CS 1704 Introduction to Data Structures and Software
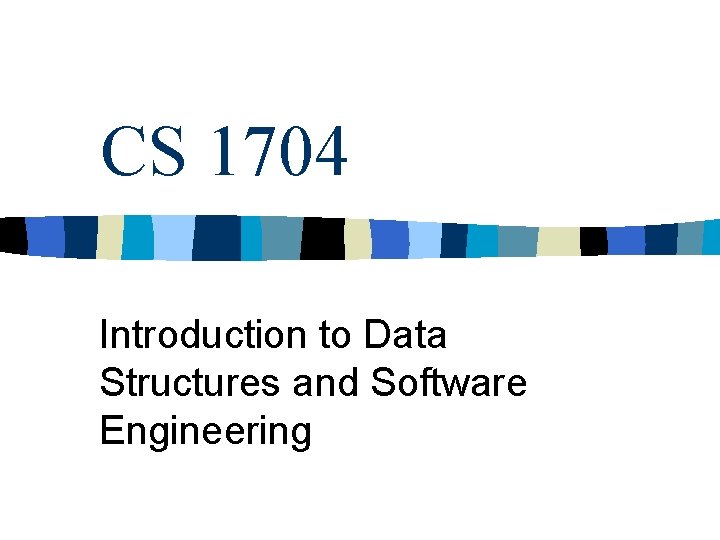
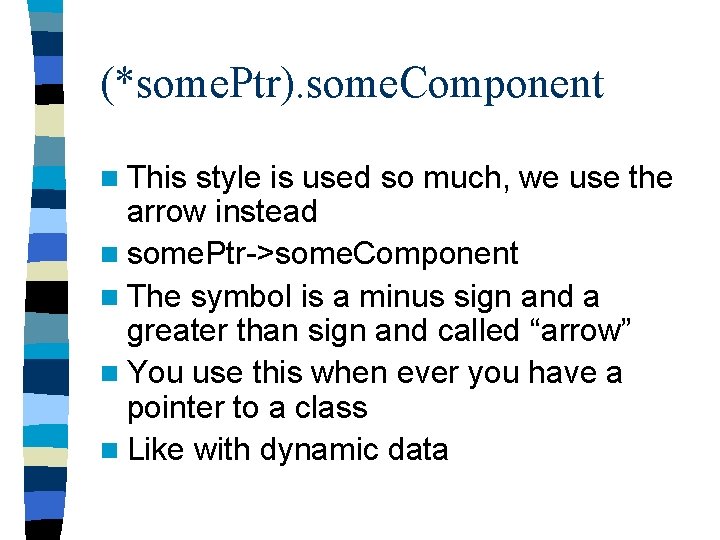
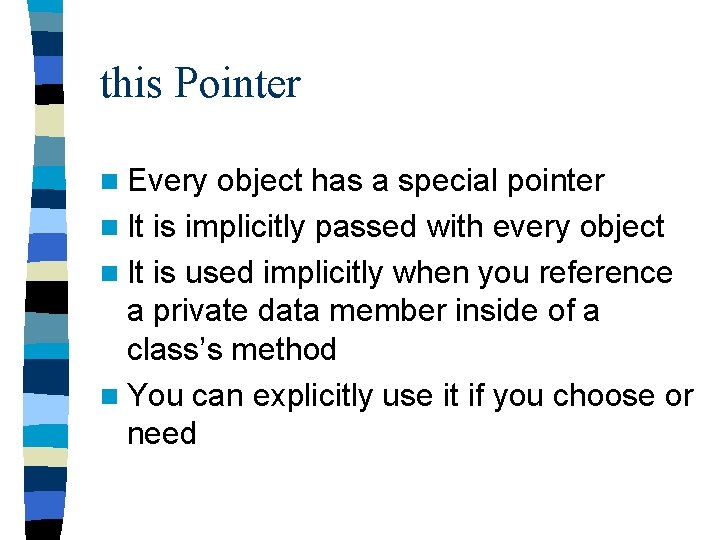
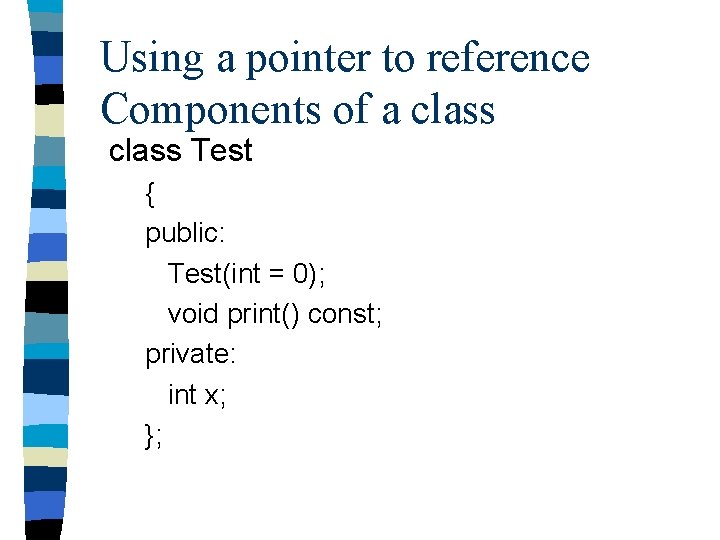
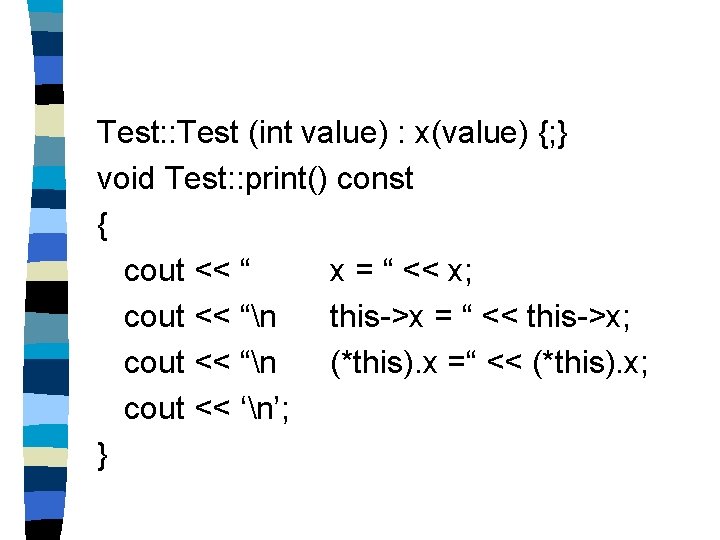
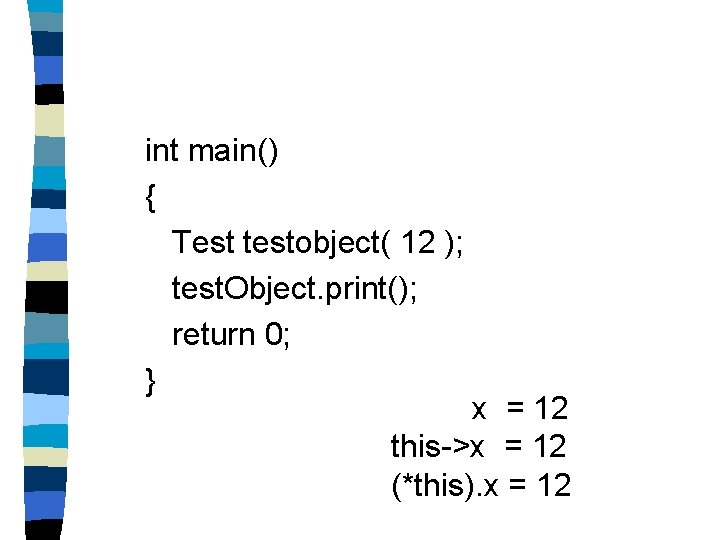
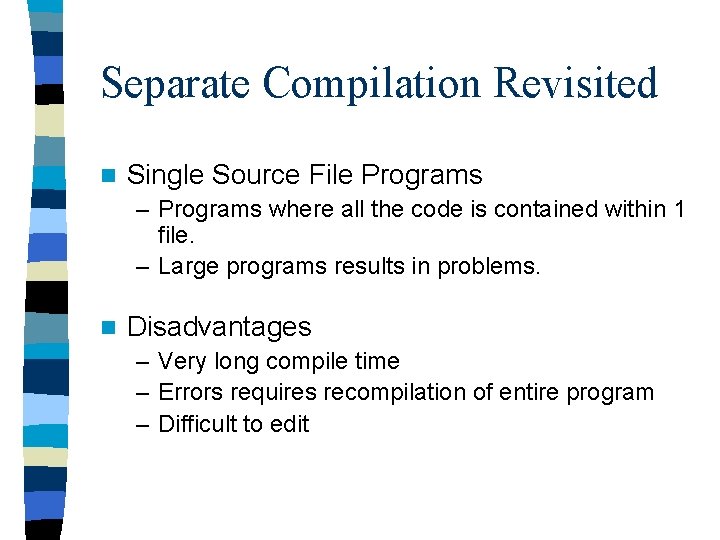
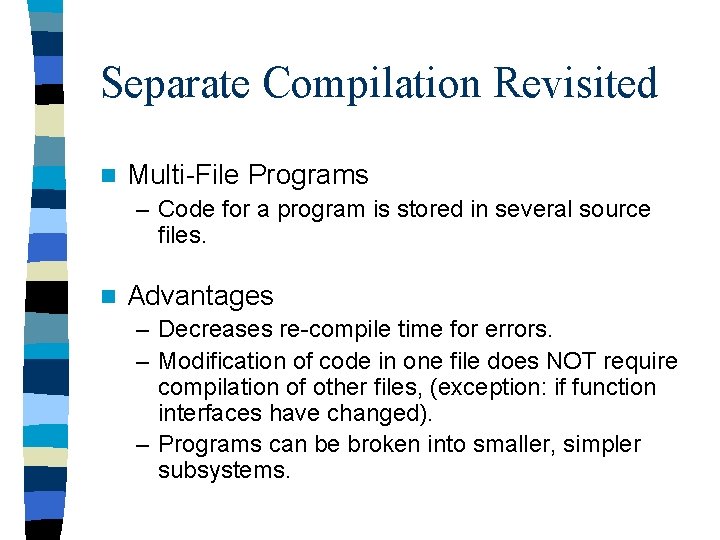
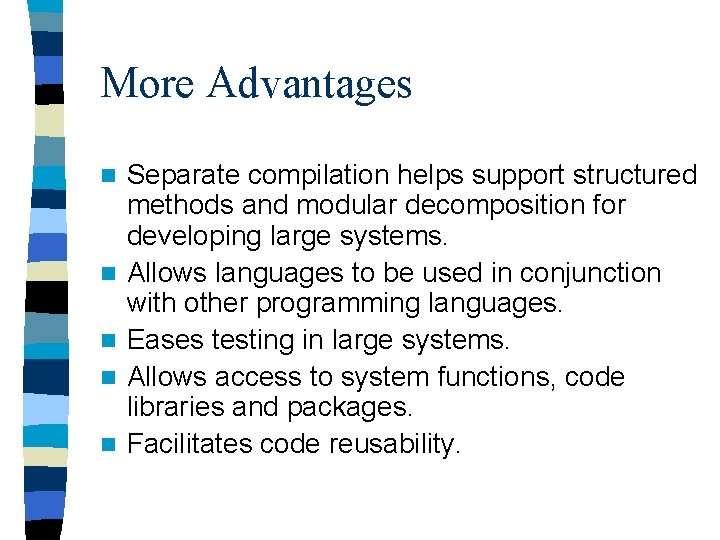
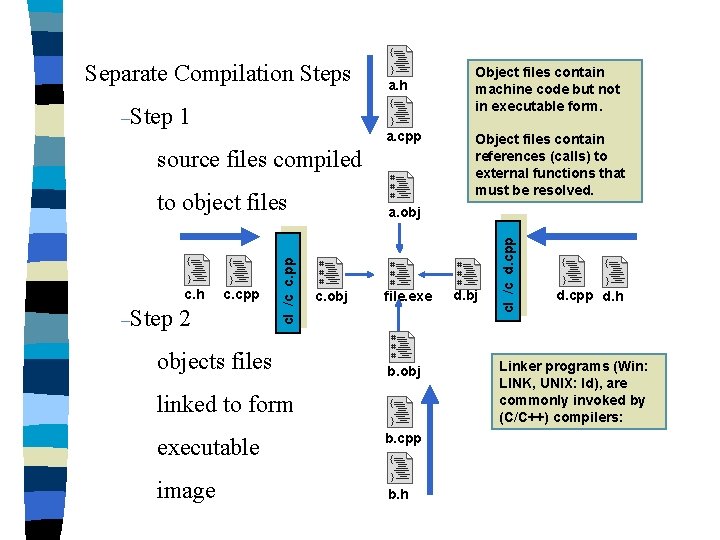
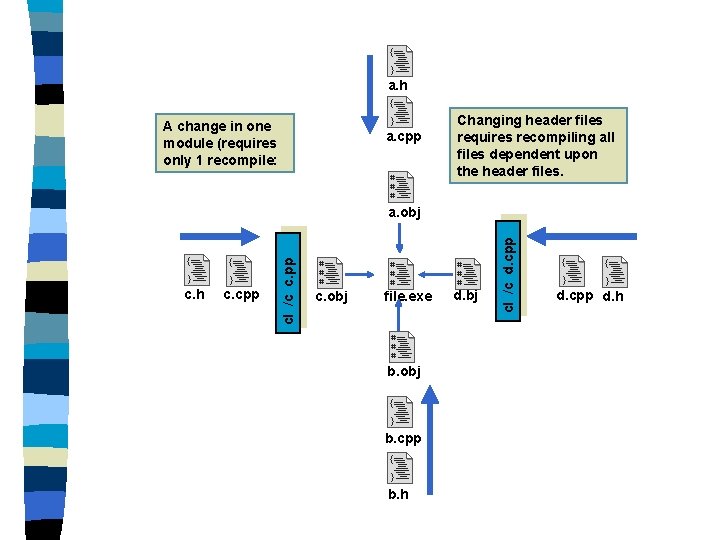
