Advanced loop controls Loop Controls Recall from last
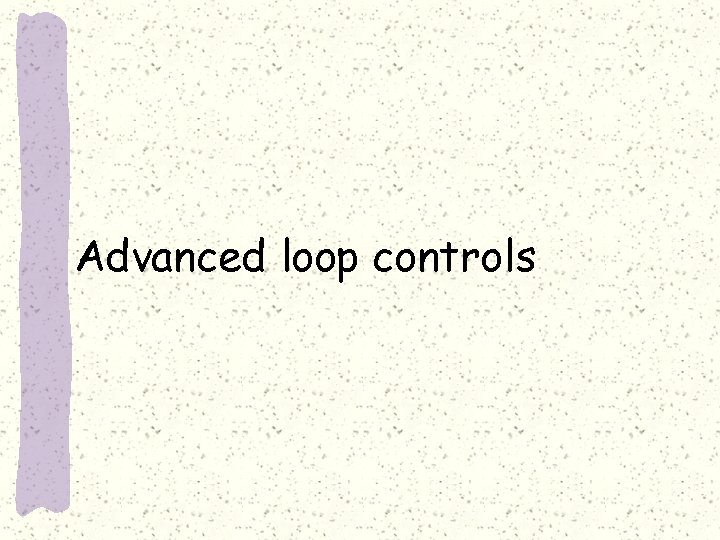
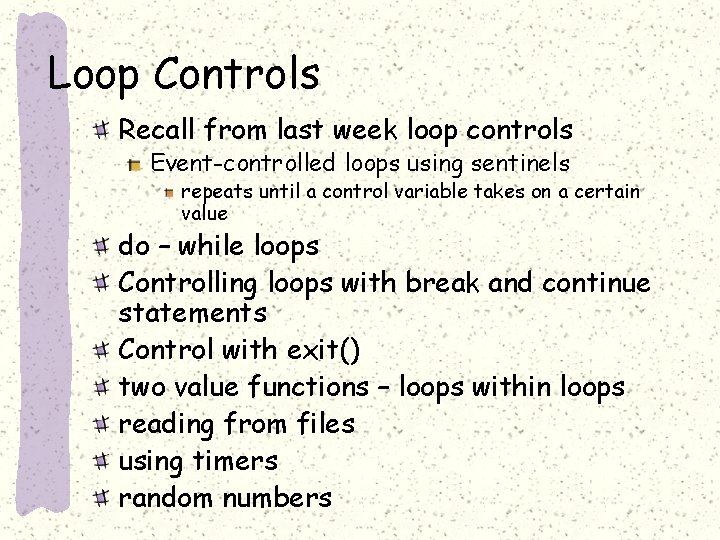
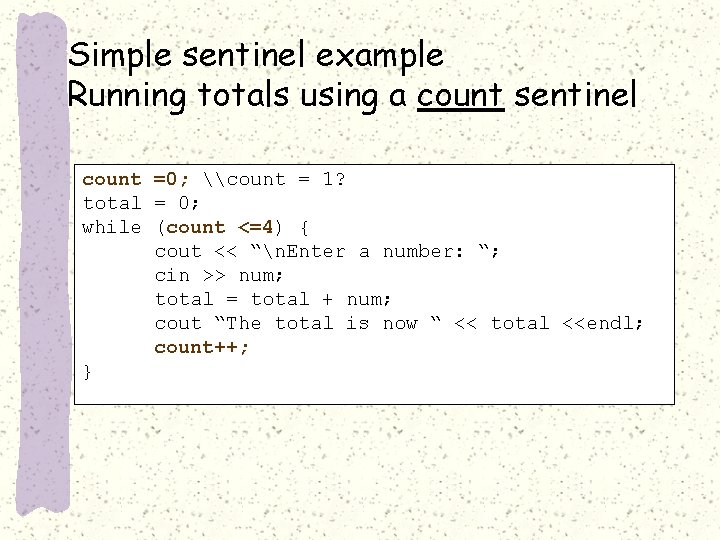
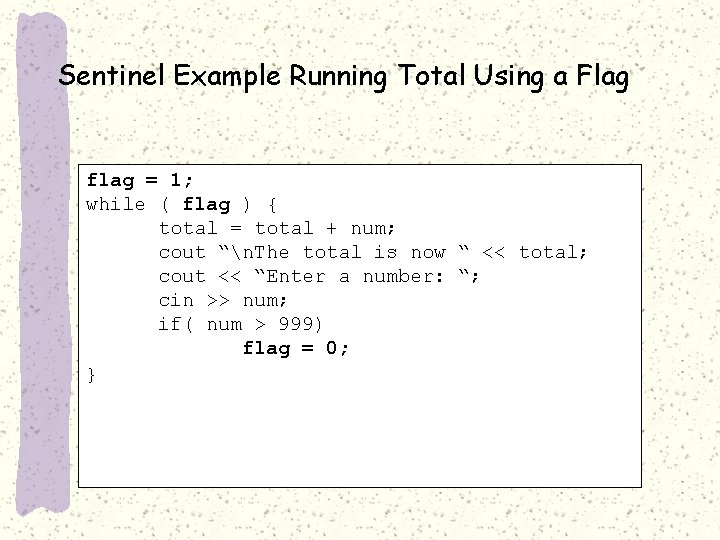
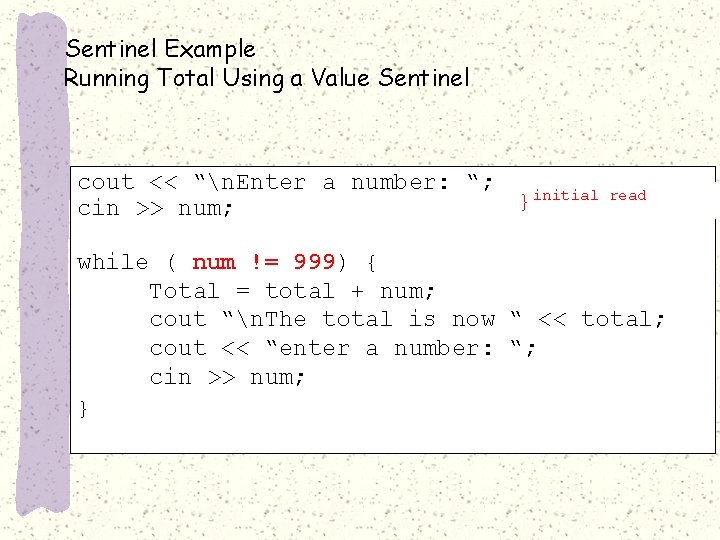
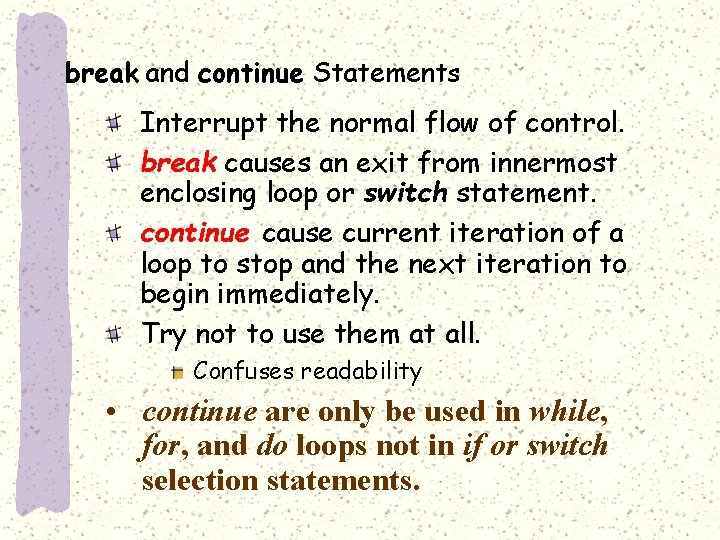
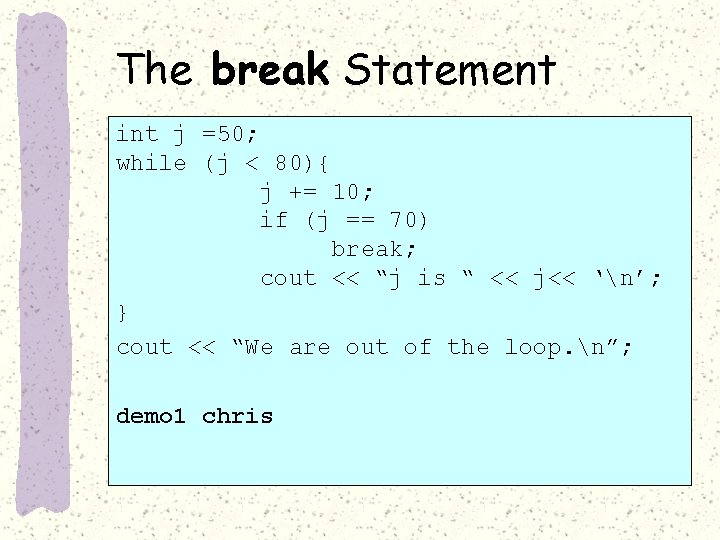
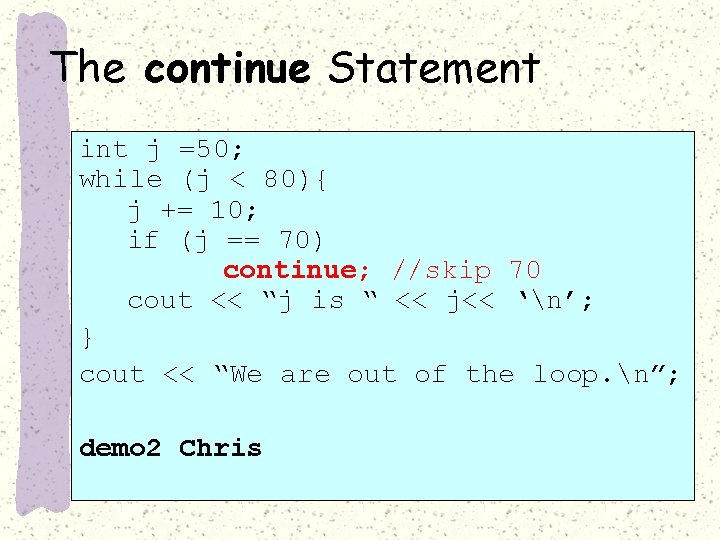
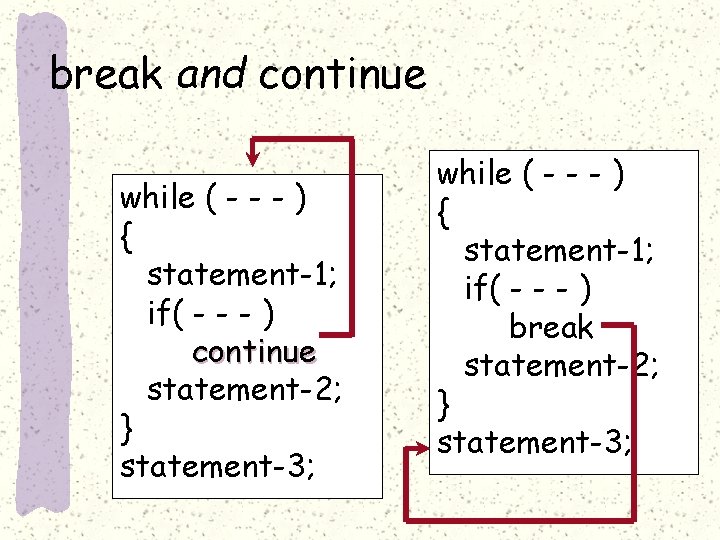
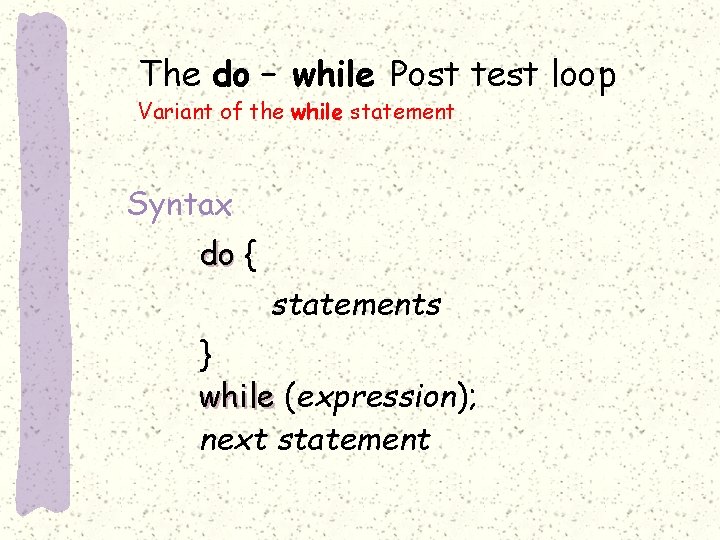
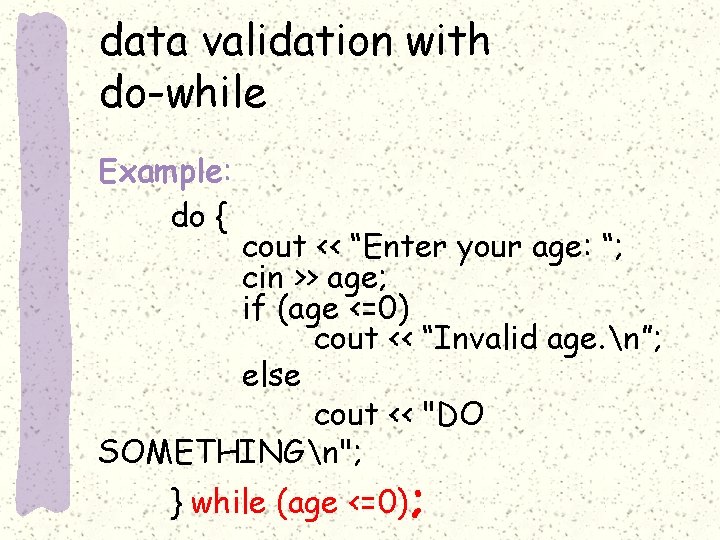
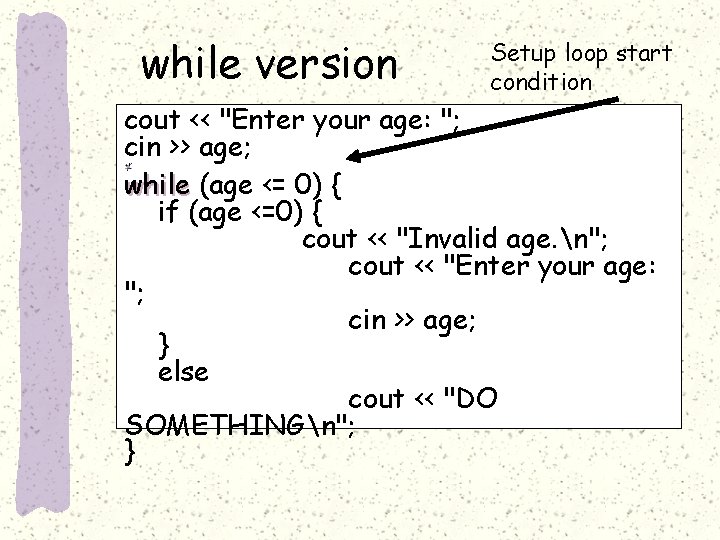
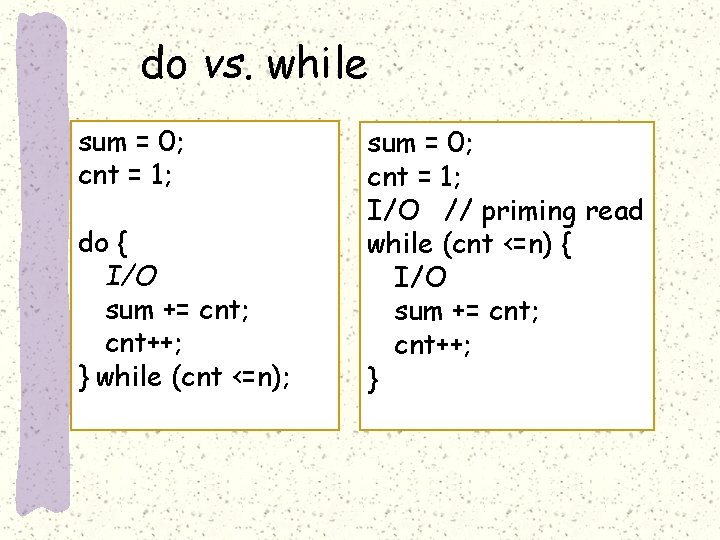
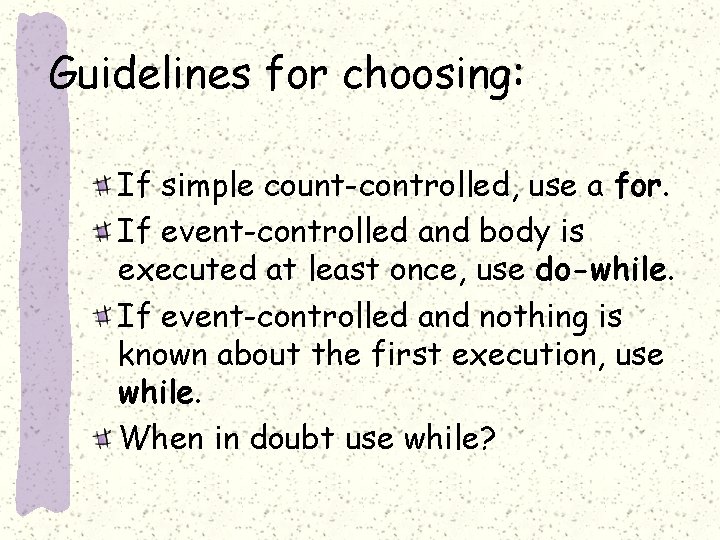
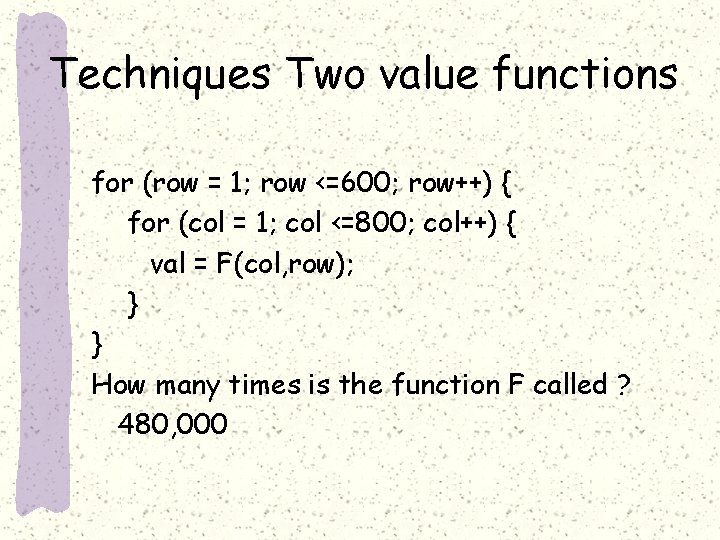
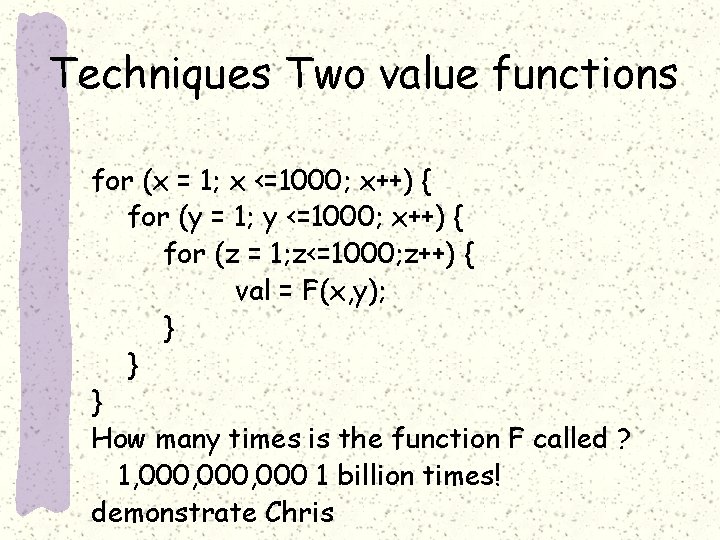
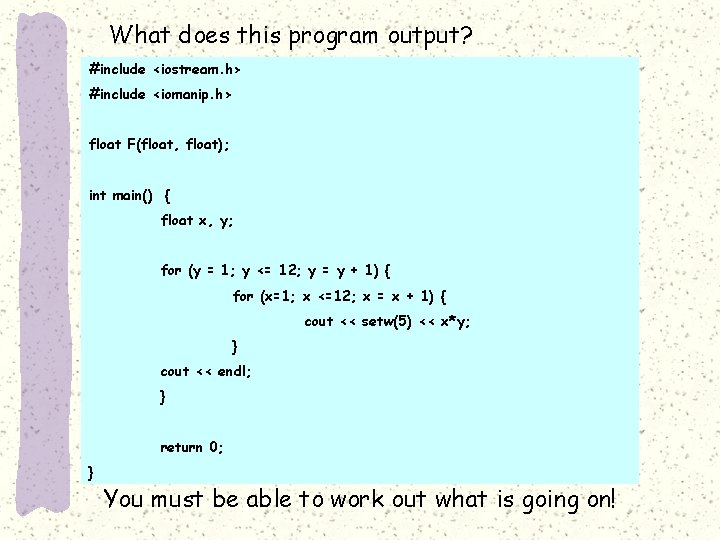
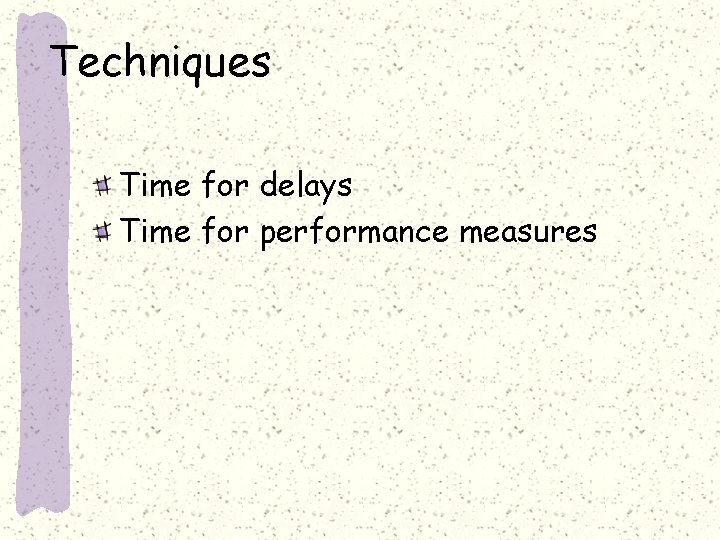
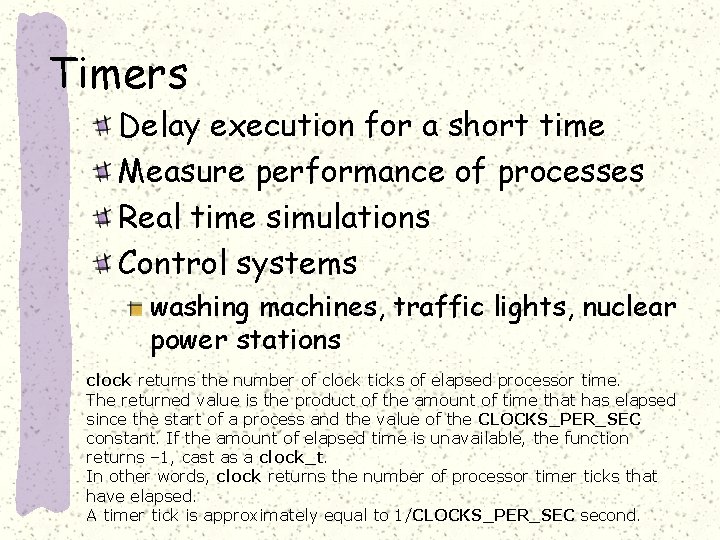
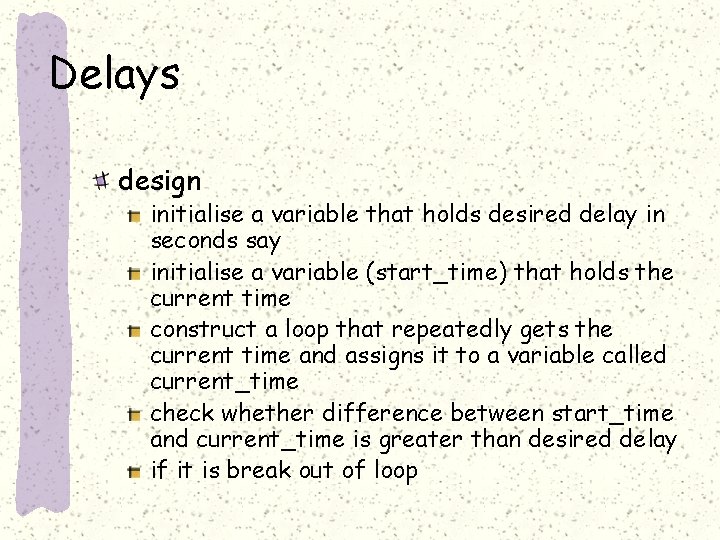
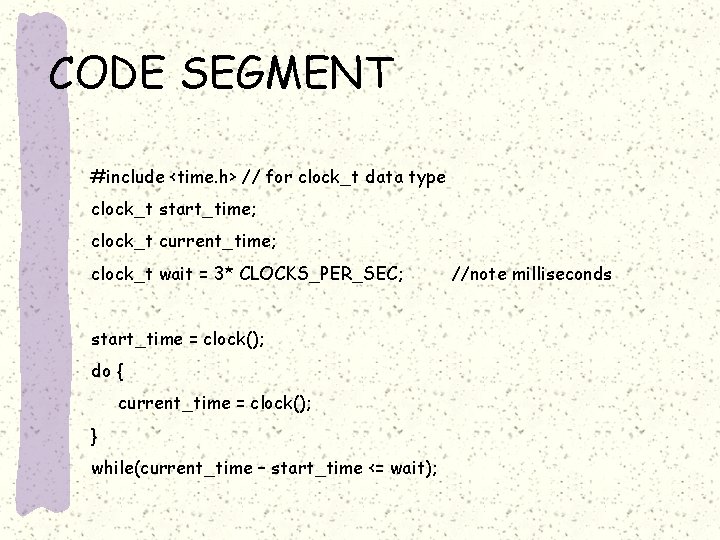
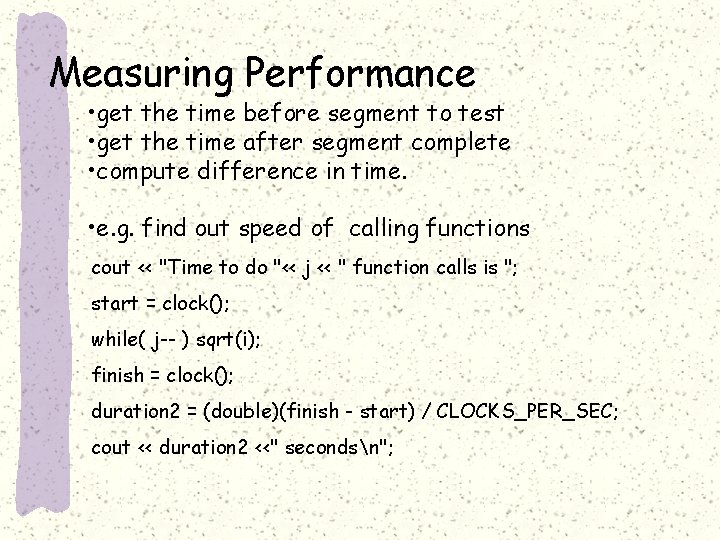
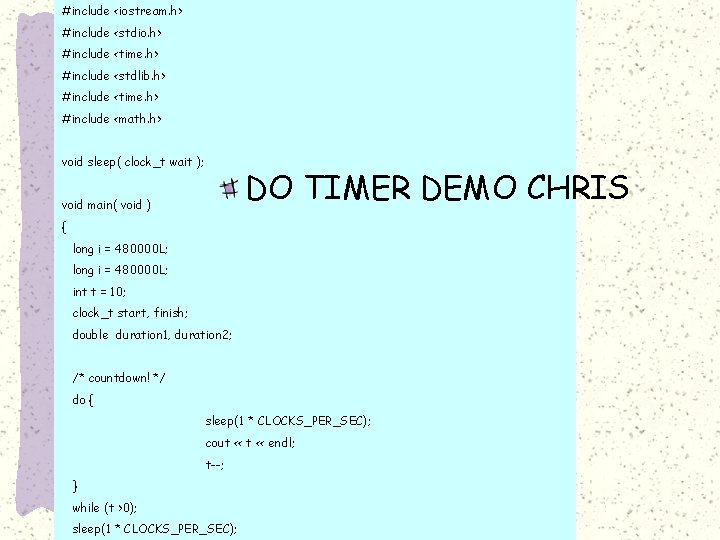
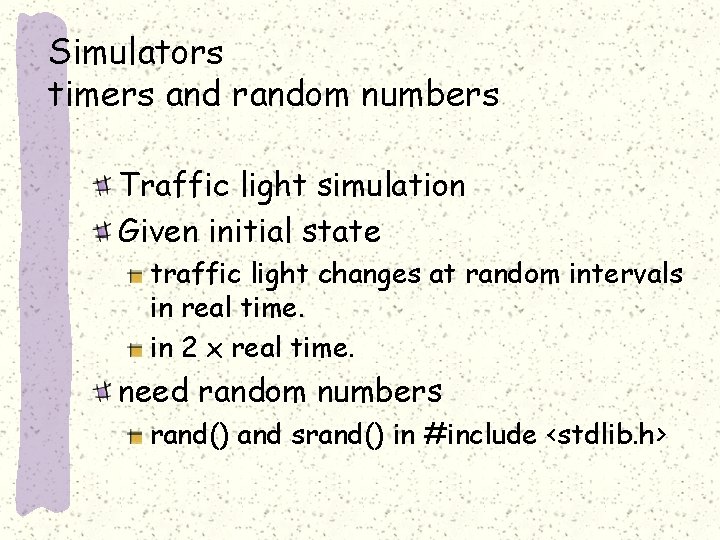
