ACM Programming Competition Sorting Algorithms ACM Programming Competition
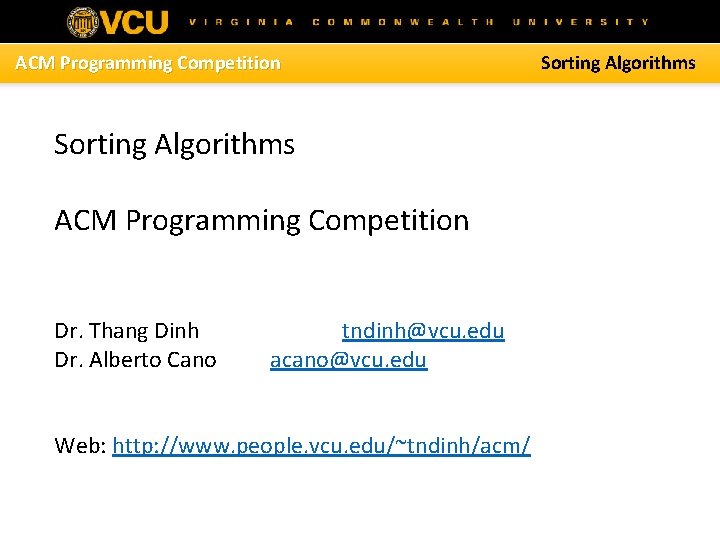
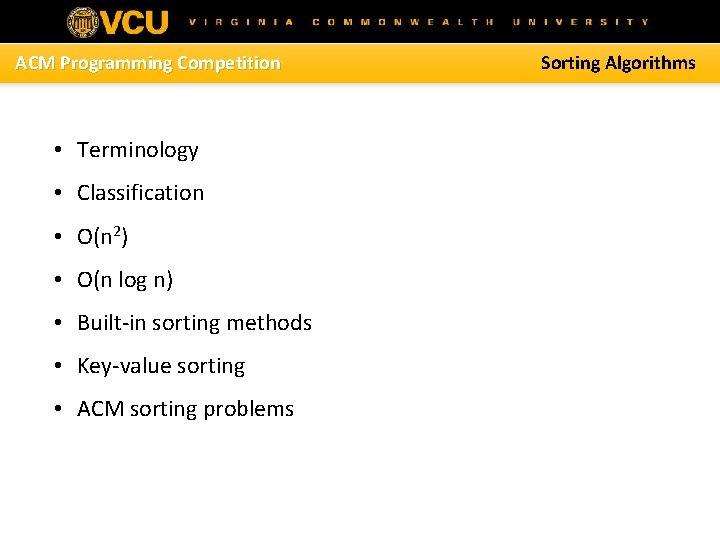
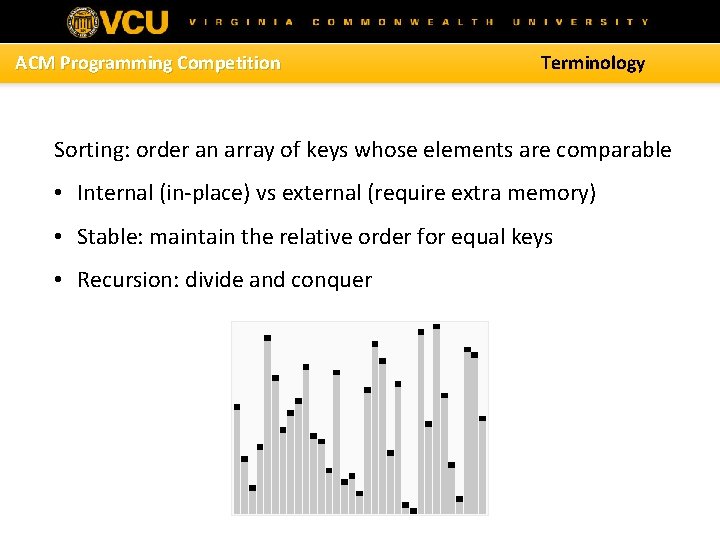
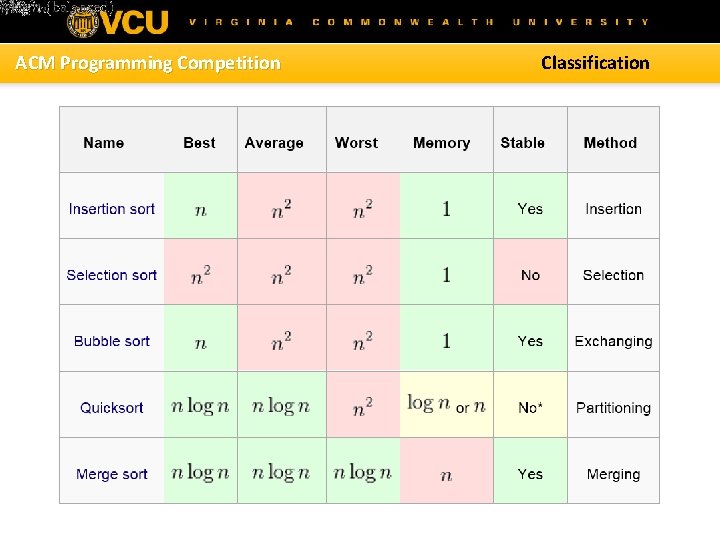
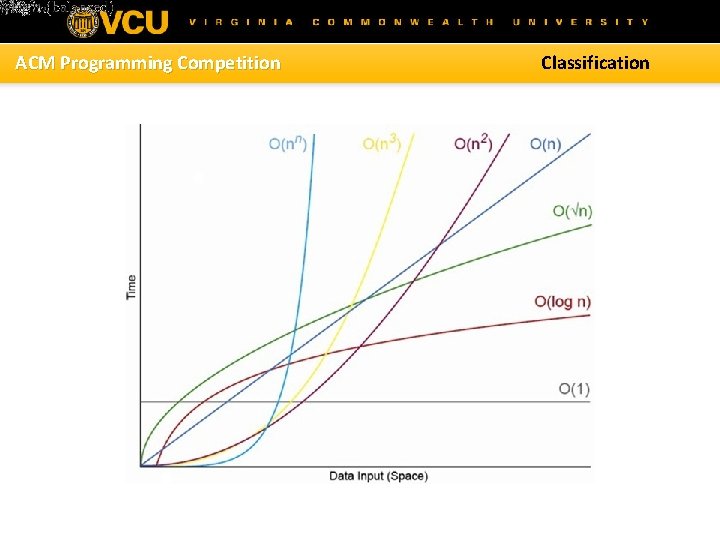
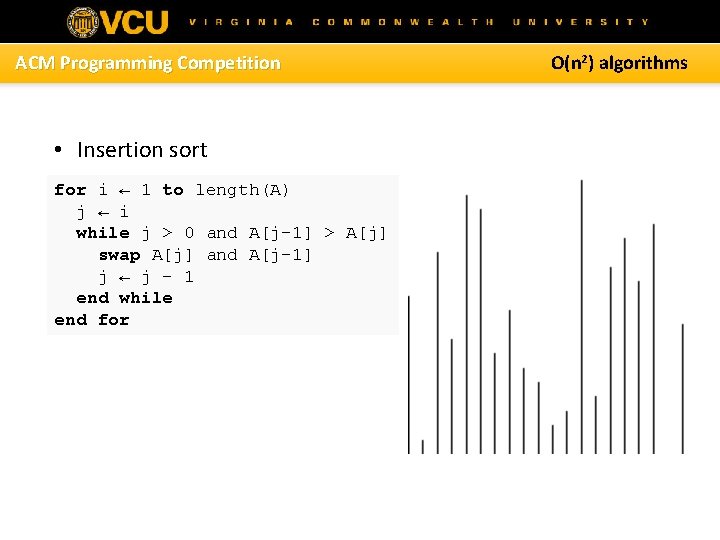
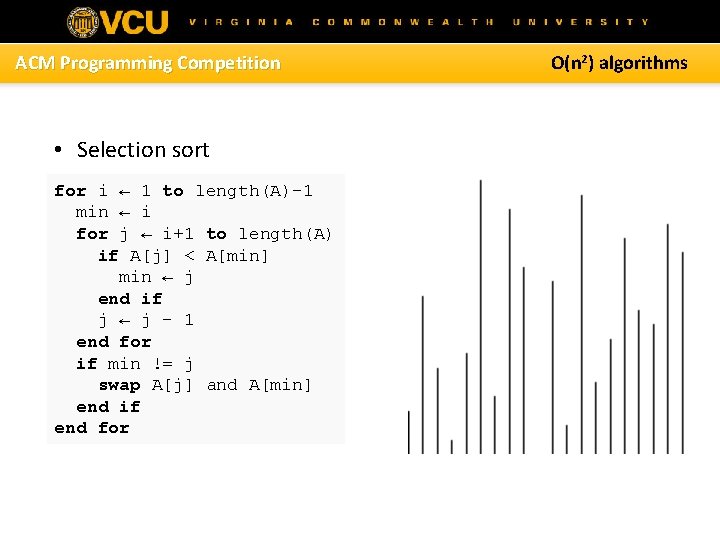
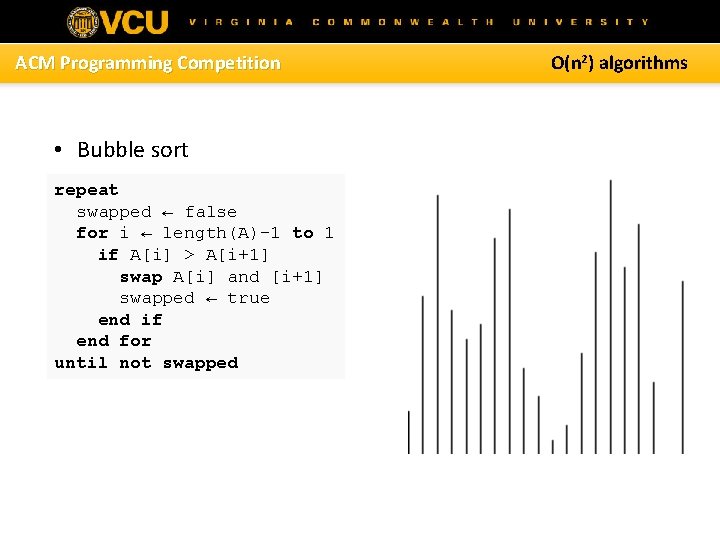
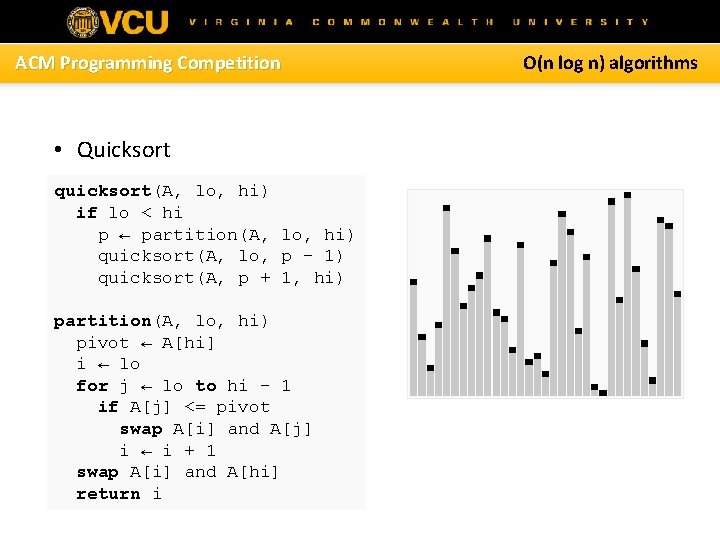
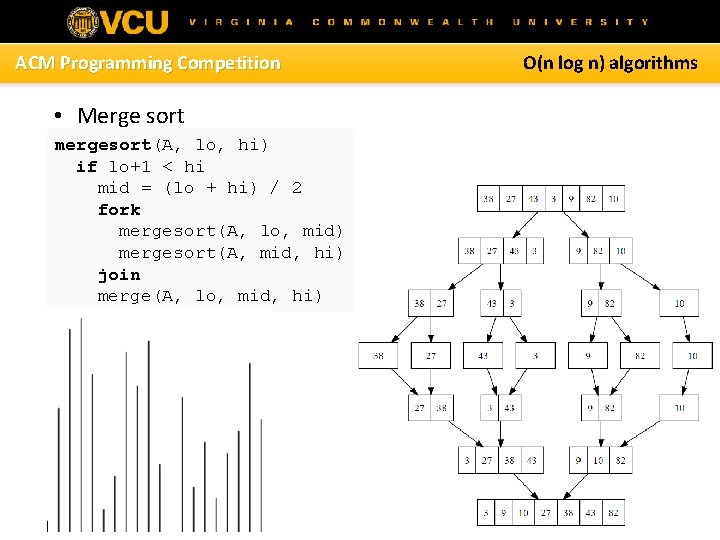
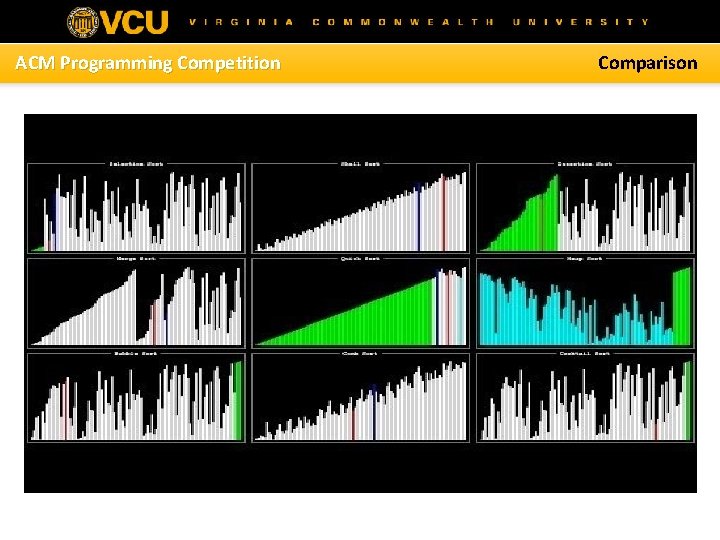
![ACM Programming Competition Built-in sorting methods • Arrays. sort(T[] A) Quicksort • Arrays. parallel. ACM Programming Competition Built-in sorting methods • Arrays. sort(T[] A) Quicksort • Arrays. parallel.](https://slidetodoc.com/presentation_image_h/d0d3b023937acf644b7124a7408f69ba/image-12.jpg)
