Synthesis Analysis and Verification Lecture 05 a Programs
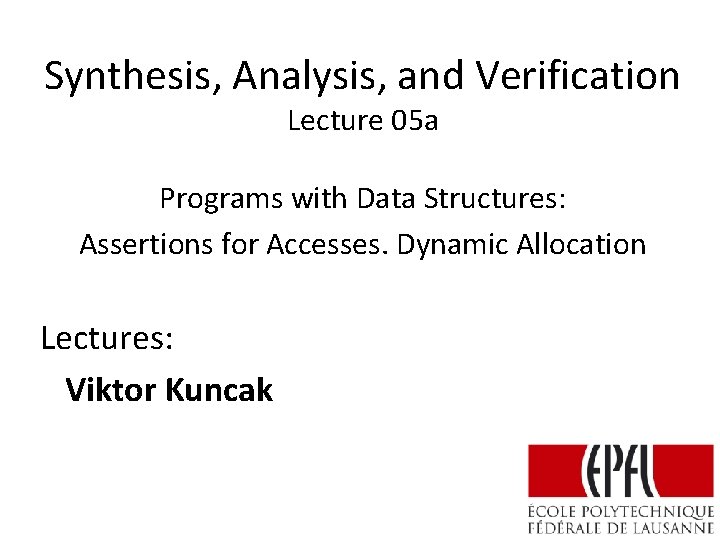
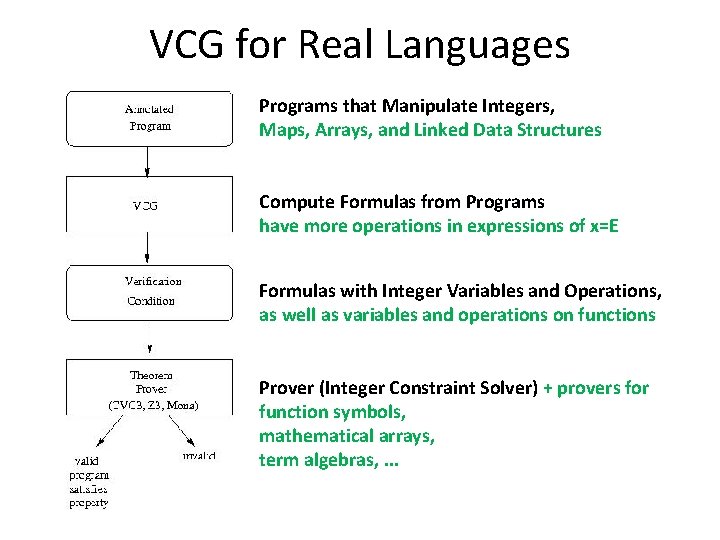
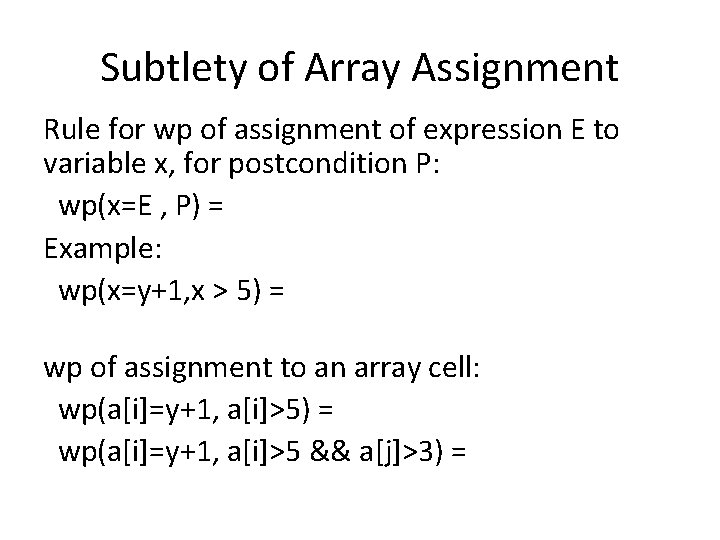
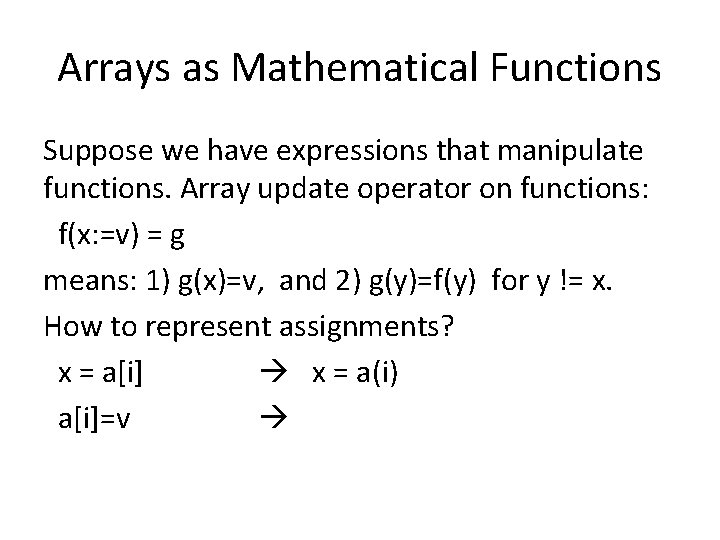
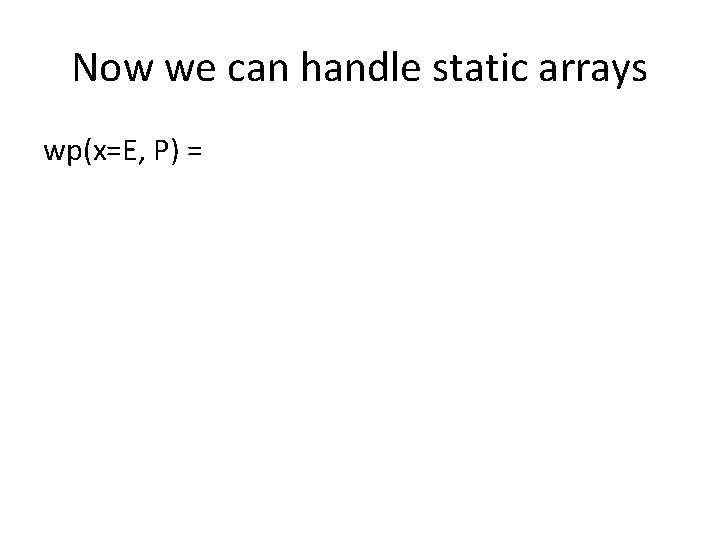
![Example with Static Arrays if (a[i] > 0) { b[k]= b[k] + a[i]; i= Example with Static Arrays if (a[i] > 0) { b[k]= b[k] + a[i]; i=](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-6.jpg)
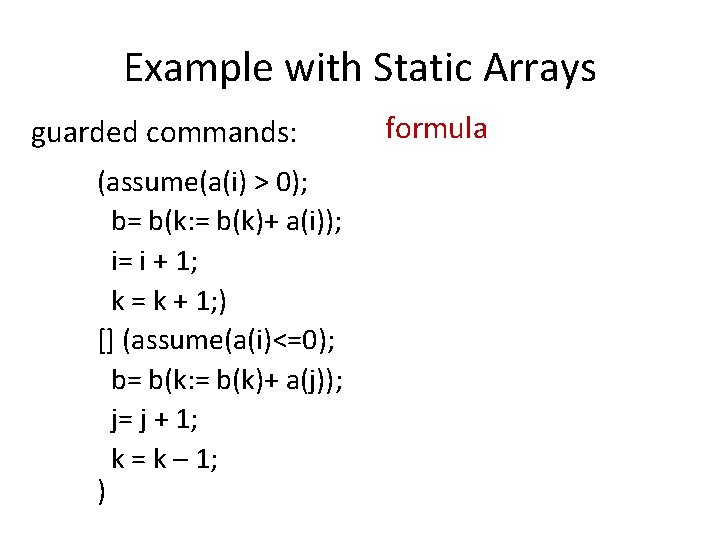
![Array Bounds Checks: Index >= 0 if (a[i] > 0) { b[k]= b[k] + Array Bounds Checks: Index >= 0 if (a[i] > 0) { b[k]= b[k] +](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-8.jpg)
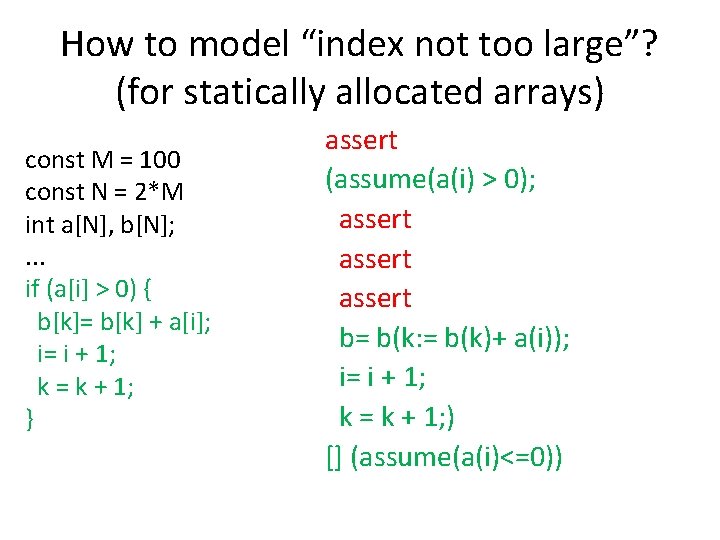
![Guarded Command Translation of Array Manipulations - with Bounds Checks x= a[i] assert(0 <= Guarded Command Translation of Array Manipulations - with Bounds Checks x= a[i] assert(0 <=](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-10.jpg)
