Introduction to OGRE 3 D Programming Main Loop
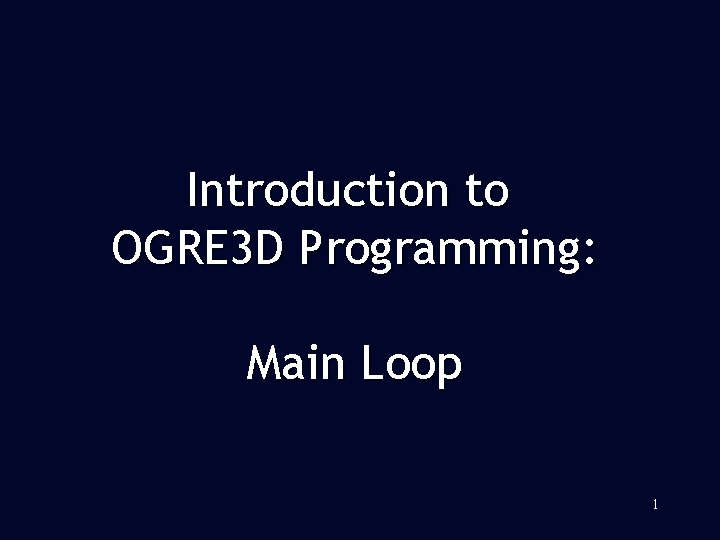
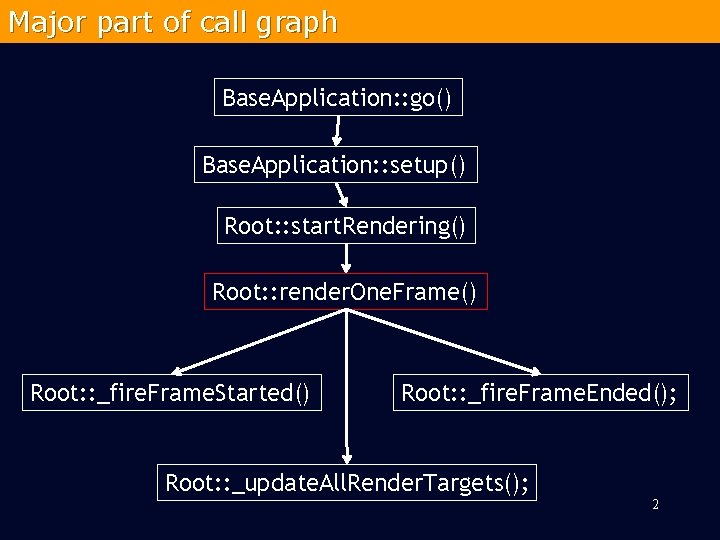
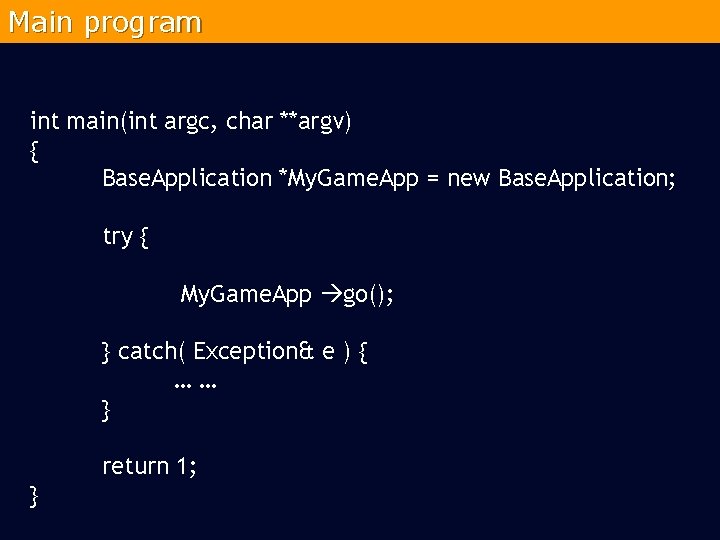
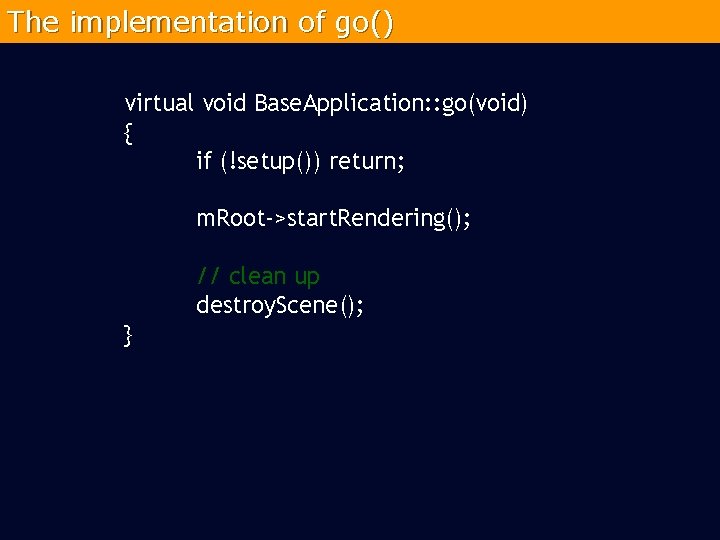
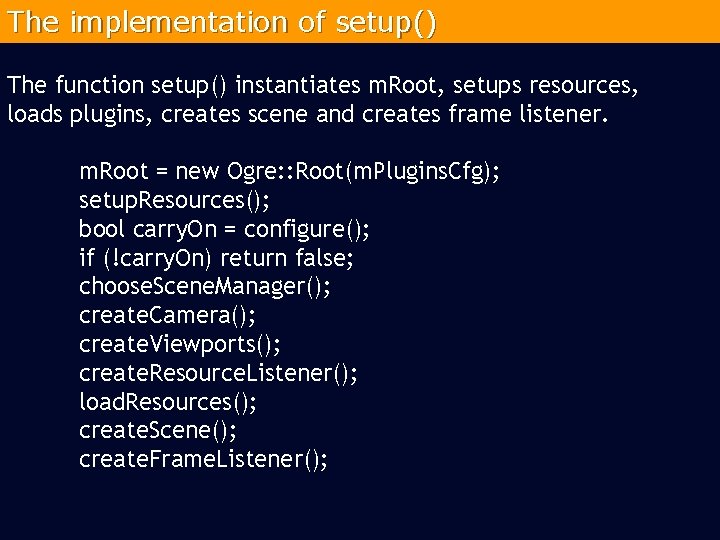
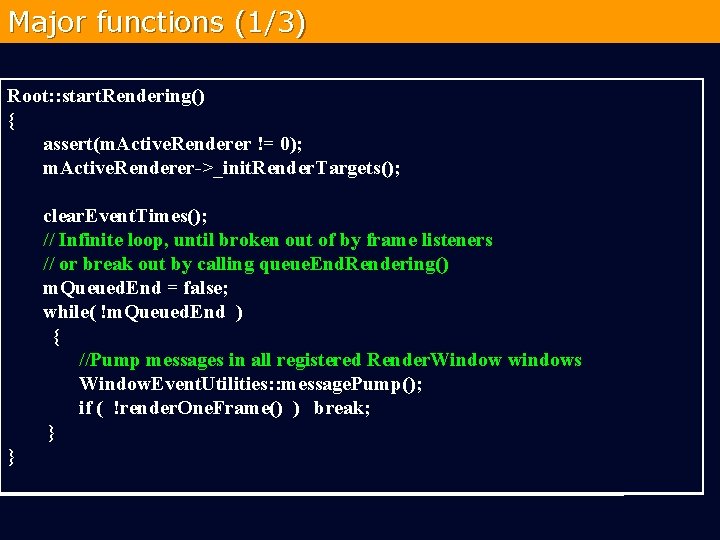
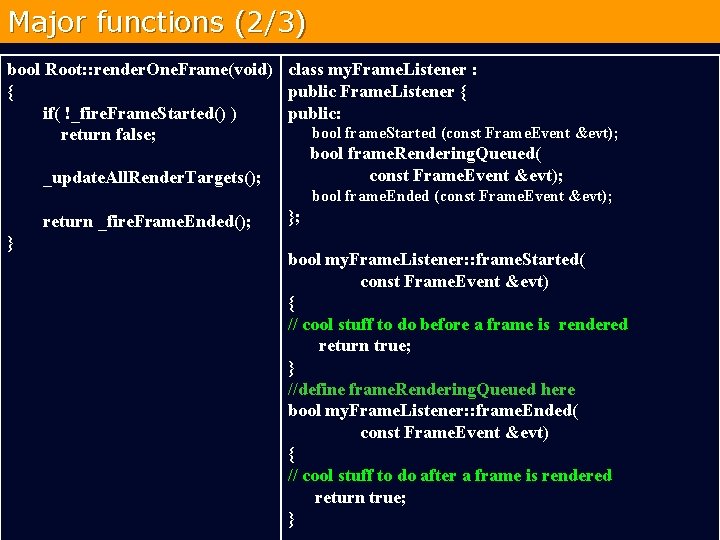
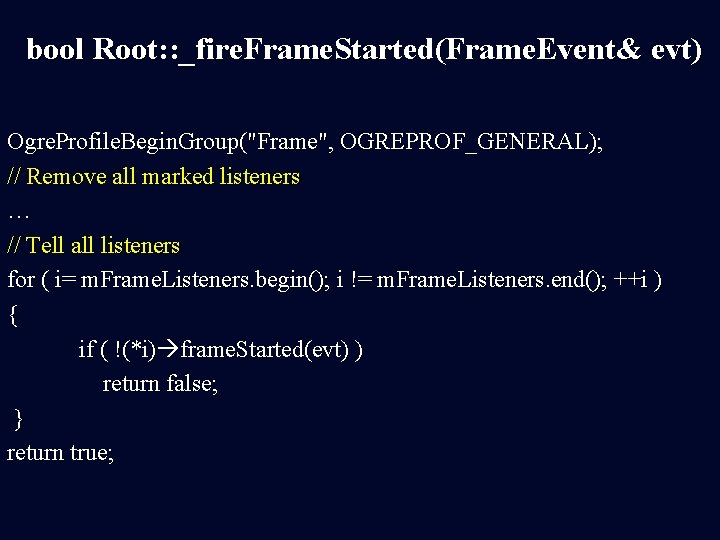
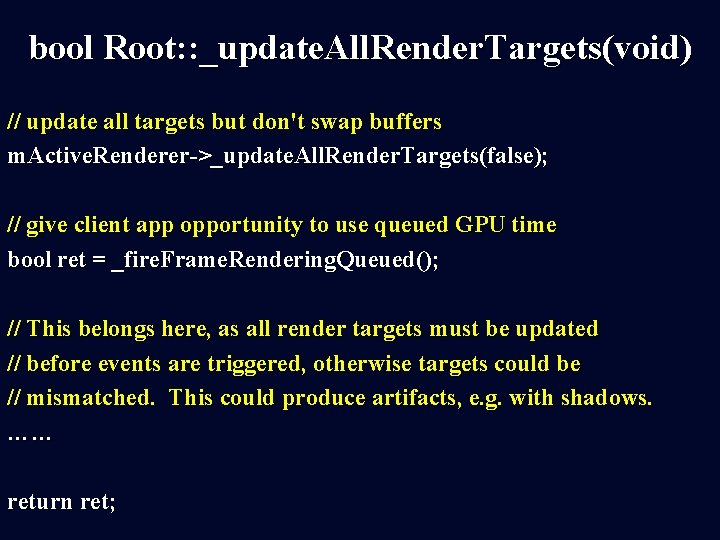
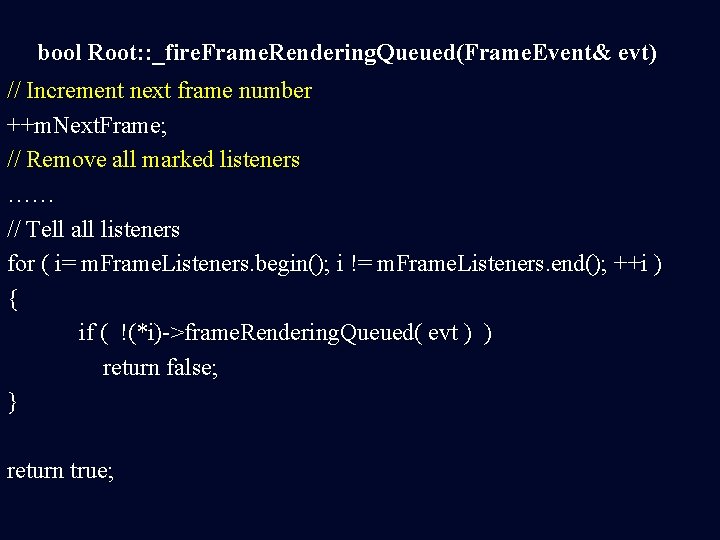
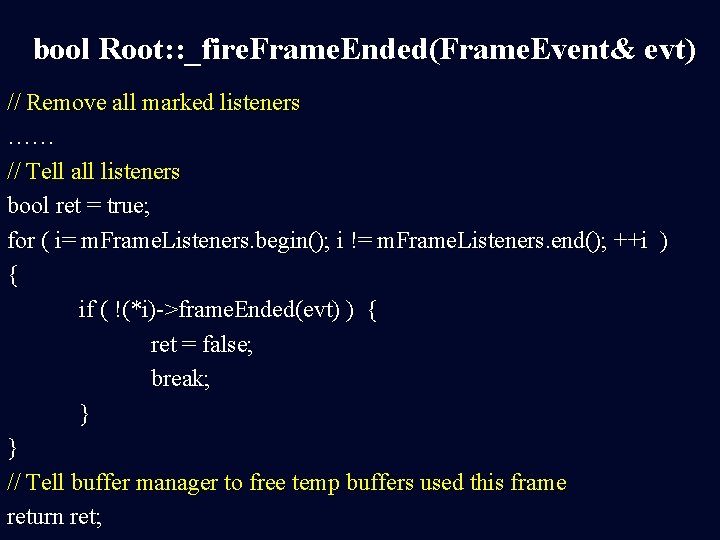
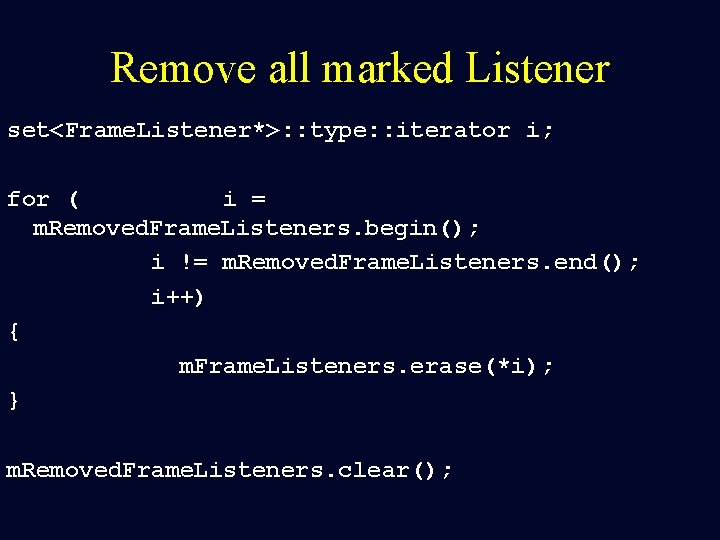
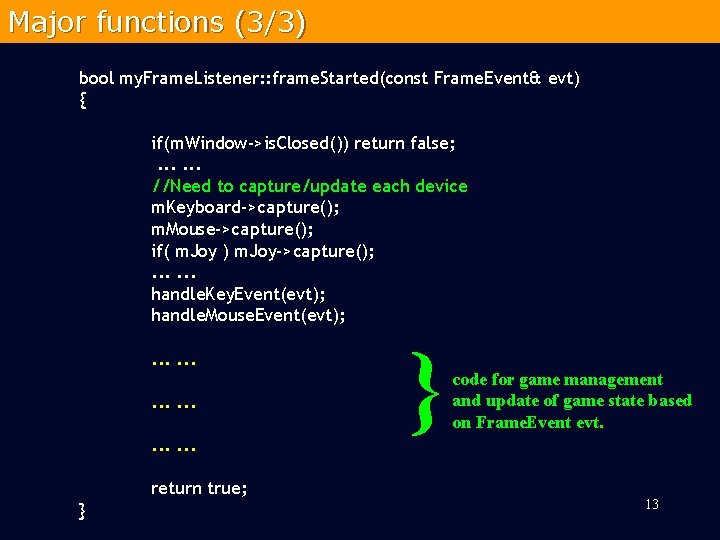
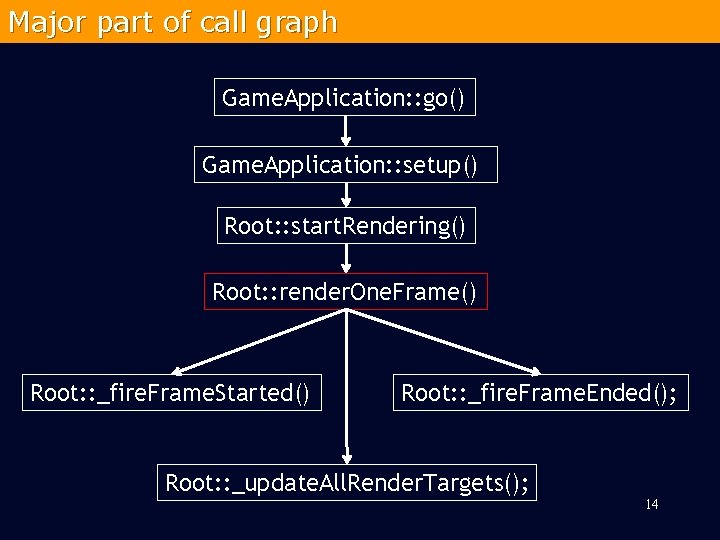
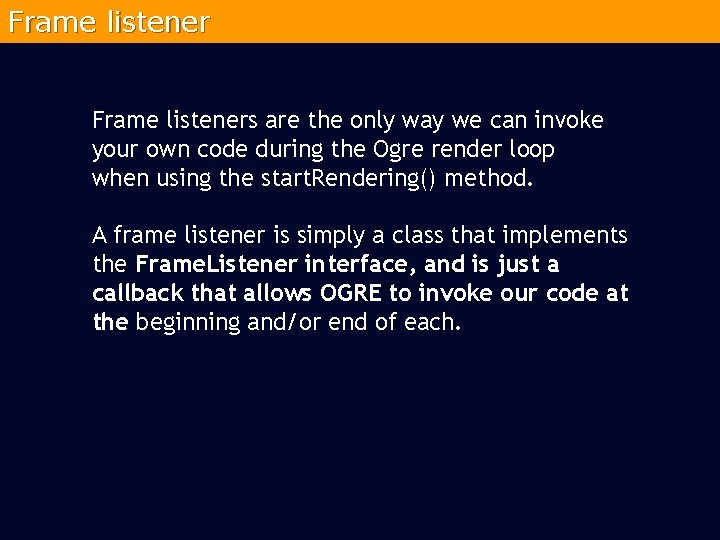
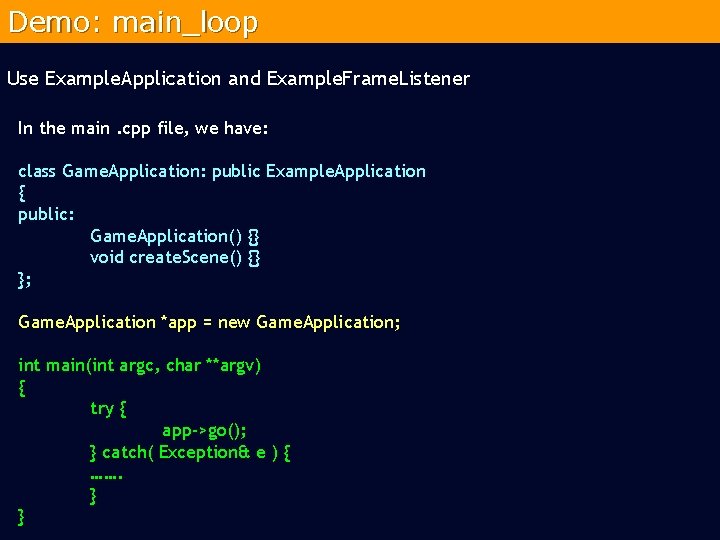
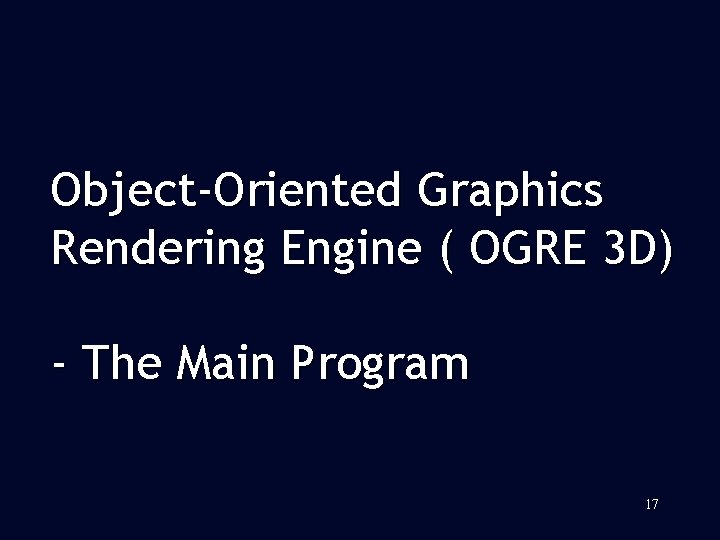
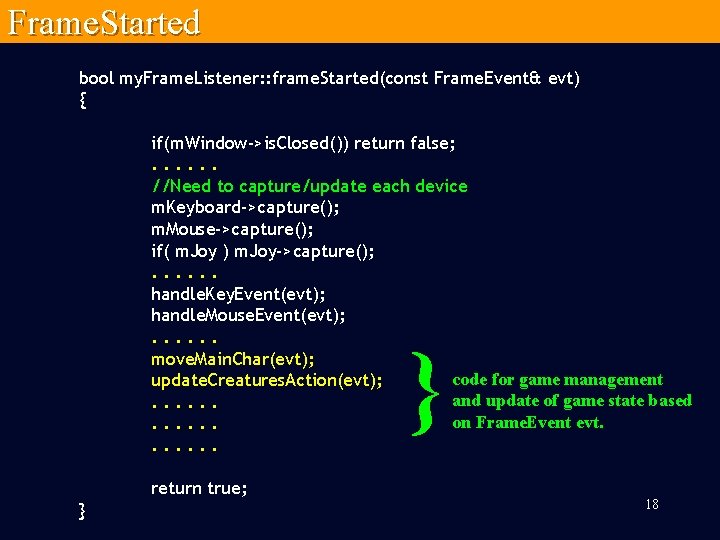
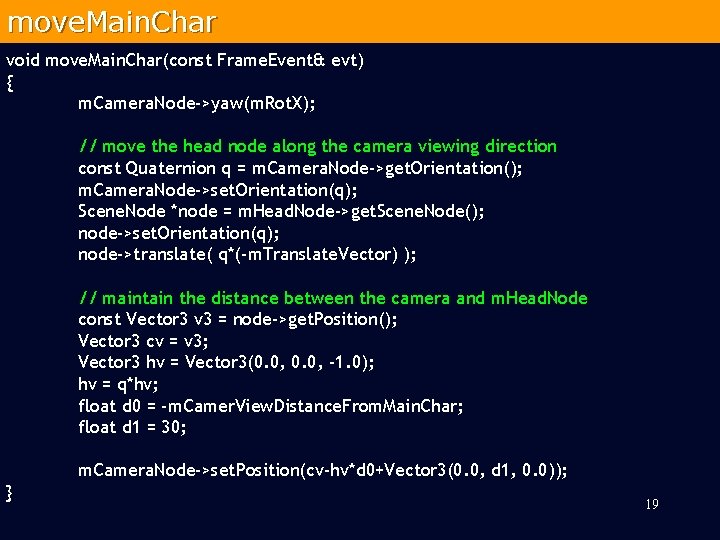
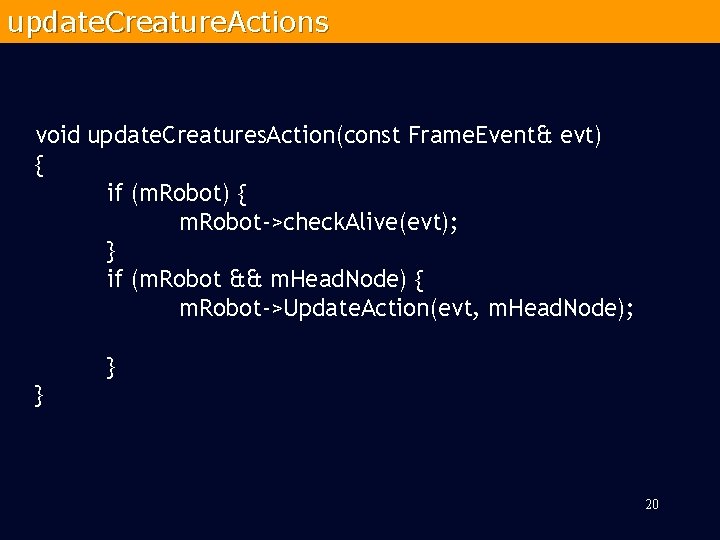
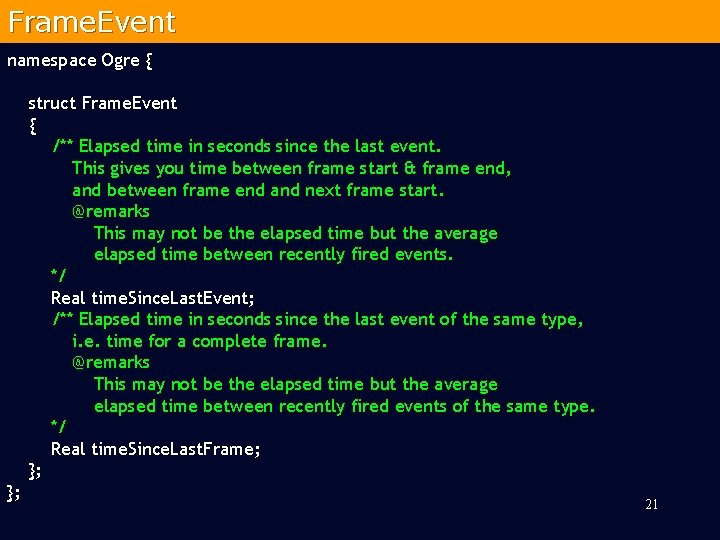
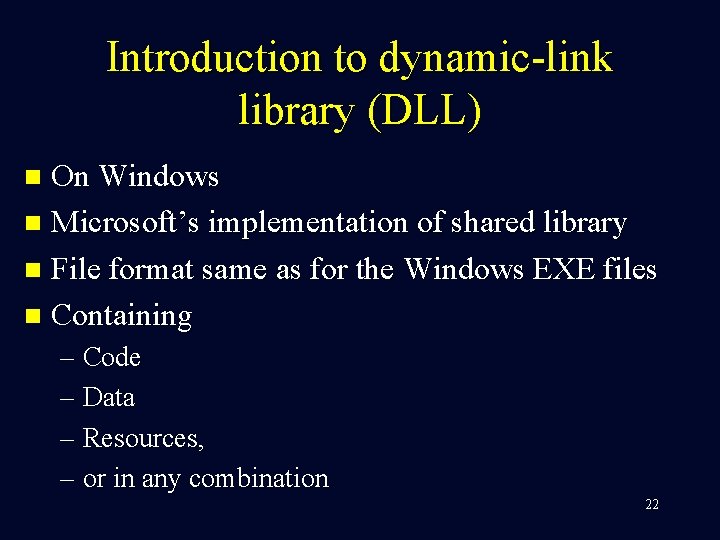
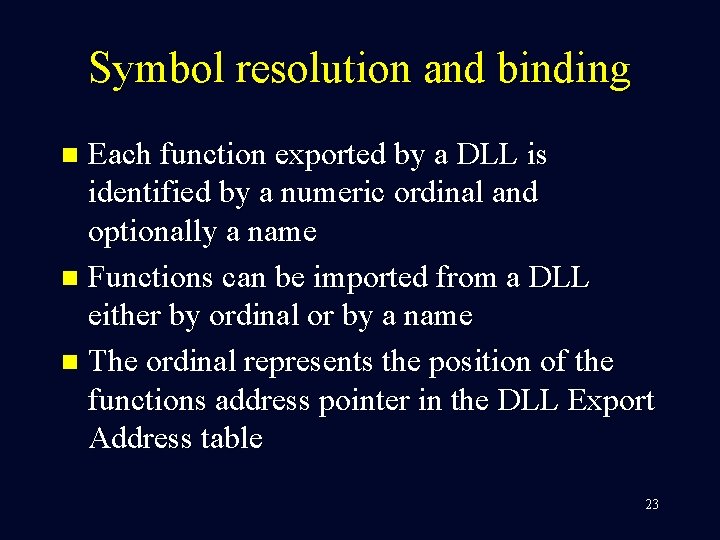
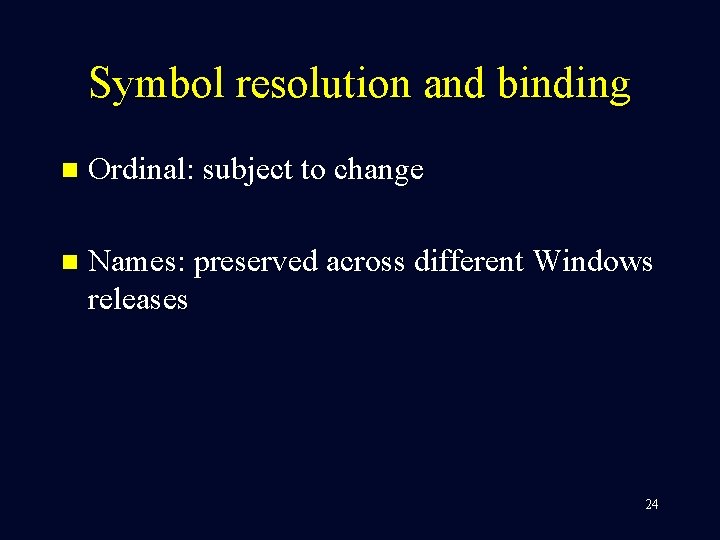
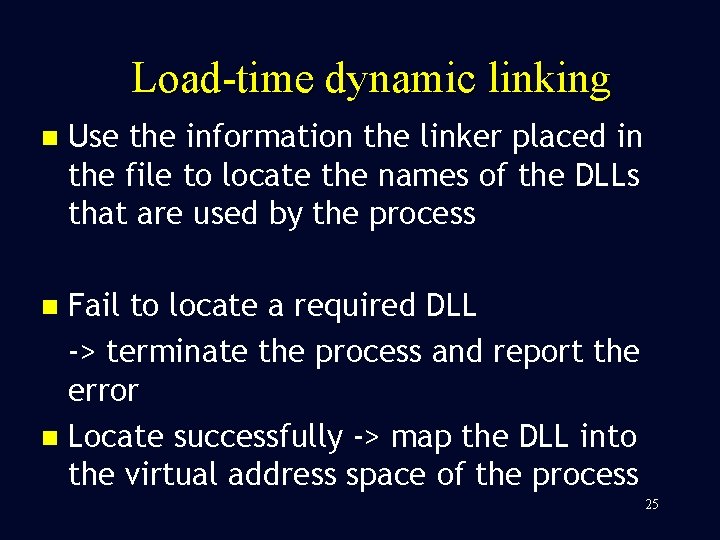
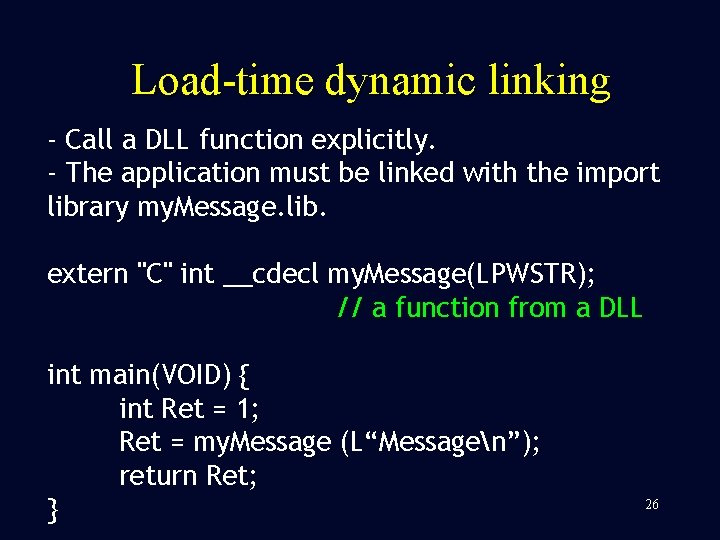
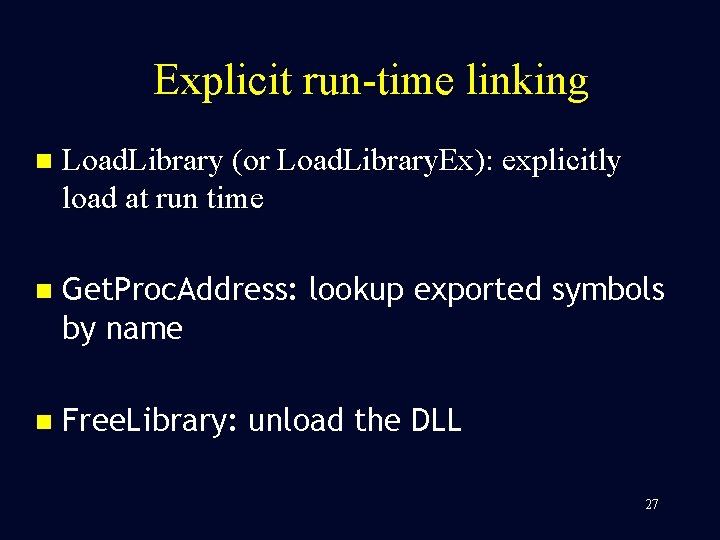
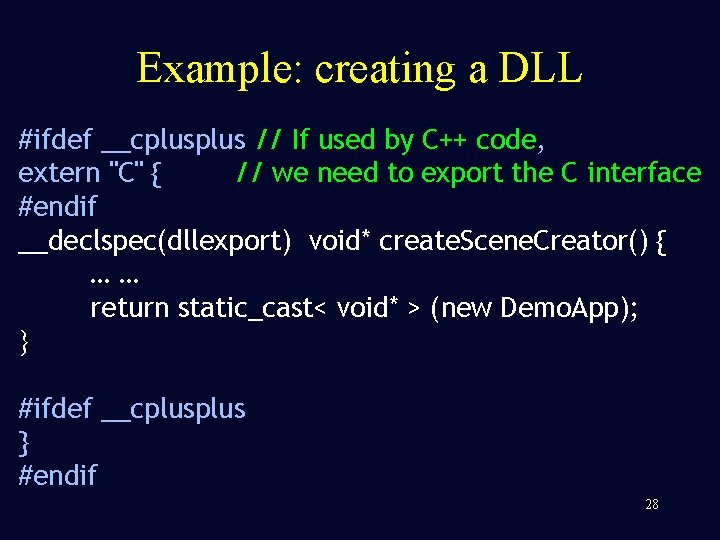
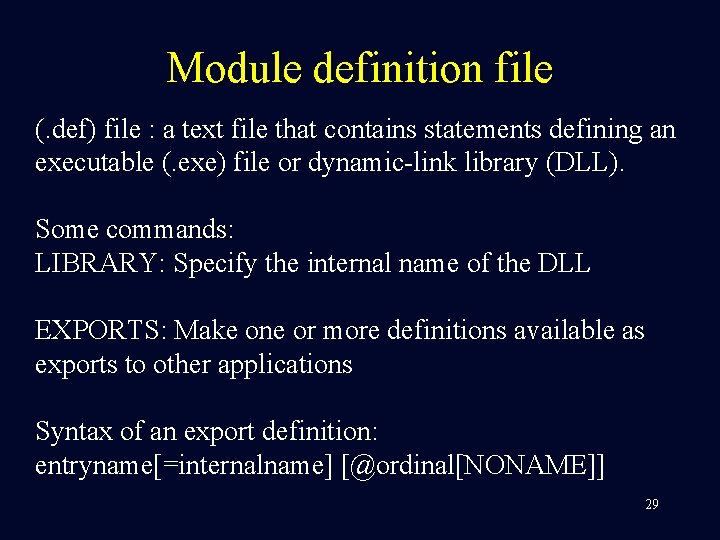
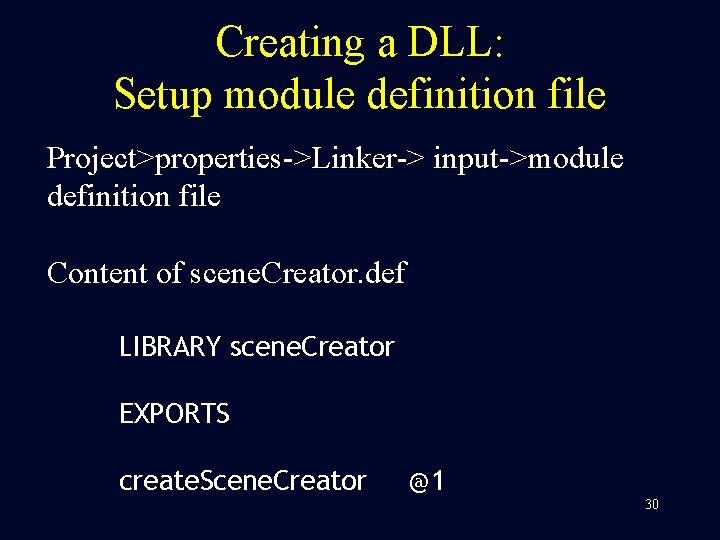
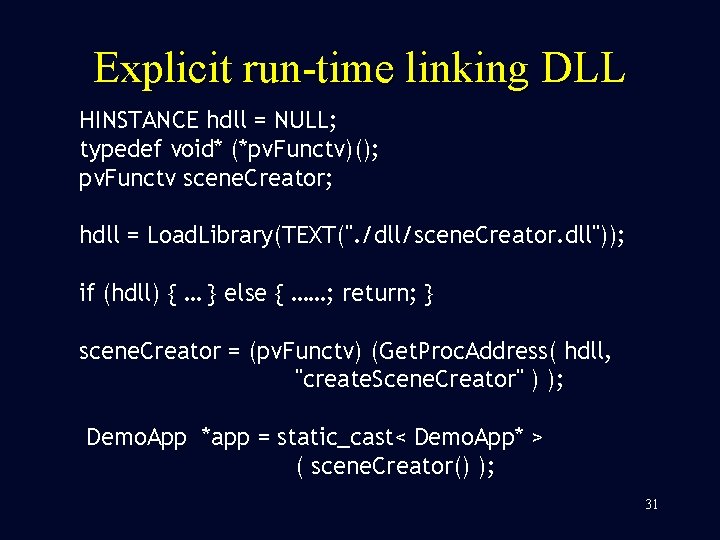
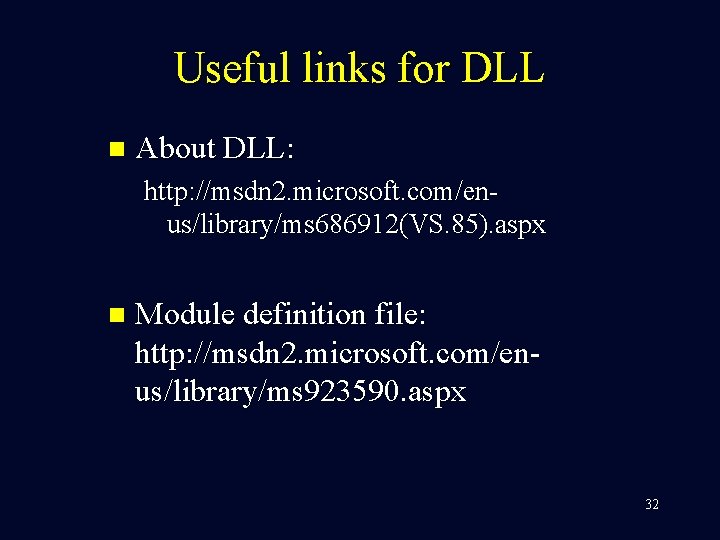
- Slides: 32
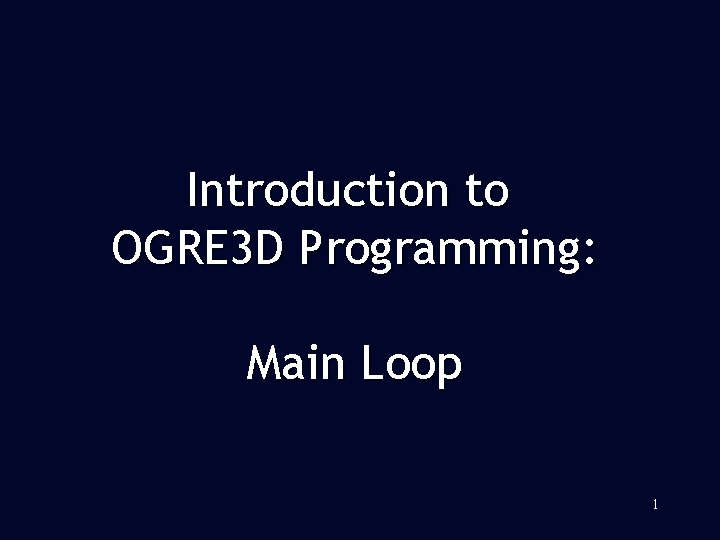
Introduction to OGRE 3 D Programming: Main Loop 1
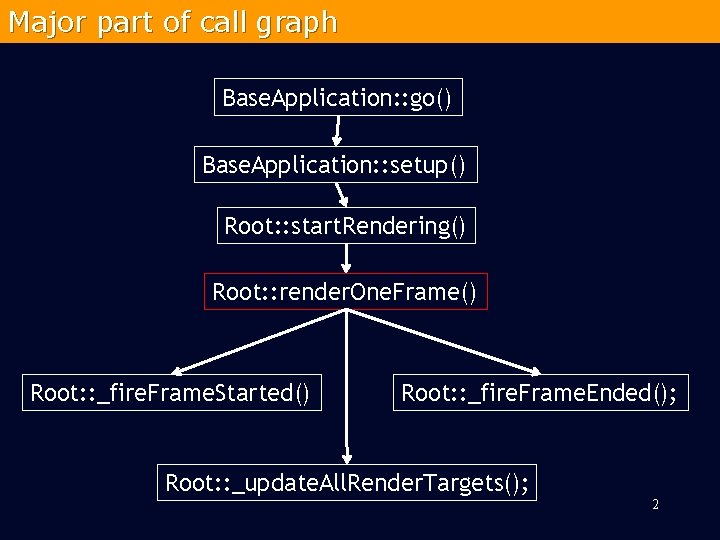
Major part of call graph Base. Application: : go() Base. Application: : setup() Root: : start. Rendering() Root: : render. One. Frame() Root: : _fire. Frame. Started() Root: : _fire. Frame. Ended(); Root: : _update. All. Render. Targets(); 2