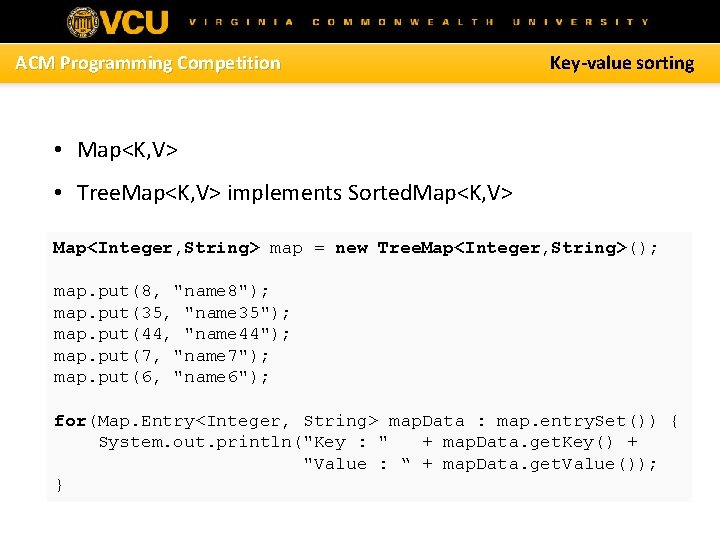
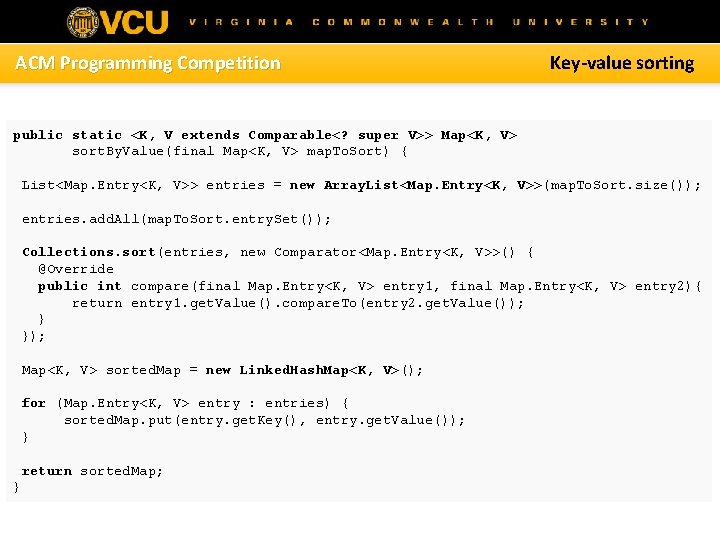
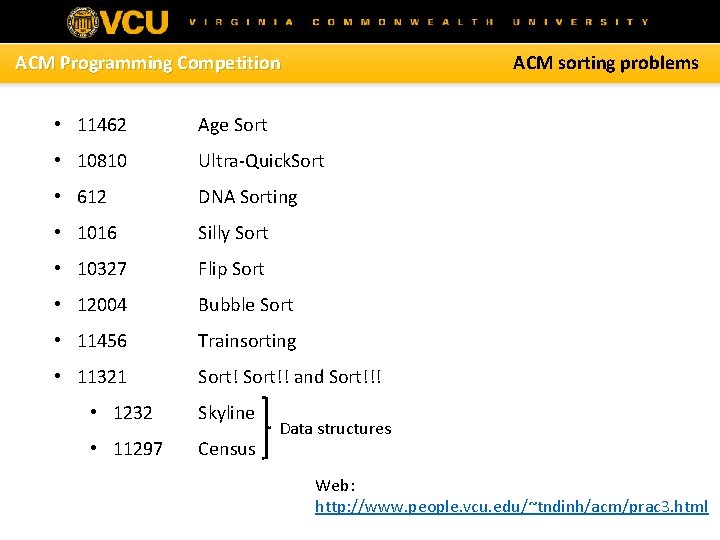
- Slides: 15
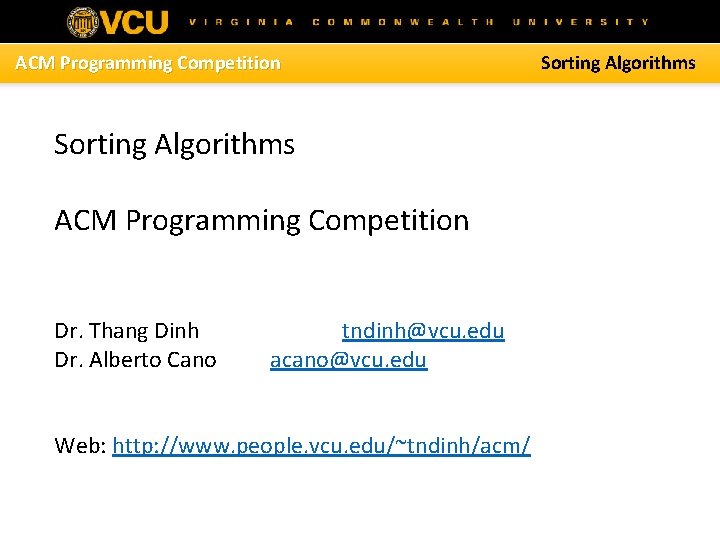
ACM Programming Competition Sorting Algorithms ACM Programming Competition Dr. Thang Dinh Dr. Alberto Cano tndinh@vcu. edu acano@vcu. edu Web: http: //www. people. vcu. edu/~tndinh/acm/ Sorting Algorithms
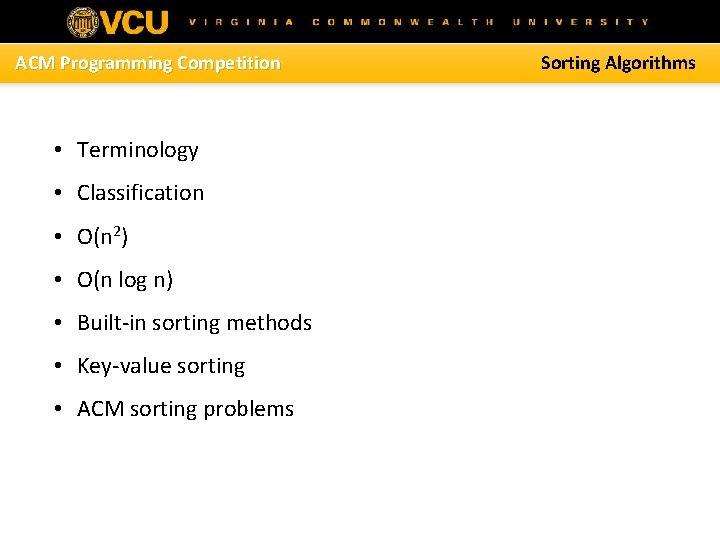
ACM Programming Competition • Terminology • Classification • O(n 2) • O(n log n) • Built-in sorting methods • Key-value sorting • ACM sorting problems Sorting Algorithms
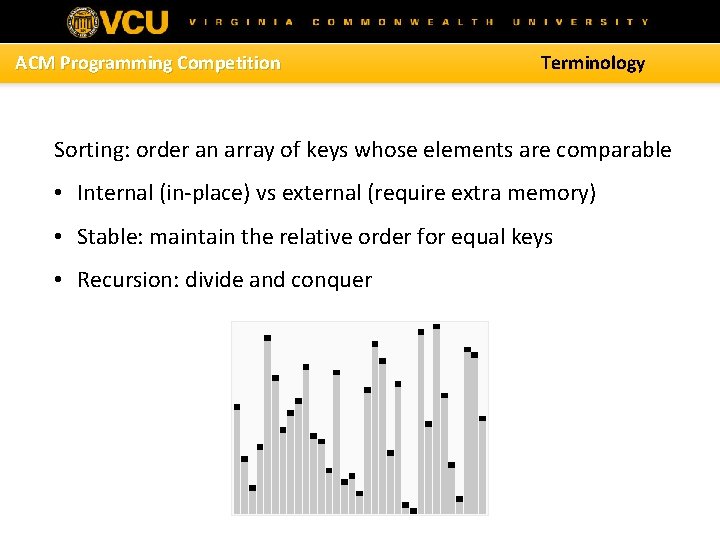
