Using Constructor Program using System namespace Using Constructor
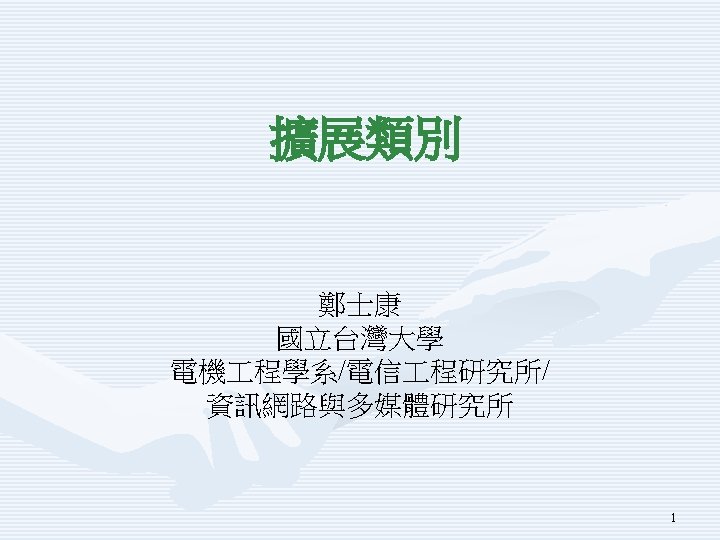
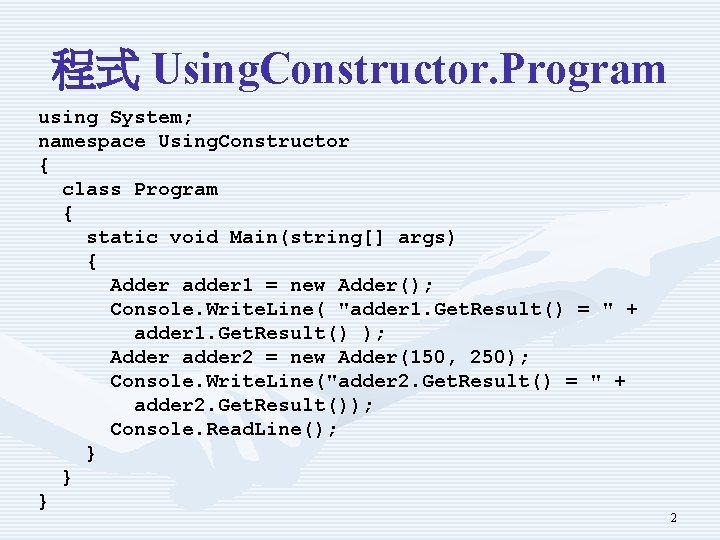
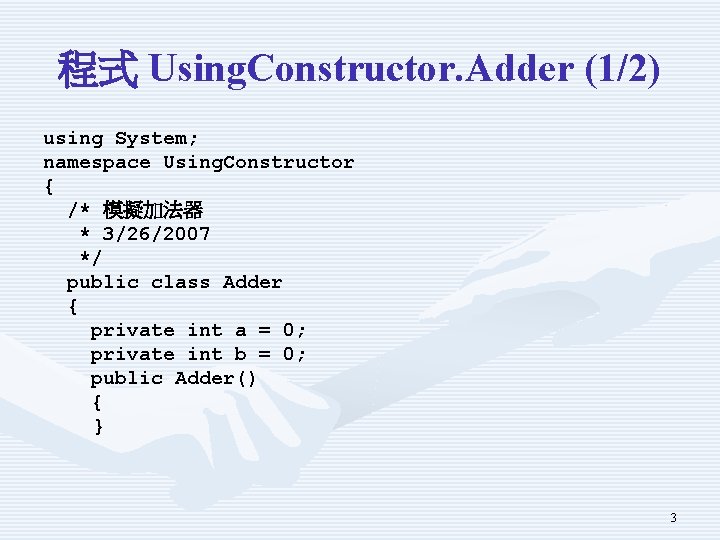
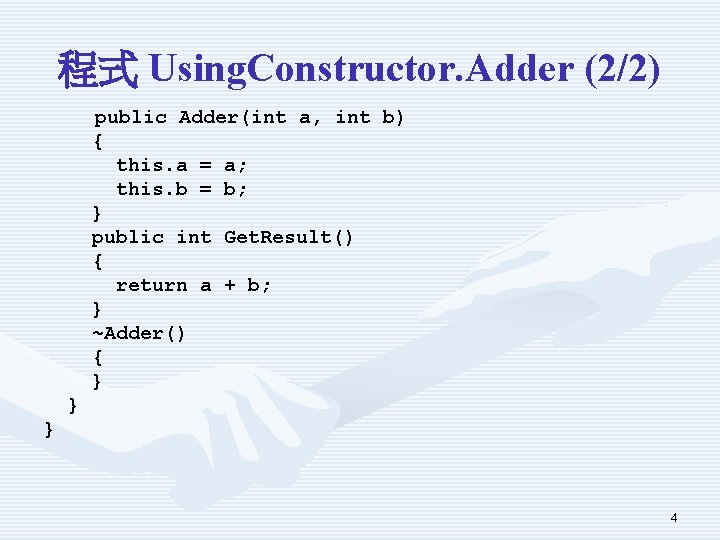
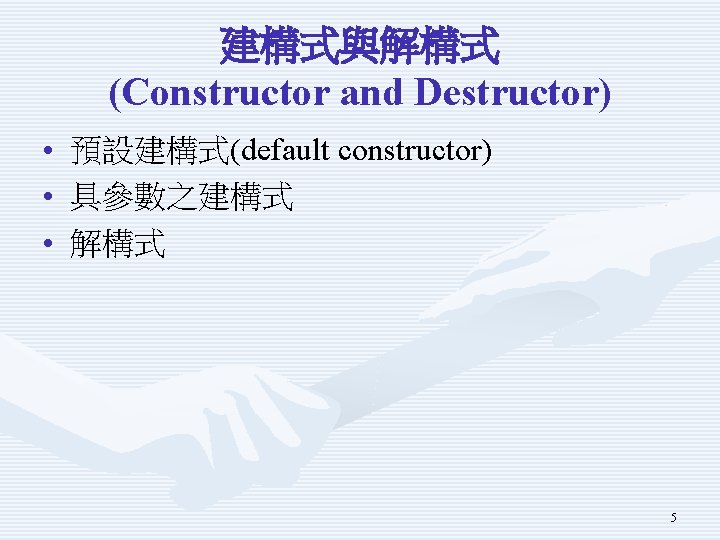
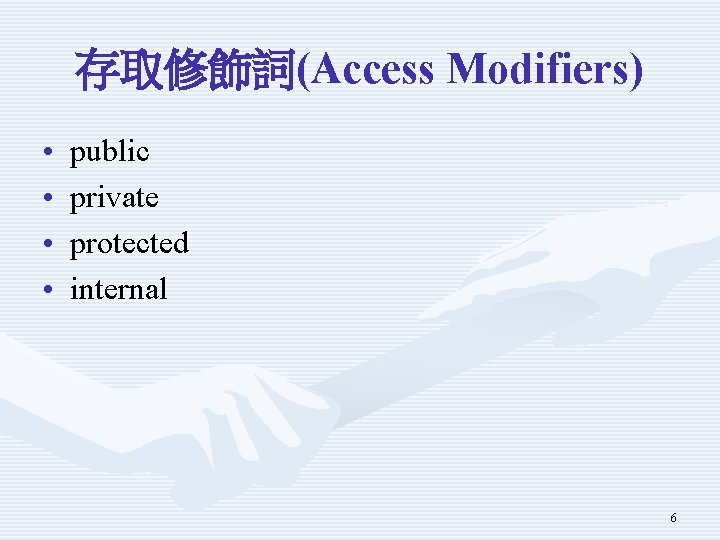
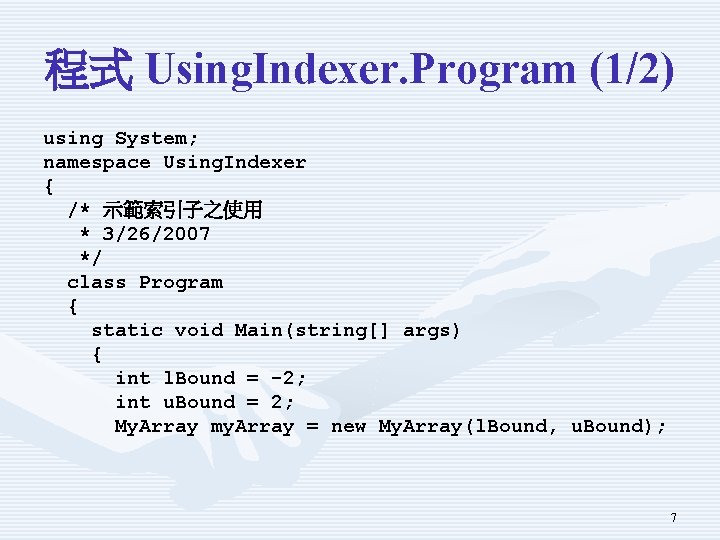
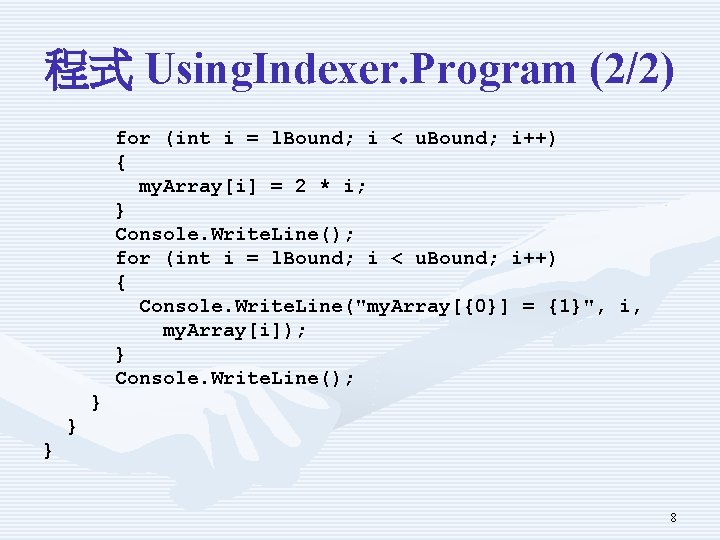
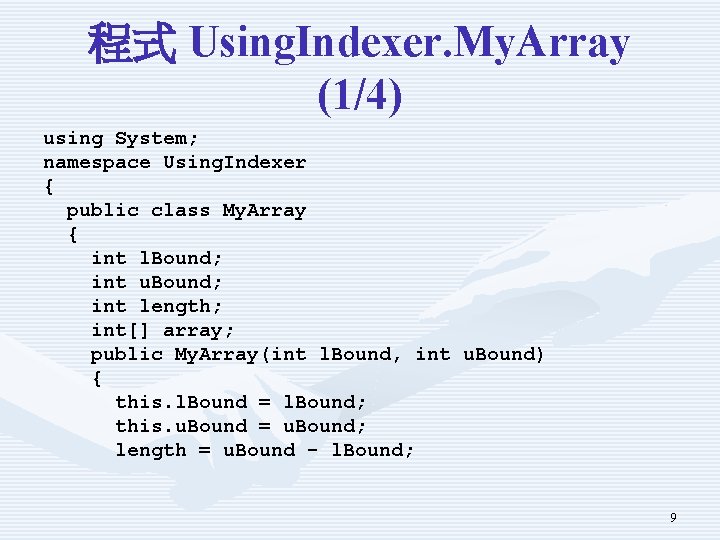
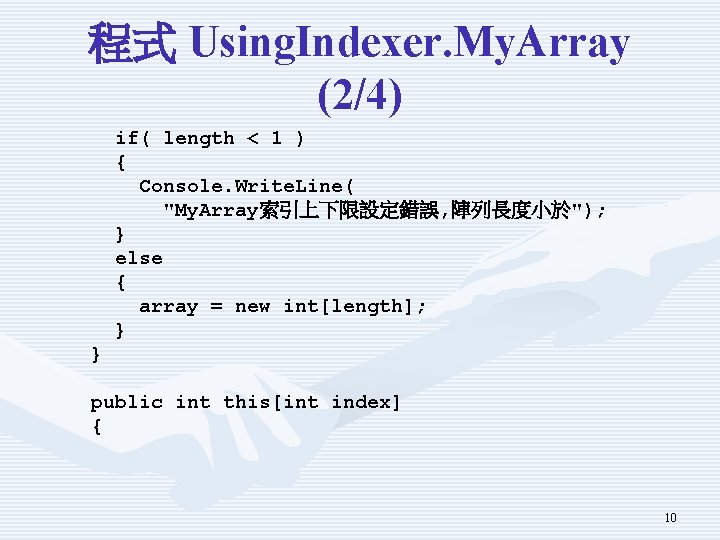
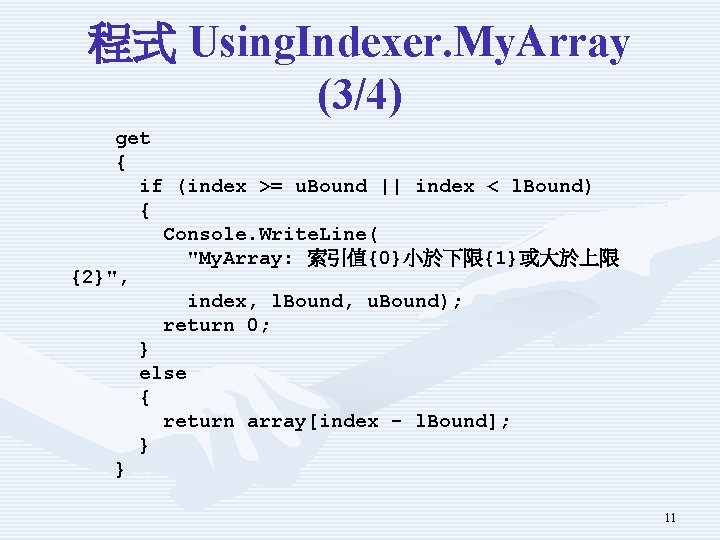
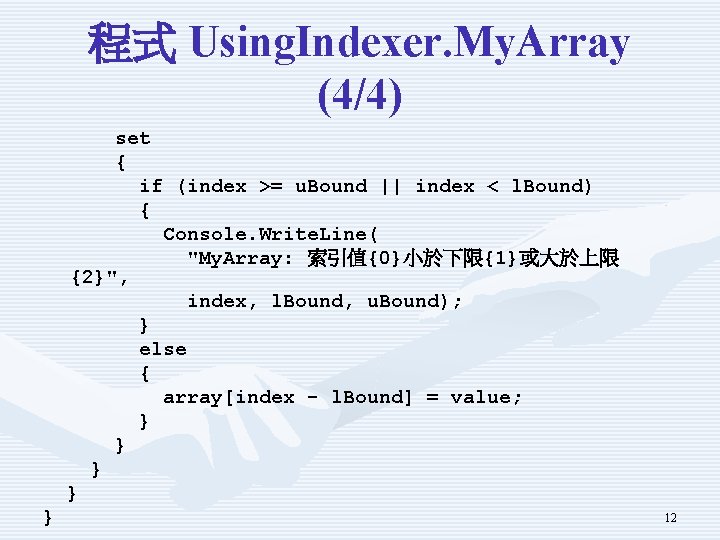
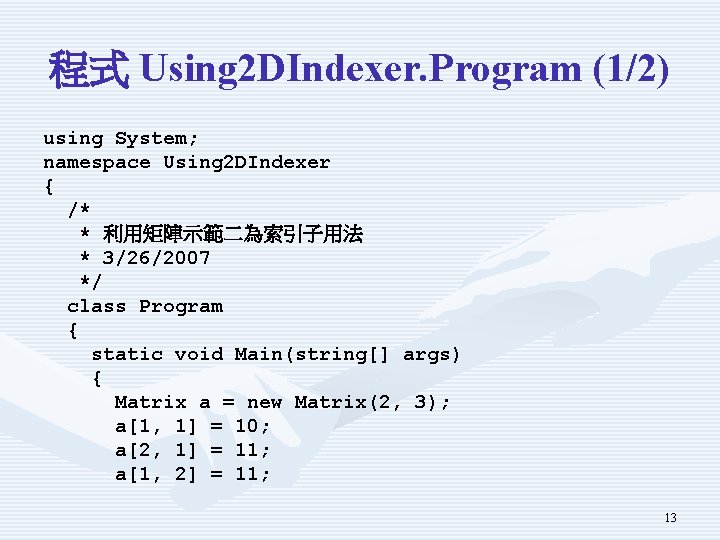
![程式 Using 2 DIndexer. Program (2/2) a[2, 2] = 12; a[1, 3] = 12; 程式 Using 2 DIndexer. Program (2/2) a[2, 2] = 12; a[1, 3] = 12;](https://slidetodoc.com/presentation_image/3173af107d9f961bb07622d8c5a445f4/image-14.jpg)
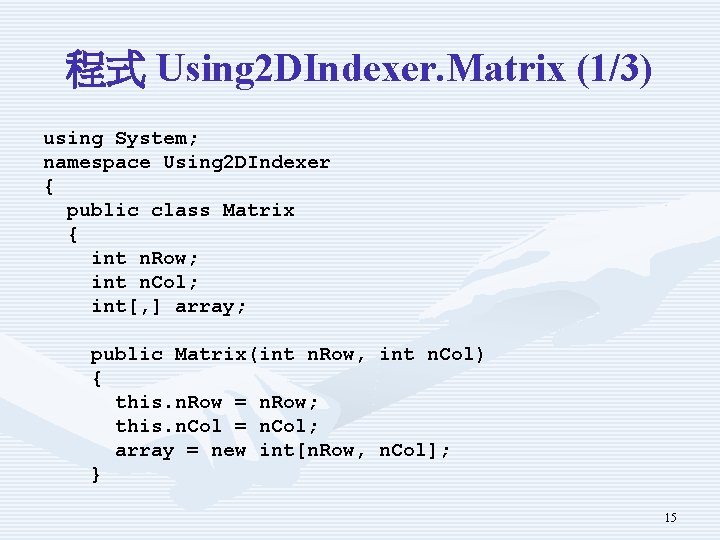
![程式 Using 2 DIndexer. Matrix (2/3) public int this[int i, int j] { get 程式 Using 2 DIndexer. Matrix (2/3) public int this[int i, int j] { get](https://slidetodoc.com/presentation_image/3173af107d9f961bb07622d8c5a445f4/image-16.jpg)
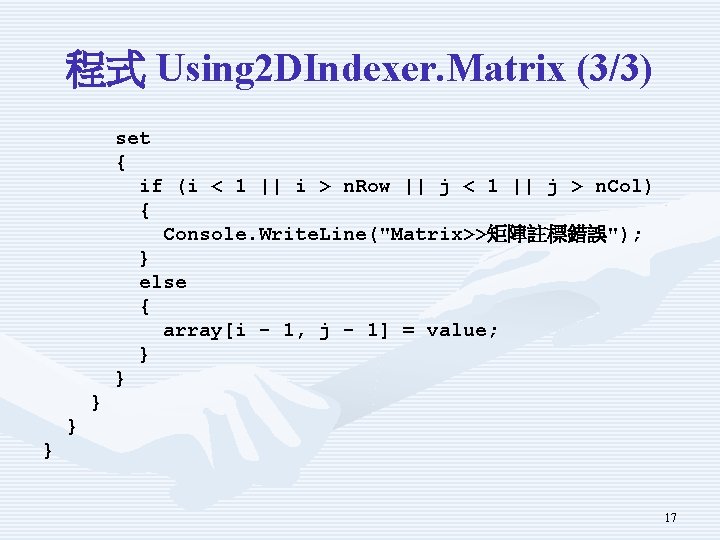
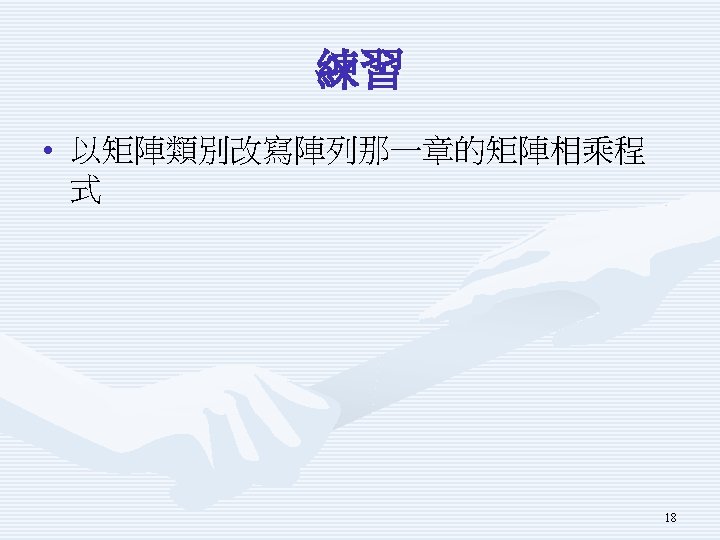
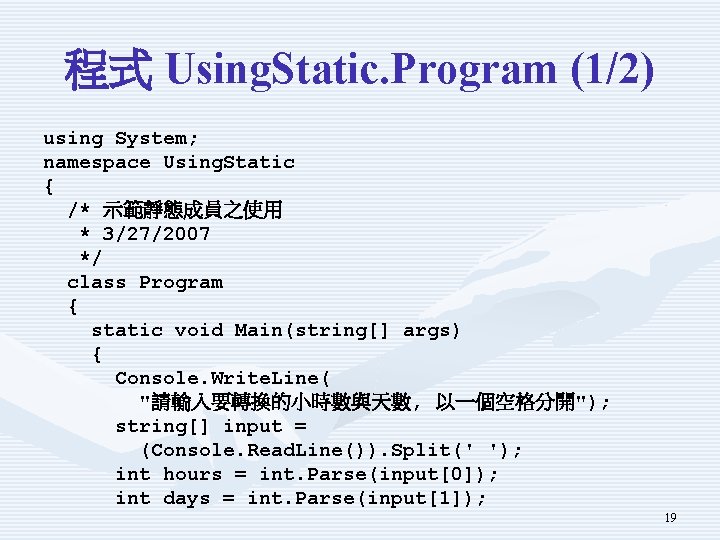
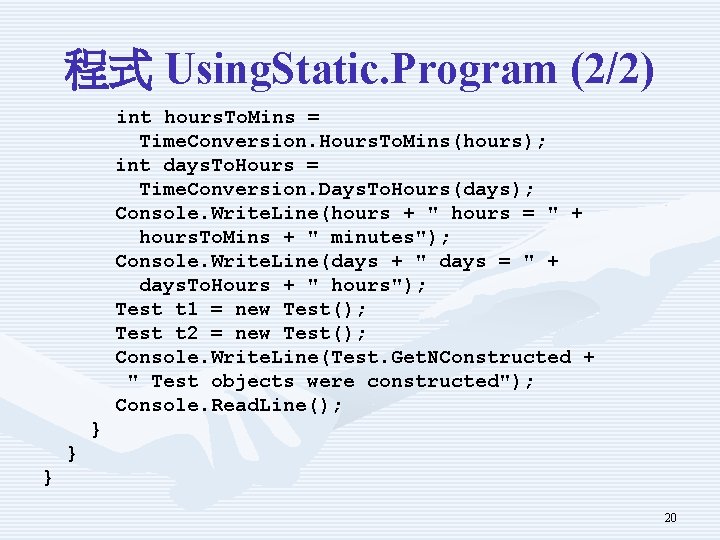
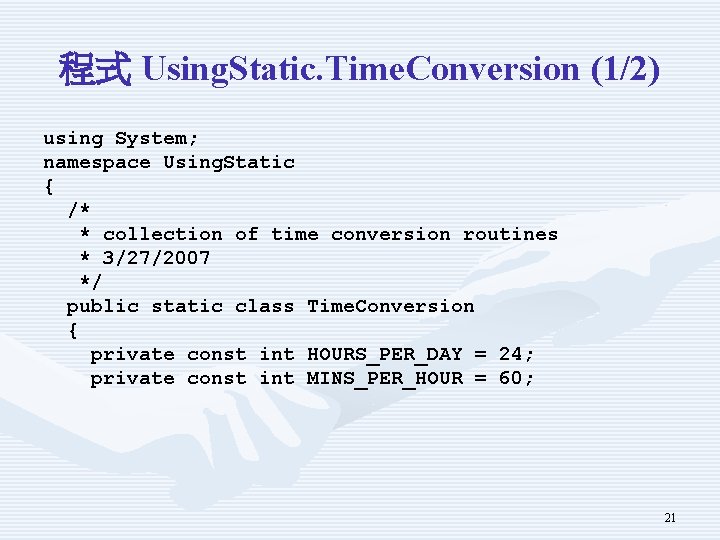
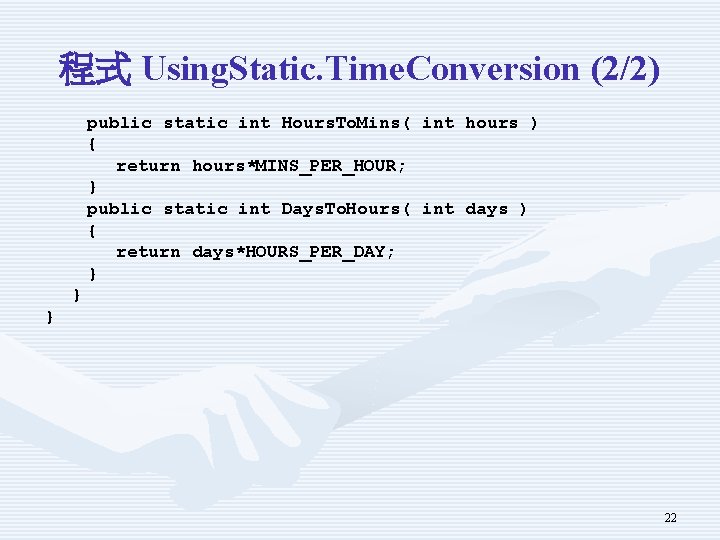
