Control Structures Topics to cover here n n
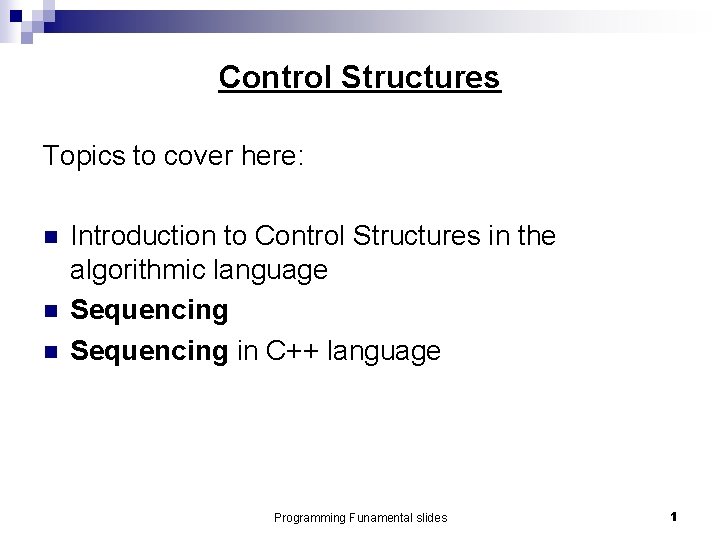
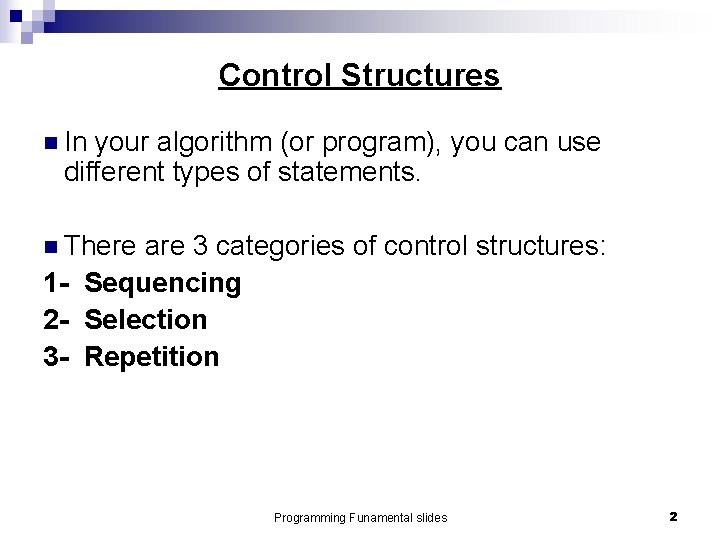
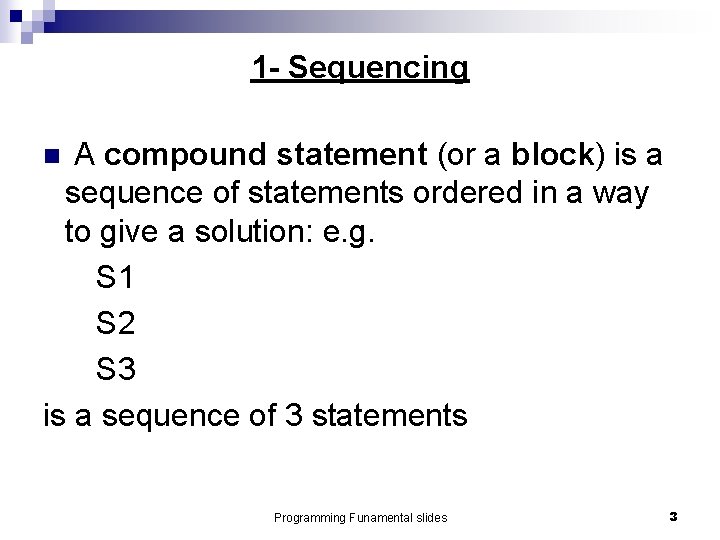
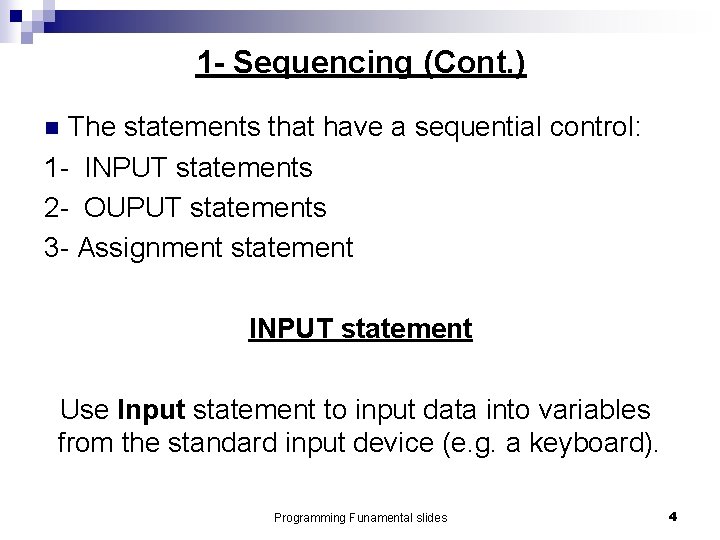
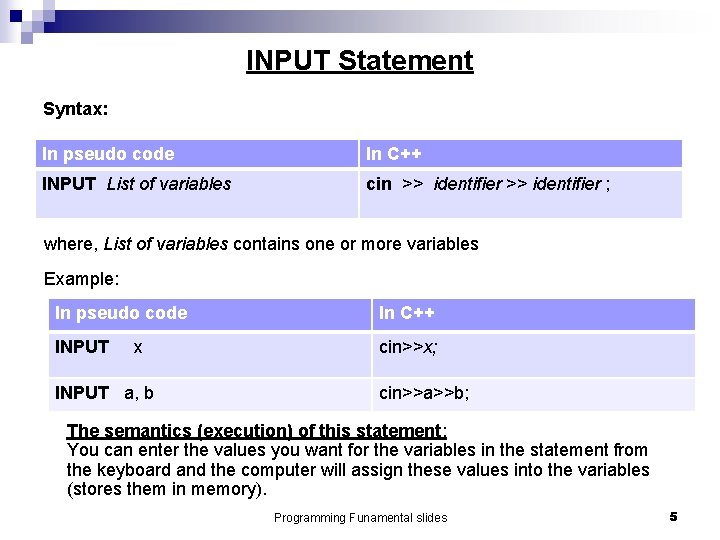
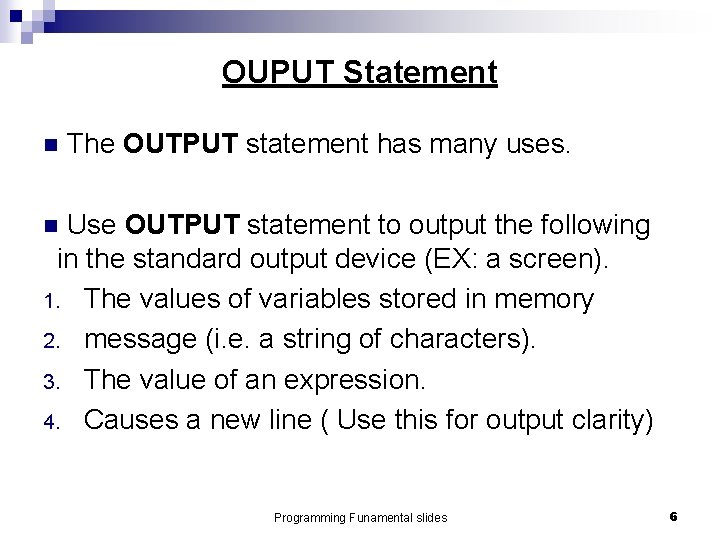
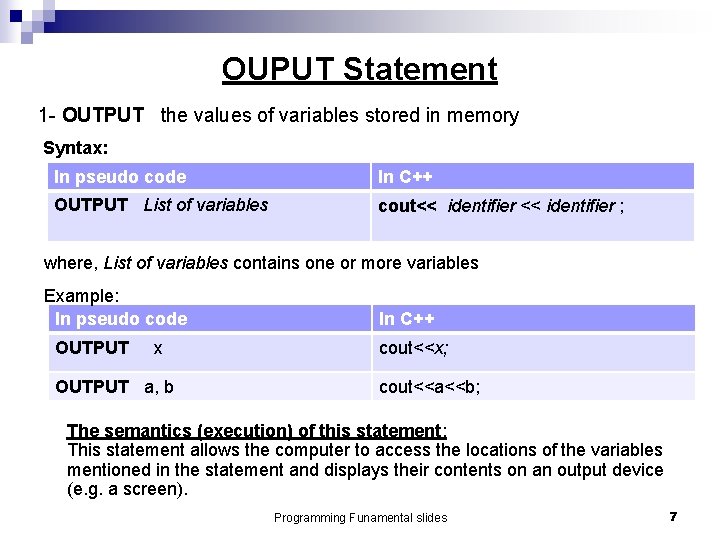
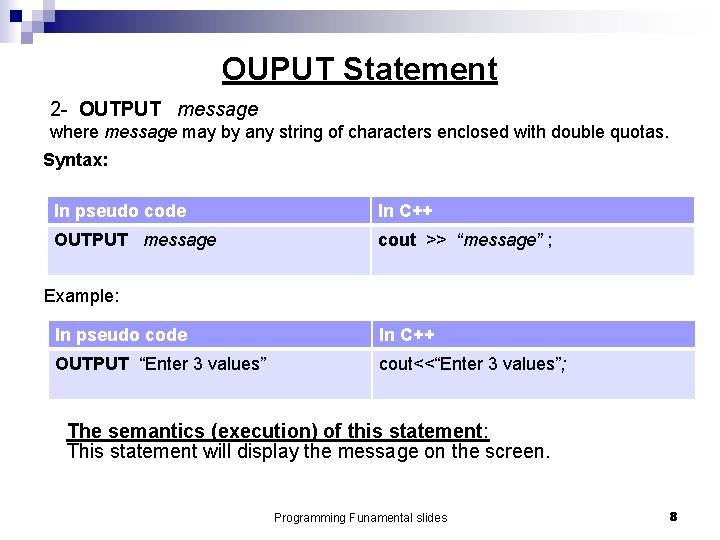
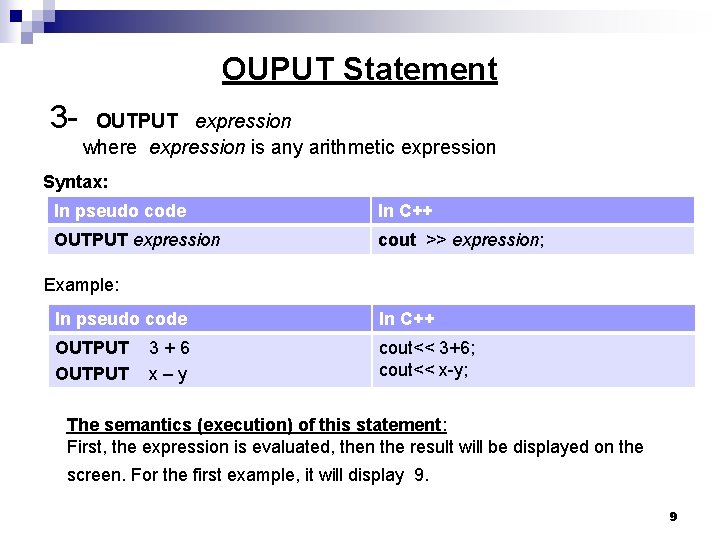
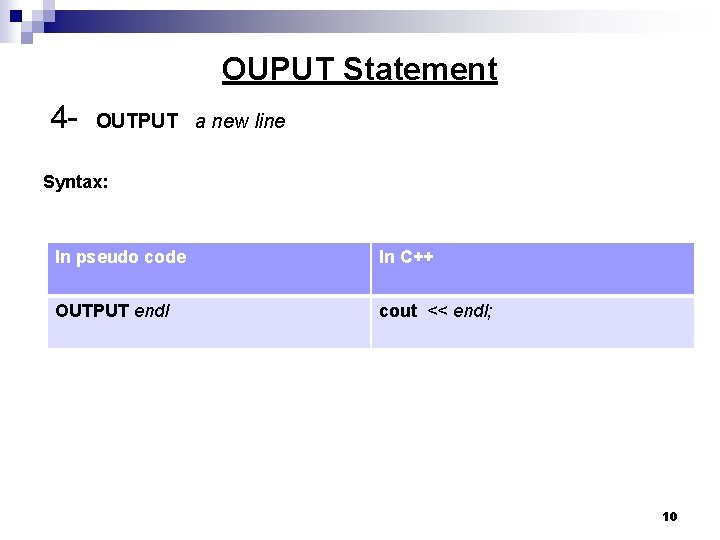
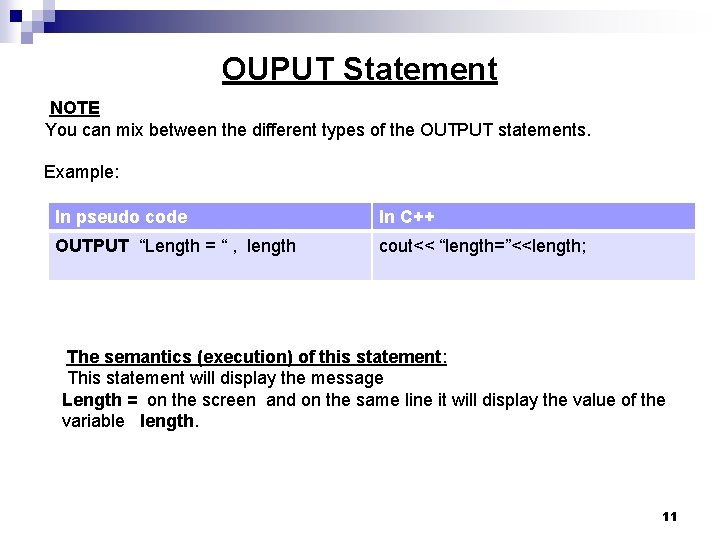
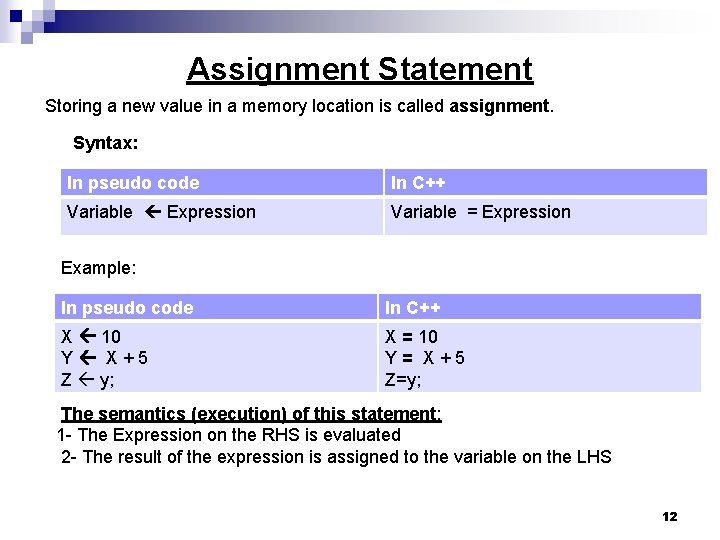
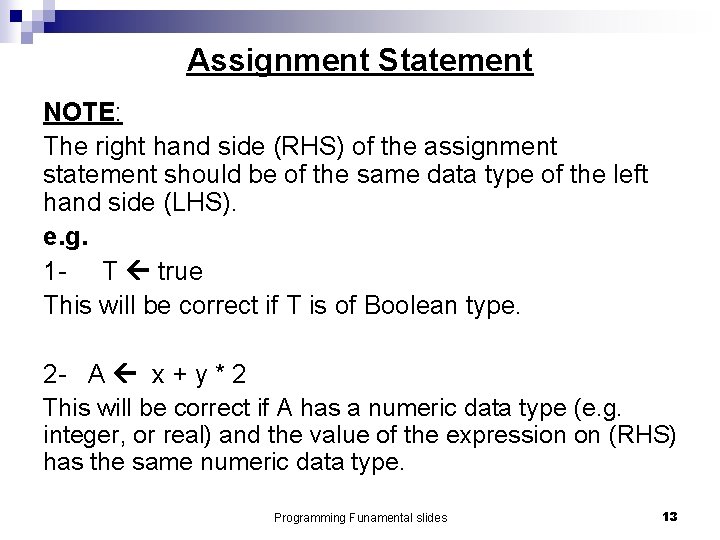
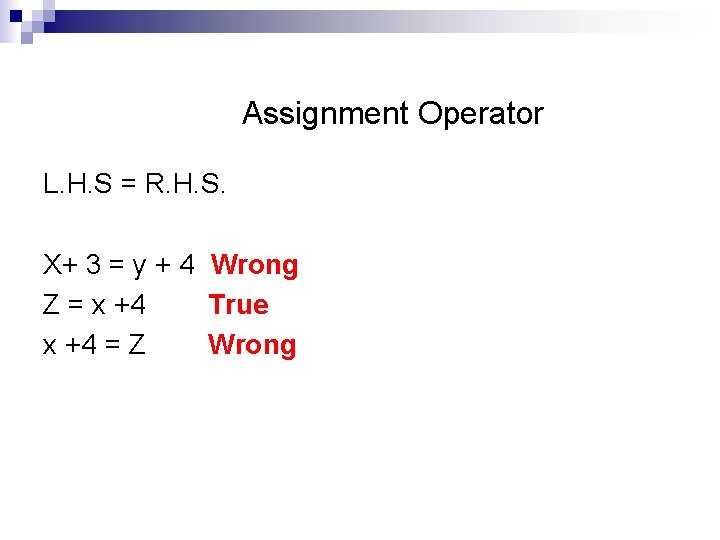
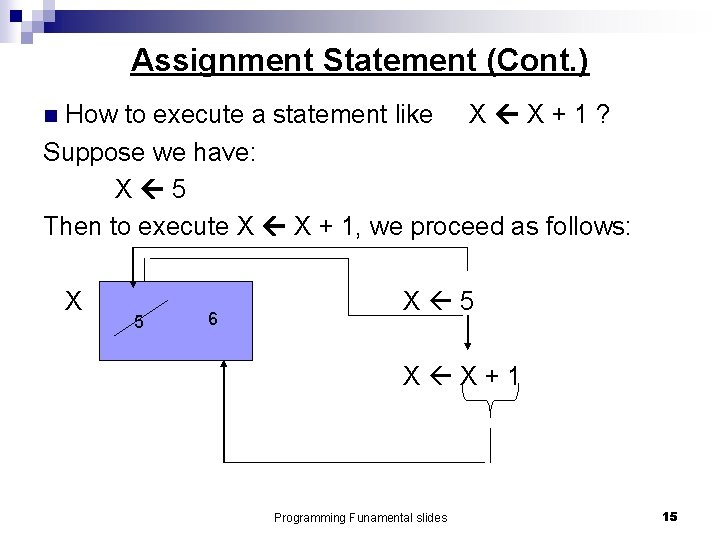
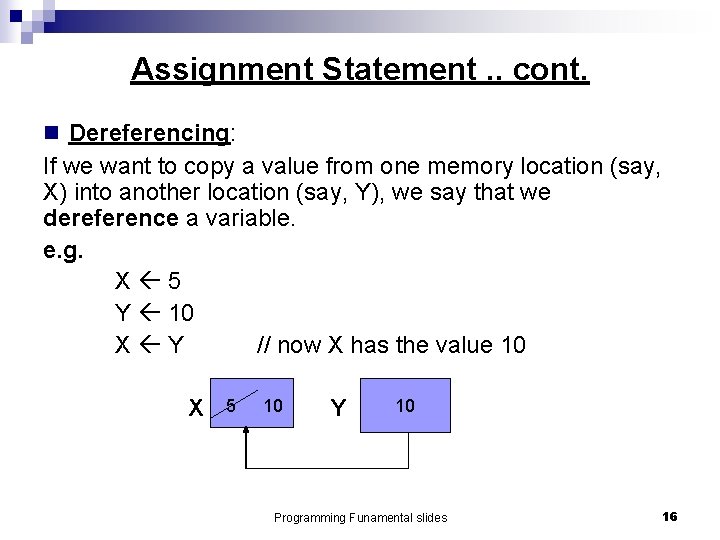
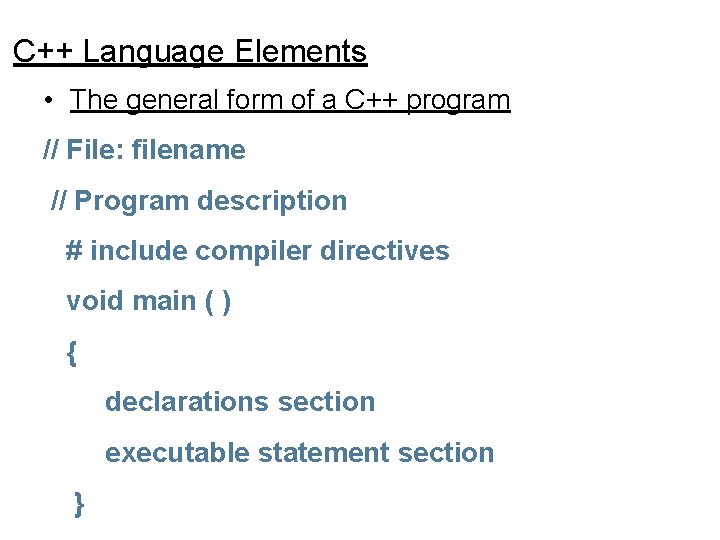
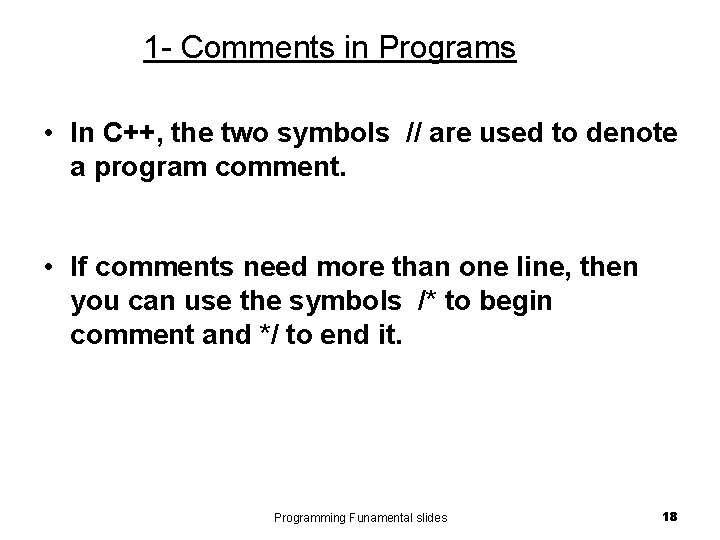
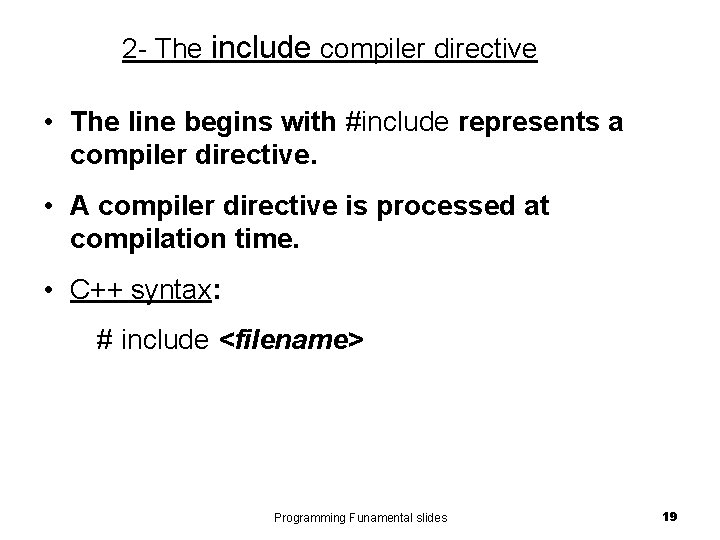
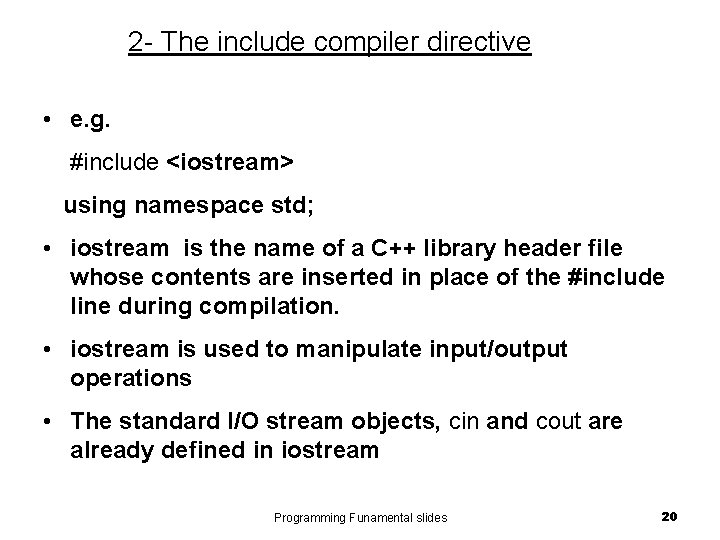
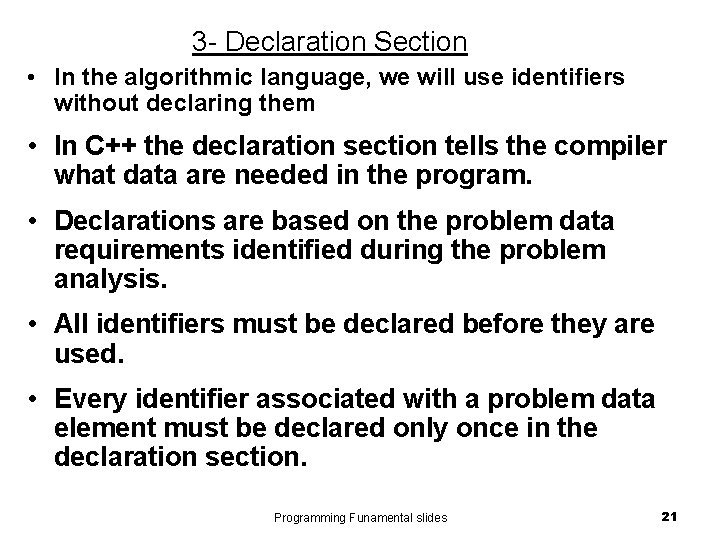
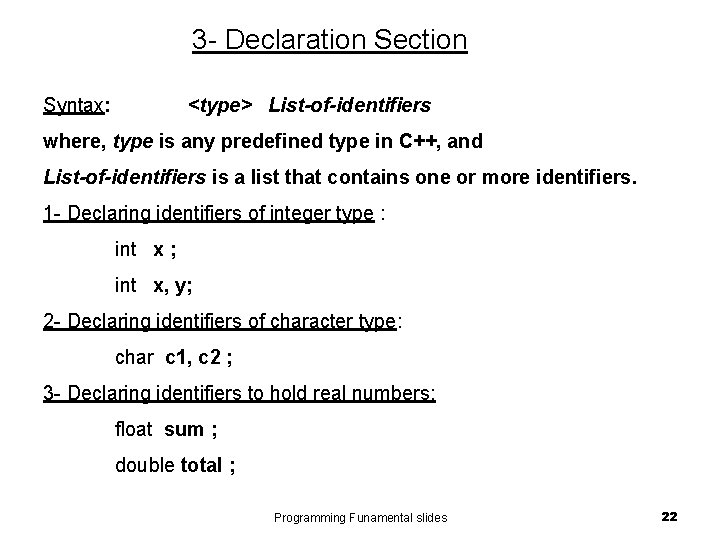
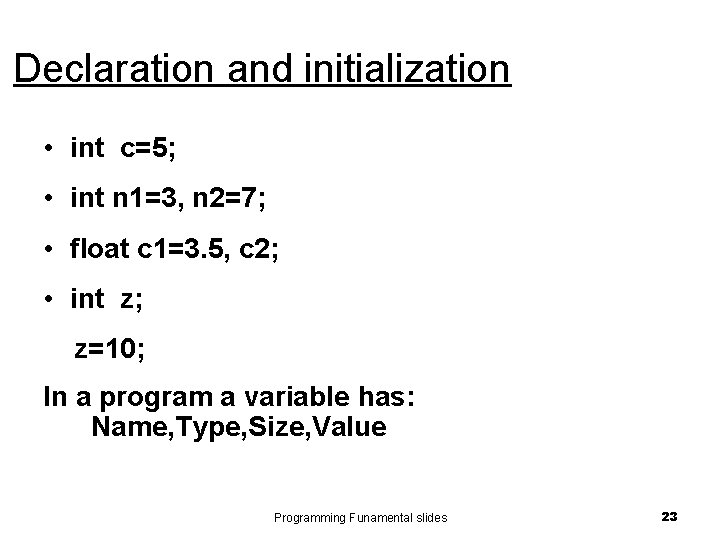
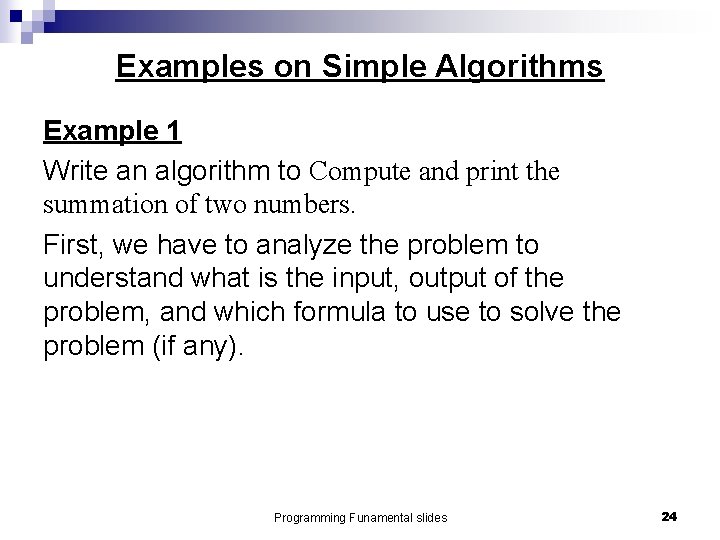
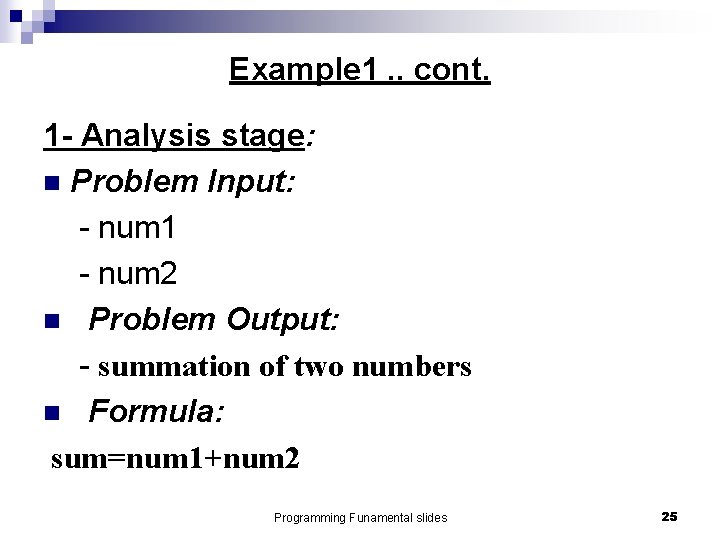
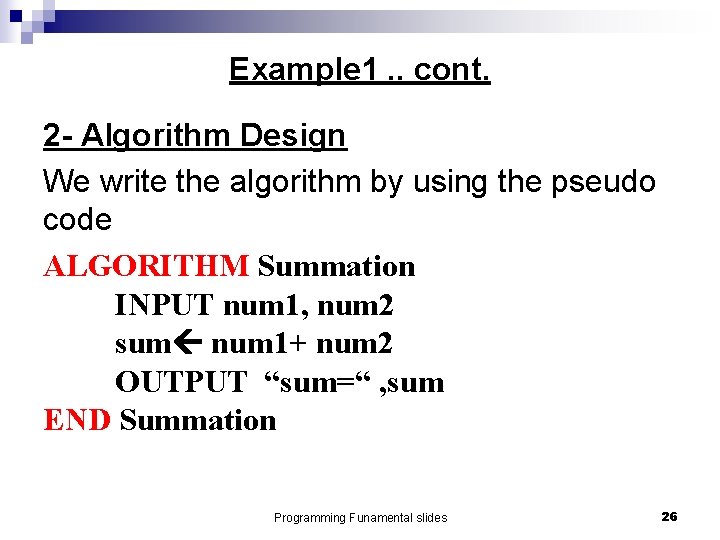
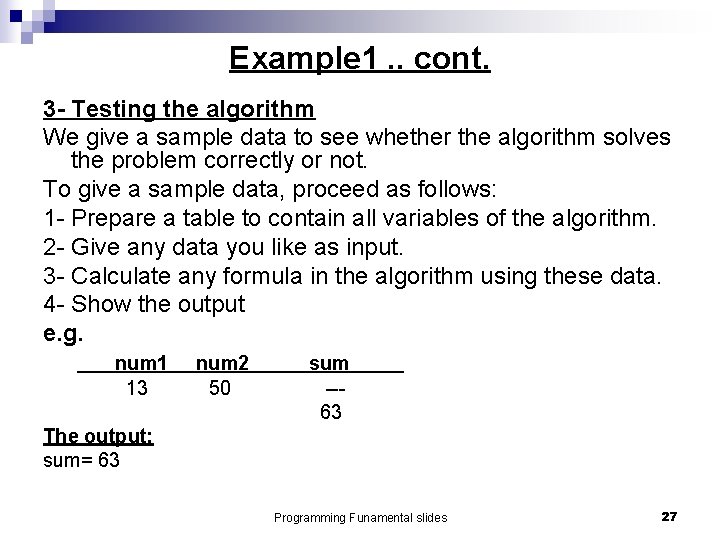
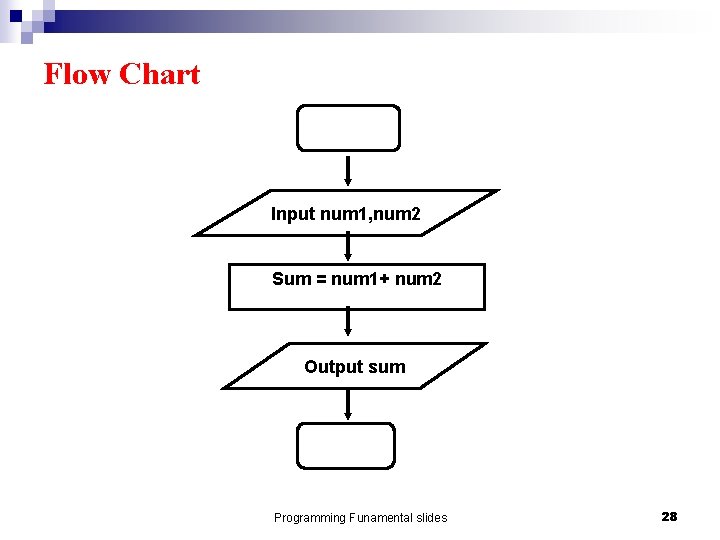
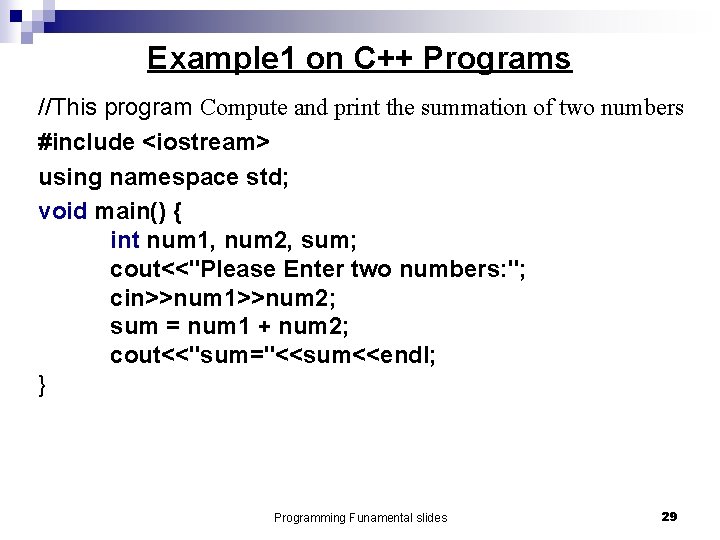
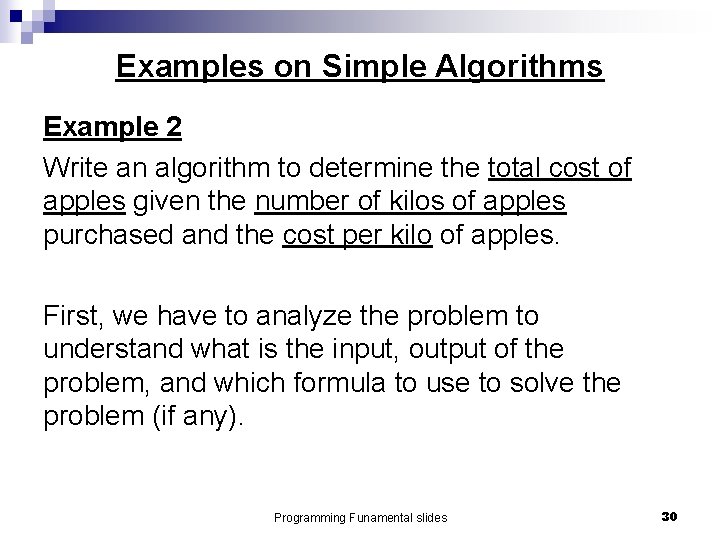
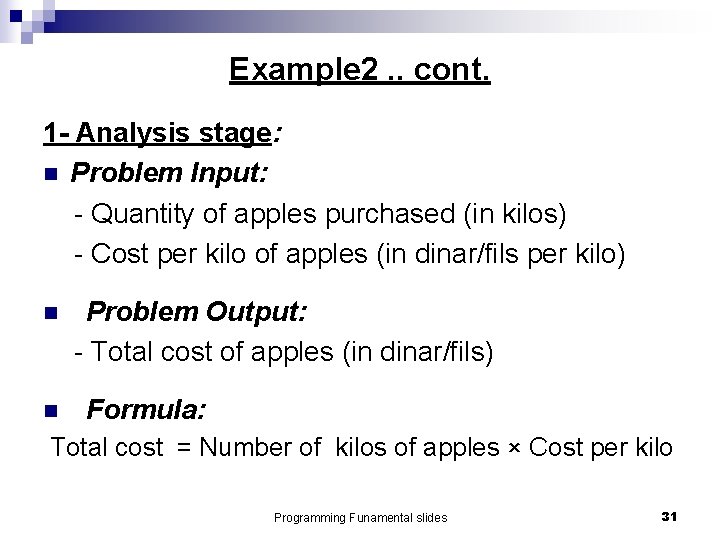
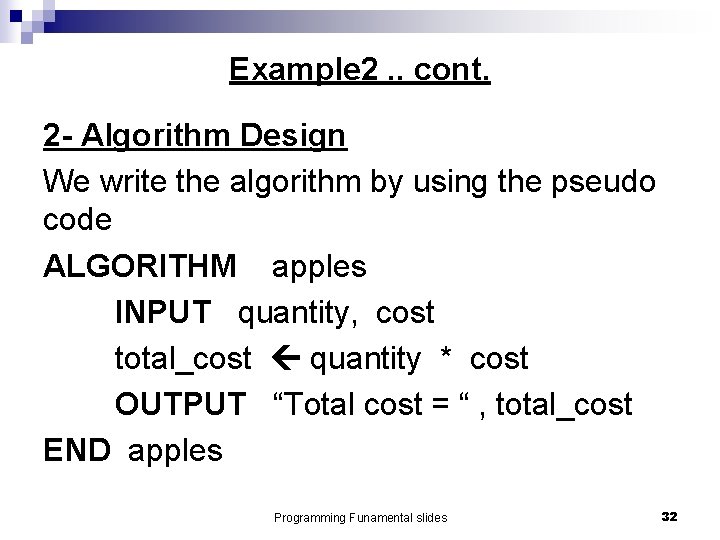
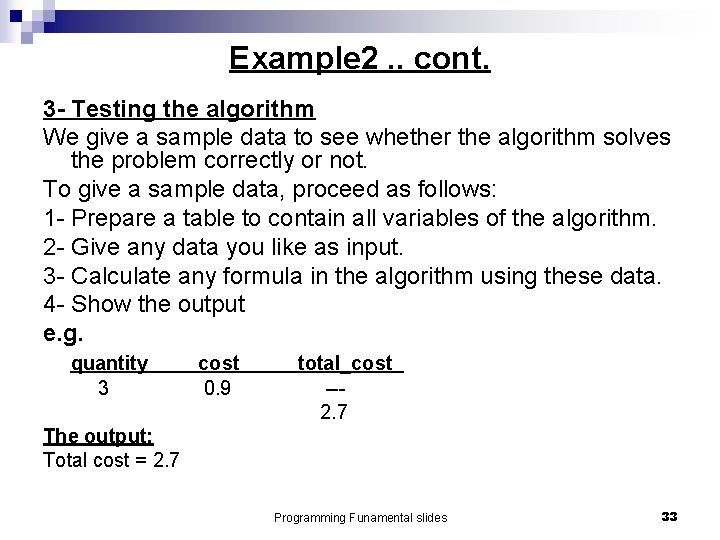
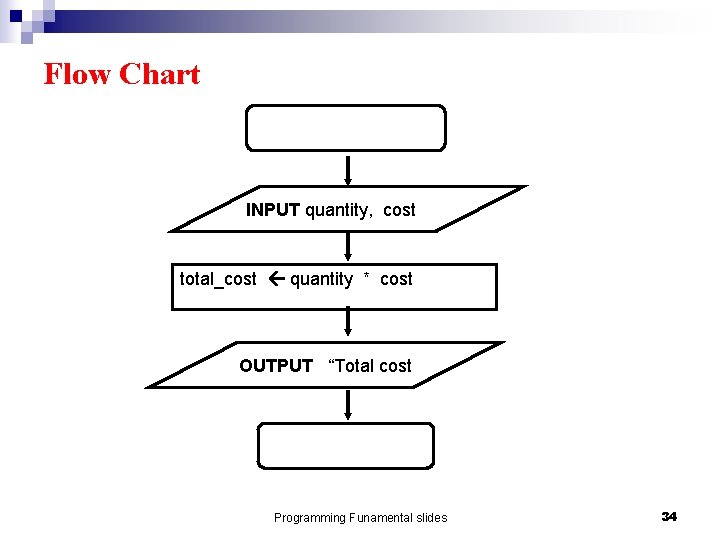
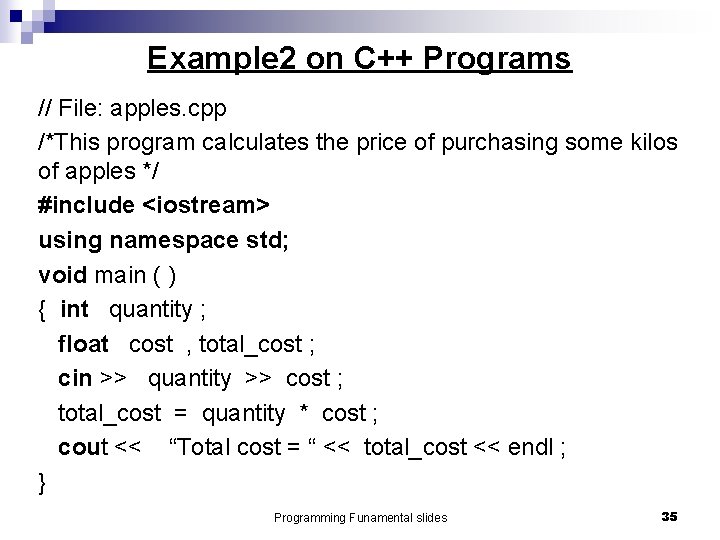
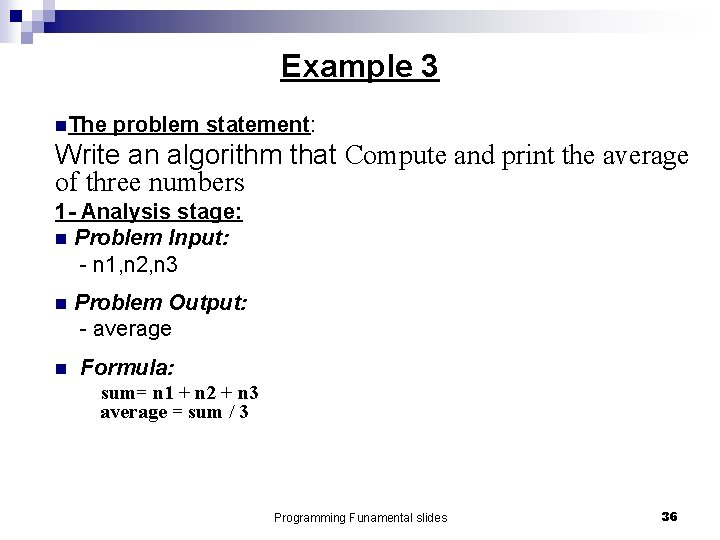
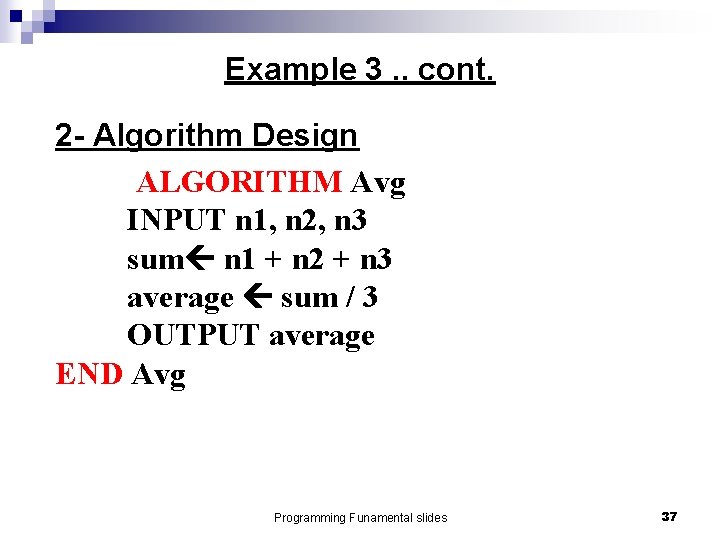
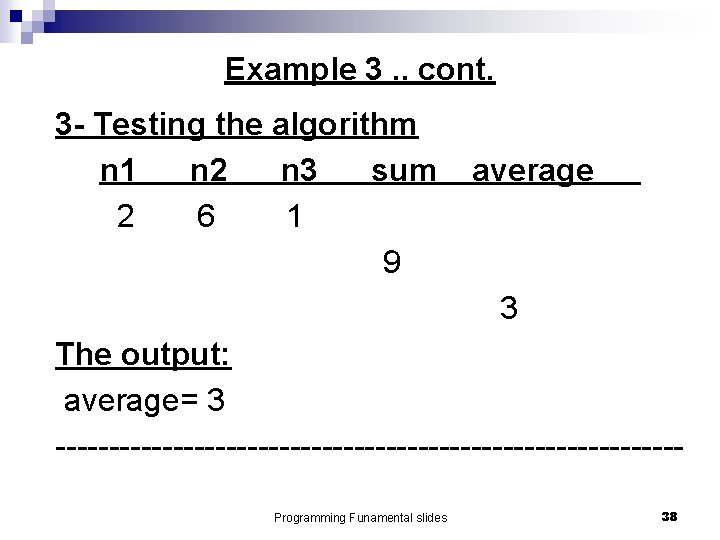
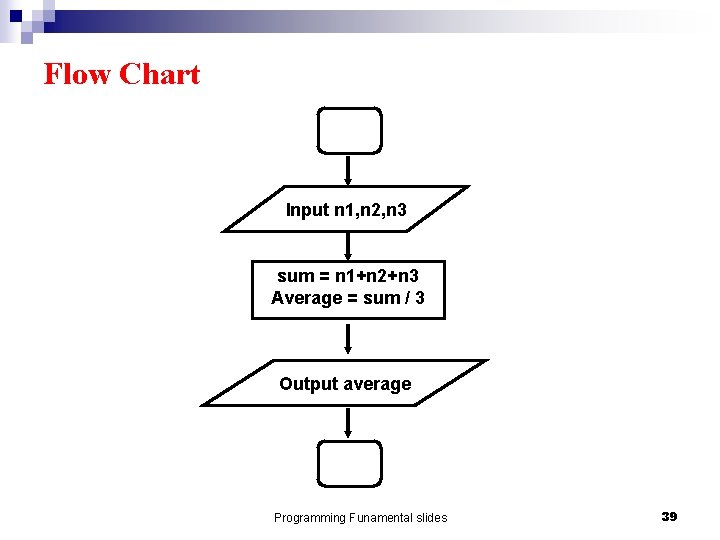
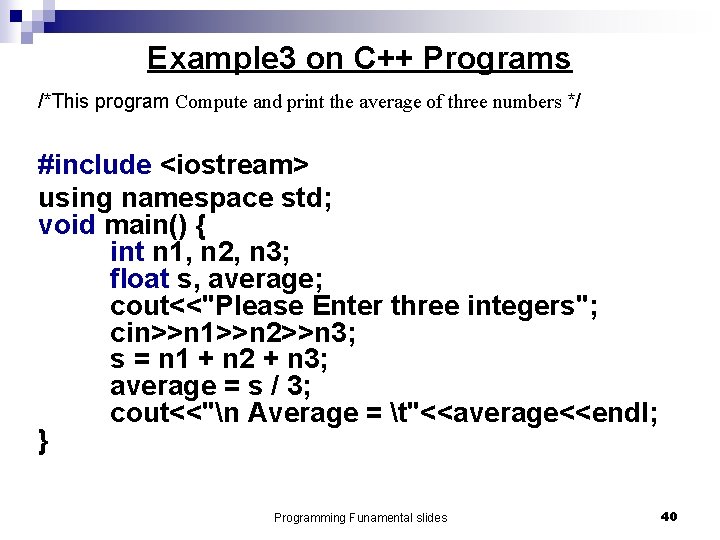
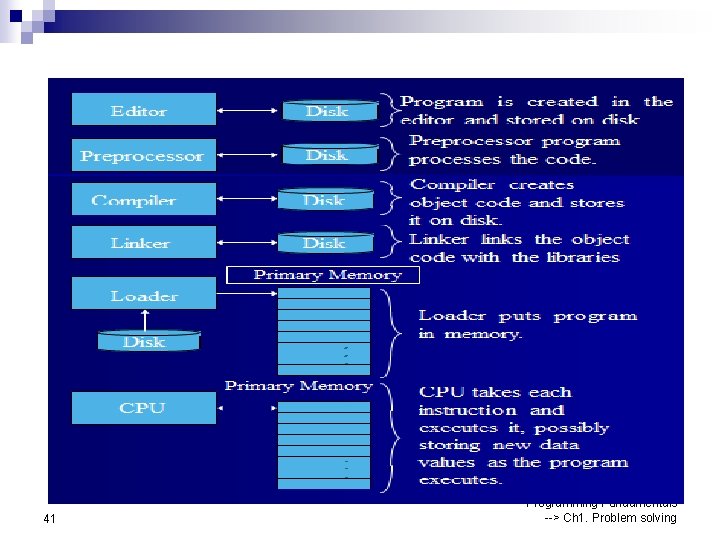
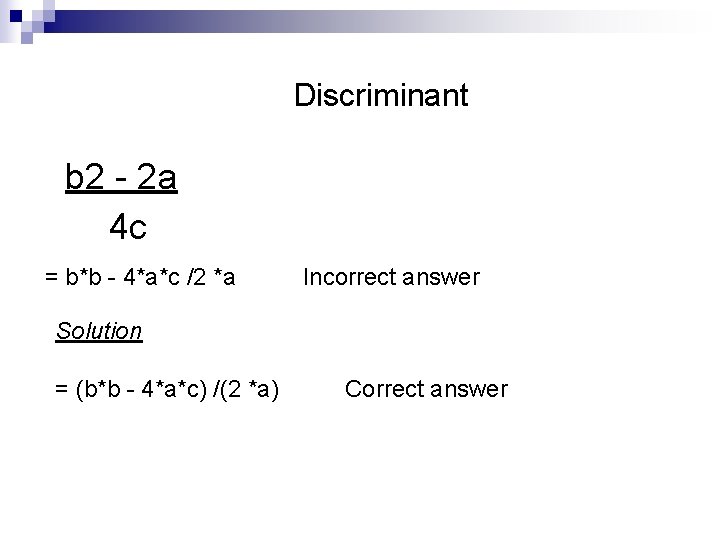
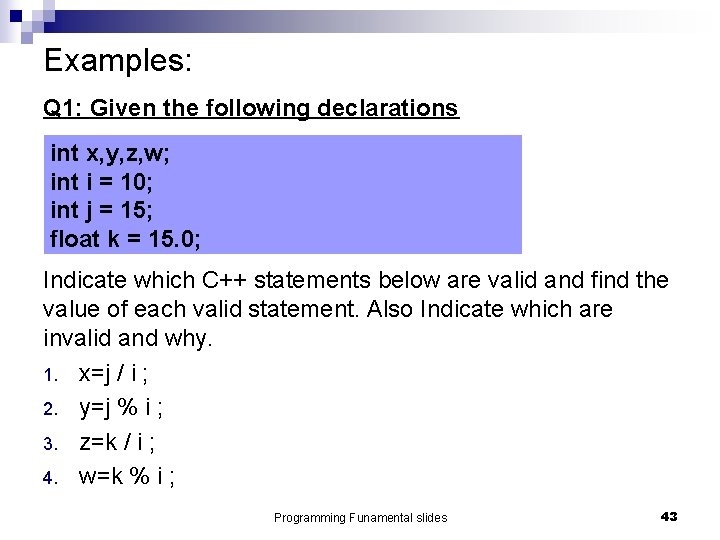
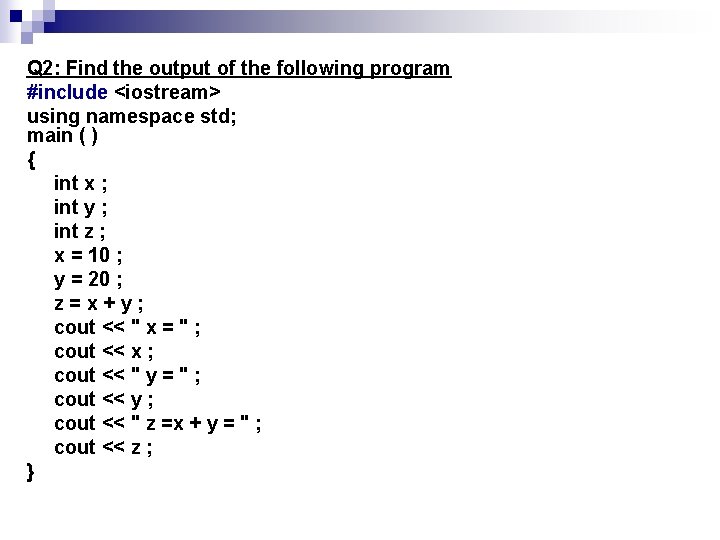
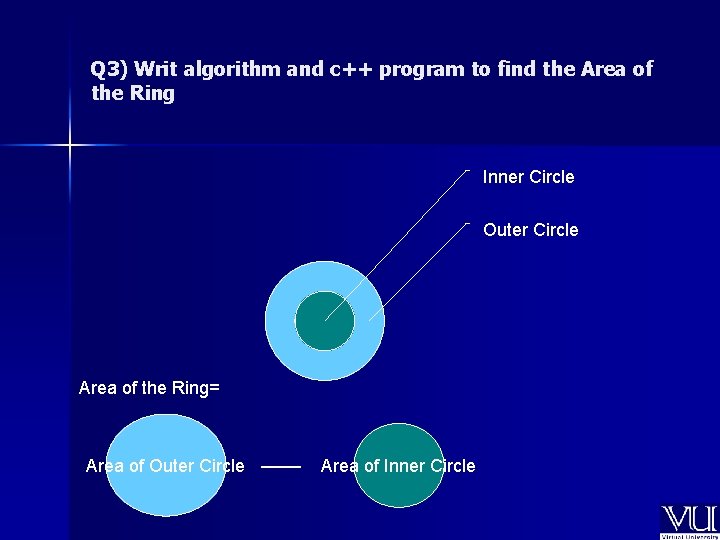
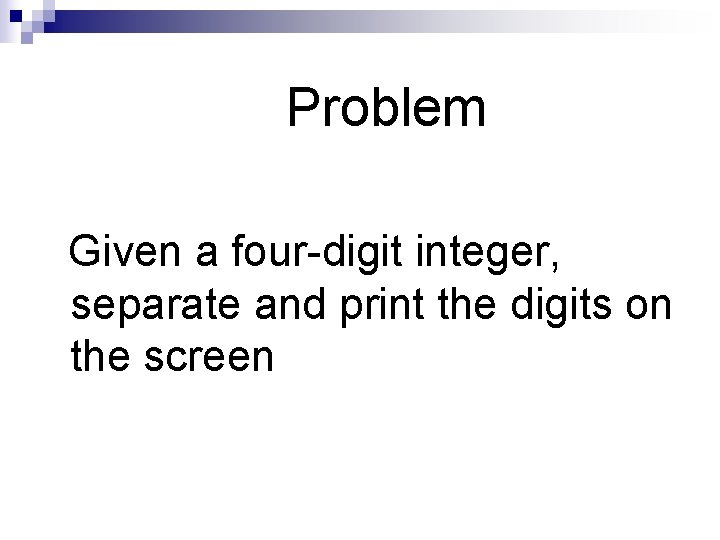
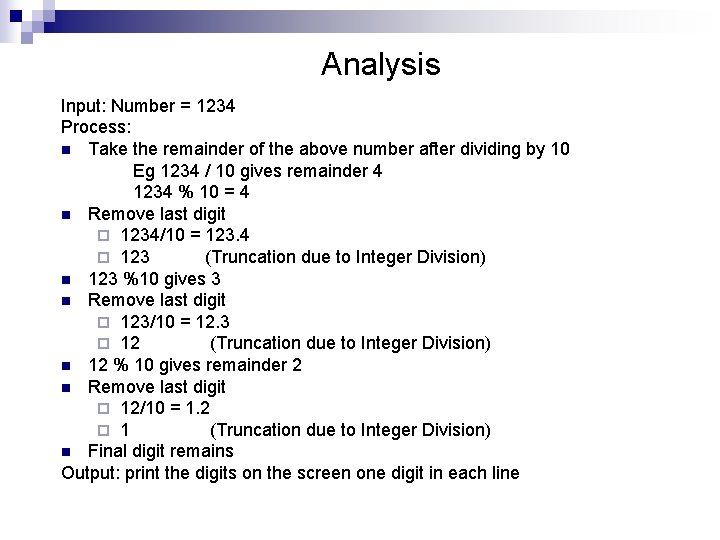
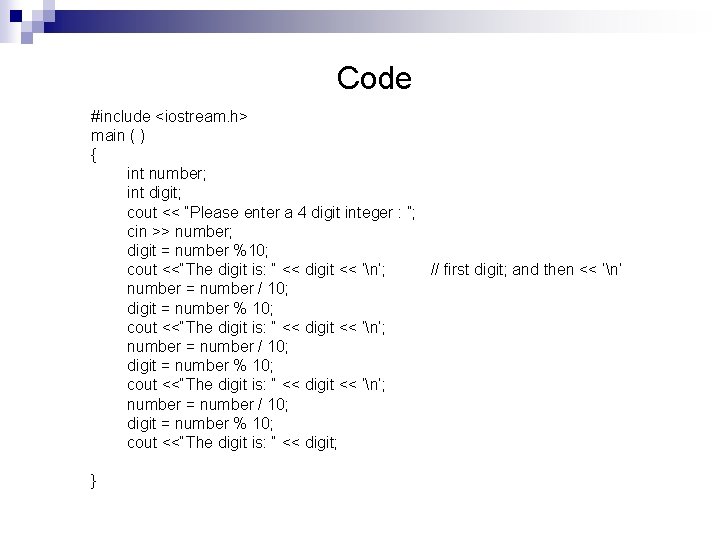
- Slides: 48
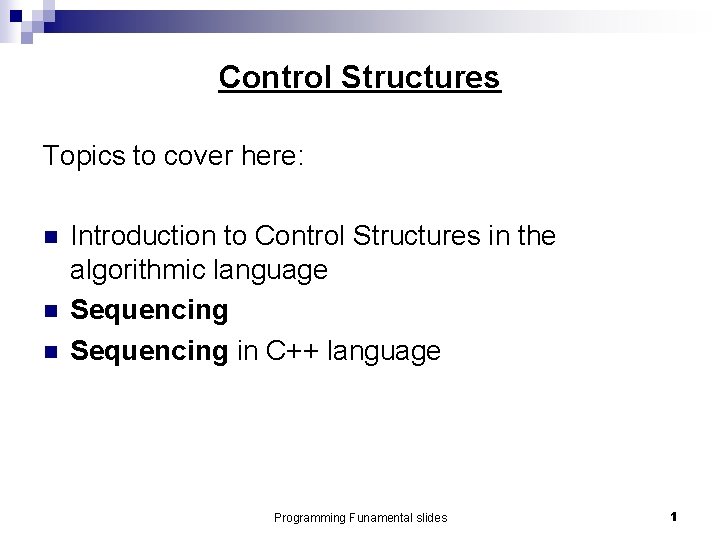
Control Structures Topics to cover here: n n n Introduction to Control Structures in the algorithmic language Sequencing in C++ language Programming Funamental slides 1
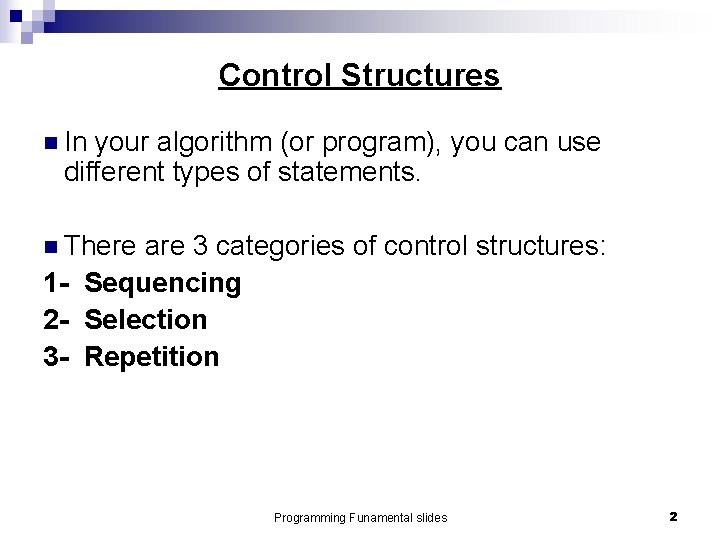