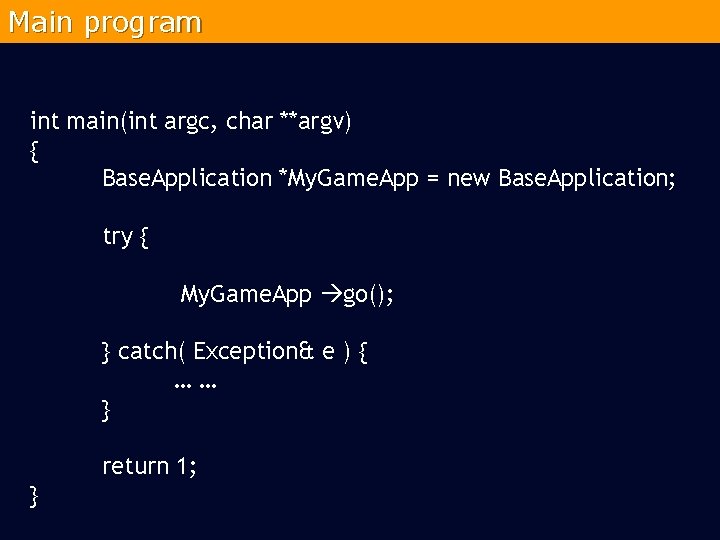
Main program int main(int argc, char **argv) { Base. Application *My. Game. App = new Base. Application; try { My. Game. App go(); } catch( Exception& e ) { …… } return 1; } 3
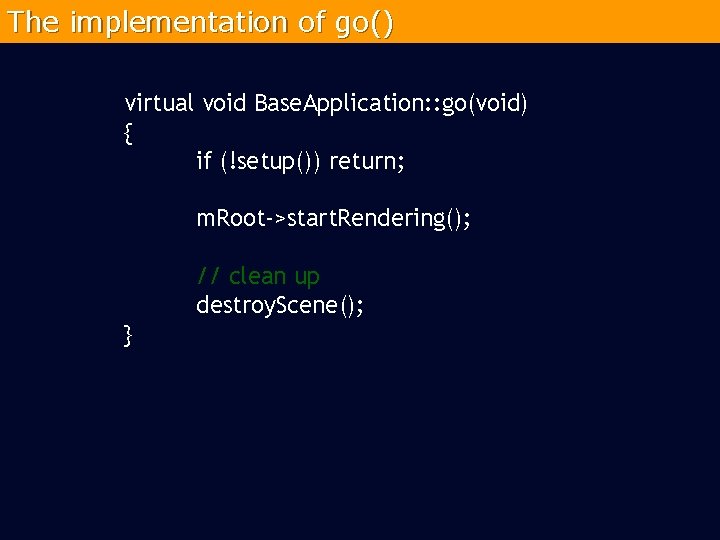
The implementation of go() virtual void Base. Application: : go(void) { if (!setup()) return; m. Root->start. Rendering(); // clean up destroy. Scene(); }
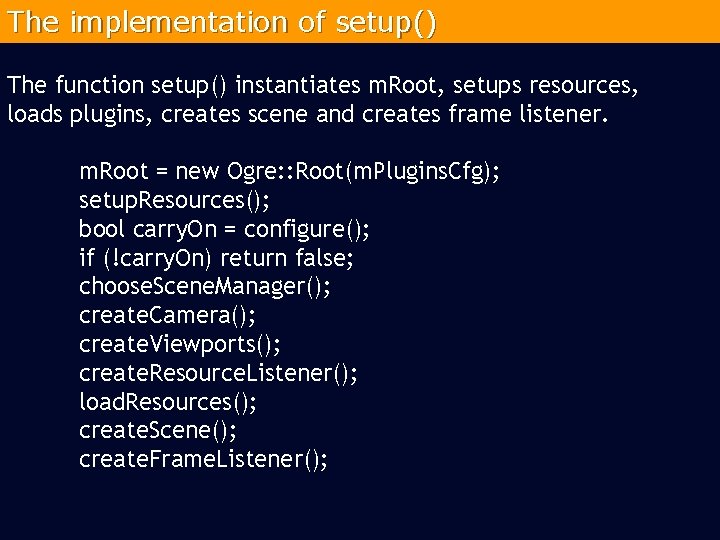
The implementation of setup() The function setup() instantiates m. Root, setups resources, loads plugins, creates scene and creates frame listener. m. Root = new Ogre: : Root(m. Plugins. Cfg); setup. Resources(); bool carry. On = configure(); if (!carry. On) return false; choose. Scene. Manager(); create. Camera(); create. Viewports(); create. Resource. Listener(); load. Resources(); create. Scene(); create. Frame. Listener();
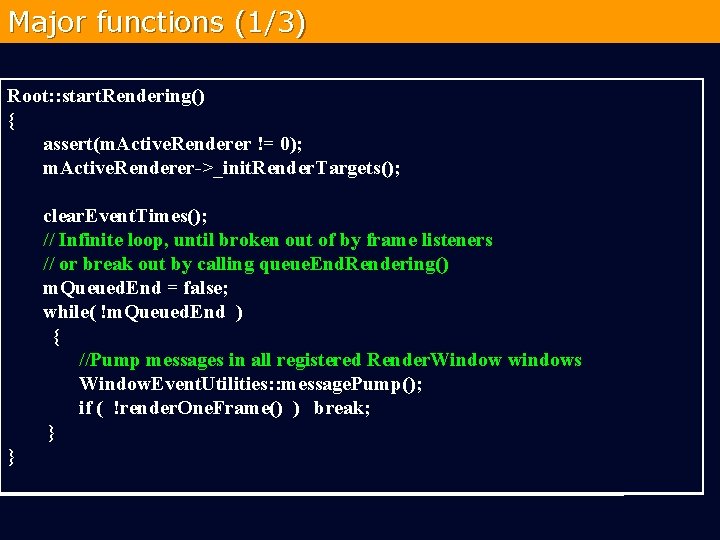
Major functions (1/3) Root: : start. Rendering() { assert(m. Active. Renderer != 0); m. Active. Renderer->_init. Render. Targets(); clear. Event. Times(); // Infinite loop, until broken out of by frame listeners // or break out by calling queue. End. Rendering() m. Queued. End = false; while( !m. Queued. End ) { //Pump messages in all registered Render. Window windows Window. Event. Utilities: : message. Pump(); if ( !render. One. Frame() ) break; } } 6
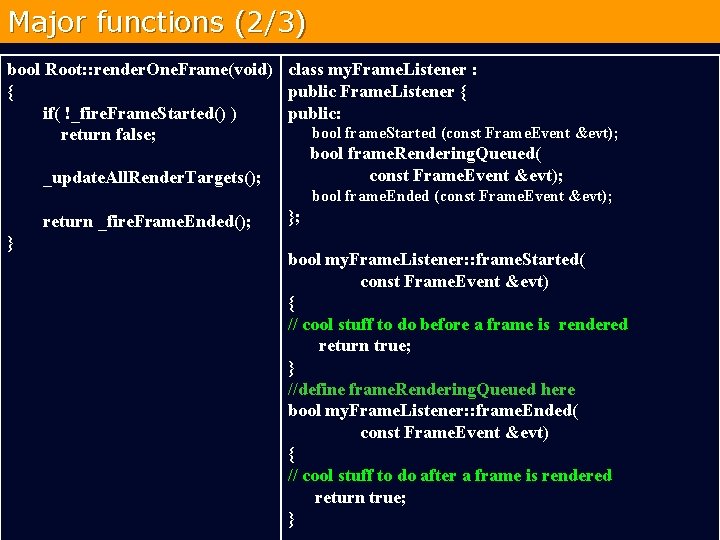
