Computing Fundamentals Instructor Engr Romana Farhan Assistant Professor
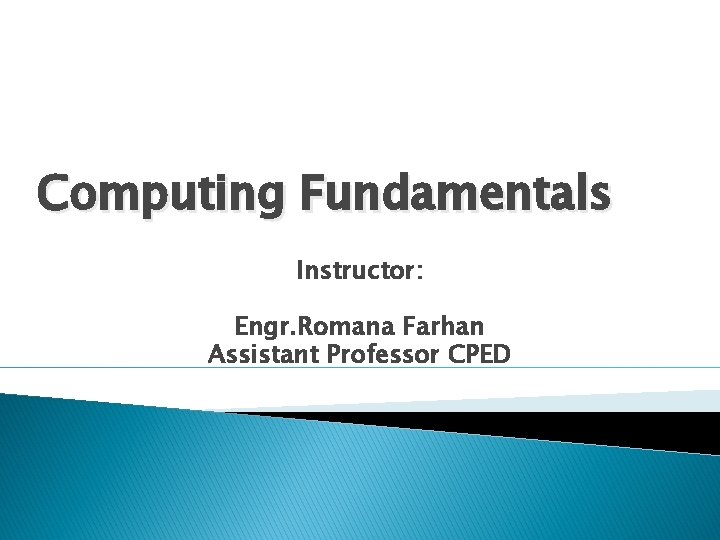
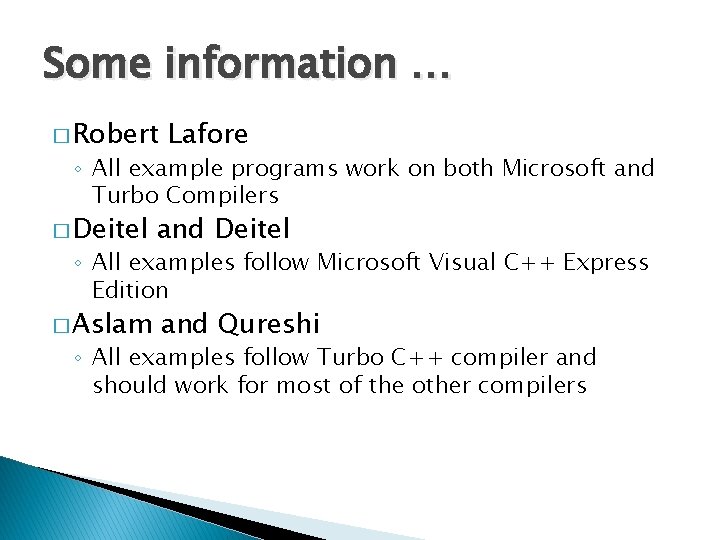
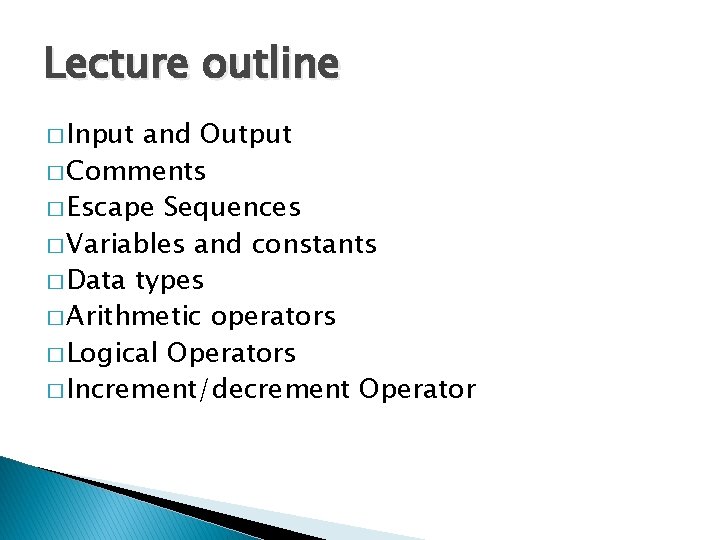
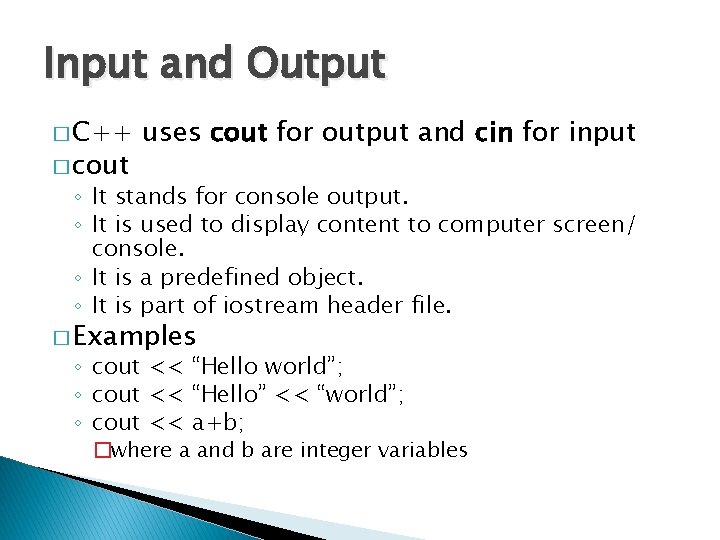
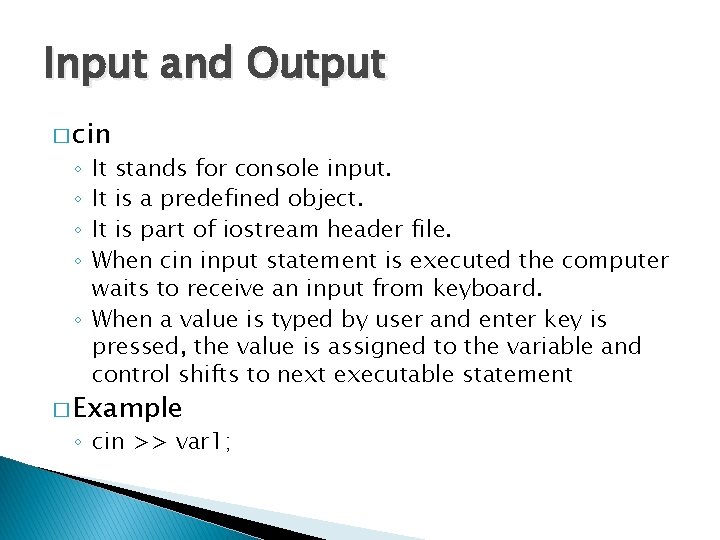
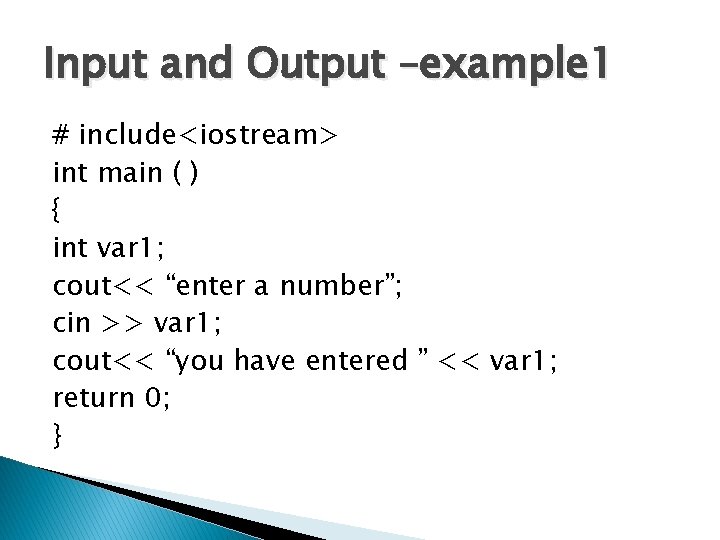
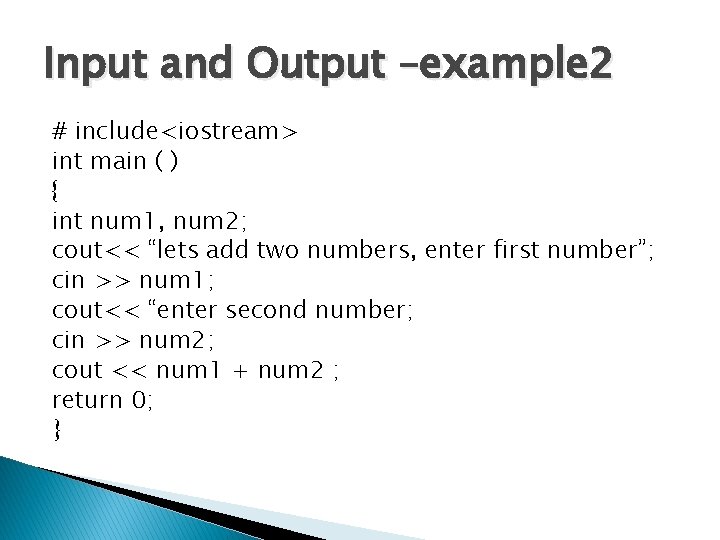
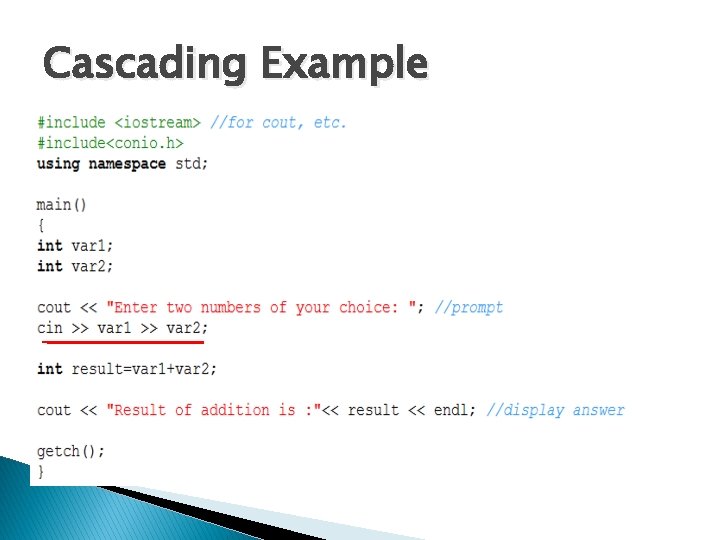
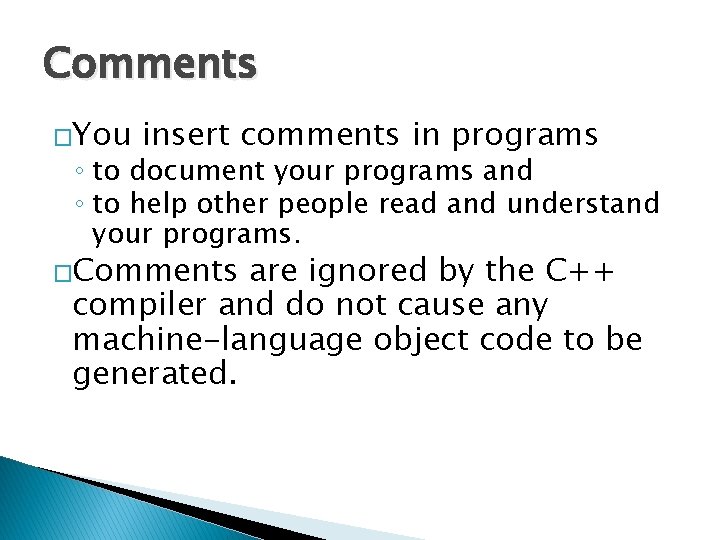
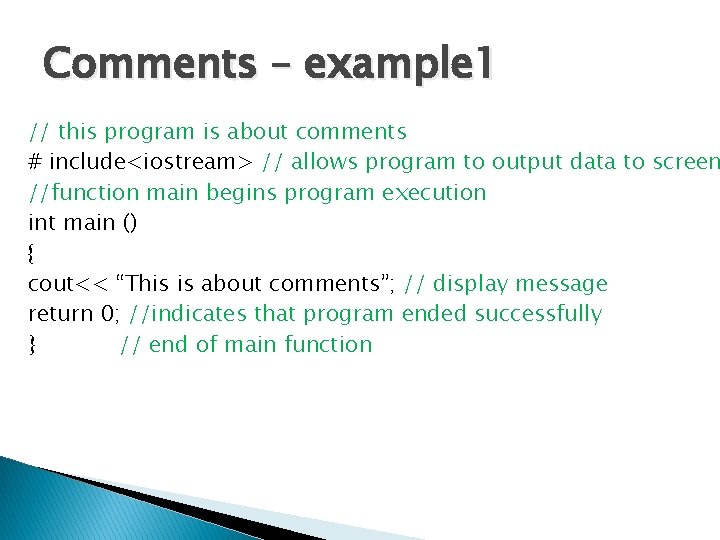
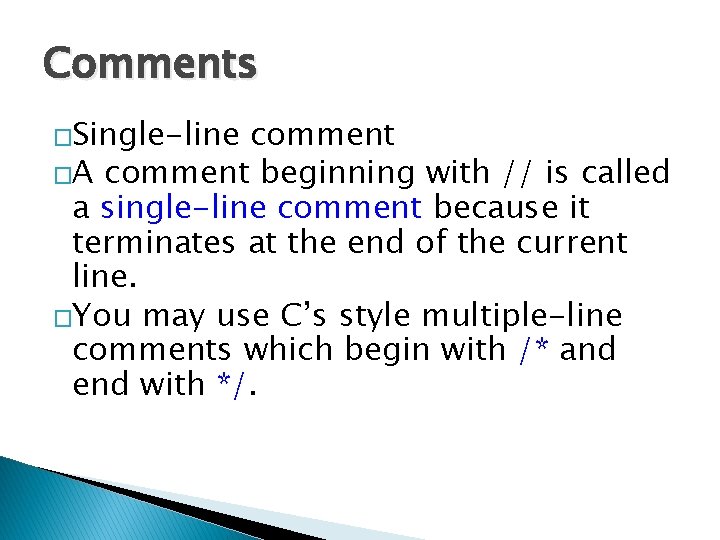
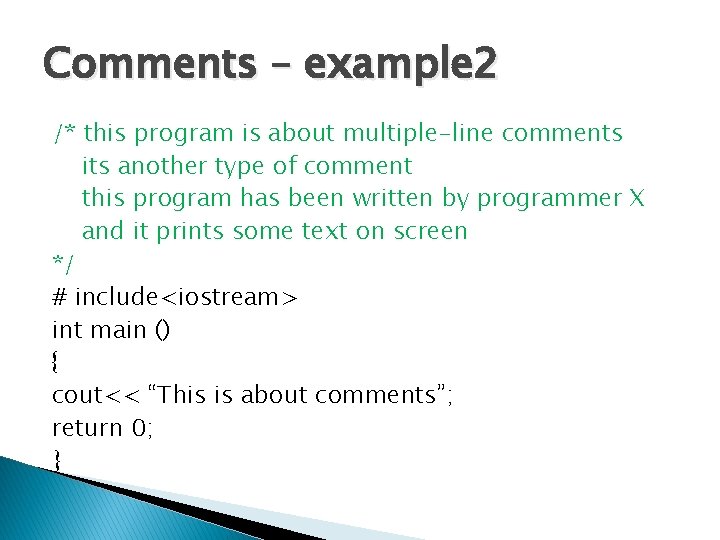
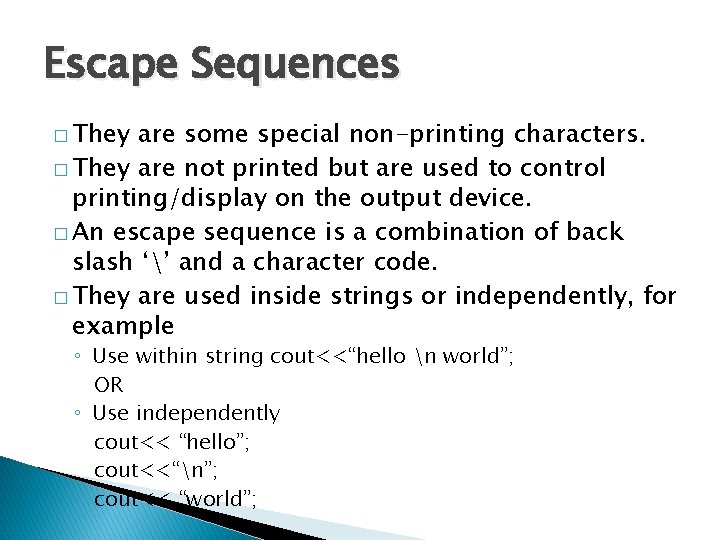
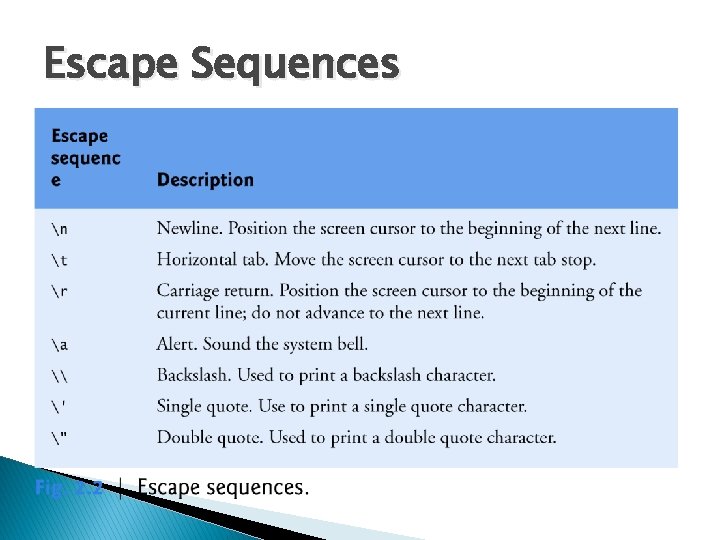
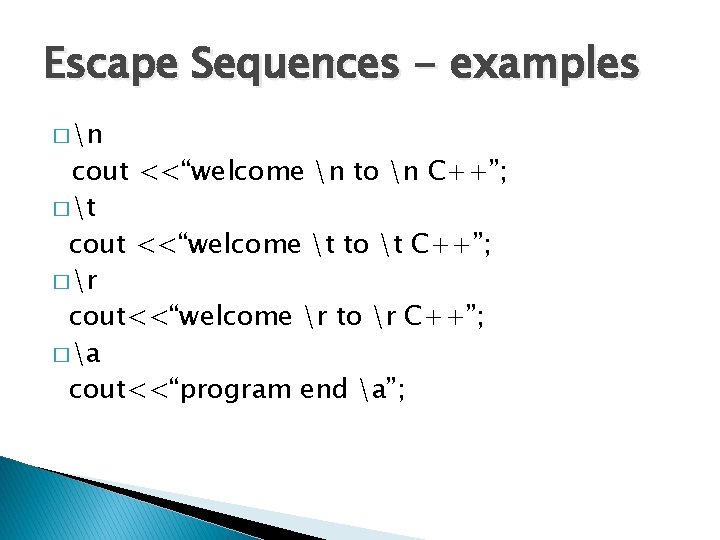
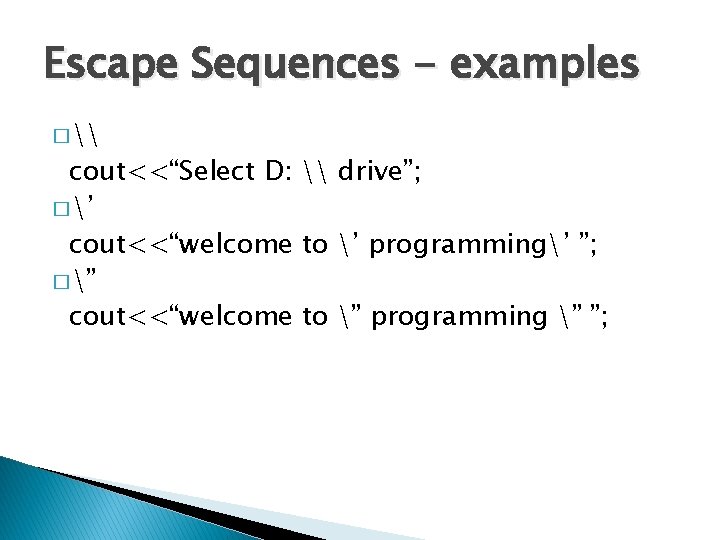
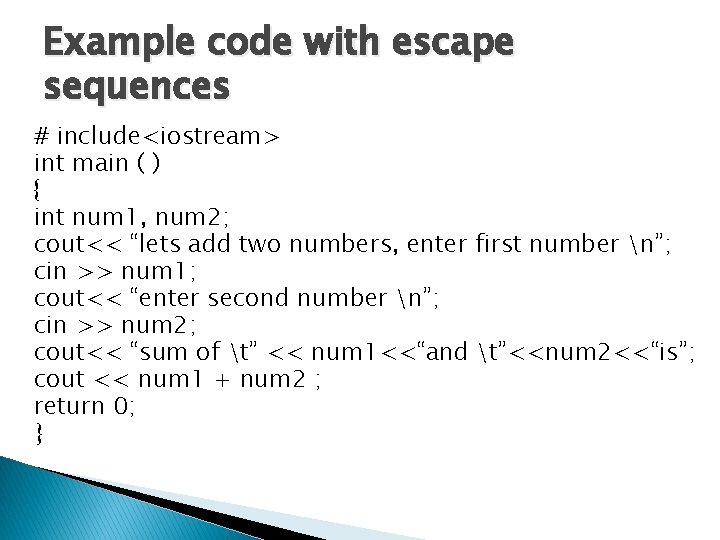
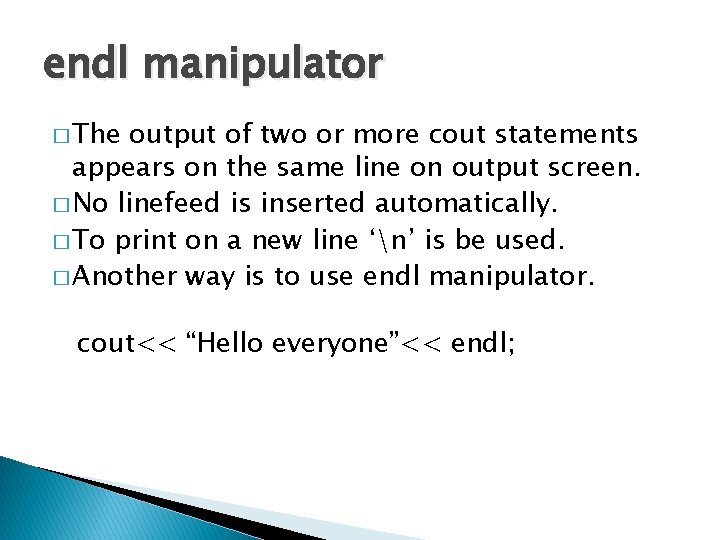
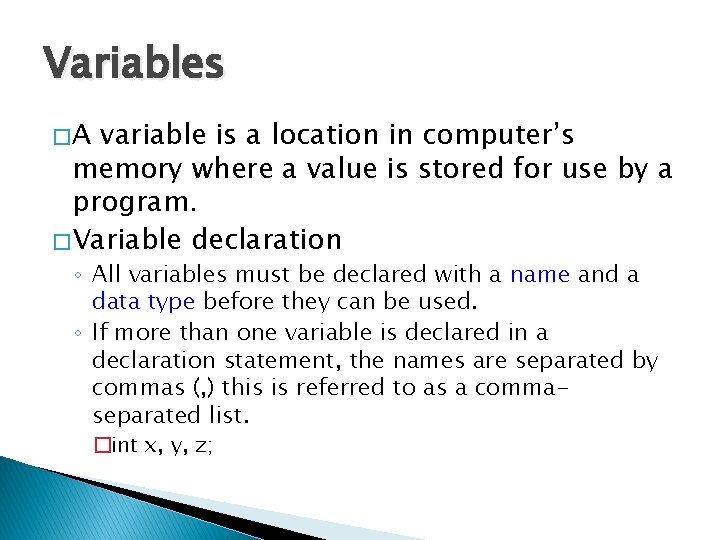
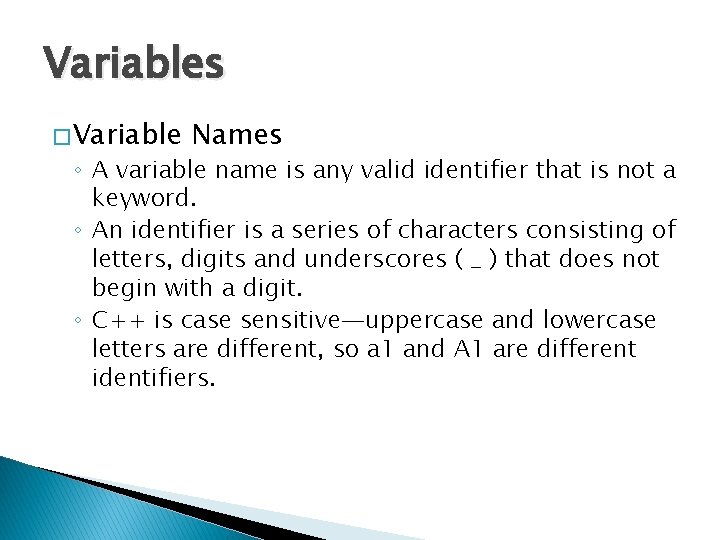
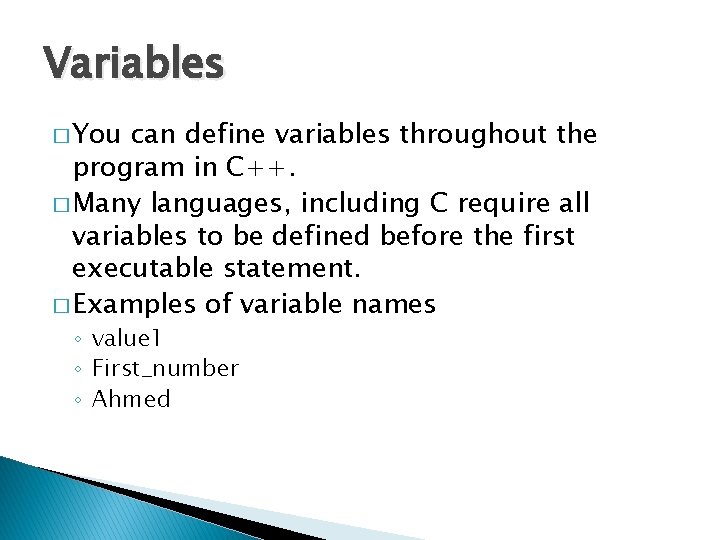