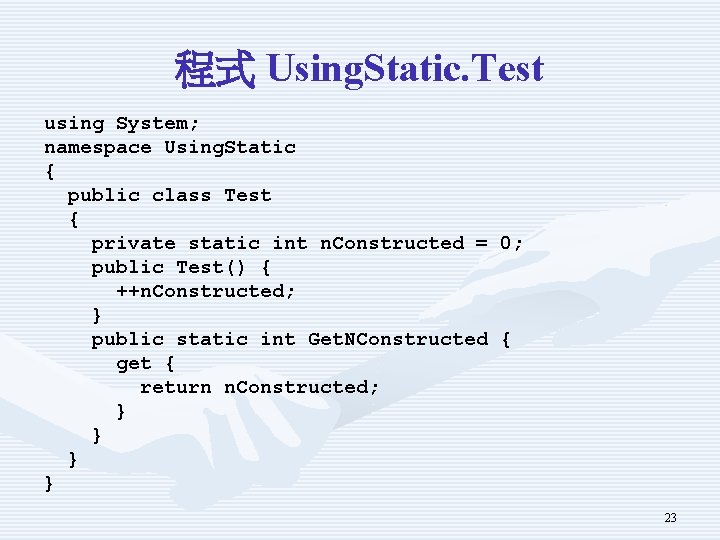
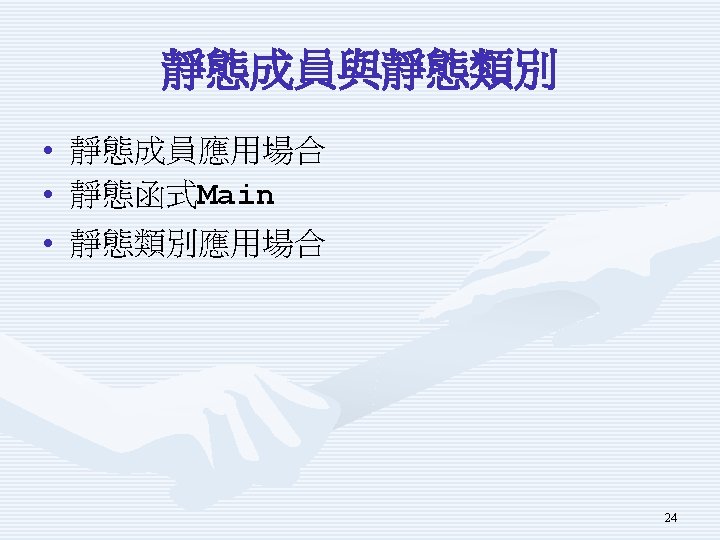
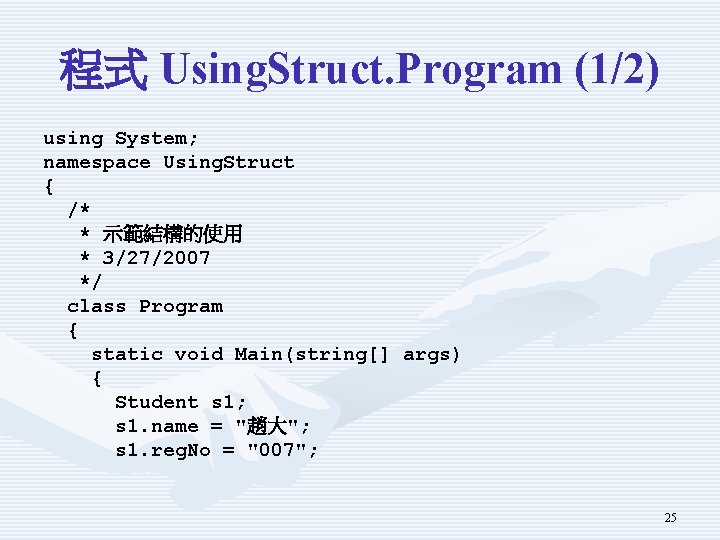
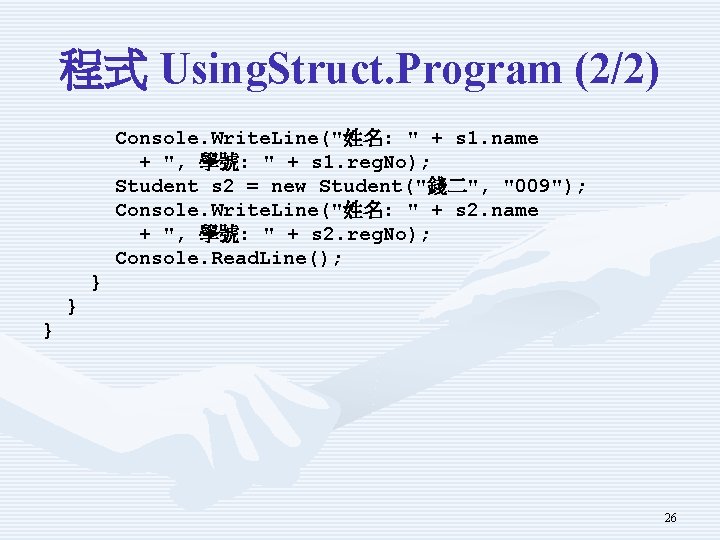
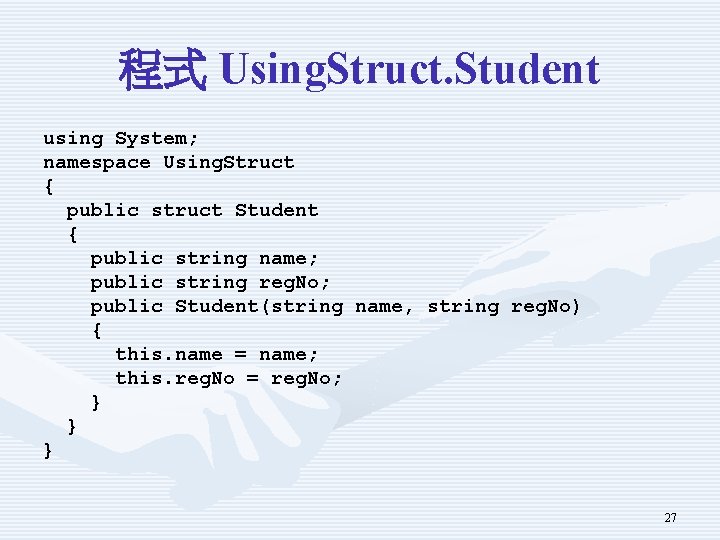
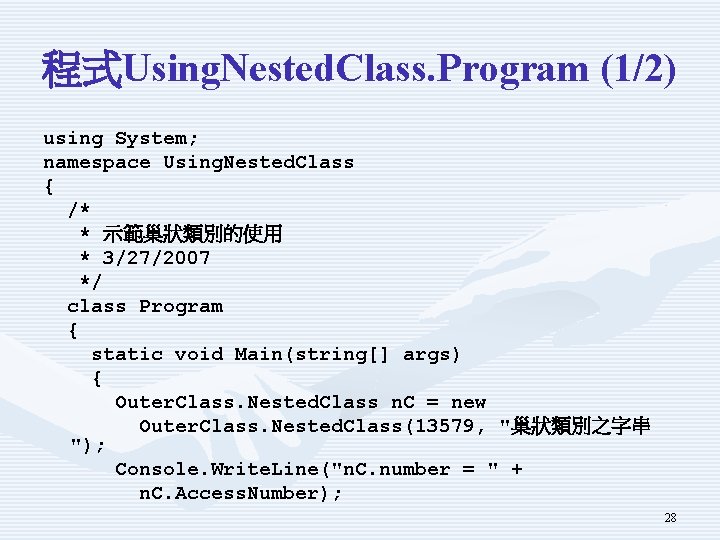
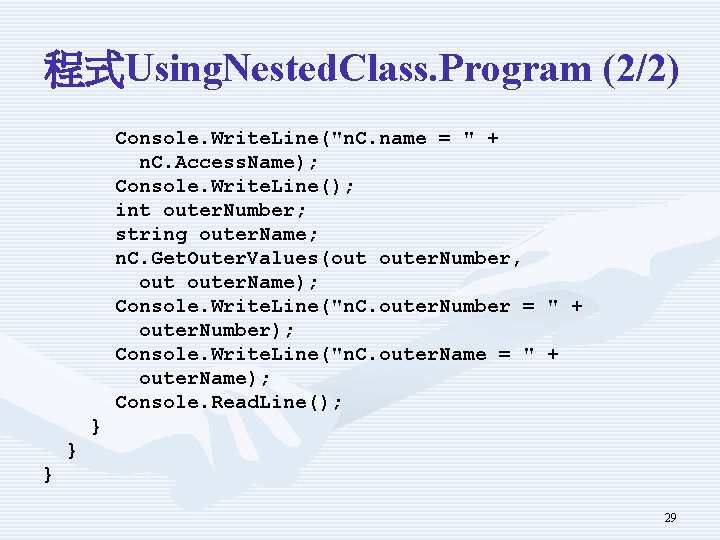
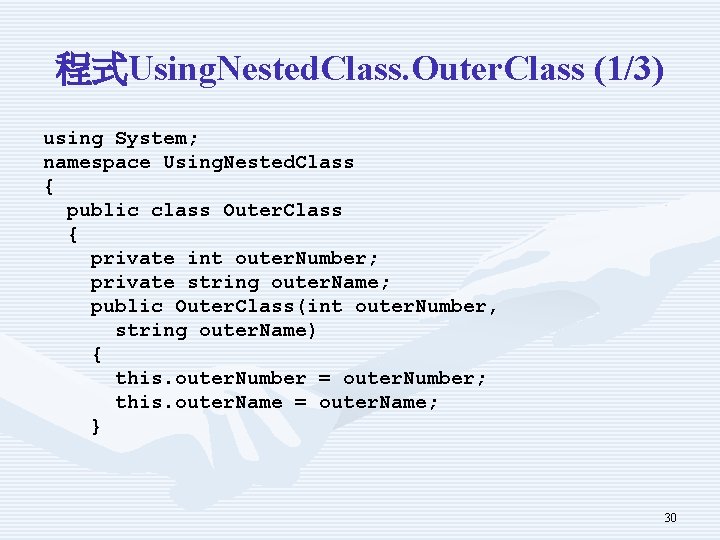
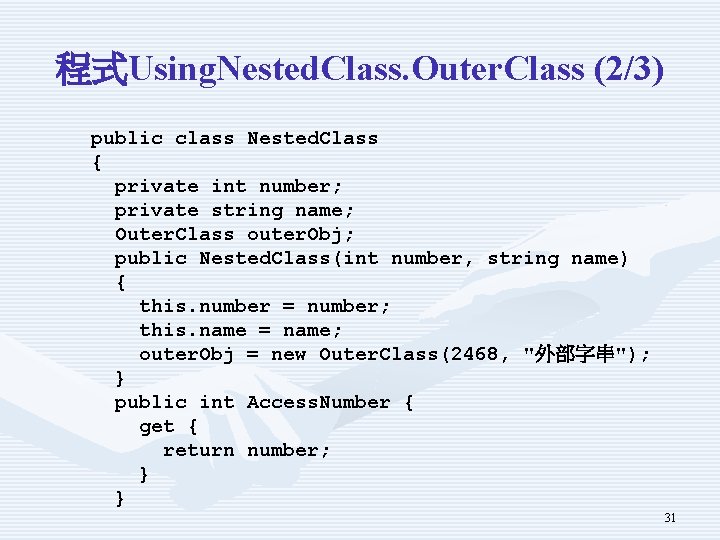
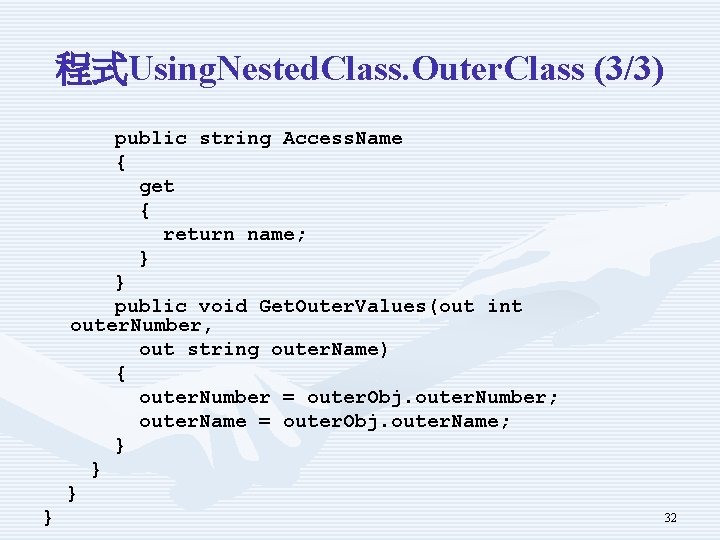
- Slides: 32
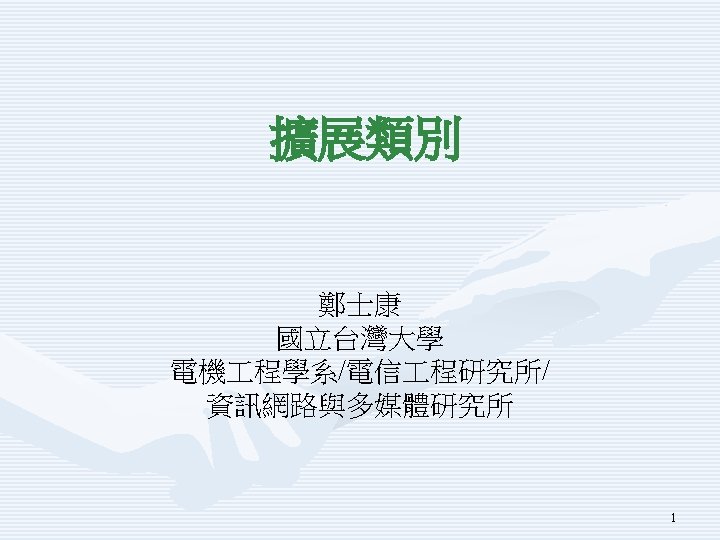
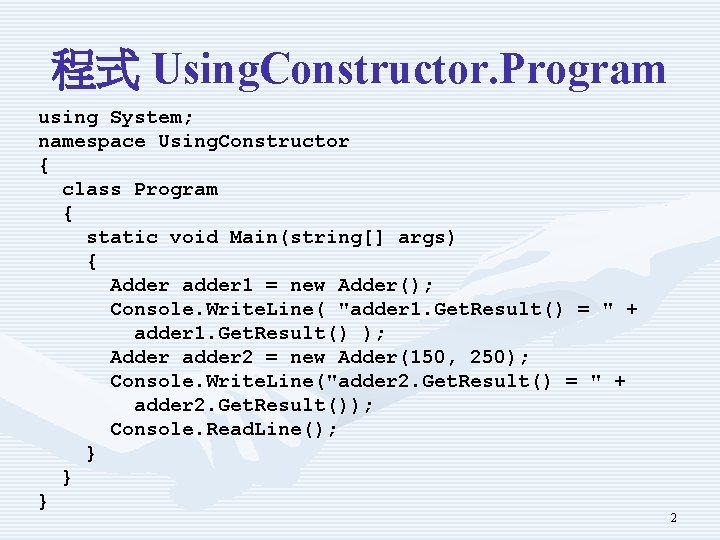
程式 Using. Constructor. Program using System; namespace Using. Constructor { class Program { static void Main(string[] args) { Adder adder 1 = new Adder(); Console. Write. Line( "adder 1. Get. Result() = " + adder 1. Get. Result() ); Adder adder 2 = new Adder(150, 250); Console. Write. Line("adder 2. Get. Result() = " + adder 2. Get. Result()); Console. Read. Line(); } } } 2
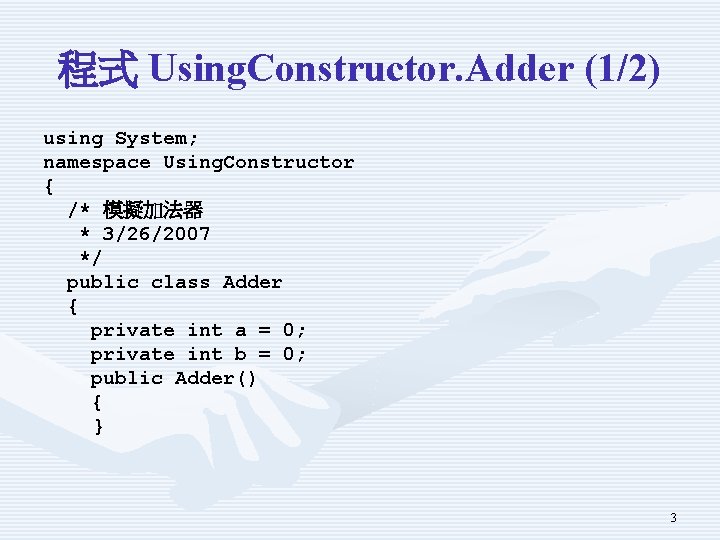
程式 Using. Constructor. Adder (1/2) using System; namespace Using. Constructor { /* 模擬加法器 * 3/26/2007 */ public class Adder { private int a = 0; private int b = 0; public Adder() { } 3
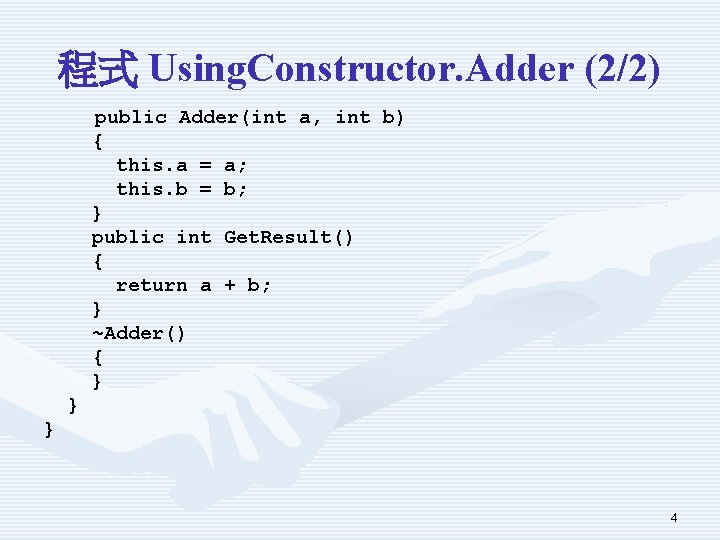
程式 Using. Constructor. Adder (2/2) public Adder(int a, int b) { this. a = a; this. b = b; } public int Get. Result() { return a + b; } ~Adder() { } } } 4
