Unit 9 Completed a whistlestop tour of programming
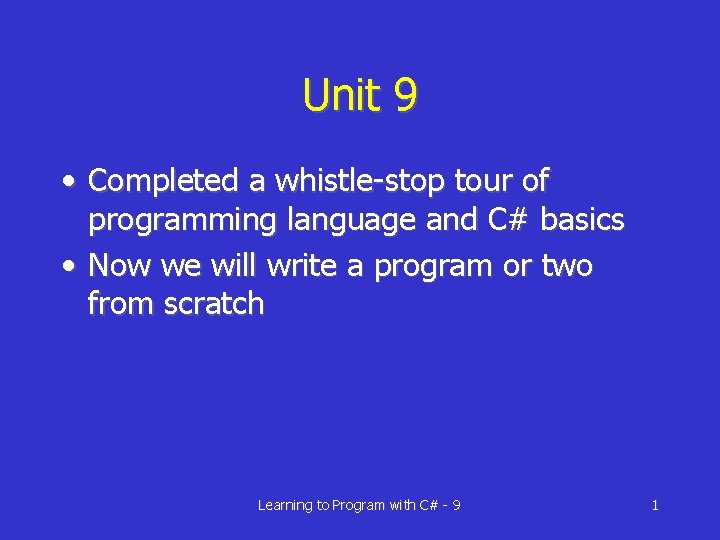
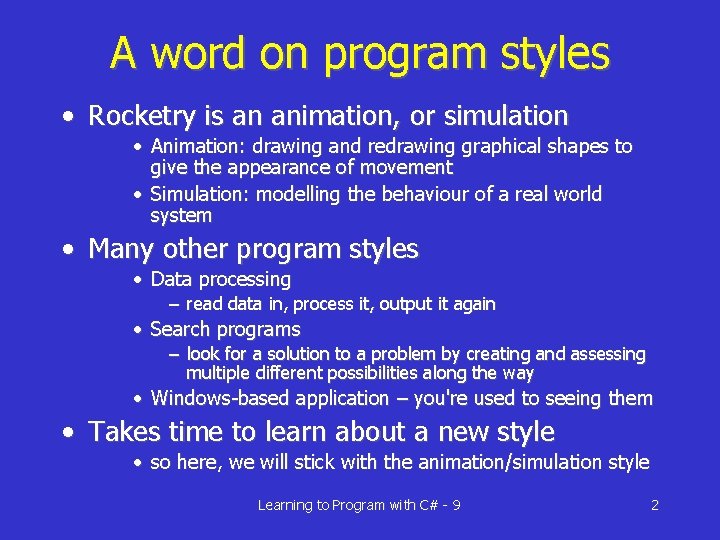
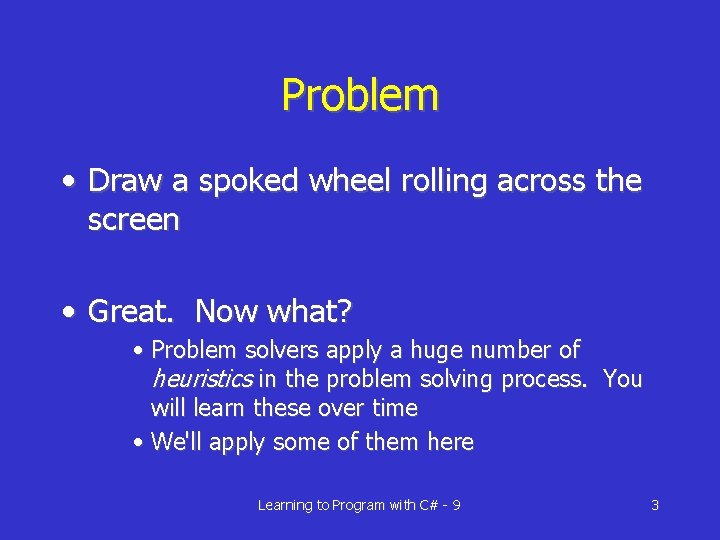
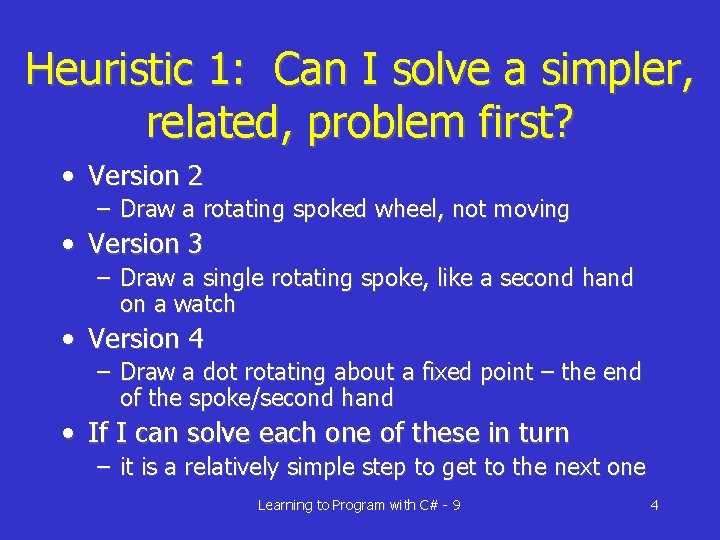
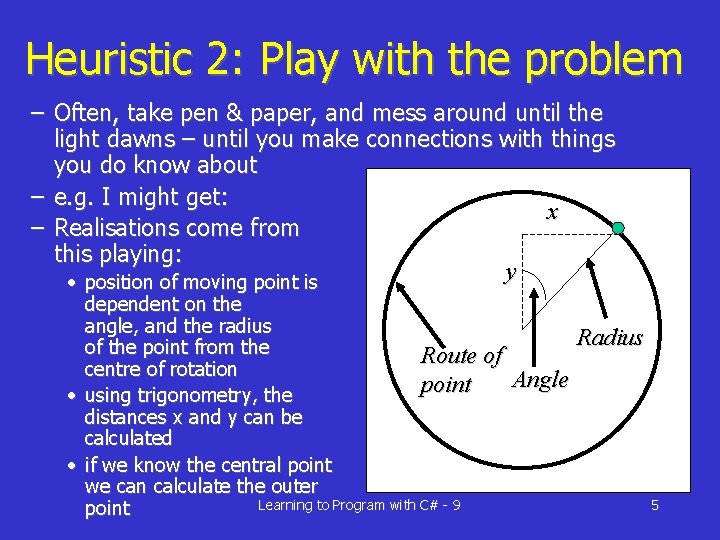
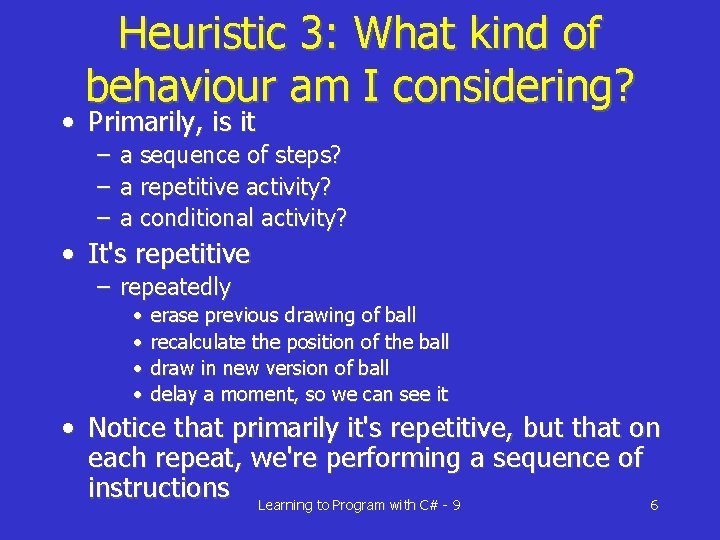
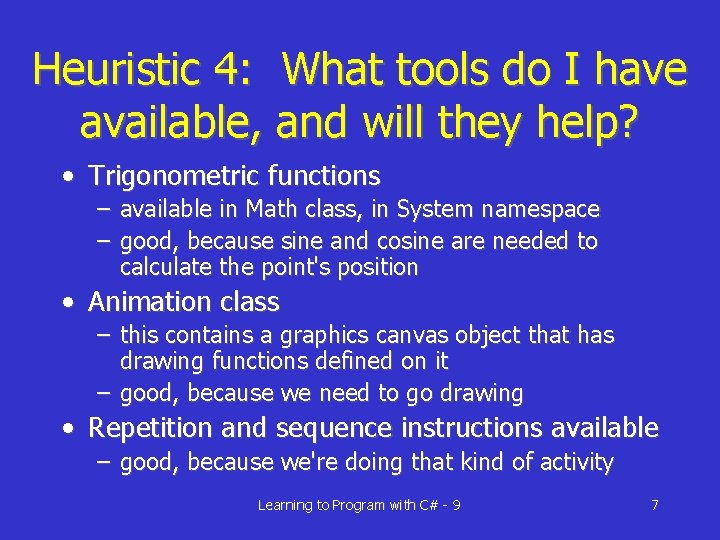
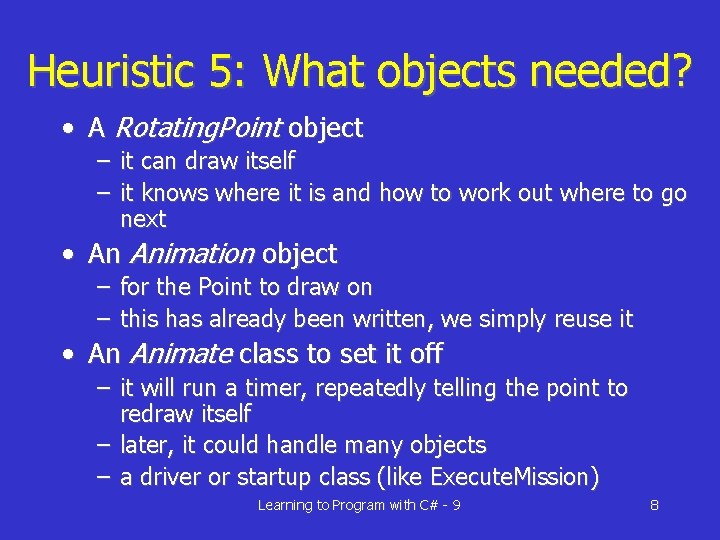
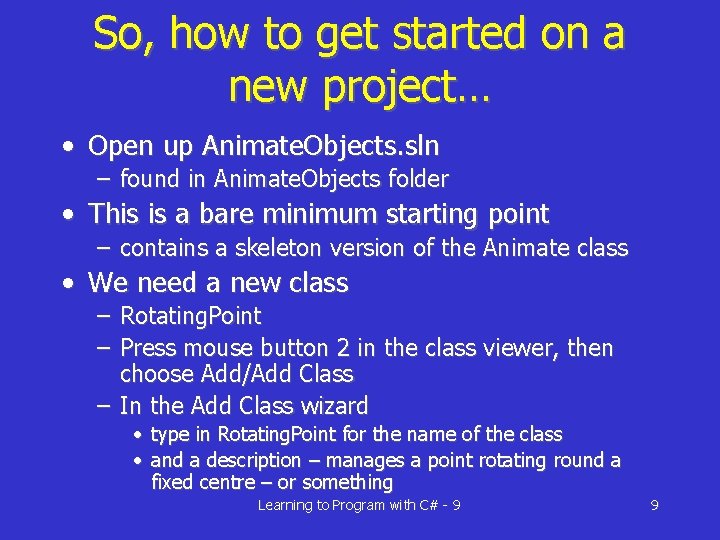
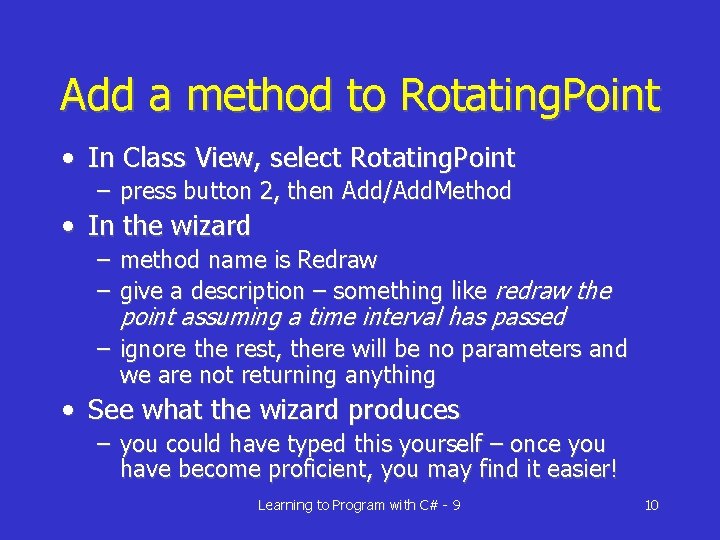
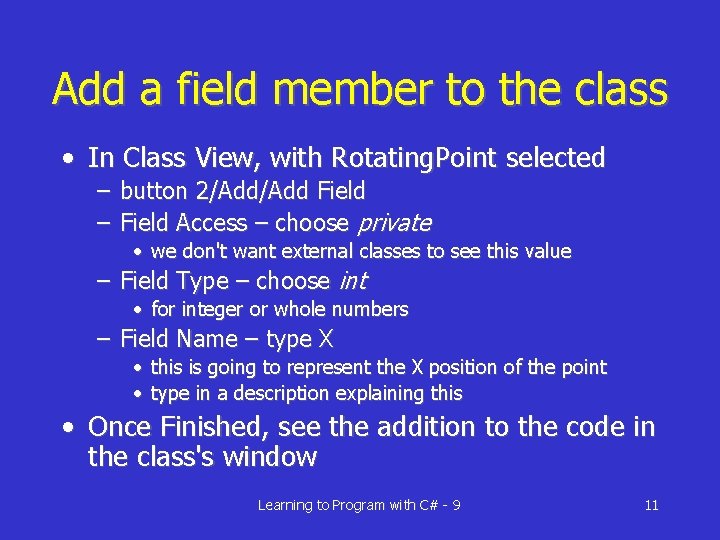
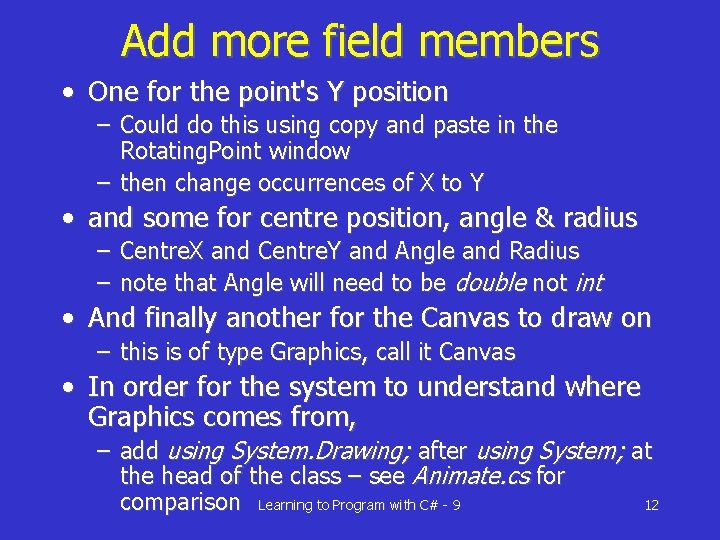
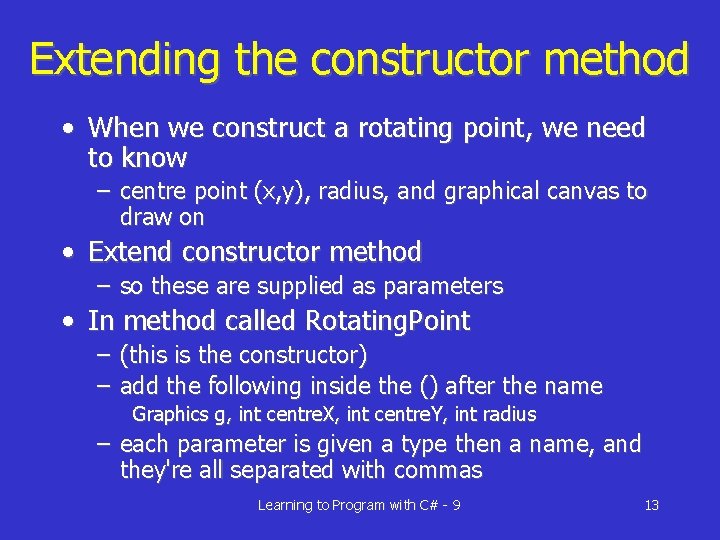
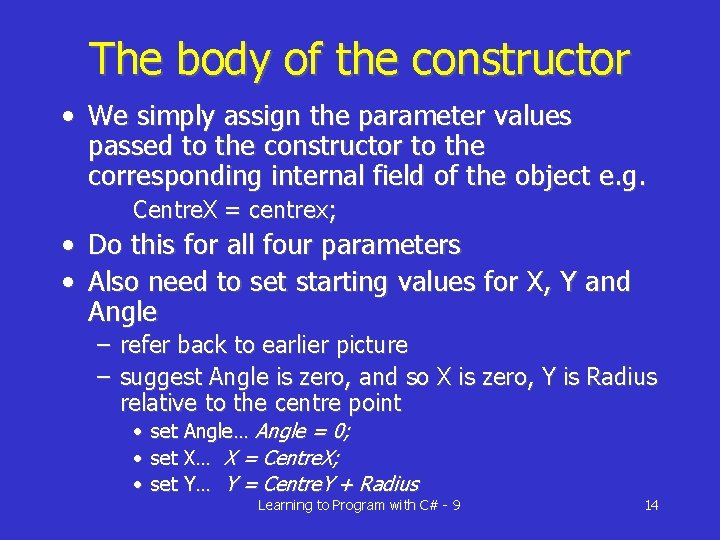
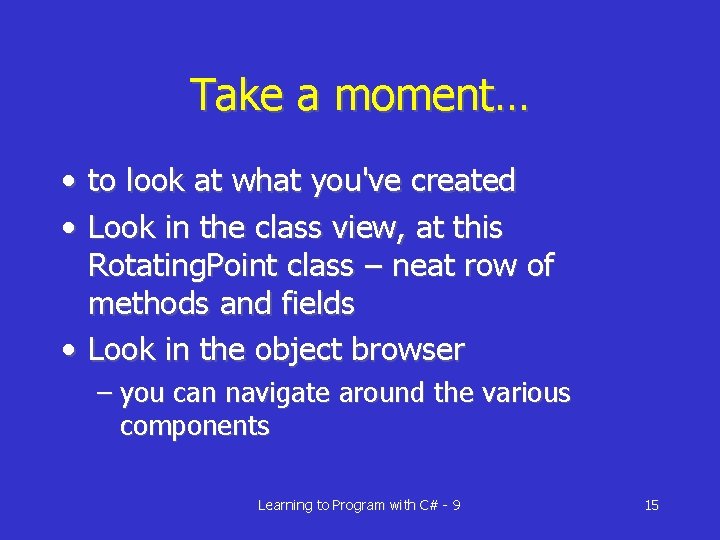
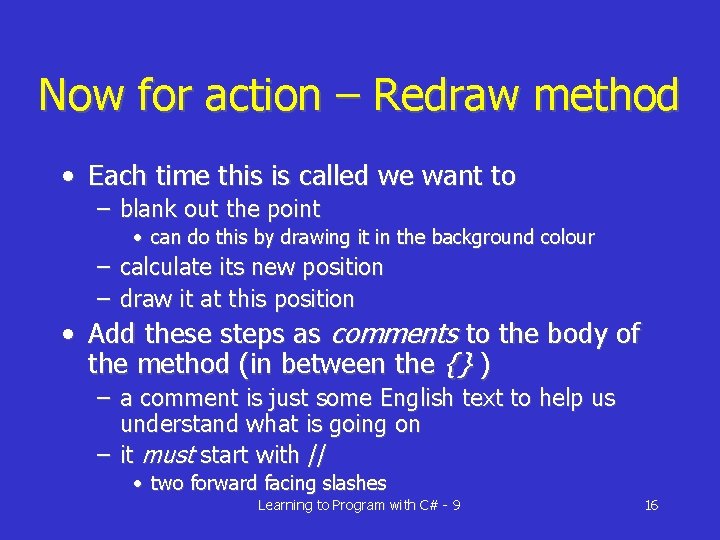
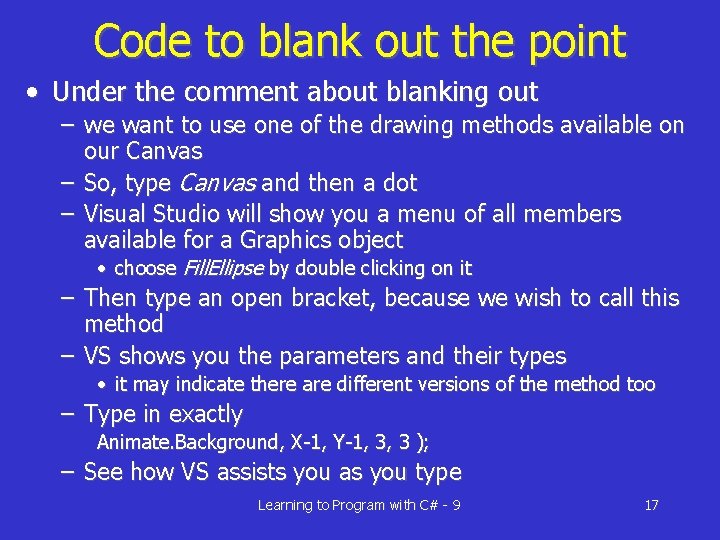
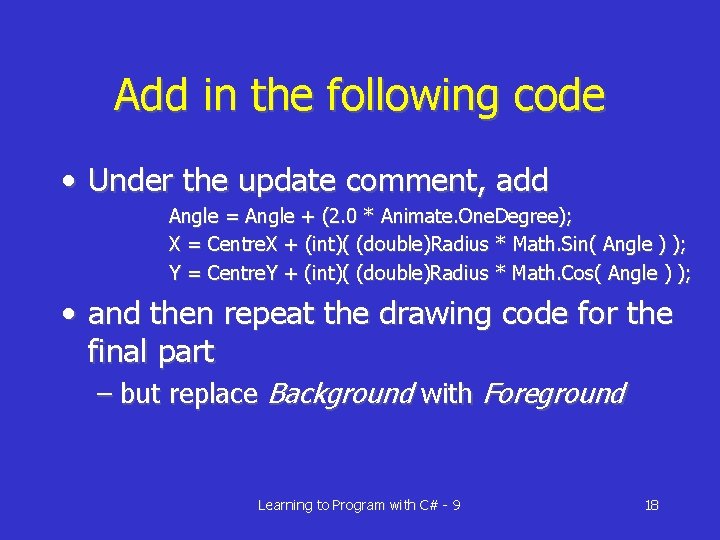
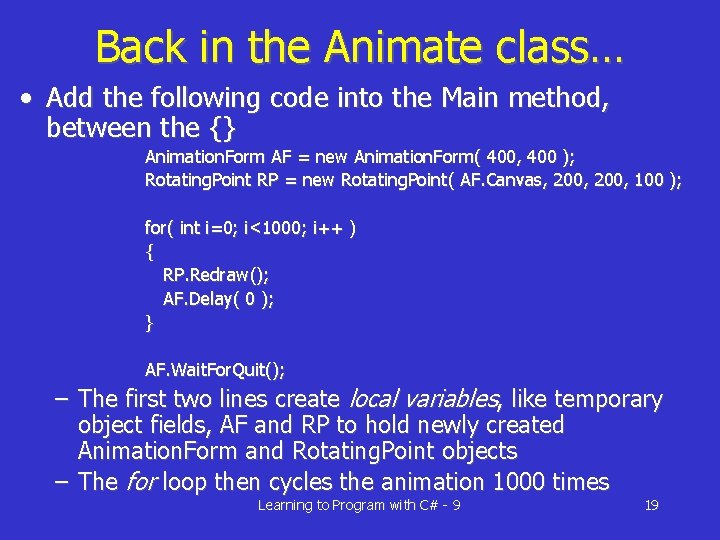
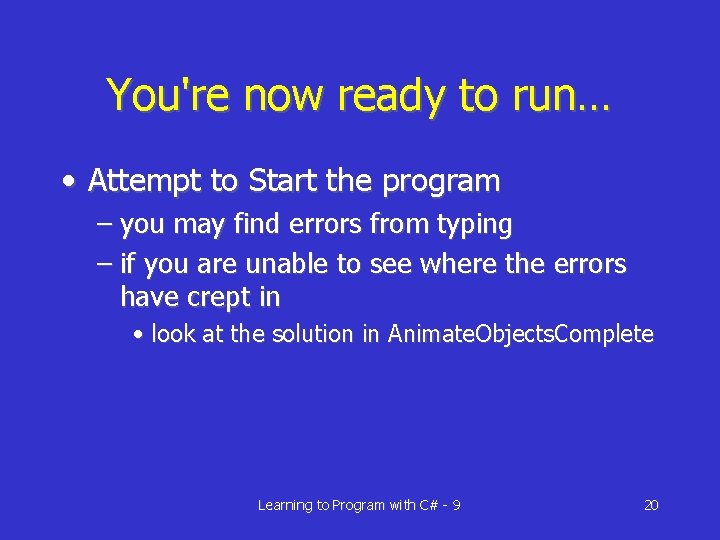
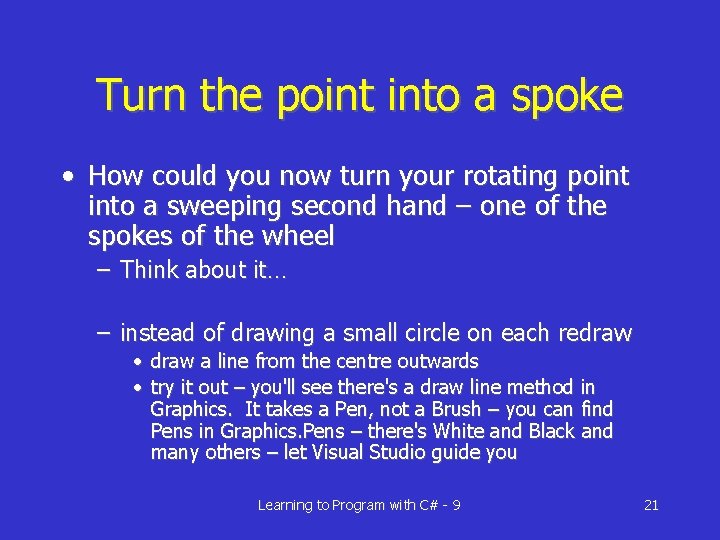
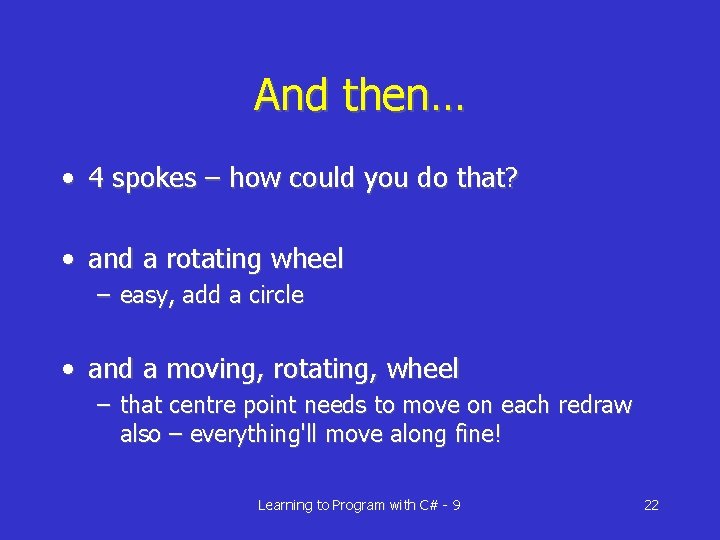
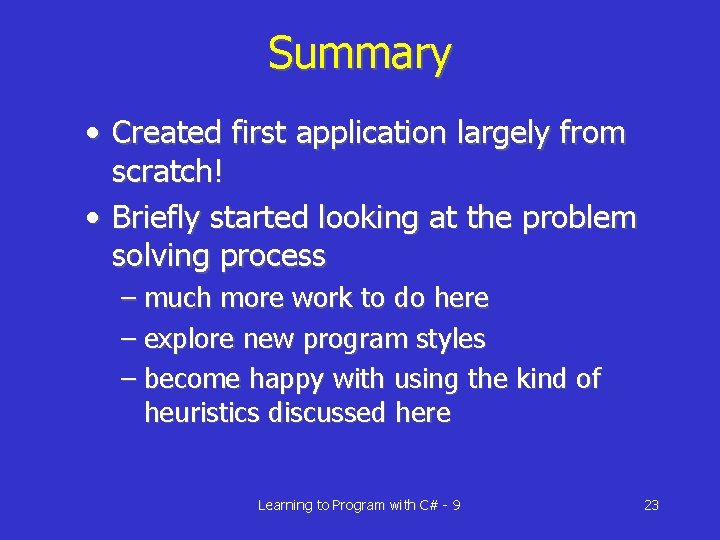
- Slides: 23
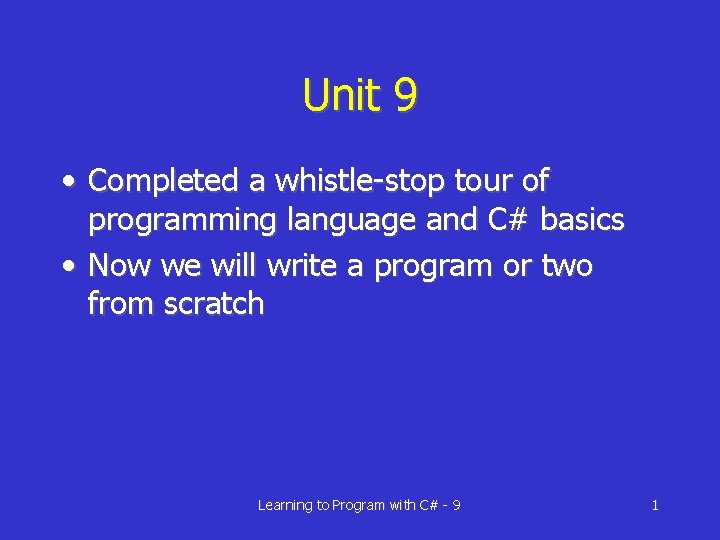
Unit 9 • Completed a whistle-stop tour of programming language and C# basics • Now we will write a program or two from scratch Learning to Program with C# - 9 1
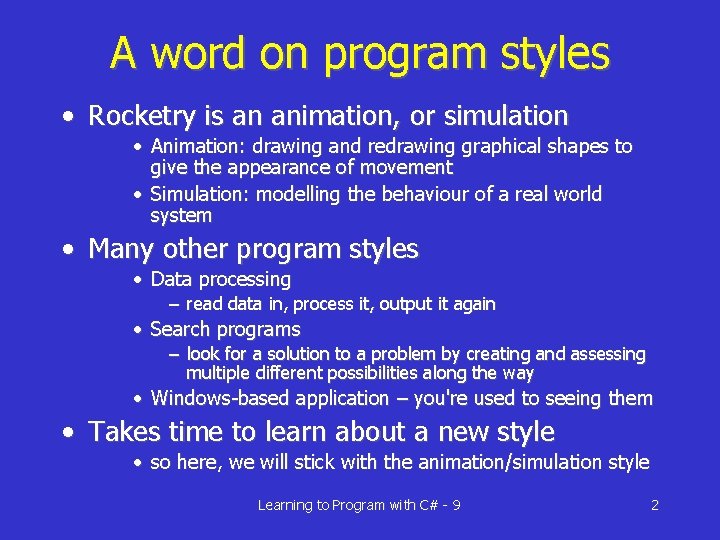
A word on program styles • Rocketry is an animation, or simulation • Animation: drawing and redrawing graphical shapes to give the appearance of movement • Simulation: modelling the behaviour of a real world system • Many