Iterators An iterator permits you to examine the
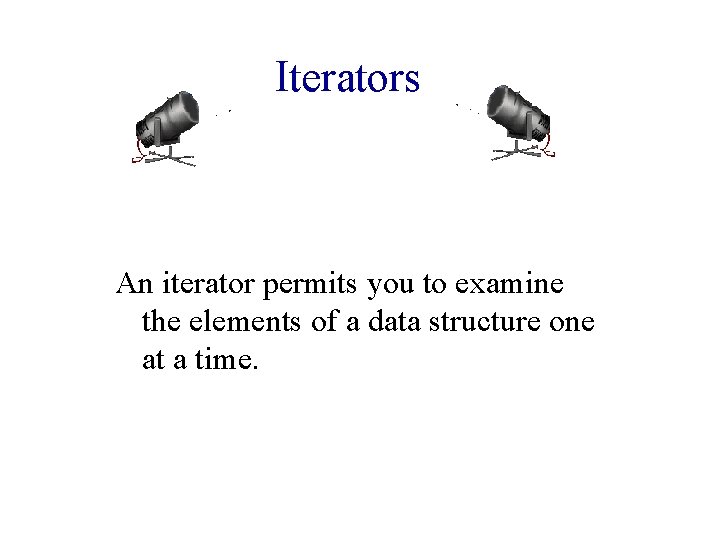
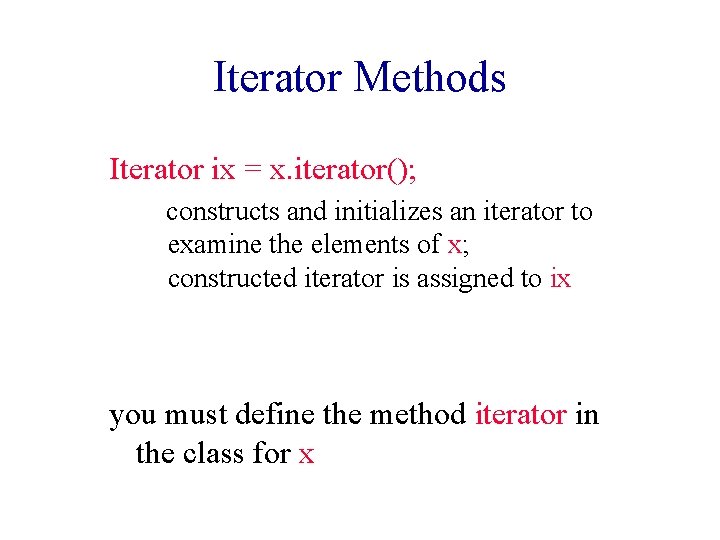
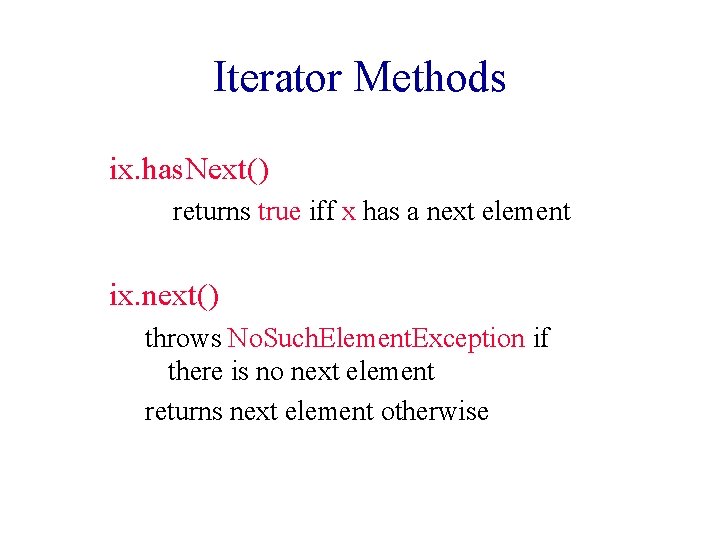
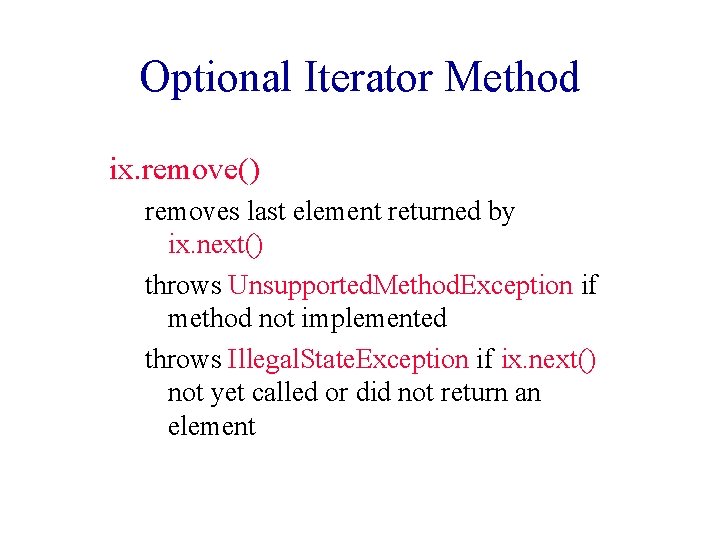
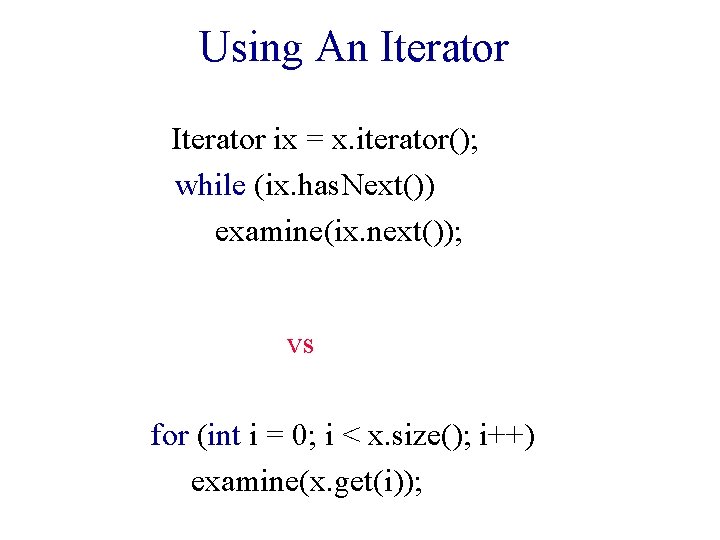
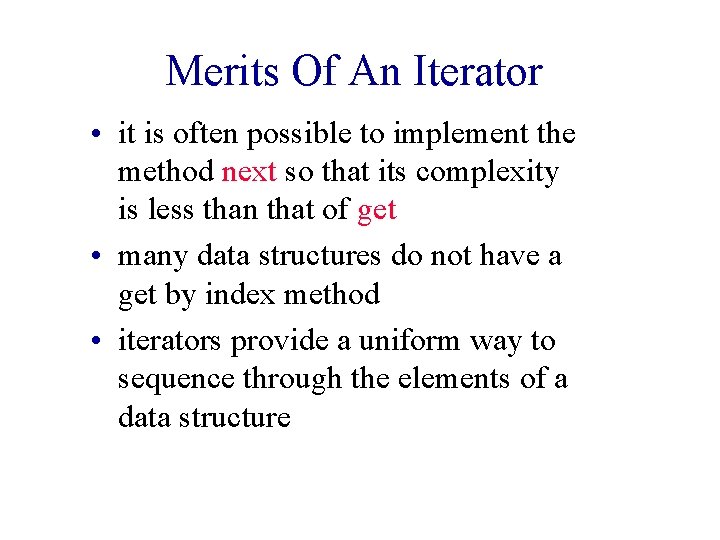
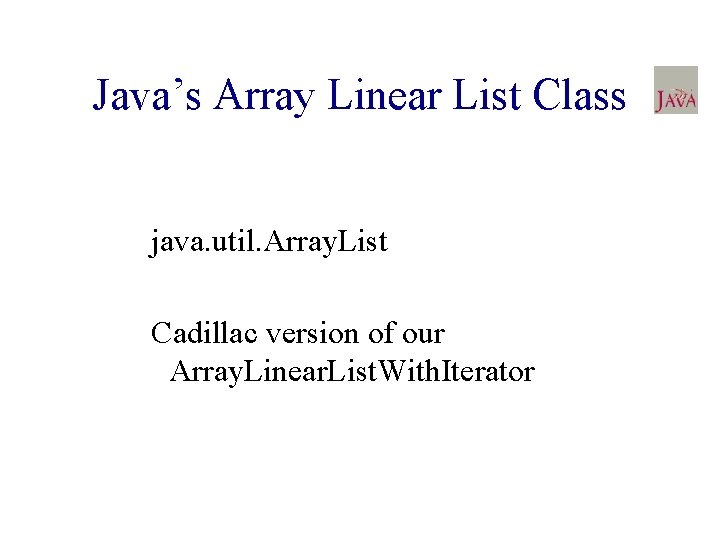
