Chapter 7 C Language Preliminaries Chapter 7 C
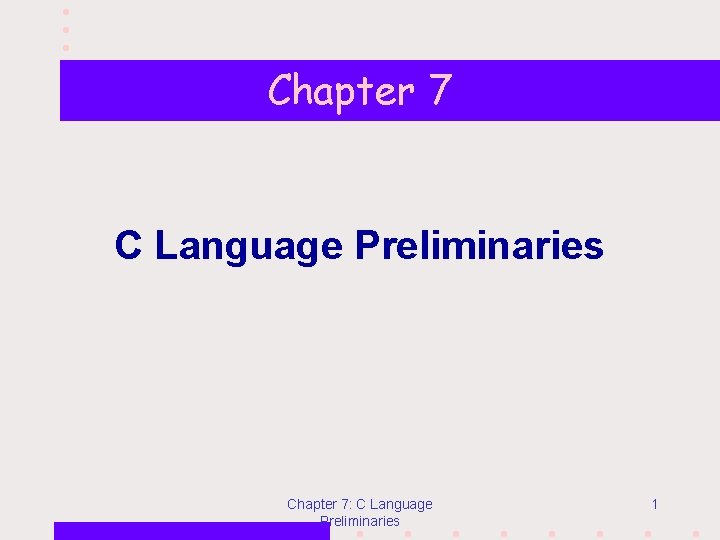
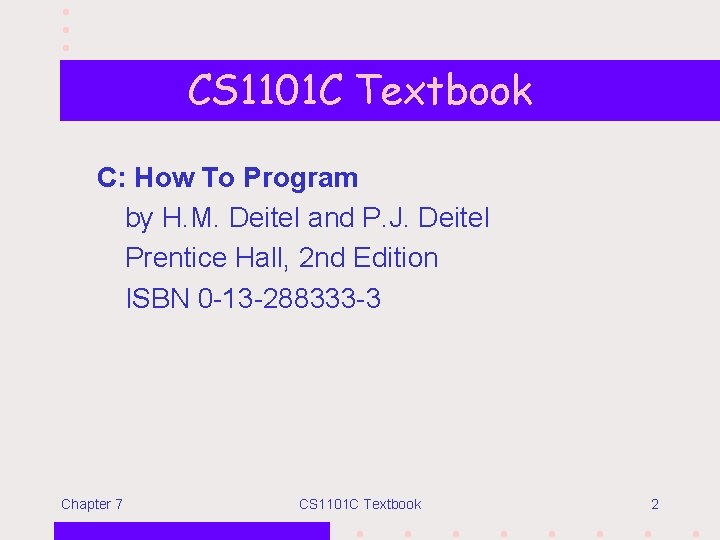
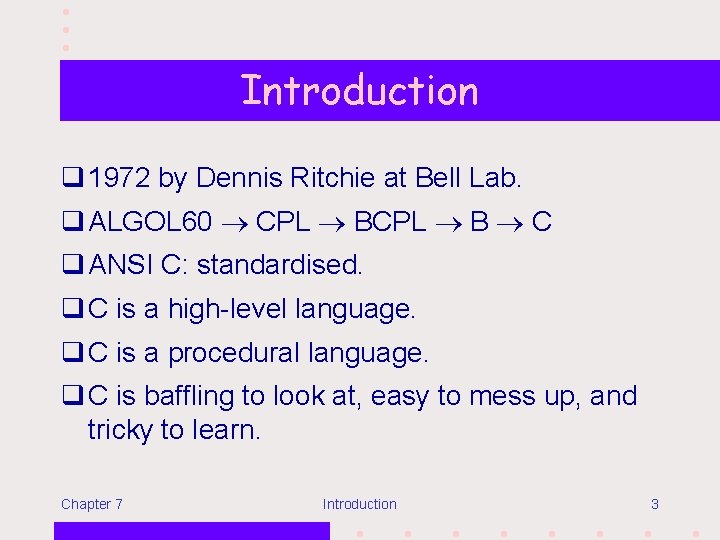
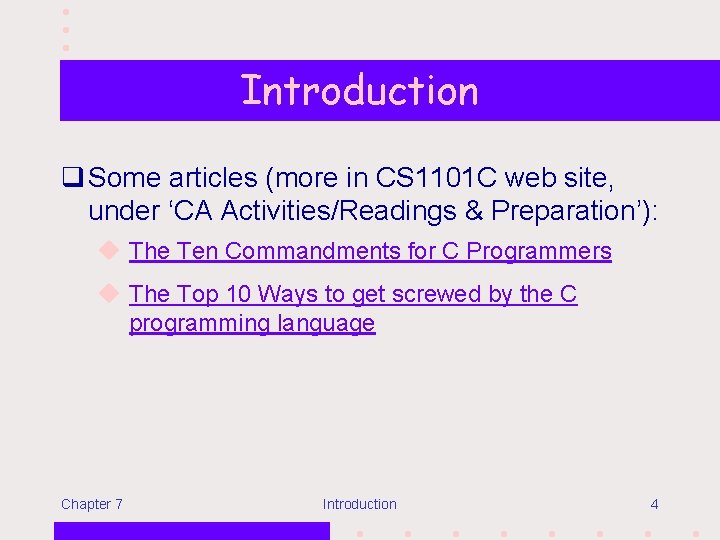
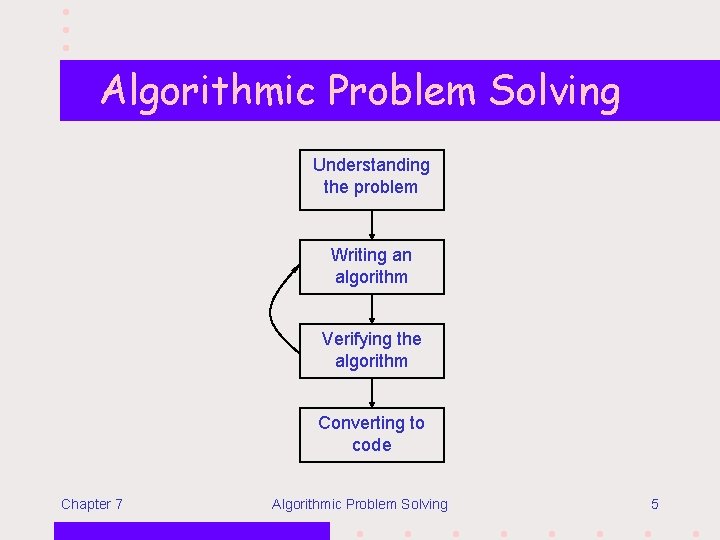
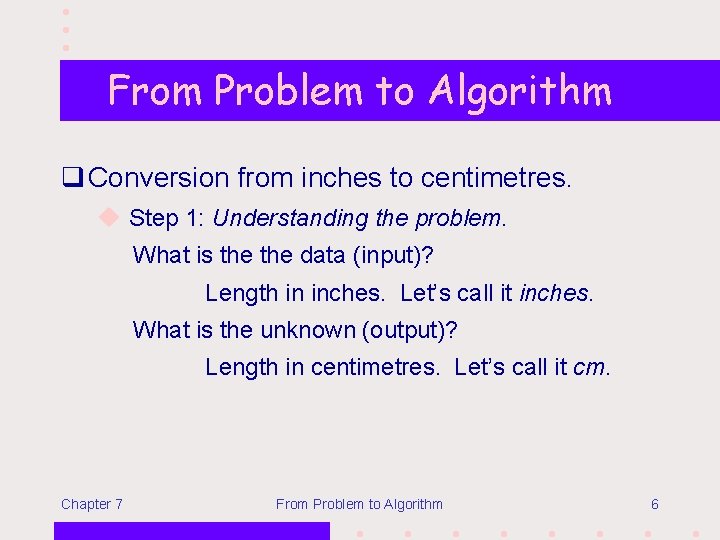
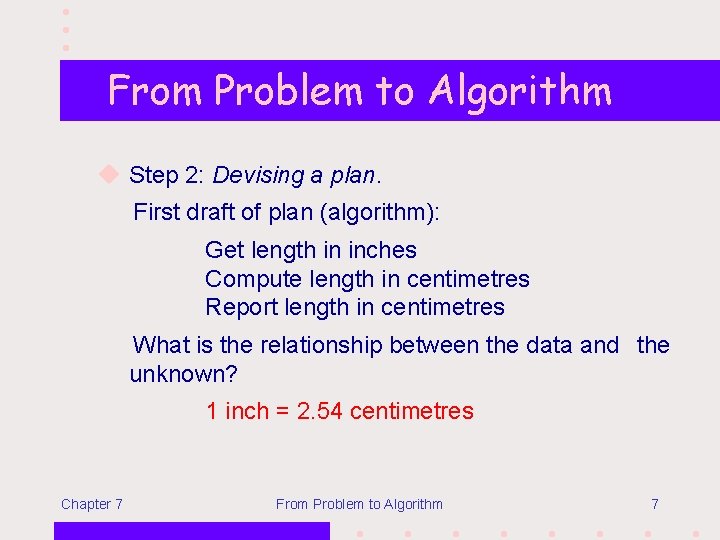
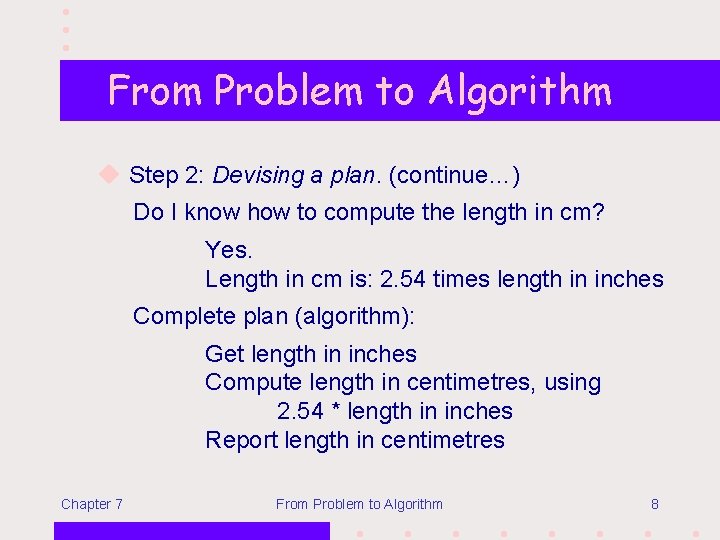
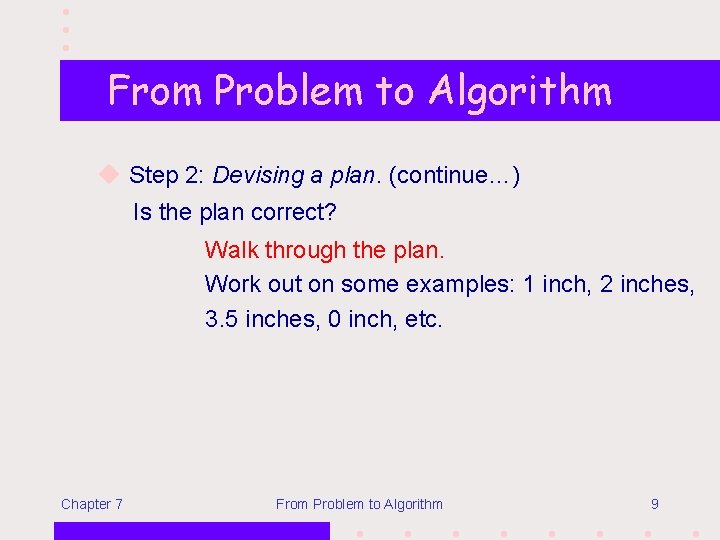
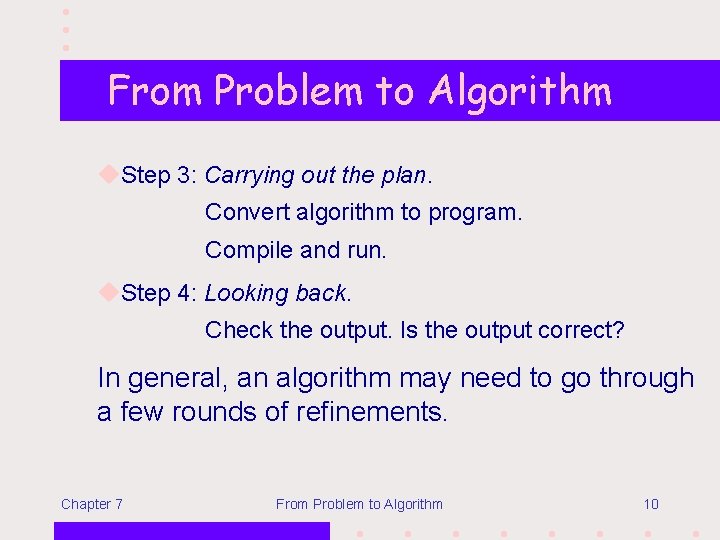
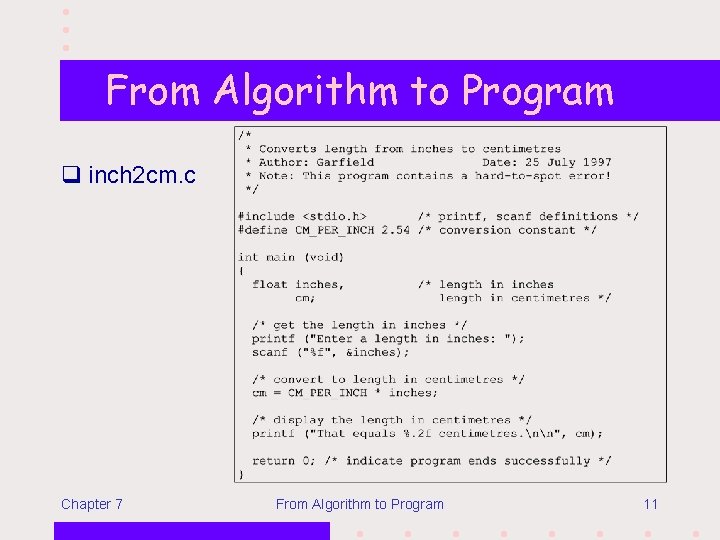
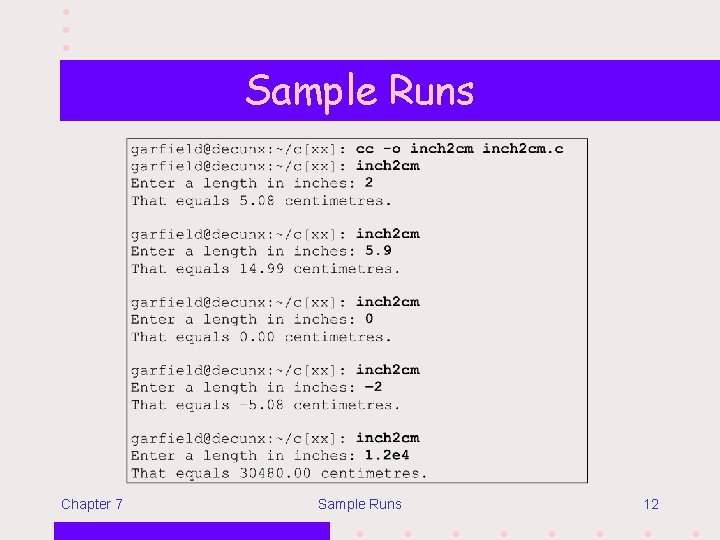
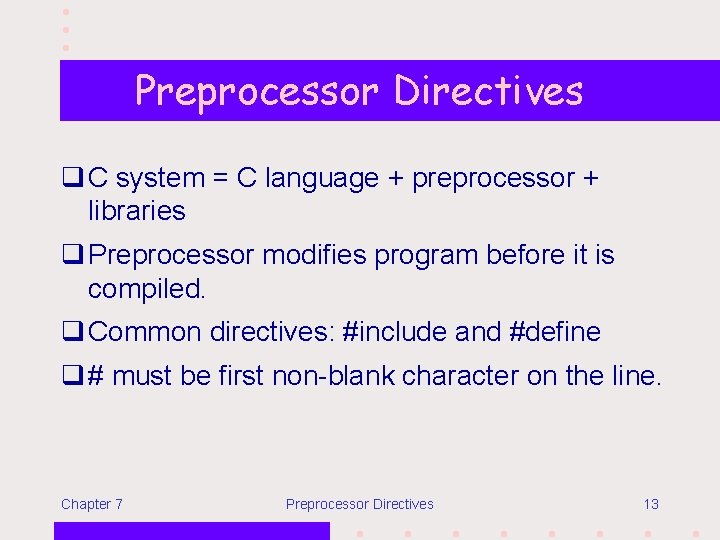
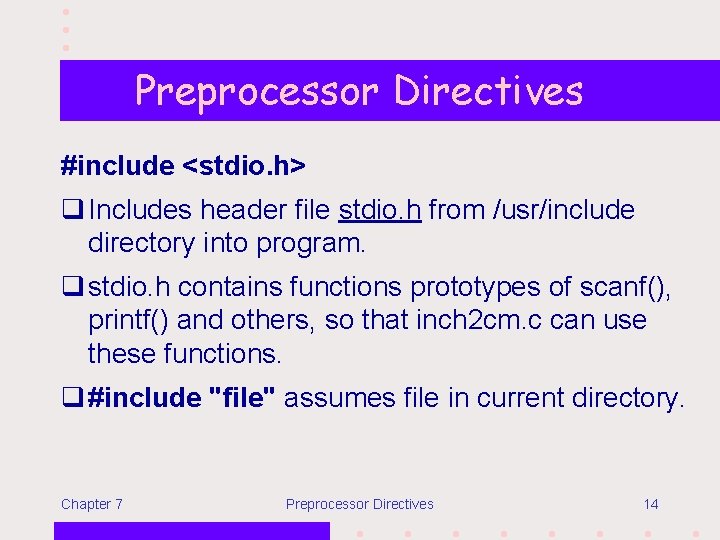
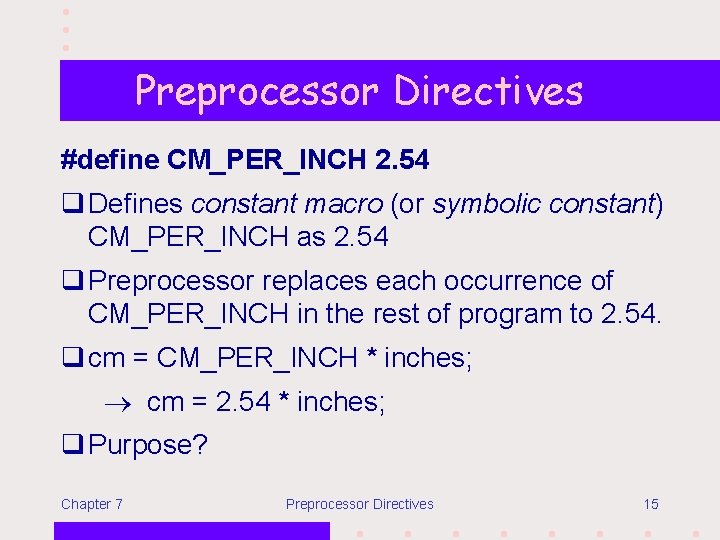
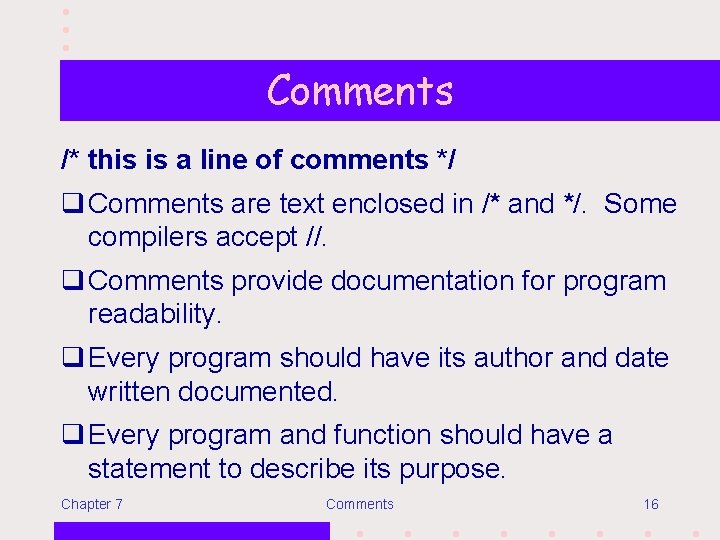
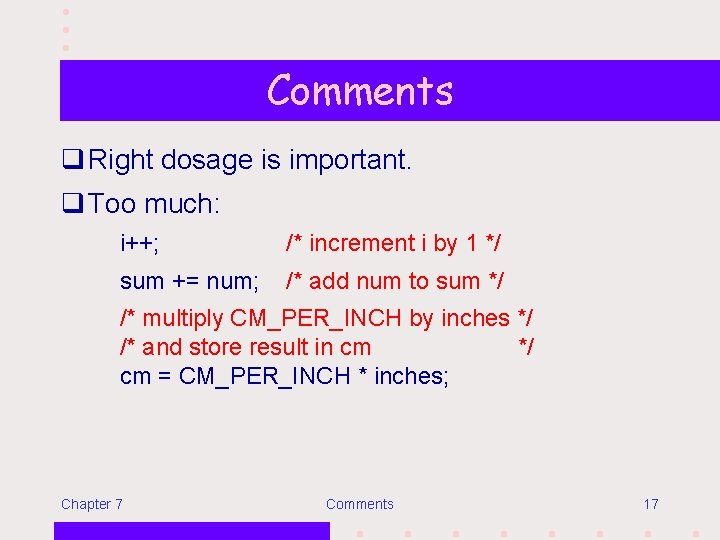
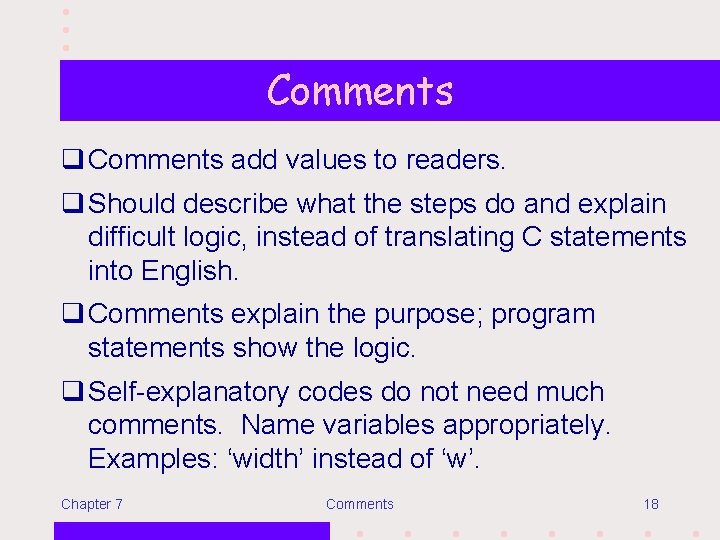
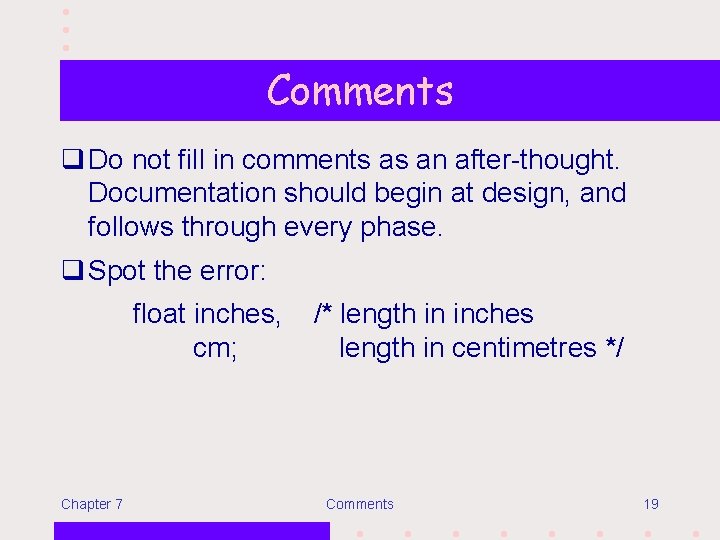
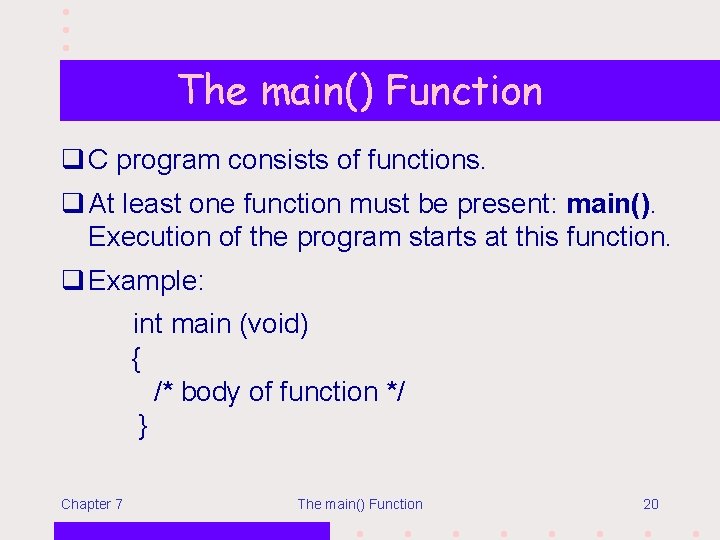
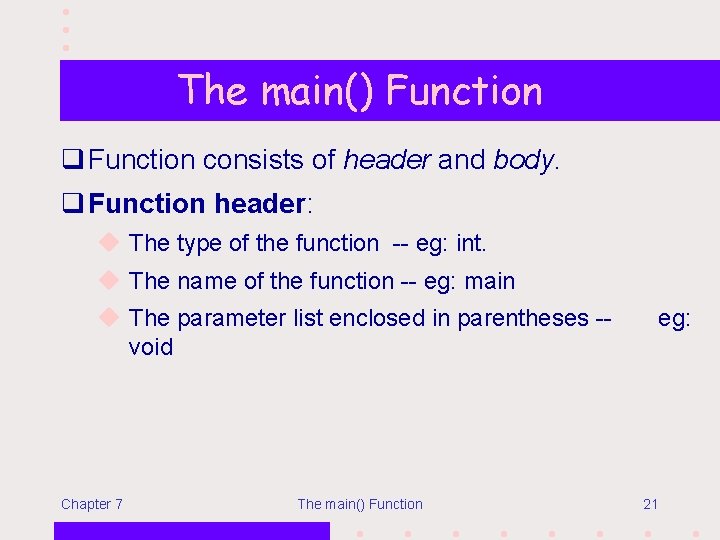
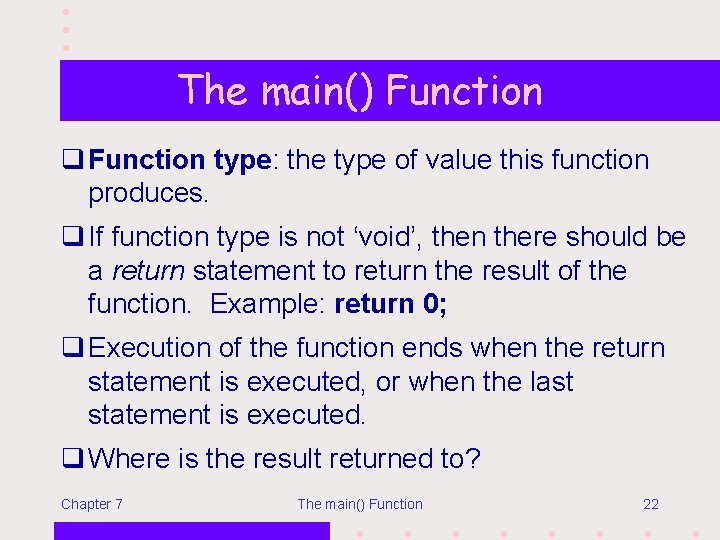
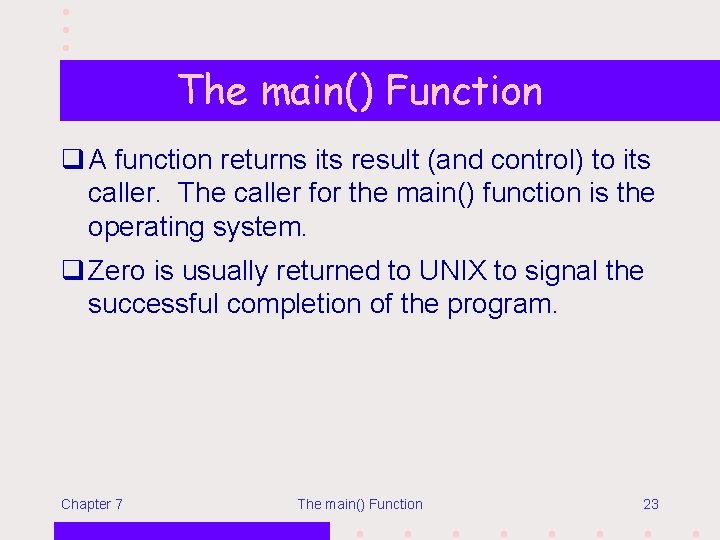
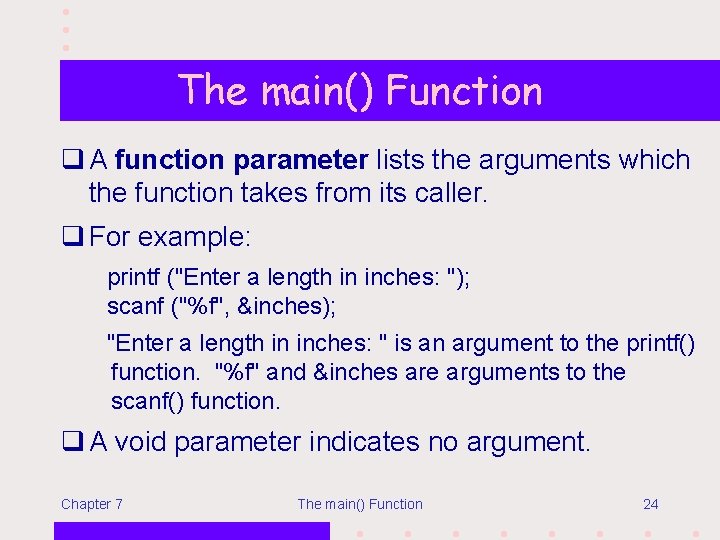
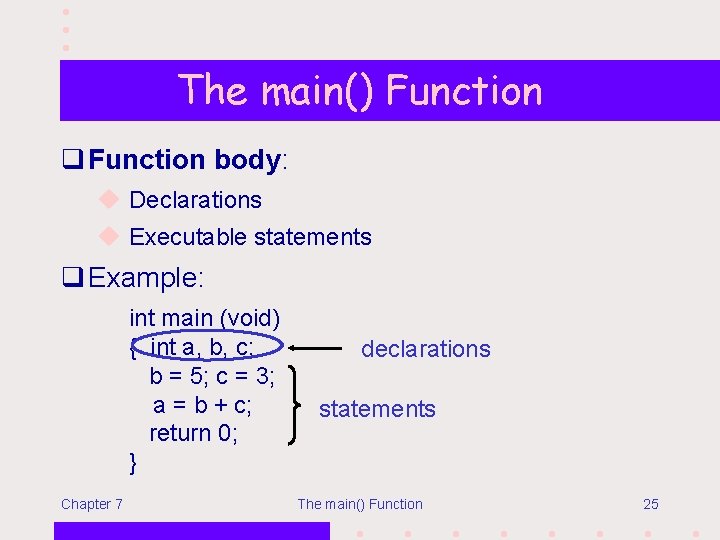
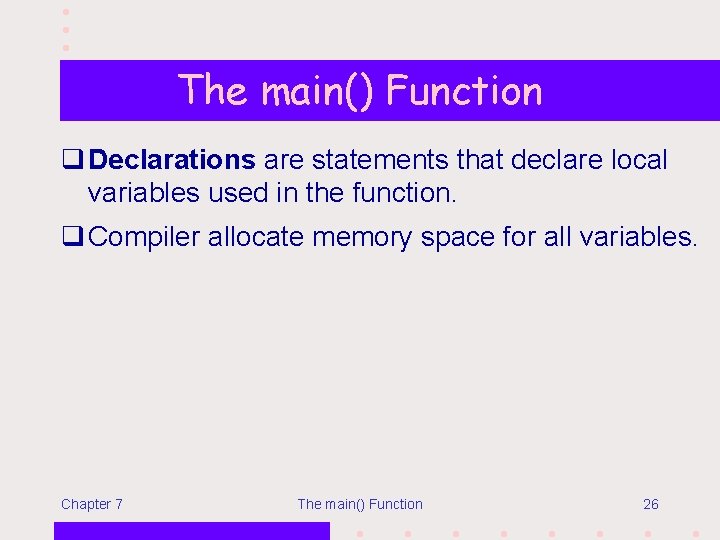
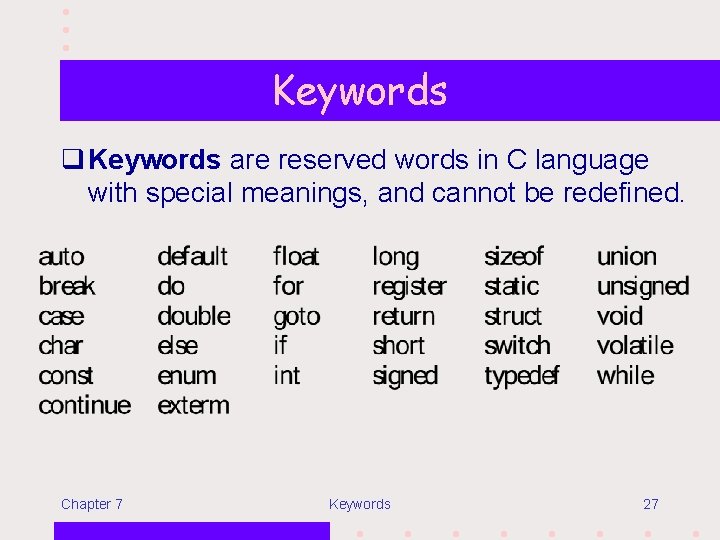
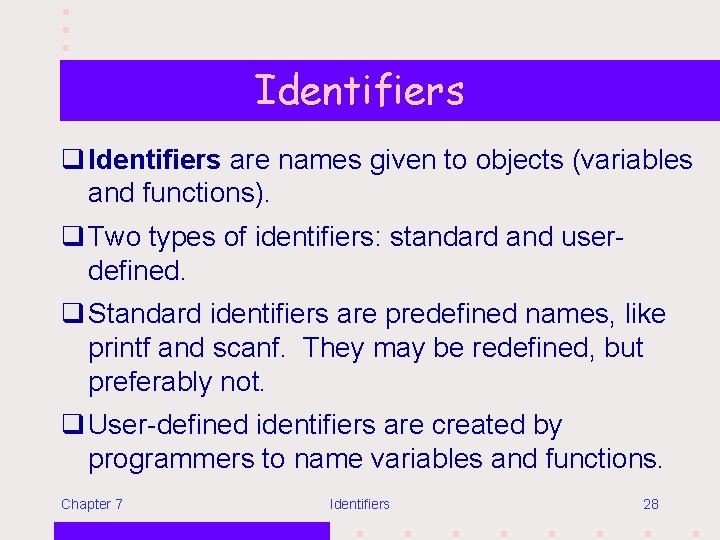
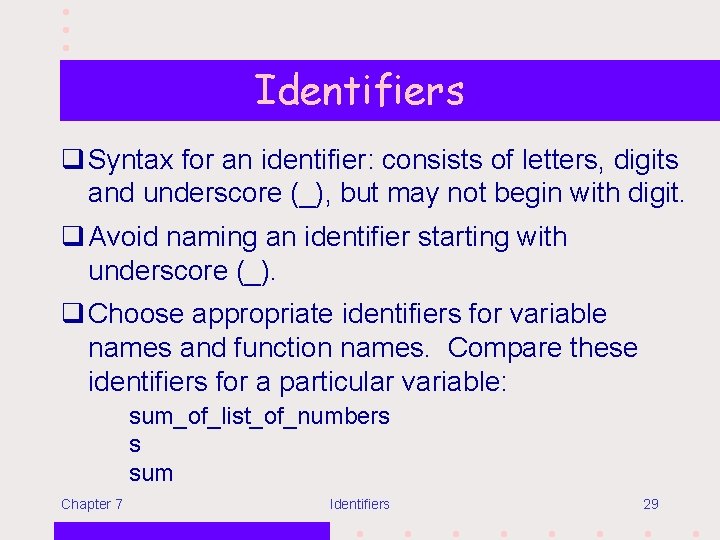
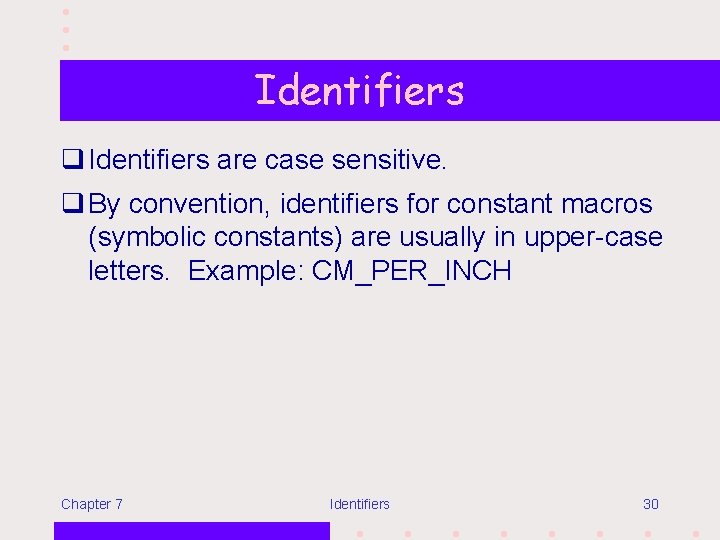
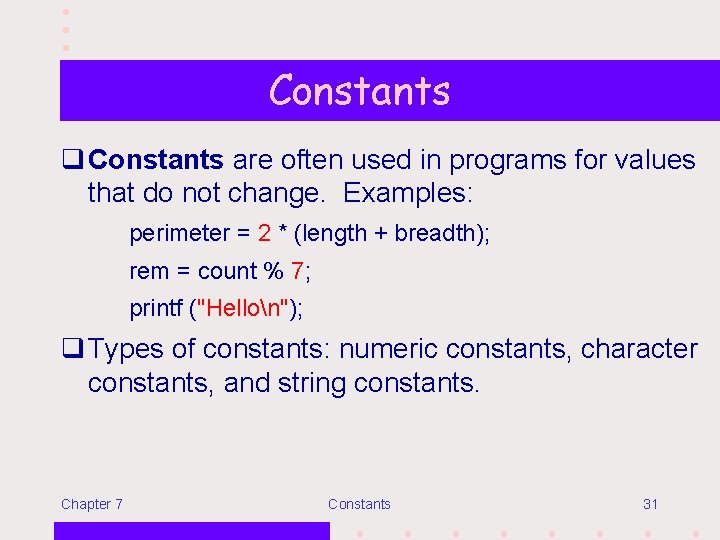
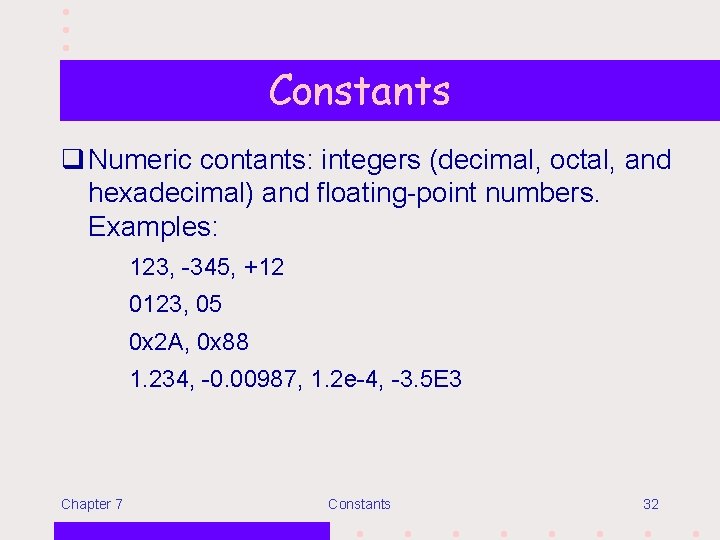
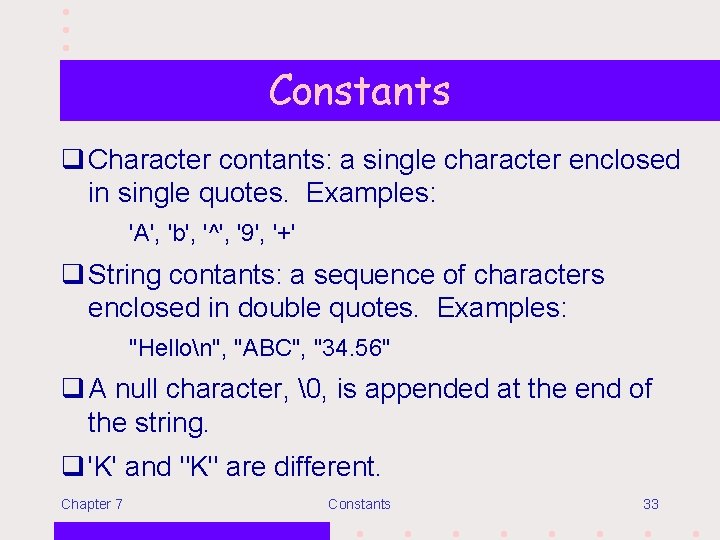
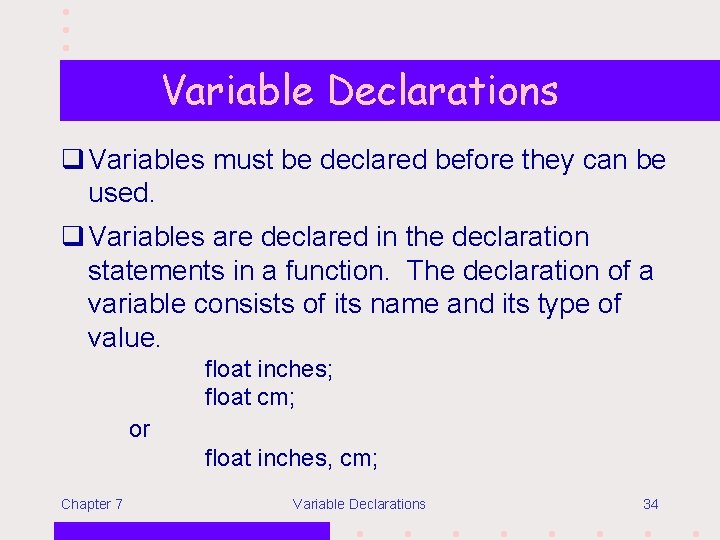
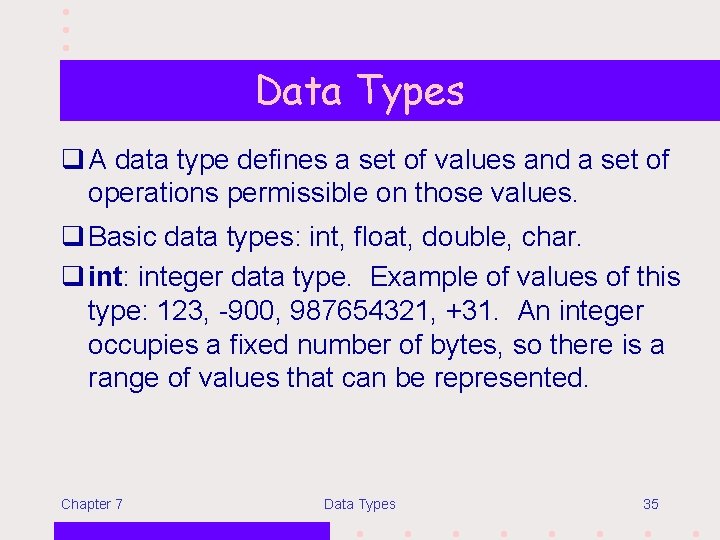
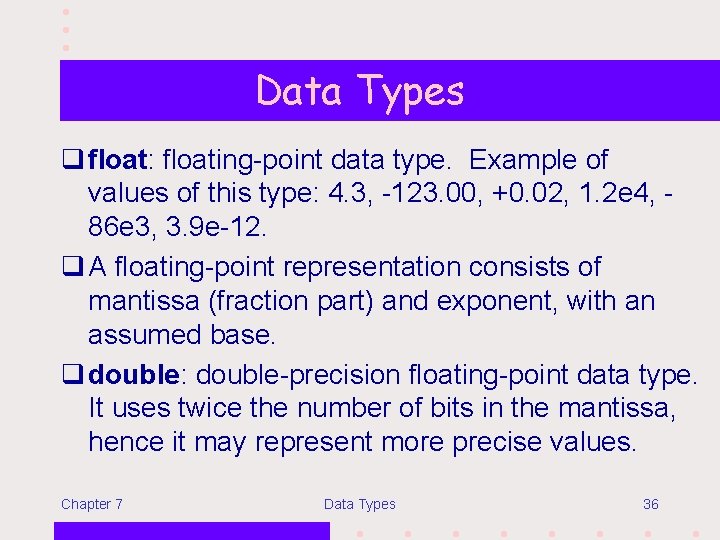
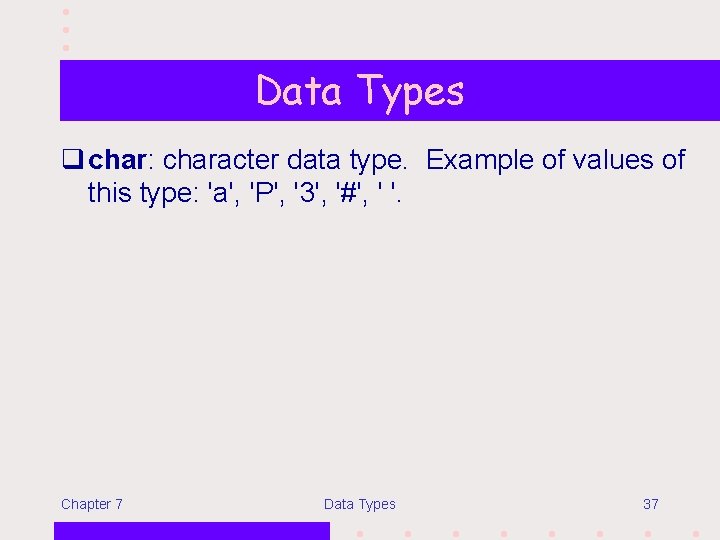
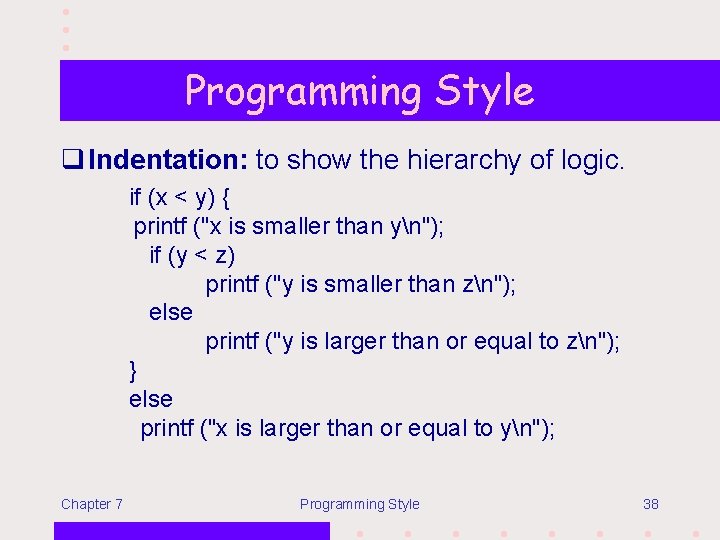
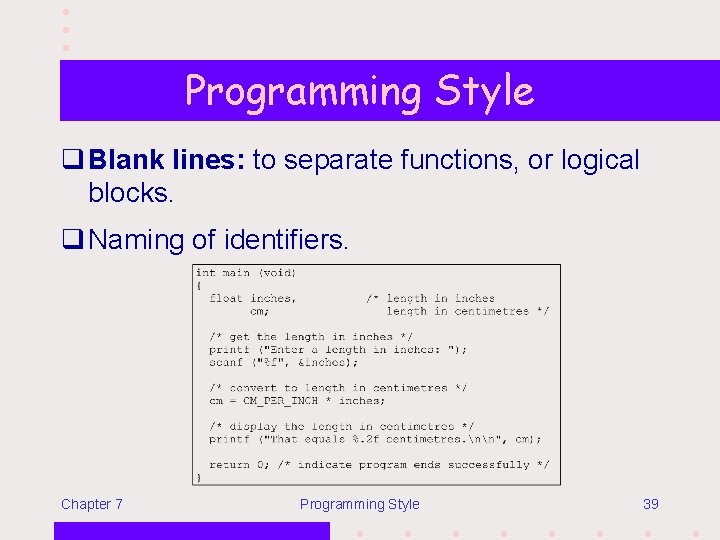
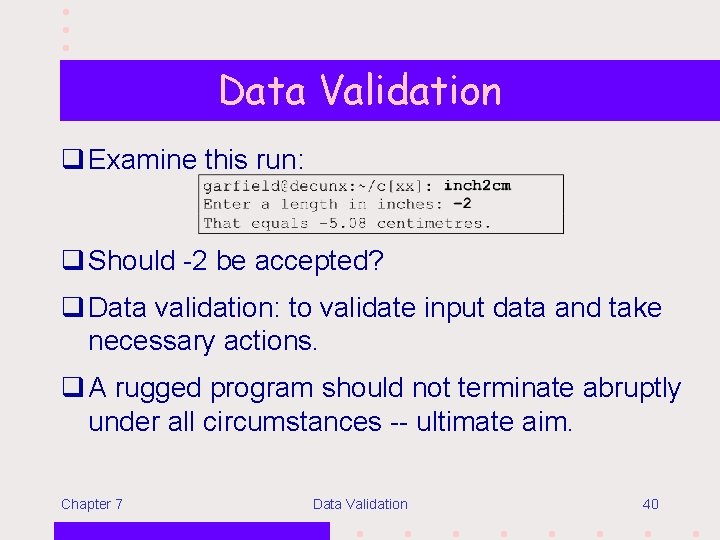
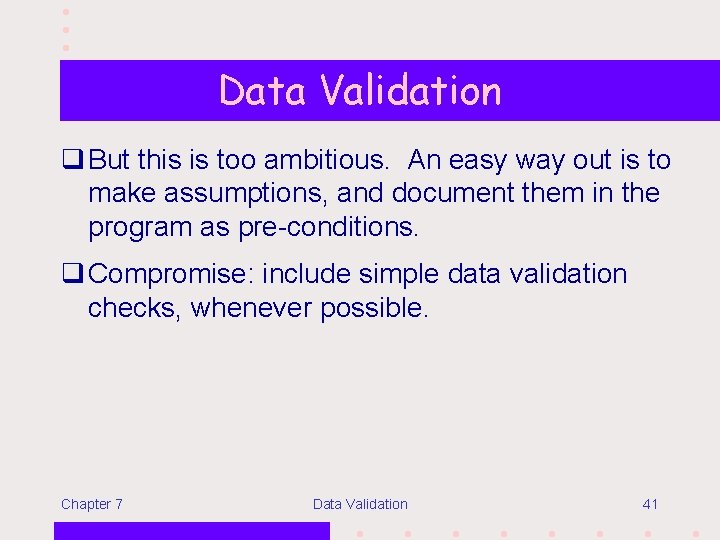
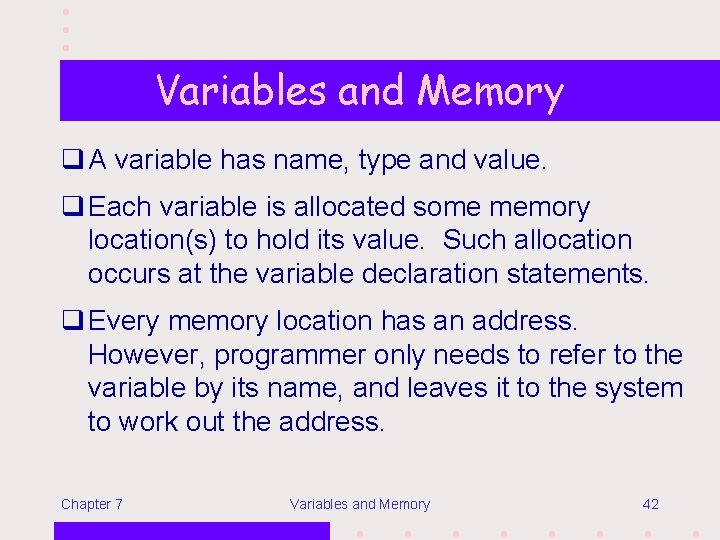
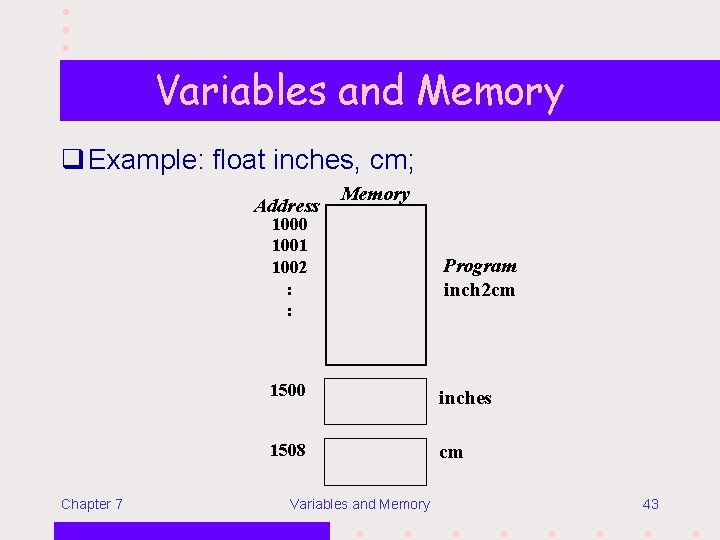
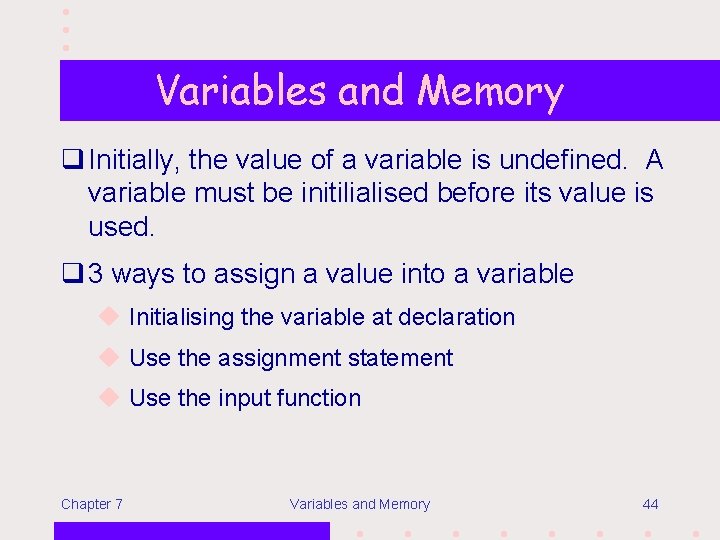
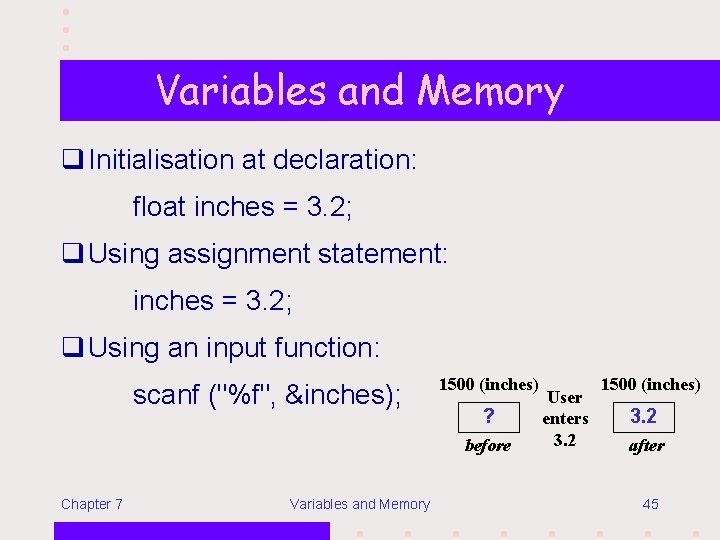
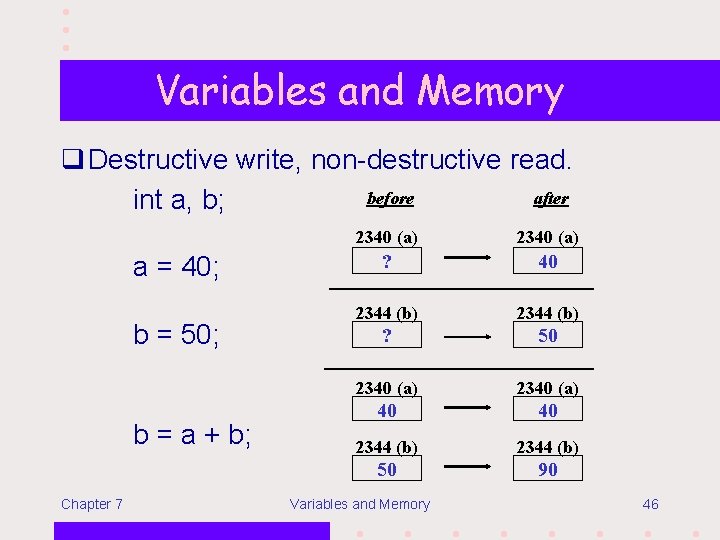
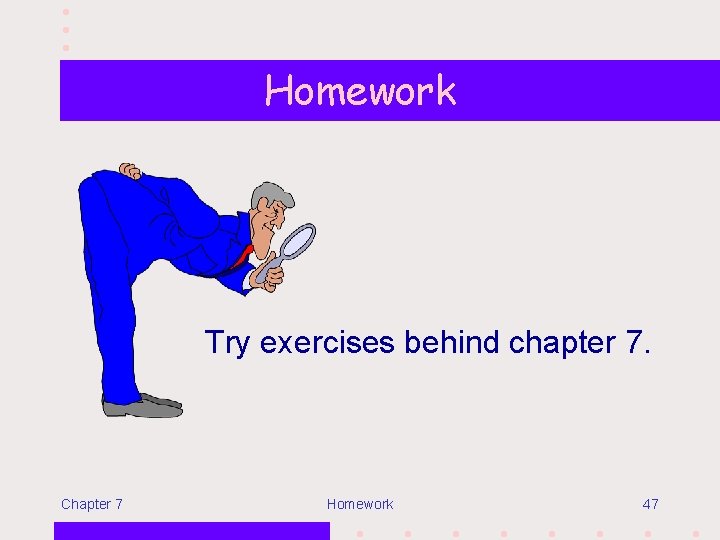
- Slides: 47
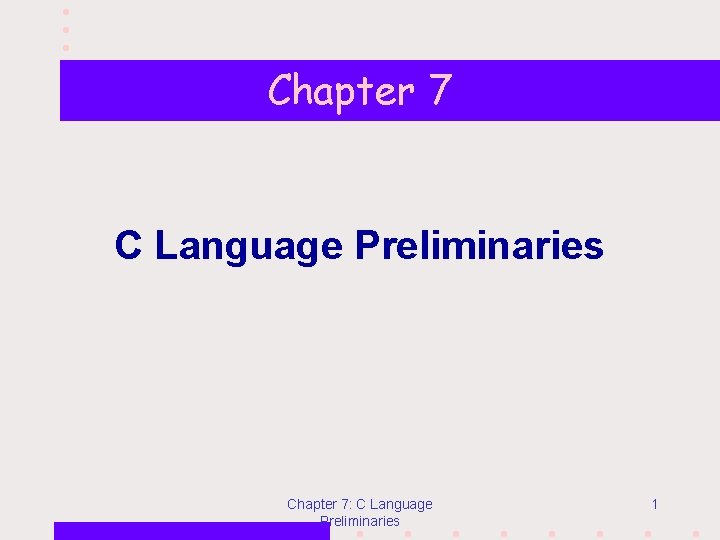
Chapter 7 C Language Preliminaries Chapter 7: C Language Preliminaries 1
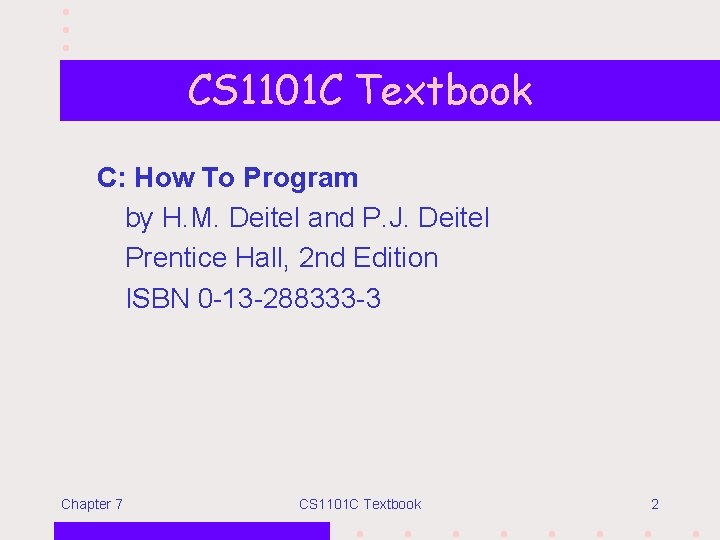