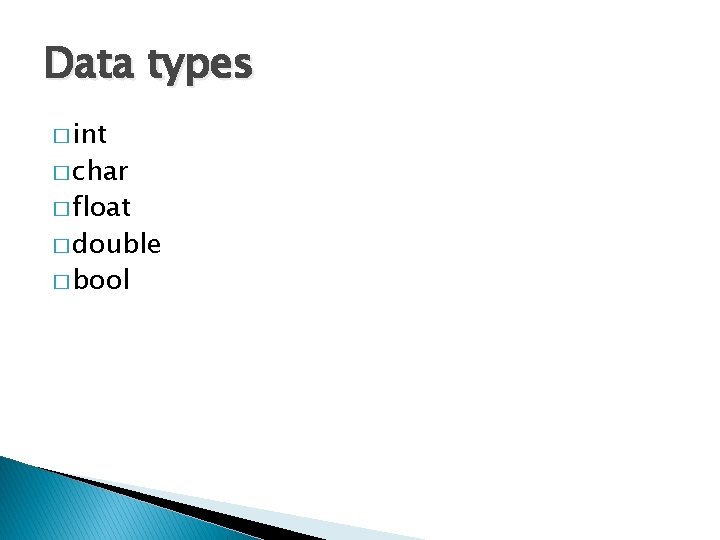
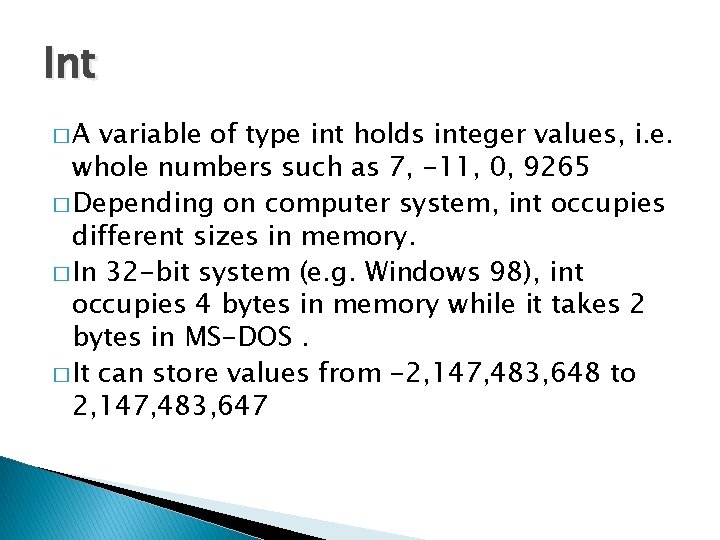
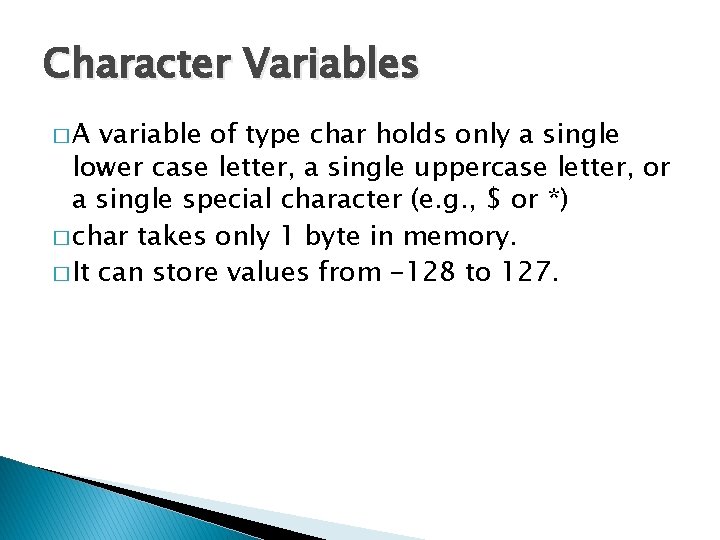
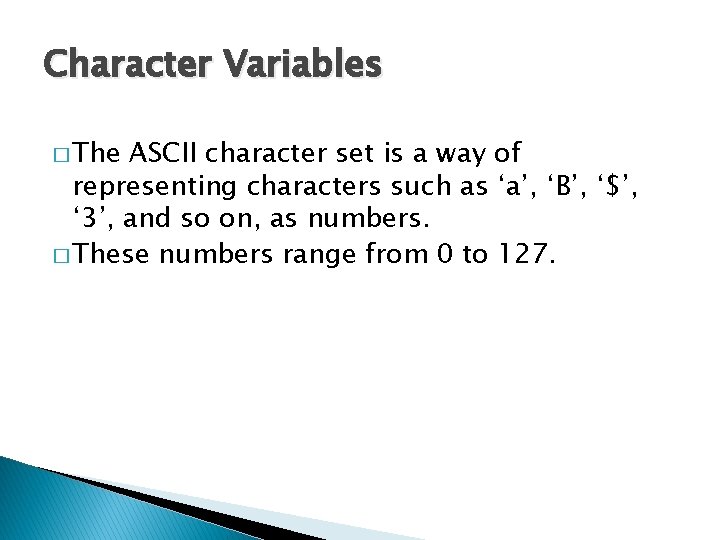
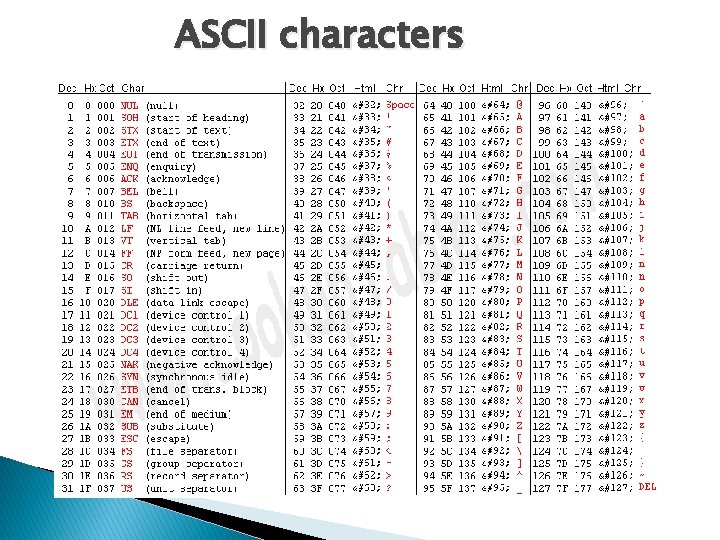
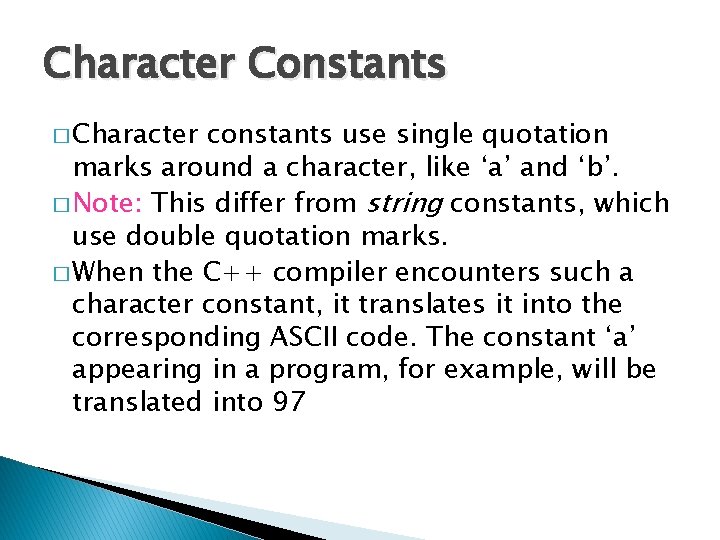
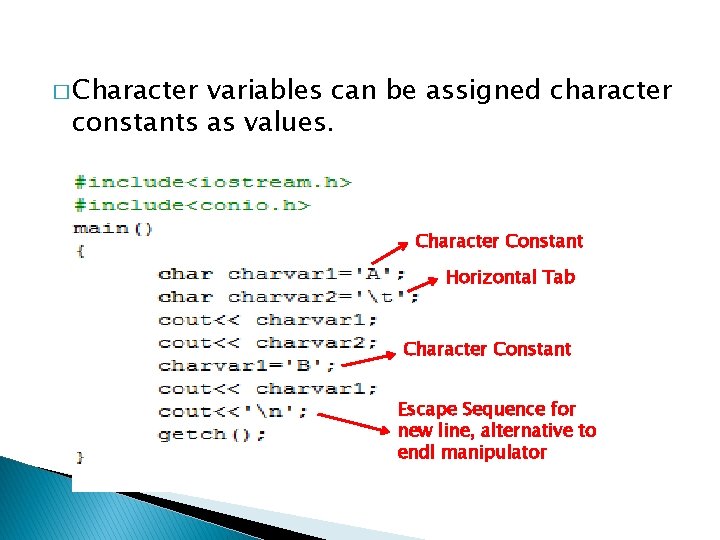
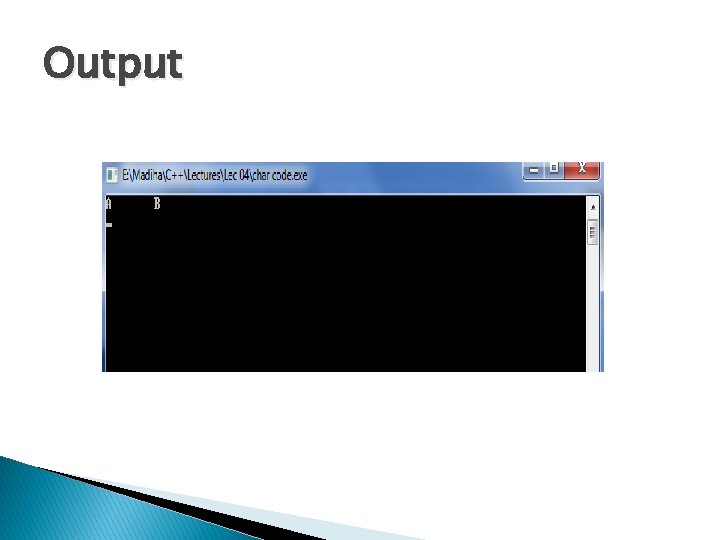
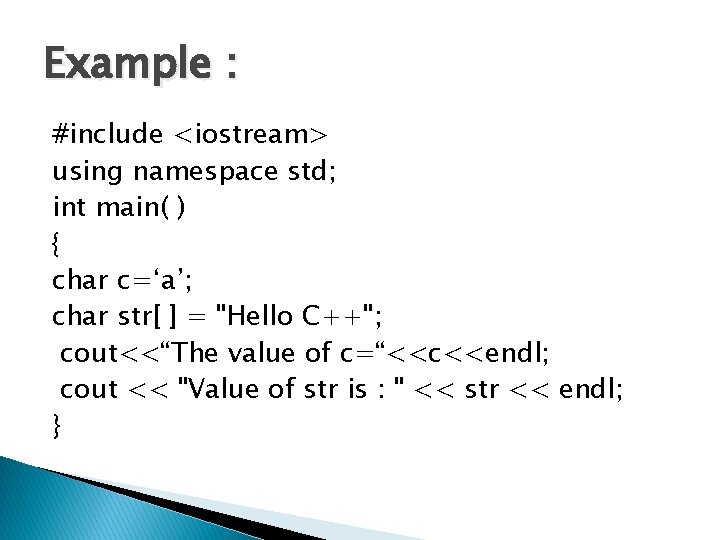
![Example : #include <iostream> using namespace std; int main( ) { char name[50]; cout Example : #include <iostream> using namespace std; int main( ) { char name[50]; cout](https://slidetodoc.com/presentation_image_h2/739fa01453916bc34e20001a02fa0133/image-31.jpg)
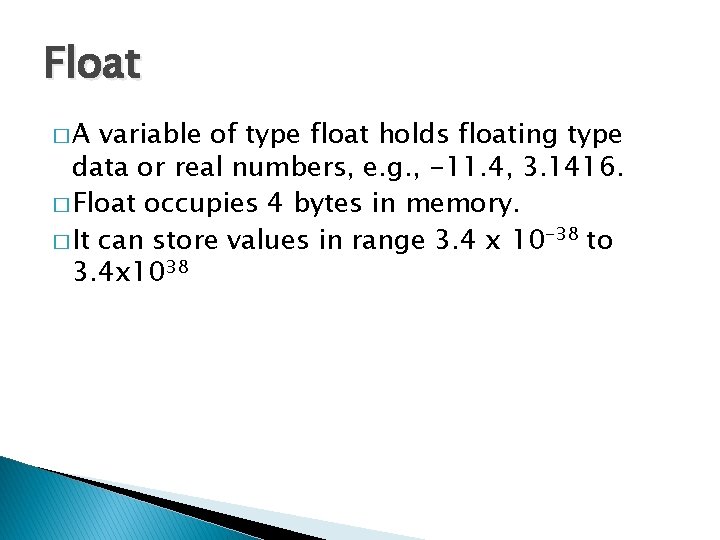
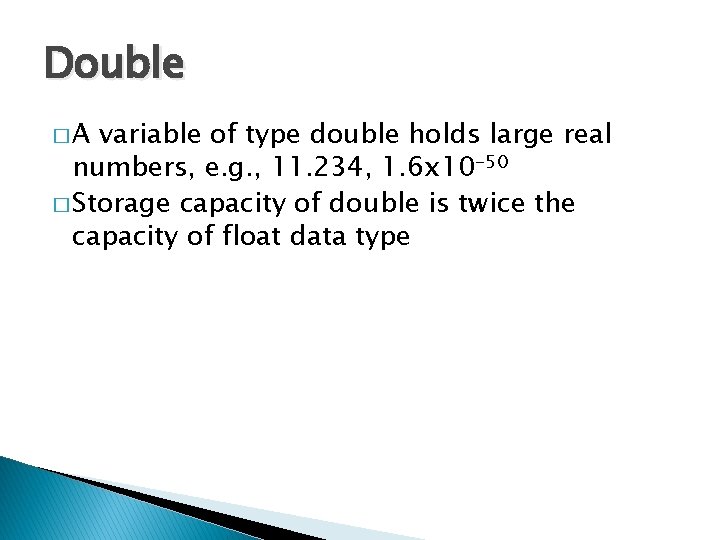
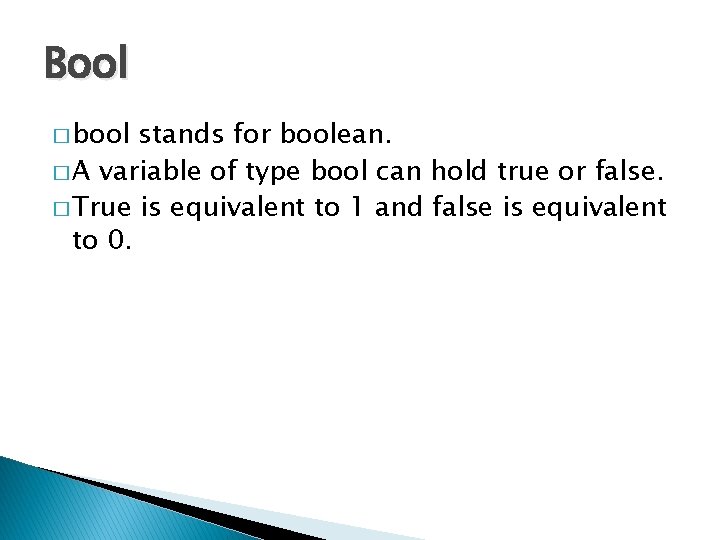
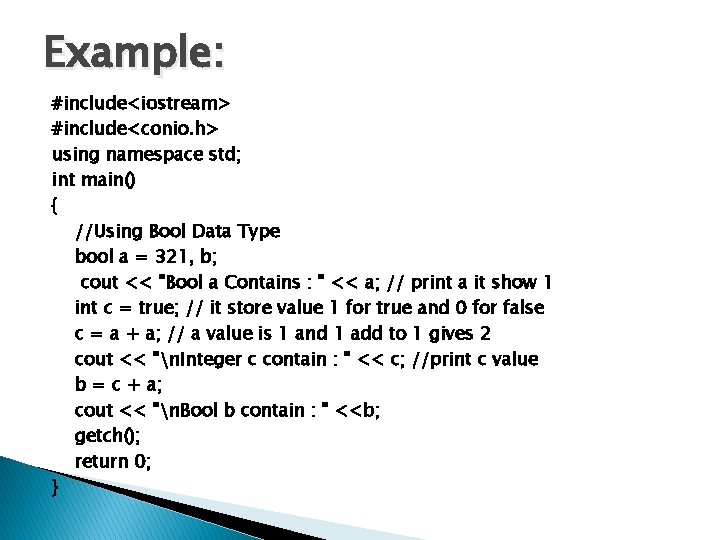
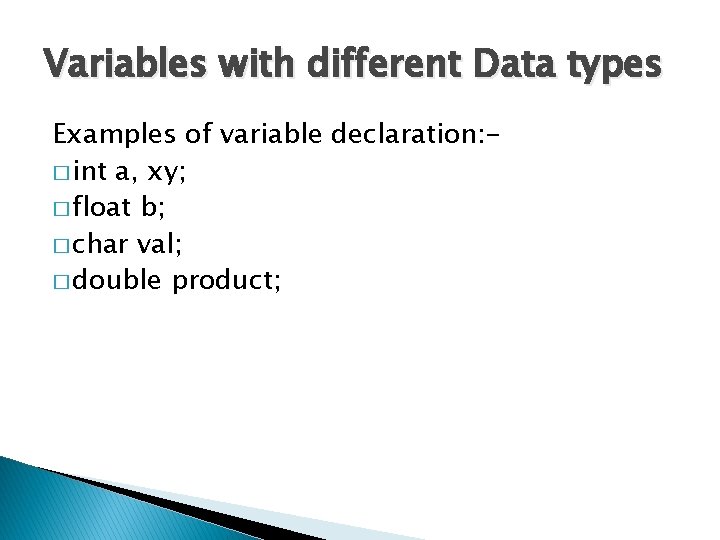
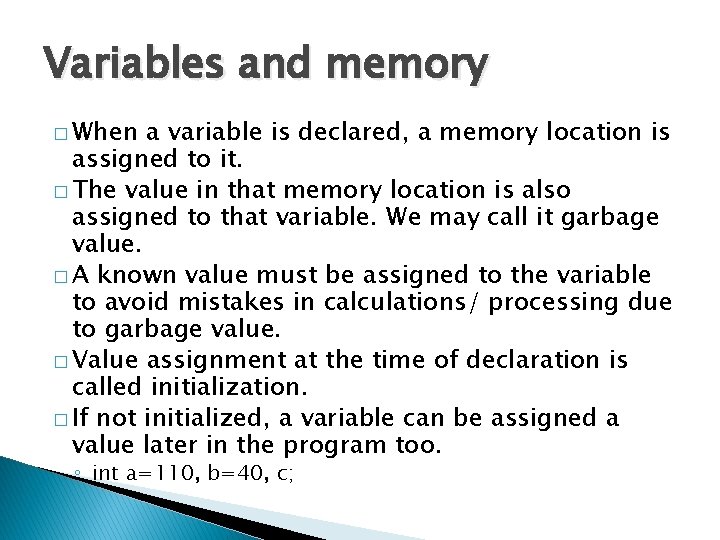
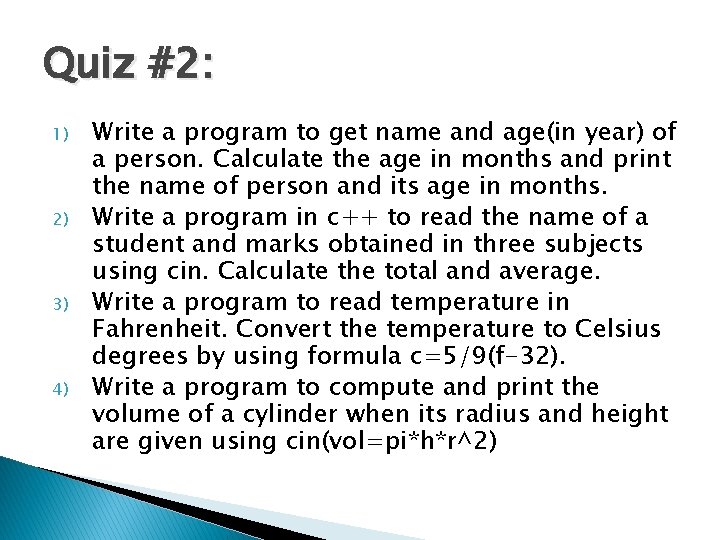
- Slides: 38
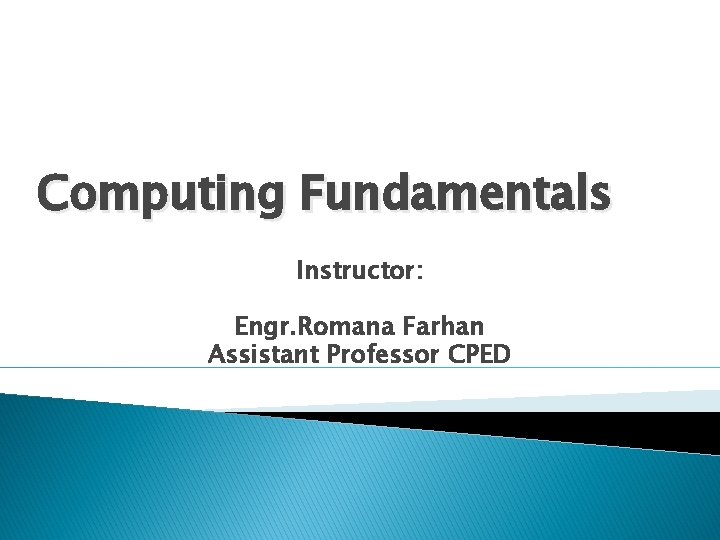
