The switch Statement 1 SWITCH MULTIPLESELECTION STATEMENT Occasionally
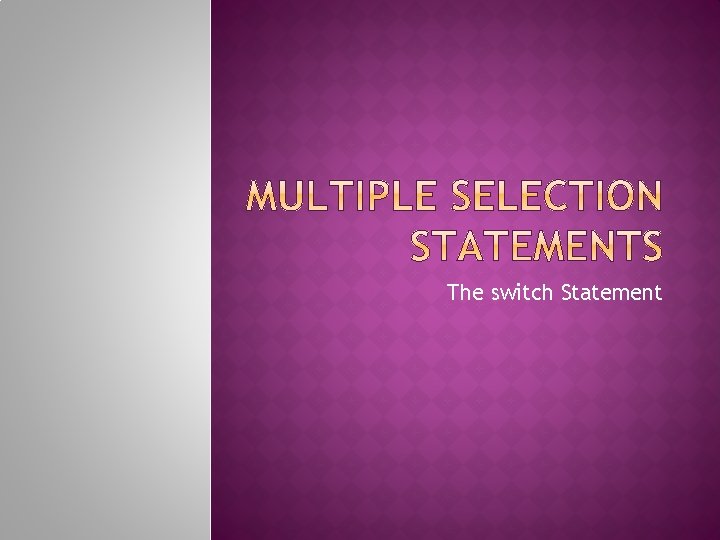
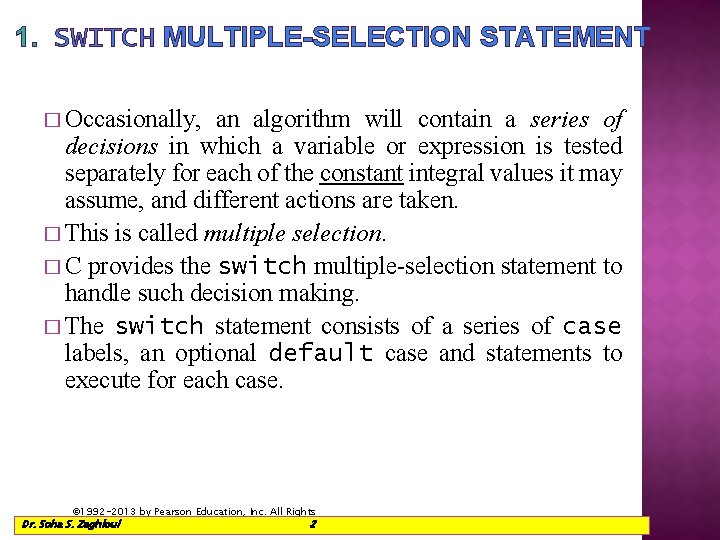
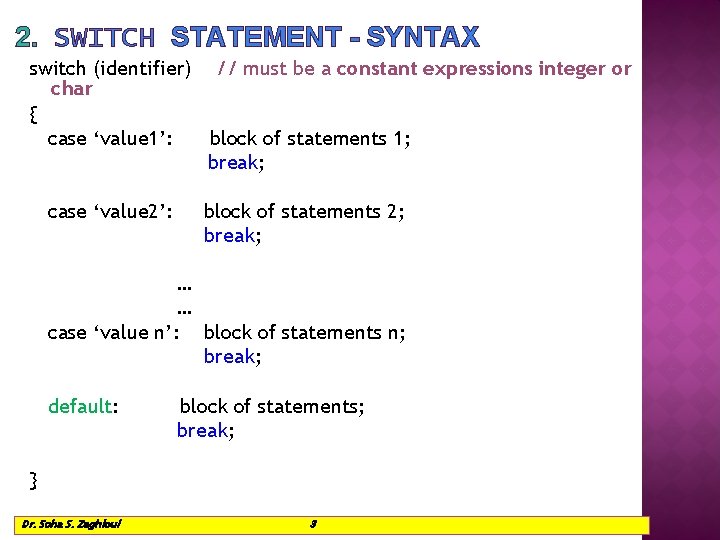
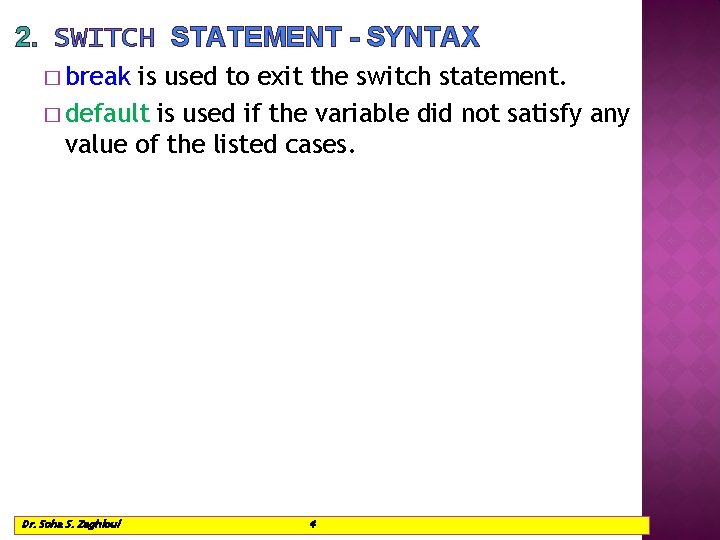
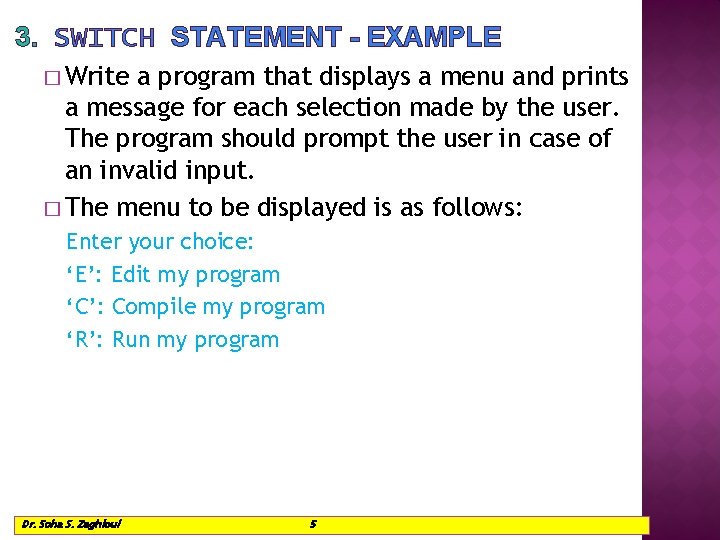
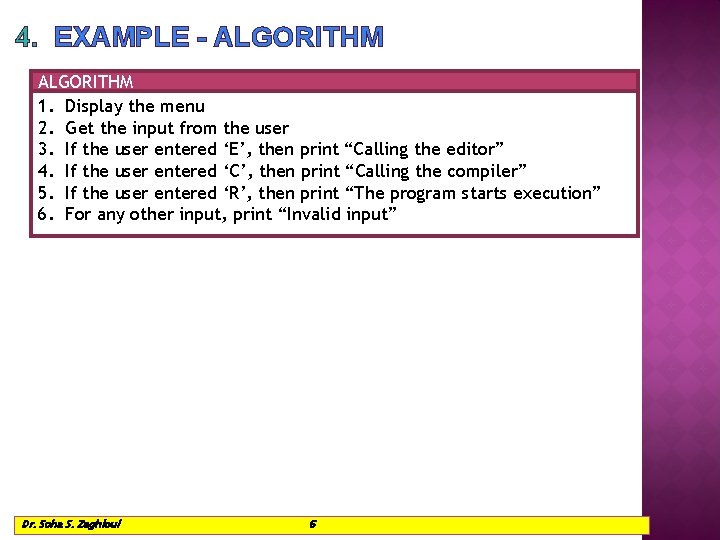
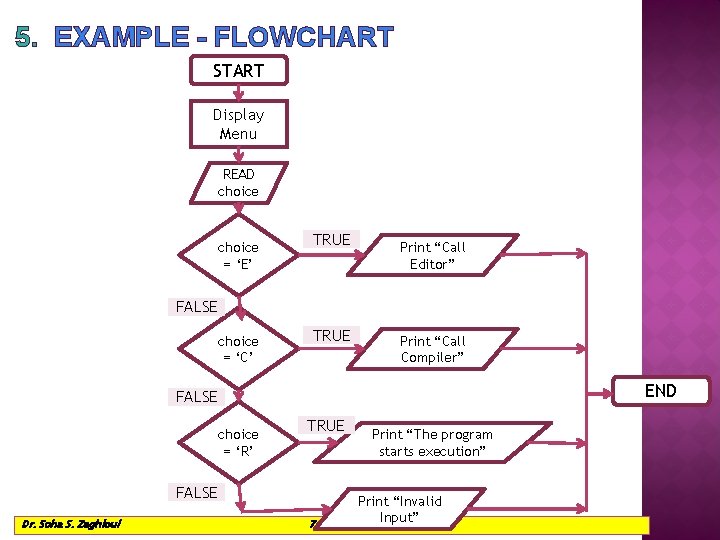
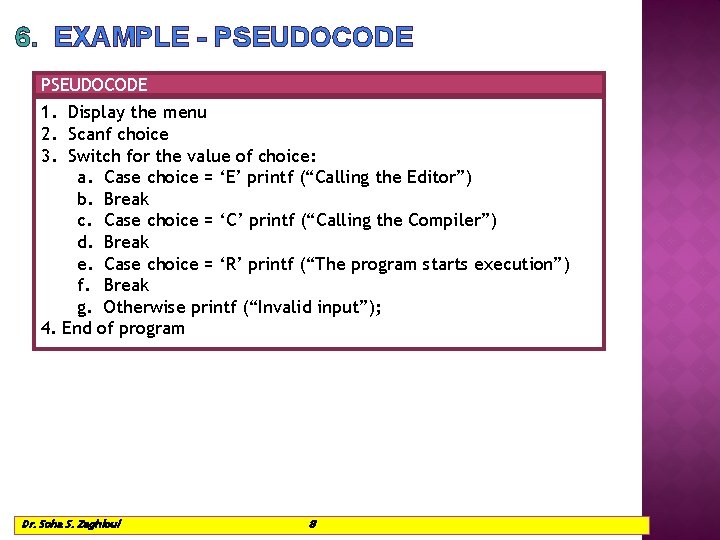
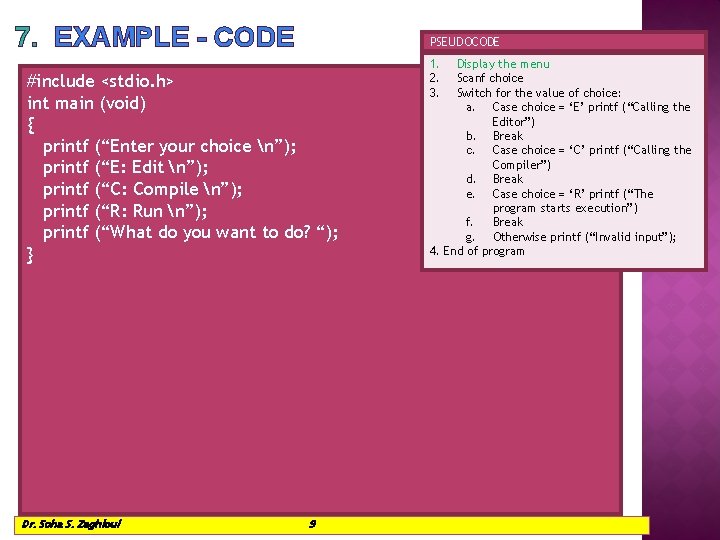
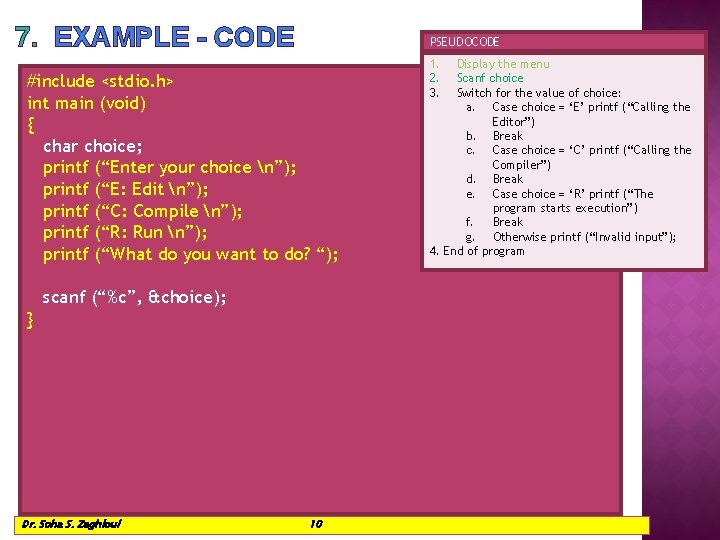
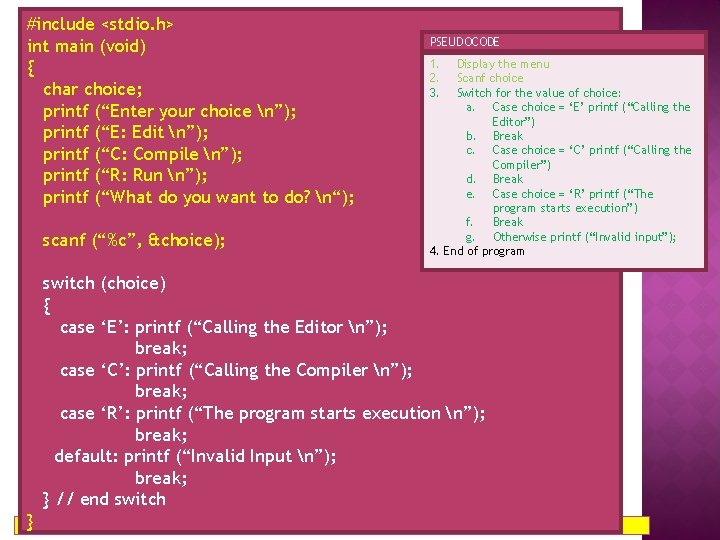
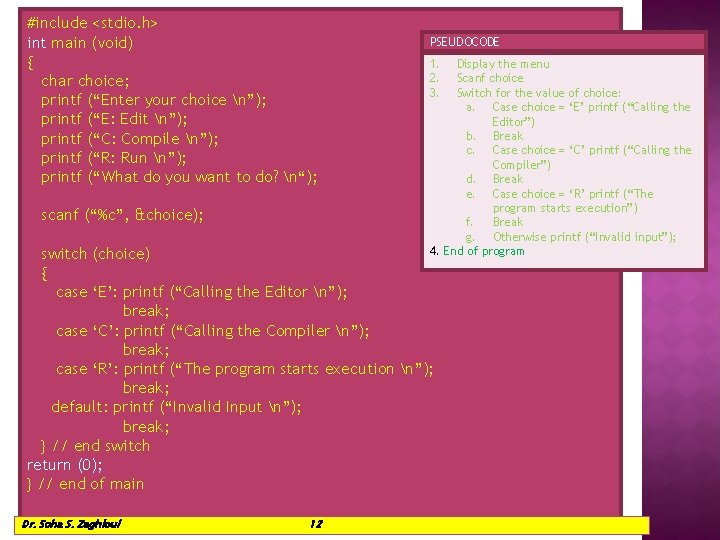
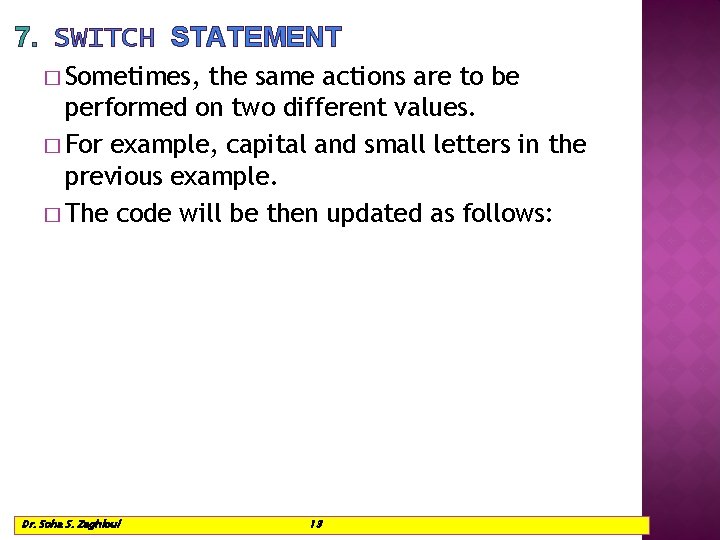
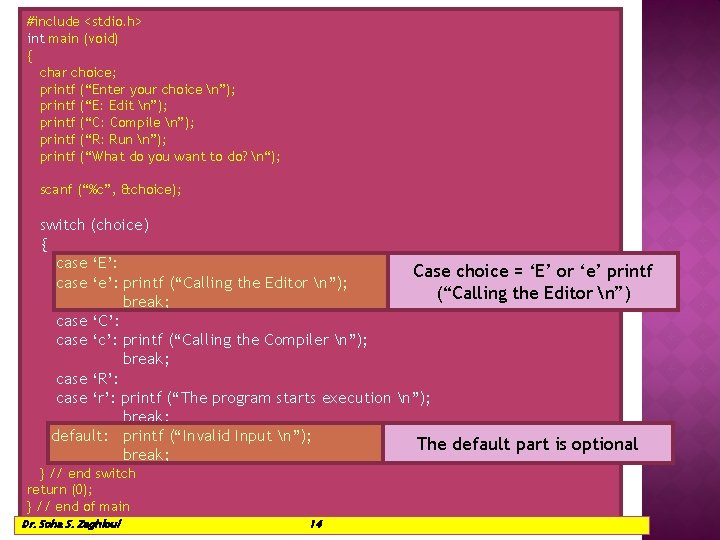
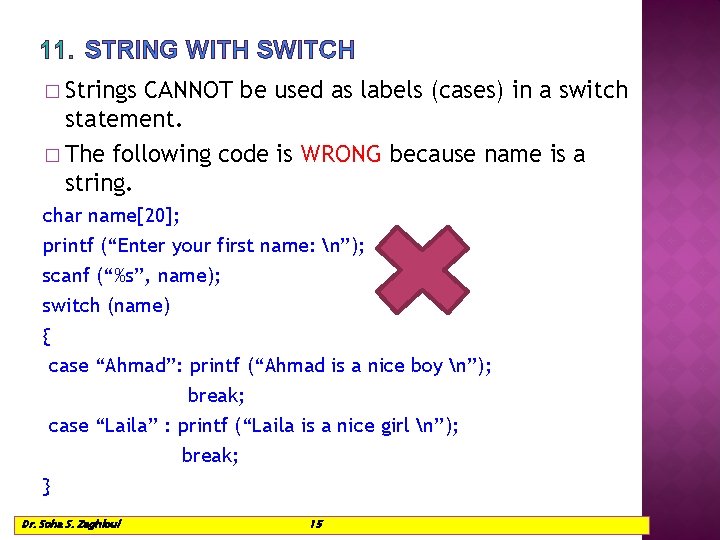
- Slides: 15
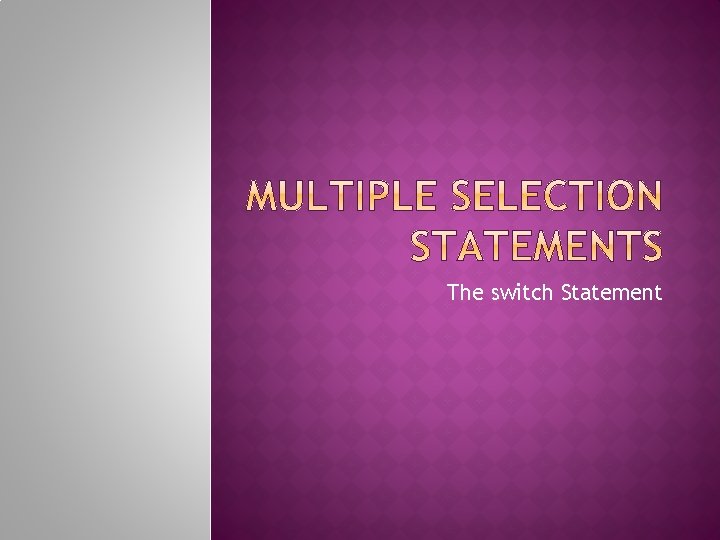
The switch Statement
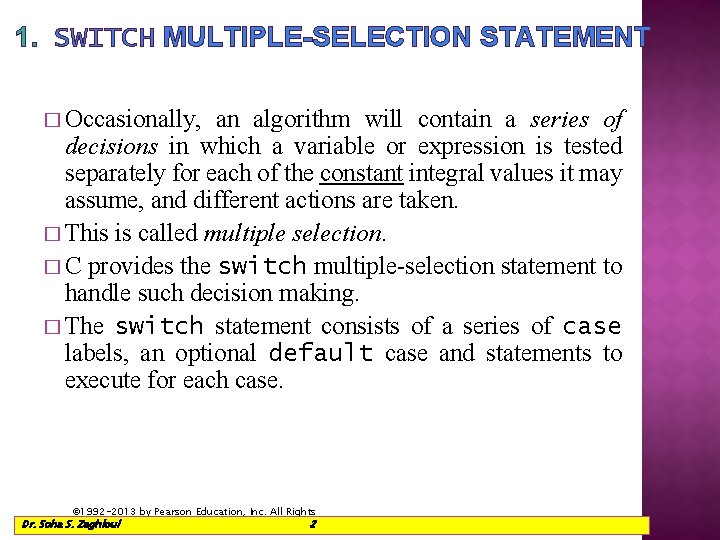
1. SWITCH MULTIPLE-SELECTION STATEMENT � Occasionally, an algorithm will contain a series of decisions in which a variable or expression is tested separately for each of the constant integral values it may assume, and