CS 1101 C Textbook C: How To Program by H. M. Deitel and P. J. Deitel Prentice Hall, 2 nd Edition ISBN 0 -13 -288333 -3 Chapter 7 CS 1101 C Textbook 2
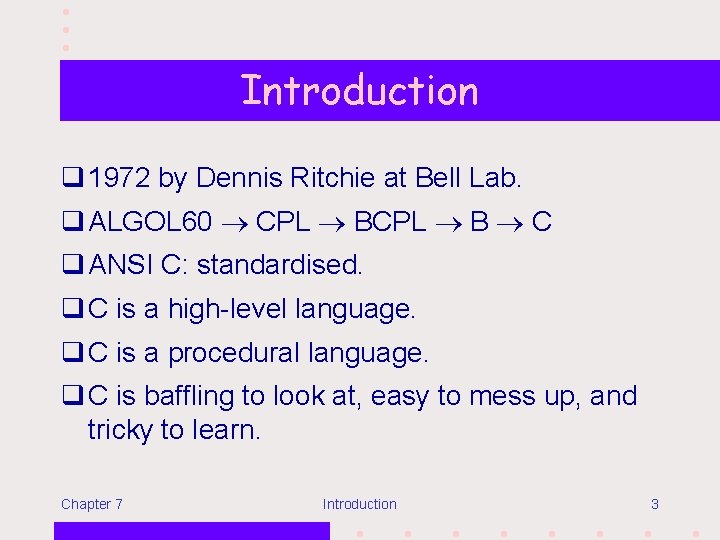
Introduction q 1972 by Dennis Ritchie at Bell Lab. q ALGOL 60 CPL B C q ANSI C: standardised. q C is a high-level language. q C is a procedural language. q C is baffling to look at, easy to mess up, and tricky to learn. Chapter 7 Introduction 3
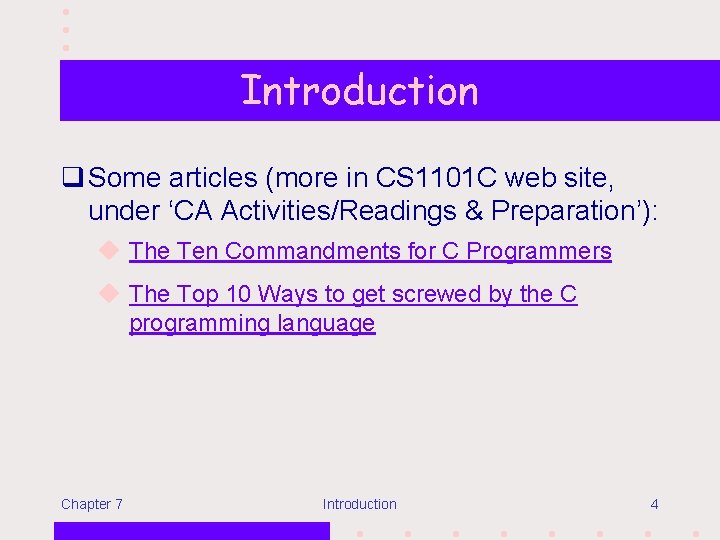
Introduction q Some articles (more in CS 1101 C web site, under ‘CA Activities/Readings & Preparation’): u The Ten Commandments for C Programmers u The Top 10 Ways to get screwed by the C programming language Chapter 7 Introduction 4
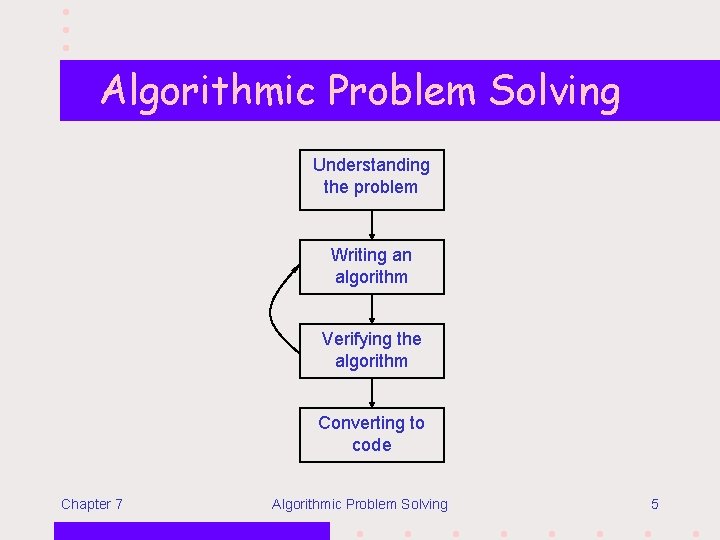
Algorithmic Problem Solving Understanding the problem Writing an algorithm Verifying the algorithm Converting to code Chapter 7 Algorithmic Problem Solving 5
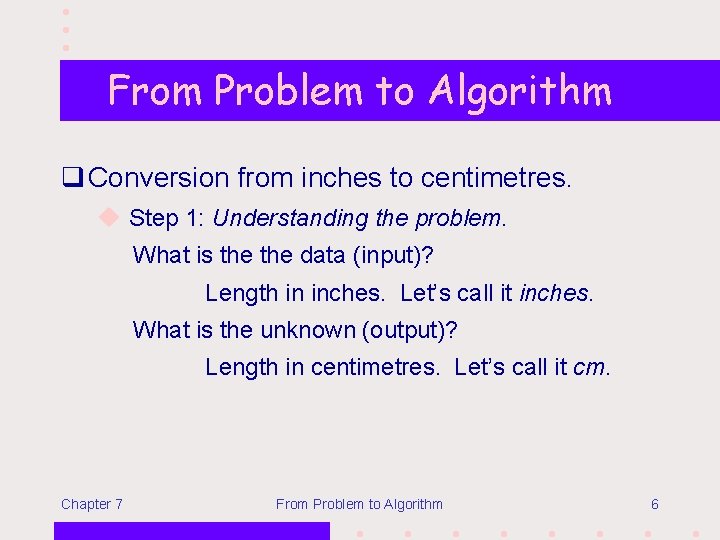
From Problem to Algorithm q Conversion from inches to centimetres. u Step 1: Understanding the problem. What is the data (input)? Length in inches. Let’s call it inches. What is the unknown (output)? Length in centimetres. Let’s call it cm. Chapter 7 From Problem to Algorithm 6
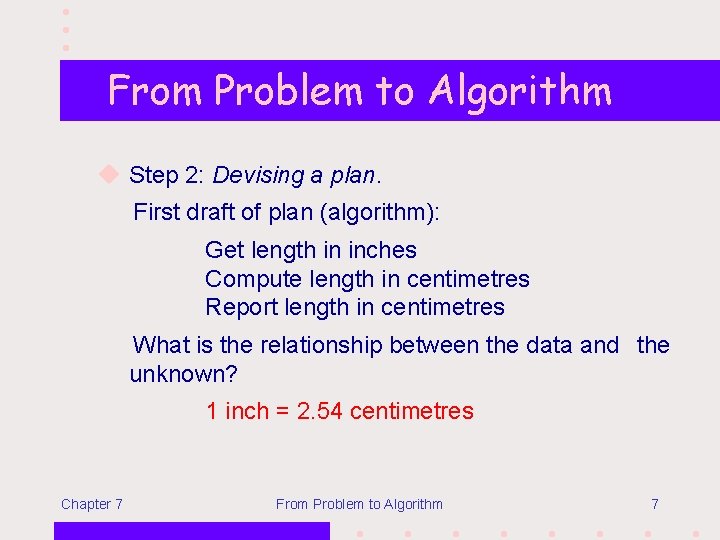
From Problem to Algorithm u Step 2: Devising a plan. First draft of plan (algorithm): Get length in inches Compute length in centimetres Report length in centimetres What is the relationship between the data and the unknown? 1 inch = 2. 54 centimetres Chapter 7 From Problem to Algorithm 7
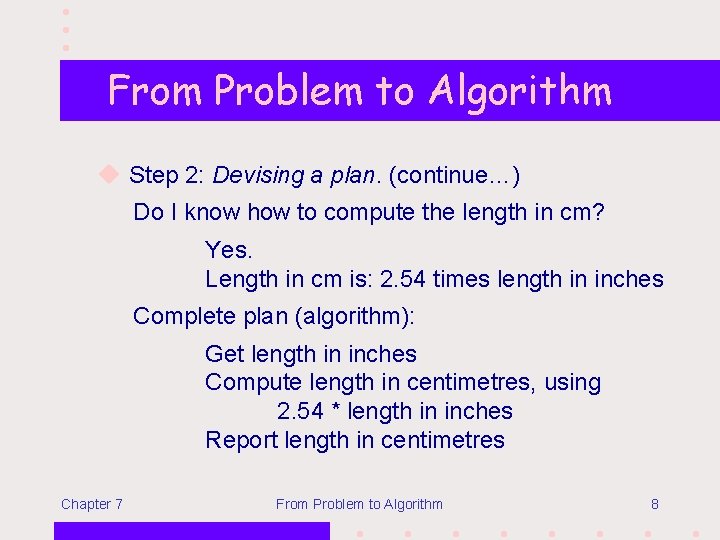
From Problem to Algorithm u Step 2: Devising a plan. (continue…) Do I know how to compute the length in cm? Yes. Length in cm is: 2. 54 times length in inches Complete plan (algorithm): Get length in inches Compute length in centimetres, using 2. 54 * length in inches Report length in centimetres Chapter 7 From Problem to Algorithm 8
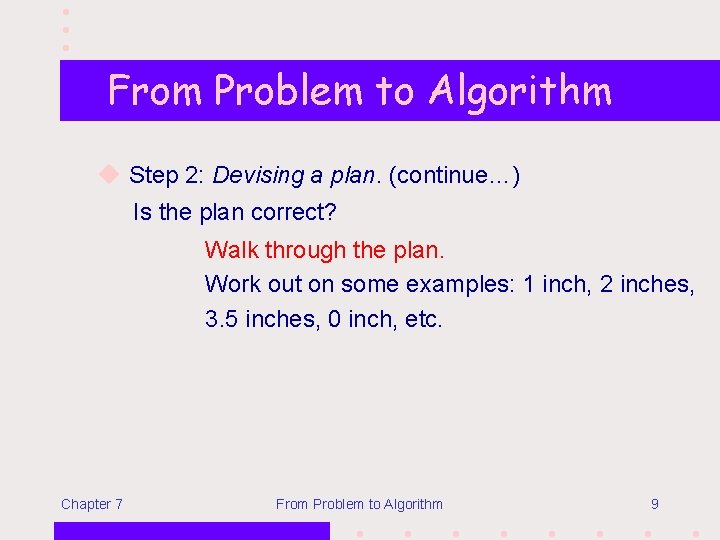
From Problem to Algorithm u Step 2: Devising a plan. (continue…) Is the plan correct? Walk through the plan. Work out on some examples: 1 inch, 2 inches, 3. 5 inches, 0 inch, etc. Chapter 7 From Problem to Algorithm 9