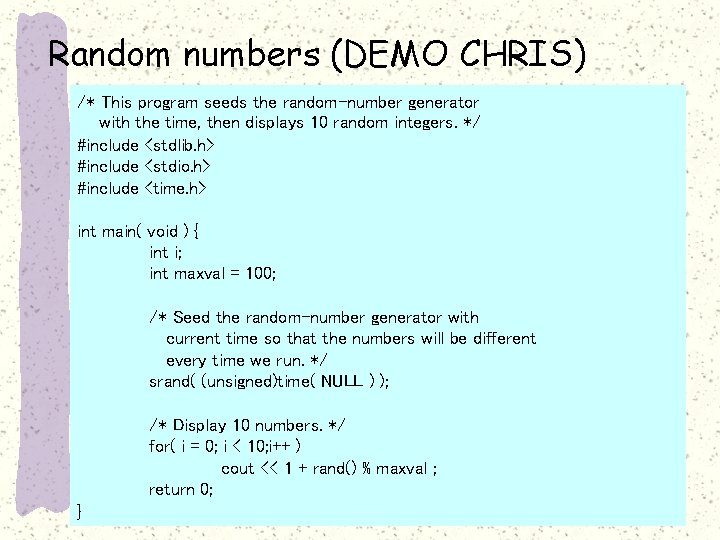
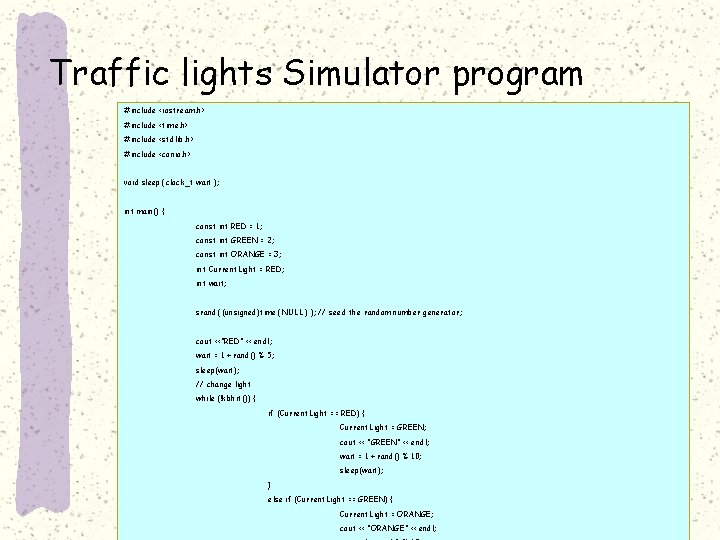
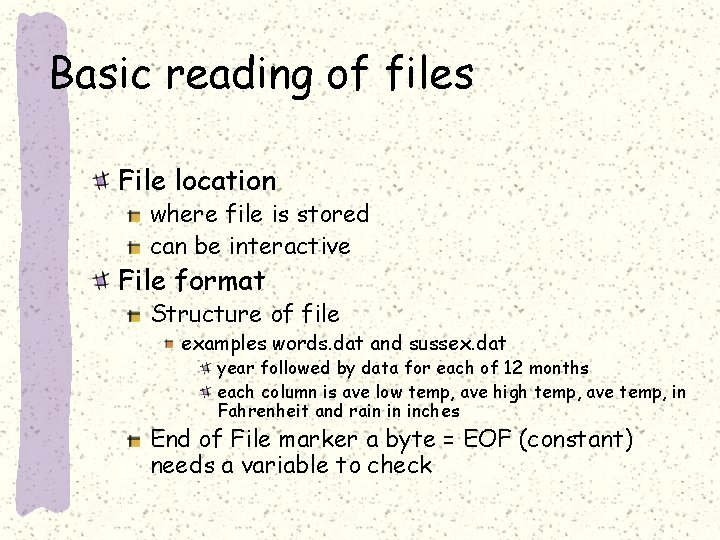
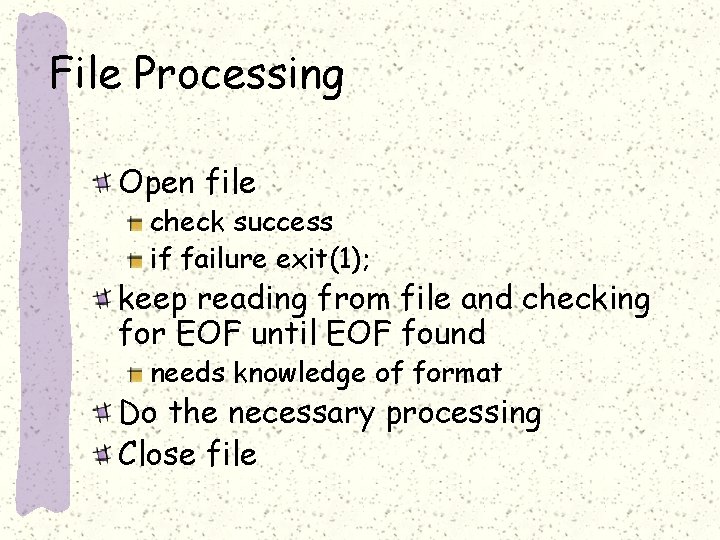
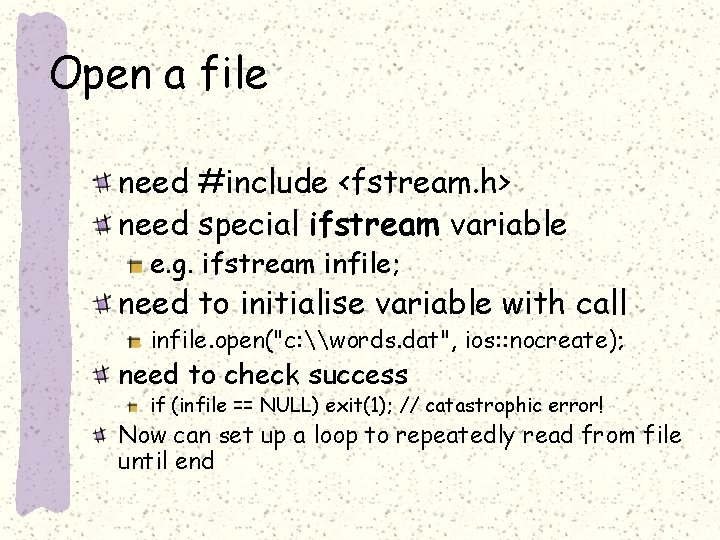
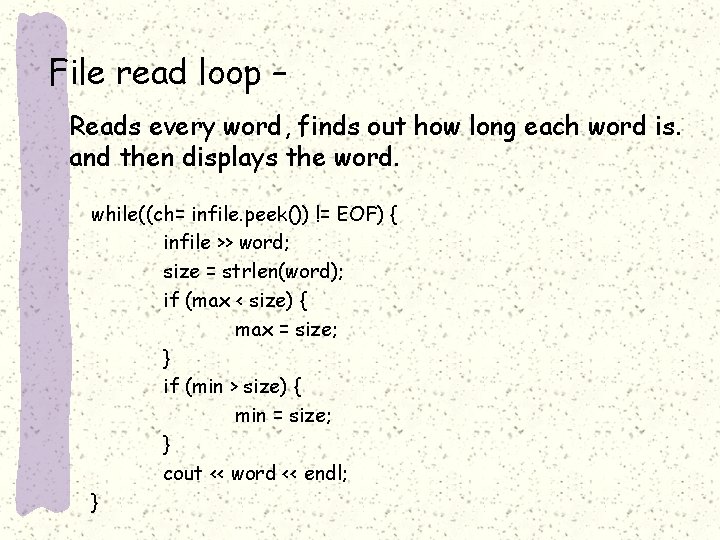
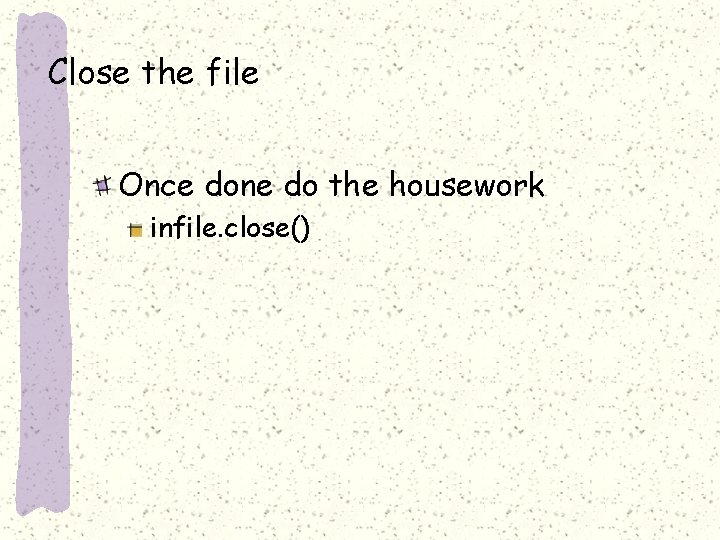
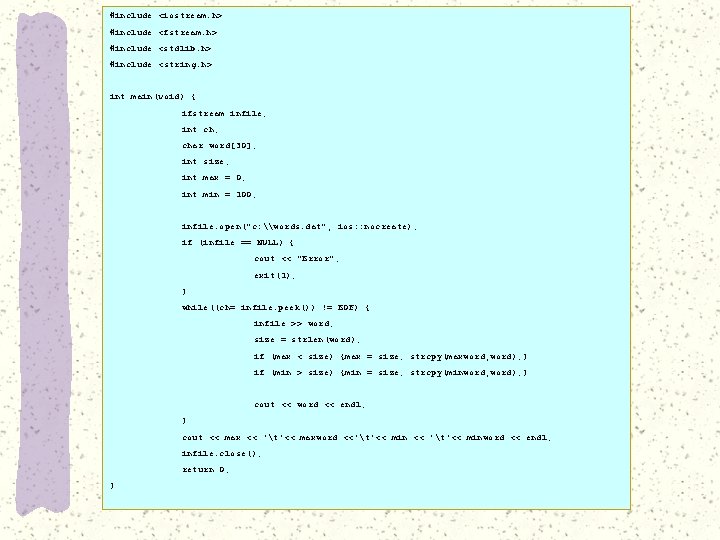
- Slides: 32
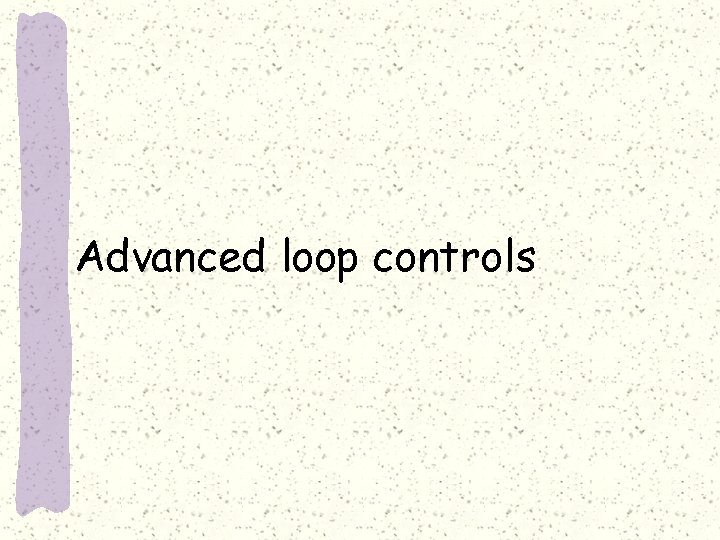
Advanced loop controls
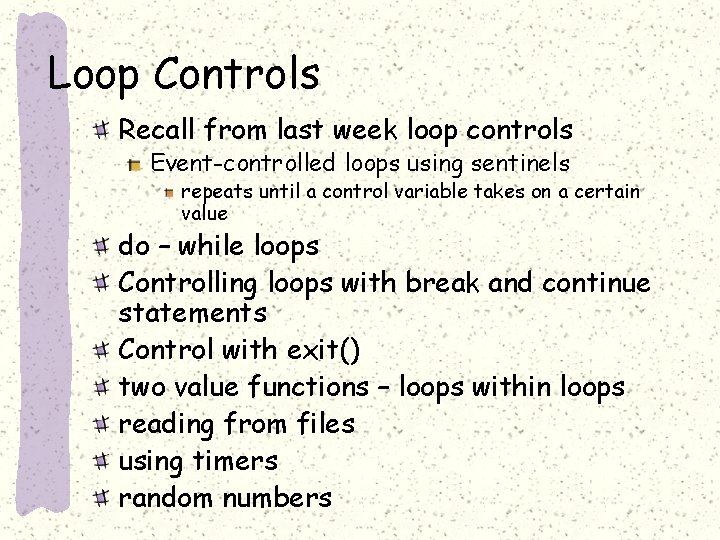
Loop Controls Recall from last week loop controls Event-controlled loops using sentinels repeats until a control variable takes on a certain value do – while loops Controlling loops with break and continue statements Control with exit() two value functions – loops within loops reading from files using timers random numbers
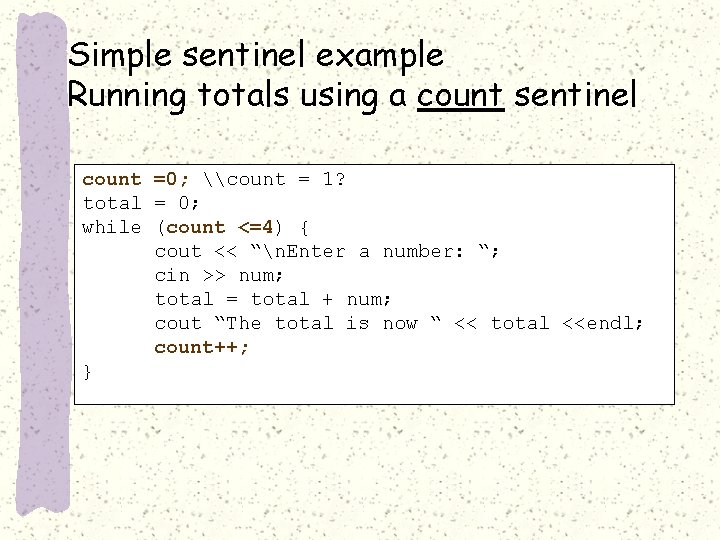
Simple sentinel example Running totals using a count sentinel count =0; \count = 1? total = 0; while (count <=4) { cout << “n. Enter a number: “; cin >> num; total = total + num; cout “The total is now “ << total <<endl; count++; }
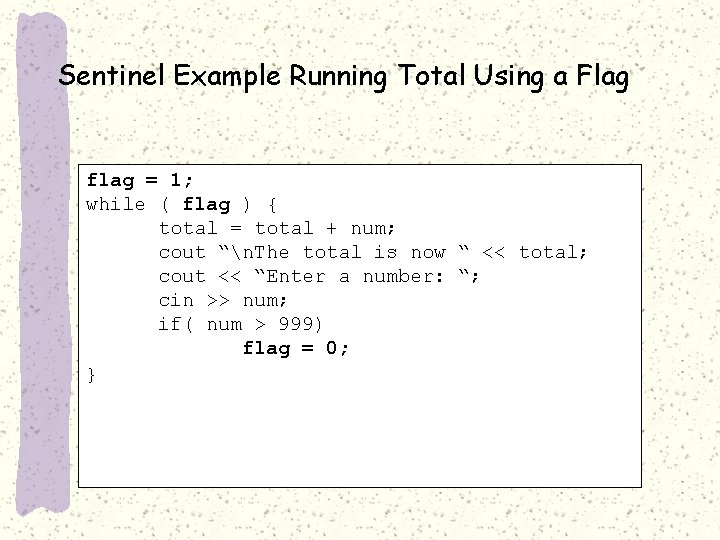
