CSCE 330 Programming Language Structures Chapter 4 Names
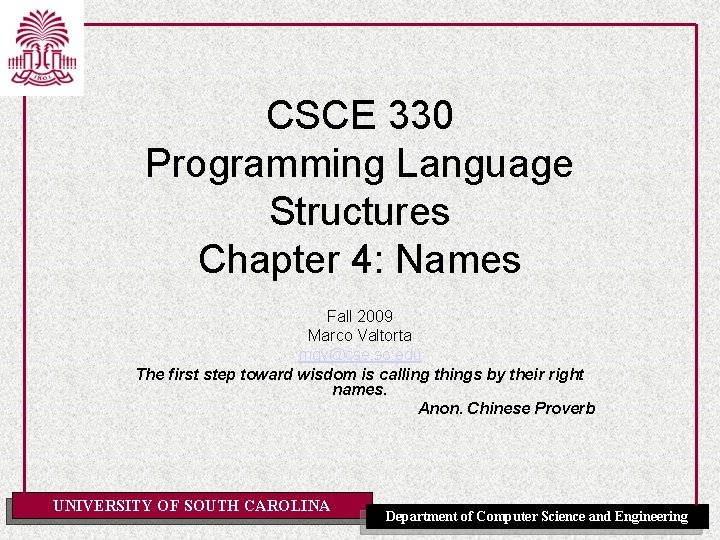
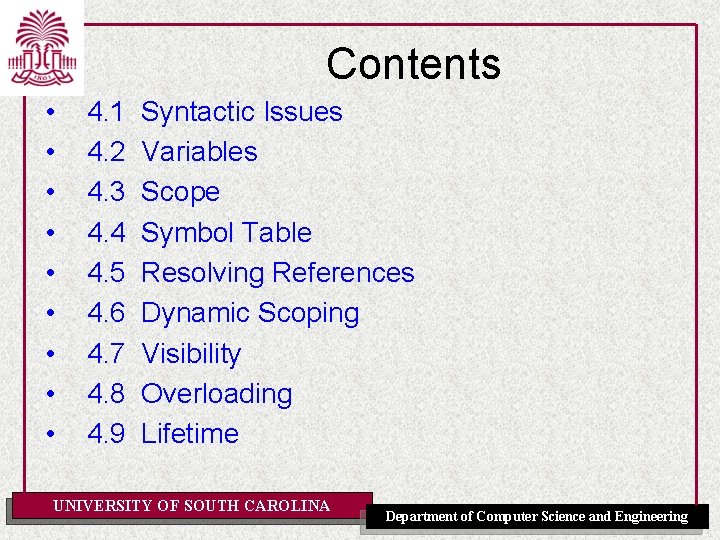
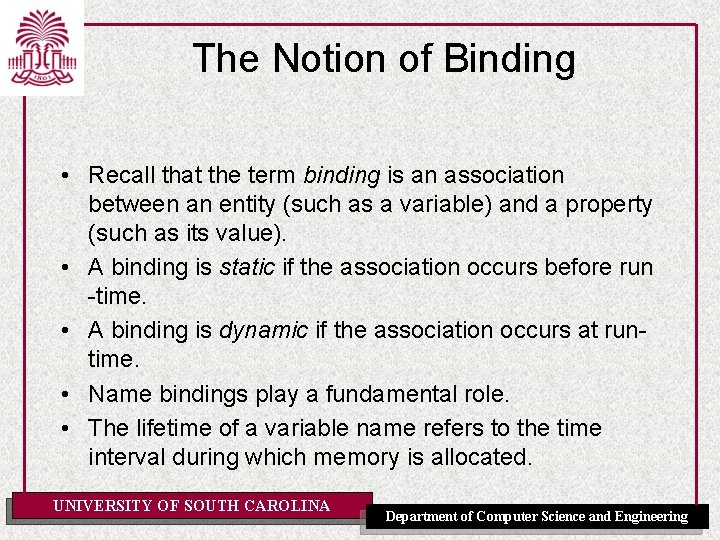
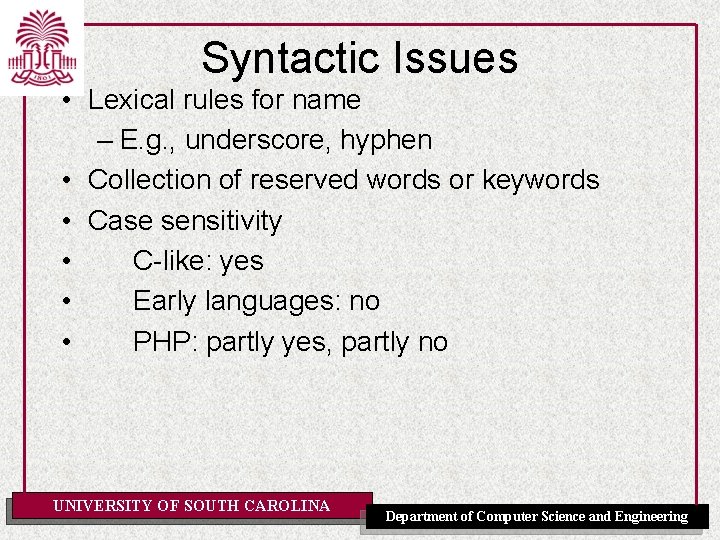
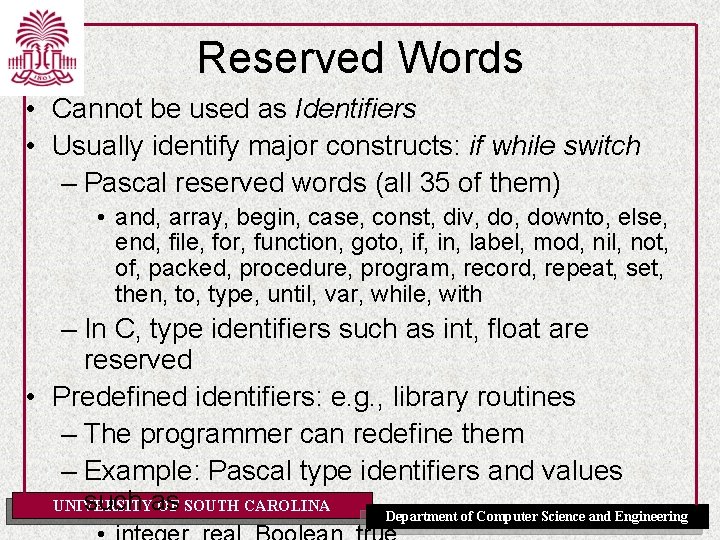
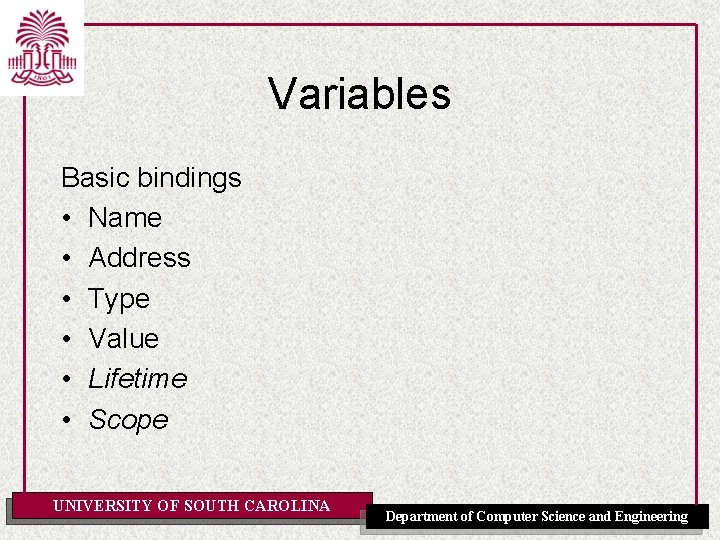
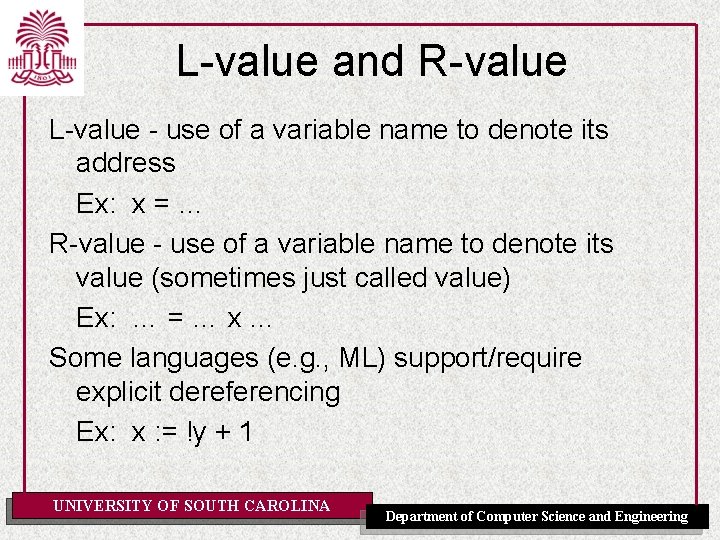
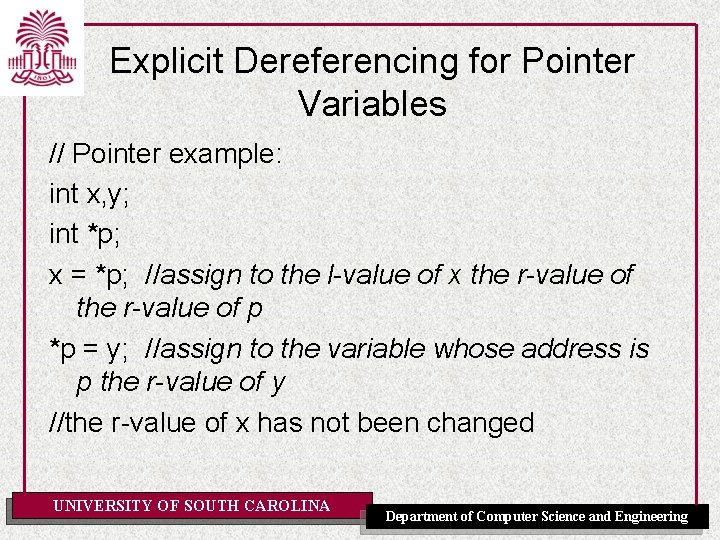
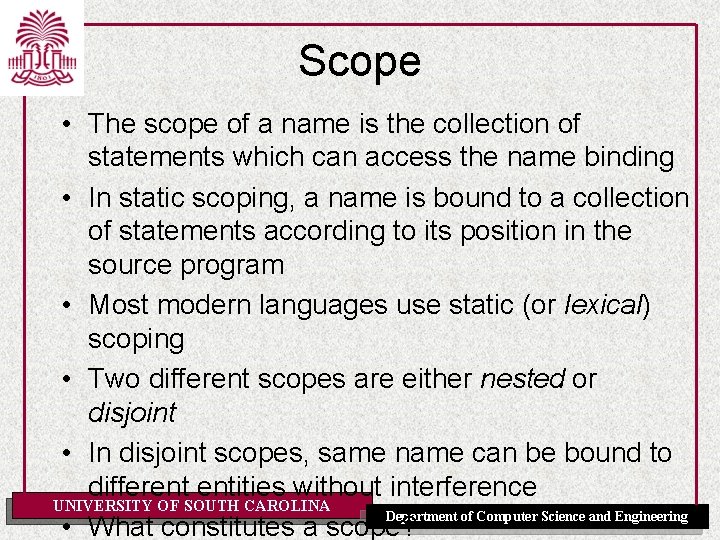
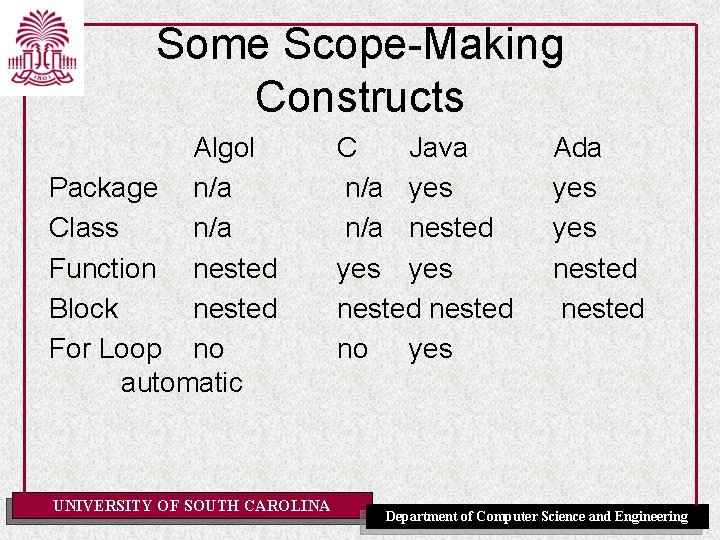
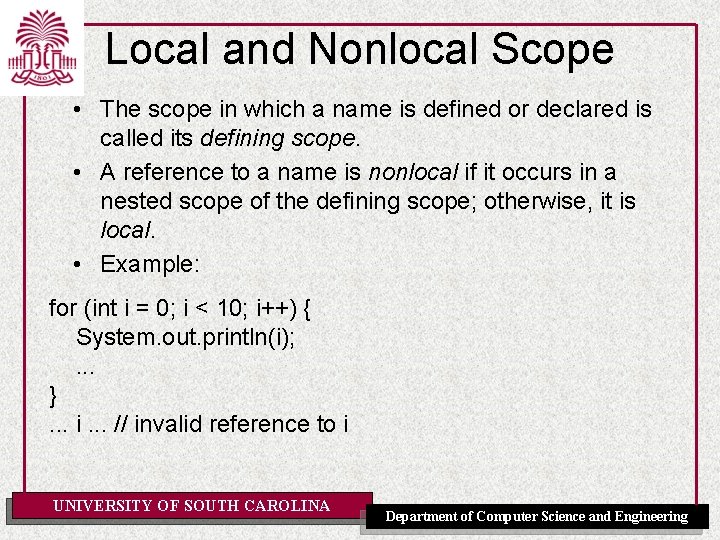
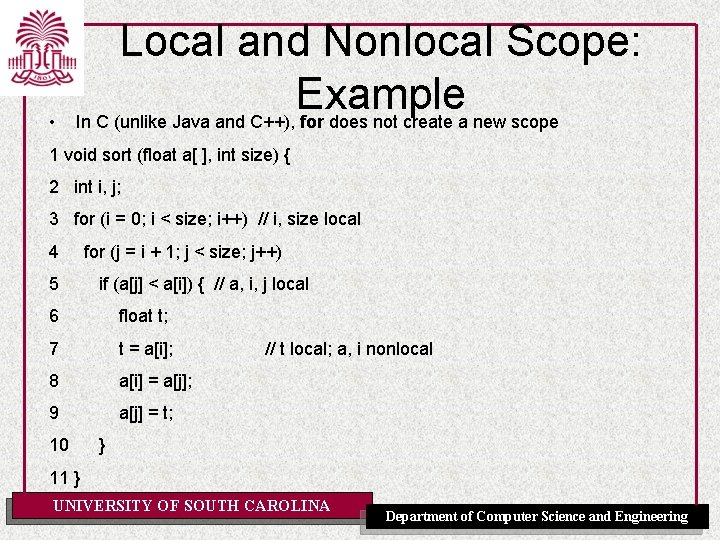
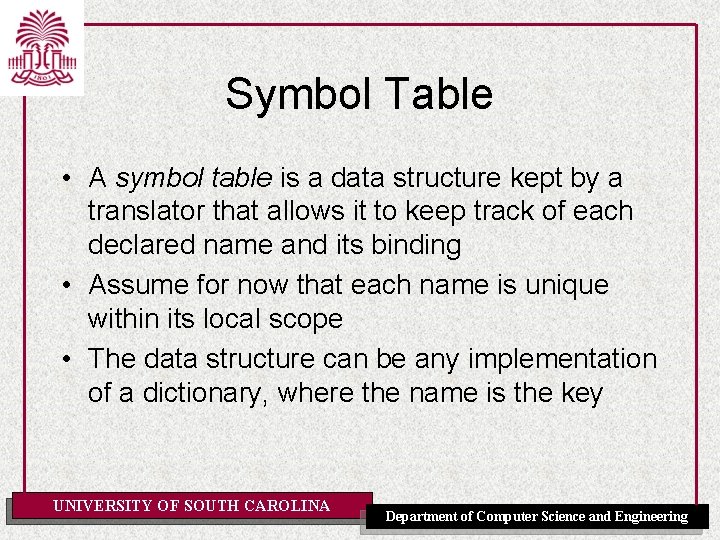
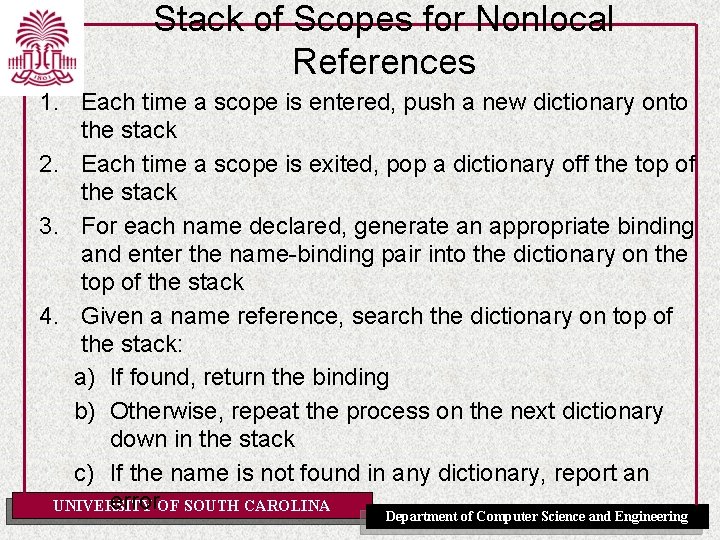
![Stack of Scope Dictionaries Example C program in Fig. 4. 1 [T], stack of Stack of Scope Dictionaries Example C program in Fig. 4. 1 [T], stack of](https://slidetodoc.com/presentation_image_h2/12a7edbc42625a0032125983929e2b65/image-15.jpg)
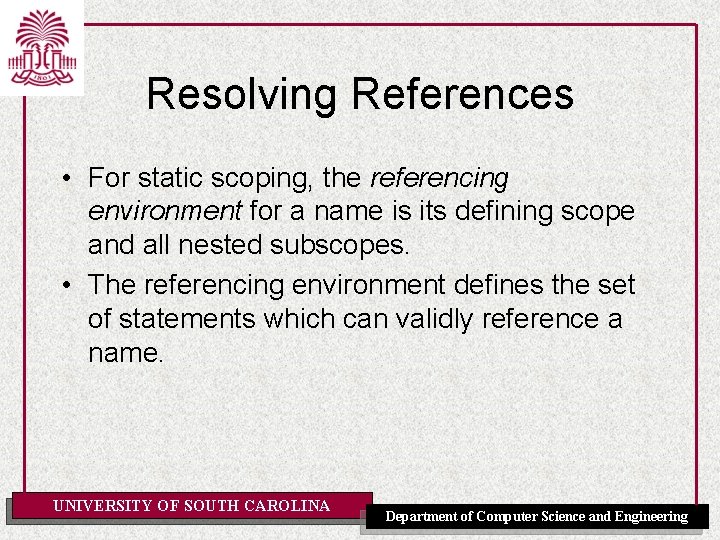
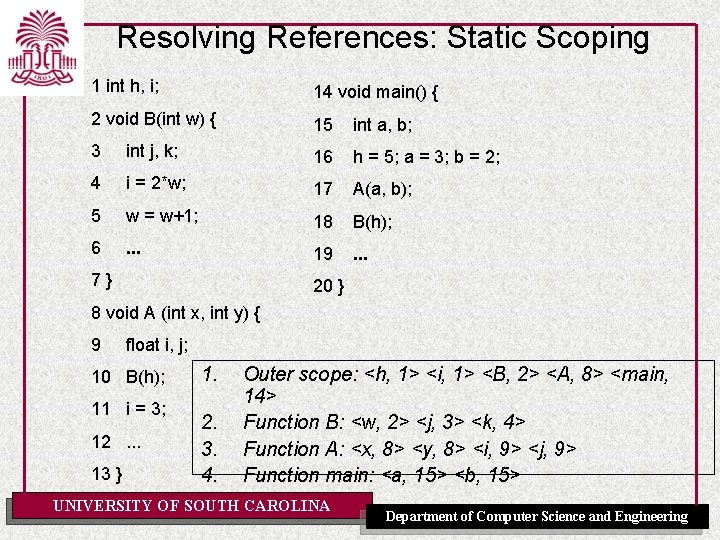
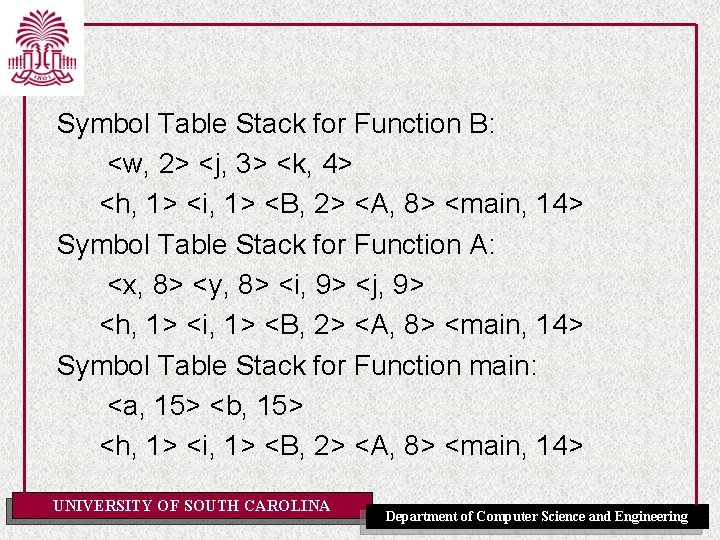
