ILM Proprietary and Confidential http www ilmservice com
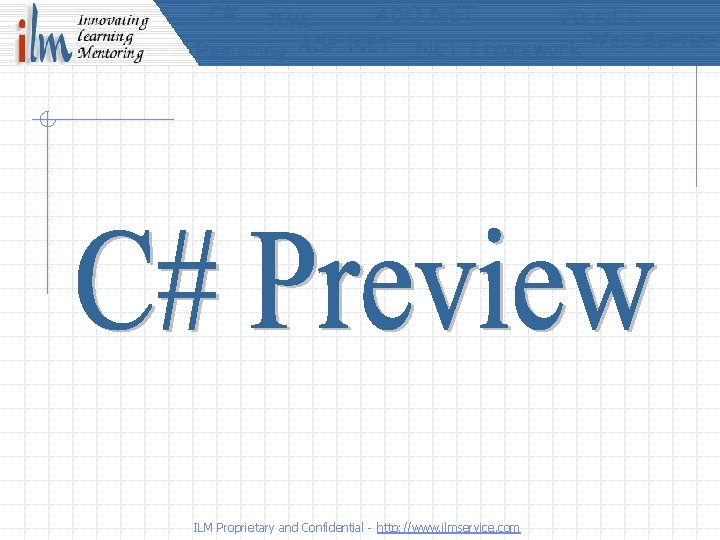
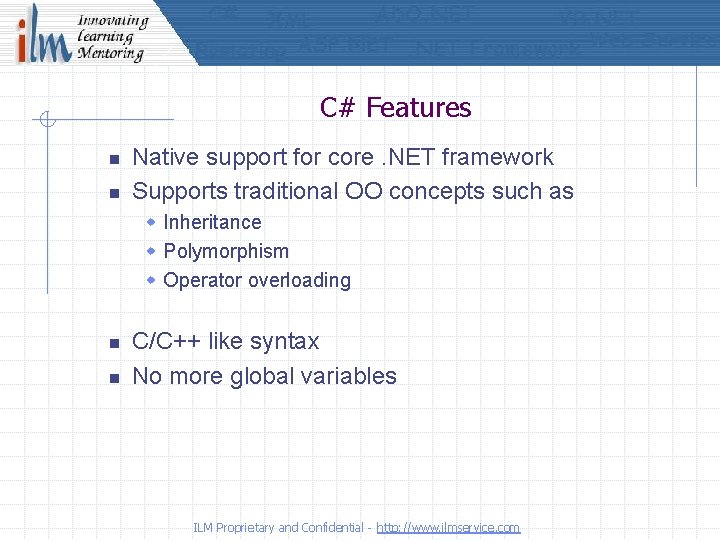
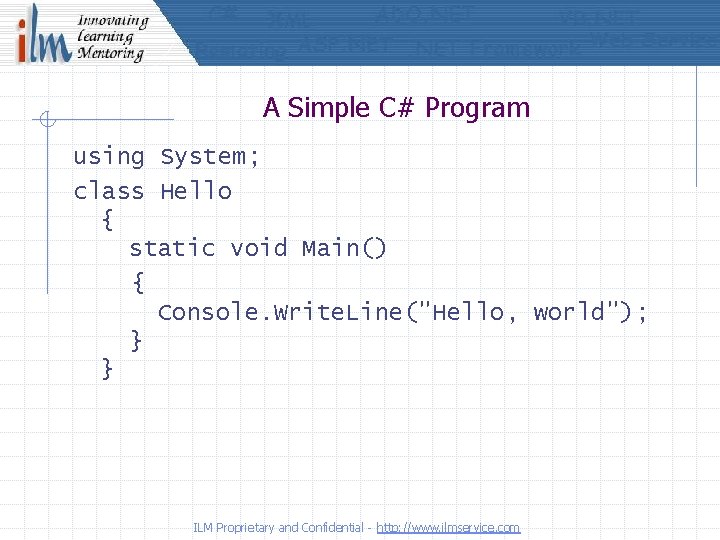
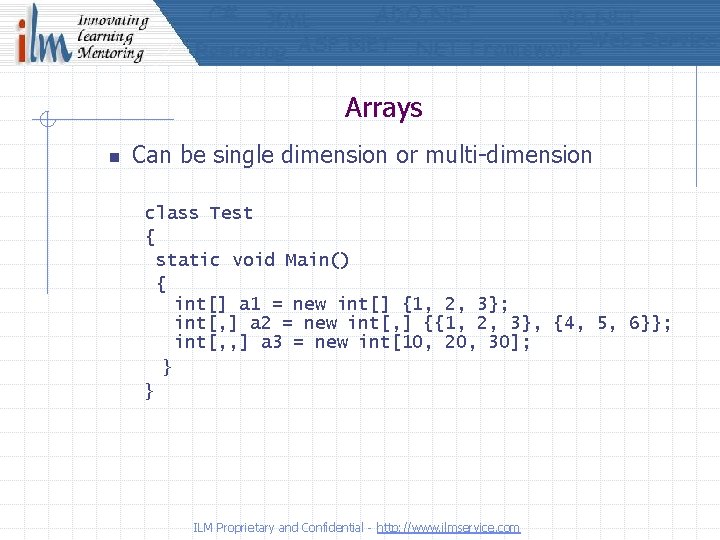
![If Statement using System; class Test { static void Main(string[] args) { if (args. If Statement using System; class Test { static void Main(string[] args) { if (args.](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-5.jpg)
![Switch Statement using System; class Test{ static void Main(string[] args) { switch (args. Length) Switch Statement using System; class Test{ static void Main(string[] args) { switch (args. Length)](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-6.jpg)
![While Statement using System; class Test{ static int Find(int value, int[] arr) { int While Statement using System; class Test{ static int Find(int value, int[] arr) { int](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-7.jpg)
