CSCICMPE 4341 Topic Programming in Python Chapter 3
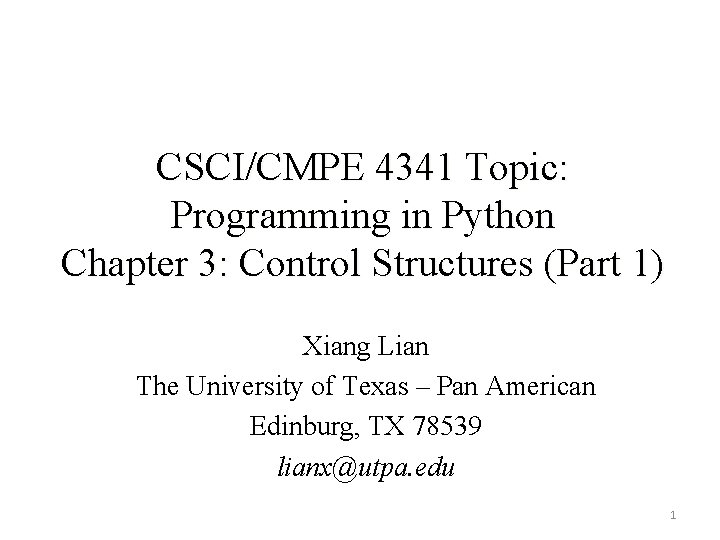
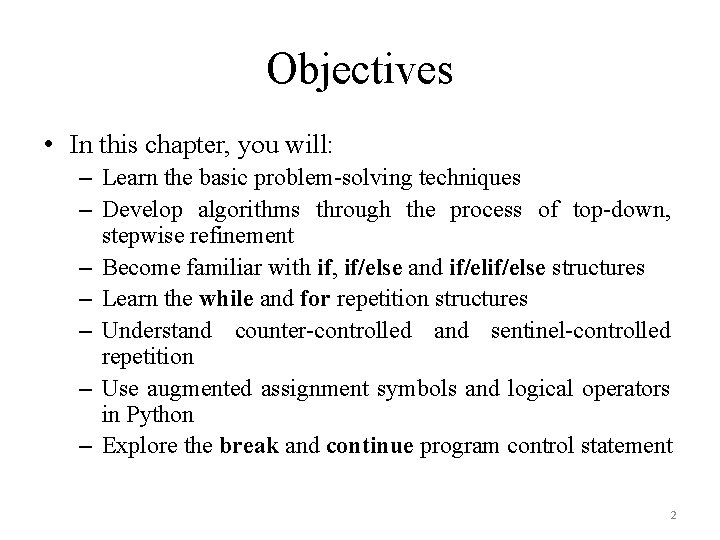
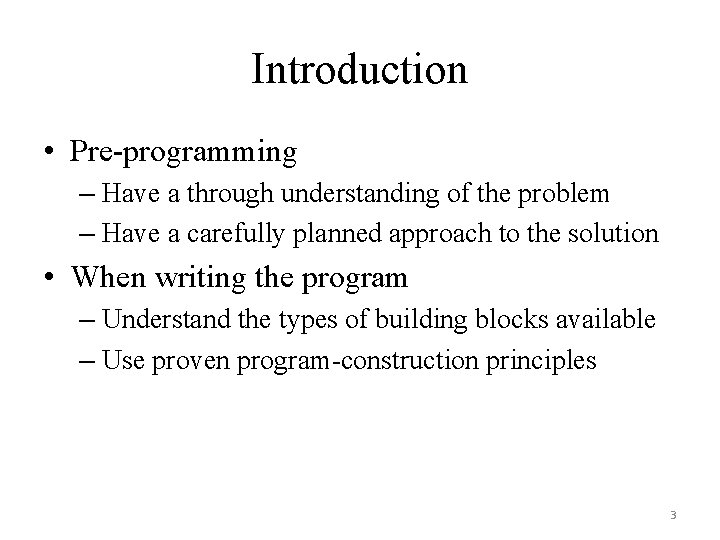
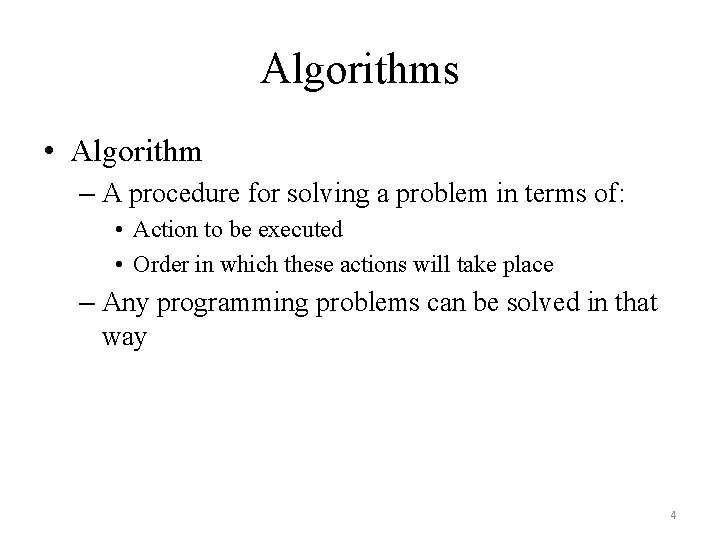
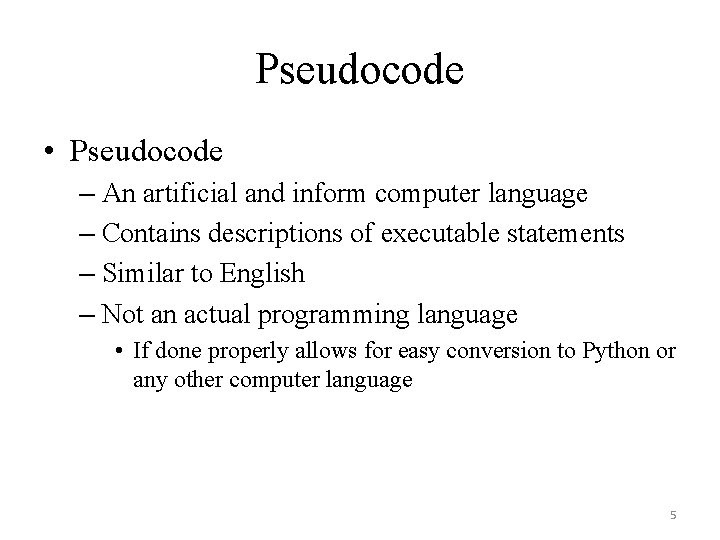
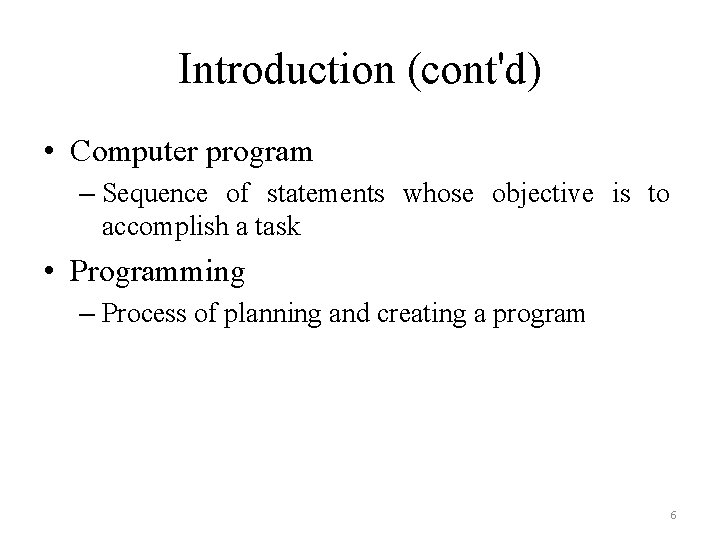
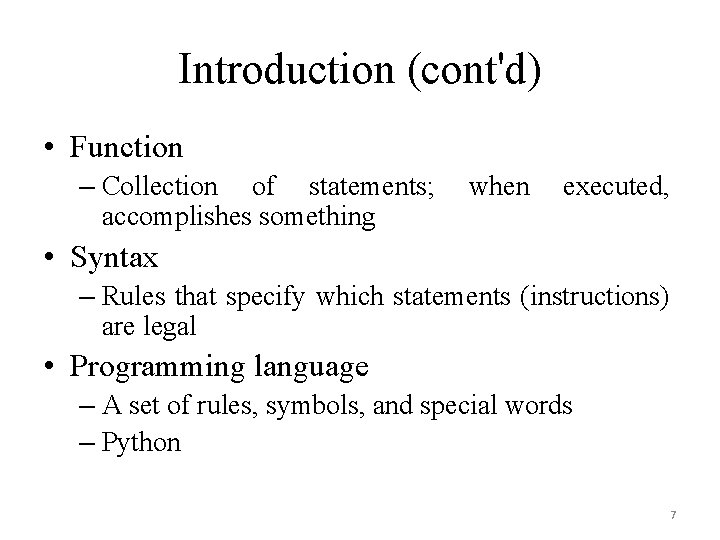
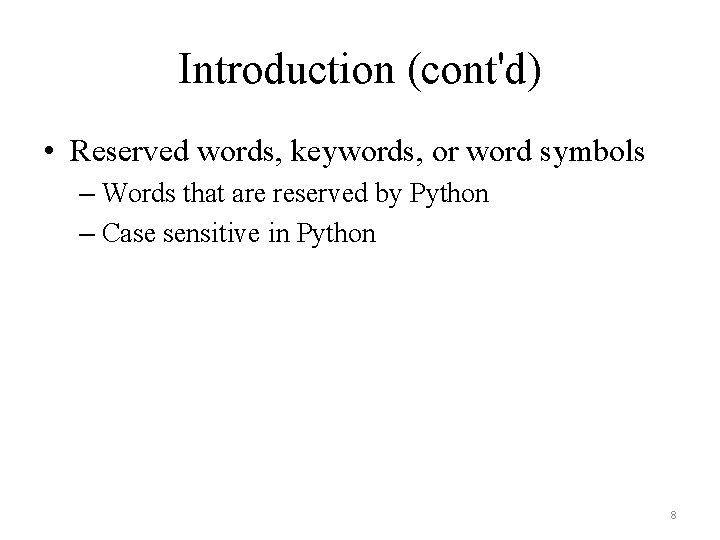
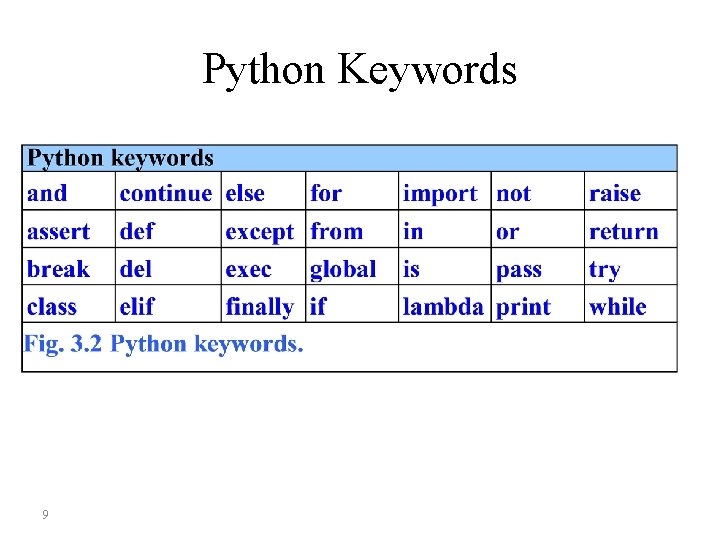
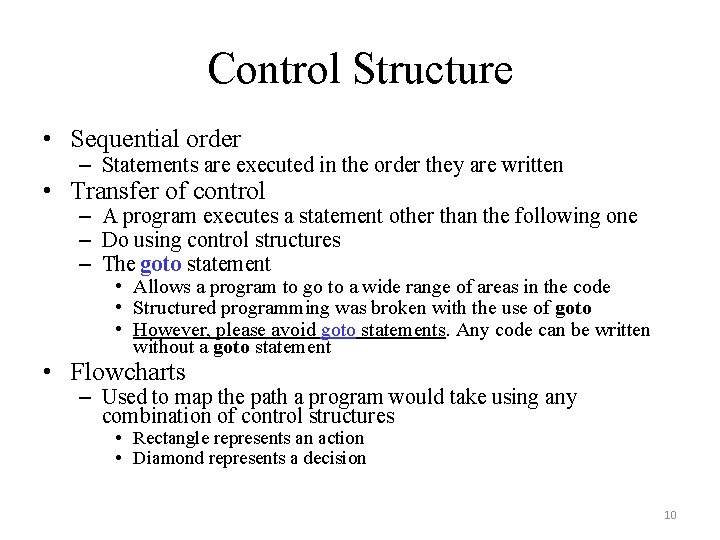
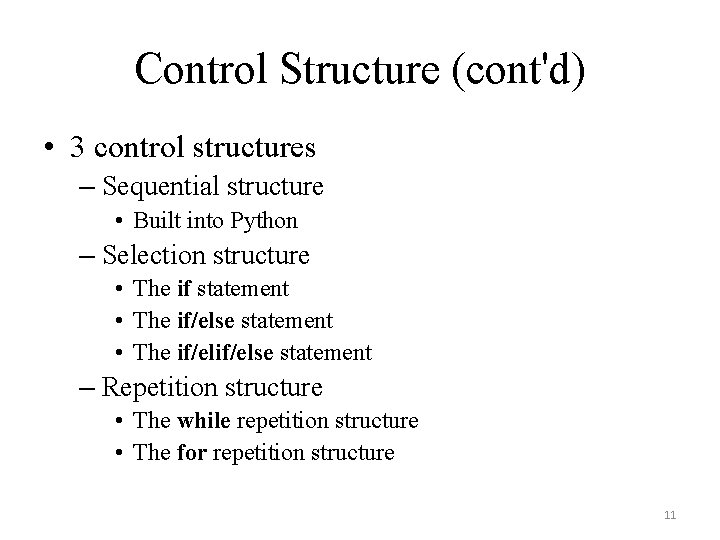
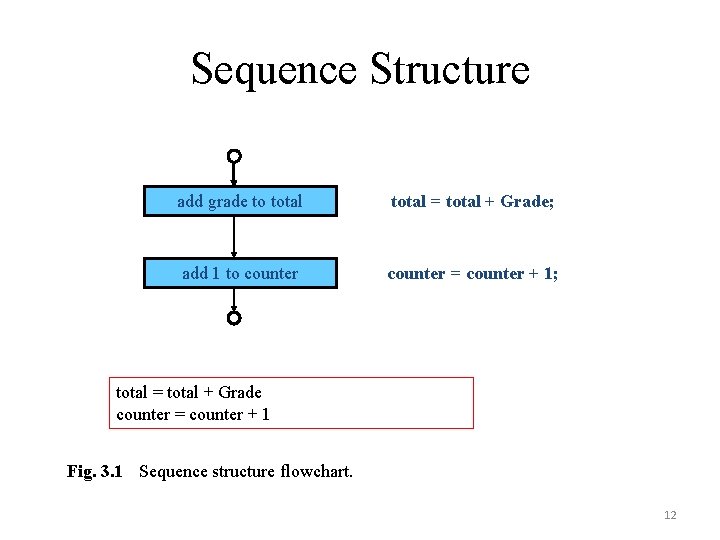
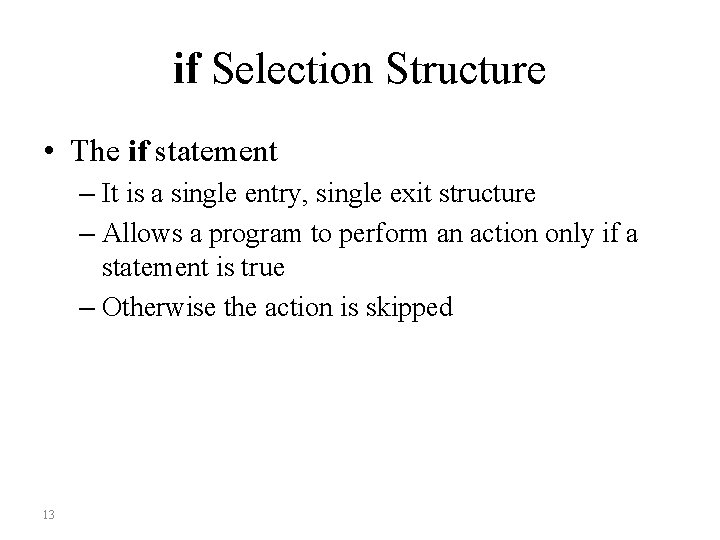
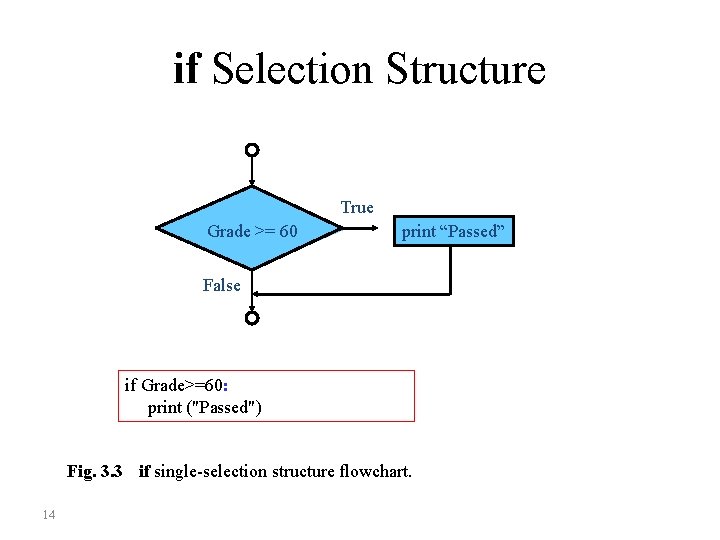
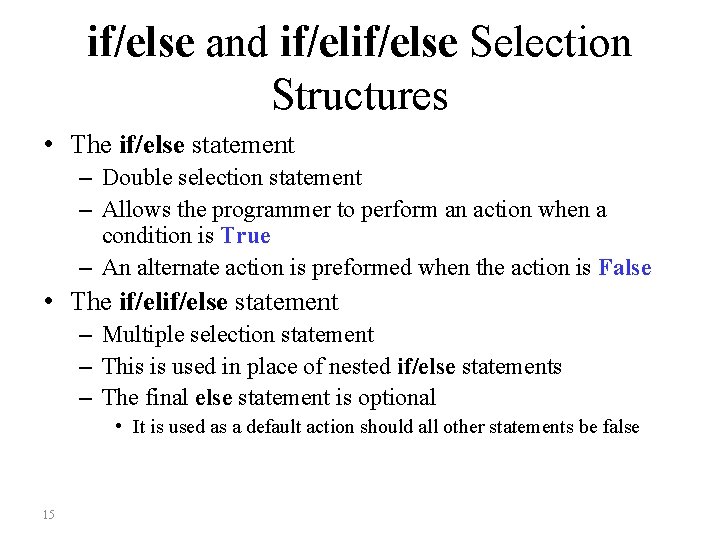
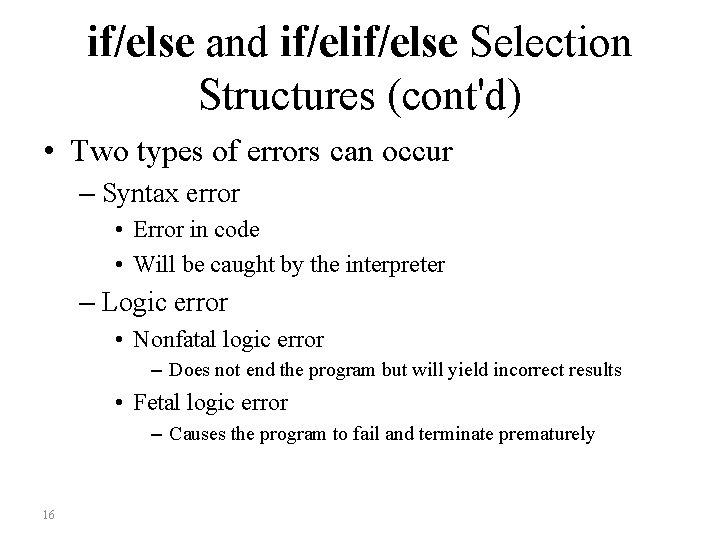
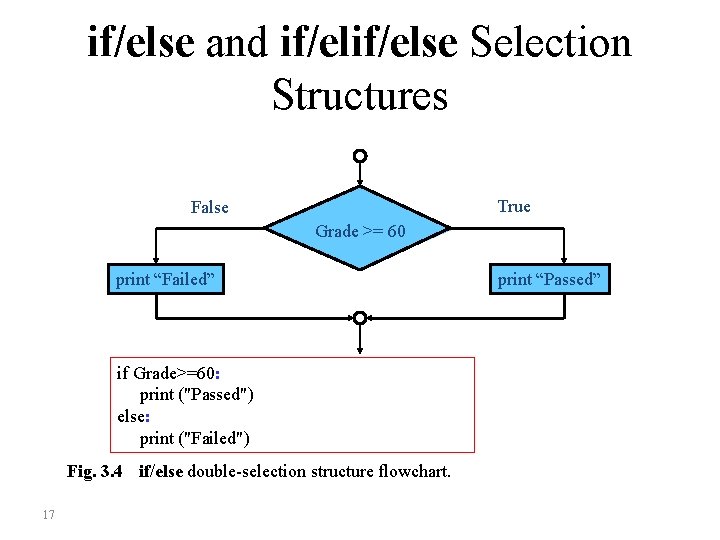
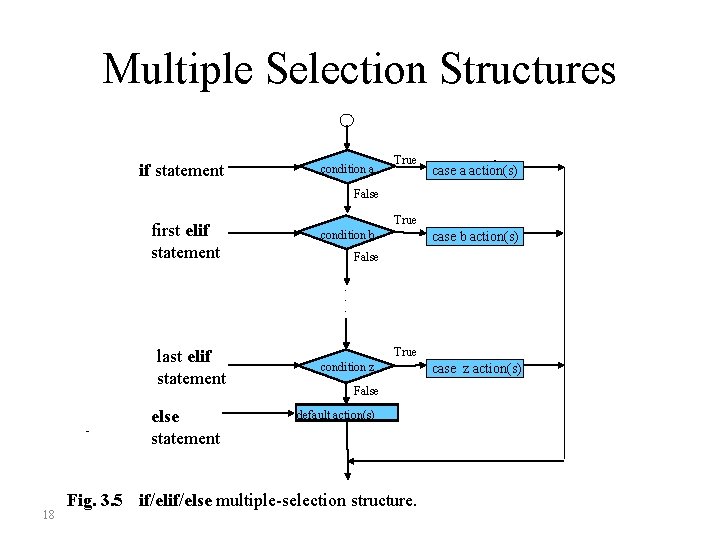
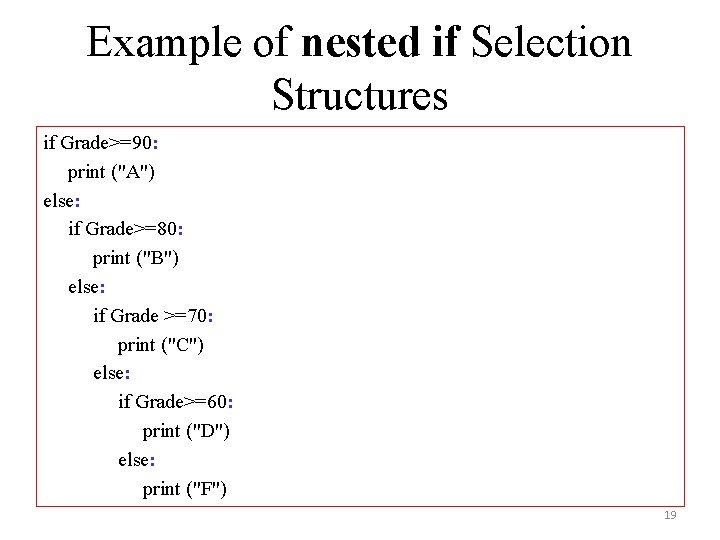
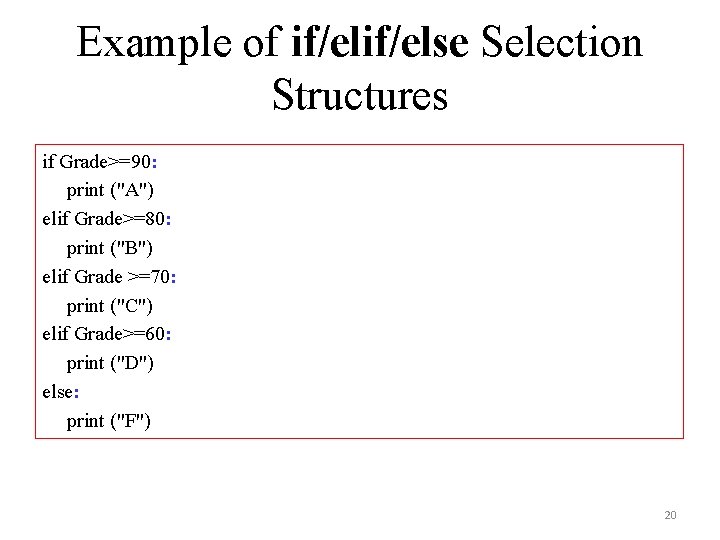
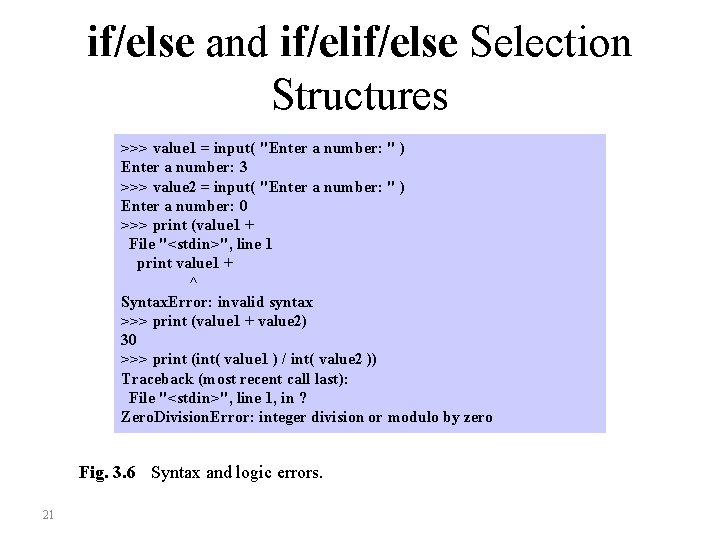
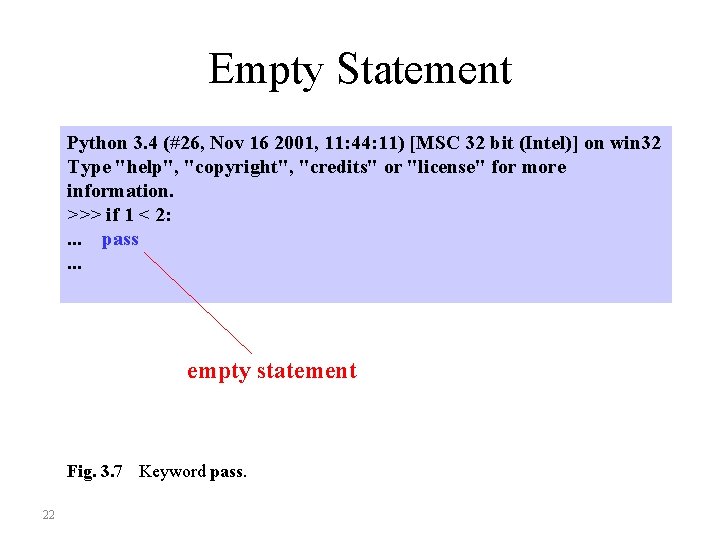
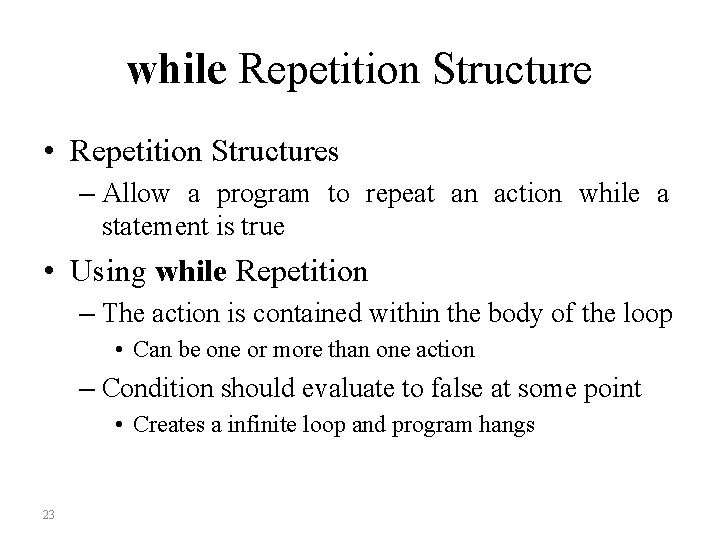
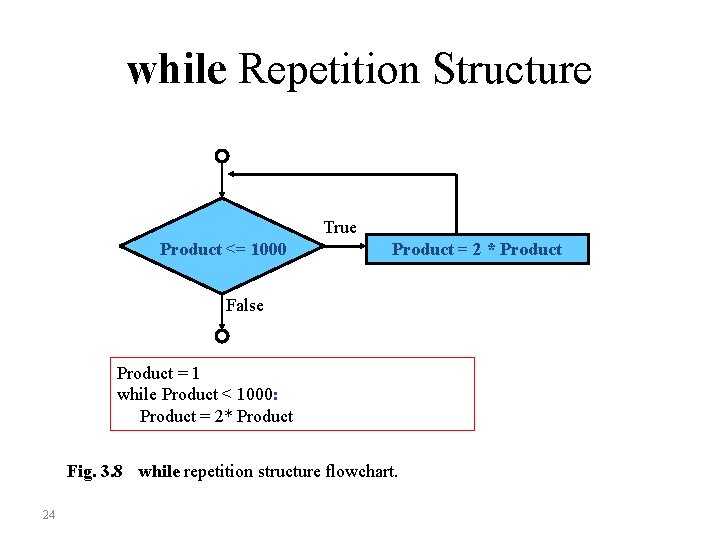
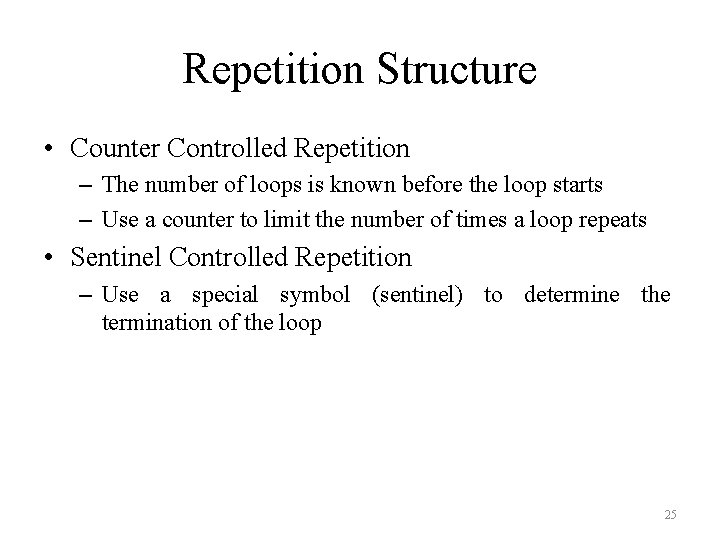
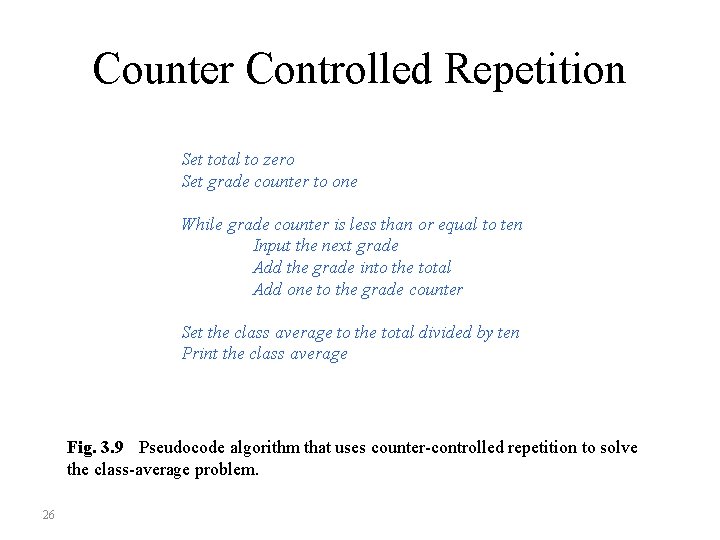
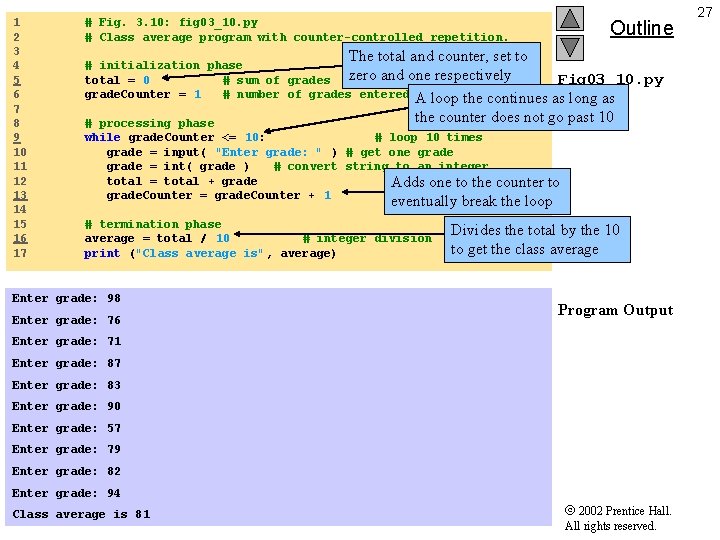
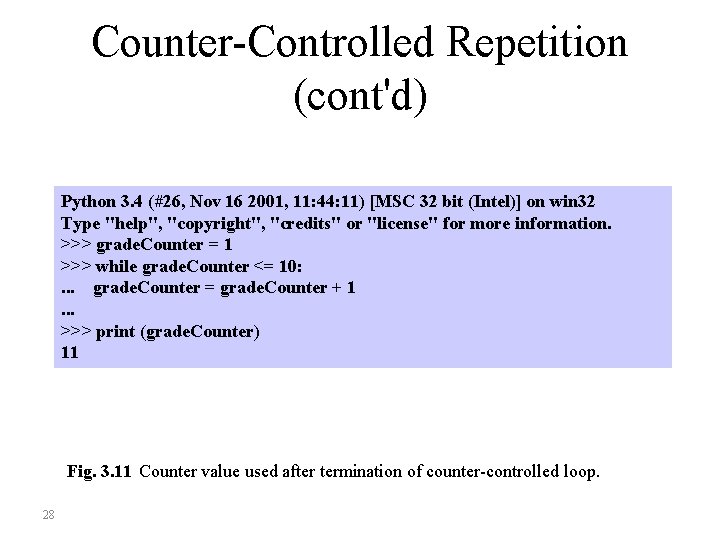
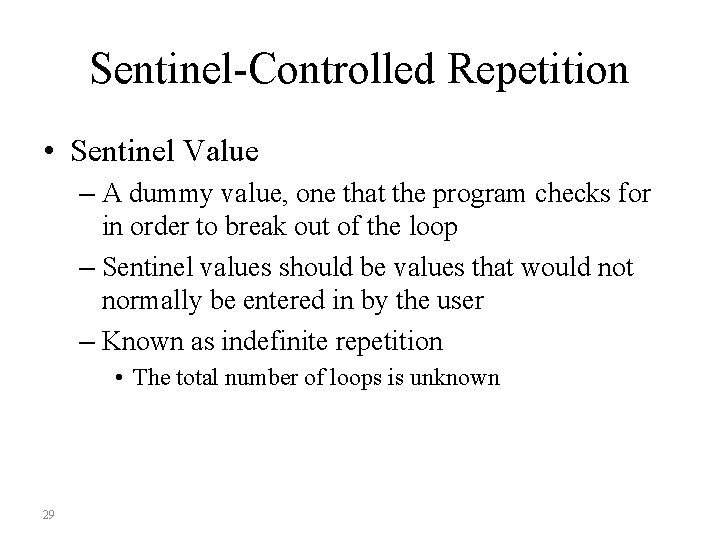
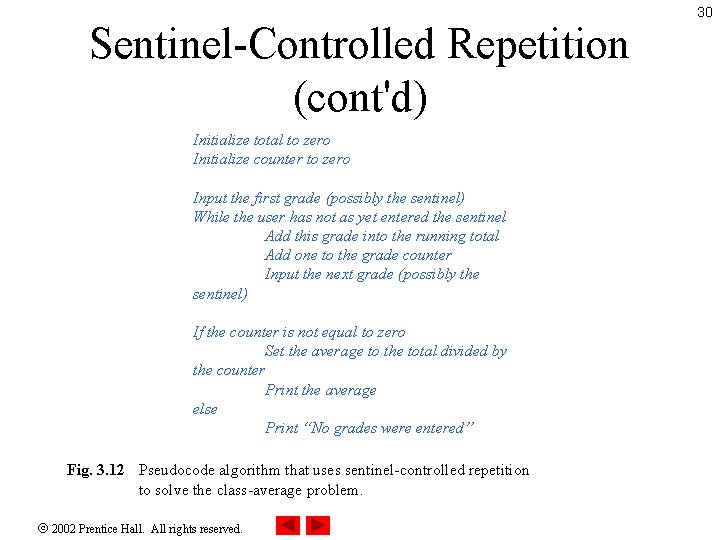
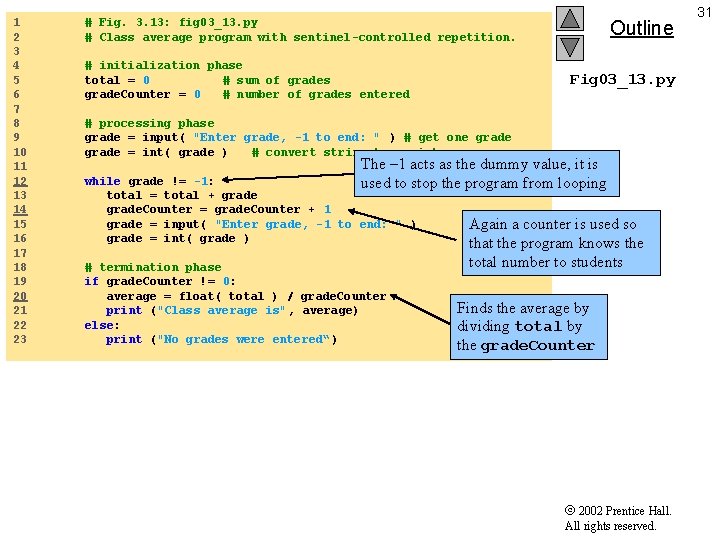
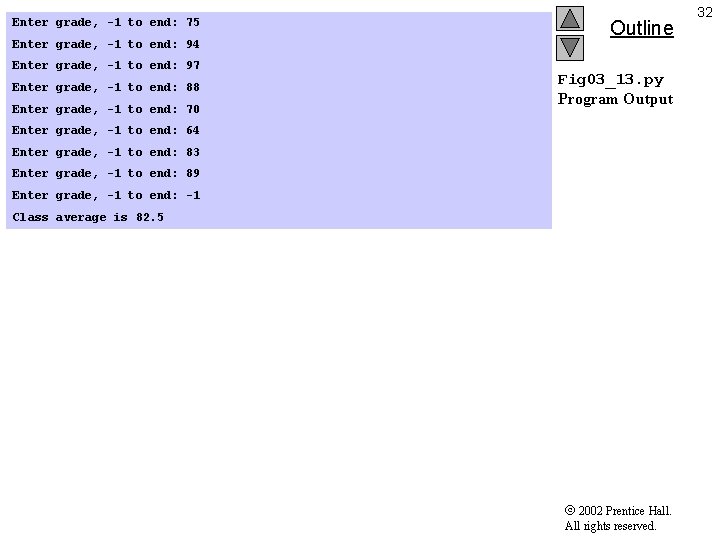
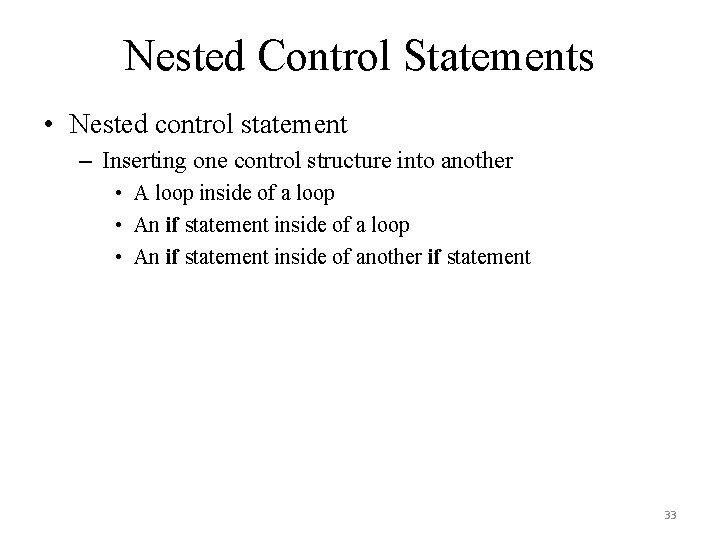
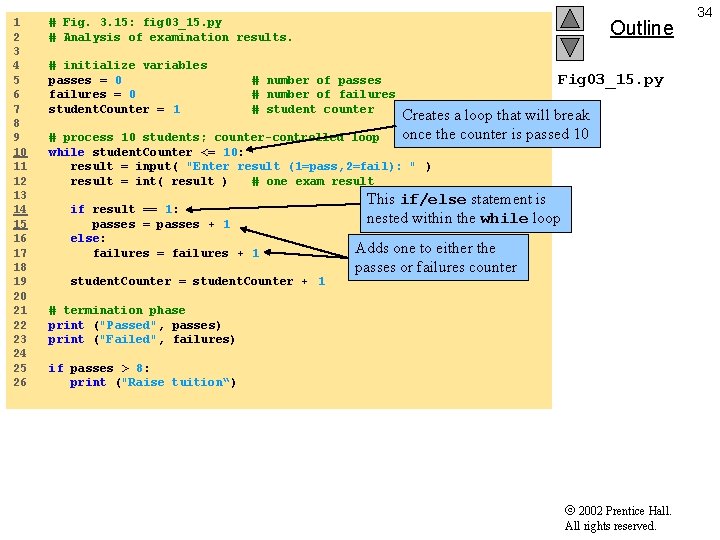
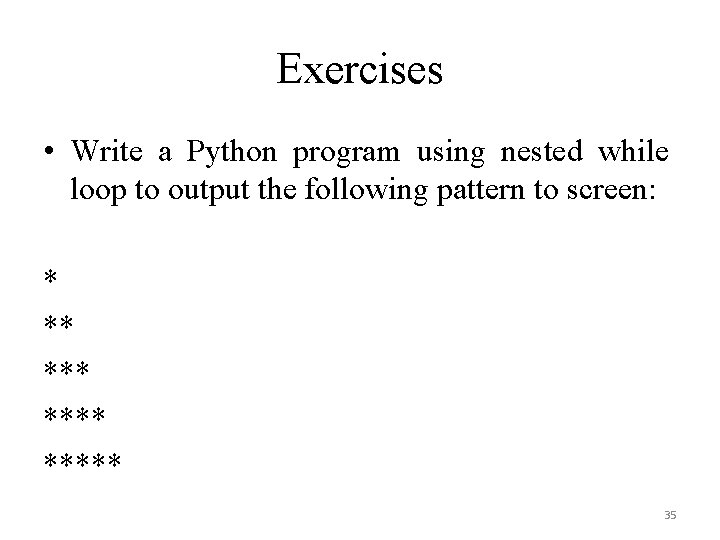
- Slides: 35
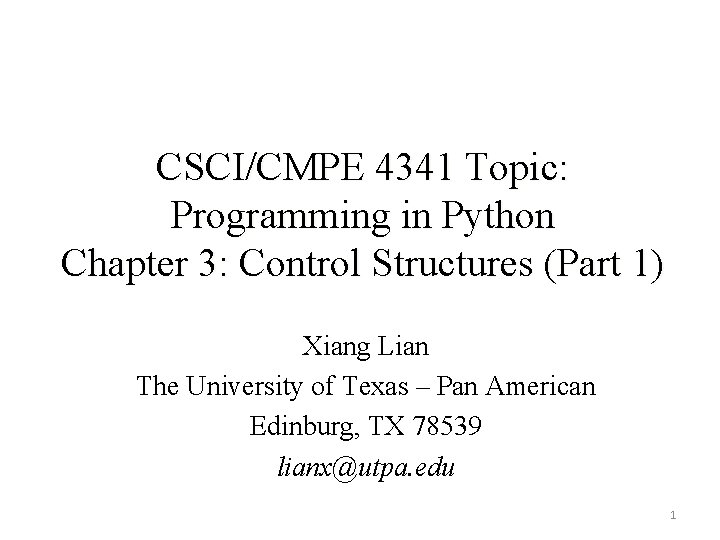
CSCI/CMPE 4341 Topic: Programming in Python Chapter 3: Control Structures (Part 1) Xiang Lian The University of Texas – Pan American Edinburg, TX 78539 lianx@utpa. edu 1
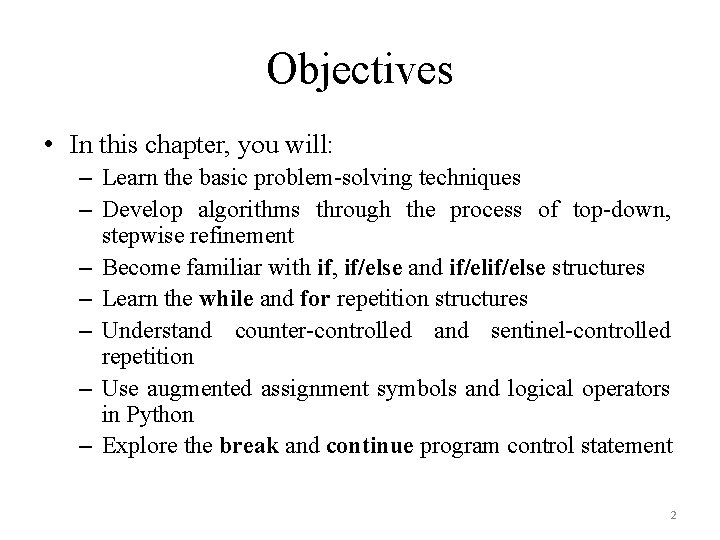