Computing Fundamentals Instructor: Engr. Romana Farhan Assistant Professor CPED
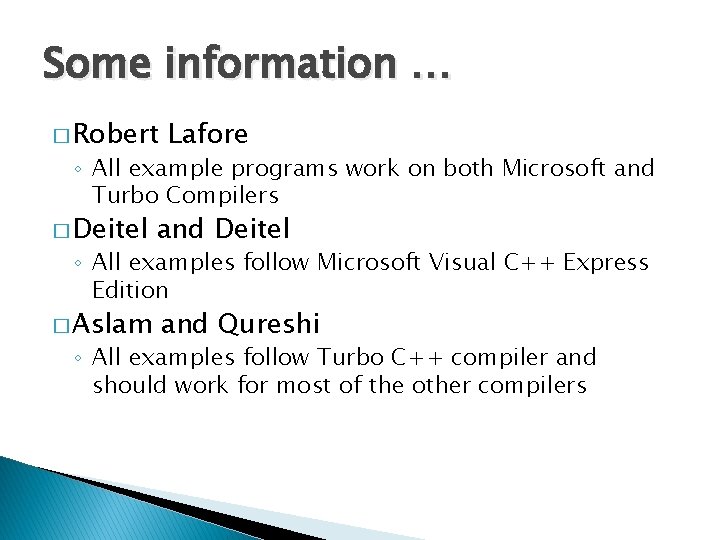
Some information … � Robert Lafore ◦ All example programs work on both Microsoft and Turbo Compilers � Deitel and Deitel � Aslam and Qureshi ◦ All examples follow Microsoft Visual C++ Express Edition ◦ All examples follow Turbo C++ compiler and should work for most of the other compilers
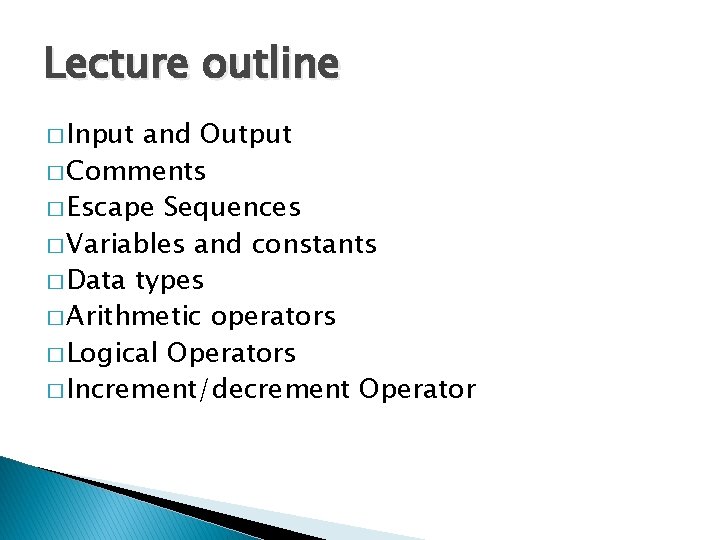
Lecture outline � Input and Output � Comments � Escape Sequences � Variables and constants � Data types � Arithmetic operators � Logical Operators � Increment/decrement Operator
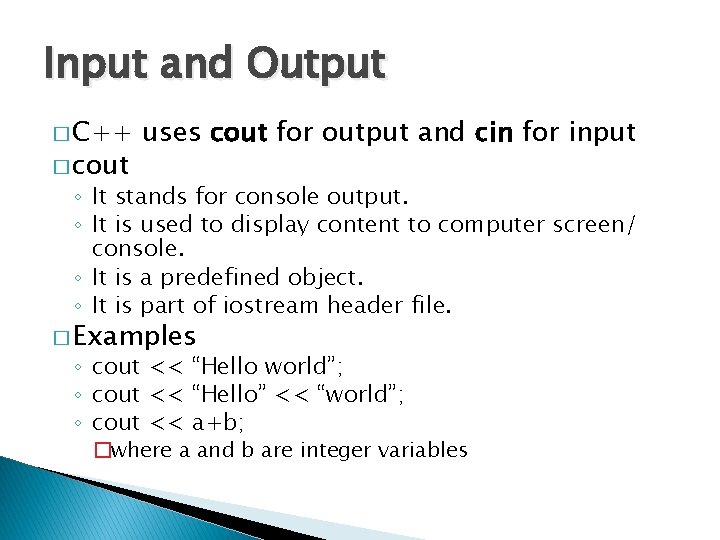
Input and Output � C++ � cout uses cout for output and cin for input ◦ It stands for console output. ◦ It is used to display content to computer screen/ console. ◦ It is a predefined object. ◦ It is part of iostream header file. � Examples ◦ cout << “Hello world”; ◦ cout << “Hello” << “world”; ◦ cout << a+b; �where a and b are integer variables