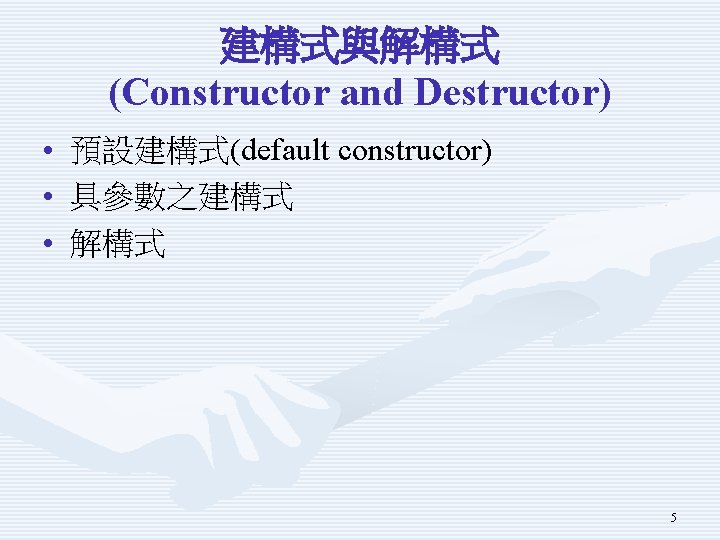
建構式與解構式 (Constructor and Destructor) • 預設建構式(default constructor) • 具參數之建構式 • 解構式 5
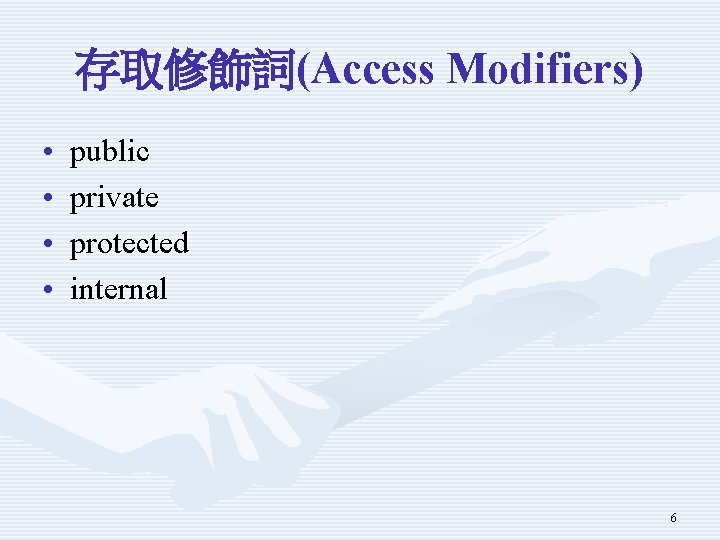
存取修飾詞(Access Modifiers) • • public private protected internal 6
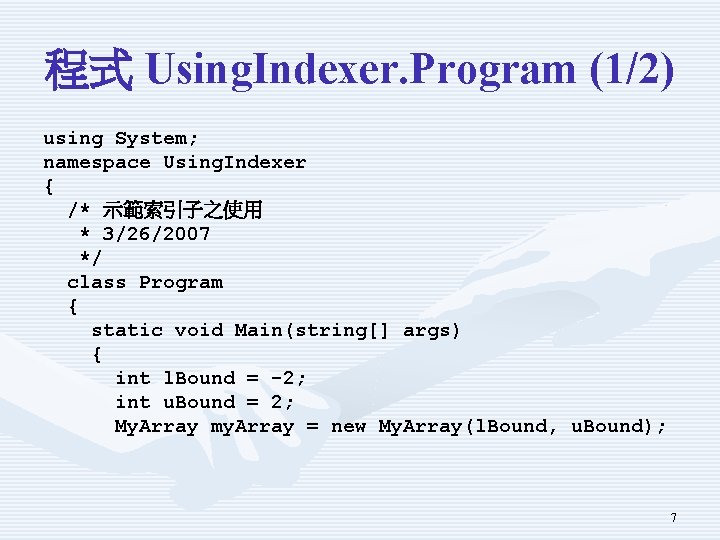
程式 Using. Indexer. Program (1/2) using System; namespace Using. Indexer { /* 示範索引子之使用 * 3/26/2007 */ class Program { static void Main(string[] args) { int l. Bound = -2; int u. Bound = 2; My. Array my. Array = new My. Array(l. Bound, u. Bound); 7
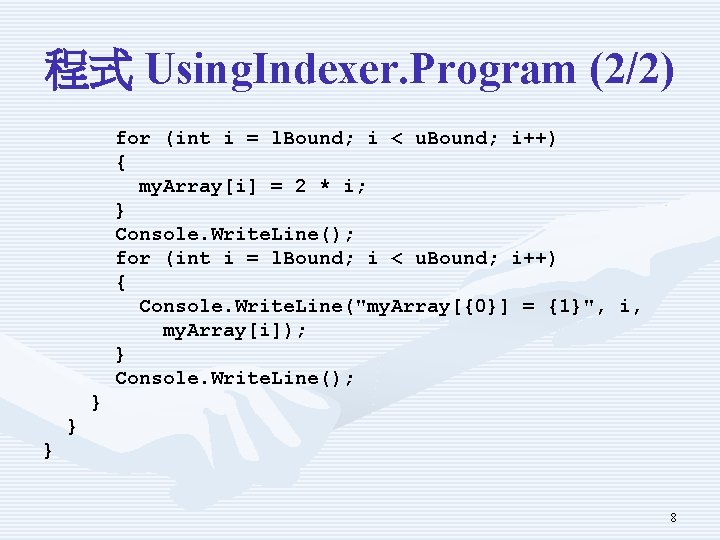
程式 Using. Indexer. Program (2/2) for (int i = l. Bound; i < u. Bound; i++) { my. Array[i] = 2 * i; } Console. Write. Line(); for (int i = l. Bound; i < u. Bound; i++) { Console. Write. Line("my. Array[{0}] = {1}", i, my. Array[i]); } Console. Write. Line(); } } } 8
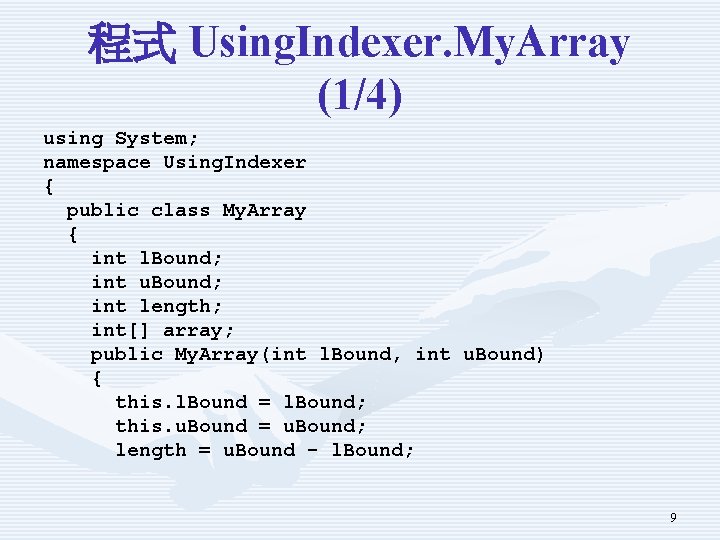
程式 Using. Indexer. My. Array (1/4) using System; namespace Using. Indexer { public class My. Array { int l. Bound; int u. Bound; int length; int[] array; public My. Array(int l. Bound, int u. Bound) { this. l. Bound = l. Bound; this. u. Bound = u. Bound; length = u. Bound - l. Bound; 9
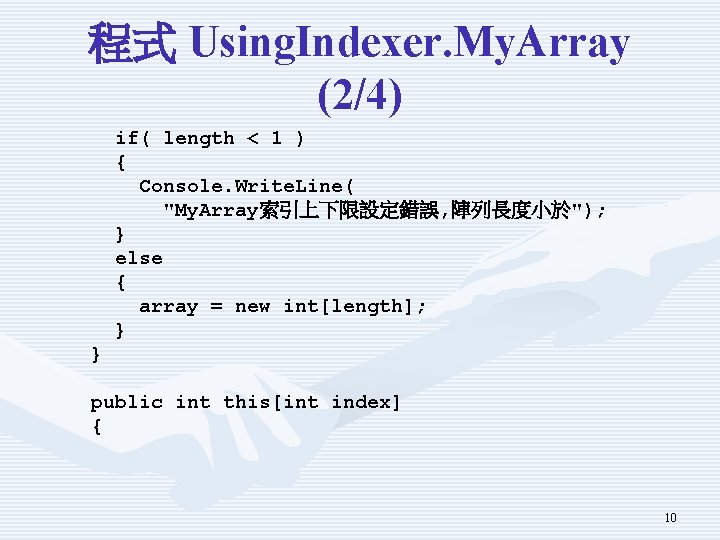
程式 Using. Indexer. My. Array (2/4) if( length < 1 ) { Console. Write. Line( "My. Array索引上下限設定錯誤, 陣列長度小於"); } else { array = new int[length]; } } public int this[int index] { 10
