CS 1704 Introduction to Data Structures and Software
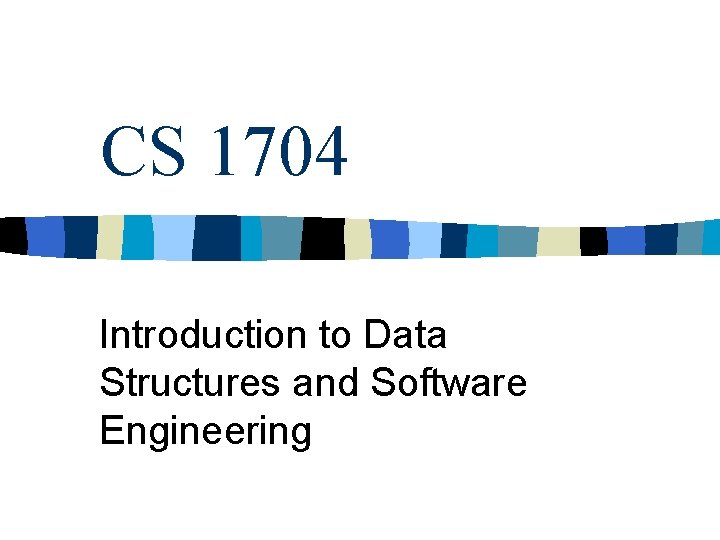
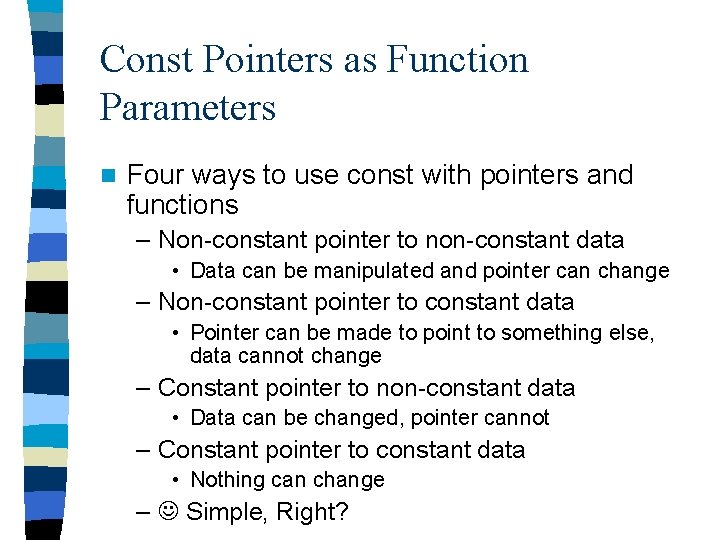
![Array Pointer n Assume we have int b[6] and int * b. Ptr n Array Pointer n Assume we have int b[6] and int * b. Ptr n](https://slidetodoc.com/presentation_image_h2/928955f2b83e9916094b488d5a71da11/image-3.jpg)
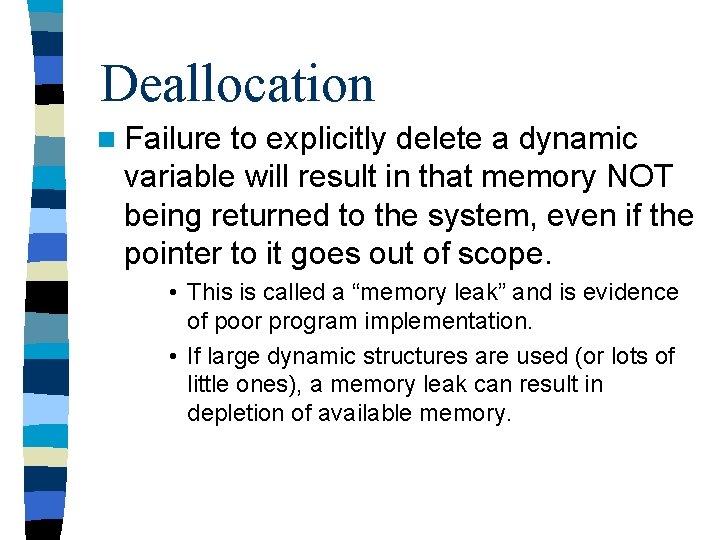