different actions are taken. � This is called multiple selection. � C provides the switch multiple-selection statement to handle such decision making. � The switch statement consists of a series of case labels, an optional default case and statements to execute for each case. © 1992 -2013 by Pearson Education, Inc. All Rights Reserved. 2 Dr. Soha S. Zaghloul
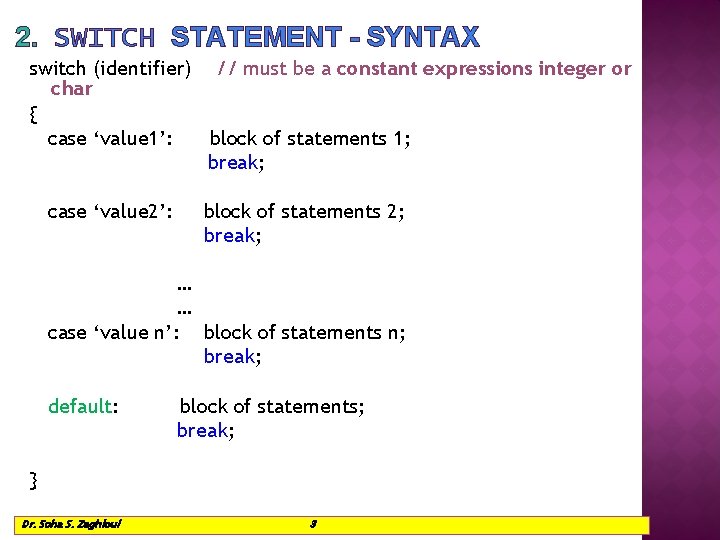
2. SWITCH STATEMENT - SYNTAX switch (identifier) // must be a constant expressions integer or char { case ‘value 1’: block of statements 1; break; case ‘value 2’: block of statements 2; break; … … case ‘value n’: block of statements n; break; default: block of statements; break; } Dr. Soha S. Zaghloul 3
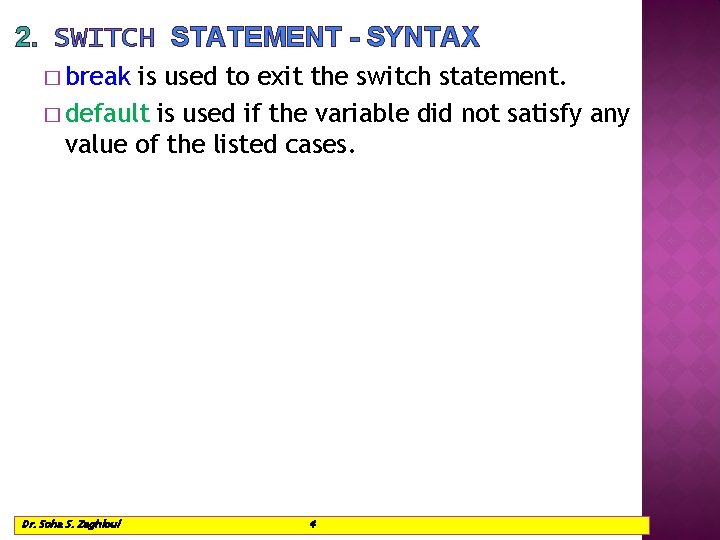
2. SWITCH STATEMENT - SYNTAX � break is used to exit the switch statement. � default is used if the variable did not satisfy any value of the listed cases. Dr. Soha S. Zaghloul 4
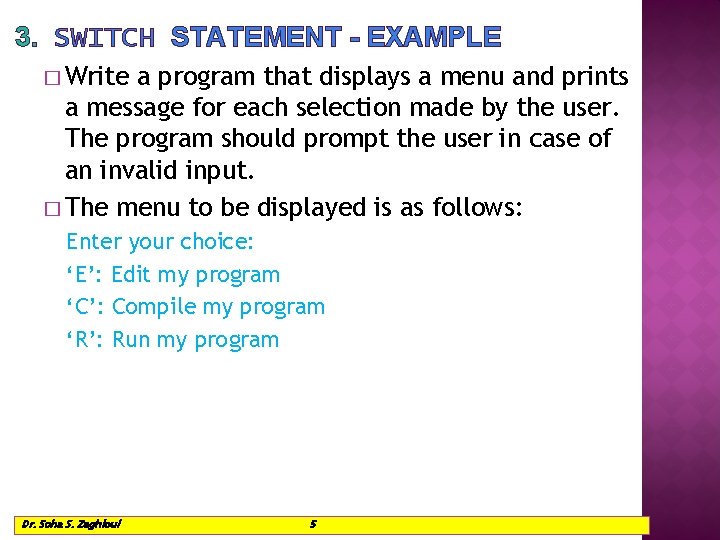