Major functions (2/3) bool Root: : render. One. Frame(void) class my. Frame. Listener : { public Frame. Listener { if( !_fire. Frame. Started() ) public: bool frame. Started (const Frame. Event &evt); return false; bool frame. Rendering. Queued( const Frame. Event &evt); _update. All. Render. Targets(); bool frame. Ended (const Frame. Event &evt); return _fire. Frame. Ended(); } }; bool my. Frame. Listener: : frame. Started( const Frame. Event &evt) { // cool stuff to do before a frame is rendered return true; } //define frame. Rendering. Queued here bool my. Frame. Listener: : frame. Ended( const Frame. Event &evt) { // cool stuff to do after a frame is rendered return true; } 7
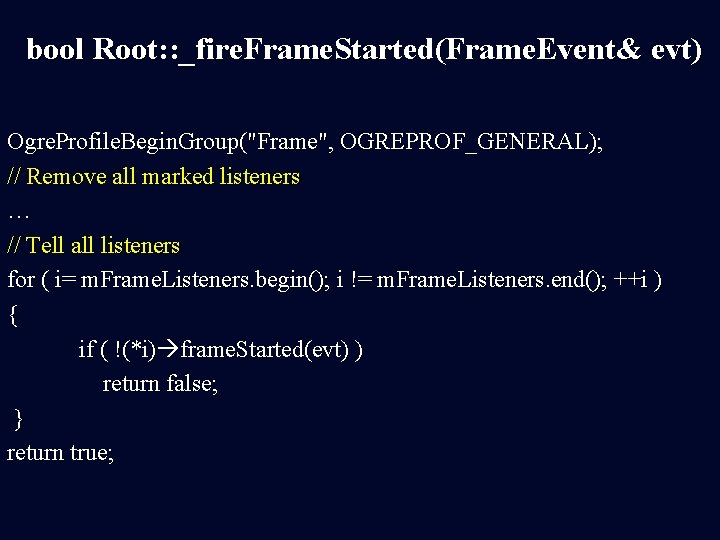
bool Root: : _fire. Frame. Started(Frame. Event& evt) Ogre. Profile. Begin. Group("Frame", OGREPROF_GENERAL); // Remove all marked listeners … // Tell all listeners for ( i= m. Frame. Listeners. begin(); i != m. Frame. Listeners. end(); ++i ) { if ( !(*i) frame. Started(evt) ) return false; } return true;
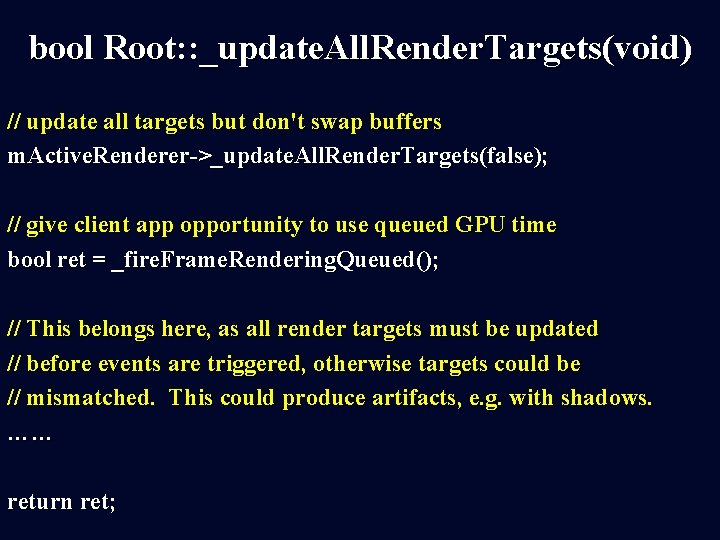
bool Root: : _update. All. Render. Targets(void) // update all targets but don't swap buffers m. Active. Renderer->_update. All. Render. Targets(false); // give client app opportunity to use queued GPU time bool ret = _fire. Frame. Rendering. Queued(); // This belongs here, as all render targets must be updated // before events are triggered, otherwise targets could be // mismatched. This could produce artifacts, e. g. with shadows. …… return ret;
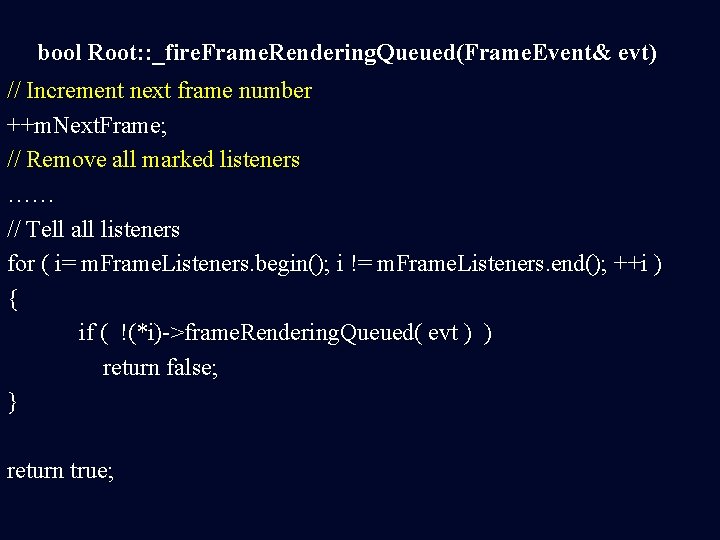
bool Root: : _fire. Frame. Rendering. Queued(Frame. Event& evt) // Increment next frame number ++m. Next. Frame; // Remove all marked listeners …… // Tell all listeners for ( i= m. Frame. Listeners. begin(); i != m. Frame. Listeners. end(); ++i ) { if ( !(*i)->frame. Rendering. Queued( evt ) ) return false; } return true;
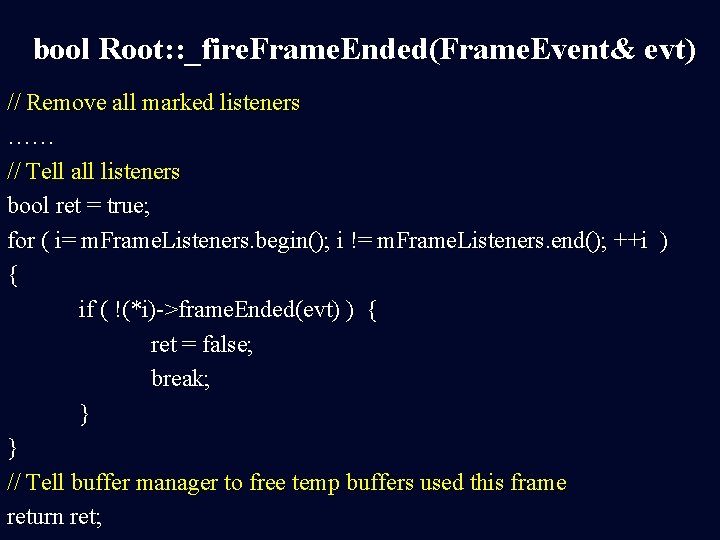