Objectives • In this chapter, you will: – Learn the basic problem-solving techniques – Develop algorithms through the process of top-down, stepwise refinement – Become familiar with if, if/else and if/else structures – Learn the while and for repetition structures – Understand counter-controlled and sentinel-controlled repetition – Use augmented assignment symbols and logical operators in Python – Explore the break and continue program control statement 2
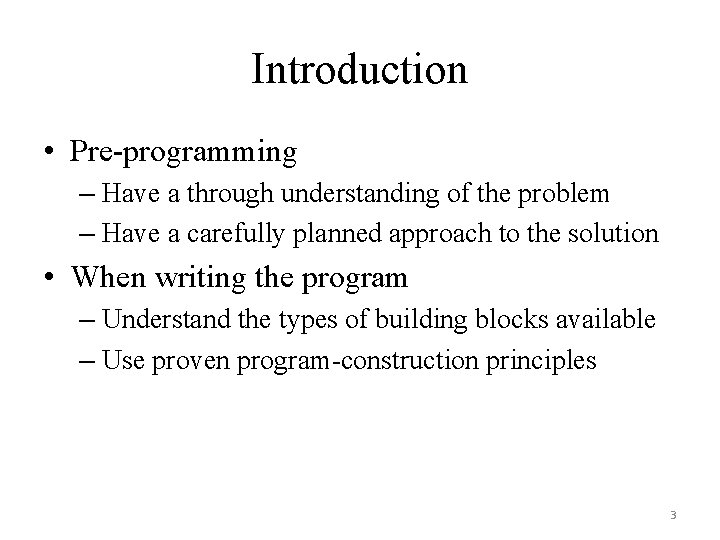
Introduction • Pre-programming – Have a through understanding of the problem – Have a carefully planned approach to the solution • When writing the program – Understand the types of building blocks available – Use proven program-construction principles 3
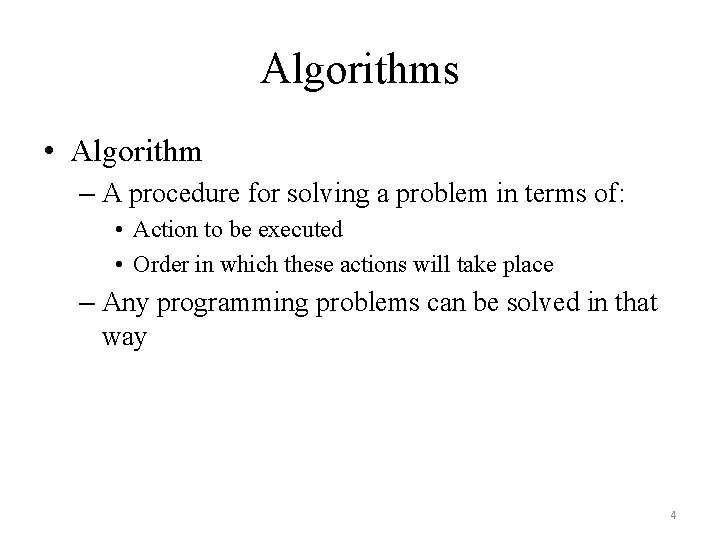
Algorithms • Algorithm – A procedure for solving a problem in terms of: • Action to be executed • Order in which these actions will take place – Any programming problems can be solved in that way 4
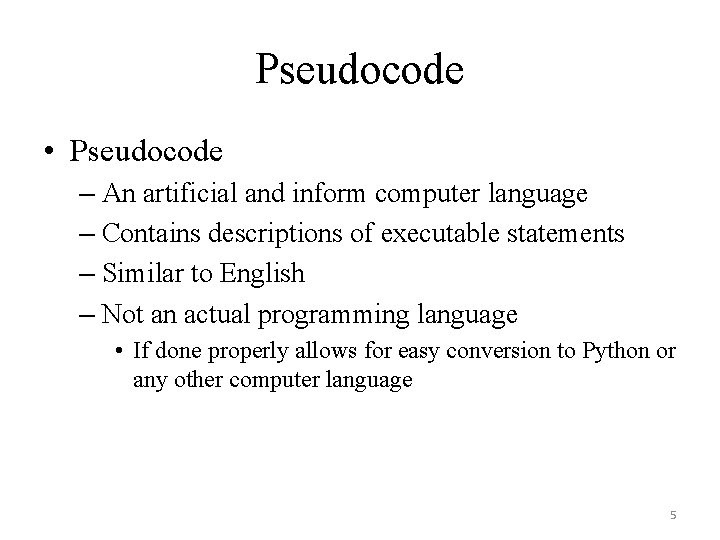
Pseudocode • Pseudocode – An artificial and inform computer language – Contains descriptions of executable statements – Similar to English – Not an actual programming language • If done properly allows for easy conversion to Python or any other computer language 5
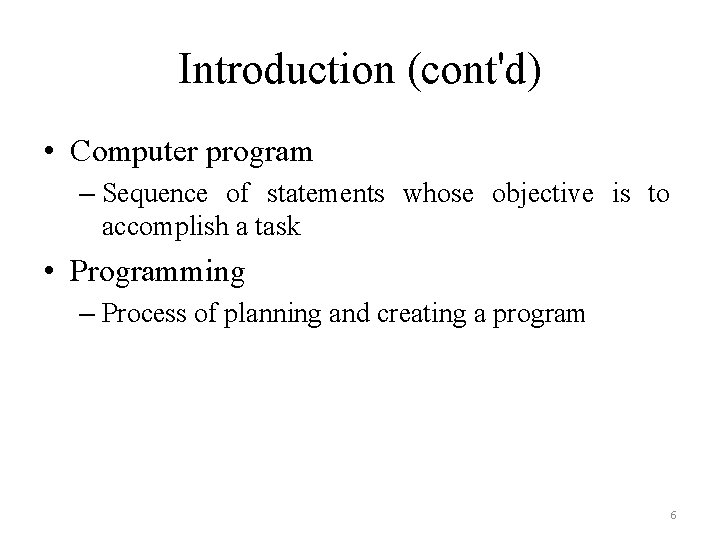
Introduction (cont'd) • Computer program – Sequence of statements whose objective is to accomplish a task • Programming – Process of planning and creating a program 6
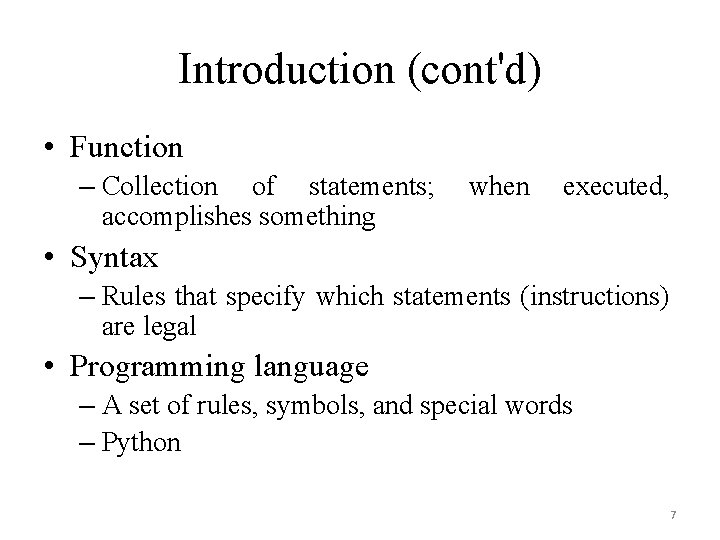
Introduction (cont'd) • Function – Collection of statements; accomplishes something when executed, • Syntax – Rules that specify which statements (instructions) are legal • Programming language – A set of rules, symbols, and special words – Python 7
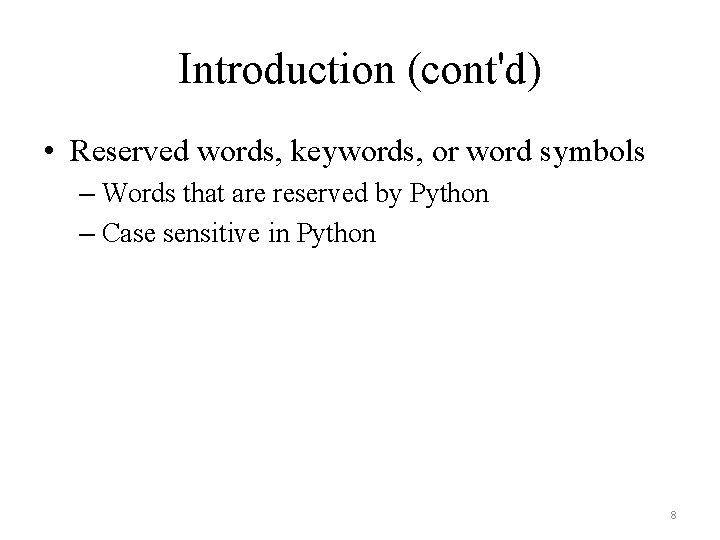
Introduction (cont'd) • Reserved words, keywords, or word symbols – Words that are reserved by Python – Case sensitive in Python 8
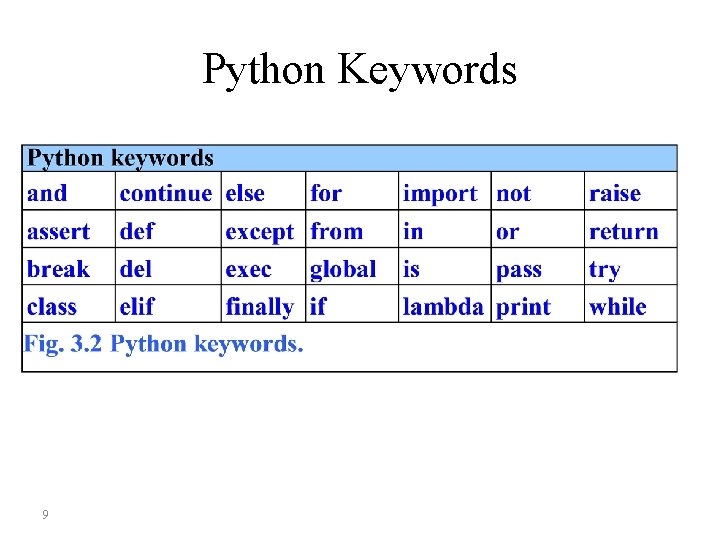
Python Keywords 9
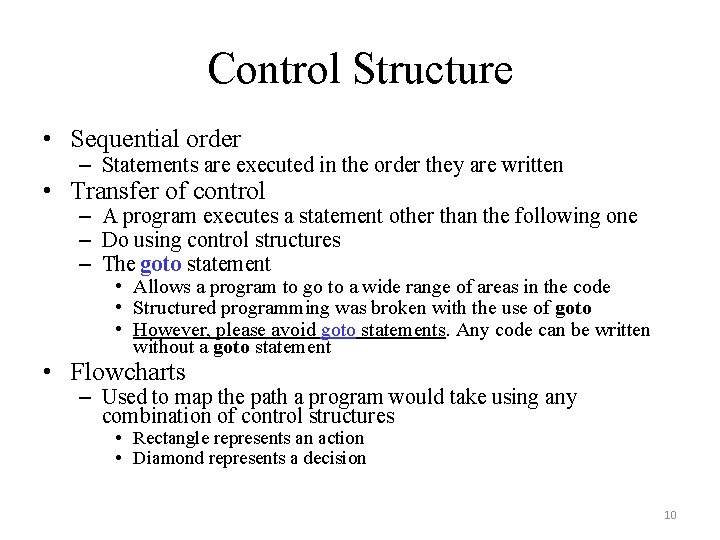
