Data Structures and Algorithms Lecture 4 Msury Mahunnah
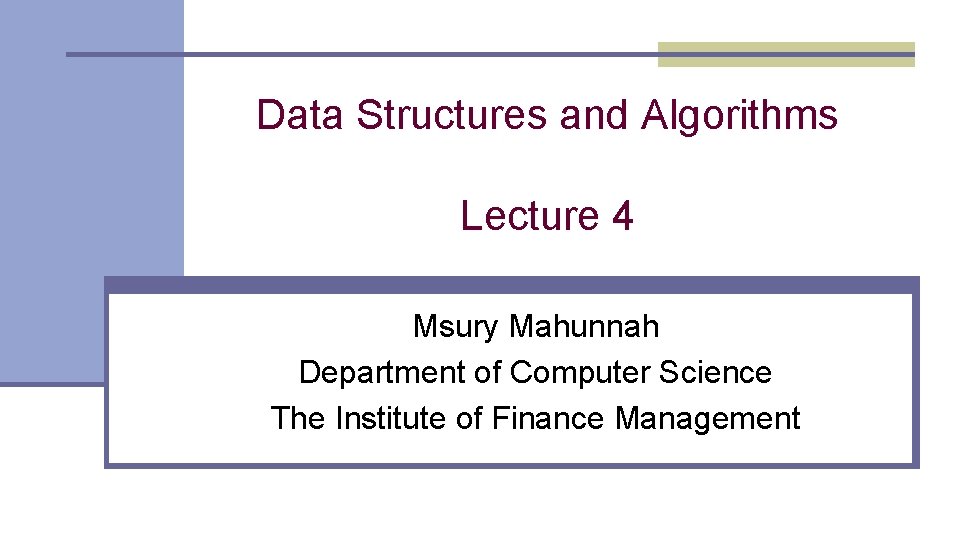
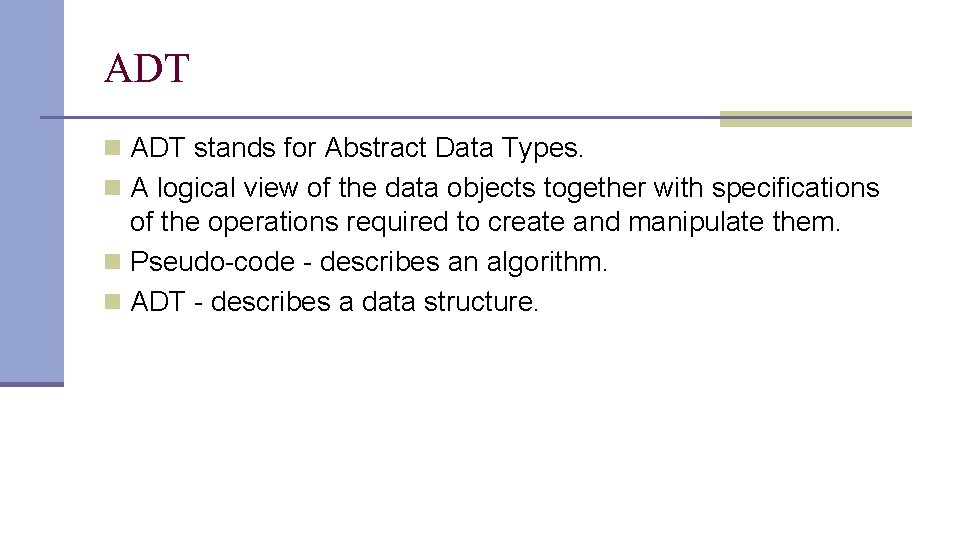
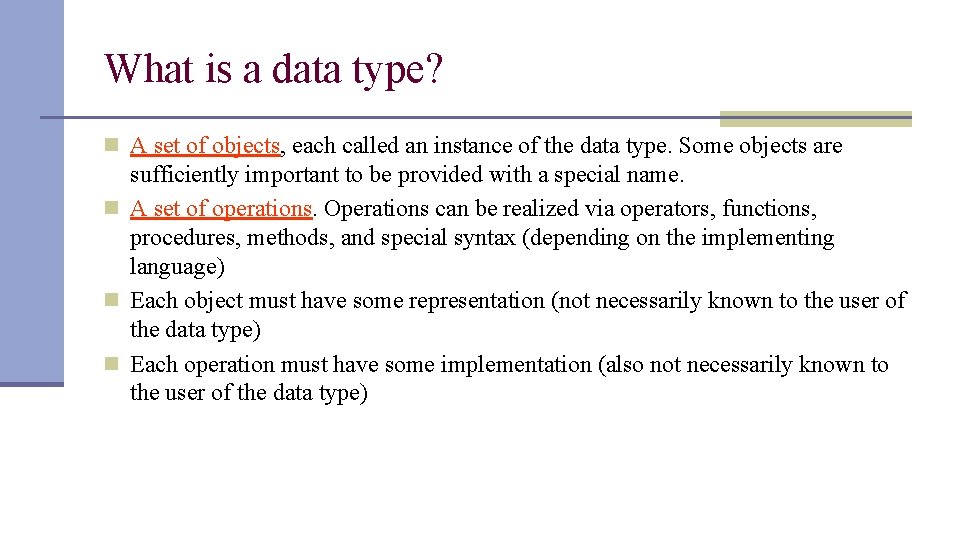
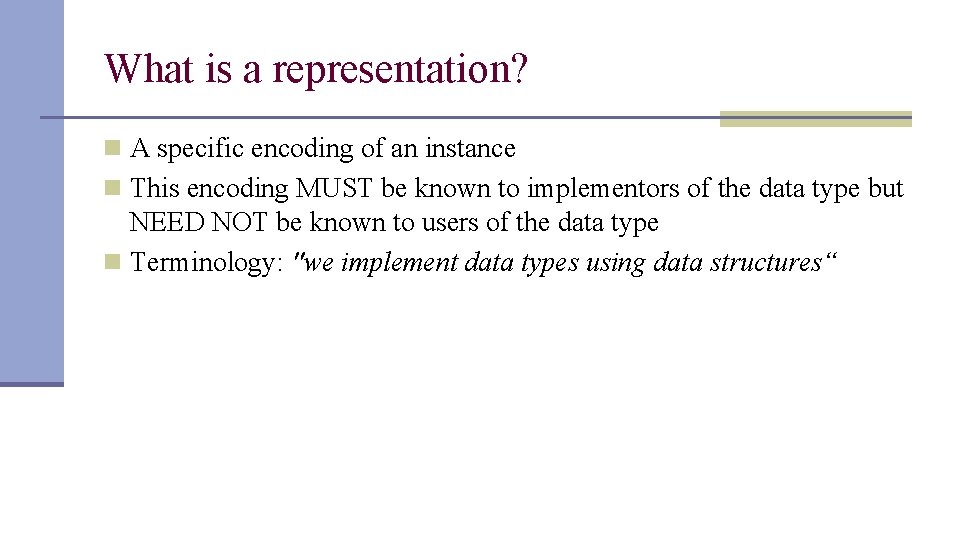
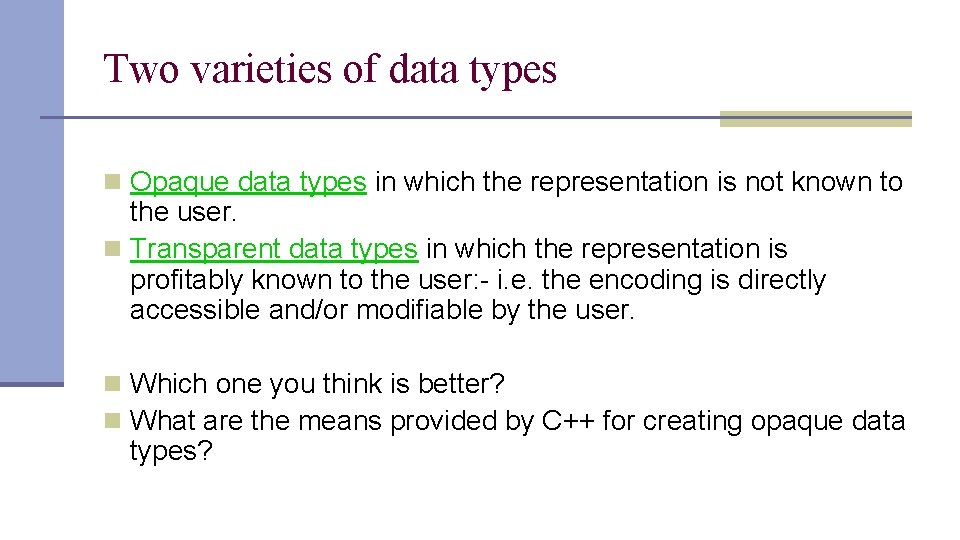
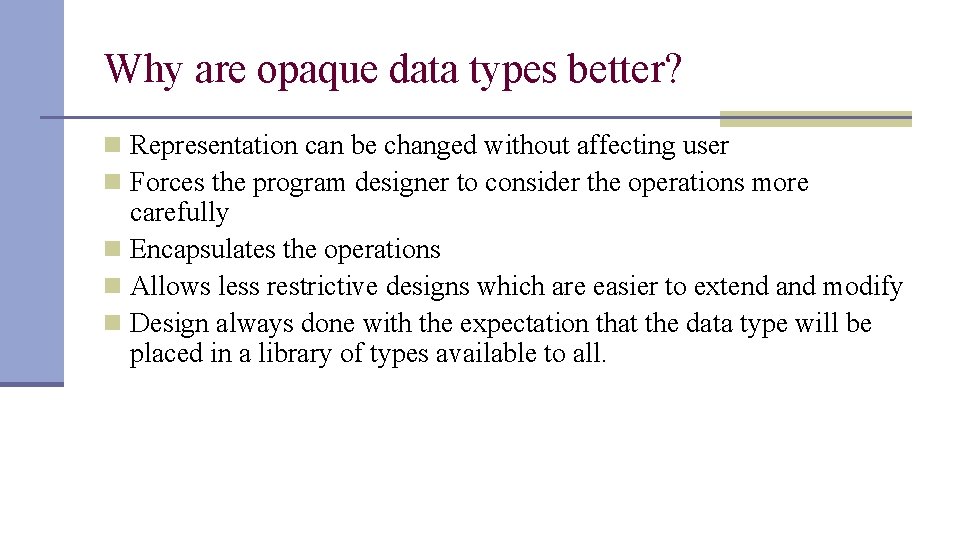
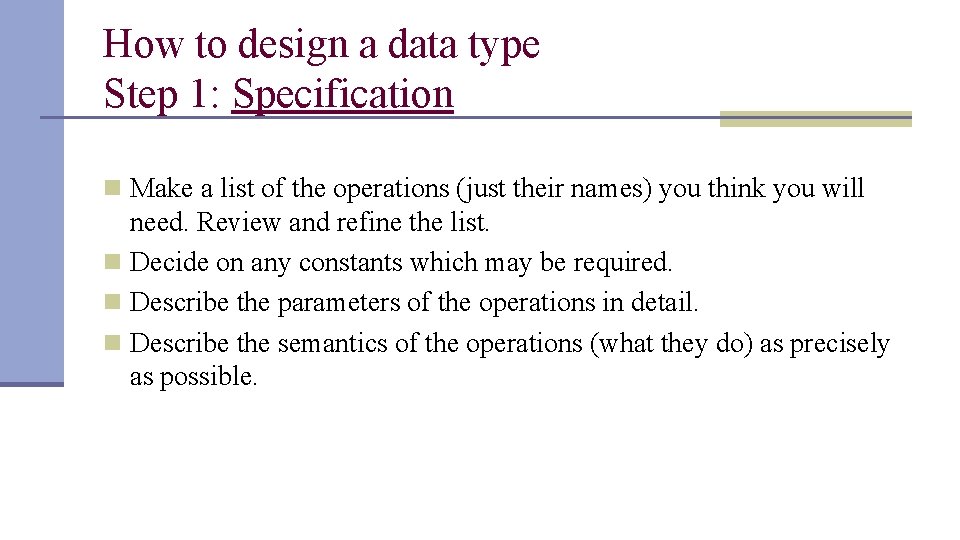
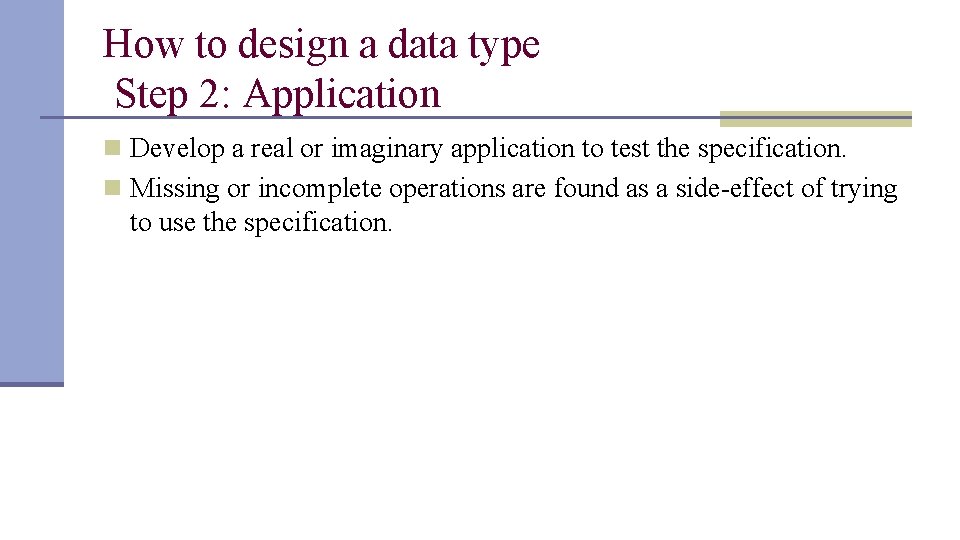
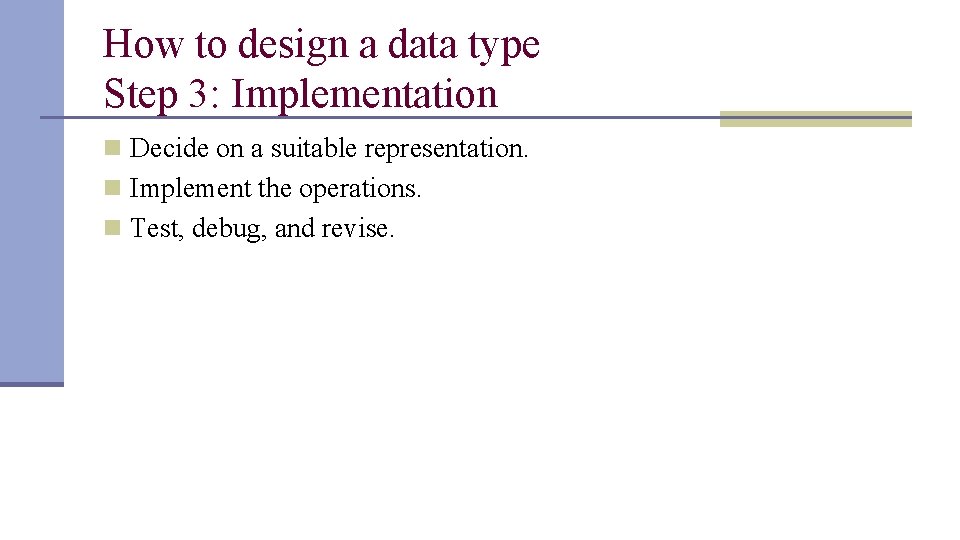
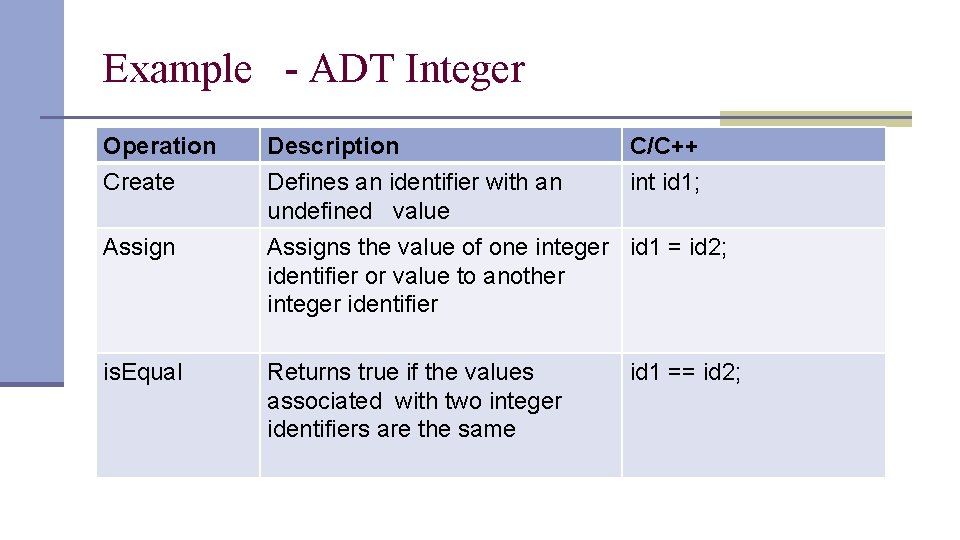