Control Structures n In your algorithm (or program), you can use different types of statements. n There are 3 categories of control structures: 1 - Sequencing 2 - Selection 3 - Repetition Programming Funamental slides 2
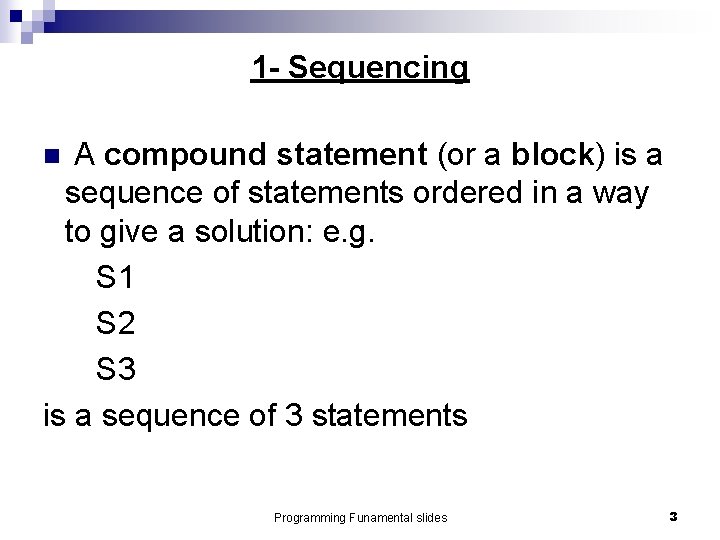
1 - Sequencing A compound statement (or a block) is a sequence of statements ordered in a way to give a solution: e. g. S 1 S 2 S 3 is a sequence of 3 statements n Programming Funamental slides 3
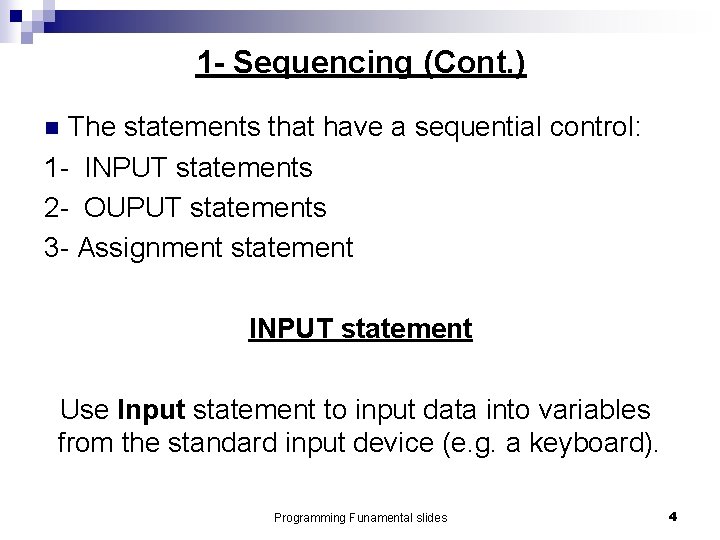
1 - Sequencing (Cont. ) The statements that have a sequential control: 1 - INPUT statements 2 - OUPUT statements 3 - Assignment statement n INPUT statement Use Input statement to input data into variables from the standard input device (e. g. a keyboard). Programming Funamental slides 4
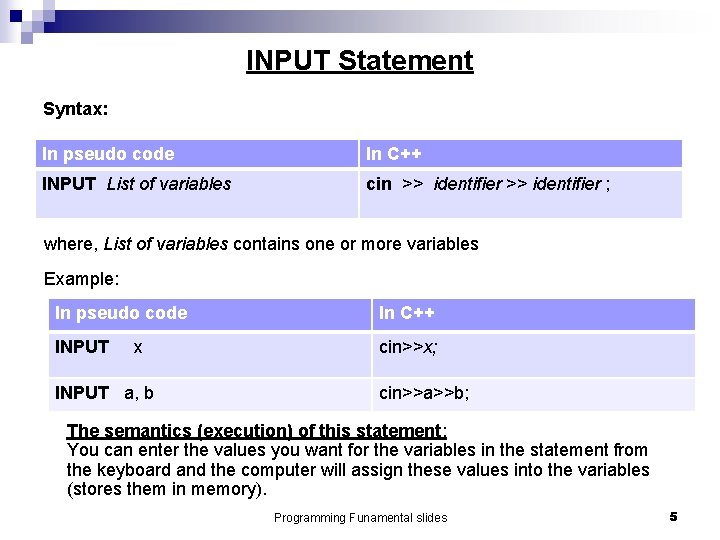
INPUT Statement Syntax: In pseudo code In C++ INPUT List of variables cin >> identifier ; where, List of variables contains one or more variables Example: In pseudo code In C++ INPUT cin>>x; x INPUT a, b cin>>a>>b; The semantics (execution) of this statement: You can enter the values you want for the variables in the statement from the keyboard and the computer will assign these values into the variables (stores them in memory). Programming Funamental slides 5
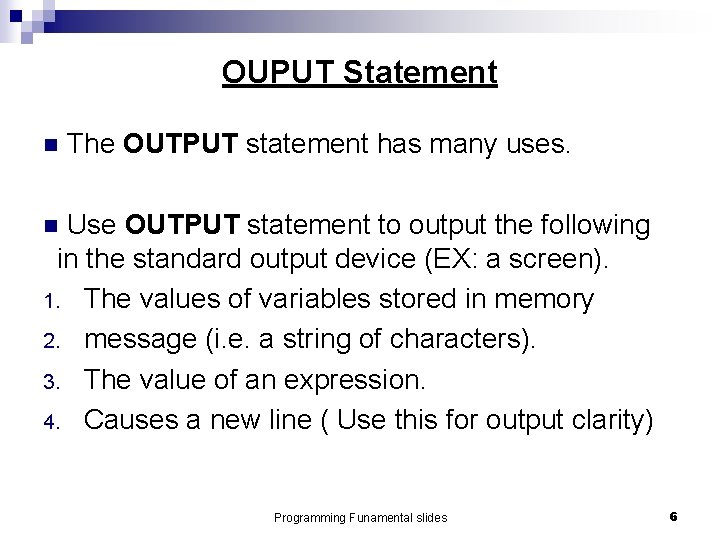
OUPUT Statement n The OUTPUT statement has many uses. Use OUTPUT statement to output the following in the standard output device (EX: a screen). 1. The values of variables stored in memory 2. message (i. e. a string of characters). 3. The value of an expression. 4. Causes a new line ( Use this for output clarity) n Programming Funamental slides 6
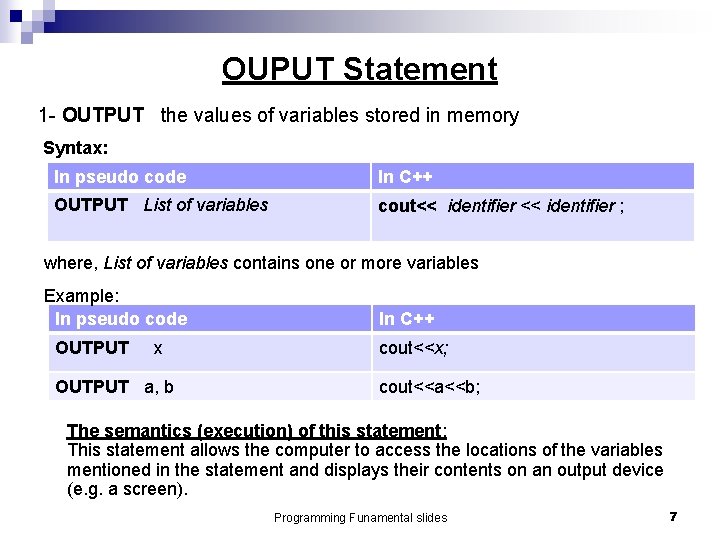
OUPUT Statement 1 - OUTPUT the values of variables stored in memory Syntax: In pseudo code In C++ OUTPUT List of variables cout<< identifier ; where, List of variables contains one or more variables Example: In pseudo code OUTPUT x OUTPUT a, b In C++ cout<<x; cout<<a<<b; The semantics (execution) of this statement: This statement allows the computer to access the locations of the variables mentioned in the statement and displays their contents on an output device (e. g. a screen). Programming Funamental slides 7
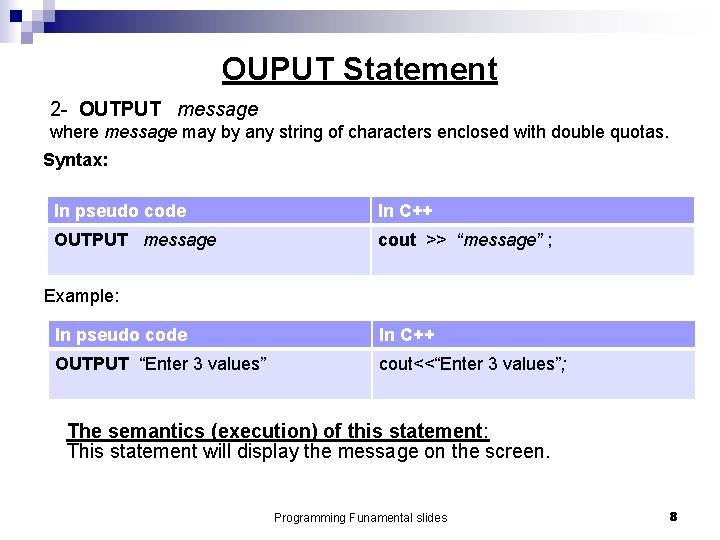
