Program Control Topics While loop For loop Switch
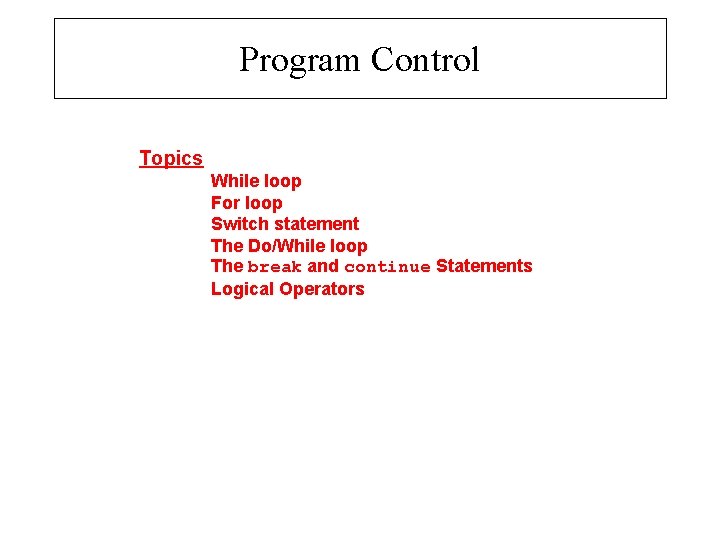
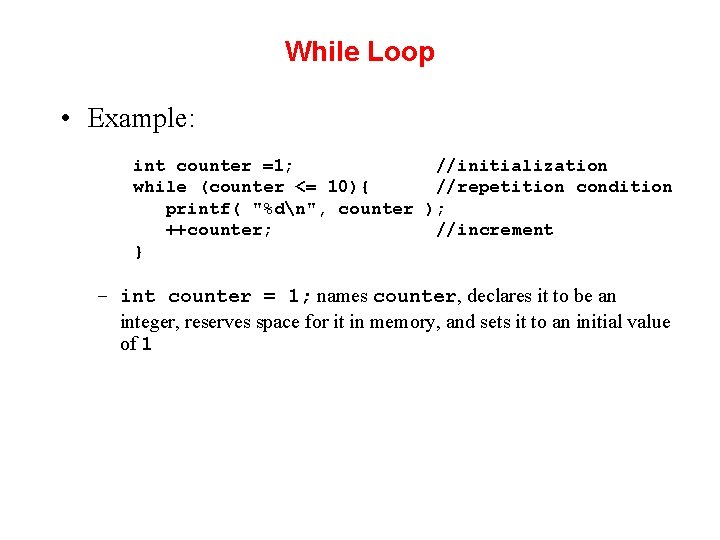
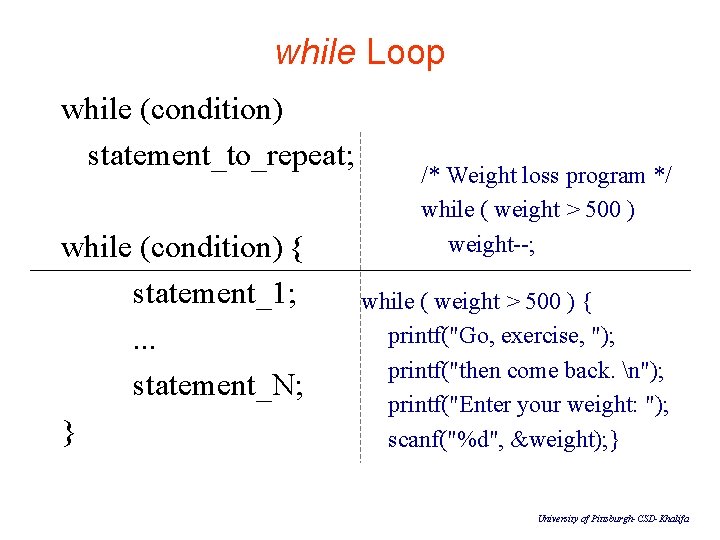
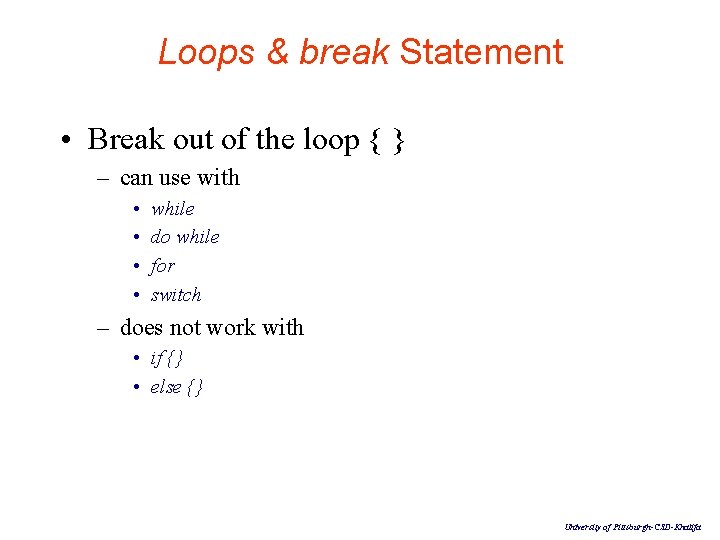
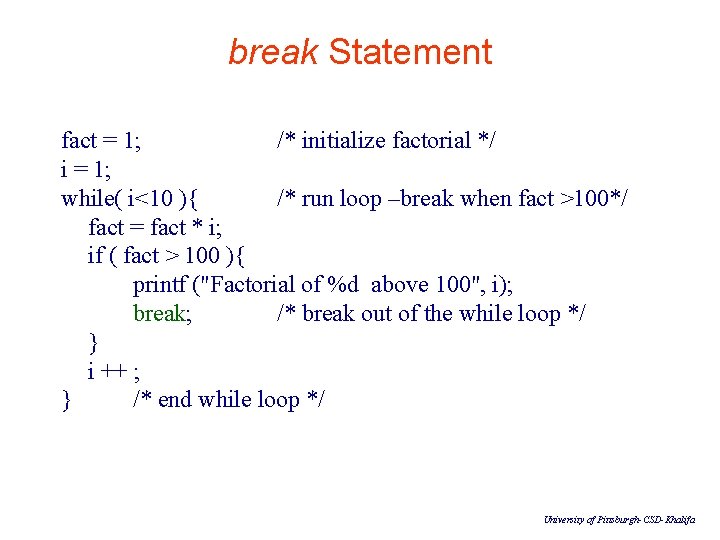
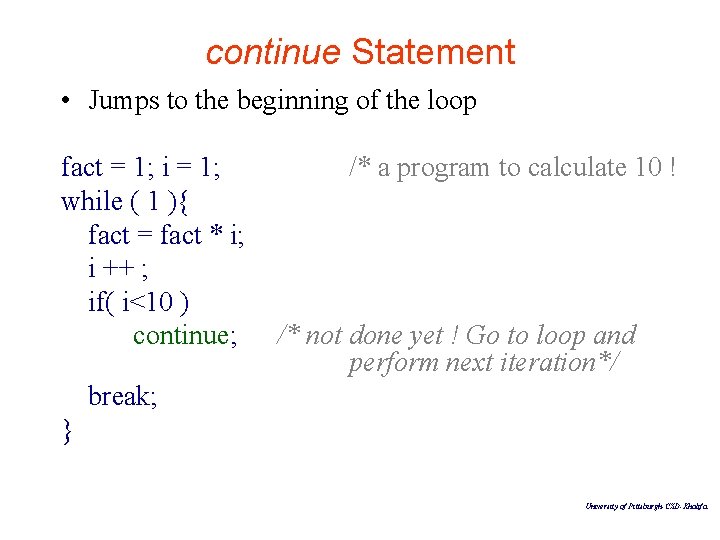
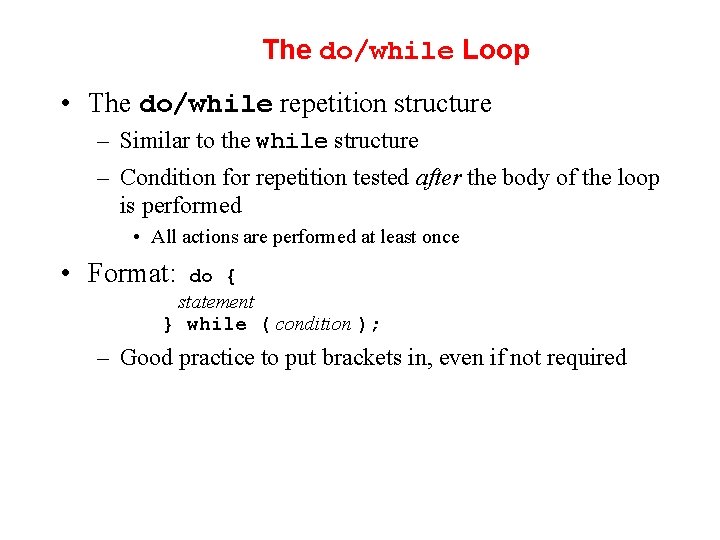
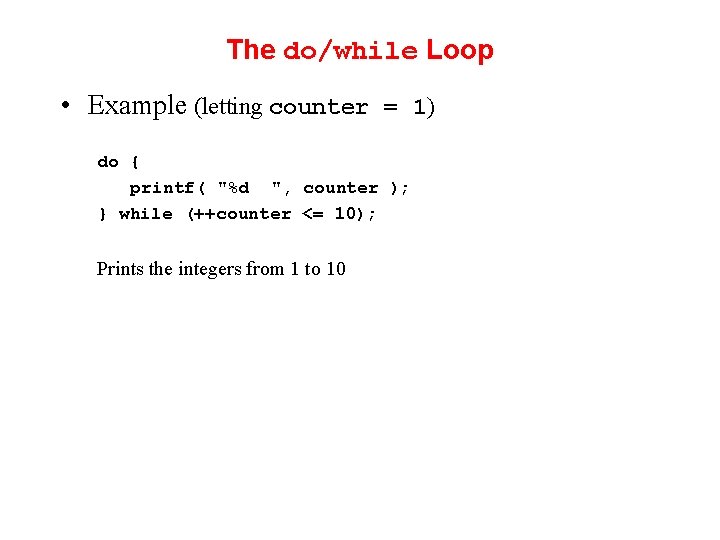
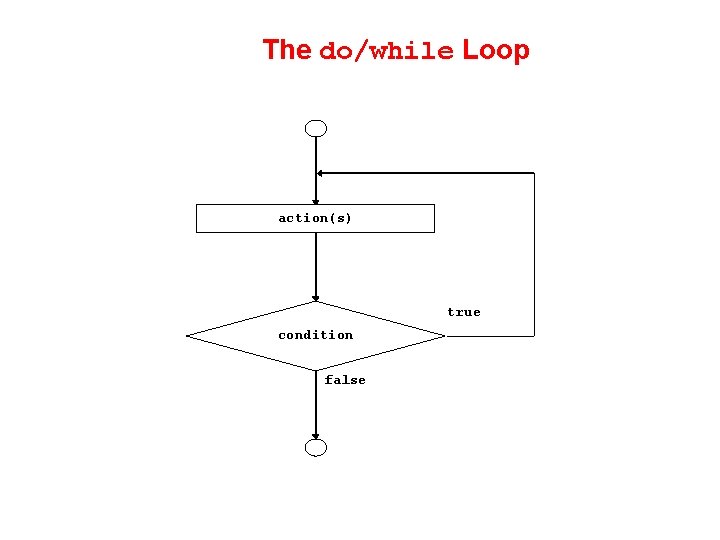
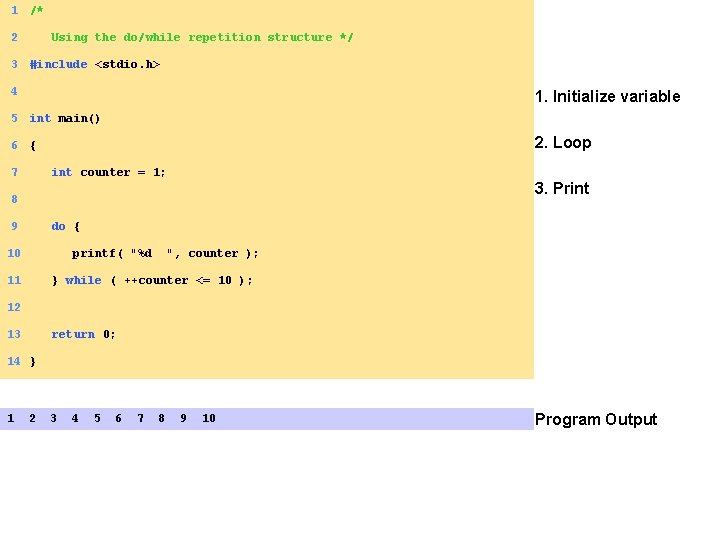
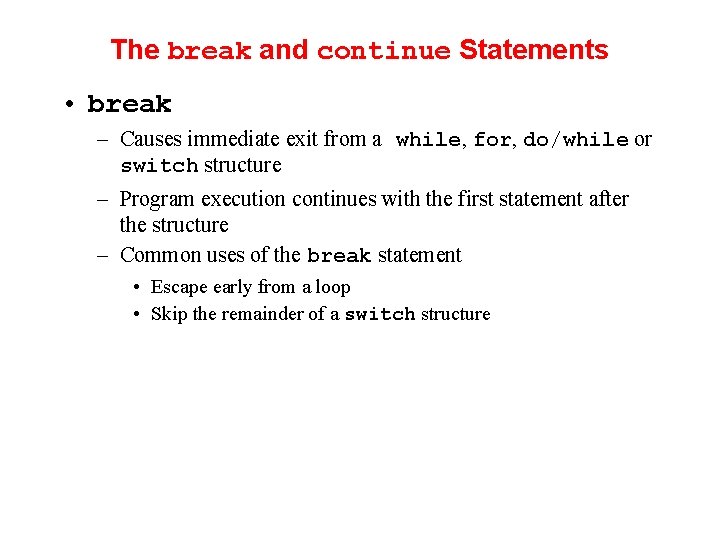
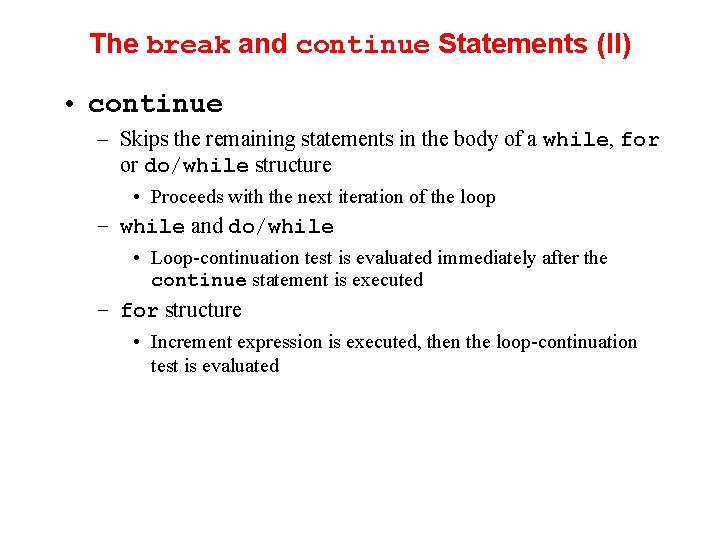
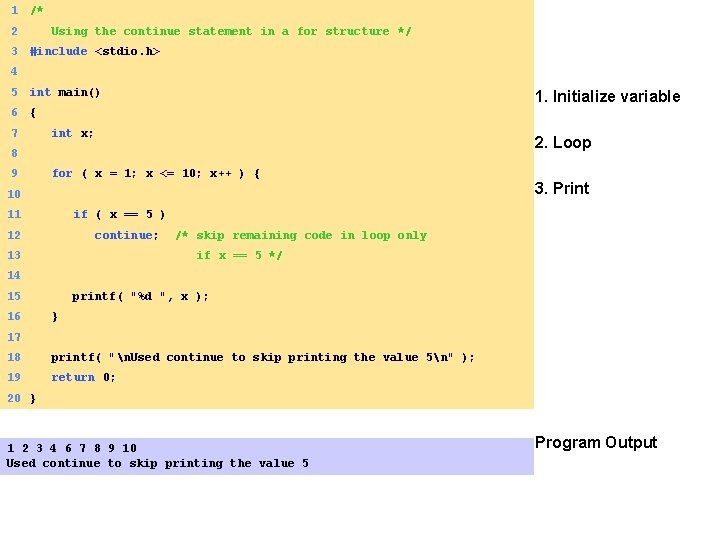
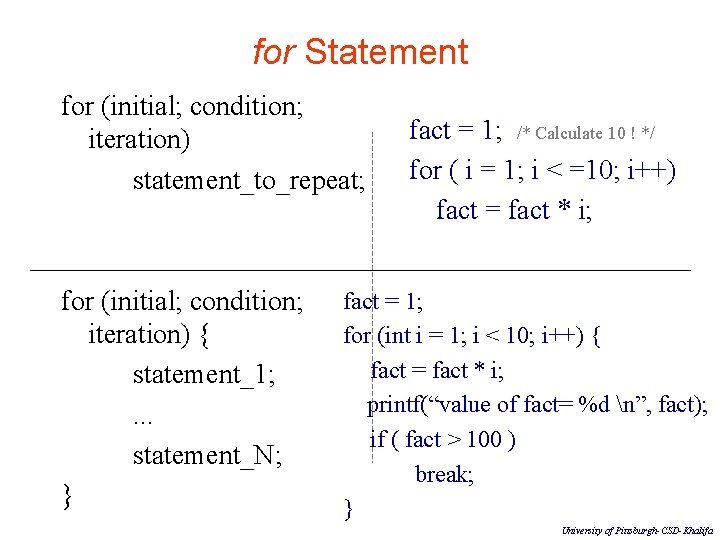
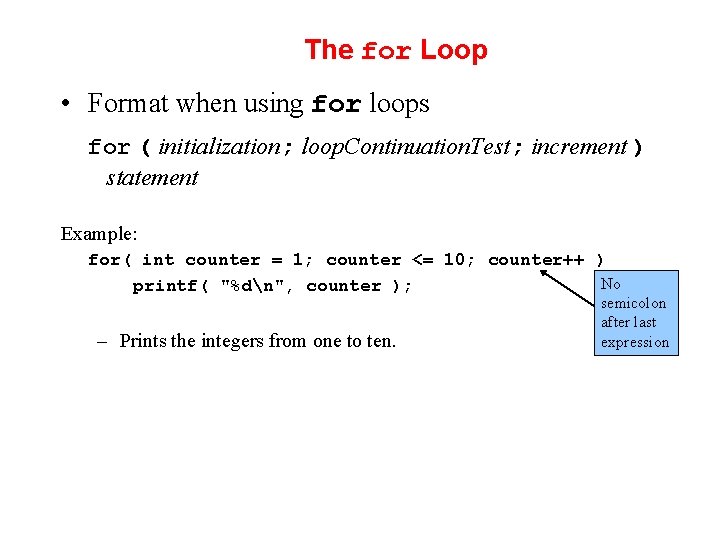
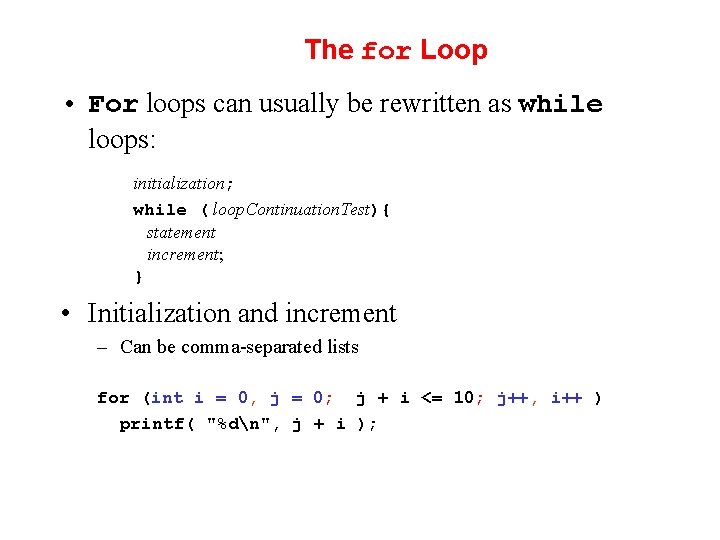
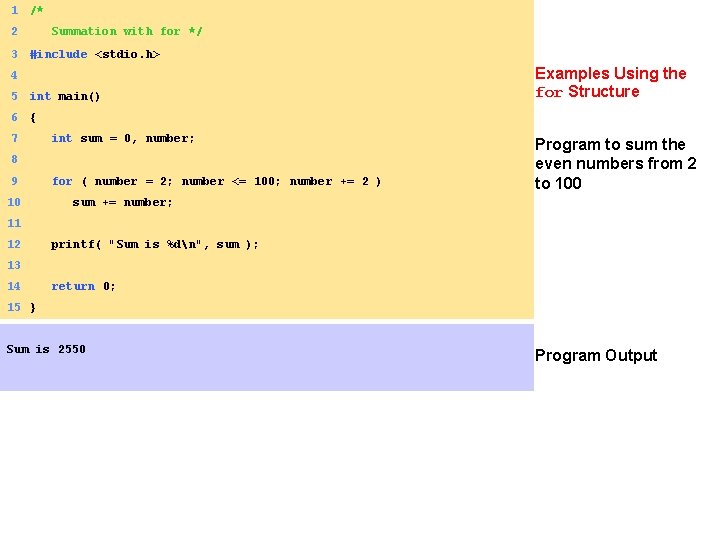
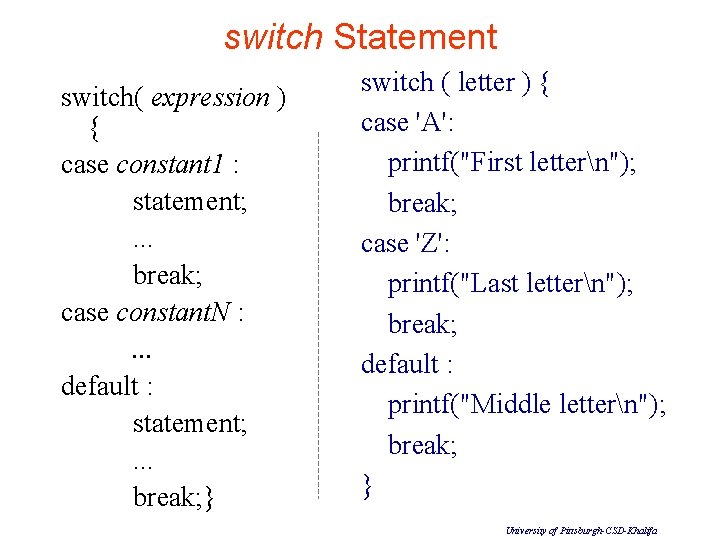
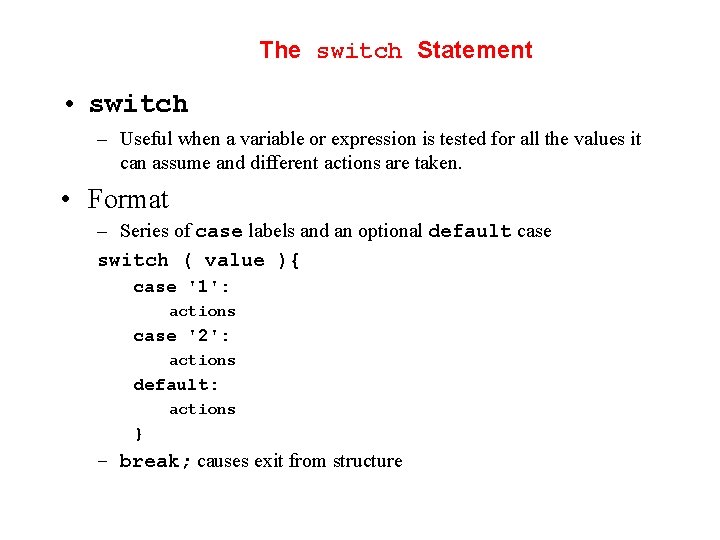
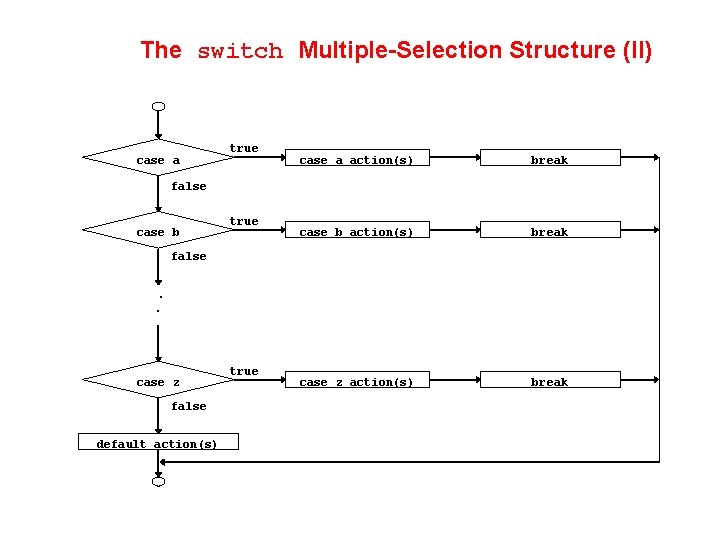
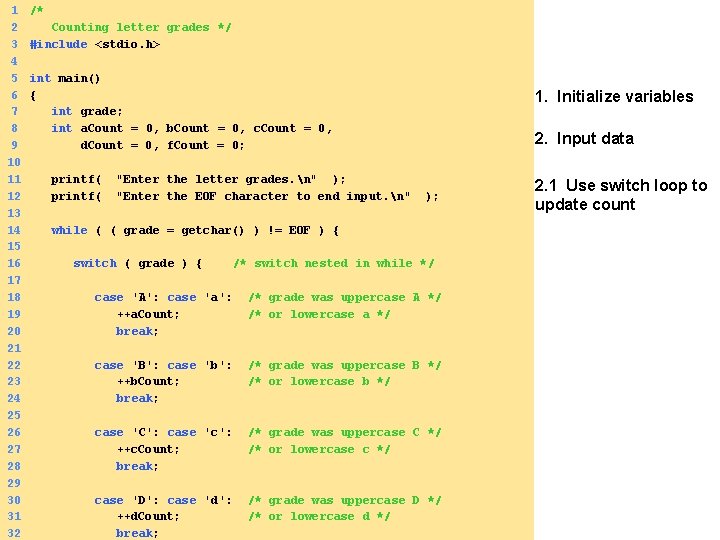
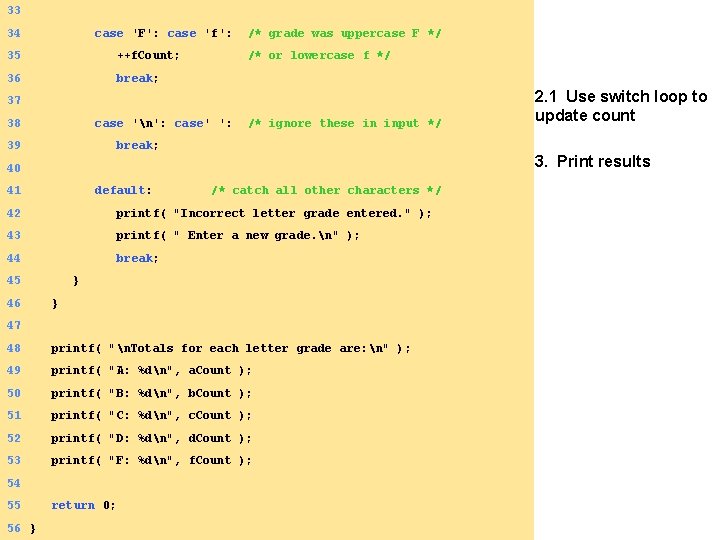
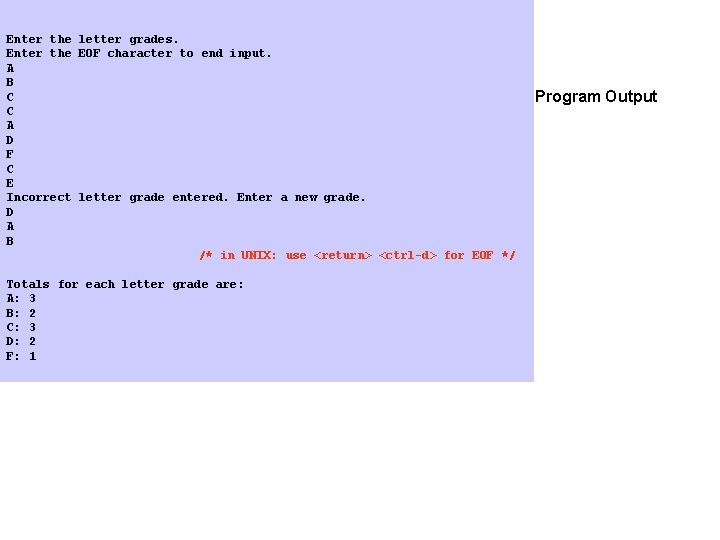
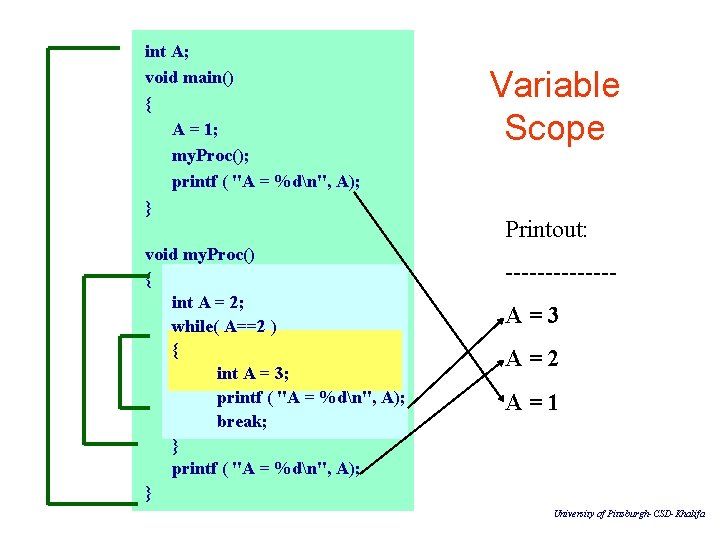
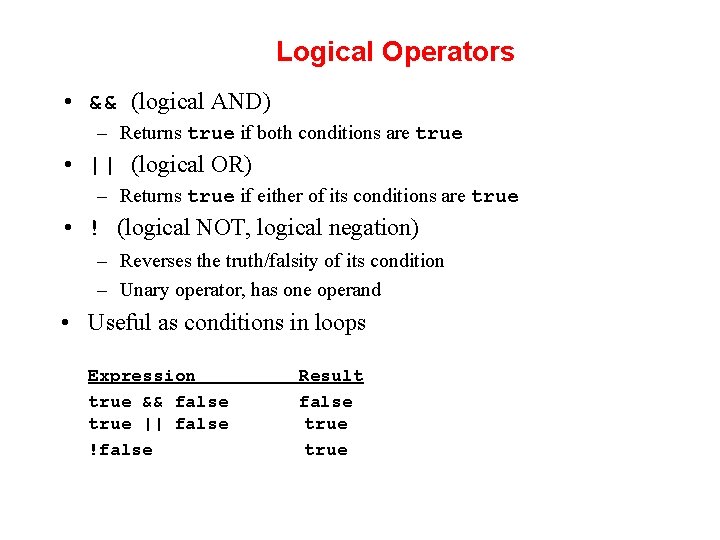
- Slides: 25
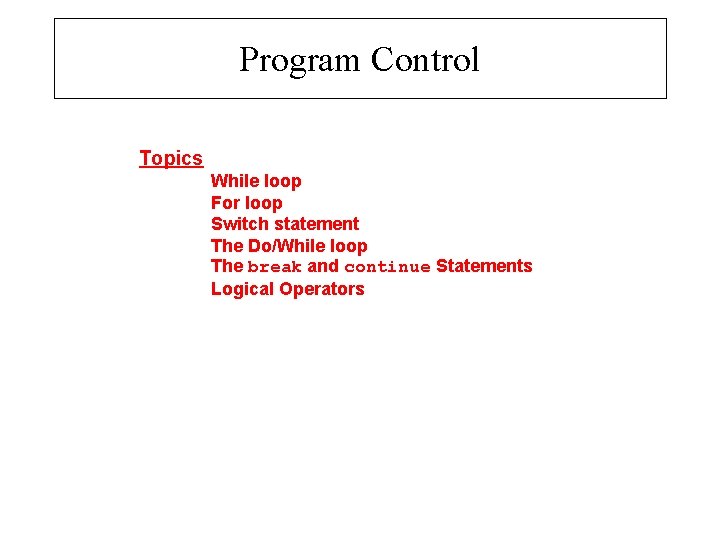
Program Control Topics While loop For loop Switch statement The Do/While loop The break and continue Statements Logical Operators