Control Structure • Sequential order – Statements are executed in the order they are written • Transfer of control – A program executes a statement other than the following one – Do using control structures – The goto statement • Allows a program to go to a wide range of areas in the code • Structured programming was broken with the use of goto • However, please avoid goto statements. Any code can be written without a goto statement • Flowcharts – Used to map the path a program would take using any combination of control structures • Rectangle represents an action • Diamond represents a decision 10
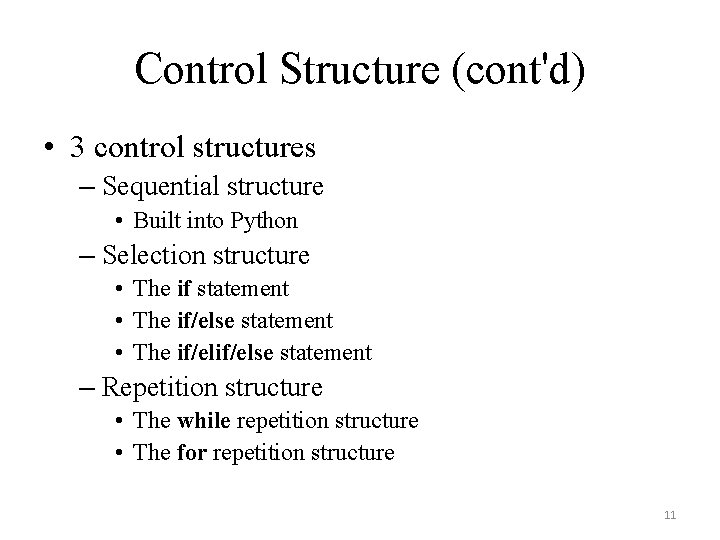
Control Structure (cont'd) • 3 control structures – Sequential structure • Built into Python – Selection structure • The if statement • The if/else statement – Repetition structure • The while repetition structure • The for repetition structure 11
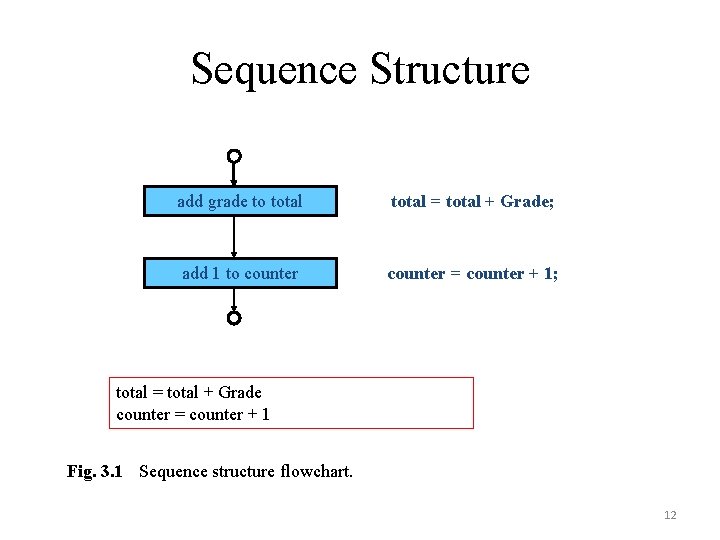
Sequence Structure add grade to total = total + Grade; add 1 to counter = counter + 1; total = total + Grade counter = counter + 1 Fig. 3. 1 Sequence structure flowchart. 12
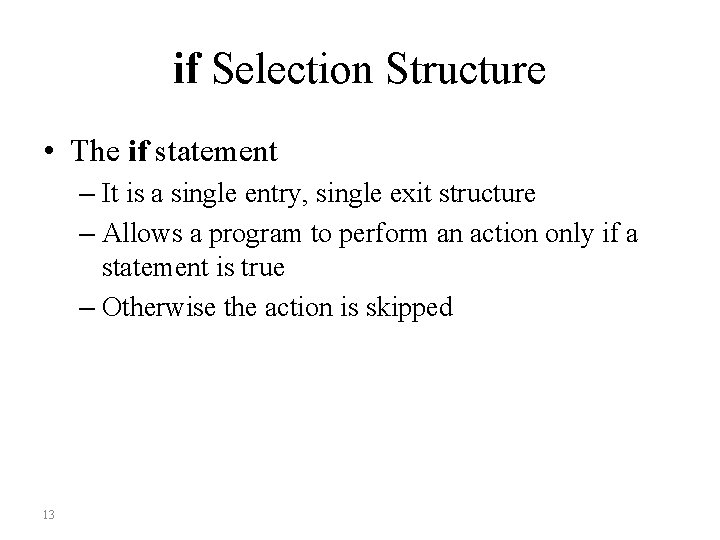
if Selection Structure • The if statement – It is a single entry, single exit structure – Allows a program to perform an action only if a statement is true – Otherwise the action is skipped 13
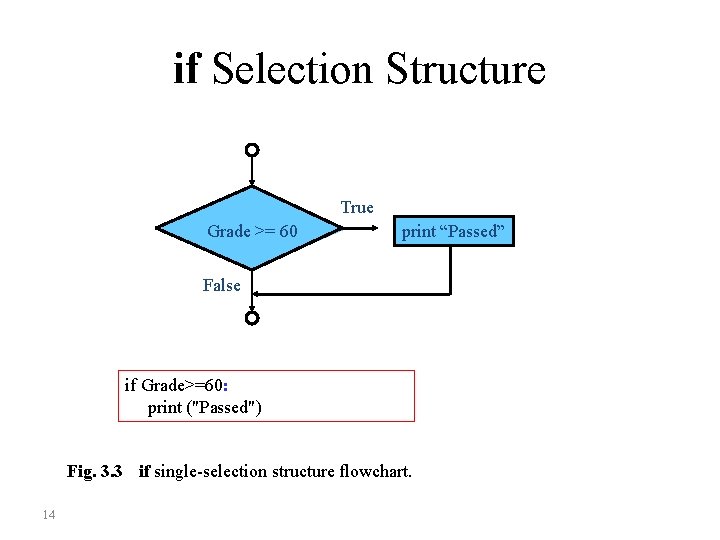
if Selection Structure True Grade >= 60 print “Passed” False if Grade>=60: print ("Passed") Fig. 3. 3 if single-selection structure flowchart. 14
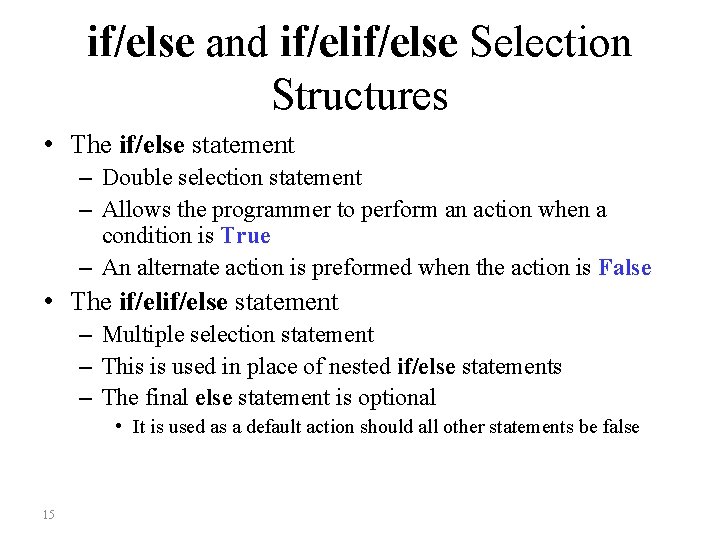
if/else and if/else Selection Structures • The if/else statement – Double selection statement – Allows the programmer to perform an action when a condition is True – An alternate action is preformed when the action is False • The if/else statement – Multiple selection statement – This is used in place of nested if/else statements – The final else statement is optional • It is used as a default action should all other statements be false 15