bool Root: : _fire. Frame. Ended(Frame. Event& evt) // Remove all marked listeners …… // Tell all listeners bool ret = true; for ( i= m. Frame. Listeners. begin(); i != m. Frame. Listeners. end(); ++i ) { if ( !(*i)->frame. Ended(evt) ) { ret = false; break; } } // Tell buffer manager to free temp buffers used this frame return ret;
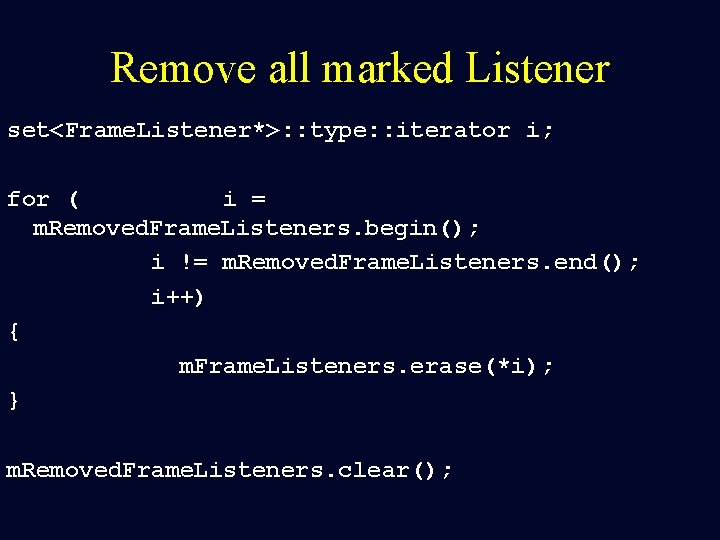
Remove all marked Listener set<Frame. Listener*>: : type: : iterator i; for ( i = m. Removed. Frame. Listeners. begin(); i != m. Removed. Frame. Listeners. end(); i++) { m. Frame. Listeners. erase(*i); } m. Removed. Frame. Listeners. clear();
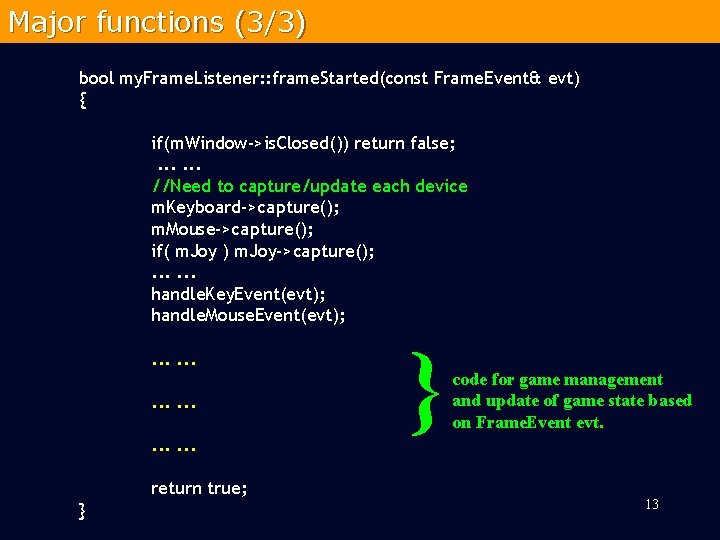
Major functions (3/3) bool my. Frame. Listener: : frame. Started(const Frame. Event& evt) { if(m. Window->is. Closed()) return false; . . . //Need to capture/update each device m. Keyboard->capture(); m. Mouse->capture(); if( m. Joy ) m. Joy->capture(); . . . handle. Key. Event(evt); handle. Mouse. Event(evt); . . . . return true; } } code for game management and update of game state based on Frame. Event evt. 13
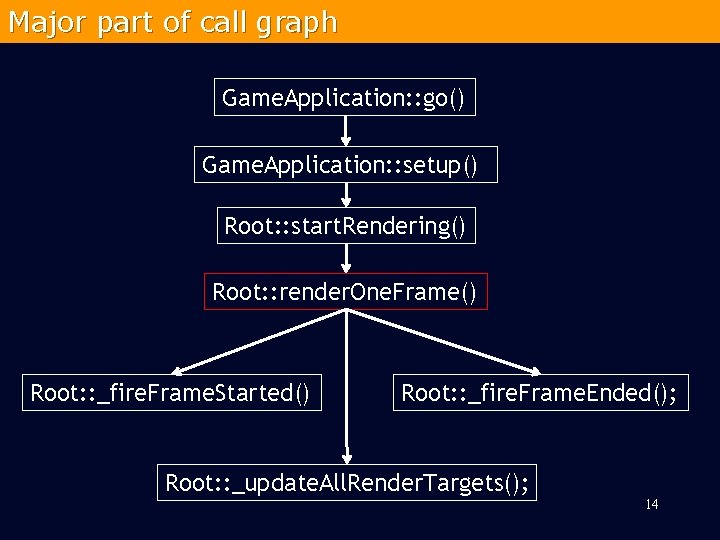
Major part of call graph Game. Application: : go() Game. Application: : setup() Root: : start. Rendering() Root: : render. One. Frame() Root: : _fire. Frame. Started() Root: : _fire. Frame. Ended(); Root: : _update. All. Render. Targets(); 14
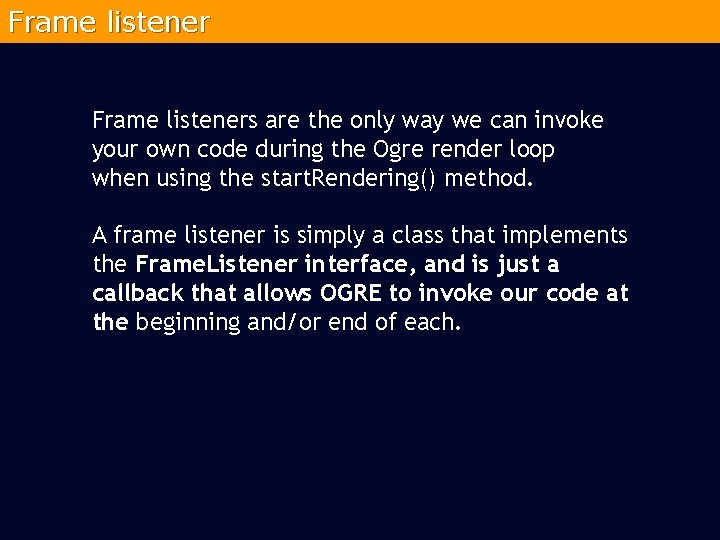
Frame listeners are the only way we can invoke your own code during the Ogre render loop when using the start. Rendering() method. A frame listener is simply a class that implements the Frame. Listener interface, and is just a callback that allows OGRE to invoke our code at the beginning and/or end of each. 15