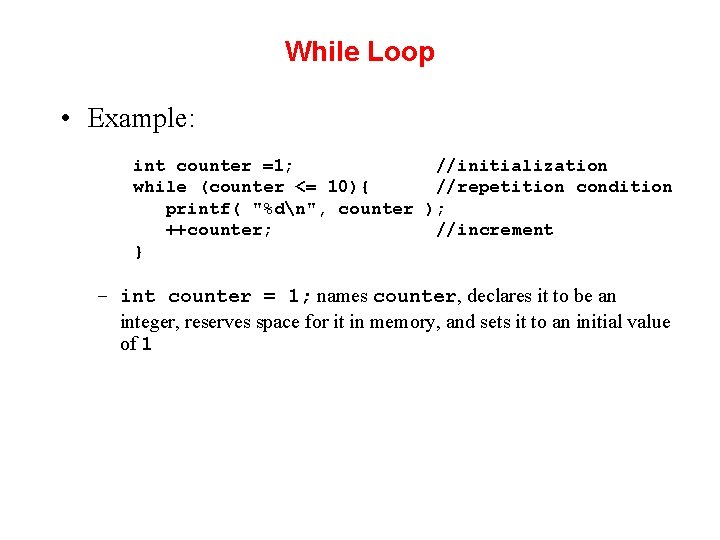
While Loop • Example: int counter =1; //initialization while (counter <= 10){ //repetition condition printf( "%dn", counter ); ++counter; //increment } – int counter = 1; names counter, declares it to be an integer, reserves space for it in memory, and sets it to an initial value of 1
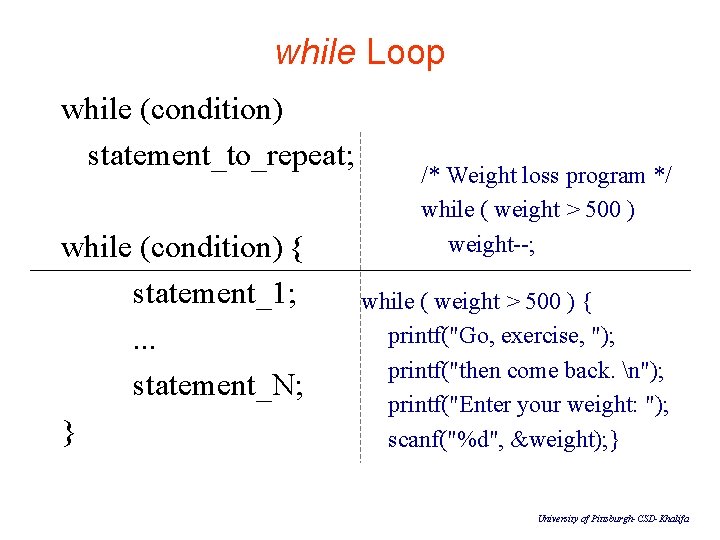
while Loop while (condition) statement_to_repeat; while (condition) { statement_1; . . . statement_N; } /* Weight loss program */ while ( weight > 500 ) weight--; while ( weight > 500 ) { printf("Go, exercise, "); printf("then come back. n"); printf("Enter your weight: "); scanf("%d", &weight); } University of Pittsburgh-CSD-Khalifa
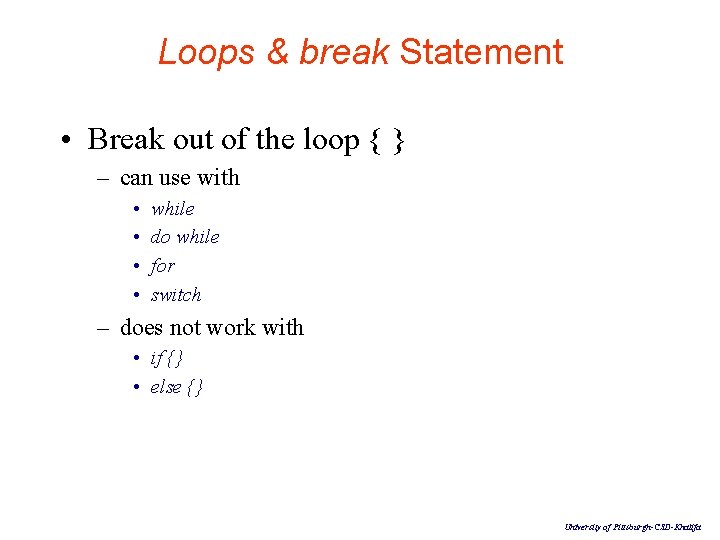
Loops & break Statement • Break out of the loop { } – can use with • • while do while for switch – does not work with • if {} • else {} University of Pittsburgh-CSD-Khalifa
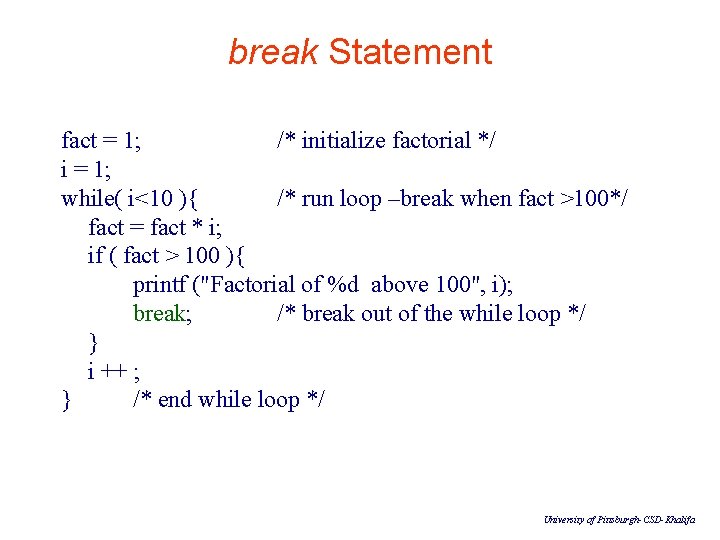
break Statement fact = 1; /* initialize factorial */ i = 1; while( i<10 ){ /* run loop –break when fact >100*/ fact = fact * i; if ( fact > 100 ){ printf ("Factorial of %d above 100", i); break; /* break out of the while loop */ } i ++ ; } /* end while loop */ University of Pittsburgh-CSD-Khalifa