other program styles • Data processing – read data in, process it, output it again • Search programs – look for a solution to a problem by creating and assessing multiple different possibilities along the way • Windows-based application – you're used to seeing them • Takes time to learn about a new style • so here, we will stick with the animation/simulation style Learning to Program with C# - 9 2
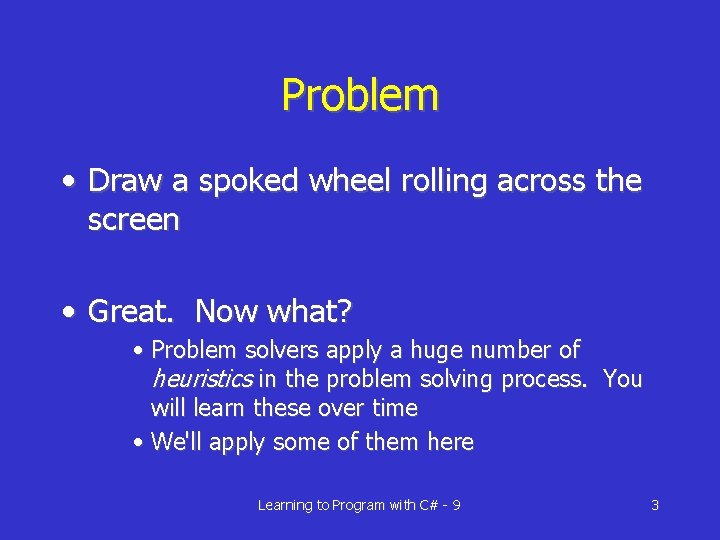
Problem • Draw a spoked wheel rolling across the screen • Great. Now what? • Problem solvers apply a huge number of heuristics in the problem solving process. You will learn these over time • We'll apply some of them here Learning to Program with C# - 9 3
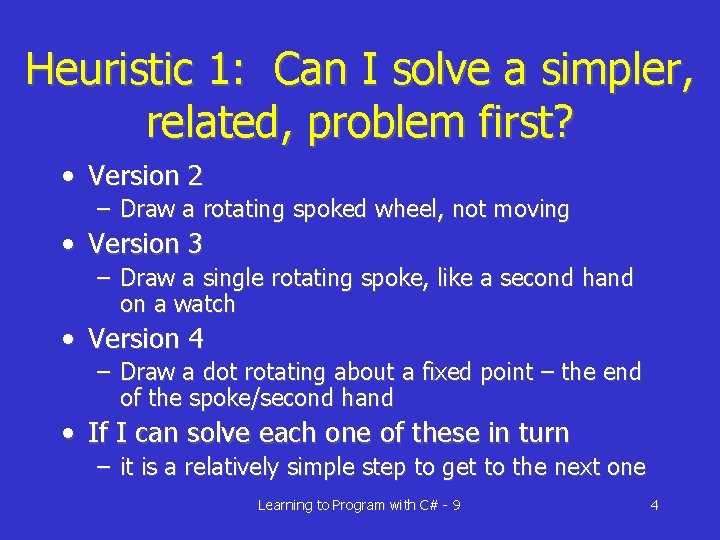
Heuristic 1: Can I solve a simpler, related, problem first? • Version 2 – Draw a rotating spoked wheel, not moving • Version 3 – Draw a single rotating spoke, like a second hand on a watch • Version 4 – Draw a dot rotating about a fixed point – the end of the spoke/second hand • If I can solve each one of these in turn – it is a relatively simple step to get to the next one Learning to Program with C# - 9 4
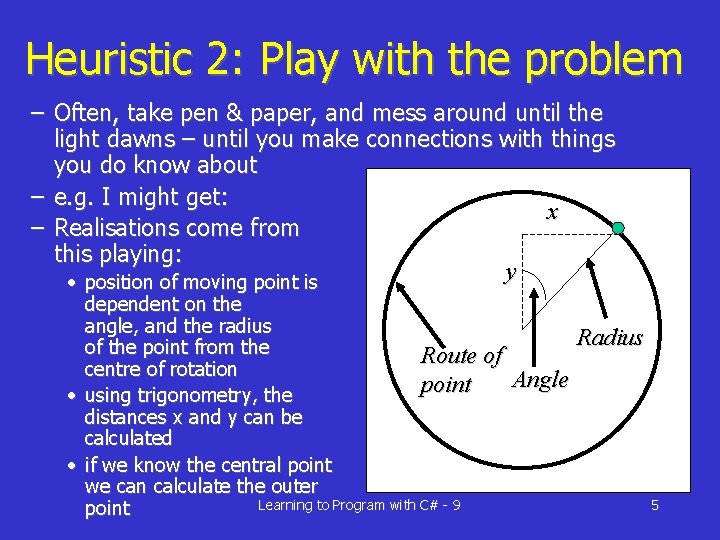
Heuristic 2: Play with the problem – Often, take pen & paper, and mess around until the light dawns – until you make connections with things you do know about – e. g. I might get: x – Realisations come from this playing: y • position of moving point is dependent on the angle, and the