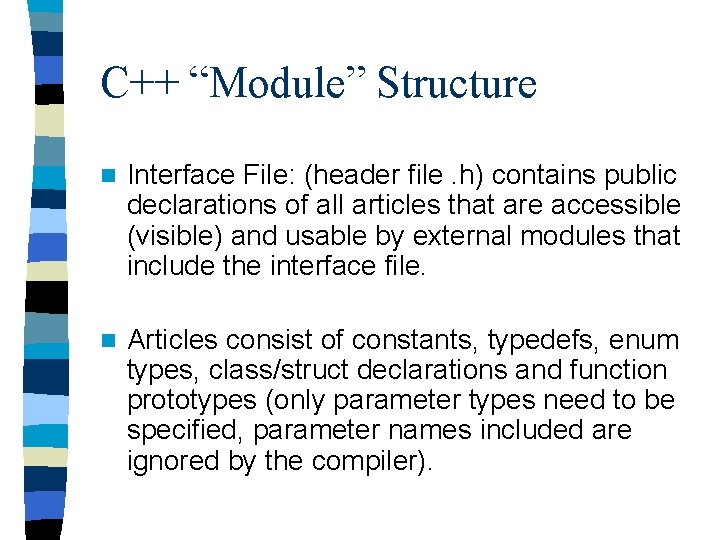
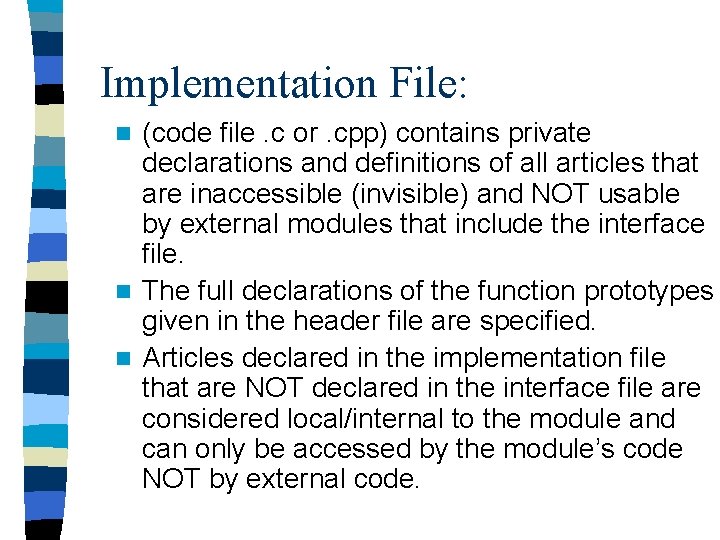
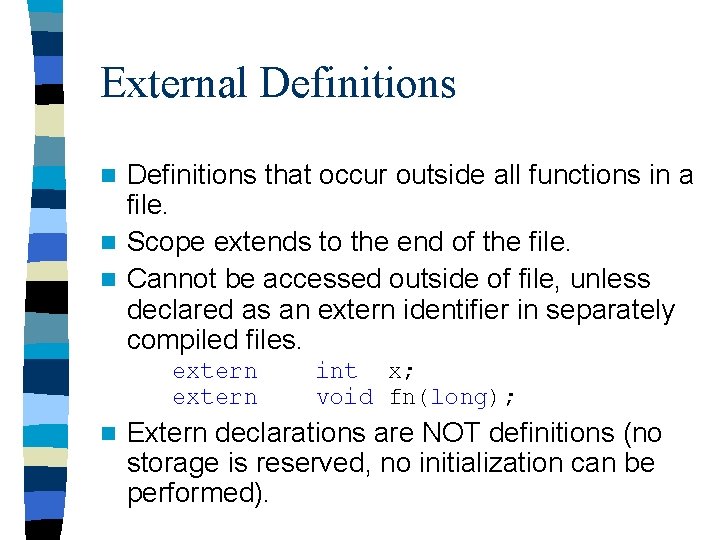
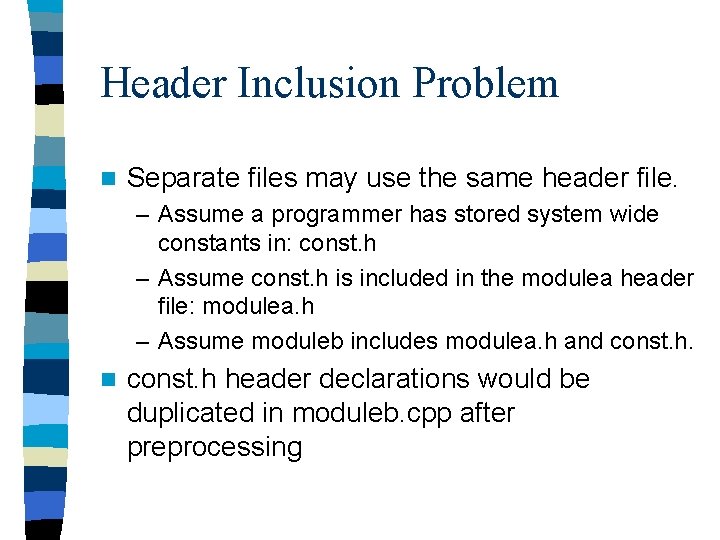
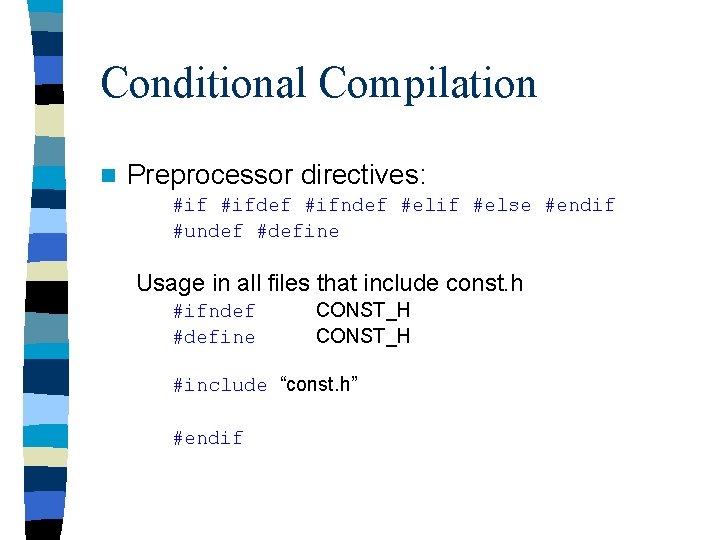
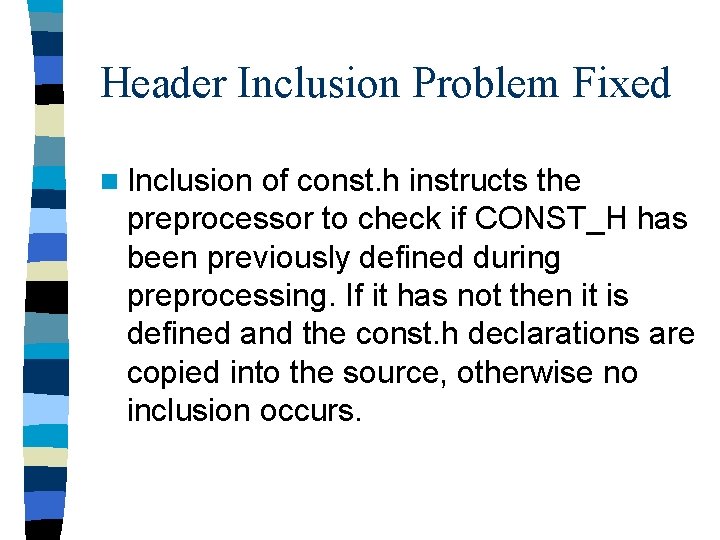
- Slides: 17
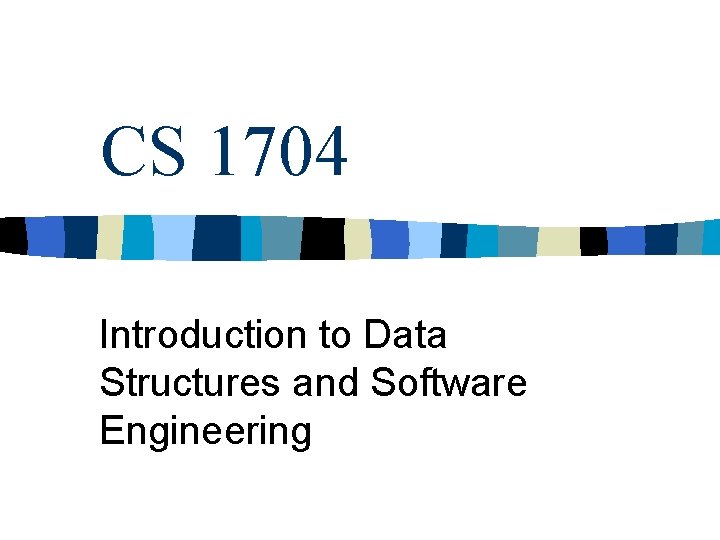
CS 1704 Introduction to Data Structures and Software Engineering
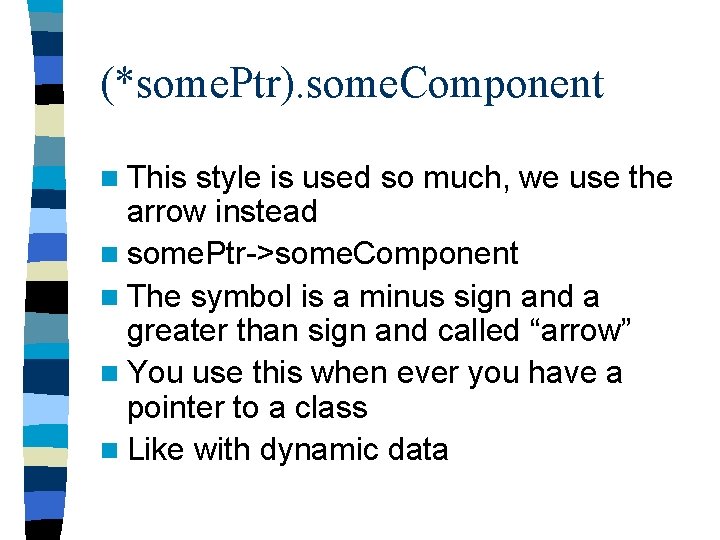
(*some. Ptr). some. Component n This style is used so much, we use the arrow instead n some. Ptr->some. Component