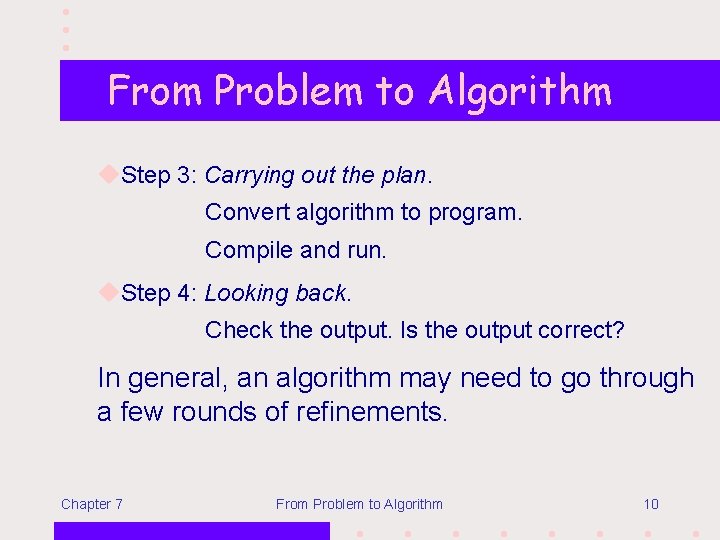
From Problem to Algorithm u. Step 3: Carrying out the plan. Convert algorithm to program. Compile and run. u. Step 4: Looking back. Check the output. Is the output correct? In general, an algorithm may need to go through a few rounds of refinements. Chapter 7 From Problem to Algorithm 10
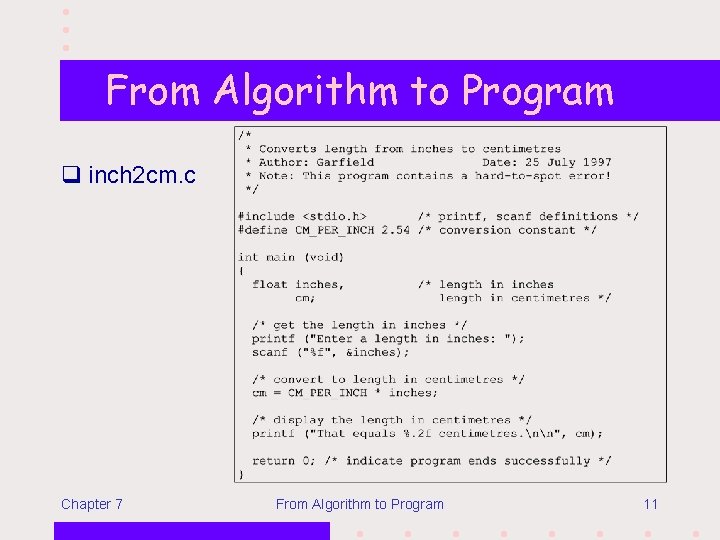
From Algorithm to Program q inch 2 cm. c Chapter 7 From Algorithm to Program 11
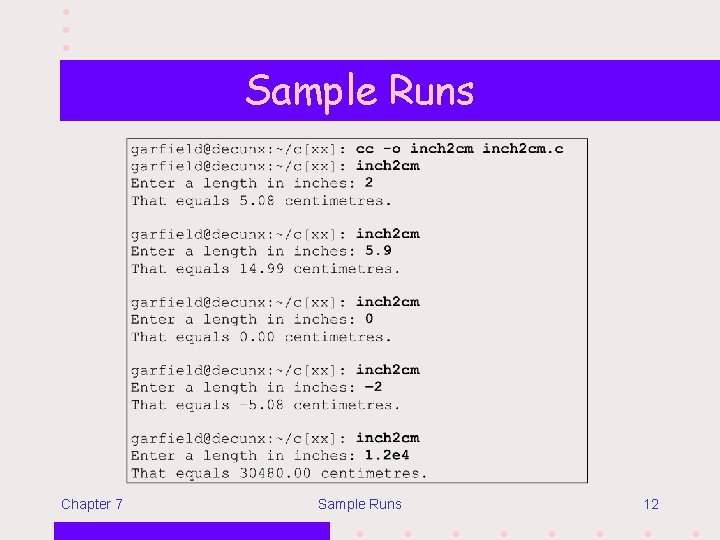
Sample Runs Chapter 7 Sample Runs 12
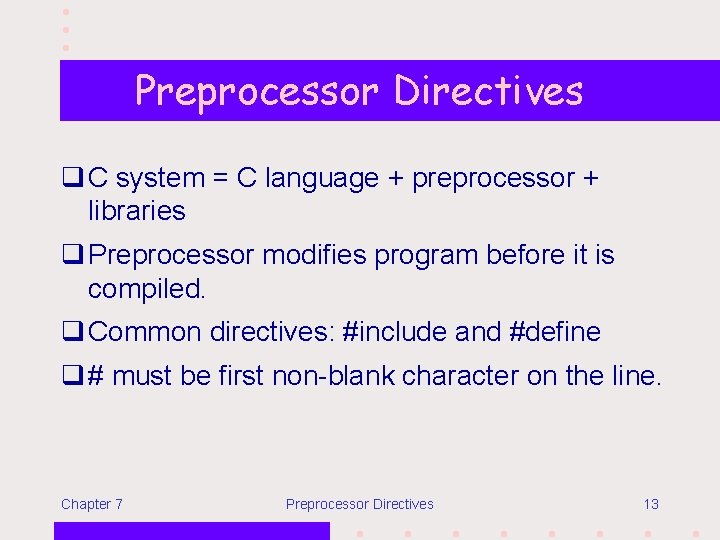
Preprocessor Directives q C system = C language + preprocessor + libraries q Preprocessor modifies program before it is compiled. q Common directives: #include and #define q # must be first non-blank character on the line. Chapter 7 Preprocessor Directives 13
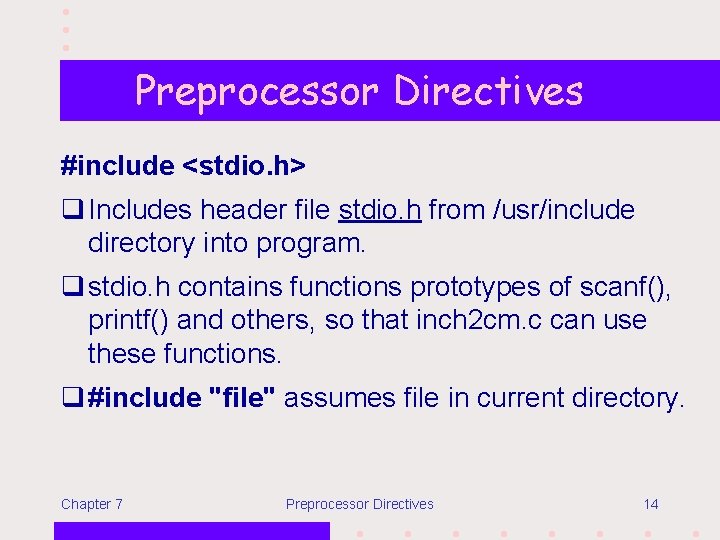
Preprocessor Directives #include <stdio. h> q Includes header file stdio. h from /usr/include directory into program. q stdio. h contains functions prototypes of scanf(), printf() and others, so that inch 2 cm. c can use these functions. q #include "file" assumes file in current directory. Chapter 7 Preprocessor Directives 14
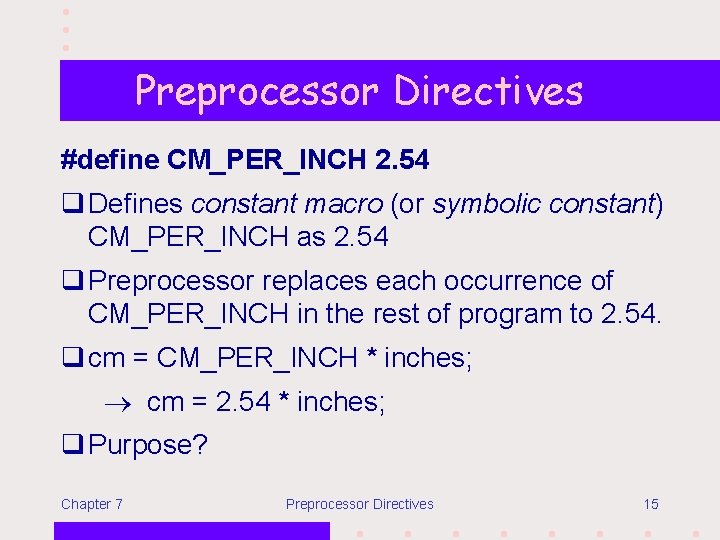
Preprocessor Directives #define CM_PER_INCH 2. 54 q Defines constant macro (or symbolic constant) CM_PER_INCH as 2. 54 q Preprocessor replaces each occurrence of CM_PER_INCH in the rest of program to 2. 54. q cm = CM_PER_INCH * inches; cm = 2. 54 * inches; q Purpose? Chapter 7 Preprocessor Directives 15
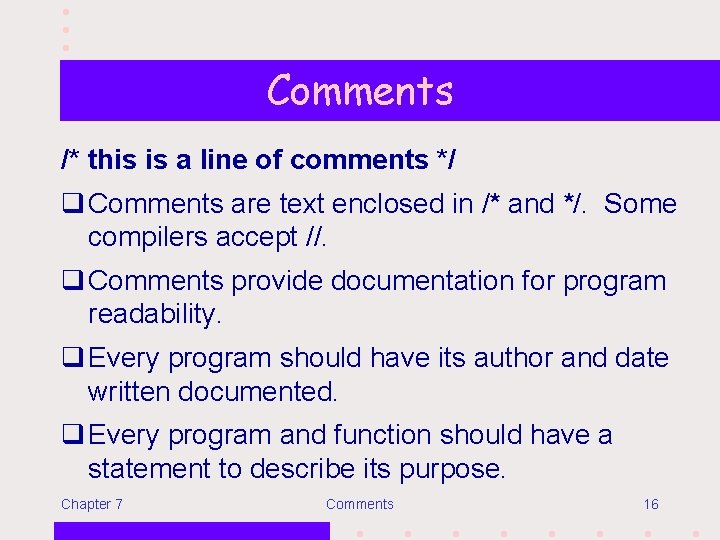
Comments /* this is a line of comments */ q Comments are text enclosed in /* and */. Some compilers accept //. q Comments provide documentation for program readability. q Every program should have its author and date written documented. q Every program and function should have a statement to describe its purpose. Chapter 7 Comments 16
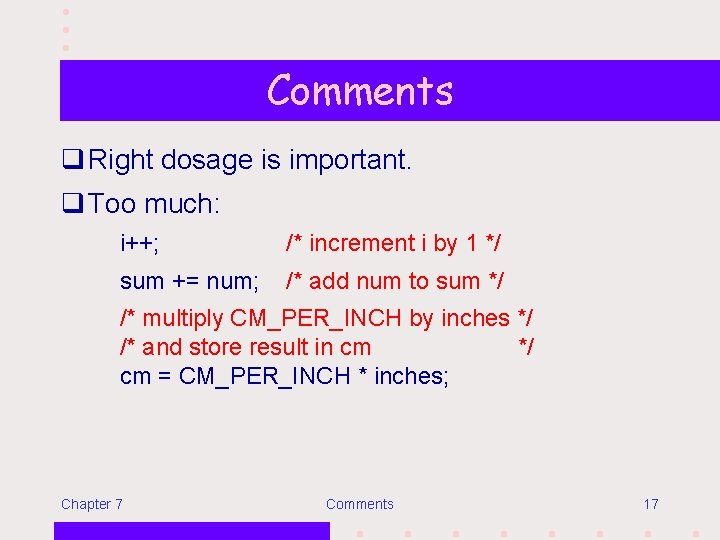
Comments q Right dosage is important. q Too much: i++; /* increment i by 1 */ sum += num; /* add num to sum */ /* multiply CM_PER_INCH by inches */ /* and store result in cm */ cm = CM_PER_INCH * inches; Chapter 7 Comments 17
