Revised 22300 Container Traversal Cmput 115 Lecture 20
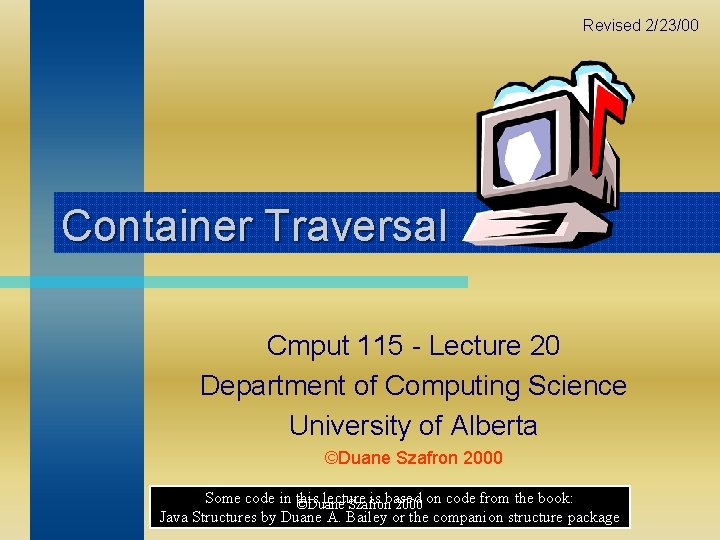
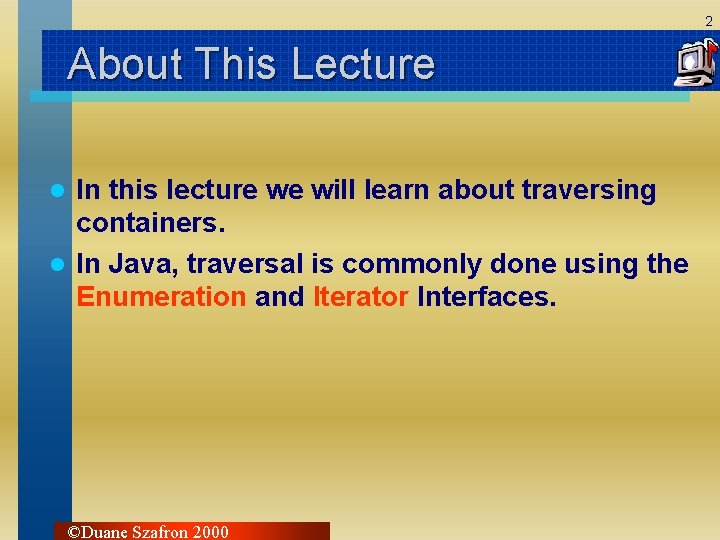
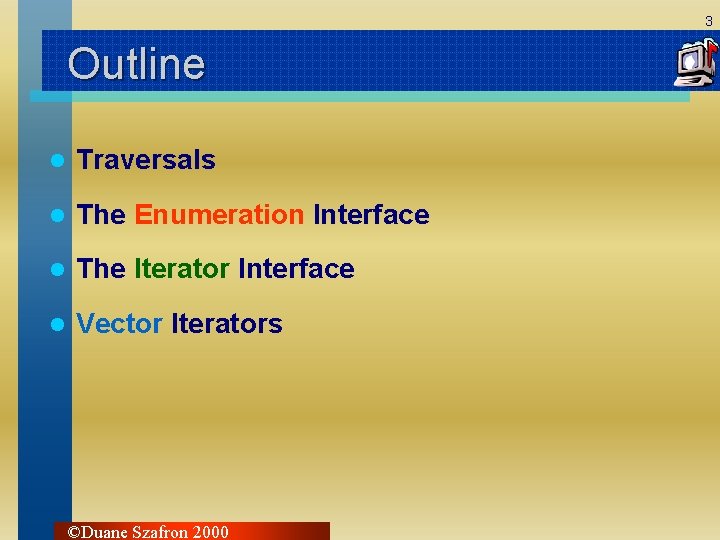
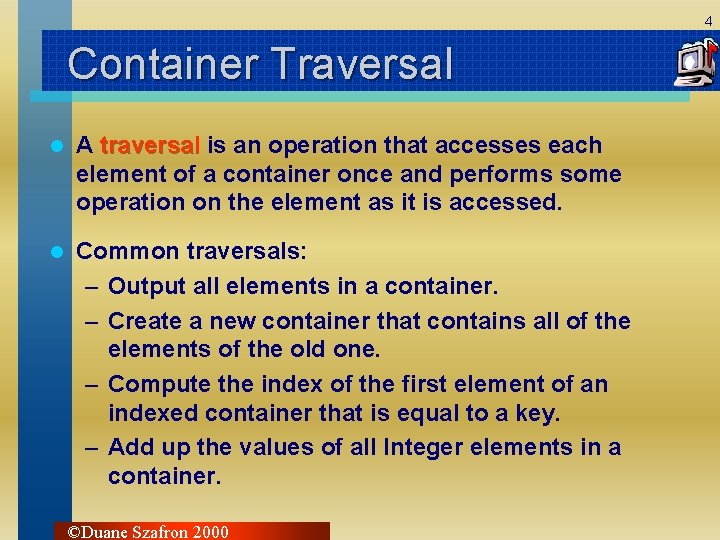
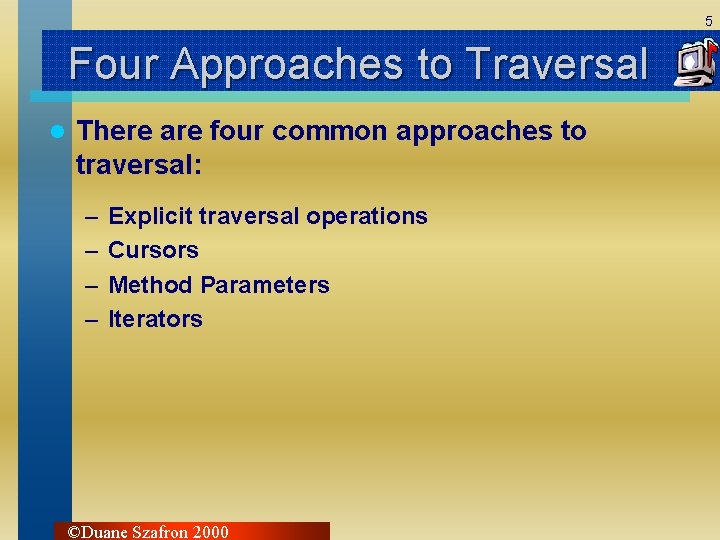
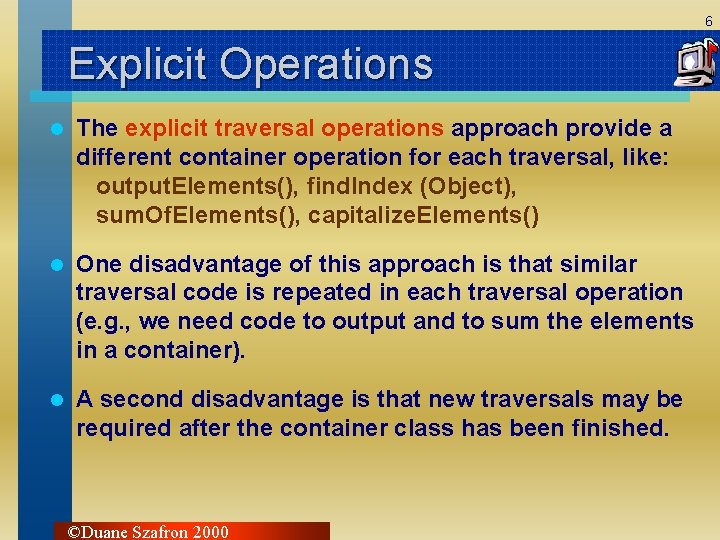
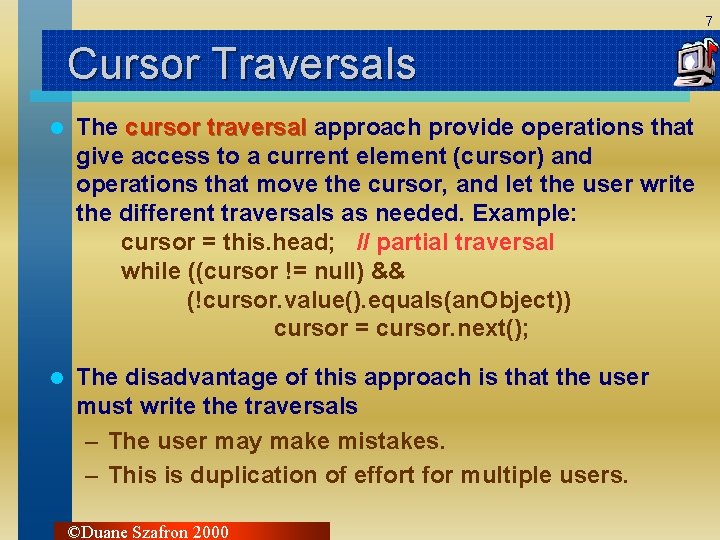
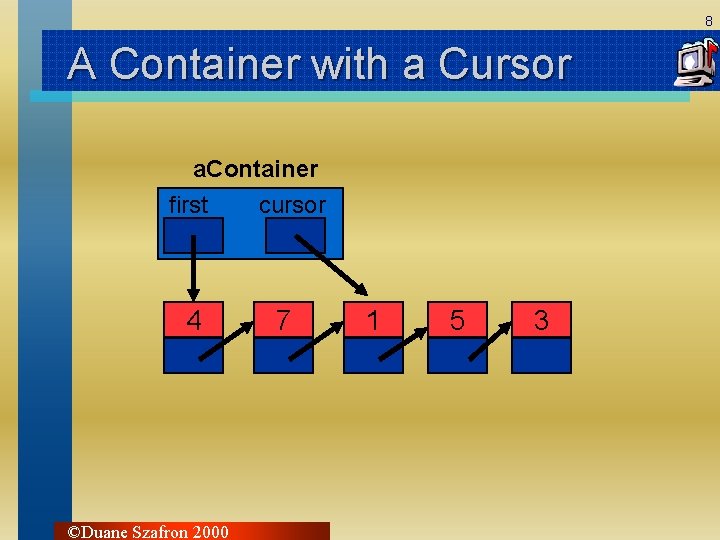
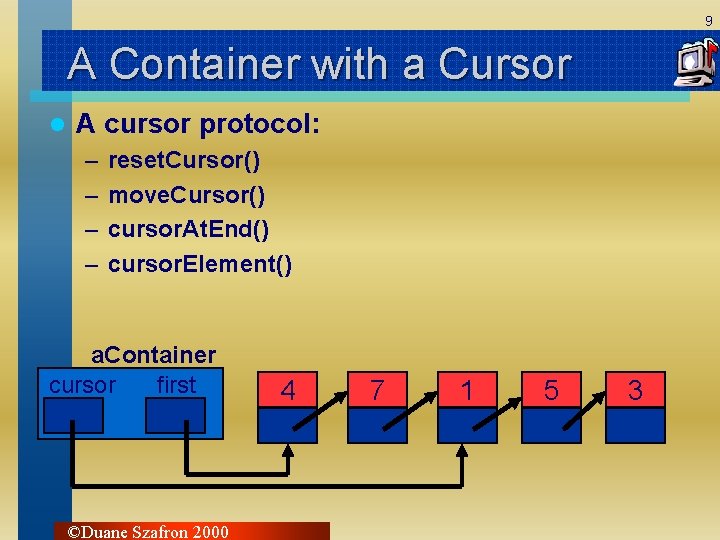
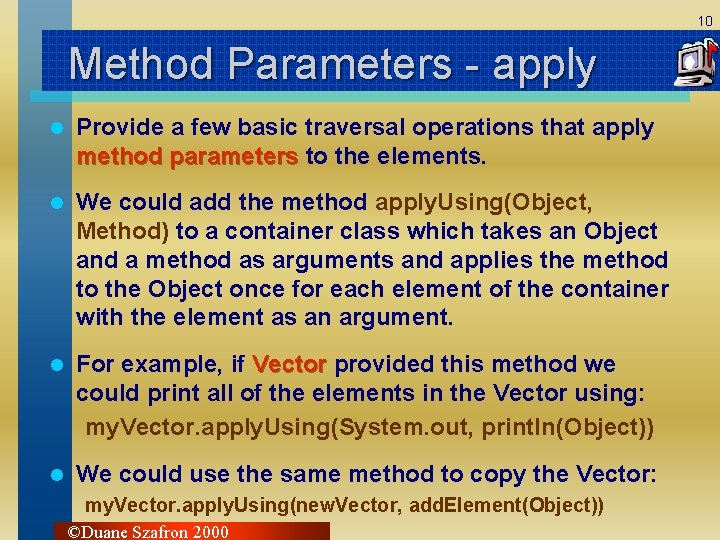
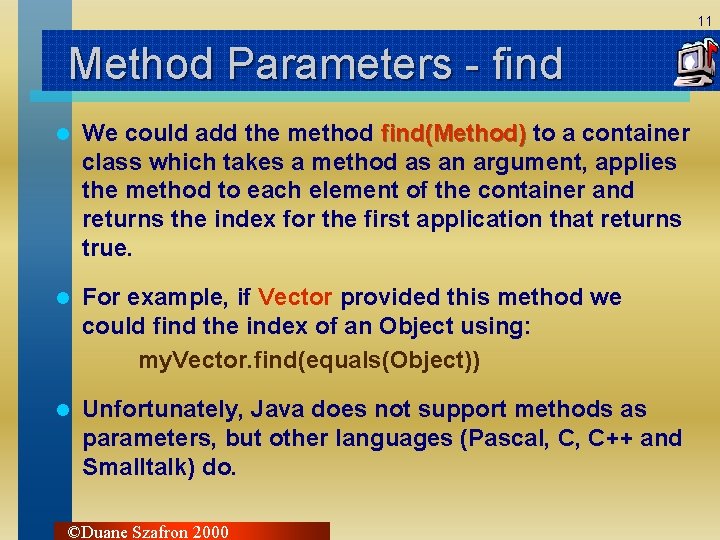
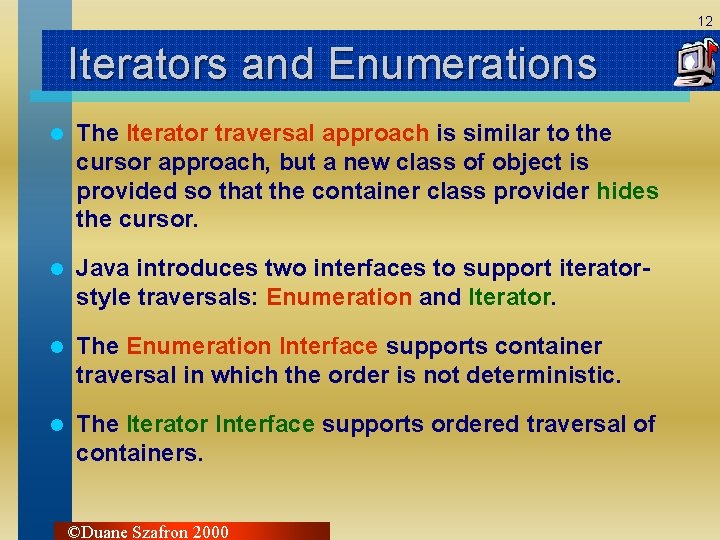
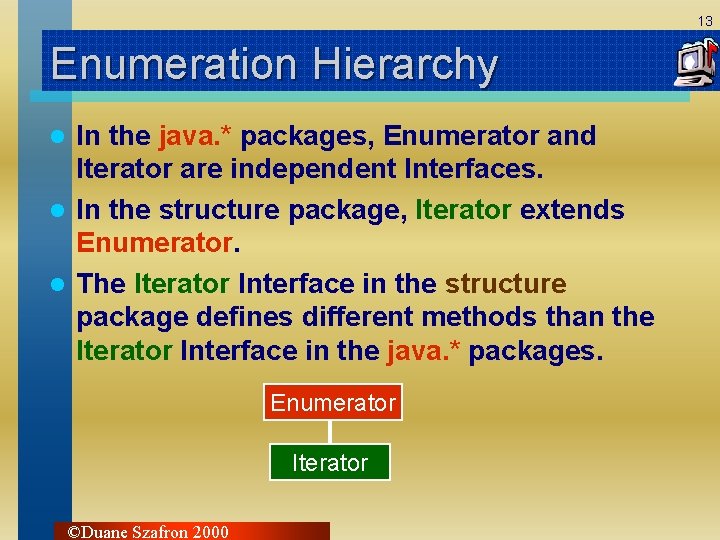
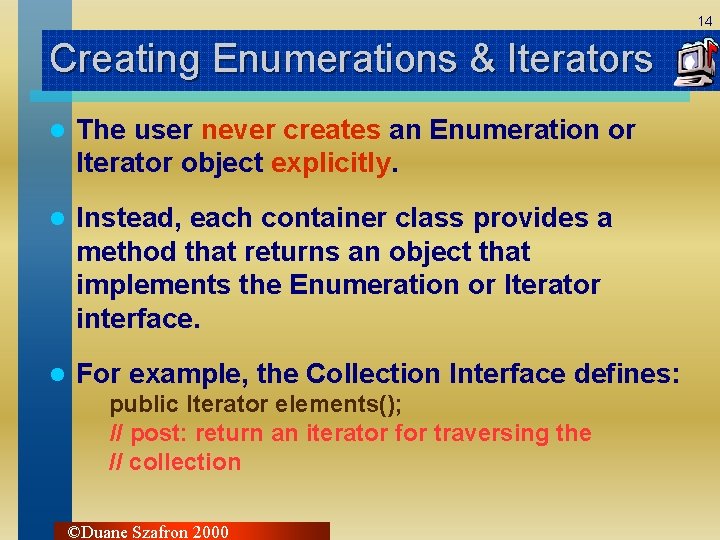
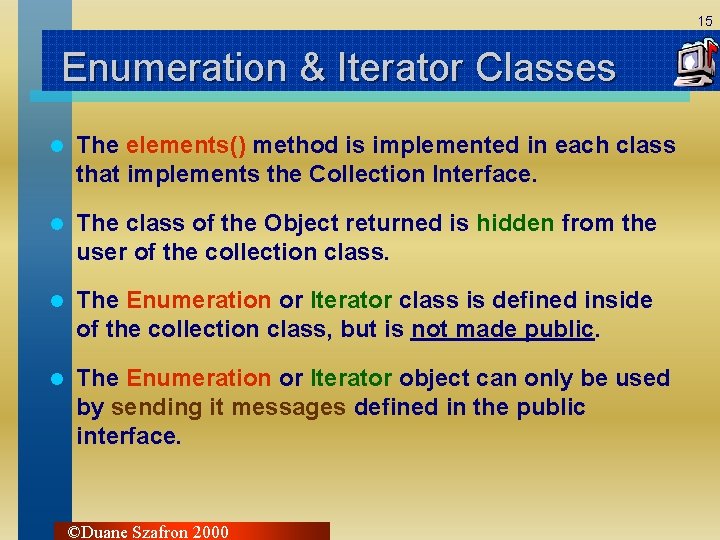
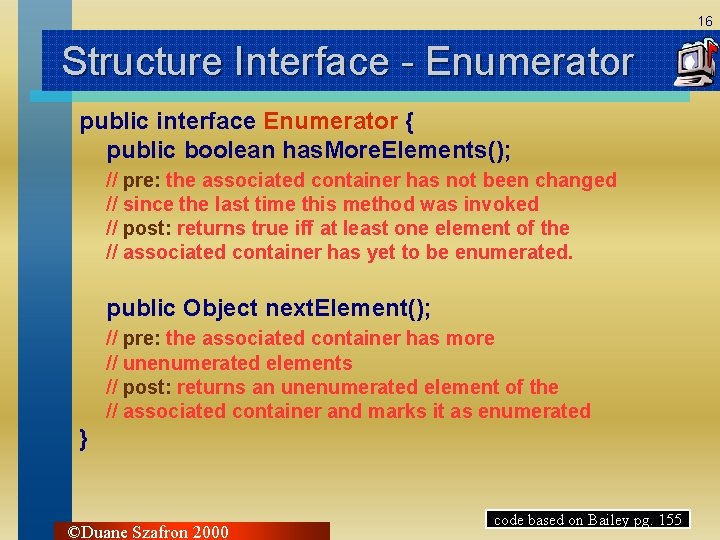
![17 Enumerator Example public static void main (String[ ] args) { Collection container; Enumeration 17 Enumerator Example public static void main (String[ ] args) { Collection container; Enumeration](https://slidetodoc.com/presentation_image_h2/77454b83a20aafdf8da922ca30096c21/image-17.jpg)
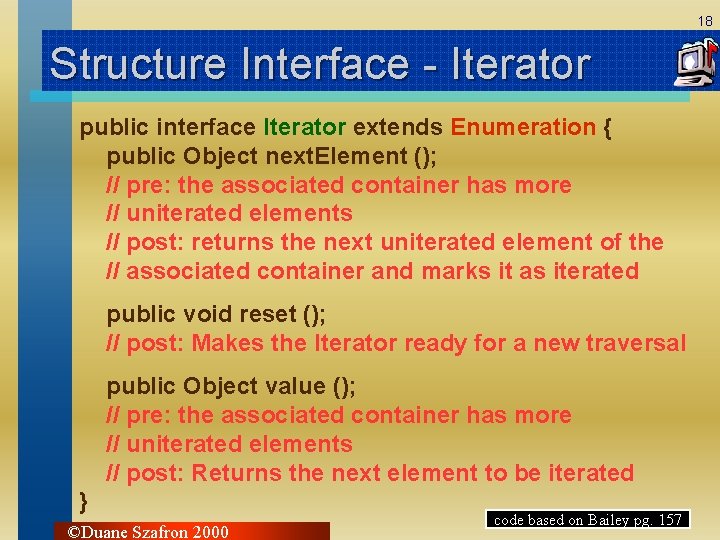
![19 Iterator Example public static void main (String[ ] args) { Vector container; Iterator 19 Iterator Example public static void main (String[ ] args) { Vector container; Iterator](https://slidetodoc.com/presentation_image_h2/77454b83a20aafdf8da922ca30096c21/image-19.jpg)
