Iterators Chain Variants Iterators An iterator permits you
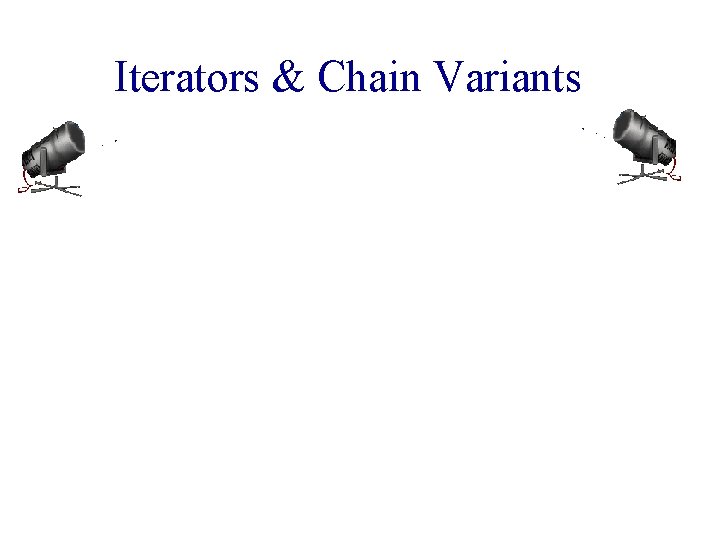
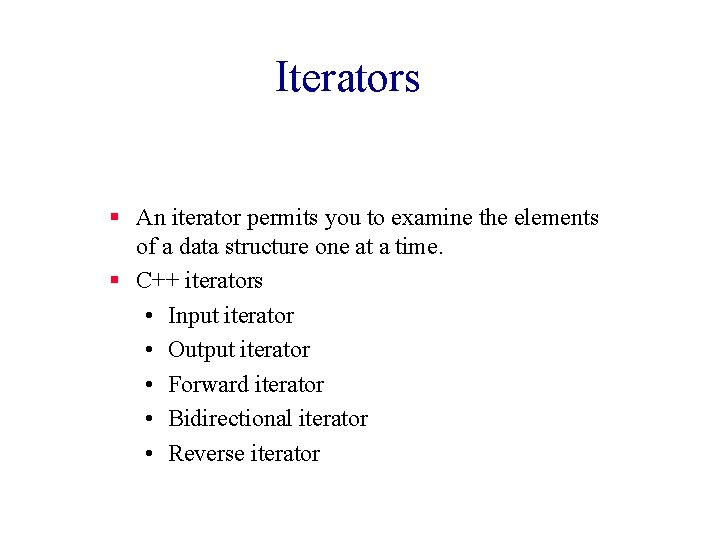
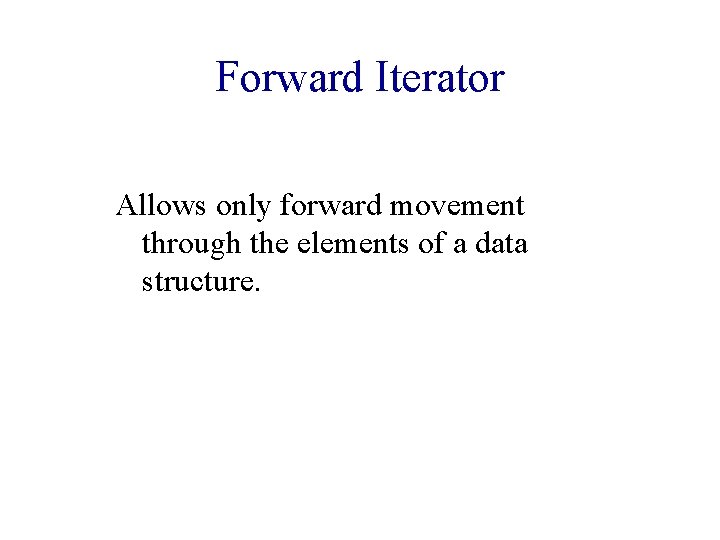
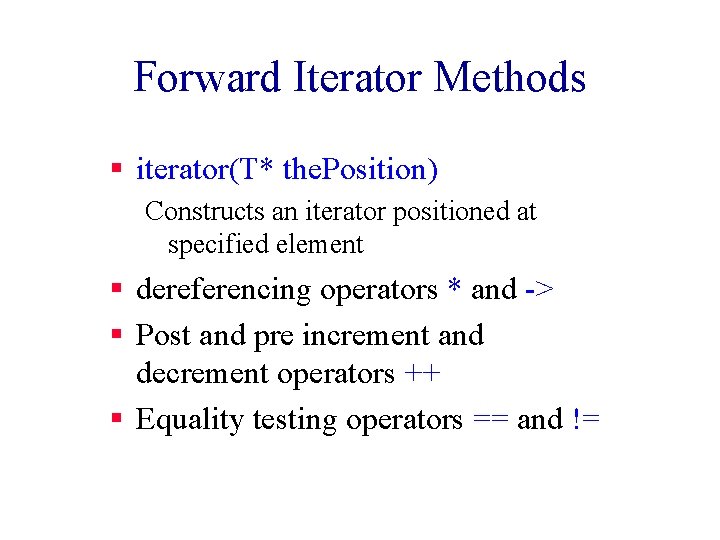
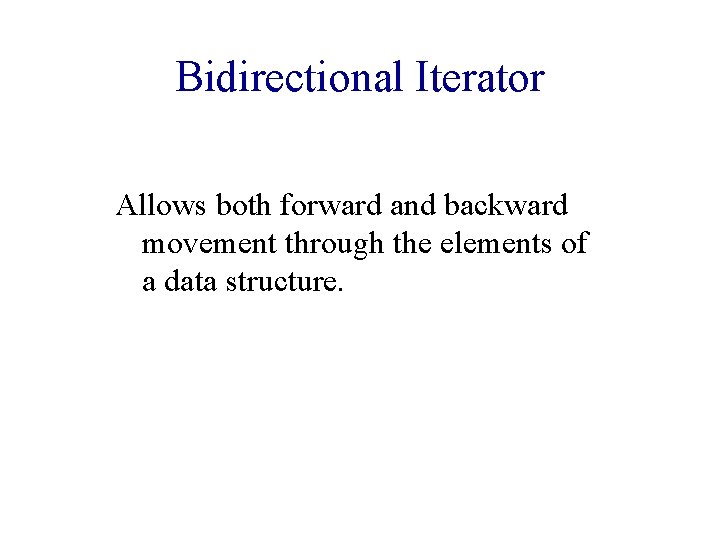
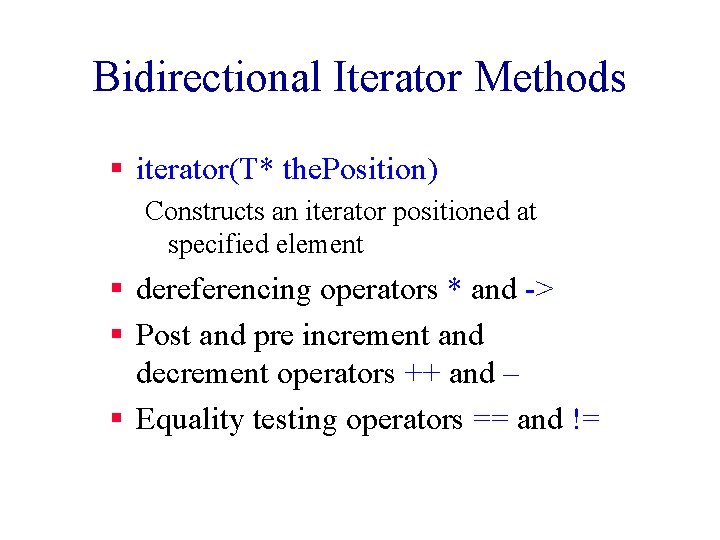
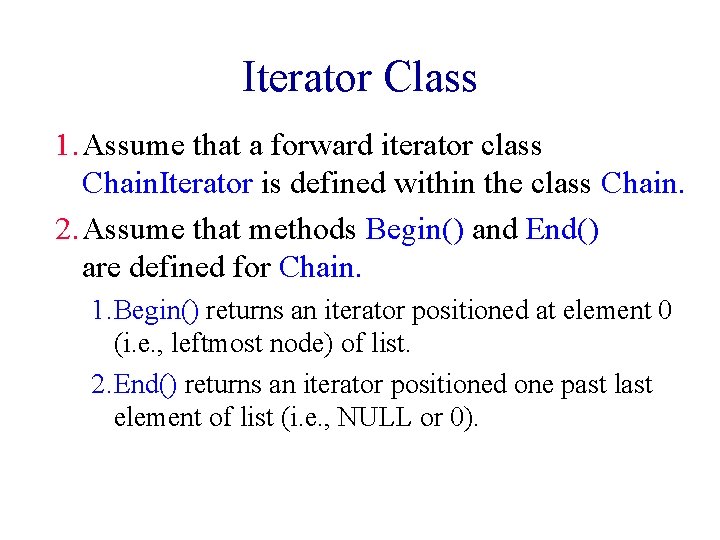
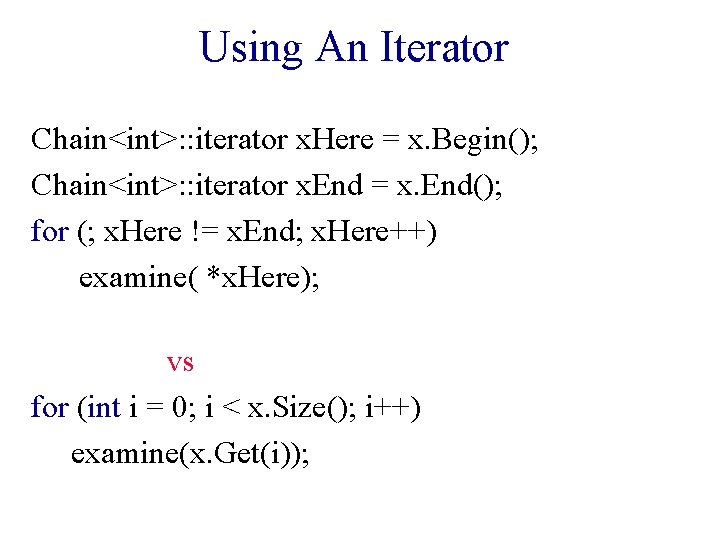
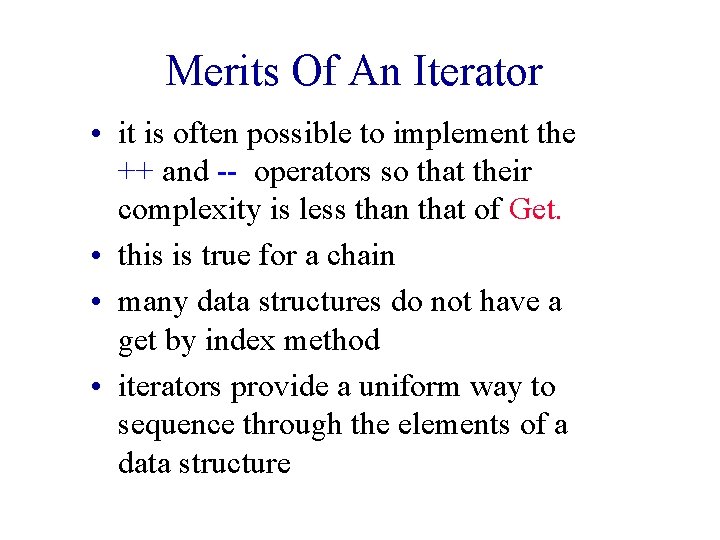
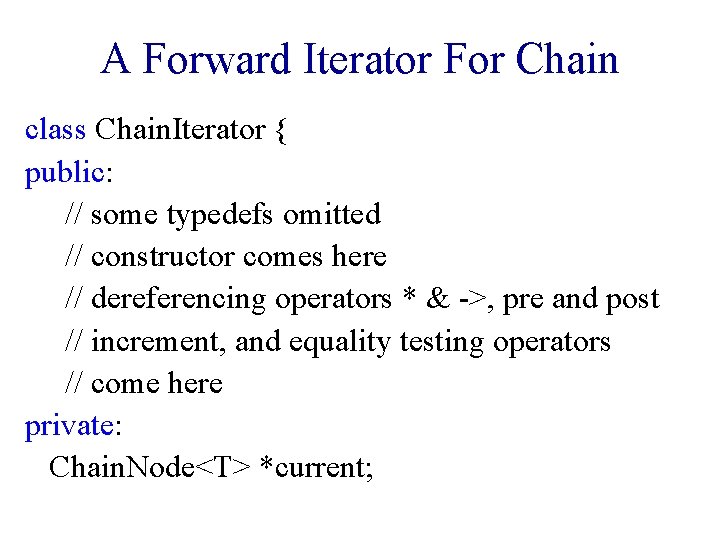
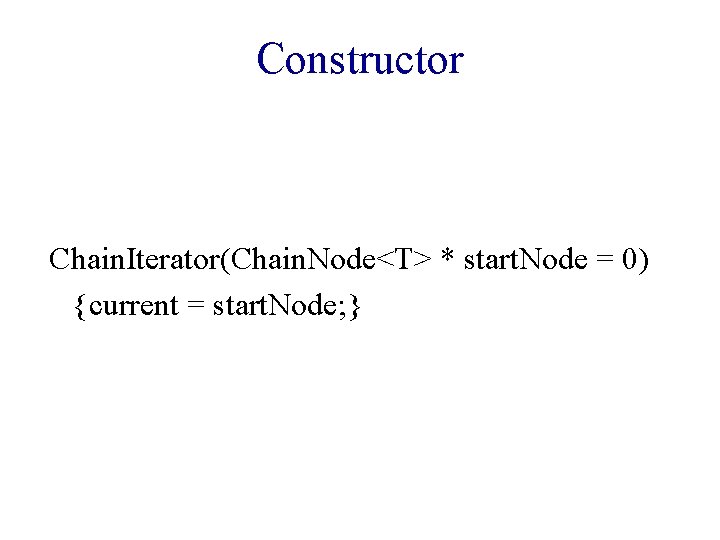