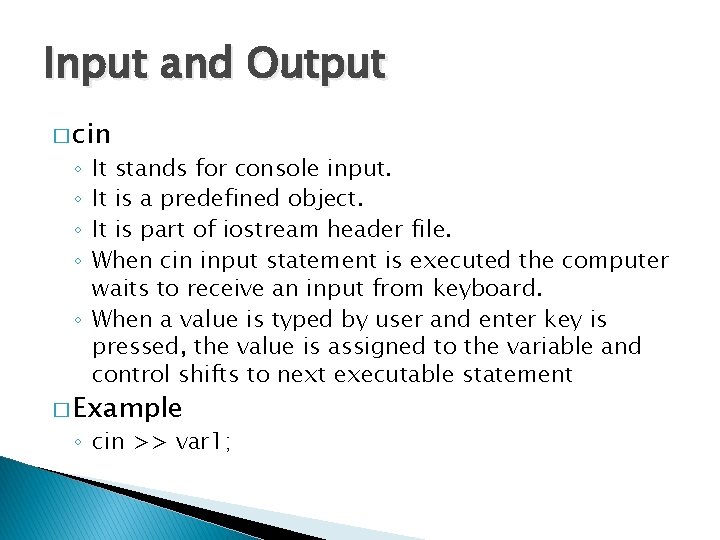
Input and Output � cin It stands for console input. It is a predefined object. It is part of iostream header file. When cin input statement is executed the computer waits to receive an input from keyboard. ◦ When a value is typed by user and enter key is pressed, the value is assigned to the variable and control shifts to next executable statement ◦ ◦ � Example ◦ cin >> var 1;
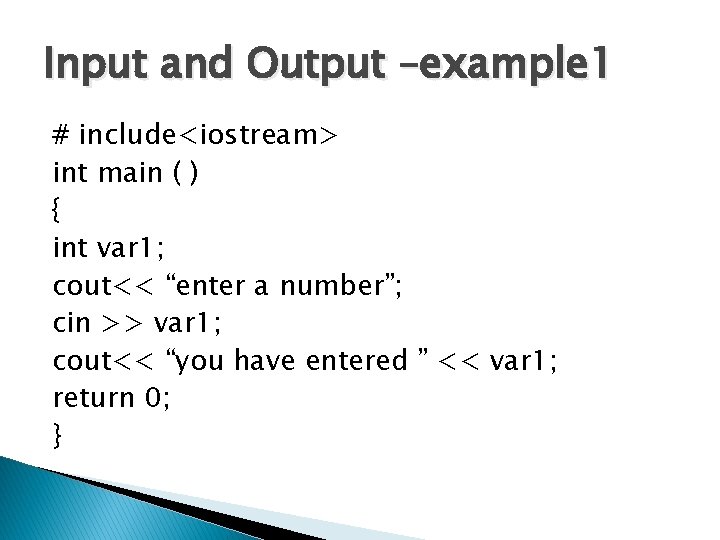
Input and Output –example 1 # include<iostream> int main ( ) { int var 1; cout<< “enter a number”; cin >> var 1; cout<< “you have entered ” << var 1; return 0; }
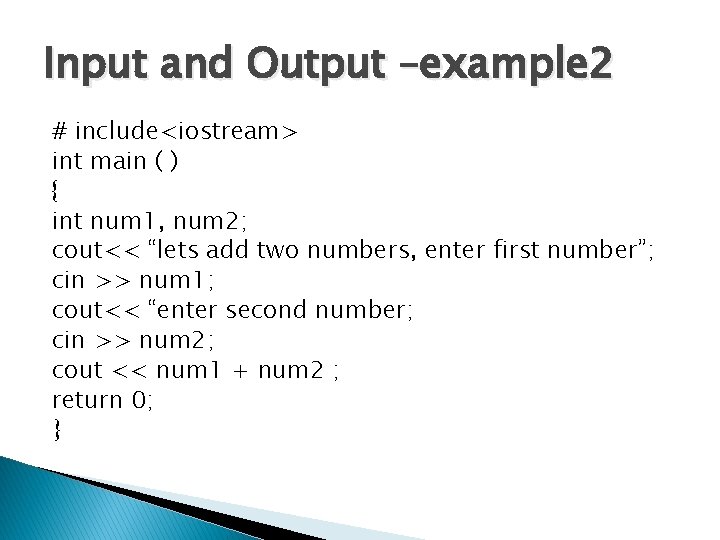
Input and Output –example 2 # include<iostream> int main ( ) { int num 1, num 2; cout<< “lets add two numbers, enter first number”; cin >> num 1; cout<< “enter second number; cin >> num 2; cout << num 1 + num 2 ; return 0; }