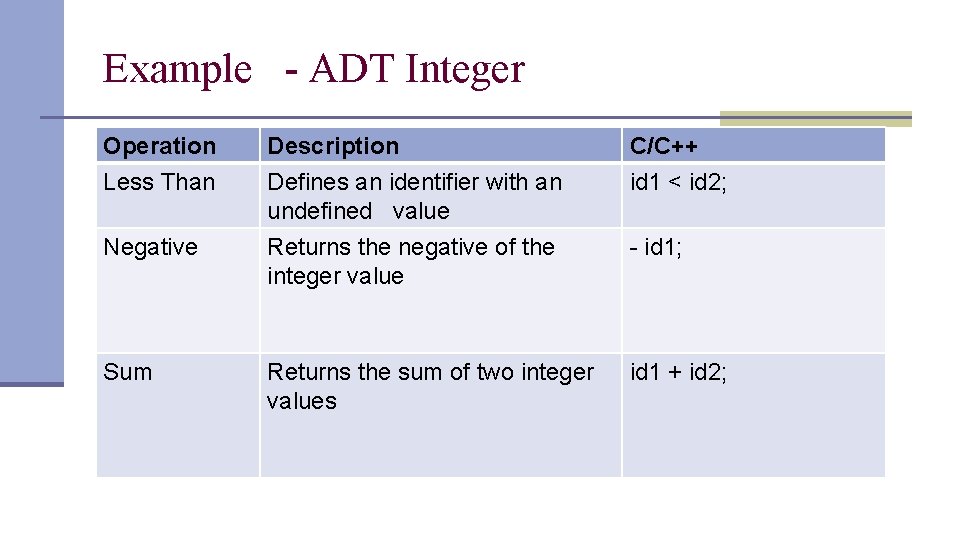
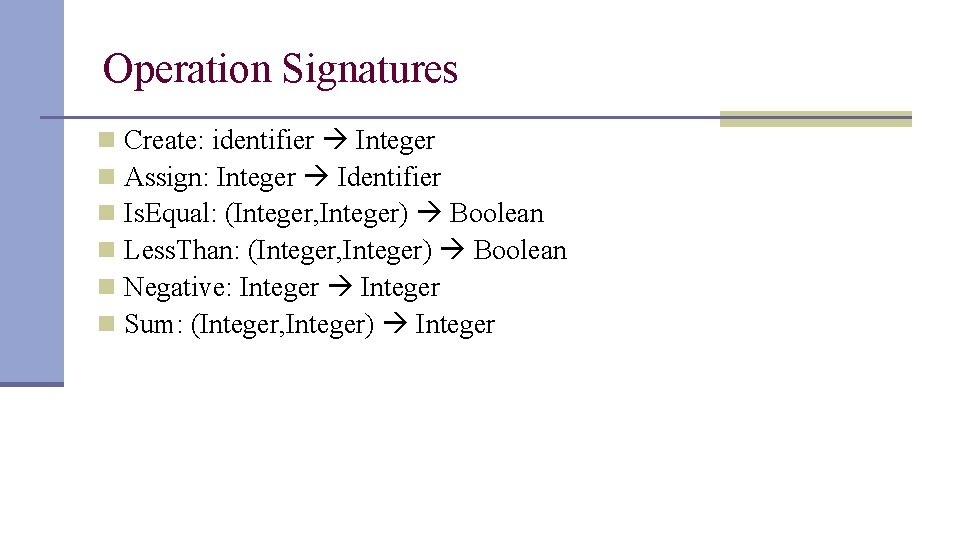
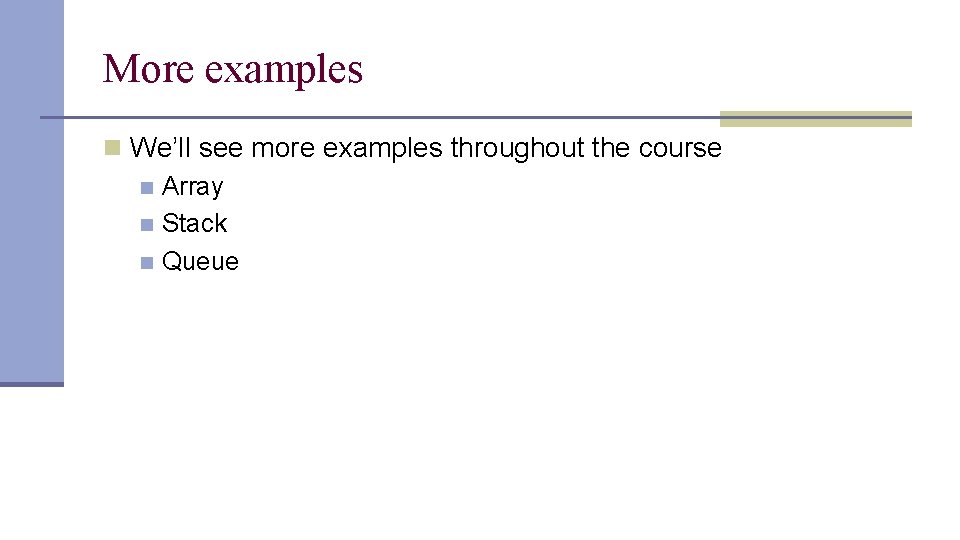
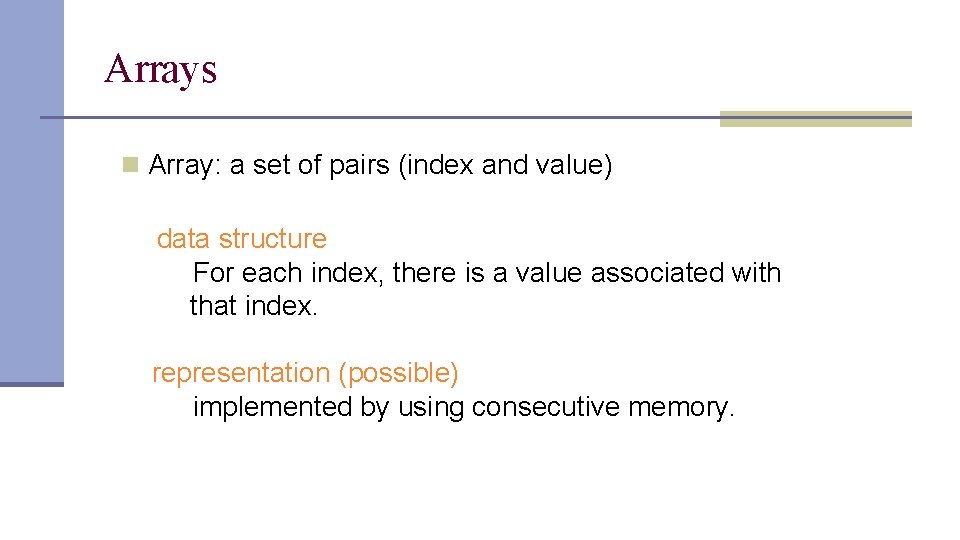
![Arrays in C++ int list[5], *plist[5]; list[5]: five integers list[0], list[1], list[2], list[3], list[4] Arrays in C++ int list[5], *plist[5]; list[5]: five integers list[0], list[1], list[2], list[3], list[4]](https://slidetodoc.com/presentation_image_h/f243d7bbb7055caad0a299ddc58cf3d4/image-15.jpg)
