Constructor LECTURE 8 Constructor Whenever a class or
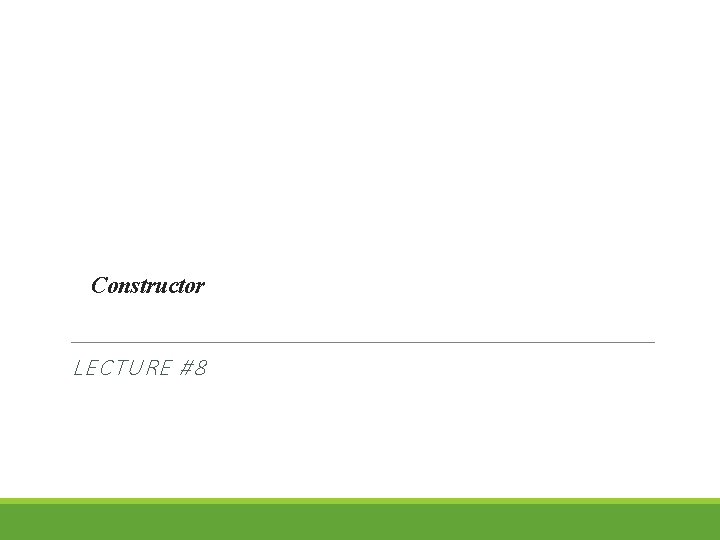
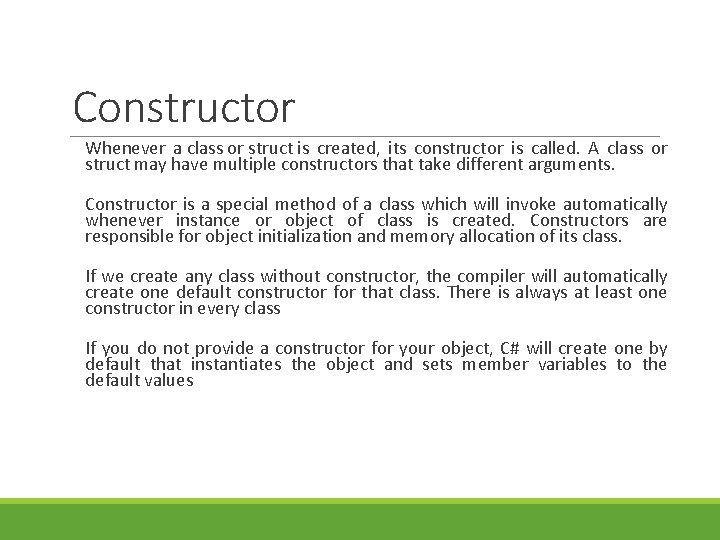
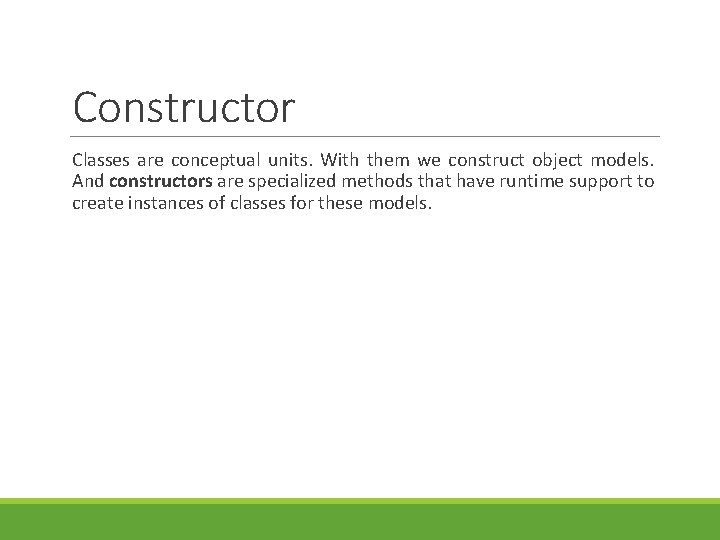
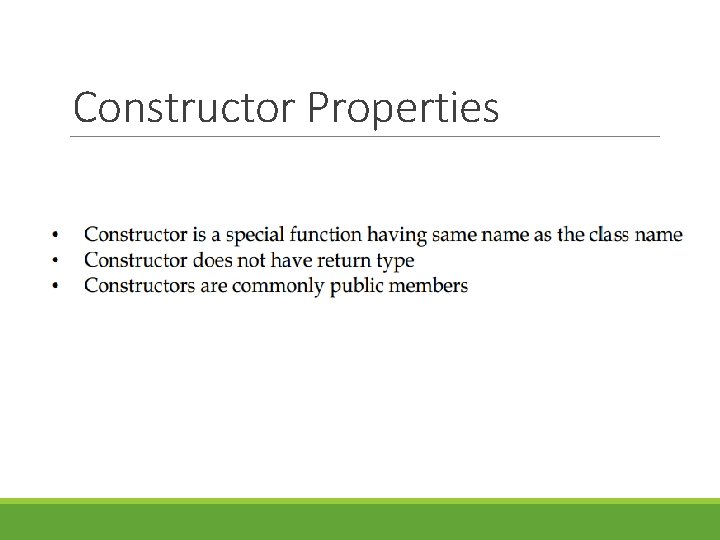
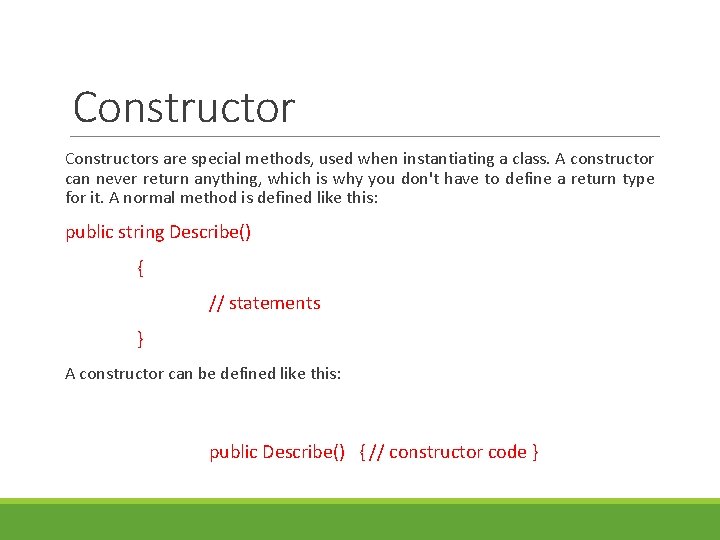
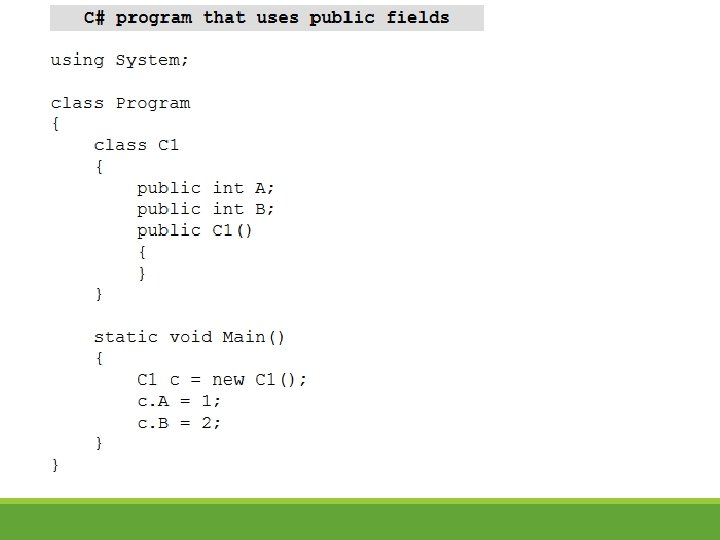
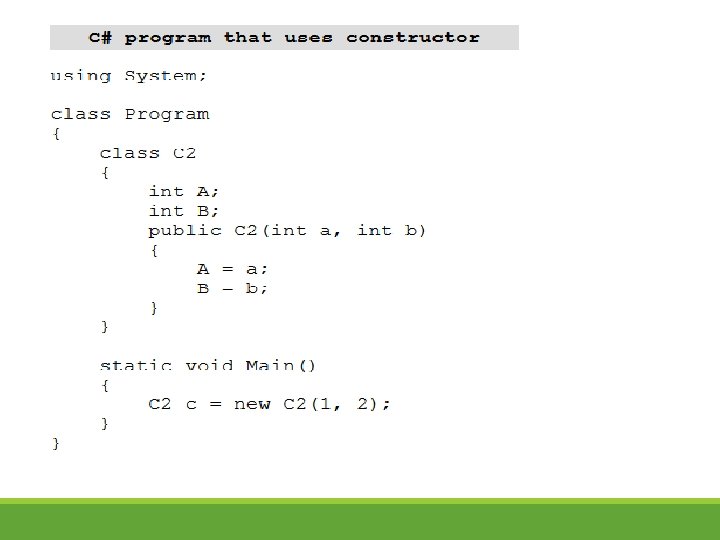
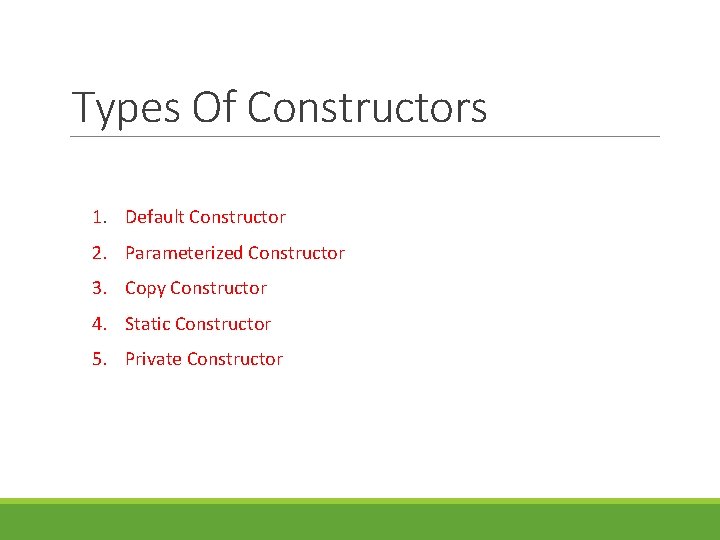
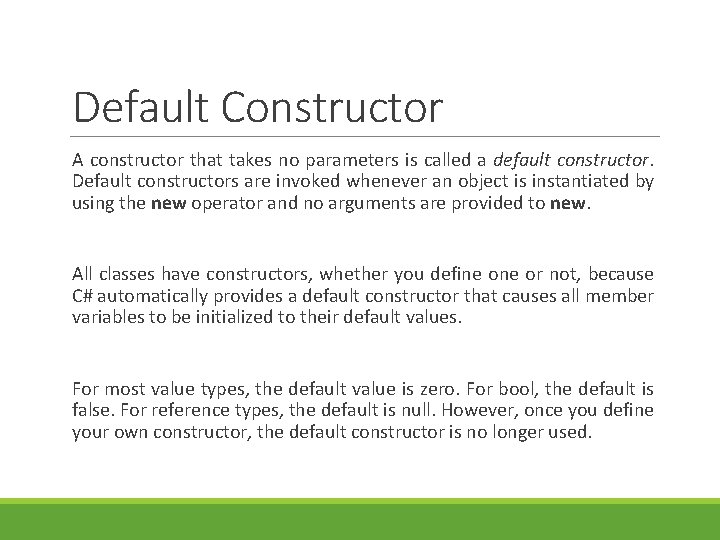
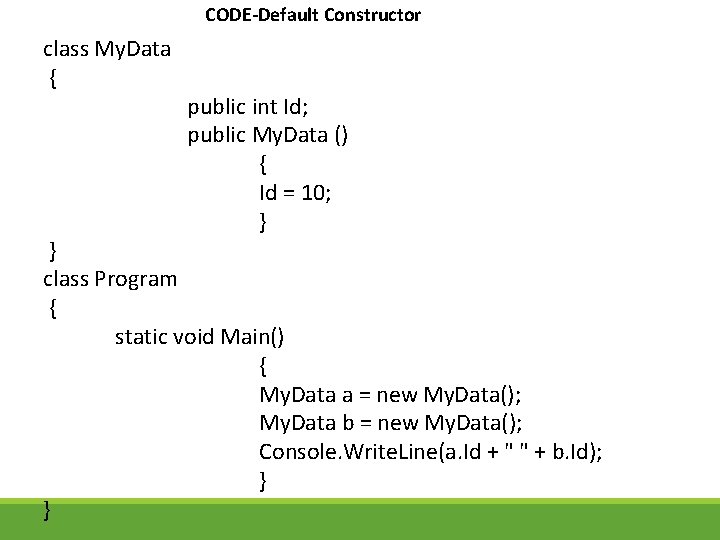
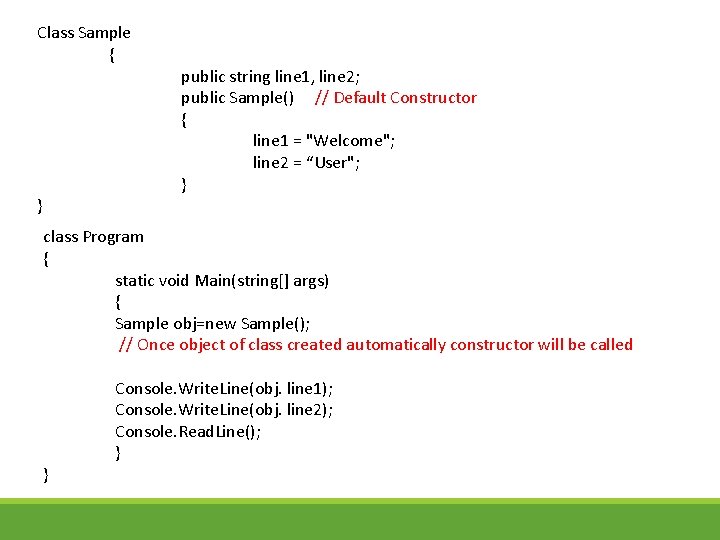
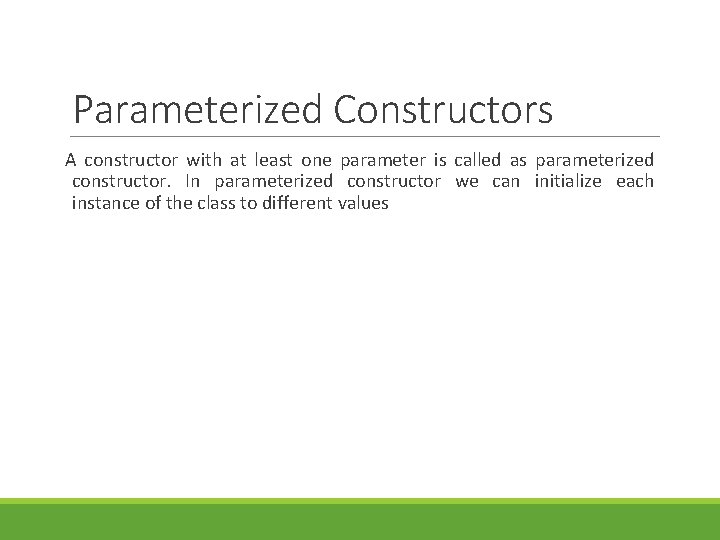
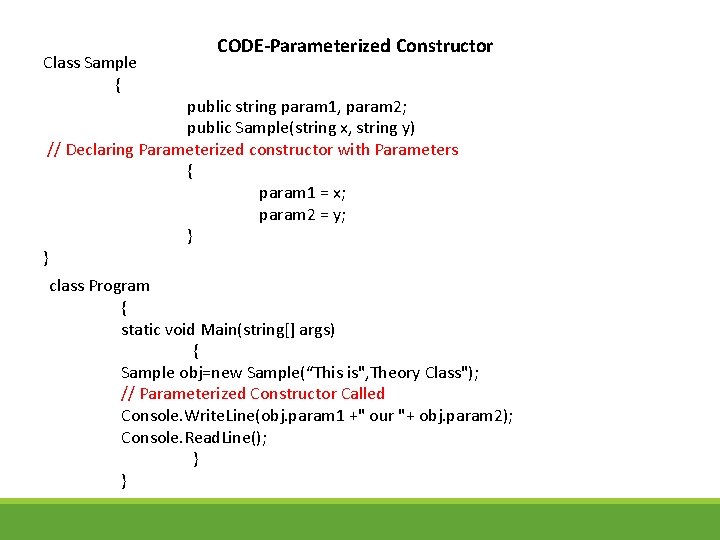
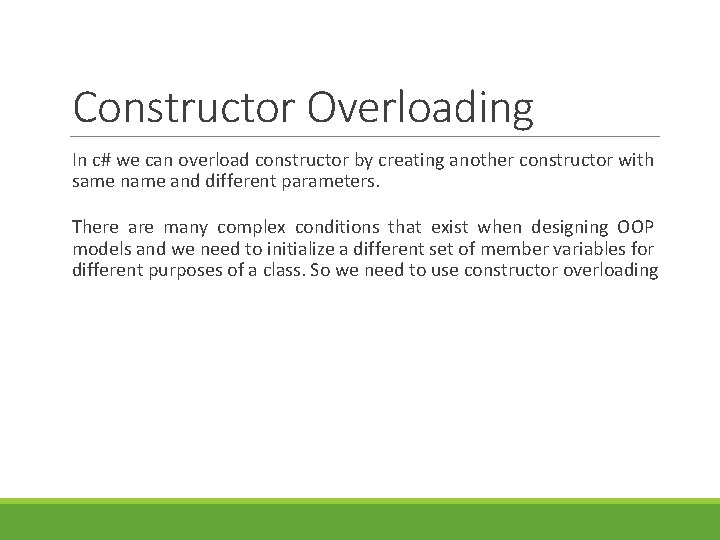