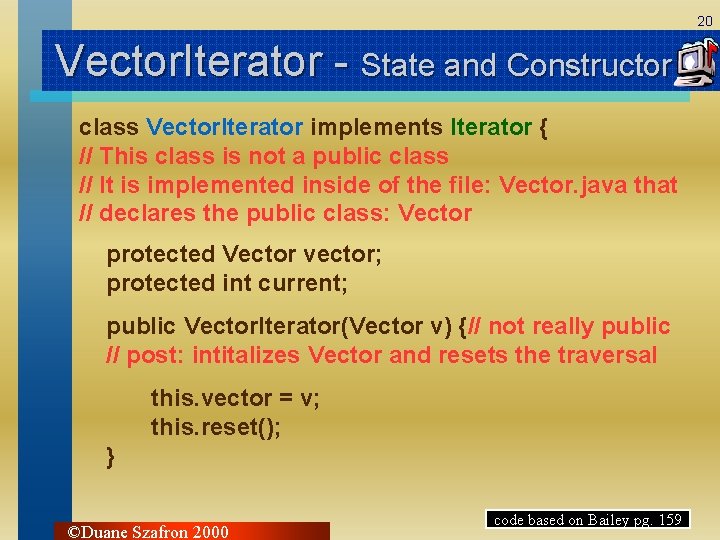
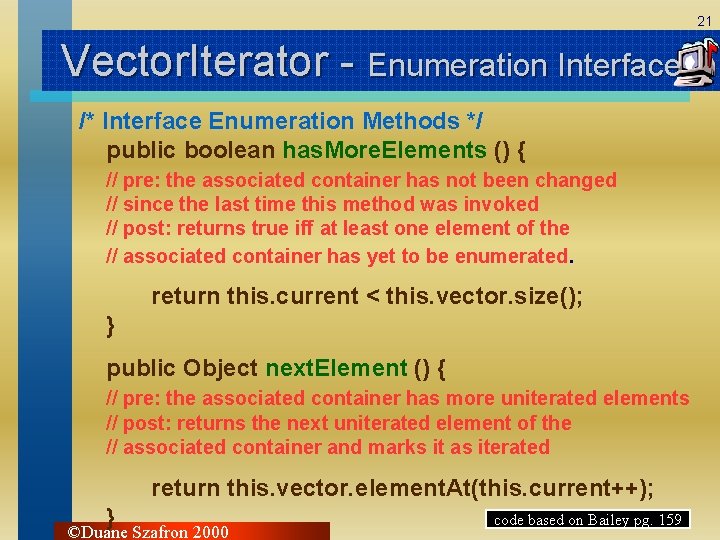
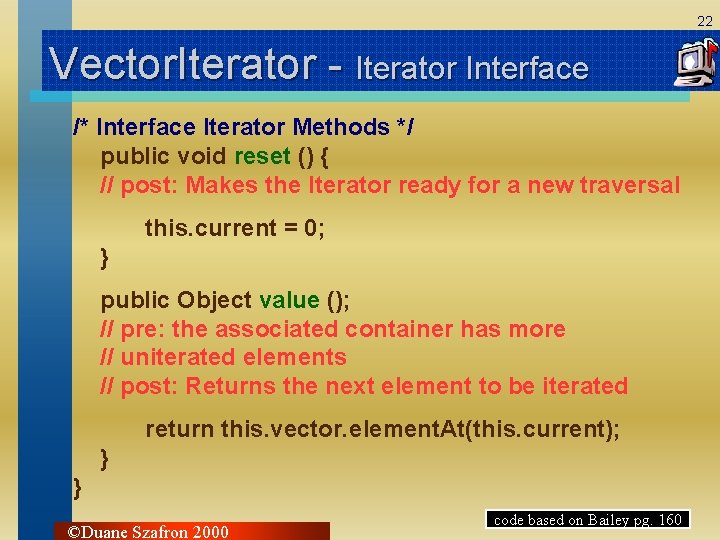
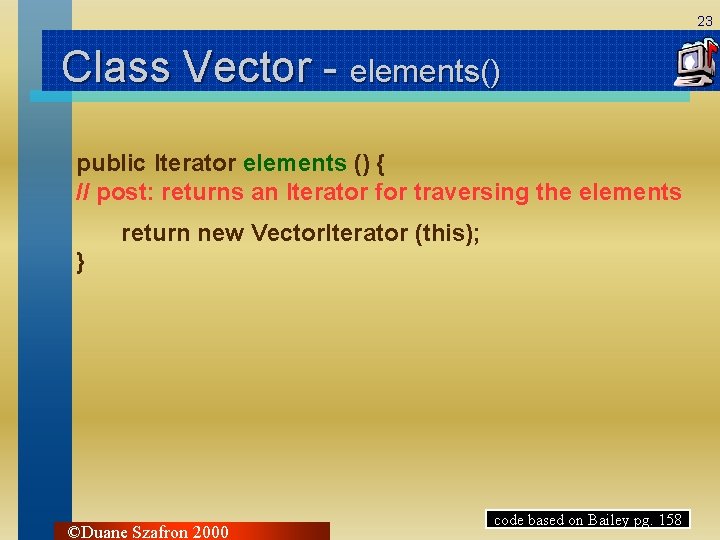
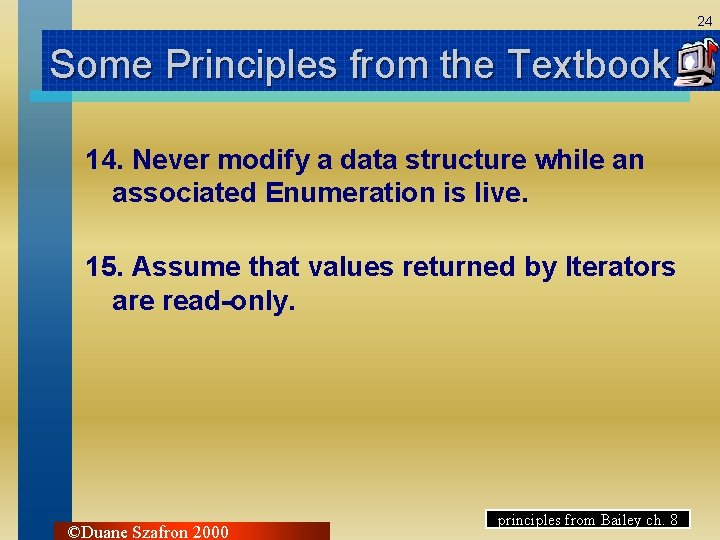
- Slides: 24
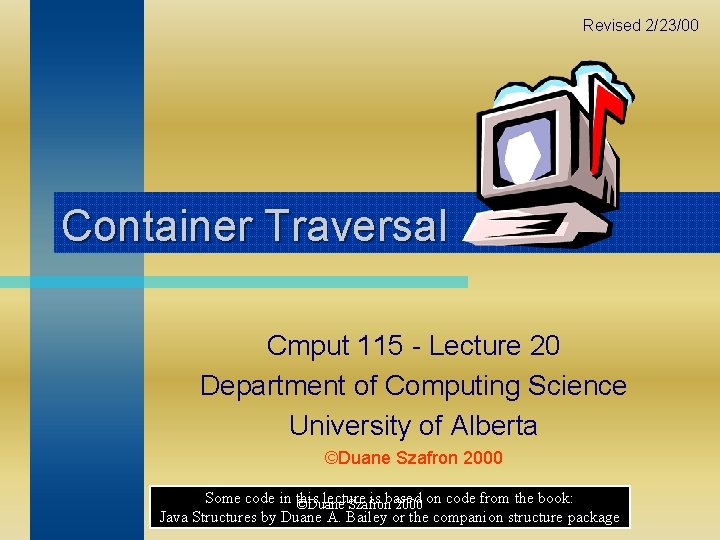
Revised 2/23/00 Container Traversal Cmput 115 - Lecture 20 Department of Computing Science University of Alberta ©Duane Szafron 2000 Some code in this lecture is based ©Duane Szafron 2000 on code from the book: Java Structures by Duane A. Bailey or the companion structure package
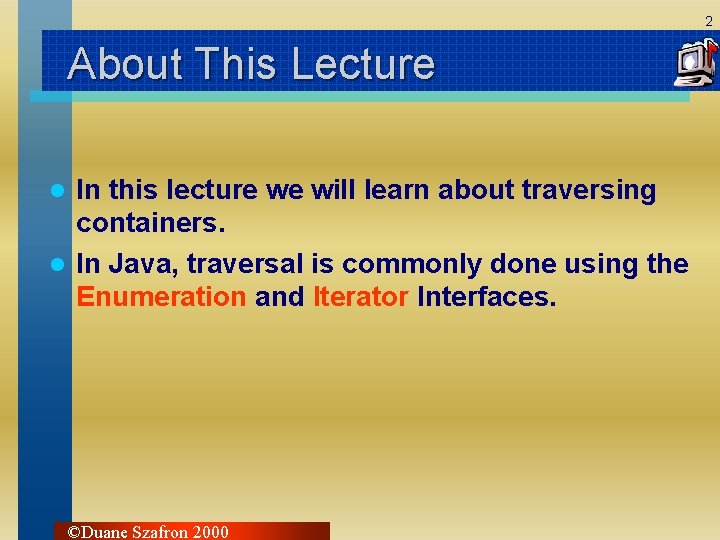
2 About This Lecture In this lecture we will learn about traversing containers. l In Java, traversal is commonly done using the Enumeration and Iterator Interfaces. l ©Duane Szafron 2000
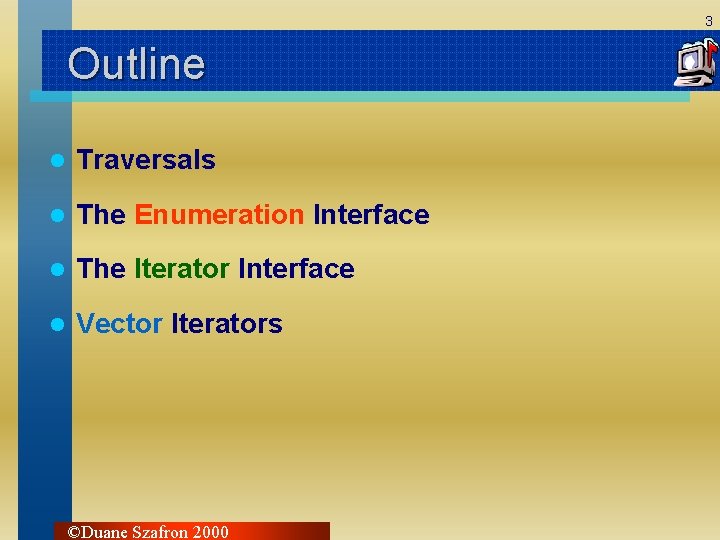
3 Outline l Traversals l The Enumeration Interface l The Iterator Interface l Vector Iterators ©Duane Szafron 2000
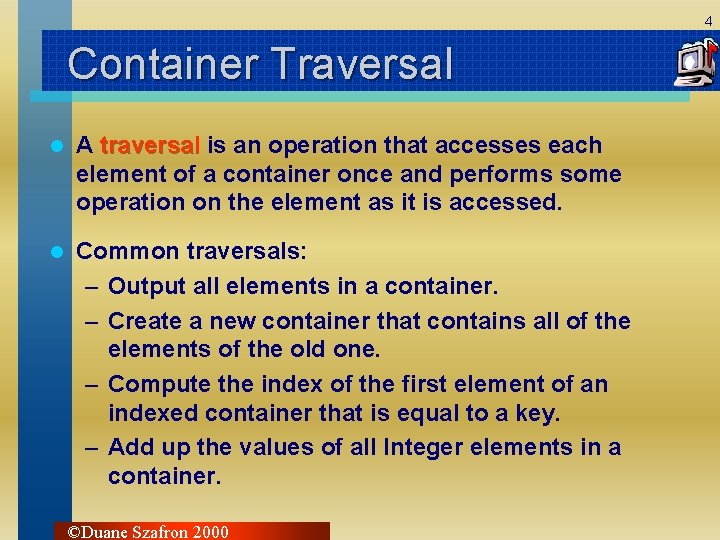