Sentinel Example Running Total Using a Flag flag = 1; while ( flag ) { total = total + num; cout “n. The total is now “ << total; cout << “Enter a number: “; cin >> num; if( num > 999) flag = 0; }
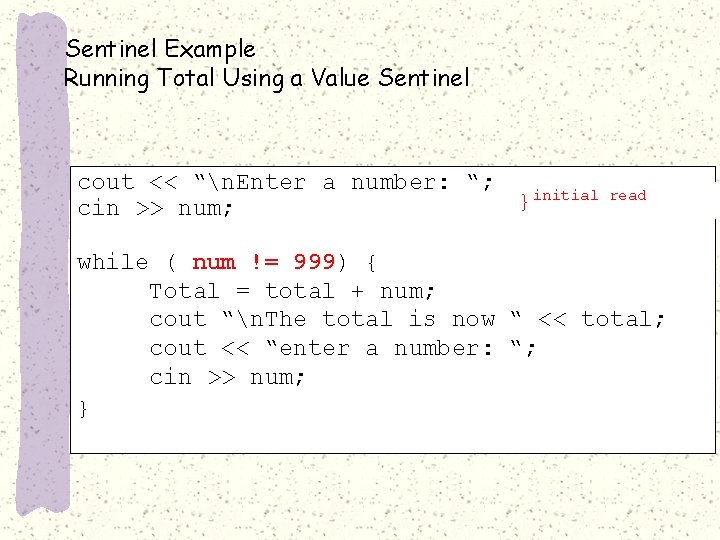
Sentinel Example Running Total Using a Value Sentinel cout << “n. Enter a number: “; }initial cin >> num; read while ( num != 999) { Total = total + num; cout “n. The total is now “ << total; cout << “enter a number: “; cin >> num; }
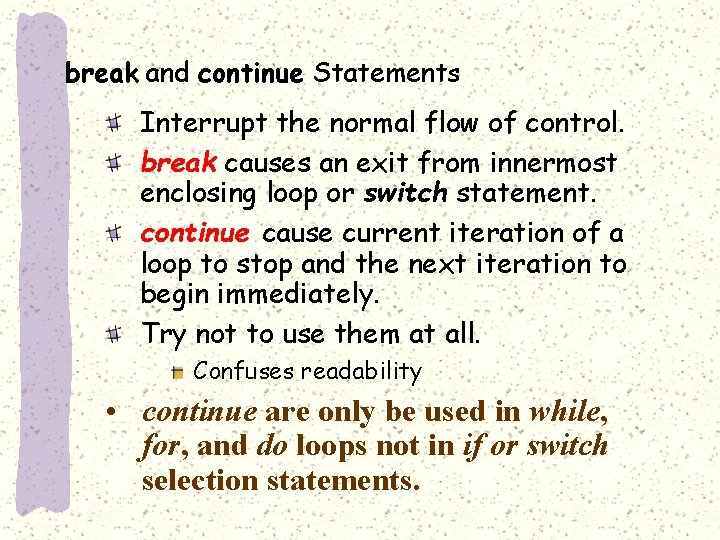
break and continue Statements Interrupt the normal flow of control. break causes an exit from innermost enclosing loop or switch statement. continue cause current iteration of a loop to stop and the next iteration to begin immediately. Try not to use them at all. Confuses readability • continue are only be used in while, for, and do loops not in if or switch selection statements.
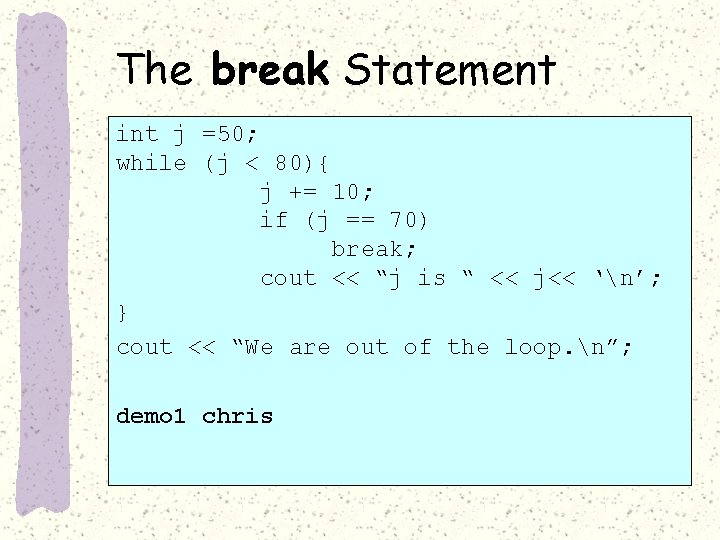
The break Statement int j =50; while (j < 80){ j += 10; if (j == 70) break; cout << “j is “ << j<< ‘n’; } cout << “We are out of the loop. n”; demo 1 chris