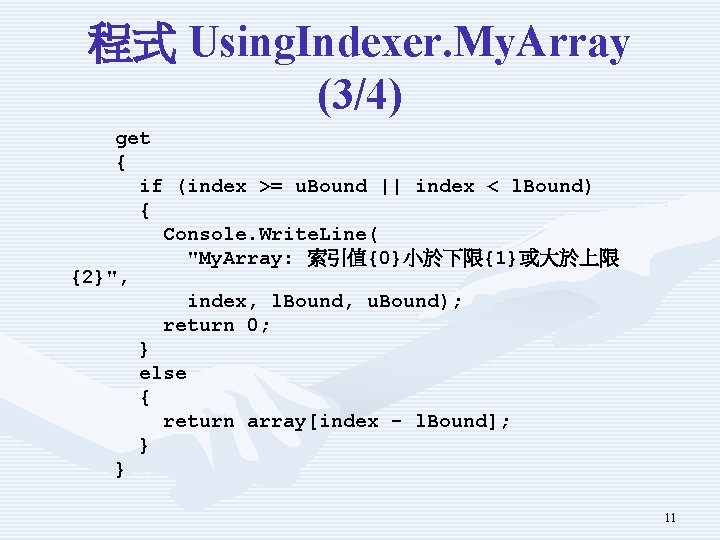
程式 Using. Indexer. My. Array (3/4) get { if (index >= u. Bound || index < l. Bound) { Console. Write. Line( "My. Array: 索引值{0}小於下限{1}或大於上限 {2}", index, l. Bound, u. Bound); return 0; } else { return array[index - l. Bound]; } } 11
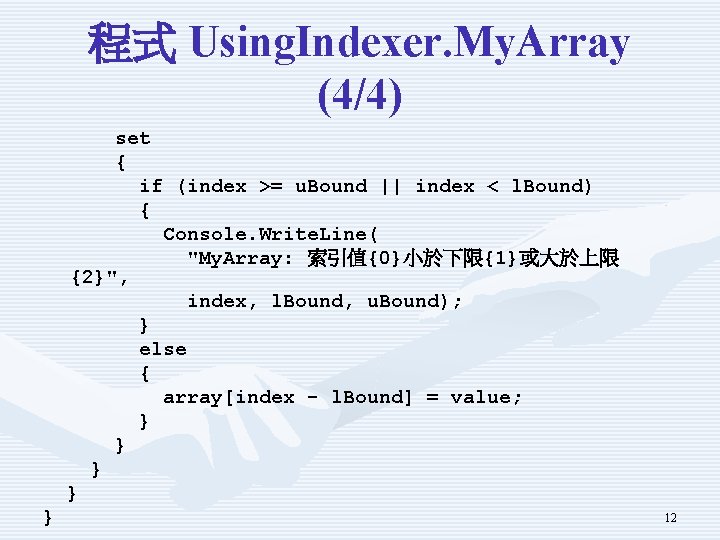
程式 Using. Indexer. My. Array (4/4) set { if (index >= u. Bound || index < l. Bound) { Console. Write. Line( "My. Array: 索引值{0}小於下限{1}或大於上限 {2}", index, l. Bound, u. Bound); } else { array[index - l. Bound] = value; } } } 12
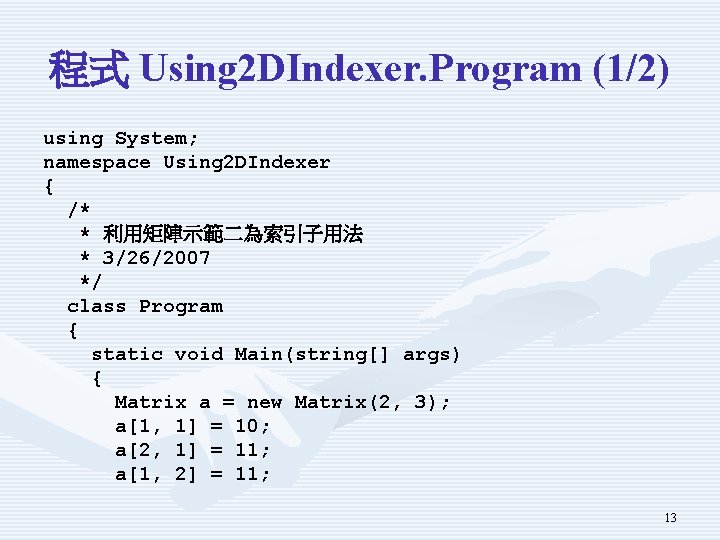
程式 Using 2 DIndexer. Program (1/2) using System; namespace Using 2 DIndexer { /* * 利用矩陣示範二為索引子用法 * 3/26/2007 */ class Program { static void Main(string[] args) { Matrix a = new Matrix(2, 3); a[1, 1] = 10; a[2, 1] = 11; a[1, 2] = 11; 13
![程式 Using 2 DIndexer Program 22 a2 2 12 a1 3 12 程式 Using 2 DIndexer. Program (2/2) a[2, 2] = 12; a[1, 3] = 12;](https://slidetodoc.com/presentation_image/3173af107d9f961bb07622d8c5a445f4/image-14.jpg)
程式 Using 2 DIndexer. Program (2/2) a[2, 2] = 12; a[1, 3] = 12; a[2, 3] = 13; for (int i = 1; i <= 2; i++) { for (int j = 1; j <= 3; j++) { Console. Write(a[i, j] + "t"); } Console. Write. Line(); } Console. Read. Line(); } } } 14
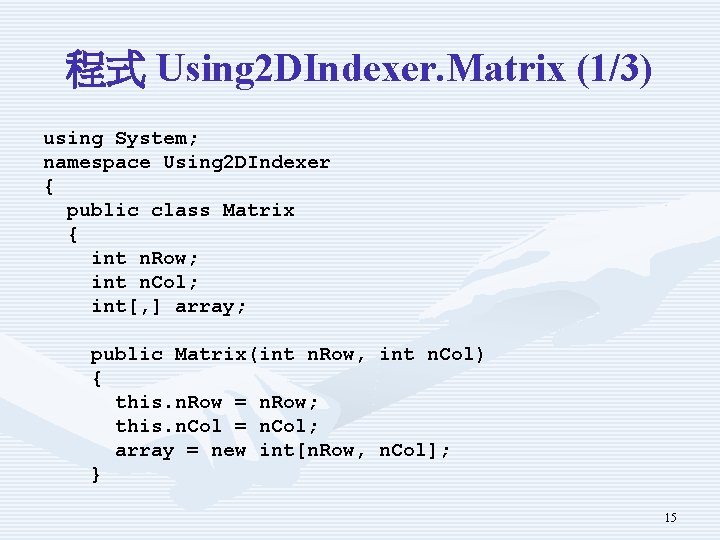
