Class Constructors l a class constructor is a
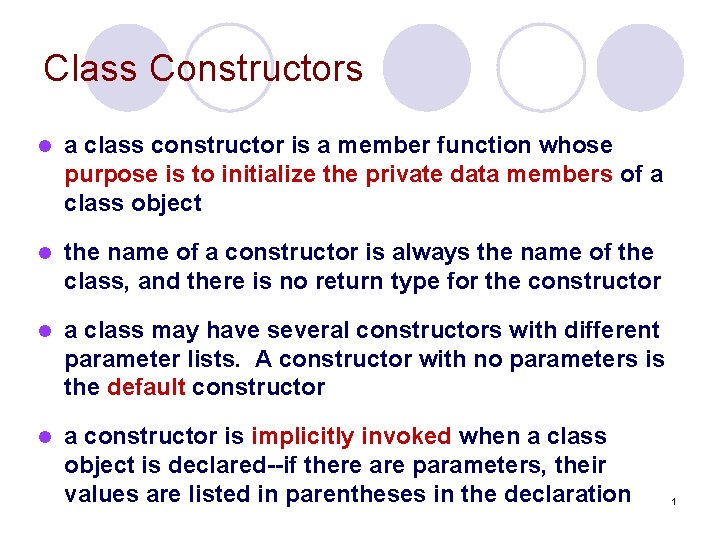
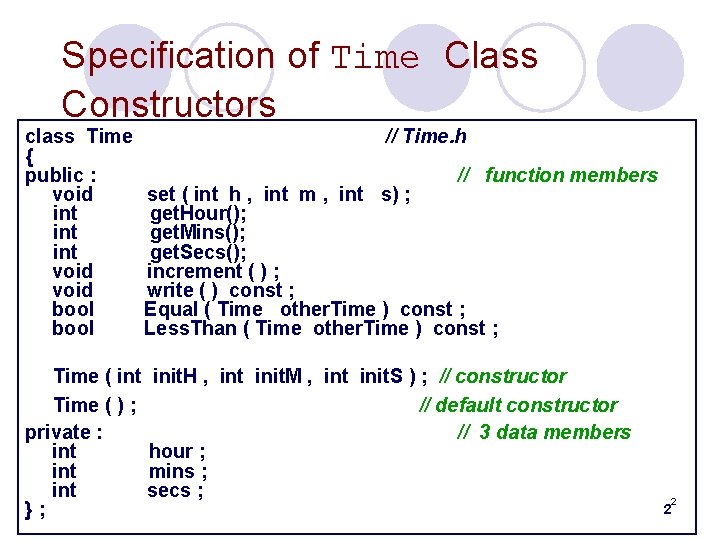
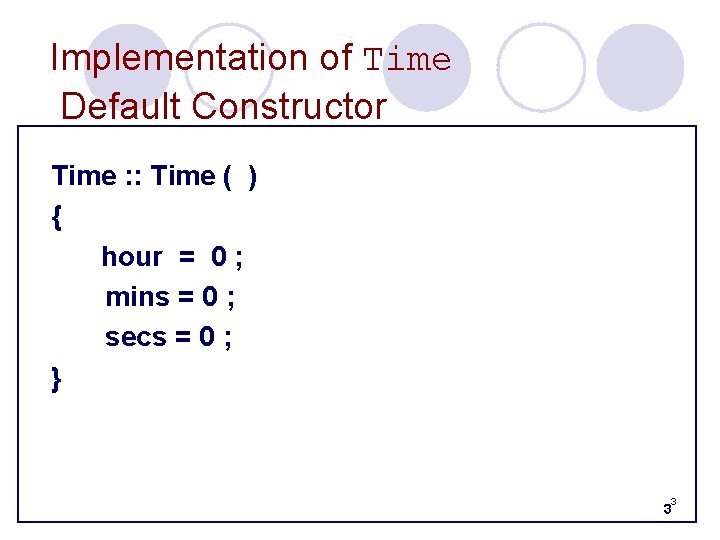
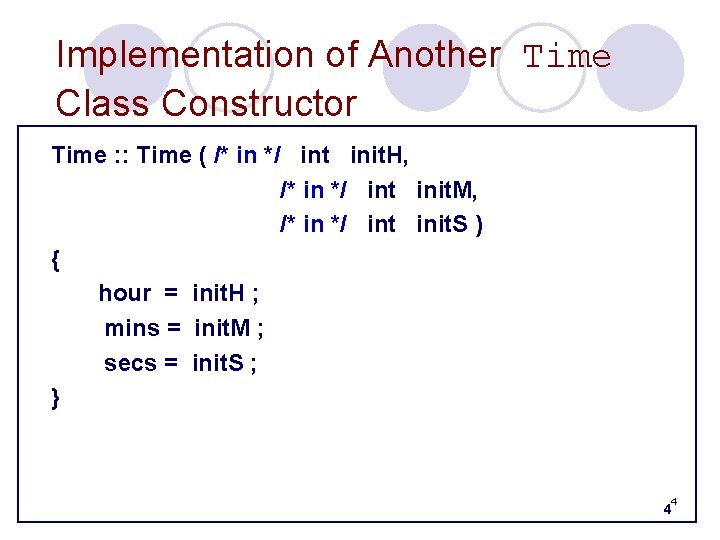
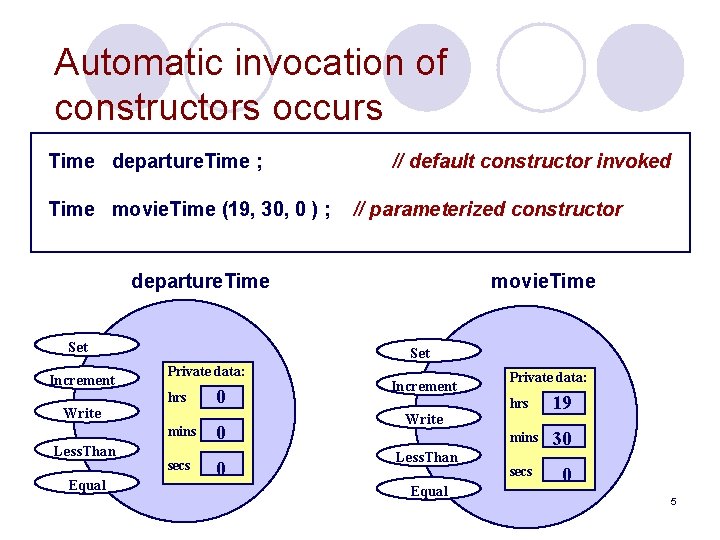
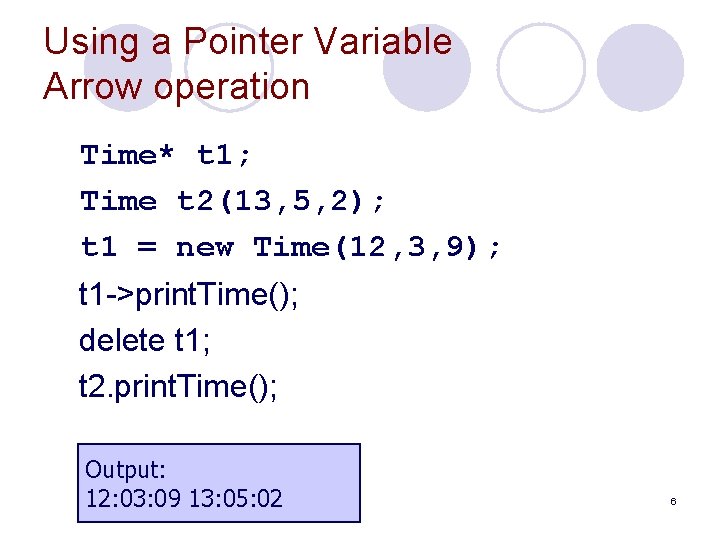
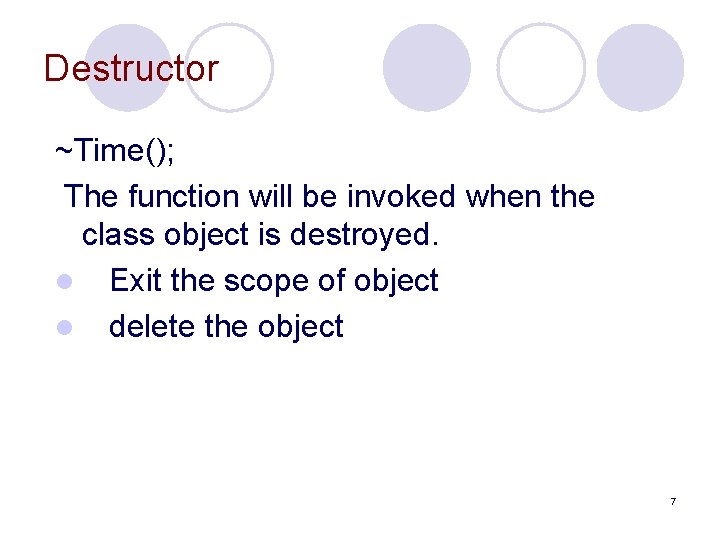
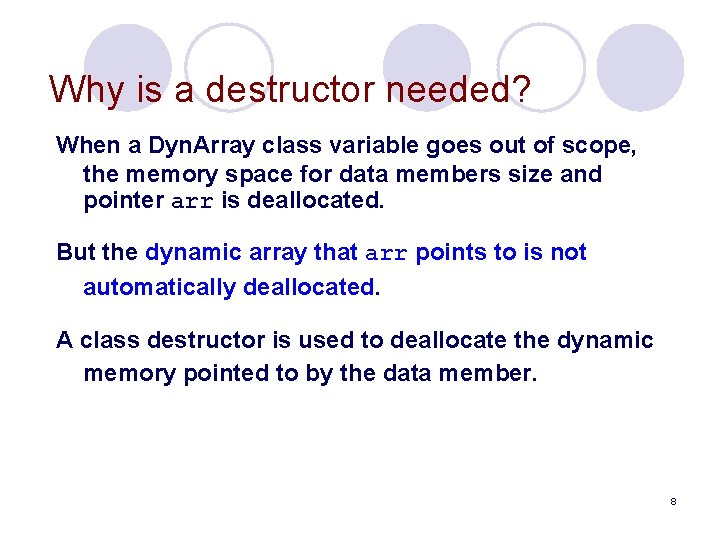
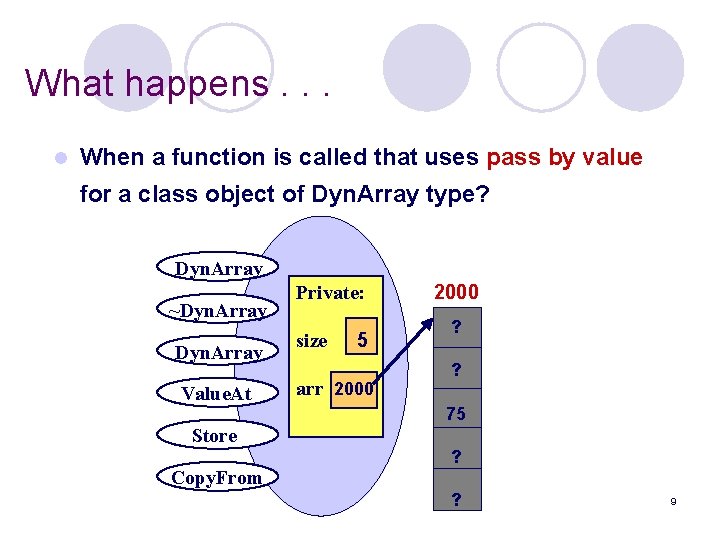
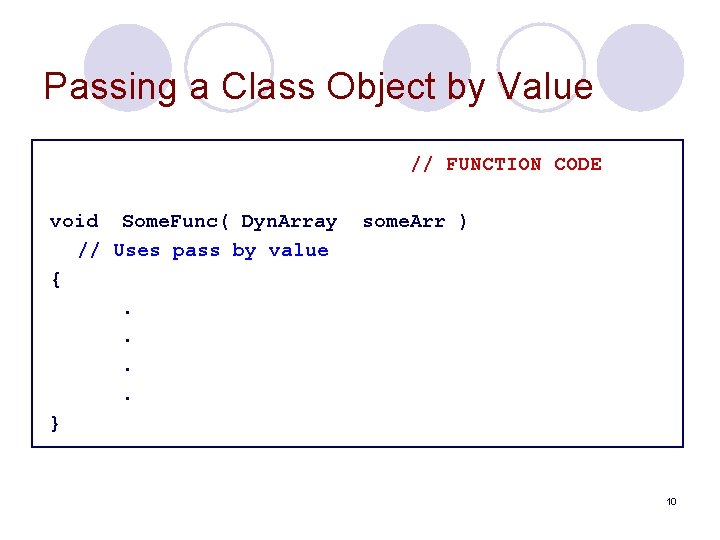
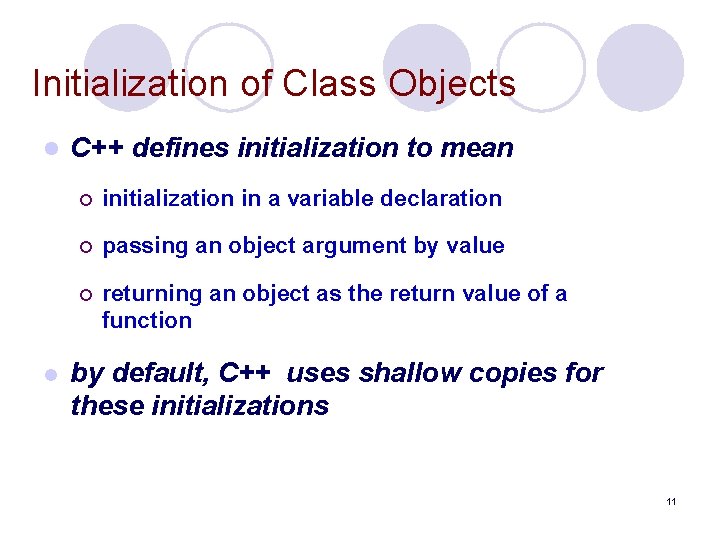
- Slides: 11