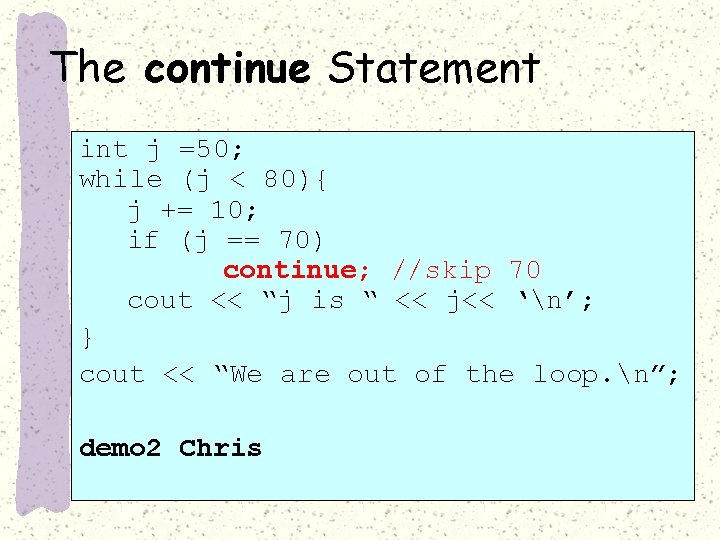
The continue Statement int j =50; while (j < 80){ j += 10; if (j == 70) continue; //skip 70 cout << “j is “ << j<< ‘n’; } cout << “We are out of the loop. n”; demo 2 Chris
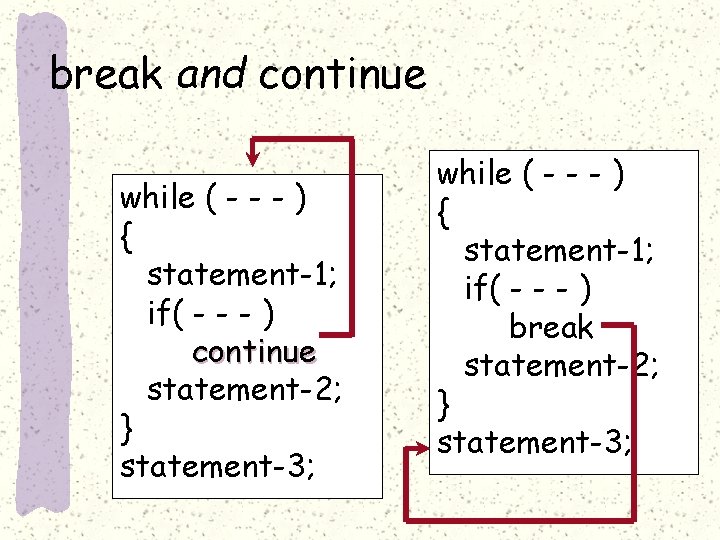
break and continue while ( - - - ) { statement-1; if( - - - ) continue statement-2; } statement-3; while ( - - - ) { statement-1; if( - - - ) break statement-2; } statement-3;
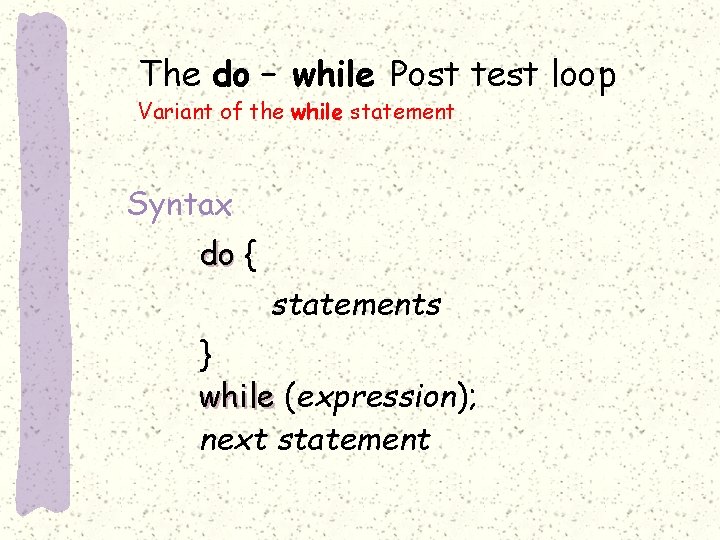
The do – while Post test loop Variant of the while statement Syntax do { statements } while (expression); next statement
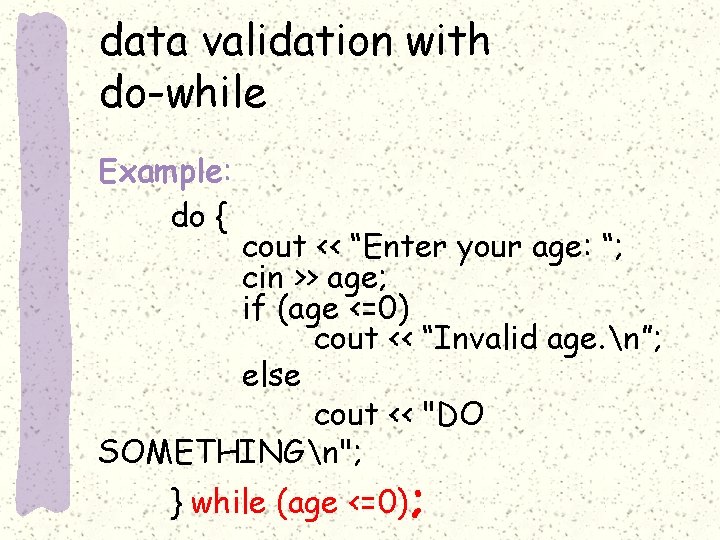
data validation with do-while Example: do { cout << “Enter your age: “; cin >> age; if (age <=0) cout << “Invalid age. n”; else cout << "DO SOMETHINGn"; } while (age <=0);
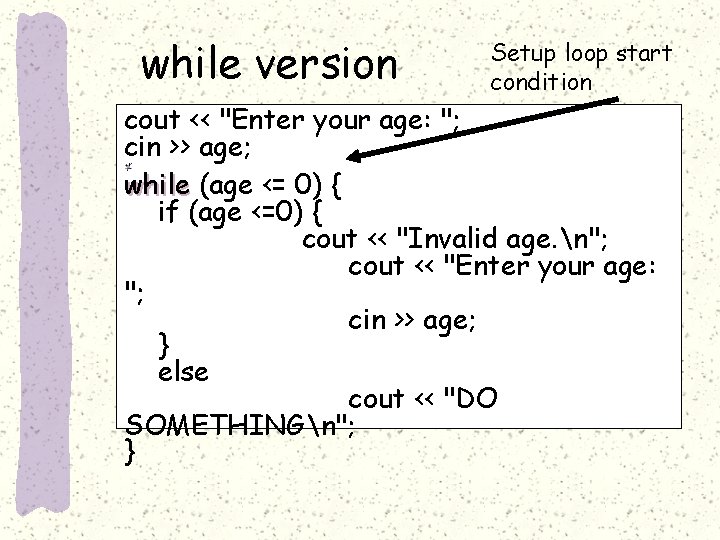
while version Setup loop start condition cout << "Enter your age: "; cin >> age; while (age <= 0) { if (age <=0) { cout << "Invalid age. n"; cout << "Enter your age: "; cin >> age; } else cout << "DO SOMETHINGn"; }