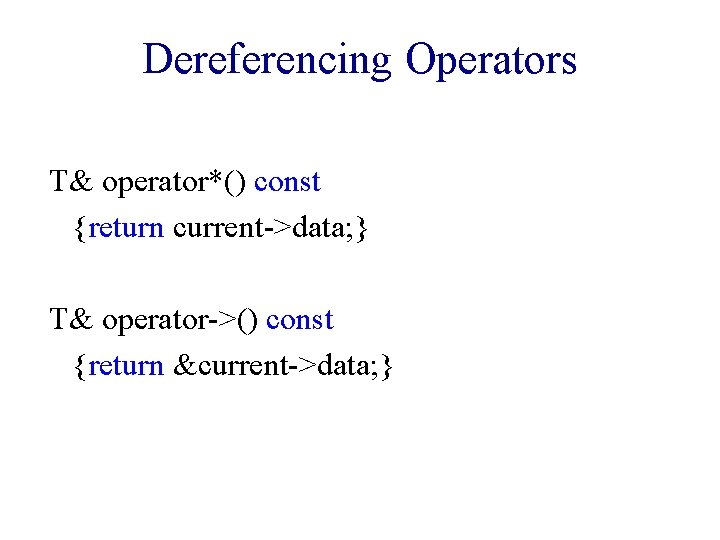
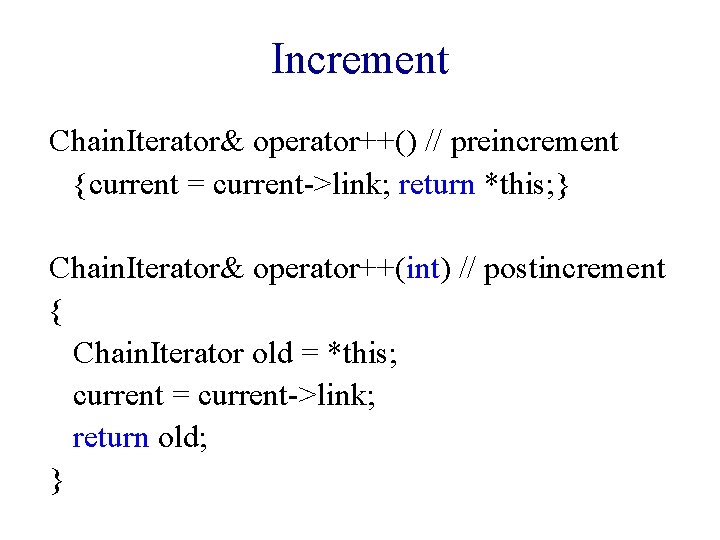
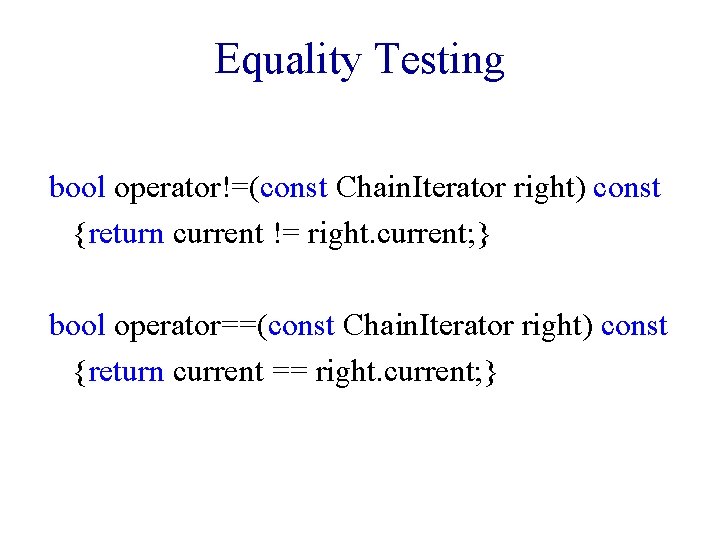
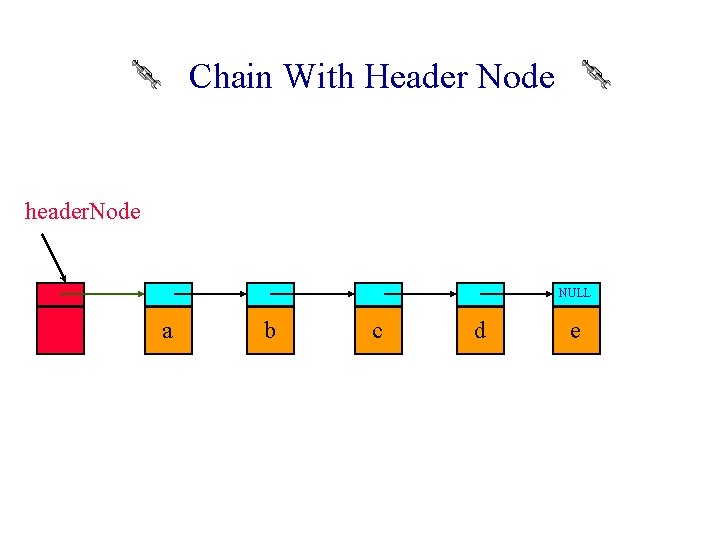
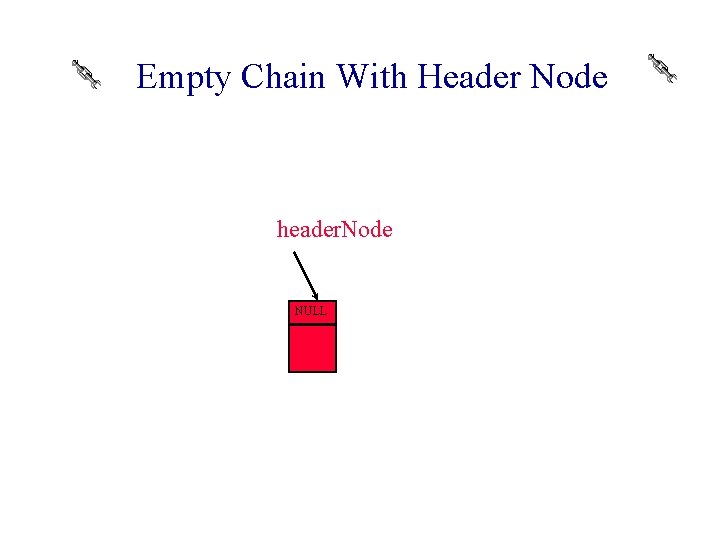
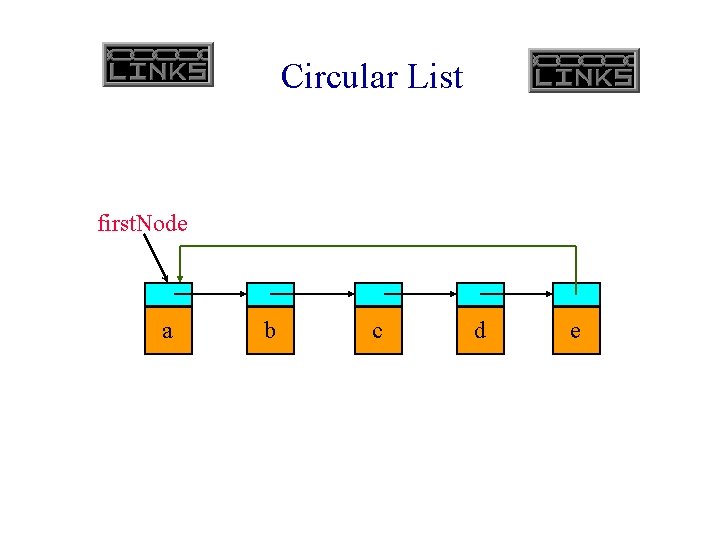
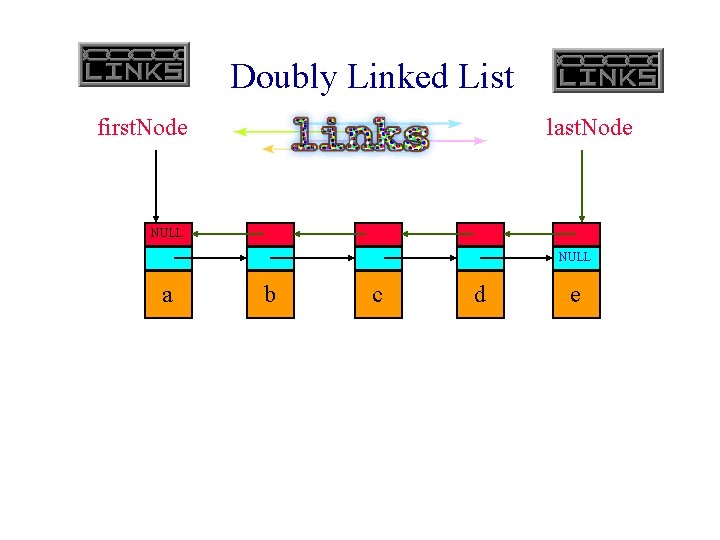
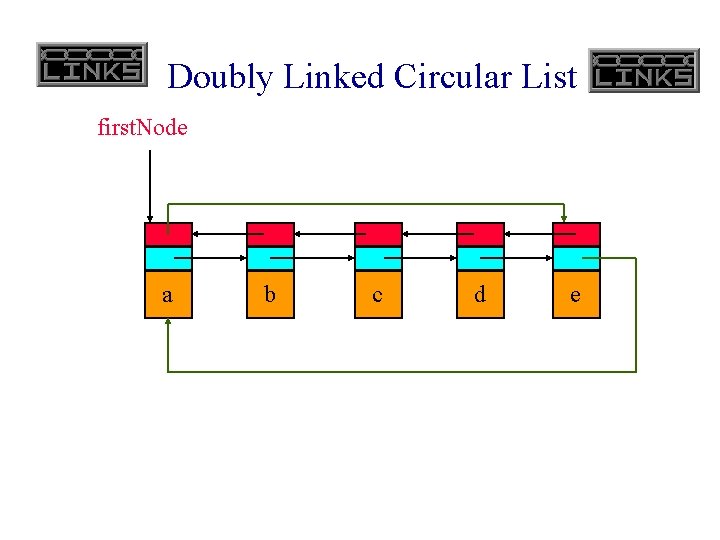
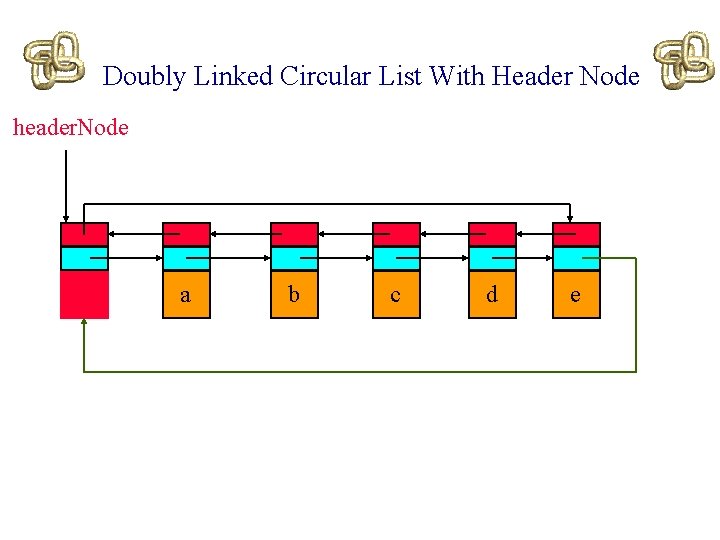
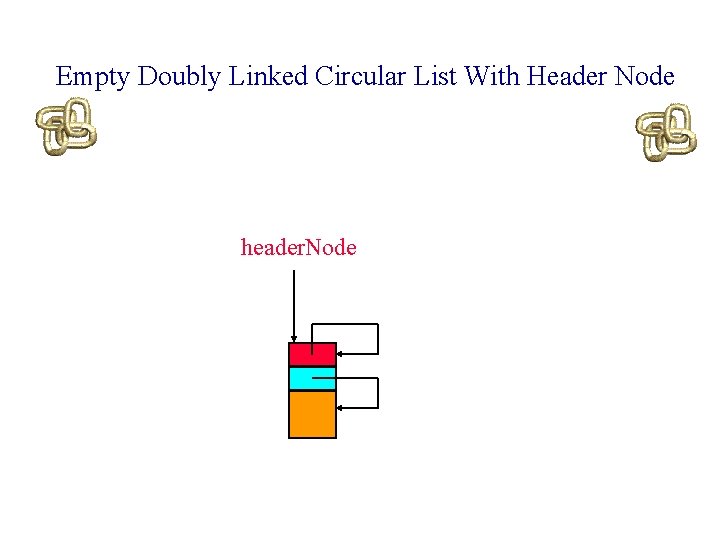
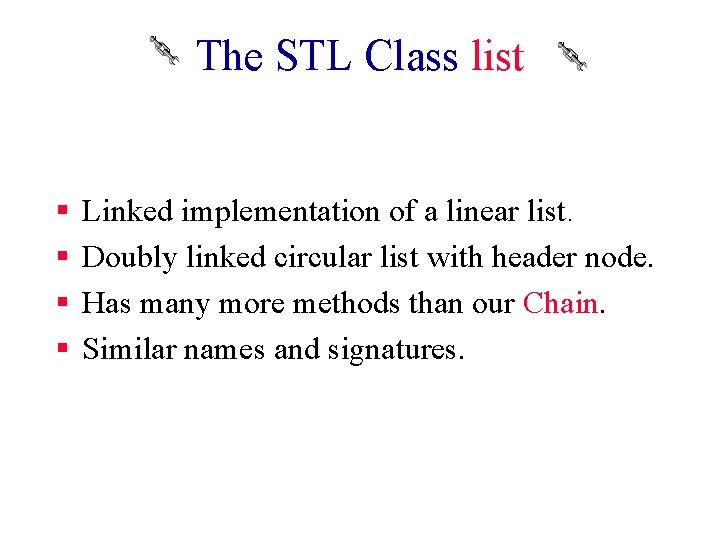
- Slides: 22
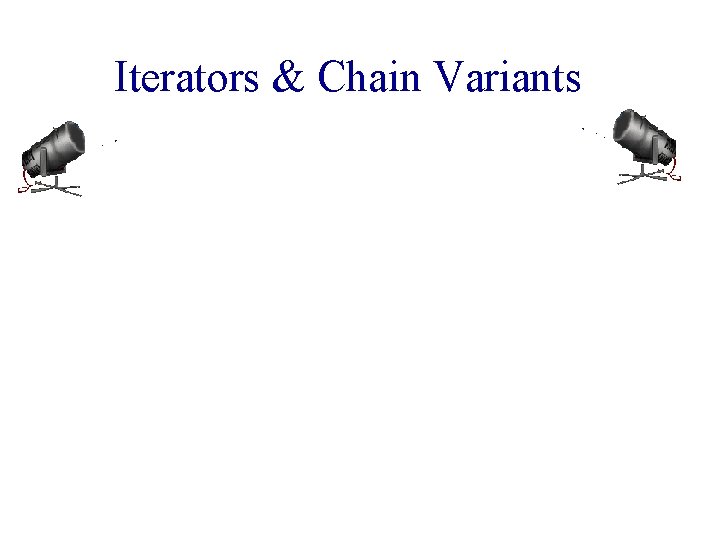