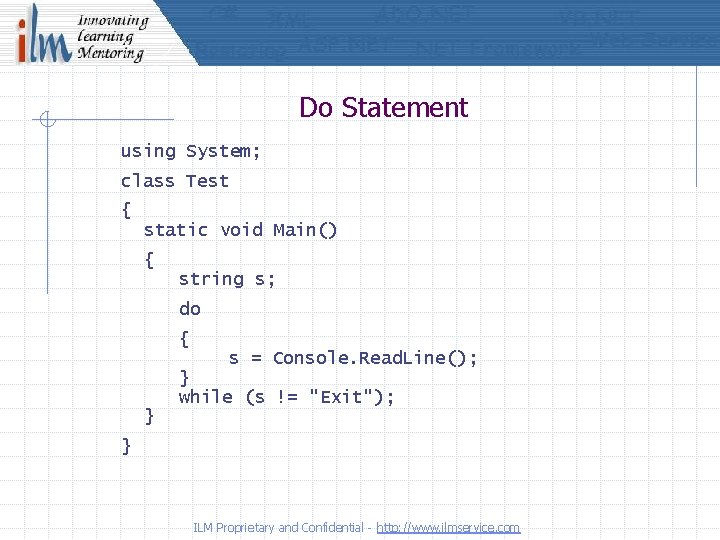
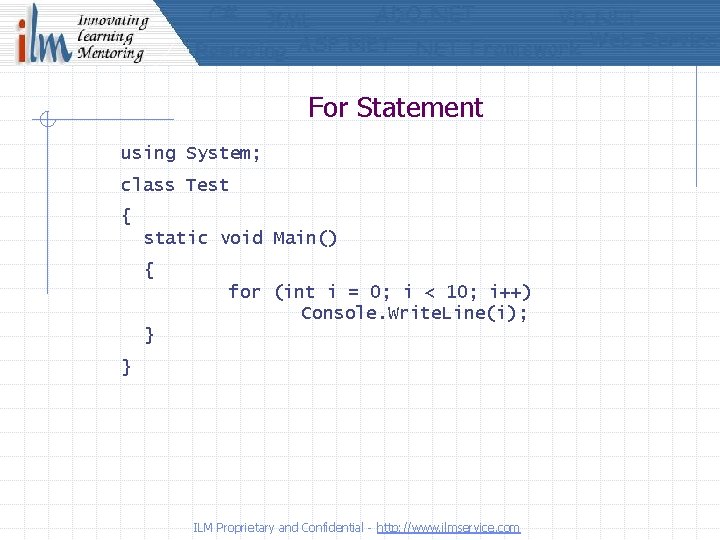
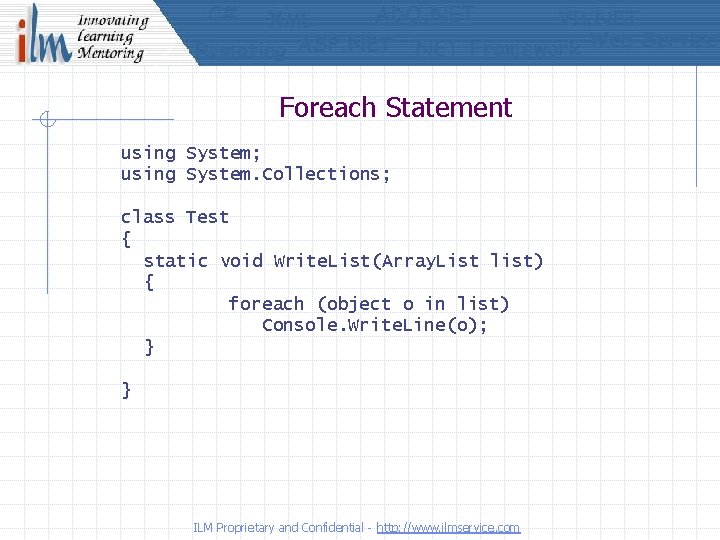
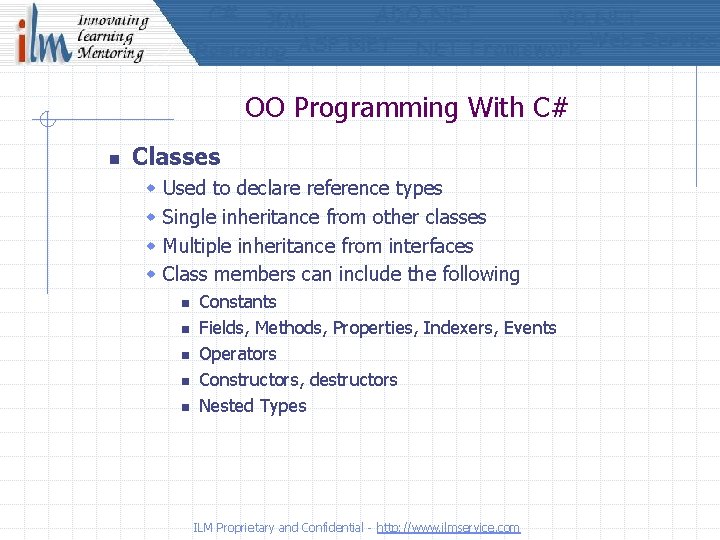
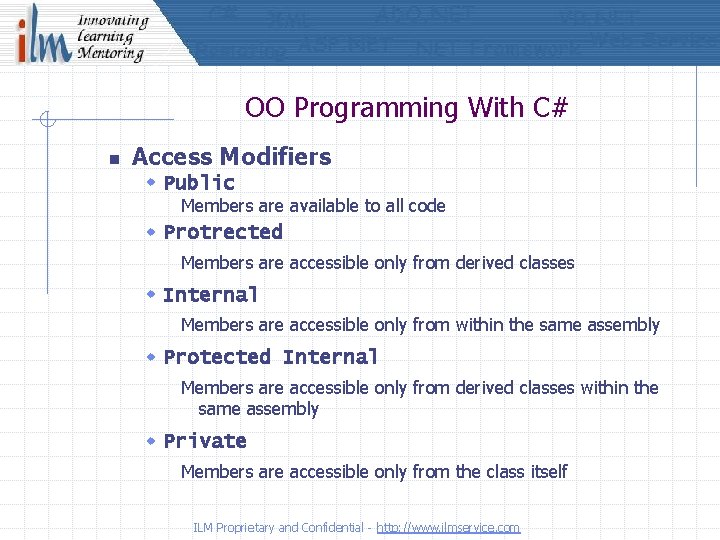
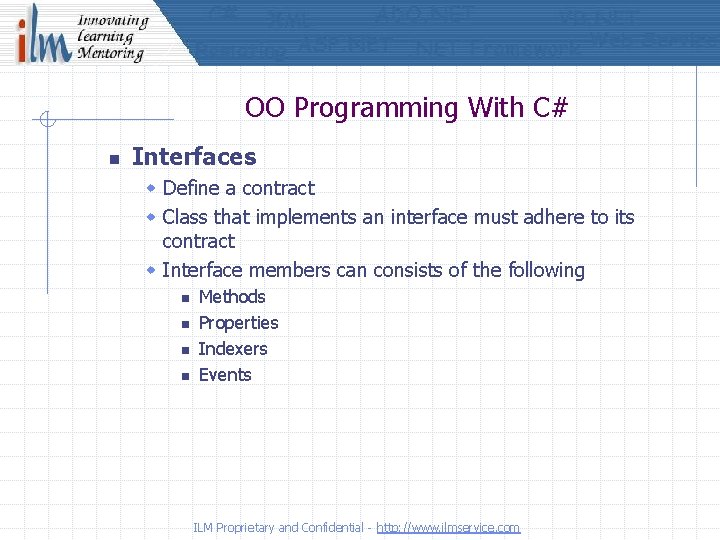
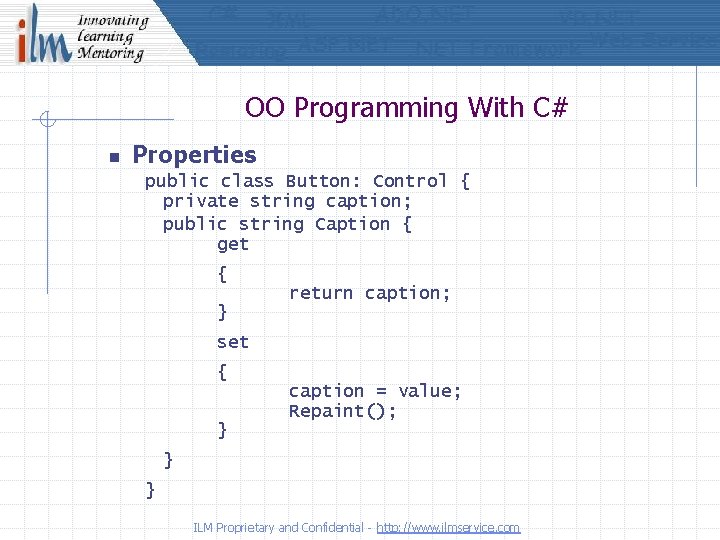
![OO Programming With C# n Indexers public class List. Box: Control { private string[] OO Programming With C# n Indexers public class List. Box: Control { private string[]](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-15.jpg)
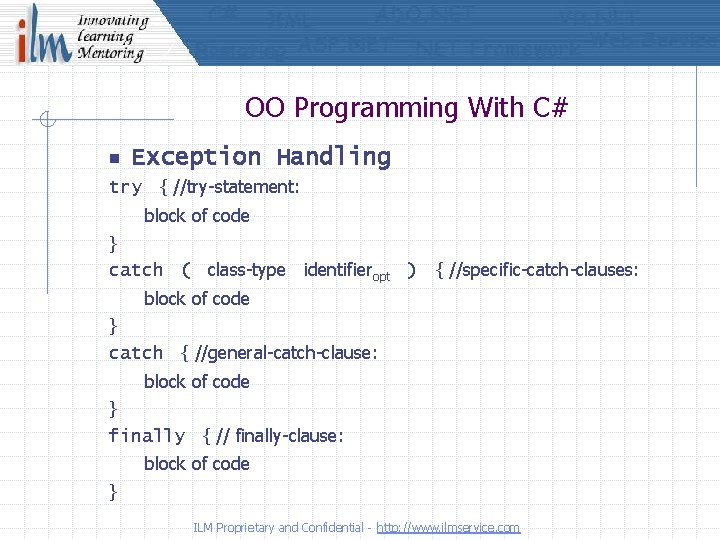
- Slides: 16
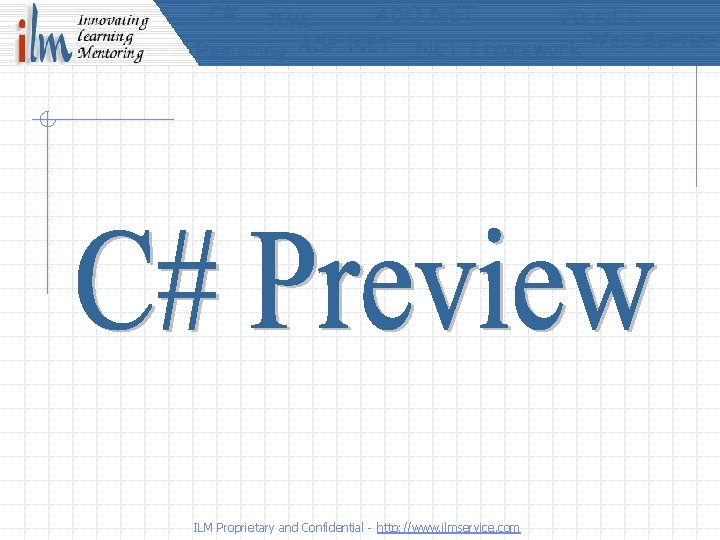
ILM Proprietary and Confidential - http: //www. ilmservice. com
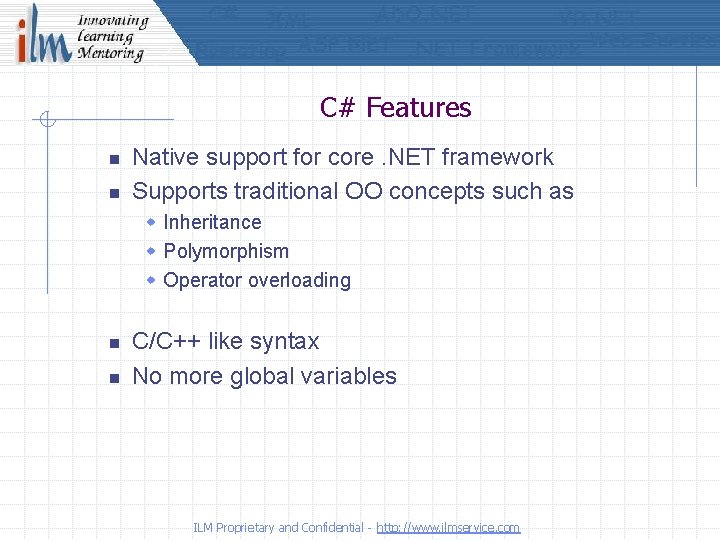
C# Features n n Native support for core. NET framework Supports traditional OO concepts such as w Inheritance w Polymorphism w Operator overloading n n C/C++ like syntax No more global variables ILM Proprietary and Confidential - http: //www. ilmservice. com