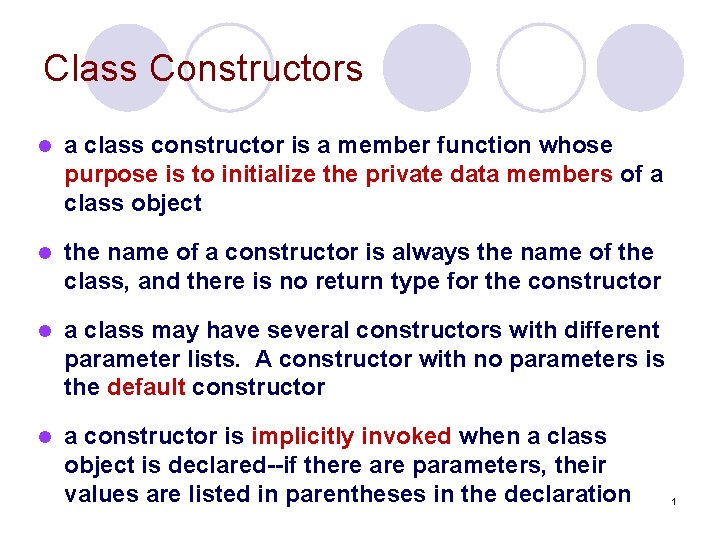
Class Constructors l a class constructor is a member function whose purpose is to initialize the private data members of a class object l the name of a constructor is always the name of the class, and there is no return type for the constructor l a class may have several constructors with different parameter lists. A constructor with no parameters is the default constructor l a constructor is implicitly invoked when a class object is declared--if there are parameters, their values are listed in parentheses in the declaration 1
