Revised 12600 Sorting Selection Sort Cmput 115 Lecture
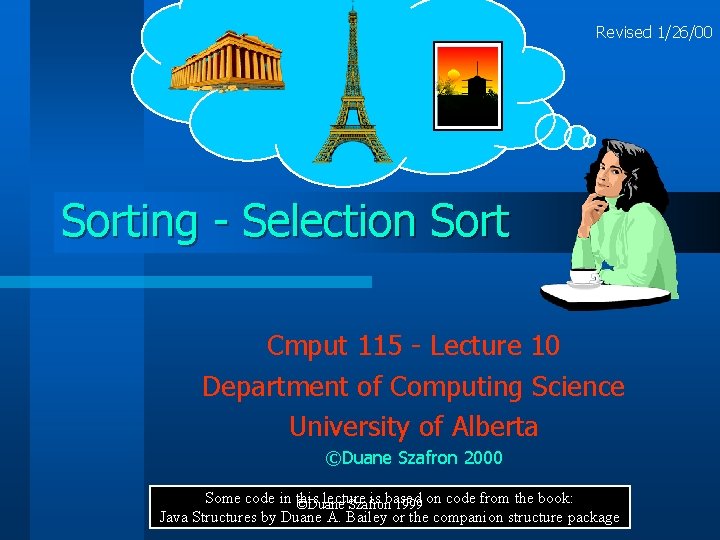
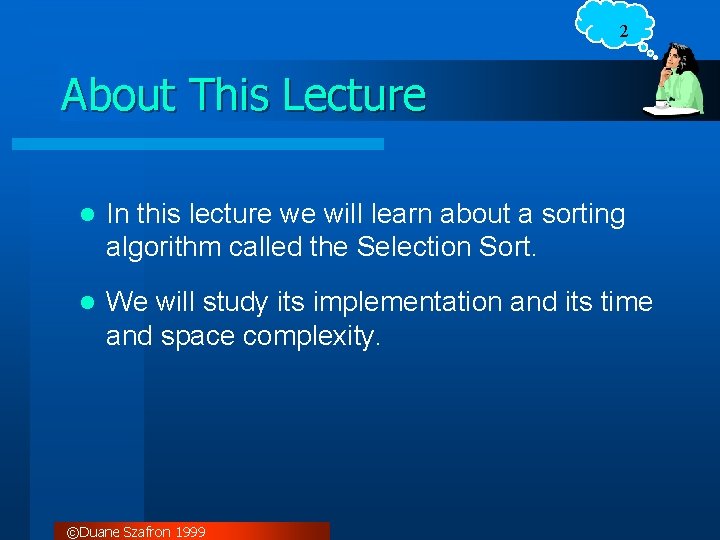
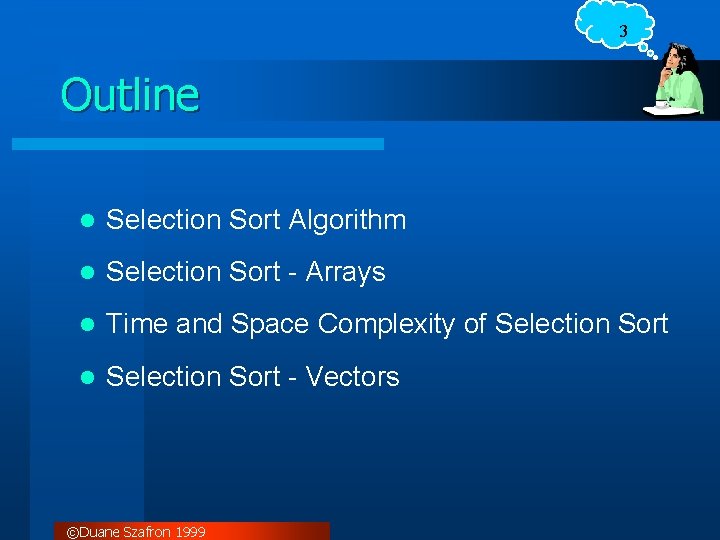
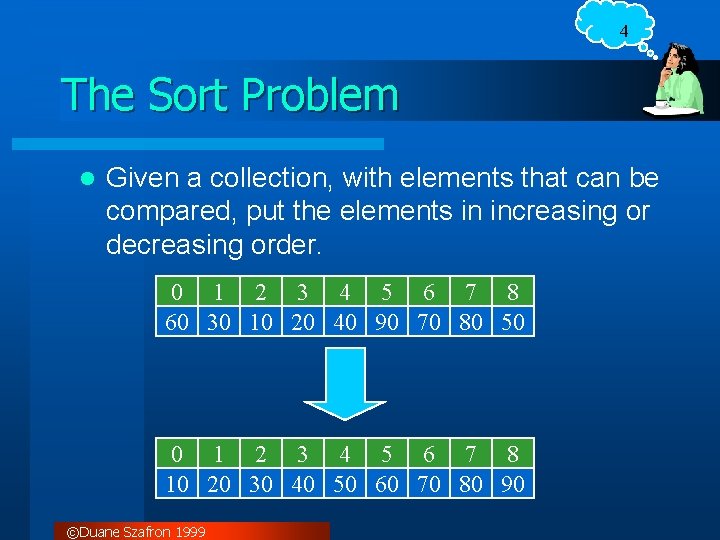
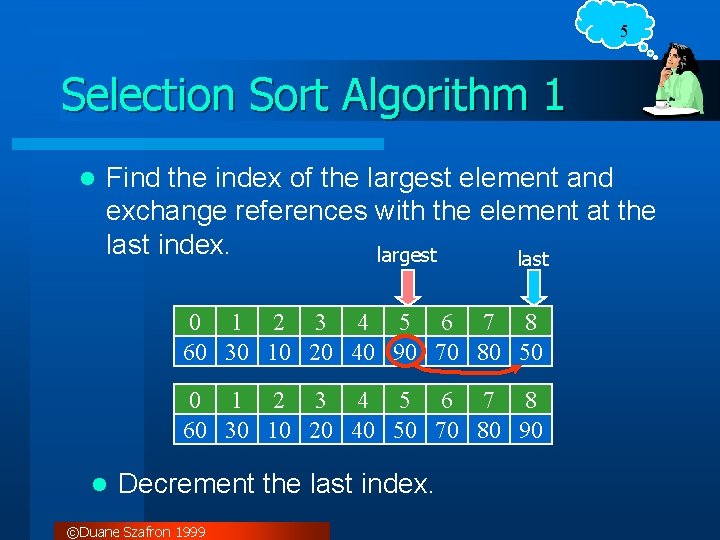
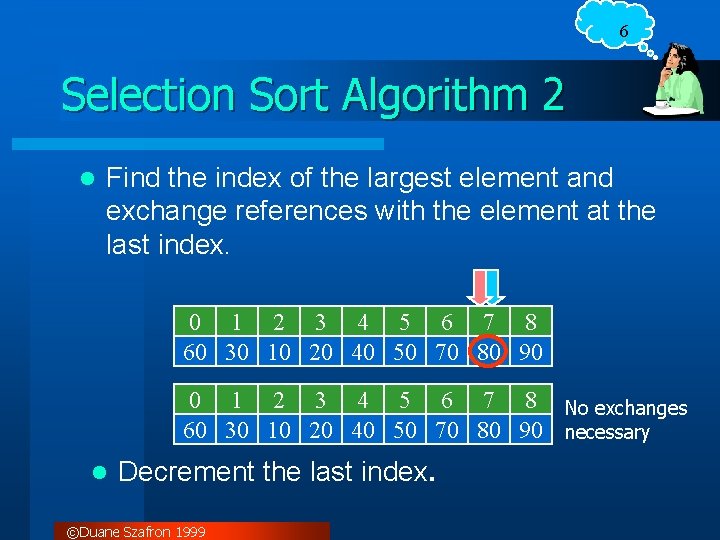
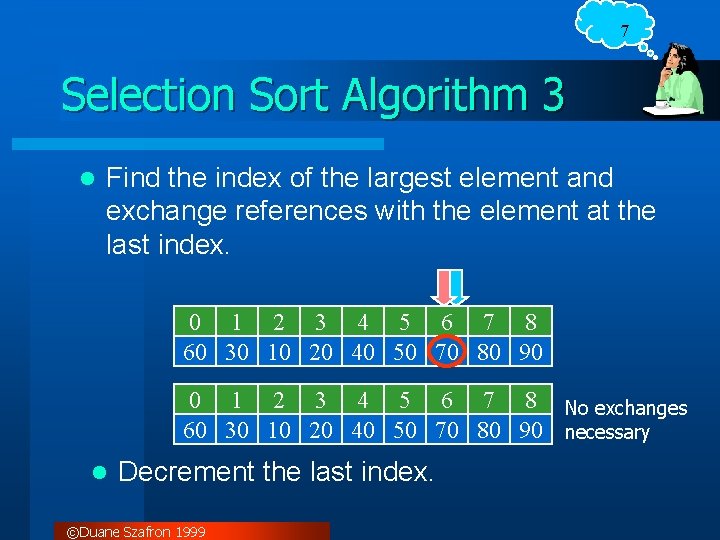
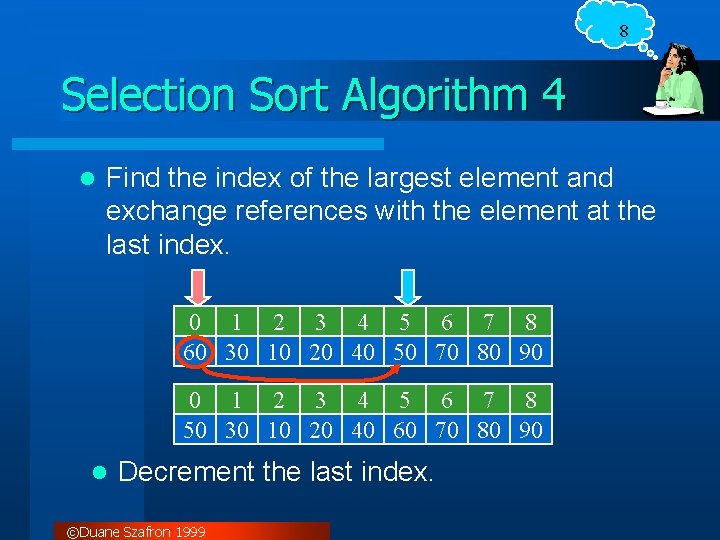
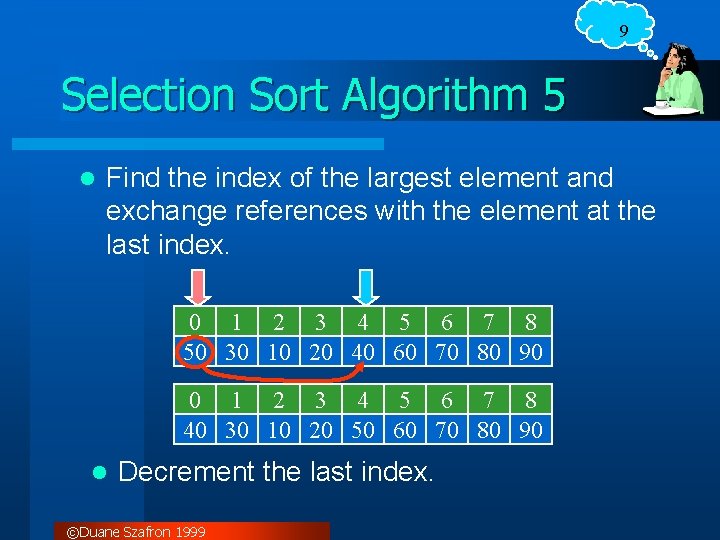
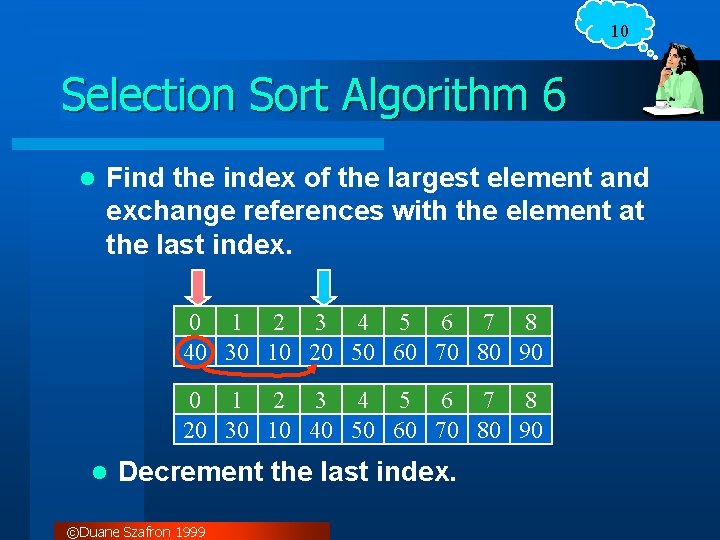
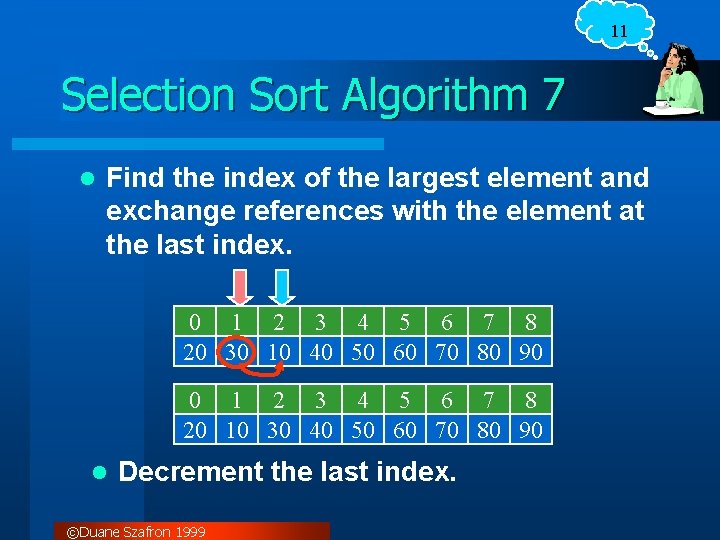
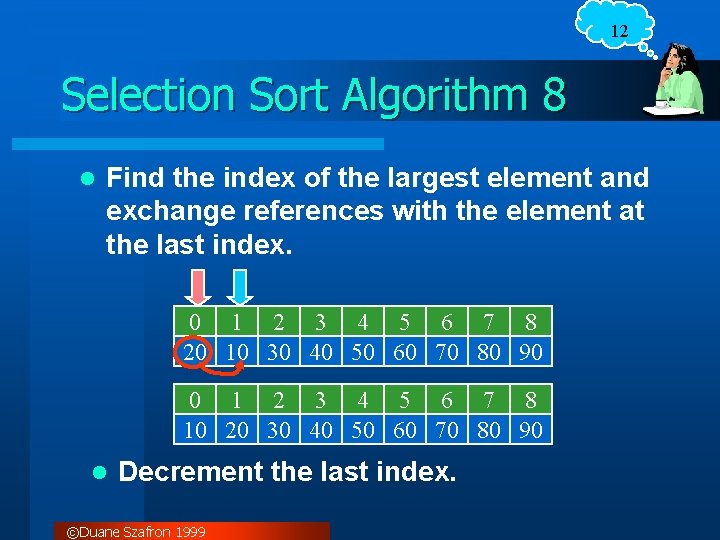
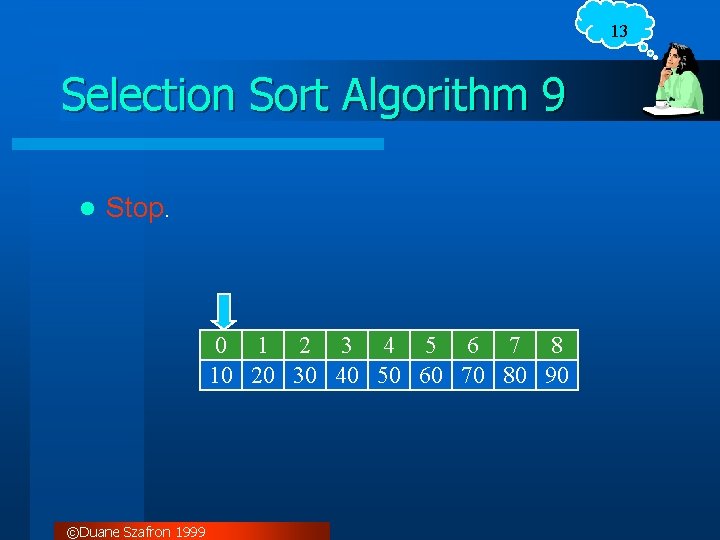
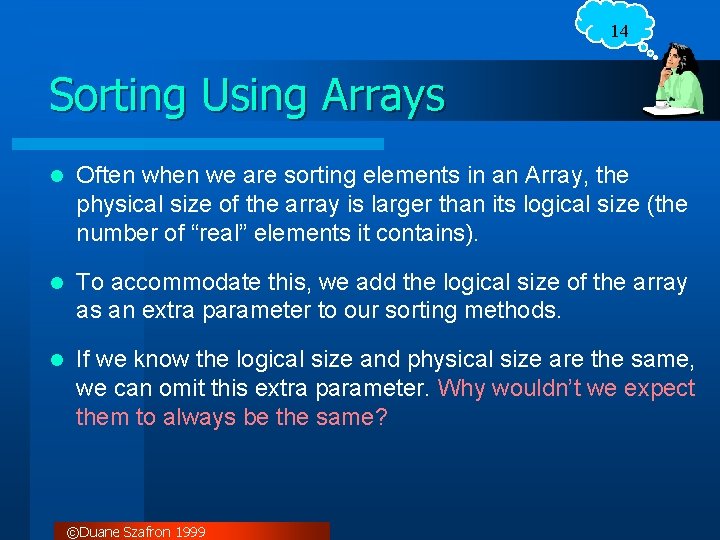
![15 Selection Sort Code - Arrays public static void selection. Sort(Comparable an. Array[ ], 15 Selection Sort Code - Arrays public static void selection. Sort(Comparable an. Array[ ],](https://slidetodoc.com/presentation_image_h2/b28111a4b24aca1d84002c74b4ba1652/image-15.jpg)
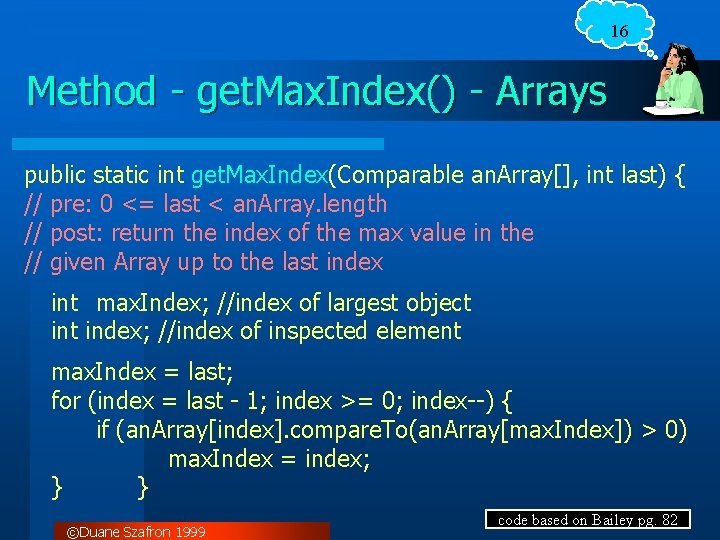
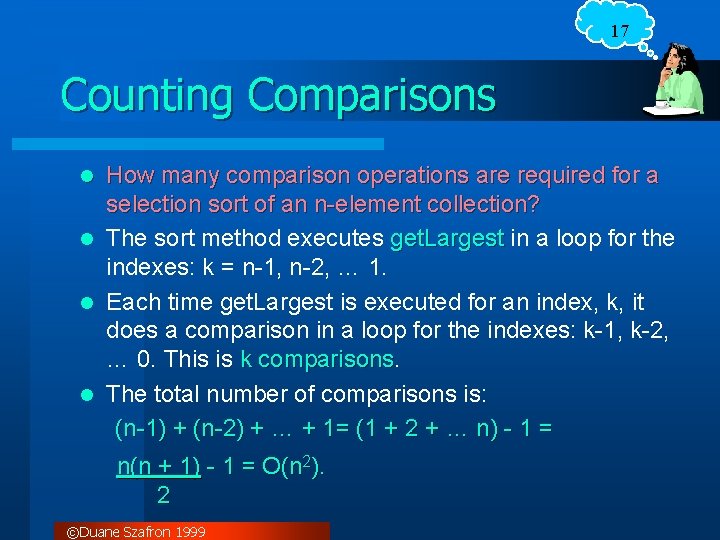
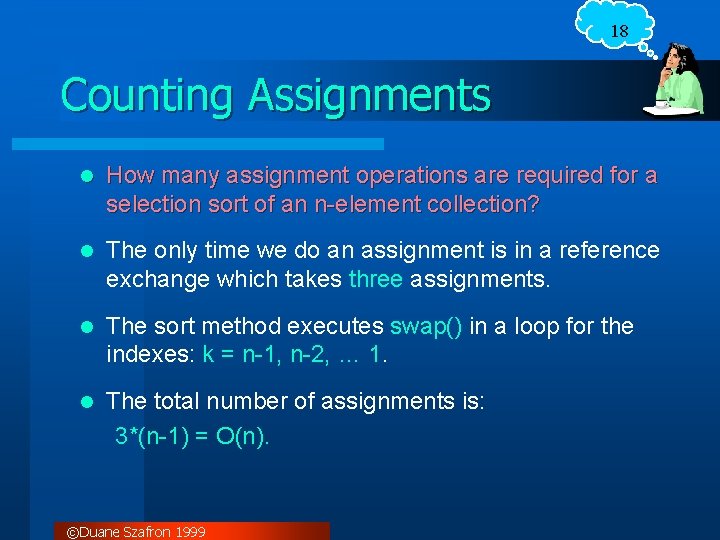
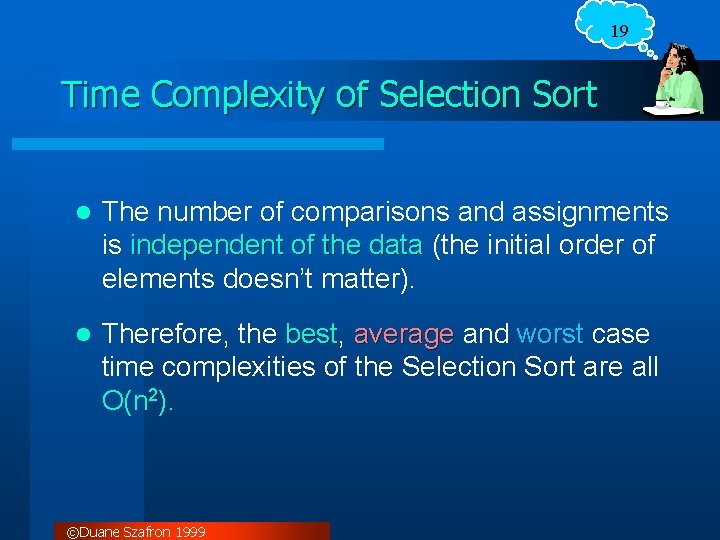
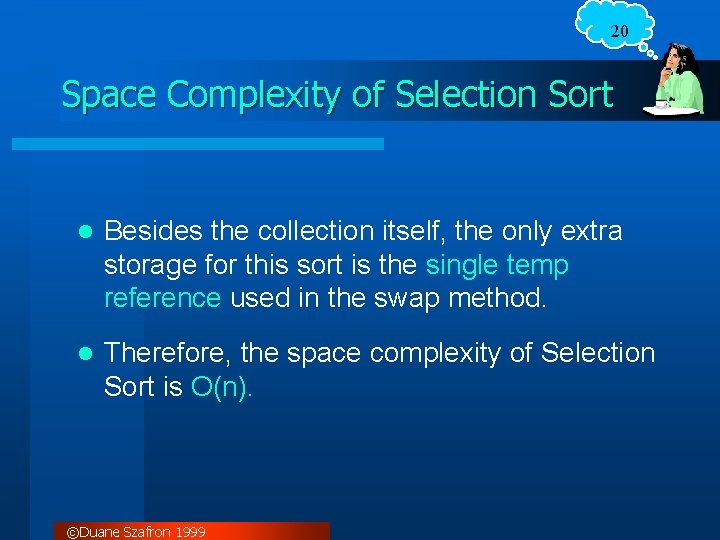
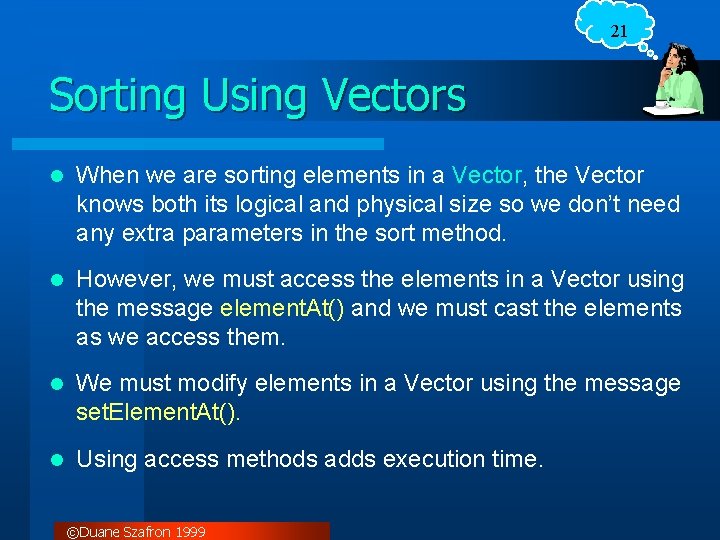
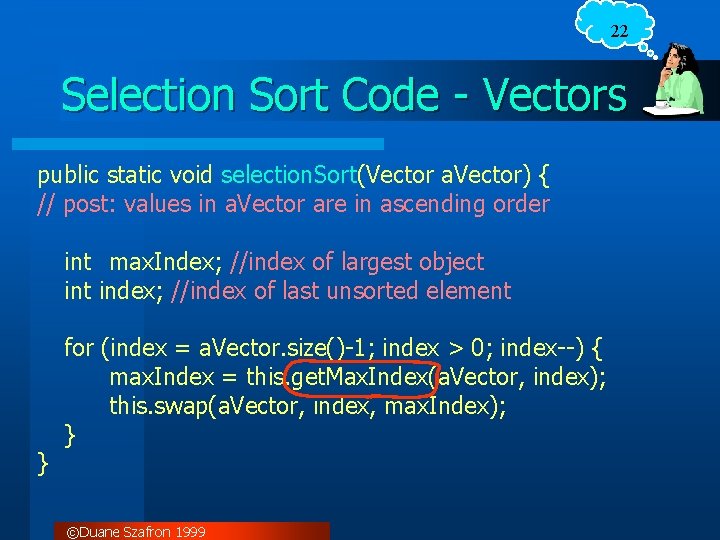
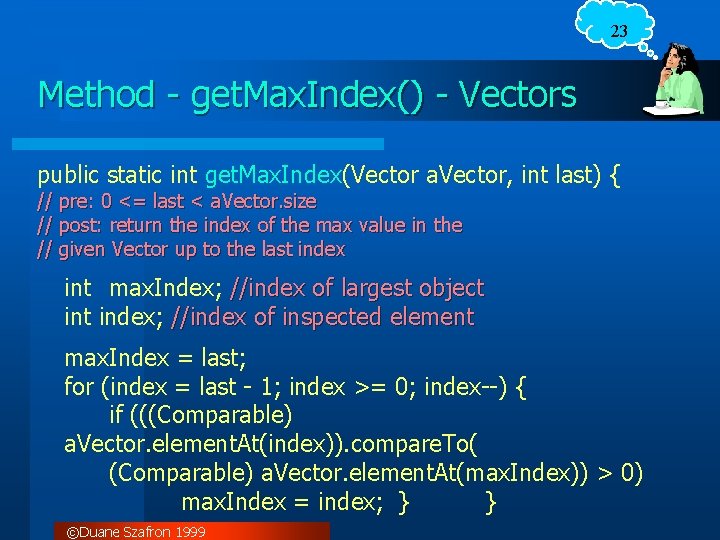
- Slides: 23
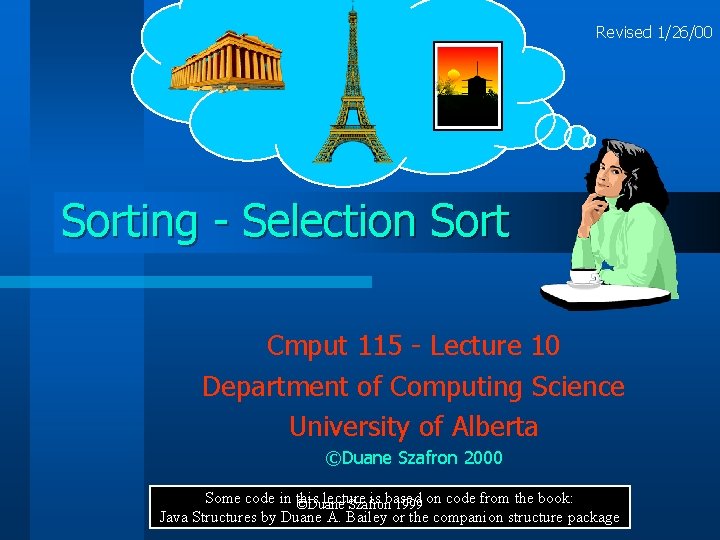