4 Container Traversal l A traversal is an operation that accesses each element of a container once and performs some operation on the element as it is accessed. l Common traversals: – Output all elements in a container. – Create a new container that contains all of the elements of the old one. – Compute the index of the first element of an indexed container that is equal to a key. – Add up the values of all Integer elements in a container. ©Duane Szafron 2000
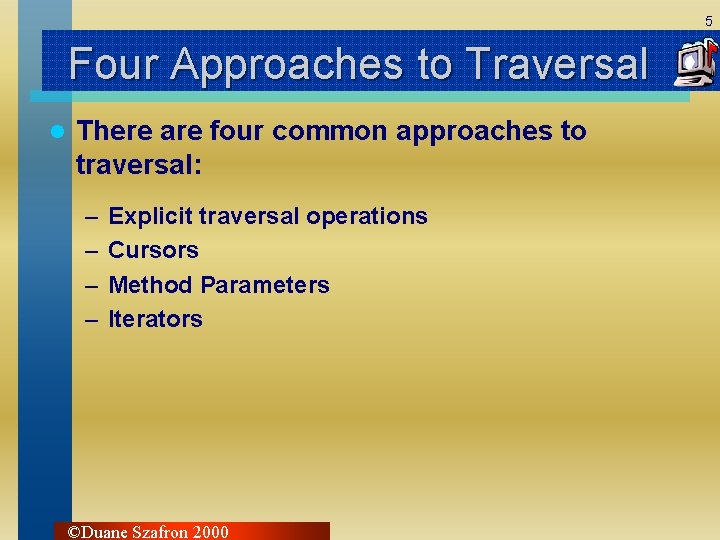
5 Four Approaches to Traversal l There are four common approaches to traversal: – – Explicit traversal operations Cursors Method Parameters Iterators ©Duane Szafron 2000
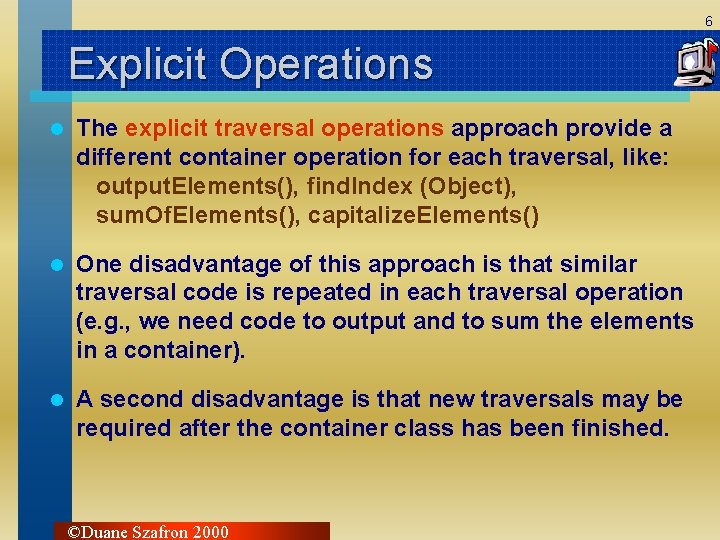
6 Explicit Operations l The explicit traversal operations approach provide a different container operation for each traversal, like: output. Elements(), find. Index (Object), sum. Of. Elements(), capitalize. Elements() l One disadvantage of this approach is that similar traversal code is repeated in each traversal operation (e. g. , we need code to output and to sum the elements in a container). l A second disadvantage is that new traversals may be required after the container class has been finished. ©Duane Szafron 2000
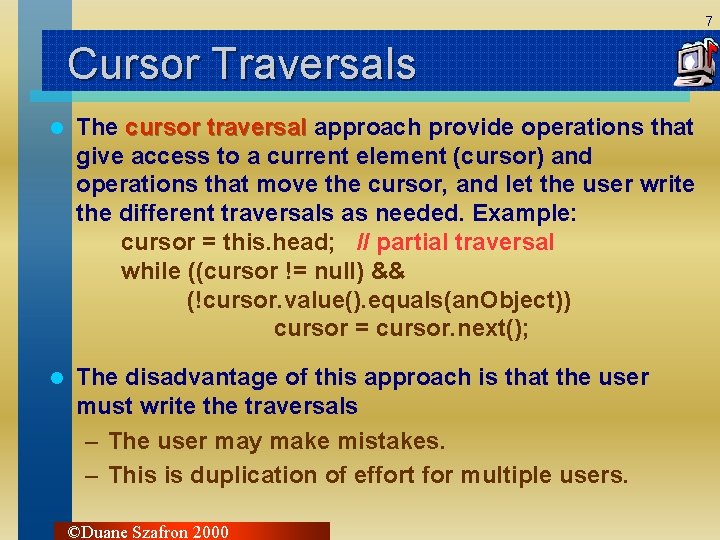