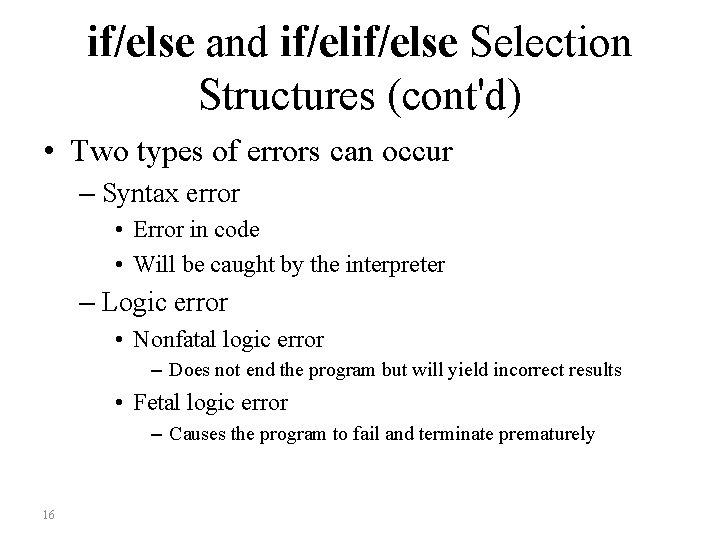
if/else and if/else Selection Structures (cont'd) • Two types of errors can occur – Syntax error • Error in code • Will be caught by the interpreter – Logic error • Nonfatal logic error – Does not end the program but will yield incorrect results • Fetal logic error – Causes the program to fail and terminate prematurely 16
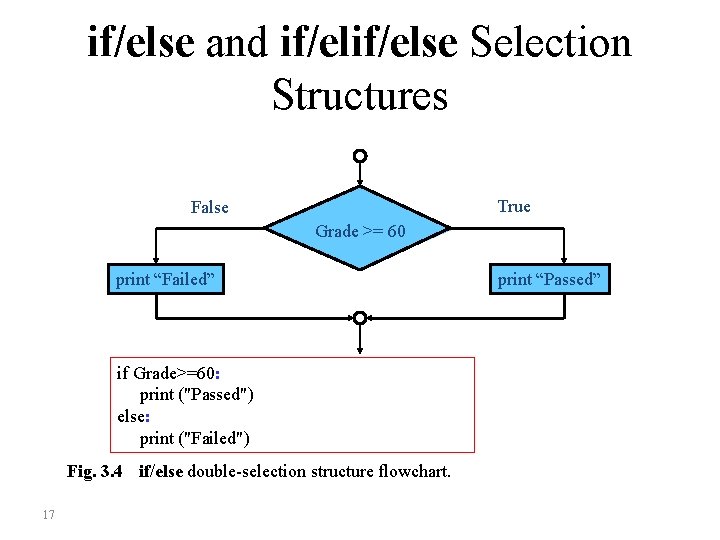
if/else and if/else Selection Structures True False Grade >= 60 print “Failed” if Grade>=60: print ("Passed") else: print ("Failed") Fig. 3. 4 if/else double-selection structure flowchart. 17 print “Passed”
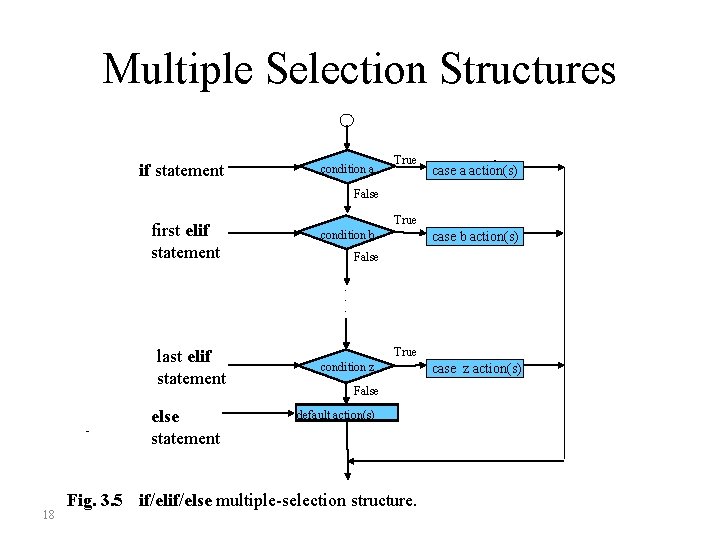
Multiple Selection Structures if statement condition a True case a action(s) False first elif statement True condition b case b action(s) False. . . last elif statement else statement 18 True condition z False default action(s) Fig. 3. 5 if/else multiple-selection structure. case z action(s)
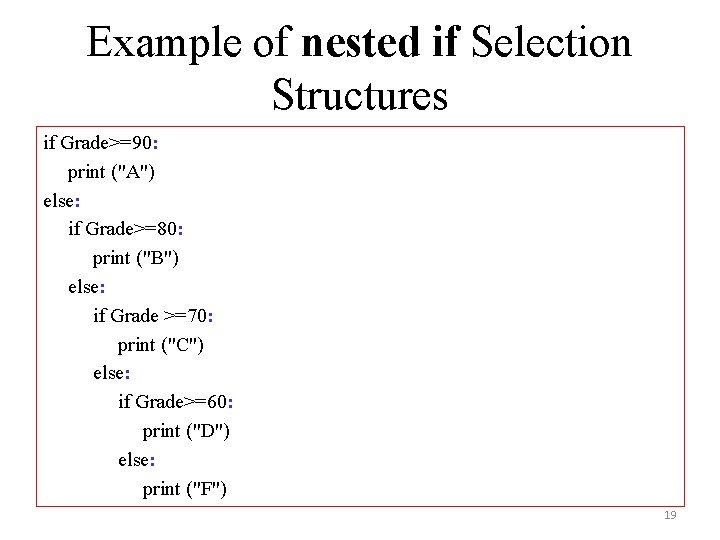
Example of nested if Selection Structures if Grade>=90: print ("A") else: if Grade>=80: print ("B") else: if Grade >=70: print ("C") else: if Grade>=60: print ("D") else: print ("F") 19
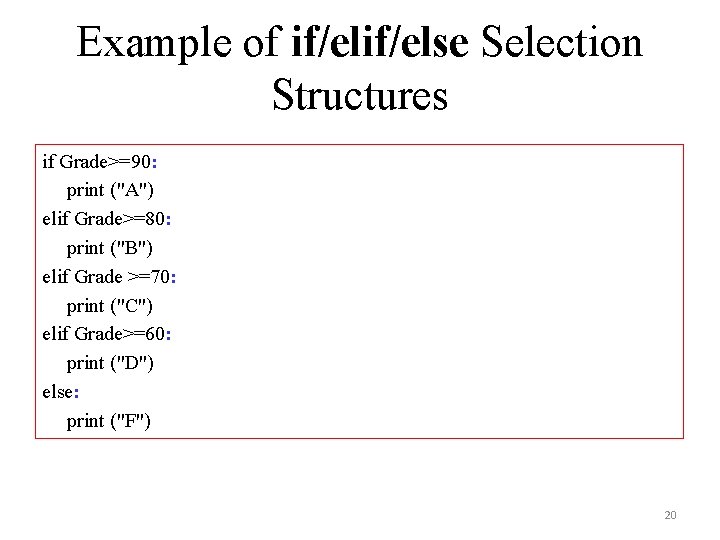
Example of if/else Selection Structures if Grade>=90: print ("A") elif Grade>=80: print ("B") elif Grade >=70: print ("C") elif Grade>=60: print ("D") else: print ("F") 20
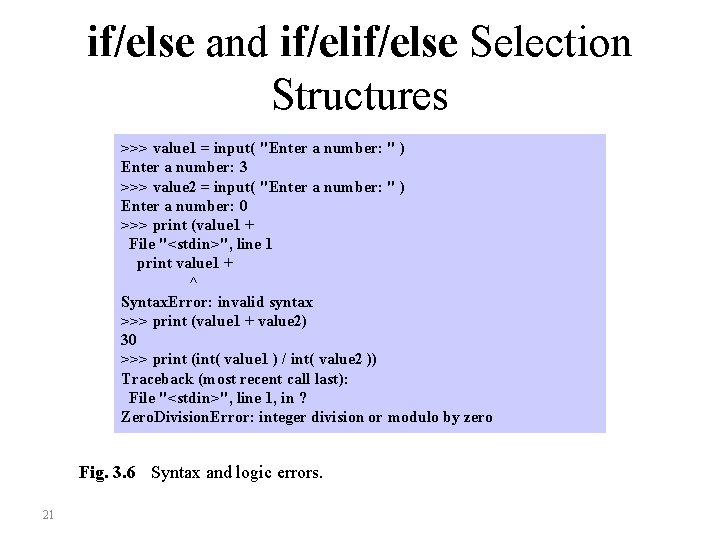
if/else and if/else Selection Structures >>> value 1 = input( "Enter a number: " ) Enter a number: 3 >>> value 2 = input( "Enter a number: " ) Enter a number: 0 >>> print (value 1 + File "<stdin>", line 1 print value 1 + ^ Syntax. Error: invalid syntax >>> print (value 1 + value 2) 30 >>> print (int( value 1 ) / int( value 2 )) Traceback (most recent call last): File "<stdin>", line 1, in ? Zero. Division. Error: integer division or modulo by zero Fig. 3. 6 Syntax and logic errors. 21
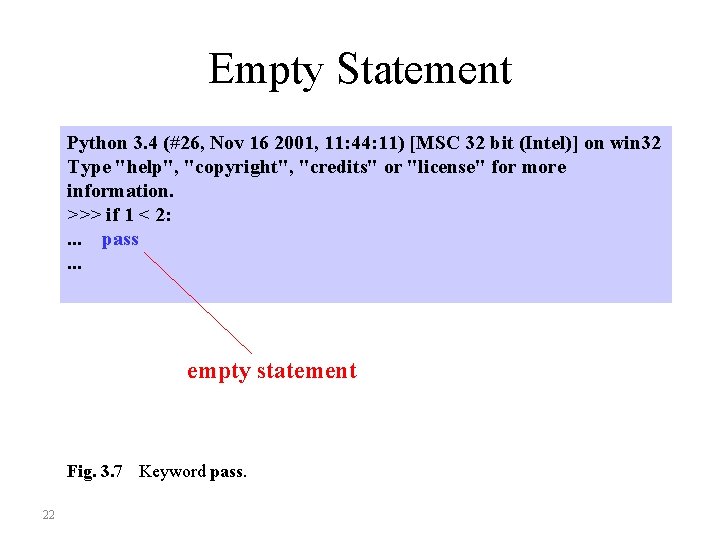