![Arrays in C++ (cont’d) Compare int *list 1 and int list 2[5] in C++. Arrays in C++ (cont’d) Compare int *list 1 and int list 2[5] in C++.](https://slidetodoc.com/presentation_image_h/f243d7bbb7055caad0a299ddc58cf3d4/image-16.jpg)
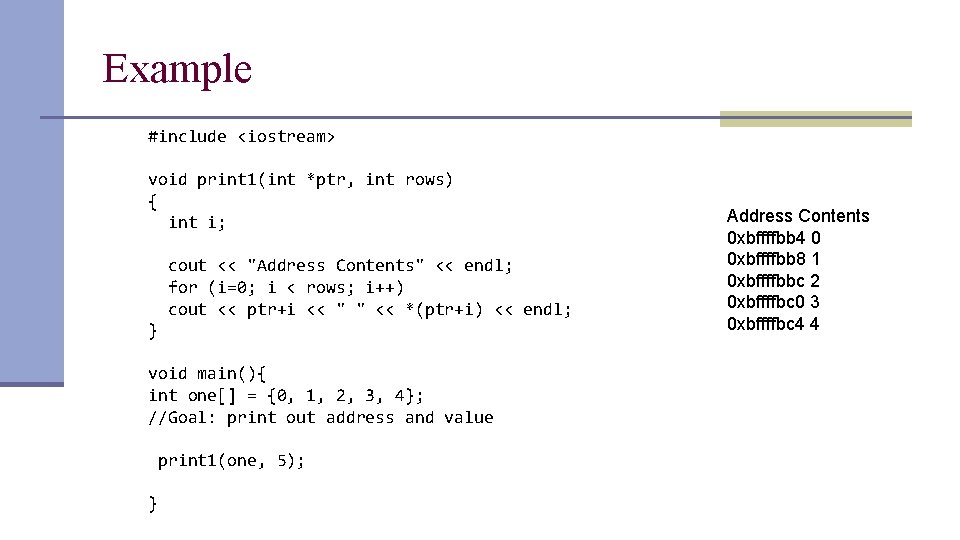
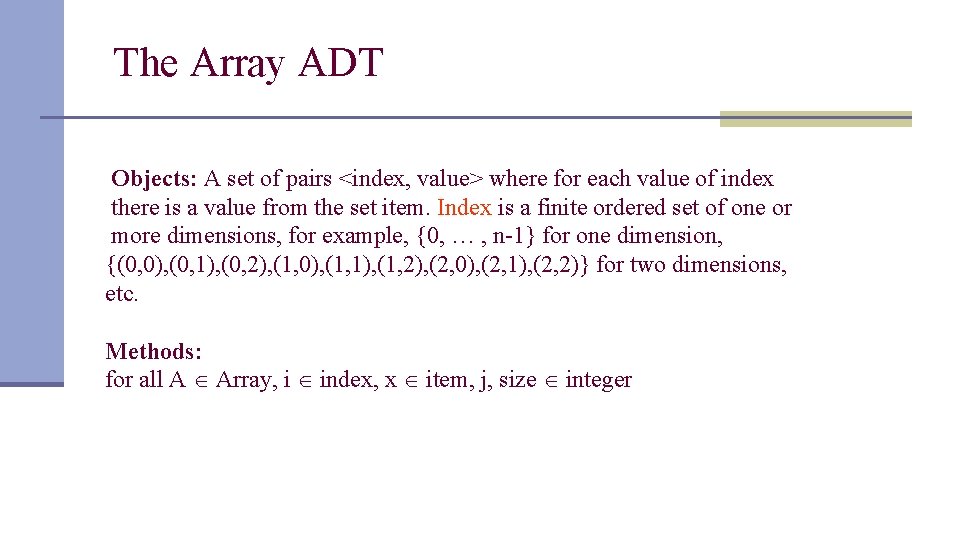
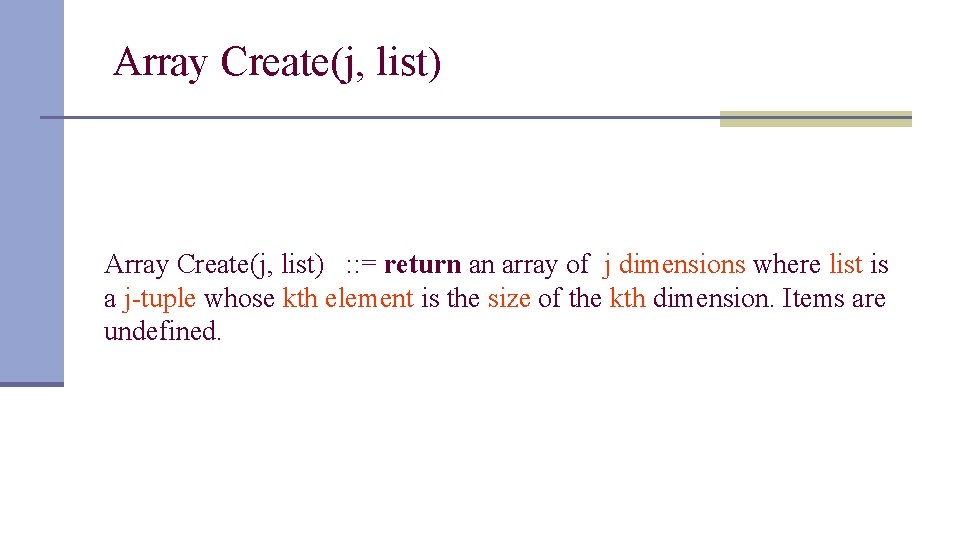
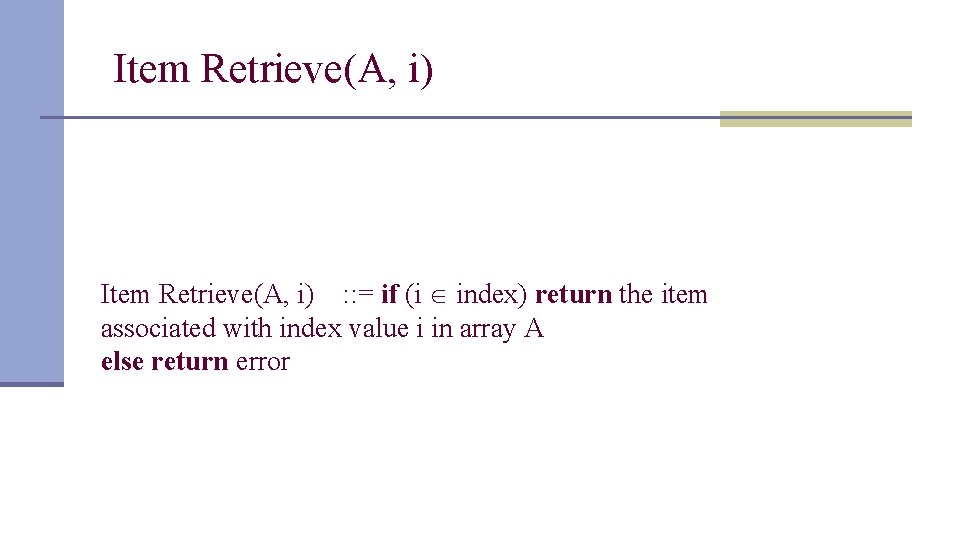
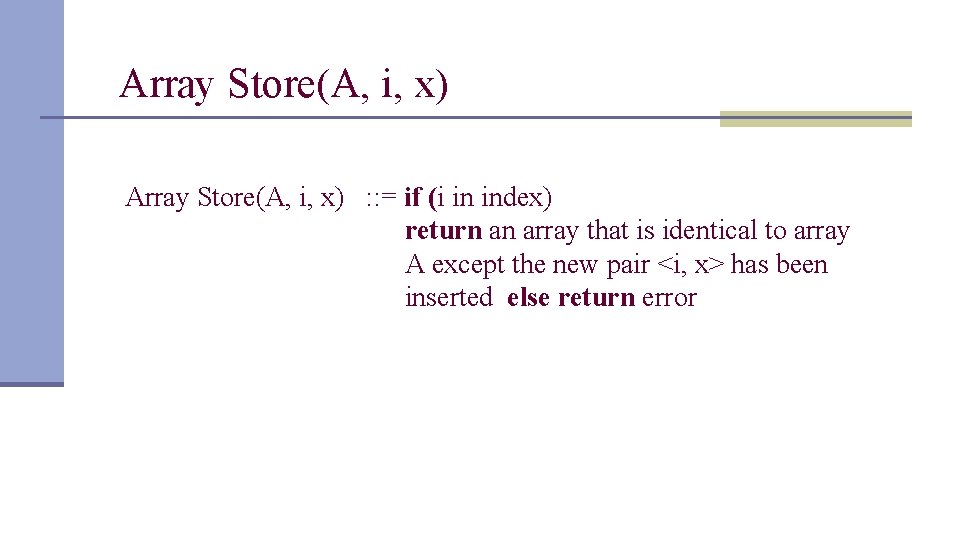