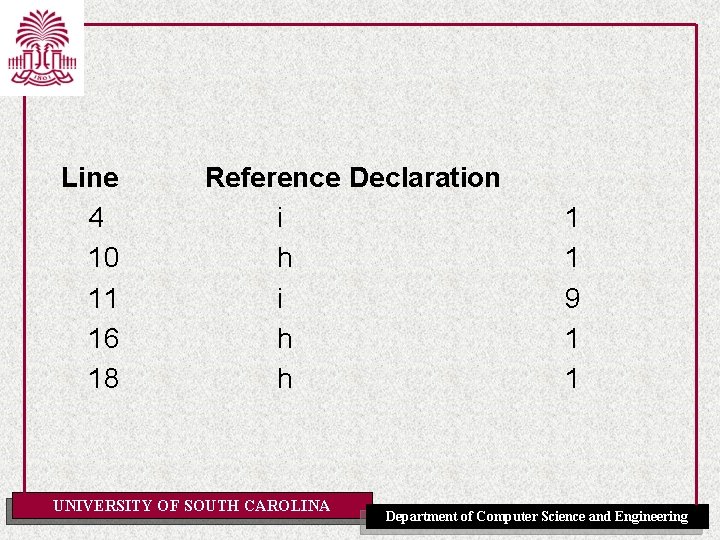
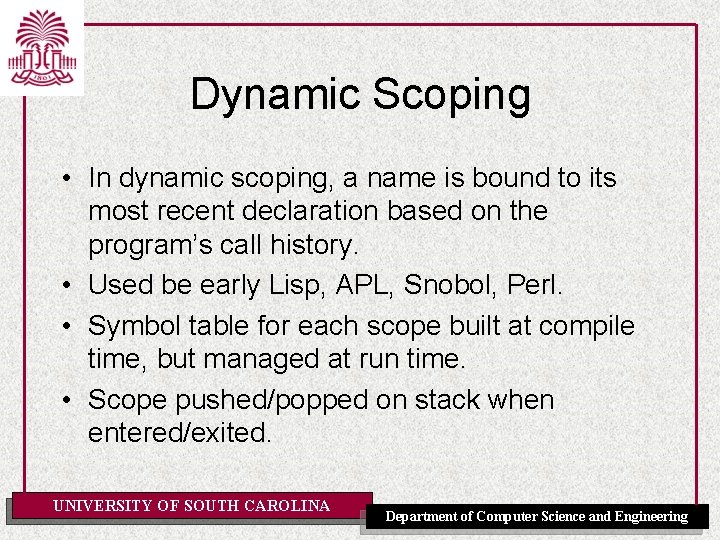
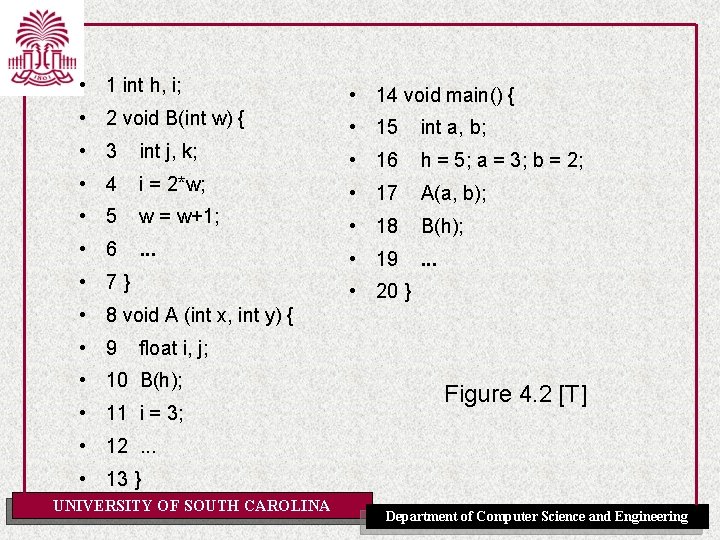
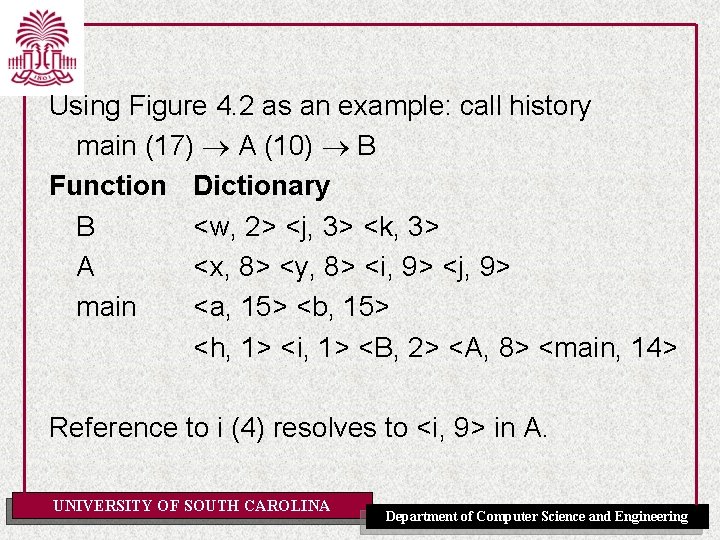
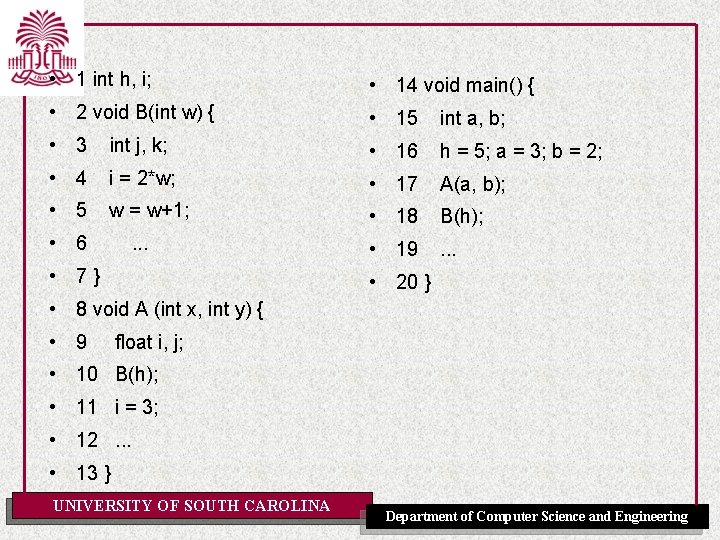
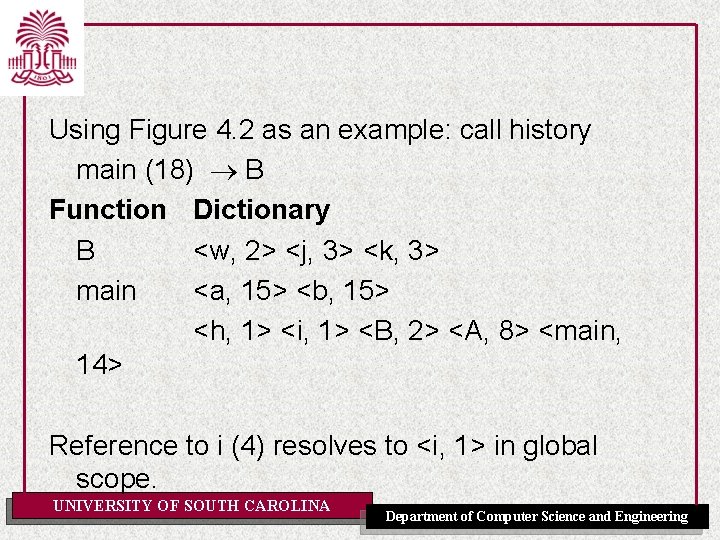
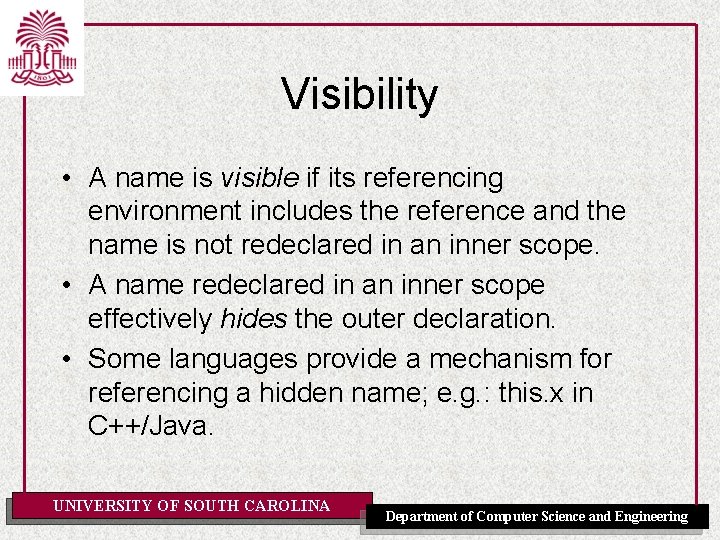
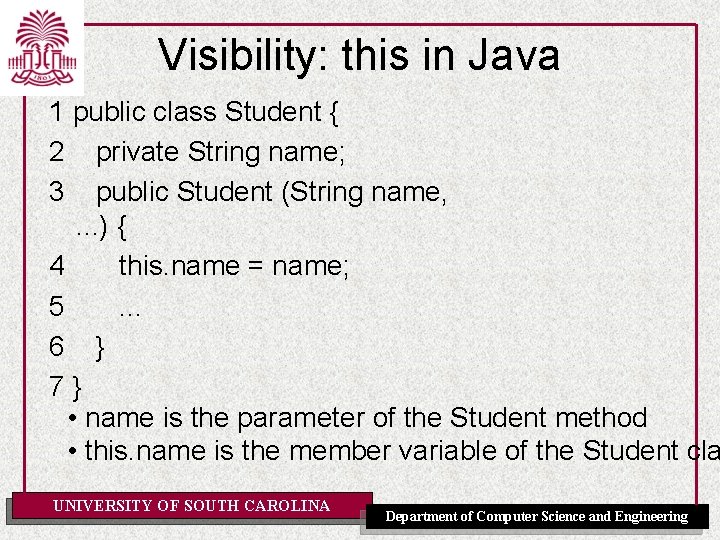
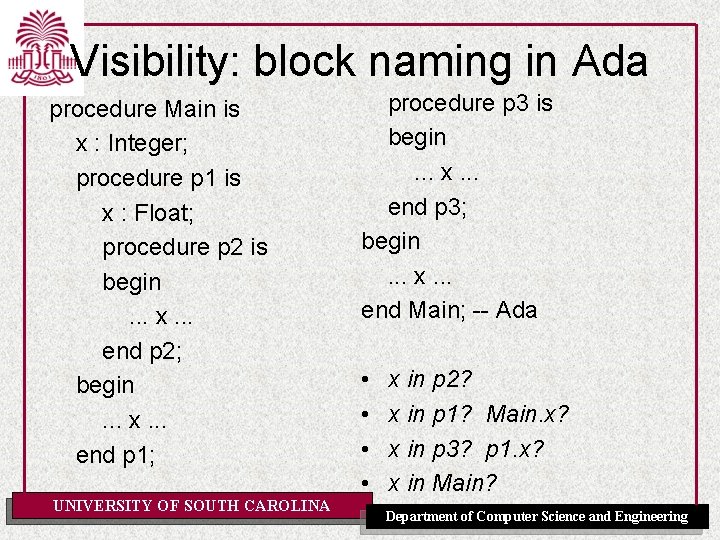
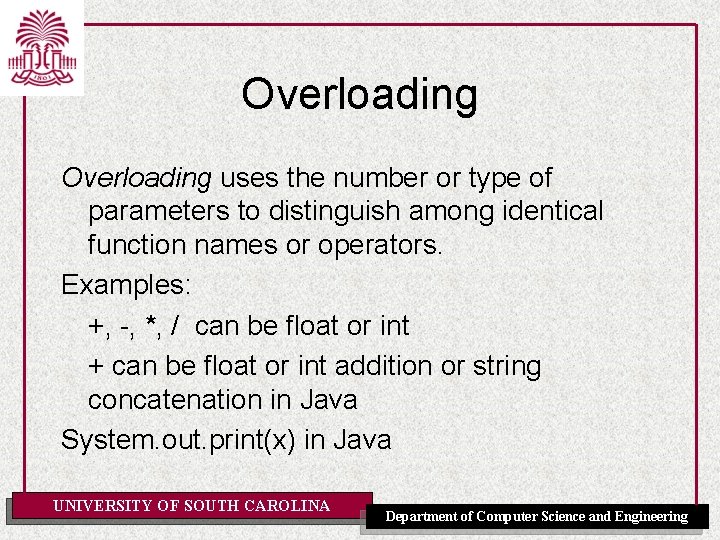
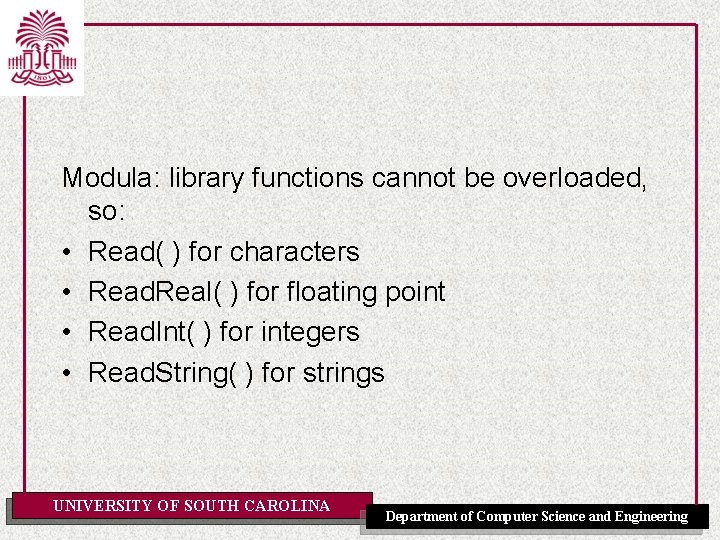
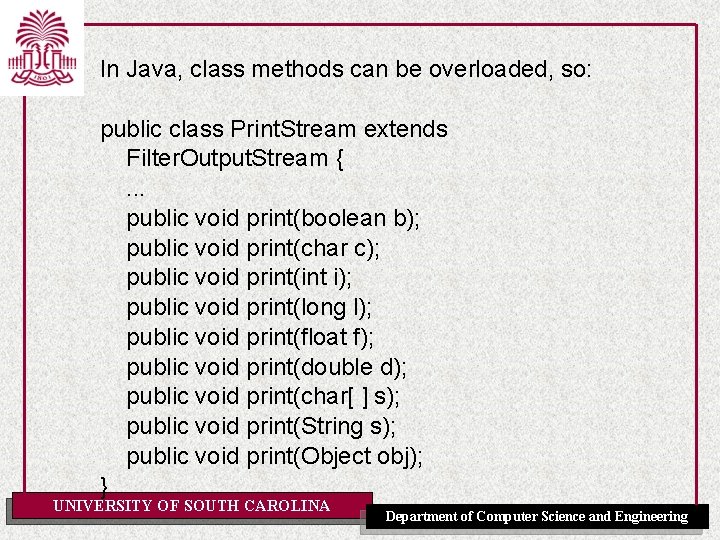
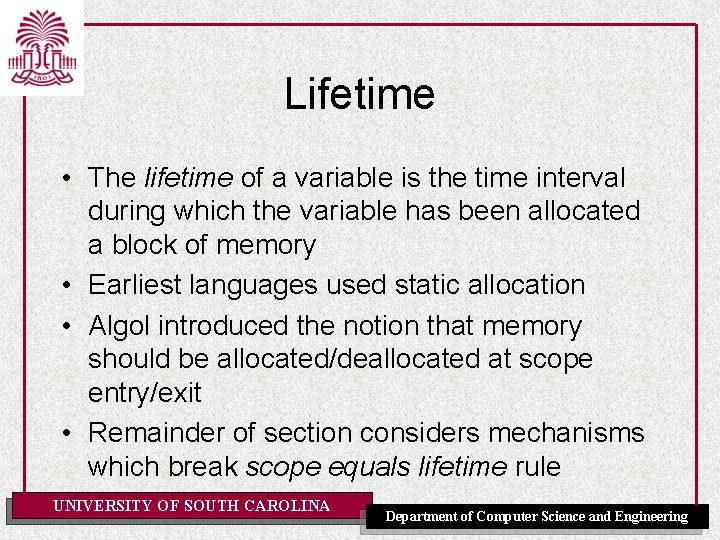
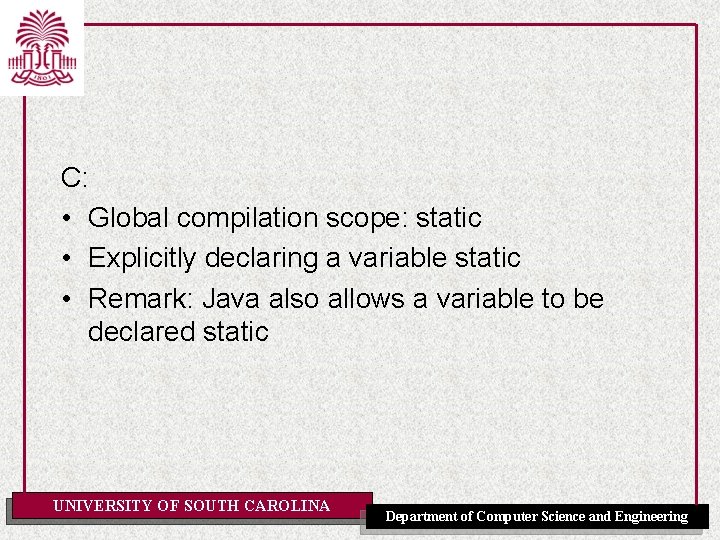
- Slides: 32
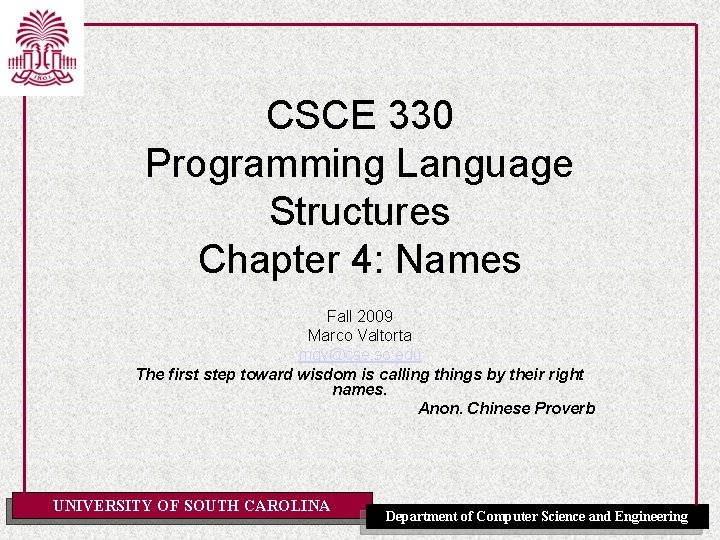
CSCE 330 Programming Language Structures Chapter 4: Names Fall 2009 Marco Valtorta mgv@cse. sc. edu The first step toward wisdom is calling things by their right names. Anon. Chinese Proverb UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
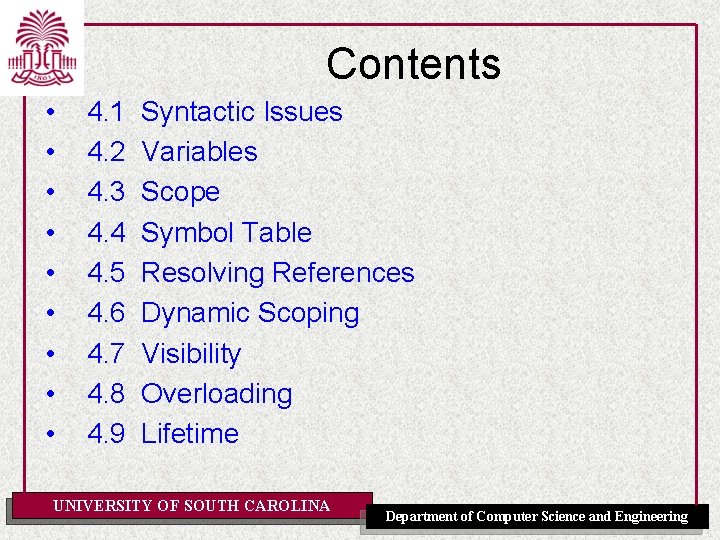