ACM Programming Competition Terminology Sorting: order an array of keys whose elements are comparable • Internal (in-place) vs external (require extra memory) • Stable: maintain the relative order for equal keys • Recursion: divide and conquer
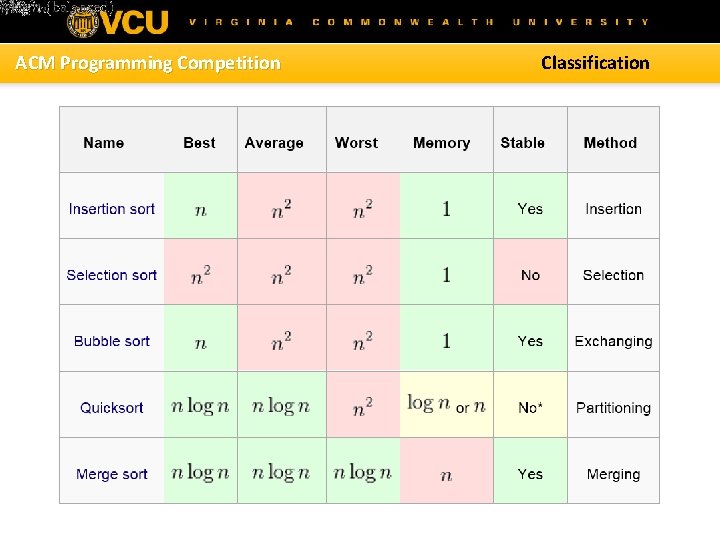
ACM Programming Competition Classification
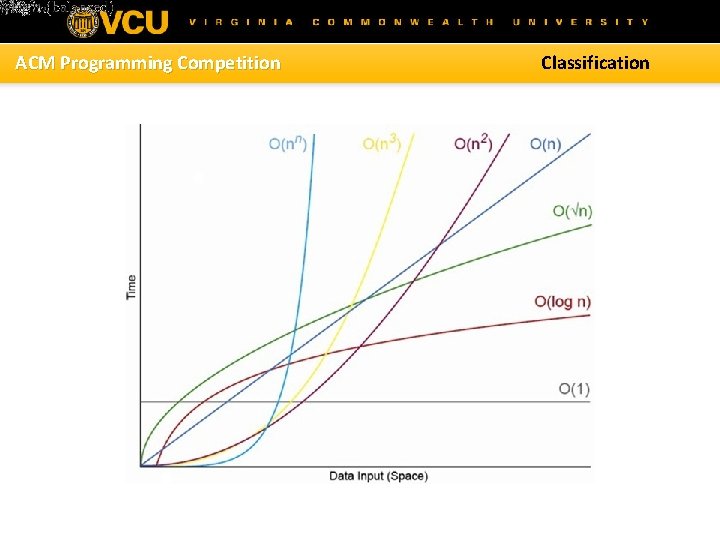
ACM Programming Competition Classification
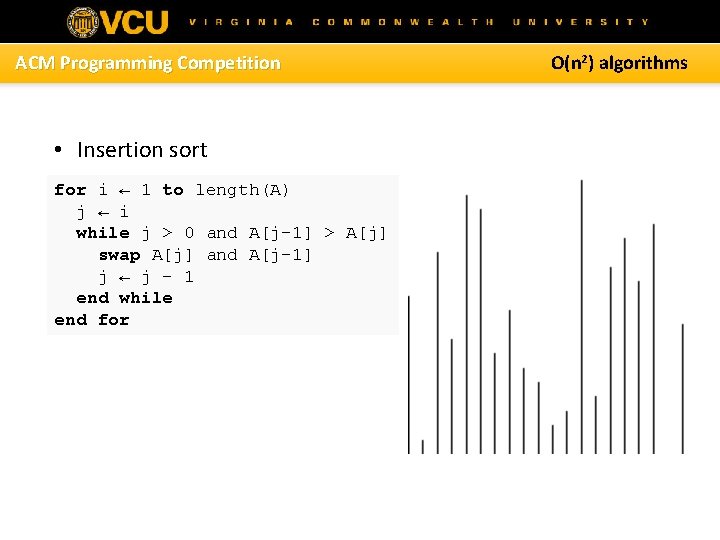
ACM Programming Competition • Insertion sort for i ← 1 to length(A) j ← i while j > 0 and A[j-1] > A[j] swap A[j] and A[j-1] j ← j – 1 end while end for O(n 2) algorithms
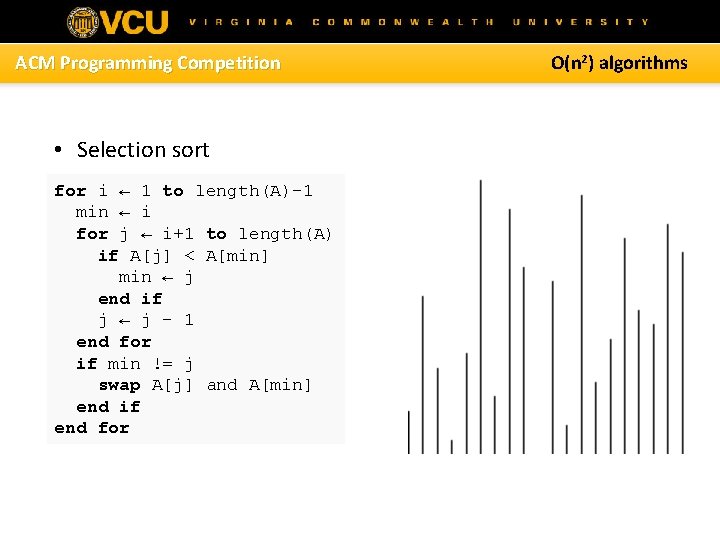
ACM Programming Competition • Selection sort for i ← 1 to length(A)-1 min ← i for j ← i+1 to length(A) if A[j] < A[min] min ← j end if j ← j – 1 end for if min != j swap A[j] and A[min] end if end for O(n 2) algorithms