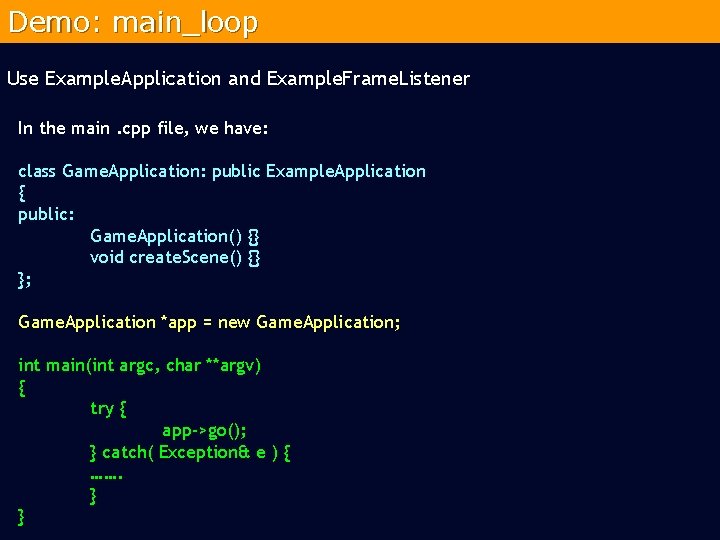
Demo: main_loop Use Example. Application and Example. Frame. Listener In the main. cpp file, we have: class Game. Application: public Example. Application { public: Game. Application() {} void create. Scene() {} }; Game. Application *app = new Game. Application; int main(int argc, char **argv) { try { app->go(); } catch( Exception& e ) { ……. } } 16
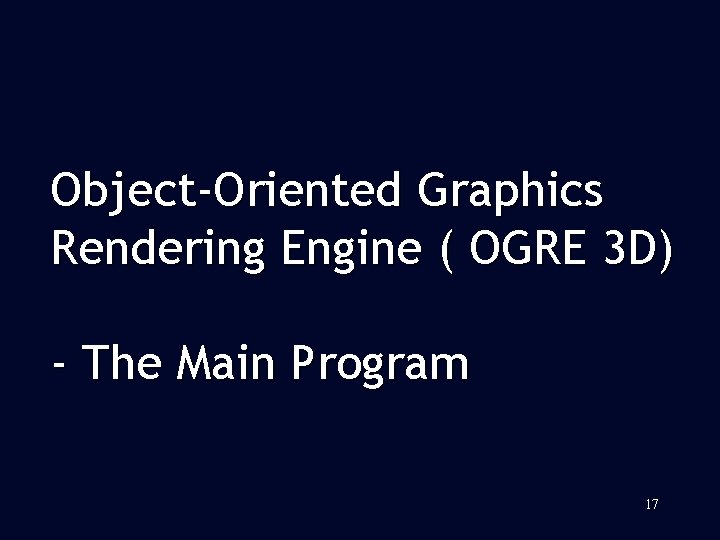
Object-Oriented Graphics Rendering Engine ( OGRE 3 D) - The Main Program 17
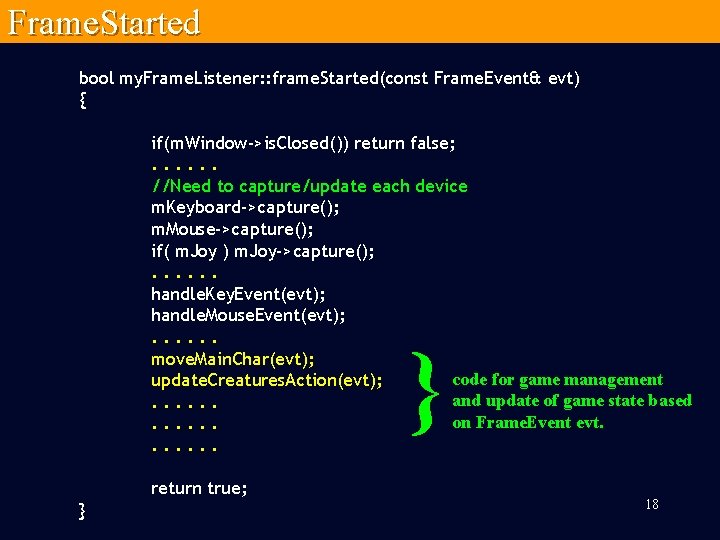
Frame. Started bool my. Frame. Listener: : frame. Started(const Frame. Event& evt) { if(m. Window->is. Closed()) return false; . . . //Need to capture/update each device m. Keyboard->capture(); m. Mouse->capture(); if( m. Joy ) m. Joy->capture(); . . . handle. Key. Event(evt); handle. Mouse. Event(evt); . . . move. Main. Char(evt); code for game management update. Creatures. Action(evt); and update of game state based. . . on Frame. Event evt. . . } return true; } 18
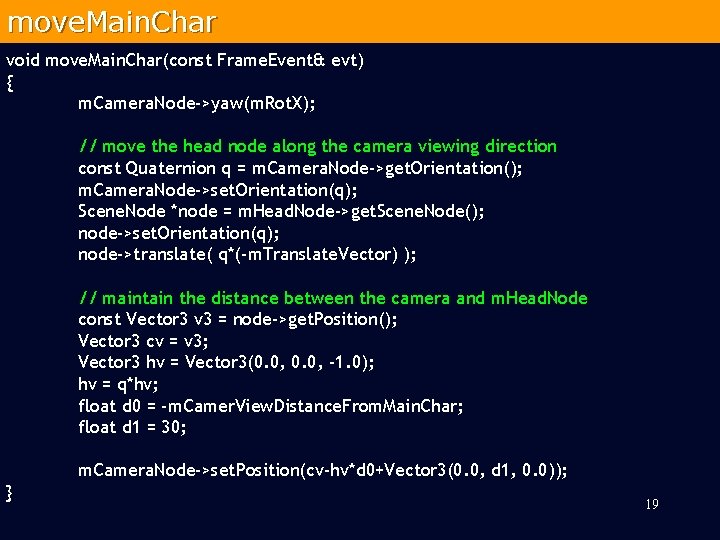
move. Main. Char void move. Main. Char(const Frame. Event& evt) { m. Camera. Node->yaw(m. Rot. X); // move the head node along the camera viewing direction const Quaternion q = m. Camera. Node->get. Orientation(); m. Camera. Node->set. Orientation(q); Scene. Node *node = m. Head. Node->get. Scene. Node(); node->set. Orientation(q); node->translate( q*(-m. Translate. Vector) ); // maintain the distance between the camera and m. Head. Node const Vector 3 v 3 = node->get. Position(); Vector 3 cv = v 3; Vector 3 hv = Vector 3(0. 0, -1. 0); hv = q*hv; float d 0 = -m. Camer. View. Distance. From. Main. Char; float d 1 = 30; m. Camera. Node->set. Position(cv-hv*d 0+Vector 3(0. 0, d 1, 0. 0)); } 19