Revised 1/26/00 Sorting - Selection Sort Cmput 115 - Lecture 10 Department of Computing Science University of Alberta ©Duane Szafron 2000 Some code in this lecture is based ©Duane Szafron 1999 on code from the book: Java Structures by Duane A. Bailey or the companion structure package
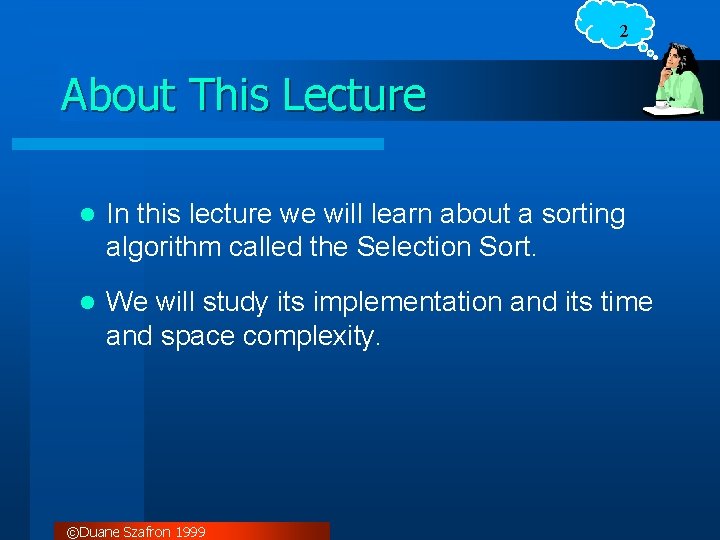
2 About This Lecture l In this lecture we will learn about a sorting algorithm called the Selection Sort. l We will study its implementation and its time and space complexity. ©Duane Szafron 1999
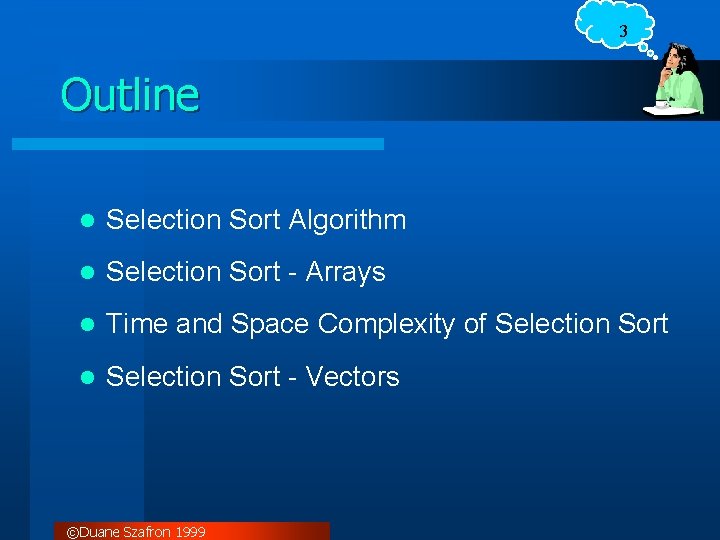
3 Outline l Selection Sort Algorithm l Selection Sort - Arrays l Time and Space Complexity of Selection Sort l Selection Sort - Vectors ©Duane Szafron 1999
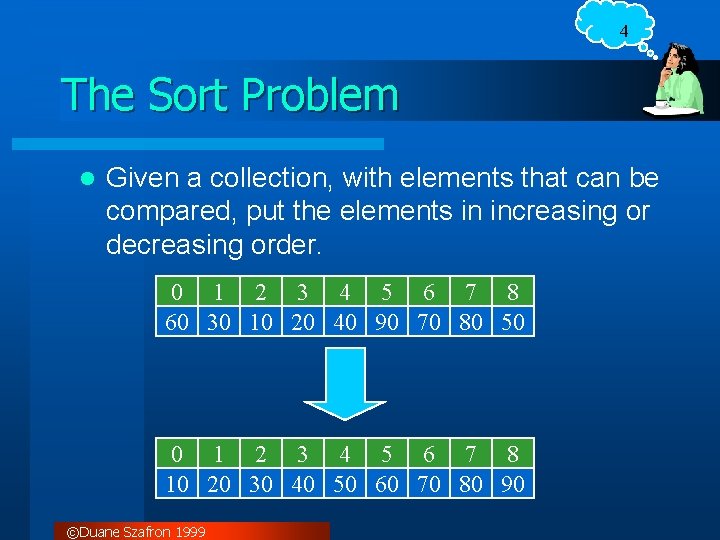
4 The Sort Problem l Given a collection, with elements that can be compared, put the elements in increasing or decreasing order. 0 1 2 3 4 5 6 7 8 60 30 10 20 40 90 70 80 50 0 1 2 3 4 5 6 7 8 10 20 30 40 50 60 70 80 90 ©Duane Szafron 1999
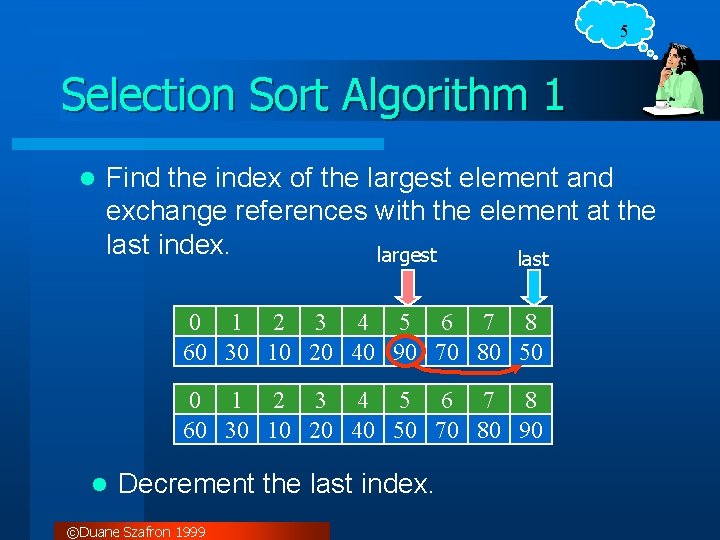
5 Selection Sort Algorithm 1 l Find the index of the largest element and exchange references with the element at the last index. largest last 0 1 2 3 4 5 6 7 8 60 30 10 20 40 90 70 80 50 0 1 2 3 4 5 6 7 8 60 30 10 20 40 50 70 80 90 l Decrement the last index. ©Duane Szafron 1999
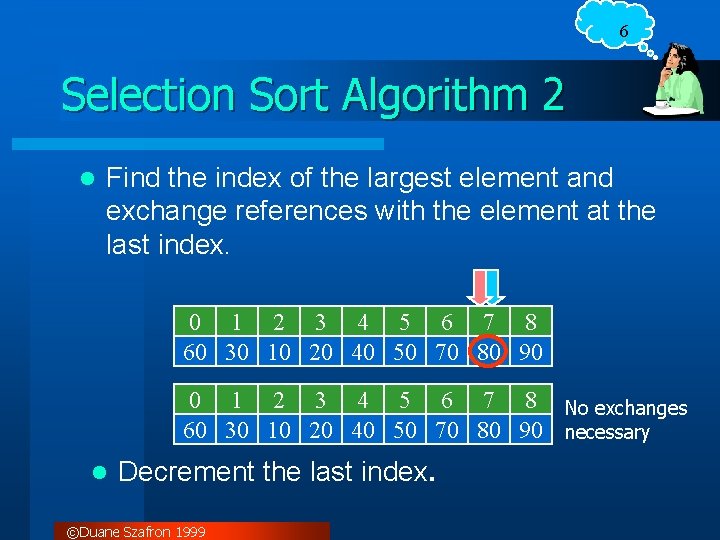