7 Cursor Traversals l The cursor traversal approach provide operations that give access to a current element (cursor) and operations that move the cursor, and let the user write the different traversals as needed. Example: cursor = this. head; // partial traversal while ((cursor != null) && (!cursor. value(). equals(an. Object)) cursor = cursor. next(); l The disadvantage of this approach is that the user must write the traversals – The user may make mistakes. – This is duplication of effort for multiple users. ©Duane Szafron 2000
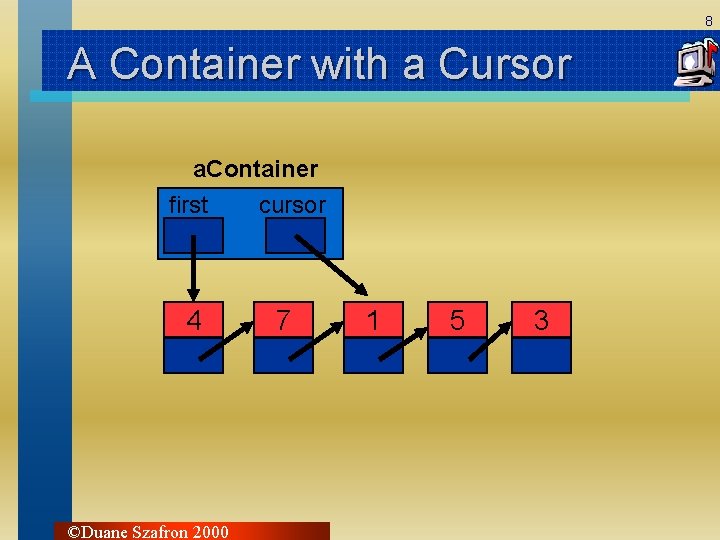
8 A Container with a Cursor a. Container first cursor 4 ©Duane Szafron 2000 7 1 5 3
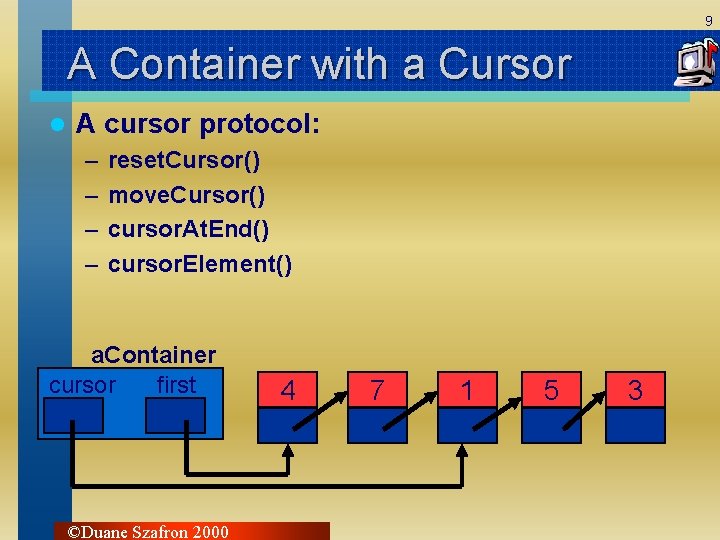
9 A Container with a Cursor l A cursor protocol: – – reset. Cursor() move. Cursor() cursor. At. End() cursor. Element() a. Container cursor first ©Duane Szafron 2000 4 7 1 5 3
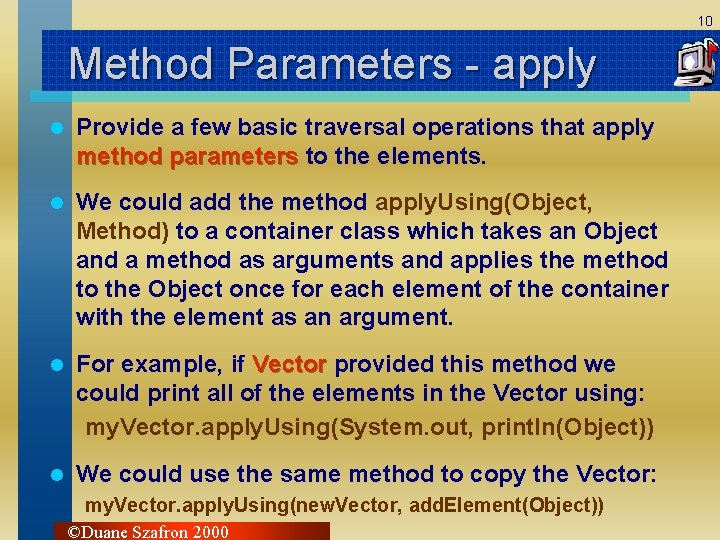
10 Method Parameters - apply l Provide a few basic traversal operations that apply method parameters to the elements. l We could add the method apply. Using(Object, Method) to a container class which takes an Object and a method as arguments and applies the method to the Object once for each element of the container with the element as an argument. l For example, if