![Checking Assertions const M = 100; const N = 2*M; int a[N], b[N]; i Checking Assertions const M = 100; const N = 2*M; int a[N], b[N]; i](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-11.jpg)
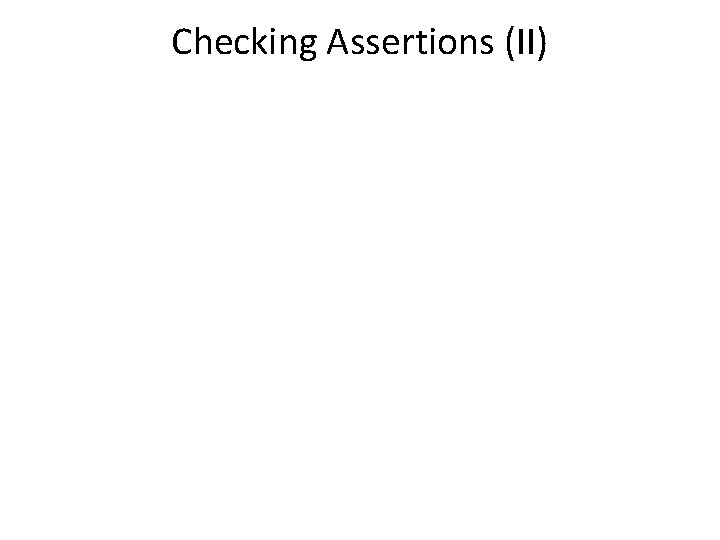
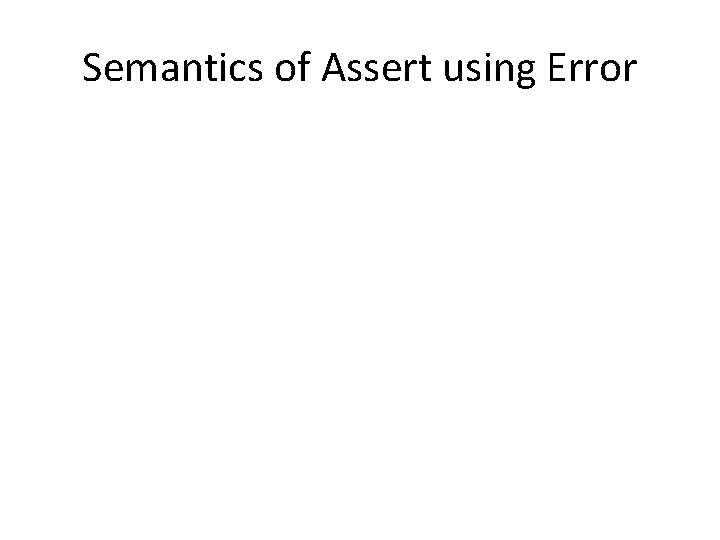
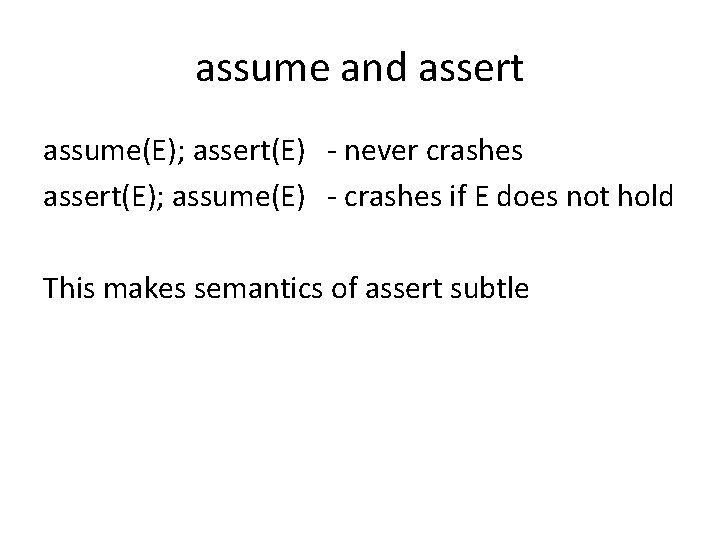
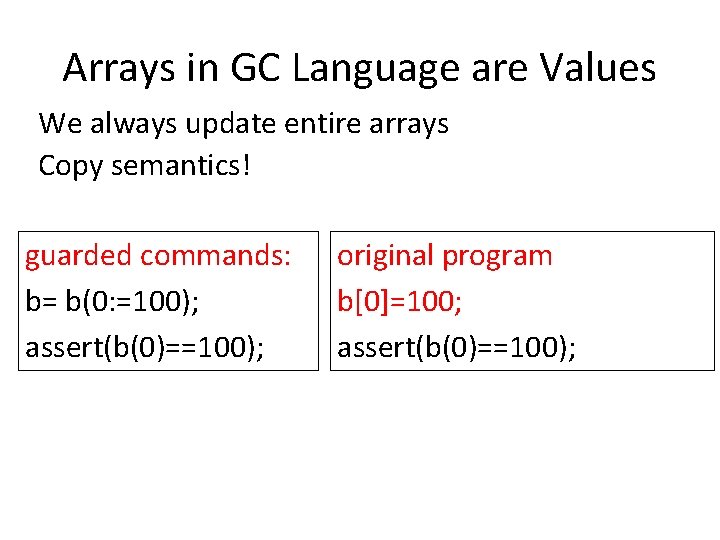
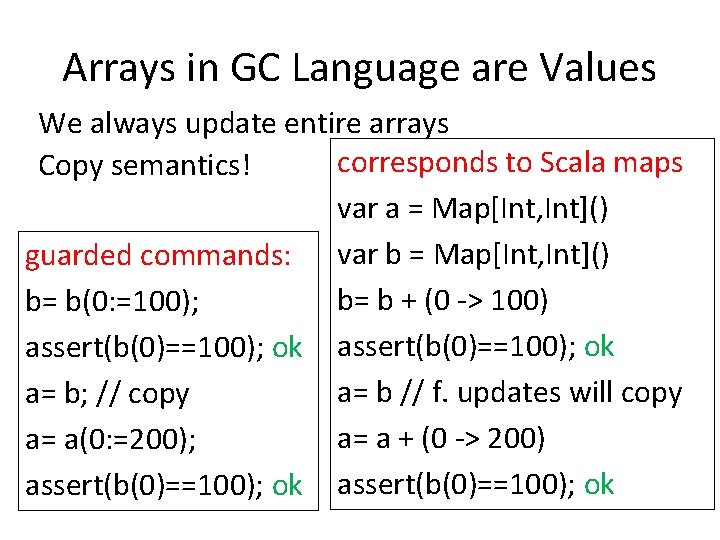
![Mutable Arrays are by Reference Java (also Scala arrays and mutable maps): b[0]= 100; Mutable Arrays are by Reference Java (also Scala arrays and mutable maps): b[0]= 100;](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-17.jpg)
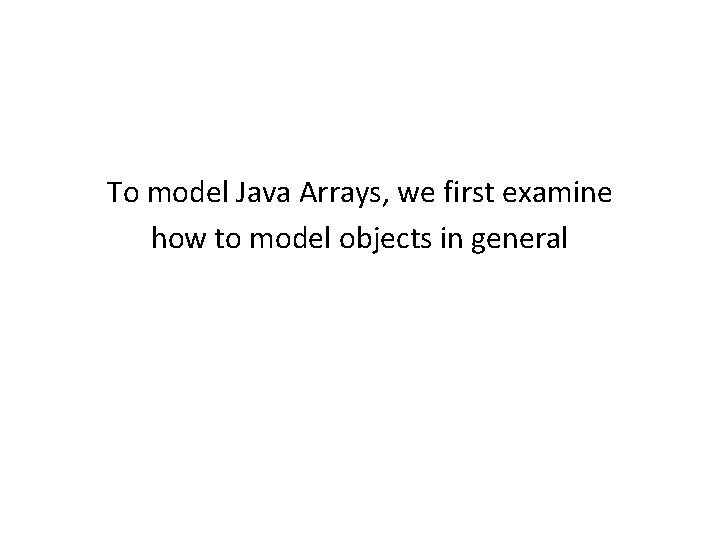
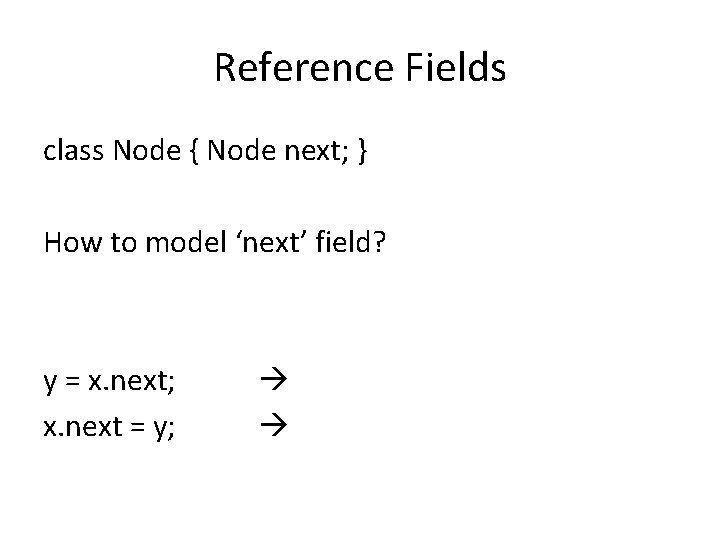
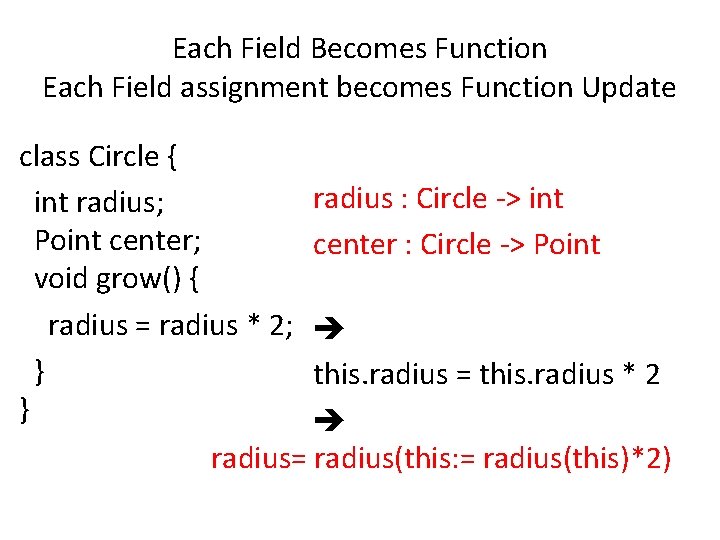
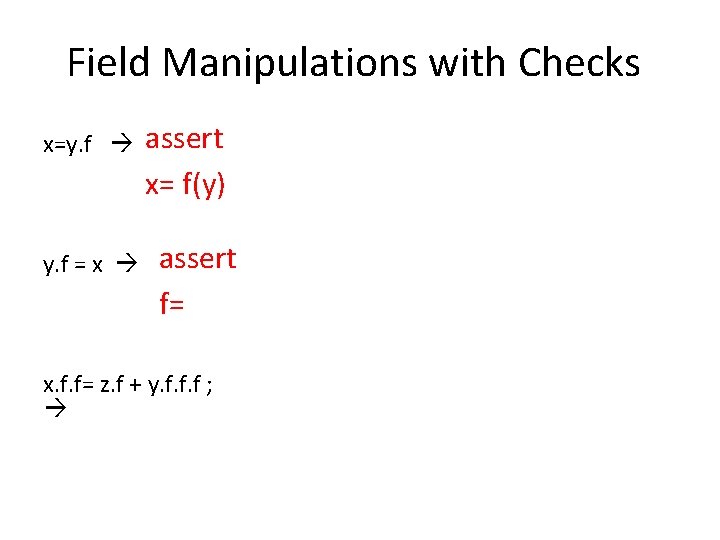