6 Selection Sort Algorithm 2 l Find the index of the largest element and exchange references with the element at the last index. 0 1 2 3 4 5 6 7 8 60 30 10 20 40 50 70 80 90 0 1 2 3 4 5 6 7 8 No exchanges 60 30 10 20 40 50 70 80 90 necessary l Decrement the last index. ©Duane Szafron 1999
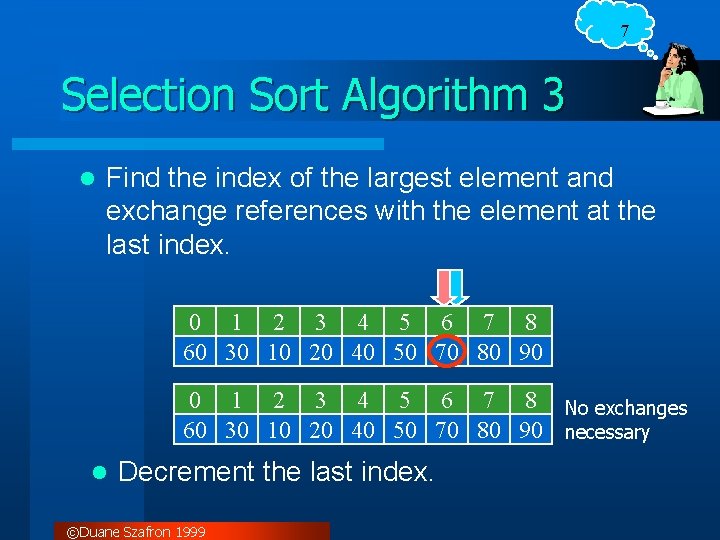
7 Selection Sort Algorithm 3 l Find the index of the largest element and exchange references with the element at the last index. 0 1 2 3 4 5 6 7 8 60 30 10 20 40 50 70 80 90 0 1 2 3 4 5 6 7 8 No exchanges 60 30 10 20 40 50 70 80 90 necessary l Decrement the last index. ©Duane Szafron 1999
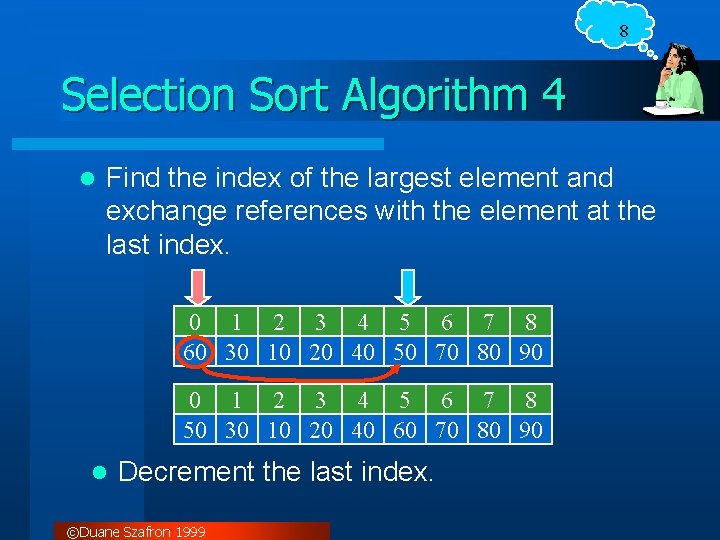
8 Selection Sort Algorithm 4 l Find the index of the largest element and exchange references with the element at the last index. 0 1 2 3 4 5 6 7 8 60 30 10 20 40 50 70 80 90 0 1 2 3 4 5 6 7 8 50 30 10 20 40 60 70 80 90 l Decrement the last index. ©Duane Szafron 1999
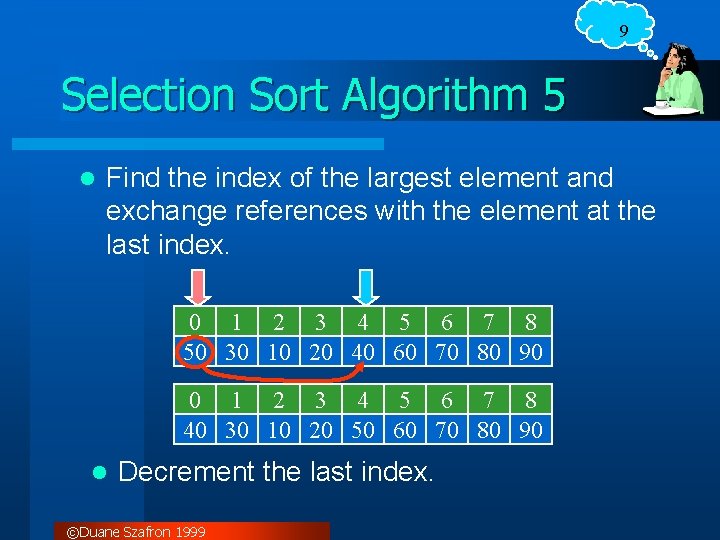
9 Selection Sort Algorithm 5 l Find the index of the largest element and exchange references with the element at the last index. 0 1 2 3 4 5 6 7 8 50 30 10 20 40 60 70 80 90 0 1 2 3 4 5 6 7 8 40 30 10 20 50 60 70 80 90 l Decrement the last index. ©Duane Szafron 1999
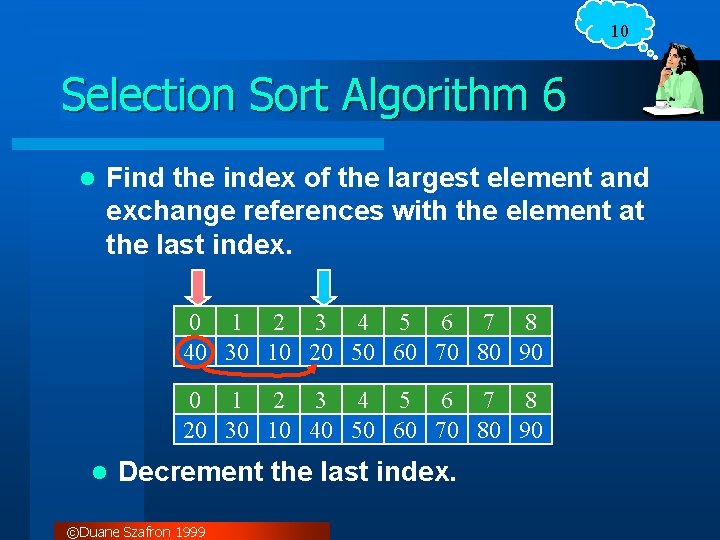