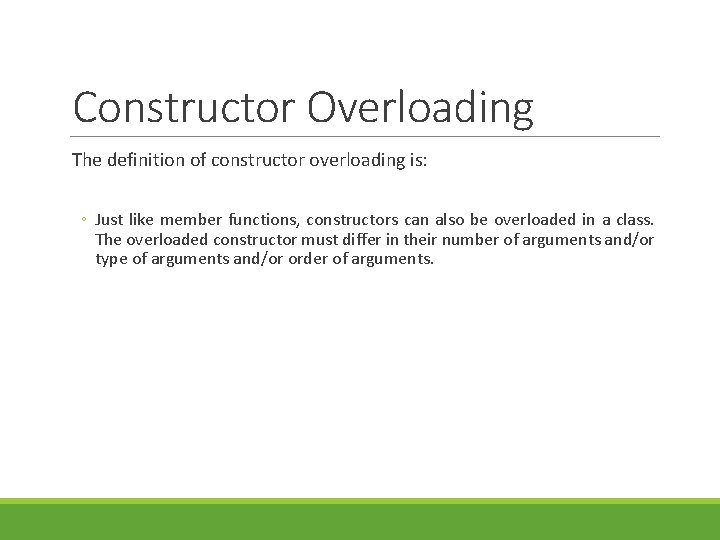
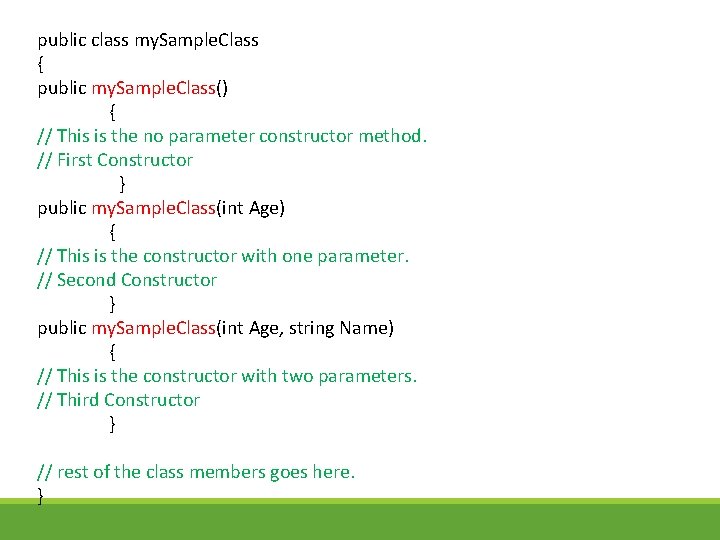
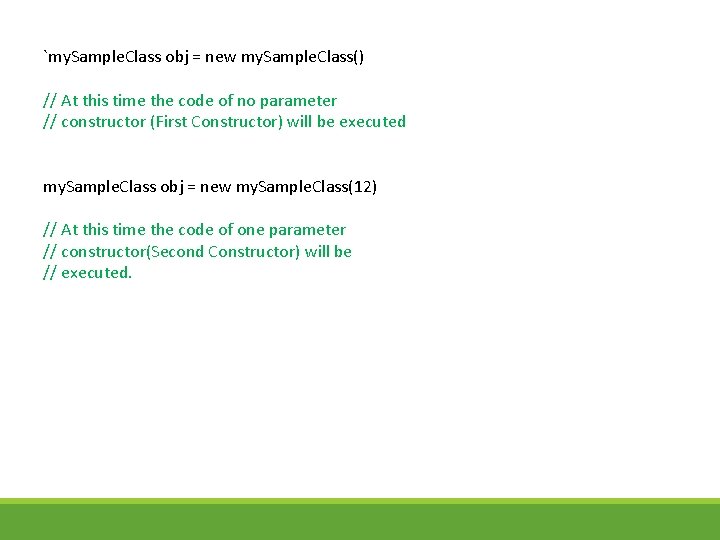
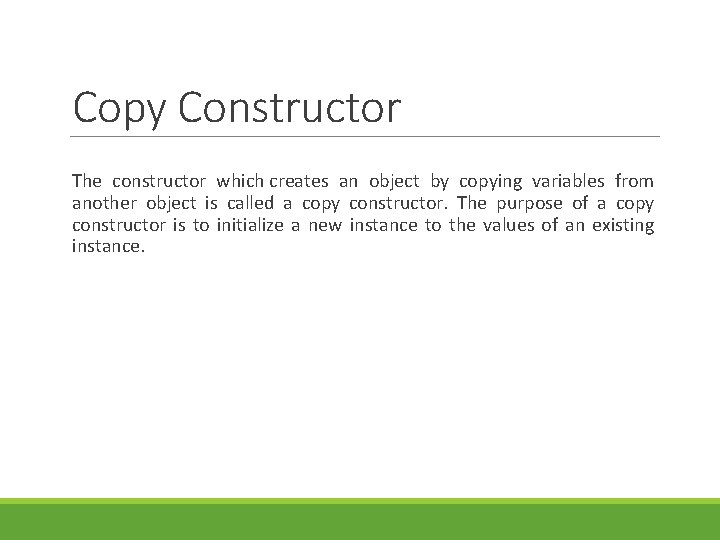
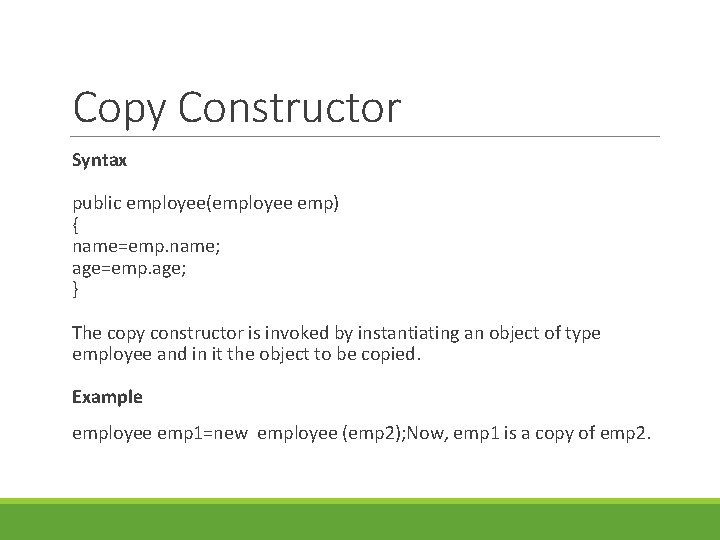
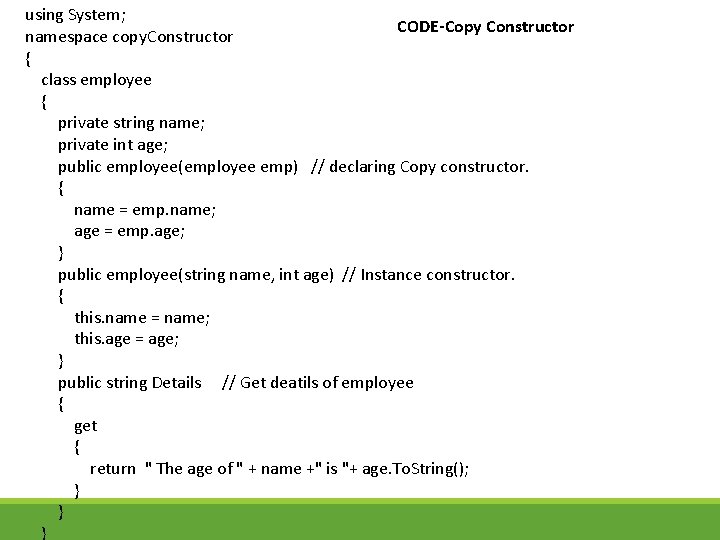
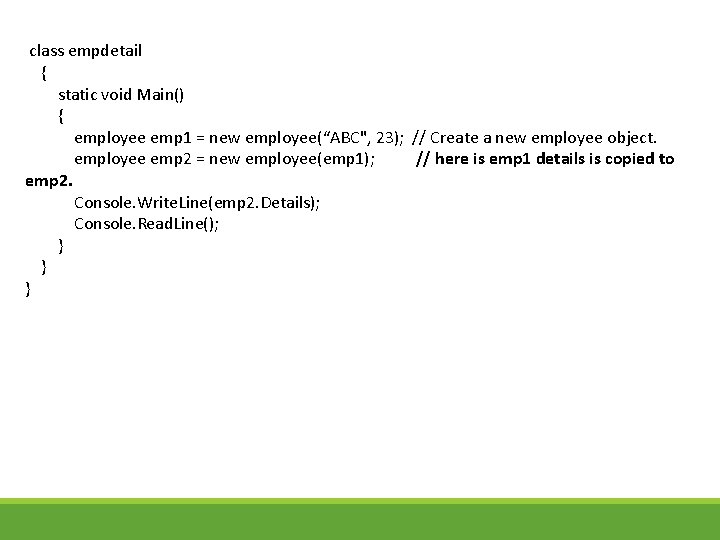
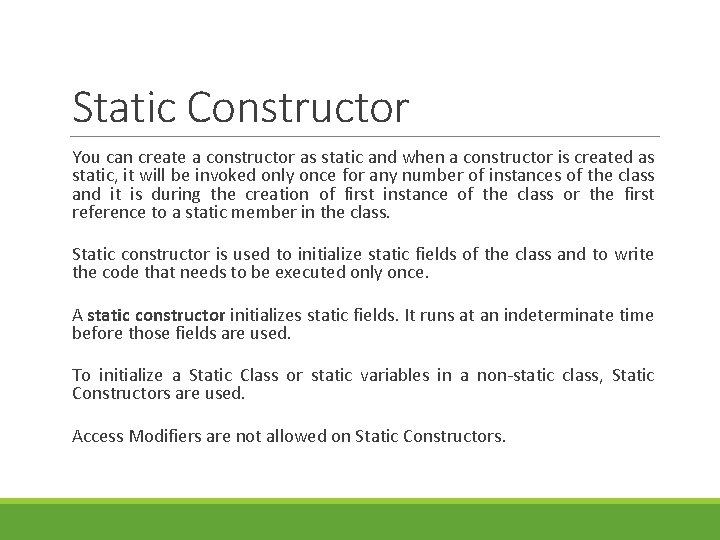
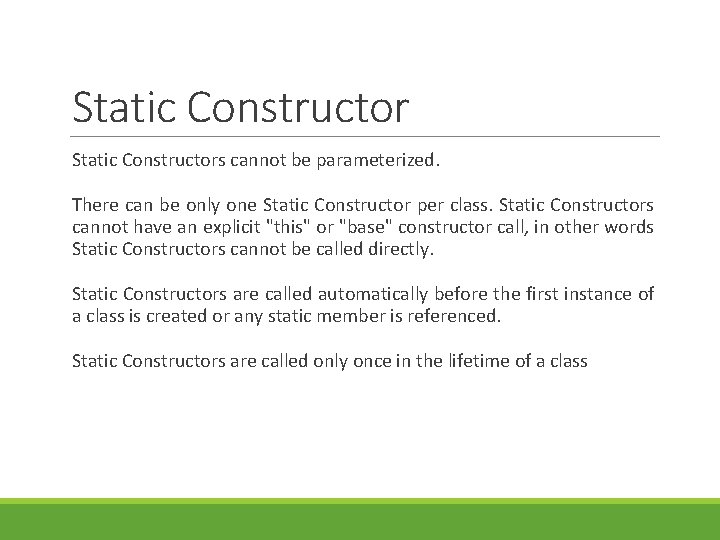
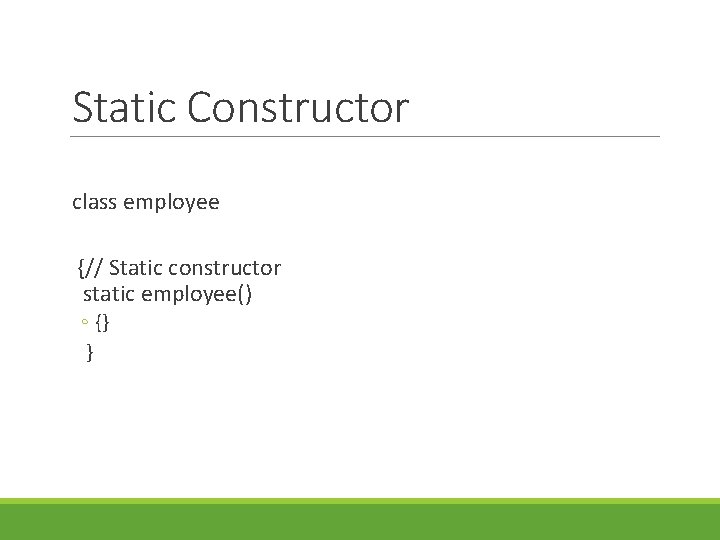
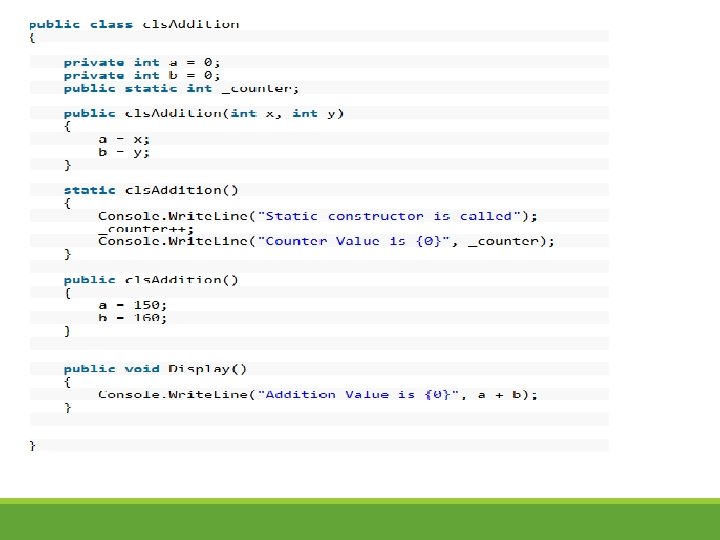
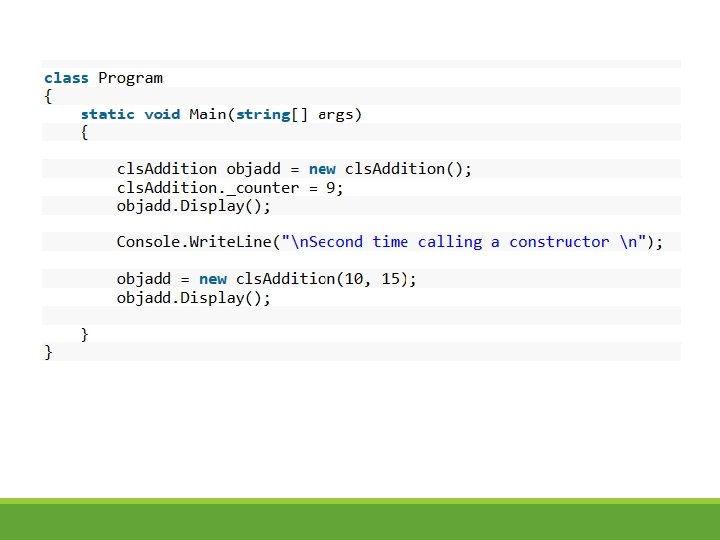
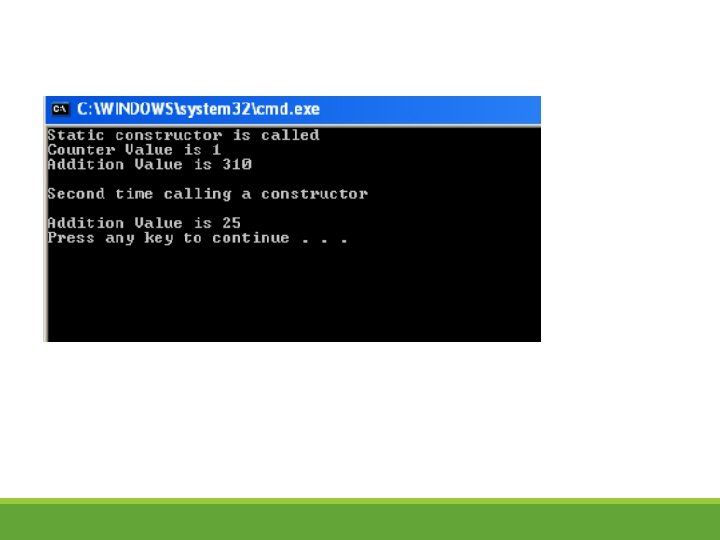
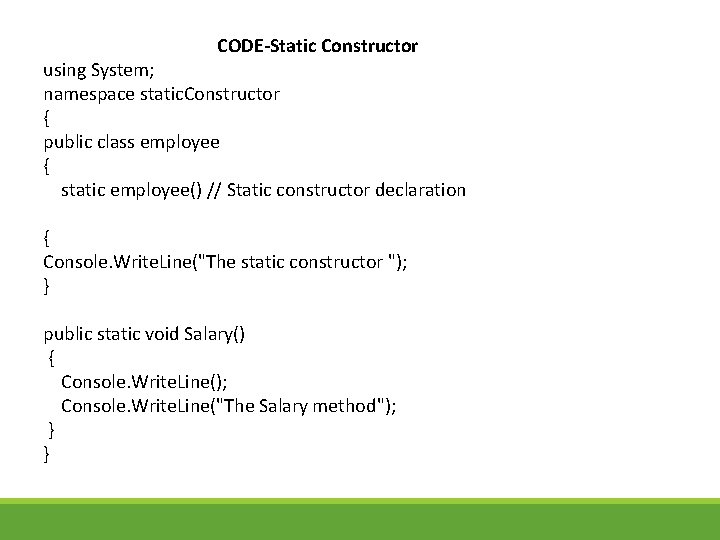
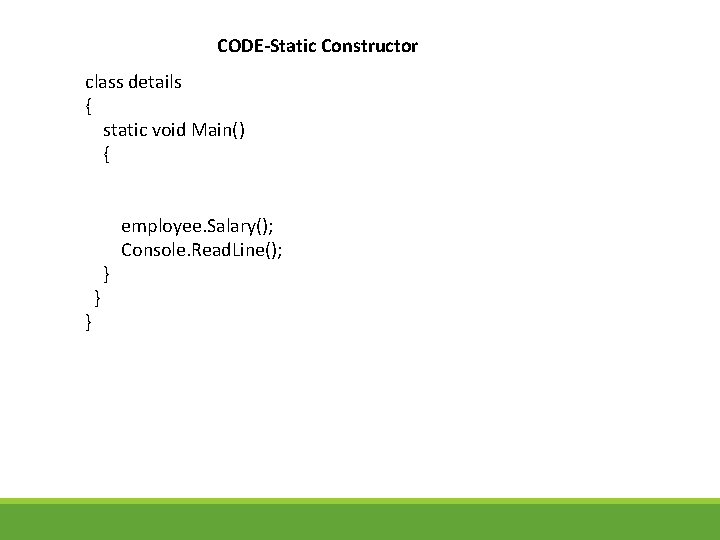