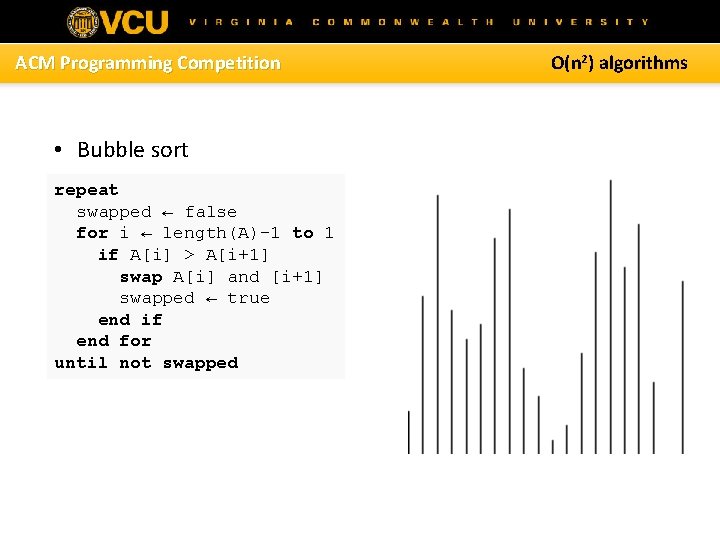
ACM Programming Competition • Bubble sort repeat swapped ← false for i ← length(A)-1 to 1 if A[i] > A[i+1] swap A[i] and [i+1] swapped ← true end if end for until not swapped O(n 2) algorithms
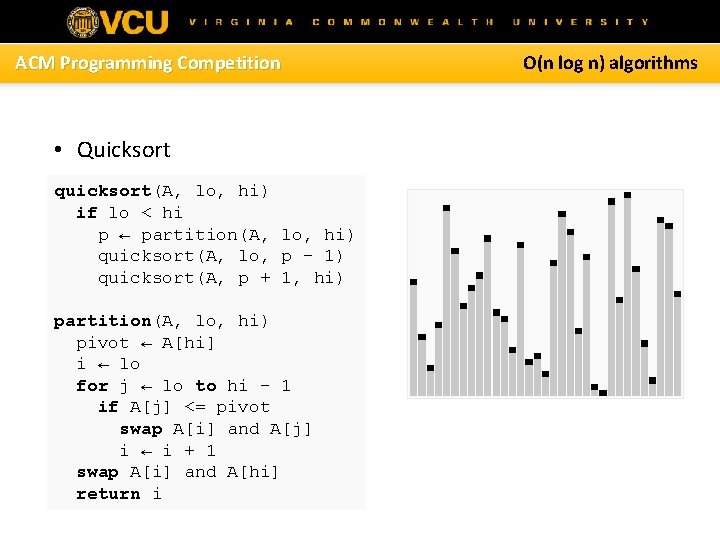
ACM Programming Competition • Quicksort quicksort(A, lo, hi) if lo < hi p ← partition(A, lo, hi) quicksort(A, lo, p - 1) quicksort(A, p + 1, hi) partition(A, lo, hi) pivot ← A[hi] i ← lo for j ← lo to hi - 1 if A[j] <= pivot swap A[i] and A[j] i ← i + 1 swap A[i] and A[hi] return i O(n log n) algorithms
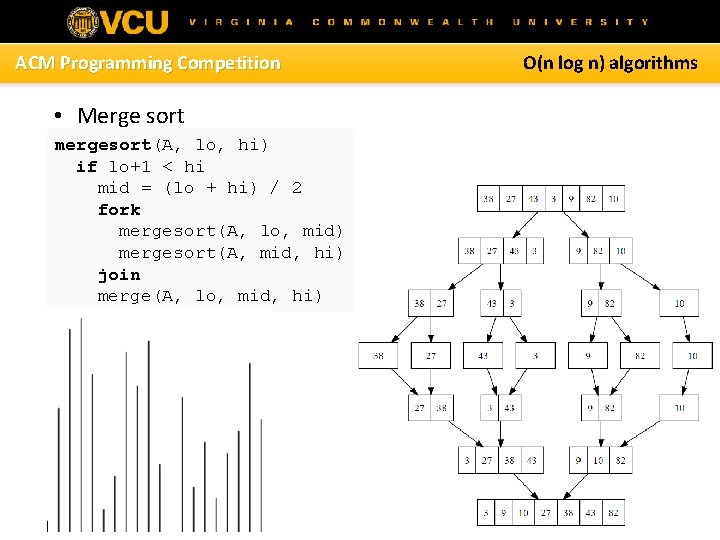