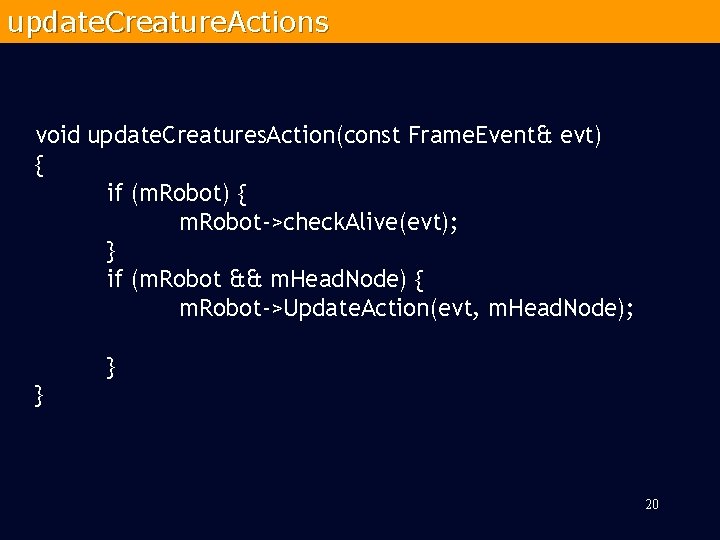
update. Creature. Actions void update. Creatures. Action(const Frame. Event& evt) { if (m. Robot) { m. Robot->check. Alive(evt); } if (m. Robot && m. Head. Node) { m. Robot->Update. Action(evt, m. Head. Node); } } 20
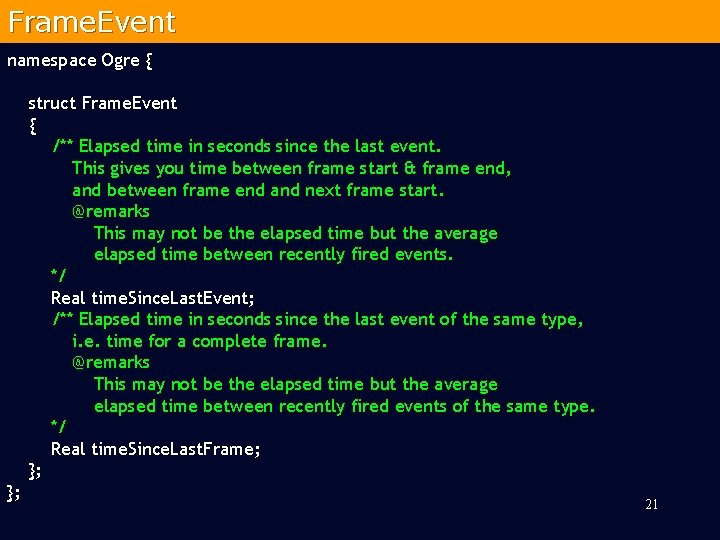
Frame. Event namespace Ogre { struct Frame. Event { /** Elapsed time in seconds since the last event. This gives you time between frame start & frame end, and between frame end and next frame start. @remarks This may not be the elapsed time but the average elapsed time between recently fired events. */ Real time. Since. Last. Event; /** Elapsed time in seconds since the last event of the same type, i. e. time for a complete frame. @remarks This may not be the elapsed time but the average elapsed time between recently fired events of the same type. */ Real time. Since. Last. Frame; }; }; 21
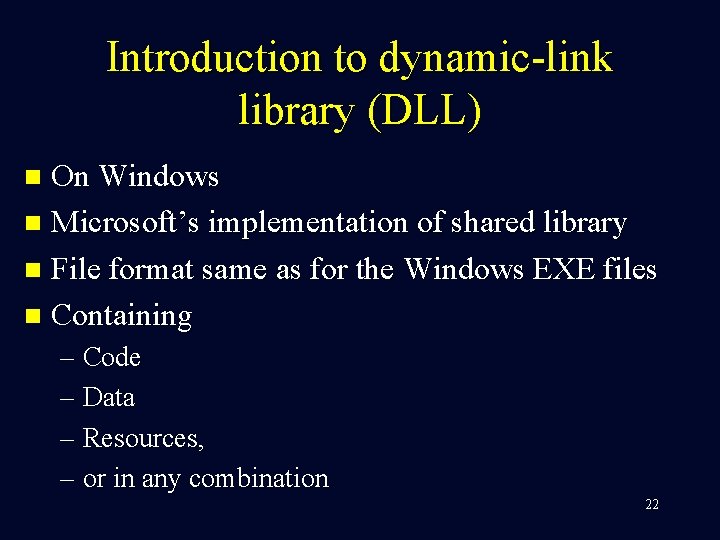
Introduction to dynamic-link library (DLL) On Windows n Microsoft’s implementation of shared library n File format same as for the Windows EXE files n Containing n – Code – Data – Resources, – or in any combination 22
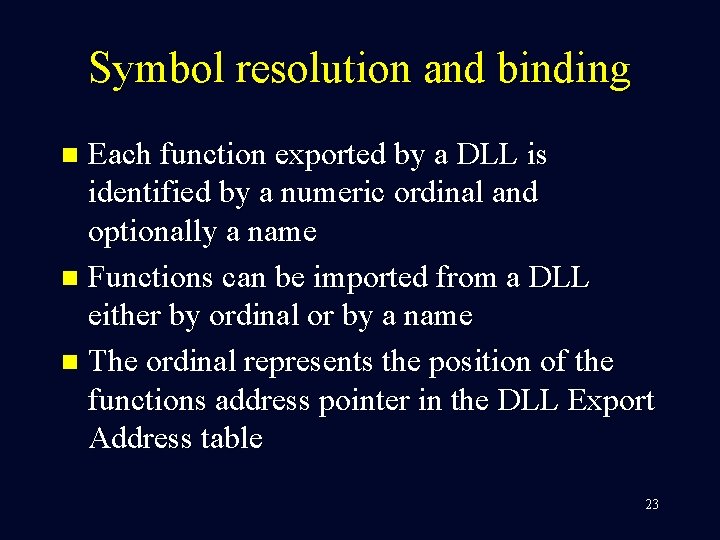
Symbol resolution and binding Each function exported by a DLL is identified by a numeric ordinal and optionally a name n Functions can be imported from a DLL either by ordinal or by a name n The ordinal represents the position of the functions address pointer in the DLL Export Address table n 23
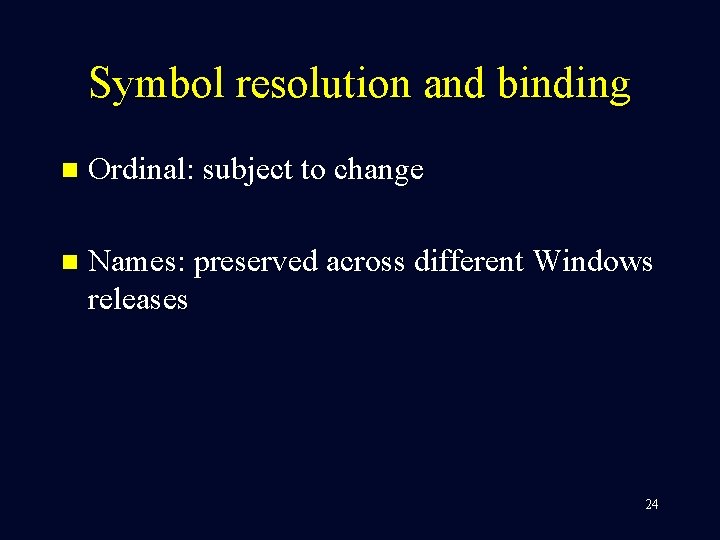
Symbol resolution and binding n Ordinal: subject to change n Names: preserved across different Windows releases 24