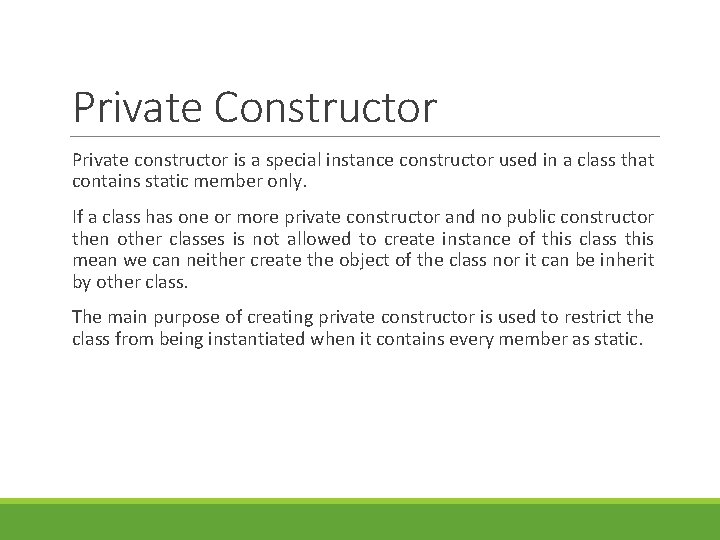
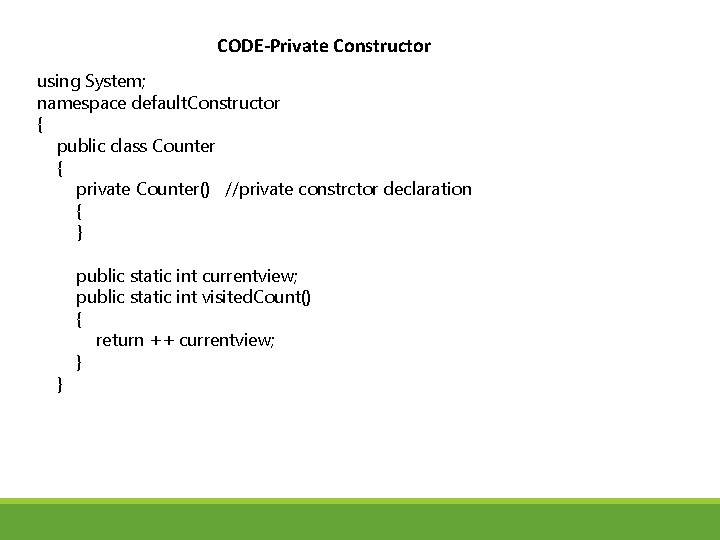
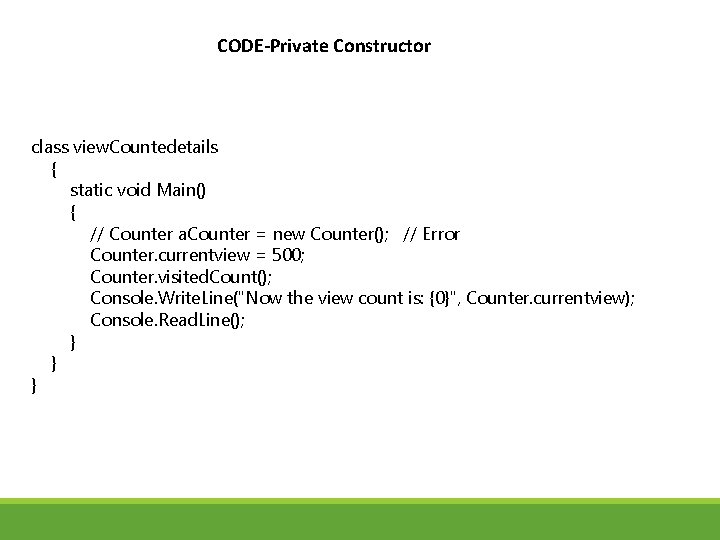
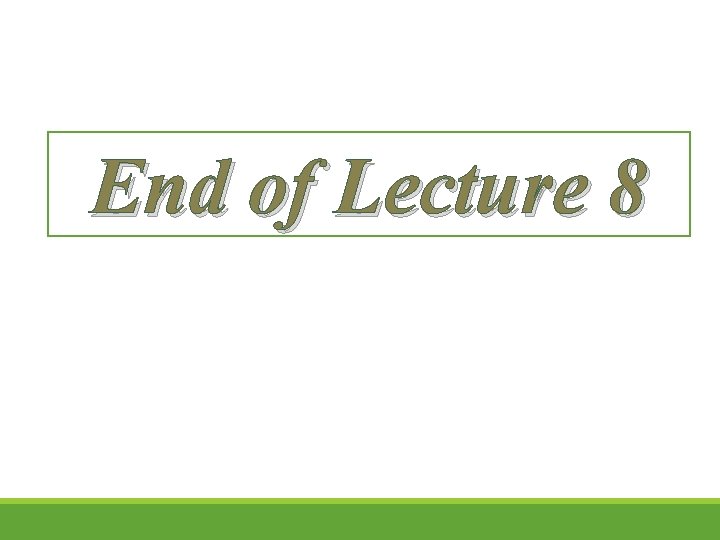
- Slides: 33
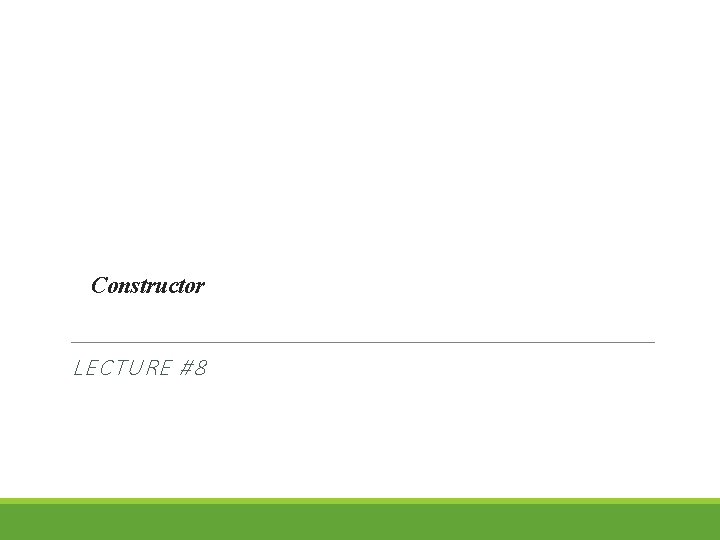
Constructor LECTURE #8
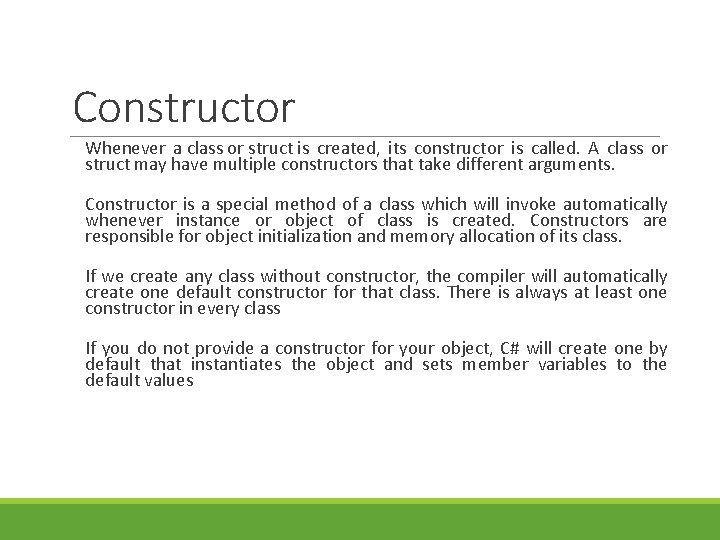
Constructor Whenever a class or struct is created, its constructor is called. A class or struct may have multiple constructors that take different arguments. Constructor is a special method of a class which will invoke automatically whenever instance or object of class is created. Constructors are responsible for object initialization