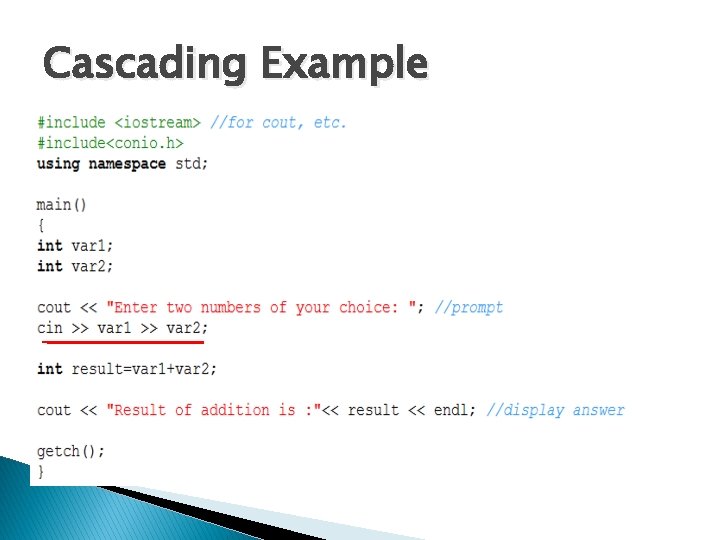
Cascading Example
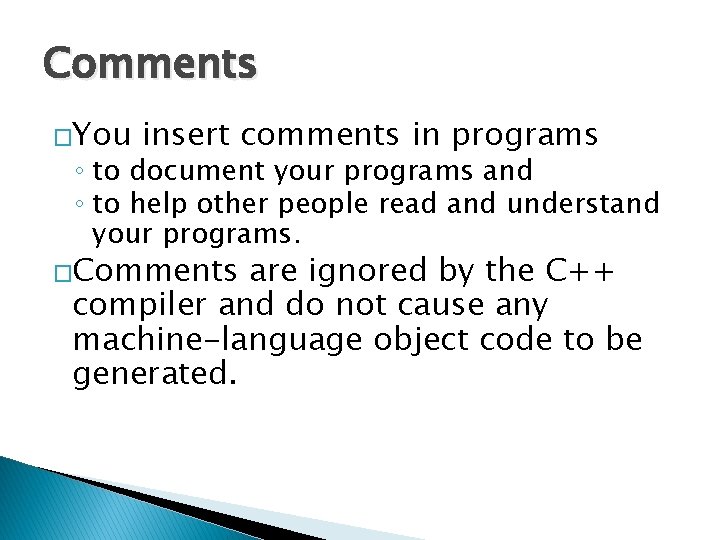
Comments �You insert comments in programs ◦ to document your programs and ◦ to help other people read and understand your programs. �Comments are ignored by the C++ compiler and do not cause any machine-language object code to be generated.
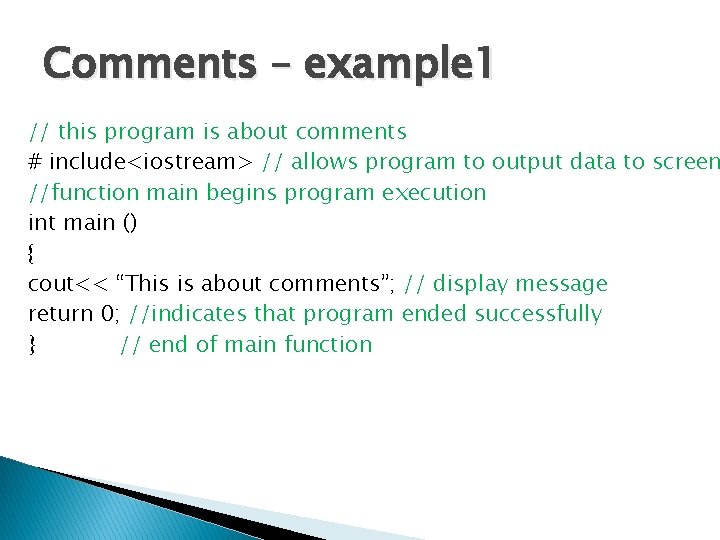
Comments – example 1 // this program is about comments # include<iostream> // allows program to output data to screen //function main begins program execution int main () { cout<< “This is about comments”; // display message return 0; //indicates that program ended successfully } // end of main function
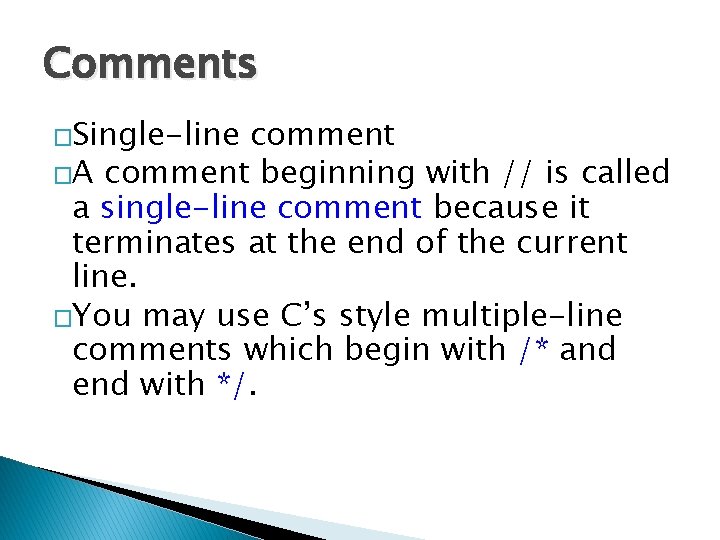
Comments �Single-line comment �A comment beginning with // is called a single-line comment because it terminates at the end of the current line. �You may use C’s style multiple-line comments which begin with /* and end with */.