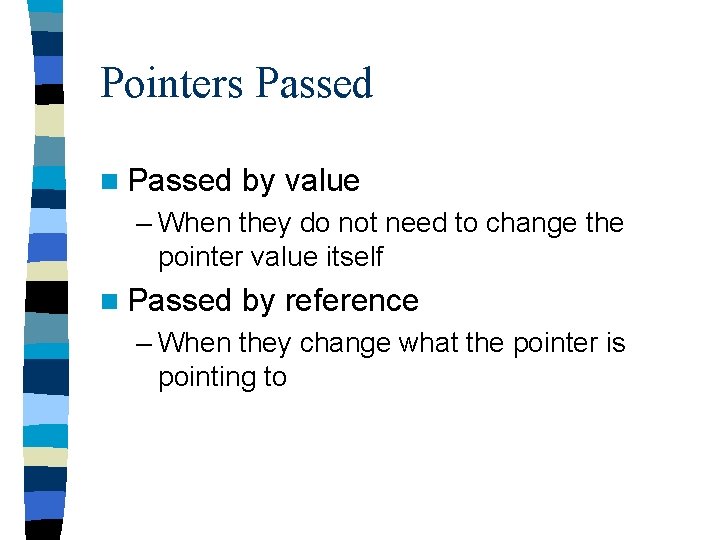
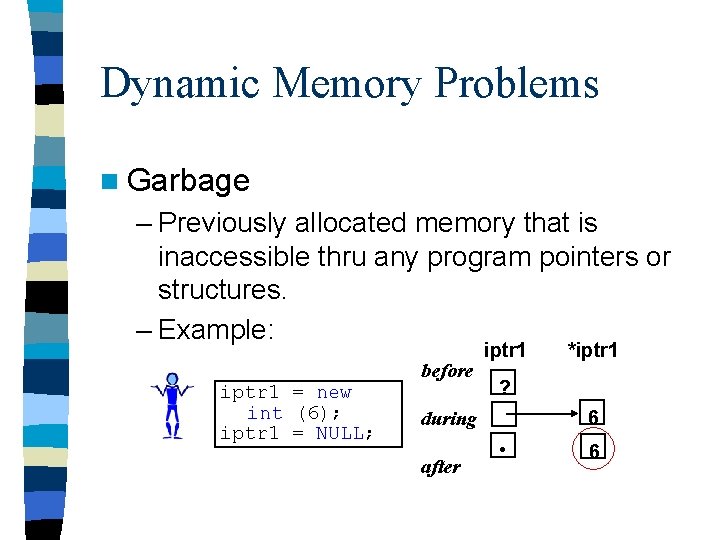
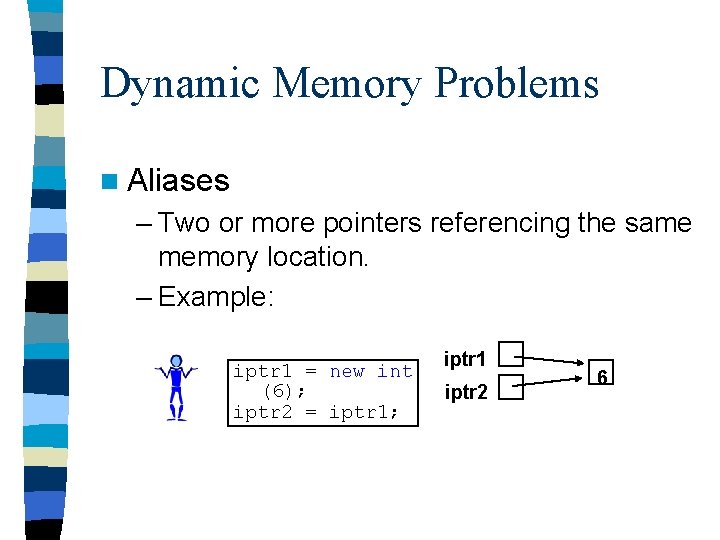
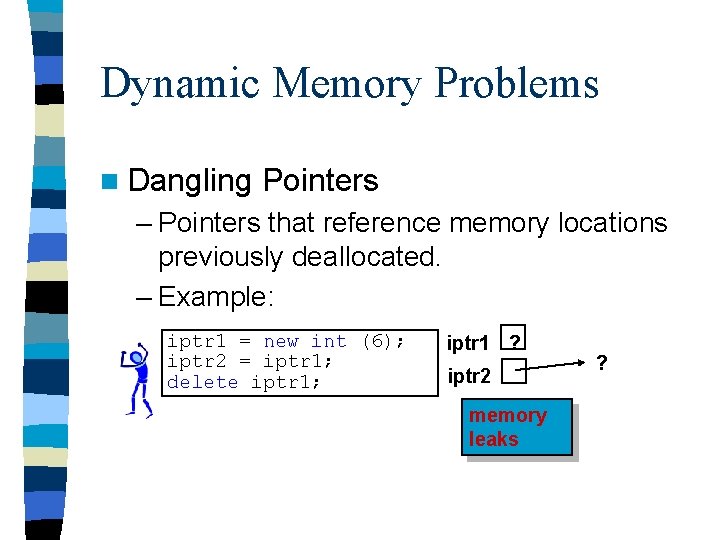
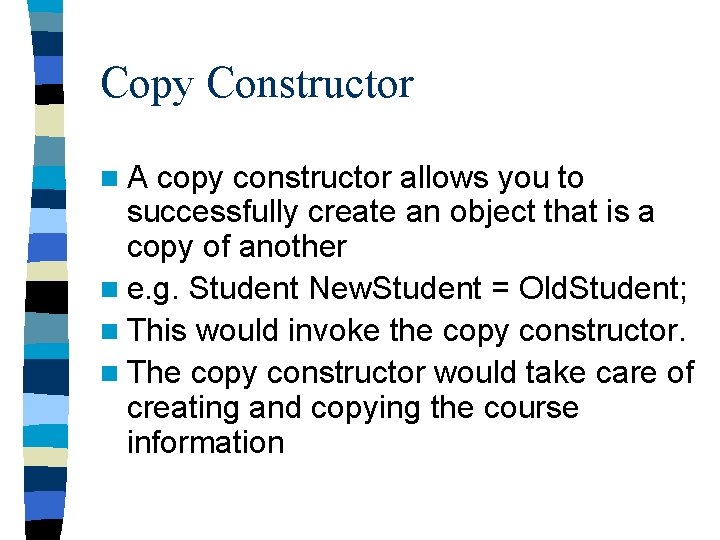
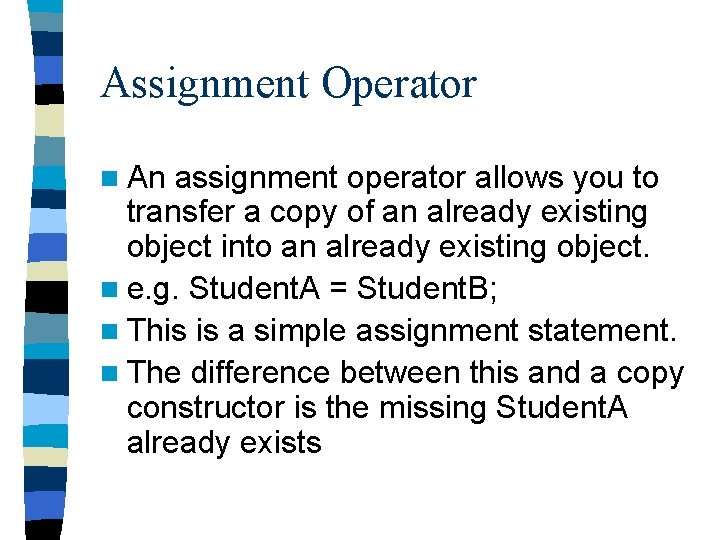
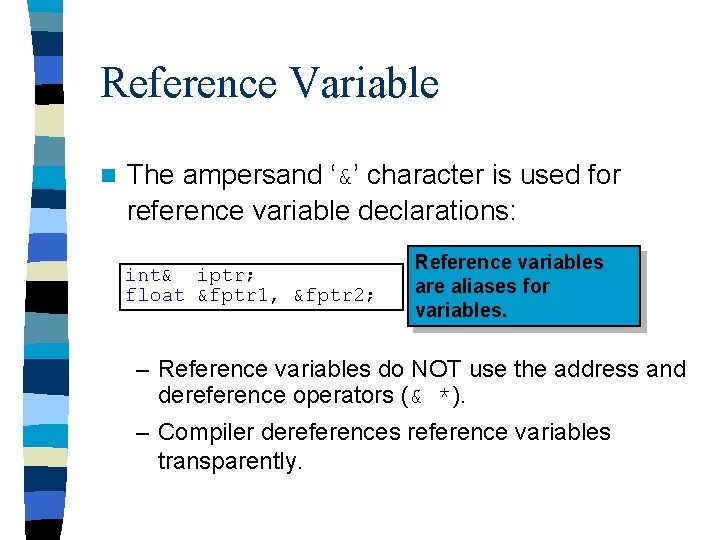
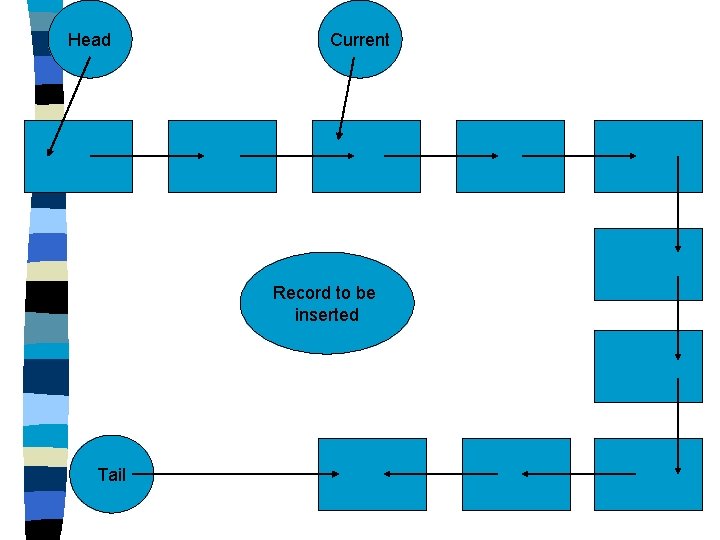
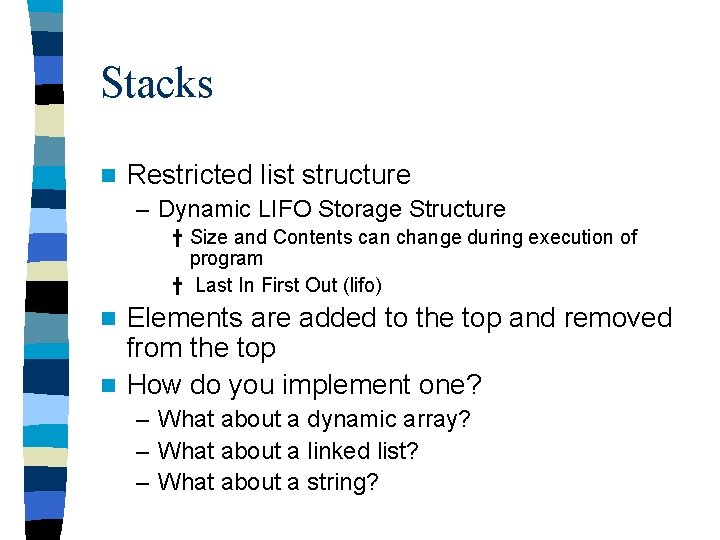
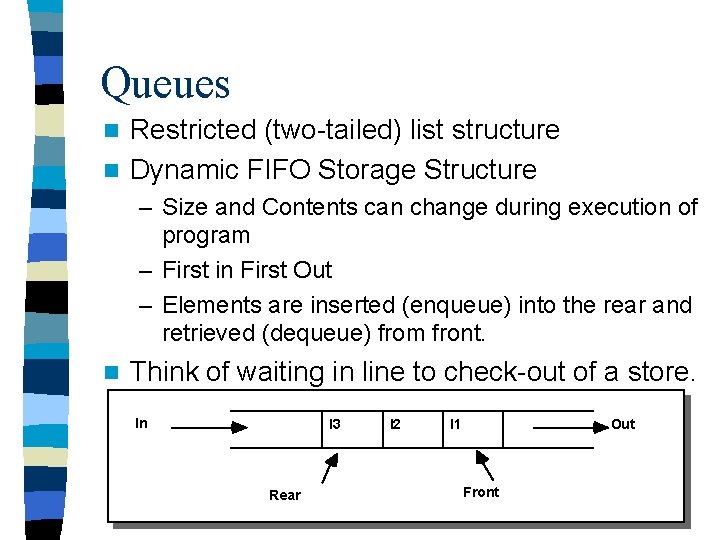