Iterators & Chain Variants
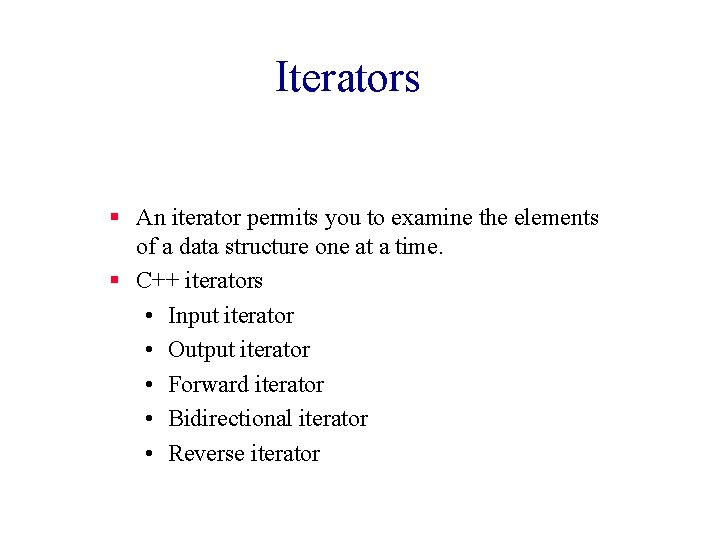
Iterators § An iterator permits you to examine the elements of a data structure one at a time. § C++ iterators • Input iterator • Output iterator • Forward iterator • Bidirectional iterator • Reverse iterator
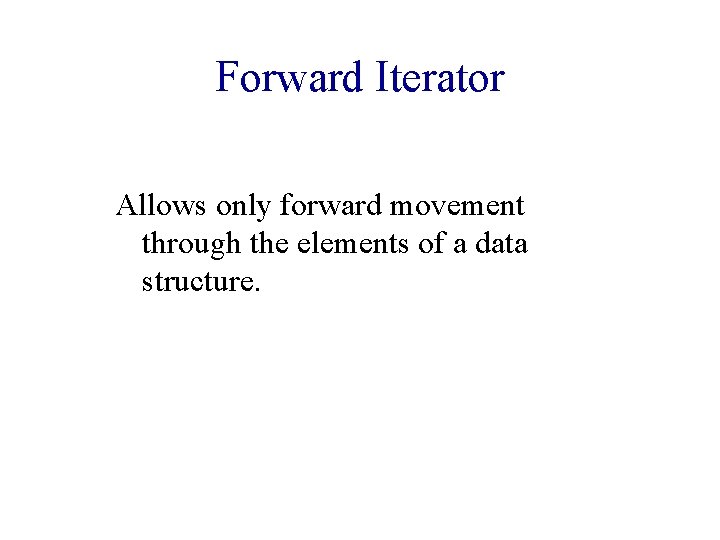
Forward Iterator Allows only forward movement through the elements of a data structure.
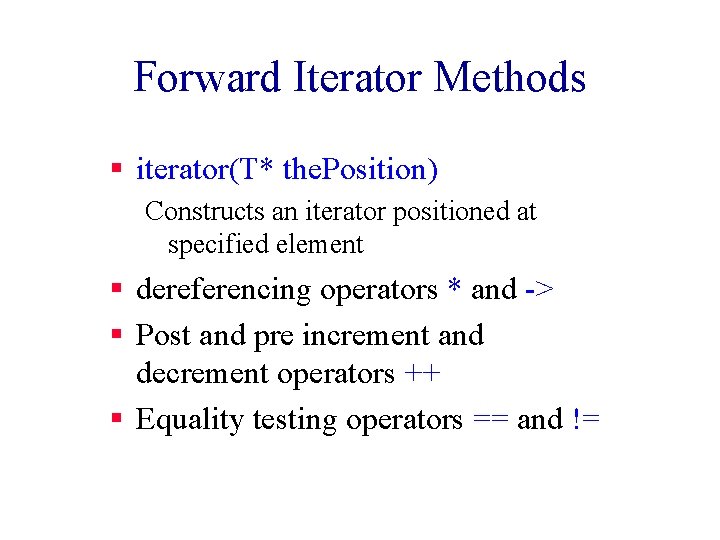