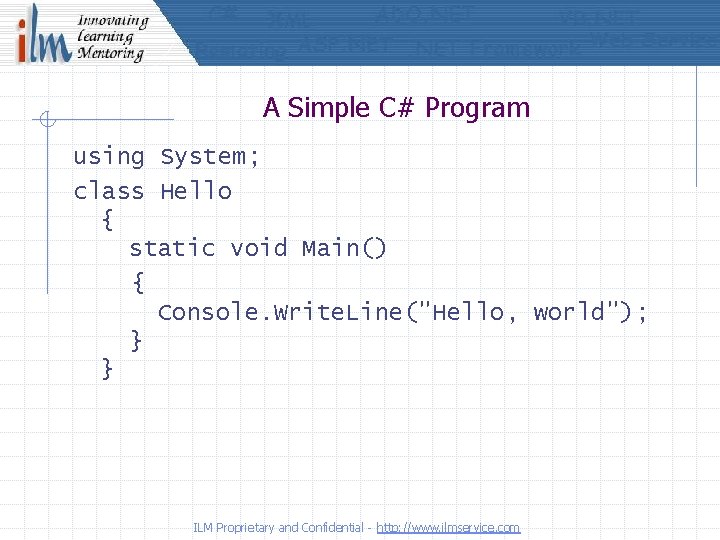
A Simple C# Program using System; class Hello { static void Main() { Console. Write. Line("Hello, world"); } } ILM Proprietary and Confidential - http: //www. ilmservice. com
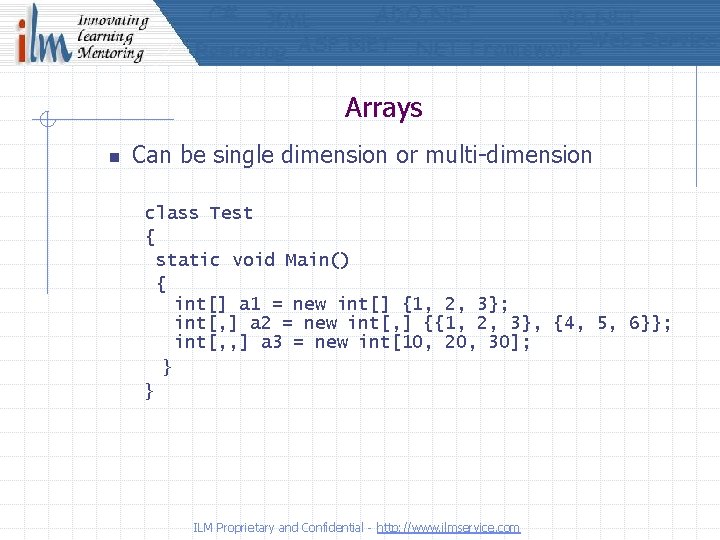
Arrays n Can be single dimension or multi-dimension class Test { static void Main() { int[] a 1 = new int[] {1, 2, 3}; int[, ] a 2 = new int[, ] {{1, 2, 3}, {4, 5, 6}}; int[, , ] a 3 = new int[10, 20, 30]; } } ILM Proprietary and Confidential - http: //www. ilmservice. com
![If Statement using System class Test static void Mainstring args if args If Statement using System; class Test { static void Main(string[] args) { if (args.](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-5.jpg)