10 Selection Sort Algorithm 6 l Find the index of the largest element and exchange references with the element at the last index. 0 1 2 3 4 5 6 7 8 40 30 10 20 50 60 70 80 90 0 1 2 3 4 5 6 7 8 20 30 10 40 50 60 70 80 90 l Decrement the last index. ©Duane Szafron 1999
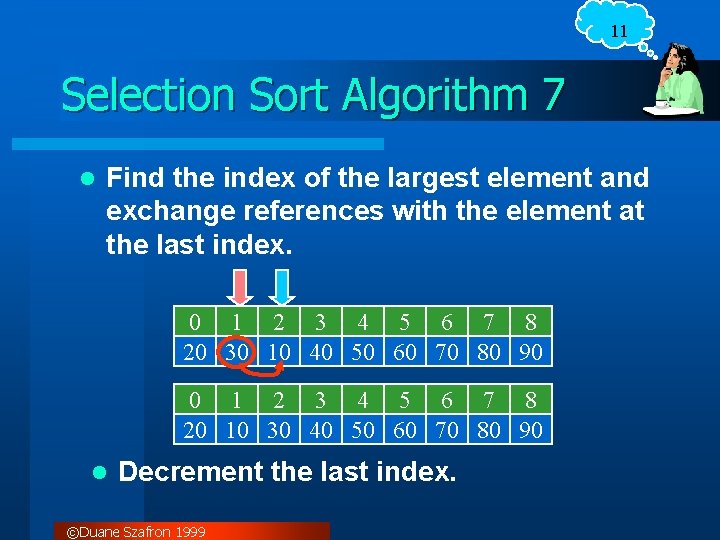
11 Selection Sort Algorithm 7 l Find the index of the largest element and exchange references with the element at the last index. 0 1 2 3 4 5 6 7 8 20 30 10 40 50 60 70 80 90 0 1 2 3 4 5 6 7 8 20 10 30 40 50 60 70 80 90 l Decrement the last index. ©Duane Szafron 1999
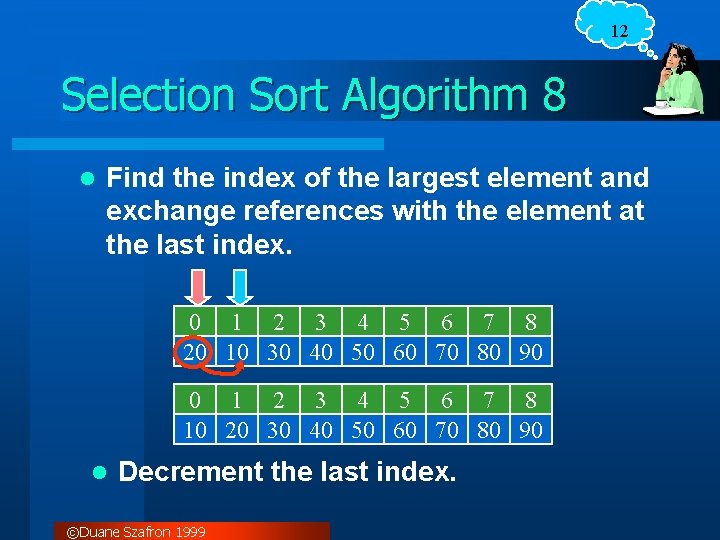
12 Selection Sort Algorithm 8 l Find the index of the largest element and exchange references with the element at the last index. 0 1 2 3 4 5 6 7 8 20 10 30 40 50 60 70 80 90 0 1 2 3 4 5 6 7 8 10 20 30 40 50 60 70 80 90 l Decrement the last index. ©Duane Szafron 1999
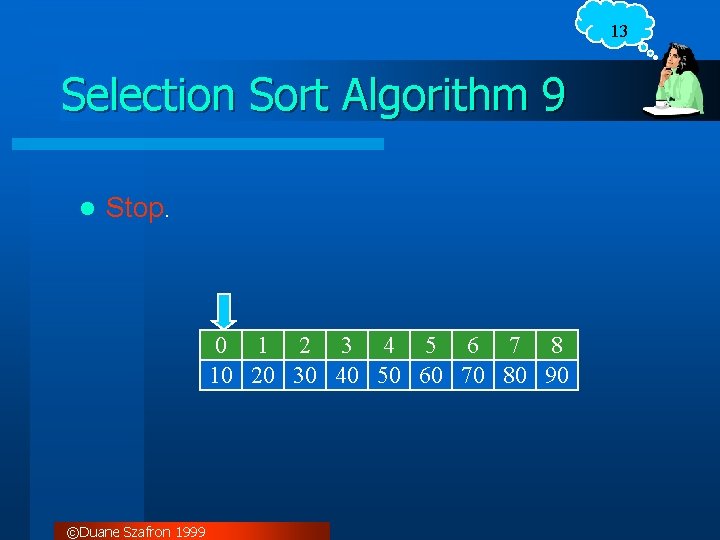
13 Selection Sort Algorithm 9 l Stop. 0 1 2 3 4 5 6 7 8 10 20 30 40 50 60 70 80 90 ©Duane Szafron 1999
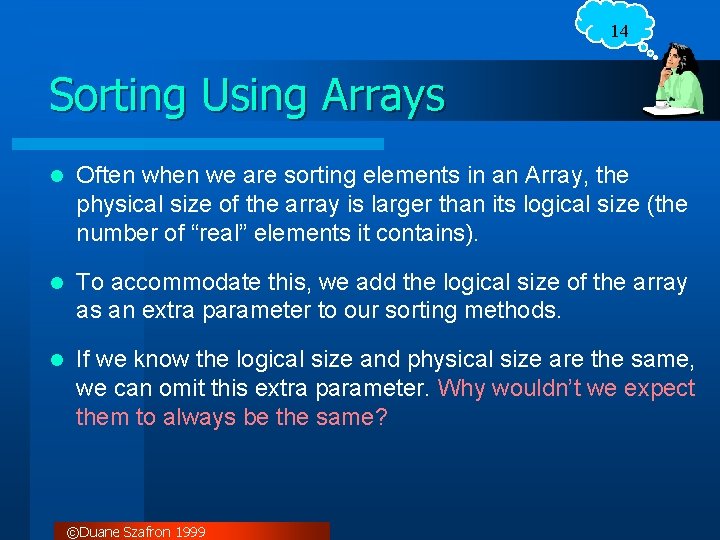
14 Sorting Using Arrays l Often when we are sorting elements in an Array, the physical size of the array is larger than its logical size (the number of “real” elements it contains). l To accommodate this, we add the logical size of the array as an extra parameter to our sorting methods. l If we know the logical size and physical size are the same, we can omit this extra parameter. Why wouldn’t we expect them to always be the same? ©Duane Szafron 1999