radius of the point from the Route of centre of rotation point • using trigonometry, the distances x and y can be calculated • if we know the central point we can calculate the outer Learning to Program with C# - 9 point Radius Angle 5
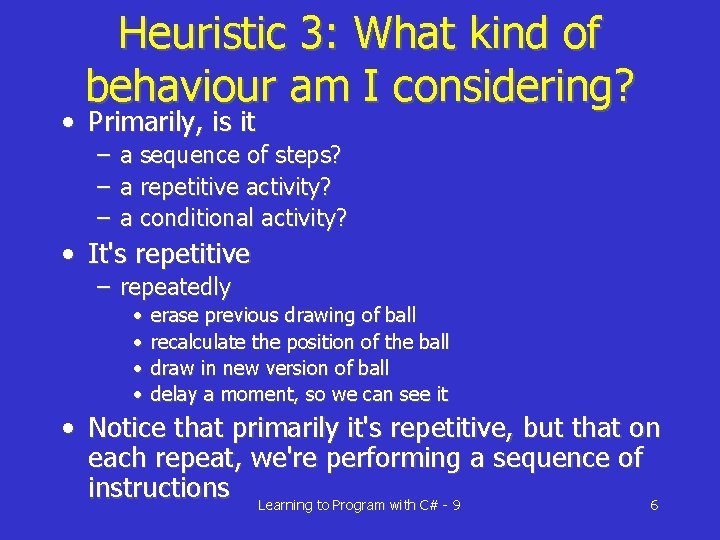
Heuristic 3: What kind of behaviour am I considering? • Primarily, is it – – – a sequence of steps? a repetitive activity? a conditional activity? • It's repetitive – repeatedly • • erase previous drawing of ball recalculate the position of the ball draw in new version of ball delay a moment, so we can see it • Notice that primarily it's repetitive, but that on each repeat, we're performing a sequence of instructions Learning to Program with C# - 9 6
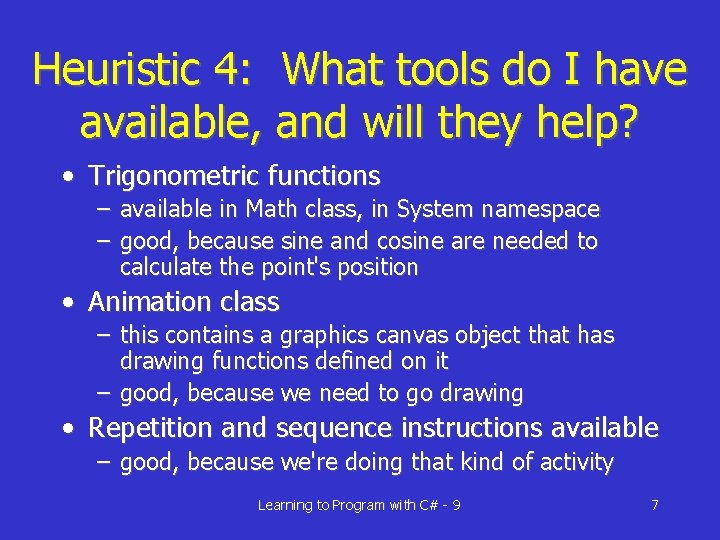
Heuristic 4: What tools do I have available, and will they help? • Trigonometric functions – available in Math class, in System namespace – good, because sine and cosine are needed to calculate the point's position • Animation class – this contains a graphics canvas object that has drawing functions defined on it – good, because we need to go drawing • Repetition and sequence instructions available – good, because we're doing that kind of activity Learning to Program with C# - 9 7
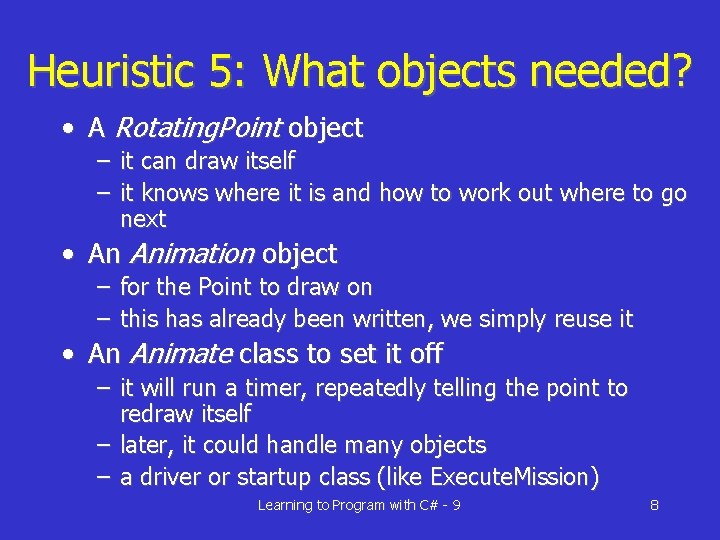