Empty Statement Python 3. 4 (#26, Nov 16 2001, 11: 44: 11) [MSC 32 bit (Intel)] on win 32 Type "help", "copyright", "credits" or "license" for more information. >>> if 1 < 2: . . . pass. . . empty statement Fig. 3. 7 Keyword pass. 22
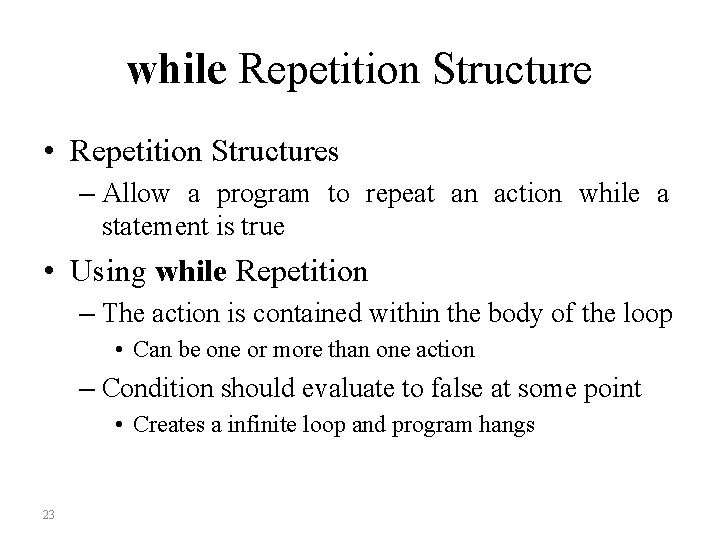
while Repetition Structure • Repetition Structures – Allow a program to repeat an action while a statement is true • Using while Repetition – The action is contained within the body of the loop • Can be one or more than one action – Condition should evaluate to false at some point • Creates a infinite loop and program hangs 23
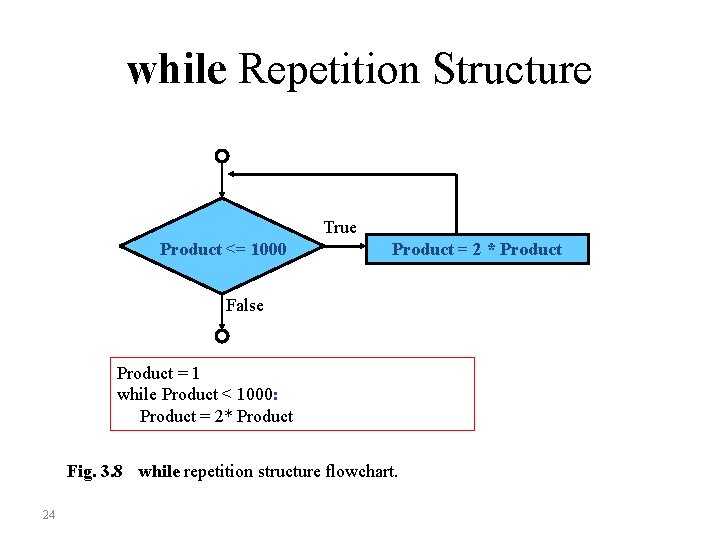
while Repetition Structure True Product <= 1000 Product = 2 * Product False Product = 1 while Product < 1000: Product = 2* Product Fig. 3. 8 while repetition structure flowchart. 24
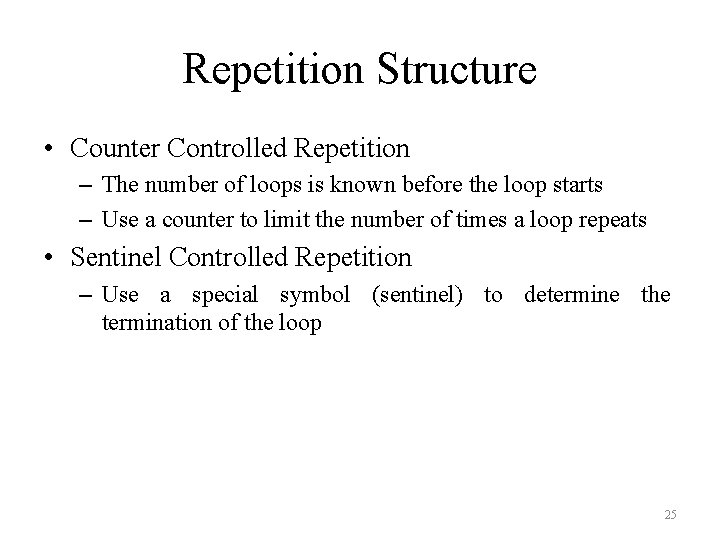
Repetition Structure • Counter Controlled Repetition – The number of loops is known before the loop starts – Use a counter to limit the number of times a loop repeats • Sentinel Controlled Repetition – Use a special symbol (sentinel) to determine the termination of the loop 25
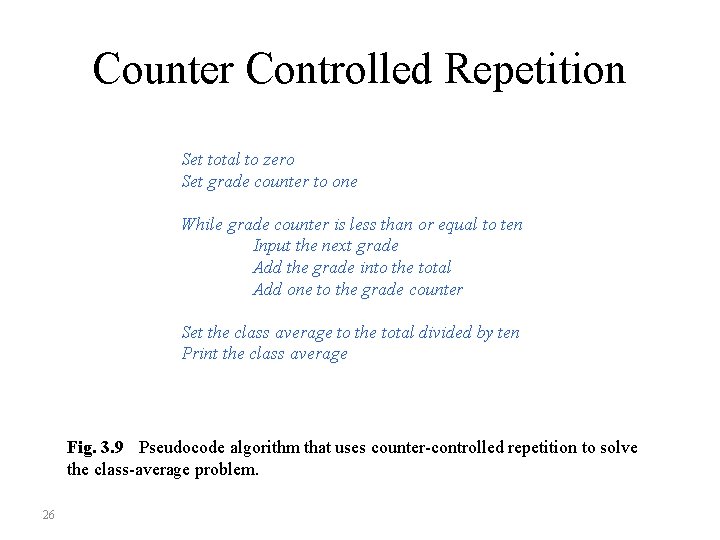
Counter Controlled Repetition Set total to zero Set grade counter to one While grade counter is less than or equal to ten Input the next grade Add the grade into the total Add one to the grade counter Set the class average to the total divided by ten Print the class average Fig. 3. 9 Pseudocode algorithm that uses counter-controlled repetition to solve the class-average problem. 26
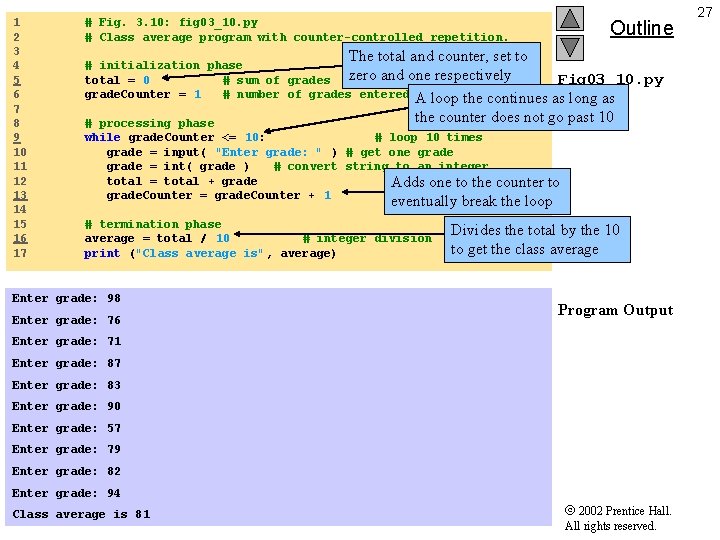
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 # Fig. 3. 