3. SWITCH STATEMENT - EXAMPLE � Write a program that displays a menu and prints a message for each selection made by the user. The program should prompt the user in case of an invalid input. � The menu to be displayed is as follows: Enter your choice: ‘E’: Edit my program ‘C’: Compile my program ‘R’: Run my program Dr. Soha S. Zaghloul 5
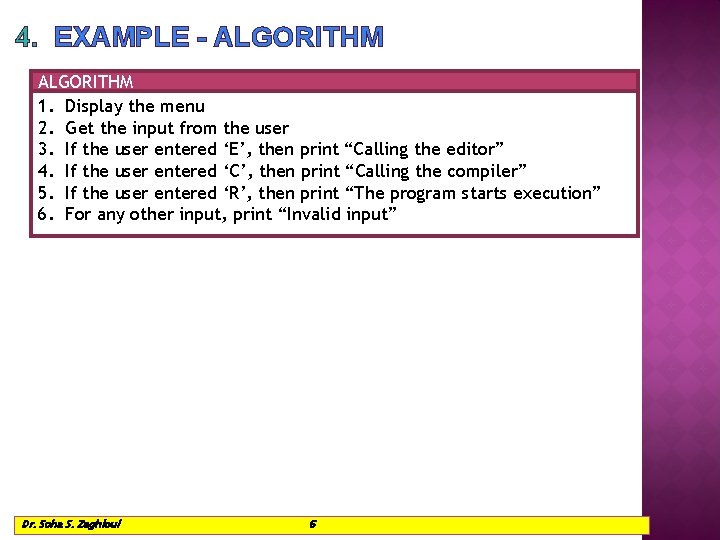
4. EXAMPLE - ALGORITHM 1. Display the menu 2. Get the input from the user 3. If the user entered ‘E’, then print “Calling the editor” 4. If the user entered ‘C’, then print “Calling the compiler” 5. If the user entered ‘R’, then print “The program starts execution” 6. For any other input, print “Invalid input” Dr. Soha S. Zaghloul 6
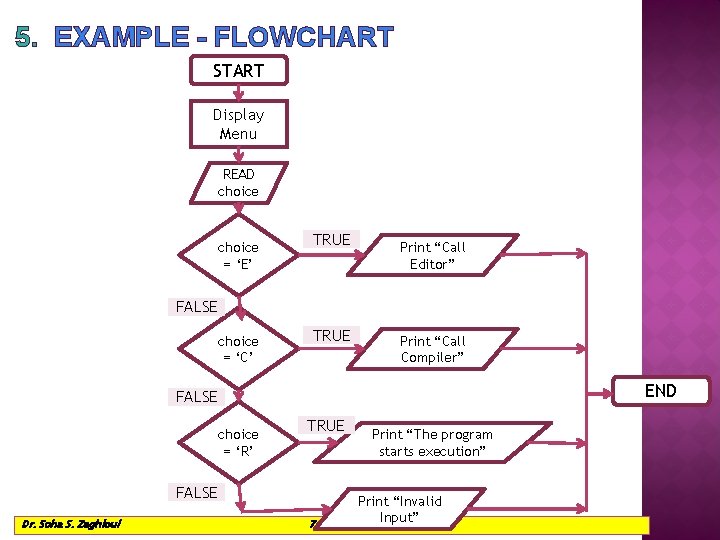
5. EXAMPLE - FLOWCHART START Display Menu READ choice = ‘E’ TRUE Print “Call Editor” FALSE choice = ‘C’ TRUE Print “Call Compiler” END FALSE choice = ‘R’ TRUE FALSE Dr. Soha S. Zaghloul 7 Print “The program starts execution” Print “Invalid Input”
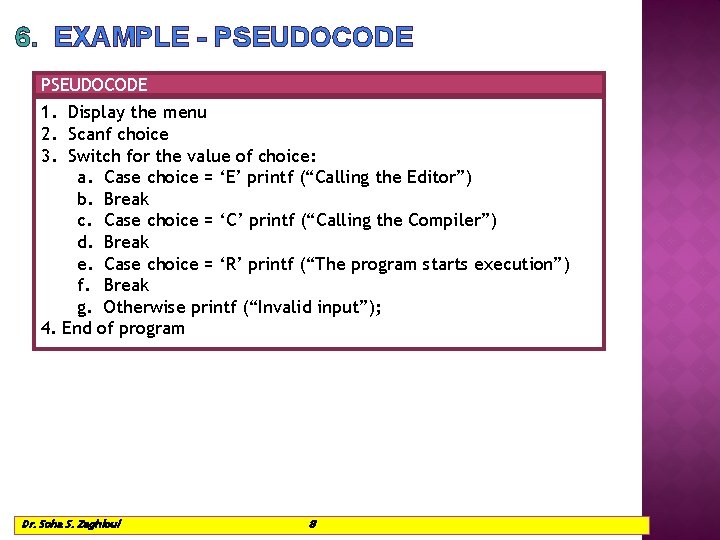