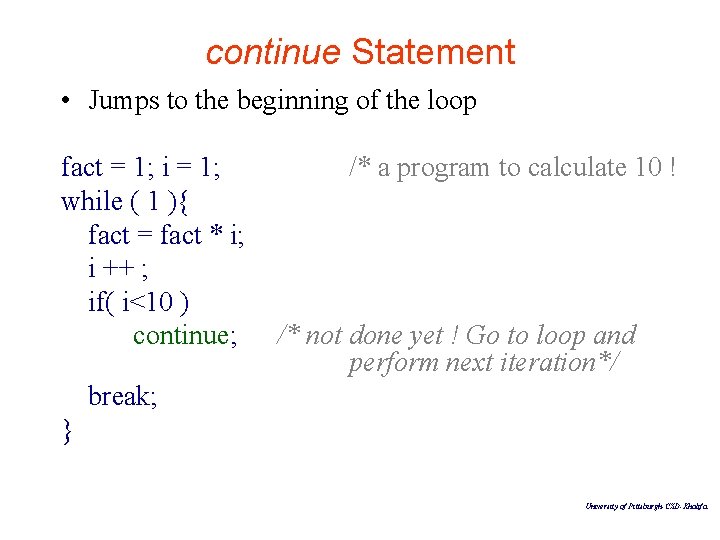
continue Statement • Jumps to the beginning of the loop fact = 1; i = 1; while ( 1 ){ fact = fact * i; i ++ ; if( i<10 ) continue; /* a program to calculate 10 ! /* not done yet ! Go to loop and perform next iteration*/ break; } University of Pittsburgh-CSD-Khalifa
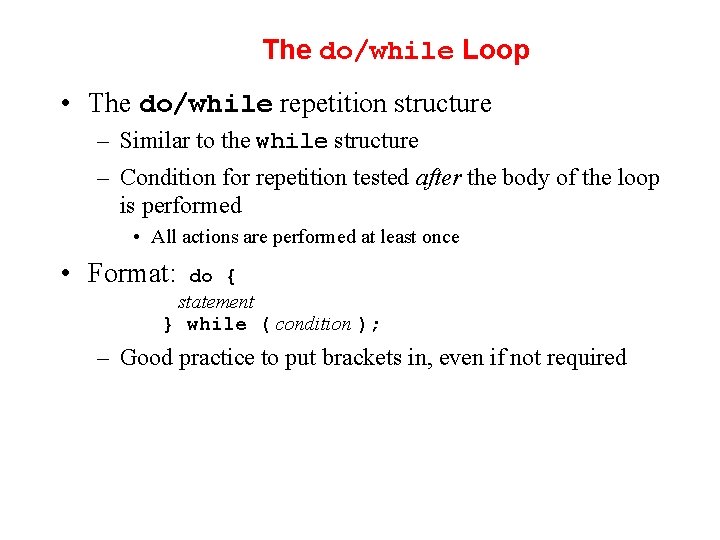
The do/while Loop • The do/while repetition structure – Similar to the while structure – Condition for repetition tested after the body of the loop is performed • All actions are performed at least once • Format: do { statement } while ( condition ); – Good practice to put brackets in, even if not required
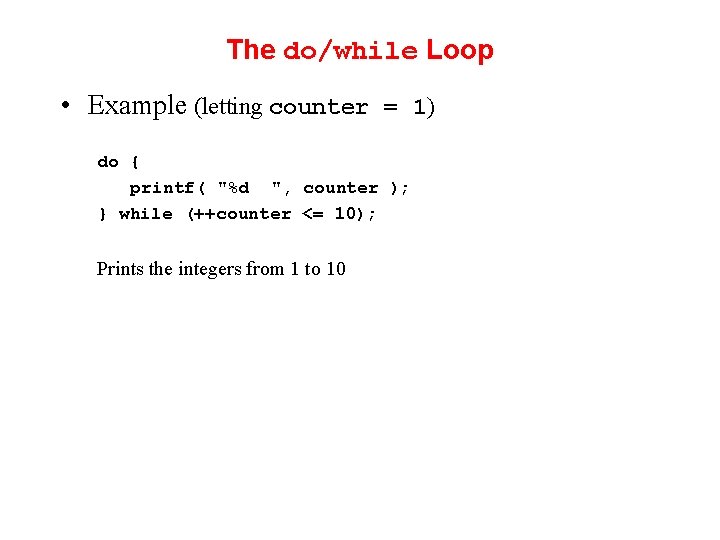
The do/while Loop • Example (letting counter = 1) do { printf( "%d ", counter ); } while (++counter <= 10); Prints the integers from 1 to 10
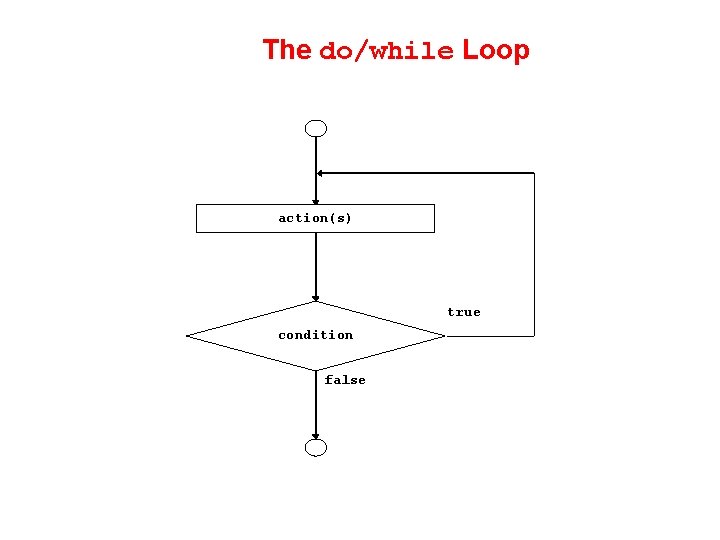
The do/while Loop action(s) true condition false
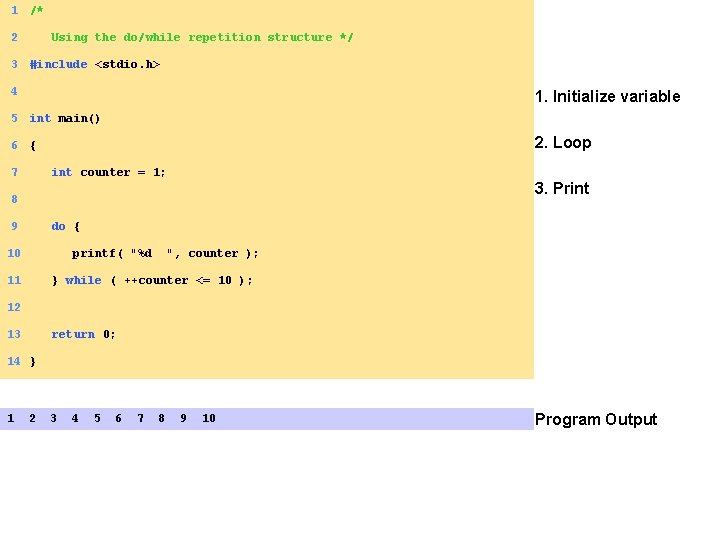
1 /* 2 Using the do/while repetition structure */ 3 #include <stdio. h> 4 1. Initialize variable 5 int main() 6 { 7 int counter = 1; 8 9 do { 2. Loop 3. Print 10 printf( "%d ", counter ); 11 } while ( ++counter <= 10 ); 12 13 return 0; 14 } 1 2 3 4 5 6 7 8 9 10 Program Output