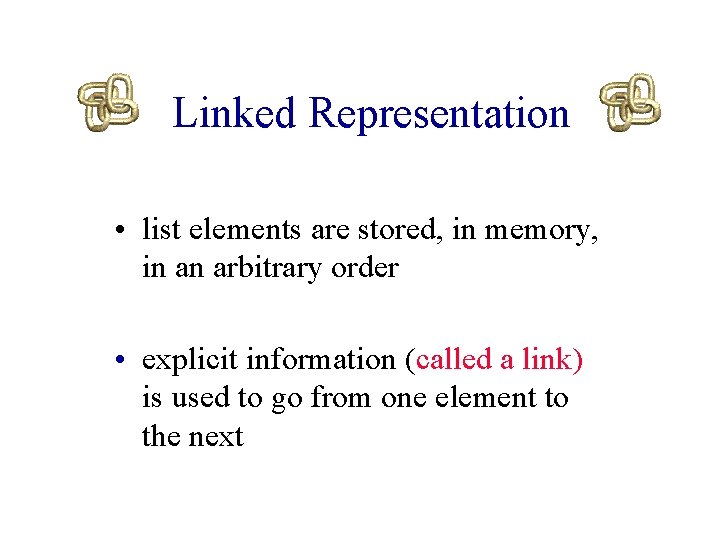
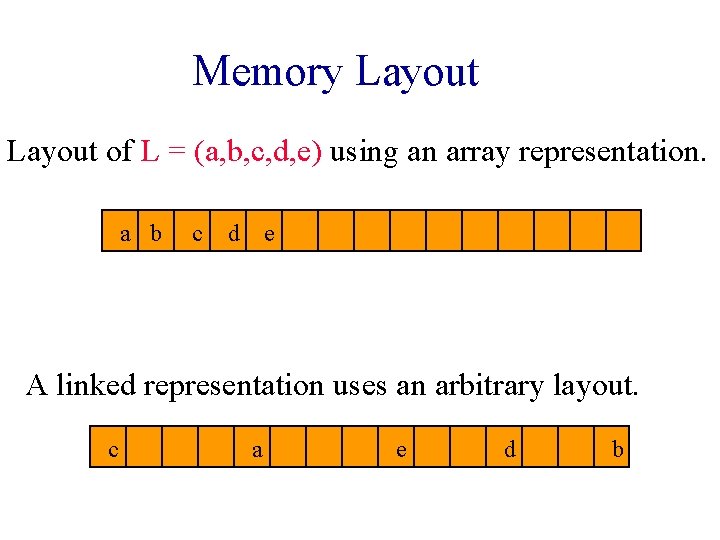
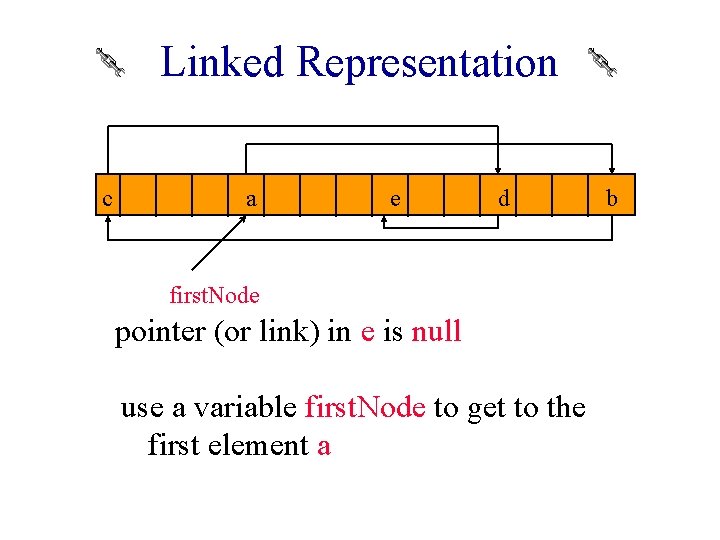
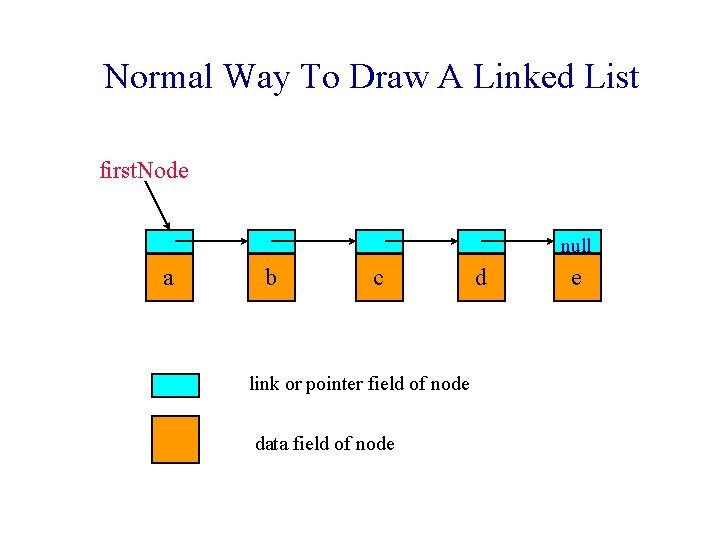
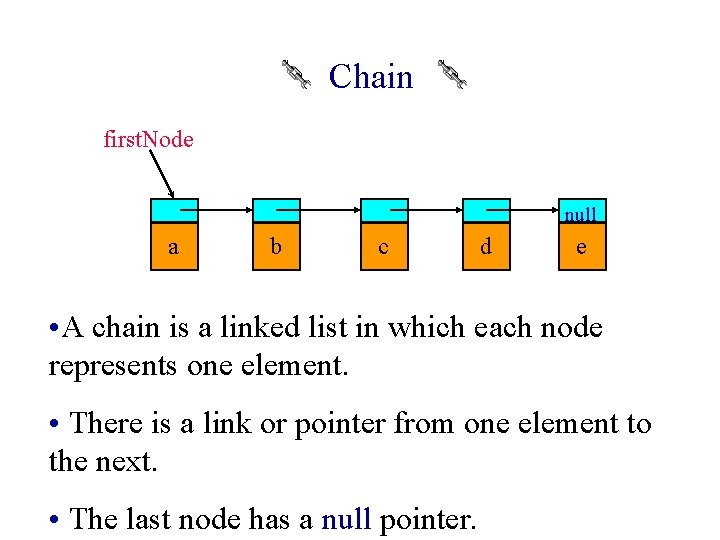
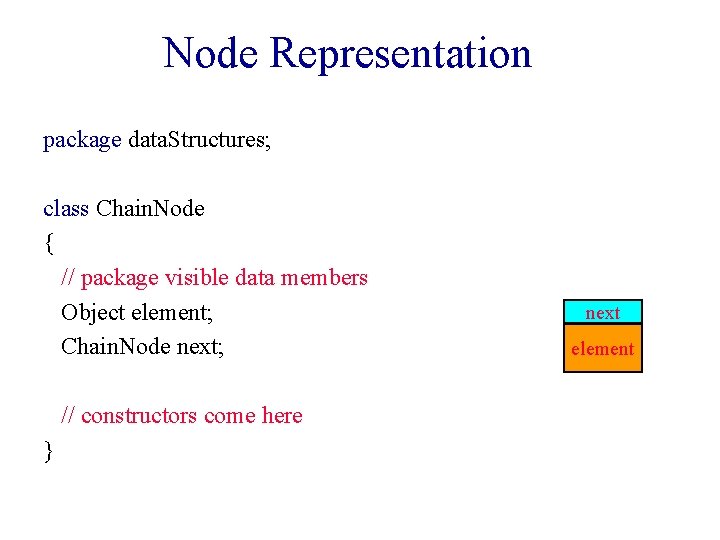
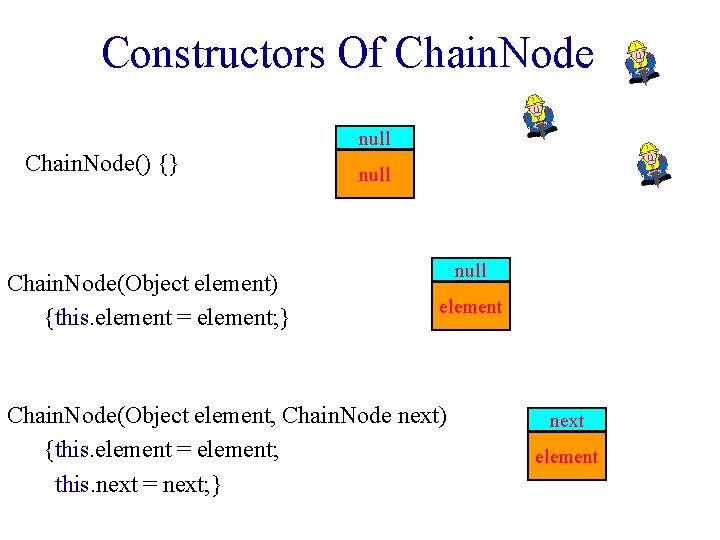
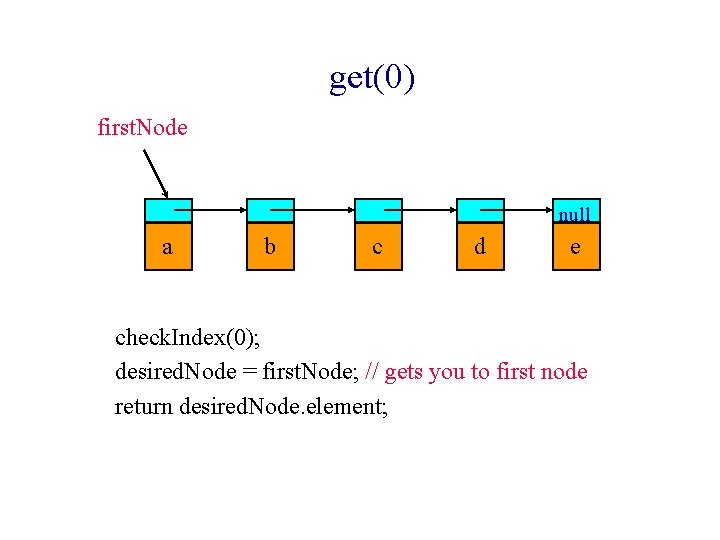