and memory allocation of its class. If we create any class without constructor, the compiler will automatically create one default constructor for that class. There is always at least one constructor in every class If you do not provide a constructor for your object, C# will create one by default that instantiates the object and sets member variables to the default values
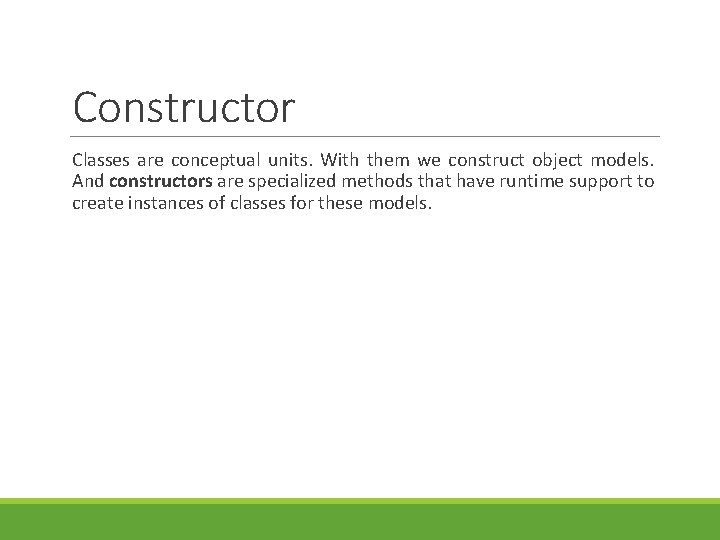
Constructor Classes are conceptual units. With them we construct object models. And constructors are specialized methods that have runtime support to create instances of classes for these models.