Contents • • • 4. 1 4. 2 4. 3 4. 4 4. 5 4. 6 4. 7 4. 8 4. 9 Syntactic Issues Variables Scope Symbol Table Resolving References Dynamic Scoping Visibility Overloading Lifetime UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
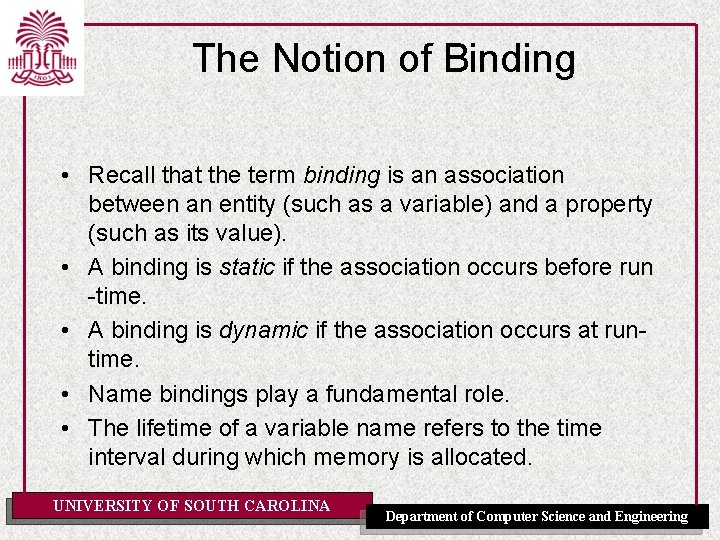
The Notion of Binding • Recall that the term binding is an association between an entity (such as a variable) and a property (such as its value). • A binding is static if the association occurs before run -time. • A binding is dynamic if the association occurs at runtime. • Name bindings play a fundamental role. • The lifetime of a variable name refers to the time interval during which memory is allocated. UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
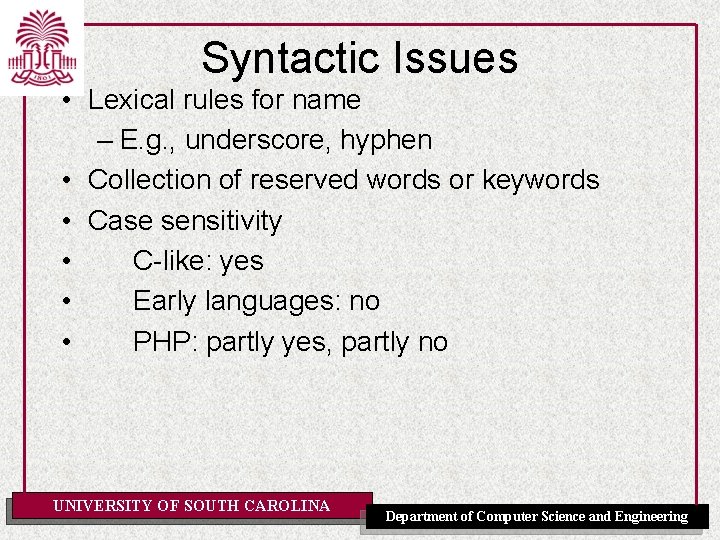
Syntactic Issues • Lexical rules for name – E. g. , underscore, hyphen • Collection of reserved words or keywords • Case sensitivity • C-like: yes • Early languages: no • PHP: partly yes, partly no UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
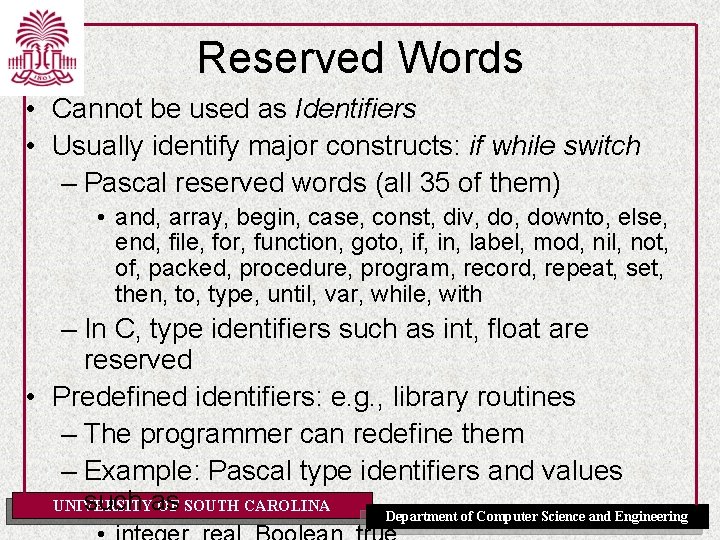