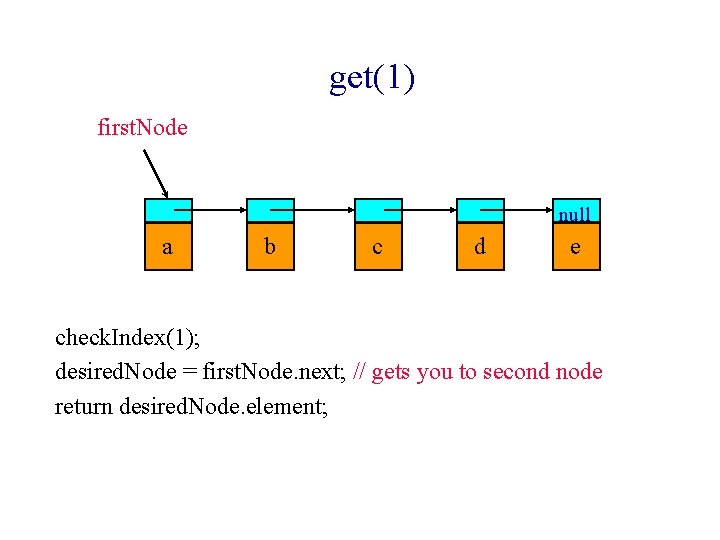
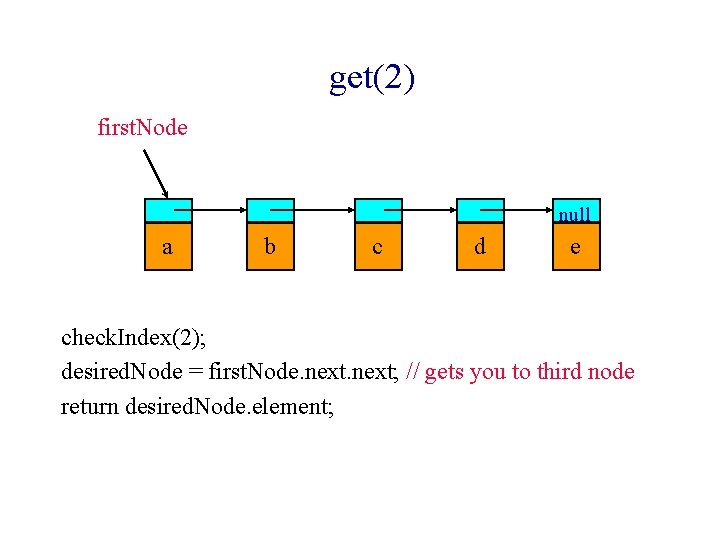
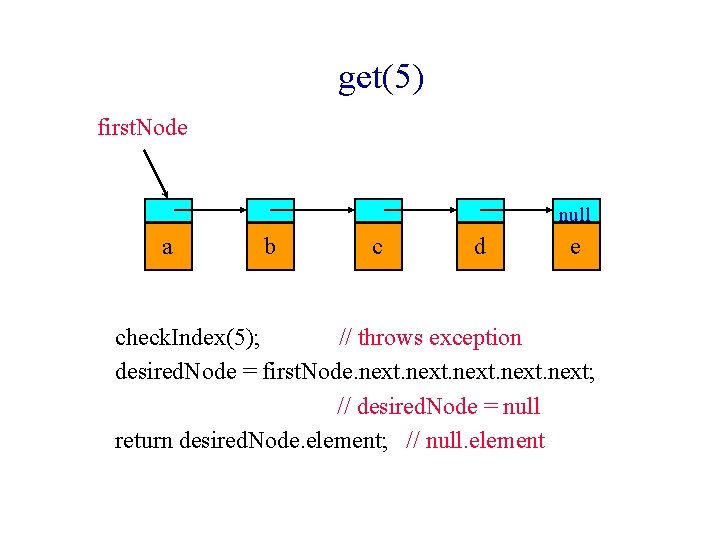
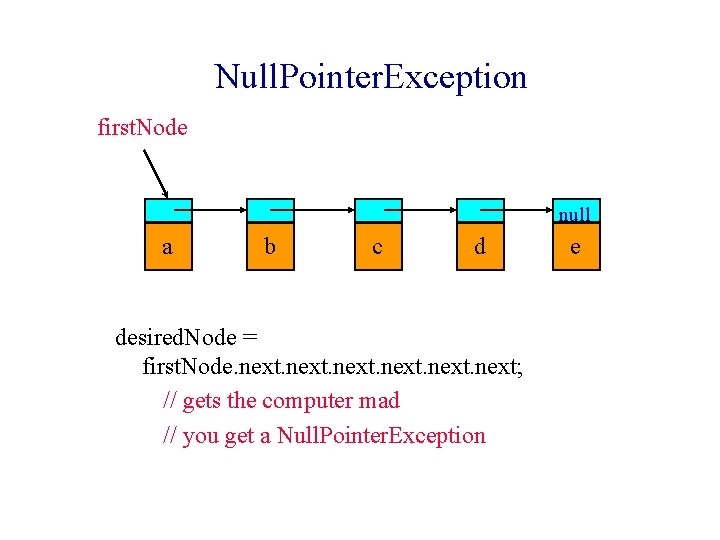
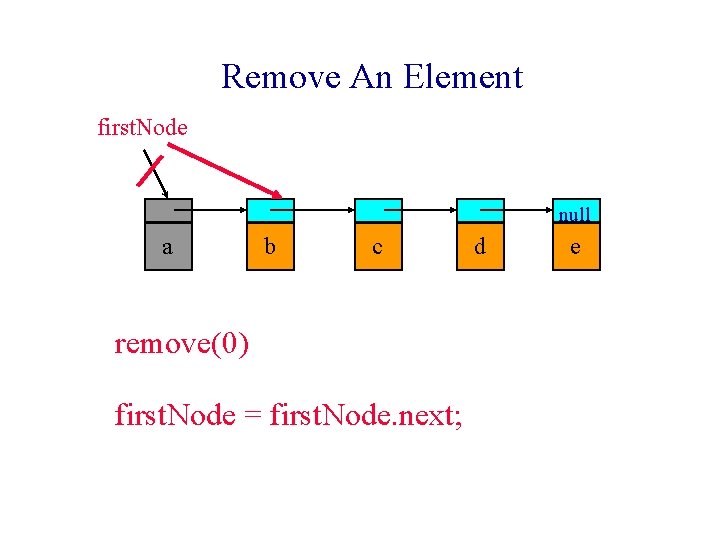
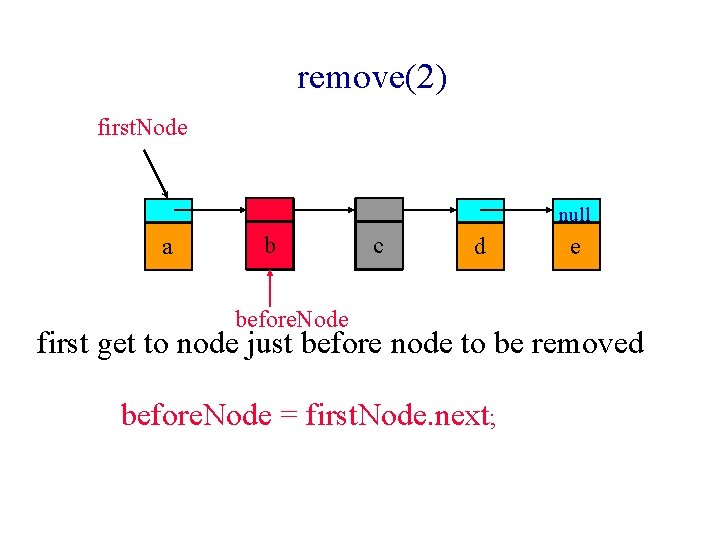
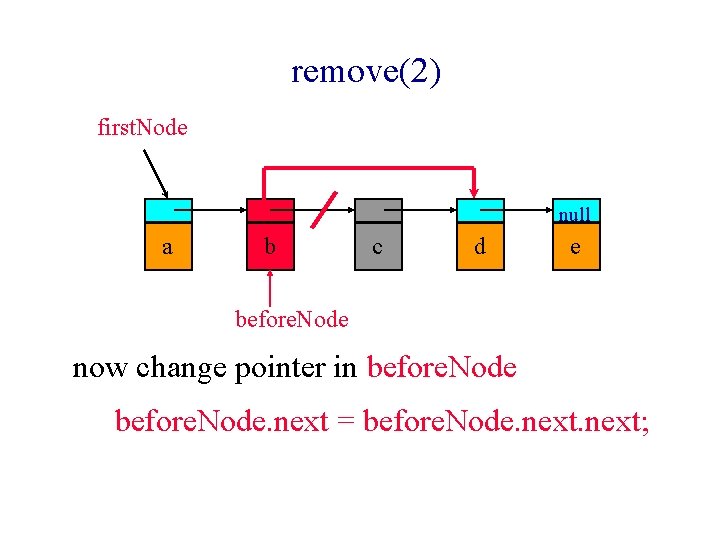
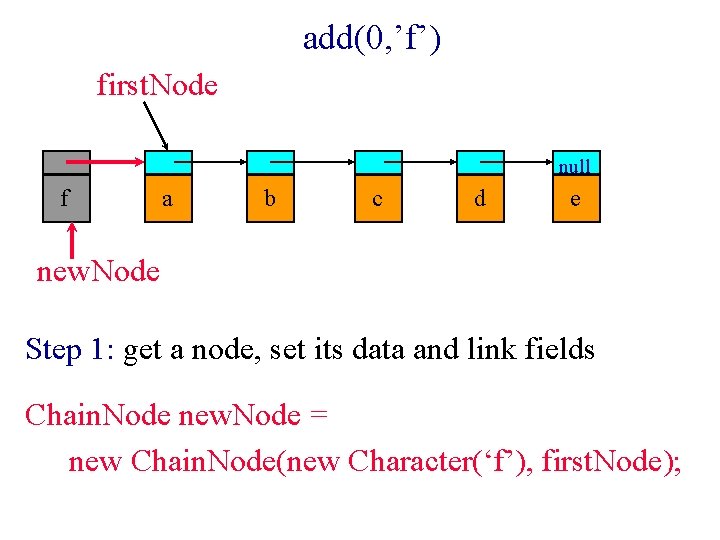