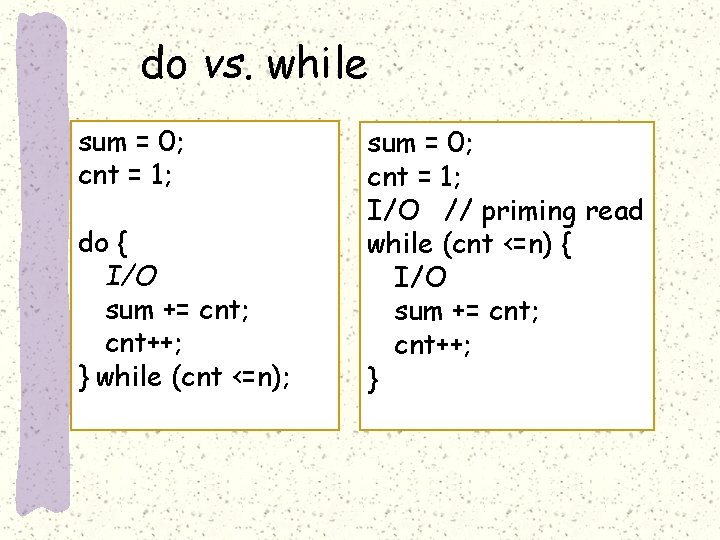
do vs. while sum = 0; cnt = 1; do { I/O sum += cnt; cnt++; } while (cnt <=n); sum = 0; cnt = 1; I/O // priming read while (cnt <=n) { I/O sum += cnt; cnt++; }
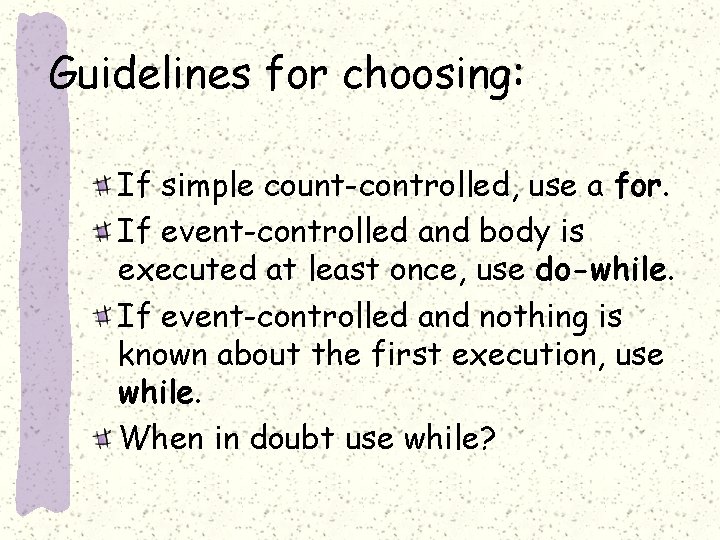
Guidelines for choosing: If simple count-controlled, use a for. If event-controlled and body is executed at least once, use do-while. If event-controlled and nothing is known about the first execution, use while. When in doubt use while?
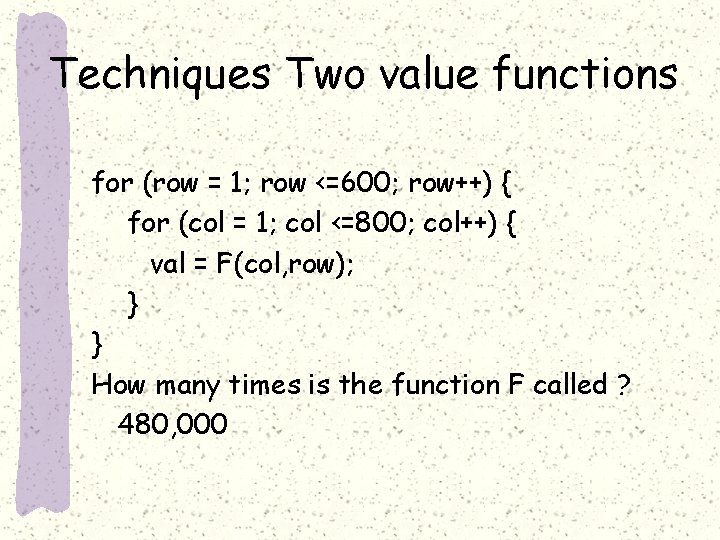
Techniques Two value functions for (row = 1; row <=600; row++) { for (col = 1; col <=800; col++) { val = F(col, row); } } How many times is the function F called ? 480, 000
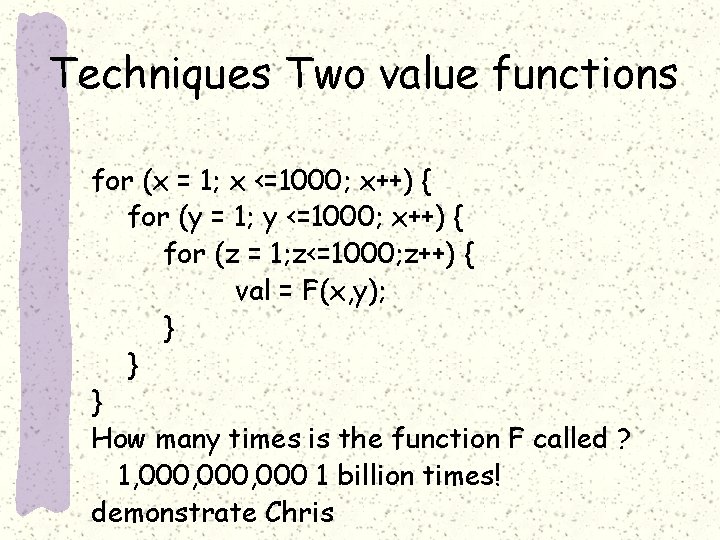
Techniques Two value functions for (x = 1; x <=1000; x++) { for (y = 1; y <=1000; x++) { for (z = 1; z<=1000; z++) { val = F(x, y); } } } How many times is the function F called ? 1, 000, 000 1 billion times! demonstrate Chris
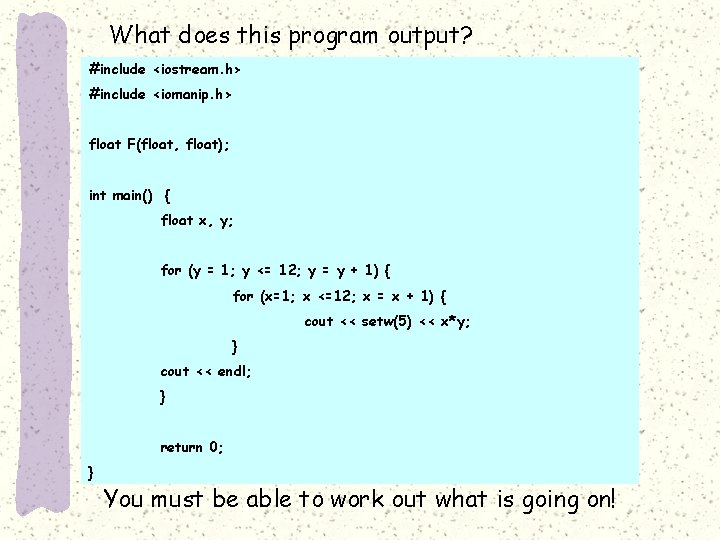