- Slides: 21
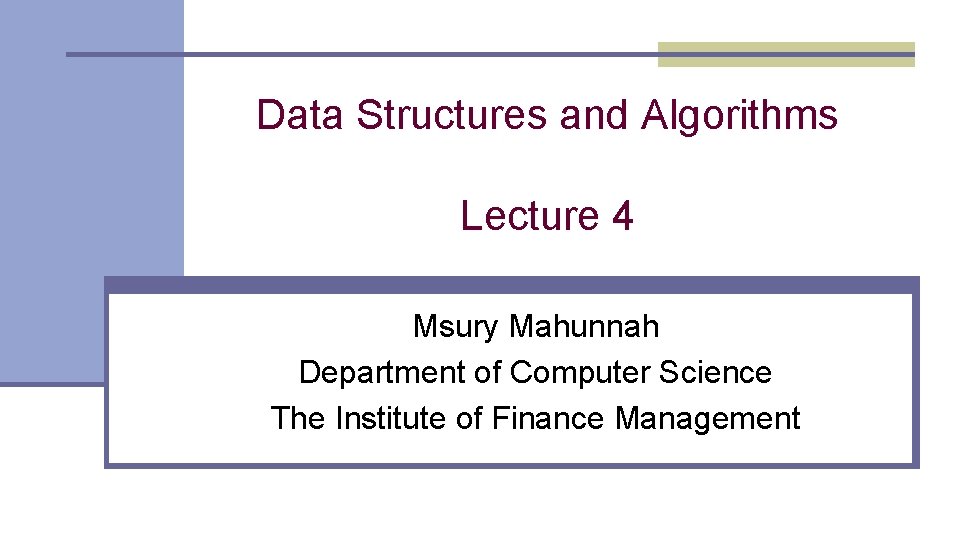
Data Structures and Algorithms Lecture 4 Msury Mahunnah Department of Computer Science The Institute of Finance Management
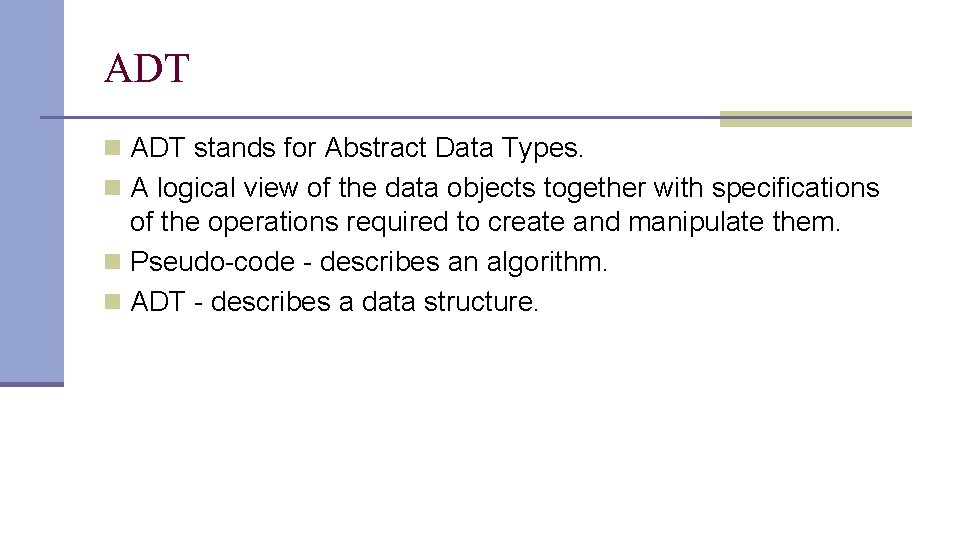
ADT n ADT stands for Abstract Data Types. n A logical view of the data objects together with specifications of the operations required to create and manipulate them. n Pseudo-code - describes an algorithm. n ADT - describes a data structure.