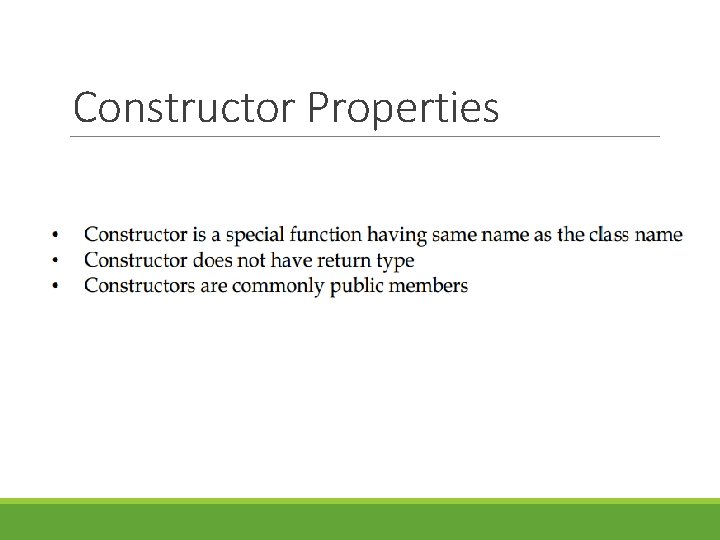
Constructor Properties
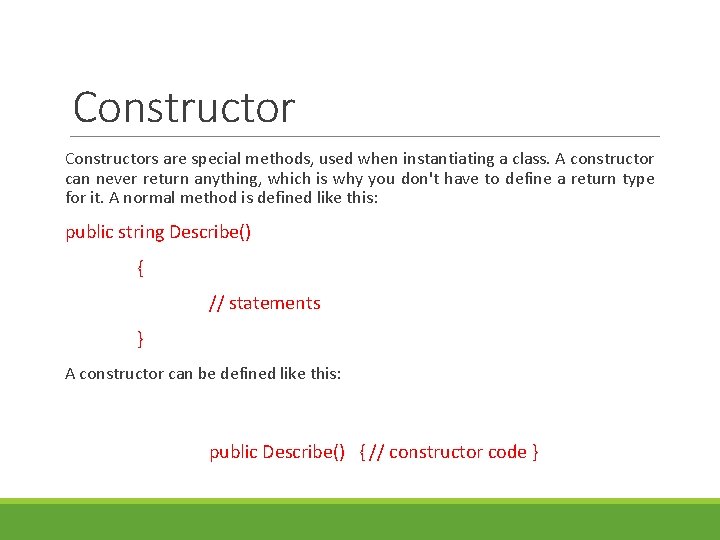
Constructors are special methods, used when instantiating a class. A constructor can never return anything, which is why you don't have to define a return type for it. A normal method is defined like this: public string Describe() { // statements } A constructor can be defined like this: public Describe() { // constructor code }
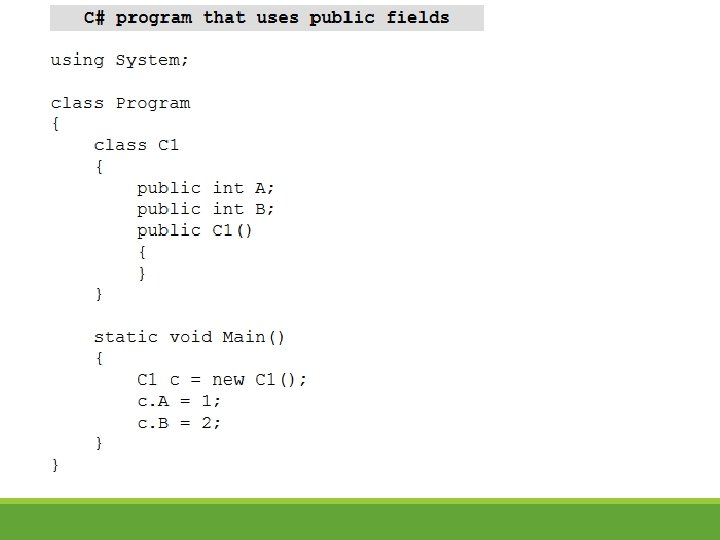
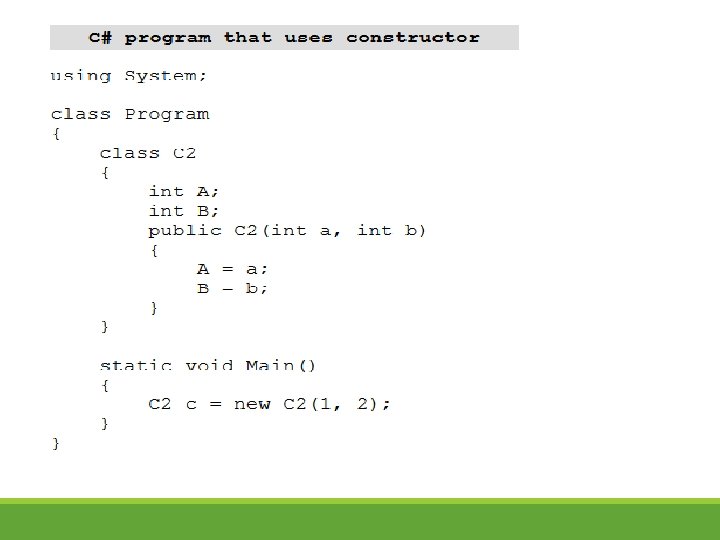
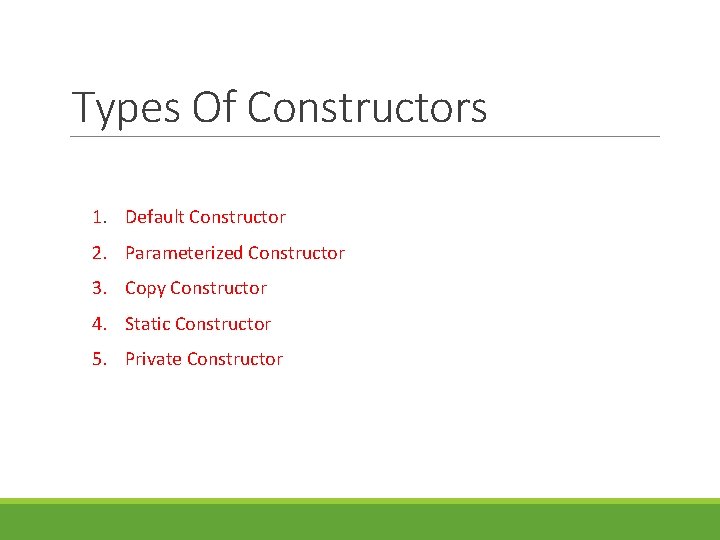
Types Of Constructors 1. Default Constructor 2. Parameterized Constructor 3. Copy Constructor 4. Static Constructor 5. Private Constructor
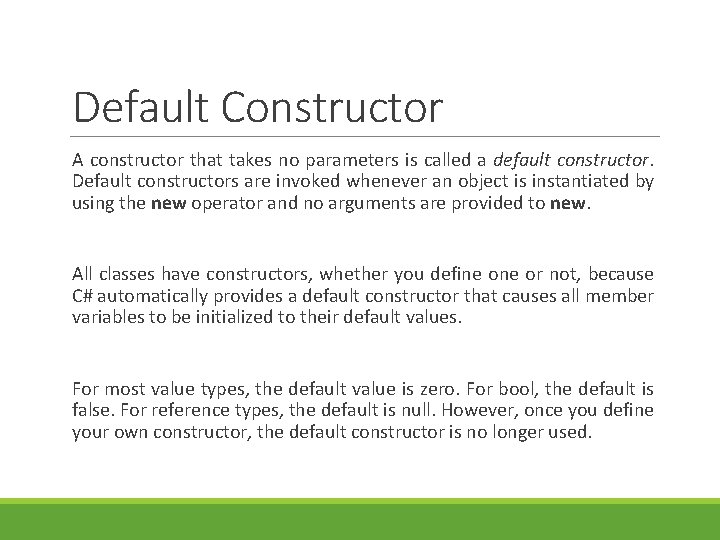
Default Constructor A constructor that takes no parameters is called a default constructor. Default constructors are invoked whenever an object is instantiated by using the new operator and no arguments are provided to new. All classes have constructors, whether you define or not, because C# automatically provides a default constructor that causes all member variables to be initialized to their default values. For most value types, the default value is zero. For bool, the default is false. For reference types, the default is null. However, once you define your own constructor, the default constructor is no longer used.