6. EXAMPLE - PSEUDOCODE 1. Display the menu 2. Scanf choice 3. Switch for the value of choice: a. Case choice = ‘E’ printf (“Calling the Editor”) b. Break c. Case choice = ‘C’ printf (“Calling the Compiler”) d. Break e. Case choice = ‘R’ printf (“The program starts execution”) f. Break g. Otherwise printf (“Invalid input”); 4. End of program Dr. Soha S. Zaghloul 8
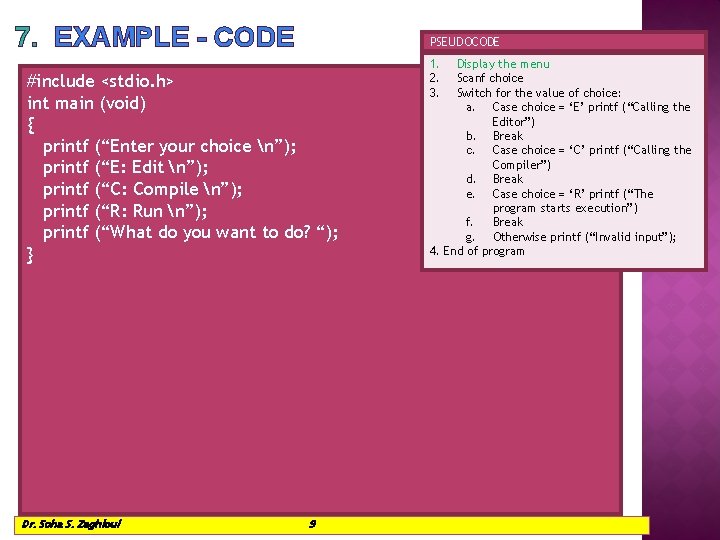
7. EXAMPLE - CODE PSEUDOCODE #include <stdio. h> int main (void) { printf (“Enter your choice n”); printf (“E: Edit n”); printf (“C: Compile n”); printf (“R: Run n”); printf (“What do you want to do? “); } Dr. Soha S. Zaghloul 9 1. 2. 3. Display the menu Scanf choice Switch for the value of choice: a. Case choice = ‘E’ printf (“Calling the Editor”) b. Break c. Case choice = ‘C’ printf (“Calling the Compiler”) d. Break e. Case choice = ‘R’ printf (“The program starts execution”) f. Break g. Otherwise printf (“Invalid input”); 4. End of program
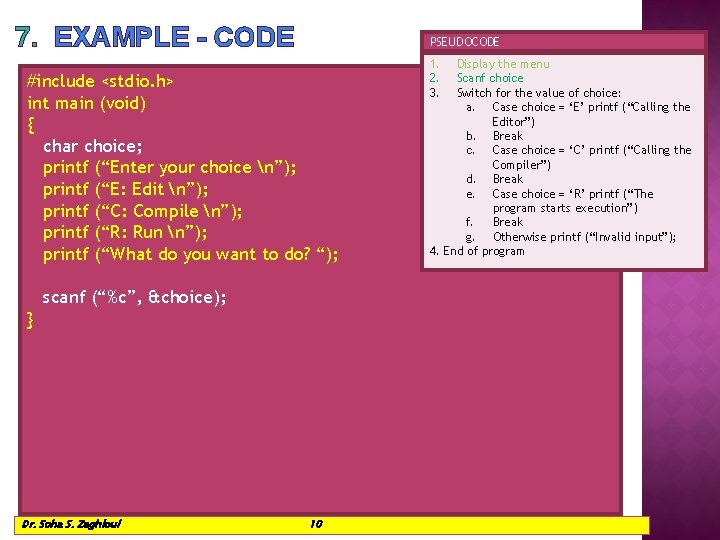