Forward Iterator Methods § iterator(T* the. Position) Constructs an iterator positioned at specified element § dereferencing operators * and -> § Post and pre increment and decrement operators ++ § Equality testing operators == and !=
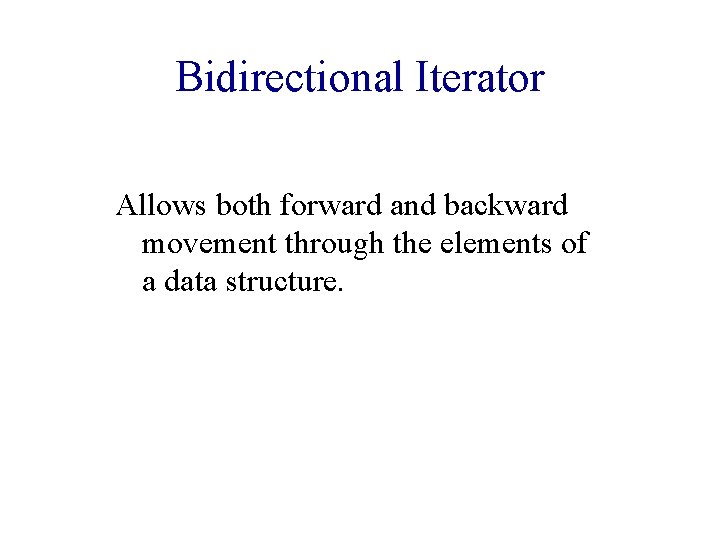
Bidirectional Iterator Allows both forward and backward movement through the elements of a data structure.
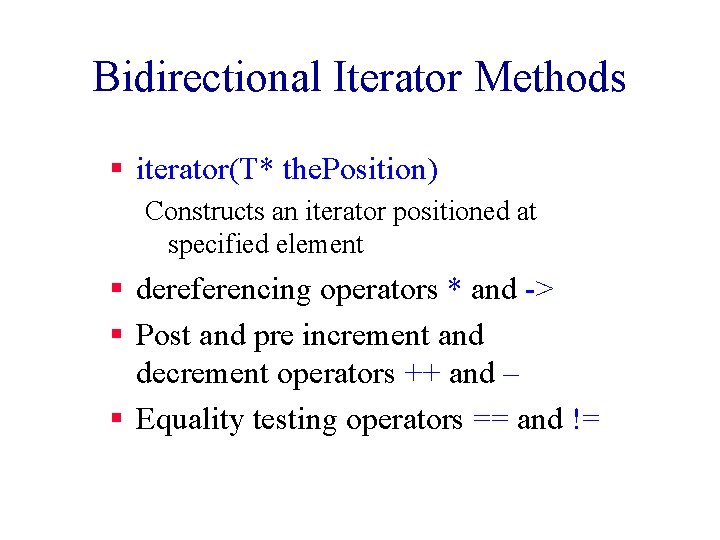
Bidirectional Iterator Methods § iterator(T* the. Position) Constructs an iterator positioned at specified element § dereferencing