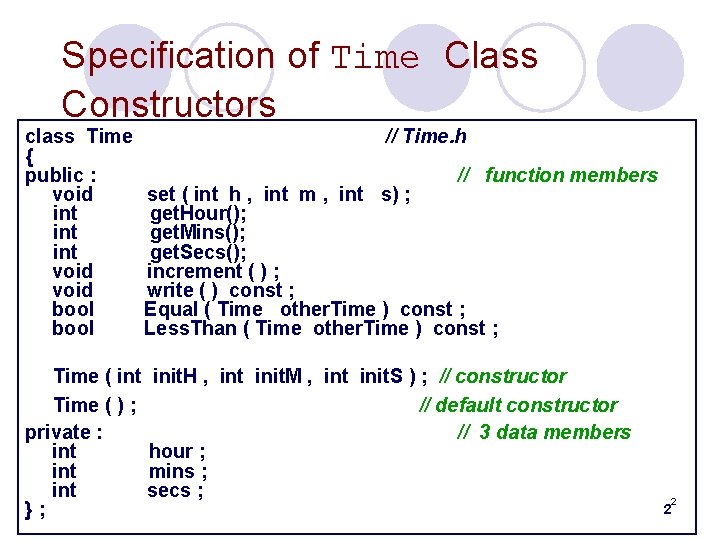
Specification of Time Class Constructors class Time { public : void int int void bool // Time. h // function members set ( int h , int m , int s) ; get. Hour(); get. Mins(); get. Secs(); increment ( ) ; write ( ) const ; Equal ( Time other. Time ) const ; Less. Than ( Time other. Time ) const ; Time ( int init. H , int init. M , int init. S ) ; // constructor Time ( ) ; // default constructor private : // 3 data members int hour ; int mins ; int secs ; }; 2 2