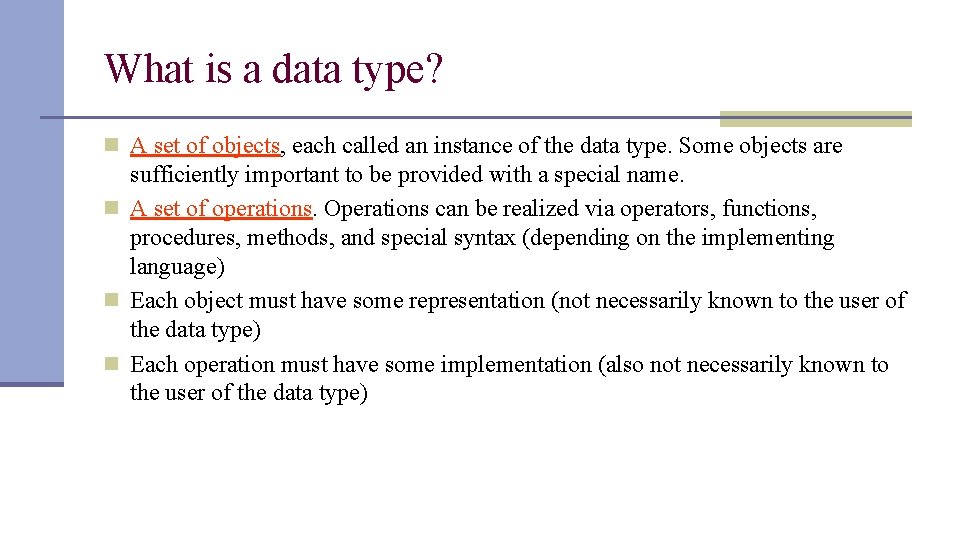
What is a data type? n A set of objects, each called an instance of the data type. Some objects are sufficiently important to be provided with a special name. n A set of operations. Operations can be realized via operators, functions, procedures, methods, and special syntax (depending on the implementing language) n Each object must have some representation (not necessarily known to the user of the data type) n Each operation must have some implementation (also not necessarily known to the user of the data type)
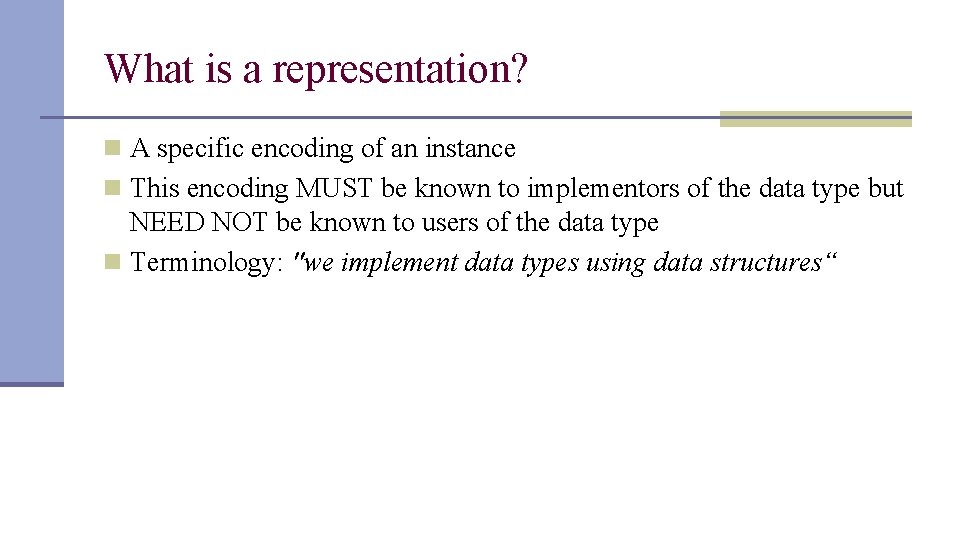
What is a representation? n A specific encoding of an instance n This encoding MUST be known to implementors of the data type but NEED NOT be known to users of the data type n Terminology: "we implement data types using data structures“
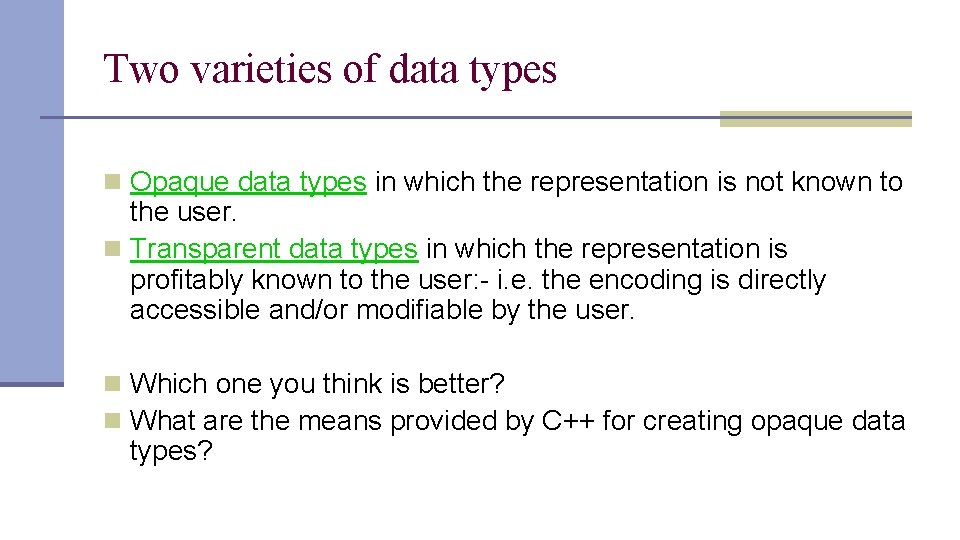
Two varieties of data types n Opaque data types in which the representation is not known to the user. n Transparent data types in which the representation is profitably known to the user: - i. e. the encoding is directly accessible and/or modifiable by the user. n Which one you think is better? n What are the means provided by C++ for creating opaque data types?
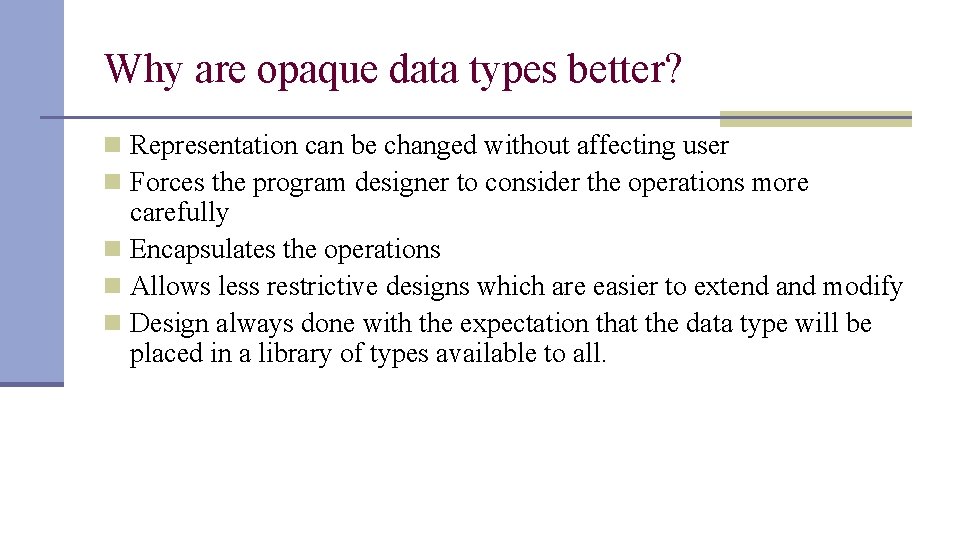
Why are opaque data types better? n Representation can be changed without affecting user n Forces the program designer to consider the operations more carefully n Encapsulates the operations n Allows less restrictive designs which are easier to extend and modify n Design always done with the expectation that the data type will be placed in a library of types available to all.