What does this program output? #include <iostream. h> #include <iomanip. h> float F(float, float); int main() { float x, y; for (y = 1; y <= 12; y = y + 1) { for (x=1; x <=12; x = x + 1) { cout << setw(5) << x*y; } cout << endl; } return 0; } You must be able to work out what is going on!
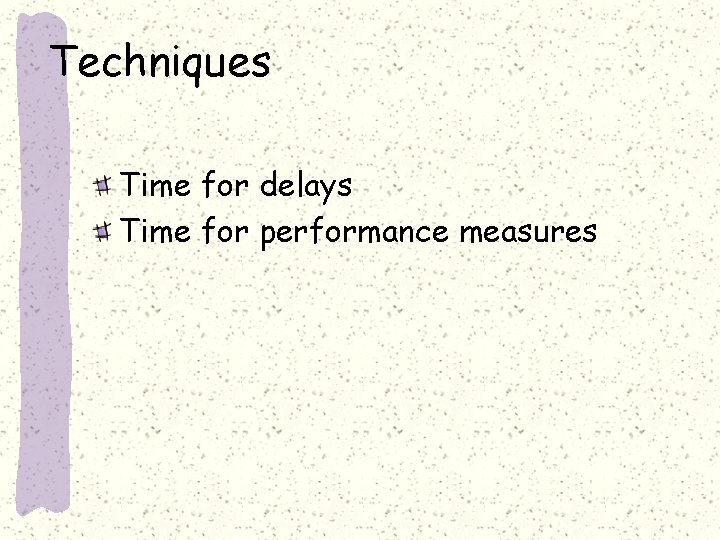
Techniques Time for delays Time for performance measures
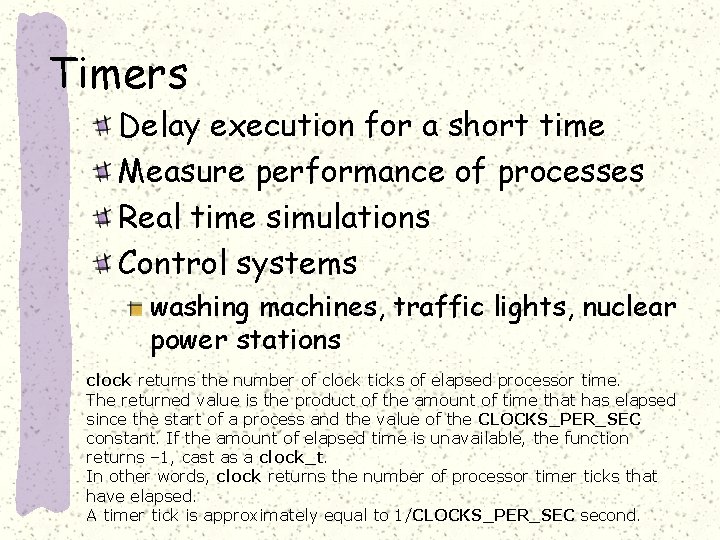
Timers Delay execution for a short time Measure performance of processes Real time simulations Control systems washing machines, traffic lights, nuclear power stations clock returns the number of clock ticks of elapsed processor time. The returned value is the product of the amount of time that has elapsed since the start of a process and the value of the CLOCKS_PER_SEC constant. If the amount of elapsed time is unavailable, the function returns – 1, cast as a clock_t. In other words, clock returns the number of processor timer ticks that have elapsed. A timer tick is approximately equal to 1/CLOCKS_PER_SEC second.