If Statement using System; class Test { static void Main(string[] args) { if (args. Length == 0) Console. Write. Line("No arguments were provided"); else Console. Write. Line("Arguments were provided"); } } ILM Proprietary and Confidential - http: //www. ilmservice. com
![Switch Statement using System class Test static void Mainstring args switch args Length Switch Statement using System; class Test{ static void Main(string[] args) { switch (args. Length)](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-6.jpg)
Switch Statement using System; class Test{ static void Main(string[] args) { switch (args. Length) { case 0: Console. Write. Line("No arguments”); break; case 1: Console. Write. Line("One arguments”); break; default: Console. Write. Line("{0} arguments”); break; } } } ILM Proprietary and Confidential - http: //www. ilmservice. com
![While Statement using System class Test static int Findint value int arr int While Statement using System; class Test{ static int Find(int value, int[] arr) { int](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-7.jpg)
While Statement using System; class Test{ static int Find(int value, int[] arr) { int i = 0; while (arr[i] != value) { if (++i > arr. Length) throw new Argument. Exception(); } return i; } } ILM Proprietary and Confidential - http: //www. ilmservice. com
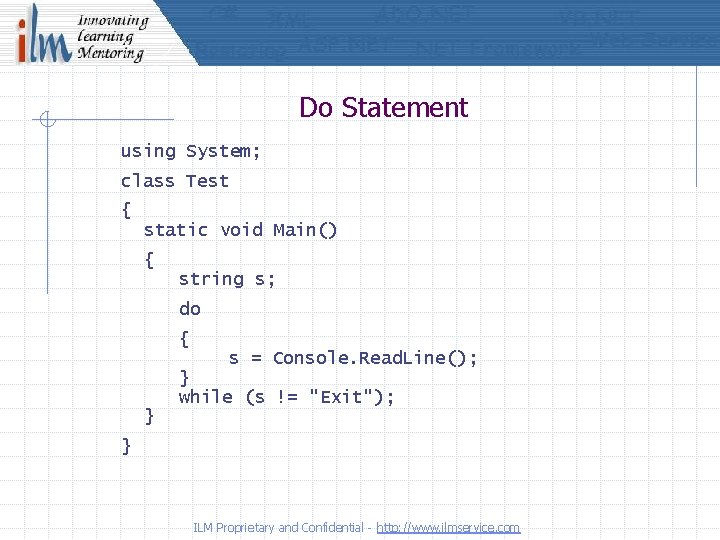
Do Statement using System; class Test { static void Main() { string s; do { s = Console. Read. Line(); } while (s != "Exit"); } } ILM Proprietary and Confidential - http: //www. ilmservice. com
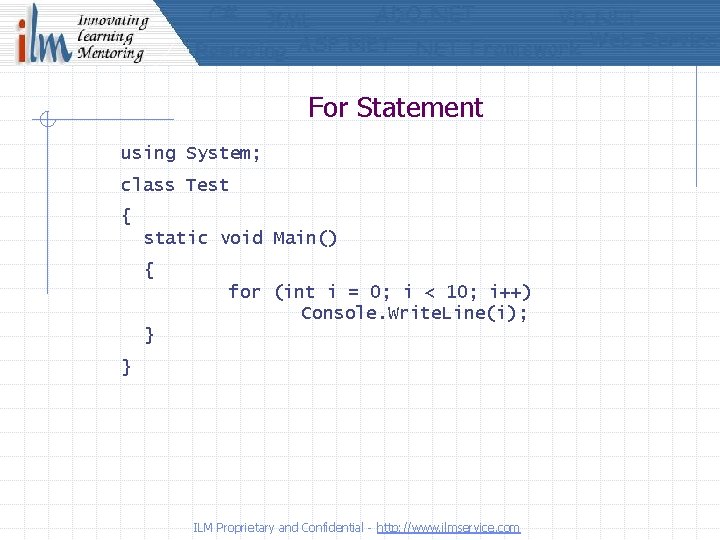
For Statement using System; class Test { static void Main() { for (int i = 0; i < 10; i++) Console. Write. Line(i); } } ILM Proprietary and Confidential - http: //www. ilmservice. com
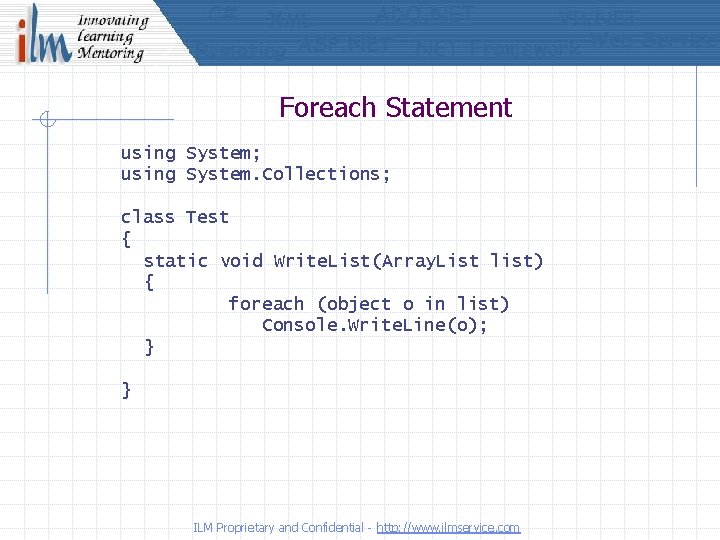
Foreach Statement using System; using System. Collections; class Test { static void Write. List(Array. List list) { foreach (object o in list) Console. Write. Line(o); } } ILM Proprietary and Confidential - http: //www. ilmservice. com