OUPUT Statement 2 - OUTPUT message where message may by any string of characters enclosed with double quotas. Syntax: In pseudo code In C++ OUTPUT message cout >> “message” ; Example: In pseudo code In C++ OUTPUT “Enter 3 values” cout<<“Enter 3 values”; The semantics (execution) of this statement: This statement will display the message on the screen. Programming Funamental slides 8
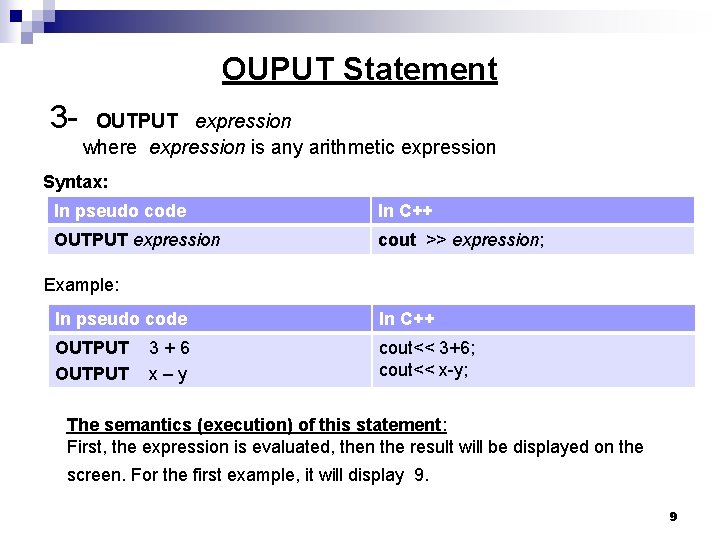
OUPUT Statement 3 - OUTPUT expression where expression is any arithmetic expression Syntax: In pseudo code In C++ OUTPUT expression cout >> expression; Example: In pseudo code In C++ OUTPUT cout<< 3+6; cout<< x-y; 3+6 x–y The semantics (execution) of this statement: First, the expression is evaluated, then the result will be displayed on the screen. For the first example, it will display 9. 9
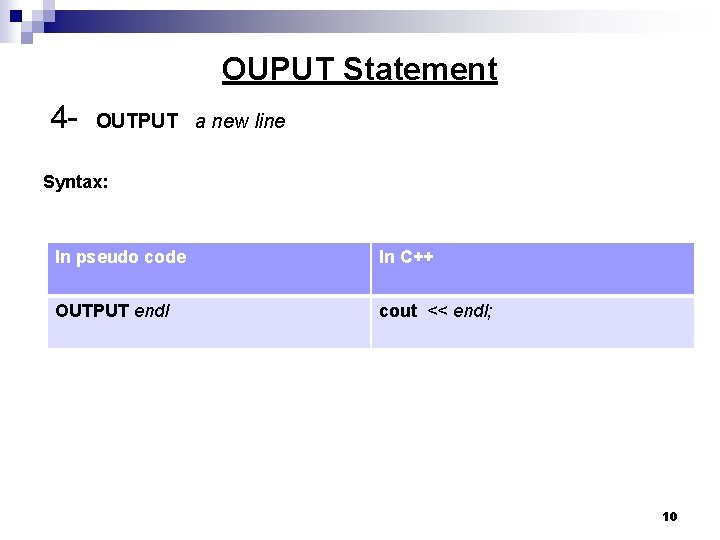
OUPUT Statement 4 - OUTPUT a new line Syntax: In pseudo code In C++ OUTPUT endl cout << endl; 10
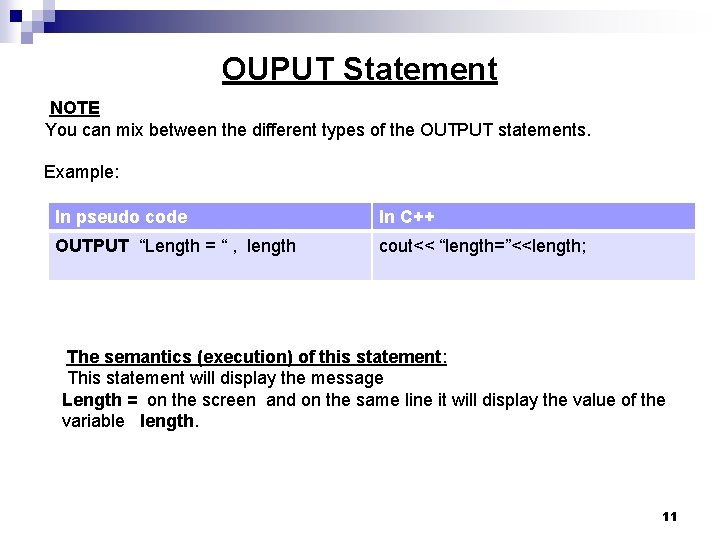
OUPUT Statement NOTE You can mix between the different types of the OUTPUT statements. Example: In pseudo code In C++ OUTPUT “Length = “ , length cout<< “length=”<<length; The semantics (execution) of this statement: This statement will display the message Length = on the screen and on the same line it will display the value of the variable length. 11
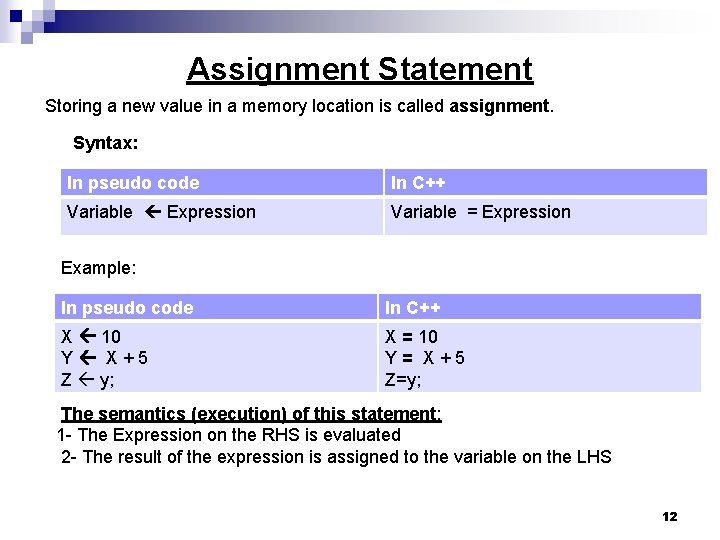
Assignment Statement Storing a new value in a memory location is called assignment. Syntax: In pseudo code In C++ Variable Expression Variable = Expression Example: In pseudo code In C++ X 10 Y X+5 Z y; X = 10 Y= X+5 Z=y; The semantics (execution) of this statement: 1 - The Expression on the RHS is evaluated 2 - The result of the expression is assigned to the variable on the LHS 12
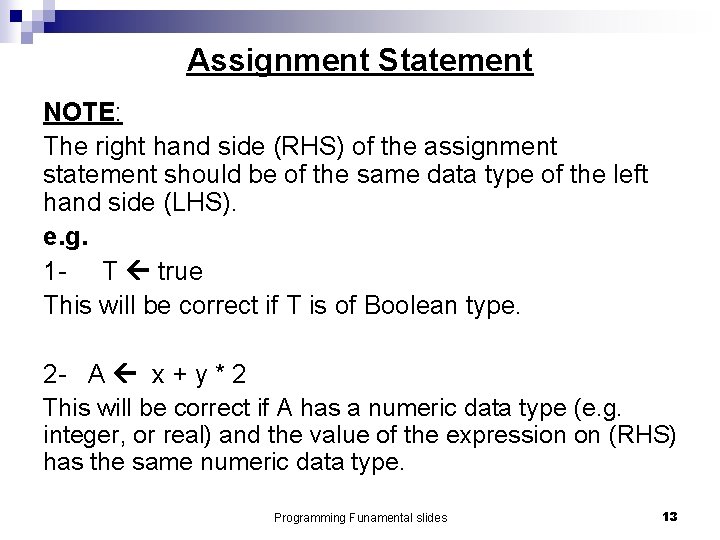
Assignment Statement NOTE: The right hand side (RHS) of the assignment statement should be of the same data type of the left hand side (LHS). e. g. 1 - T true This will be correct if T is of Boolean type. 2 - A x + y * 2 This will be correct if A has a numeric data type (e. g. integer, or real) and the value of the expression on (RHS) has the same numeric data type. Programming Funamental slides 13
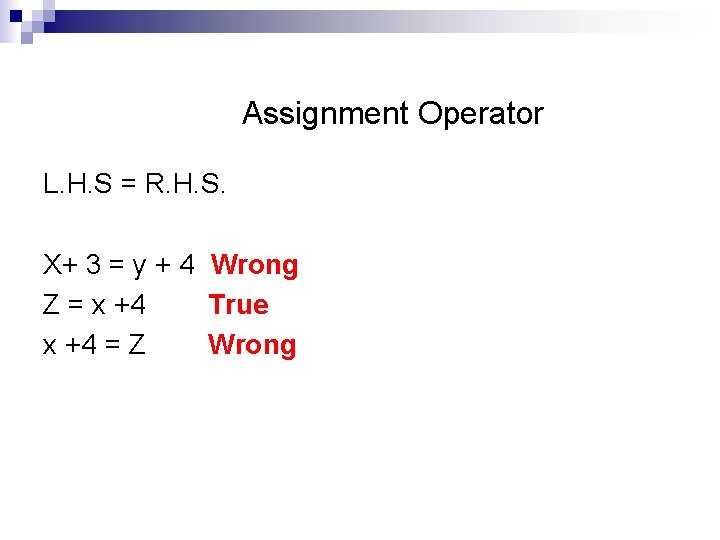
Assignment Operator L. H. S = R. H. S. X+ 3 = y + 4 Wrong Z = x +4 True x +4 = Z Wrong
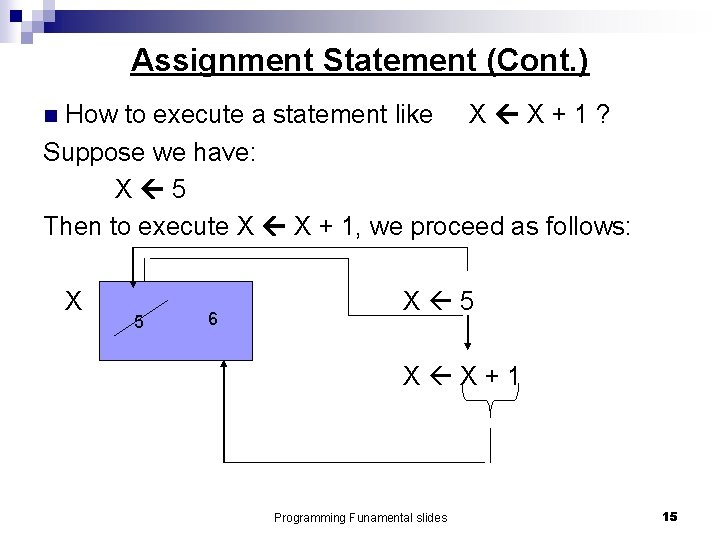