ACM Programming Competition • Merge sort mergesort(A, lo, hi) if lo+1 < hi mid = (lo + hi) / 2 fork mergesort(A, lo, mid) mergesort(A, mid, hi) join merge(A, lo, mid, hi) O(n log n) algorithms
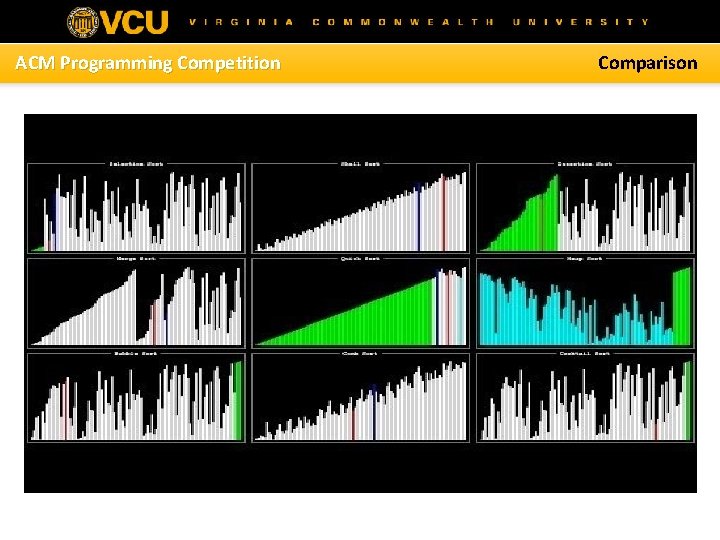
ACM Programming Competition Comparison
![ACM Programming Competition Builtin sorting methods Arrays sortT A Quicksort Arrays parallel ACM Programming Competition Built-in sorting methods • Arrays. sort(T[] A) Quicksort • Arrays. parallel.](https://slidetodoc.com/presentation_image_h/d0d3b023937acf644b7124a7408f69ba/image-12.jpg)
ACM Programming Competition Built-in sorting methods • Arrays. sort(T[] A) Quicksort • Arrays. parallel. Sort(T[] A) Parallel Quicksort • Collections. sort(List<T> list) Merge sort Collections. sort(person. List, new