Vector provided this method we could print all of the elements in the Vector using: my. Vector. apply. Using(System. out, println(Object)) l We could use the same method to copy the Vector: my. Vector. apply. Using(new. Vector, add. Element(Object)) ©Duane Szafron 2000
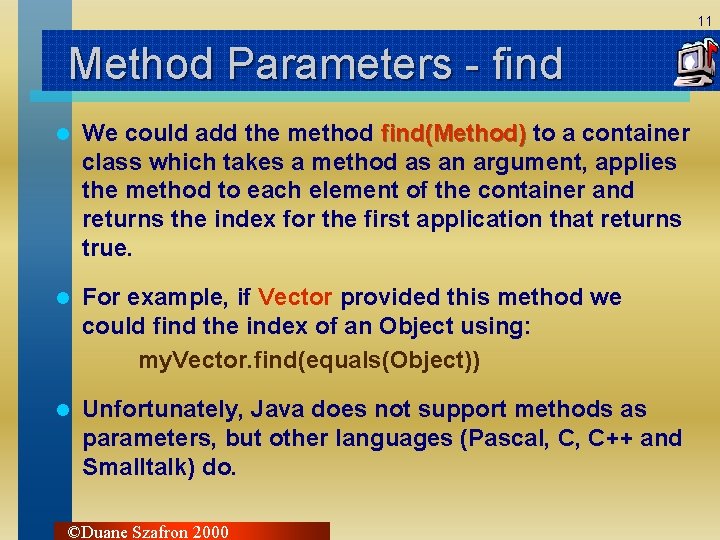
11 Method Parameters - find l We could add the method find(Method) to a container class which takes a method as an argument, applies the method to each element of the container and returns the index for the first application that returns true. l For example, if Vector provided this method we could find the index of an Object using: my. Vector. find(equals(Object)) l Unfortunately, Java does not support methods as parameters, but other languages (Pascal, C, C++ and Smalltalk) do. ©Duane Szafron 2000
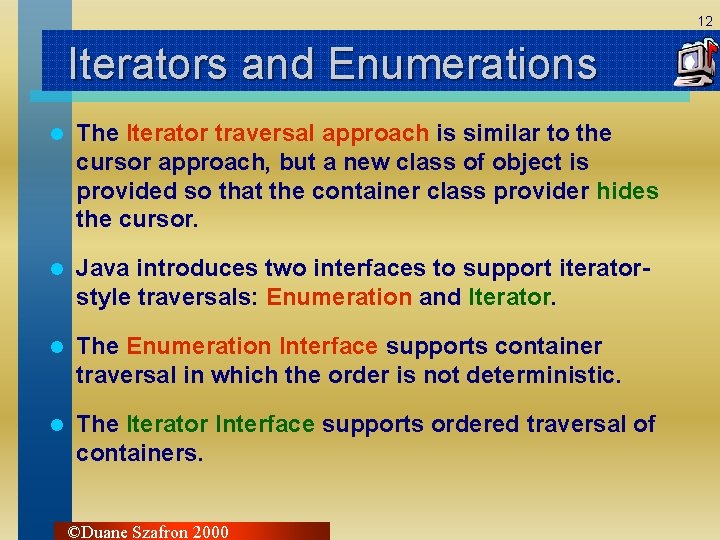
12 Iterators and Enumerations l The Iterator traversal approach is similar to the cursor approach, but a new class of object is provided so that the container class provider hides the cursor. l Java introduces two interfaces to support iteratorstyle traversals: Enumeration and Iterator. l The Enumeration Interface supports container traversal in which the order is not deterministic. l The Iterator Interface supports ordered traversal of containers. ©Duane Szafron 2000