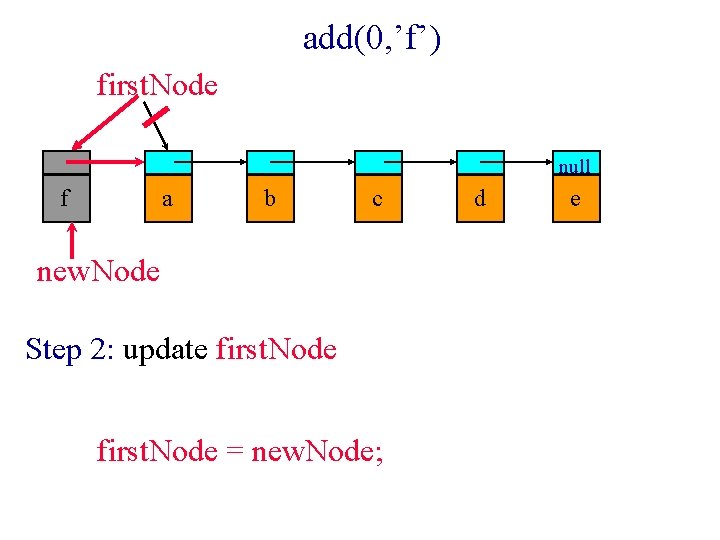
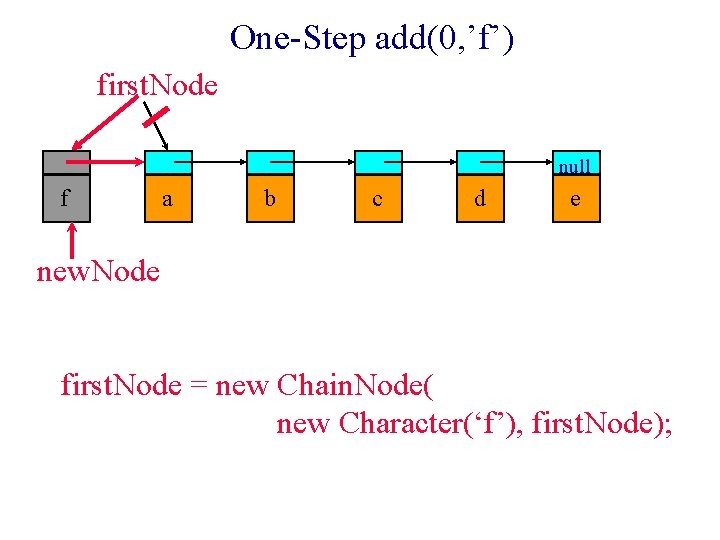
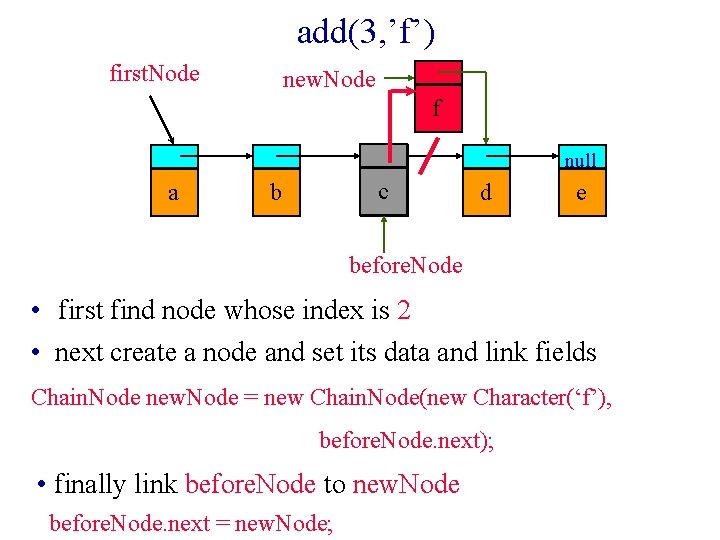
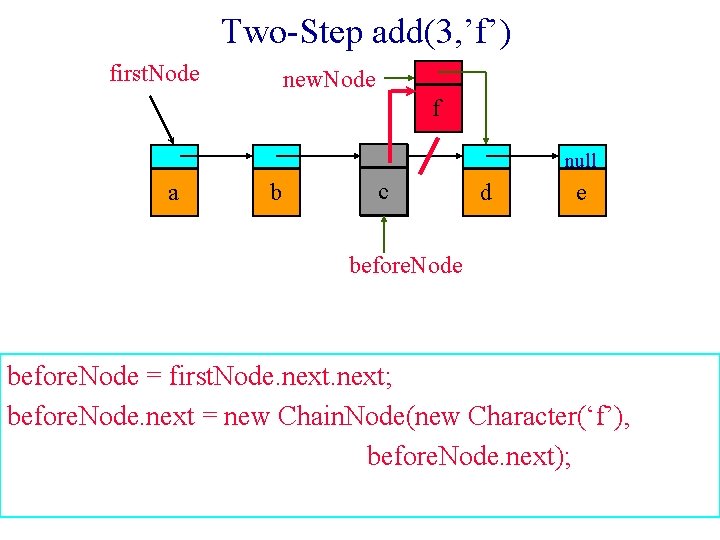
- Slides: 27
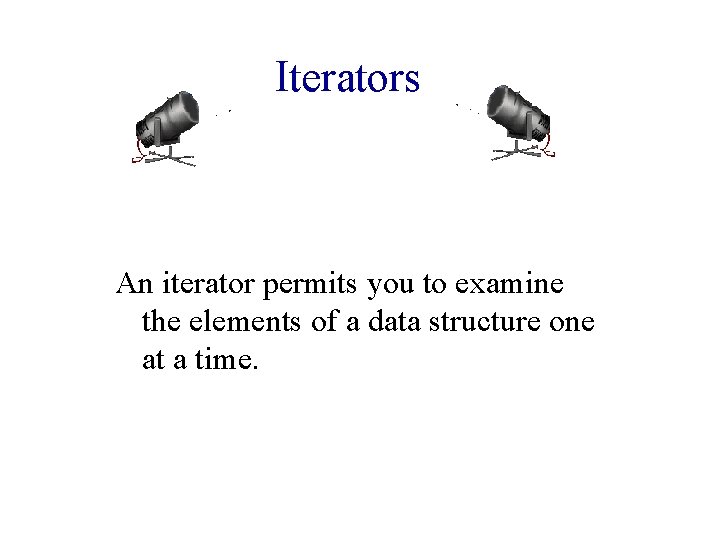
Iterators An iterator permits you to examine the elements of a data structure one at a time.