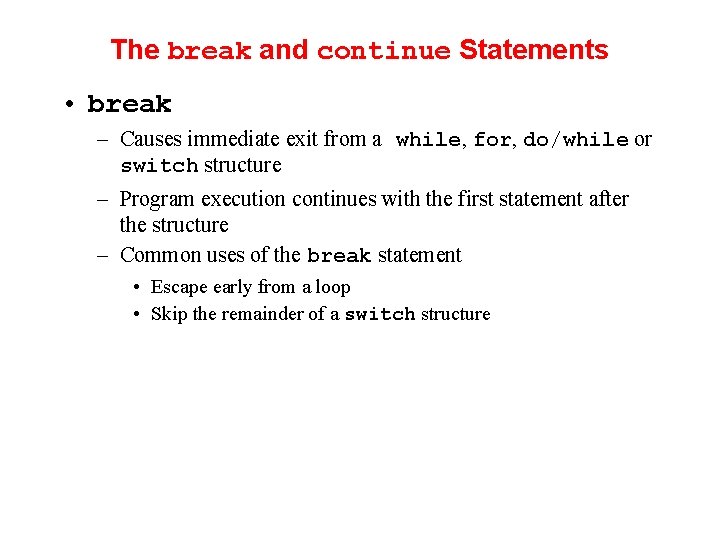
The break and continue Statements • break – Causes immediate exit from a while, for, do/while or switch structure – Program execution continues with the first statement after the structure – Common uses of the break statement • Escape early from a loop • Skip the remainder of a switch structure
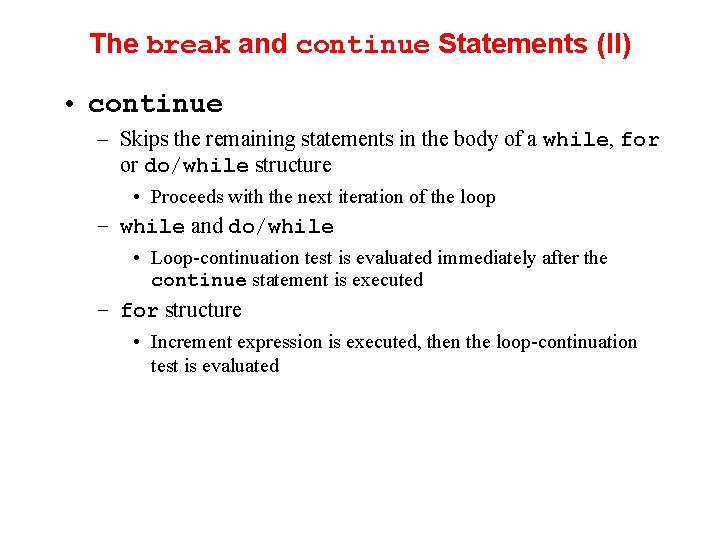
The break and continue Statements (II) • continue – Skips the remaining statements in the body of a while, for or do/while structure • Proceeds with the next iteration of the loop – while and do/while • Loop-continuation test is evaluated immediately after the continue statement is executed – for structure • Increment expression is executed, then the loop-continuation test is evaluated
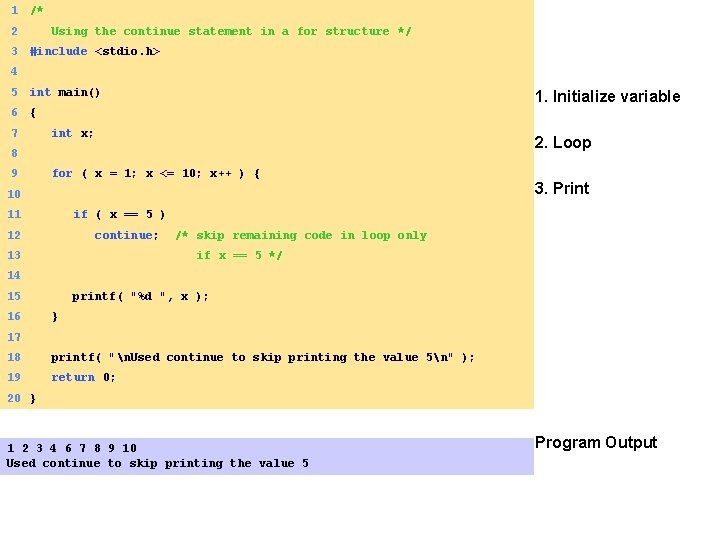
1 /* 2 Using the continue statement in a for structure */ 3 #include <stdio. h> 4 5 int main() 6 { 7 int x; 8 9 for ( x = 1; x <= 10; x++ ) { 10 1. Initialize variable 2. Loop 3. Print 11 if ( x == 5 ) 12 continue; /* skip remaining code in loop only 13 if x == 5 */ 14 15 printf( "%d ", x ); 16 } 17 18 printf( "n. Used continue to skip printing the value 5n" ); 19 return 0; 20 } 1 2 3 4 6 7 8 9 10 Used continue to skip printing the value 5 Program Output