Heuristic 5: What objects needed? • A Rotating. Point object – it can draw itself – it knows where it is and how to work out where to go next • An Animation object – – for the Point to draw on this has already been written, we simply reuse it • An Animate class to set it off – it will run a timer, repeatedly telling the point to redraw itself – later, it could handle many objects – a driver or startup class (like Execute. Mission) Learning to Program with C# - 9 8
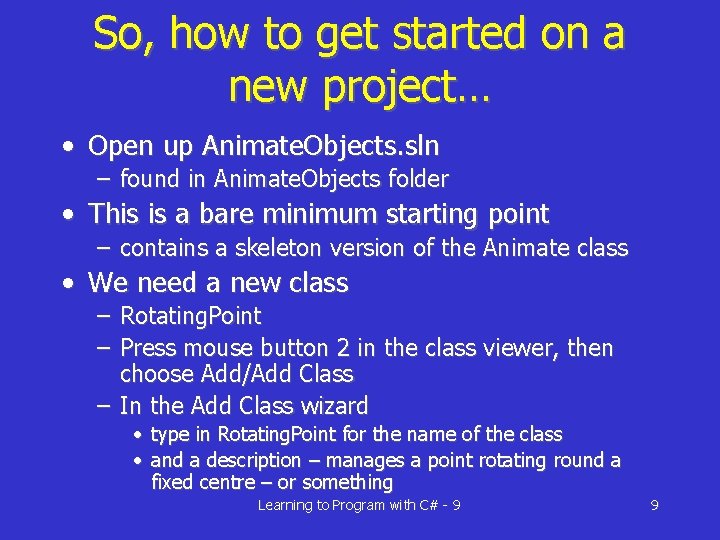
So, how to get started on a new project… • Open up Animate. Objects. sln – found in Animate. Objects folder • This is a bare minimum starting point – contains a skeleton version of the Animate class • We need a new class – Rotating. Point – Press mouse button 2 in the class viewer, then choose Add/Add Class – In the Add Class wizard • type in Rotating. Point for the name of the class • and a description – manages a point rotating round a fixed centre – or something Learning to Program with C# - 9 9
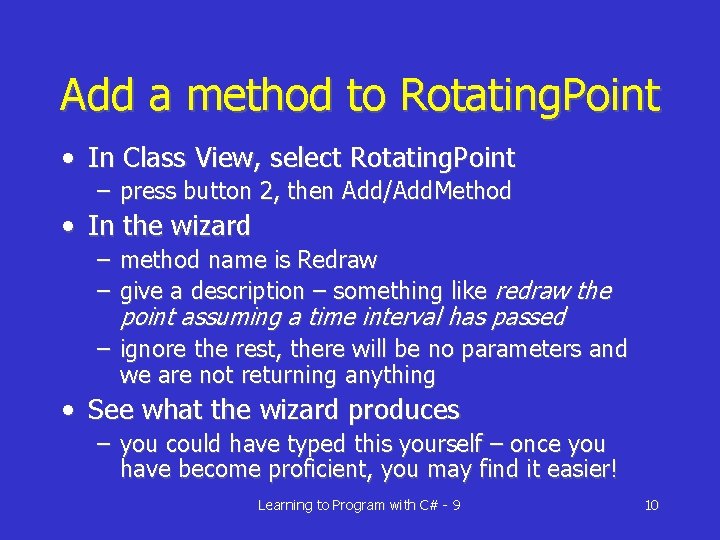
Add a method to Rotating. Point • In Class View, select Rotating. Point – press button 2, then Add/Add. Method • In the wizard – – method name is Redraw give a description – something like redraw the point assuming a time interval has passed – ignore the rest, there will be no parameters and we are not returning anything • See what the wizard produces – you could have typed this yourself – once you have become proficient, you may find it easier! Learning to Program with C# - 9 10
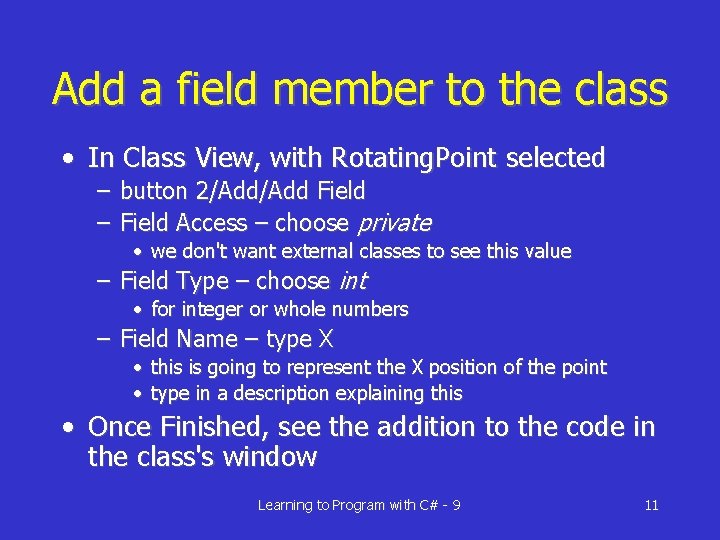