operators * and -> § Post and pre increment and decrement operators ++ and – § Equality testing operators == and !=
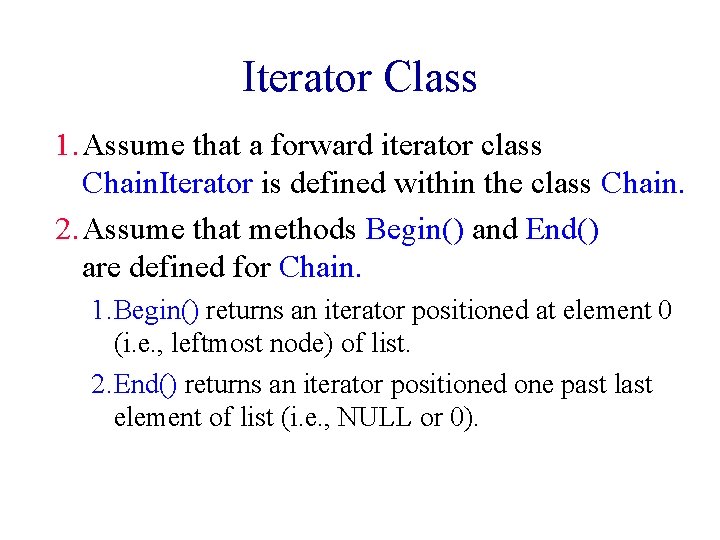
Iterator Class 1. Assume that a forward iterator class Chain. Iterator is defined within the class Chain. 2. Assume that methods Begin() and End() are defined for Chain. 1. Begin() returns an iterator positioned at element 0 (i. e. , leftmost node) of list. 2. End() returns an iterator positioned one past last element of list (i. e. , NULL or 0).