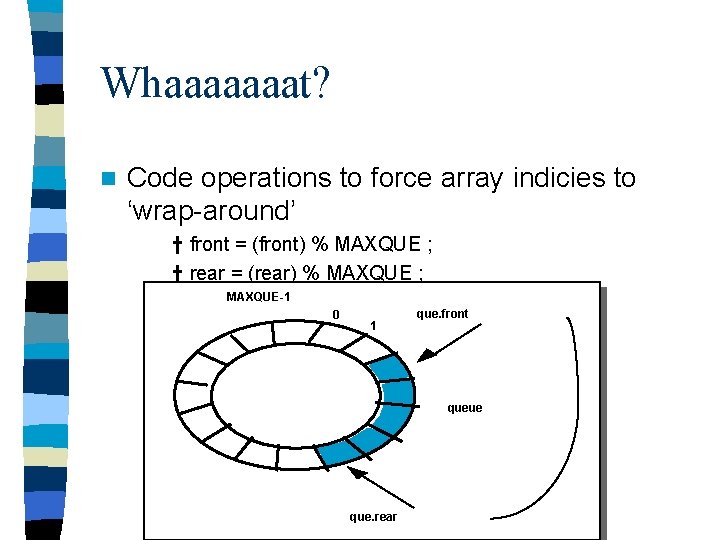
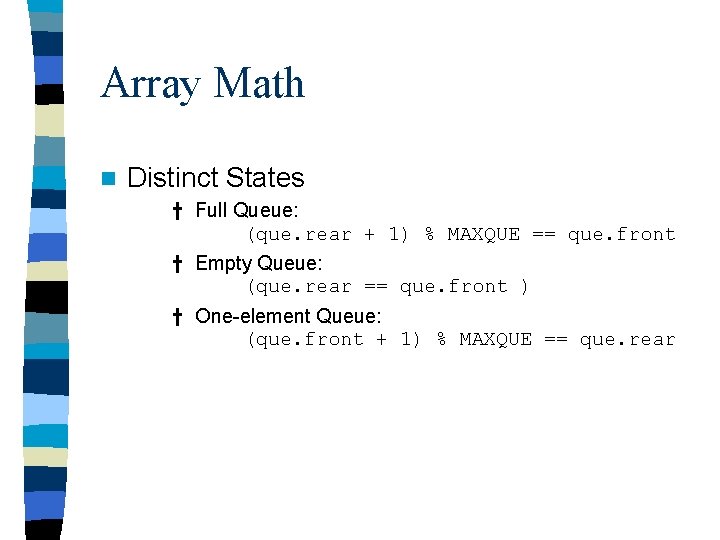
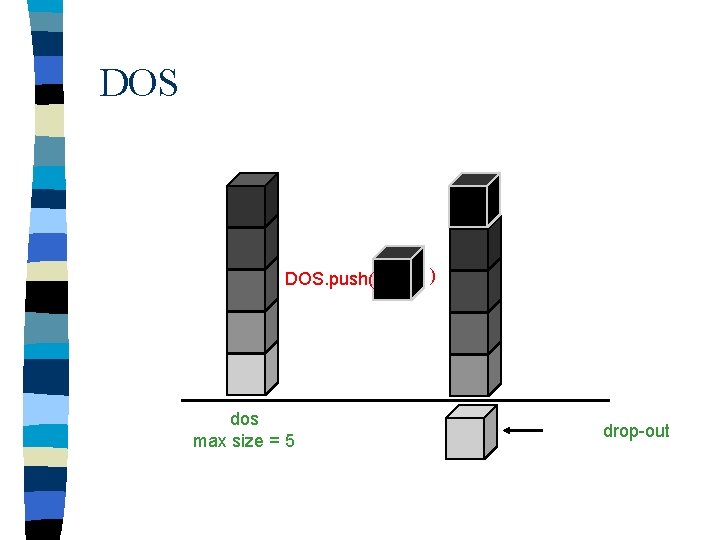
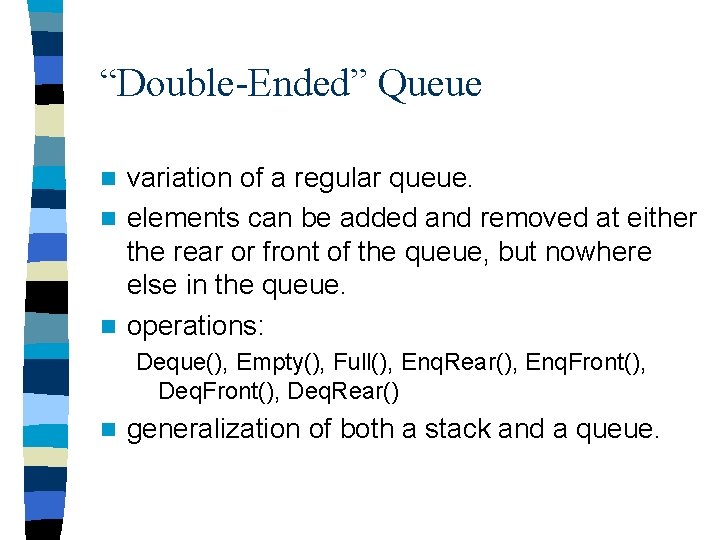
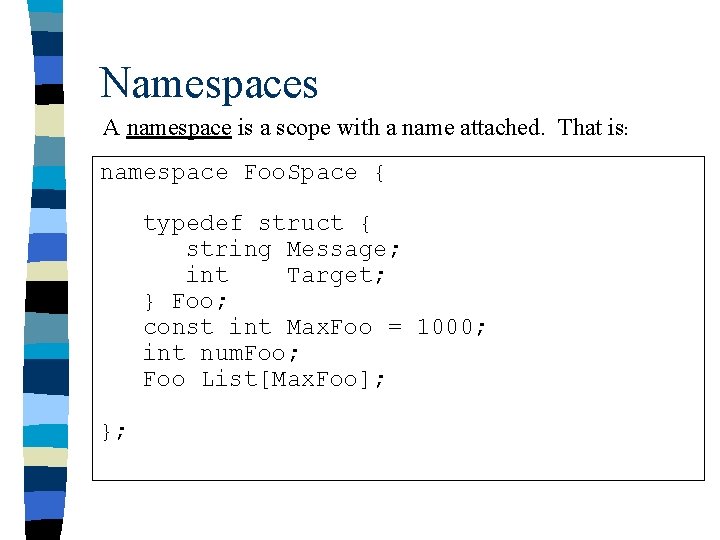
- Slides: 19
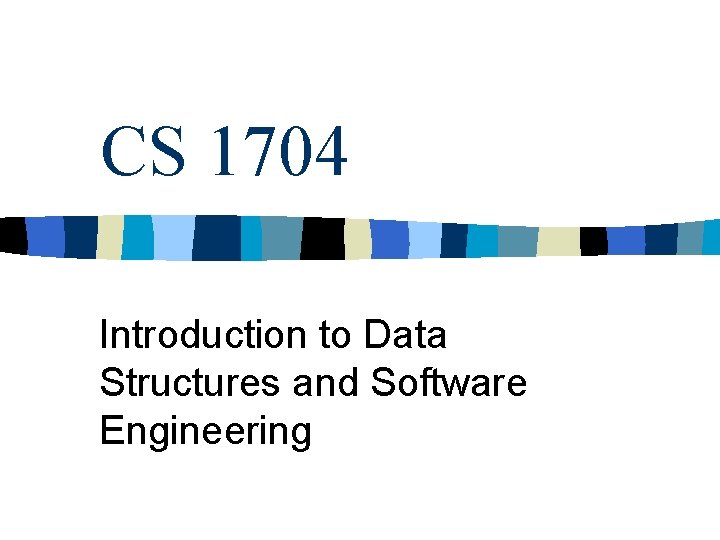
CS 1704 Introduction to Data Structures and Software Engineering
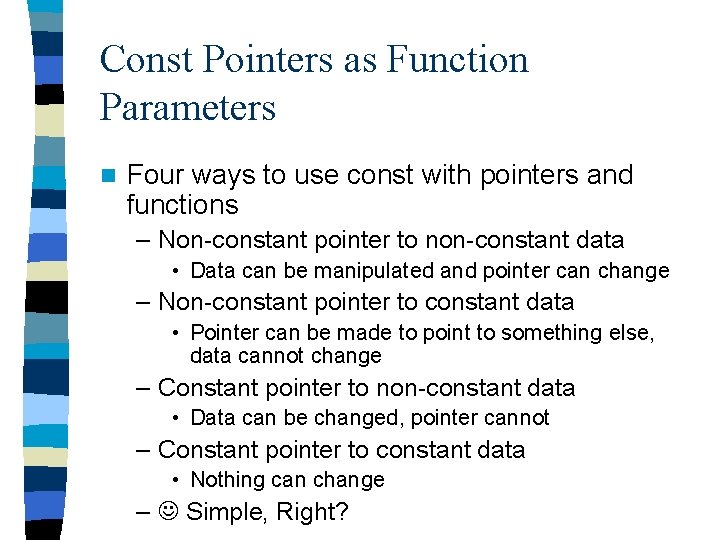
Const Pointers as Function Parameters n Four ways to use const with pointers and functions – Non-constant pointer to non-constant data • Data can be manipulated and pointer can change – Non-constant pointer to constant data • Pointer can be made to point to something else, data cannot change – Constant pointer to non-constant data • Data can be changed, pointer cannot – Constant pointer to constant data • Nothing can change – Simple, Right?