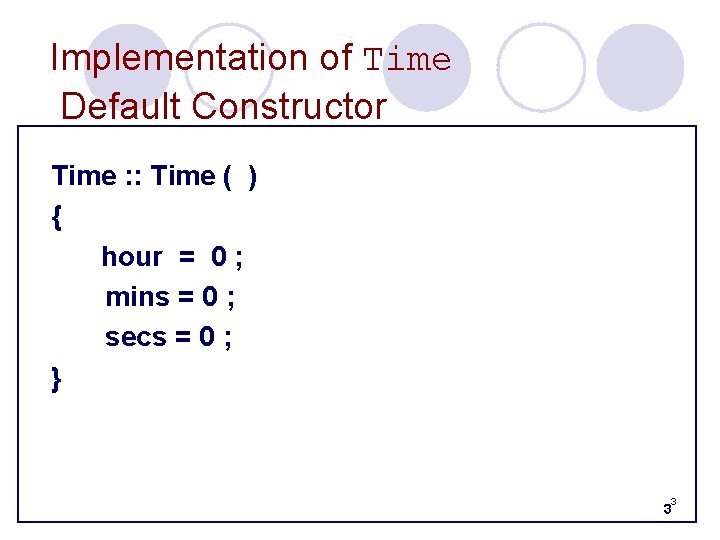
Implementation of Time Default Constructor Time : : Time ( ) { hour = 0 ; mins = 0 ; secs = 0 ; } 3 3
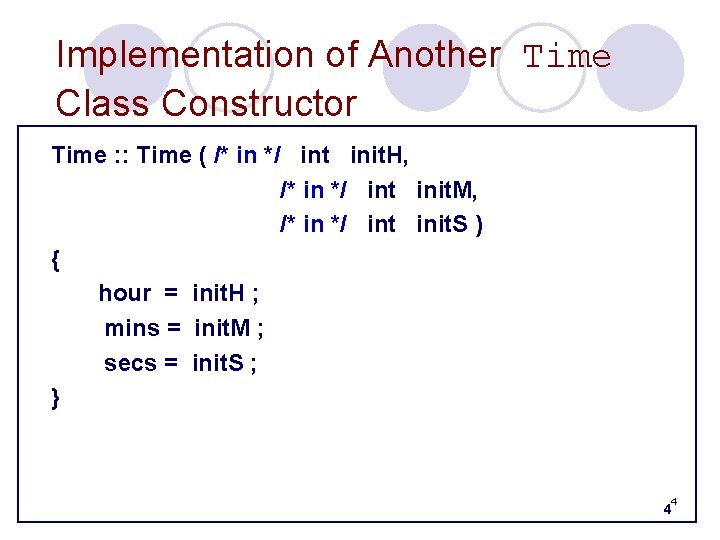
Implementation of Another Time Class Constructor Time : : Time ( /* in */ int init. H, /* in */ int init. M, /* in */ int init. S ) { hour = init. H ; mins = init. M ; secs = init. S ; } 4 4
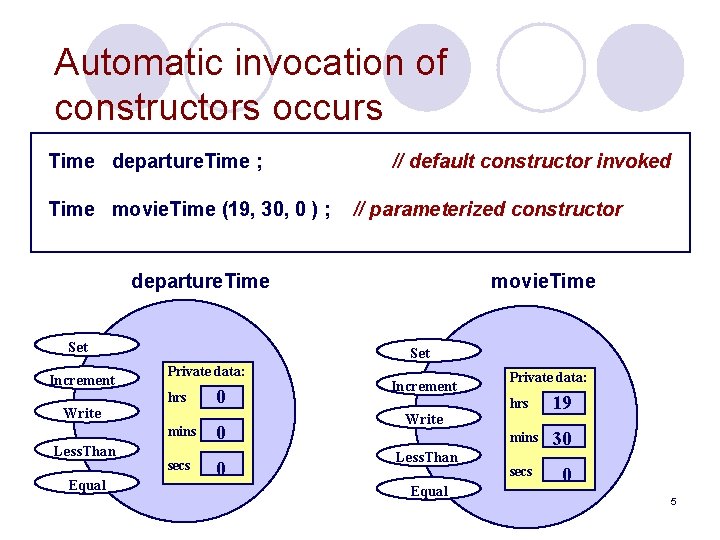