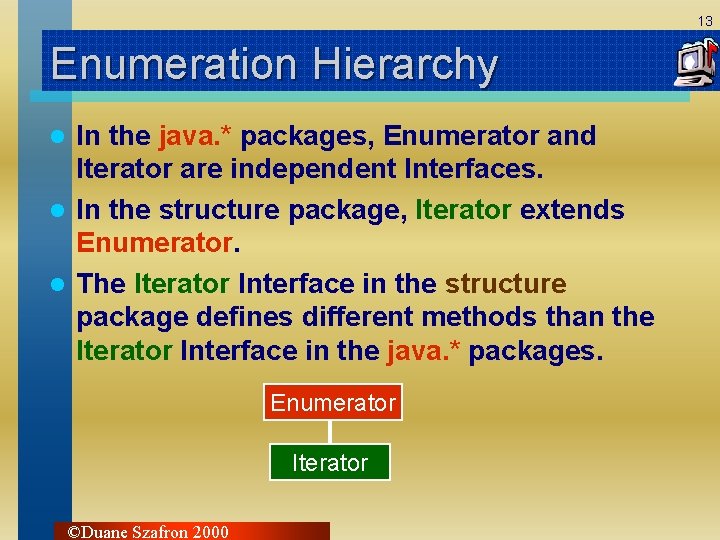
13 Enumeration Hierarchy In the java. * packages, Enumerator and Iterator are independent Interfaces. l In the structure package, Iterator extends Enumerator. l The Iterator Interface in the structure package defines different methods than the Iterator Interface in the java. * packages. l Enumerator Iterator ©Duane Szafron 2000
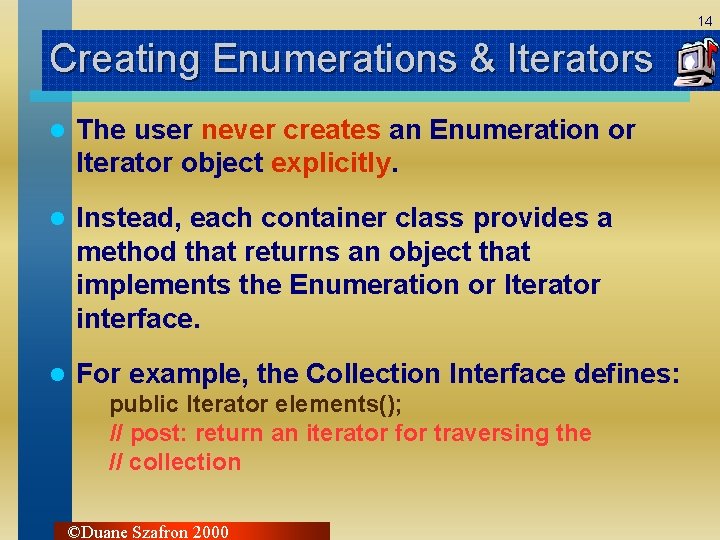
14 Creating Enumerations & Iterators l The user never creates an Enumeration or Iterator object explicitly. l Instead, each container class provides a method that returns an object that implements the Enumeration or Iterator interface. l For example, the Collection Interface defines: public Iterator elements(); // post: return an iterator for traversing the // collection ©Duane Szafron 2000
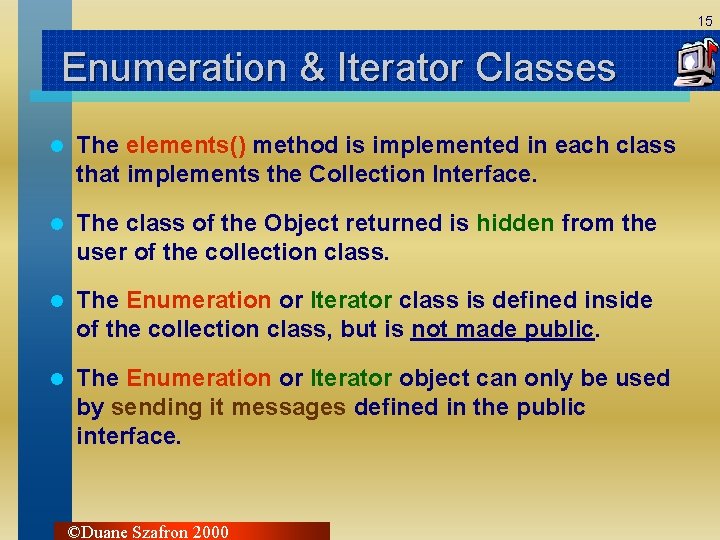
15 Enumeration & Iterator Classes l The elements() method is implemented in each class that implements the Collection Interface. l The class of the Object returned is hidden from the user of the collection class. l The Enumeration or Iterator class