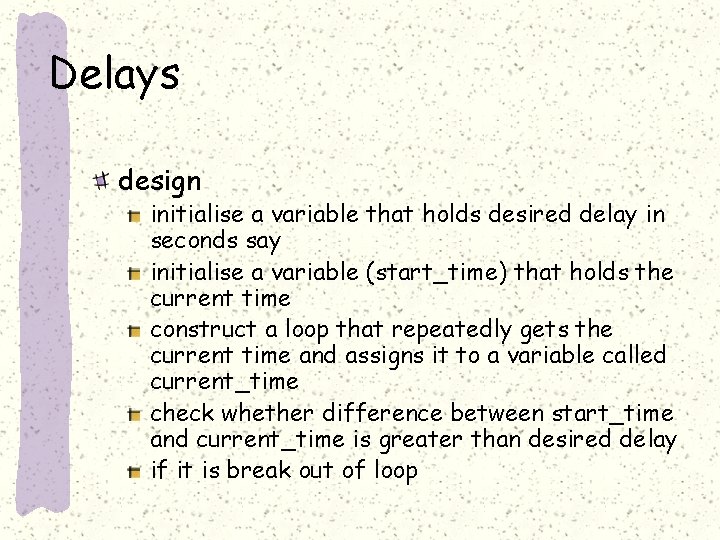
Delays design initialise a variable that holds desired delay in seconds say initialise a variable (start_time) that holds the current time construct a loop that repeatedly gets the current time and assigns it to a variable called current_time check whether difference between start_time and current_time is greater than desired delay if it is break out of loop
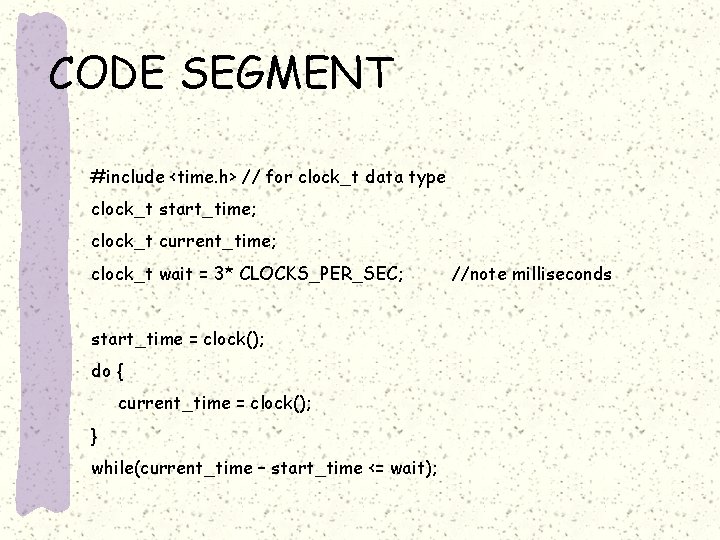
CODE SEGMENT #include <time. h> // for clock_t data type clock_t start_time; clock_t current_time; clock_t wait = 3* CLOCKS_PER_SEC; start_time = clock(); do { current_time = clock(); } while(current_time – start_time <= wait); //note milliseconds
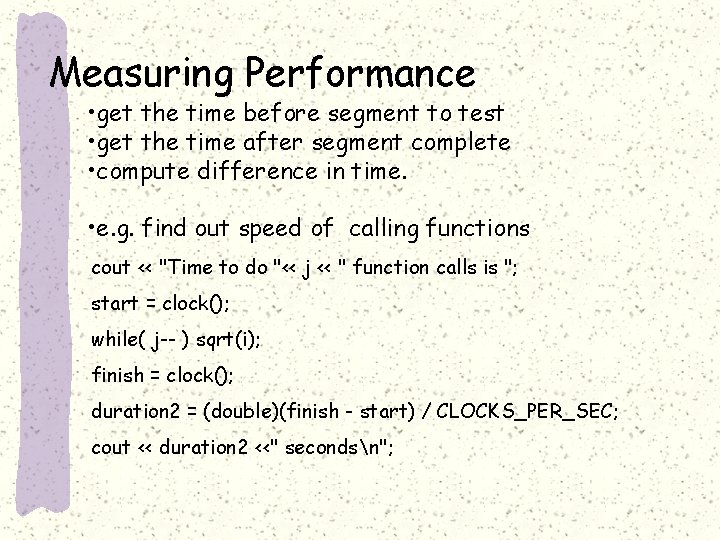
Measuring Performance • get the time before segment to test • get the time after segment complete • compute difference in time. • e. g. find out speed of calling functions cout << "Time to do "<< j << " function calls is "; start = clock(); while( j-- ) sqrt(i); finish = clock(); duration 2 = (double)(finish - start) / CLOCKS_PER_SEC; cout << duration 2 <<" secondsn";
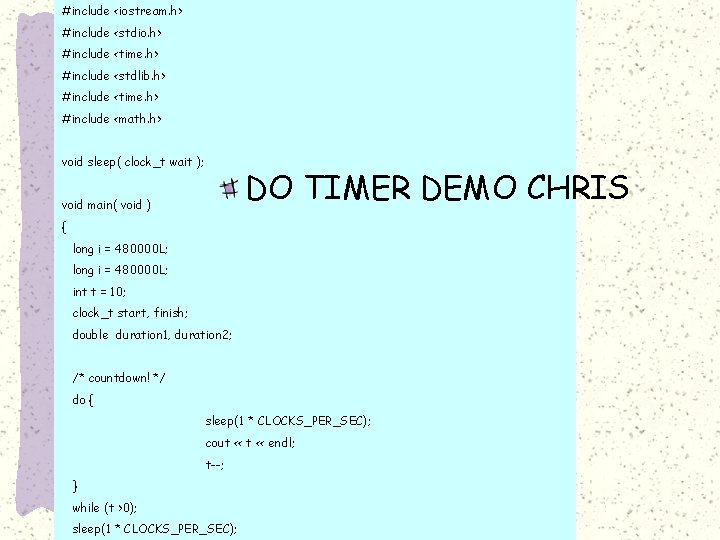
#include <iostream. h> #include <stdio. h> #include <time. h> #include <stdlib. h> #include <time. h> #include <math. h> void sleep( clock_t wait ); DO TIMER DEMO CHRIS void main( void ) { long i = 480000 L; int t = 10; clock_t start, finish; double duration 1, duration 2; /* countdown! */ do { sleep(1 * CLOCKS_PER_SEC); cout << endl; t--; } while (t >0); sleep(1 * CLOCKS_PER_SEC);
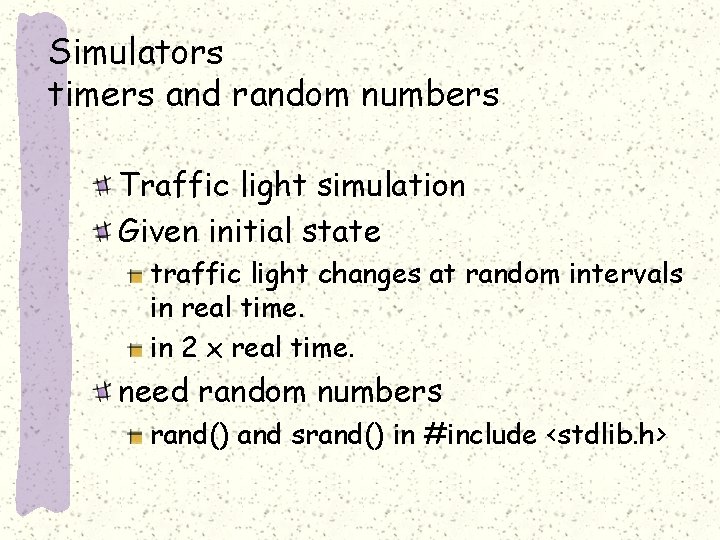
Simulators timers and random numbers Traffic light simulation Given initial state traffic light changes at random intervals in real time. in 2 x real time. need random numbers rand() and srand() in #include <stdlib. h>
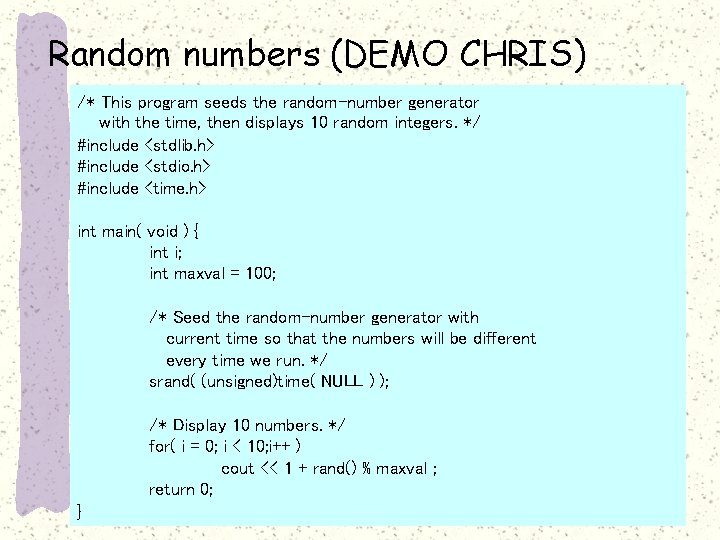
Random numbers (DEMO CHRIS) /* This program seeds the random-number generator with the time, then displays 10 random integers. */ #include <stdlib. h> #include <stdio. h> #include <time. h> int main( void ) { int i; int maxval = 100; /* Seed the random-number generator with current time so that the numbers will be different every time we run. */ srand( (unsigned)time( NULL ) ); /* Display 10 numbers. */ for( i = 0; i < 10; i++ ) cout << 1 + rand() % maxval ; return 0; }
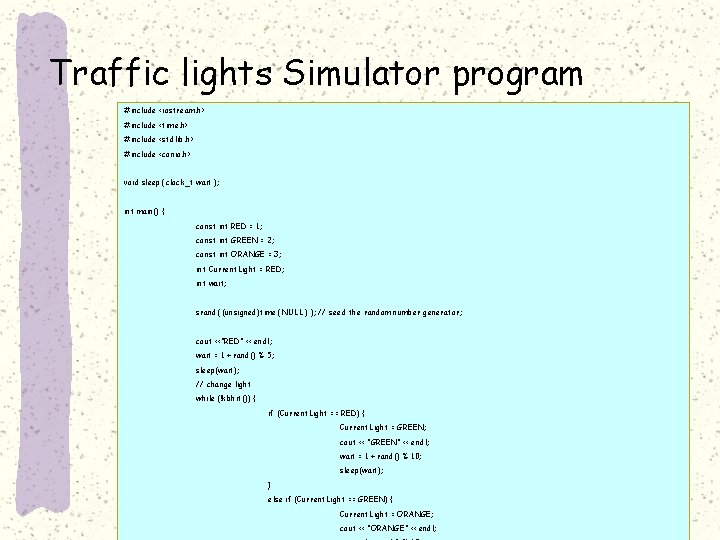
Traffic lights Simulator program #include <iostream. h> #include <time. h> #include <stdlib. h> #include <conio. h> void sleep( clock_t wait ); int main() { const int RED = 1; const int GREEN = 2; const int ORANGE = 3; int Current. Light = RED; int wait; srand( (unsigned)time( NULL ) ); // seed the random number generator; cout <<"RED" << endl; wait = 1 + rand() % 5; sleep(wait); // change light while (!kbhit()) { if (Current. Light == RED) { Current. Light = GREEN; cout << "GREEN" << endl; wait = 1 + rand() % 10; sleep(wait); } else if (Current. Light == GREEN) { Current. Light = ORANGE; cout << "ORANGE" << endl;