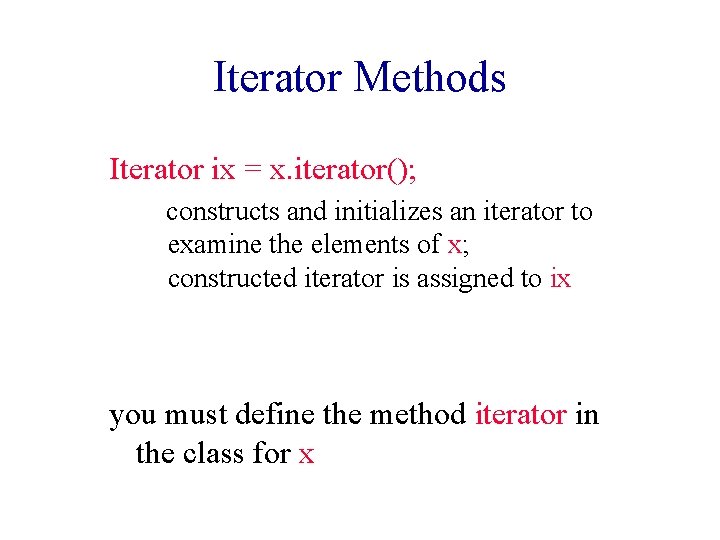
Iterator Methods Iterator ix = x. iterator(); constructs and initializes an iterator to examine the elements of x; constructed iterator is assigned to ix you must define the method iterator in the class for x
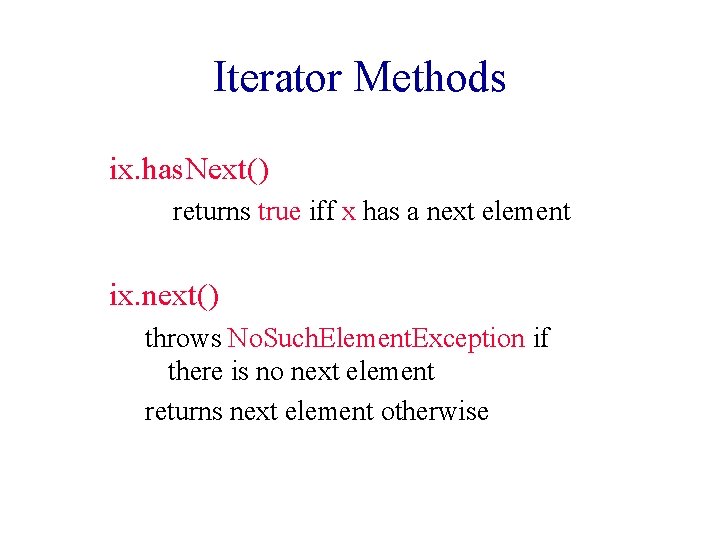
Iterator Methods ix. has. Next() returns true iff x has a next element ix. next() throws No. Such. Element. Exception if there is no next element returns next element otherwise
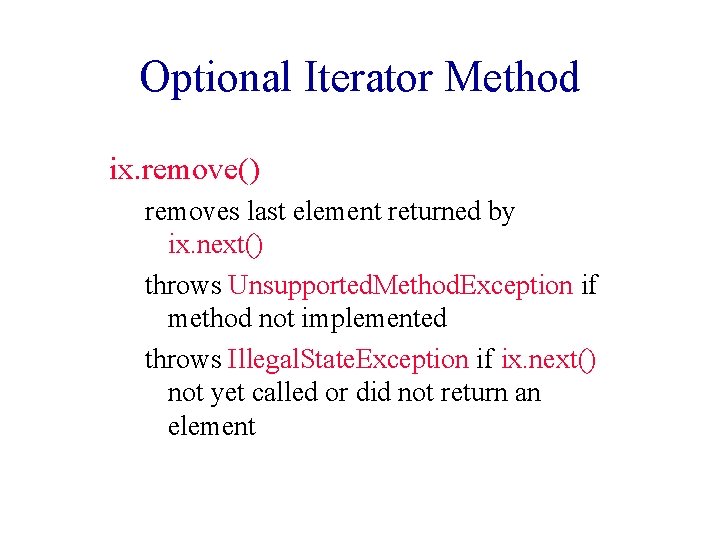
Optional Iterator Method ix. remove() removes last element returned by ix. next() throws Unsupported. Method. Exception if method not implemented throws Illegal. State. Exception if ix. next() not yet called or did not return an element
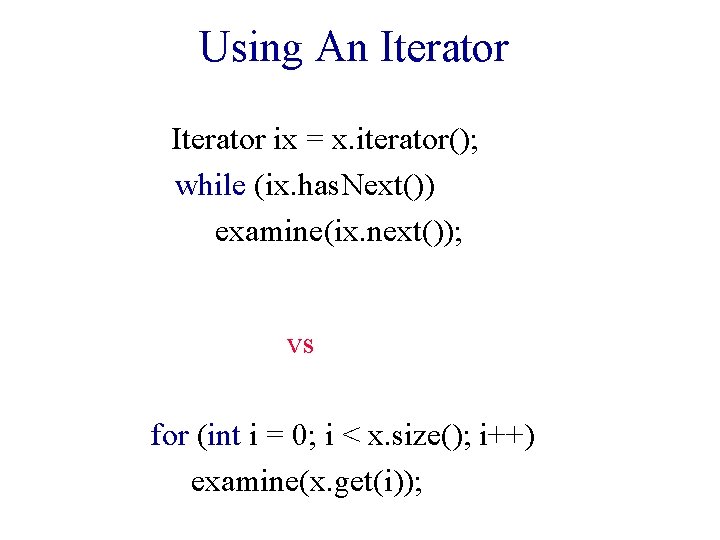
Using An Iterator ix = x. iterator(); while (ix. has. Next()) examine(ix. next()); vs for (int i = 0; i < x. size(); i++) examine(x. get(i));
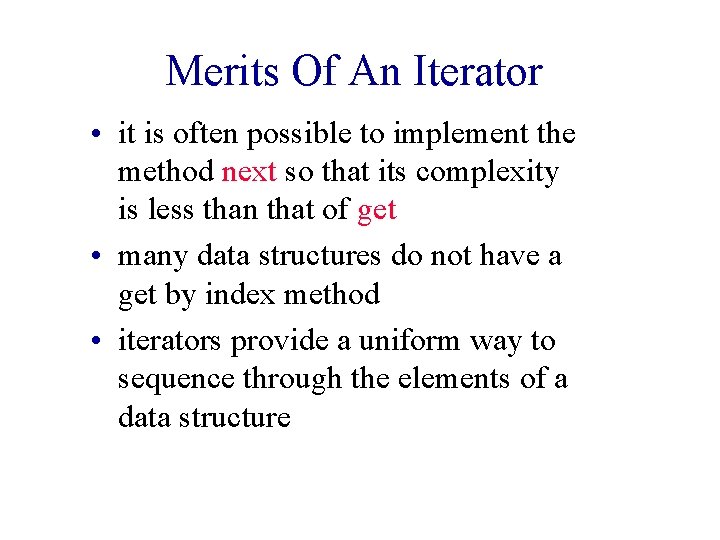
Merits Of An Iterator • it is often possible to implement the method next so that its complexity is less than that of get • many data structures do not have a get by index method • iterators provide a uniform way to sequence through the elements of a data structure
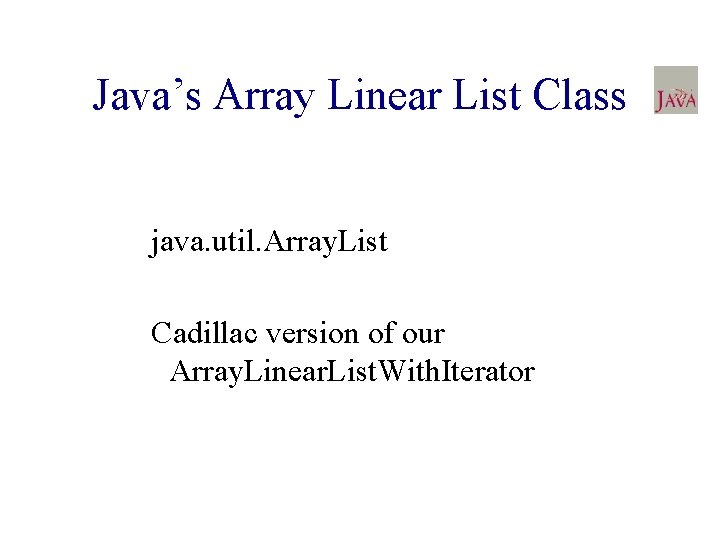
Java’s Array Linear List Class java. util. Array. List Cadillac version of our Array. Linear. List. With. Iterator
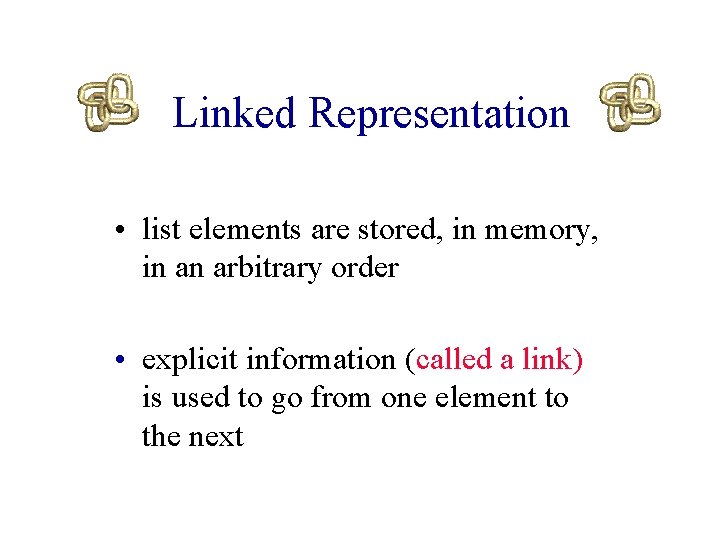