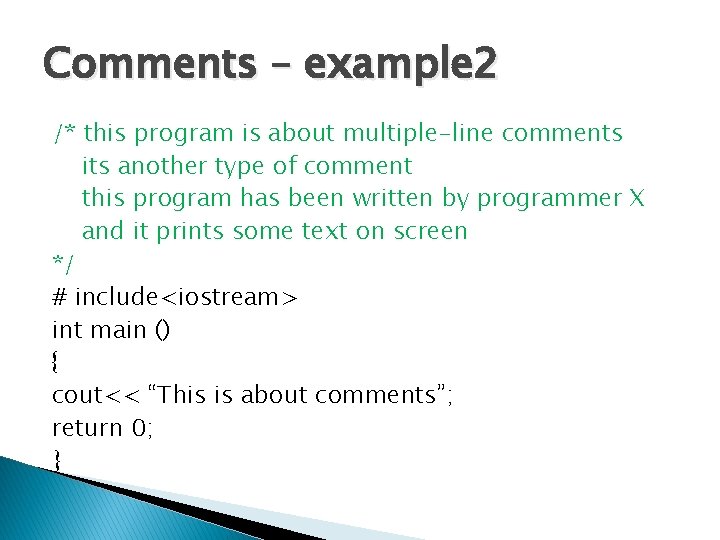
Comments – example 2 /* this program is about multiple-line comments its another type of comment this program has been written by programmer X and it prints some text on screen */ # include<iostream> int main () { cout<< “This is about comments”; return 0; }
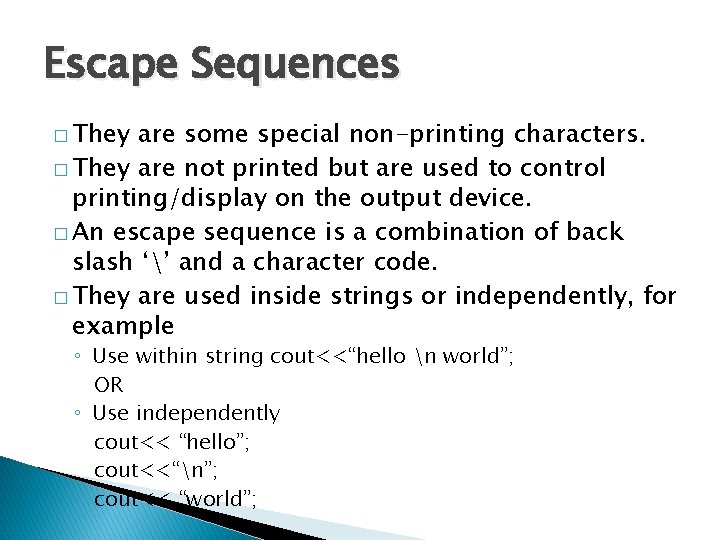
Escape Sequences � They are some special non-printing characters. � They are not printed but are used to control printing/display on the output device. � An escape sequence is a combination of back slash ‘’ and a character code. � They are used inside strings or independently, for example ◦ Use within string cout<<“hello n world”; OR ◦ Use independently cout<< “hello”; cout<<“n”; cout<< “world”;
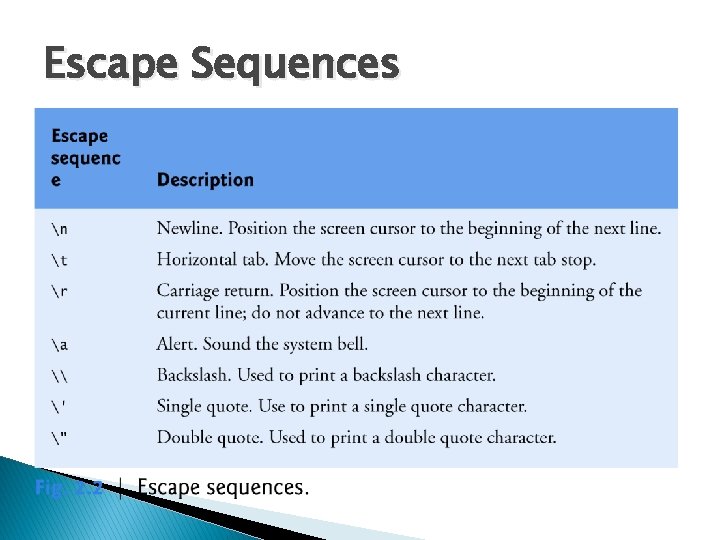
Escape Sequences
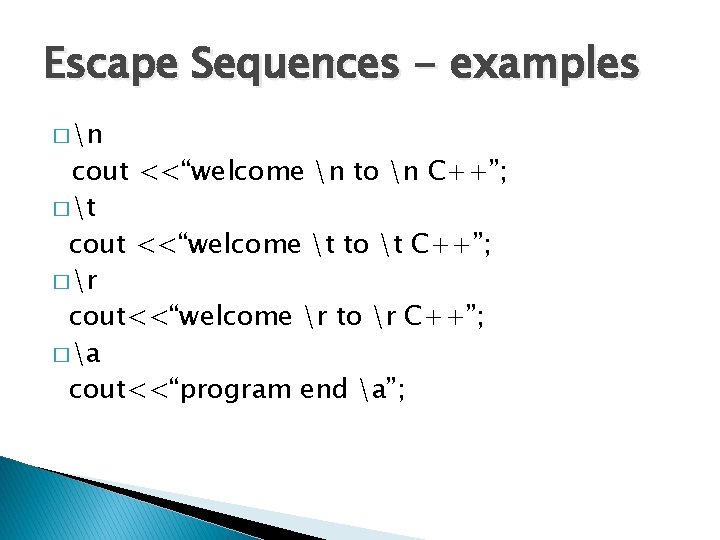
Escape Sequences - examples � n cout <<“welcome n to n C++”; � t cout <<“welcome t to t C++”; � r cout<<“welcome r to r C++”; � a cout<<“program end a”;
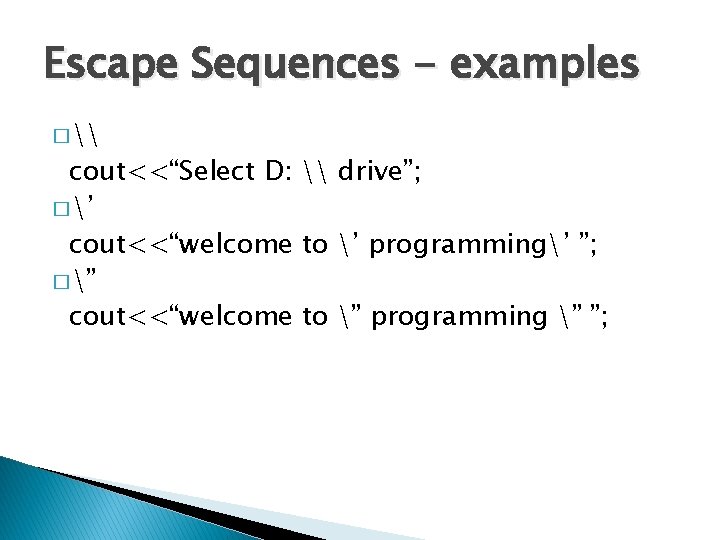
Escape Sequences - examples � \ cout<<“Select D: \ drive”; � ’ cout<<“welcome to ’ programming’ ”; � ” cout<<“welcome to ” programming ” ”;
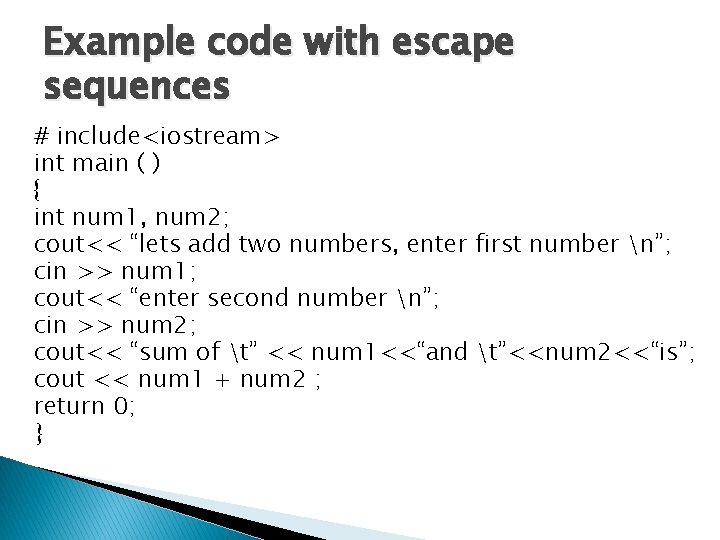
Example code with escape sequences # include<iostream> int main ( ) { int num 1, num 2; cout<< “lets add two numbers, enter first number n”; cin >> num 1; cout<< “enter second number n”; cin >> num 2; cout<< “sum of t” << num 1<<“and t”<<num 2<<“is”; cout << num 1 + num 2 ; return 0; }
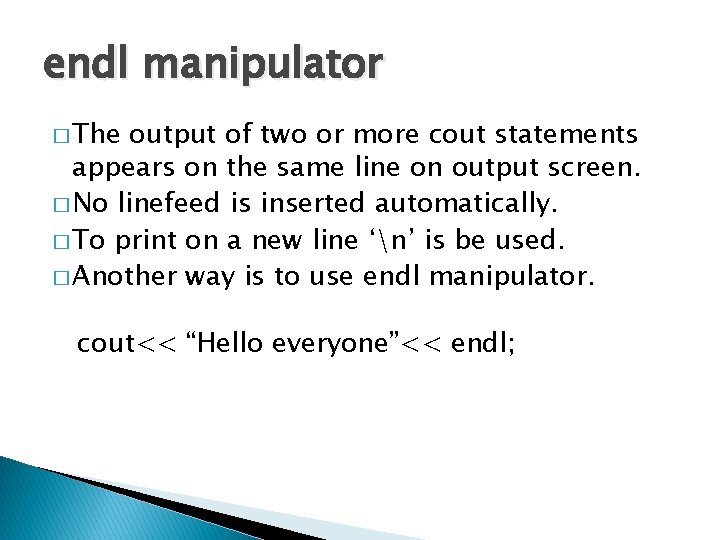
endl manipulator � The output of two or more cout statements appears on the same line on output screen. � No linefeed is inserted automatically. � To print on a new line ‘n’ is be used. � Another way is to use endl manipulator. cout<< “Hello everyone”<< endl;
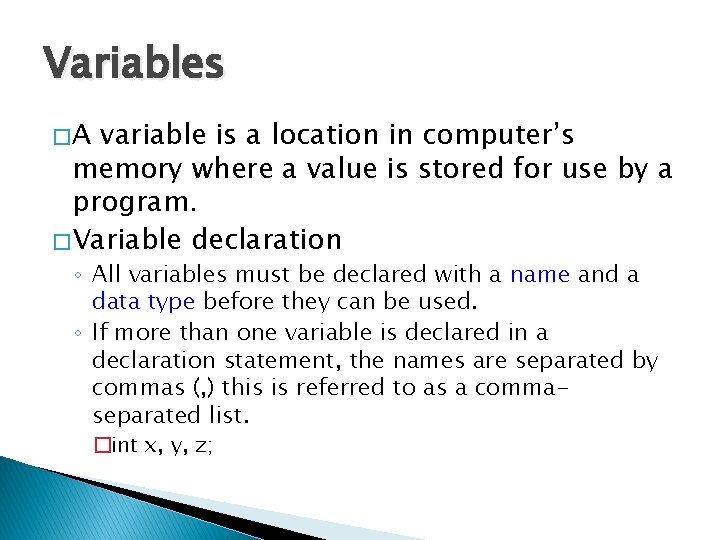
Variables �A variable is a location in computer’s memory where a value is stored for use by a program. � Variable declaration ◦ All variables must be declared with a name and a data type before they can be used. ◦ If more than one variable is declared in a declaration statement, the names are separated by commas (, ) this is referred to as a commaseparated list. �int x, y, z;
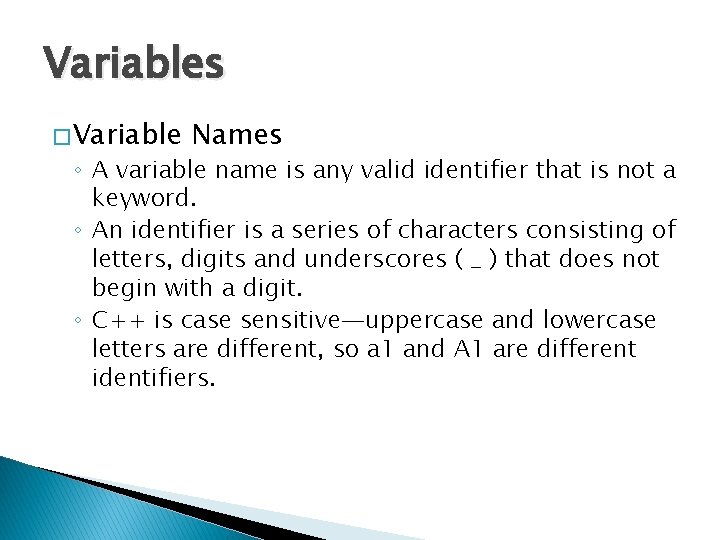
Variables � Variable Names ◦ A variable name is any valid identifier that is not a keyword. ◦ An identifier is a series of characters consisting of letters, digits and underscores ( _ ) that does not begin with a digit. ◦ C++ is case sensitive—uppercase and lowercase letters are different, so a 1 and A 1 are different identifiers.