Comparator<Person>(){ public int compare(Person p 1, Person p 2){ return p 1. first. Name. compare. To(p 2. first. Name); } });
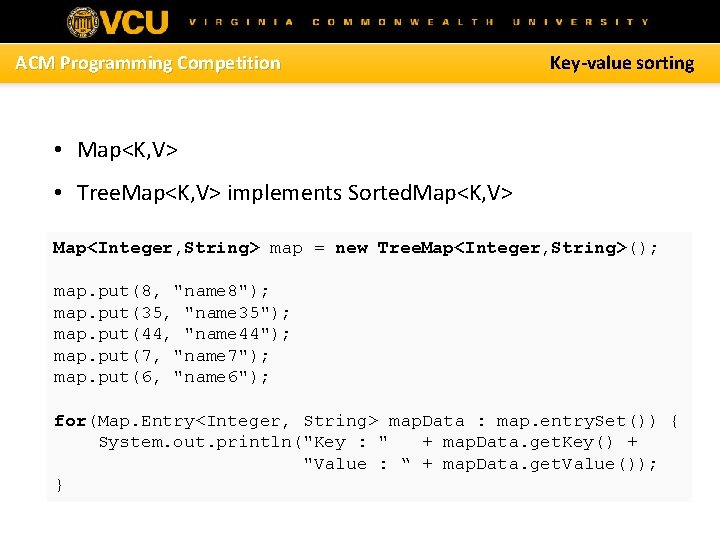
ACM Programming Competition Key-value sorting • Map<K, V> • Tree. Map<K, V> implements Sorted. Map<K, V> Map<Integer, String> map = new Tree. Map<Integer, String>(); map. put(8, "name 8"); map. put(35, "name 35"); map. put(44, "name 44"); map. put(7, "name 7"); map. put(6, "name 6"); for(Map. Entry<Integer, String> map. Data : map. entry. Set()) { System. out. println("Key : " + map. Data. get. Key() + "Value : “ + map. Data. get. Value()); }
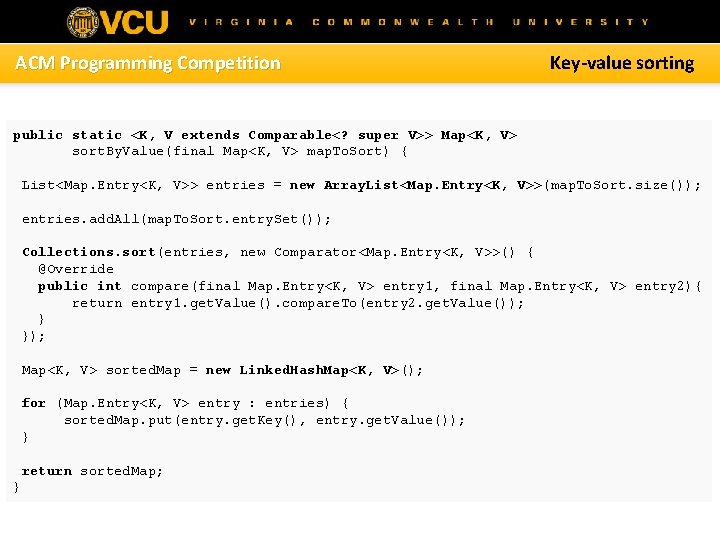