Reserved Words • Cannot be used as Identifiers • Usually identify major constructs: if while switch – Pascal reserved words (all 35 of them) • and, array, begin, case, const, div, downto, else, end, file, for, function, goto, if, in, label, mod, nil, not, of, packed, procedure, program, record, repeat, set, then, to, type, until, var, while, with – In C, type identifiers such as int, float are reserved • Predefined identifiers: e. g. , library routines – The programmer can redefine them – Example: Pascal type identifiers and values such as UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
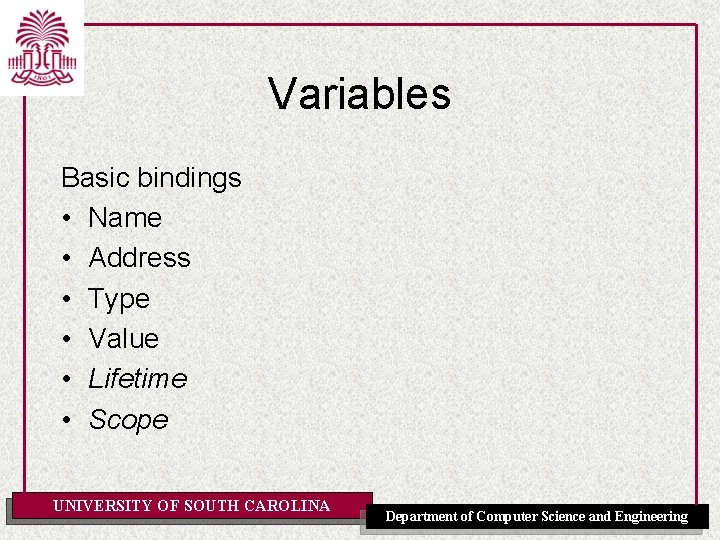
Variables Basic bindings • Name • Address • Type • Value • Lifetime • Scope UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
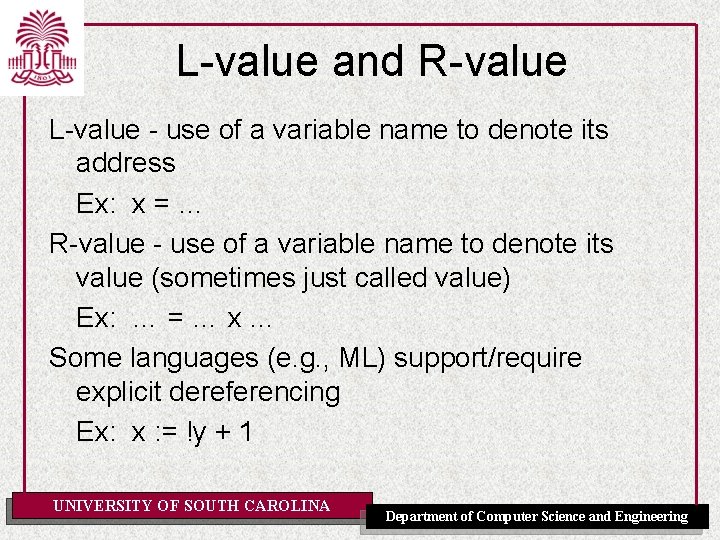
L-value and R-value L-value - use of a variable name to denote its address Ex: x = … R-value - use of a variable name to denote its value (sometimes just called value) Ex: … = … x … Some languages (e. g. , ML) support/require explicit dereferencing Ex: x : = !y + 1 UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