10: fig 03_10. py # Class average program with counter-controlled repetition. Outline The total and counter, set to # initialization phase zero and one respectively total = 0 # sum of grades grade. Counter = 1 # number of grades entered A loop the continues Fig 03_10. py as long as the counter does not go past 10 # processing phase while grade. Counter <= 10: # loop 10 times grade = input( "Enter grade: " ) # get one grade = int( grade ) # convert string to an integer total = total + grade Adds one to the grade. Counter = grade. Counter + 1 counter to eventually break the loop # termination phase average = total / 10 # integer division print ("Class average is", average) Enter grade: 98 Enter grade: 76 Divides the total by the 10 to get the class average Program Output Enter grade: 71 Enter grade: 87 Enter grade: 83 Enter grade: 90 Enter grade: 57 Enter grade: 79 Enter grade: 82 Enter grade: 94 Class average is 81 2002 Prentice Hall. All rights reserved. 27
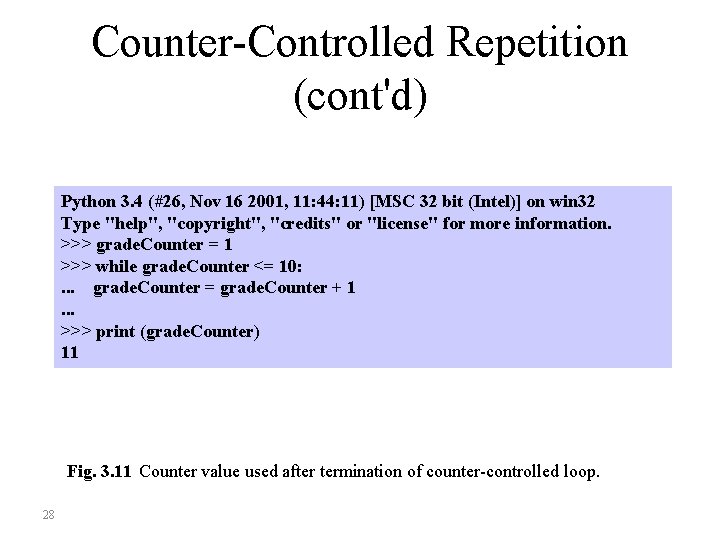
Counter-Controlled Repetition (cont'd) Python 3. 4 (#26, Nov 16 2001, 11: 44: 11) [MSC 32 bit (Intel)] on win 32 Type "help", "copyright", "credits" or "license" for more information. >>> grade. Counter = 1 >>> while grade. Counter <= 10: . . . grade. Counter = grade. Counter + 1. . . >>> print (grade. Counter) 11 Fig. 3. 11 Counter value used after termination of counter-controlled loop. 28
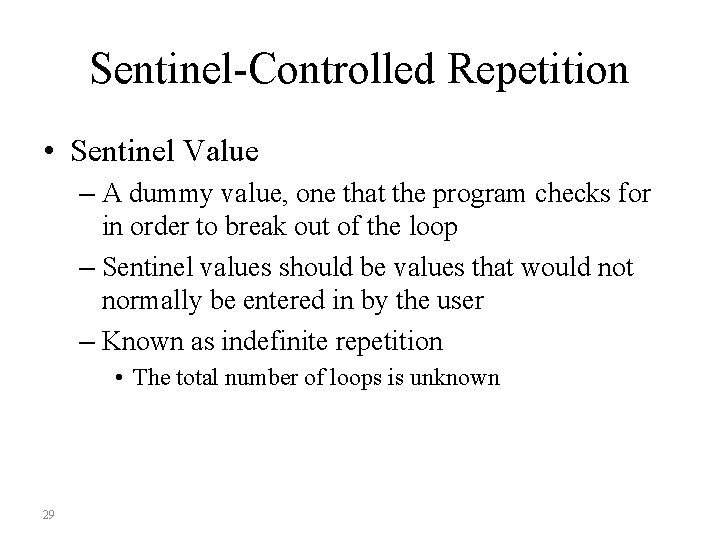
Sentinel-Controlled Repetition • Sentinel Value – A dummy value, one that the program checks for in order to break out of the loop – Sentinel values should be values that would not normally be entered in by the user – Known as indefinite repetition • The total number of loops is unknown 29
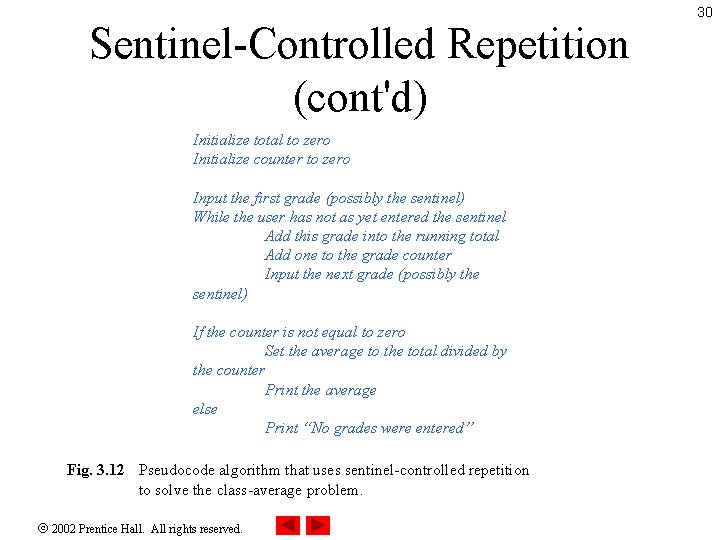
Sentinel-Controlled Repetition (cont'd) Initialize total to zero Initialize counter to zero Input the first grade (possibly the sentinel) While the user has not as yet entered the sentinel Add this grade into the running total Add one to the grade counter Input the next grade (possibly the sentinel) If the counter is not equal to zero Set the average to the total divided by the counter Print the average else Print “No grades were entered” Fig. 3. 12 Pseudocode algorithm that uses sentinel-controlled repetition to solve the class-average problem. 2002 Prentice Hall. All rights reserved. 30