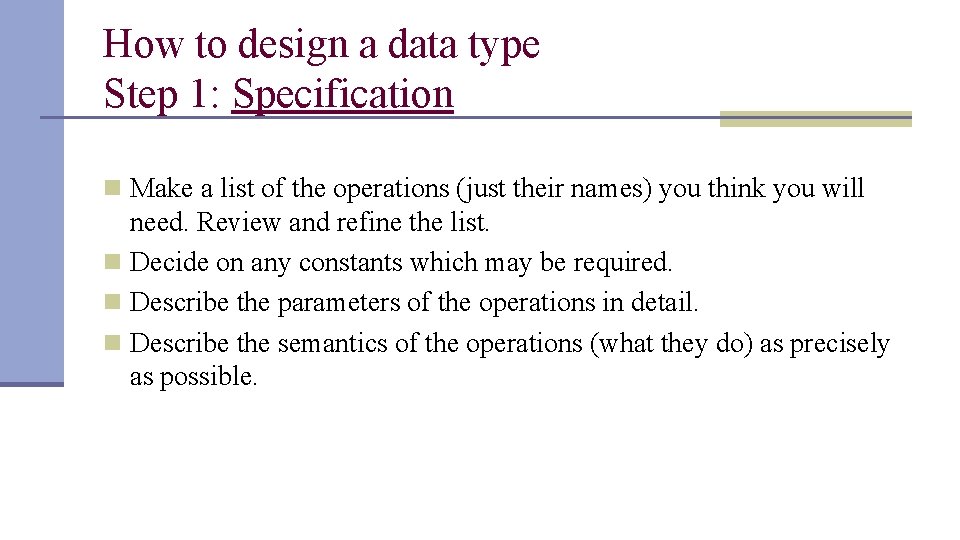
How to design a data type Step 1: Specification n Make a list of the operations (just their names) you think you will need. Review and refine the list. n Decide on any constants which may be required. n Describe the parameters of the operations in detail. n Describe the semantics of the operations (what they do) as precisely as possible.
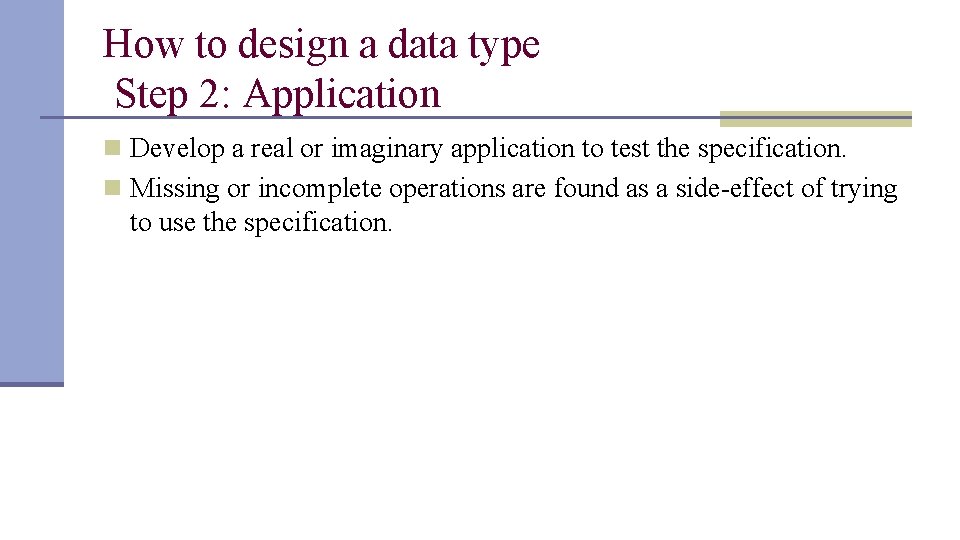
How to design a data type Step 2: Application n Develop a real or imaginary application to test the specification. n Missing or incomplete operations are found as a side-effect of trying to use the specification.
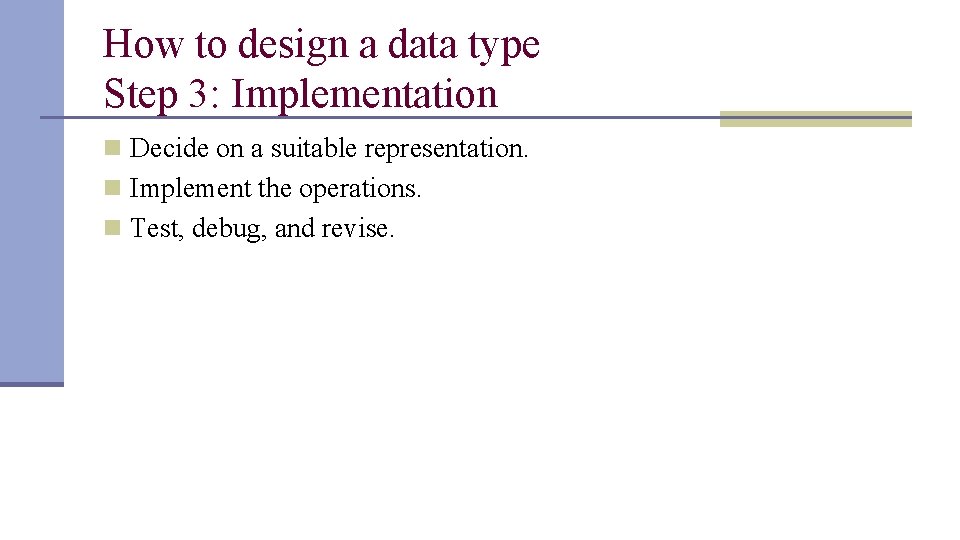
How to design a data type Step 3: Implementation n Decide on a suitable representation. n Implement the operations. n Test, debug, and revise.
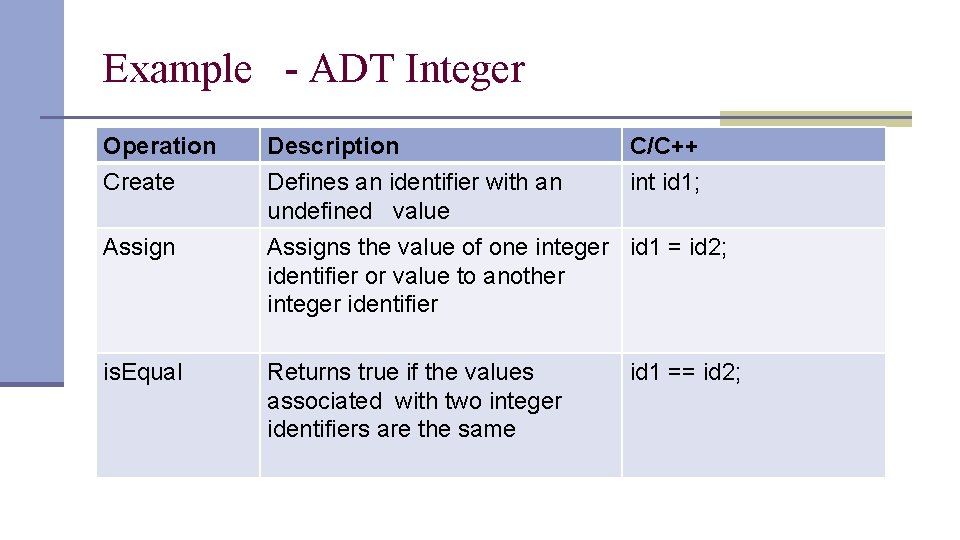
Example - ADT Integer Operation Create Description Defines an identifier with an undefined value C/C++ int id 1; Assigns the value of one integer id 1 = id 2; identifier or value to another integer identifier is. Equal Returns true if the values associated with two integer identifiers are the same id 1 == id 2;