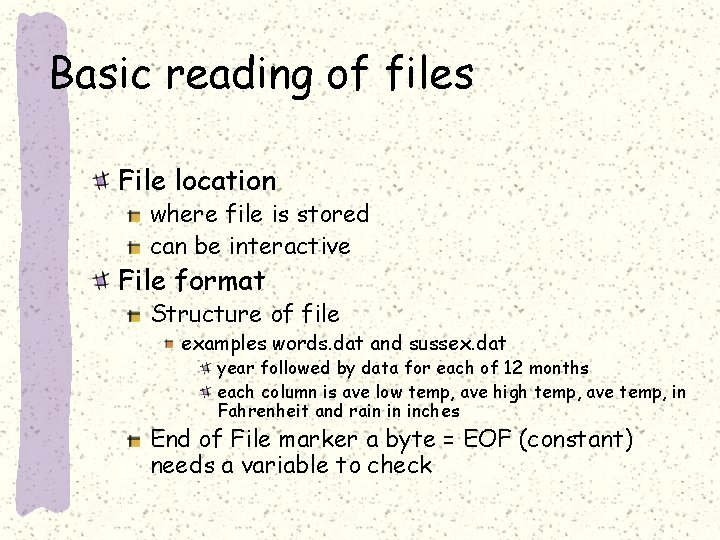
Basic reading of files File location where file is stored can be interactive File format Structure of file examples words. dat and sussex. dat year followed by data for each of 12 months each column is ave low temp, ave high temp, ave temp, in Fahrenheit and rain in inches End of File marker a byte = EOF (constant) needs a variable to check
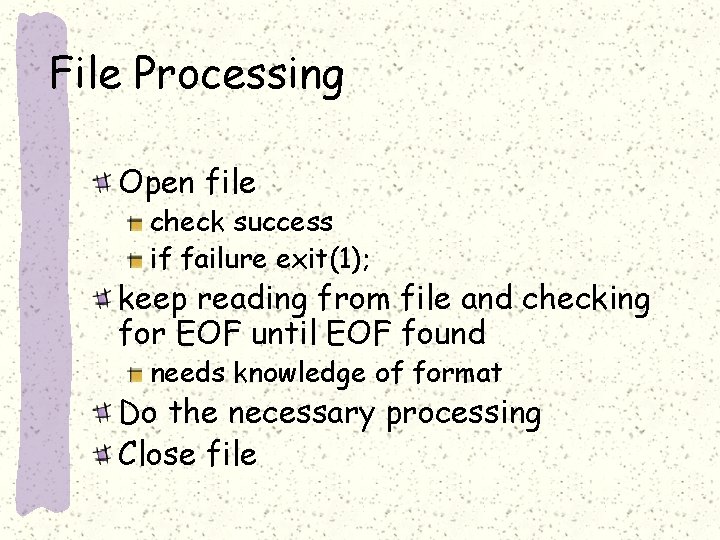
File Processing Open file check success if failure exit(1); keep reading from file and checking for EOF until EOF found needs knowledge of format Do the necessary processing Close file
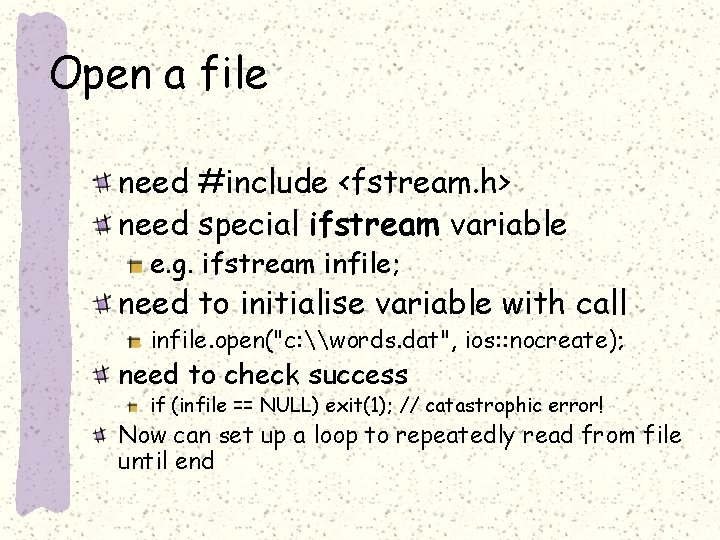
Open a file need #include <fstream. h> need special ifstream variable e. g. ifstream infile; need to initialise variable with call infile. open("c: \words. dat", ios: : nocreate); need to check success if (infile == NULL) exit(1); // catastrophic error! Now can set up a loop to repeatedly read from file until end
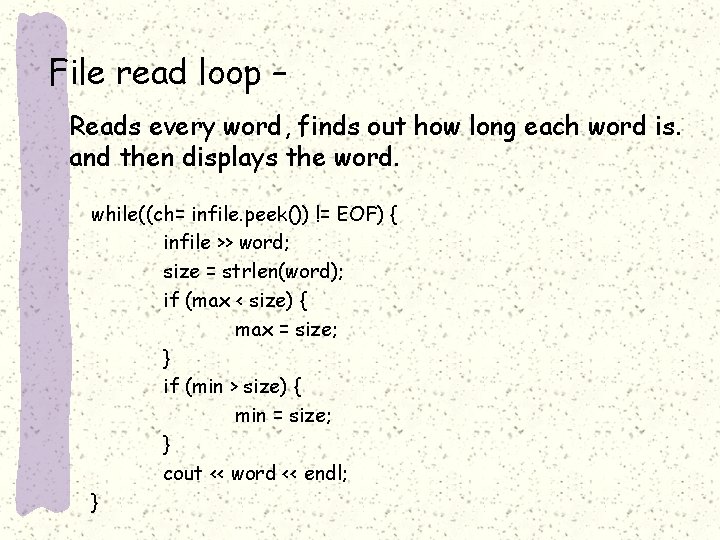
File read loop – Reads every word, finds out how long each word is. and then displays the word. while((ch= infile. peek()) != EOF) { infile >> word; size = strlen(word); if (max < size) { max = size; } if (min > size) { min = size; } cout << word << endl; }
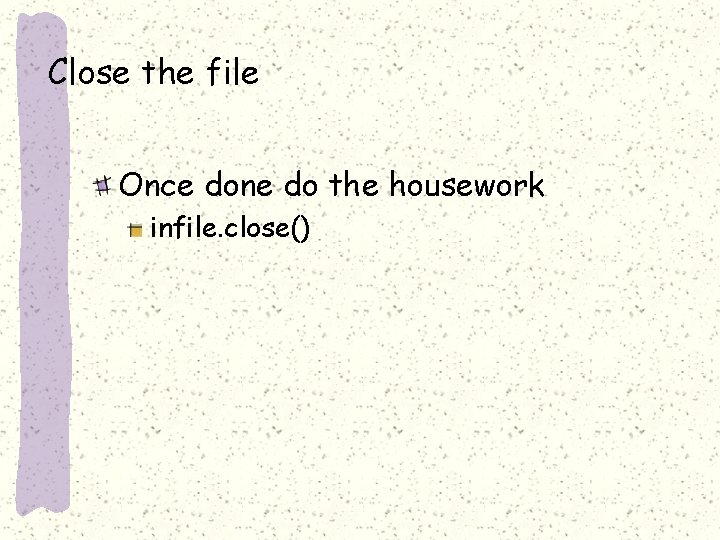
Close the file Once done do the housework infile. close()
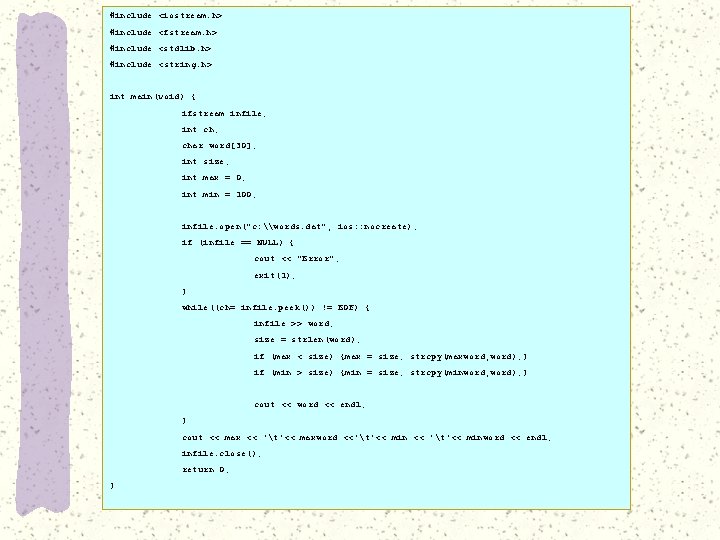
#include <iostream. h> #include <fstream. h> #include <stdlib. h> #include <string. h> int main(void) { ifstream infile; int ch; char word[30]; int size; int max = 0; int min = 100; infile. open("c: \words. dat", ios: : nocreate); if (infile == NULL) { cout << "Error"; exit(1); } while((ch= infile. peek()) != EOF) { infile >> word; size = strlen(word); if (max < size) {max = size; strcpy(maxword, word); } if (min > size) {min = size; strcpy(minword, word); } cout << word << endl; } cout << max << 't'<< maxword <<'t'<< min << 't'<< minword << endl; infile. close(); return 0; }
General controls vs application controls
He who controls the past controls the future
Diagram close loop
Statement while... wend digunakan untuk
Fifth gear loop the loop
Radial and ulnar loop
Open loop vs closed loop in cars
Multi loop pid controller regolatore pid multi loop
Manakah yang lebih baik open loop atau close loop system
Gos infant formula
Beef recall
Recall coordinator
3 step recall method
Recall the rules of probability
Gianttoby
Difference between recall and recognition
Active recall and spaced repetition
Aviane recall
Fight club recall formula
What is product mapping
Type of feature story
Let us reflect
Ionic recall
Recall column
Recall vs recognition
Recall lesson 6
Precision recall information retrieval
Recall
Beef recall
Beef recall
An inspector calls recall questions
Recall bias
Acb and bca puzzle