Add a field member to the class • In Class View, with Rotating. Point selected – button 2/Add Field – Field Access – choose private • we don't want external classes to see this value – Field Type – choose int • for integer or whole numbers – Field Name – type X • this is going to represent the X position of the point • type in a description explaining this • Once Finished, see the addition to the code in the class's window Learning to Program with C# - 9 11
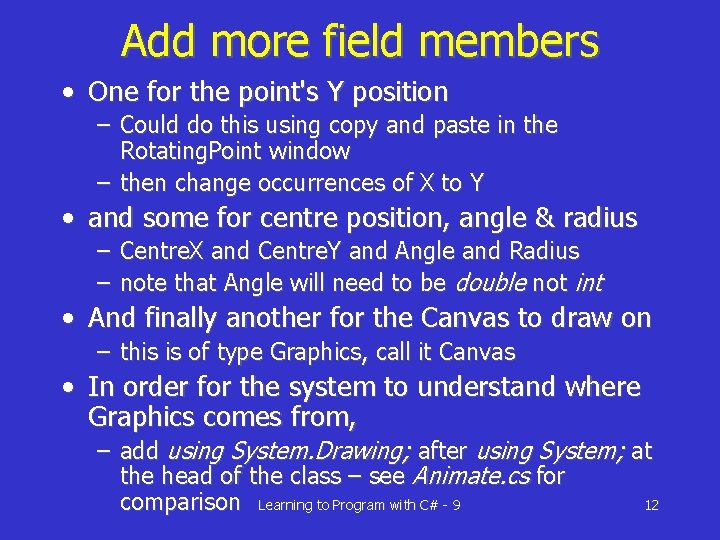
Add more field members • One for the point's Y position – Could do this using copy and paste in the Rotating. Point window – then change occurrences of X to Y • and some for centre position, angle & radius – – Centre. X and Centre. Y and Angle and Radius note that Angle will need to be double not int • And finally another for the Canvas to draw on – this is of type Graphics, call it Canvas • In order for the system to understand where Graphics comes from, – add using System. Drawing; after using System; at the head of the class – see Animate. cs for 12 comparison Learning to Program with C# - 9
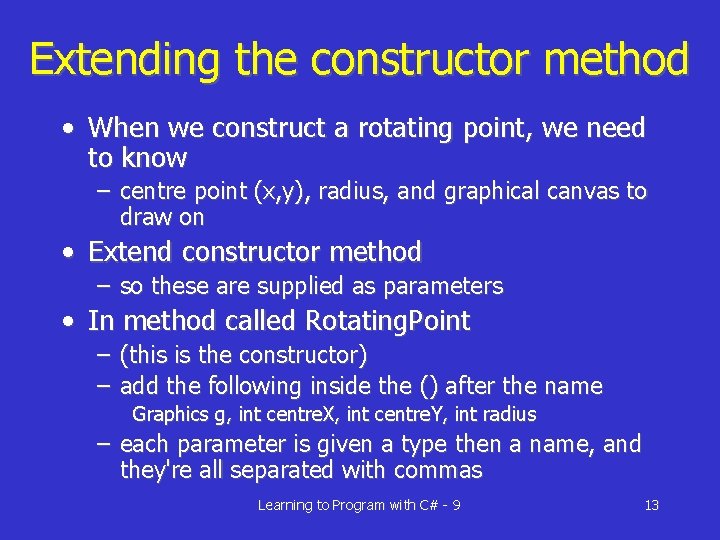
Extending the constructor method • When we construct a rotating point, we need to know – centre point (x, y), radius, and graphical canvas to draw on • Extend constructor method – so these are supplied as parameters • In method called Rotating. Point – (this is the constructor) – add the following inside the () after the name Graphics g, int centre. X, int centre. Y, int radius – each parameter is given a type then a name, and they're all separated with commas Learning to Program with C# - 9 13