7. EXAMPLE - CODE PSEUDOCODE #include <stdio. h> int main (void) { char choice; printf (“Enter your choice n”); printf (“E: Edit n”); printf (“C: Compile n”); printf (“R: Run n”); printf (“What do you want to do? “); scanf (“%c”, &choice); } Dr. Soha S. Zaghloul 10 1. 2. 3. Display the menu Scanf choice Switch for the value of choice: a. Case choice = ‘E’ printf (“Calling the Editor”) b. Break c. Case choice = ‘C’ printf (“Calling the Compiler”) d. Break e. Case choice = ‘R’ printf (“The program starts execution”) f. Break g. Otherwise printf (“Invalid input”); 4. End of program
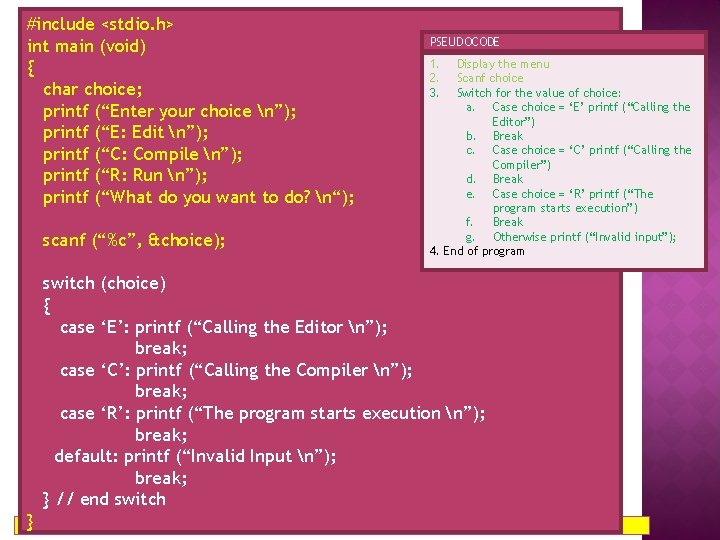
#include <stdio. h> int main (void) { char choice; printf (“Enter your choice n”); printf (“E: Edit n”); printf (“C: Compile n”); printf (“R: Run n”); printf (“What do you want to do? n“); scanf (“%c”, &choice); PSEUDOCODE 1. 2. 3. Display the menu Scanf choice Switch for the value of choice: a. Case choice = ‘E’ printf (“Calling the Editor”) b. Break c. Case choice = ‘C’ printf (“Calling the Compiler”) d. Break e. Case