程式 Using 2 DIndexer. Matrix (1/3) using System; namespace Using 2 DIndexer { public class Matrix { int n. Row; int n. Col; int[, ] array; public Matrix(int n. Row, { this. n. Row = n. Row; this. n. Col = n. Col; array = new int[n. Row, } int n. Col) n. Col]; 15
![程式 Using 2 DIndexer Matrix 23 public int thisint i int j get 程式 Using 2 DIndexer. Matrix (2/3) public int this[int i, int j] { get](https://slidetodoc.com/presentation_image/3173af107d9f961bb07622d8c5a445f4/image-16.jpg)
程式 Using 2 DIndexer. Matrix (2/3) public int this[int i, int j] { get { if (i < 1 || i > n. Row || j < 1 || j > n. Col) { Console. Write. Line("Matrix>>矩陣註標錯誤"); return 0; } else { return array[i - 1, j - 1]; } } 16
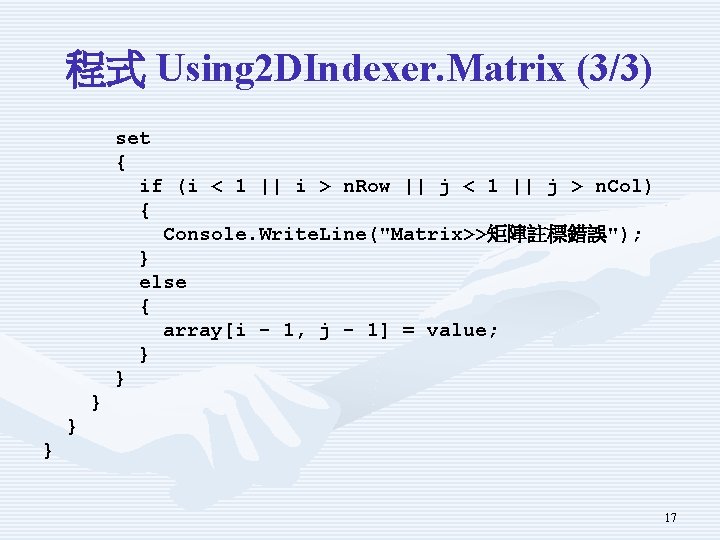
程式 Using 2 DIndexer. Matrix (3/3) set { if (i < 1 || i > n. Row || j < 1 || j > n. Col) { Console. Write. Line("Matrix>>矩陣註標錯誤"); } else { array[i - 1, j - 1] = value; } } } 17
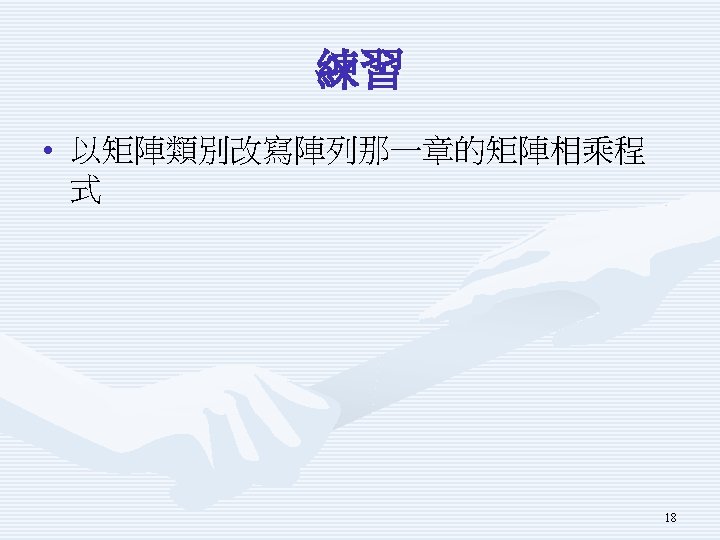
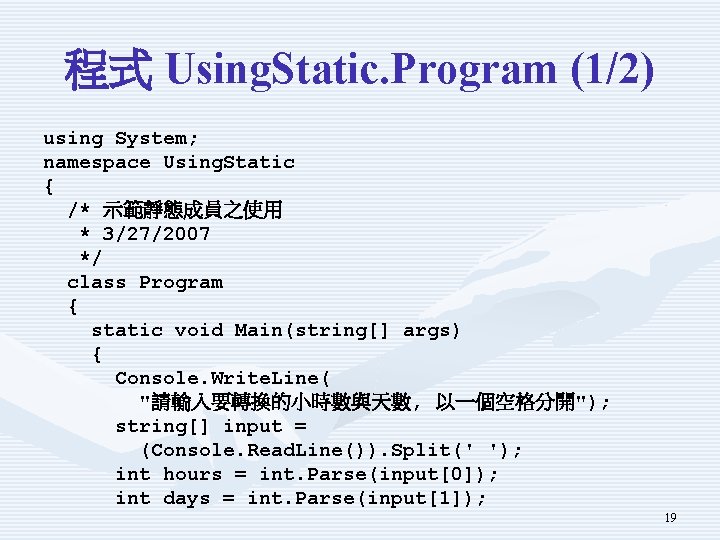
程式 Using. Static. Program (1/2) using System; namespace Using. Static { /* 示範靜態成員之使用 * 3/27/2007 */ class Program { static void Main(string[] args) { Console. Write. Line( "請輸入要轉換的小時數與天數, 以一個空格分開"); string[] input = (Console. Read. Line()). Split(' '); int hours = int. Parse(input[0]); int days = int. Parse(input[1]); 19