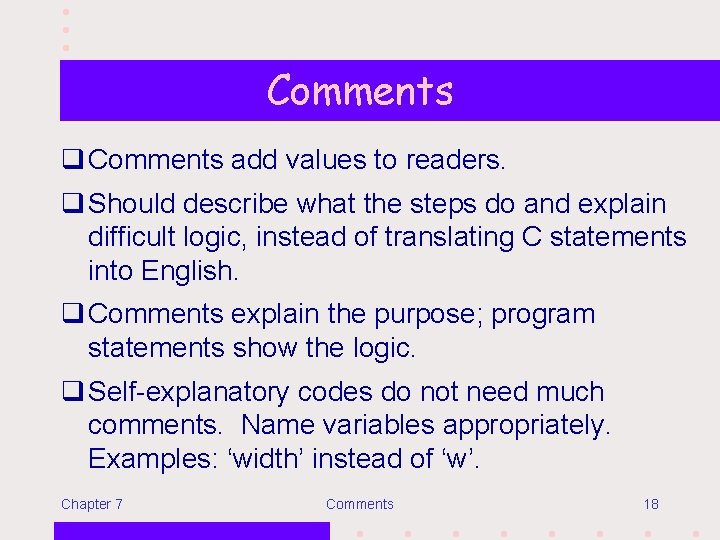
Comments q Comments add values to readers. q Should describe what the steps do and explain difficult logic, instead of translating C statements into English. q Comments explain the purpose; program statements show the logic. q Self-explanatory codes do not need much comments. Name variables appropriately. Examples: ‘width’ instead of ‘w’. Chapter 7 Comments 18
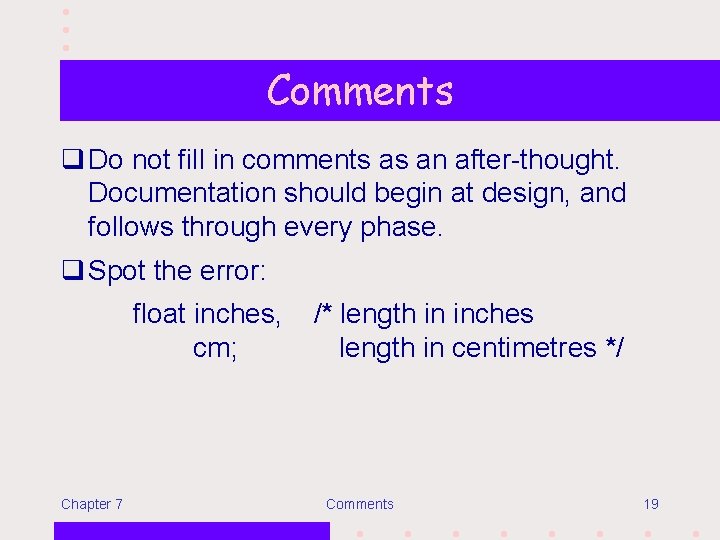
Comments q Do not fill in comments as an after-thought. Documentation should begin at design, and follows through every phase. q Spot the error: float inches, cm; Chapter 7 /* length in inches length in centimetres */ Comments 19
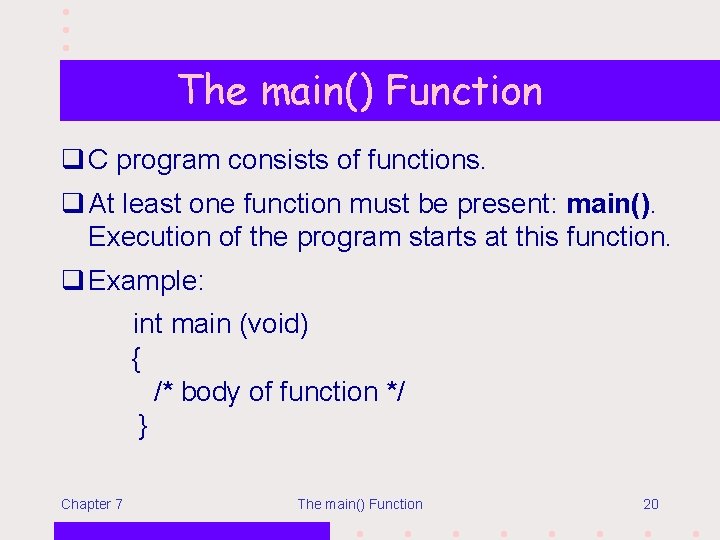
The main() Function q C program consists of functions. q At least one function must be present: main(). Execution of the program starts at this function. q Example: int main (void) { /* body of function */ } Chapter 7 The main() Function 20
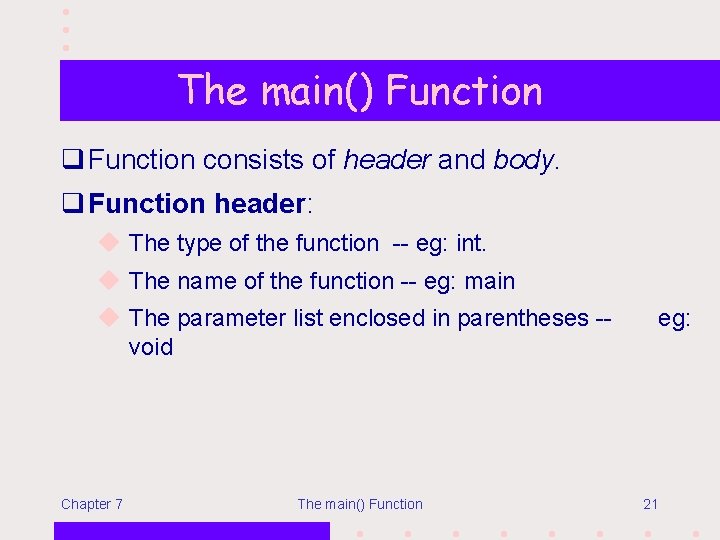
The main() Function q Function consists of header and body. q Function header: u The type of the function -- eg: int. u The name of the function -- eg: main u The parameter list enclosed in parentheses -void Chapter 7 The main() Function eg: 21
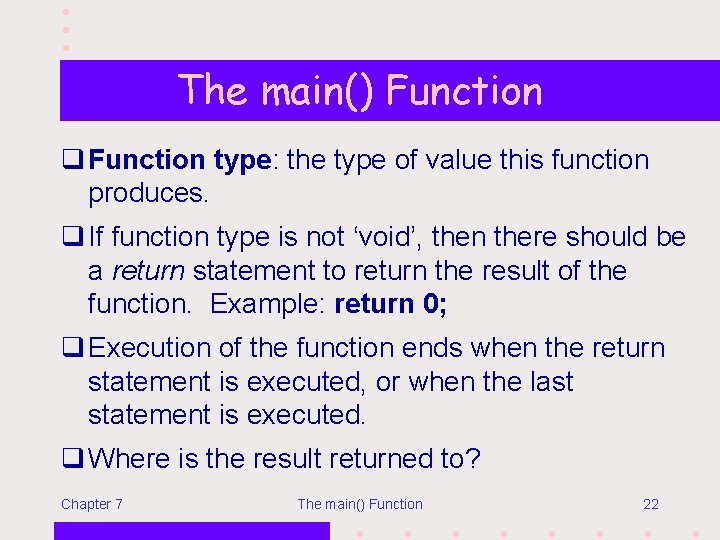
The main() Function q Function type: the type of value this function produces. q If function type is not ‘void’, then there should be a return statement to return the result of the function. Example: return 0; q Execution of the function ends when the return statement is executed, or when the last statement is executed. q Where is the result returned to? Chapter 7 The main() Function 22
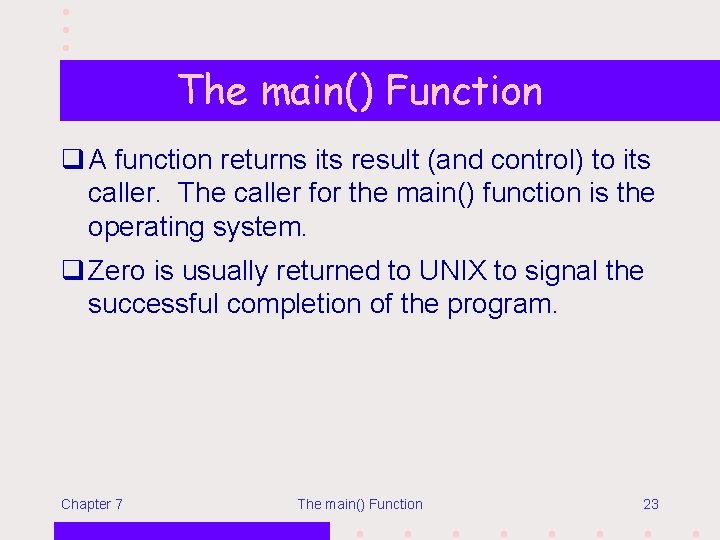
The main() Function q A function returns its result (and control) to its caller. The caller for the main() function is the operating system. q Zero is usually returned to UNIX to signal the successful completion of the program. Chapter 7 The main() Function 23
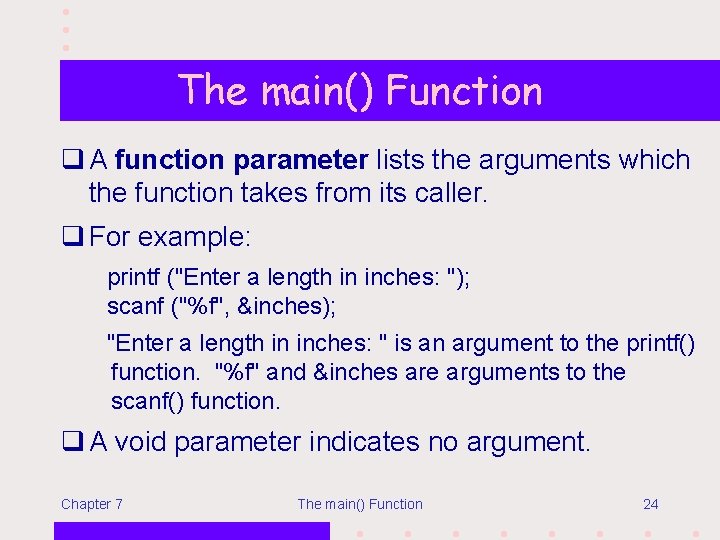