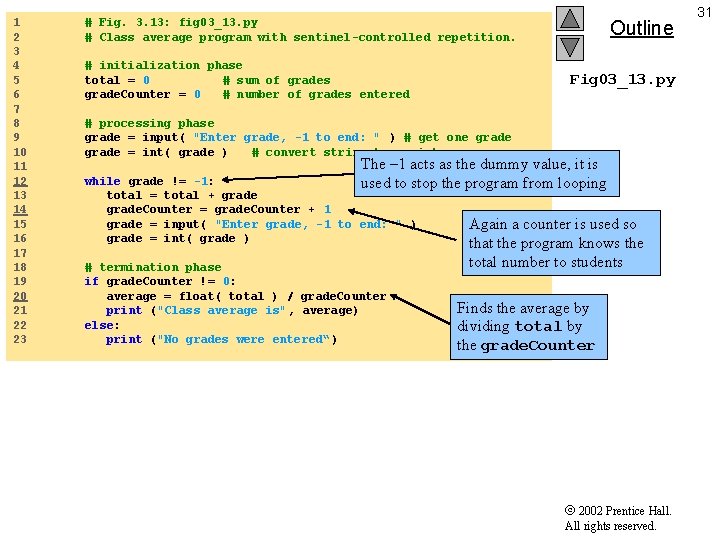
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 # Fig. 3. 13: fig 03_13. py # Class average program with sentinel-controlled repetition. # initialization phase total = 0 # sum of grades grade. Counter = 0 # number of grades entered Outline Fig 03_13. py # processing phase grade = input( "Enter grade, -1 to end: " ) # get one grade = int( grade ) # convert string to an integer The – 1 acts as the dummy value, it is used to stop the program from looping while grade != -1: total = total + grade. Counter = grade. Counter + 1 grade = input( "Enter grade, -1 to end: " ) grade = int( grade ) # termination phase if grade. Counter != 0: average = float( total ) / grade. Counter print ("Class average is", average) else: print ("No grades were entered“ ) Again a counter is used so that the program knows the total number to students Finds the average by dividing total by the grade. Counter 2002 Prentice Hall. All rights reserved. 31
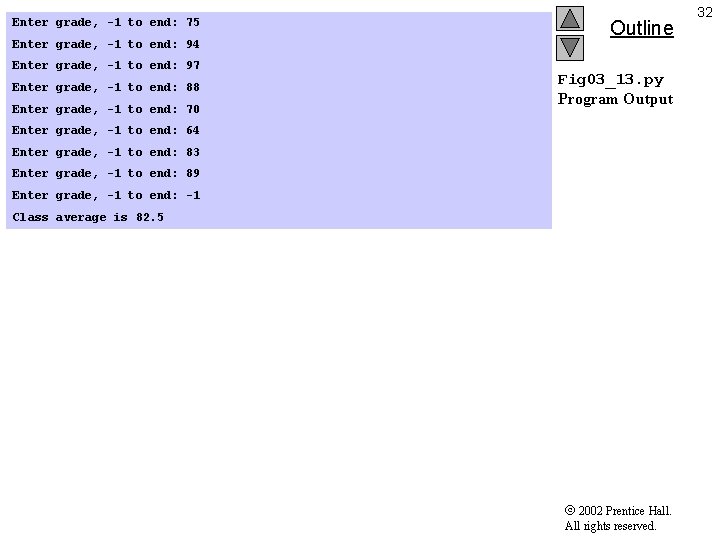
Enter grade, -1 to end: 75 Enter grade, -1 to end: 94 Enter grade, -1 to end: 97 Enter grade, -1 to end: 88 Enter grade, -1 to end: 70 Outline Fig 03_13. py Program Output Enter grade, -1 to end: 64 Enter grade, -1 to end: 83 Enter grade, -1 to end: 89 Enter grade, -1 to end: -1 Class average is 82. 5 2002 Prentice Hall. All rights reserved. 32
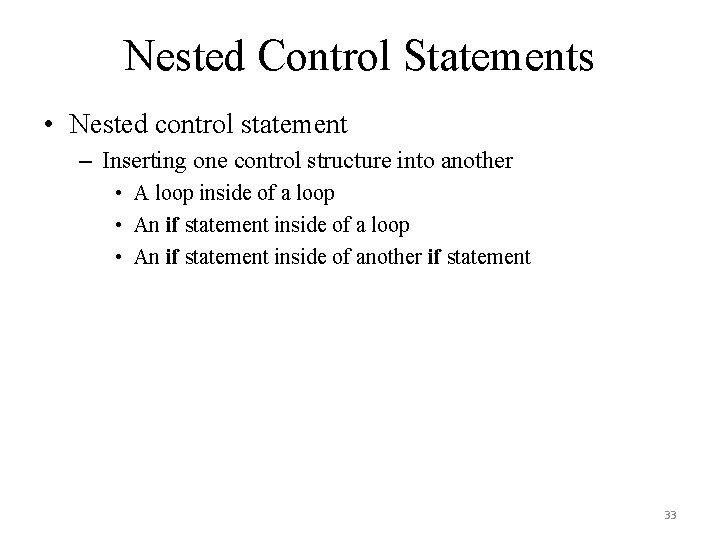
Nested Control Statements • Nested control statement – Inserting one control structure into another • A loop inside of a loop • An if statement inside of another if statement 33
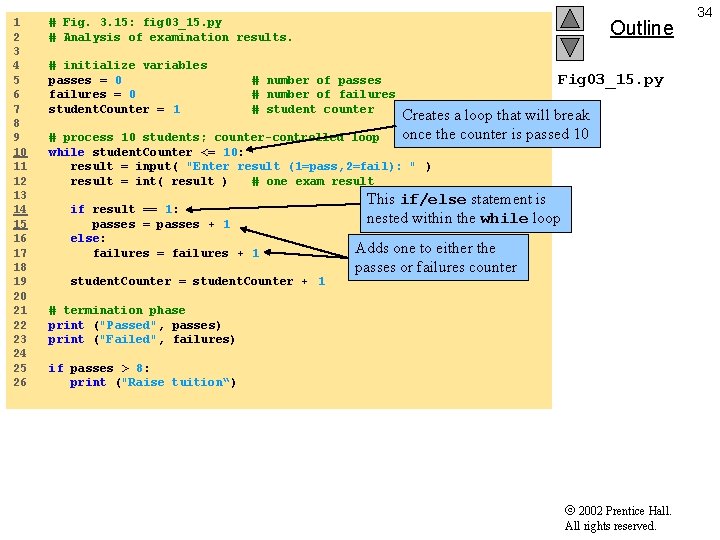