![15 Selection Sort Code Arrays public static void selection SortComparable an Array 15 Selection Sort Code - Arrays public static void selection. Sort(Comparable an. Array[ ],](https://slidetodoc.com/presentation_image_h2/b28111a4b24aca1d84002c74b4ba1652/image-15.jpg)
15 Selection Sort Code - Arrays public static void selection. Sort(Comparable an. Array[ ], int size) { // pre: 0 <= size <= an. Array. length // post: values in an. Array are in ascending order int max. Index; //index of largest object index; //index of last unsorted element } for (index = size - 1; index > 0; index--) { max. Index = this. get. Max. Index(an. Array, index); this. swap(an. Array, index, max. Index); } ©Duane Szafron 1999 code based on Bailey pg. 82
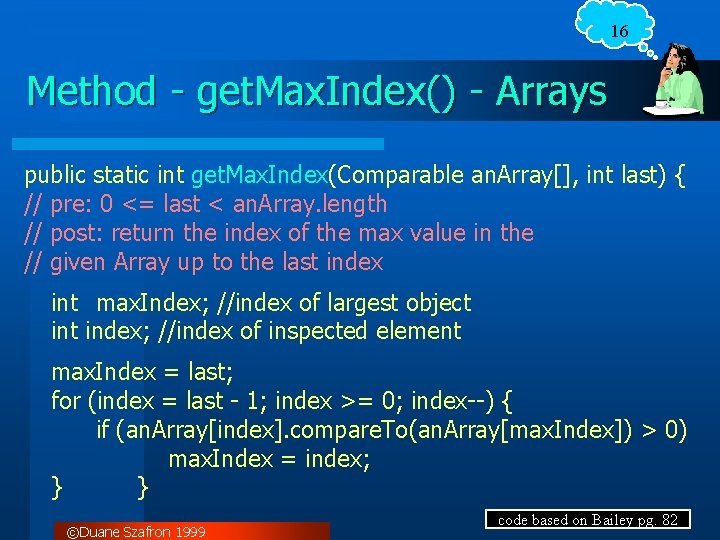
16 Method - get. Max. Index() - Arrays public static int get. Max. Index(Comparable an. Array[], int last) { // pre: 0 <= last < an. Array. length // post: return the index of the max value in the // given Array up to the last index int max. Index; //index of largest object index; //index of inspected element max. Index = last; for (index = last - 1; index >= 0; index--) { if (an. Array[index]. compare. To(an. Array[max. Index]) > 0) max. Index = index; } } ©Duane Szafron 1999 code based on Bailey pg. 82