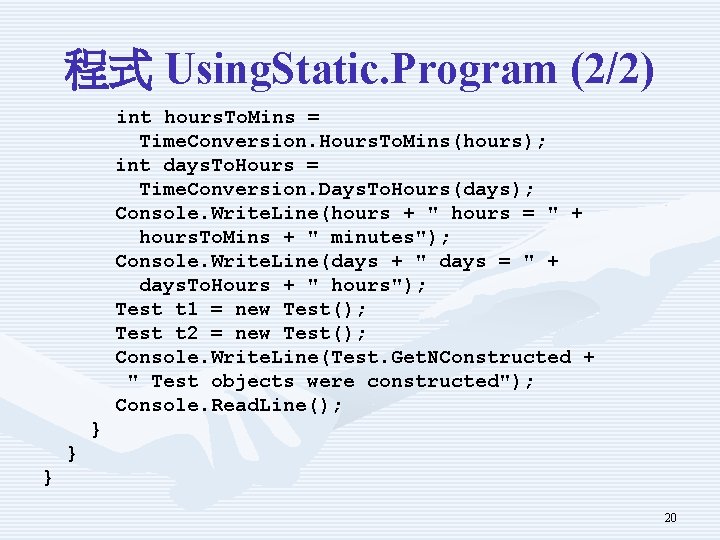
程式 Using. Static. Program (2/2) int hours. To. Mins = Time. Conversion. Hours. To. Mins(hours); int days. To. Hours = Time. Conversion. Days. To. Hours(days); Console. Write. Line(hours + " hours = " + hours. To. Mins + " minutes"); Console. Write. Line(days + " days = " + days. To. Hours + " hours"); Test t 1 = new Test(); Test t 2 = new Test(); Console. Write. Line(Test. Get. NConstructed + " Test objects were constructed"); Console. Read. Line(); } } } 20
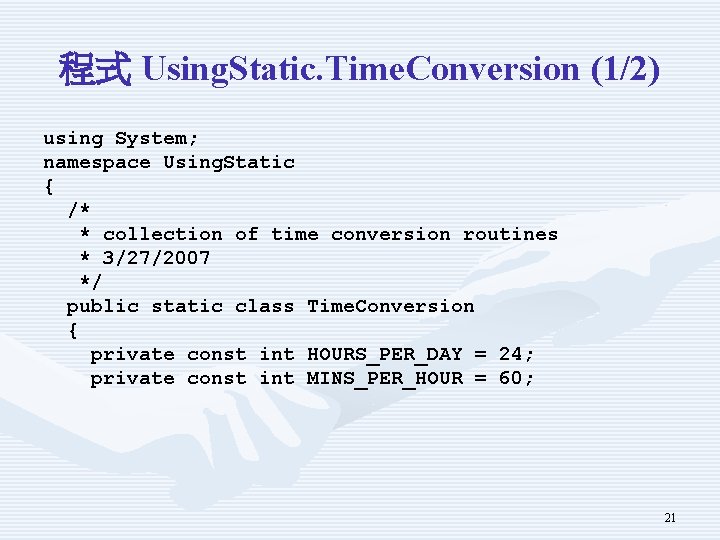
程式 Using. Static. Time. Conversion (1/2) using System; namespace Using. Static { /* * collection of time conversion routines * 3/27/2007 */ public static class Time. Conversion { private const int HOURS_PER_DAY = 24; private const int MINS_PER_HOUR = 60; 21
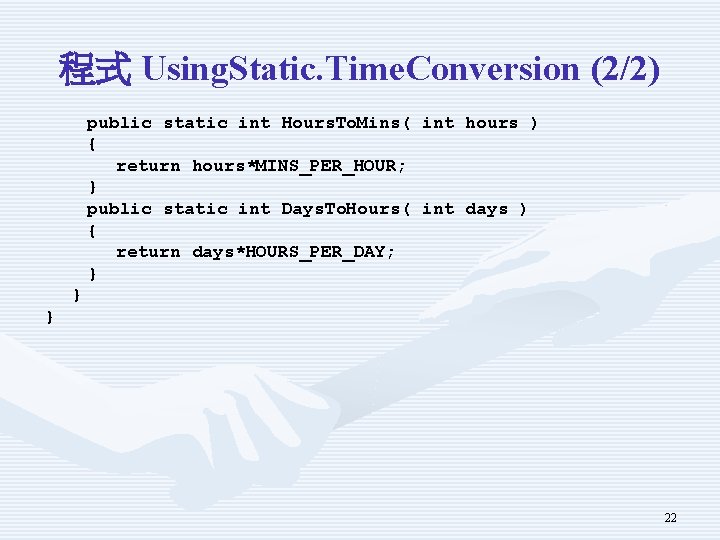
程式 Using. Static. Time. Conversion (2/2) public static int Hours. To. Mins( int hours ) { return hours*MINS_PER_HOUR; } public static int Days. To. Hours( int days ) { return days*HOURS_PER_DAY; } } } 22
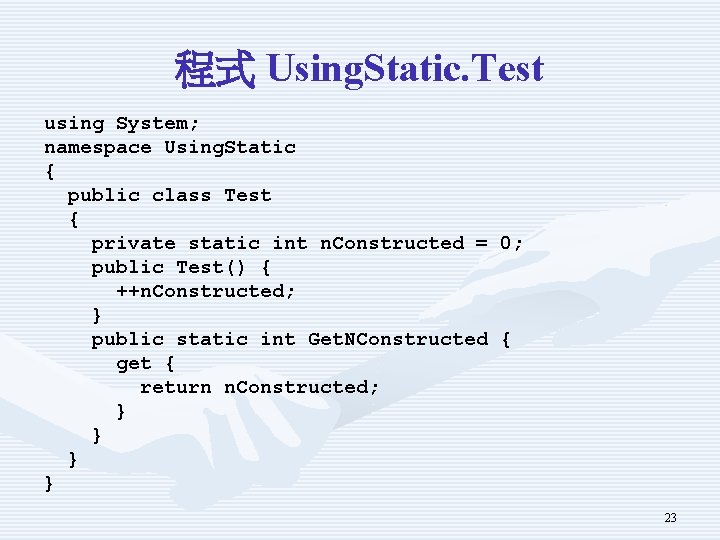
程式 Using. Static. Test using System; namespace Using. Static { public class Test { private static int n. Constructed = 0; public Test() { ++n. Constructed; } public static int Get. NConstructed { get { return n. Constructed; } } 23