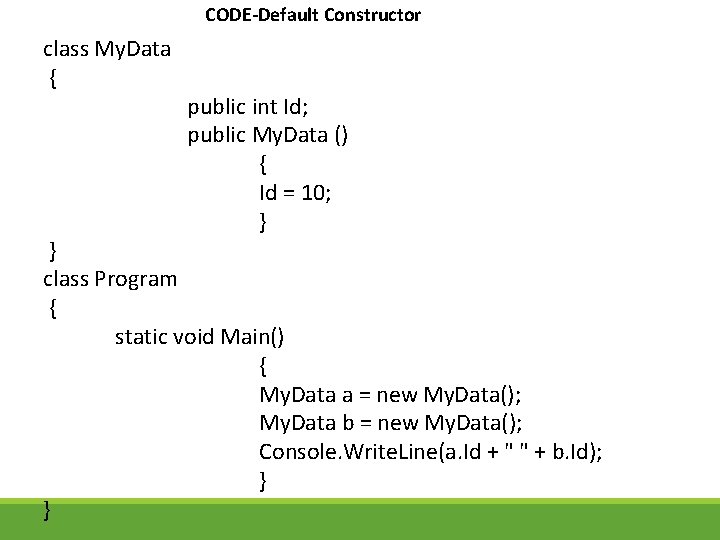
CODE-Default Constructor class My. Data { public int Id; public My. Data () { Id = 10; } } class Program { static void Main() { My. Data a = new My. Data(); My. Data b = new My. Data(); Console. Write. Line(a. Id + " " + b. Id); } }
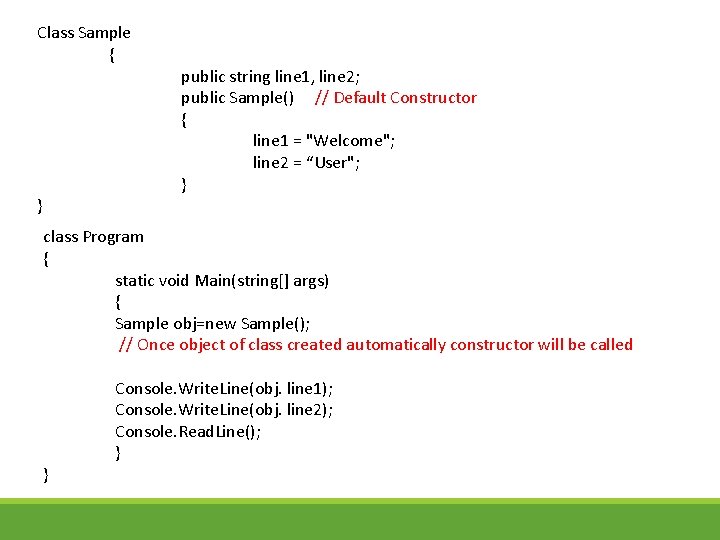
Class Sample { } public string line 1, line 2; public Sample() // Default Constructor { line 1 = "Welcome"; line 2 = “User"; } class Program { static void Main(string[] args) { Sample obj=new Sample(); // Once object of class created automatically constructor will be called } Console. Write. Line(obj. line 1); Console. Write. Line(obj. line 2); Console. Read. Line(); }
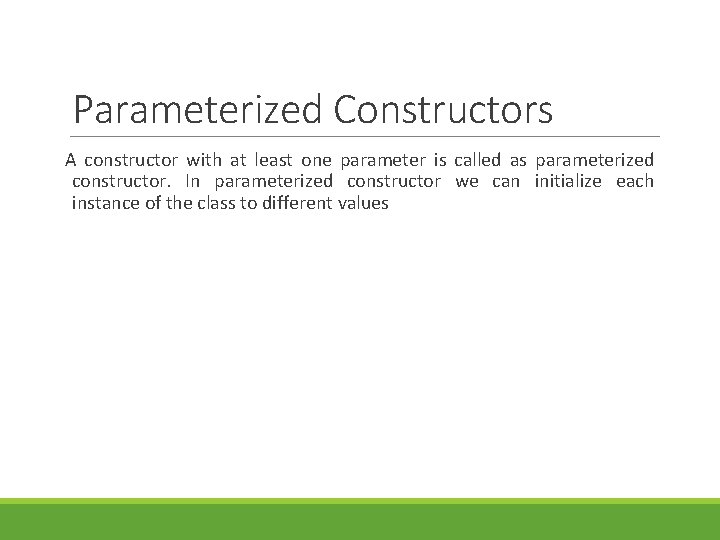
Parameterized Constructors A constructor with at least one parameter is called as parameterized constructor. In parameterized constructor we can initialize each instance of the class to different values
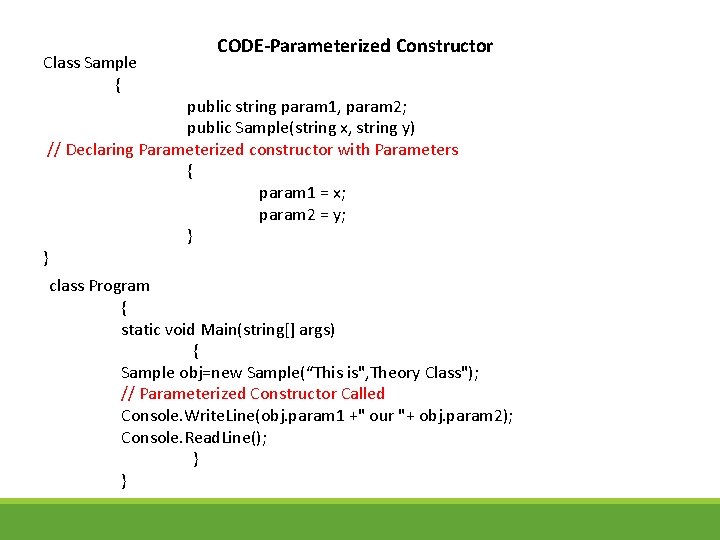
Class Sample { CODE-Parameterized Constructor public string param 1, param 2; public Sample(string x, string y) // Declaring Parameterized constructor with Parameters { param 1 = x; param 2 = y; } } class Program { static void Main(string[] args) { Sample obj=new Sample(“This is", Theory Class"); // Parameterized Constructor Called Console. Write. Line(obj. param 1 +" our "+ obj. param 2); Console. Read. Line(); } }
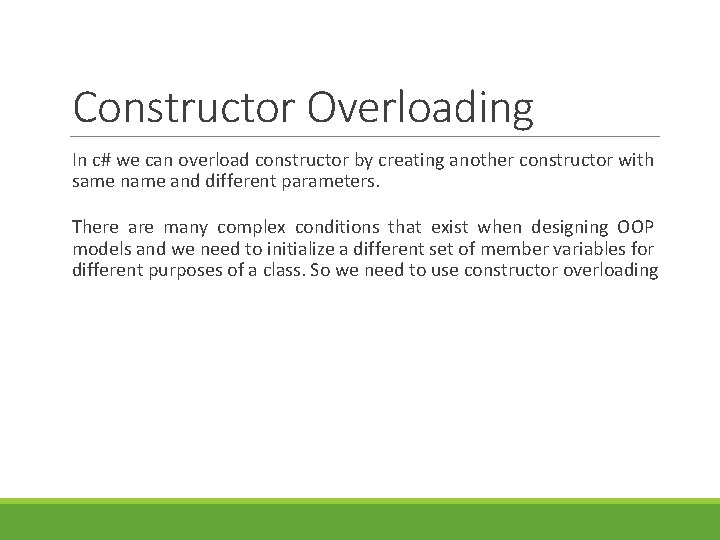
Constructor Overloading In c# we can overload constructor by creating another constructor with same name and different parameters. There are many complex conditions that exist when designing OOP models and we need to initialize a different set of member variables for different purposes of a class. So we need to use constructor overloading
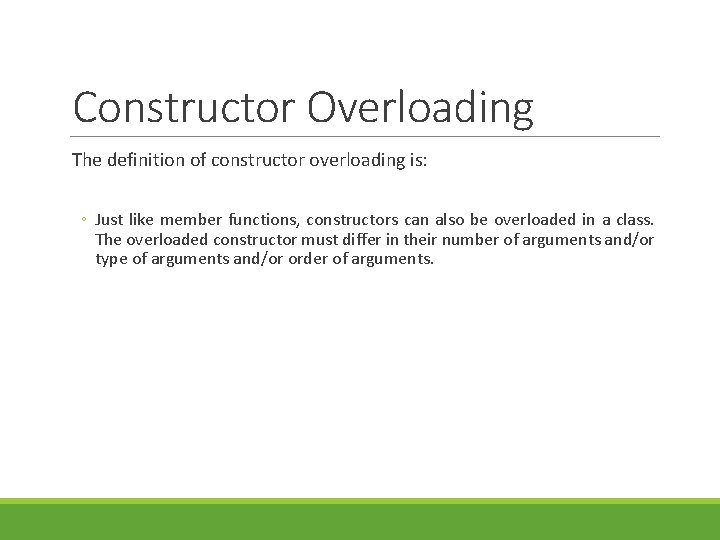
Constructor Overloading The definition of constructor overloading is: ◦ Just like member functions, constructors can also be overloaded in a class. The overloaded constructor must differ in their number of arguments and/or type of arguments and/or order of arguments.
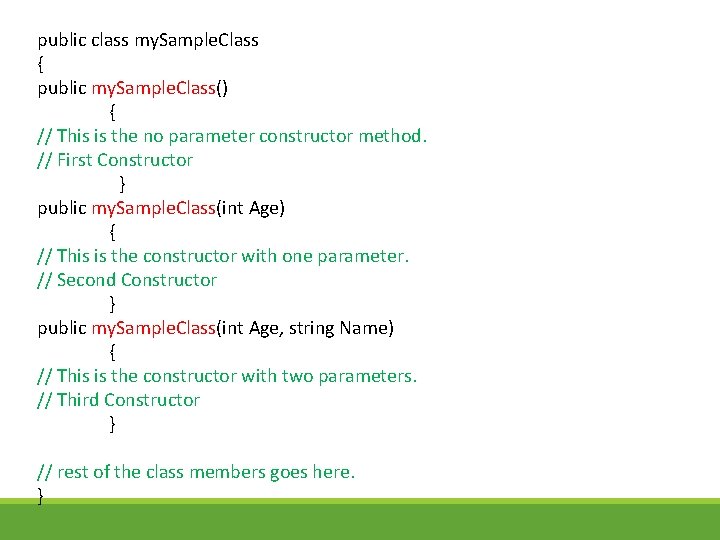
public class my. Sample. Class { public my. Sample. Class() { // This is the no parameter constructor method. // First Constructor } public my. Sample. Class(int Age) { // This is the constructor with one parameter. // Second Constructor } public my. Sample. Class(int Age, string Name) { // This is the constructor with two parameters. // Third Constructor } // rest of the class members goes here. }
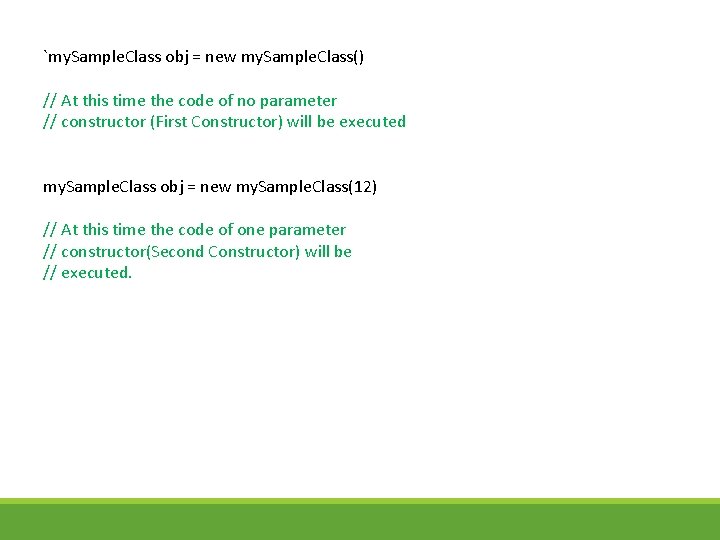
`my. Sample. Class obj = new my. Sample. Class() // At this time the code of no parameter // constructor (First Constructor) will be executed my. Sample. Class obj = new my. Sample. Class(12) // At this time the code of one parameter // constructor(Second Constructor) will be // executed.
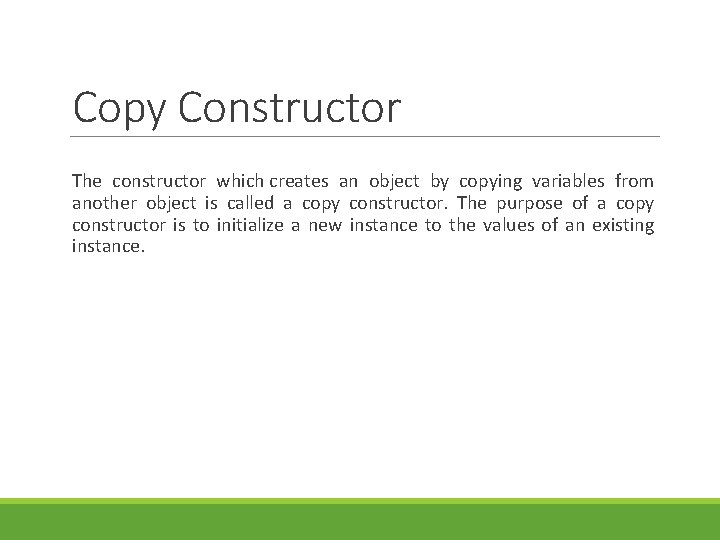
Copy Constructor The constructor which creates an object by copying variables from another object is called a copy constructor. The purpose of a copy constructor is to initialize a new instance to the values of an existing instance.