is defined inside of the collection class, but is not made public. l The Enumeration or Iterator object can only be used by sending it messages defined in the public interface. ©Duane Szafron 2000
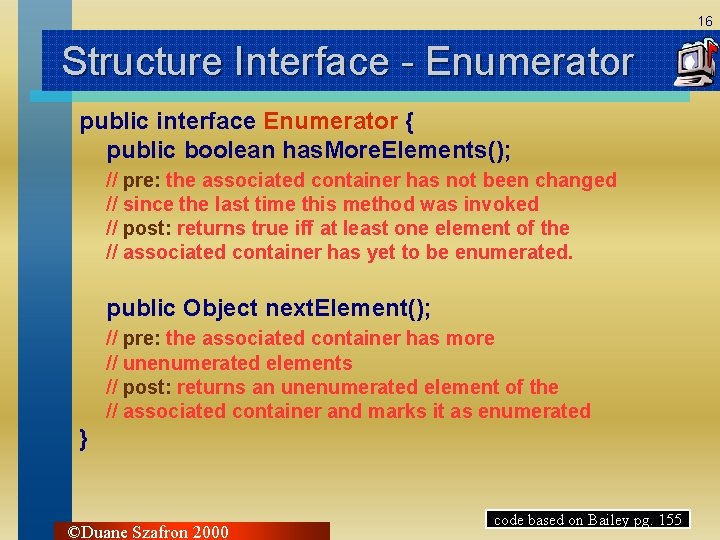
16 Structure Interface - Enumerator public interface Enumerator { public boolean has. More. Elements(); // pre: the associated container has not been changed // since the last time this method was invoked // post: returns true iff at least one element of the // associated container has yet to be enumerated. public Object next. Element(); // pre: the associated container has more // unenumerated elements // post: returns an unenumerated element of the // associated container and marks it as enumerated } ©Duane Szafron 2000 code based on Bailey pg. 155
![17 Enumerator Example public static void main String args Collection container Enumeration 17 Enumerator Example public static void main (String[ ] args) { Collection container; Enumeration](https://slidetodoc.com/presentation_image_h2/77454b83a20aafdf8da922ca30096c21/image-17.jpg)