![Array Pointer n Assume we have int b6 and int b Ptr n Array Pointer n Assume we have int b[6] and int * b. Ptr n](https://slidetodoc.com/presentation_image_h2/928955f2b83e9916094b488d5a71da11/image-3.jpg)
Array Pointer n Assume we have int b[6] and int * b. Ptr n We can do this: – b. Ptr = b; – b. Ptr = &b[ 0 ]; n Also, for example b[3] is: – ( b. Ptr + 3 ) – *( b + 3 )
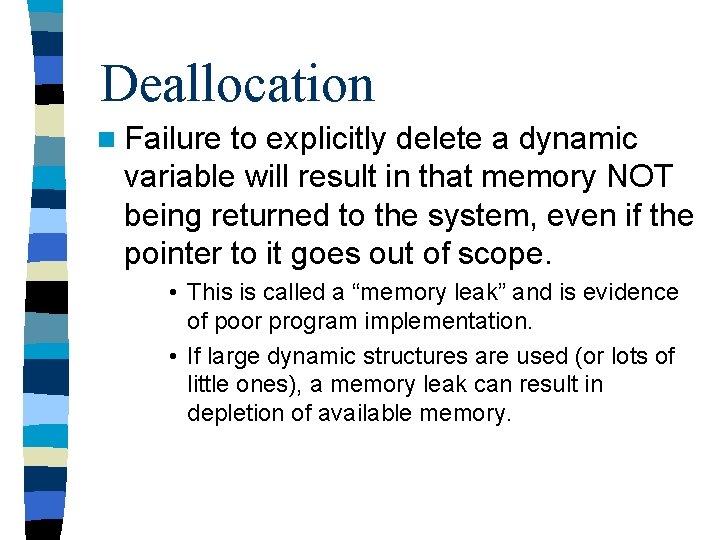
Deallocation n Failure to explicitly delete a dynamic variable will result in that memory NOT being returned to the system, even if the pointer to it goes out of scope. • This is called a “memory leak” and is evidence of poor program implementation. • If large dynamic structures are used (or lots of little ones), a memory leak can result in depletion of available memory.
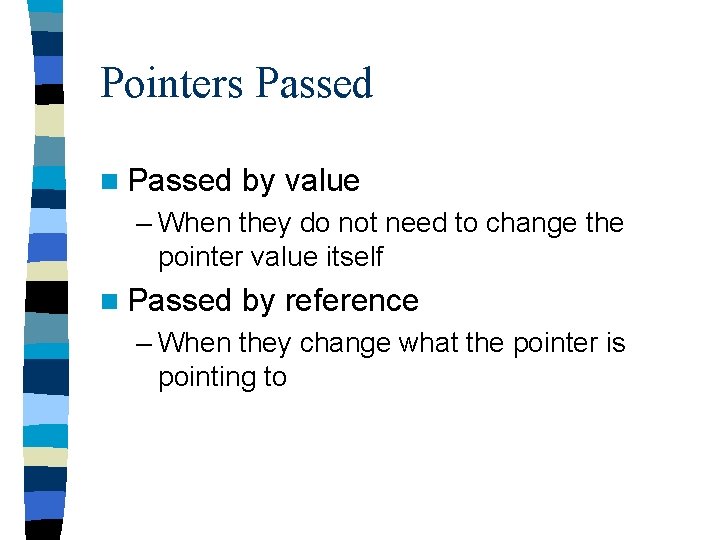
Pointers Passed n Passed by value – When they do not need to change the pointer value itself n Passed by reference – When they change what the pointer is pointing to
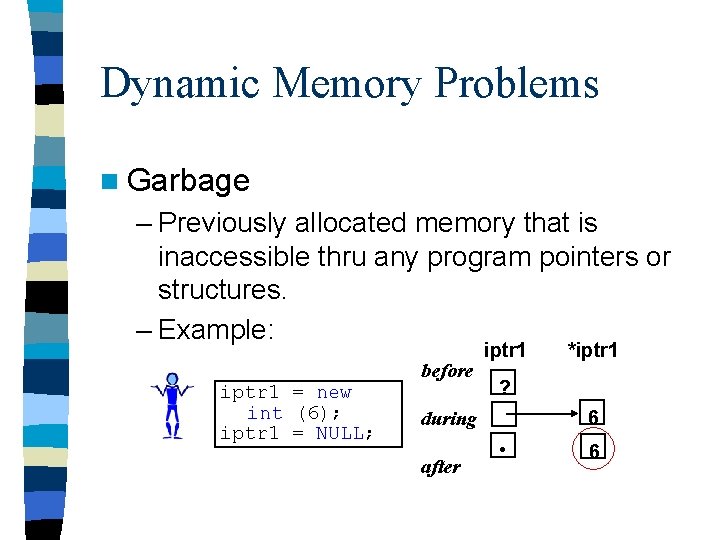
Dynamic Memory Problems n Garbage – Previously allocated memory that is inaccessible thru any program pointers or structures. – Example: before iptr 1 = new int (6); iptr 1 = NULL; iptr 1 ? 6 during after *iptr 1 • 6
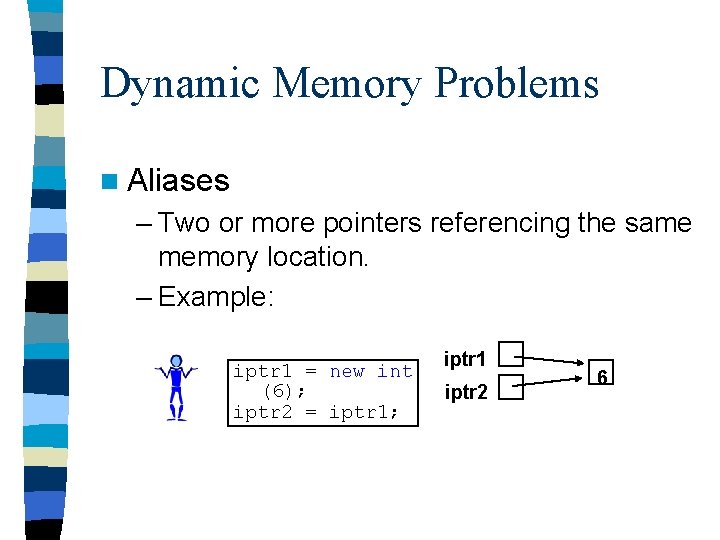