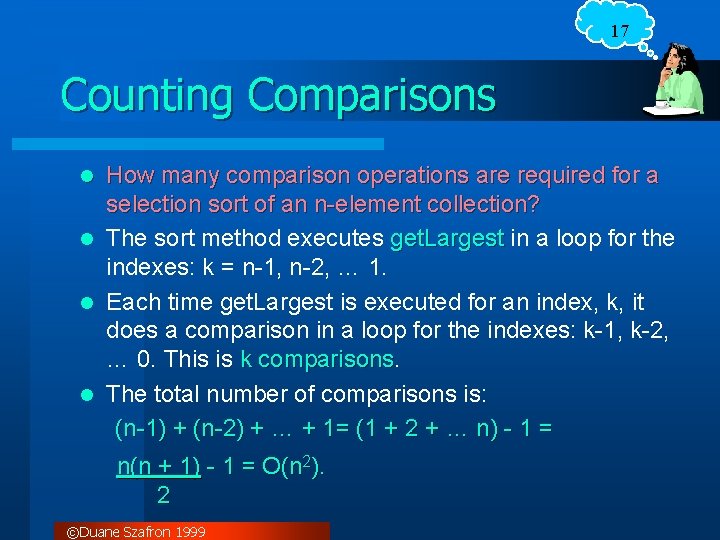
17 Counting Comparisons How many comparison operations are required for a selection sort of an n-element collection? l The sort method executes get. Largest in a loop for the indexes: k = n-1, n-2, … 1. l Each time get. Largest is executed for an index, k, it does a comparison in a loop for the indexes: k-1, k-2, … 0. This is k comparisons l The total number of comparisons is: (n-1) + (n-2) + … + 1= (1 + 2 + … n) - 1 = l n(n + 1) - 1 = O(n 2). 2 ©Duane Szafron 1999
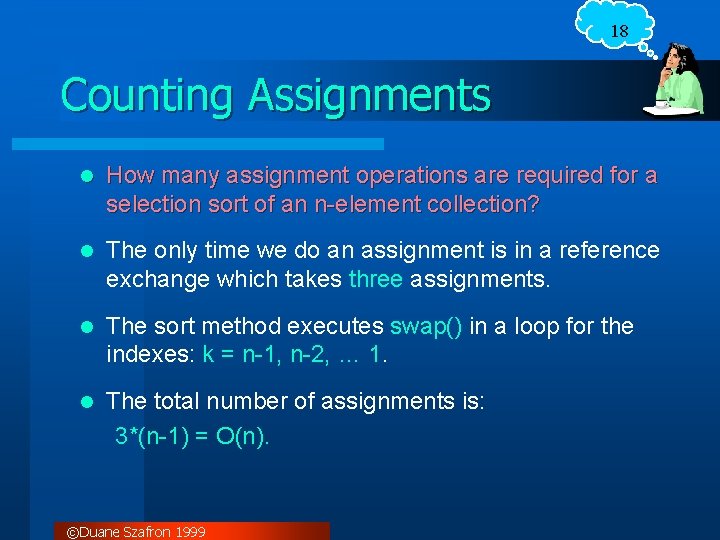
18 Counting Assignments l How many assignment operations are required for a selection sort of an n-element collection? l The only time we do an assignment is in a reference exchange which takes three assignments. l The sort method executes swap() in a loop for the indexes: k = n-1, n-2, … 1. l The total number of assignments is: 3*(n-1) = O(n). ©Duane Szafron 1999
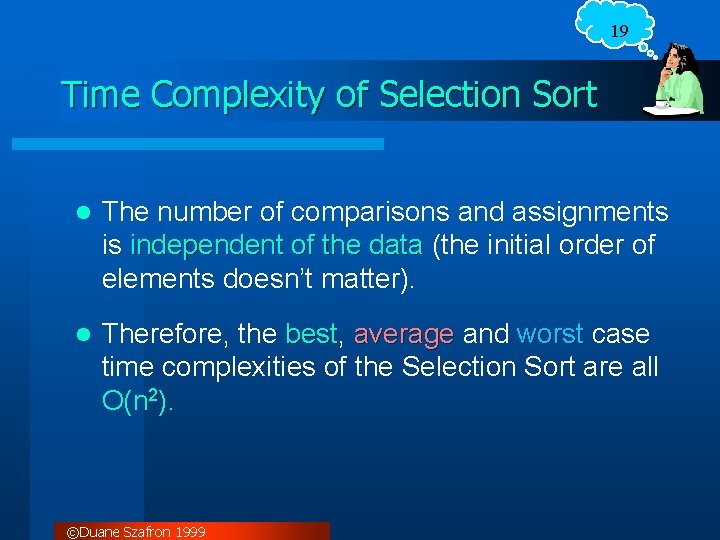
19 Time Complexity of Selection Sort l The number of comparisons and assignments is independent of the data (the initial order of elements doesn’t matter). l Therefore, the best, best average and worst case time complexities of the Selection Sort are all O(n 2). ©Duane Szafron 1999