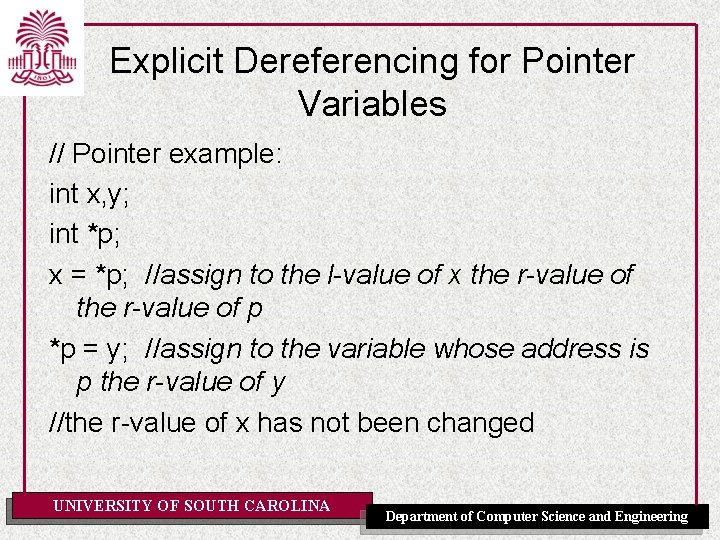
Explicit Dereferencing for Pointer Variables // Pointer example: int x, y; int *p; x = *p; //assign to the l-value of x the r-value of p *p = y; //assign to the variable whose address is p the r-value of y //the r-value of x has not been changed UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
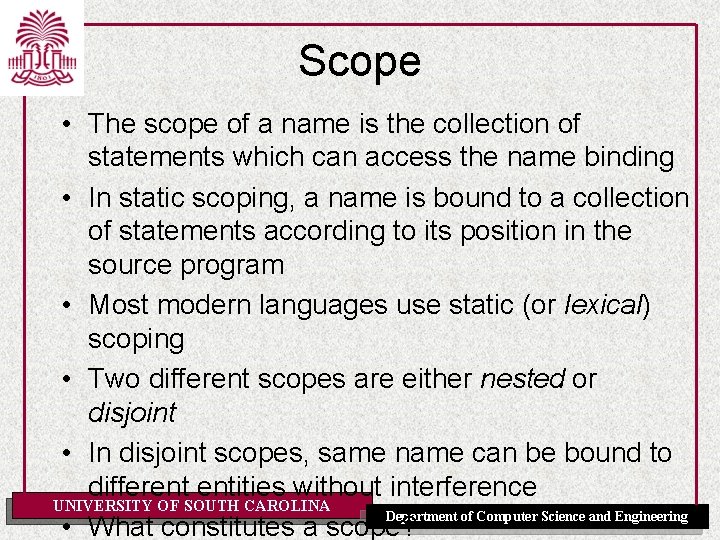
Scope • The scope of a name is the collection of statements which can access the name binding • In static scoping, a name is bound to a collection of statements according to its position in the source program • Most modern languages use static (or lexical) scoping • Two different scopes are either nested or disjoint • In disjoint scopes, same name can be bound to different entities without interference UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering • What constitutes a scope?