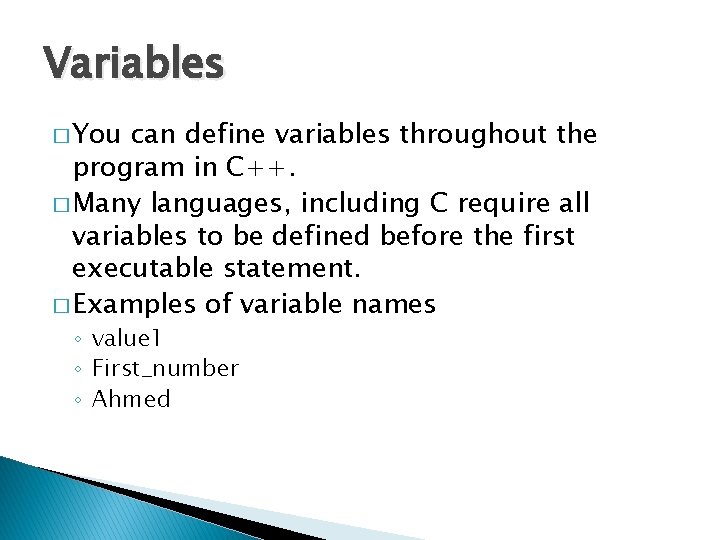
Variables � You can define variables throughout the program in C++. � Many languages, including C require all variables to be defined before the first executable statement. � Examples of variable names ◦ value 1 ◦ First_number ◦ Ahmed
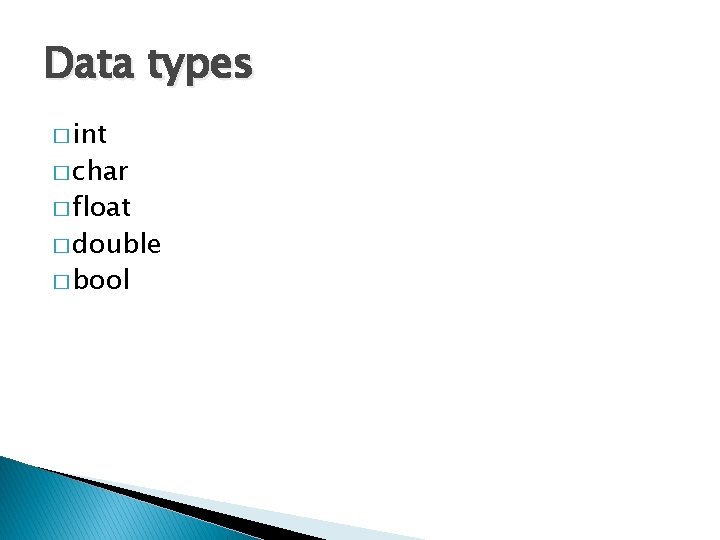
Data types � int � char � float � double � bool
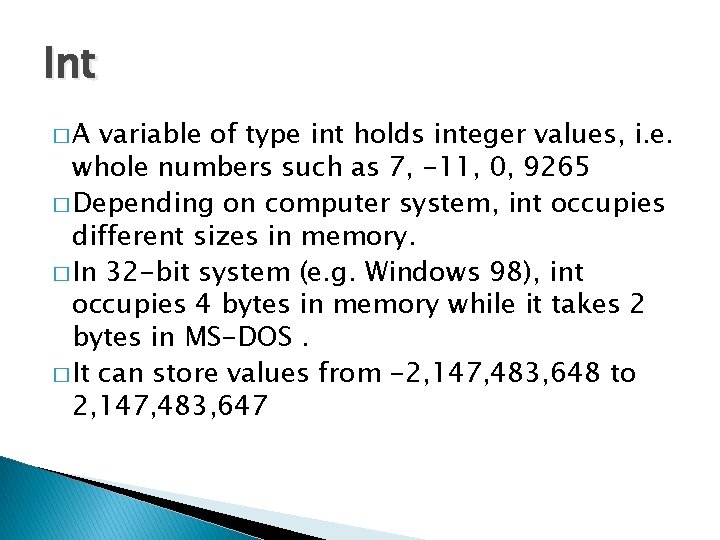
Int �A variable of type int holds integer values, i. e. whole numbers such as 7, -11, 0, 9265 � Depending on computer system, int occupies different sizes in memory. � In 32 -bit system (e. g. Windows 98), int occupies 4 bytes in memory while it takes 2 bytes in MS-DOS. � It can store values from -2, 147, 483, 648 to 2, 147, 483, 647
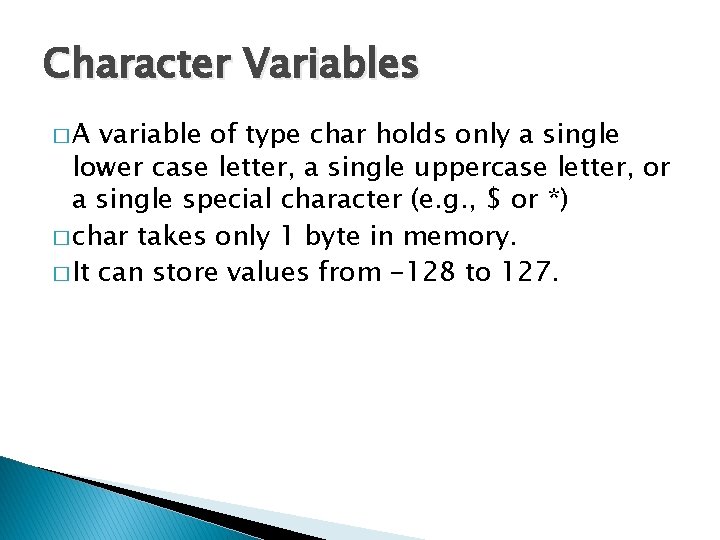
Character Variables �A variable of type char holds only a single lower case letter, a single uppercase letter, or a single special character (e. g. , $ or *) � char takes only 1 byte in memory. � It can store values from -128 to 127.
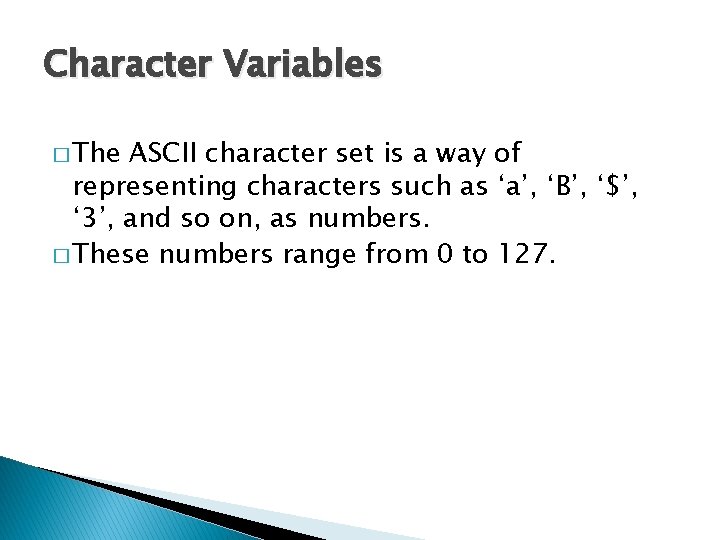
Character Variables � The ASCII character set is a way of representing characters such as ‘a’, ‘B’, ‘$’, ‘ 3’, and so on, as numbers. � These numbers range from 0 to 127.
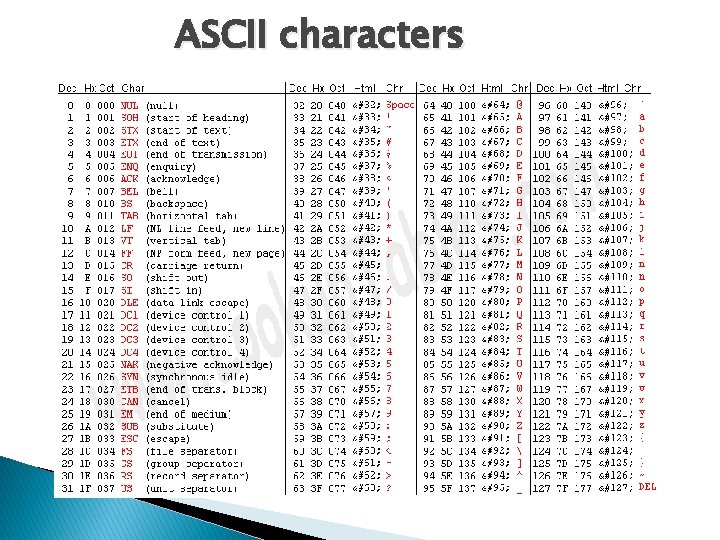
ASCII characters
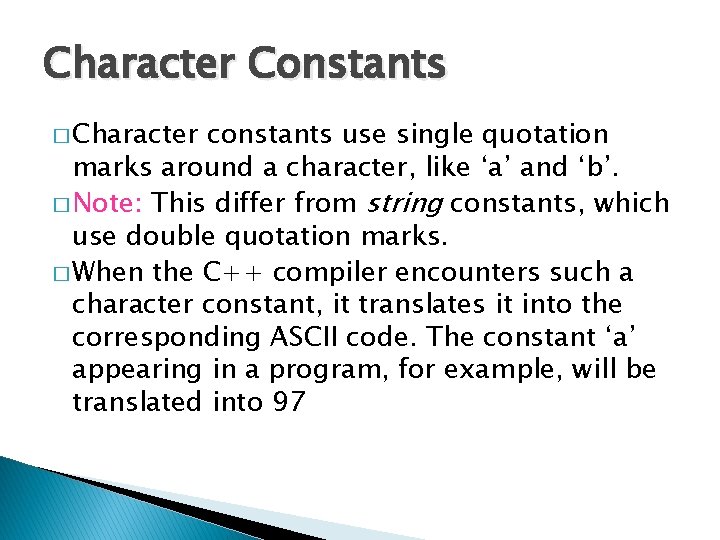
Character Constants � Character constants use single quotation marks around a character, like ‘a’ and ‘b’. � Note: This differ from string constants, which use double quotation marks. � When the C++ compiler encounters such a character constant, it translates it into the corresponding ASCII code. The constant ‘a’ appearing in a program, for example, will be translated into 97
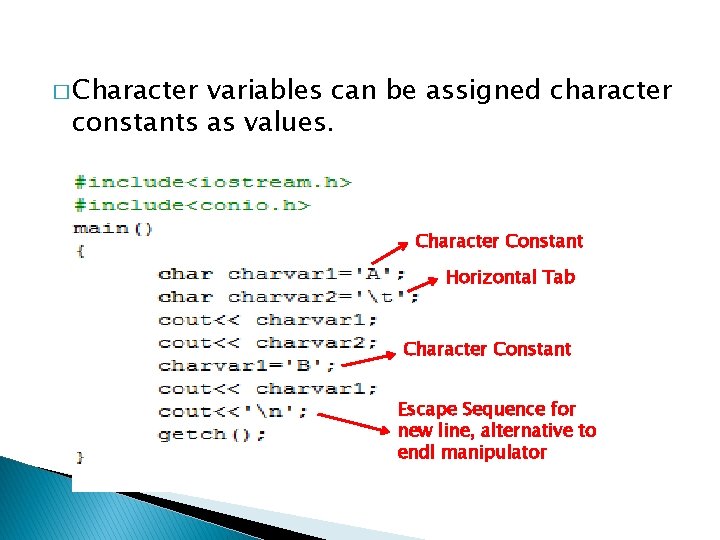
� Character variables can be assigned character constants as values. Character Constant Horizontal Tab Character Constant Escape Sequence for new line, alternative to endl manipulator
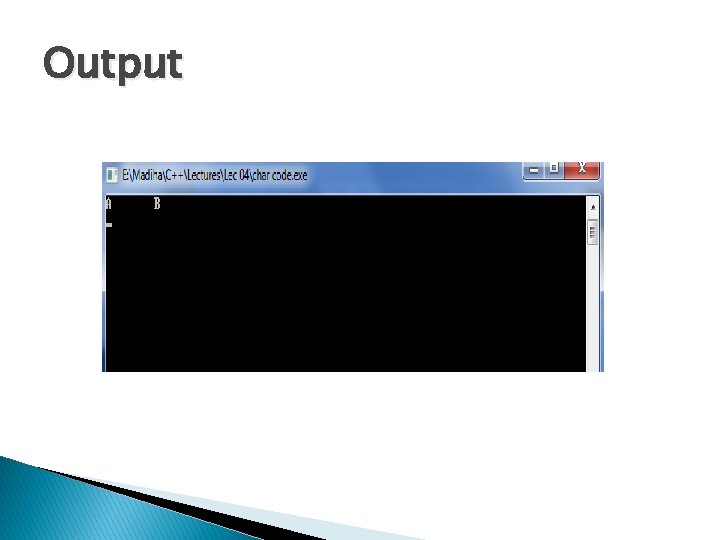
Output
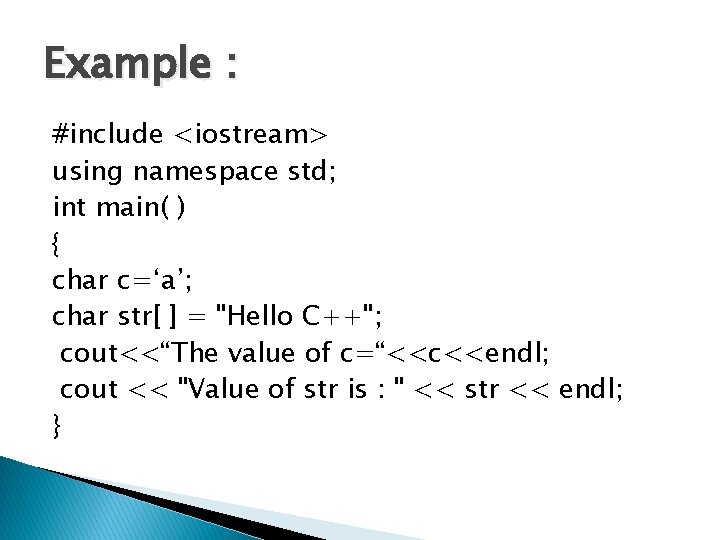
Example : #include <iostream> using namespace std; int main( ) { char c=‘a’; char str[ ] = "Hello C++"; cout<<“The value of c=“<<c<<endl; cout << "Value of str is : " << str << endl; }
![Example include iostream using namespace std int main char name50 cout Example : #include <iostream> using namespace std; int main( ) { char name[50]; cout](https://slidetodoc.com/presentation_image_h2/739fa01453916bc34e20001a02fa0133/image-31.jpg)
Example : #include <iostream> using namespace std; int main( ) { char name[50]; cout << "Please enter your name: "; cin >> name; cout << "Your name is: " << name << endl; }
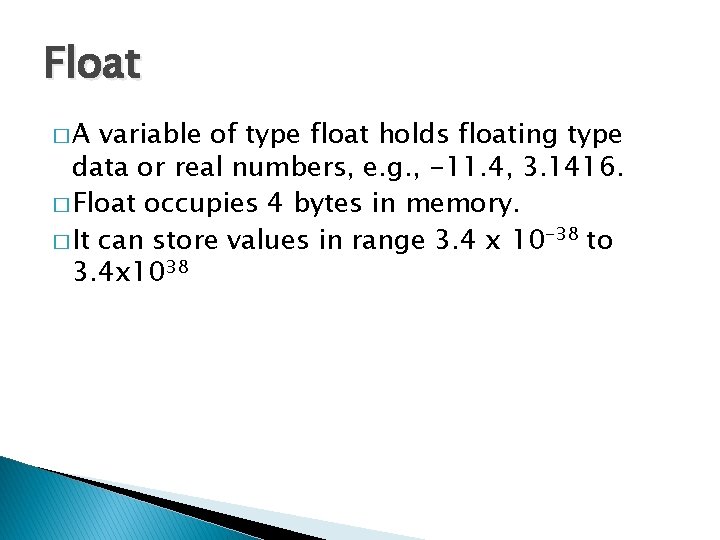
Float �A variable of type float holds floating type data or real numbers, e. g. , -11. 4, 3. 1416. � Float occupies 4 bytes in memory. � It can store values in range 3. 4 x 10 -38 to 3. 4 x 1038
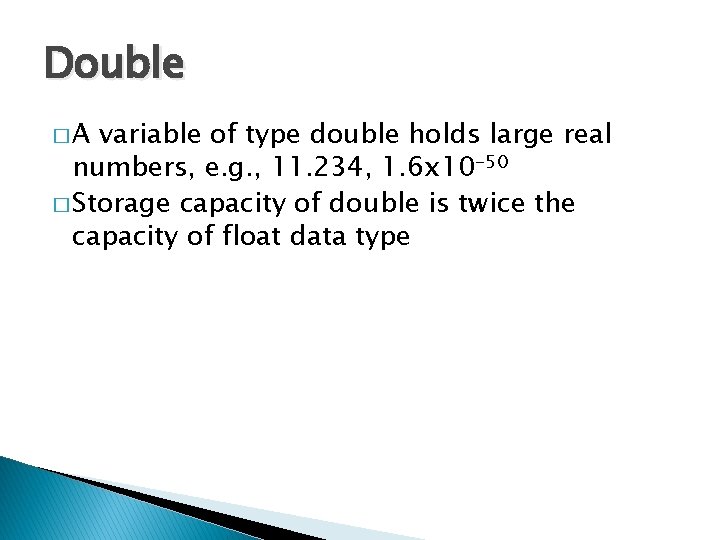