Assignment Statement (Cont. ) How to execute a statement like X X + 1 ? Suppose we have: X 5 Then to execute X X + 1, we proceed as follows: n X 5 6 X 5 X X+1 Programming Funamental slides 15
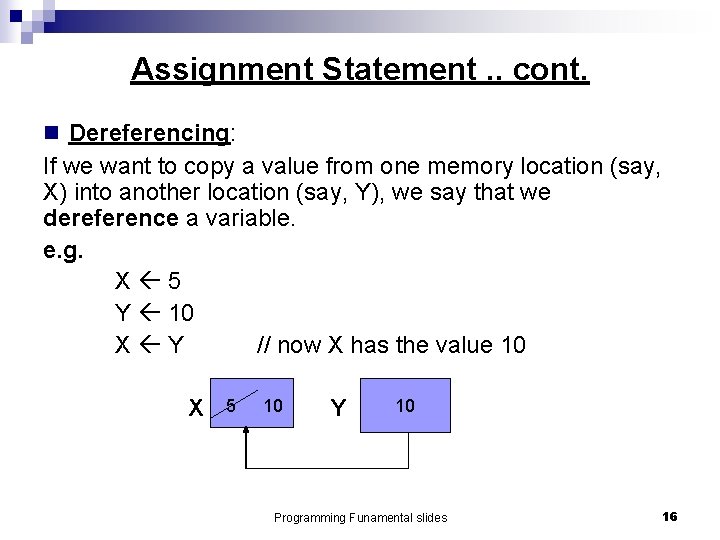
Assignment Statement. . cont. n Dereferencing: If we want to copy a value from one memory location (say, X) into another location (say, Y), we say that we dereference a variable. e. g. X 5 Y 10 X Y // now X has the value 10 X 5 10 Y 10 Programming Funamental slides 16
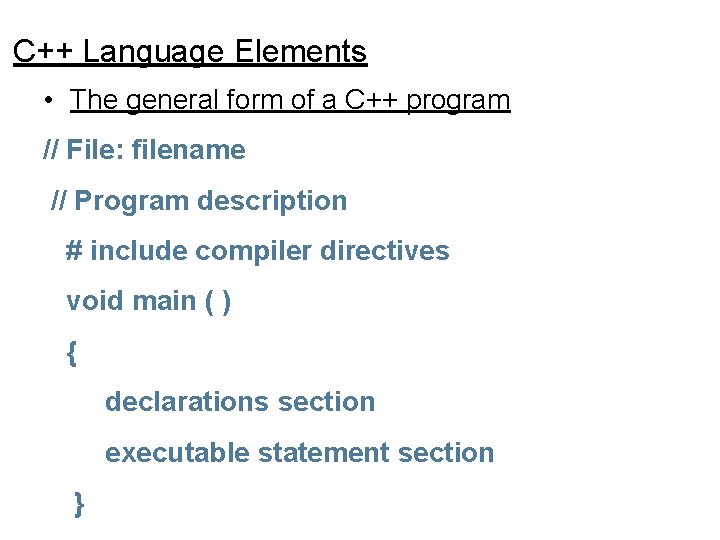
C++ Language Elements • The general form of a C++ program // File: filename // Program description # include compiler directives void main ( ) { declarations section executable statement section } Programming Fundamentals --> Ch 1. Problem solving 17
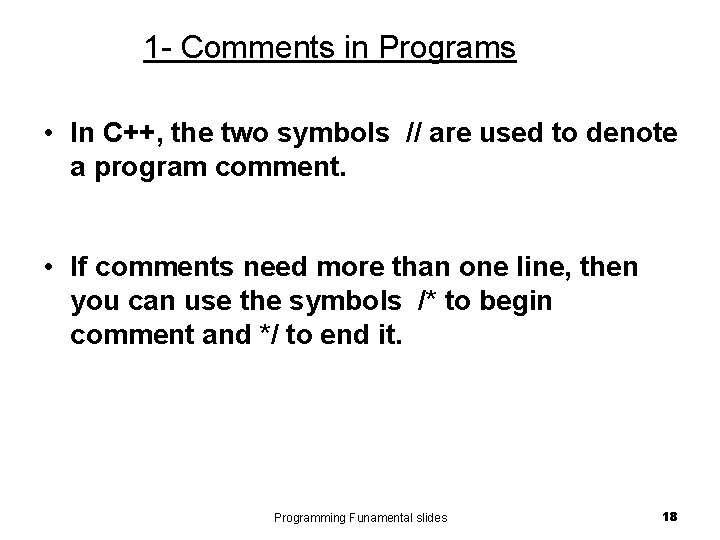
1 - Comments in Programs • In C++, the two symbols // are used to denote a program comment. • If comments need more than one line, then you can use the symbols /* to begin comment and */ to end it. Programming Funamental slides 18
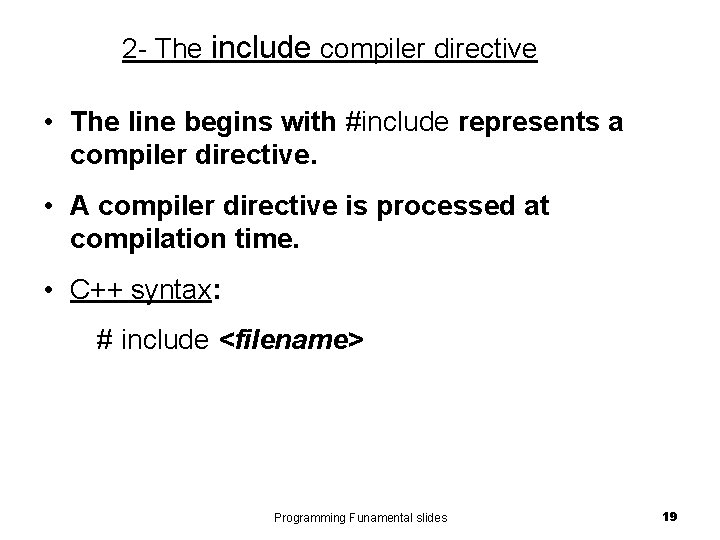
2 - The include compiler directive • The line begins with #include represents a compiler directive. • A compiler directive is processed at compilation time. • C++ syntax: # include <filename> Programming Funamental slides 19
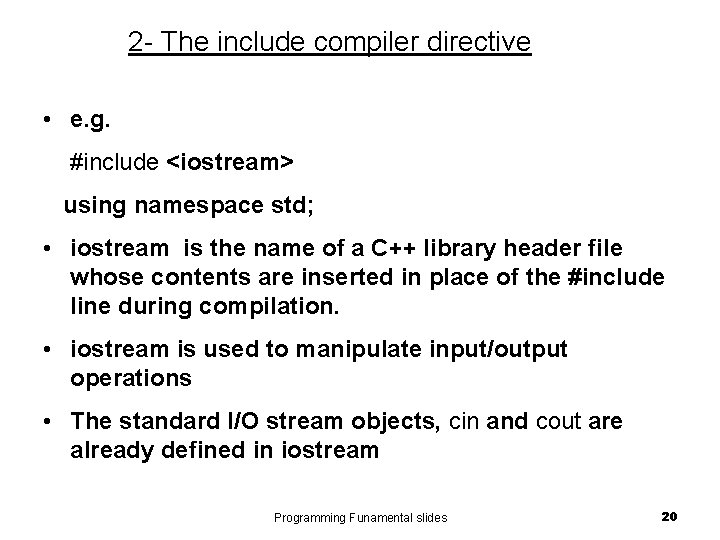
2 - The include compiler directive • e. g. #include <iostream> using namespace std; • iostream is the name of a C++ library header file whose contents are inserted in place of the #include line during compilation. • iostream is used to manipulate input/output operations • The standard I/O stream objects, cin and cout are already defined in iostream Programming Funamental slides 20
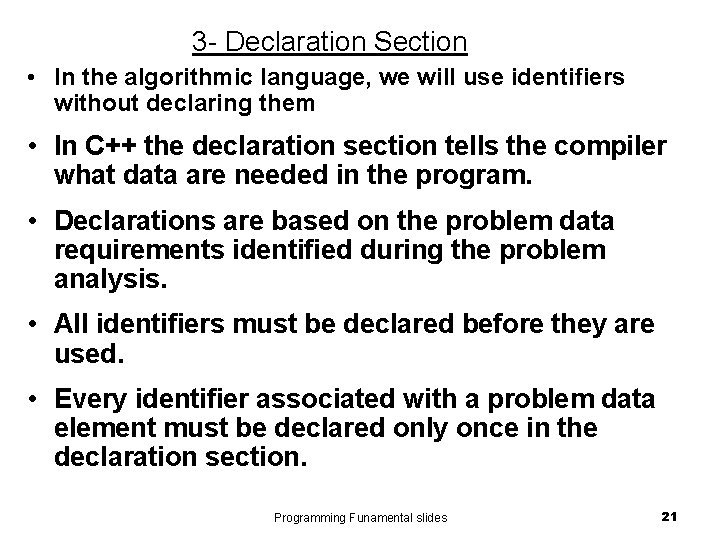
3 - Declaration Section • In the algorithmic language, we will use identifiers without declaring them • In C++ the declaration section tells the compiler what data are needed in the program. • Declarations are based on the problem data requirements identified during the problem analysis. • All identifiers must be declared before they are used. • Every identifier associated with a problem data element must be declared only once in the declaration section. Programming Funamental slides 21
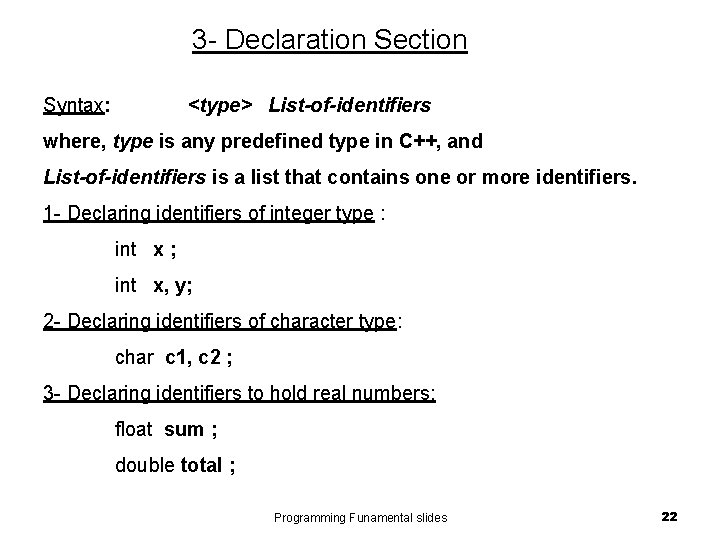