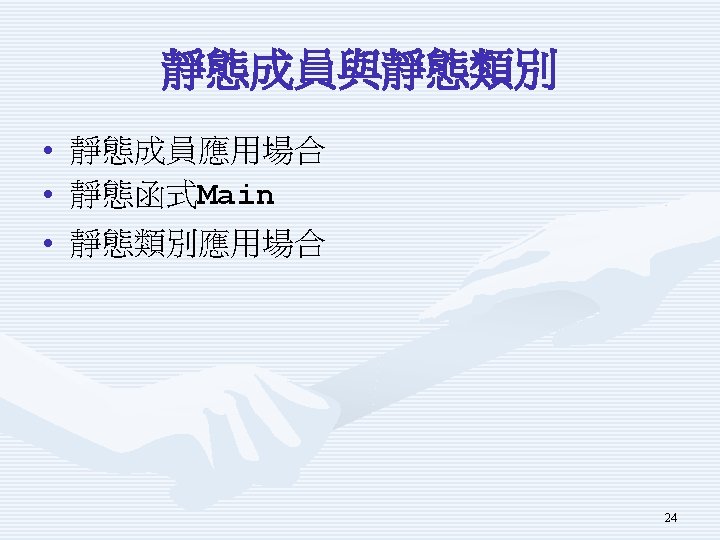
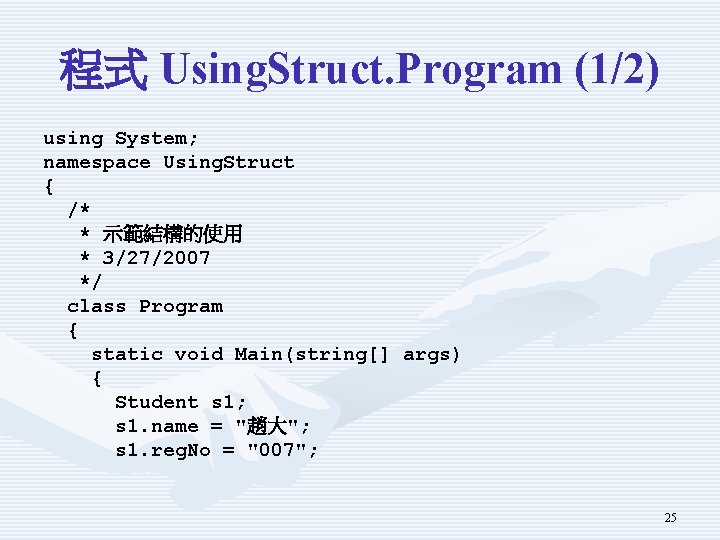
程式 Using. Struct. Program (1/2) using System; namespace Using. Struct { /* * 示範結構的使用 * 3/27/2007 */ class Program { static void Main(string[] args) { Student s 1; s 1. name = "趙大"; s 1. reg. No = "007"; 25
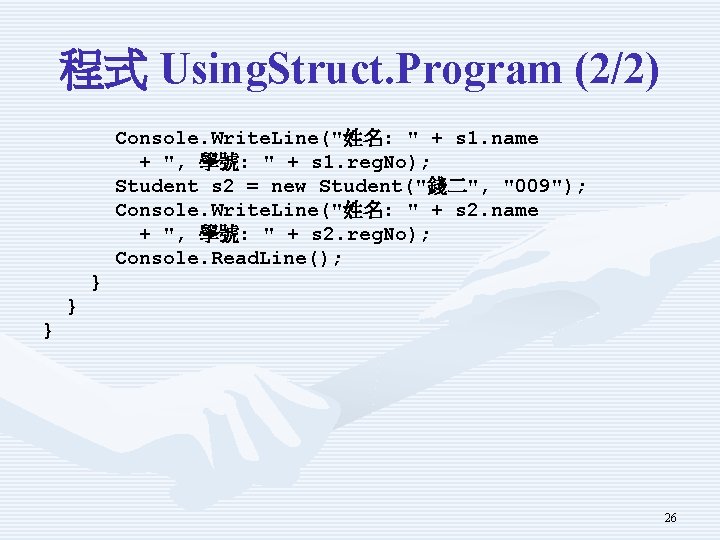
程式 Using. Struct. Program (2/2) Console. Write. Line("姓名: " + s 1. name + ", 學號: " + s 1. reg. No); Student s 2 = new Student("錢二", "009"); Console. Write. Line("姓名: " + s 2. name + ", 學號: " + s 2. reg. No); Console. Read. Line(); } } } 26
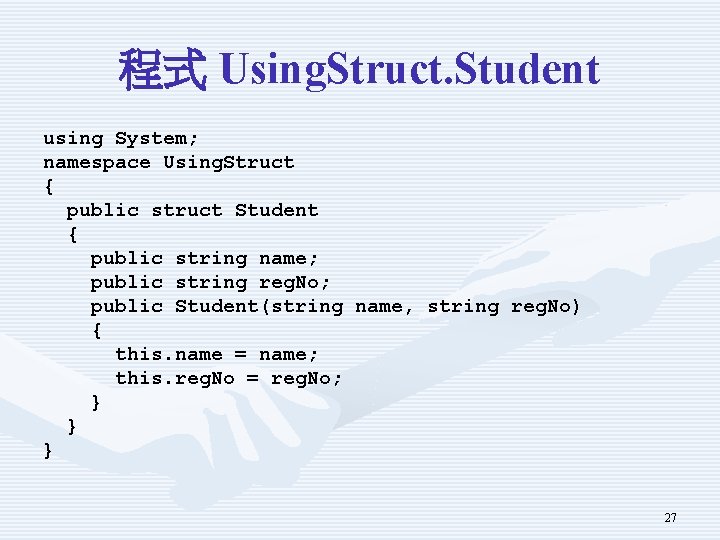
程式 Using. Struct. Student using System; namespace Using. Struct { public struct Student { public string name; public string reg. No; public Student(string name, string reg. No) { this. name = name; this. reg. No = reg. No; } } } 27
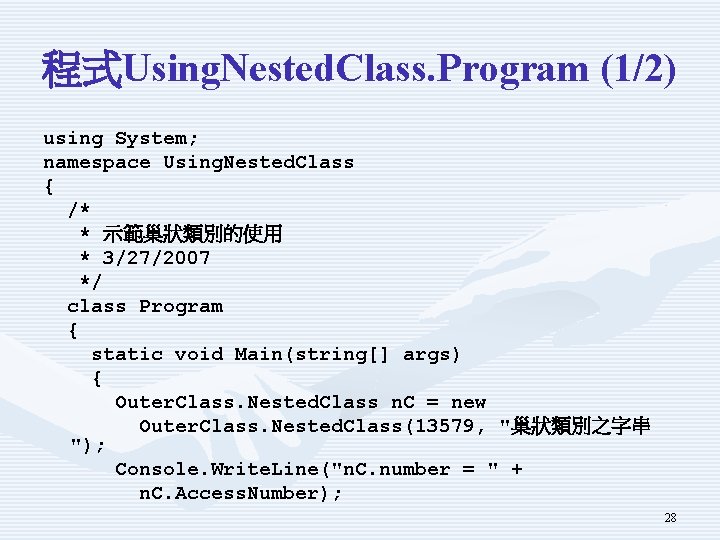
程式Using. Nested. Class. Program (1/2) using System; namespace Using. Nested. Class { /* * 示範巢狀類別的使用 * 3/27/2007 */ class Program { static void Main(string[] args) { Outer. Class. Nested. Class n. C = new Outer. Class. Nested. Class(13579, "巢狀類別之字串 "); Console. Write. Line("n. C. number = " + n. C. Access. Number); 28
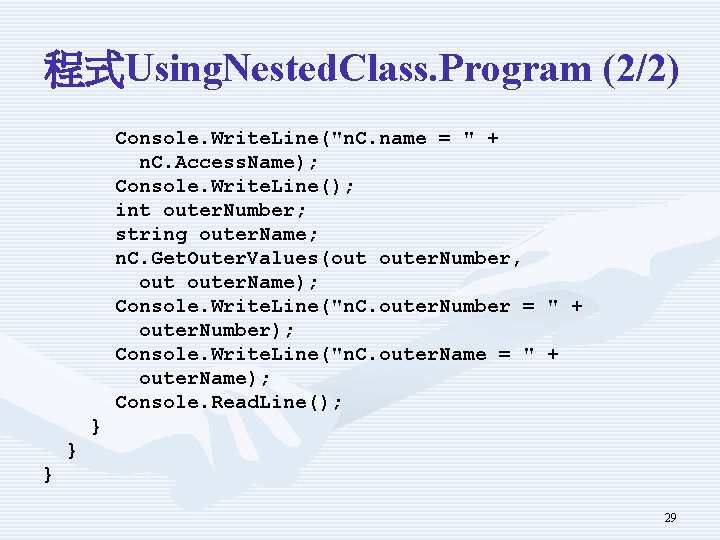