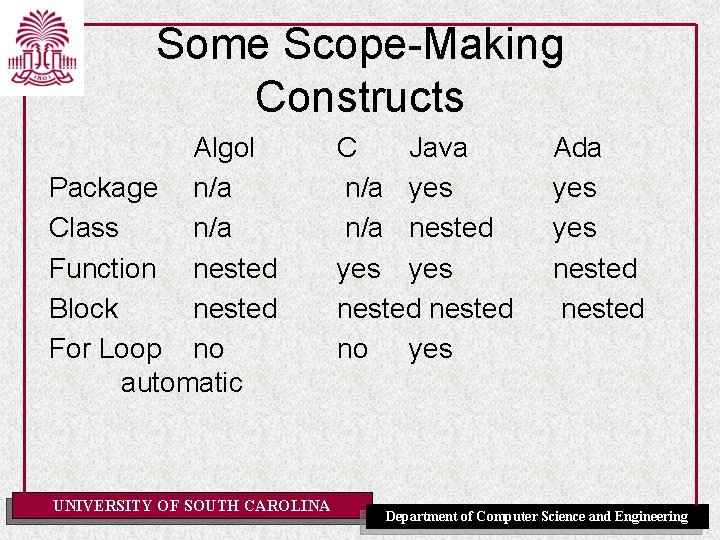
Some Scope-Making Constructs Algol Package n/a Class n/a Function nested Block nested For Loop no automatic UNIVERSITY OF SOUTH CAROLINA C Java n/a yes n/a nested yes nested no yes Ada yes nested Department of Computer Science and Engineering
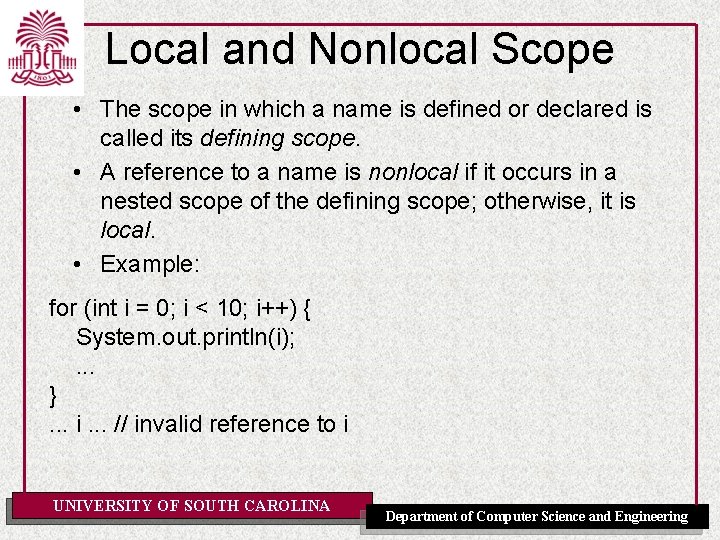
Local and Nonlocal Scope • The scope in which a name is defined or declared is called its defining scope. • A reference to a name is nonlocal if it occurs in a nested scope of the defining scope; otherwise, it is local. • Example: for (int i = 0; i < 10; i++) { System. out. println(i); . . . }. . . i. . . // invalid reference to i UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
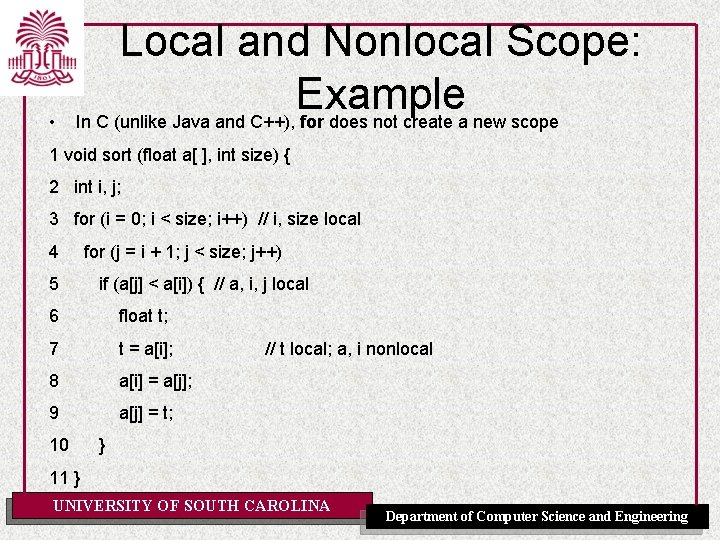
• Local and Nonlocal Scope: Example In C (unlike Java and C++), for does not create a new scope 1 void sort (float a[ ], int size) { 2 int i, j; 3 for (i = 0; i < size; i++) // i, size local 4 5 for (j = i + 1; j < size; j++) if (a[j] < a[i]) { // a, i, j local 6 float t; 7 t = a[i]; 8 a[i] = a[j]; 9 a[j] = t; 10 // t local; a, i nonlocal } 11 } UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
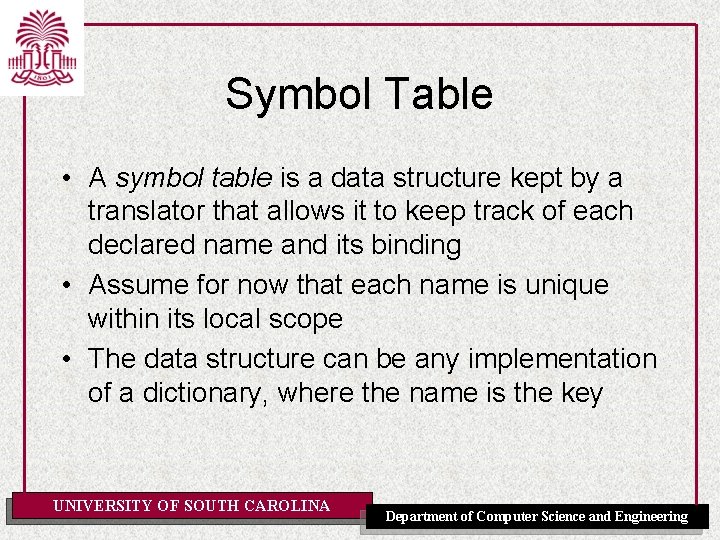
Symbol Table • A symbol table is a data structure kept by a translator that allows it to keep track of each declared name and its binding • Assume for now that each name is unique within its local scope • The data structure can be any implementation of a dictionary, where the name is the key UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
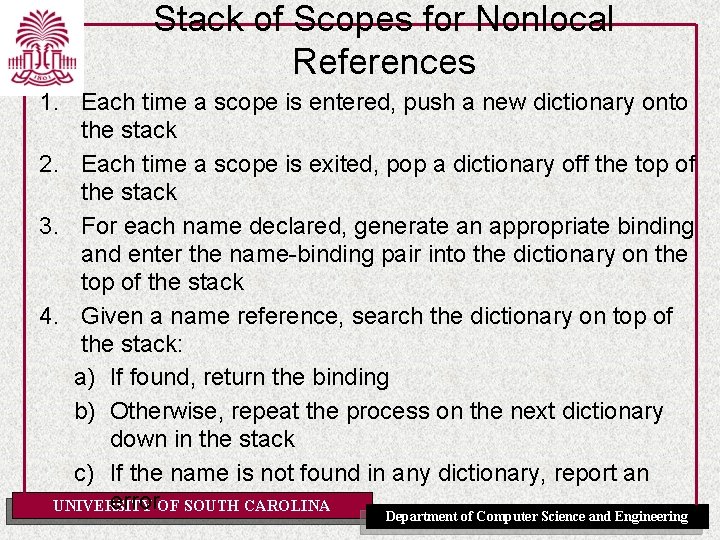
Stack of Scopes for Nonlocal References 1. Each time a scope is entered, push a new dictionary onto the stack 2. Each time a scope is exited, pop a dictionary off the top of the stack 3. For each name declared, generate an appropriate binding and enter the name-binding pair into the dictionary on the top of the stack 4. Given a name reference, search the dictionary on top of the stack: a) If found, return the binding b) Otherwise, repeat the process on the next dictionary down in the stack c) If the name is not found in any dictionary, report an error. OF SOUTH CAROLINA UNIVERSITY Department of Computer Science and Engineering
![Stack of Scope Dictionaries Example C program in Fig 4 1 T stack of Stack of Scope Dictionaries Example C program in Fig. 4. 1 [T], stack of](https://slidetodoc.com/presentation_image_h2/12a7edbc42625a0032125983929e2b65/image-15.jpg)
Stack of Scope Dictionaries Example C program in Fig. 4. 1 [T], stack of dictionaries at line 7: 1 void sort (float a[ ], int size) { <t, 6> 2 int i, j; <j, 2> <i, 2> <size, 1> <a, 1> 3 for (i = 0; i < size; i++) // i, size local <sort, 1> 4 for (j = i + 1; j < size; j++) At line 4 and 11: 5 if (a[j] < a[i]) { // a, i, j local <j, 2> <i, 2> <size, 1> <a, 6 float t; 1> 7 t = a[i]; // t local; a, i nonlocal <sort, 1> 8 a[i] = a[j]; 9 a[j] = t; 10 } Note error in text: The for statement does not create a new scope in C! 11 } UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
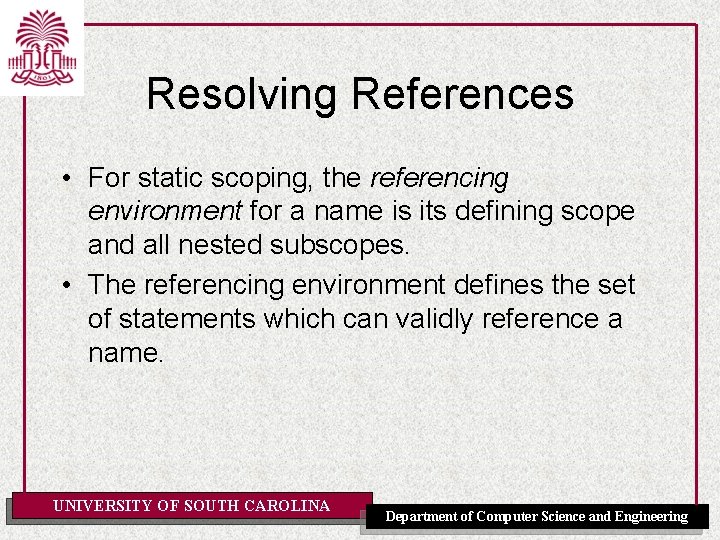
Resolving References • For static scoping, the referencing environment for a name is its defining scope and all nested subscopes. • The referencing environment defines the set of statements which can validly reference a name. UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