ACM Programming Competition Key-value sorting public static <K, V extends Comparable<? super V>> Map<K, V> sort. By. Value(final Map<K, V> map. To. Sort) { List<Map. Entry<K, V>> entries = new Array. List<Map. Entry<K, V>>(map. To. Sort. size()); entries. add. All(map. To. Sort. entry. Set()); Collections. sort(entries, new Comparator<Map. Entry<K, V>>() { @Override public int compare(final Map. Entry<K, V> entry 1, final Map. Entry<K, V> entry 2){ return entry 1. get. Value(). compare. To(entry 2. get. Value()); } }); Map<K, V> sorted. Map = new Linked. Hash. Map<K, V>(); for (Map. Entry<K, V> entry : entries) { sorted. Map. put(entry. get. Key(), entry. get. Value()); } return sorted. Map; }
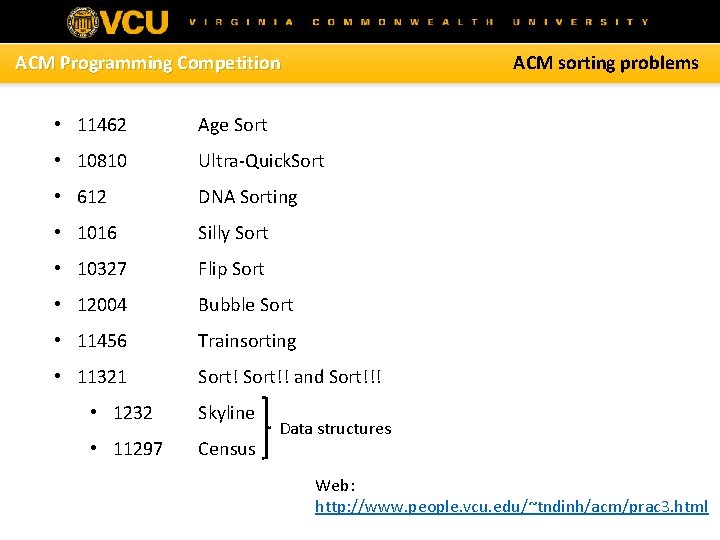
ACM Programming Competition ACM sorting problems • 11462 Age Sort • 10810 Ultra-Quick. Sort • 612 DNA Sorting • 1016 Silly Sort • 10327 Flip Sort • 12004 Bubble Sort • 11456 Trainsorting • 11321 Sort!! and Sort!!! • 1232 Skyline • 11297 Census Data structures Web: http: //www. people. vcu. edu/~tndinh/acm/prac 3. html
Internal sorting and external sorting
Introduction to sorting algorithms
Efficiency of sorting algorithms
Bsort
Insertion sort decision tree 4 elements
Sorting algorithms in c
External sorting algorithms
Recursive sorting algorithms
Log n
Most common sorting algorithms
Quadratic sorting algorithms
Place:sort=8&redirectsmode=2&maxresults=10/
Game programming algorithms and techniques
Computational thinking algorithms and programming
Synchronization algorithms and concurrent programming
Lump sum subsidy