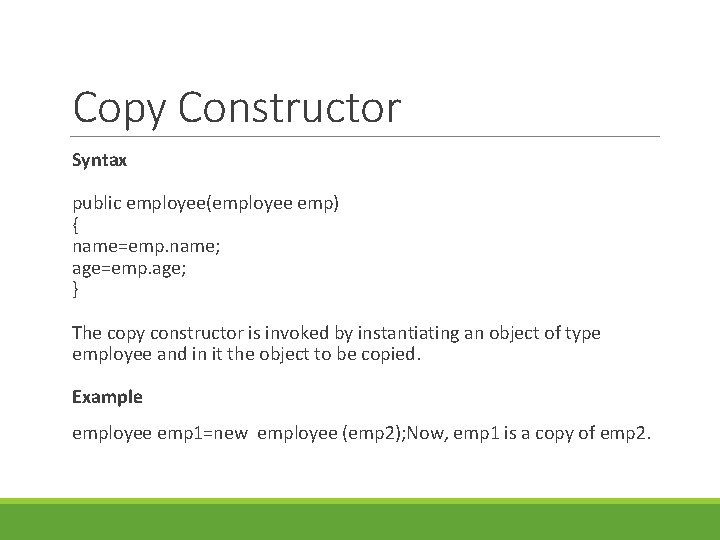
Copy Constructor Syntax public employee(employee emp) { name=emp. name; age=emp. age; } The copy constructor is invoked by instantiating an object of type employee and in it the object to be copied. Example employee emp 1=new employee (emp 2); Now, emp 1 is a copy of emp 2.
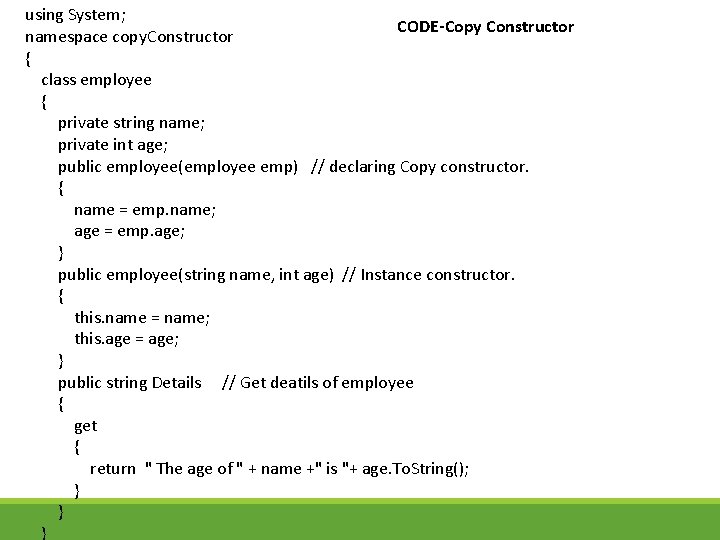
using System; CODE-Copy Constructor namespace copy. Constructor { class employee { private string name; private int age; public employee(employee emp) // declaring Copy constructor. { name = emp. name; age = emp. age; } public employee(string name, int age) // Instance constructor. { this. name = name; this. age = age; } public string Details // Get deatils of employee { get { return " The age of " + name +" is "+ age. To. String(); } } }
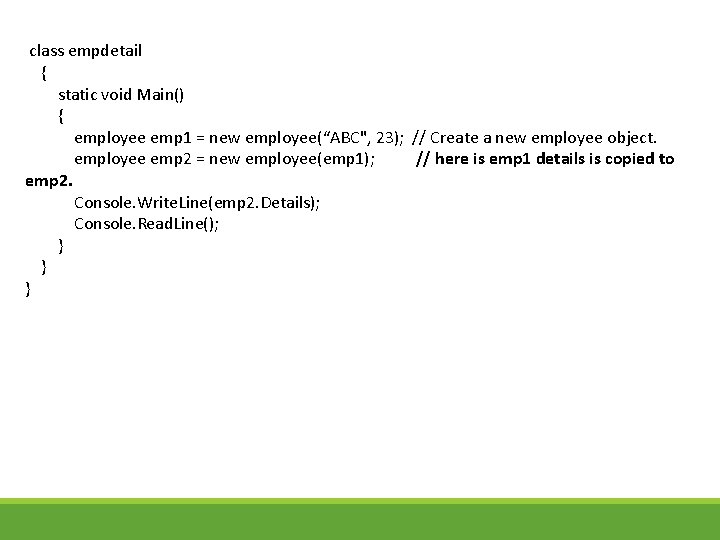
class empdetail { static void Main() { employee emp 1 = new employee(“ABC", 23); // Create a new employee object. employee emp 2 = new employee(emp 1); // here is emp 1 details is copied to emp 2. Console. Write. Line(emp 2. Details); Console. Read. Line(); } } }
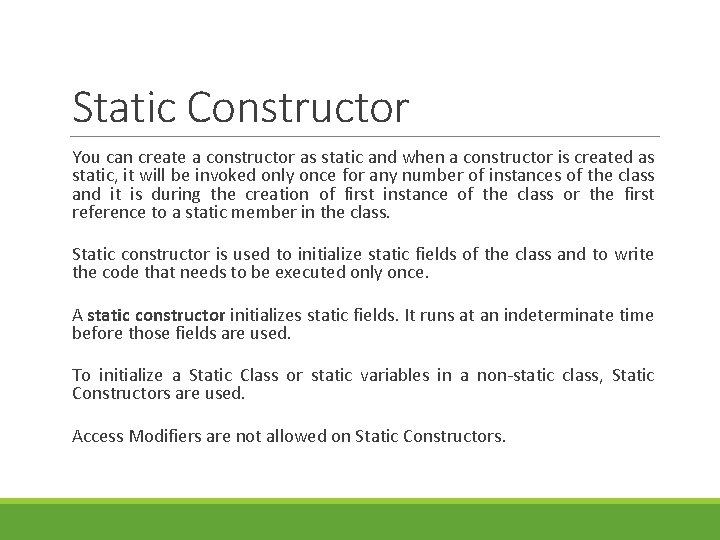
Static Constructor You can create a constructor as static and when a constructor is created as static, it will be invoked only once for any number of instances of the class and it is during the creation of first instance of the class or the first reference to a static member in the class. Static constructor is used to initialize static fields of the class and to write the code that needs to be executed only once. A static constructor initializes static fields. It runs at an indeterminate time before those fields are used. To initialize a Static Class or static variables in a non-static class, Static Constructors are used. Access Modifiers are not allowed on Static Constructors.
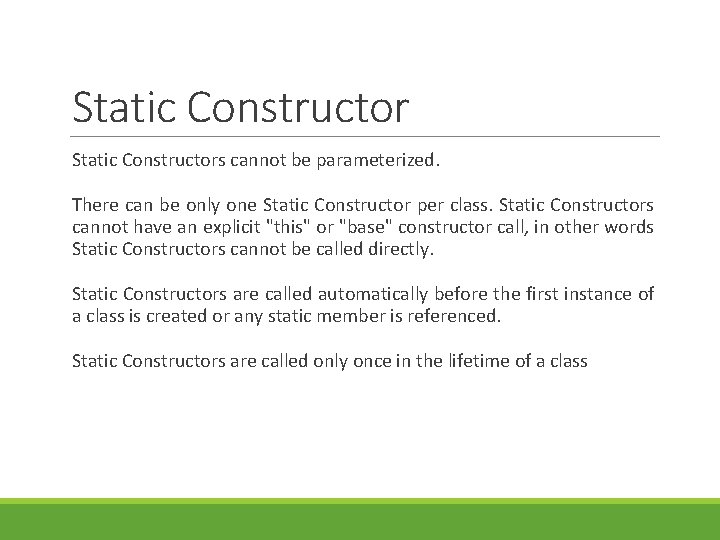
Static Constructors cannot be parameterized. There can be only one Static Constructor per class. Static Constructors cannot have an explicit "this" or "base" constructor call, in other words Static Constructors cannot be called directly. Static Constructors are called automatically before the first instance of a class is created or any static member is referenced. Static Constructors are called only once in the lifetime of a class
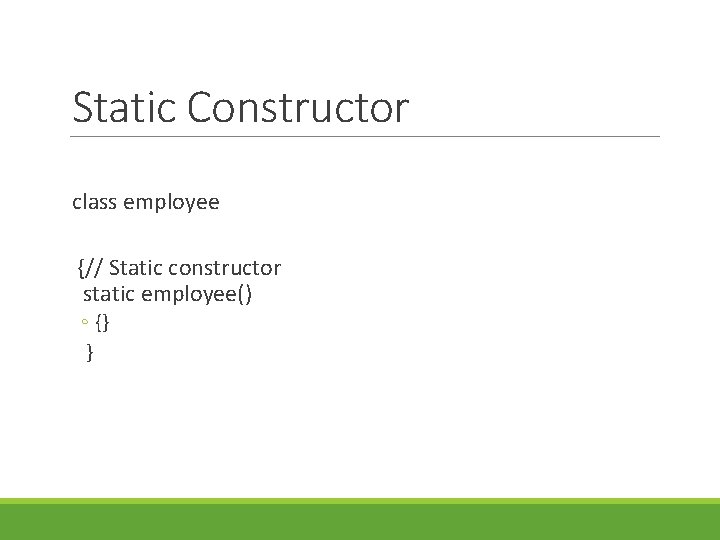
Static Constructor class employee {// Static constructor static employee() ◦ {} }
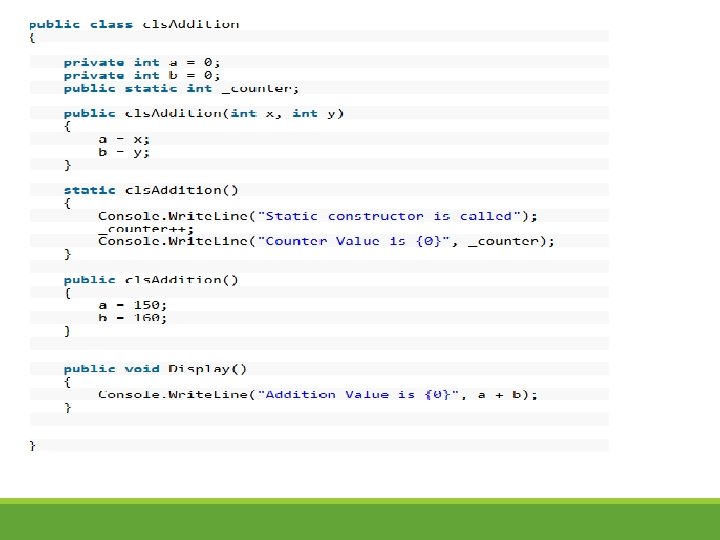
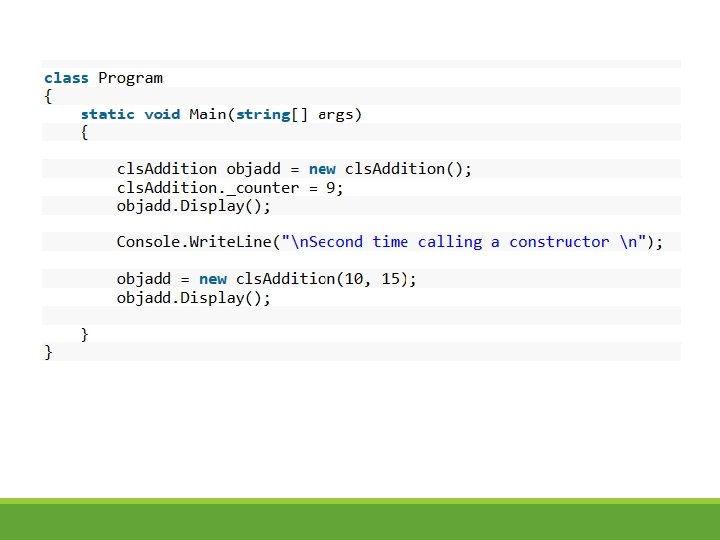
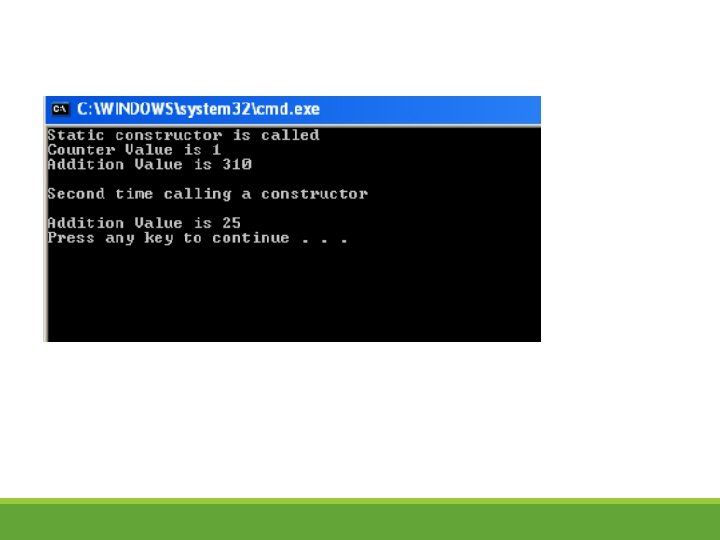
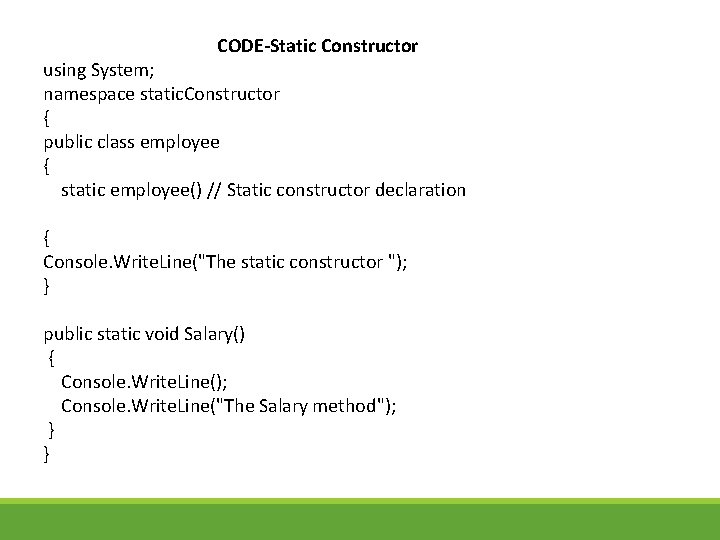