choice = ‘R’ printf (“The program starts execution”) f. Break g. Otherwise printf (“Invalid input”); 4. End of program switch (choice) { case ‘E’: printf (“Calling the Editor n”); break; case ‘C’: printf (“Calling the Compiler n”); break; case ‘R’: printf (“The program starts execution n”); break; default: printf (“Invalid Input n”); break; } // end switch } Dr. Soha S. Zaghloul 11
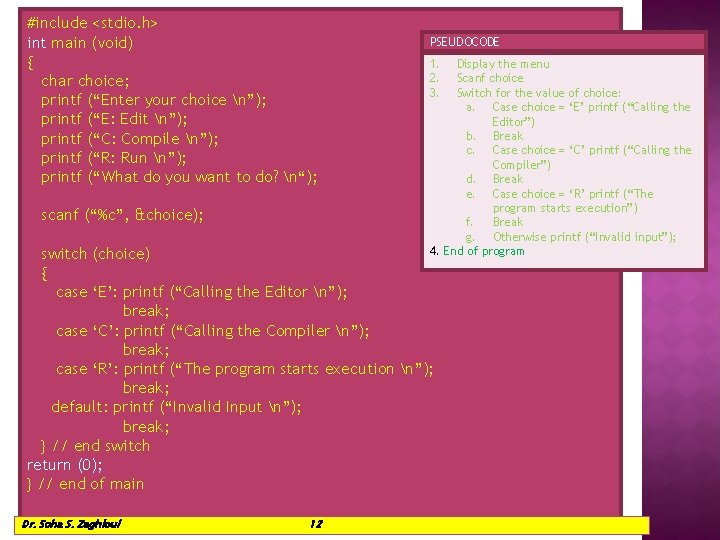
#include <stdio. h> int main (void) { char choice; printf (“Enter your choice n”); printf (“E: Edit n”); printf (“C: Compile n”); printf (“R: Run n”); printf (“What do you want to do? n“); scanf (“%c”, &choice); PSEUDOCODE 1. 2. 3. Display the menu Scanf choice Switch for the value of choice: a. Case choice = ‘E’ printf (“Calling the Editor”) b. Break c. Case choice = ‘C’ printf (“Calling the Compiler”) d. Break e. Case choice = ‘R’ printf (“The program starts execution”) f. Break g. Otherwise printf (“Invalid input”); 4. End of program switch (choice) { case ‘E’: printf (“Calling