程式Using. Nested. Class. Program (2/2) Console. Write. Line("n. C. name = " + n. C. Access. Name); Console. Write. Line(); int outer. Number; string outer. Name; n. C. Get. Outer. Values(out outer. Number, outer. Name); Console. Write. Line("n. C. outer. Number = outer. Number); Console. Write. Line("n. C. outer. Name = " outer. Name); Console. Read. Line(); " + + } } } 29
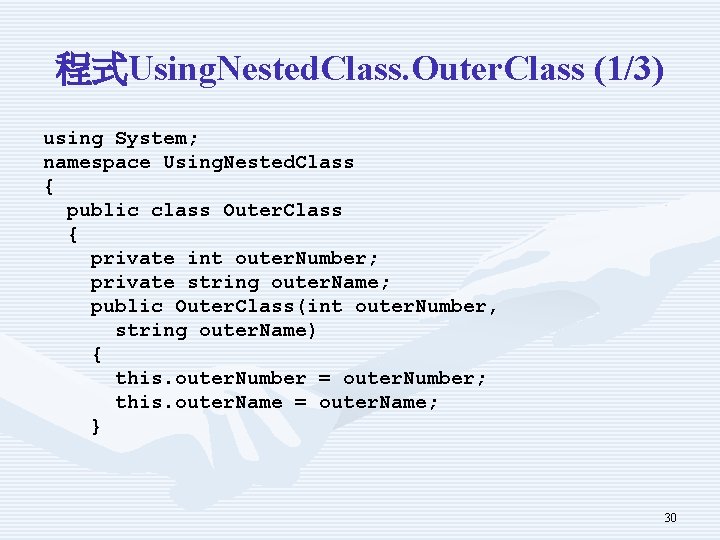
程式Using. Nested. Class. Outer. Class (1/3) using System; namespace Using. Nested. Class { public class Outer. Class { private int outer. Number; private string outer. Name; public Outer. Class(int outer. Number, string outer. Name) { this. outer. Number = outer. Number; this. outer. Name = outer. Name; } 30
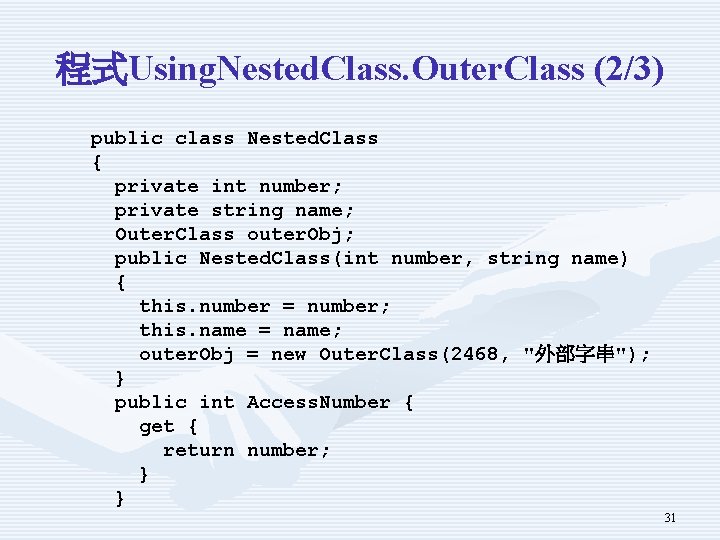
程式Using. Nested. Class. Outer. Class (2/3) public class Nested. Class { private int number; private string name; Outer. Class outer. Obj; public Nested. Class(int number, string name) { this. number = number; this. name = name; outer. Obj = new Outer. Class(2468, "外部字串"); } public int Access. Number { get { return number; } } 31
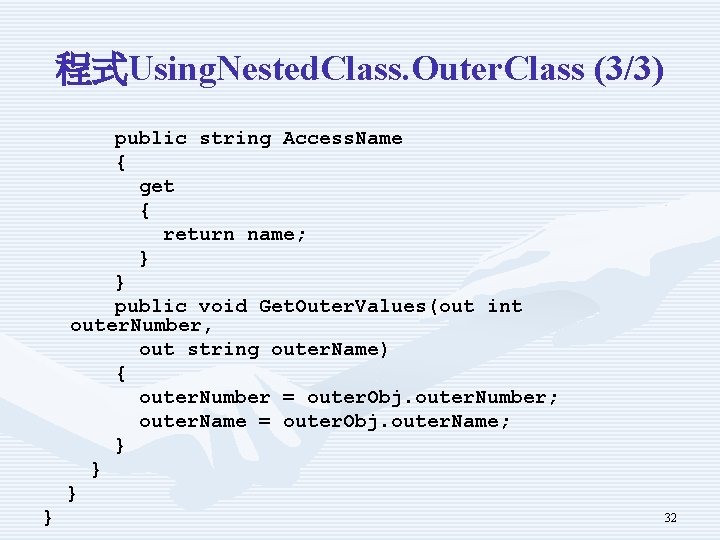
程式Using. Nested. Class. Outer. Class (3/3) public string Access. Name { get { return name; } } public void Get. Outer. Values(out int outer. Number, out string outer. Name) { outer. Number = outer. Obj. outer. Number; outer. Name = outer. Obj. outer. Name; } } 32