Dynamic Memory Problems n Aliases – Two or more pointers referencing the same memory location. – Example: iptr 1 = new int (6); iptr 2 = iptr 1; iptr 1 iptr 2 6
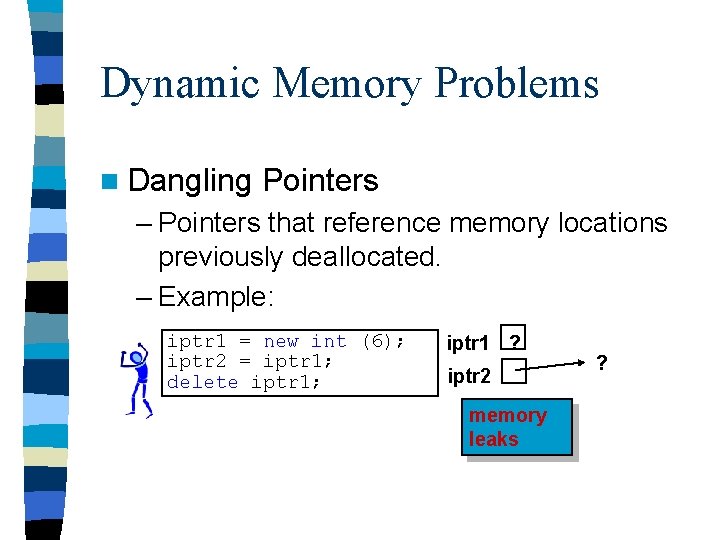
Dynamic Memory Problems n Dangling Pointers – Pointers that reference memory locations previously deallocated. – Example: iptr 1 = new int (6); iptr 2 = iptr 1; delete iptr 1; iptr 1 ? iptr 2 memory leaks ?
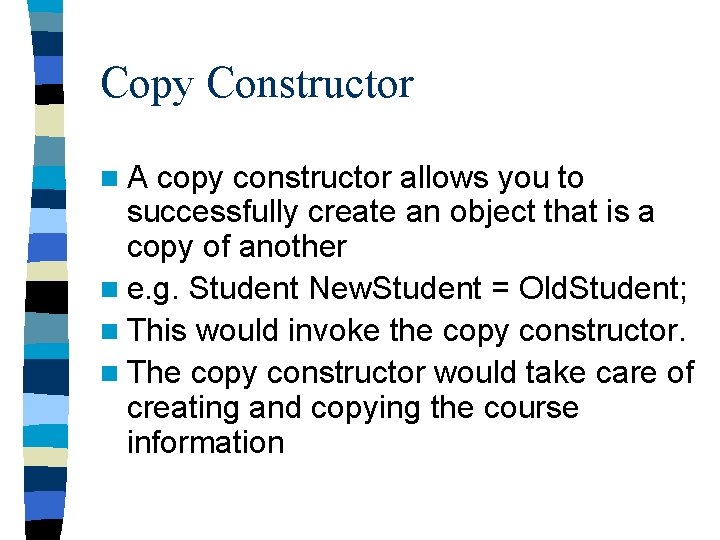
Copy Constructor n. A copy constructor allows you to successfully create an object that is a copy of another n e. g. Student New. Student = Old. Student; n This would invoke the copy constructor. n The copy constructor would take care of creating and copying the course information
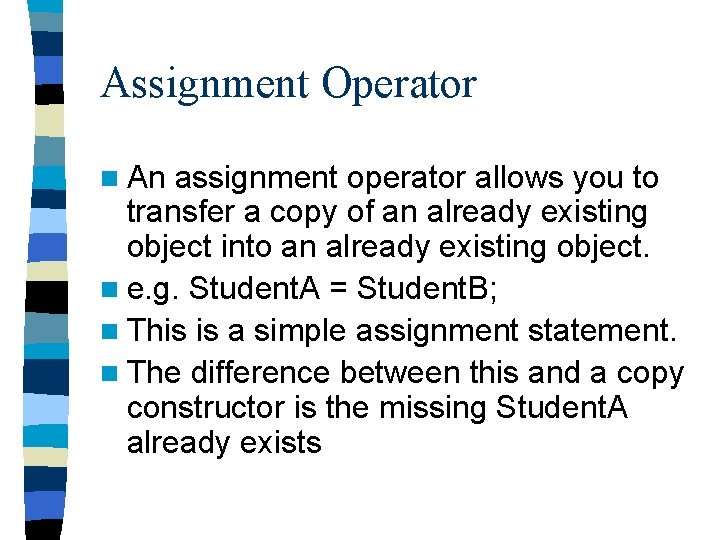
Assignment Operator n An assignment operator allows you to transfer a copy of an already existing object into an already existing object. n e. g. Student. A = Student. B; n This is a simple assignment statement. n The difference between this and a copy constructor is the missing Student. A already exists
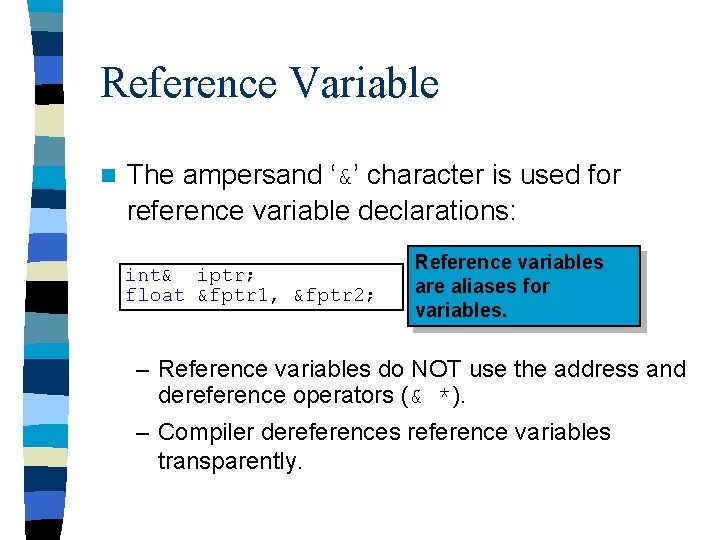
Reference Variable n The ampersand ‘&’ character is used for reference variable declarations: int& iptr; float &fptr 1, &fptr 2; Reference variables are aliases for variables. – Reference variables do NOT use the address and dereference operators (& *). – Compiler dereferences reference variables transparently.