the Editor n”); break; case ‘C’: printf (“Calling the Compiler n”); break; case ‘R’: printf (“The program starts execution n”); break; default: printf (“Invalid Input n”); break; } // end switch return (0); } // end of main Dr. Soha S. Zaghloul 12
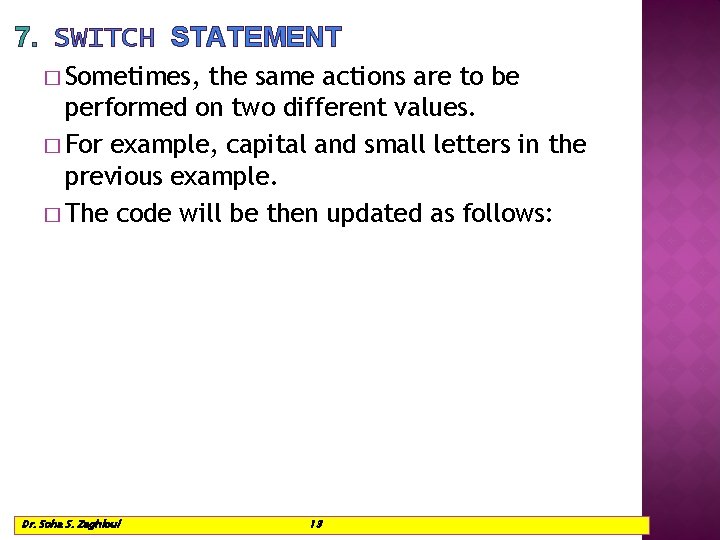
7. SWITCH STATEMENT � Sometimes, the same actions are to be performed on two different values. � For example, capital and small letters in the previous example. � The code will be then updated as follows: Dr. Soha S. Zaghloul 13
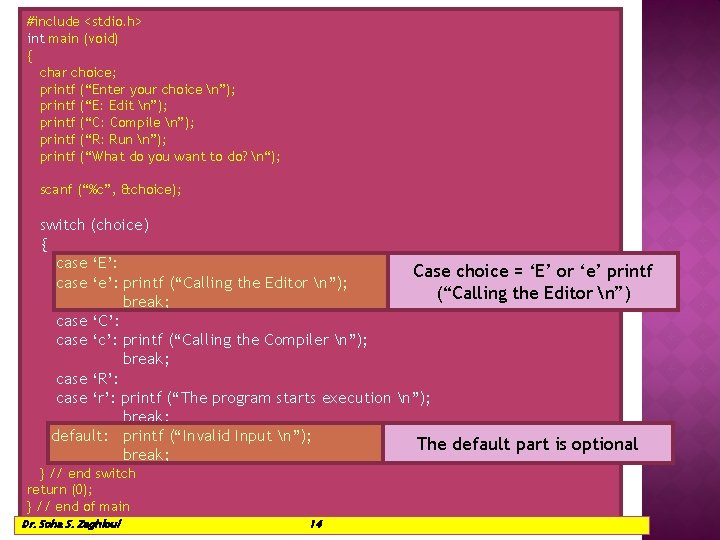
#include <stdio. h> int main (void) { char choice; printf (“Enter your choice n”); printf (“E: Edit n”); printf (“C: Compile n”); printf (“R: Run n”); printf (“What do you want to do? n“); scanf (“%c”, &choice); switch (choice) { case ‘E’: Case choice = ‘E’ or ‘e’ printf case ‘e’: printf (“Calling the Editor n”); (“Calling the Editor n”) break; case ‘C’: case ‘c’: printf (“Calling the Compiler n”); break; case ‘R’: case ‘r’: printf (“The program starts