Linked Representation • list elements are stored, in memory, in an arbitrary order • explicit information (called a link) is used to go from one element to the next
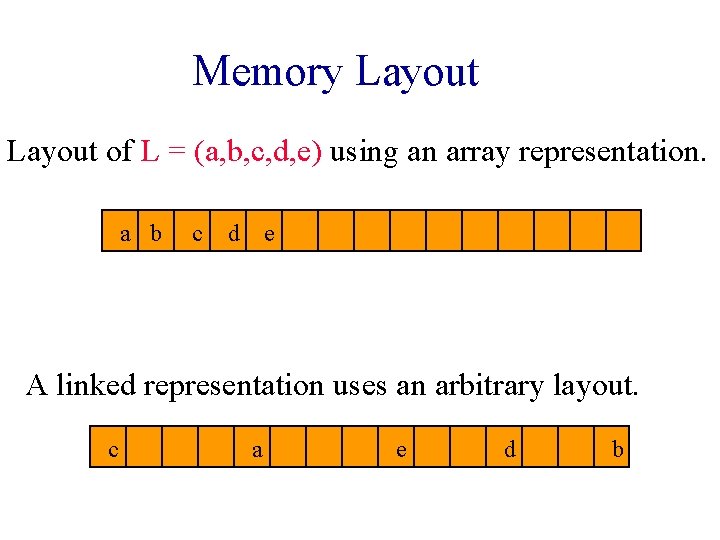
Memory Layout of L = (a, b, c, d, e) using an array representation. a b c d e A linked representation uses an arbitrary layout. c a e d b
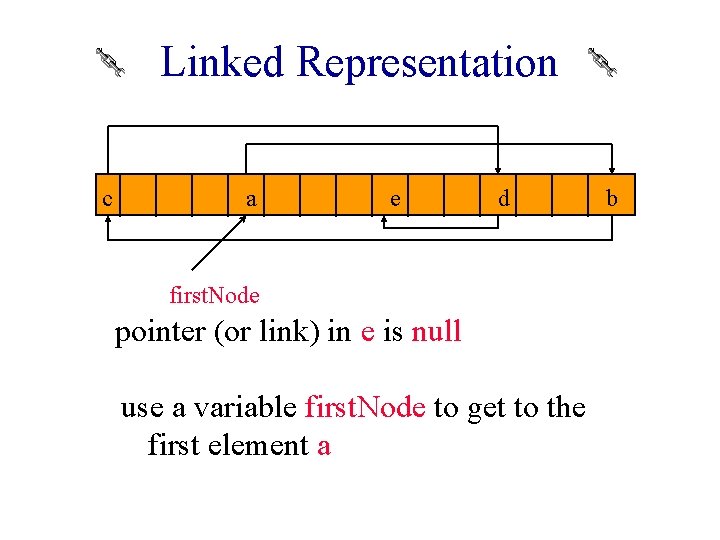
Linked Representation c a e d first. Node pointer (or link) in e is null use a variable first. Node to get to the first element a b
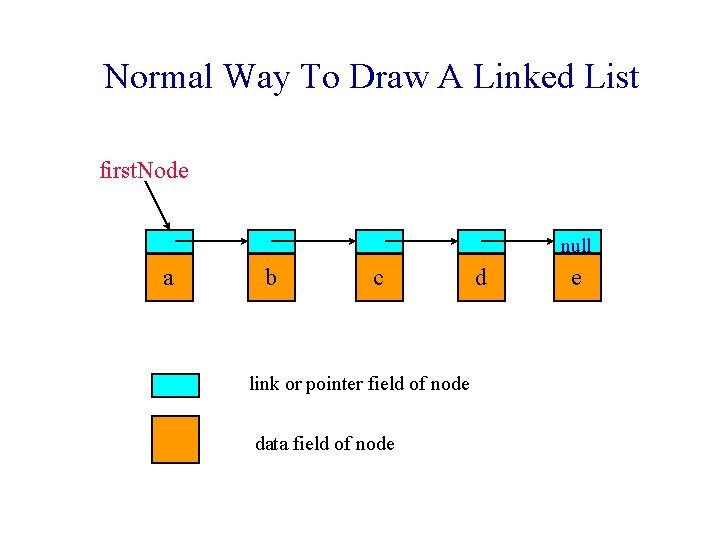
Normal Way To Draw A Linked List first. Node null a b c link or pointer field of node data field of node d e
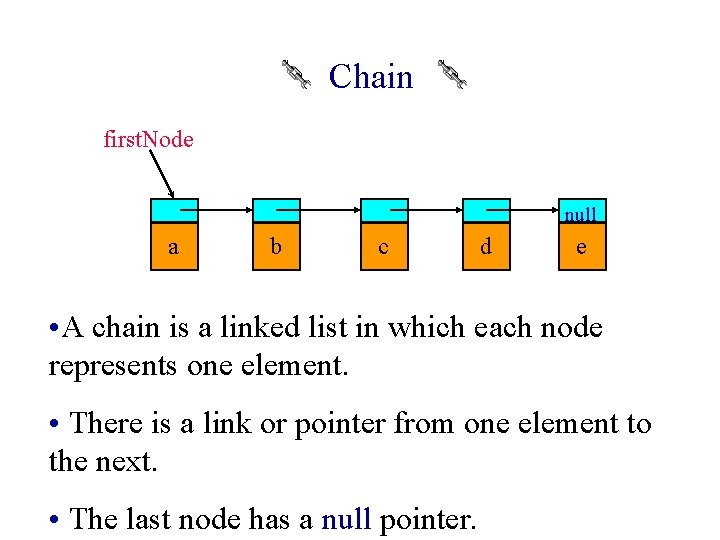
Chain first. Node null a b c d e • A chain is a linked list in which each node represents one element. • There is a link or pointer from one element to the next. • The last node has a null pointer.
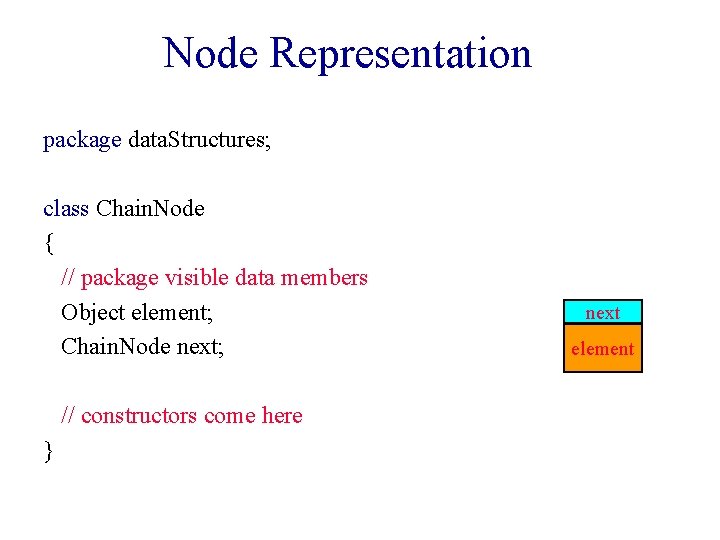
Node Representation package data. Structures; class Chain. Node { // package visible data members Object element; Chain. Node next; // constructors come here } next element
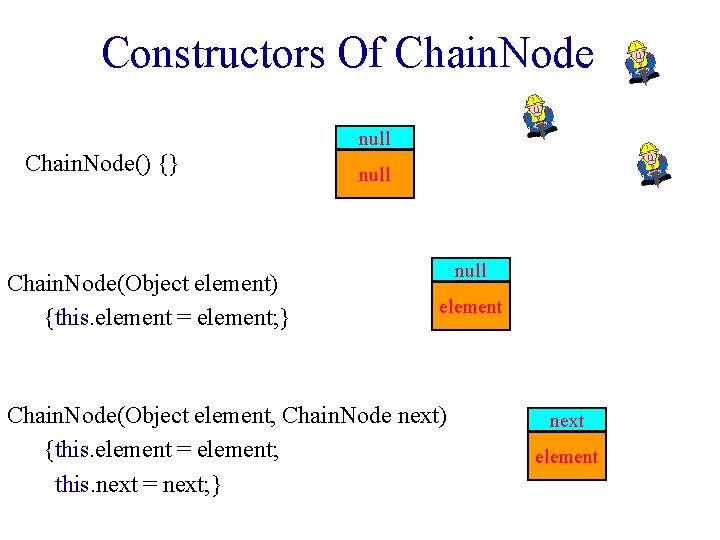
Constructors Of Chain. Node null Chain. Node() {} Chain. Node(Object element) {this. element = element; } null element Chain. Node(Object element, Chain. Node next) {this. element = element; this. next = next; } next element
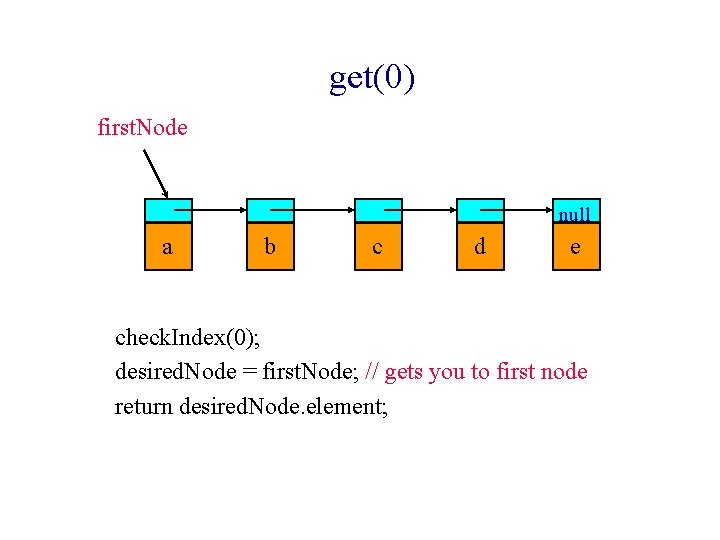
get(0) first. Node null a b c d e check. Index(0); desired. Node = first. Node; // gets you to first node return desired. Node. element;
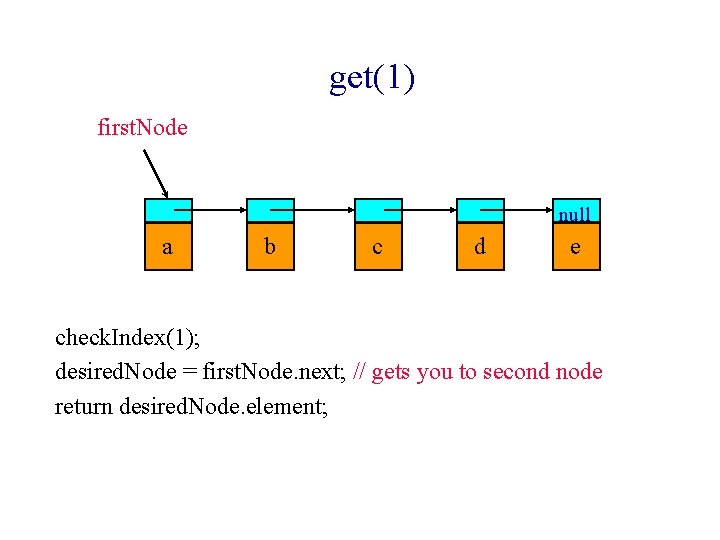