3 - Declaration Section Syntax: <type> List-of-identifiers where, type is any predefined type in C++, and List-of-identifiers is a list that contains one or more identifiers. 1 - Declaring identifiers of integer type : int x ; int x, y; 2 - Declaring identifiers of character type: char c 1, c 2 ; 3 - Declaring identifiers to hold real numbers: float sum ; double total ; Programming Funamental slides 22
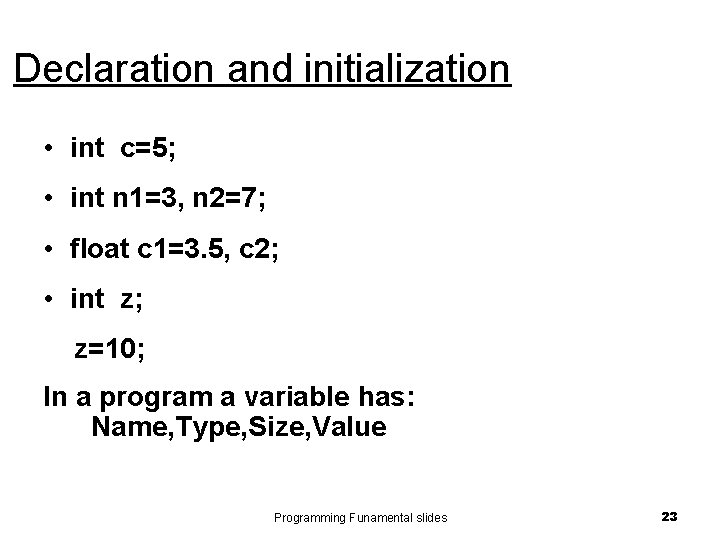
Declaration and initialization • int c=5; • int n 1=3, n 2=7; • float c 1=3. 5, c 2; • int z; z=10; In a program a variable has: Name, Type, Size, Value Programming Funamental slides 23
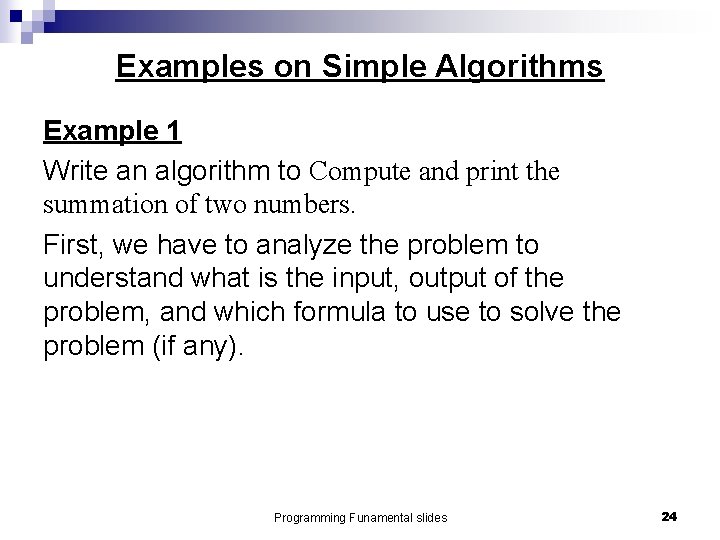
Examples on Simple Algorithms Example 1 Write an algorithm to Compute and print the summation of two numbers. First, we have to analyze the problem to understand what is the input, output of the problem, and which formula to use to solve the problem (if any). Programming Funamental slides 24
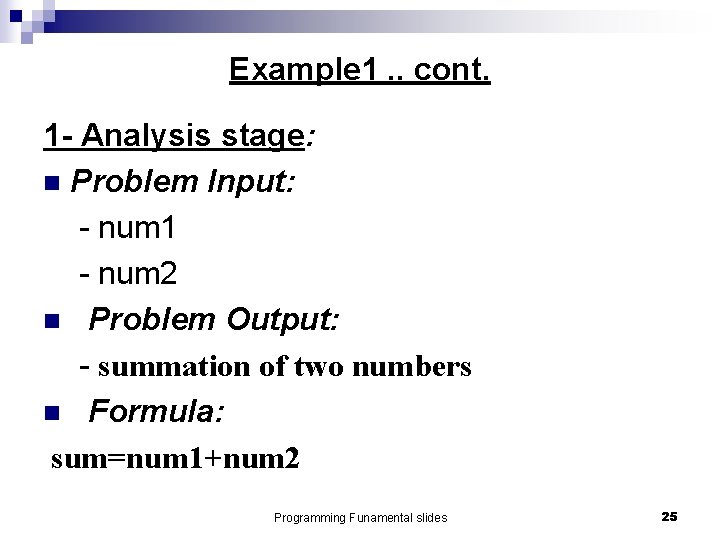
Example 1. . cont. 1 - Analysis stage: n Problem Input: - num 1 - num 2 n Problem Output: - summation of two numbers n Formula: sum=num 1+num 2 Programming Funamental slides 25
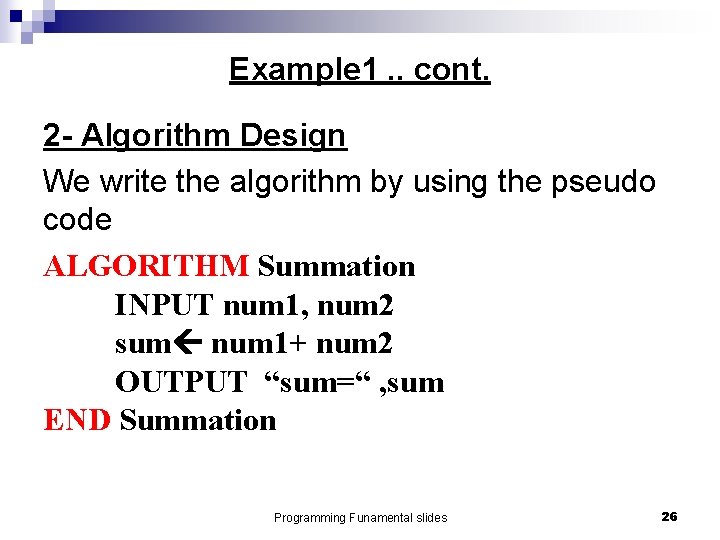
Example 1. . cont. 2 - Algorithm Design We write the algorithm by using the pseudo code ALGORITHM Summation INPUT num 1, num 2 sum num 1+ num 2 OUTPUT “sum=“ , sum END Summation Programming Funamental slides 26
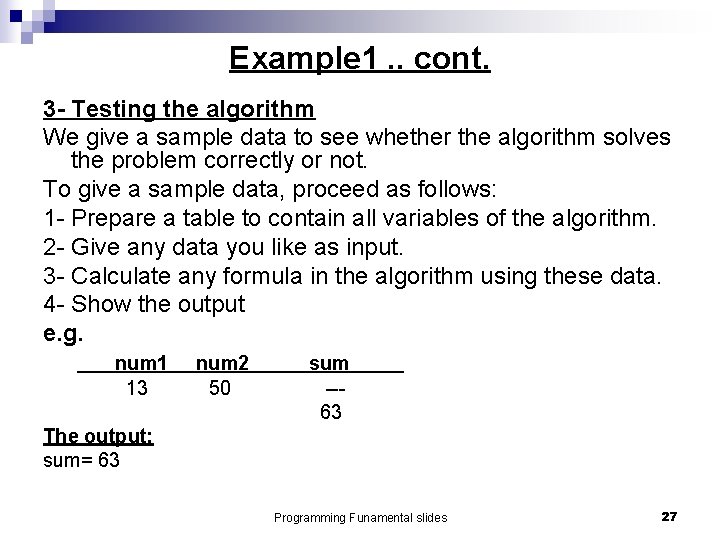
Example 1. . cont. 3 - Testing the algorithm We give a sample data to see whether the algorithm solves the problem correctly or not. To give a sample data, proceed as follows: 1 - Prepare a table to contain all variables of the algorithm. 2 - Give any data you like as input. 3 - Calculate any formula in the algorithm using these data. 4 - Show the output e. g. num 1 13 num 2 50 sum --63 The output: sum= 63 Programming Funamental slides 27
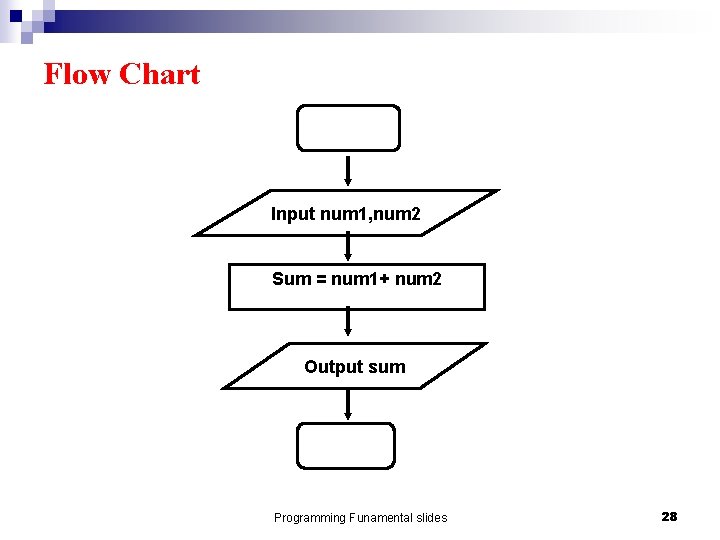
Flow Chart Input num 1, num 2 Sum = num 1+ num 2 Output sum Programming Funamental slides 28
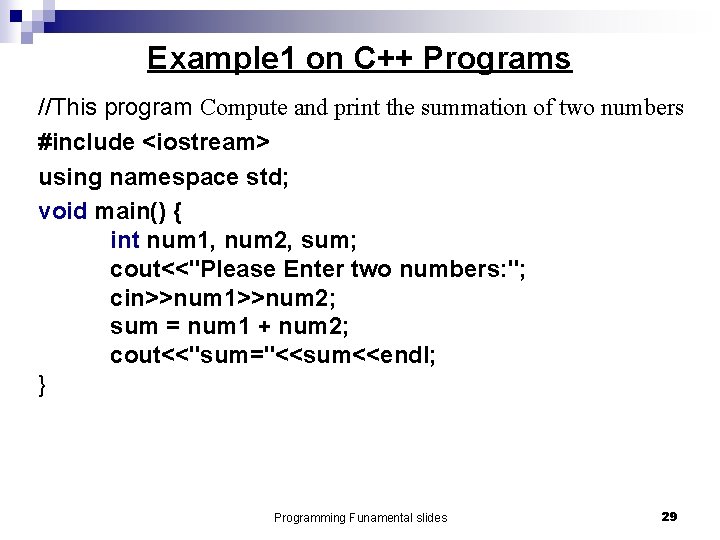
Example 1 on C++ Programs //This program Compute and print the summation of two numbers #include <iostream> using namespace std; void main() { int num 1, num 2, sum; cout<<"Please Enter two numbers: "; cin>>num 1>>num 2; sum = num 1 + num 2; cout<<"sum="<<sum<<endl; } Programming Funamental slides 29
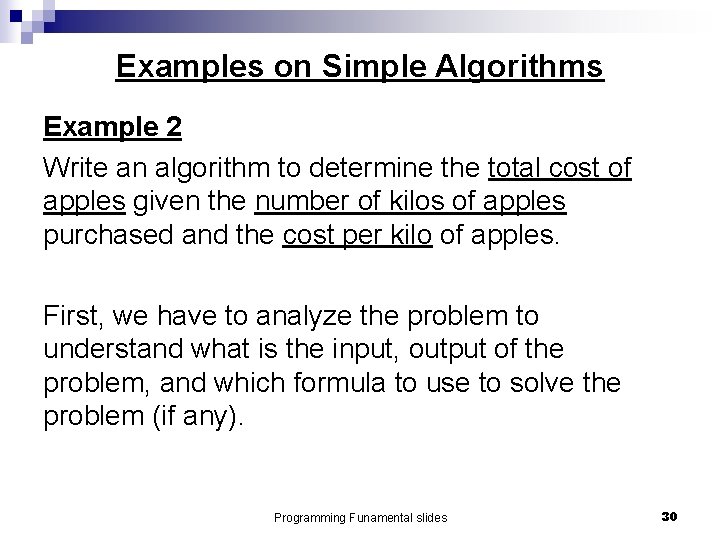