Automatic invocation of constructors occurs Time departure. Time ; Time movie. Time (19, 30, 0 ) ; // default constructor invoked // parameterized constructor departure. Time Set Increment Write Less. Than Equal movie. Time Set Private data: hrs 0 mins 0 secs 0 Increment Write Less. Than Equal Private data: hrs 19 mins 30 secs 0 5
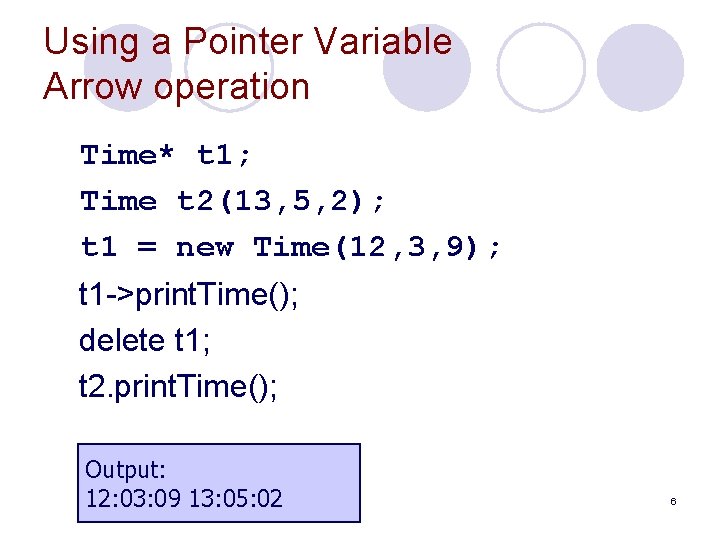
Using a Pointer Variable Arrow operation Time* t 1; Time t 2(13, 5, 2); t 1 = new Time(12, 3, 9); t 1 ->print. Time(); delete t 1; t 2. print. Time(); Output: 12: 03: 09 13: 05: 02 6
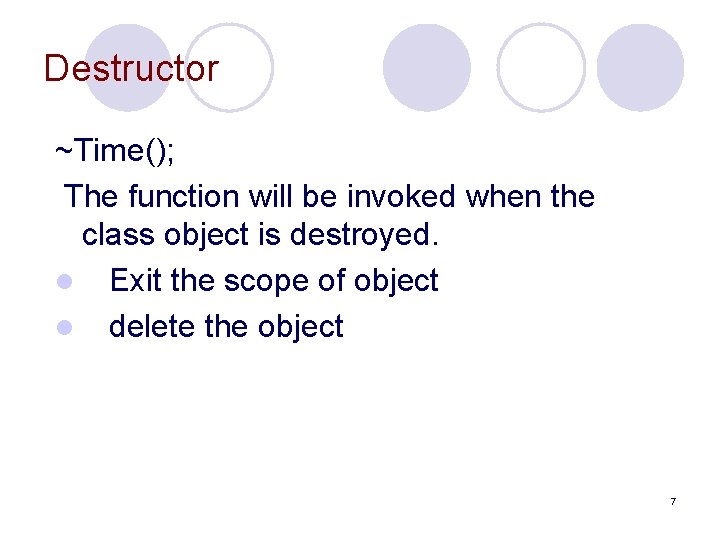