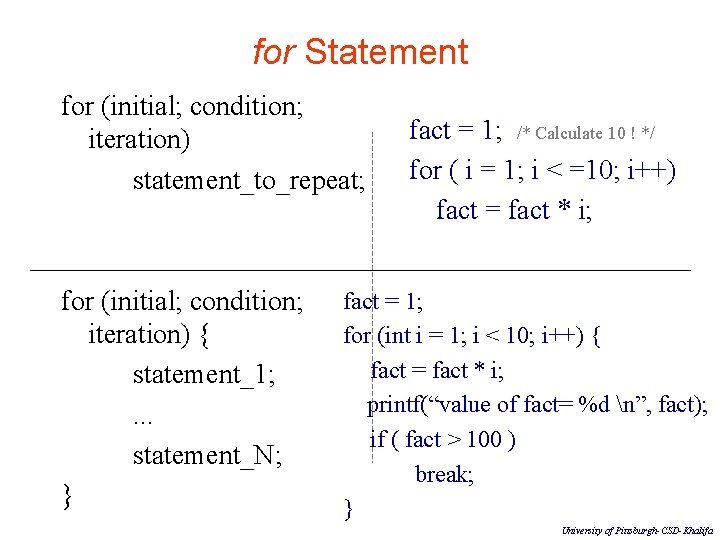
for Statement for (initial; condition; iteration) statement_to_repeat; for (initial; condition; iteration) { statement_1; . . . statement_N; } fact = 1; /* Calculate 10 ! */ for ( i = 1; i < =10; i++) fact = fact * i; fact = 1; for (int i = 1; i < 10; i++) { fact = fact * i; printf(“value of fact= %d n”, fact); if ( fact > 100 ) break; } University of Pittsburgh-CSD-Khalifa
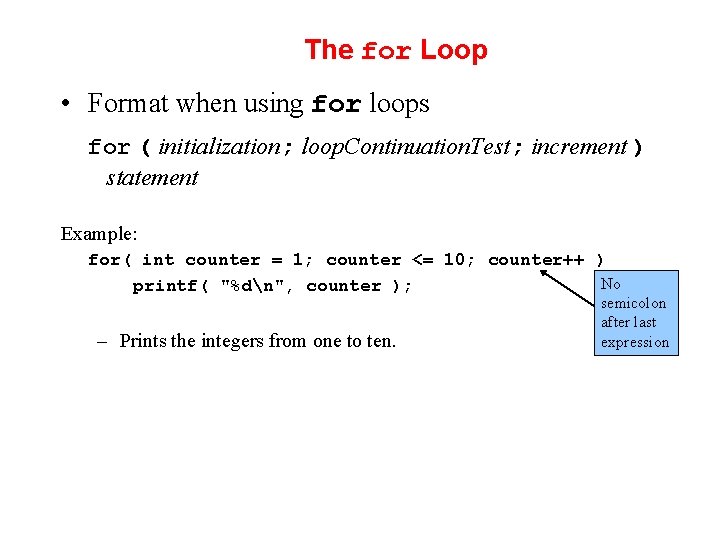
The for Loop • Format when using for loops for ( initialization; loop. Continuation. Test; increment ) statement Example: for( int counter = 1; counter <= 10; counter++ ) No printf( "%dn", counter ); – Prints the integers from one to ten. semicolon after last expression
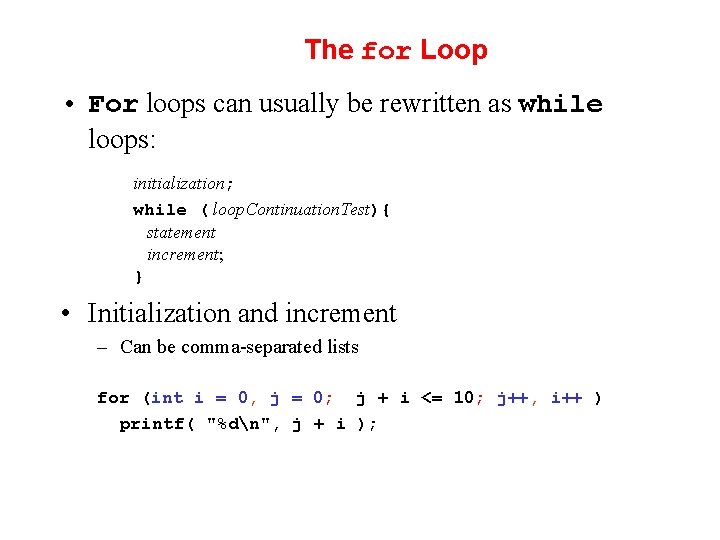
The for Loop • For loops can usually be rewritten as while loops: initialization; while ( loop. Continuation. Test){ statement increment; } • Initialization and increment – Can be comma-separated lists for (int i = 0, j = 0; j + i <= 10; j++, i++ ) printf( "%dn", j + i );
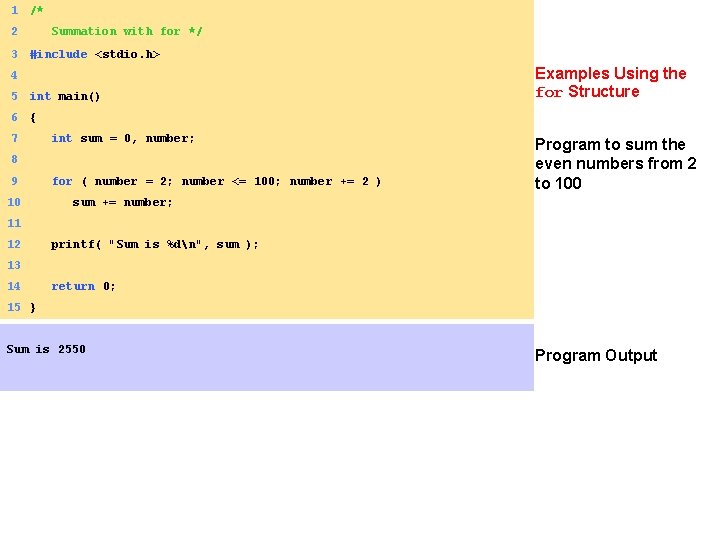