n The symbol is a minus sign and a greater than sign and called “arrow” n You use this when ever you have a pointer to a class n Like with dynamic data
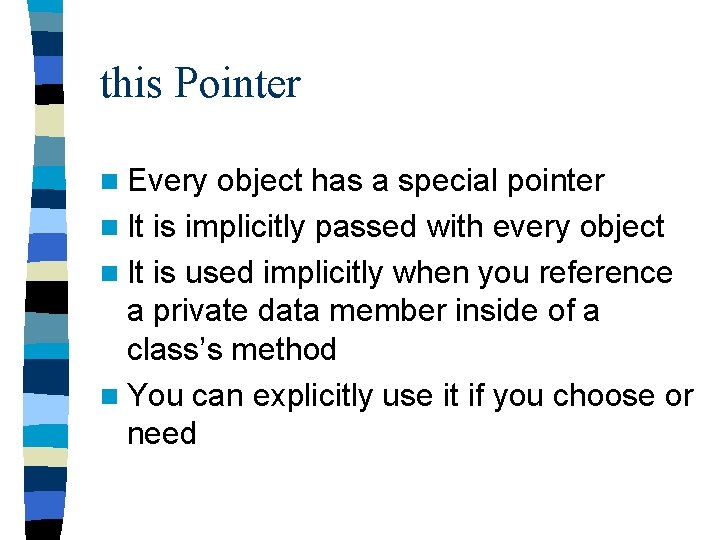
this Pointer n Every object has a special pointer n It is implicitly passed with every object n It is used implicitly when you reference a private data member inside of a class’s method n You can explicitly use it if you choose or need
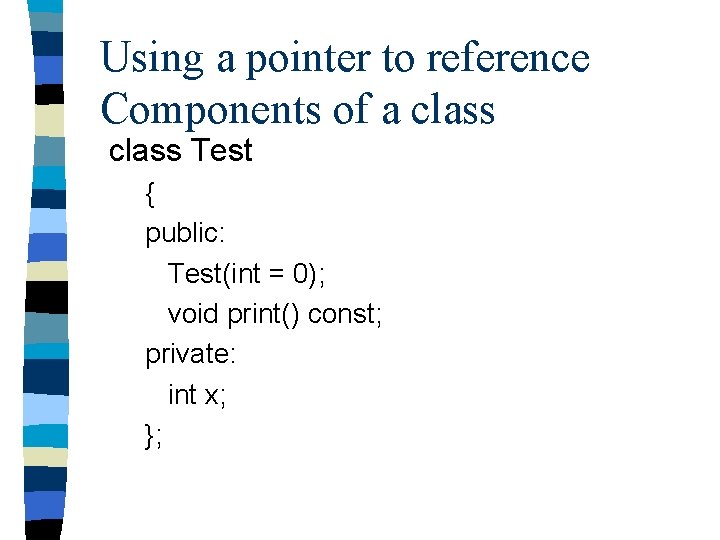