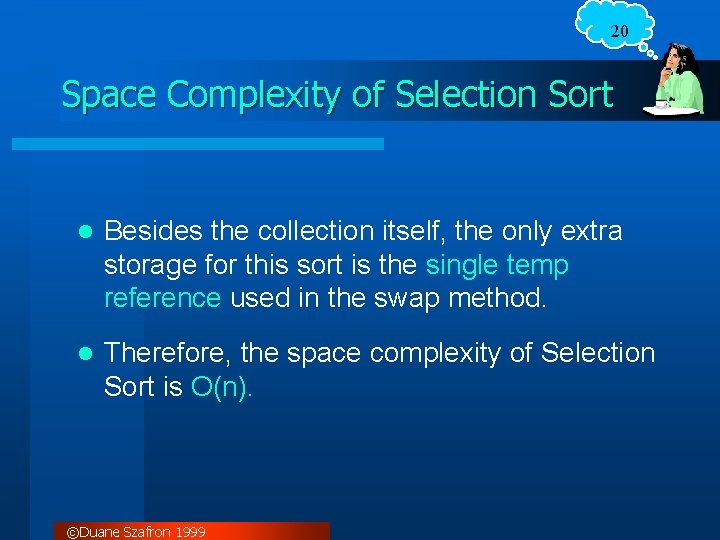
20 Space Complexity of Selection Sort l Besides the collection itself, the only extra storage for this sort is the single temp reference used in the swap method. l Therefore, the space complexity of Selection Sort is O(n). ©Duane Szafron 1999
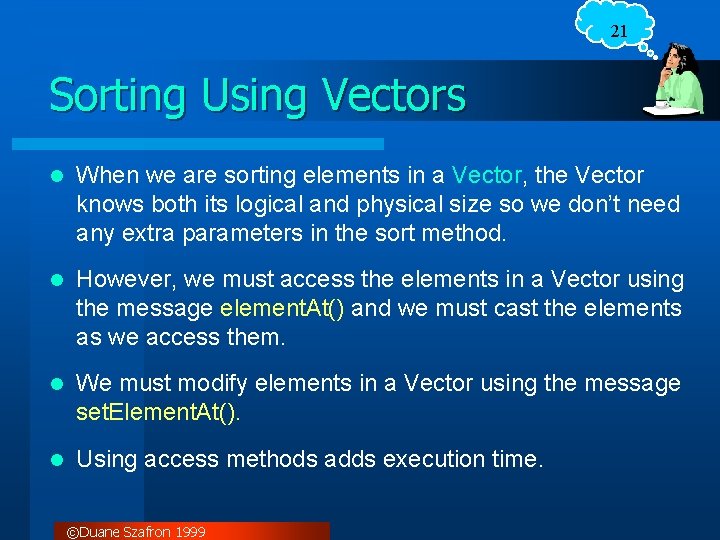
21 Sorting Using Vectors l When we are sorting elements in a Vector, the Vector knows both its logical and physical size so we don’t need any extra parameters in the sort method. l However, we must access the elements in a Vector using the message element. At() and we must cast the elements as we access them. l We must modify elements in a Vector using the message set. Element. At(). l Using access methods adds execution time. ©Duane Szafron 1999
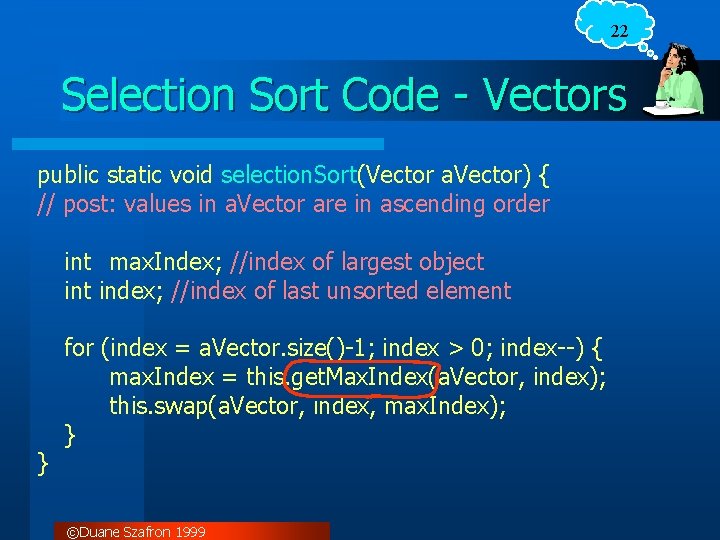
22 Selection Sort Code - Vectors public static void selection. Sort(Vector a. Vector) { // post: values in a. Vector are in ascending order int max. Index; //index of largest object index; //index of last unsorted element } for (index = a. Vector. size()-1; index > 0; index--) { max. Index = this. get. Max. Index(a. Vector, index); this. swap(a. Vector, index, max. Index); } ©Duane Szafron 1999