Examples on Simple Algorithms Example 2 Write an algorithm to determine the total cost of apples given the number of kilos of apples purchased and the cost per kilo of apples. First, we have to analyze the problem to understand what is the input, output of the problem, and which formula to use to solve the problem (if any). Programming Funamental slides 30
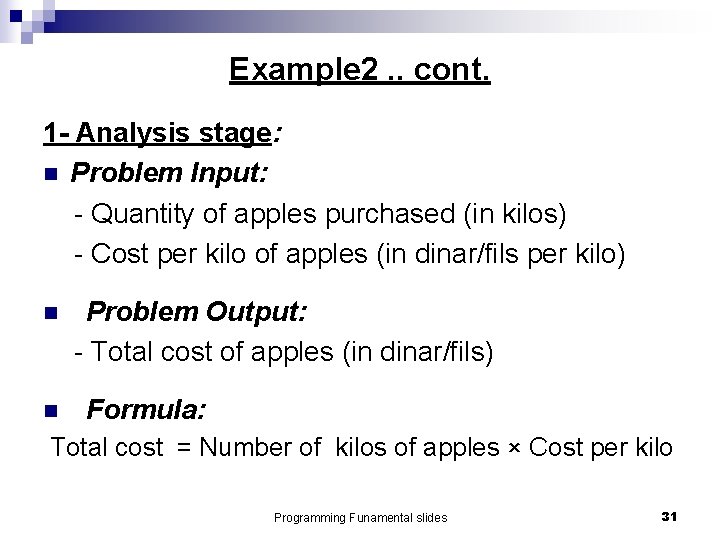
Example 2. . cont. 1 - Analysis stage: n Problem Input: - Quantity of apples purchased (in kilos) - Cost per kilo of apples (in dinar/fils per kilo) n n Problem Output: - Total cost of apples (in dinar/fils) Formula: Total cost = Number of kilos of apples × Cost per kilo Programming Funamental slides 31
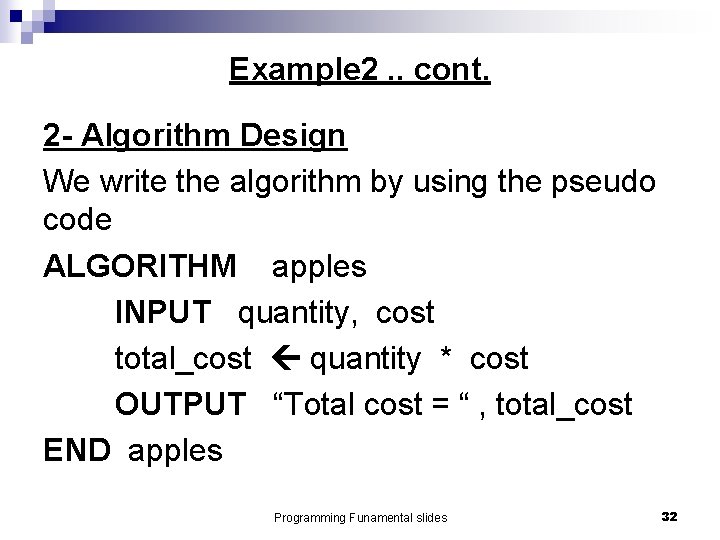
Example 2. . cont. 2 - Algorithm Design We write the algorithm by using the pseudo code ALGORITHM apples INPUT quantity, cost total_cost quantity * cost OUTPUT “Total cost = “ , total_cost END apples Programming Funamental slides 32
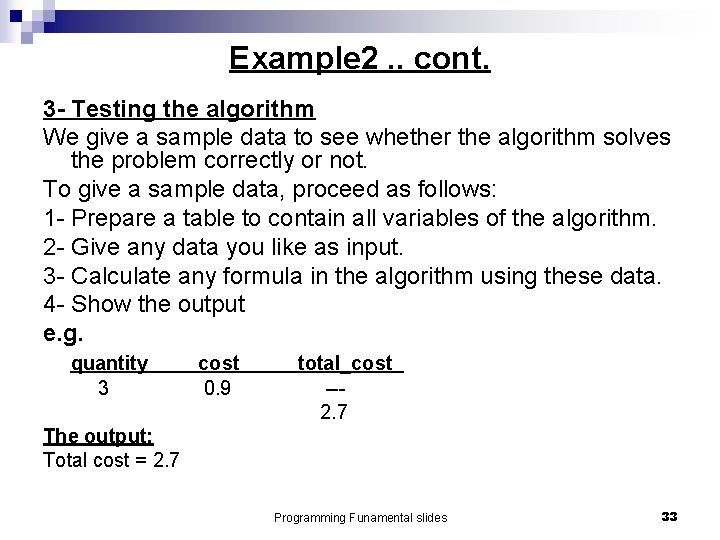
Example 2. . cont. 3 - Testing the algorithm We give a sample data to see whether the algorithm solves the problem correctly or not. To give a sample data, proceed as follows: 1 - Prepare a table to contain all variables of the algorithm. 2 - Give any data you like as input. 3 - Calculate any formula in the algorithm using these data. 4 - Show the output e. g. quantity 3 cost 0. 9 total_cost --2. 7 The output: Total cost = 2. 7 Programming Funamental slides 33
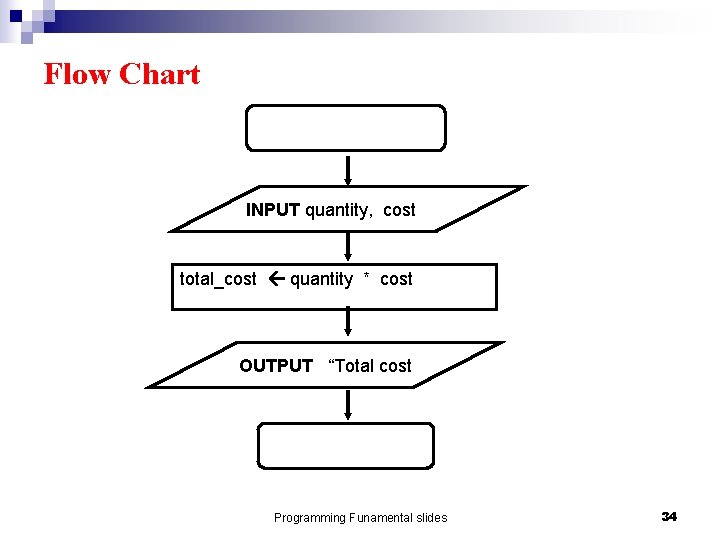
Flow Chart INPUT quantity, cost total_cost quantity * cost OUTPUT “Total cost Programming Funamental slides 34
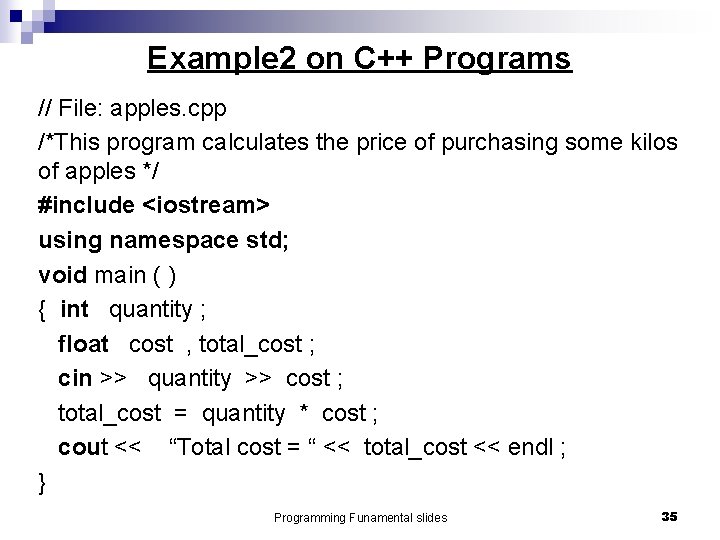
Example 2 on C++ Programs // File: apples. cpp /*This program calculates the price of purchasing some kilos of apples */ #include <iostream> using namespace std; void main ( ) { int quantity ; float cost , total_cost ; cin >> quantity >> cost ; total_cost = quantity * cost ; cout << “Total cost = “ << total_cost << endl ; } Programming Funamental slides 35
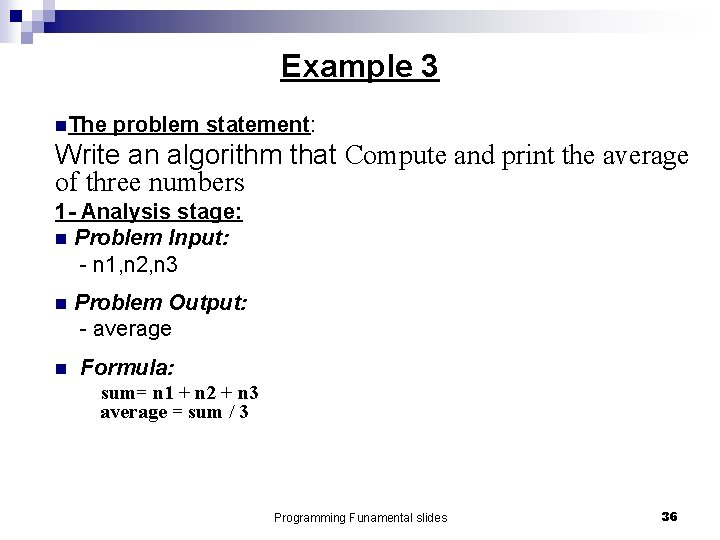
Example 3 n. The problem statement: Write an algorithm that Compute and print the average of three numbers 1 - Analysis stage: n Problem Input: - n 1, n 2, n 3 n n Problem Output: - average Formula: sum= n 1 + n 2 + n 3 average = sum / 3 Programming Funamental slides 36