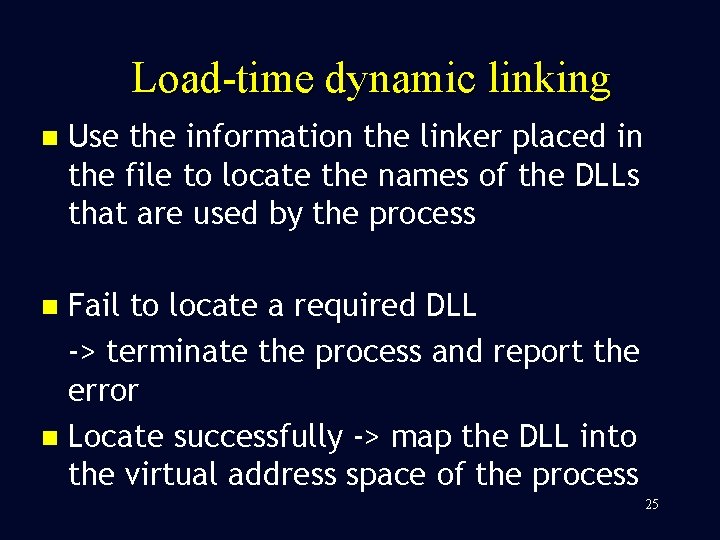
Load-time dynamic linking n Use the information the linker placed in the file to locate the names of the DLLs that are used by the process Fail to locate a required DLL -> terminate the process and report the error n Locate successfully -> map the DLL into the virtual address space of the process n 25
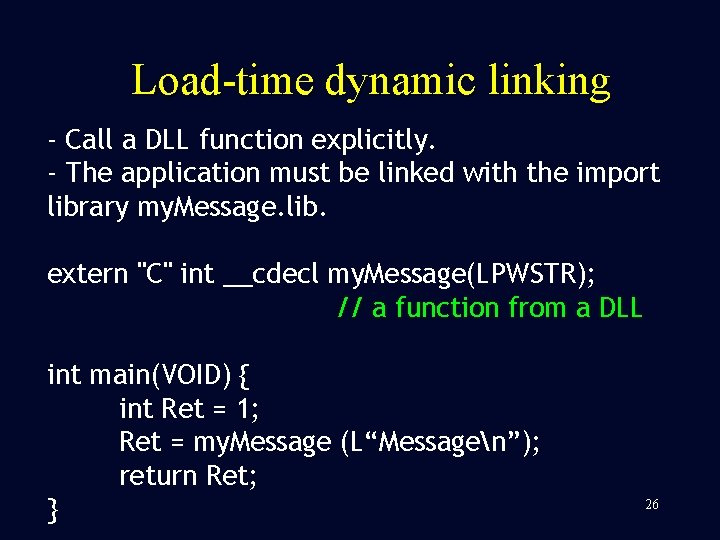
Load-time dynamic linking - Call a DLL function explicitly. - The application must be linked with the import library my. Message. lib. extern "C" int __cdecl my. Message(LPWSTR); // a function from a DLL int main(VOID) { int Ret = 1; Ret = my. Message (L“Messagen”); return Ret; } 26
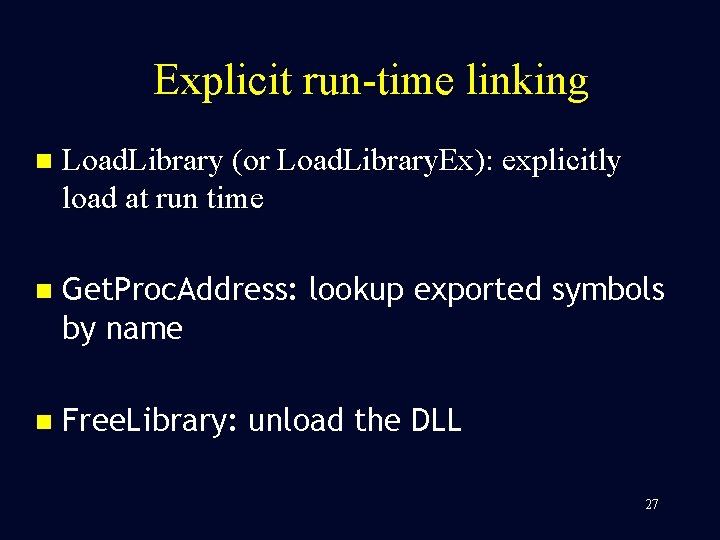
Explicit run-time linking n Load. Library (or Load. Library. Ex): explicitly load at run time n Get. Proc. Address: lookup exported symbols by name n Free. Library: unload the DLL 27
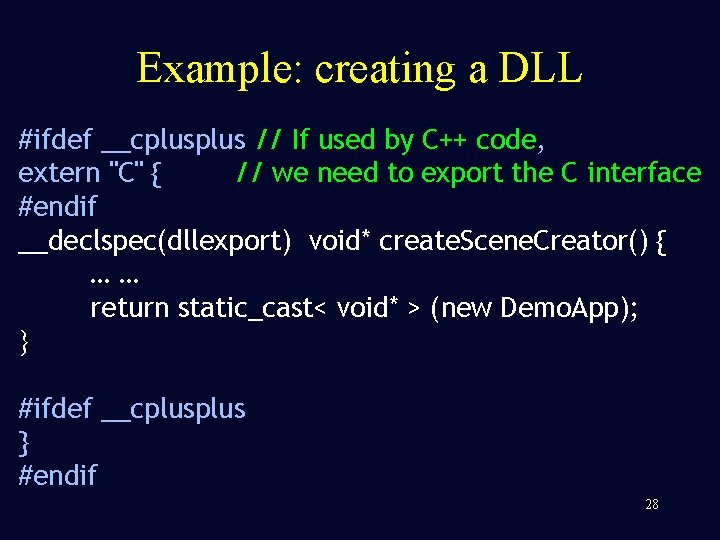
Example: creating a DLL #ifdef __cplus // If used by C++ code, extern "C" { // we need to export the C interface #endif __declspec(dllexport) void* create. Scene. Creator() { …… return static_cast< void* > (new Demo. App); } #ifdef __cplus } #endif 28
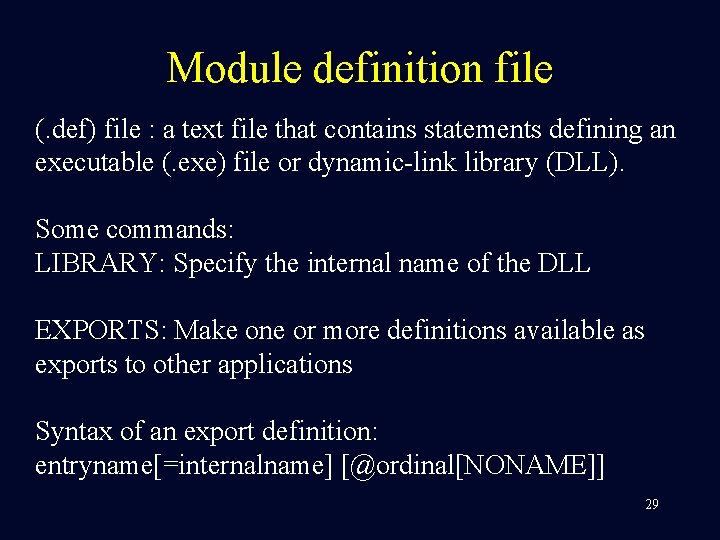
Module definition file (. def) file : a text file that contains statements defining an executable (. exe) file or dynamic-link library (DLL). Some commands: LIBRARY: Specify the internal name of the DLL EXPORTS: Make one or more definitions available as M exports to other applications Syntax of an export definition: entryname[=internalname] [@ordinal[NONAME]] 29
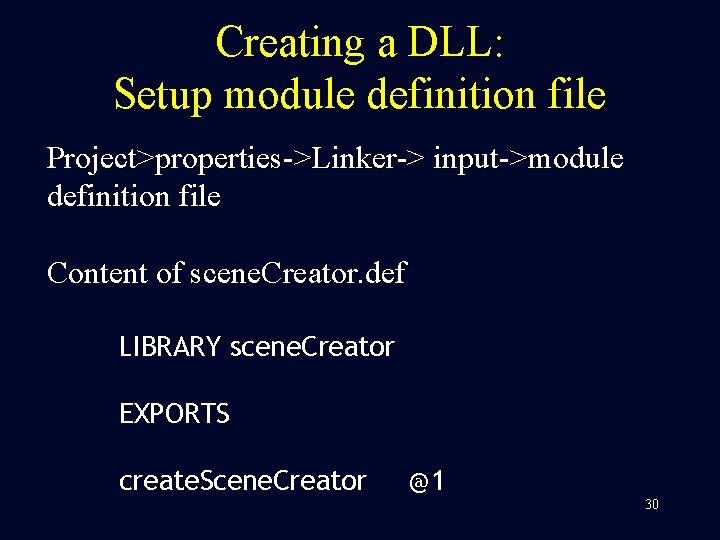