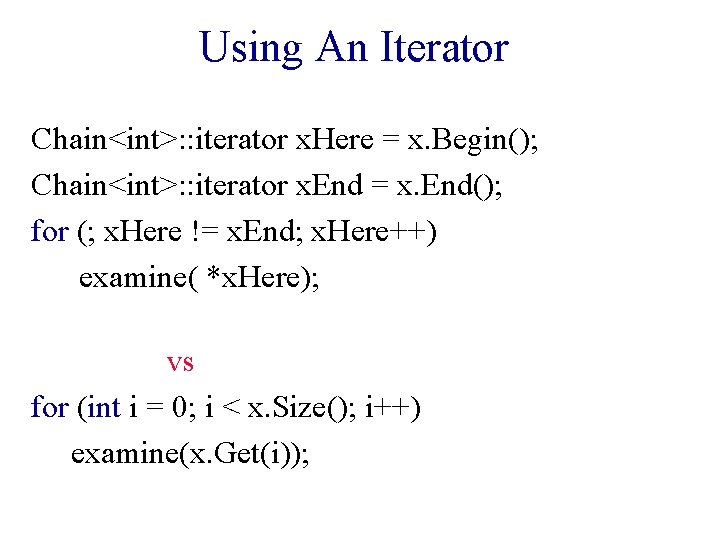
Using An Iterator Chain<int>: : iterator x. Here = x. Begin(); Chain<int>: : iterator x. End = x. End(); for (; x. Here != x. End; x. Here++) examine( *x. Here); vs for (int i = 0; i < x. Size(); i++) examine(x. Get(i));
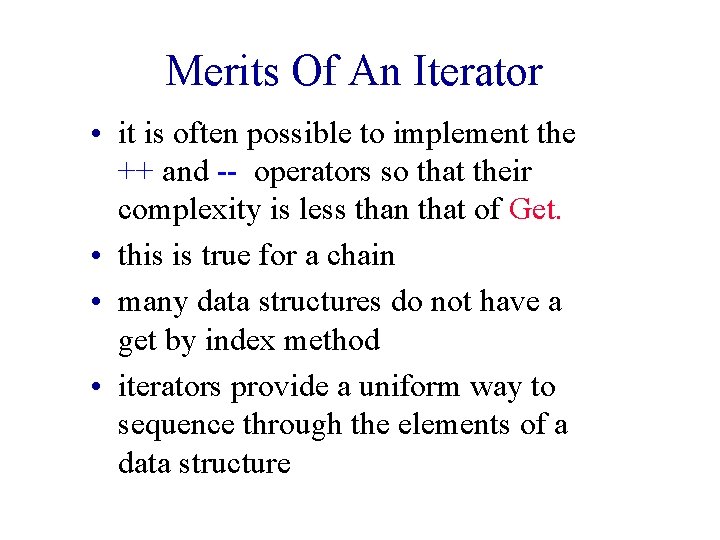
Merits Of An Iterator • it is often possible to implement the ++ and -- operators so that their complexity is less than that of Get. • this is true for a chain • many data structures do not have a get by index method • iterators provide a uniform way to sequence through the elements of a data structure
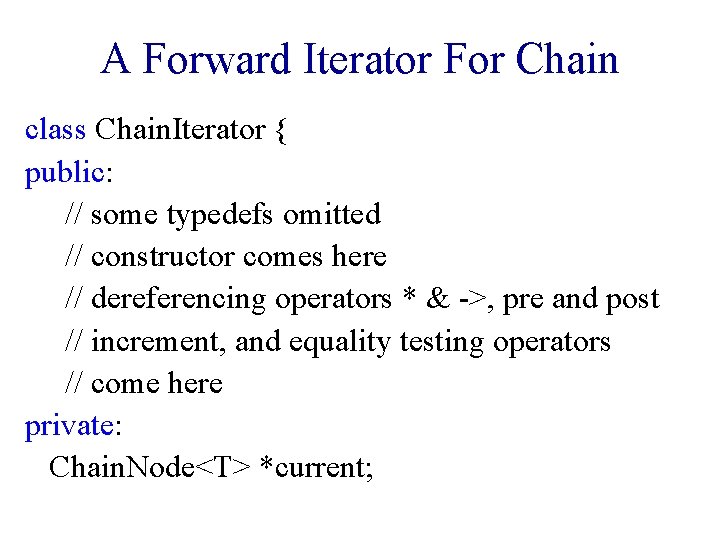
A Forward Iterator For Chain class Chain. Iterator { public: // some typedefs omitted // constructor comes here // dereferencing operators * & ->, pre and post // increment, and equality testing operators // come here private: Chain. Node<T> *current;
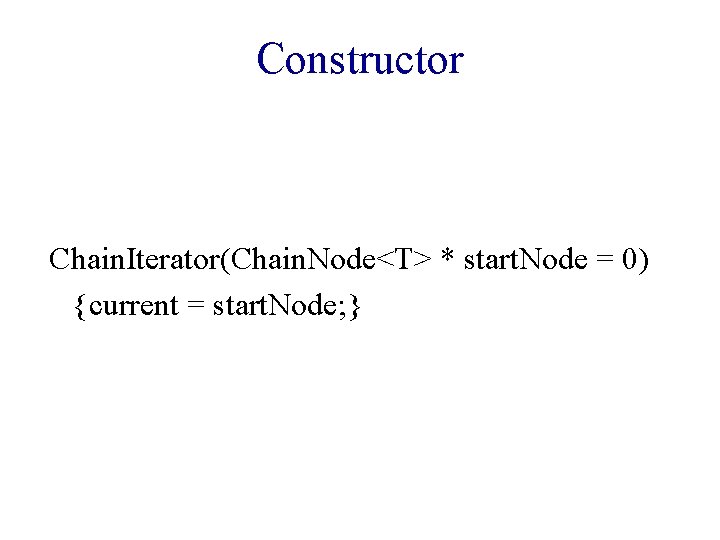
Constructor Chain. Iterator(Chain. Node<T> * start. Node = 0) {current = start. Node; }