CODE-Static Constructor using System; namespace static. Constructor { public class employee { static employee() // Static constructor declaration { Console. Write. Line("The static constructor "); } public static void Salary() { Console. Write. Line(); Console. Write. Line("The Salary method"); } }
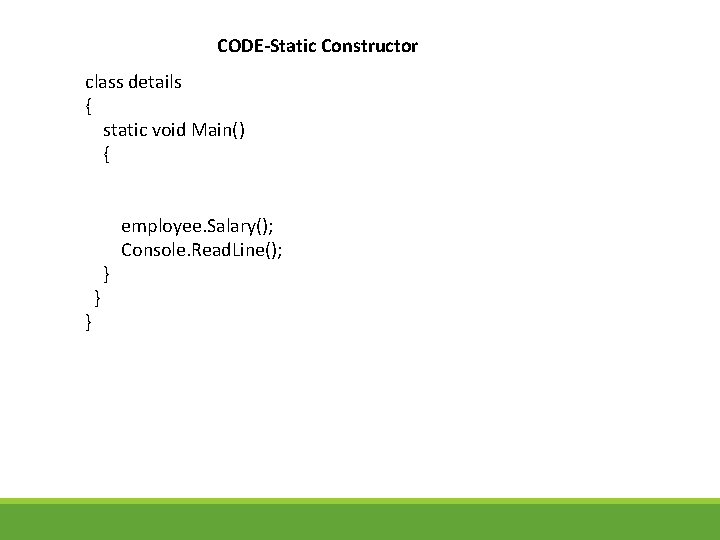
CODE-Static Constructor class details { static void Main() { } } } employee. Salary(); Console. Read. Line();
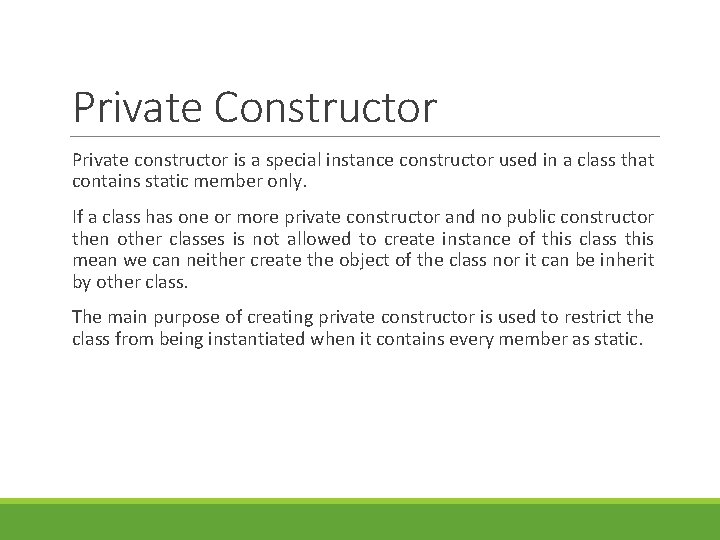
Private Constructor Private constructor is a special instance constructor used in a class that contains static member only. If a class has one or more private constructor and no public constructor then other classes is not allowed to create instance of this class this mean we can neither create the object of the class nor it can be inherit by other class. The main purpose of creating private constructor is used to restrict the class from being instantiated when it contains every member as static.
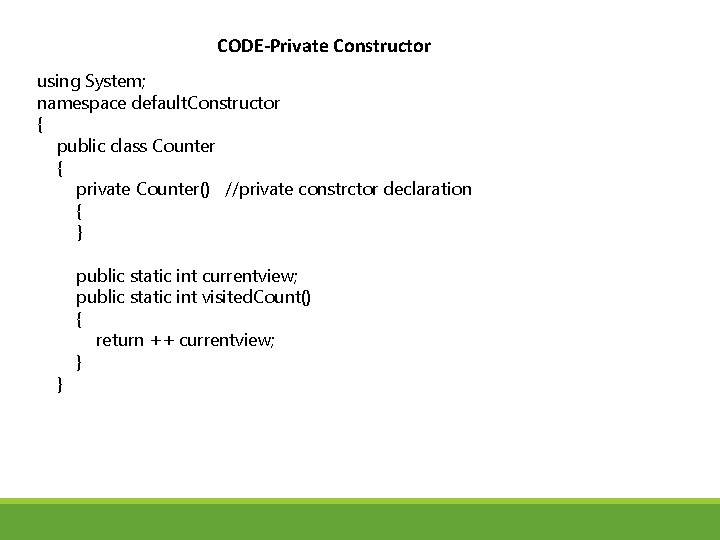
CODE-Private Constructor using System; namespace default. Constructor { public class Counter { private Counter() //private constrctor declaration { } } public static int currentview; public static int visited. Count() { return ++ currentview; }
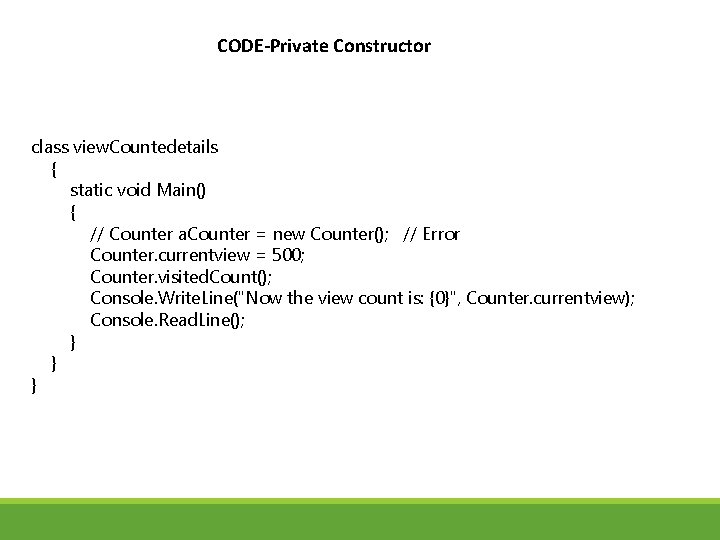
CODE-Private Constructor class view. Countedetails { static void Main() { // Counter a. Counter = new Counter(); // Error Counter. currentview = 500; Counter. visited. Count(); Console. Write. Line("Now the view count is: {0}", Counter. currentview); Console. Read. Line(); } } }
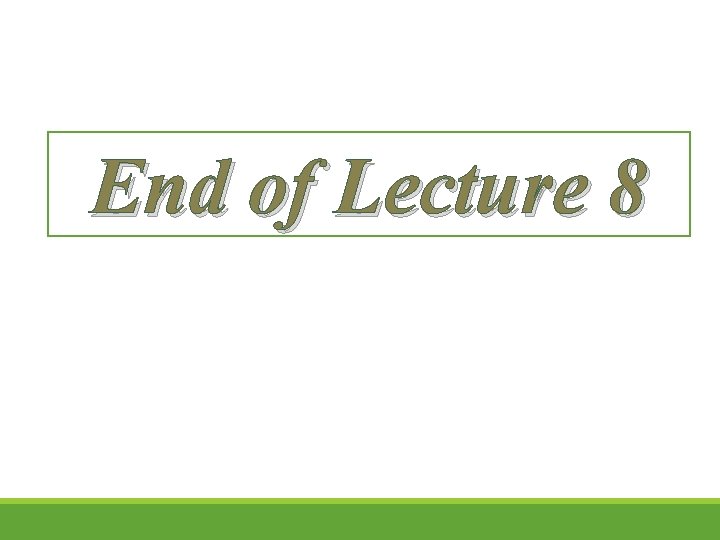
End of Lecture 8
01:640:244 lecture notes - lecture 15: plat, idah, farad
Padi quiz 1 answers
Contending nationalist loyalties exist whenever
Conflict occurs whenever
Whenever the weather is cold
Whenever an interaction occurs in a system
Whenever jon delivers a newspaper to the zapanta house
Fanboys and swabi
Whenever possible, child car safety seats should be placed
Heat energy is transferred by conduction whenever molecules
Whenever there is too little friction or traction
An interaction occurs when:
Whenever or when ever
11factorial
Urgent move emt
Interaction occurs when
Whenever
Speak right to my heart
I will follow you wherever you may go
A current is said to exist whenever _____.
Section 2-4 chemical reactions and enzymes
Copy constructor in java
Difference between default and parameterized constructor
Shallow copy constructor
Deep copy constructor c++
Characteristics of constructor
Characteristics of constructor
Constructors and destructors in c
Copy constructor vs assignment operator
Abstraction in java
Glm multiply vector by scalar
Sketsa prototype
Filip zavoral
Java string constructor