Destructor ~Time(); The function will be invoked when the class object is destroyed. l Exit the scope of object l delete the object 7
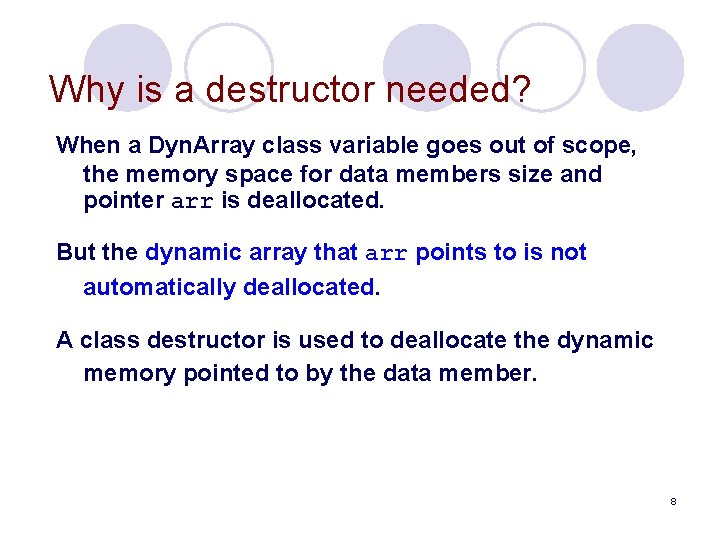
Why is a destructor needed? When a Dyn. Array class variable goes out of scope, the memory space for data members size and pointer arr is deallocated. But the dynamic array that arr points to is not automatically deallocated. A class destructor is used to deallocate the dynamic memory pointed to by the data member. 8