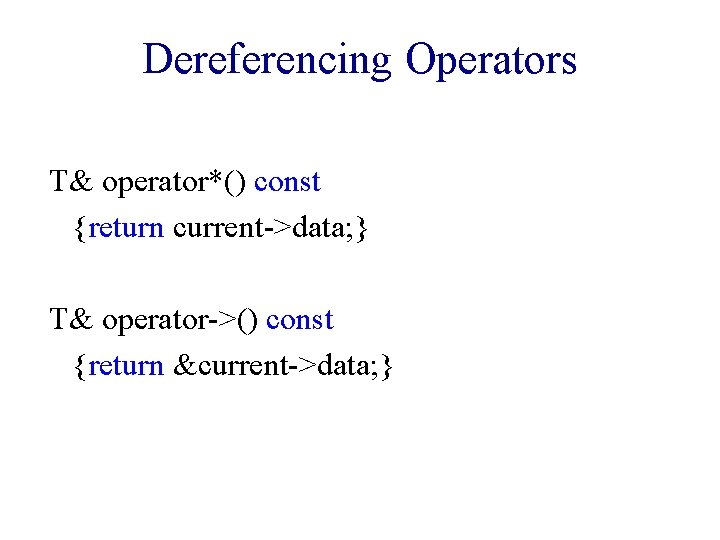
Dereferencing Operators T& operator*() const {return current->data; } T& operator->() const {return ¤t->data; }
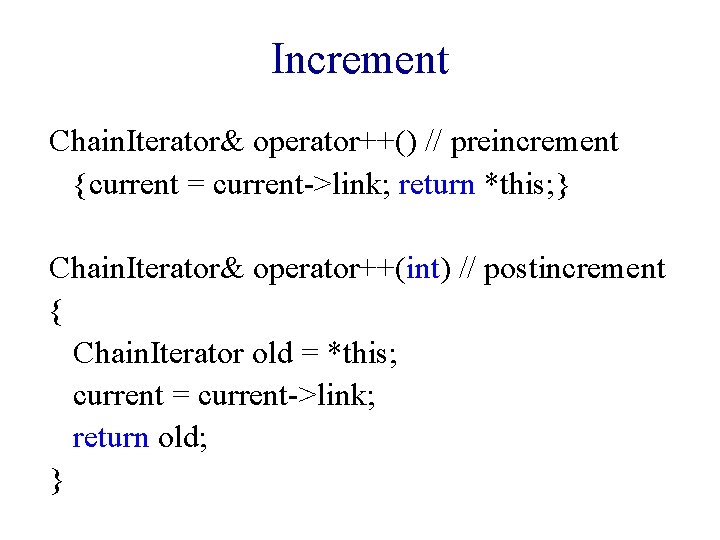
Increment Chain. Iterator& operator++() // preincrement {current = current->link; return *this; } Chain. Iterator& operator++(int) // postincrement { Chain. Iterator old = *this; current = current->link; return old; }
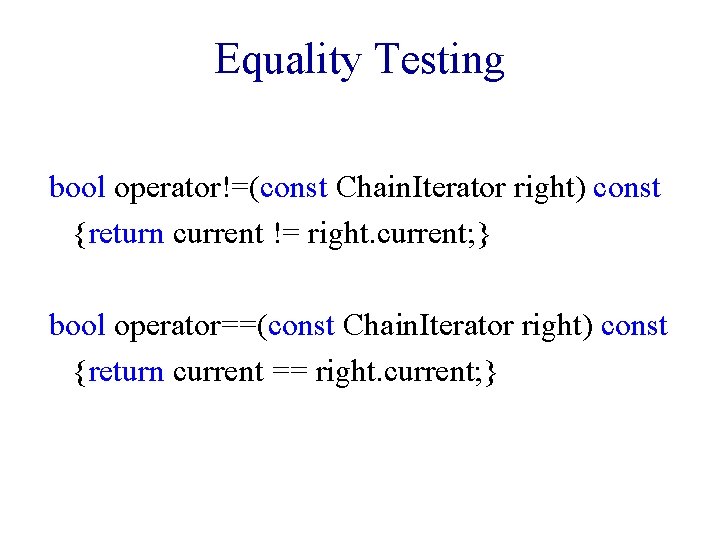
Equality Testing bool operator!=(const Chain. Iterator right) const {return current != right. current; } bool operator==(const Chain. Iterator right) const {return current == right. current; }
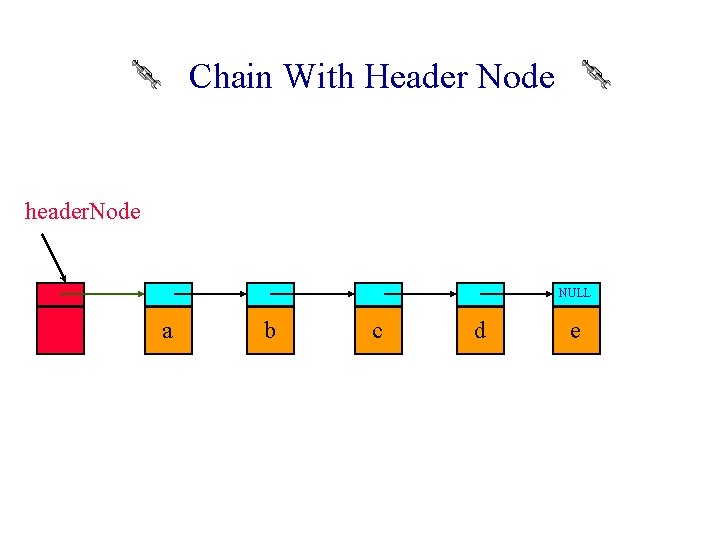
Chain With Header Node header. Node NULL a b c d e
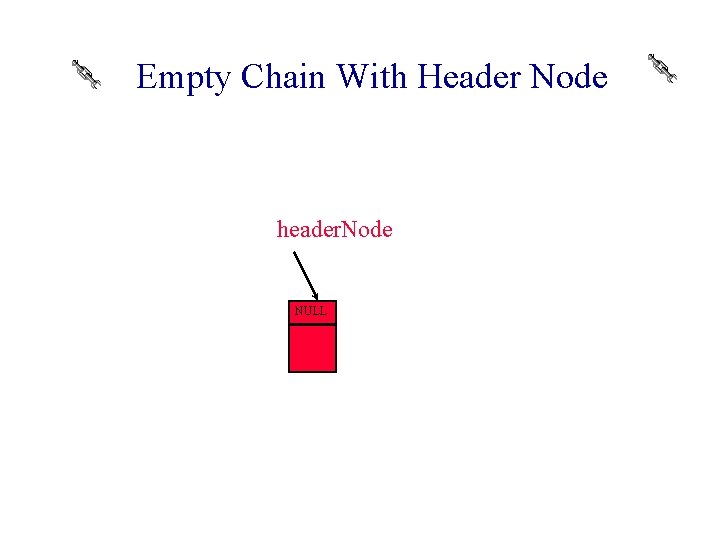
Empty Chain With Header Node header. Node NULL
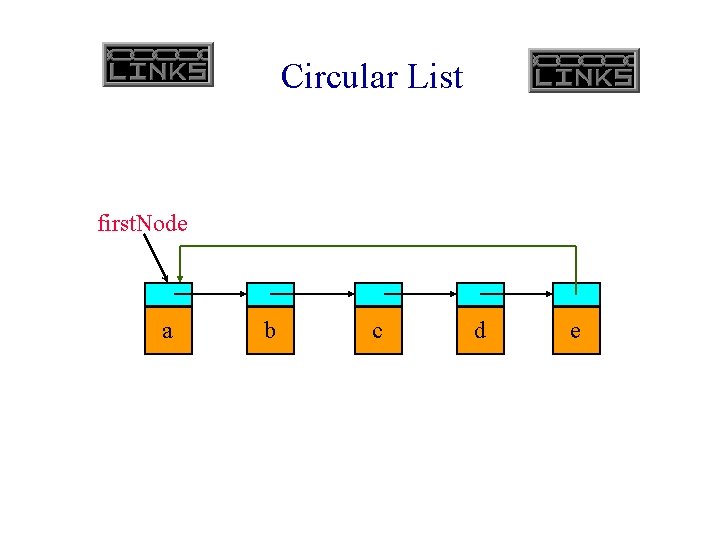
Circular List first. Node a b c d e
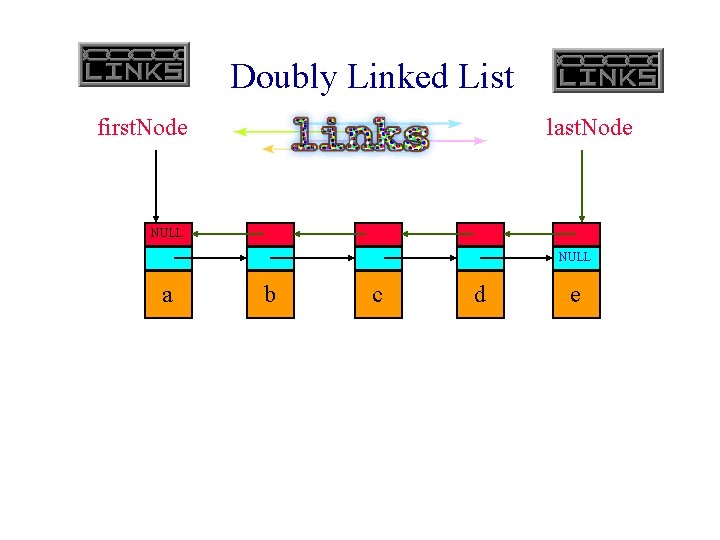
Doubly Linked List first. Node last. Node NULL a b c d e
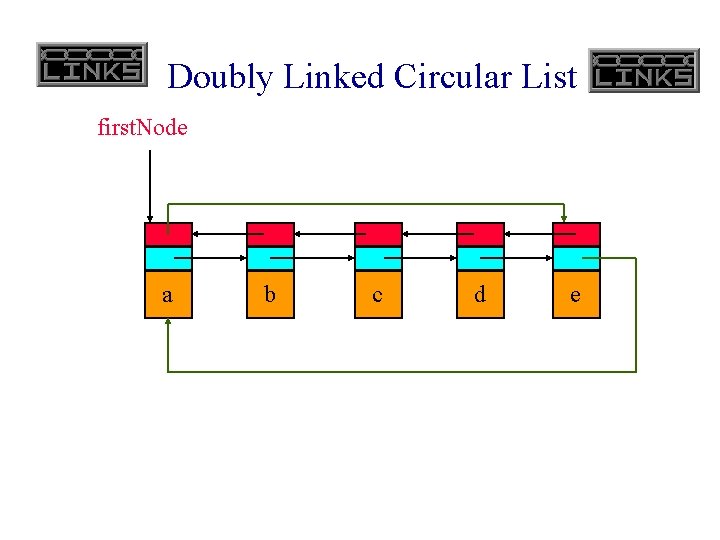