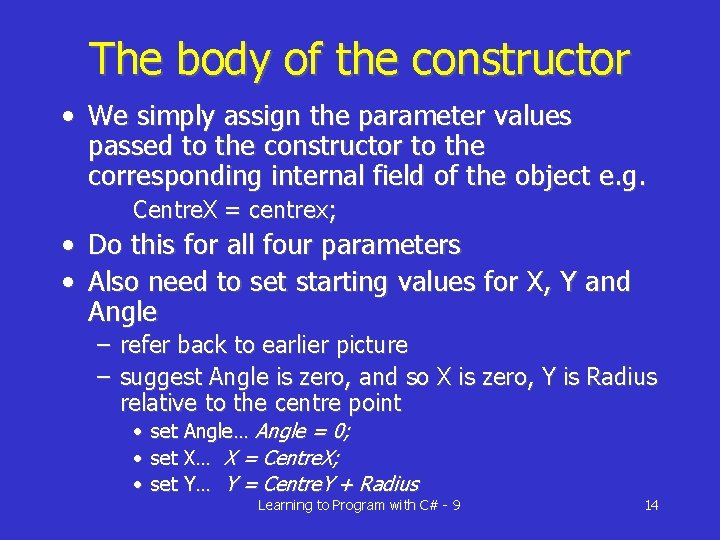
The body of the constructor • We simply assign the parameter values passed to the constructor to the corresponding internal field of the object e. g. Centre. X = centrex; • Do this for all four parameters • Also need to set starting values for X, Y and Angle – refer back to earlier picture – suggest Angle is zero, and so X is zero, Y is Radius relative to the centre point • set Angle… Angle = 0; • set X… X = Centre. X; • set Y… Y = Centre. Y + Radius Learning to Program with C# - 9 14
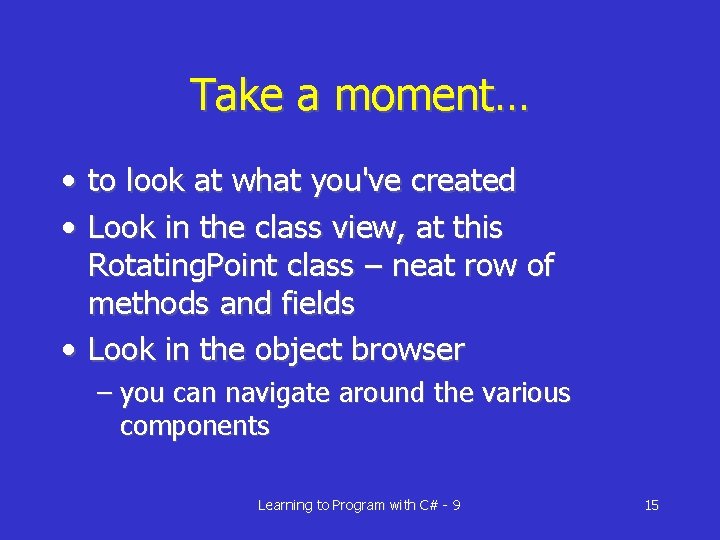
Take a moment… • to look at what you've created • Look in the class view, at this Rotating. Point class – neat row of methods and fields • Look in the object browser – you can navigate around the various components Learning to Program with C# - 9 15
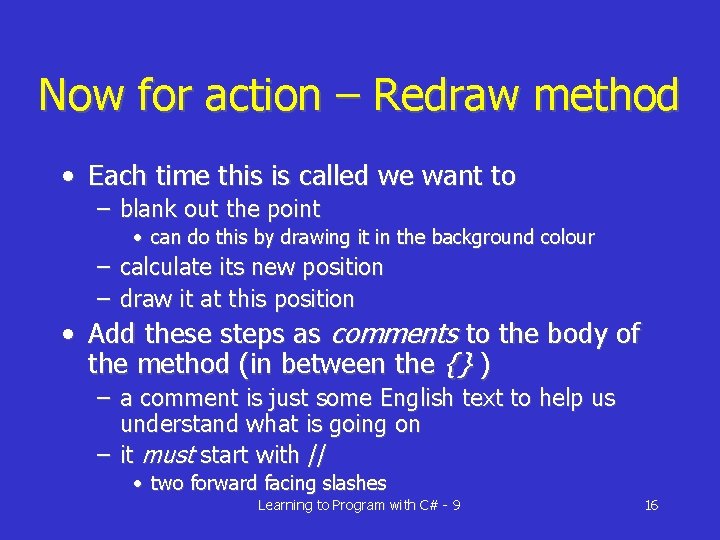
Now for action – Redraw method • Each time this is called we want to – blank out the point • can do this by drawing it in the background colour – calculate its new position – draw it at this position • Add these steps as comments to the body of the method (in between the {} ) – a comment is just some English text to help us understand what is going on – it must start with // • two forward facing slashes Learning to Program with C# - 9 16
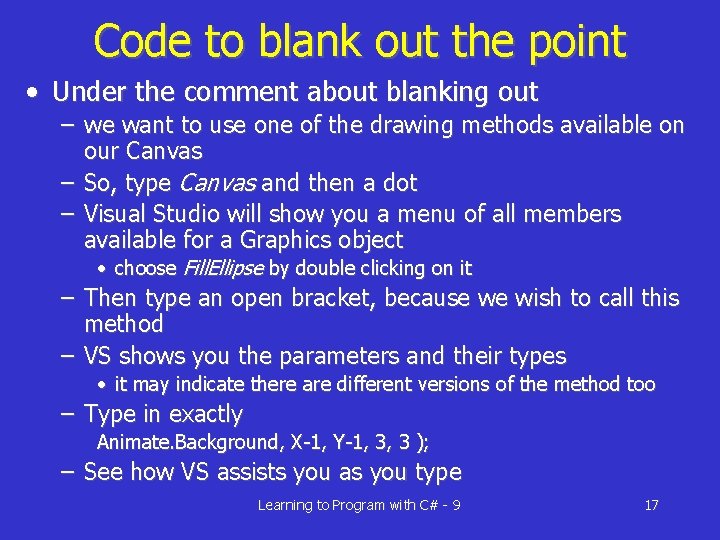