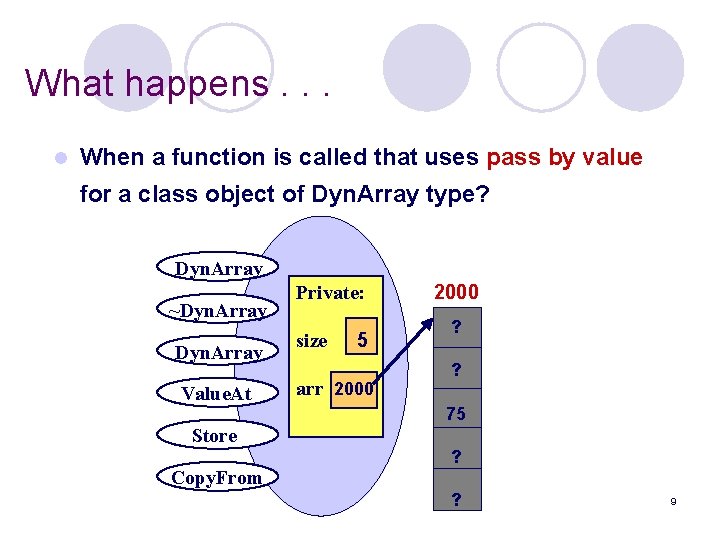
What happens. . . l When a function is called that uses pass by value for a class object of Dyn. Array type? Dyn. Array ~Dyn. Array Value. At Private: size 5 arr 2000 ? ? 75 Store Copy. From ? ? 9
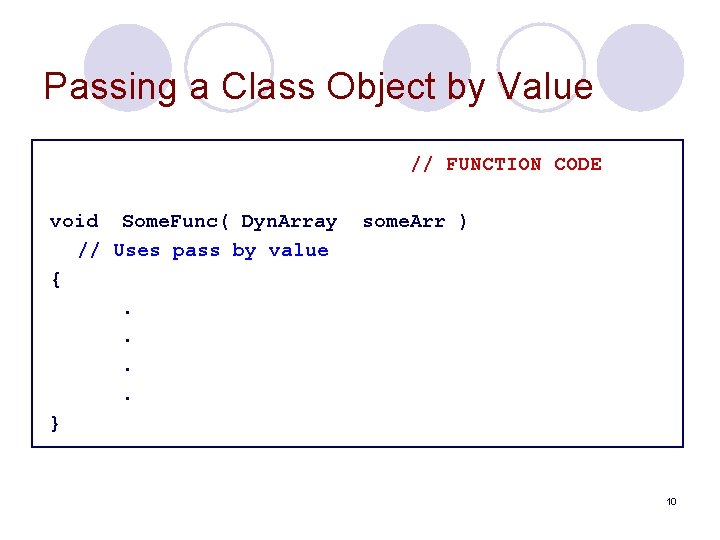
Passing a Class Object by Value // FUNCTION CODE void Some. Func( Dyn. Array // Uses pass by value {. . } some. Arr ) 10
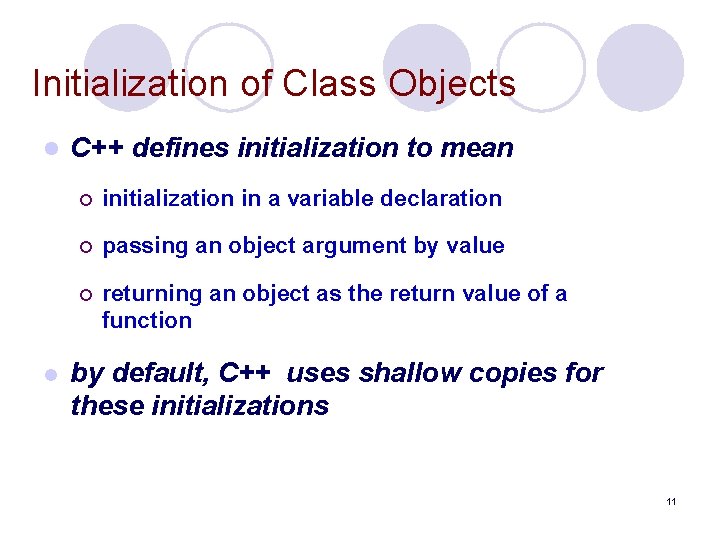
Initialization of Class Objects l l C++ defines initialization to mean ¡ initialization in a variable declaration ¡ passing an object argument by value ¡ returning an object as the return value of a function by default, C++ uses shallow copies for these initializations 11
Method signature consists of
Considerate constructors scoring matrix
Constructor
Considerate constructors best practice hub
Constructors and destructors in c
Difference between default and parameterized constructor
Slope field constructor
Dios constructor
Characteristics of constructor
Constructor in java
Recodex gchd
Desain prototype adalah