execution n”); break; default: printf (“Invalid Input n”); The default part is optional break; } // end switch return (0); } // end of main Dr. Soha S. Zaghloul 14
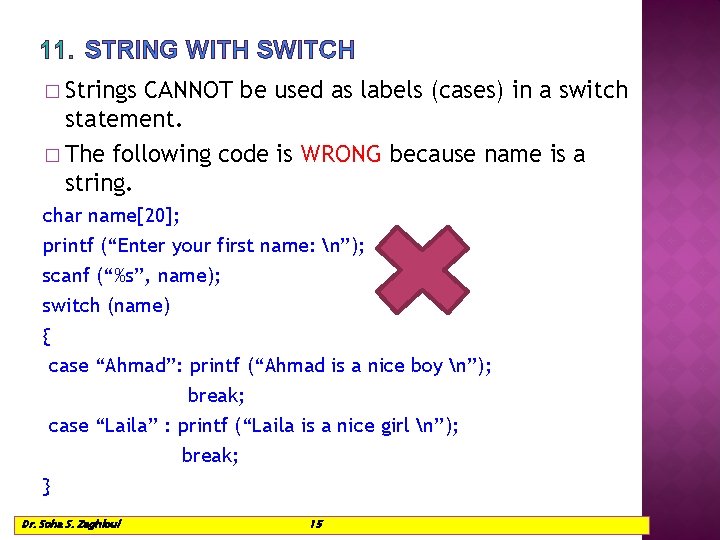
11. STRING WITH SWITCH � Strings CANNOT be used as labels (cases) in a switch statement. � The following code is WRONG because name is a string. char name[20]; printf (“Enter your first name: n”); scanf (“%s”, name); switch (name) { case “Ahmad”: printf (“Ahmad is a nice boy n”); break; case “Laila” : printf (“Laila is a nice girl n”); break; } Dr. Soha S. Zaghloul 15
Old opie occasionally tries
Old opie cranial nerves
Motor efferent division
Old opie occasionally tries
Opening in the earth's crust where magma occasionally exits
The resources need to be reserved during the setup phase in
Go switch model 11
Từ ngữ thể hiện lòng nhân hậu
Hổ đẻ mỗi lứa mấy con
Diễn thế sinh thái là
Vẽ hình chiếu vuông góc của vật thể sau
Ng-html
Ví dụ về giọng cùng tên
101012 bằng
Lời thề hippocrates
Thang điểm glasgow