get(1) first. Node null a b c d e check. Index(1); desired. Node = first. Node. next; // gets you to second node return desired. Node. element;
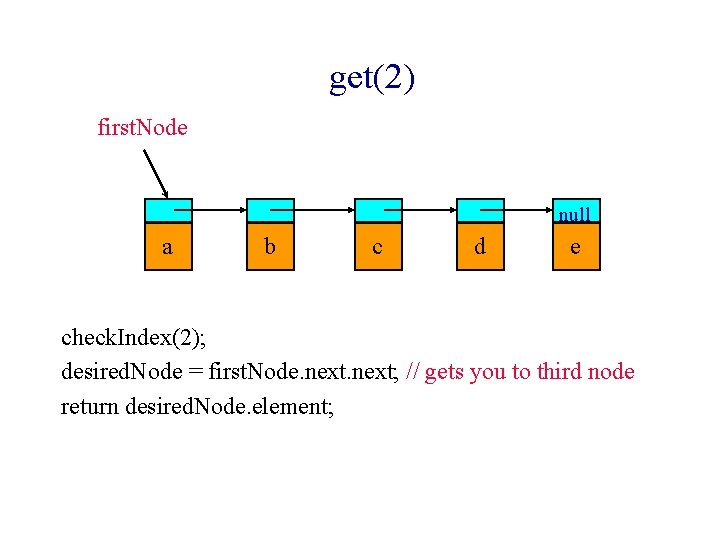
get(2) first. Node null a b c d e check. Index(2); desired. Node = first. Node. next; // gets you to third node return desired. Node. element;
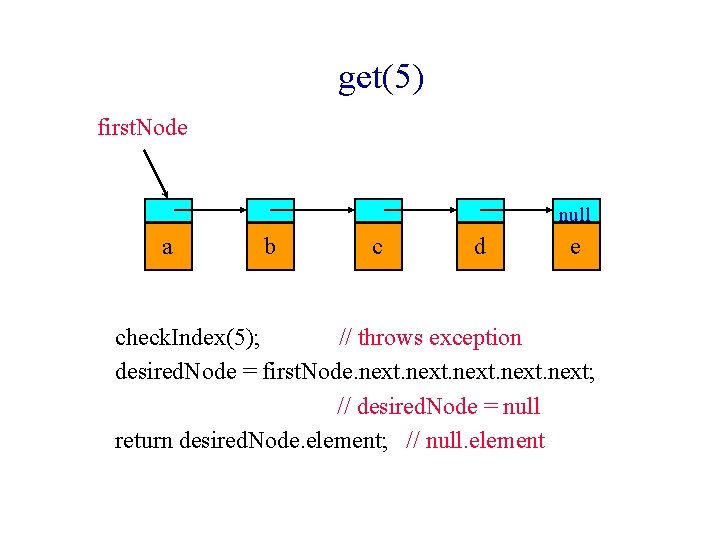
get(5) first. Node null a b c d e check. Index(5); // throws exception desired. Node = first. Node. next; // desired. Node = null return desired. Node. element; // null. element
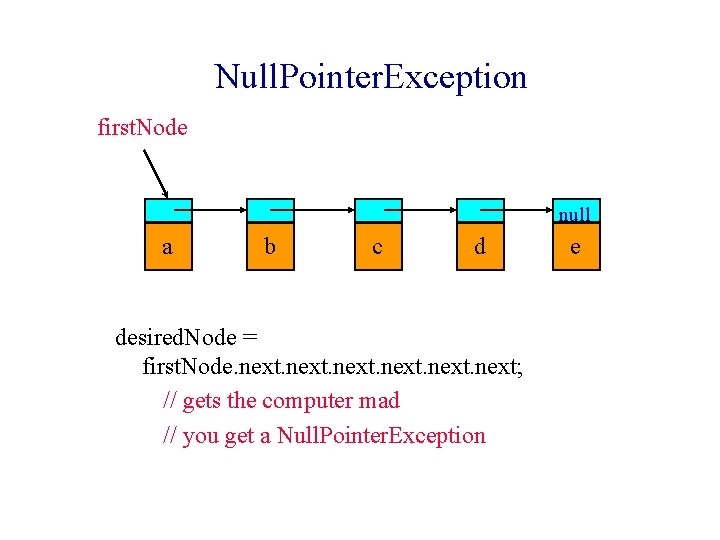
Null. Pointer. Exception first. Node null a b c d desired. Node = first. Node. next; // gets the computer mad // you get a Null. Pointer. Exception e
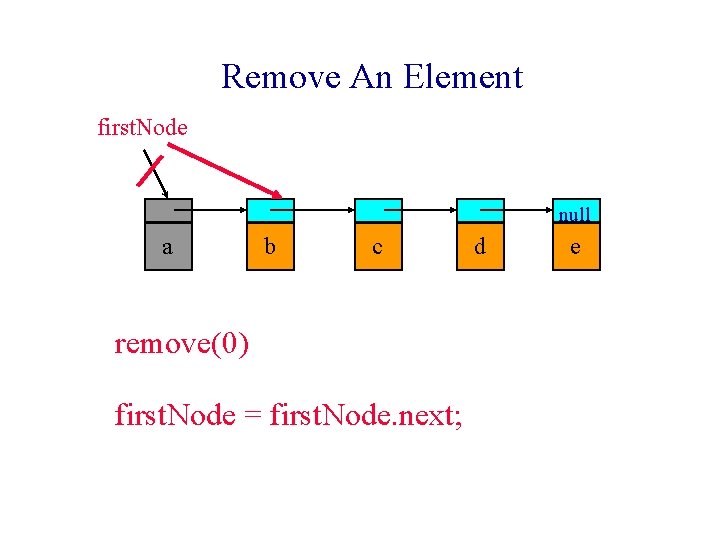
Remove An Element first. Node null a b c remove(0) first. Node = first. Node. next; d e
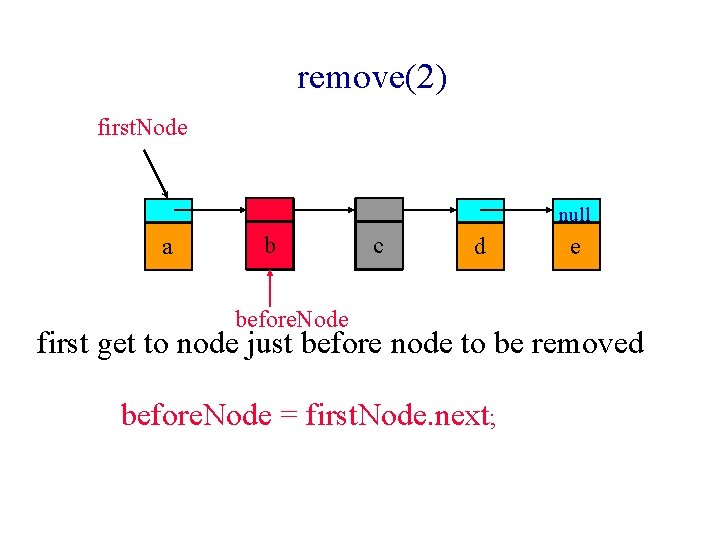
remove(2) first. Node a b c null c d before. Node e first get to node just before node to be removed before. Node = first. Node. next;
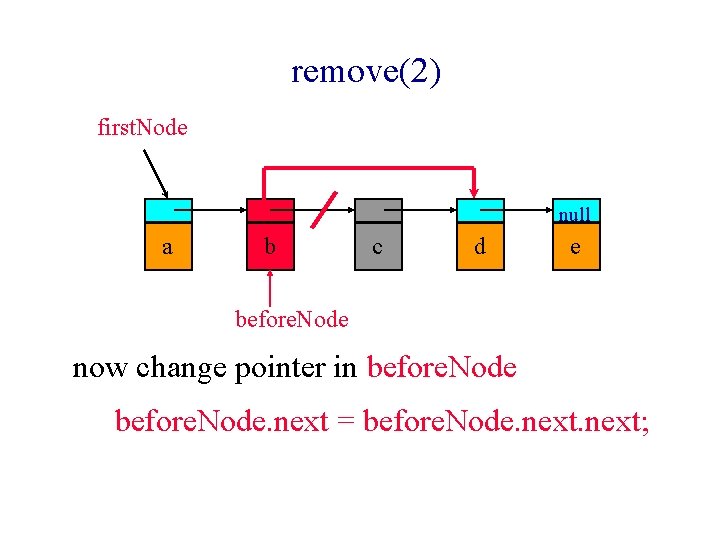
remove(2) first. Node null a b c d e before. Node now change pointer in before. Node. next = before. Node. next;
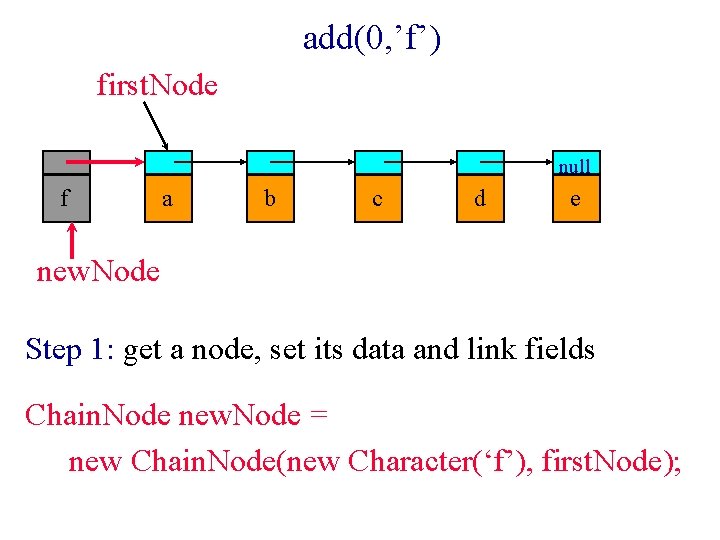
add(0, ’f’) first. Node null f a b c d e new. Node Step 1: get a node, set its data and link fields Chain. Node new. Node = new Chain. Node(new Character(‘f’), first. Node);
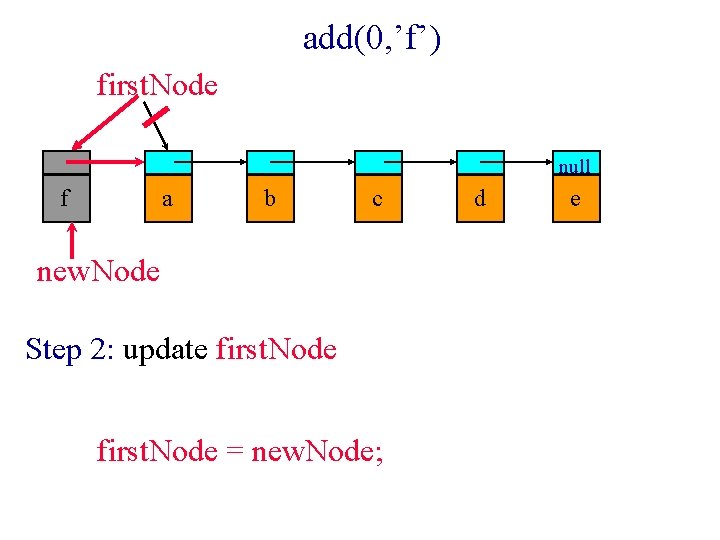
add(0, ’f’) first. Node null f a b c new. Node Step 2: update first. Node = new. Node; d e