1 /* 2 Summation with for */ 3 #include <stdio. h> 4 5 int main() 6 { 7 int sum = 0, number; 8 9 for ( number = 2; number <= 100; number += 2 ) Examples Using the for Structure Program to sum the even numbers from 2 to 100 10 sum += number; 11 12 printf( "Sum is %dn", sum ); 13 14 return 0; 15 } Sum is 2550 Program Output
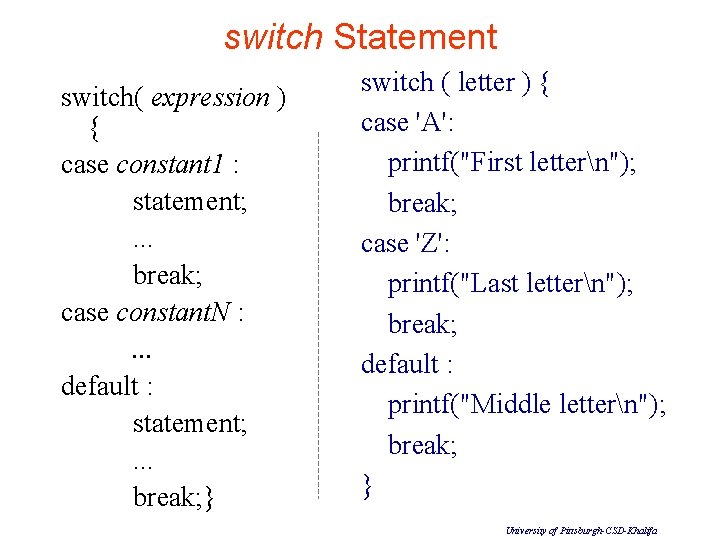
switch Statement switch( expression ) { case constant 1 : statement; . . . break; case constant. N : . . . default : statement; . . . break; } switch ( letter ) { case 'A': printf("First lettern"); break; case 'Z': printf("Last lettern"); break; default : printf("Middle lettern"); break; } University of Pittsburgh-CSD-Khalifa
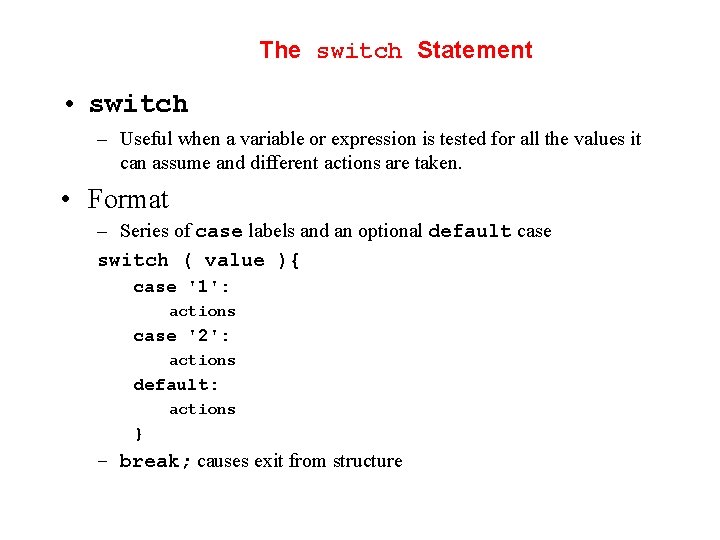
The switch Statement • switch – Useful when a variable or expression is tested for all the values it can assume and different actions are taken. • Format – Series of case labels and an optional default case switch ( value ){ case '1': actions case '2': actions default: actions } – break; causes exit from structure
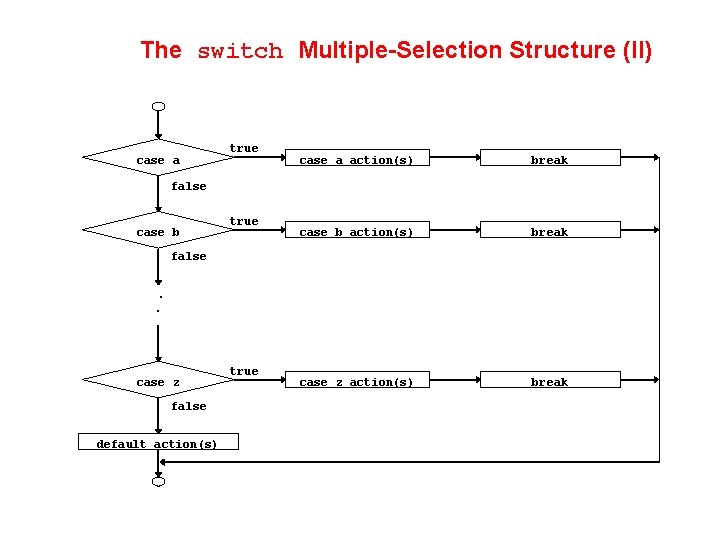
The switch Multiple-Selection Structure (II) case a true case a action(s) break case b action(s) break case z action(s) break false case b true false. . . case z false default action(s) true
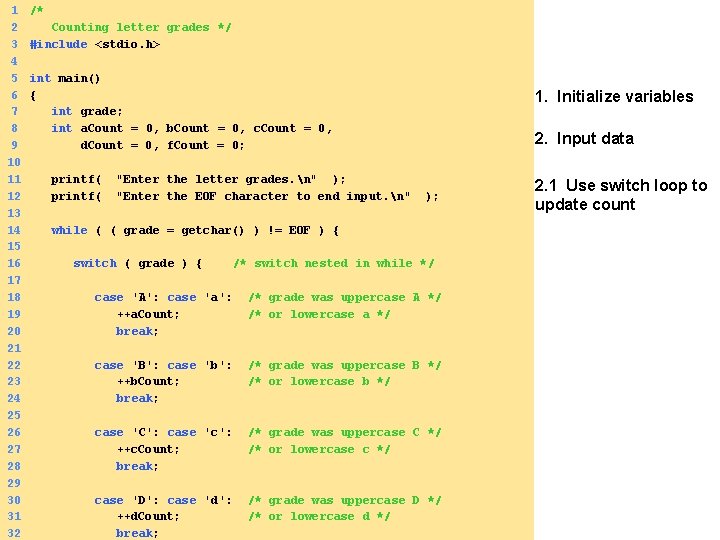