Using a pointer to reference Components of a class Test { public: Test(int = 0); void print() const; private: int x; };
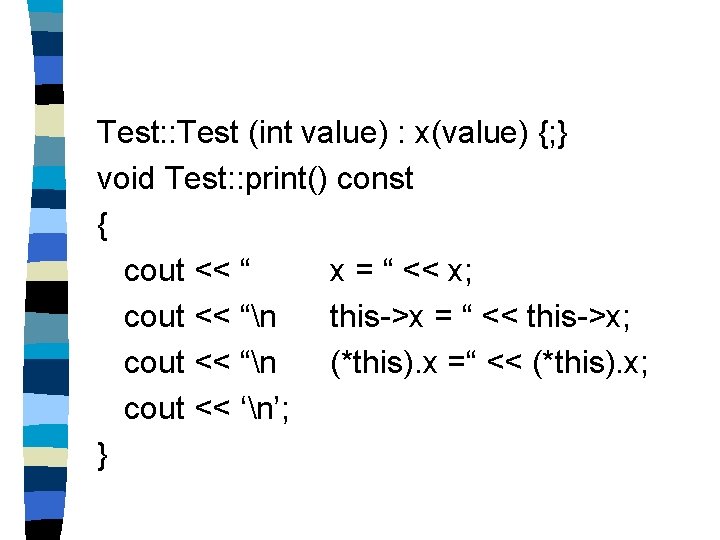
Test: : Test (int value) : x(value) {; } void Test: : print() const { cout << “ x = “ << x; cout << “n this->x = “ << this->x; cout << “n (*this). x =“ << (*this). x; cout << ‘n’; }
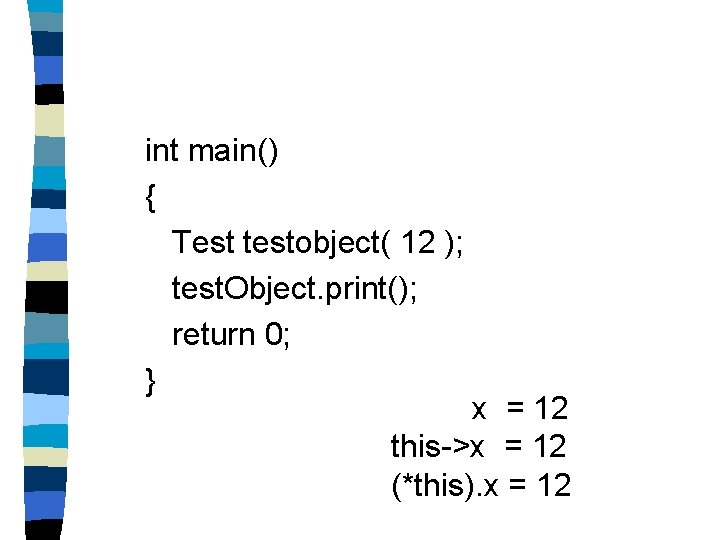
int main() { Test testobject( 12 ); test. Object. print(); return 0; } x = 12 this->x = 12 (*this). x = 12
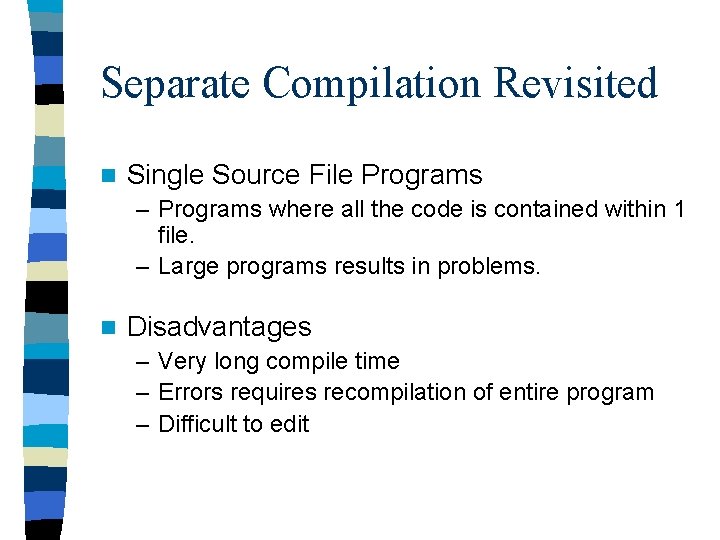