Code to blank out the point • Under the comment about blanking out – we want to use one of the drawing methods available on our Canvas – So, type Canvas and then a dot – Visual Studio will show you a menu of all members available for a Graphics object • choose Fill. Ellipse by double clicking on it – Then type an open bracket, because we wish to call this method – VS shows you the parameters and their types • it may indicate there are different versions of the method too – Type in exactly Animate. Background, X-1, Y-1, 3, 3 ); – See how VS assists you as you type Learning to Program with C# - 9 17
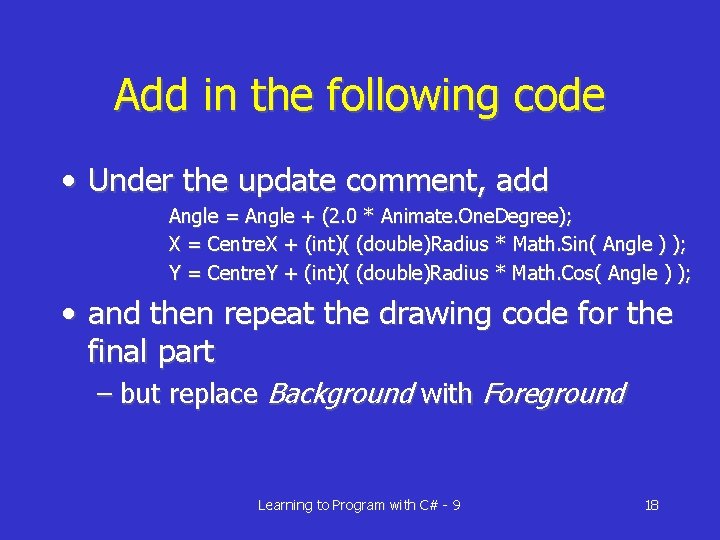
Add in the following code • Under the update comment, add Angle = Angle + (2. 0 * Animate. One. Degree); X = Centre. X + (int)( (double)Radius * Math. Sin( Angle ) ); Y = Centre. Y + (int)( (double)Radius * Math. Cos( Angle ) ); • and then repeat the drawing code for the final part – but replace Background with Foreground Learning to Program with C# - 9 18
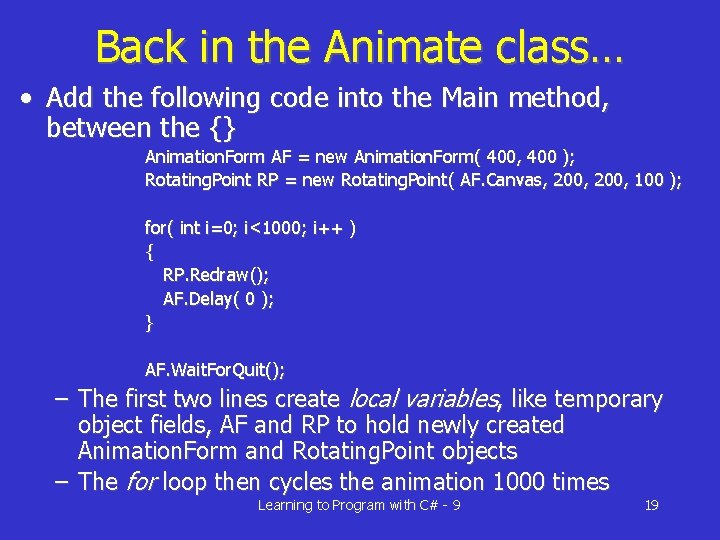
Back in the Animate class… • Add the following code into the Main method, between the {} Animation. Form AF = new Animation. Form( 400, 400 ); Rotating. Point RP = new Rotating. Point( AF. Canvas, 200, 100 ); for( int i=0; i<1000; i++ ) { RP. Redraw(); AF. Delay( 0 ); } AF. Wait. For. Quit(); – The first two lines create local variables, like temporary object fields, AF and RP to hold newly created Animation. Form and Rotating. Point objects – The for loop then cycles the animation 1000 times Learning to Program with C# - 9 19