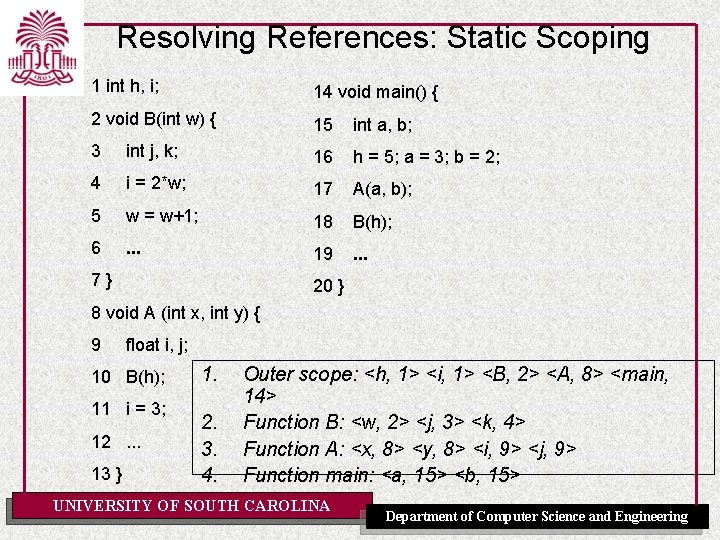
Resolving References: Static Scoping 1 int h, i; 14 void main() { 2 void B(int w) { 15 int a, b; 3 int j, k; 16 h = 5; a = 3; b = 2; 4 i = 2*w; 17 A(a, b); 5 w = w+1; 18 B(h); 6 . . . 19 . . . 7} 20 } 8 void A (int x, int y) { 9 float i, j; 10 B(h); 11 i = 3; 12. . . 13 } 1. 2. 3. 4. Outer scope: <h, 1> <i, 1> <B, 2> <A, 8> <main, 14> Function B: <w, 2> <j, 3> <k, 4> Function A: <x, 8> <y, 8> <i, 9> <j, 9> Function main: <a, 15> <b, 15> UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
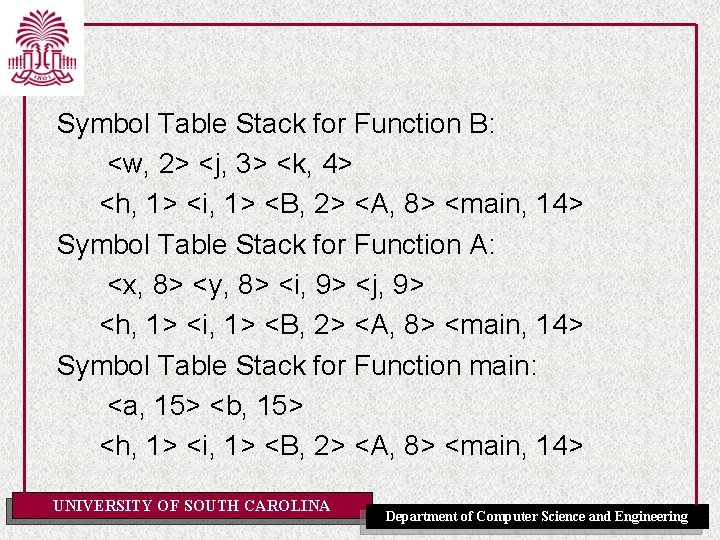
Symbol Table Stack for Function B: <w, 2> <j, 3> <k, 4> <h, 1> <i, 1> <B, 2> <A, 8> <main, 14> Symbol Table Stack for Function A: <x, 8> <y, 8> <i, 9> <j, 9> <h, 1> <i, 1> <B, 2> <A, 8> <main, 14> Symbol Table Stack for Function main: <a, 15> <b, 15> <h, 1> <i, 1> <B, 2> <A, 8> <main, 14> UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
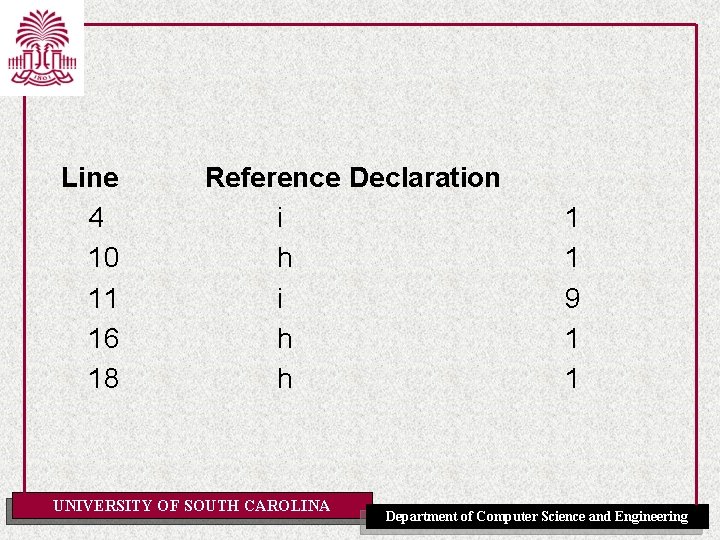
Line 4 10 11 16 18 Reference Declaration i h h UNIVERSITY OF SOUTH CAROLINA 1 1 9 1 1 Department of Computer Science and Engineering
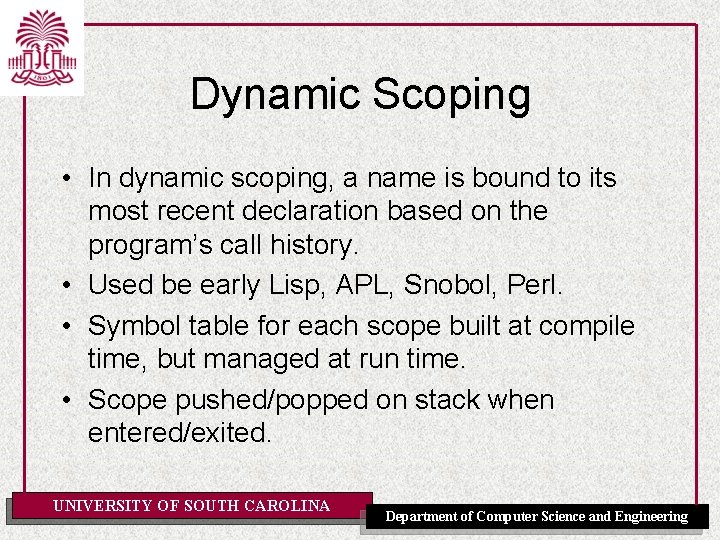
Dynamic Scoping • In dynamic scoping, a name is bound to its most recent declaration based on the program’s call history. • Used be early Lisp, APL, Snobol, Perl. • Symbol table for each scope built at compile time, but managed at run time. • Scope pushed/popped on stack when entered/exited. UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
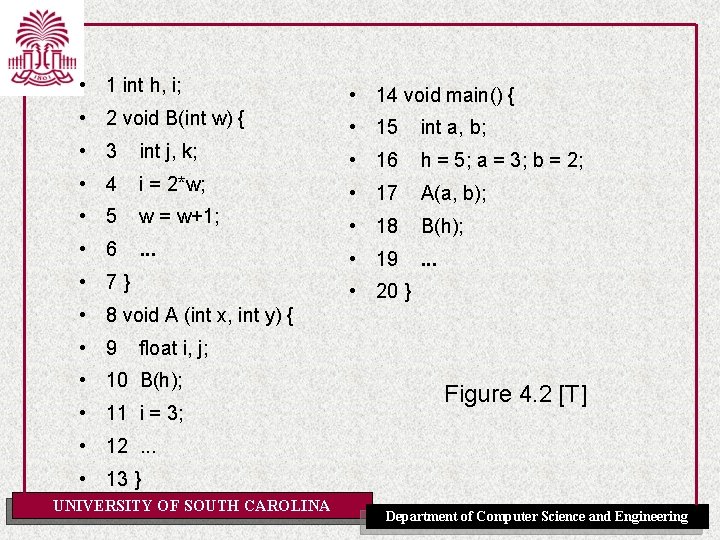
• 1 int h, i; • 14 void main() { • 2 void B(int w) { • 15 int a, b; • 3 int j, k; • 16 h = 5; a = 3; b = 2; • 4 i = 2*w; • 17 A(a, b); • 5 w = w+1; • 18 B(h); • 6 . . . • 19 . . . • 7} • 20 } • 8 void A (int x, int y) { • 9 float i, j; • 10 B(h); • 11 i = 3; Figure 4. 2 [T] • 12. . . • 13 } UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
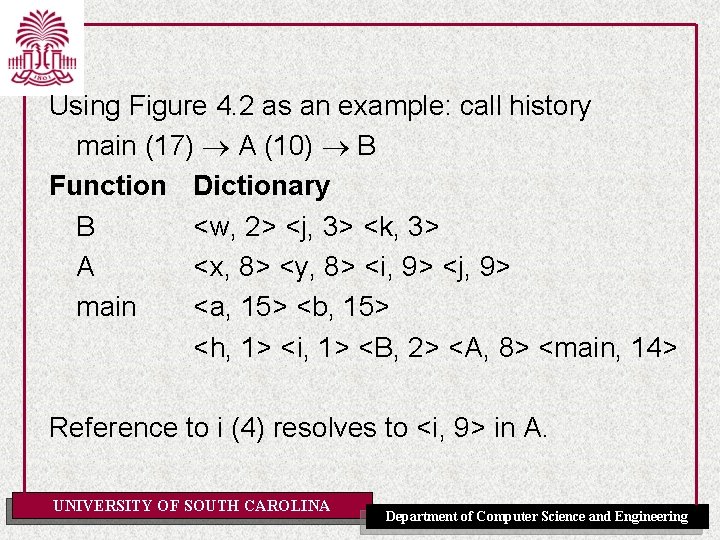
Using Figure 4. 2 as an example: call history main (17) A (10) B Function Dictionary B <w, 2> <j, 3> <k, 3> A <x, 8> <y, 8> <i, 9> <j, 9> main <a, 15> <b, 15> <h, 1> <i, 1> <B, 2> <A, 8> <main, 14> Reference to i (4) resolves to <i, 9> in A. UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
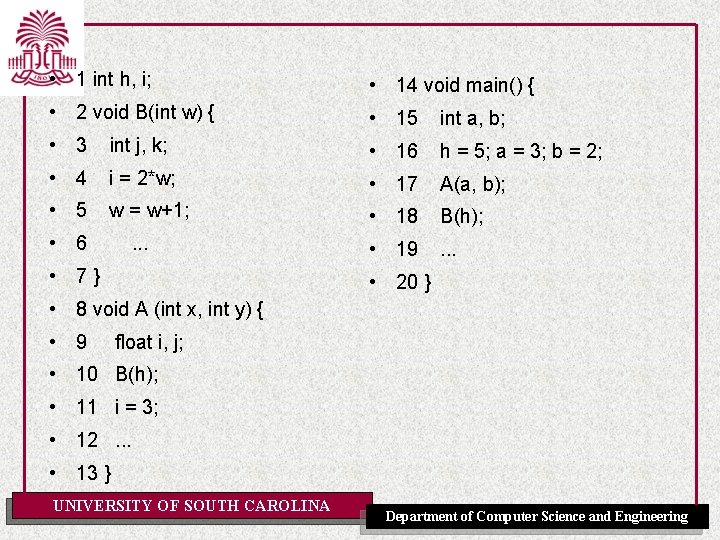
• 1 int h, i; • 14 void main() { • 2 void B(int w) { • 15 int a, b; • 3 int j, k; • 16 h = 5; a = 3; b = 2; • 4 i = 2*w; • 17 A(a, b); • 5 w = w+1; • 18 B(h); • 19 . . . • 6 . . . • 7} • 20 } • 8 void A (int x, int y) { • 9 float i, j; • 10 B(h); • 11 i = 3; • 12. . . • 13 } UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
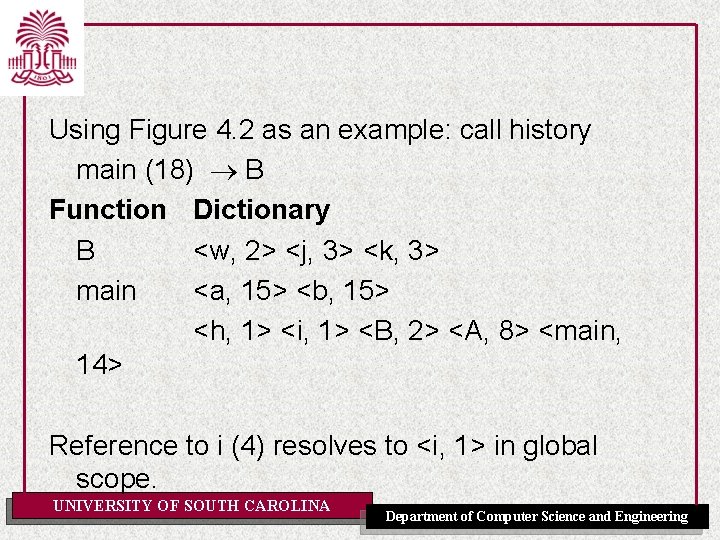
Using Figure 4. 2 as an example: call history main (18) B Function Dictionary B <w, 2> <j, 3> <k, 3> main <a, 15> <b, 15> <h, 1> <i, 1> <B, 2> <A, 8> <main, 14> Reference to i (4) resolves to <i, 1> in global scope. UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
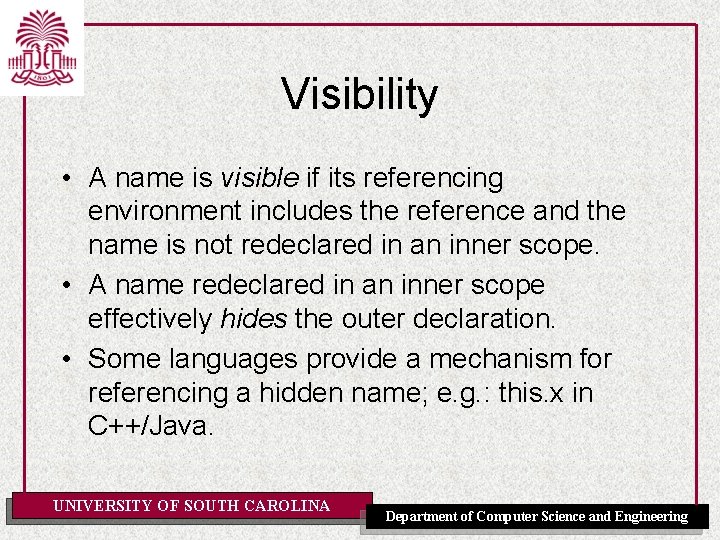
Visibility • A name is visible if its referencing environment includes the reference and the name is not redeclared in an inner scope. • A name redeclared in an inner scope effectively hides the outer declaration. • Some languages provide a mechanism for referencing a hidden name; e. g. : this. x in C++/Java. UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