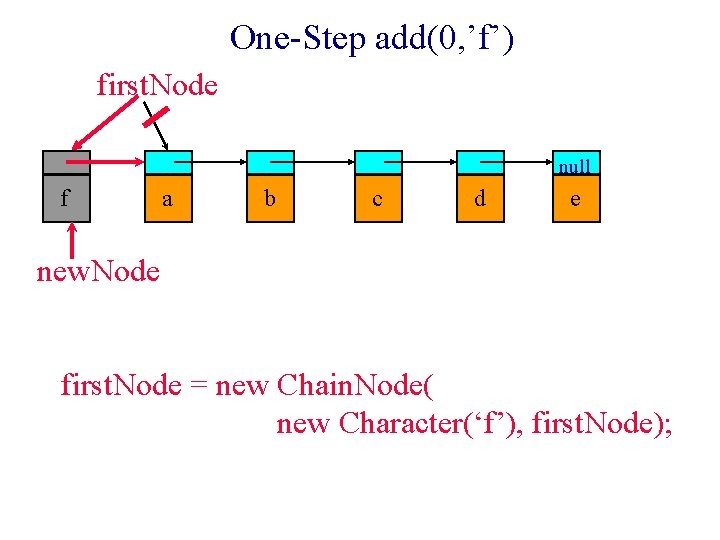
One-Step add(0, ’f’) first. Node null f a b c d e new. Node first. Node = new Chain. Node( new Character(‘f’), first. Node);
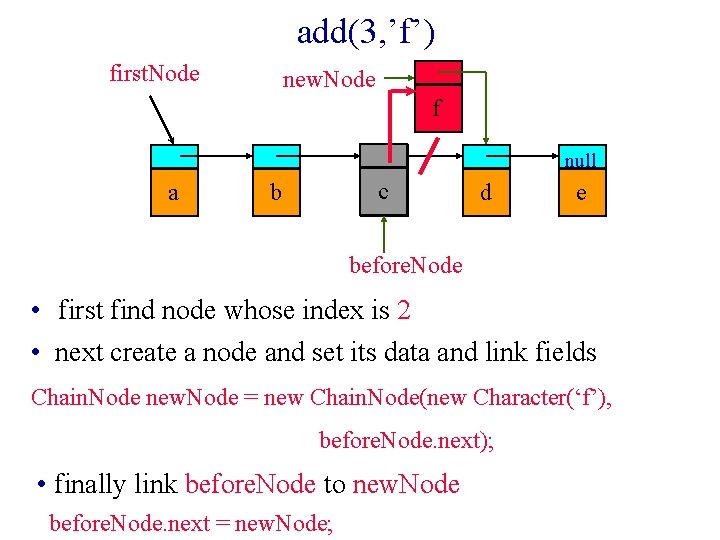
add(3, ’f’) first. Node new. Node f null a c b d e before. Node • first find node whose index is 2 • next create a node and set its data and link fields Chain. Node new. Node = new Chain. Node(new Character(‘f’), before. Node. next); • finally link before. Node to new. Node before. Node. next = new. Node;
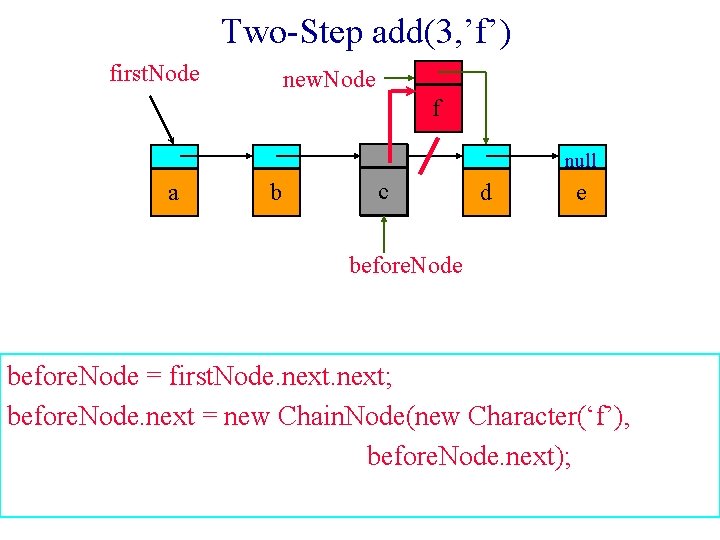
Two-Step add(3, ’f’) first. Node new. Node f null a b c d e before. Node = first. Node. next; before. Node. next = new Chain. Node(new Character(‘f’), before. Node. next);
Maps and sets support bidirectional iterators.
Python iterators and generators
Iterator inner class java
Python iterator pattern
Inorder iterator
Rapid json
Iterator entwurfsmuster
Iterator
Volcano iterator model
Zig iterator
Informatica def
Code blocks in python
Examine yourselves to see if you are in the faith
St mary's county tree removal permit
If time permits quotes
Alabama oversize regulations
Riverside building and safety
Permits meaning
Title v air permits 101
The material that permits the flow of electricity
Texas air quality permits
Texas railroad commission pipeline permits
Modot motor carrier services
Transferable discharge permits
Riverside permit
What exception if any permits a private pilot
Denver quick permits
Apras permits