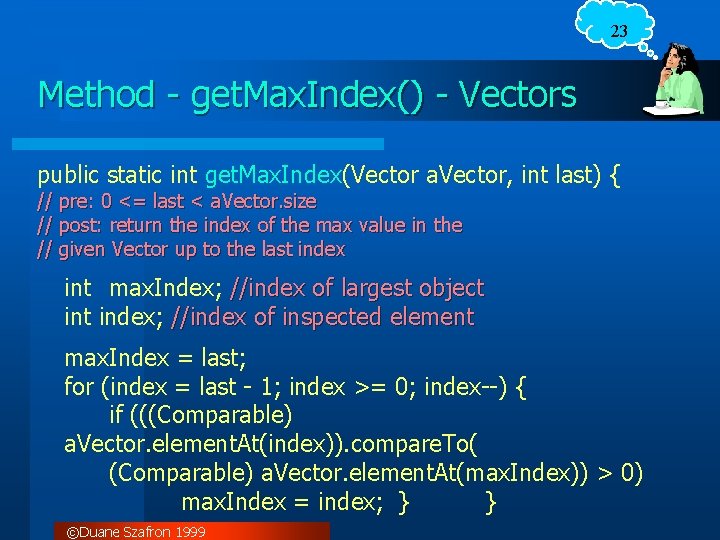
23 Method - get. Max. Index() - Vectors public static int get. Max. Index(Vector a. Vector, int last) { // pre: 0 <= last < a. Vector. size // post: return the index of the max value in the // given Vector up to the last index int max. Index; //index of largest object index; //index of inspected element max. Index = last; for (index = last - 1; index >= 0; index--) { if (((Comparable) a. Vector. element. At(index)). compare. To( (Comparable) a. Vector. element. At(max. Index)) > 0) max. Index = index; } } ©Duane Szafron 1999
Bubble sort vs selection sort time complexity
Bubble sort vs selection sort
Bubble sort and selection sort
Difference between selection sort and bubble sort
Heap sort vs selection sort
Selection sort vs bubble sort
Differentiate between bubble and quick sorting
Internal and external sort
Checkpoint 4800
Sort meaning
Quick sort merge sort
Quick sort merge sort
Radix sort animation
Bucket sort vs radix sort
01:640:244 lecture notes - lecture 15: plat, idah, farad
Selectionsort
Recurrence relation of recursive selection sort
Selection sort number of comparisons
Replacement selection sort
Insertion sort recurrence relation
Mips selection sort
Albero di decisione bubblesort
Difference between selection and insertion sort
Selection sort worst case