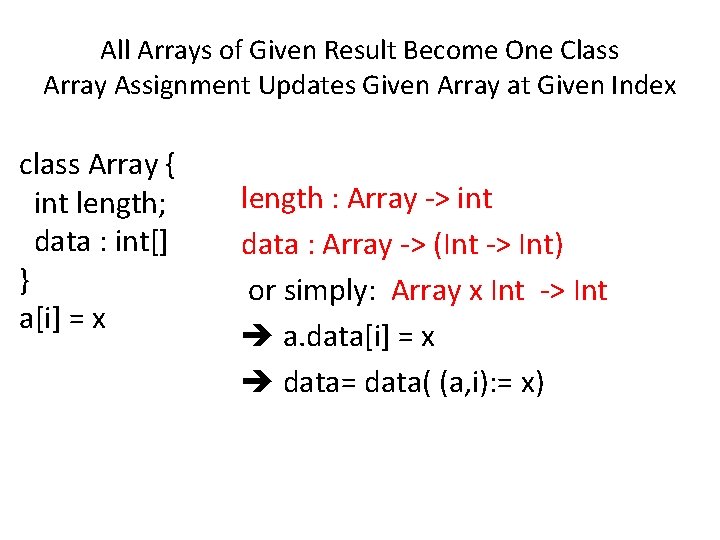
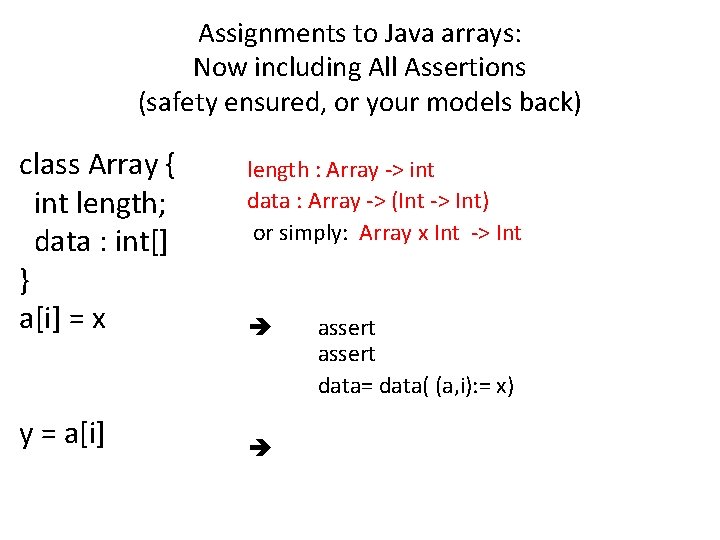
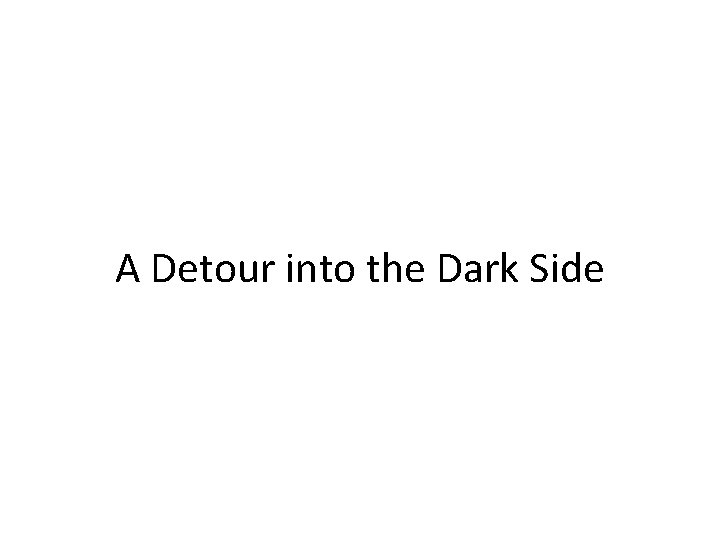
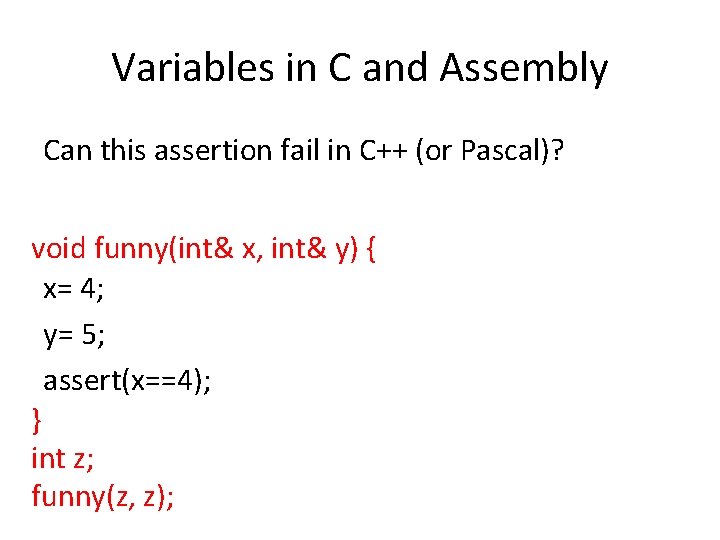
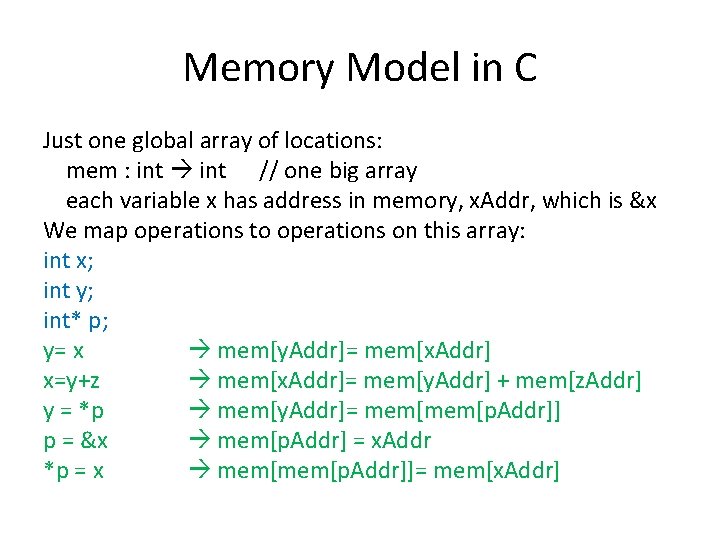
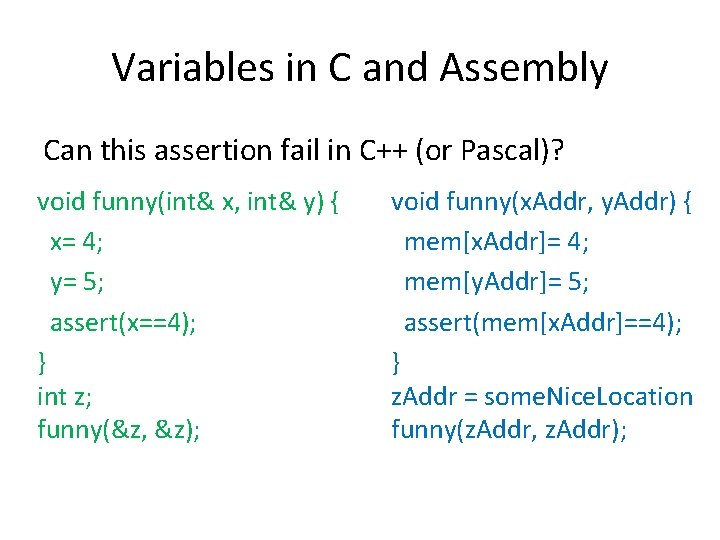
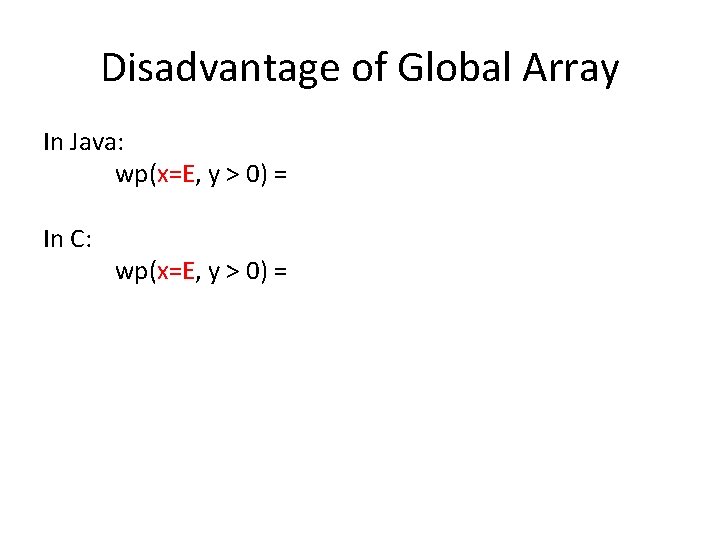
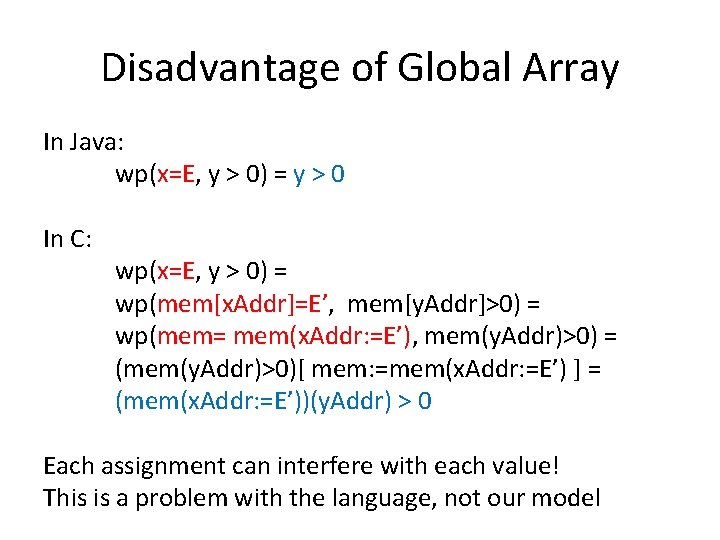
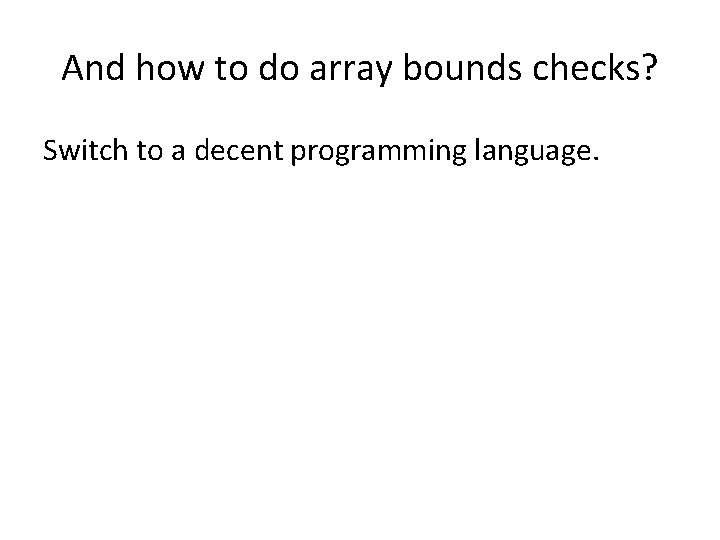
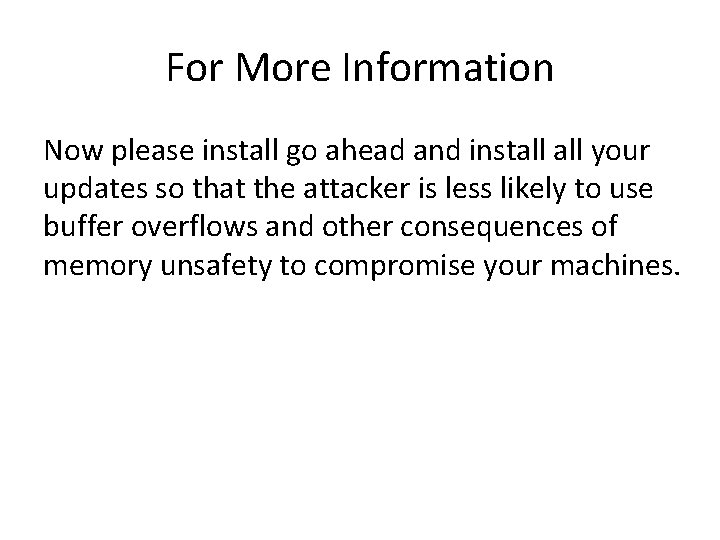
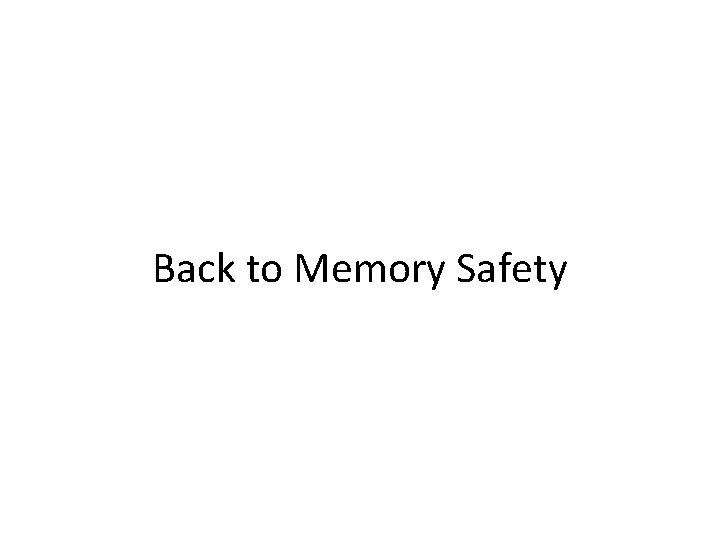
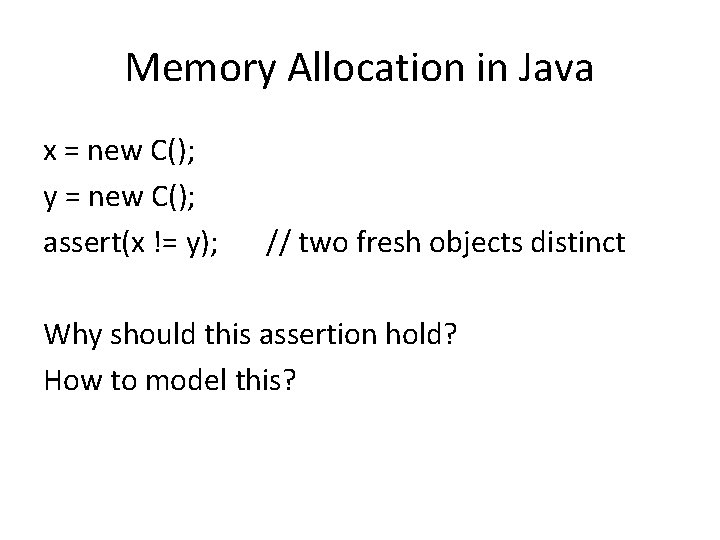
![How to represent fresh objects? assume(N > 50); a = new Object[N]; i = How to represent fresh objects? assume(N > 50); a = new Object[N]; i =](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-34.jpg)
![Alloc Set (Function) alloc : Obj Boolean i. e. alloc : Set[Obj] x = Alloc Set (Function) alloc : Obj Boolean i. e. alloc : Set[Obj] x =](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-35.jpg)