Creating a DLL: Setup module definition file Project>properties->Linker-> input->module definition file Content of scene. Creator. def LIBRARY scene. Creator EXPORTS create. Scene. Creator @1 30
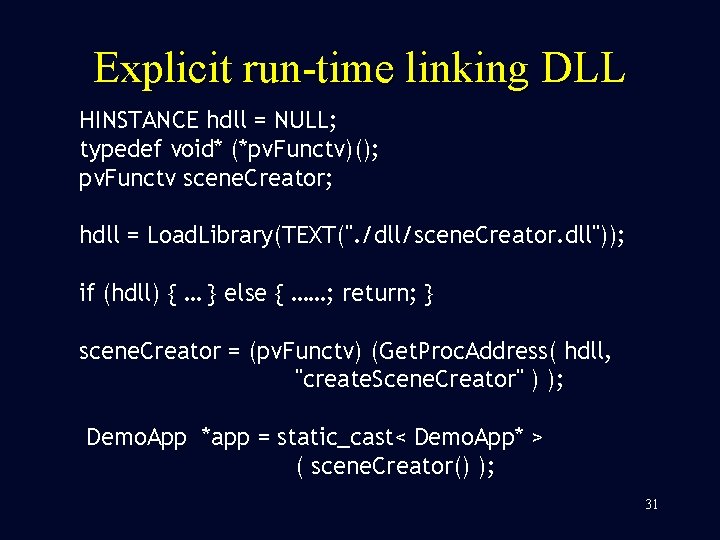
Explicit run-time linking DLL HINSTANCE hdll = NULL; typedef void* (*pv. Functv)(); pv. Functv scene. Creator; hdll = Load. Library(TEXT(". /dll/scene. Creator. dll")); if (hdll) { … } else { ……; return; } scene. Creator = (pv. Functv) (Get. Proc. Address( hdll, "create. Scene. Creator" ) ); Demo. App *app = static_cast< Demo. App* > ( scene. Creator() ); 31
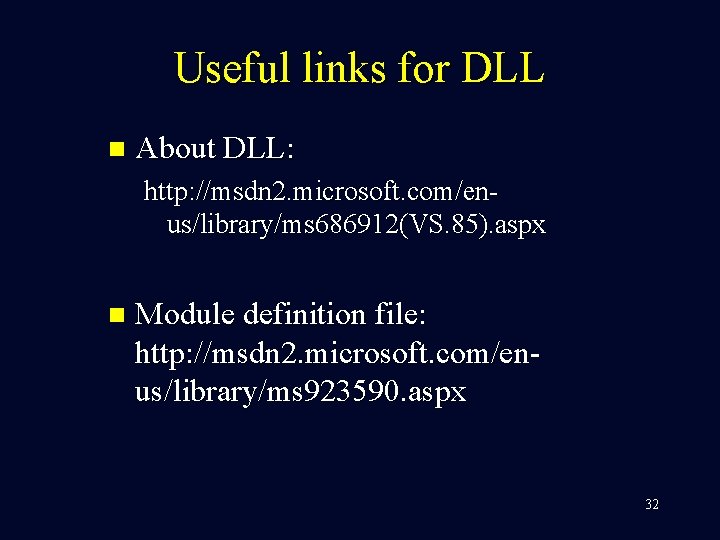
Useful links for DLL n About DLL: http: //msdn 2. microsoft. com/enus/library/ms 686912(VS. 85). aspx n Module definition file: http: //msdn 2. microsoft. com/enus/library/ms 923590. aspx 32
Ogres sociālais dienests
Sky fog ogre
Ogre game engine tutorial
Metaphor figure of speech
Shrek 2
Citra grafik
Cegui tutorial
Diagram blok open loop
Fifth gear loop the loop
Open loop vs closed loop in cars
Manakah yang lebih baik open loop atau close loop system
Perbedaan for while do while
L
Multi loop pid controller regolatore pid multi loop
Looping algoritma
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
System programming definition
Integer programming vs linear programming
Definisi integer
Expressing the future
Main ideas examples
Void main int main
Introduction to server side programming
Java introduction to problem solving and programming
Introduction to programming languages
Elementary programming in java
An introduction to parallel programming peter pacheco
Introduction to visual basic programming
What does plc stand for
Programming and problem solving with java
Console programming
Introduction to programming