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 /* Counting letter grades */ #include <stdio. h> int main() { int grade; int a. Count = 0, b. Count = 0, c. Count = 0, d. Count = 0, f. Count = 0; printf( "Enter the letter grades. n" ); printf( "Enter the EOF character to end input. n" ); while ( ( grade = getchar() ) != EOF ) { switch ( grade ) { /* switch nested in while */ case 'A': case 'a': /* grade was uppercase A */ ++a. Count; /* or lowercase a */ break; case 'B': case 'b': /* grade was uppercase B */ ++b. Count; /* or lowercase b */ break; case 'C': case 'c': /* grade was uppercase C */ ++c. Count; /* or lowercase c */ break; case 'D': case 'd': /* grade was uppercase D */ ++d. Count; /* or lowercase d */ break; 1. Initialize variables 2. Input data 2. 1 Use switch loop to update count
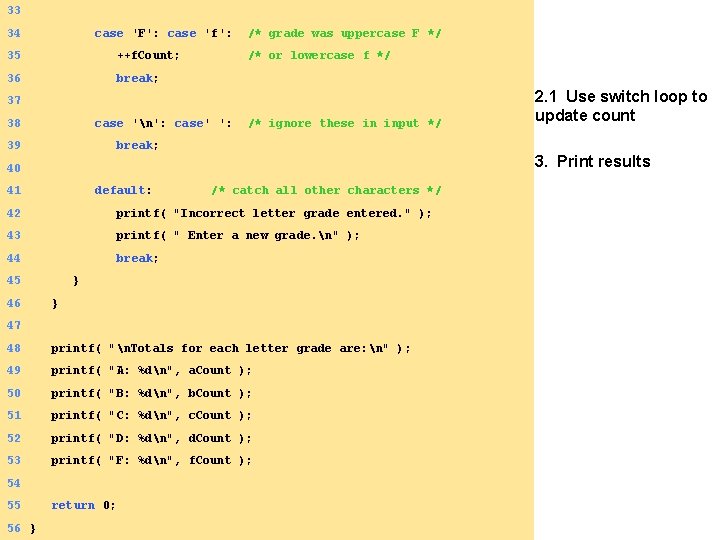
33 34 case 'F': case 'f': /* grade was uppercase F */ 35 ++f. Count; /* or lowercase f */ 36 break; 37 38 case 'n': case' ': /* ignore these in input */ 2. 1 Use switch loop to update count 39 break; 40 41 default: /* catch all other characters */ 42 printf( "Incorrect letter grade entered. " ); 43 printf( " Enter a new grade. n" ); 44 break; 45 } 46 } 47 48 printf( "n. Totals