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 # Fig. 3. 15: fig 03_15. py # Analysis of examination results. # initialize variables passes = 0 failures = 0 student. Counter = 1 Outline # number of passes # number of failures # student counter Fig 03_15. py Creates a loop that will break once the counter is passed 10 # process 10 students; counter-controlled loop while student. Counter <= 10: result = input( "Enter result (1=pass, 2=fail): " ) result = int( result ) # one exam result if result == 1: passes = passes + 1 else: failures = failures + 1 student. Counter = student. Counter + 1 This if/else statement is nested within the while loop Adds one to either the passes or failures counter # termination phase print ("Passed", passes) print ("Failed", failures) if passes > 8: print ("Raise tuition“) 2002 Prentice Hall. All rights reserved. 34
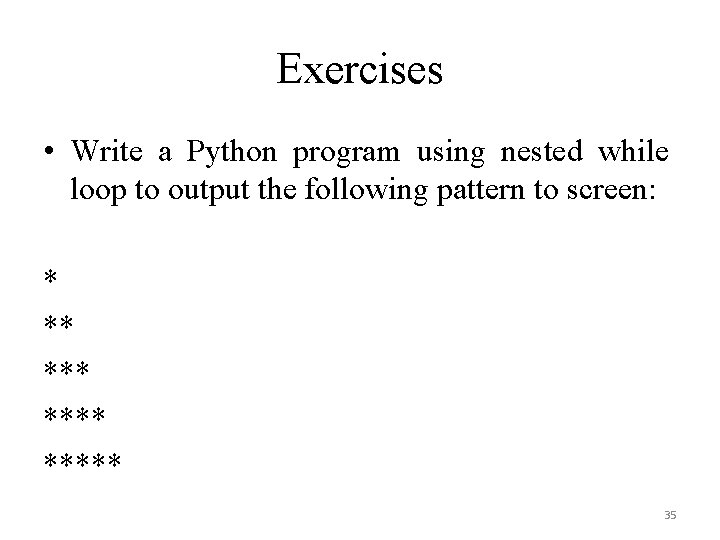
Exercises • Write a Python program using nested while loop to output the following pattern to screen: * ** ***** 35
Python chapter 5 programming exercises
Concluding sentence
Narrowing down a topic exercises
Python procedural language
Second order cone programming python
Python programming in context
Constraint programming python
Rapid gui programming with python and qt
Python programming in context
Python cgi programming examples
Audiolab python
Python programming
Programming essentials in python
Python blackjack oop
Python programming an introduction to computer science
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
System programming definition
Linear vs integer programming
Definisi integer
Chapter 5 selecting a topic and a purpose
Specific purpose statements
What is non fruitful function in python
Chapter 3 python for everybody
Py4e chapter 5
Decode the words
Cj-zj meaning
Logical programming language
Chapter 16 programming language
Four special cases in linear programming
Computer programming chapter 1
Chapter 1 introduction to computers and programming
Chapter 1 introduction to computers and programming
History of python
Chapter 8 linear programming applications solutions
Chapter 2 elementary programming