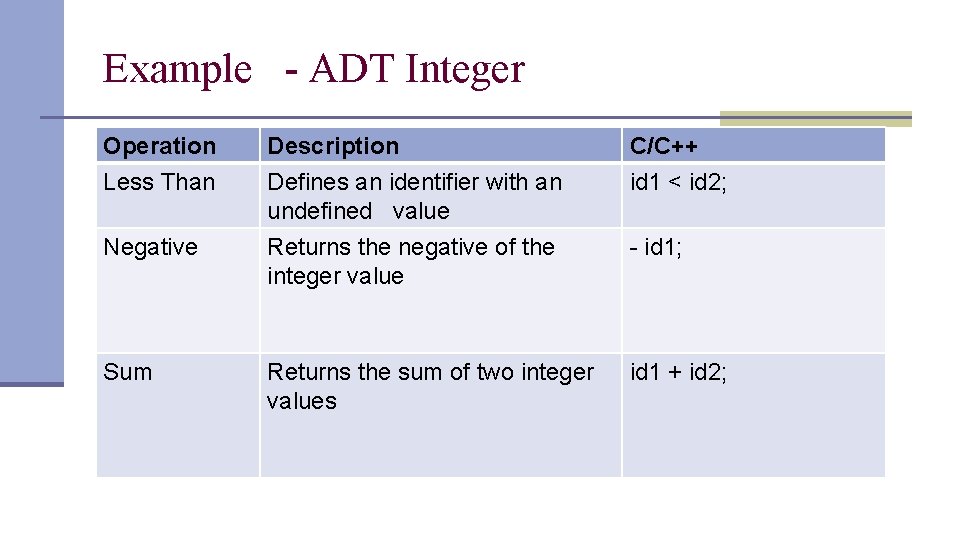
Example - ADT Integer Operation Less Than Description Defines an identifier with an undefined value C/C++ id 1 < id 2; Negative Returns the negative of the integer value - id 1; Sum Returns the sum of two integer values id 1 + id 2;
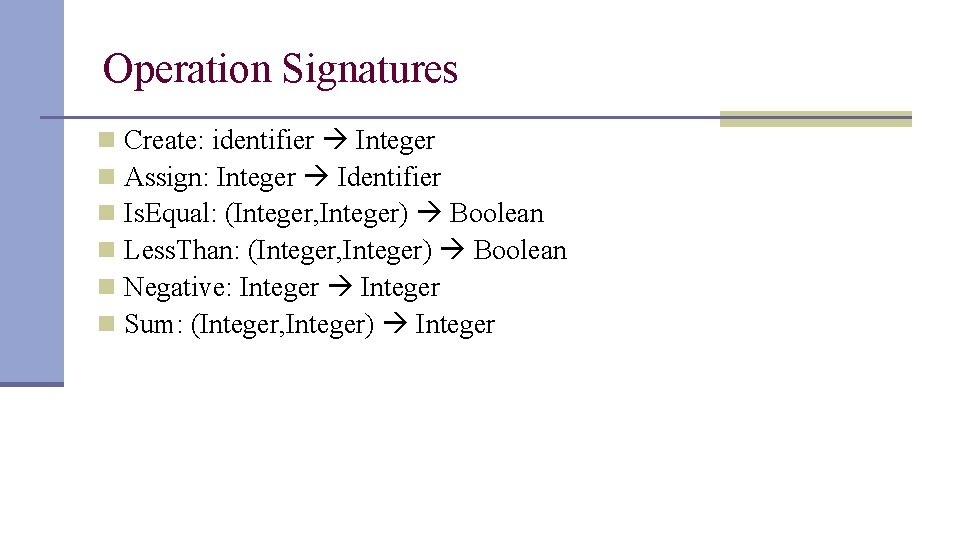
Operation Signatures n n n Create: identifier Integer Assign: Integer Identifier Is. Equal: (Integer, Integer) Boolean Less. Than: (Integer, Integer) Boolean Negative: Integer Sum: (Integer, Integer) Integer
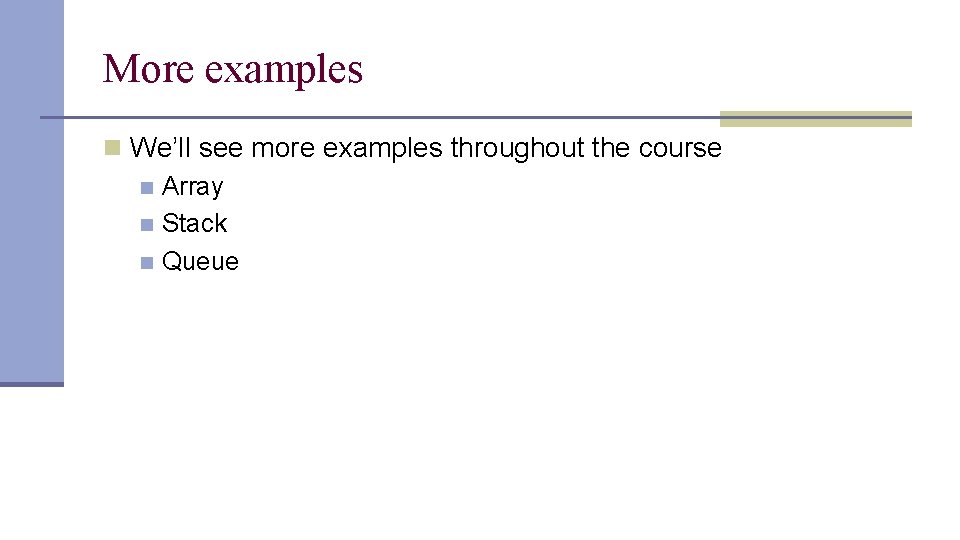
More examples n We’ll see more examples throughout the course n Array n Stack n Queue
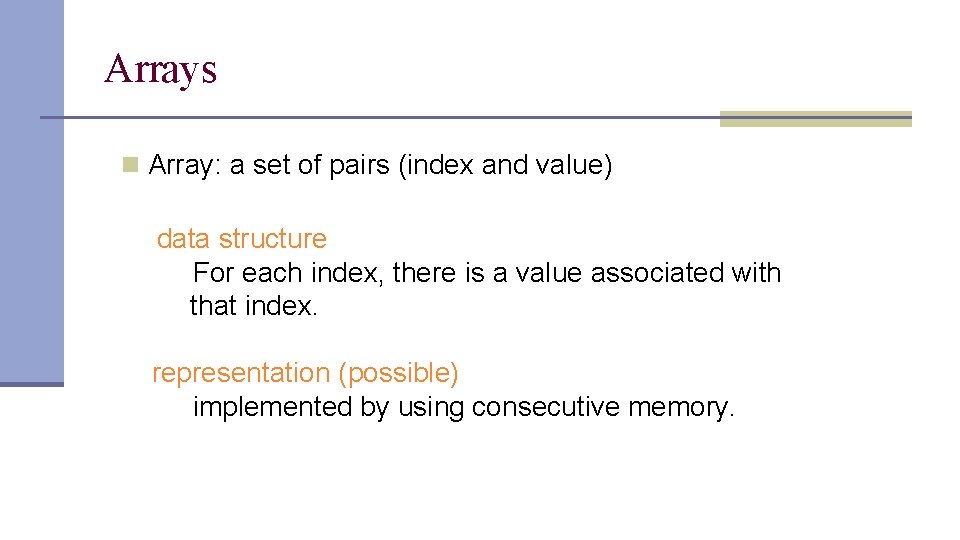
Arrays n Array: a set of pairs (index and value) data structure For each index, there is a value associated with that index. representation (possible) implemented by using consecutive memory.