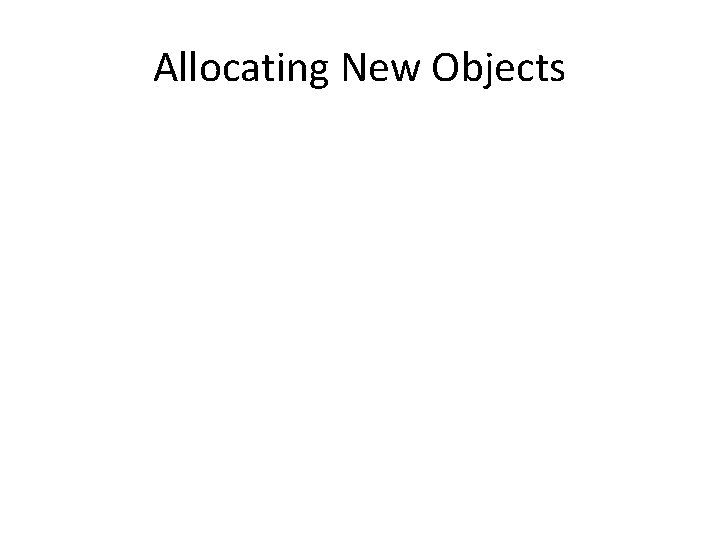
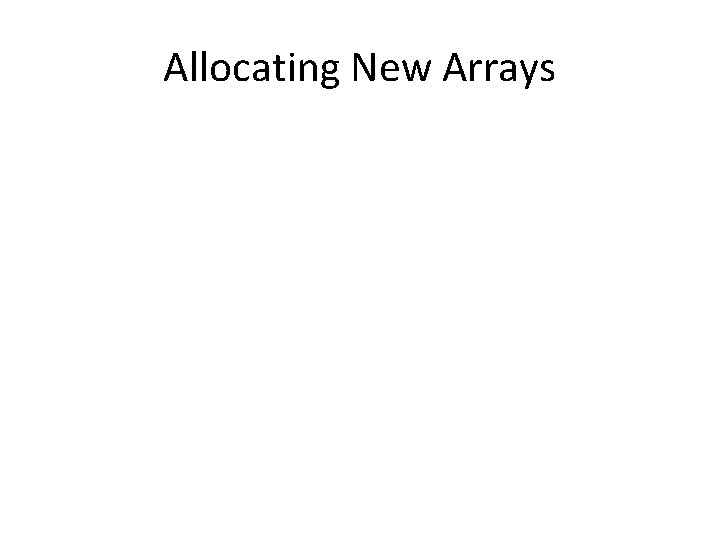
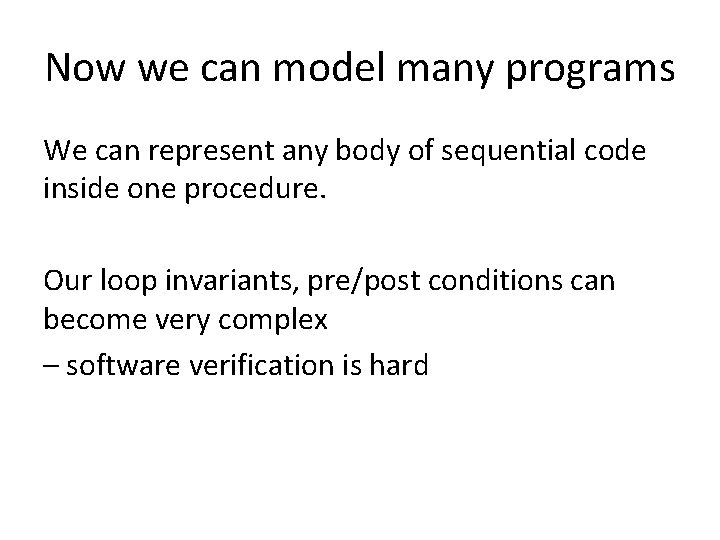
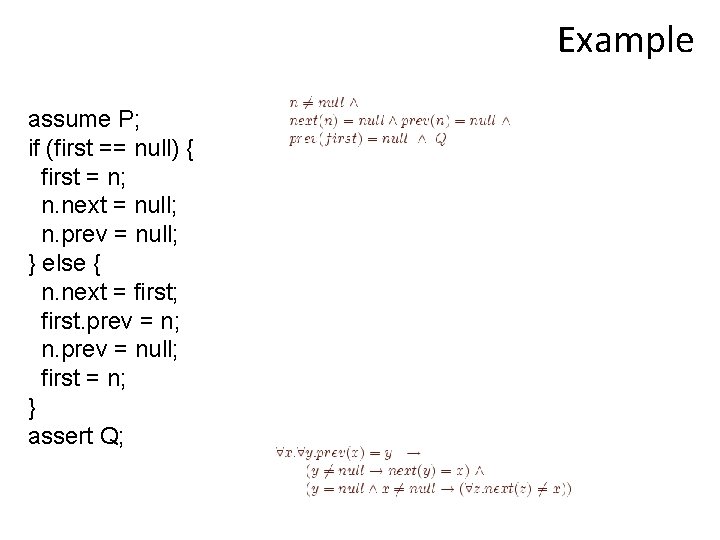
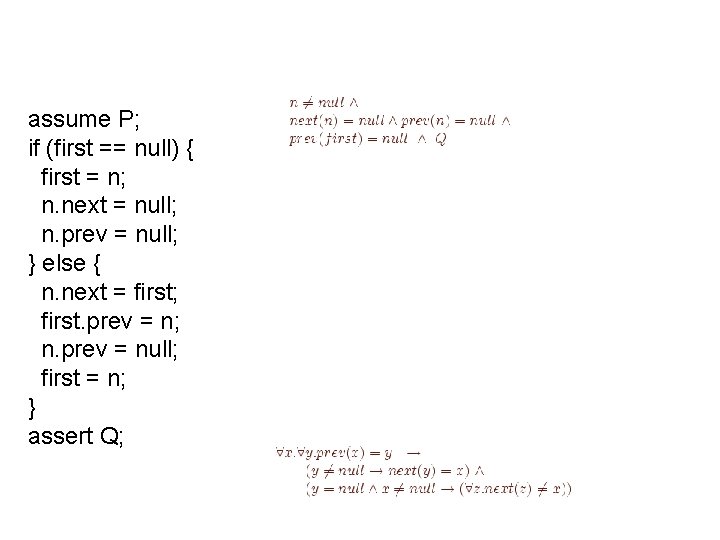
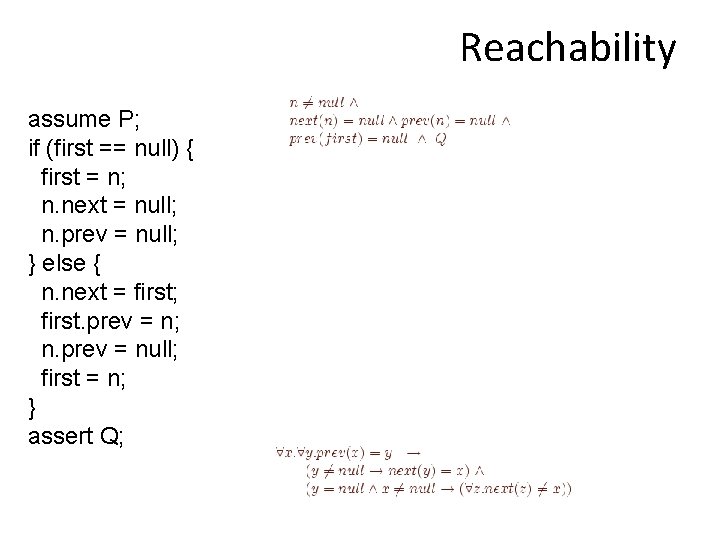
- Slides: 41
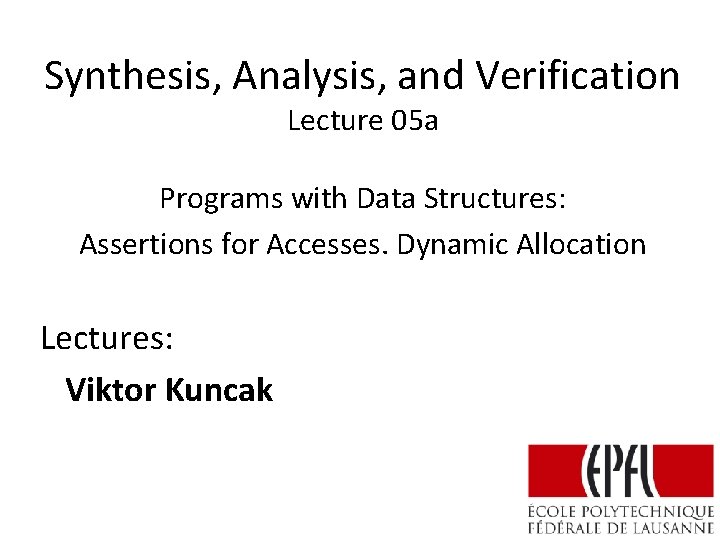
Synthesis, Analysis, and Verification Lecture 05 a Programs with Data Structures: Assertions for Accesses. Dynamic Allocation Lectures: Viktor Kuncak
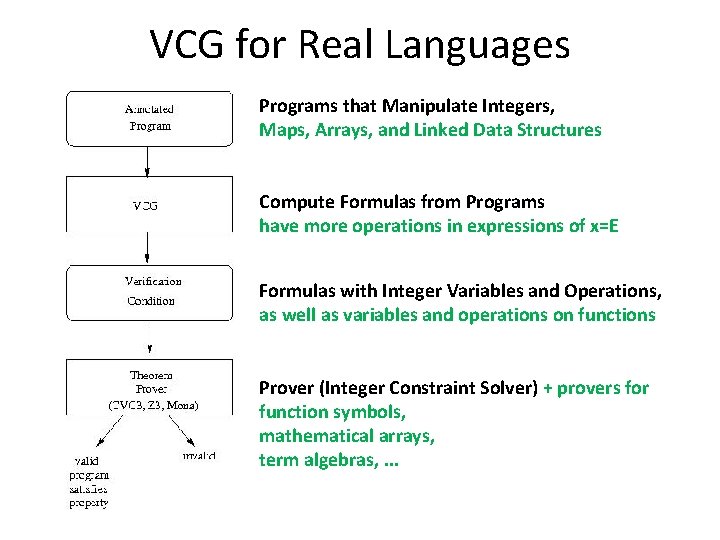
VCG for Real Languages Programs that Manipulate Integers, Maps, Arrays, and Linked Data Structures Compute Formulas from Programs have more operations in expressions of x=E Formulas with Integer Variables and Operations, as well as variables and operations on functions Prover (Integer Constraint Solver) + provers for function symbols, mathematical arrays, term algebras, . . .
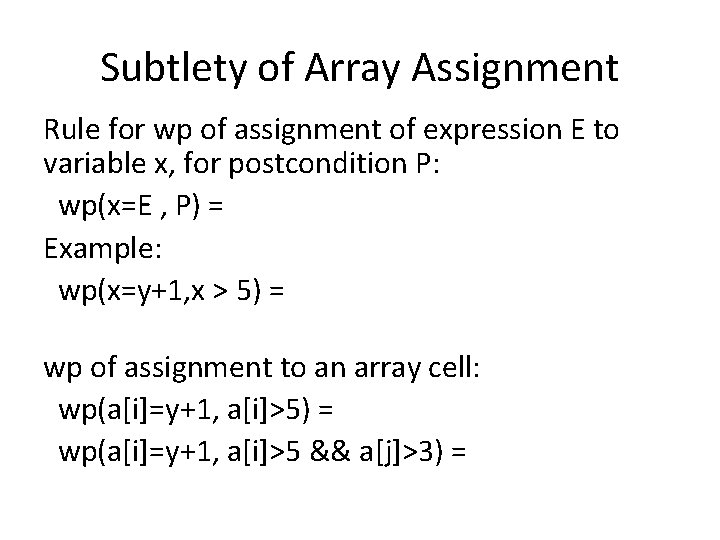
Subtlety of Array Assignment Rule for wp of assignment of expression E to variable x, for postcondition P: wp(x=E , P) = Example: wp(x=y+1, x > 5) = wp of assignment to an array cell: wp(a[i]=y+1, a[i]>5) = wp(a[i]=y+1, a[i]>5 && a[j]>3) =
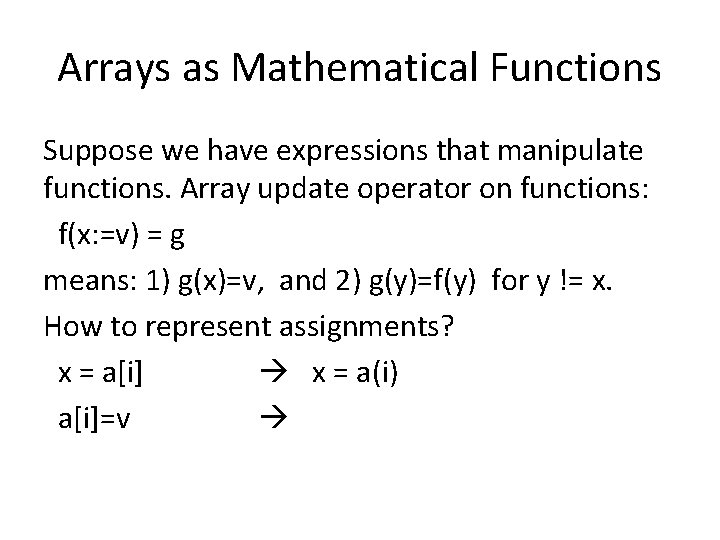
Arrays as Mathematical Functions Suppose we have expressions that manipulate functions. Array update operator on functions: f(x: =v) = g means: 1) g(x)=v, and 2) g(y)=f(y) for y != x. How to represent assignments? x = a[i] x = a(i) a[i]=v
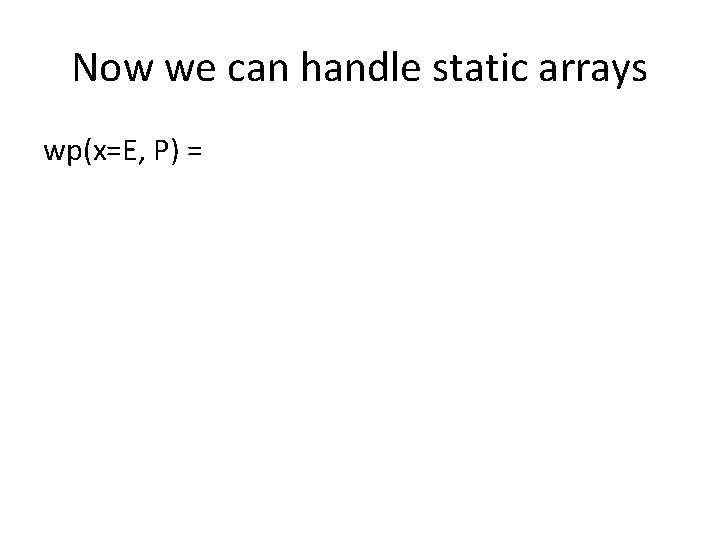
Now we can handle static arrays wp(x=E, P) =
![Example with Static Arrays if ai 0 bk bk ai i Example with Static Arrays if (a[i] > 0) { b[k]= b[k] + a[i]; i=](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-6.jpg)
Example with Static Arrays if (a[i] > 0) { b[k]= b[k] + a[i]; i= i + 1; k = k + 1; } else { b[k] = b[k] + a[j]; j= j + 1; k = k – 1; }
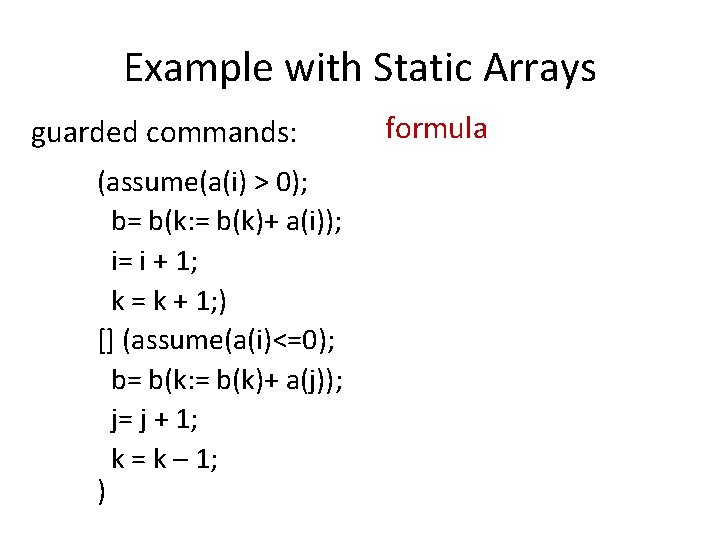
Example with Static Arrays guarded commands: (assume(a(i) > 0); b= b(k: = b(k)+ a(i)); i= i + 1; k = k + 1; ) [] (assume(a(i)<=0); b= b(k: = b(k)+ a(j)); j= j + 1; k = k – 1; ) formula
![Array Bounds Checks Index 0 if ai 0 bk bk Array Bounds Checks: Index >= 0 if (a[i] > 0) { b[k]= b[k] +](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-8.jpg)
Array Bounds Checks: Index >= 0 if (a[i] > 0) { b[k]= b[k] + a[i]; i= i + 1; k = k + 1; } else { b[k] = b[k] + a[j]; j= j + 1; k = k – 1; } assert(i >= 0) (assume(a(i) > 0); assert b= b(k: = b(k)+ a(i)); i= i + 1; k = k + 1; ) [] (assume(a(i)<=0); assert b= b(k: = b(k)+ a(j)); j= j + 1; k = k – 1; )
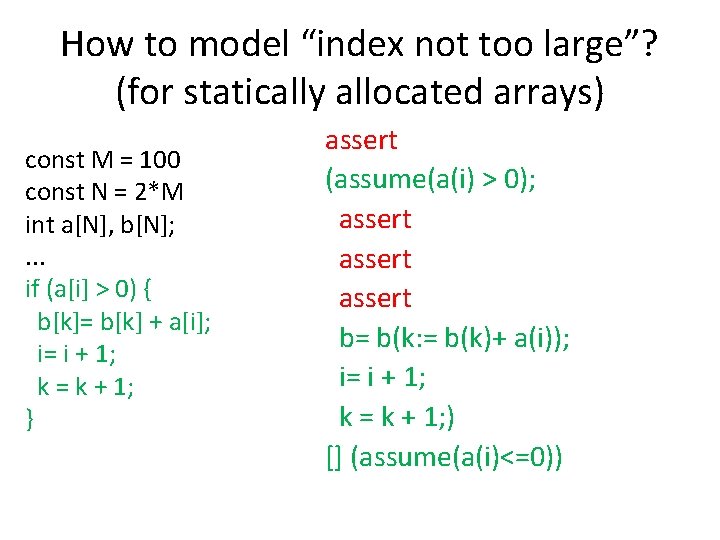
How to model “index not too large”? (for statically allocated arrays) const M = 100 const N = 2*M int a[N], b[N]; . . . if (a[i] > 0) { b[k]= b[k] + a[i]; i= i + 1; k = k + 1; } assert (assume(a(i) > 0); assert b= b(k: = b(k)+ a(i)); i= i + 1; k = k + 1; ) [] (assume(a(i)<=0))
![Guarded Command Translation of Array Manipulations with Bounds Checks x ai assert0 Guarded Command Translation of Array Manipulations - with Bounds Checks x= a[i] assert(0 <=](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-10.jpg)
Guarded Command Translation of Array Manipulations - with Bounds Checks x= a[i] assert(0 <= i); assert(i < a_size); x = a(i); a[i] = y assert(0 <= i); . . .
![Checking Assertions const M 100 const N 2M int aN bN i Checking Assertions const M = 100; const N = 2*M; int a[N], b[N]; i](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-11.jpg)
Checking Assertions const M = 100; const N = 2*M; int a[N], b[N]; i = -1; while (i < N) { i= i + 1; if (a[i] > 0) { k = k + 1; b[k]= b[k] + a[i]; } 1. Translate to guarded commands } 2. Find a loop invariant and prove it inductive 3. Show that the invariant implies assertions
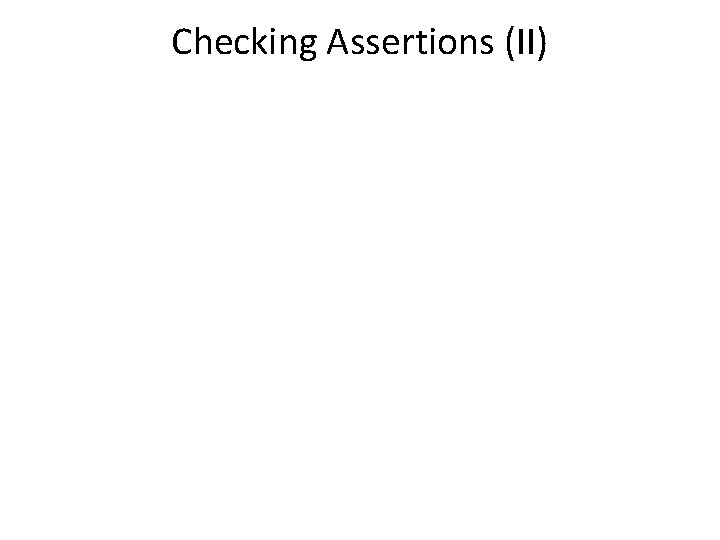
Checking Assertions (II)
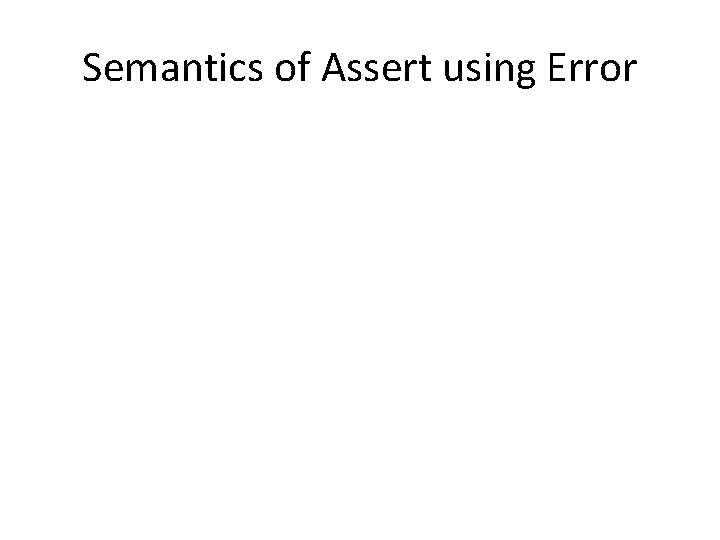
Semantics of Assert using Error
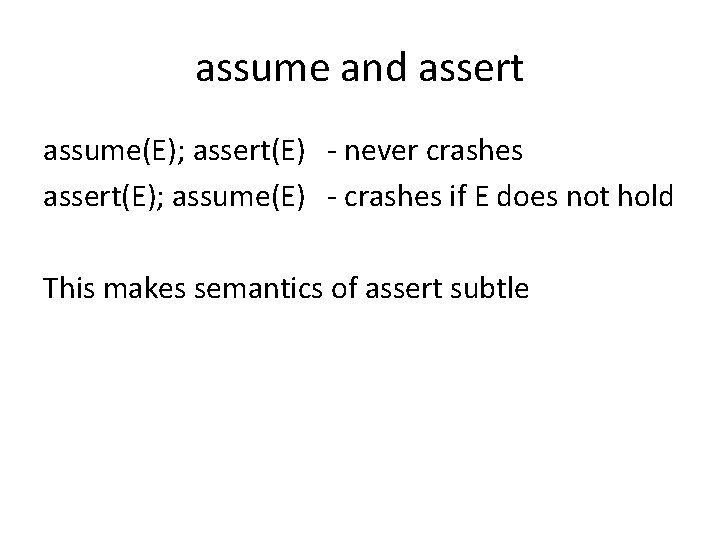
assume and assert assume(E); assert(E) - never crashes assert(E); assume(E) - crashes if E does not hold This makes semantics of assert subtle
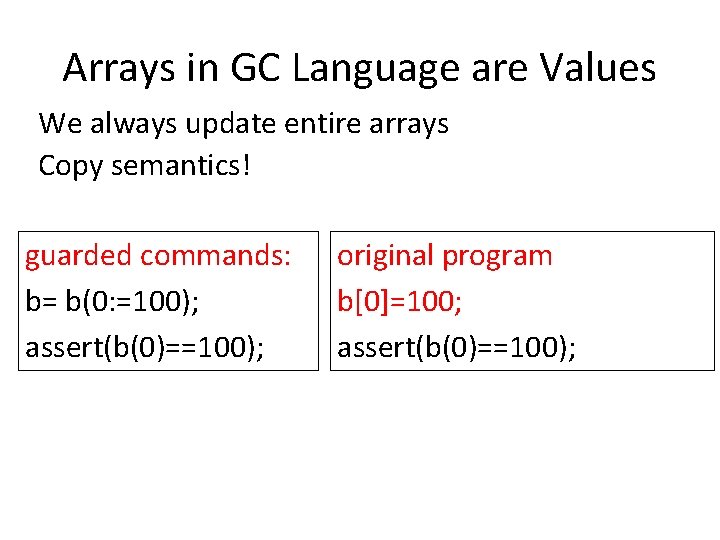
Arrays in GC Language are Values We always update entire arrays Copy semantics! guarded commands: b= b(0: =100); assert(b(0)==100); original program b[0]=100; assert(b(0)==100);
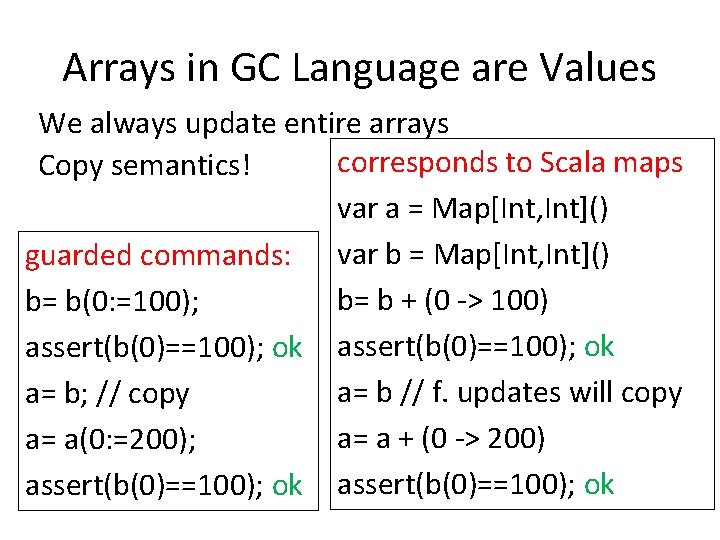
Arrays in GC Language are Values We always update entire arrays corresponds to Scala maps Copy semantics! var a = Map[Int, Int]() guarded commands: var b = Map[Int, Int]() b= b + (0 -> 100) b= b(0: =100); assert(b(0)==100); ok a= b // f. updates will copy a= b; // copy a= a + (0 -> 200) a= a(0: =200); assert(b(0)==100); ok
![Mutable Arrays are by Reference Java also Scala arrays and mutable maps b0 100 Mutable Arrays are by Reference Java (also Scala arrays and mutable maps): b[0]= 100;](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-17.jpg)
Mutable Arrays are by Reference Java (also Scala arrays and mutable maps): b[0]= 100; assert(b[0]==100); a= b; // make references point to same array a[0]= 200; assert(b[0]==100); // fails, b[0]==a[0]==200
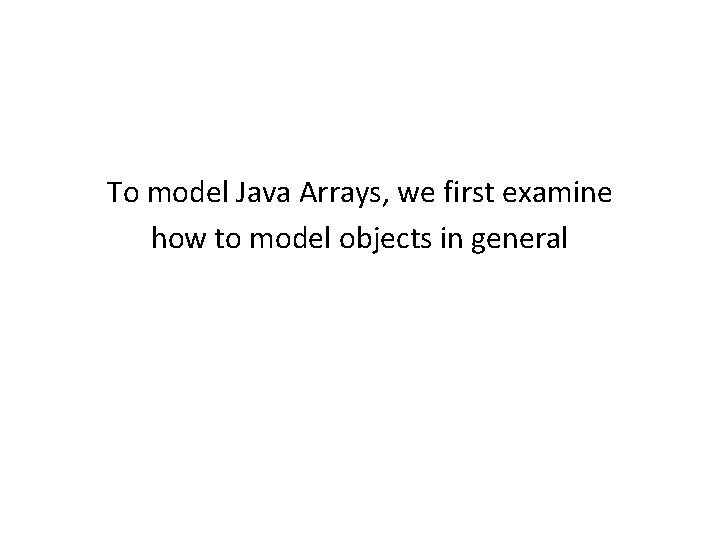
To model Java Arrays, we first examine how to model objects in general
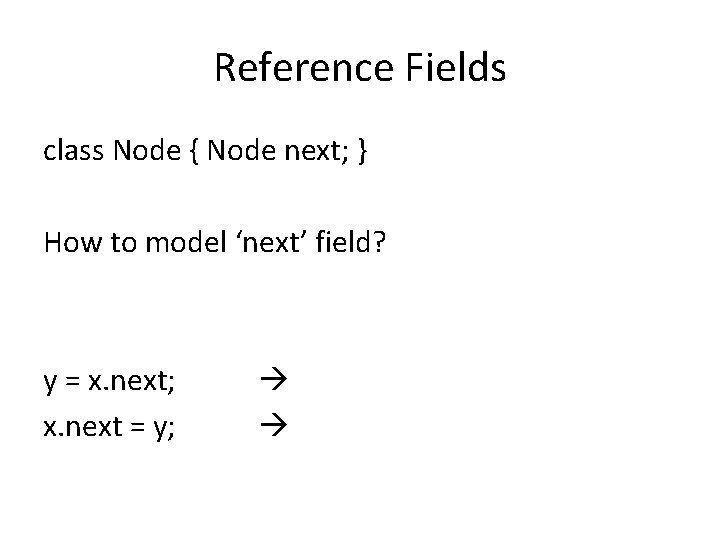
Reference Fields class Node { Node next; } How to model ‘next’ field? y = x. next; x. next = y;
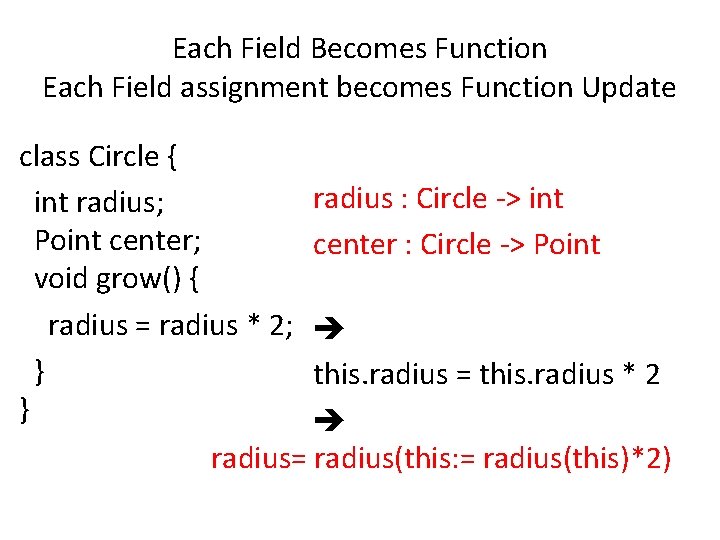
Each Field Becomes Function Each Field assignment becomes Function Update class Circle { int radius; Point center; void grow() { radius = radius * 2; } } radius : Circle -> int center : Circle -> Point this. radius = this. radius * 2 radius= radius(this: = radius(this)*2)
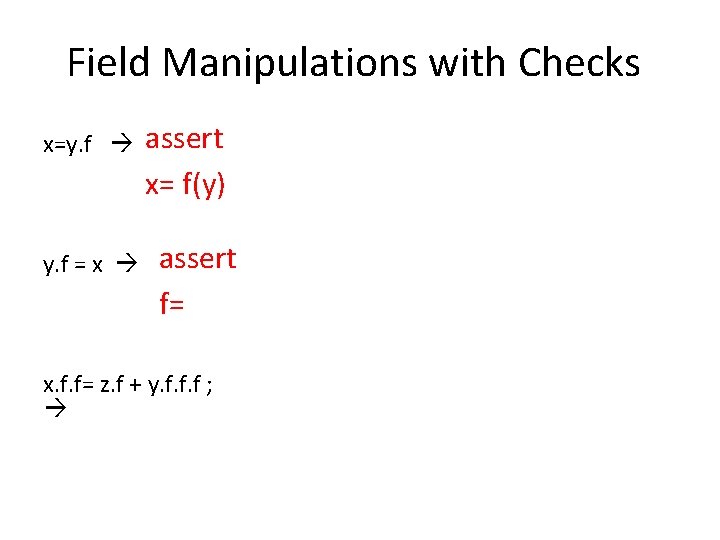
Field Manipulations with Checks x=y. f = x assert x= f(y) assert f= x. f. f= z. f + y. f. f. f ;
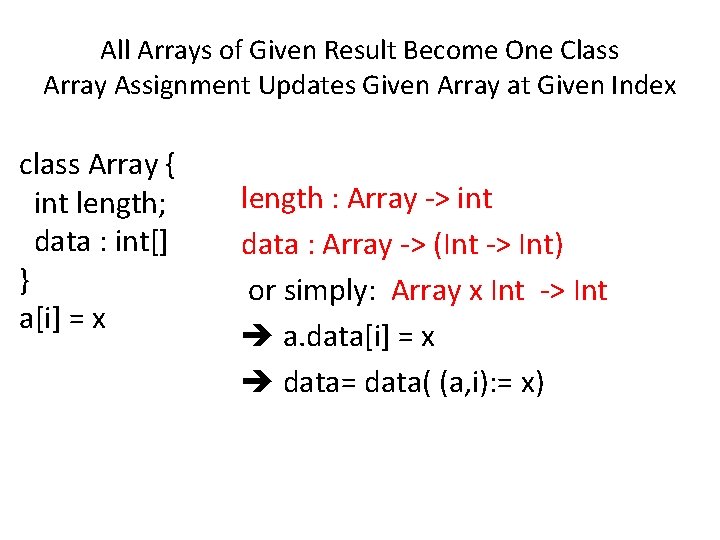
All Arrays of Given Result Become One Class Array Assignment Updates Given Array at Given Index class Array { int length; data : int[] } a[i] = x length : Array -> int data : Array -> (Int -> Int) or simply: Array x Int -> Int a. data[i] = x data= data( (a, i): = x)
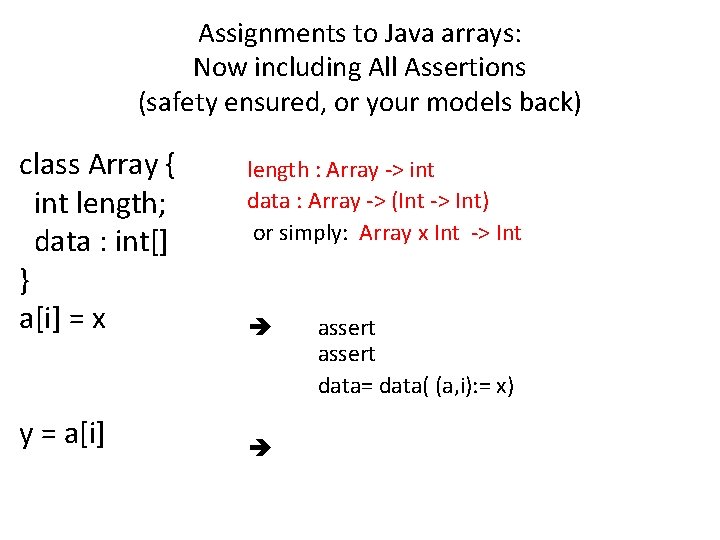
Assignments to Java arrays: Now including All Assertions (safety ensured, or your models back) class Array { int length; data : int[] } a[i] = x y = a[i] length : Array -> int data : Array -> (Int -> Int) or simply: Array x Int -> Int assert data= data( (a, i): = x)
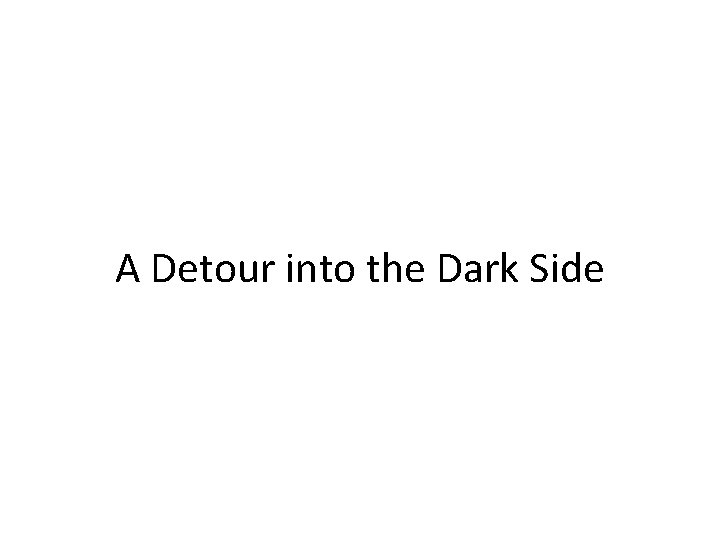
A Detour into the Dark Side
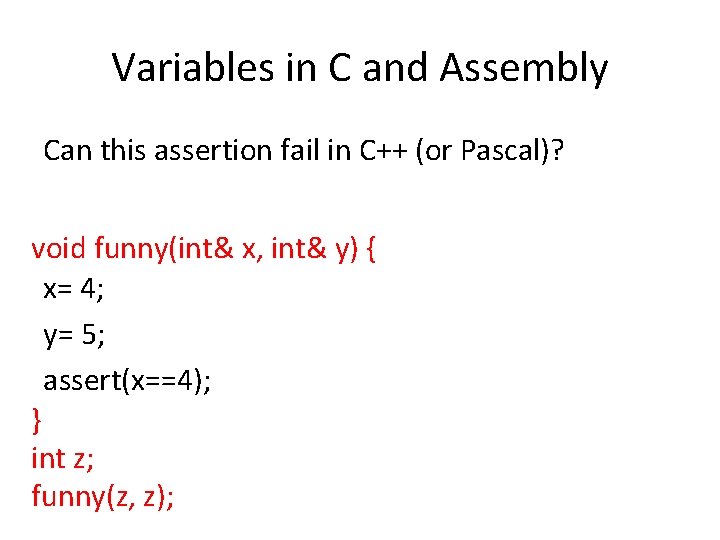
Variables in C and Assembly Can this assertion fail in C++ (or Pascal)? void funny(int& x, int& y) { x= 4; y= 5; assert(x==4); } int z; funny(z, z);
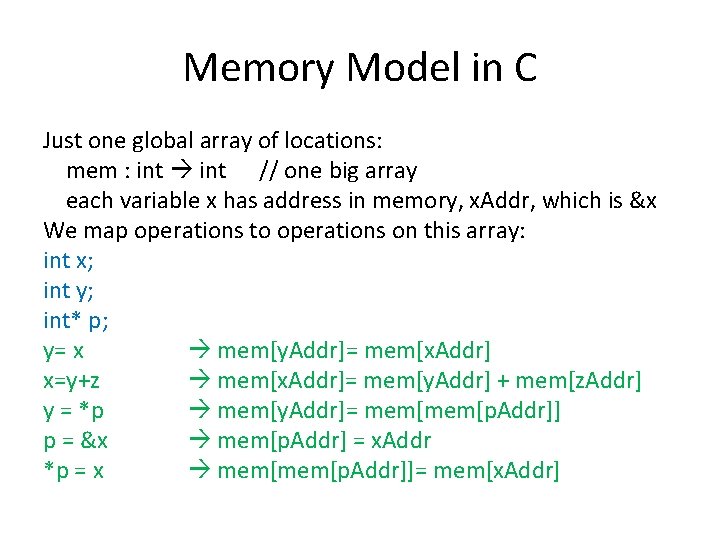
Memory Model in C Just one global array of locations: mem : int // one big array each variable x has address in memory, x. Addr, which is &x We map operations to operations on this array: int x; int y; int* p; y= x mem[y. Addr]= mem[x. Addr] x=y+z mem[x. Addr]= mem[y. Addr] + mem[z. Addr] y = *p mem[y. Addr]= mem[p. Addr]] p = &x mem[p. Addr] = x. Addr *p = x mem[p. Addr]]= mem[x. Addr]
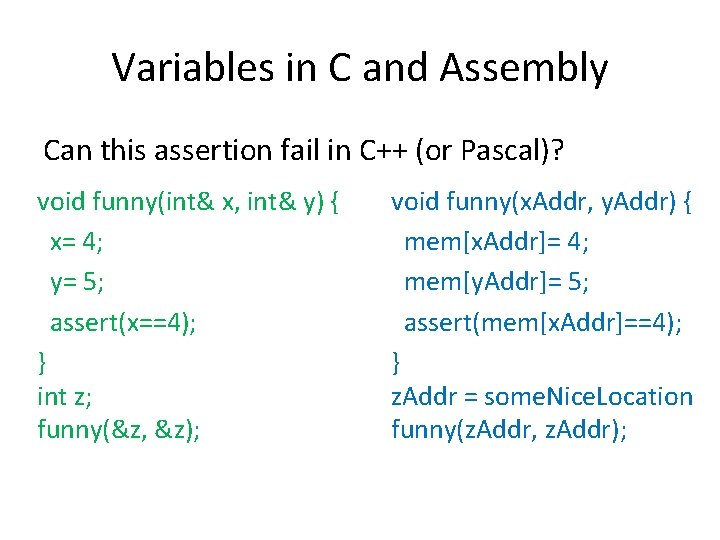
Variables in C and Assembly Can this assertion fail in C++ (or Pascal)? void funny(int& x, int& y) { x= 4; y= 5; assert(x==4); } int z; funny(&z, &z); void funny(x. Addr, y. Addr) { mem[x. Addr]= 4; mem[y. Addr]= 5; assert(mem[x. Addr]==4); } z. Addr = some. Nice. Location funny(z. Addr, z. Addr);
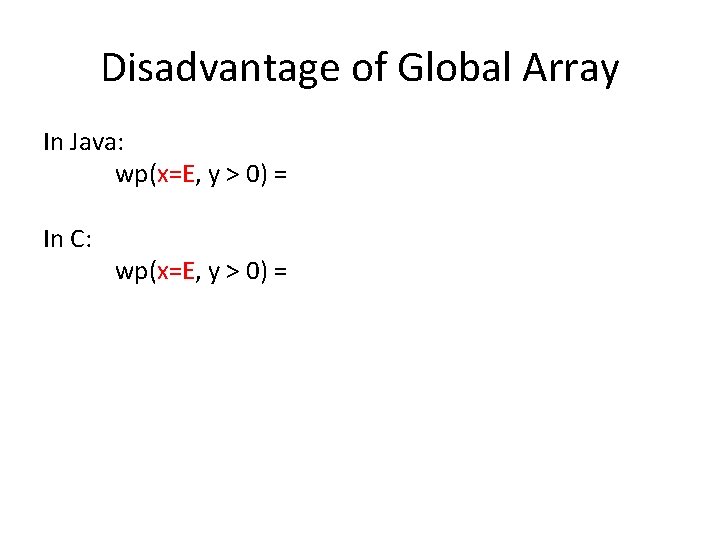
Disadvantage of Global Array In Java: wp(x=E, y > 0) = In C: wp(x=E, y > 0) =
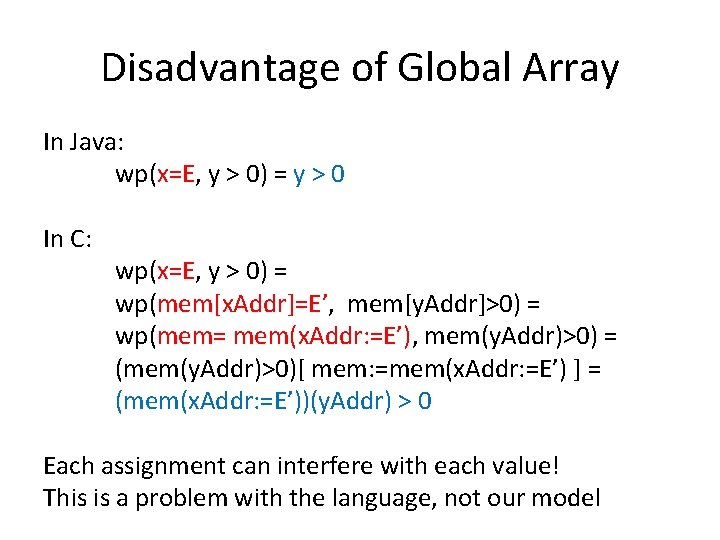
Disadvantage of Global Array In Java: wp(x=E, y > 0) = y > 0 In C: wp(x=E, y > 0) = wp(mem[x. Addr]=E’, mem[y. Addr]>0) = wp(mem= mem(x. Addr: =E’), mem(y. Addr)>0) = (mem(y. Addr)>0)[ mem: =mem(x. Addr: =E’) ] = (mem(x. Addr: =E’))(y. Addr) > 0 Each assignment can interfere with each value! This is a problem with the language, not our model
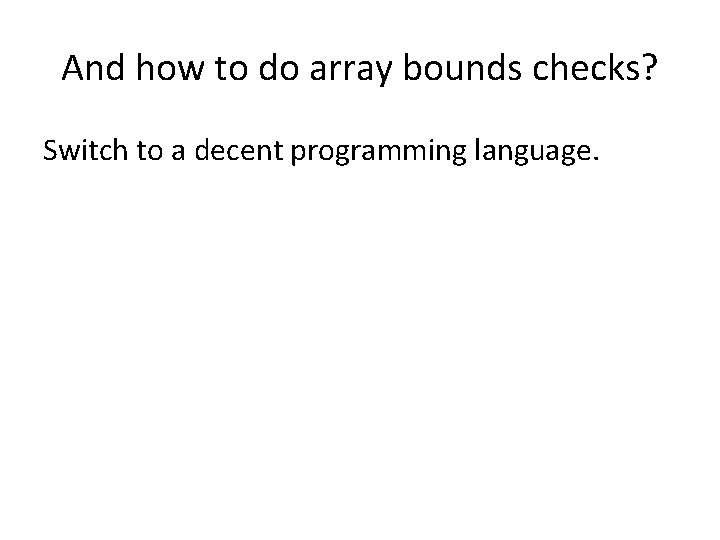
And how to do array bounds checks? Switch to a decent programming language.
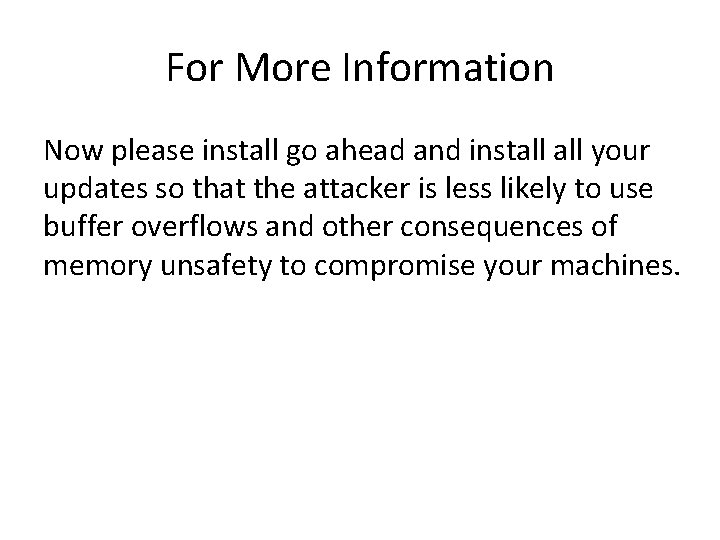
For More Information Now please install go ahead and install your updates so that the attacker is less likely to use buffer overflows and other consequences of memory unsafety to compromise your machines.
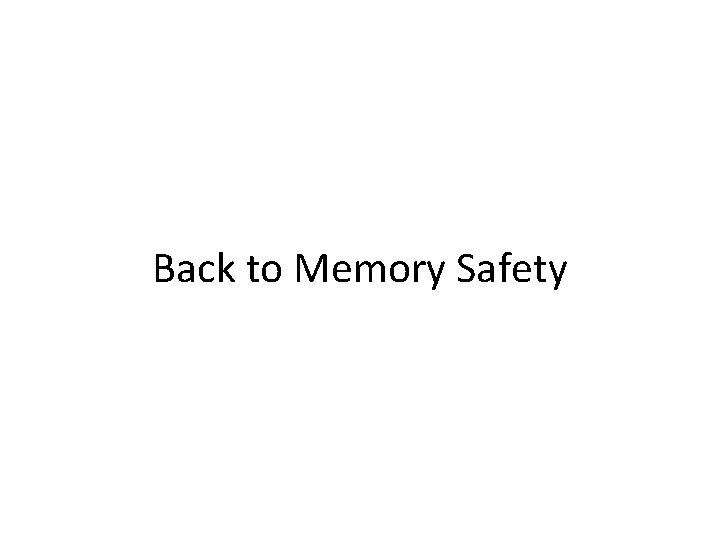
Back to Memory Safety
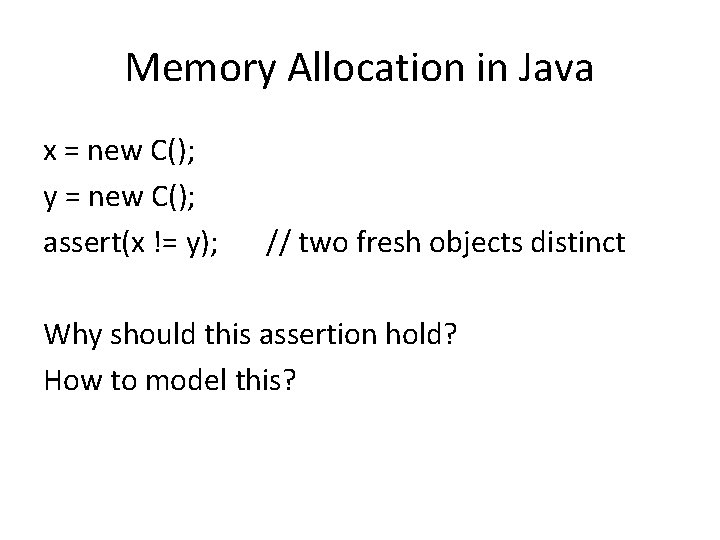
Memory Allocation in Java x = new C(); y = new C(); assert(x != y); // two fresh objects distinct Why should this assertion hold? How to model this?
![How to represent fresh objects assumeN 50 a new ObjectN i How to represent fresh objects? assume(N > 50); a = new Object[N]; i =](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-34.jpg)
How to represent fresh objects? assume(N > 50); a = new Object[N]; i = 0; while (i < N) { a[i] = new Object(); i = i + 1; } assert(a[5] != a[15]);
![Alloc Set Function alloc Obj Boolean i e alloc SetObj x Alloc Set (Function) alloc : Obj Boolean i. e. alloc : Set[Obj] x =](https://slidetodoc.com/presentation_image_h2/d687a65cb4df94dcf9b3771fb896289f/image-35.jpg)
Alloc Set (Function) alloc : Obj Boolean i. e. alloc : Set[Obj] x = new C();
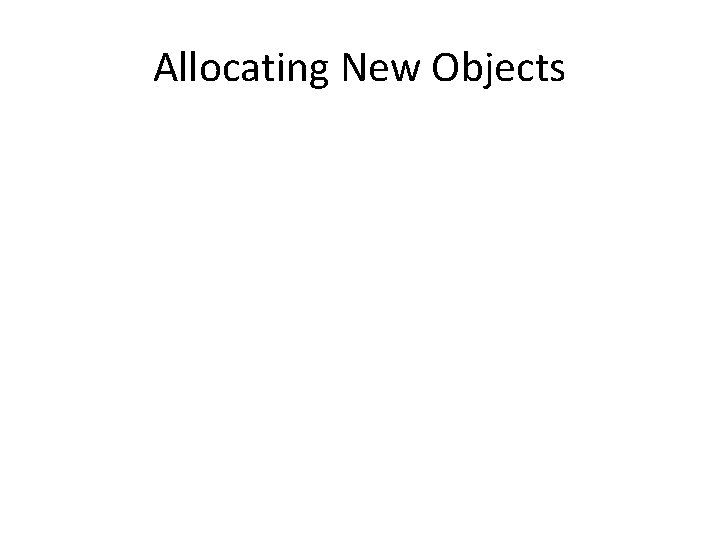
Allocating New Objects
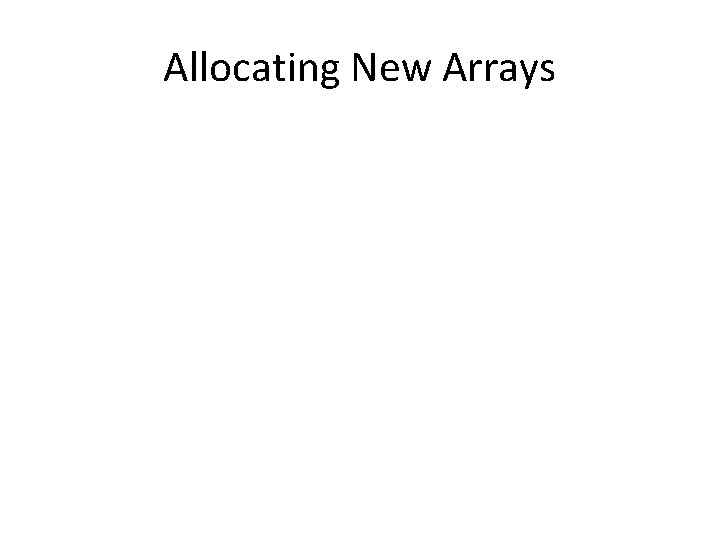
Allocating New Arrays
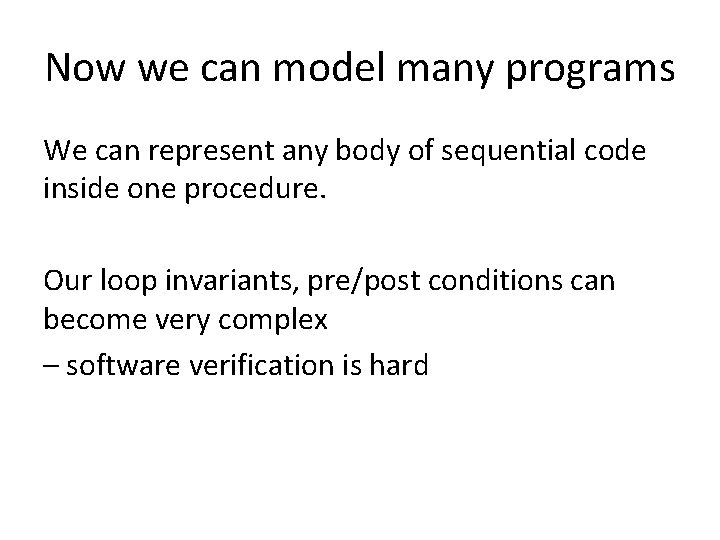
Now we can model many programs We can represent any body of sequential code inside one procedure. Our loop invariants, pre/post conditions can become very complex – software verification is hard
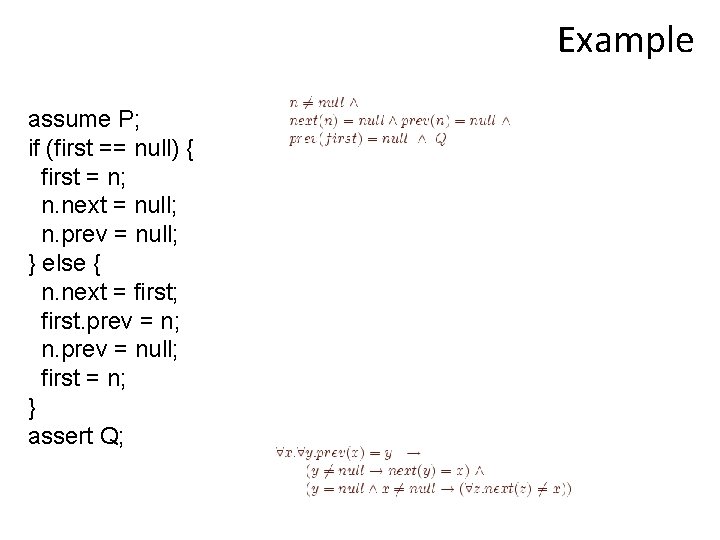
Example assume P; if (first == null) { first = n; n. next = null; n. prev = null; } else { n. next = first; first. prev = n; n. prev = null; first = n; } assert Q;
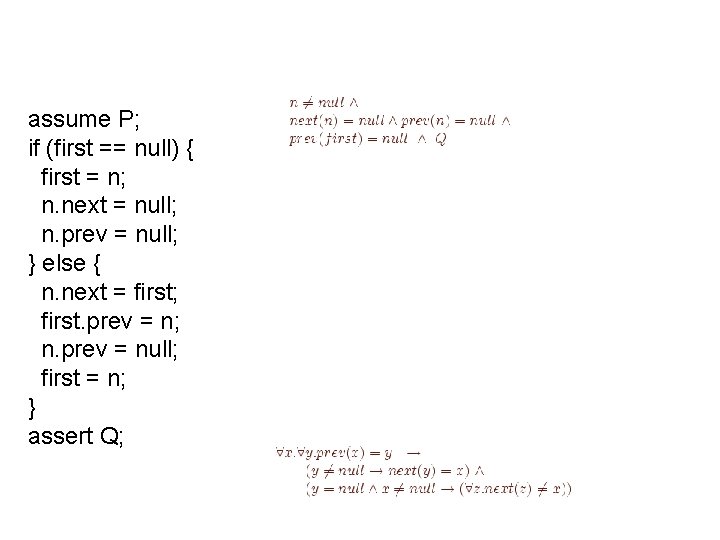
assume P; if (first == null) { first = n; n. next = null; n. prev = null; } else { n. next = first; first. prev = n; n. prev = null; first = n; } assert Q;
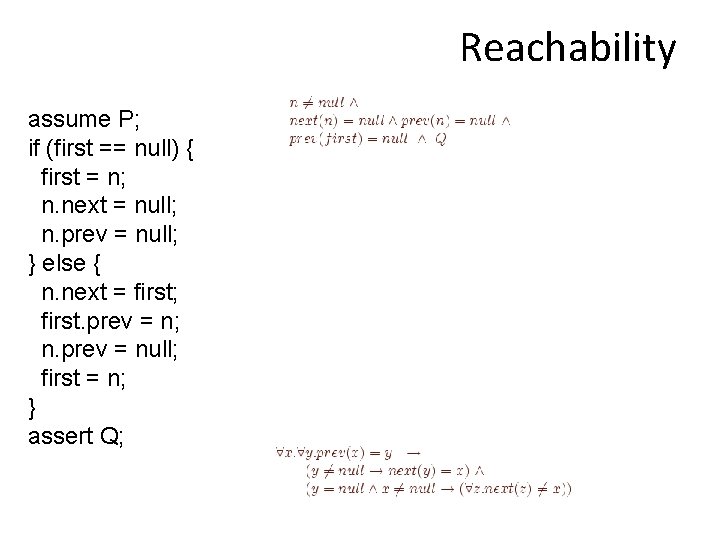
Reachability assume P; if (first == null) { first = n; n. next = null; n. prev = null; } else { n. next = first; first. prev = n; n. prev = null; first = n; } assert Q;
Collection of programs written to service other programs.
01:640:244 lecture notes - lecture 15: plat, idah, farad
It is an integrated analysis and synthesis
Application comprehension
Applied behavior analysis programs
Rhetorical vs synthesis essay
Meta synthesis vs meta analysis
Robert van engelen
Exploratory data analysis lecture notes
Sensitivity analysis lecture notes
Factor analysis lecture notes
Analysis of algorithms lecture notes
Quadrant streak
Power system analysis lecture notes
Illumination in entrepreneurship
Verification and validation
Verification and validation
Testing types in software engineering
Validation plan
Verification principle strengths and weaknesses
Shelving and shelf rectification
Asme v&v 10
Verification and validation
Verification and validation
Verification and validation plan
Verification and validation
Calibration verification material
A software verification and validation method. section 19
Software verification and validation plan
What is stock verification
Semi formal verification
Is unit testing verification or validation
Twic nexgen
Tncompass
Cpa confirmation letter
Dta verification documents
Smart meter verification
Sla certification
Roster verification ohio
Means of verification
Dea number verification
Applicative vs normal order