for each letter grade are: n" ); 49 printf( "A: %dn", a. Count ); 50 printf( "B: %dn", b. Count ); 51 printf( "C: %dn", c. Count ); 52 printf( "D: %dn", d. Count ); 53 printf( "F: %dn", f. Count ); 54 55 return 0; 56 } 3. Print results
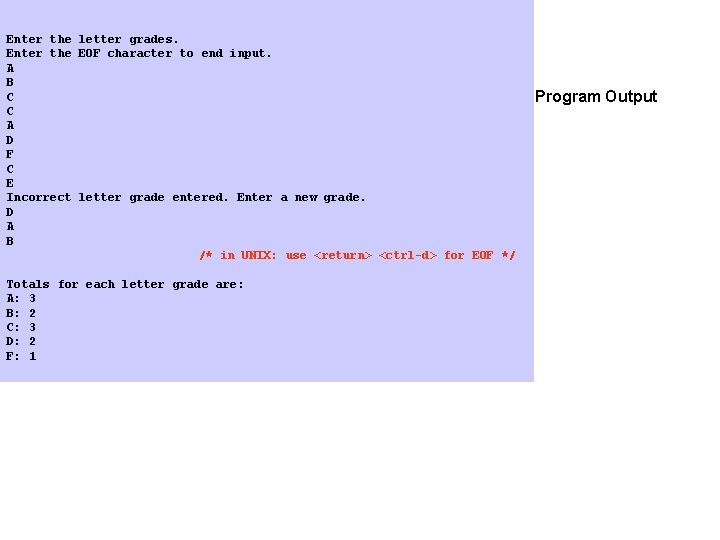
Enter the letter grades. Enter the EOF character to end input. A B C C A D F C E Incorrect letter grade entered. Enter a new grade. D A B /* in UNIX: use <return> <ctrl-d> for EOF */ Totals for each letter grade are: A: 3 B: 2 C: 3 D: 2 F: 1 Program Output
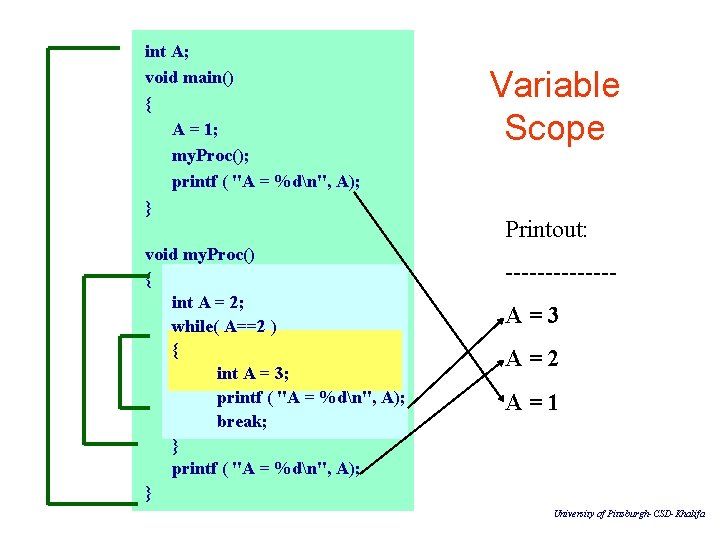
int A; void main() { A = 1; my. Proc(); printf ( "A = %dn", A); } void my. Proc() { int A = 2; while( A==2 ) { int A = 3; printf ( "A = %dn", A); break; } printf ( "A = %dn", A); } Variable Scope Printout: -------A=3 A=2 A=1 University of Pittsburgh-CSD-Khalifa
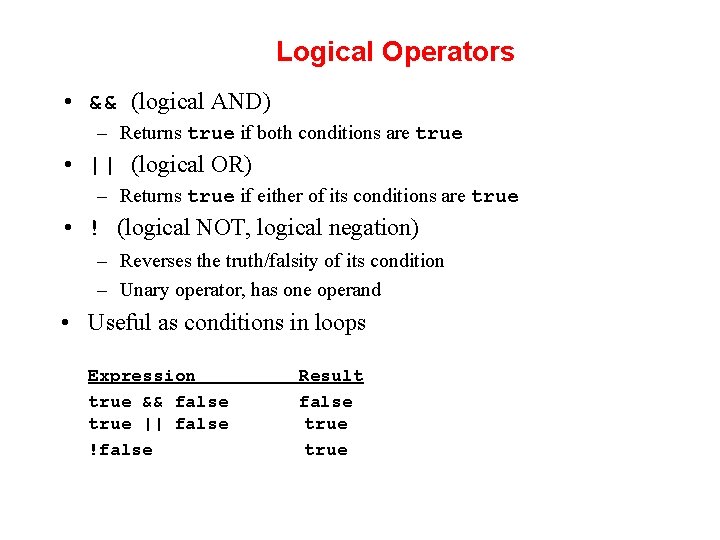
Logical Operators • && (logical AND) – Returns true if both conditions are true • || (logical OR) – Returns true if either of its conditions are true • ! (logical NOT, logical negation) – Reverses the truth/falsity of its condition – Unary operator, has one operand • Useful as conditions in loops Expression Result true && false true || false true !false true