![Arrays in C int list5 plist5 list5 five integers list0 list1 list2 list3 list4 Arrays in C++ int list[5], *plist[5]; list[5]: five integers list[0], list[1], list[2], list[3], list[4]](https://slidetodoc.com/presentation_image_h/f243d7bbb7055caad0a299ddc58cf3d4/image-15.jpg)
Arrays in C++ int list[5], *plist[5]; list[5]: five integers list[0], list[1], list[2], list[3], list[4] *plist[5]: five pointers to integers plist[0], plist[1], plist[2], plist[3], plist[4] implementation of 1 -D array list[0] base address = list[1] + sizeof(int) list[2] + 2*sizeof(int) list[3] + 3*sizeof(int) list[4] + 4*size(int)
![Arrays in C contd Compare int list 1 and int list 25 in C Arrays in C++ (cont’d) Compare int *list 1 and int list 2[5] in C++.](https://slidetodoc.com/presentation_image_h/f243d7bbb7055caad0a299ddc58cf3d4/image-16.jpg)
Arrays in C++ (cont’d) Compare int *list 1 and int list 2[5] in C++. Same: list 1 and list 2 are pointers. Difference: list 2 reserves five locations. Notations: list 2 - a pointer to list 2[0] (list 2 + i) - a pointer to list 2[i] (&list 2[i]) *(list 2 + i) - list 2[i]
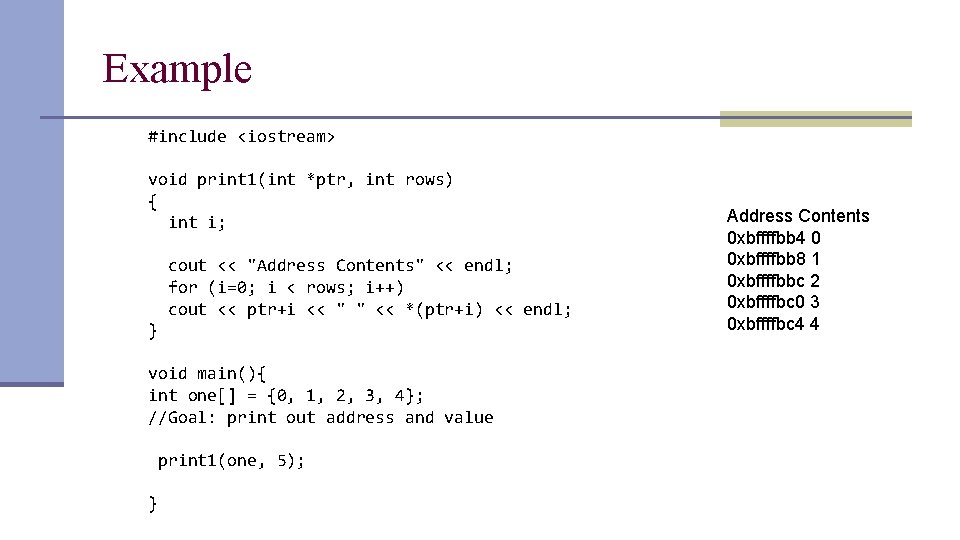
Example #include <iostream> void print 1(int *ptr, int rows) { int i; cout << "Address Contents" << endl; for (i=0; i < rows; i++) cout << ptr+i << " " << *(ptr+i) << endl; } void main(){ int one[] = {0, 1, 2, 3, 4}; //Goal: print out address and value print 1(one, 5); } Address Contents 0 xbffffbb 4 0 0 xbffffbb 8 1 0 xbffffbbc 2 0 xbffffbc 0 3 0 xbffffbc 4 4
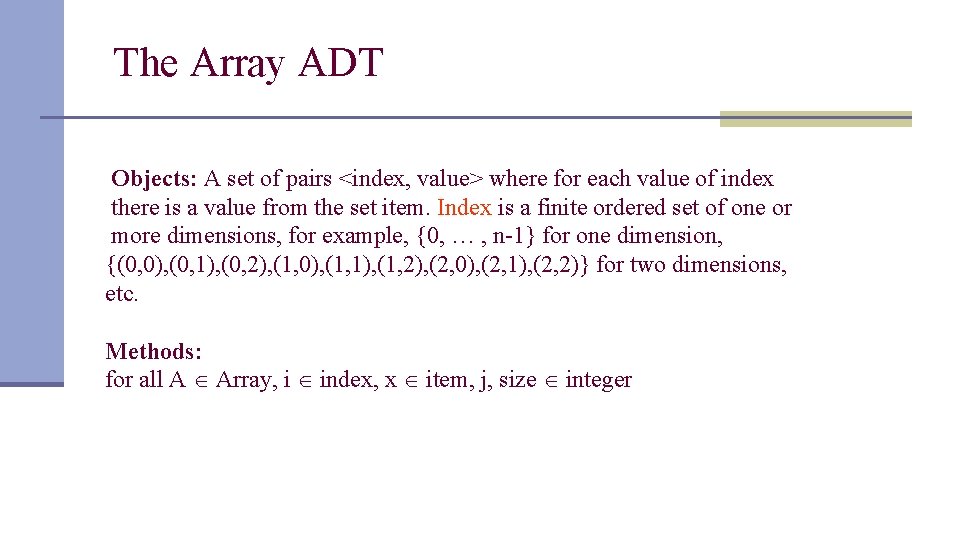
The Array ADT Objects: A set of pairs <index, value> where for each value of index there is a value from the set item. Index is a finite ordered set of one or more dimensions, for example, {0, … , n-1} for one dimension, {(0, 0), (0, 1), (0, 2), (1, 0), (1, 1), (1, 2), (2, 0), (2, 1), (2, 2)} for two dimensions, etc. Methods: for all A Array, i index, x item, j, size integer
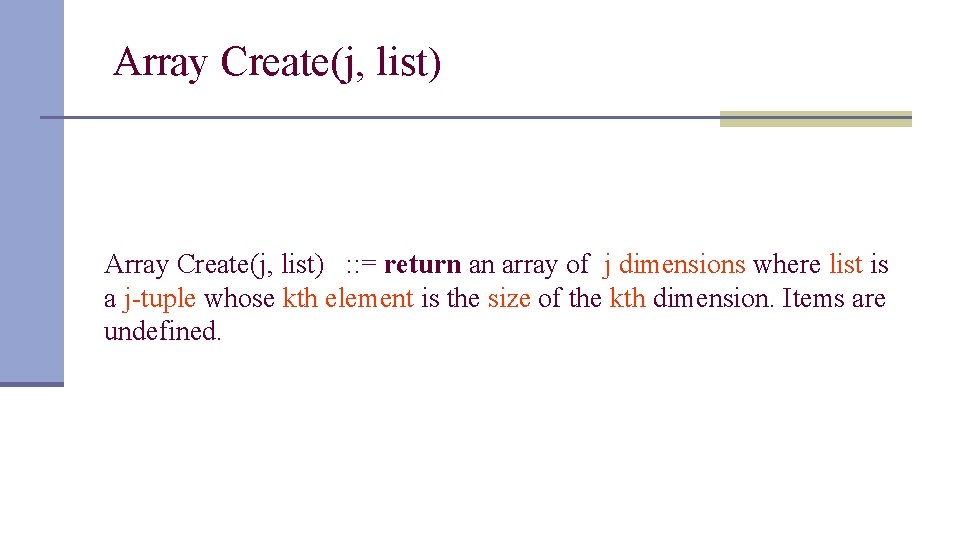
Array Create(j, list) : : = return an array of j dimensions where list is a j-tuple whose kth element is the size of the kth dimension. Items are undefined.
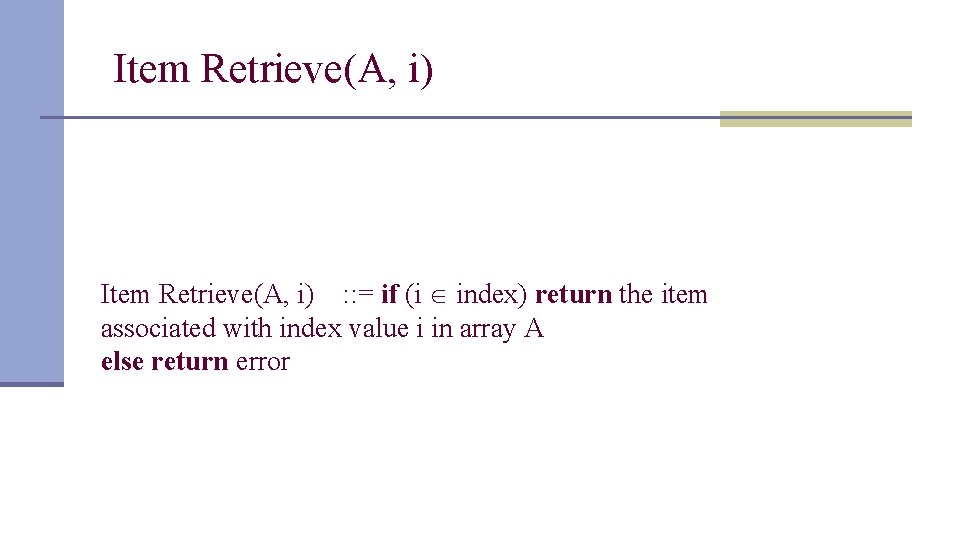
Item Retrieve(A, i) : : = if (i index) return the item associated with index value i in array A else return error
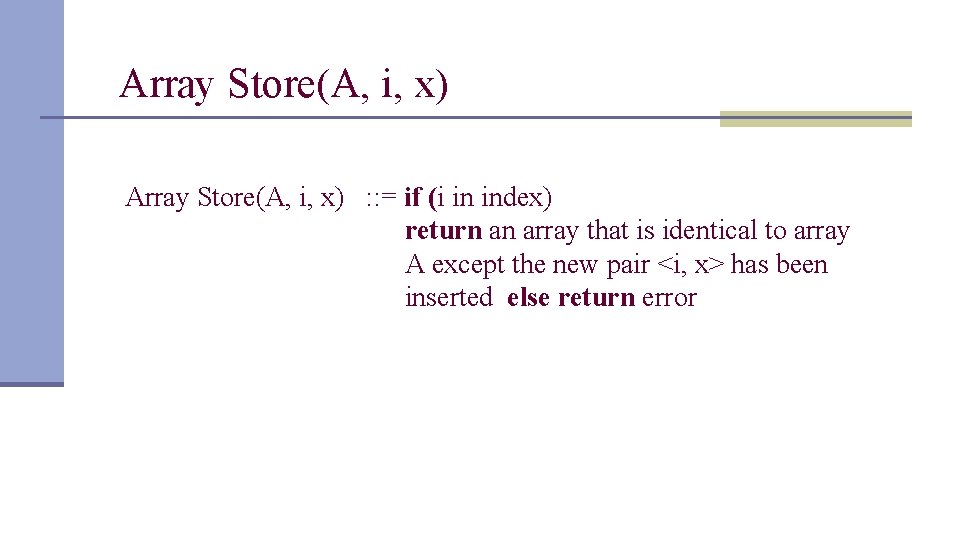
Array Store(A, i, x) : : = if (i in index) return an array that is identical to array A except the new pair <i, x> has been inserted else return error
Data structures and algorithms iit bombay
Cos423
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Data structures and algorithms iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Signature file structure in information retrieval system
Data structures and algorithms
Algorithms + data structures = programs
Msury
Msury
Analysis of algorithms lecture notes
Introduction to algorithms lecture notes
01:640:244 lecture notes - lecture 15: plat, idah, farad
Analogous structure
Muthukrishnan data stream algorithms
Macro processors
Assembler data structures
Data structures and abstractions with java