Separate Compilation Revisited n Single Source File Programs – Programs where all the code is contained within 1 file. – Large programs results in problems. n Disadvantages – Very long compile time – Errors requires recompilation of entire program – Difficult to edit
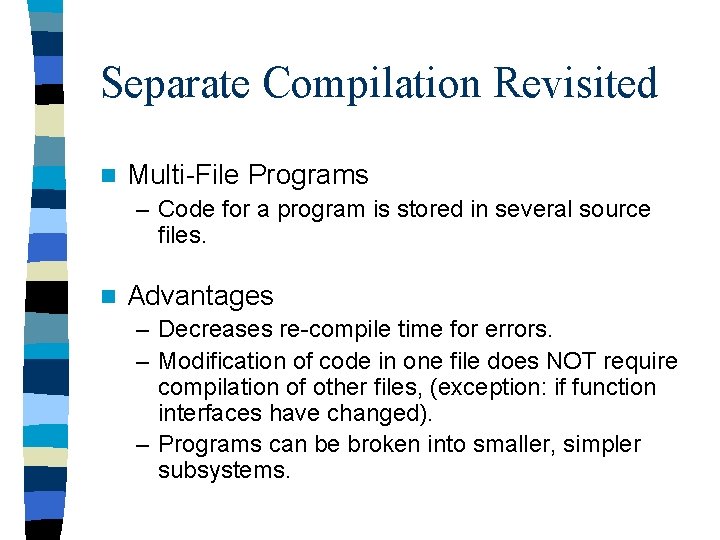
Separate Compilation Revisited n Multi-File Programs – Code for a program is stored in several source files. n Advantages – Decreases re-compile time for errors. – Modification of code in one file does NOT require