The main() Function q A function parameter lists the arguments which the function takes from its caller. q For example: printf ("Enter a length in inches: "); scanf ("%f", &inches); "Enter a length in inches: " is an argument to the printf() function. "%f" and &inches are arguments to the scanf() function. q A void parameter indicates no argument. Chapter 7 The main() Function 24
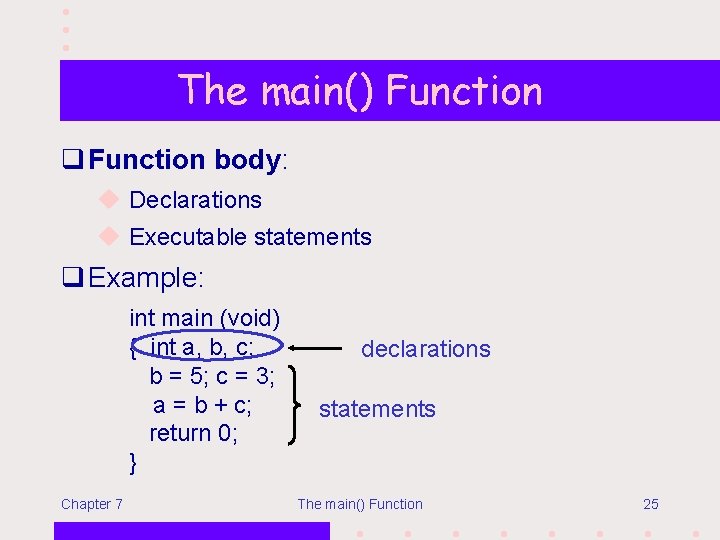
The main() Function q Function body: u Declarations u Executable statements q Example: int main (void) { int a, b, c; b = 5; c = 3; a = b + c; return 0; } Chapter 7 declarations statements The main() Function 25
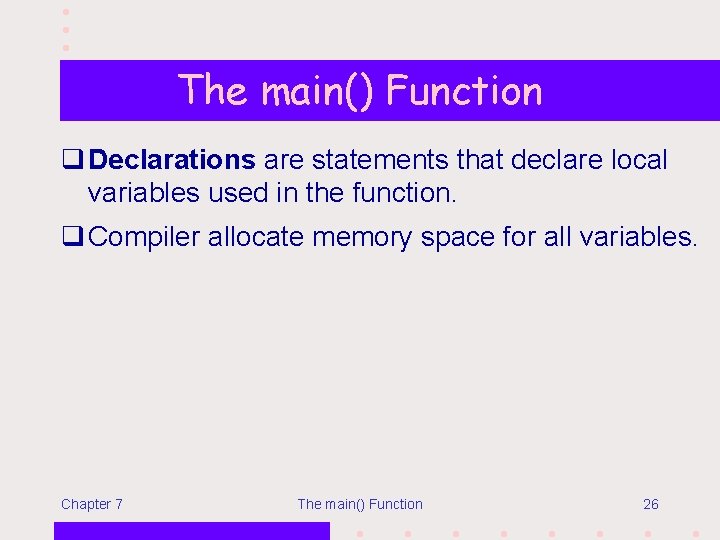
The main() Function q Declarations are statements that declare local variables used in the function. q Compiler allocate memory space for all variables. Chapter 7 The main() Function 26
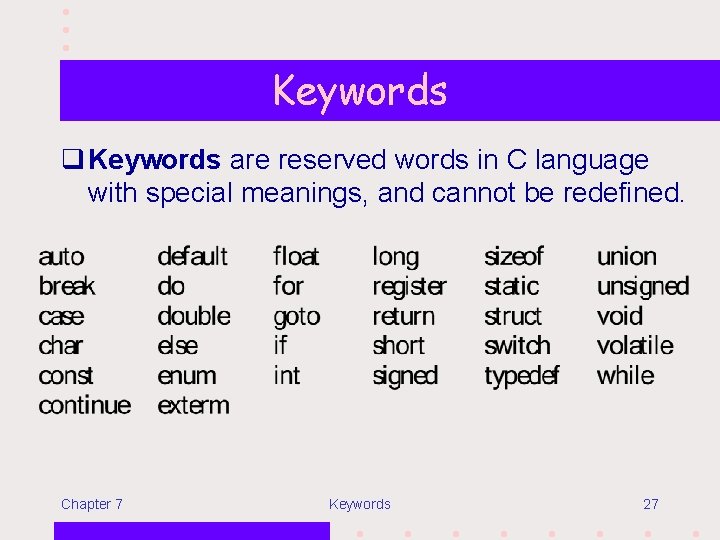
Keywords q Keywords are reserved words in C language with special meanings, and cannot be redefined. Chapter 7 Keywords 27
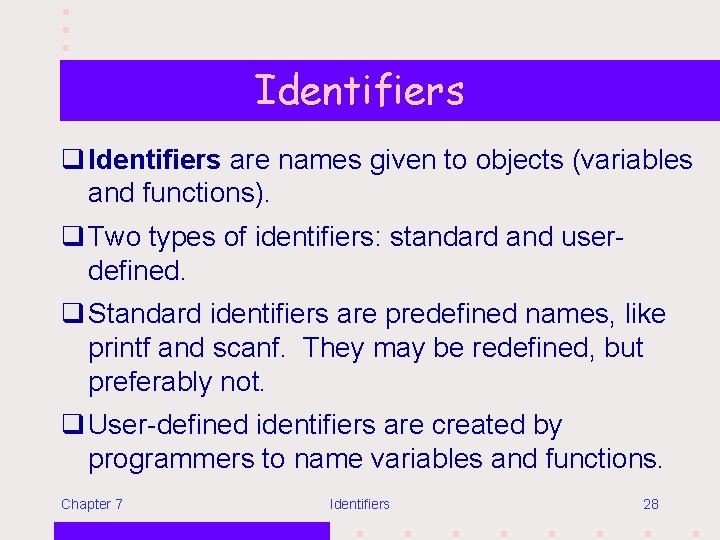
Identifiers q Identifiers are names given to objects (variables and functions). q Two types of identifiers: standard and userdefined. q Standard identifiers are predefined names, like printf and scanf. They may be redefined, but preferably not. q User-defined identifiers are created by programmers to name variables and functions. Chapter 7 Identifiers 28
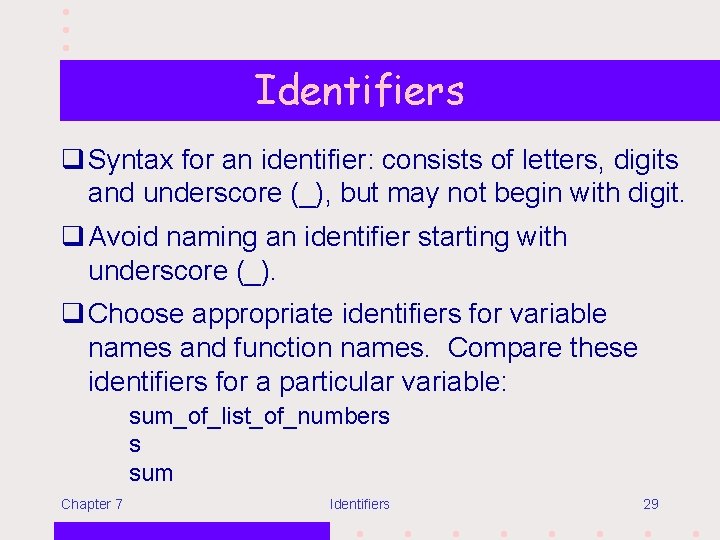
Identifiers q Syntax for an identifier: consists of letters, digits and underscore (_), but may not begin with digit. q Avoid naming an identifier starting with underscore (_). q Choose appropriate identifiers for variable names and function names. Compare these identifiers for a particular variable: sum_of_list_of_numbers s sum Chapter 7 Identifiers 29
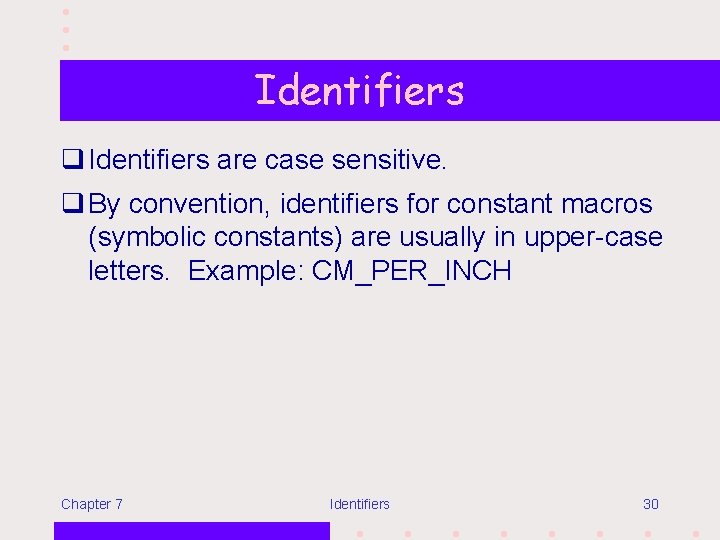
Identifiers q Identifiers are case sensitive. q By convention, identifiers for constant macros (symbolic constants) are usually in upper-case letters. Example: CM_PER_INCH Chapter 7 Identifiers 30
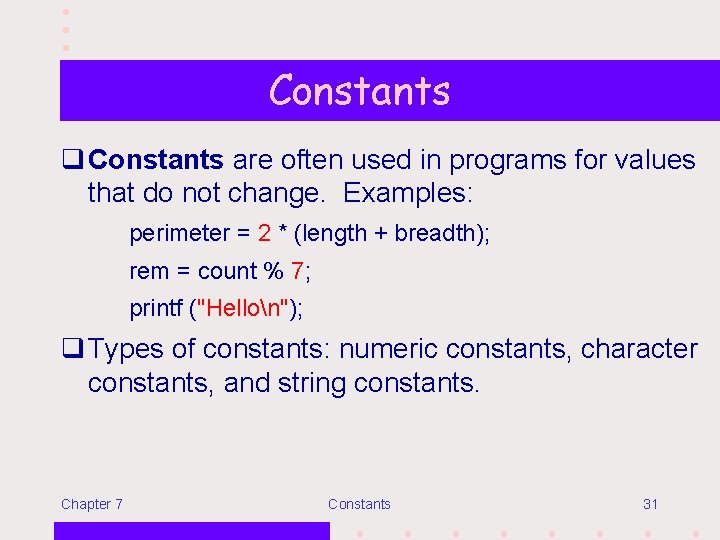
Constants q Constants are often used in programs for values that do not change. Examples: perimeter = 2 * (length + breadth); rem = count % 7; printf ("Hellon"); q Types of constants: numeric constants, character constants, and string constants. Chapter 7 Constants 31