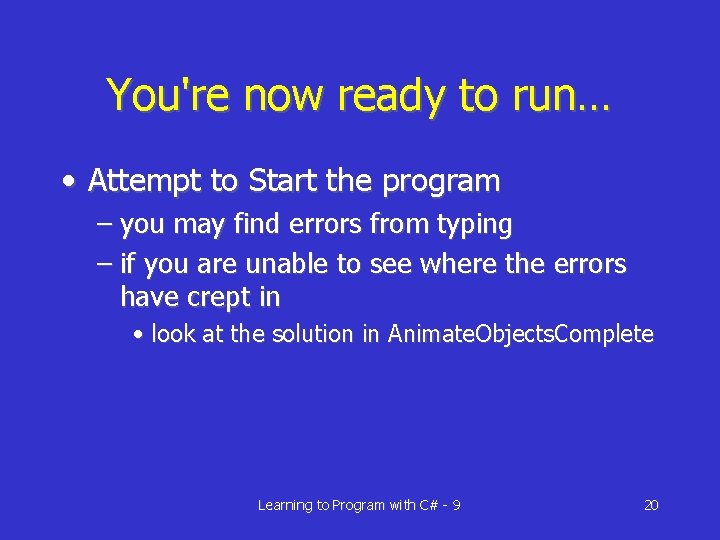
You're now ready to run… • Attempt to Start the program – you may find errors from typing – if you are unable to see where the errors have crept in • look at the solution in Animate. Objects. Complete Learning to Program with C# - 9 20
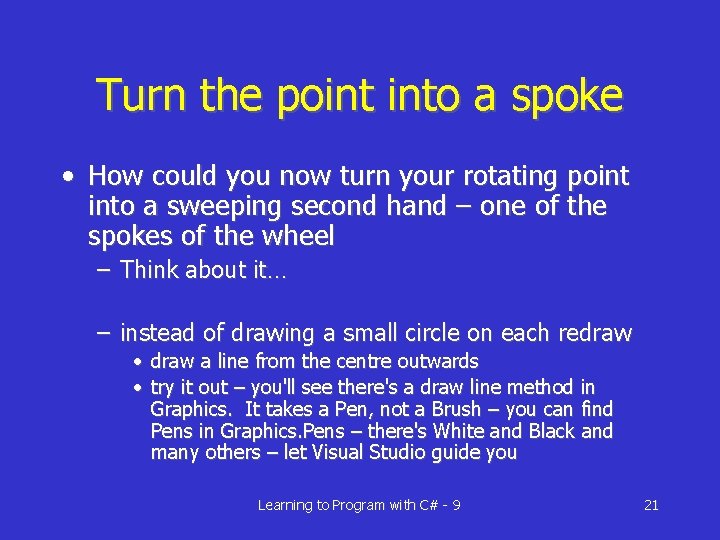
Turn the point into a spoke • How could you now turn your rotating point into a sweeping second hand – one of the spokes of the wheel – Think about it… – instead of drawing a small circle on each redraw • draw a line from the centre outwards • try it out – you'll see there's a draw line method in Graphics. It takes a Pen, not a Brush – you can find Pens in Graphics. Pens – there's White and Black and many others – let Visual Studio guide you Learning to Program with C# - 9 21
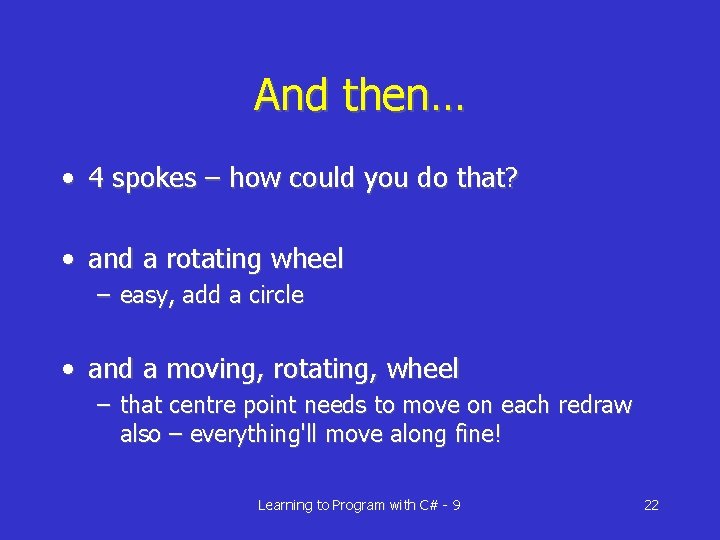
And then… • 4 spokes – how could you do that? • and a rotating wheel – easy, add a circle • and a moving, rotating, wheel – that centre point needs to move on each redraw also – everything'll move along fine! Learning to Program with C# - 9 22
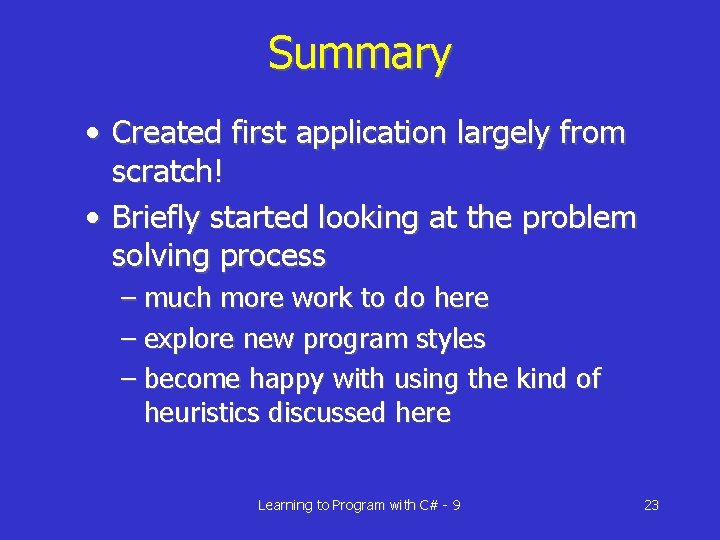
Summary • Created first application largely from scratch! • Briefly started looking at the problem solving process – much more work to do here – explore new program styles – become happy with using the kind of heuristics discussed here Learning to Program with C# - 9 23