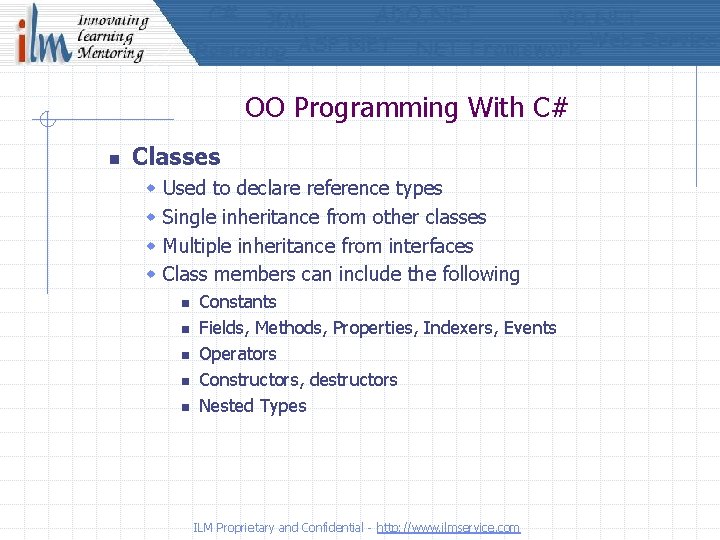
OO Programming With C# n Classes w w Used to declare reference types Single inheritance from other classes Multiple inheritance from interfaces Class members can include the following n n n Constants Fields, Methods, Properties, Indexers, Events Operators Constructors, destructors Nested Types ILM Proprietary and Confidential - http: //www. ilmservice. com
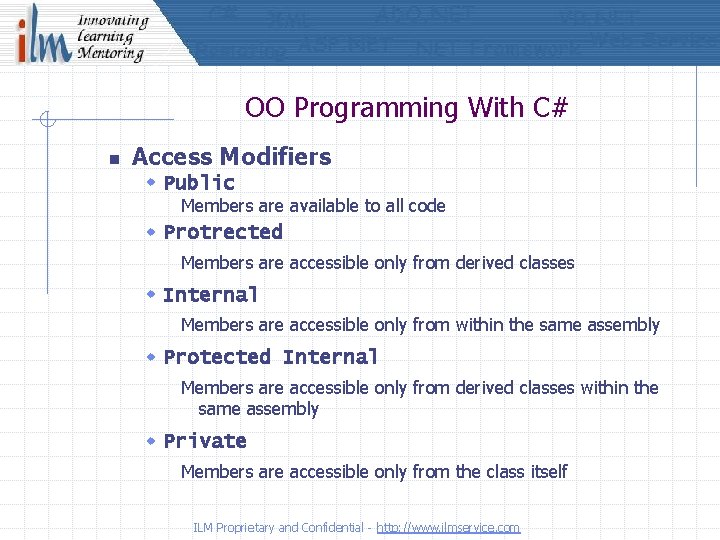
OO Programming With C# n Access Modifiers w Public Members are available to all code w Protrected Members are accessible only from derived classes w Internal Members are accessible only from within the same assembly w Protected Internal Members are accessible only from derived classes within the same assembly w Private Members are accessible only from the class itself ILM Proprietary and Confidential - http: //www. ilmservice. com