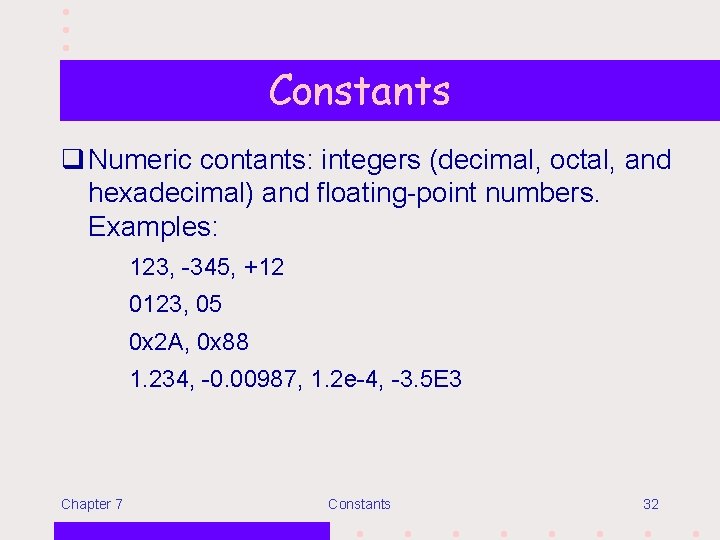
Constants q Numeric contants: integers (decimal, octal, and hexadecimal) and floating-point numbers. Examples: 123, -345, +12 0123, 05 0 x 2 A, 0 x 88 1. 234, -0. 00987, 1. 2 e-4, -3. 5 E 3 Chapter 7 Constants 32
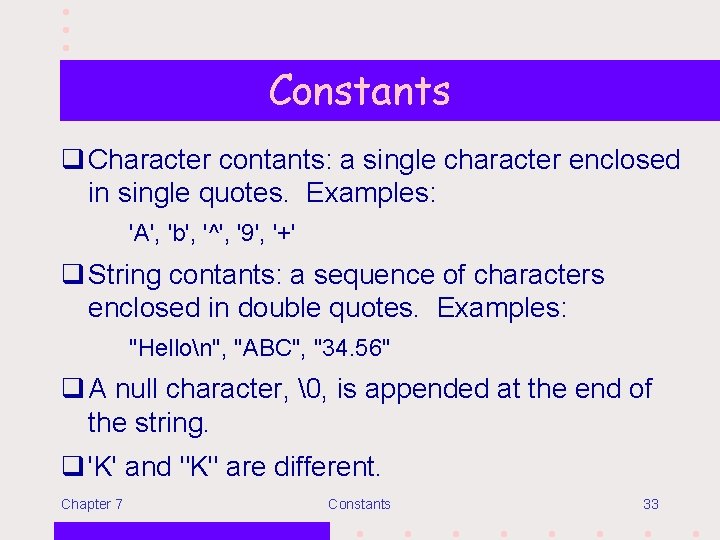
Constants q Character contants: a single character enclosed in single quotes. Examples: 'A', 'b', '^', '9', '+' q String contants: a sequence of characters enclosed in double quotes. Examples: "Hellon", "ABC", "34. 56" q A null character, , is appended at the end of the string. q 'K' and "K" are different. Chapter 7 Constants 33
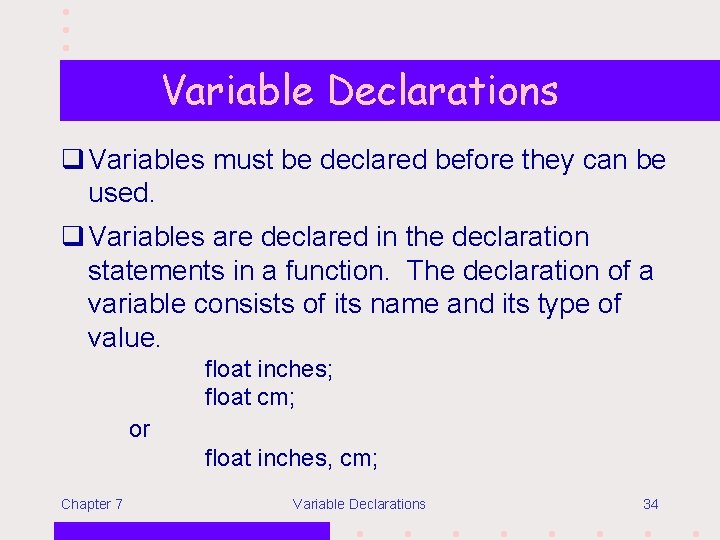
Variable Declarations q Variables must be declared before they can be used. q Variables are declared in the declaration statements in a function. The declaration of a variable consists of its name and its type of value. float inches; float cm; or float inches, cm; Chapter 7 Variable Declarations 34
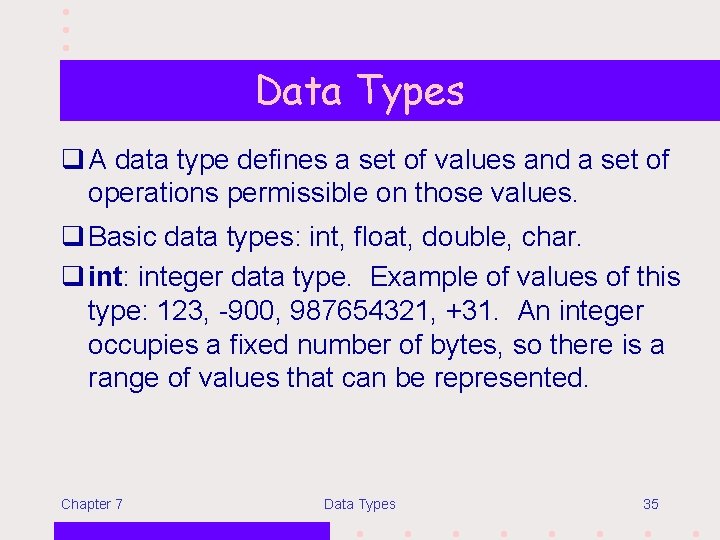
Data Types q A data type defines a set of values and a set of operations permissible on those values. q Basic data types: int, float, double, char. q int: integer data type. Example of values of this type: 123, -900, 987654321, +31. An integer occupies a fixed number of bytes, so there is a range of values that can be represented. Chapter 7 Data Types 35
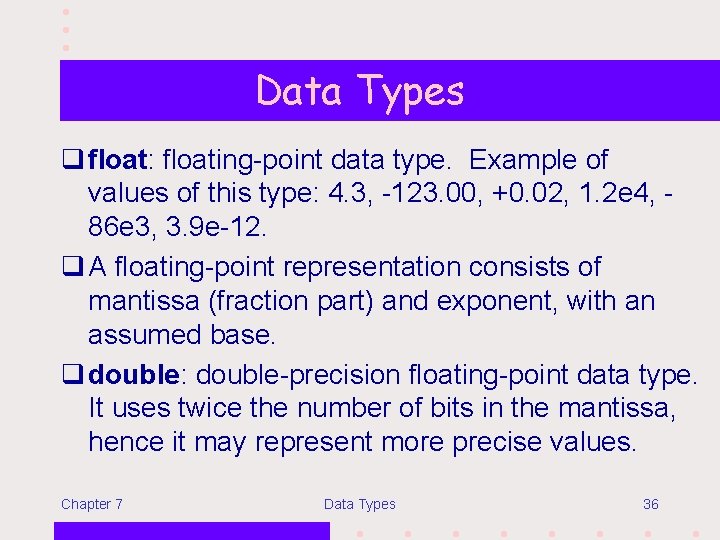
Data Types q float: floating-point data type. Example of values of this type: 4. 3, -123. 00, +0. 02, 1. 2 e 4, 86 e 3, 3. 9 e-12. q A floating-point representation consists of mantissa (fraction part) and exponent, with an assumed base. q double: double-precision floating-point data type. It uses twice the number of bits in the mantissa, hence it may represent more precise values. Chapter 7 Data Types 36
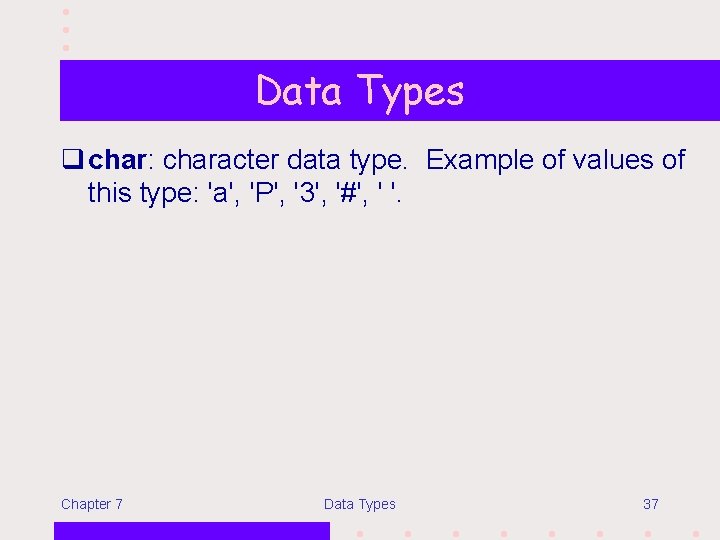
Data Types q char: character data type. Example of values of this type: 'a', 'P', '3', '#', ' '. Chapter 7 Data Types 37
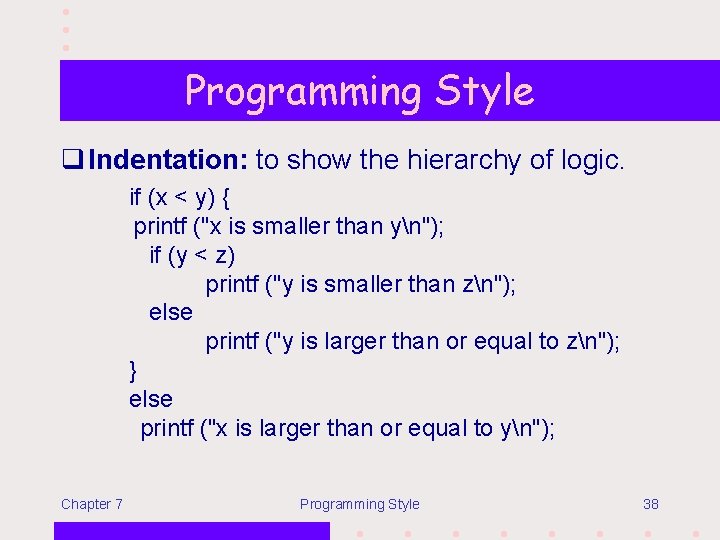