compilation of other files, (exception: if function interfaces have changed). – Programs can be broken into smaller, simpler subsystems.
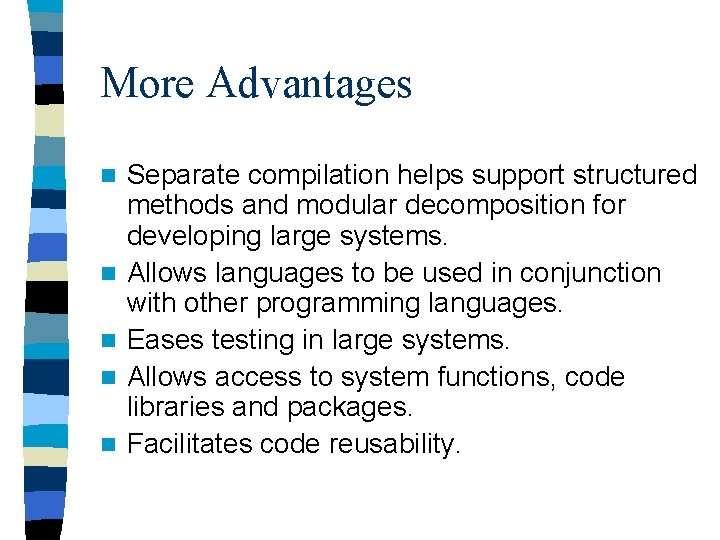
More Advantages n n n Separate compilation helps support structured methods and modular decomposition for developing large systems. Allows languages to be used in conjunction with other programming languages. Eases testing in large systems. Allows access to system functions, code libraries and packages. Facilitates code reusability.