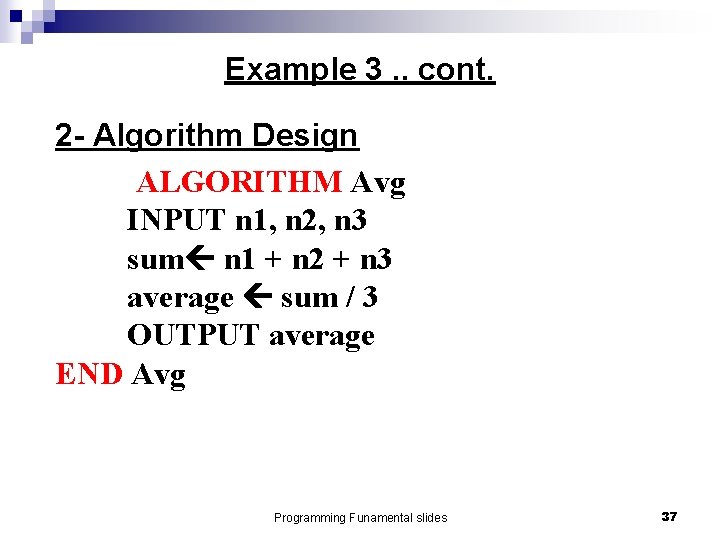
Example 3. . cont. 2 - Algorithm Design ALGORITHM Avg INPUT n 1, n 2, n 3 sum n 1 + n 2 + n 3 average sum / 3 OUTPUT average END Avg Programming Funamental slides 37
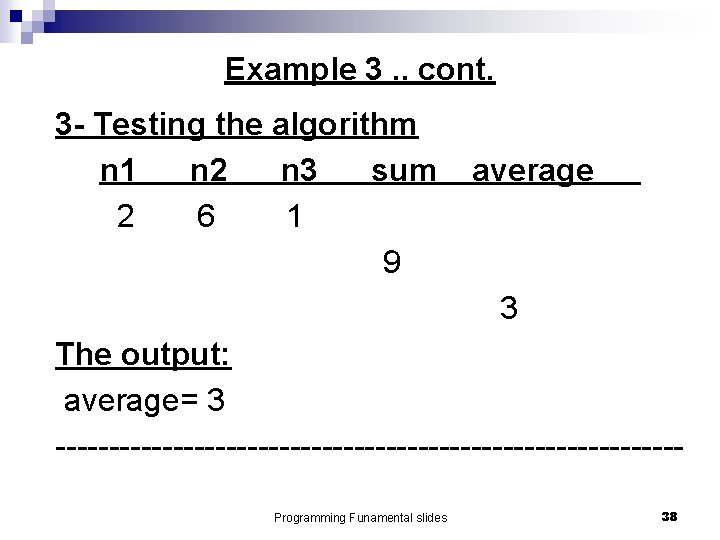
Example 3. . cont. 3 - Testing the algorithm n 1 n 2 n 3 sum 2 6 1 9 average 3 The output: average= 3 -----------------------------Programming Funamental slides 38
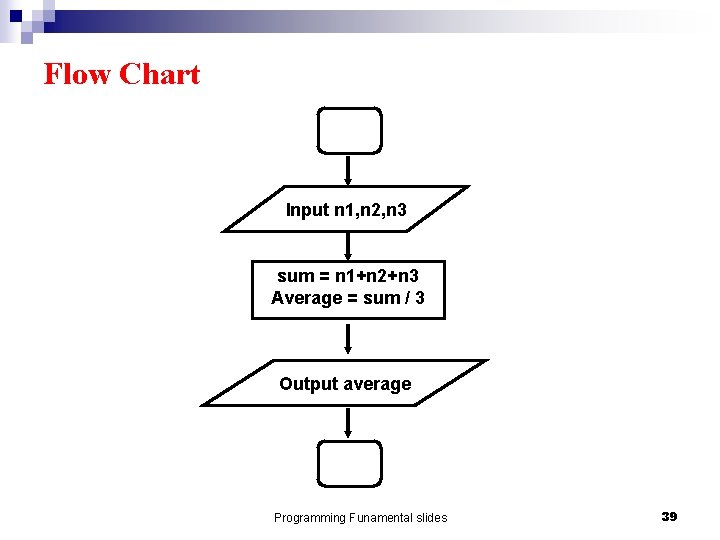
Flow Chart Input n 1, n 2, n 3 sum = n 1+n 2+n 3 Average = sum / 3 Output average Programming Funamental slides 39
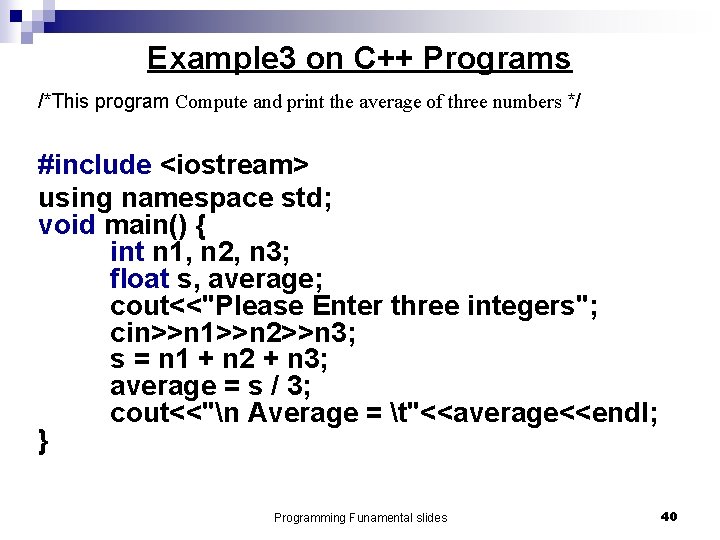
Example 3 on C++ Programs /*This program Compute and print the average of three numbers */ #include <iostream> using namespace std; void main() { int n 1, n 2, n 3; float s, average; cout<<"Please Enter three integers"; cin>>n 1>>n 2>>n 3; s = n 1 + n 2 + n 3; average = s / 3; cout<<"n Average = t"<<average<<endl; } Programming Funamental slides 40
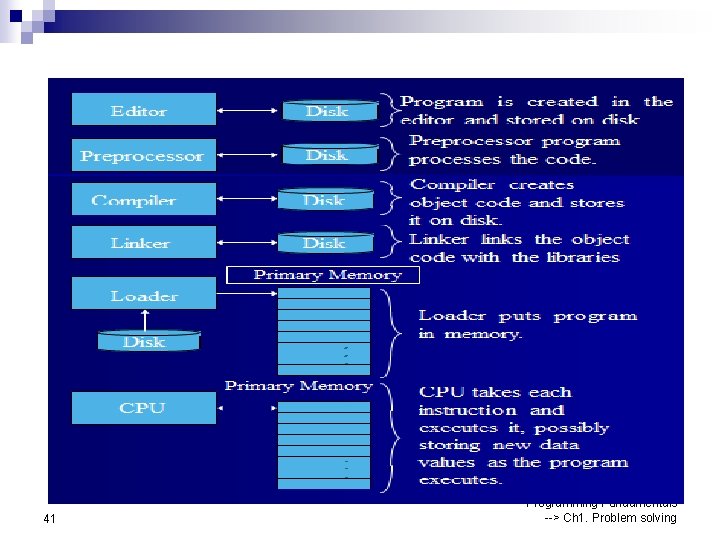
Integrated Development Environment 41 Programming Fundamentals --> Ch 1. Problem solving
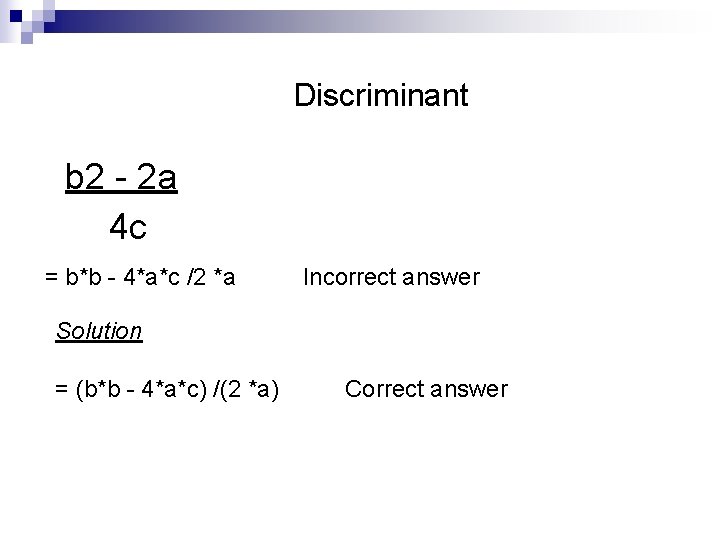
Discriminant b 2 - 2 a 4 c = b*b - 4*a*c /2 *a Incorrect answer Solution = (b*b - 4*a*c) /(2 *a) Correct answer
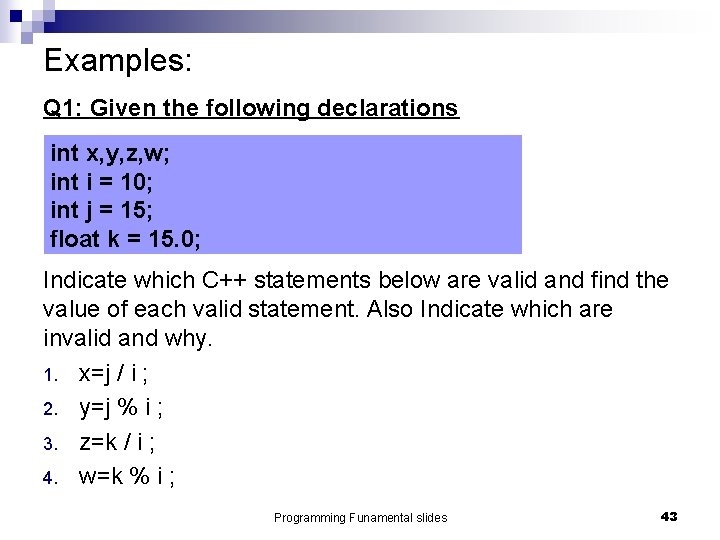
Examples: Q 1: Given the following declarations int x, y, z, w; int i = 10; int j = 15; float k = 15. 0; Indicate which C++ statements below are valid and find the value of each valid statement. Also Indicate which are invalid and why. 1. x=j / i ; 2. y=j % i ; 3. z=k / i ; 4. w=k % i ; Programming Funamental slides 43
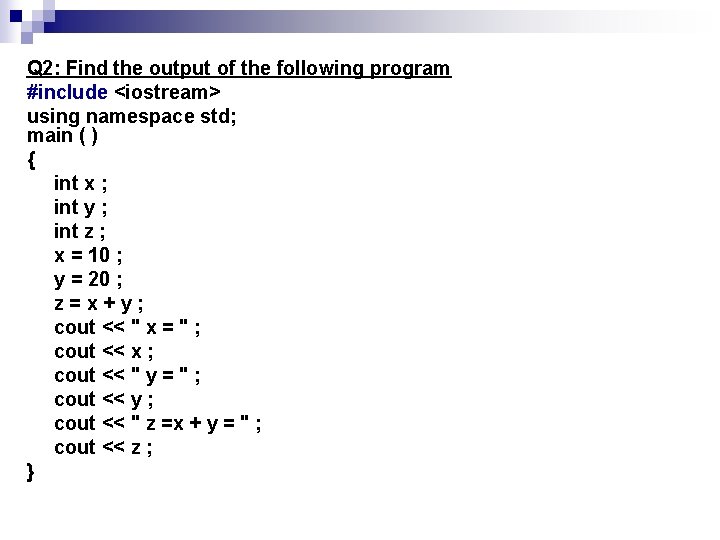
Q 2: Find the output of the following program #include <iostream> using namespace std; main ( ) { int x ; int y ; int z ; x = 10 ; y = 20 ; z=x+y; cout << " x = " ; cout << x ; cout << " y = " ; cout << y ; cout << " z =x + y = " ; cout << z ; }
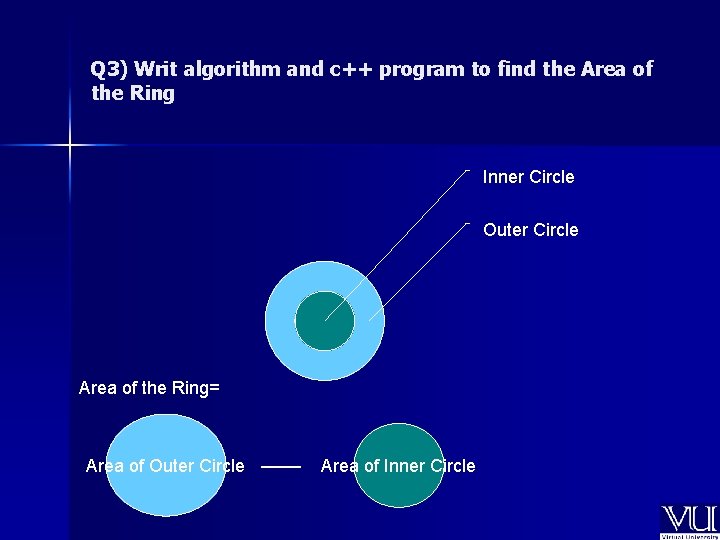
Q 3) Writ algorithm and c++ program to find the Area of the Ring Inner Circle Outer Circle Area of the Ring= Area of Outer Circle ____ Area of Inner Circle
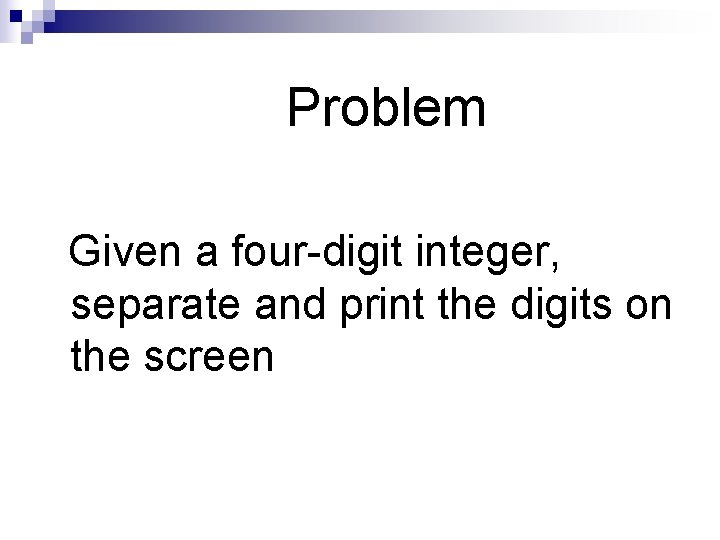
Problem Given a four-digit integer, separate and print the digits on the screen
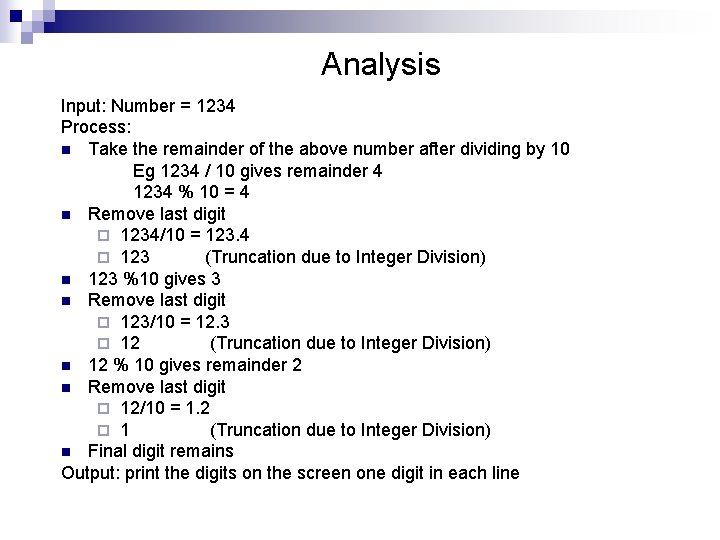