Programming Style q Indentation: to show the hierarchy of logic. if (x < y) { printf ("x is smaller than yn"); if (y < z) printf ("y is smaller than zn"); else printf ("y is larger than or equal to zn"); } else printf ("x is larger than or equal to yn"); Chapter 7 Programming Style 38
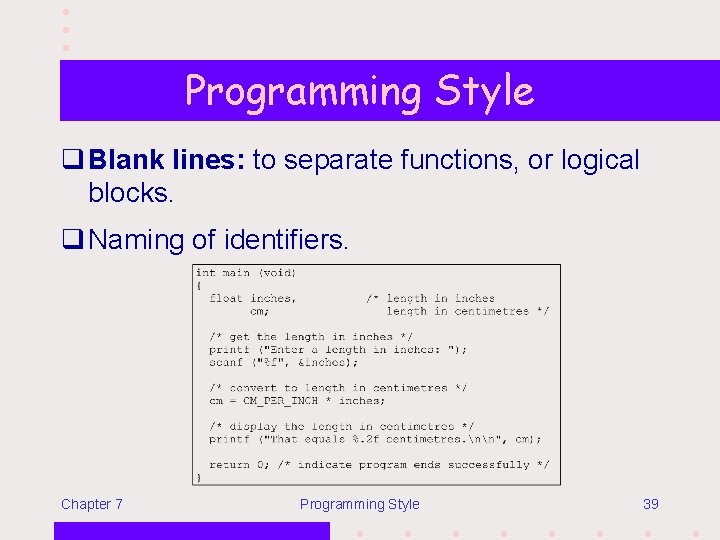
Programming Style q Blank lines: to separate functions, or logical blocks. q Naming of identifiers. Chapter 7 Programming Style 39
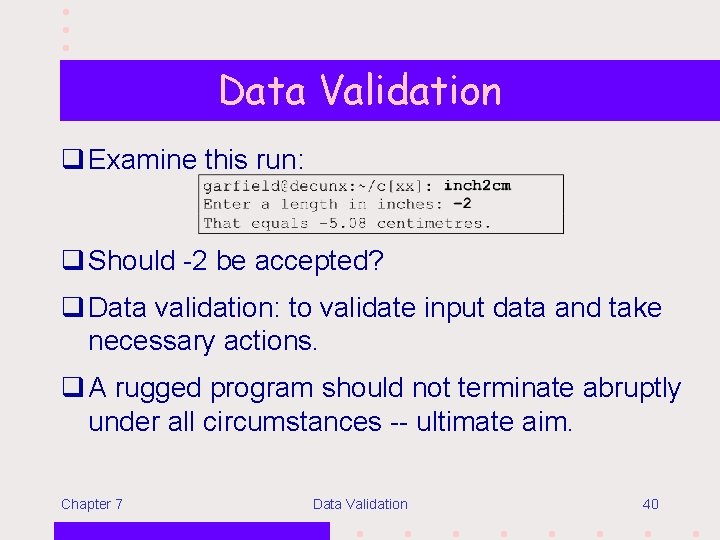
Data Validation q Examine this run: q Should -2 be accepted? q Data validation: to validate input data and take necessary actions. q A rugged program should not terminate abruptly under all circumstances -- ultimate aim. Chapter 7 Data Validation 40
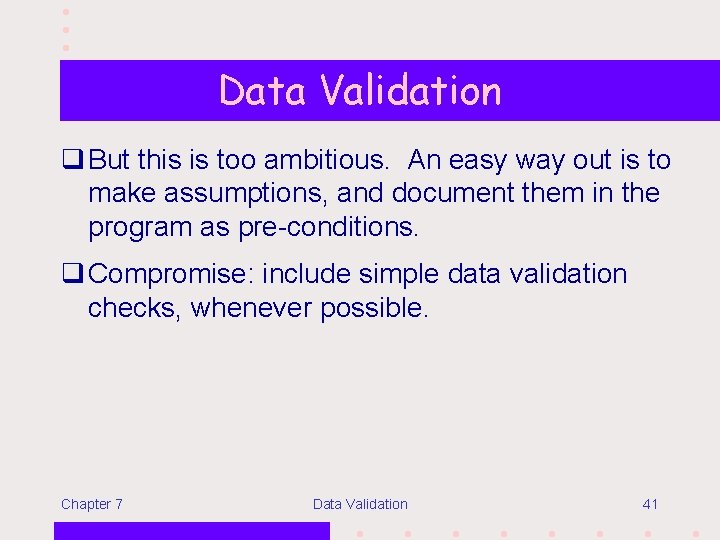
Data Validation q But this is too ambitious. An easy way out is to make assumptions, and document them in the program as pre-conditions. q Compromise: include simple data validation checks, whenever possible. Chapter 7 Data Validation 41
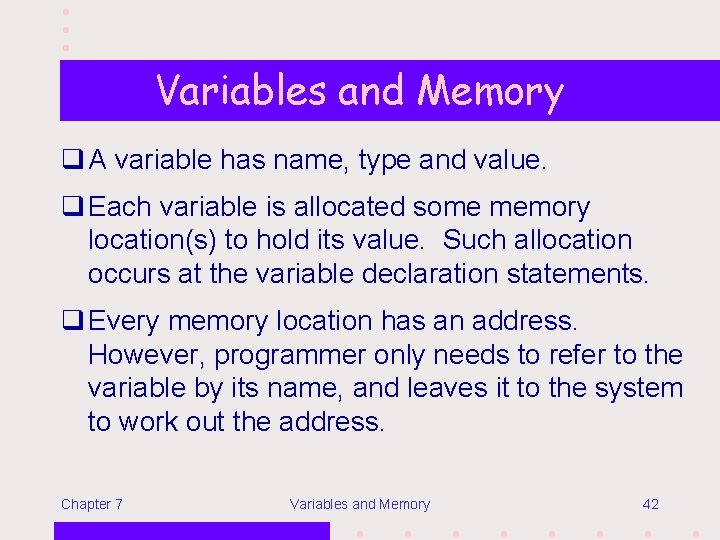
Variables and Memory q A variable has name, type and value. q Each variable is allocated some memory location(s) to hold its value. Such allocation occurs at the variable declaration statements. q Every memory location has an address. However, programmer only needs to refer to the variable by its name, and leaves it to the system to work out the address. Chapter 7 Variables and Memory 42
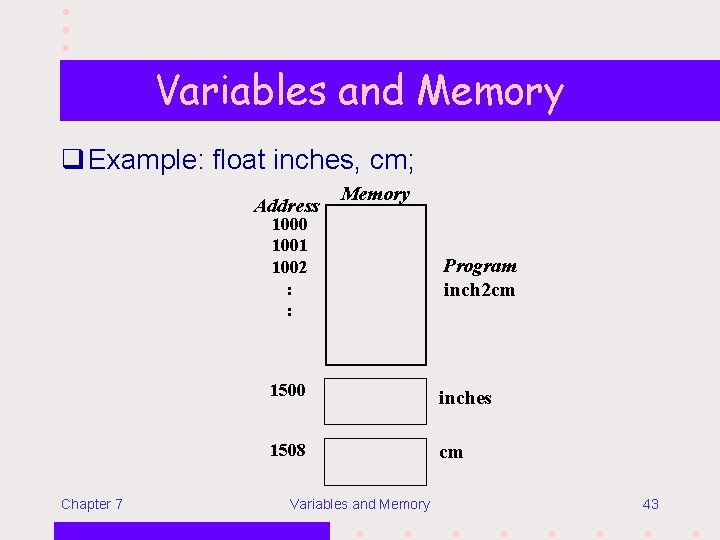
Variables and Memory q Example: float inches, cm; Address Memory 1000 1001 1002 : : Chapter 7 Program inch 2 cm 1500 inches 1508 cm Variables and Memory 43
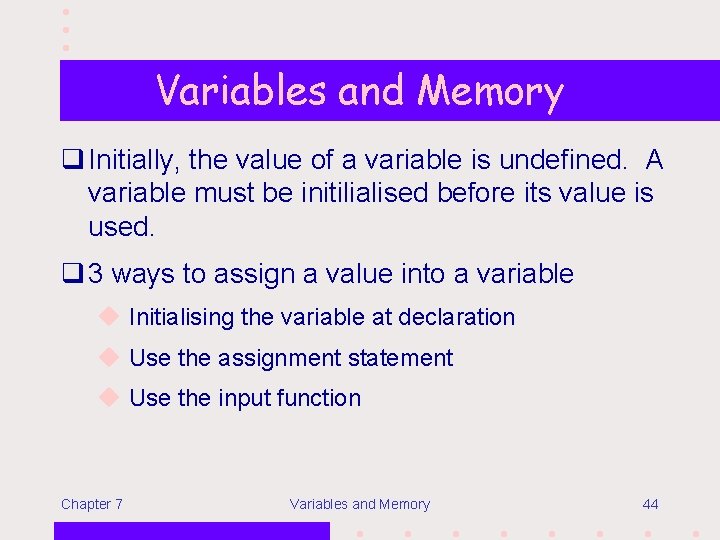
Variables and Memory q Initially, the value of a variable is undefined. A variable must be initilialised before its value is used. q 3 ways to assign a value into a variable u Initialising the variable at declaration u Use the assignment statement u Use the input function Chapter 7 Variables and Memory 44
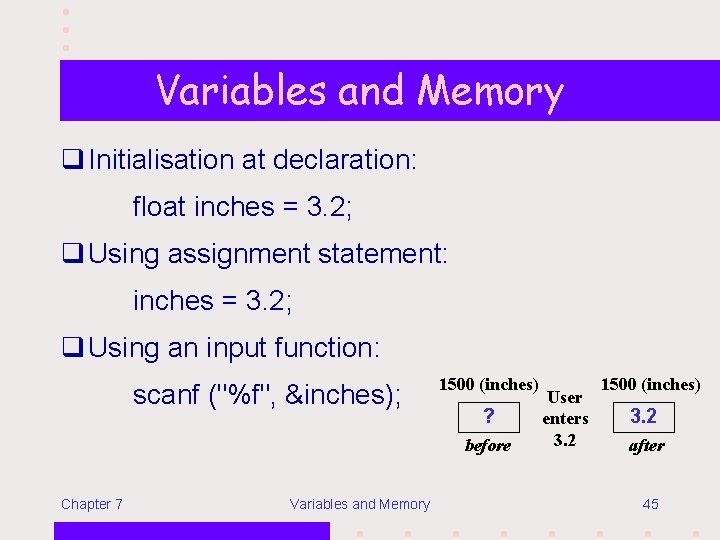