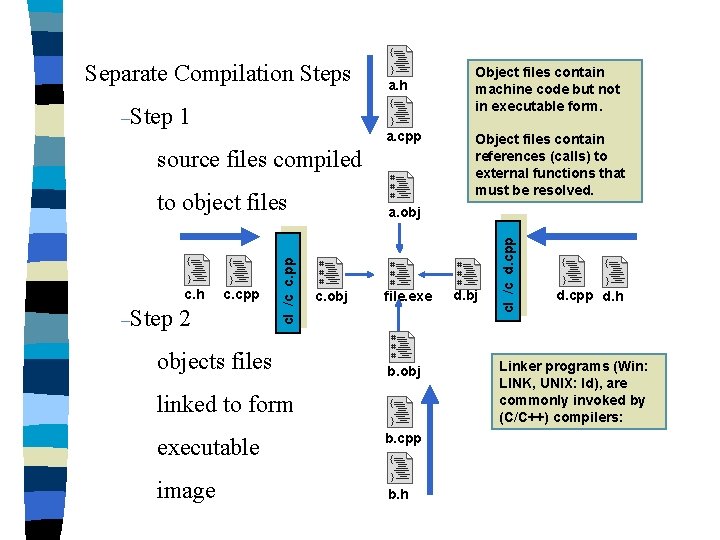
Separate Compilation Steps 1 a. cpp source files compiled c. h –Step c. cpp 2 cl /c c. pp to object files objects files image Object files contain references (calls) to external functions that must be resolved. a. obj c. obj file. exe b. obj linked to form executable Object files contain machine code but not in executable form. b. cpp b. h d. bj cl /c d. cpp –Step a. h d. cpp d. h Linker programs (Win: LINK, UNIX: ld), are commonly invoked by (C/C++) compilers:
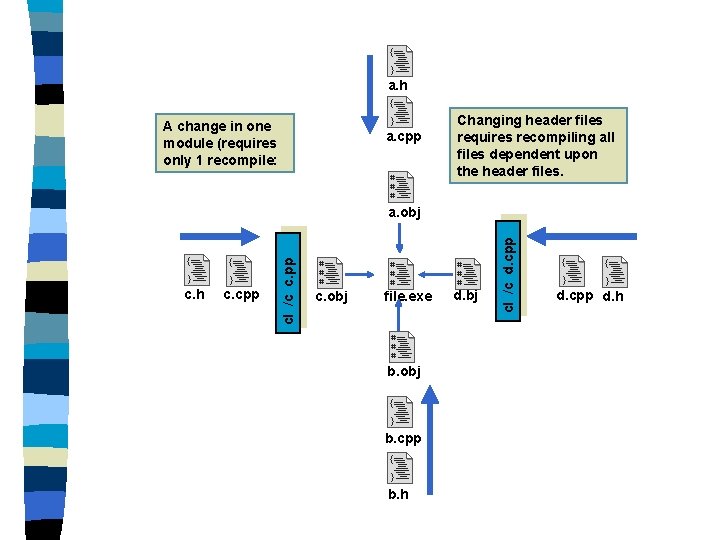
a. h A change in one module (requires only 1 recompile: a. cpp Changing header files requires recompiling all files dependent upon the header files. c. cpp c. obj file. exe b. obj b. cpp b. h d. bj cl /c d. cpp c. h cl /c c. pp a. obj d. cpp d. h
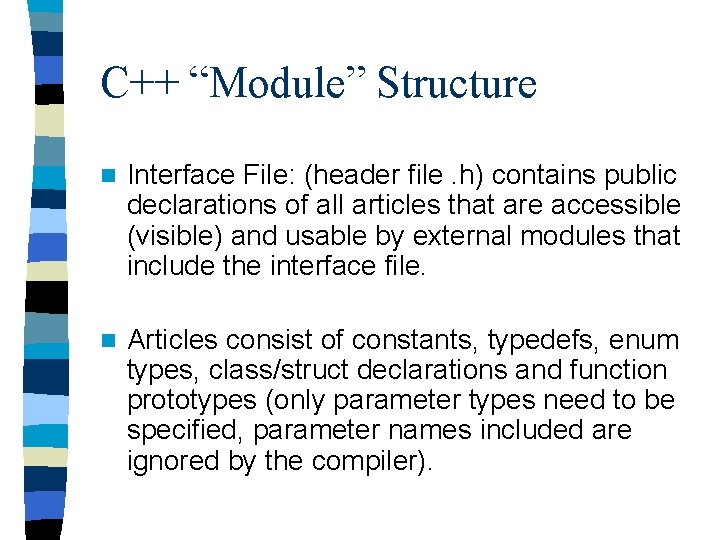
C++ “Module” Structure n Interface File: (header file. h) contains public declarations of all articles that are accessible (visible) and usable by external modules that include the interface file. n Articles consist of constants, typedefs, enum types, class/struct declarations and function prototypes (only parameter types need to be specified, parameter names included are ignored by the compiler).
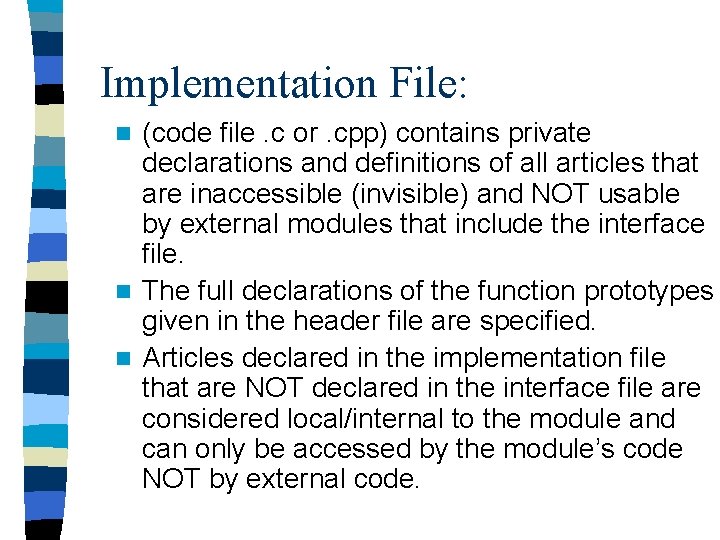
Implementation File: (code file. c or. cpp) contains private declarations and definitions of all articles that are inaccessible (invisible) and NOT usable by external modules that include the interface file. n The full declarations of the function prototypes given in the header file are specified. n Articles declared in the implementation file that are NOT declared in the interface file are considered local/internal to the module and can only be accessed by the module’s code NOT by external code. n
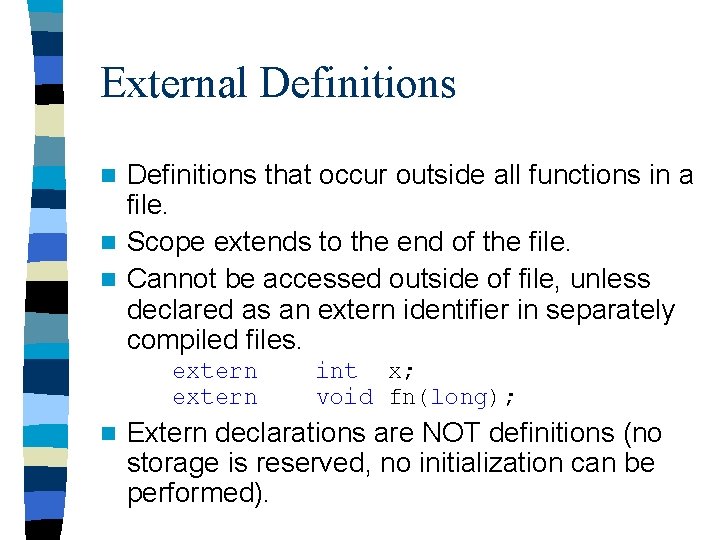
External Definitions that occur outside all functions in a file. n Scope extends to the end of the file. n Cannot be accessed outside of file, unless declared as an extern identifier in separately compiled files. n extern n int x; void fn(long); Extern declarations are NOT definitions (no storage is reserved, no initialization can be performed).