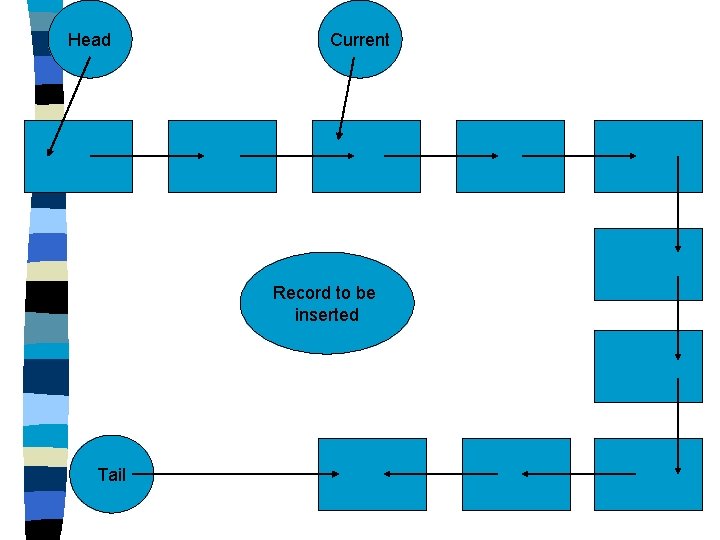
Head Current Record to be inserted Tail
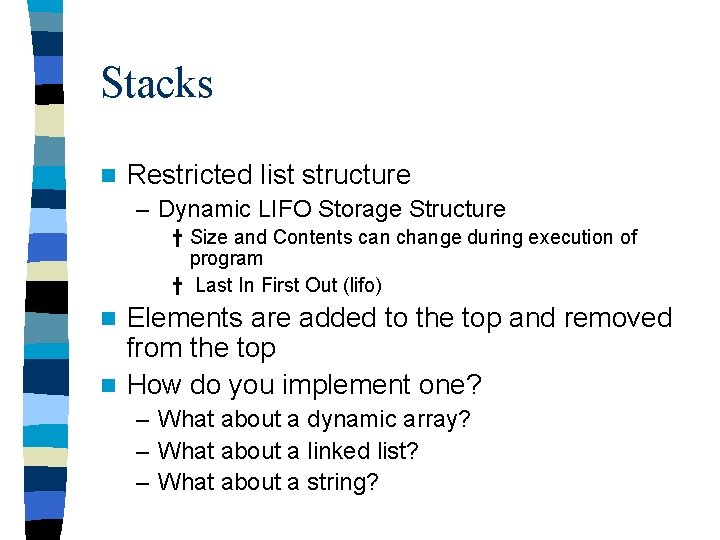
Stacks n Restricted list structure – Dynamic LIFO Storage Structure † Size and Contents can change during execution of program † Last In First Out (lifo) Elements are added to the top and removed from the top n How do you implement one? n – What about a dynamic array? – What about a linked list? – What about a string?
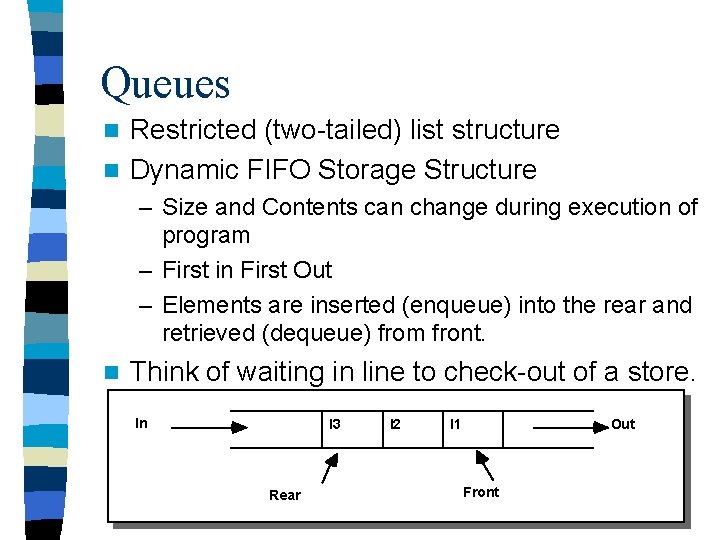
Queues Restricted (two-tailed) list structure n Dynamic FIFO Storage Structure n – Size and Contents can change during execution of program – First in First Out – Elements are inserted (enqueue) into the rear and retrieved (dequeue) from front. n Think of waiting in line to check-out of a store. In I 3 Rear I 2 I 1 Out Front
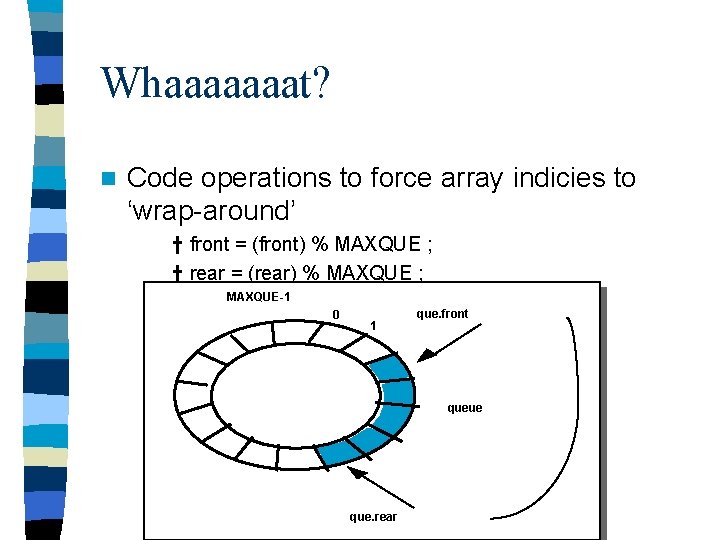
Whaaaaaaat? n Code operations to force array indicies to ‘wrap-around’ † front = (front) % MAXQUE ; † rear = (rear) % MAXQUE ; MAXQUE-1 0 1 que. front queue que. rear
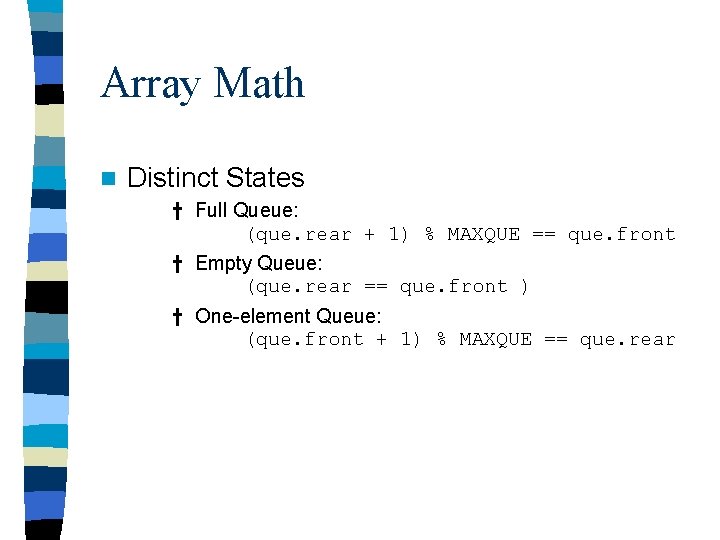
Array Math n Distinct States † Full Queue: (que. rear + 1) % MAXQUE == que. front † Empty Queue: (que. rear == que. front ) † One-element Queue: (que. front + 1) % MAXQUE == que. rear