Variables and Memory q Initialisation at declaration: float inches = 3. 2; q Using assignment statement: inches = 3. 2; q Using an input function: scanf ("%f", &inches); 1500 (inches) ? before Chapter 7 Variables and Memory User enters 3. 2 1500 (inches) 3. 2 after 45
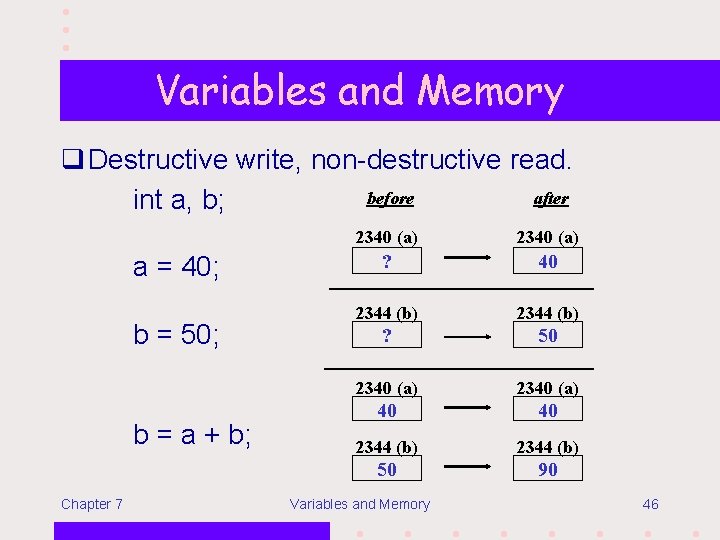
Variables and Memory q Destructive write, non-destructive read. before after int a, b; a = 40; b = 50; b = a + b; Chapter 7 2340 (a) ? 40 2344 (b) ? 50 2340 (a) 40 40 2344 (b) 50 90 Variables and Memory 46
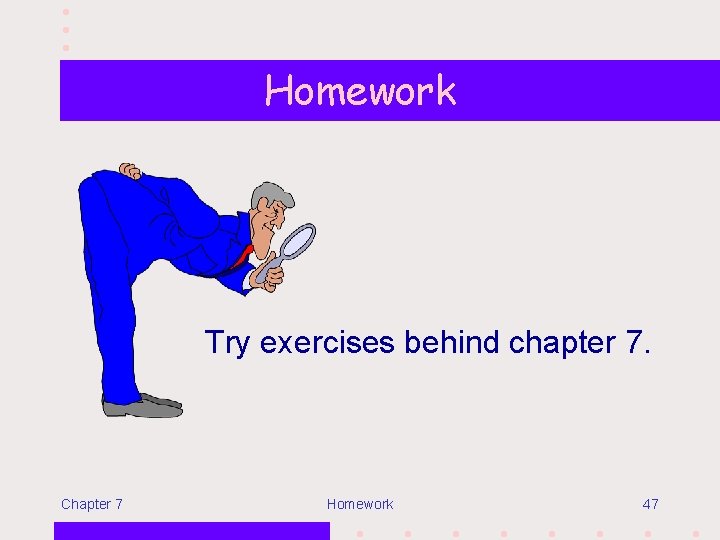
Homework Try exercises behind chapter 7. Chapter 7 Homework 47
Chapter 1 mathematical preliminaries
Apa itu preliminaries
Preliminary materials
Halaman preliminaries
Mathematical preliminaries in numerical computing
Contributes synoynm
Monitor model
Difference between second language and foreign language
Interpreted language vs compiled language
What is a standard language
Low-level programming language
Hdl language
Grant always turns in his homework
Difference between assembly language and machine language
Difference between assembly language and machine language
Assembly language consists of
Hyperbole about trees
What is formal language
Corso di laurea in scienze della formazione primaria
Is prolog declarative language
Prolog is a declarative language.
Literal language vs figurative language
13)turing machine is language recognizer of language.
Difference of first language and second language
Language
Literal language
A level english language language change
Absolute implicational universal
Formal vs informal language
Figurative or literal language
Sapir whorf theory
Reactive language vs proactive language
What is the difference between idiom and hyperbole
Is figurative language a language feature
Communicative signals and informative signals
Social language vs academic language
Habit 1: be proactive examples
Proactive language vs reactive language
Similes in chapter 1 of mice and men
Night chapter 4 literary devices
Figurative language hatchet
Chapter 17 hatchet
Chapter 8 thinking language and intelligence
Chapter 7 cognition thinking intelligence and language
Logical programming language
Chapter 16 programming language
Language of composition chapter 3
Metaphors in hatchet chapters 5-6