Double �A variable of type double holds large real numbers, e. g. , 11. 234, 1. 6 x 10 -50 � Storage capacity of double is twice the capacity of float data type
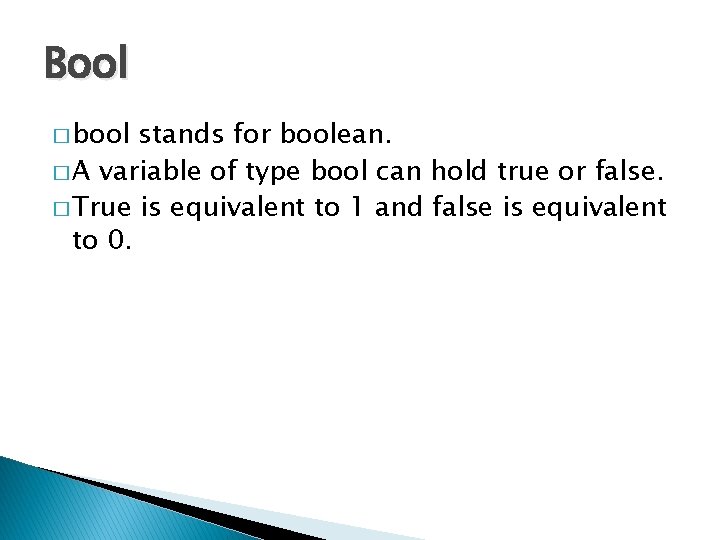
Bool � bool stands for boolean. � A variable of type bool can hold true or false. � True is equivalent to 1 and false is equivalent to 0.
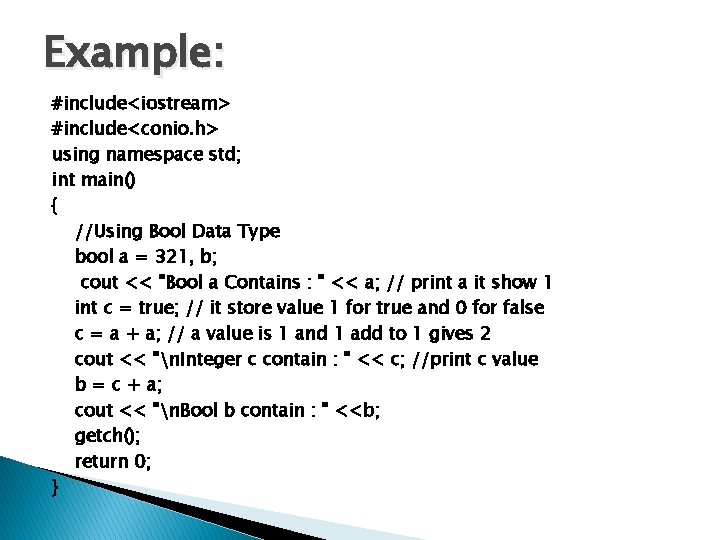
Example: #include<iostream> #include<conio. h> using namespace std; int main() { //Using Bool Data Type bool a = 321, b; cout << "Bool a Contains : " << a; // print a it show 1 int c = true; // it store value 1 for true and 0 for false c = a + a; // a value is 1 and 1 add to 1 gives 2 cout << "n. Integer c contain : " << c; //print c value b = c + a; cout << "n. Bool b contain : " <<b; getch(); return 0; }
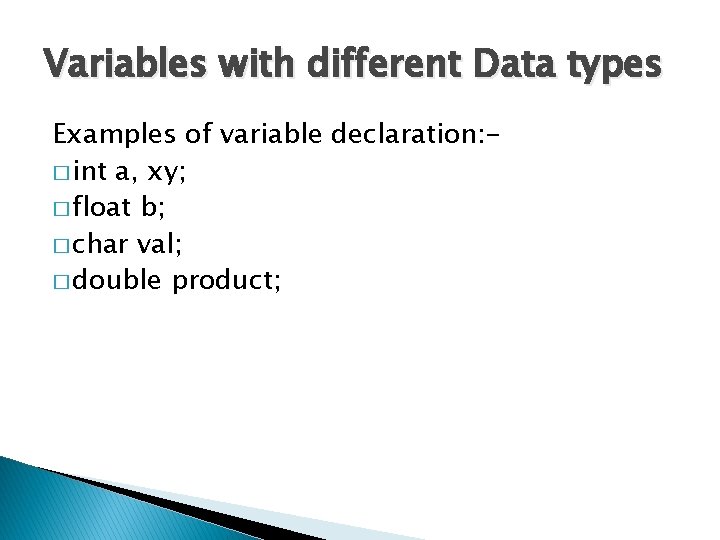
Variables with different Data types Examples of variable declaration: � int a, xy; � float b; � char val; � double product;
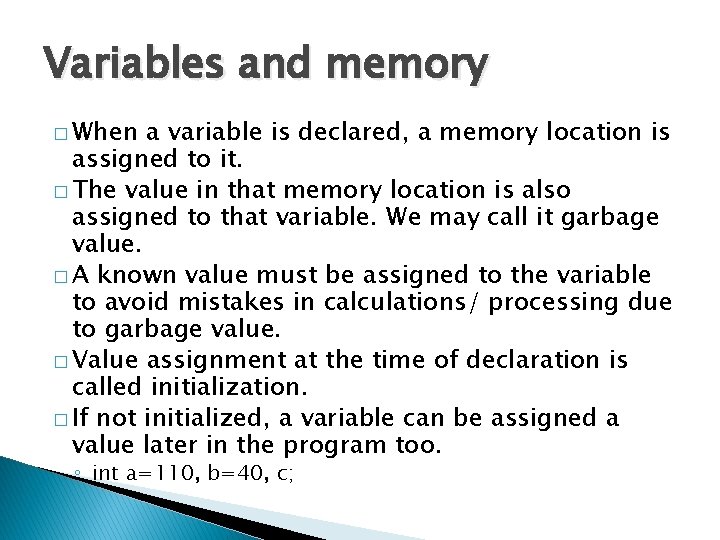
Variables and memory � When a variable is declared, a memory location is assigned to it. � The value in that memory location is also assigned to that variable. We may call it garbage value. � A known value must be assigned to the variable to avoid mistakes in calculations/ processing due to garbage value. � Value assignment at the time of declaration is called initialization. � If not initialized, a variable can be assigned a value later in the program too. ◦ int a=110, b=40, c;
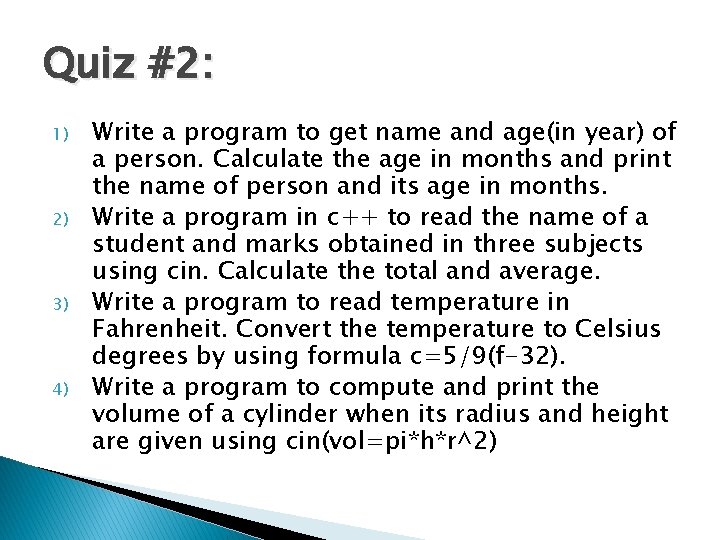
Quiz #2: 1) 2) 3) 4) Write a program to get name and age(in year) of a person. Calculate the age in months and print the name of person and its age in months. Write a program in c++ to read the name of a student and marks obtained in three subjects using cin. Calculate the total and average. Write a program to read temperature in Fahrenheit. Convert the temperature to Celsius degrees by using formula c=5/9(f-32). Write a program to compute and print the volume of a cylinder when its radius and height are given using cin(vol=pi*h*r^2)
Xxx mian
Cuhk assistant professor salary
Promotion from assistant to associate professor
Mian ahmad farhan
Farhan helmy
Quarter turn belt drive
Farhan sajjad
Logic and computer design fundamentals
Engr 350
Engr 1181
Engr 112
Engineering 10 sjsu
Mala tiskana slova
Performance task: roller coaster design
Engr 248
Perfectly plastic collision
Engr 201
Unr cse
Engr 1330
Tamu engr 482
Engr 10 sjsu
Engr 1182
Engr 1181
Engr 1330
Engr 1181
Engr 1181
Conventional computing and intelligent computing
Naismith was an instructor of
Instructor operating station
Ospfv
Jrotc marksmanship instructor course online
Cisco instructor certification
Virtual instructor.com
Basic instructor course texas
Pepperball launcher nomenclature
Basic instructor course #1014
Please clean your own room
Catia instructor
Tcole 1014 basic instructor course