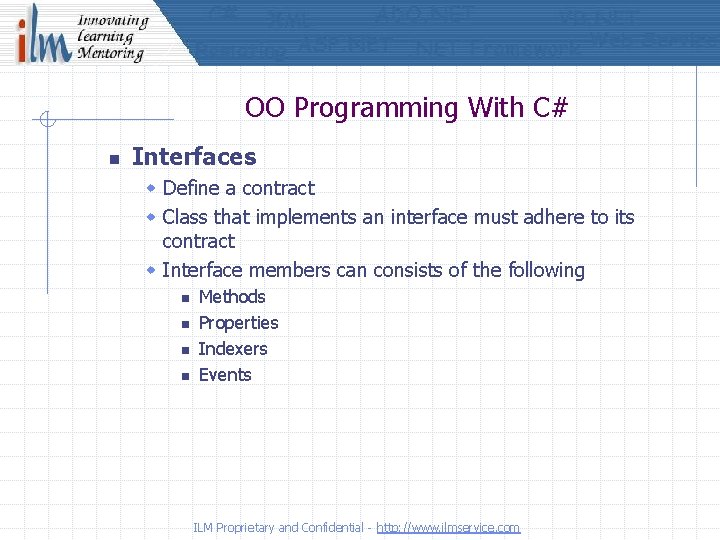
OO Programming With C# n Interfaces w Define a contract w Class that implements an interface must adhere to its contract w Interface members can consists of the following n n Methods Properties Indexers Events ILM Proprietary and Confidential - http: //www. ilmservice. com
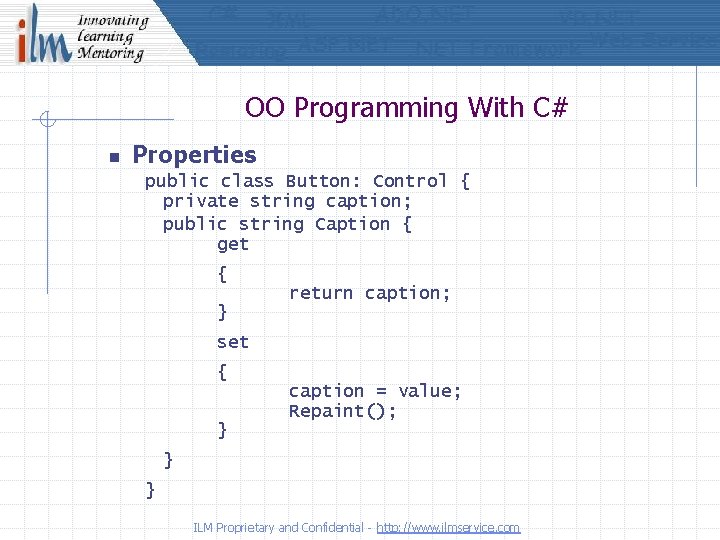
OO Programming With C# n Properties public class Button: Control { private string caption; public string Caption { get { return caption; } set { caption = value; Repaint(); } } } ILM Proprietary and Confidential - http: //www. ilmservice. com
![OO Programming With C n Indexers public class List Box Control private string OO Programming With C# n Indexers public class List. Box: Control { private string[]](https://slidetodoc.com/presentation_image_h2/00086c263d8731c3286f02ba5444a1e7/image-15.jpg)