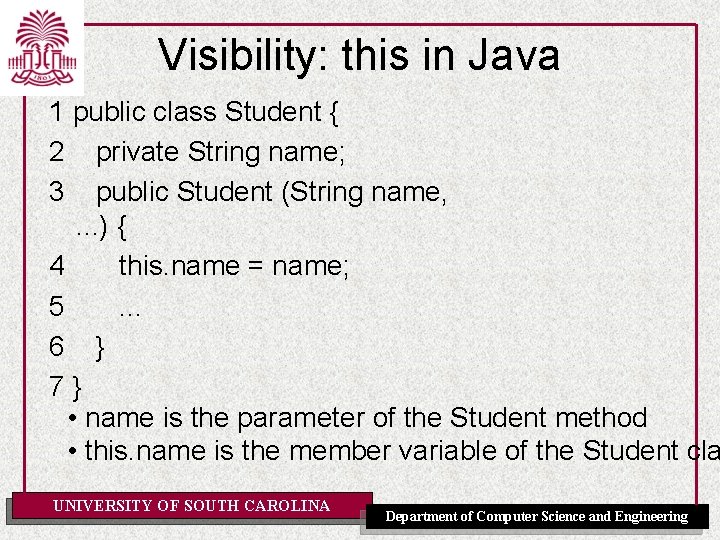
Visibility: this in Java 1 public class Student { 2 private String name; 3 public Student (String name, . . . ) { 4 this. name = name; 5. . . 6 } 7} • name is the parameter of the Student method • this. name is the member variable of the Student cla UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
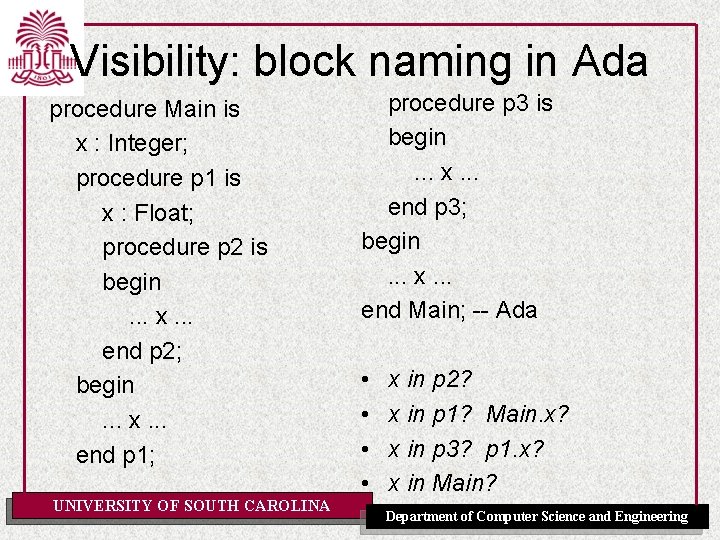
Visibility: block naming in Ada procedure Main is x : Integer; procedure p 1 is x : Float; procedure p 2 is begin. . . x. . . end p 2; begin. . . x. . . end p 1; UNIVERSITY OF SOUTH CAROLINA procedure p 3 is begin. . . x. . . end p 3; begin. . . x. . . end Main; -- Ada • • x in p 2? x in p 1? Main. x? x in p 3? p 1. x? x in Main? Department of Computer Science and Engineering
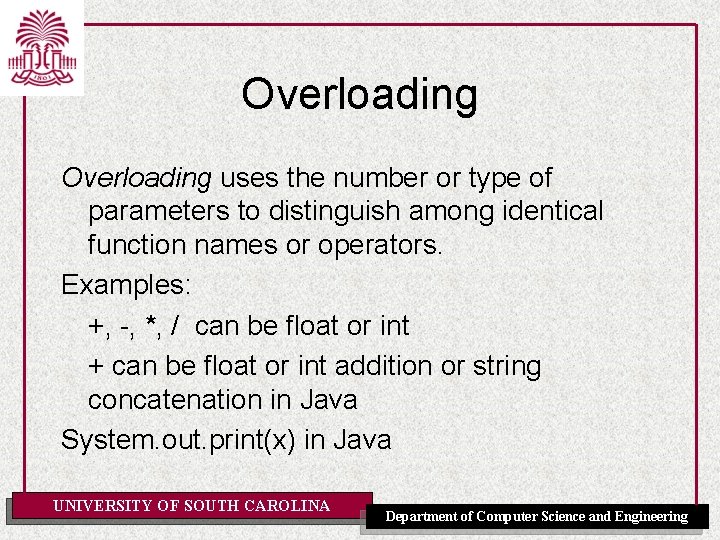
Overloading uses the number or type of parameters to distinguish among identical function names or operators. Examples: +, -, *, / can be float or int + can be float or int addition or string concatenation in Java System. out. print(x) in Java UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
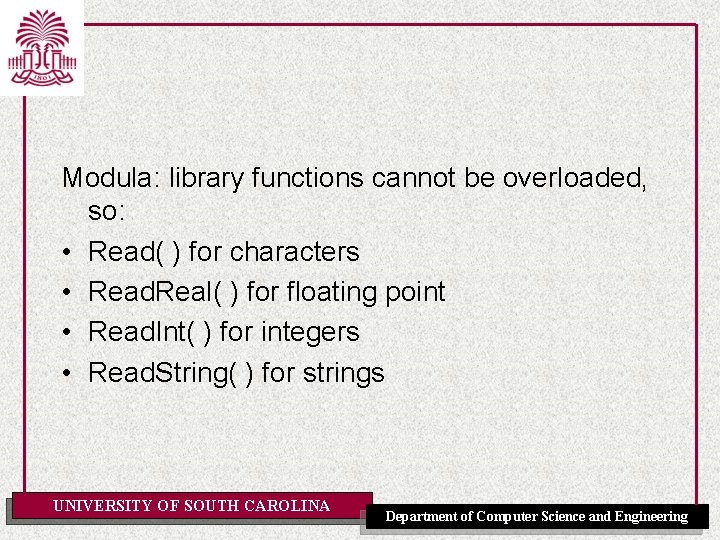
Modula: library functions cannot be overloaded, so: • Read( ) for characters • Read. Real( ) for floating point • Read. Int( ) for integers • Read. String( ) for strings UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
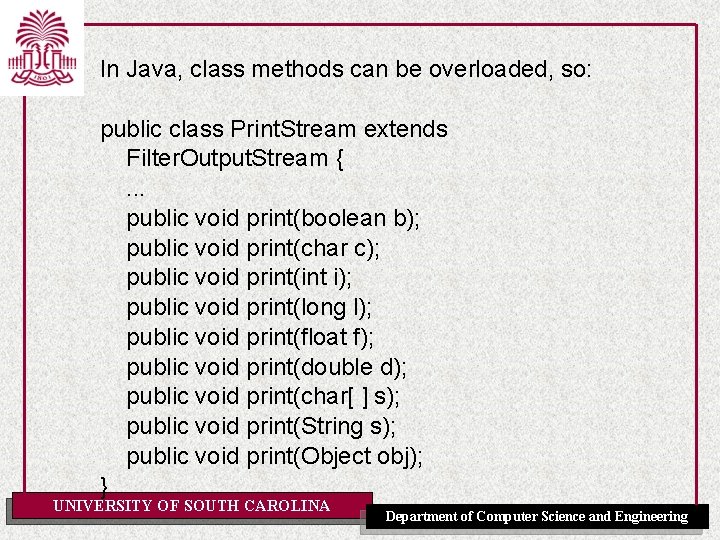
In Java, class methods can be overloaded, so: public class Print. Stream extends Filter. Output. Stream {. . . public void print(boolean b); public void print(char c); public void print(int i); public void print(long l); public void print(float f); public void print(double d); public void print(char[ ] s); public void print(String s); public void print(Object obj); } UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
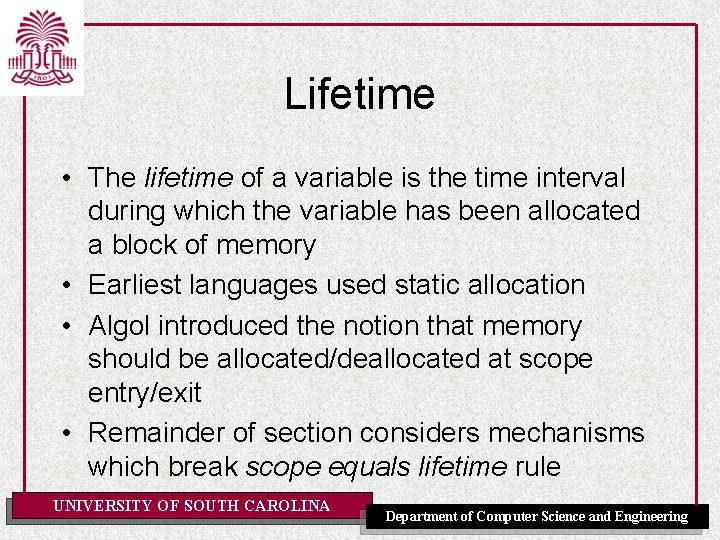
Lifetime • The lifetime of a variable is the time interval during which the variable has been allocated a block of memory • Earliest languages used static allocation • Algol introduced the notion that memory should be allocated/deallocated at scope entry/exit • Remainder of section considers mechanisms which break scope equals lifetime rule UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
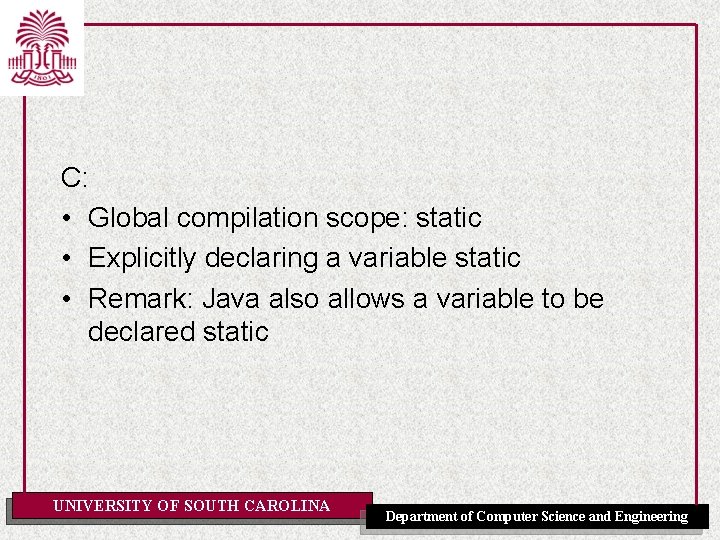
C: • Global compilation scope: static • Explicitly declaring a variable static • Remark: Java also allows a variable to be declared static UNIVERSITY OF SOUTH CAROLINA Department of Computer Science and Engineering
Homologous structures definition
Oval flow chart
Language
Control structures in c
9 chemical names and formulas
Prolog reverse list
Chapter 16 programming language
Chapter 16 programming language
Perbedaan linear programming dan integer programming
Greedy vs dynamic
Windows 10 system programming, part 1
Linear vs integer programming
Programing adalah
Nwcg s-330
S330 nwcg
Desacato a funcionário público
Great schism 1054
1453-330
395-1453
Beräkna kapitalstorlek
Nuremberg code
Stats 330
Oracle 11g client download
Csci 330
Indica la forma correcta de cada verbo en el imperfecto.
The roman principate (31 bce- 330 ce) was installed by
Bts 330
Stats 330
Nep 530
Isa 330 bahasa indonesia
Astm c 330
Definition of byzantium
Astm c 330