Doubly Linked Circular List first. Node a b c d e
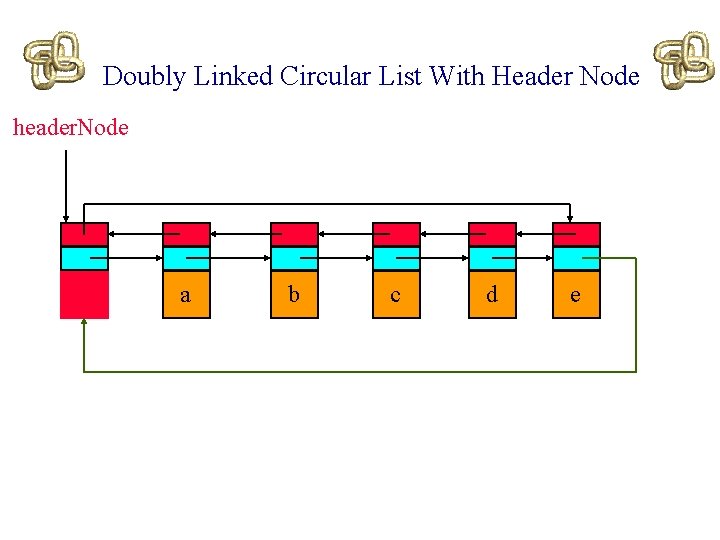
Doubly Linked Circular List With Header Node header. Node a b c d e
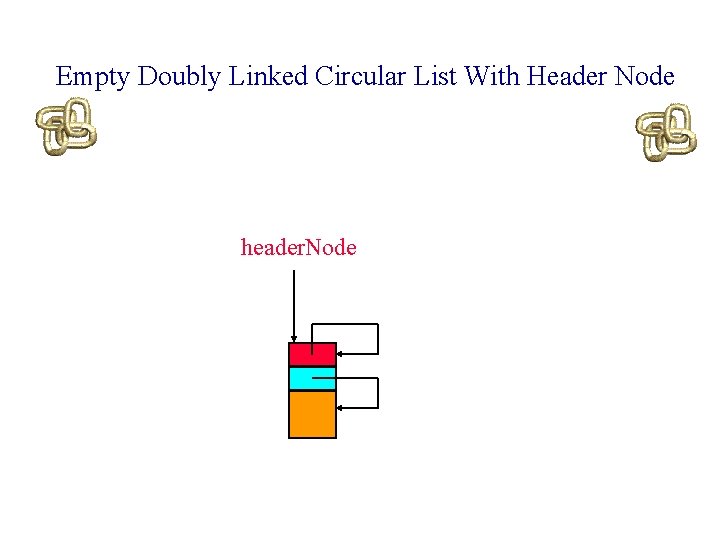
Empty Doubly Linked Circular List With Header Node header. Node
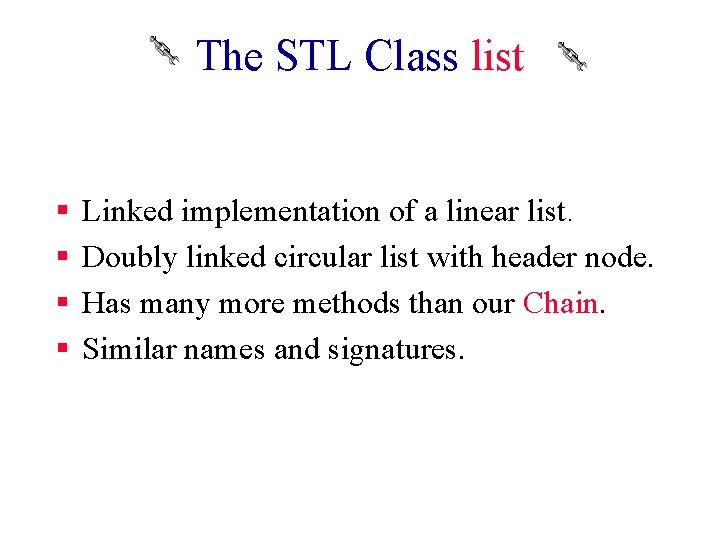
The STL Class list § § Linked implementation of a linear list. Doubly linked circular list with header node. Has many more methods than our Chain. Similar names and signatures.
Maps and sets support bidirectional iterators.
Yield vs return python
Food chain sequence
Iterator inner class java
Iterator pattern python
Inorder iterator
Rapidjson iterator
Iterator entwurfsmuster
Iterator
Volcano iterator model
Zig iterator
Junit before after
Code blocks in python
Jewish variants
Hemoglobin variants
Efficient variants of the icp algorithm
Efficient variants of the icp algorithm
Pram variants
Variants of english language
Copy number variants
F35 variants
St mary's county critical area permits
If time permits quotes