OO Programming With C# n Indexers public class List. Box: Control { private string[] items; public string this[int index] { get { return items[index]; } set { items[index] = value; Repaint(); } } } ILM Proprietary and Confidential - http: //www. ilmservice. com
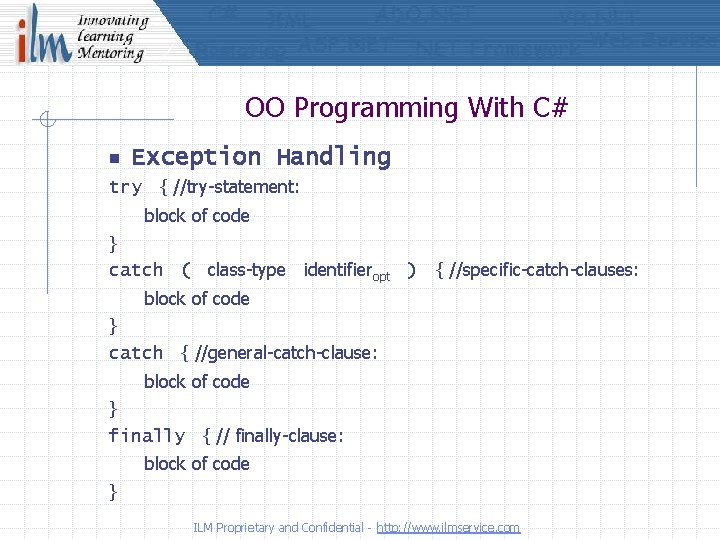
OO Programming With C# n Exception Handling try { //try-statement: block of code } catch ( class-type identifieropt ) { //specific-catch-clauses: block of code } catch { //general-catch-clause: block of code } finally { // finally-clause: block of code } ILM Proprietary and Confidential - http: //www. ilmservice. com
Ruboiy janri
Confidential do not copy or distribute
Confidential and proprietary
Bài thơ mẹ đi làm từ sáng sớm
Cơm
Open source disadvantages
Manzara janri haqida malumot
What is hadith literally and technically
Epapack
Charxpalak texnologiyasi
Kitob beminnat do st insho
Ilm sahroda do'st
Proprietary freeware
Proprietary grief
Proprietary format
Define proprietary colony
Proprietary theory