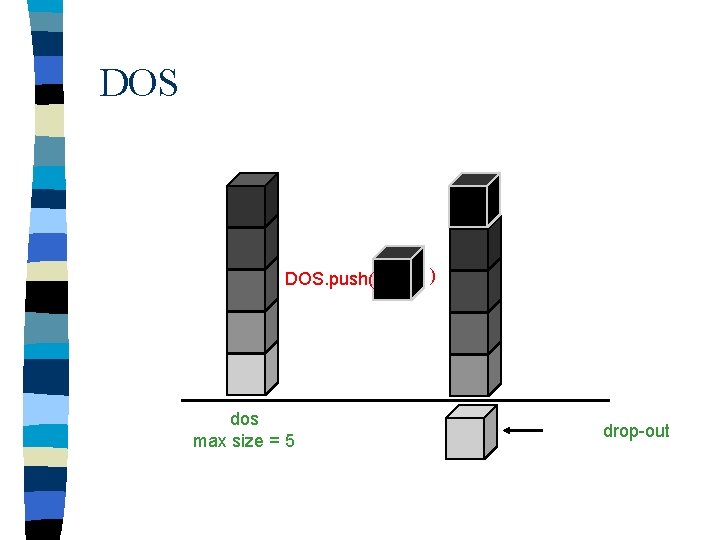
DOS DOS. push( dos max size = 5 ) drop-out
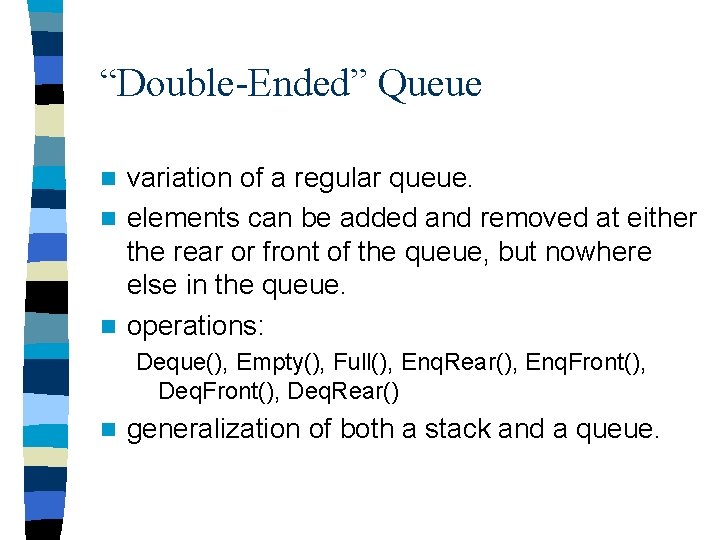
“Double-Ended” Queue variation of a regular queue. n elements can be added and removed at either the rear or front of the queue, but nowhere else in the queue. n operations: n Deque(), Empty(), Full(), Enq. Rear(), Enq. Front(), Deq. Rear() n generalization of both a stack and a queue.
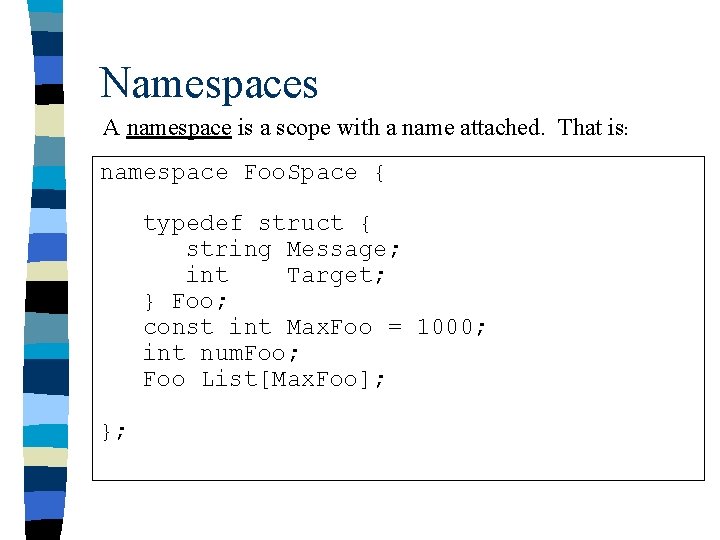
Namespaces A namespace is a scope with a name attached. That is: namespace Foo. Space { typedef struct { string Message; int Target; } Foo; const int Max. Foo = 1000; int num. Foo; Foo List[Max. Foo]; };
Poolse koning 1704
Vip room standard life
Examples of homologous
Introduction to data structures
Introduction to data structures
Introduction to data mining and data warehousing
Data structures and algorithms iit bombay
Cos 423 princeton
Data structures and algorithms tutorial
Conditional macro expansion
Assembler data structures
Information retrieval data structures and algorithms
Data structures and abstractions with java
Data structures and algorithms bits pilani
Adts, data structures, and problem solving with c++
Ajit diwan iitb
Data structures and algorithm
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo