Stl iterators 1 The iterator concept A generalization
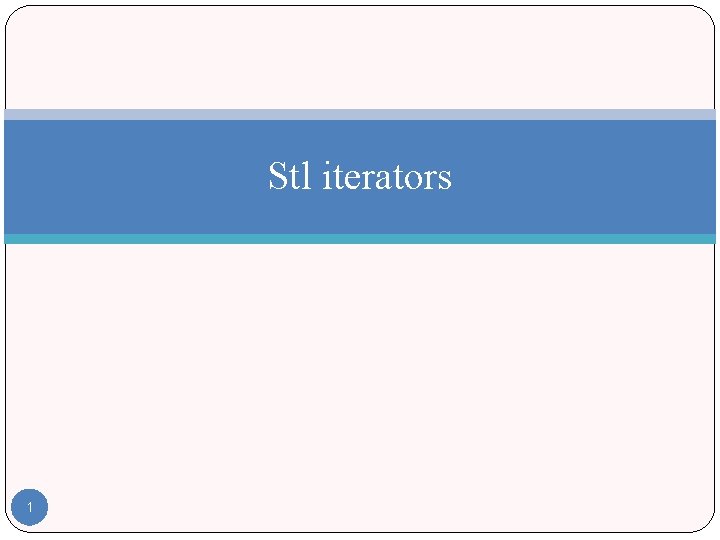
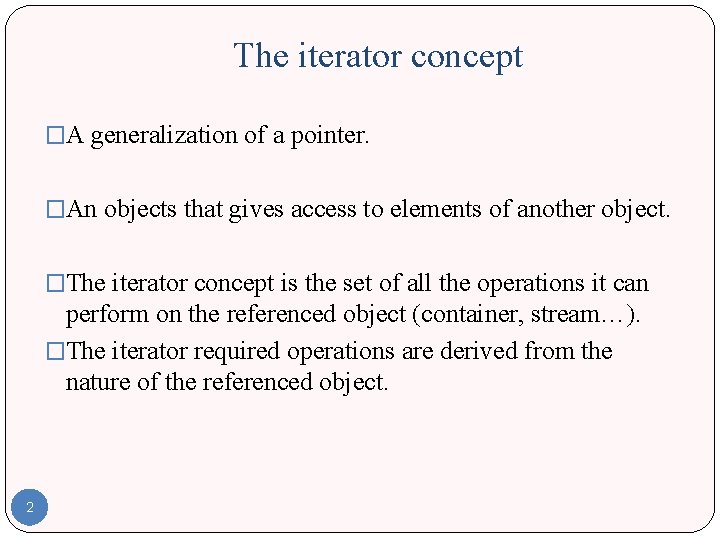
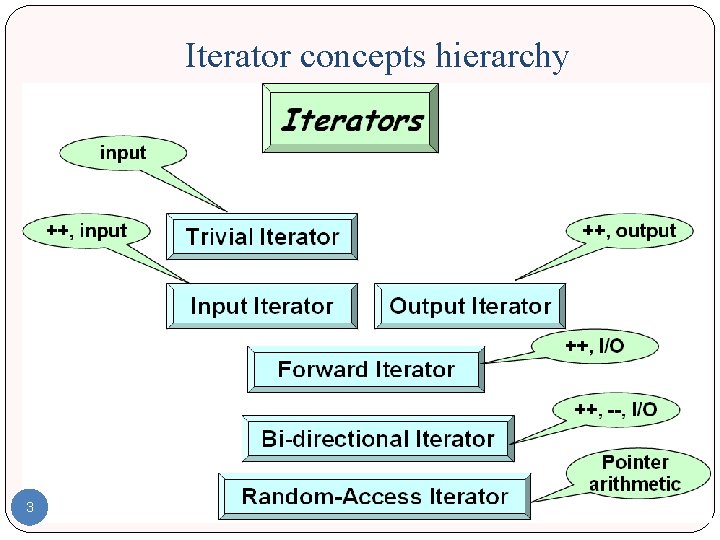
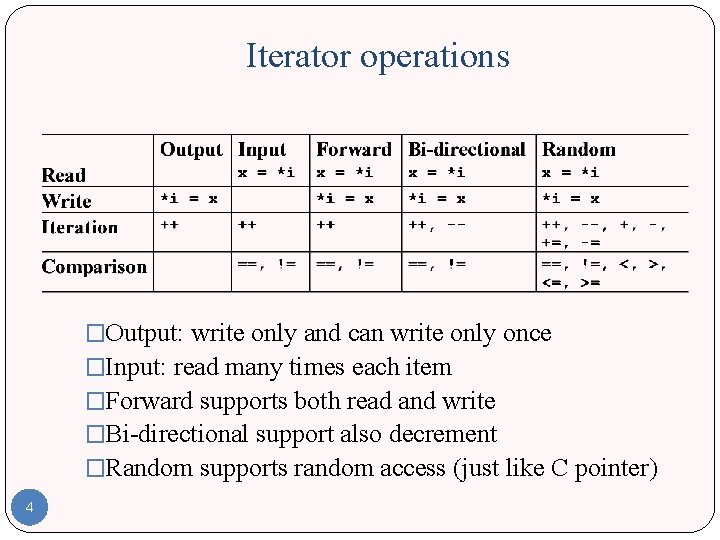
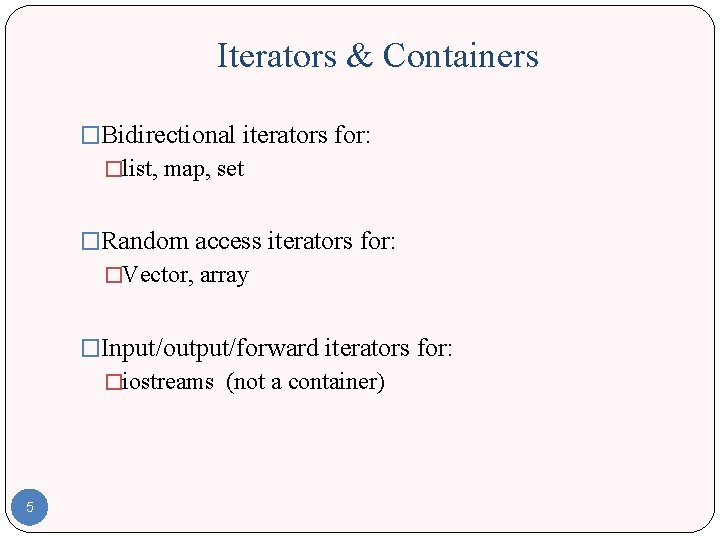
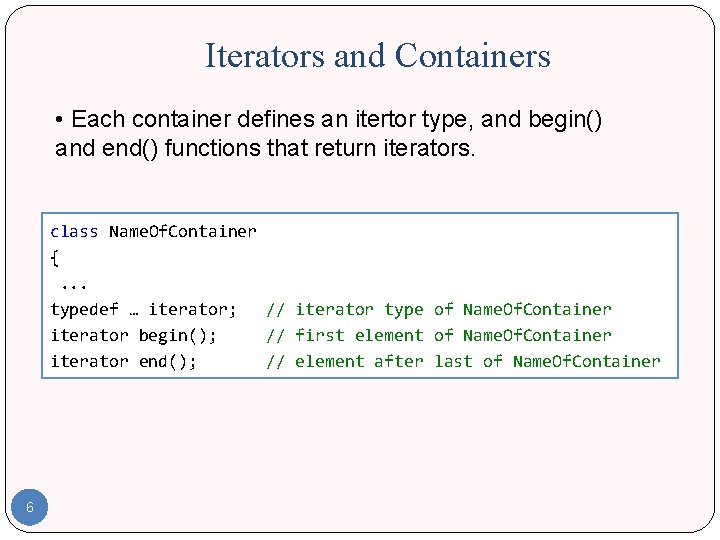
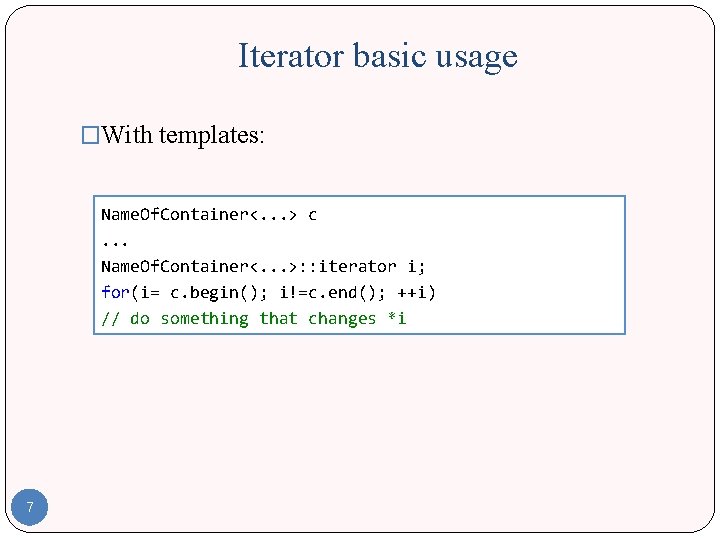
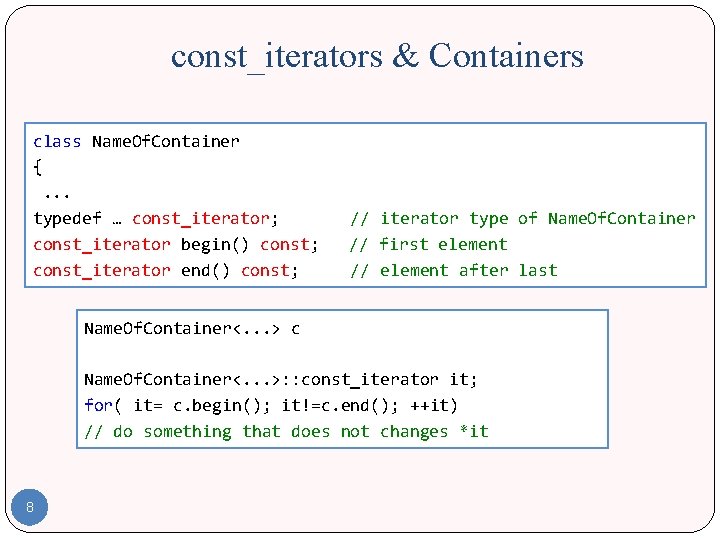
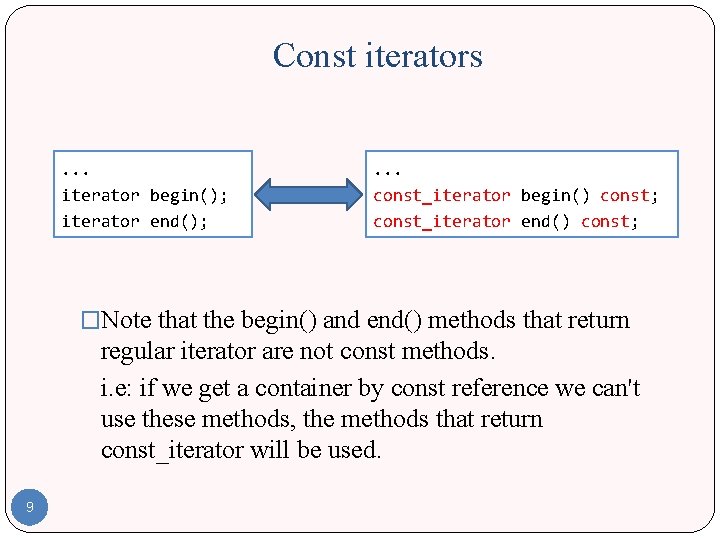
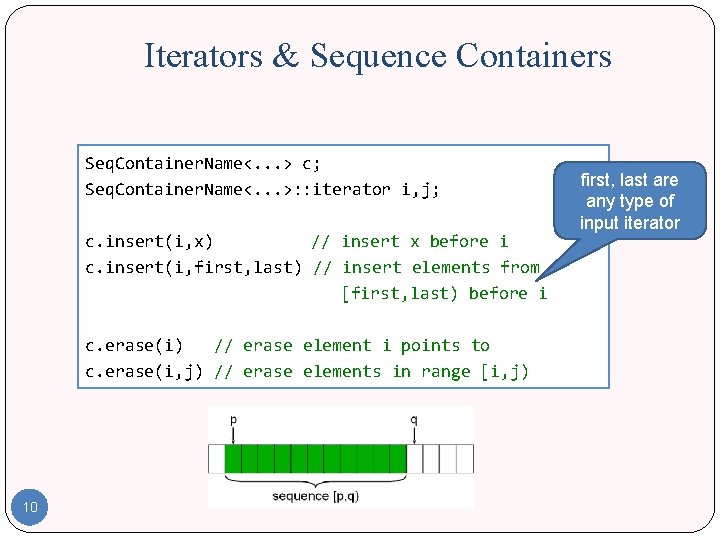
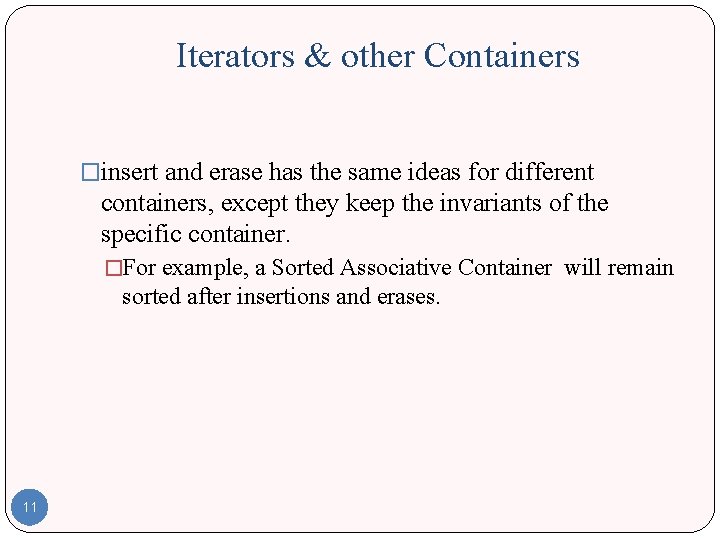
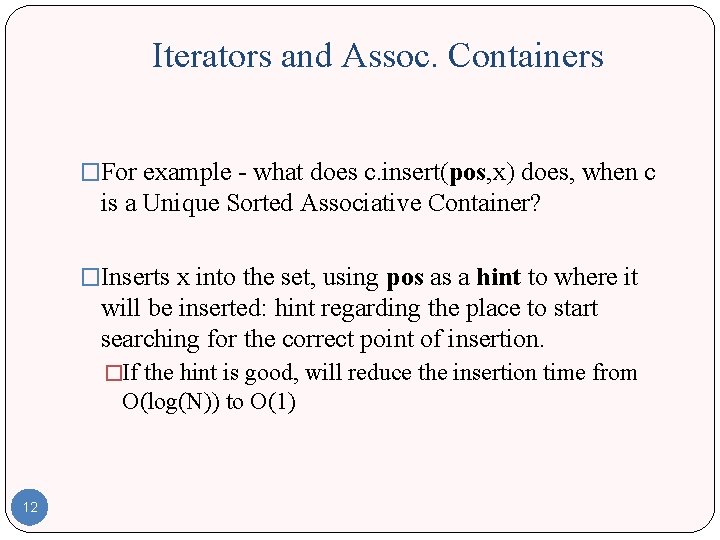
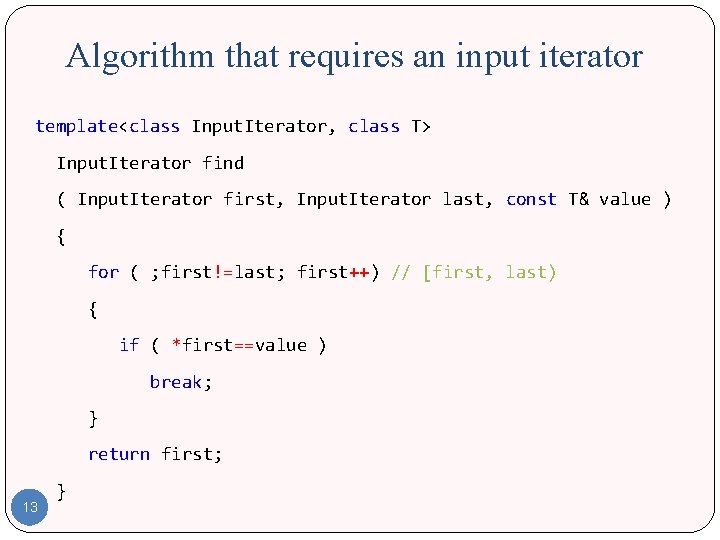
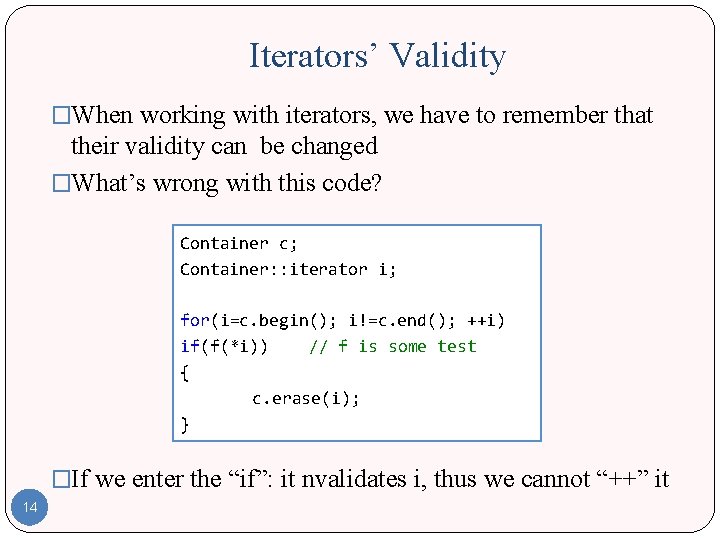
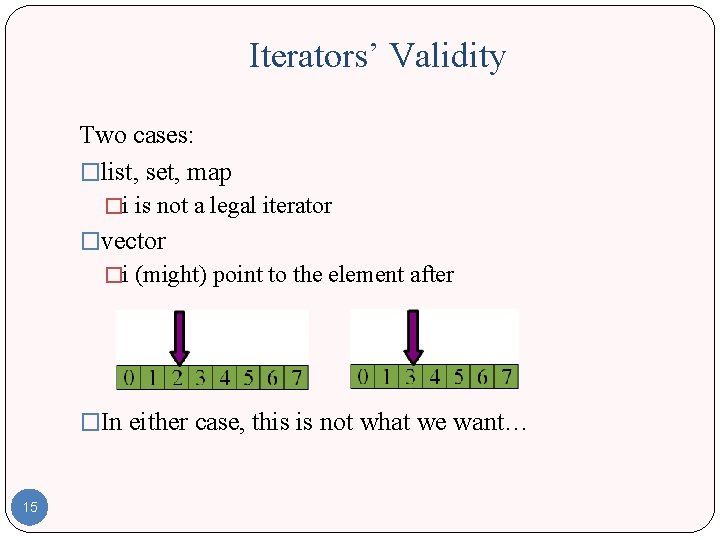
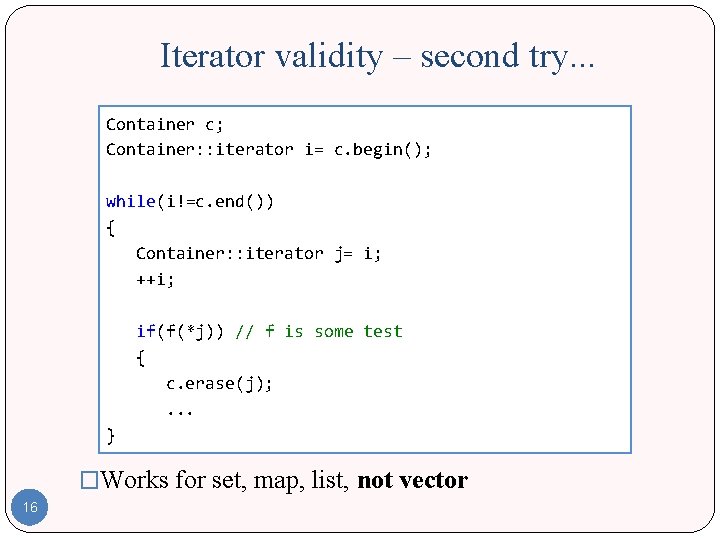
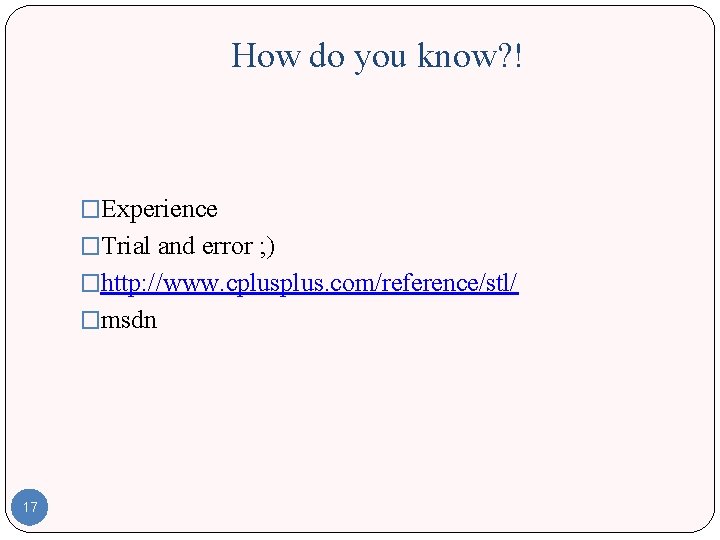
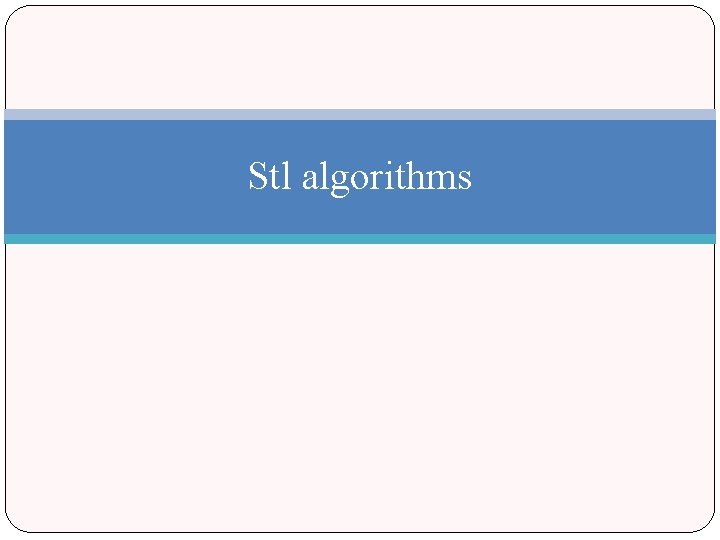
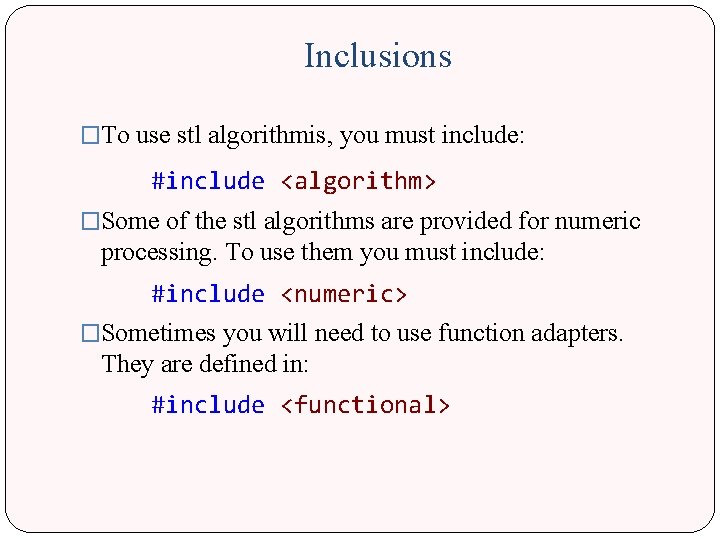
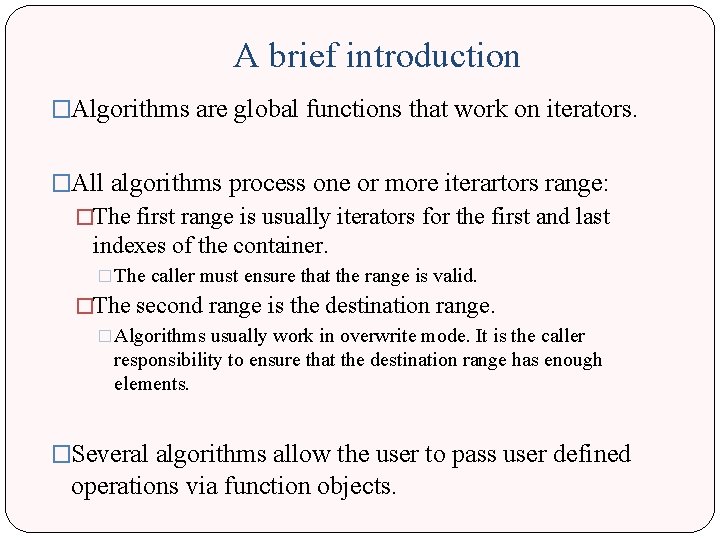
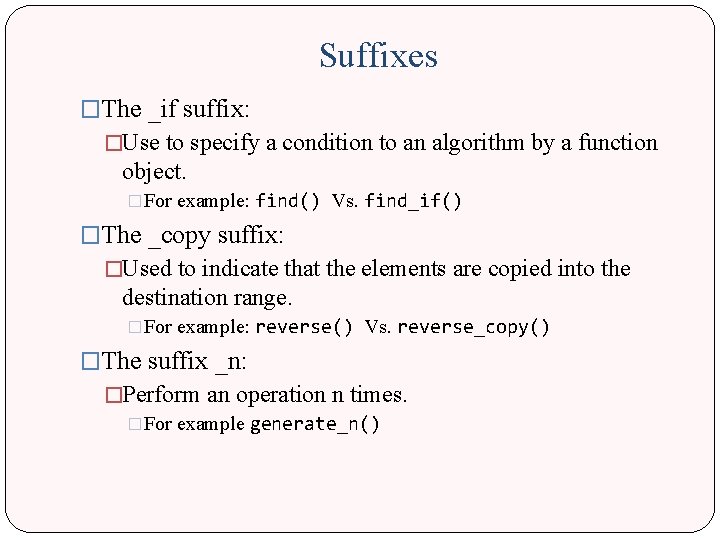
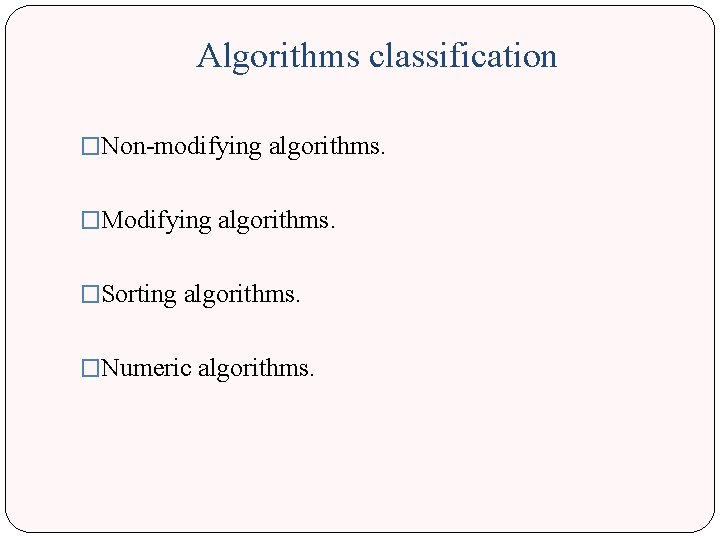
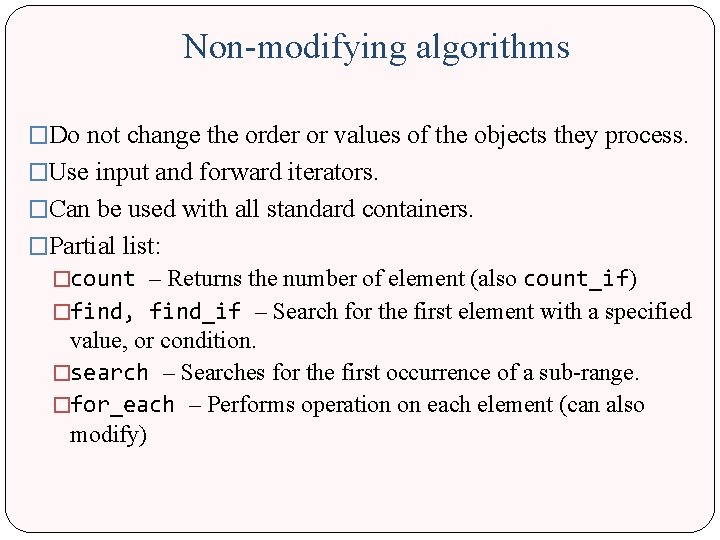
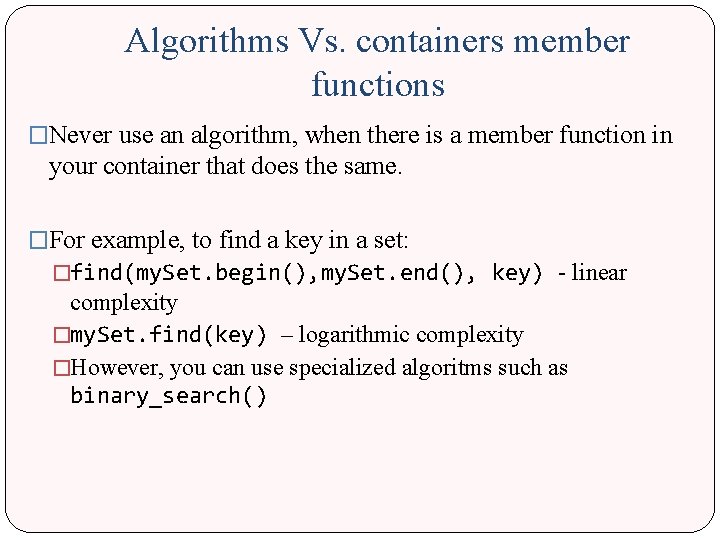
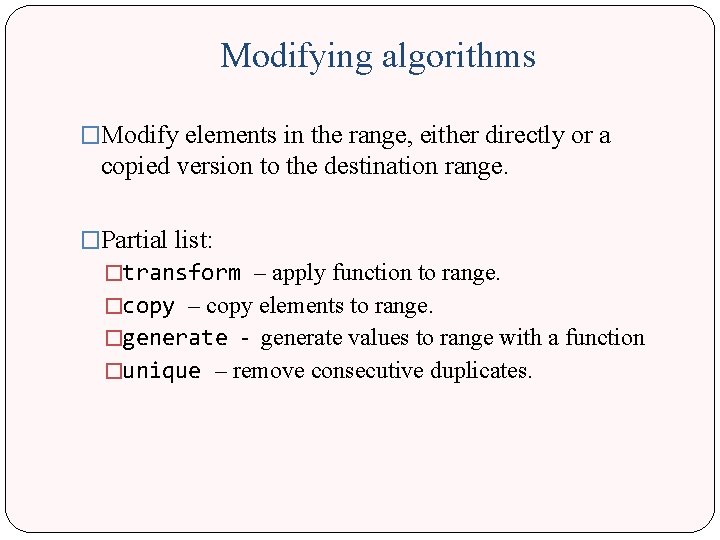
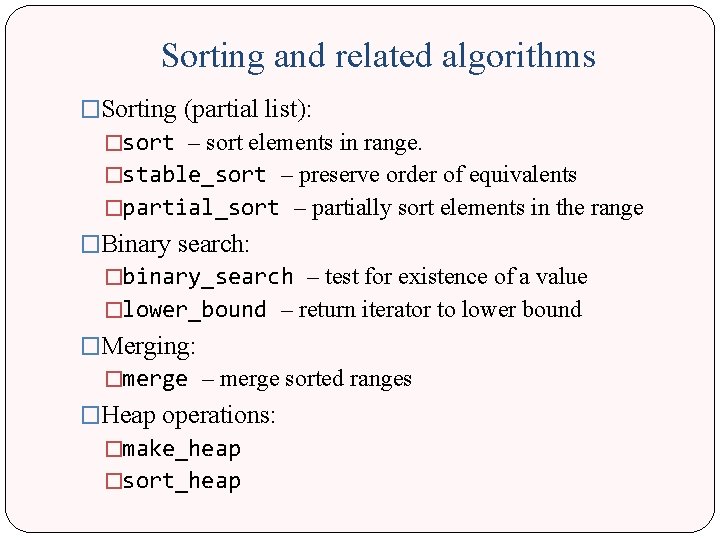
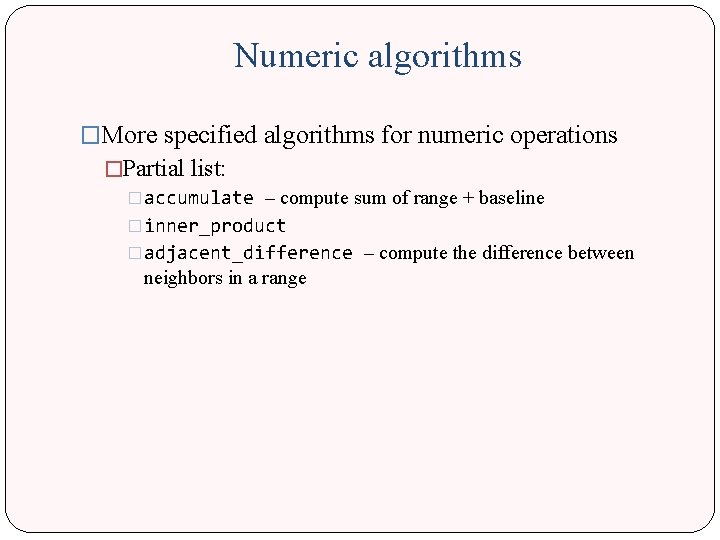
- Slides: 27
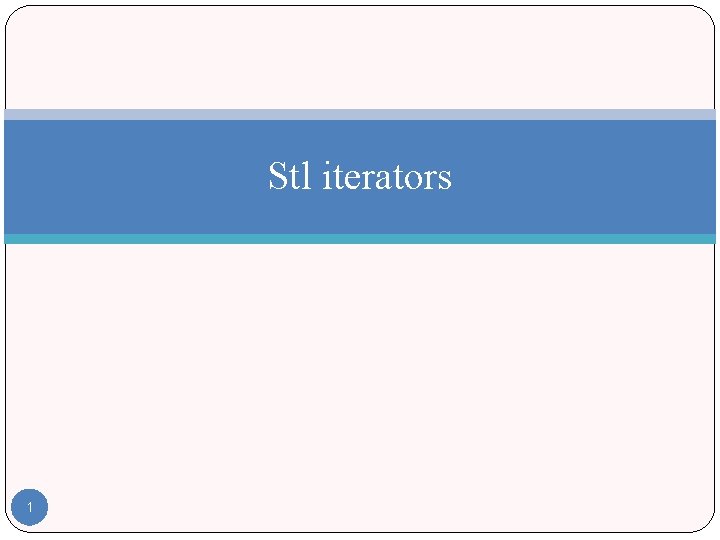
Stl iterators 1
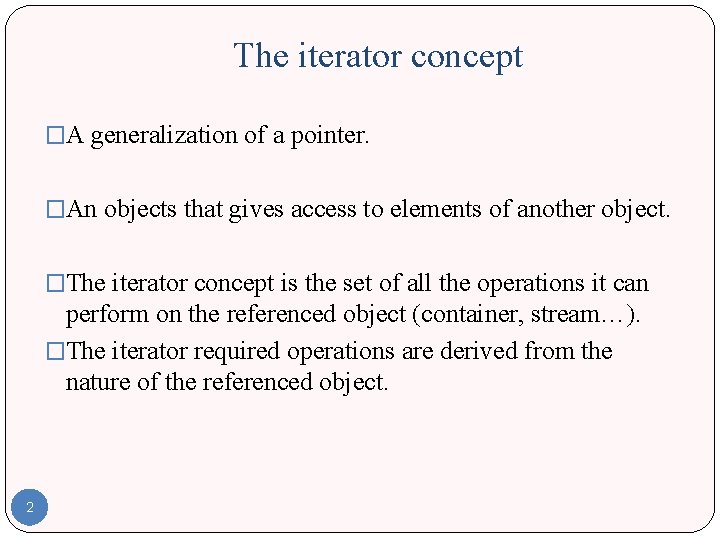
The iterator concept �A generalization of a pointer. �An objects that gives access to elements of another object. �The iterator concept is the set of all the operations it can perform on the referenced object (container, stream…). �The iterator required operations are derived from the nature of the referenced object. 2
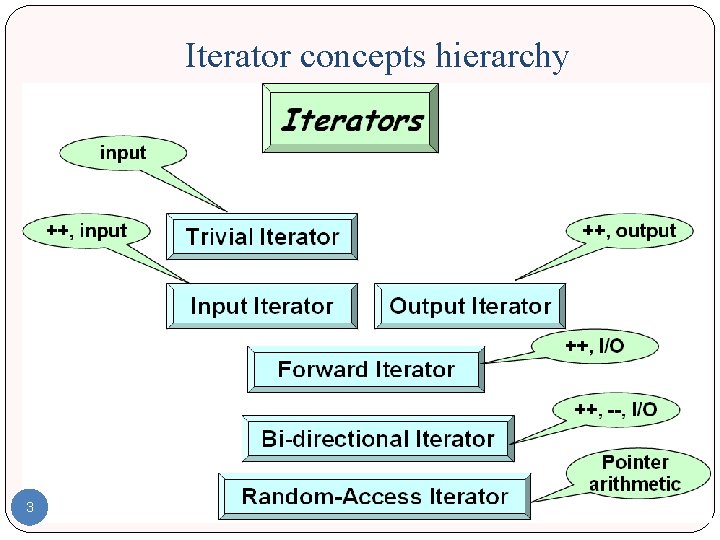
Iterator concepts hierarchy 3
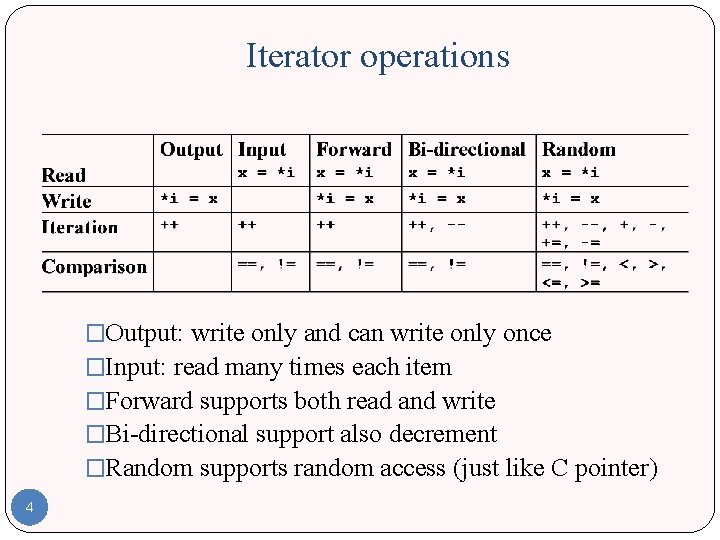
Iterator operations �Output: write only and can write only once �Input: read many times each item �Forward supports both read and write �Bi-directional support also decrement �Random supports random access (just like C pointer) 4
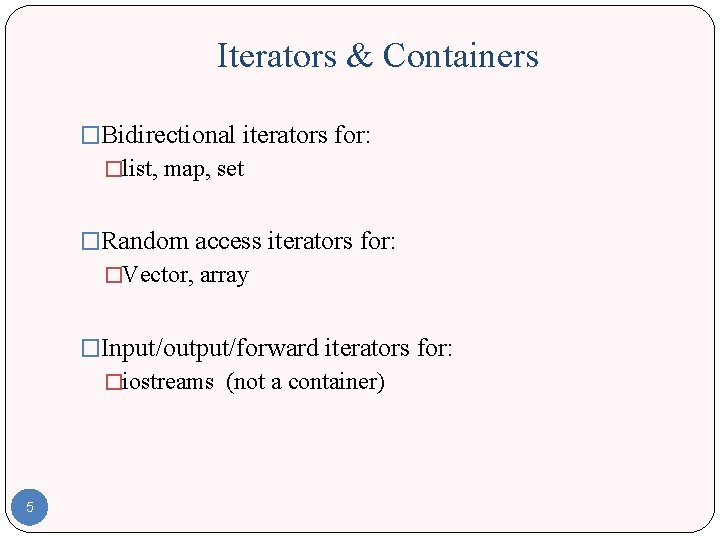
Iterators & Containers �Bidirectional iterators for: �list, map, set �Random access iterators for: �Vector, array �Input/output/forward iterators for: �iostreams (not a container) 5
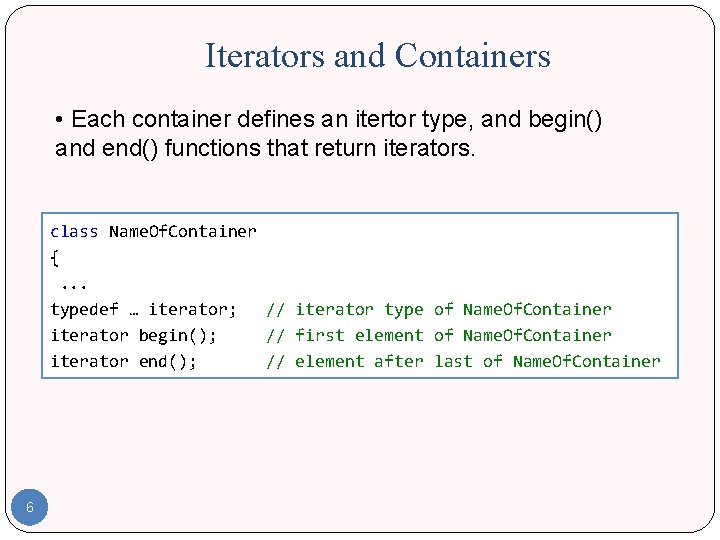
Iterators and Containers • Each container defines an itertor type, and begin() and end() functions that return iterators. class Name. Of. Container {. . . typedef … iterator; // iterator type of Name. Of. Container iterator begin(); // first element of Name. Of. Container iterator end(); // element after last of Name. Of. Container 6
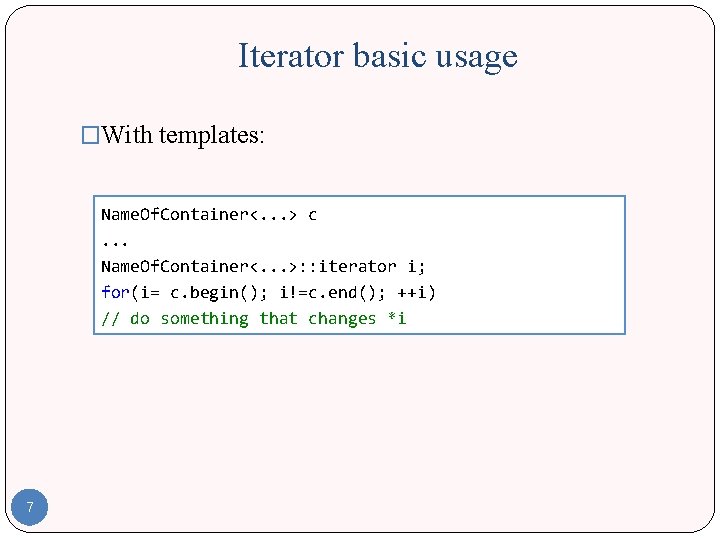
Iterator basic usage �With templates: Name. Of. Container<. . . > c. . . Name. Of. Container<. . . >: : iterator i; for(i= c. begin(); i!=c. end(); ++i) // do something that changes *i 7
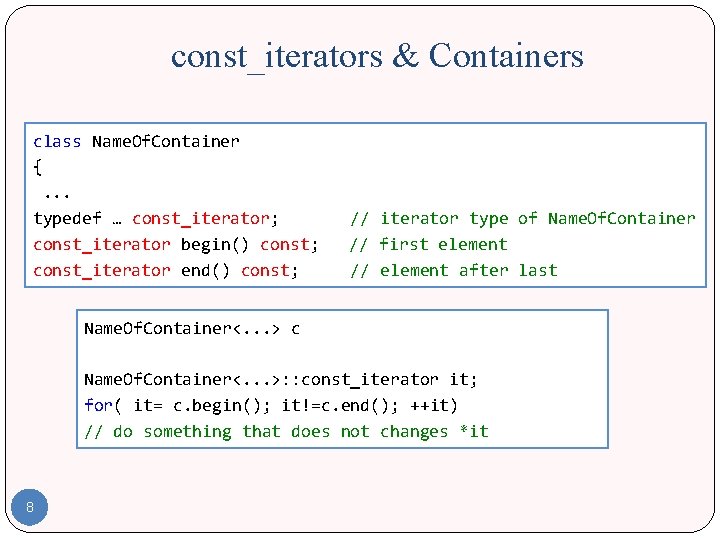
const_iterators & Containers class Name. Of. Container {. . . typedef … const_iterator; const_iterator begin() const; const_iterator end() const; // iterator type of Name. Of. Container // first element // element after last Name. Of. Container<. . . > c Name. Of. Container<. . . >: : const_iterator it; for( it= c. begin(); it!=c. end(); ++it) // do something that does not changes *it 8
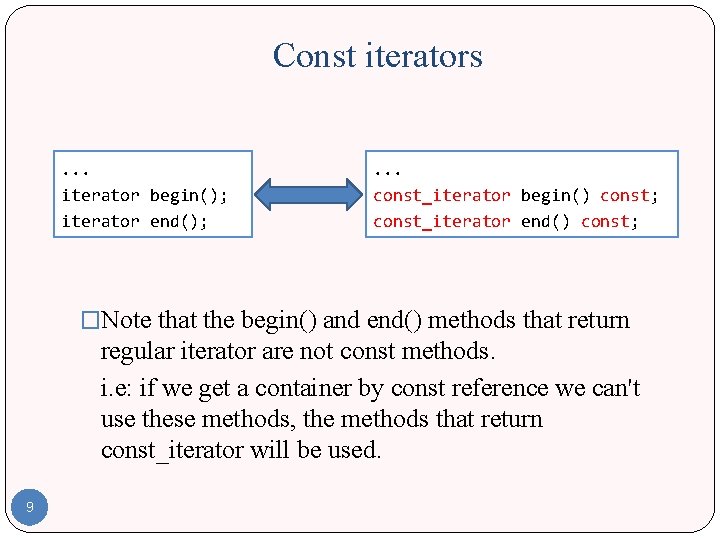
Const iterators. . . iterator begin(); iterator end(); . . . const_iterator begin() const; const_iterator end() const; �Note that the begin() and end() methods that return regular iterator are not const methods. i. e: if we get a container by const reference we can't use these methods, the methods that return const_iterator will be used. 9
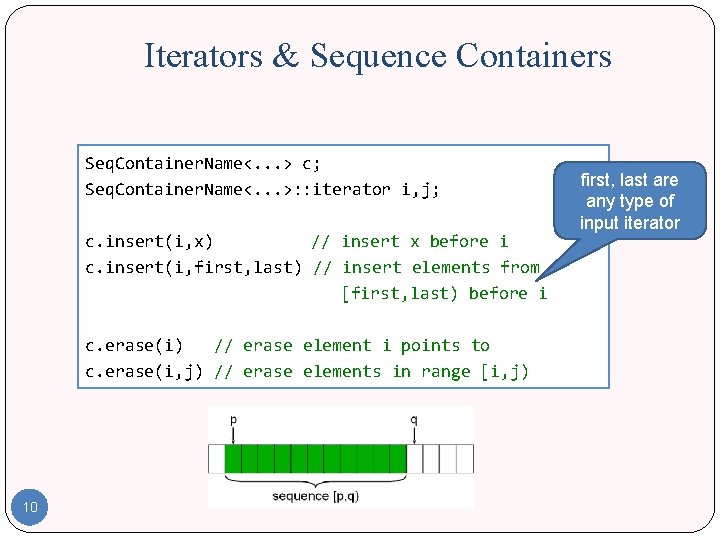
Iterators & Sequence Containers Seq. Container. Name<. . . > c; Seq. Container. Name<. . . >: : iterator i, j; c. insert(i, x) // insert x before i c. insert(i, first, last) // insert elements from [first, last) before i c. erase(i) // erase element i points to c. erase(i, j) // erase elements in range [i, j) 10 first, last are any type of input iterator
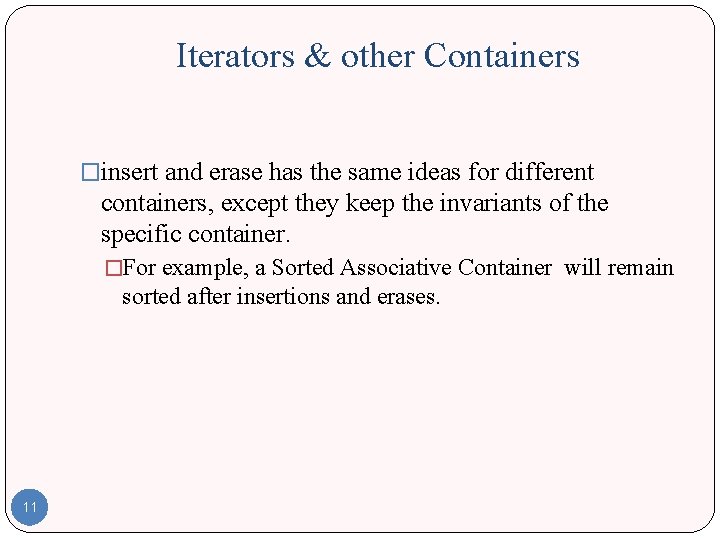
Iterators & other Containers �insert and erase has the same ideas for different containers, except they keep the invariants of the specific container. �For example, a Sorted Associative Container will remain sorted after insertions and erases. 11
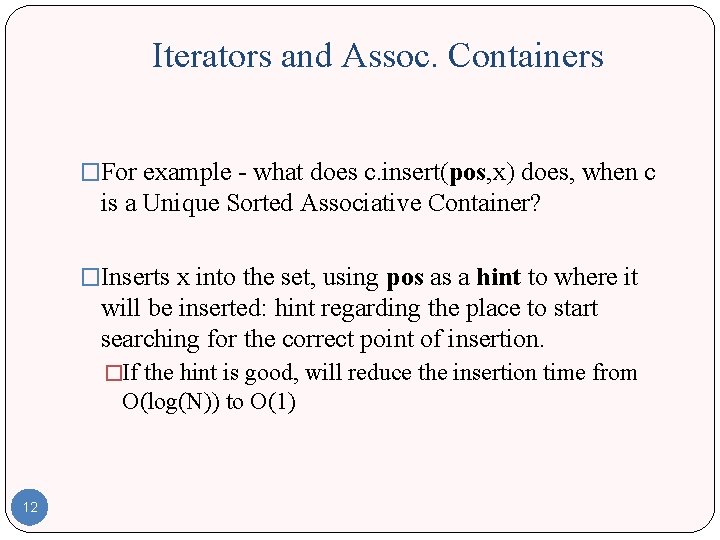
Iterators and Assoc. Containers �For example - what does c. insert(pos, x) does, when c is a Unique Sorted Associative Container? �Inserts x into the set, using pos as a hint to where it will be inserted: hint regarding the place to start searching for the correct point of insertion. �If the hint is good, will reduce the insertion time from O(log(N)) to O(1) 12
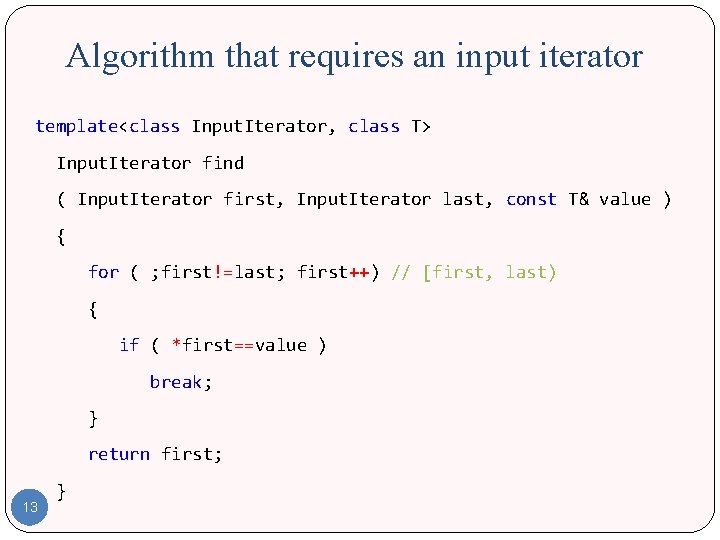
Algorithm that requires an input iterator template<class Input. Iterator, class T> Input. Iterator find ( Input. Iterator first, Input. Iterator last, const T& value ) { for ( ; first!=last; first++) // [first, last) { if ( *first==value ) break; } return first; 13 }
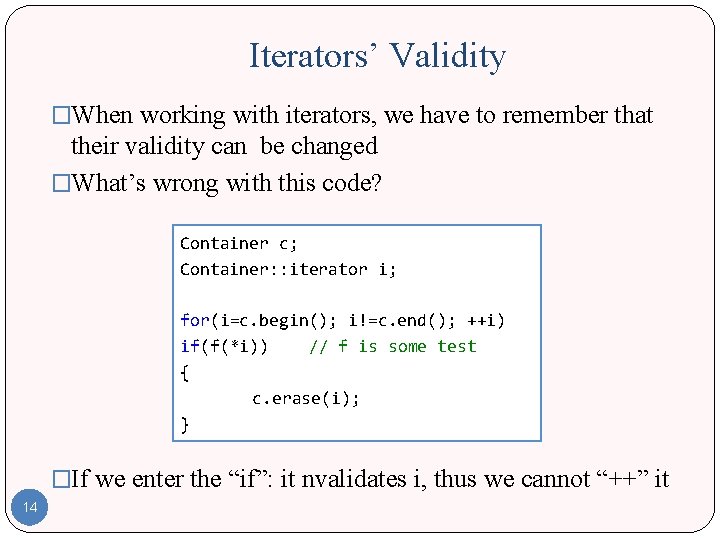
Iterators’ Validity �When working with iterators, we have to remember that their validity can be changed �What’s wrong with this code? Container c; Container: : iterator i; for(i=c. begin(); i!=c. end(); ++i) if(f(*i)) // f is some test { c. erase(i); } �If we enter the “if”: it nvalidates i, thus we cannot “++” it 14
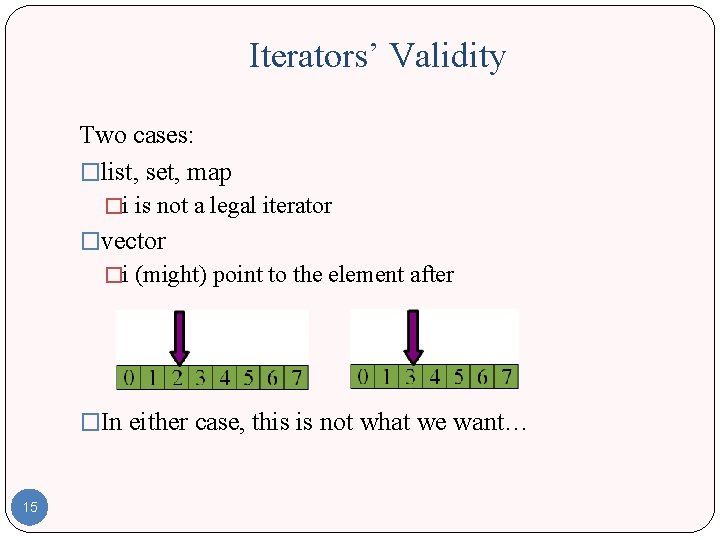
Iterators’ Validity Two cases: �list, set, map �i is not a legal iterator �vector �i (might) point to the element after �In either case, this is not what we want… 15
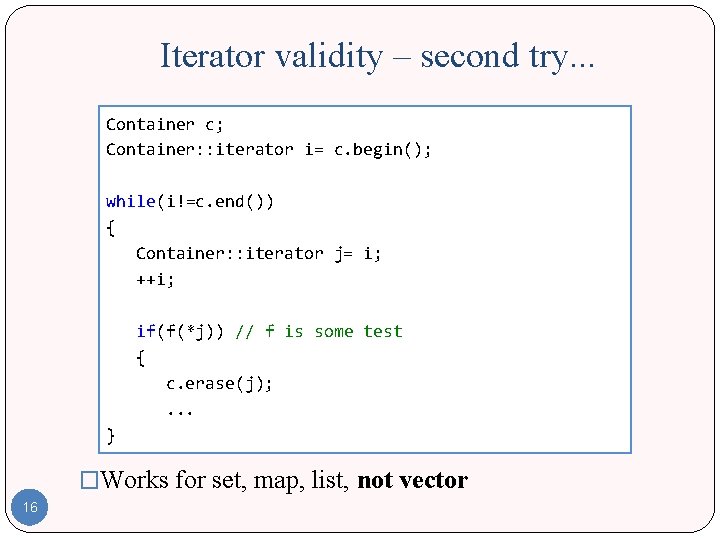
Iterator validity – second try. . . Container c; Container: : iterator i= c. begin(); while(i!=c. end()) { Container: : iterator j= i; ++i; if(f(*j)) // f is some test { c. erase(j); . . . } �Works for set, map, list, not vector 16
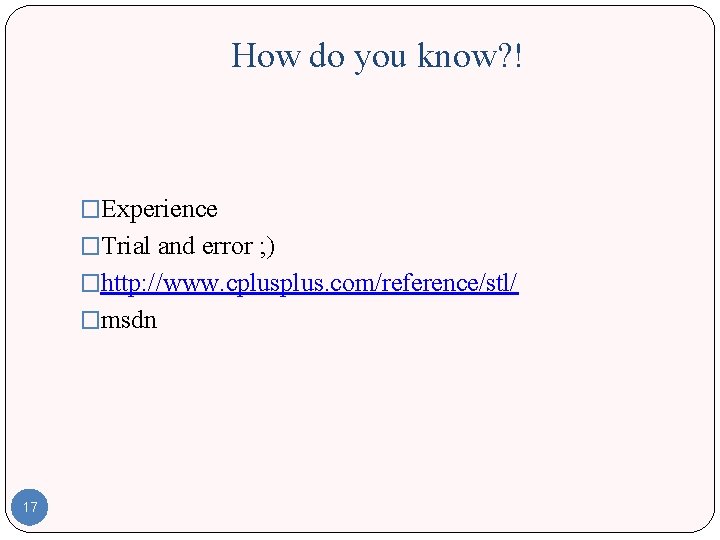
How do you know? ! �Experience �Trial and error ; ) �http: //www. cplus. com/reference/stl/ �msdn 17
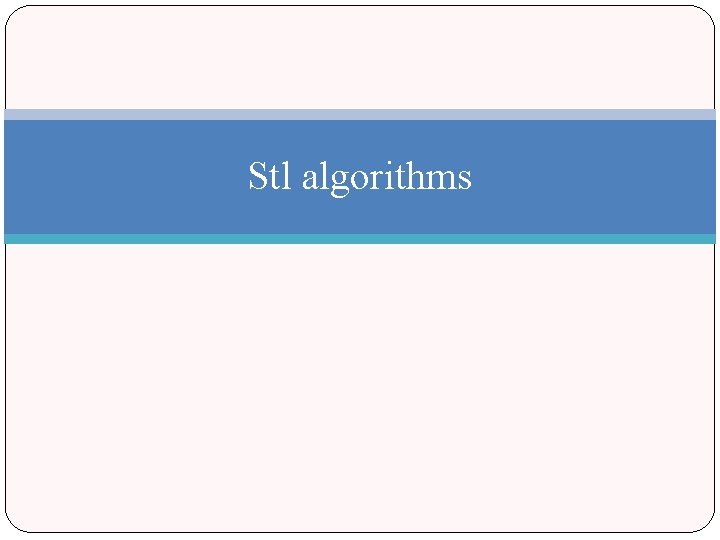
Stl algorithms
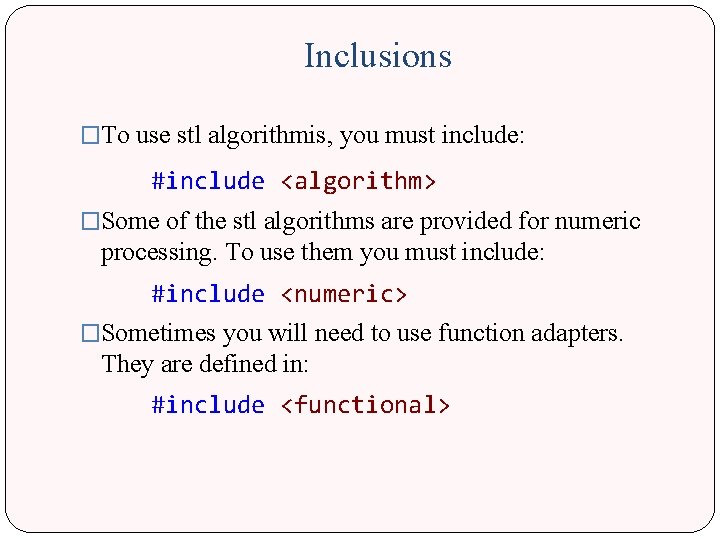
Inclusions �To use stl algorithmis, you must include: #include <algorithm> �Some of the stl algorithms are provided for numeric processing. To use them you must include: #include <numeric> �Sometimes you will need to use function adapters. They are defined in: #include <functional>
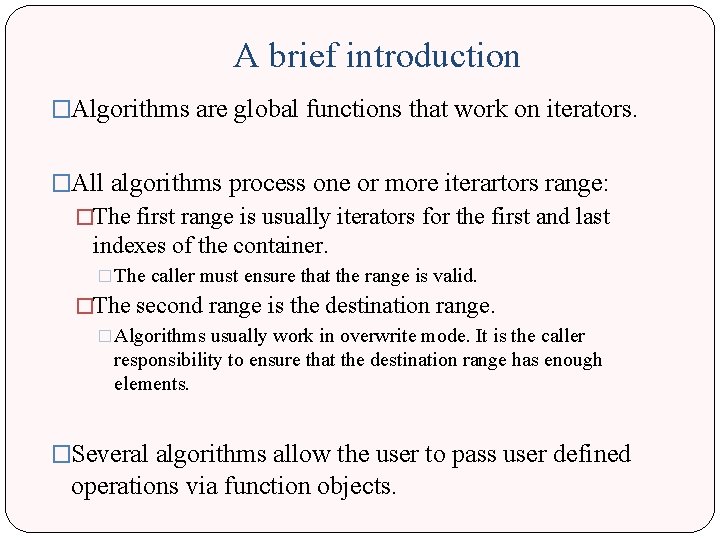
A brief introduction �Algorithms are global functions that work on iterators. �All algorithms process one or more iterartors range: �The first range is usually iterators for the first and last indexes of the container. �The caller must ensure that the range is valid. �The second range is the destination range. �Algorithms usually work in overwrite mode. It is the caller responsibility to ensure that the destination range has enough elements. �Several algorithms allow the user to pass user defined operations via function objects.
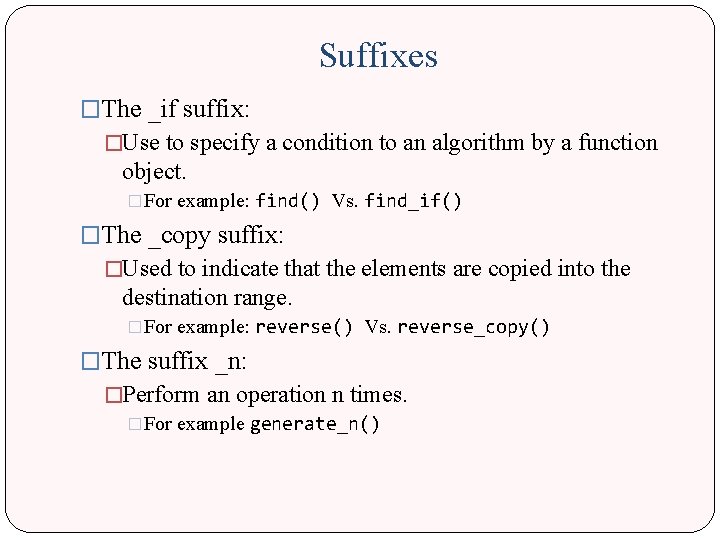
Suffixes �The _if suffix: �Use to specify a condition to an algorithm by a function object. �For example: find() Vs. find_if() �The _copy suffix: �Used to indicate that the elements are copied into the destination range. �For example: reverse() Vs. reverse_copy() �The suffix _n: �Perform an operation n times. �For example generate_n()
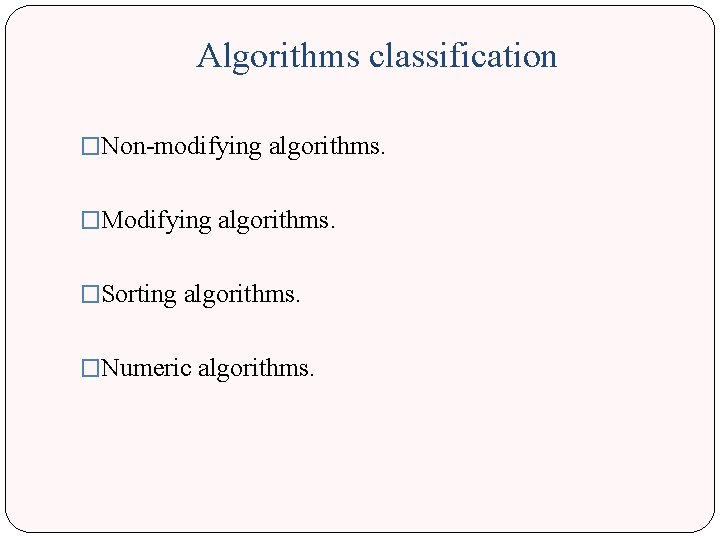
Algorithms classification �Non-modifying algorithms. �Modifying algorithms. �Sorting algorithms. �Numeric algorithms.
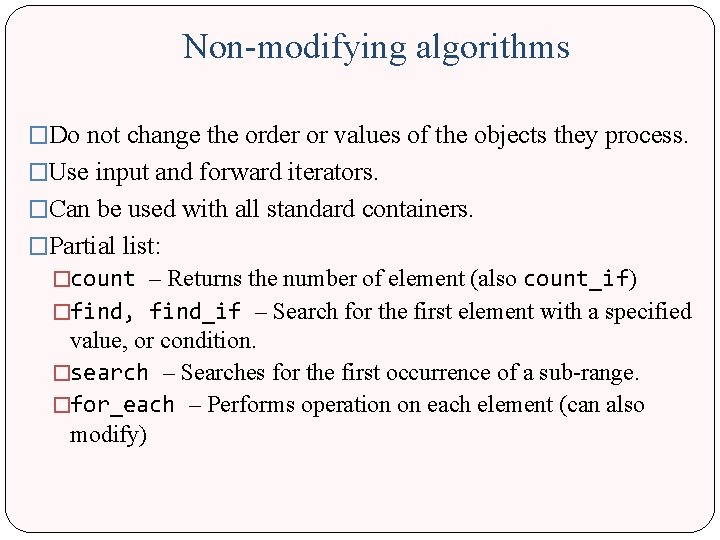
Non-modifying algorithms �Do not change the order or values of the objects they process. �Use input and forward iterators. �Can be used with all standard containers. �Partial list: �count – Returns the number of element (also count_if) �find, find_if – Search for the first element with a specified value, or condition. �search – Searches for the first occurrence of a sub-range. �for_each – Performs operation on each element (can also modify)
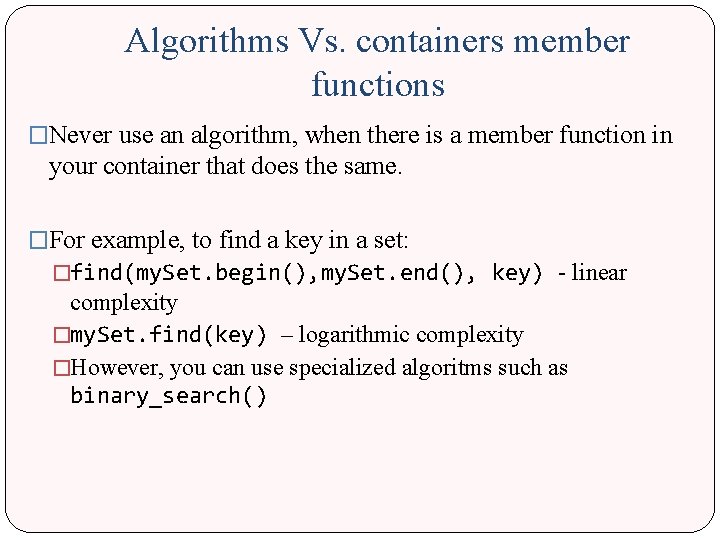
Algorithms Vs. containers member functions �Never use an algorithm, when there is a member function in your container that does the same. �For example, to find a key in a set: �find(my. Set. begin(), my. Set. end(), key) - linear complexity �my. Set. find(key) – logarithmic complexity �However, you can use specialized algoritms such as binary_search()
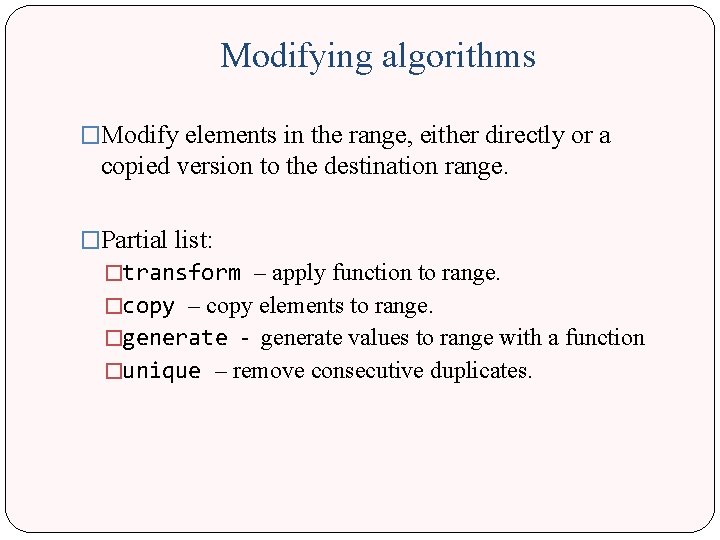
Modifying algorithms �Modify elements in the range, either directly or a copied version to the destination range. �Partial list: �transform – apply function to range. �copy – copy elements to range. �generate - generate values to range with a function �unique – remove consecutive duplicates.
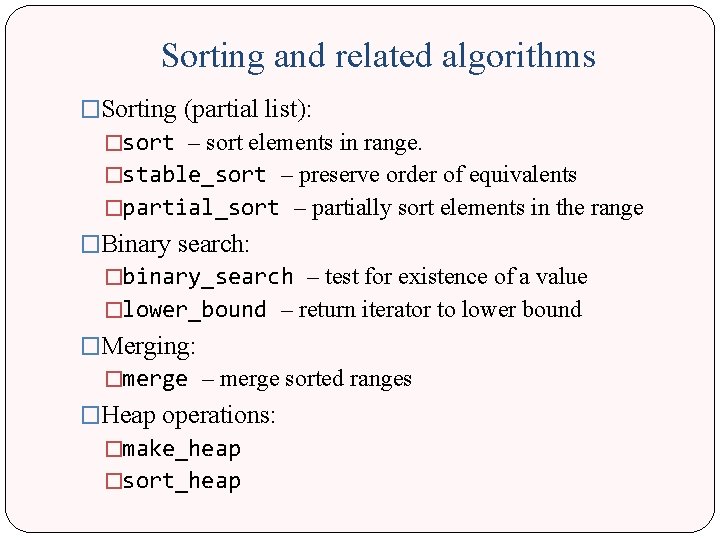
Sorting and related algorithms �Sorting (partial list): �sort – sort elements in range. �stable_sort – preserve order of equivalents �partial_sort – partially sort elements in the range �Binary search: �binary_search – test for existence of a value �lower_bound – return iterator to lower bound �Merging: �merge – merge sorted ranges �Heap operations: �make_heap �sort_heap
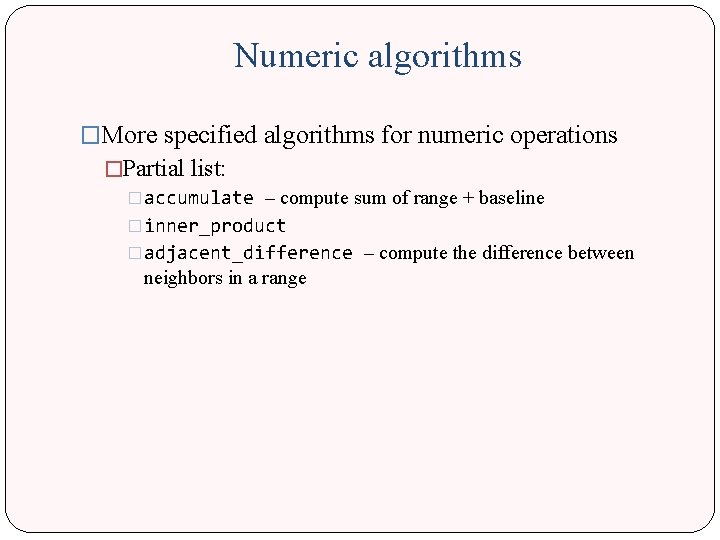
Numeric algorithms �More specified algorithms for numeric operations �Partial list: �accumulate – compute sum of range + baseline �inner_product �adjacent_difference – compute the difference between neighbors in a range
Maps and sets support bidirectional iterators.
Iterators and generators in python
Iterator inner class java
Iterator entwurfsmuster
Junit before after
Iterator pattern python
Iterator
Ghidra codeblock iterator
Inorder iterator
Volcano iterator model
Rapidjson assert
Zig iterator
Std sort
Stl bgb
Język stl komendy
Overenie stl
Parallel stl
From statsmodels.tsa.seasonal import stl
Blender reduce polygons
Stl bgb
Thư viện stl
Stl biblioteka
C++ functor
Stl biblioteka
Generic programming and the stl
Stl components are grouped under
Stl stands for in c++
Terminale stl spcl systèmes et procédés