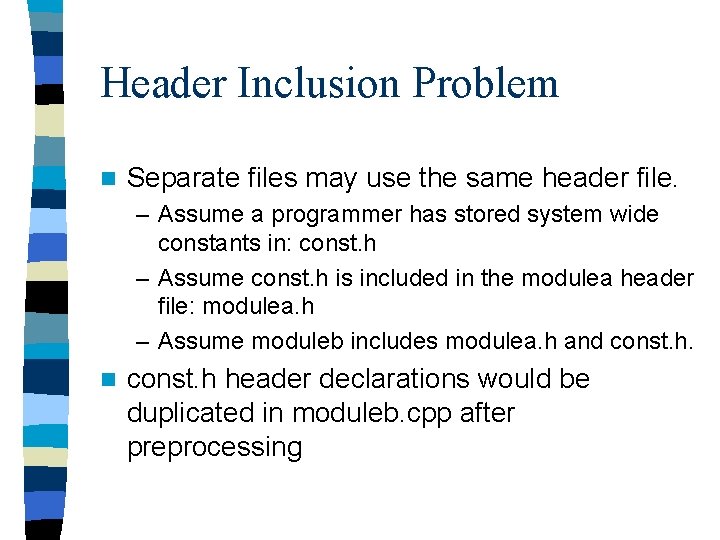
Header Inclusion Problem n Separate files may use the same header file. – Assume a programmer has stored system wide constants in: const. h – Assume const. h is included in the modulea header file: modulea. h – Assume moduleb includes modulea. h and const. h. n const. h header declarations would be duplicated in moduleb. cpp after preprocessing
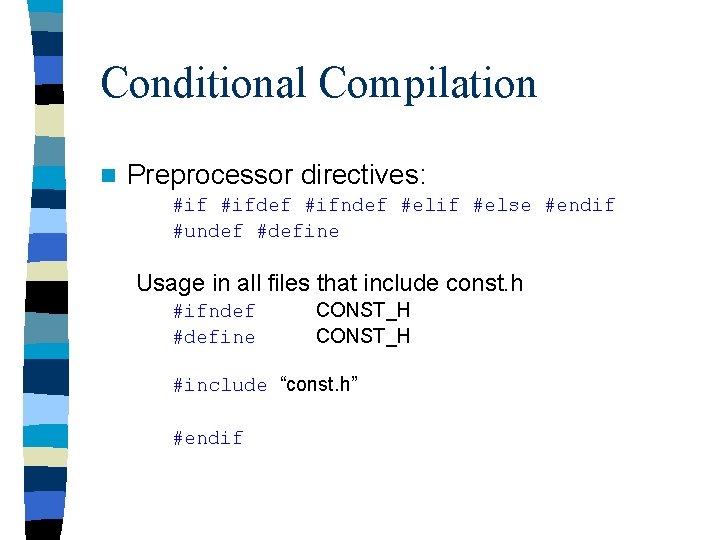
Conditional Compilation n Preprocessor directives: #ifdef #ifndef #elif #else #endif #undef #define Usage in all files that include const. h #ifndef #define CONST_H #include “const. h” #endif
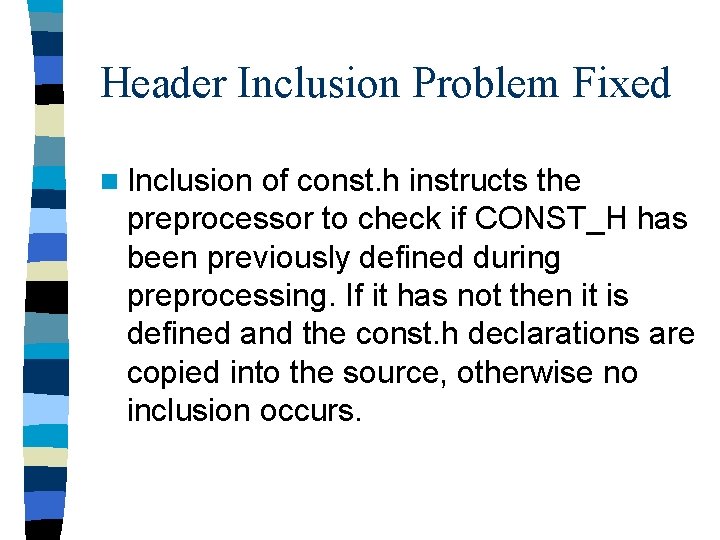
Header Inclusion Problem Fixed n Inclusion of const. h instructs the preprocessor to check if CONST_H has been previously defined during preprocessing. If it has not then it is defined and the const. h declarations are copied into the source, otherwise no inclusion occurs.