Analysis Input: Number = 1234 Process: n Take the remainder of the above number after dividing by 10 Eg 1234 / 10 gives remainder 4 1234 % 10 = 4 n Remove last digit ¨ 1234/10 = 123. 4 ¨ 123 (Truncation due to Integer Division) n 123 %10 gives 3 n Remove last digit ¨ 123/10 = 12. 3 ¨ 12 (Truncation due to Integer Division) n 12 % 10 gives remainder 2 n Remove last digit ¨ 12/10 = 1. 2 ¨ 1 (Truncation due to Integer Division) n Final digit remains Output: print the digits on the screen one digit in each line
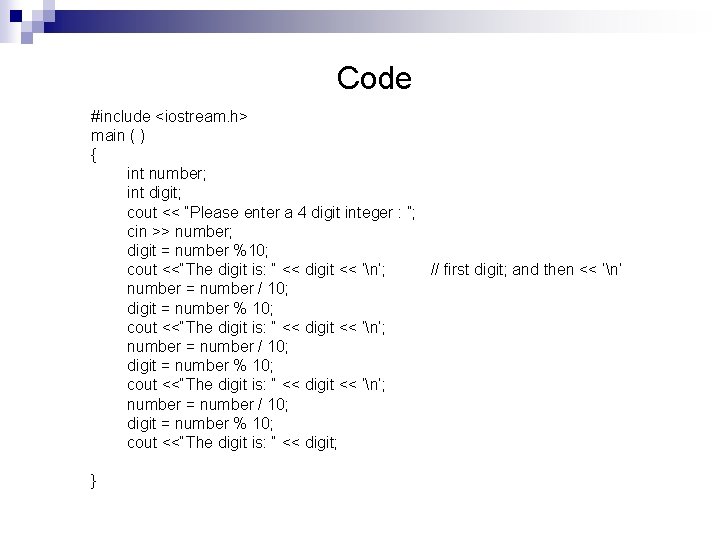
Code #include <iostream. h> main ( ) { int number; int digit; cout << “Please enter a 4 digit integer : ”; cin >> number; digit = number %10; cout <<“The digit is: “ << digit << ‘n’; // first digit; and then << ‘n’ number = number / 10; digit = number % 10; cout <<“The digit is: “ << digit << ‘n’; number = number / 10; digit = number % 10; cout <<“The digit is: “ << digit; }
Six cardinal fields of gaze
Vike vicente
De 4 kundetyper
The vegetation and manufactured structures that cover land
Here i bow down here i bow down
Homologous
Hardware and control structures
Statement level control structures
Control structures in php
Setprecision in c++
Repetition control structure in c++
Types of control structures
Assembly language programming
Statement level control structures
Control structures in php
Iteration control structures
Which is not a repetition control structure in java?
Iteration control structures
Entity life history
Repetition pseudocode example
Control structure in visual basic
Primary control vs secondary control
Dairy plant management
Reynold’s transport theorem
Stock control e flow control
Control volume vs control surface
Positive vs negative controls
What is a negative control in an experiment
Data link control
Control de flujo y control de errores
Negative control vs positive control examples
Flow control and error control
Scalar control vs vector control
Komponen ltspice
School magazine examples
How to write an essay grade 9
Thematic paragraph example
Behavioural training topics
Skill 21 draw conclusions about who what when where
What is competency based interview
Surprising reversal essay example
Theme of death in the great gatsby
What is a thematic topic
Table topics questions toastmasters
Subjective and objective writing
Solo talk examples
Software project management topics
Sociolinguistics topics
Smaw topics