17 Enumerator Example public static void main (String[ ] args) { Collection container; Enumeration enumerator; container = new Set. List(); Barney container. add(“Fred”); Fred container. add(“Barney”); enumerator = container. elements(); while (enumerator. has. More. Elements()) System. out. println(enumerator. next. Element()); } ©Duane Szafron 2000 code based on Bailey pg. 158
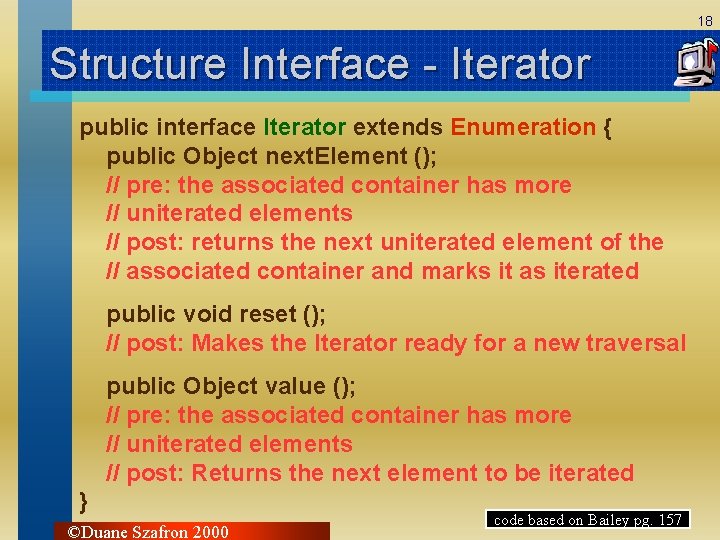
18 Structure Interface - Iterator public interface Iterator extends Enumeration { public Object next. Element (); // pre: the associated container has more // uniterated elements // post: returns the next uniterated element of the // associated container and marks it as iterated public void reset (); // post: Makes the Iterator ready for a new traversal public Object value (); // pre: the associated container has more // uniterated elements // post: Returns the next element to be iterated } ©Duane Szafron 2000 code based on Bailey pg. 157
![19 Iterator Example public static void main String args Vector container Iterator 19 Iterator Example public static void main (String[ ] args) { Vector container; Iterator](https://slidetodoc.com/presentation_image_h2/77454b83a20aafdf8da922ca30096c21/image-19.jpg)
19 Iterator Example public static void main (String[ ] args) { Vector container; Iterator iterator; container = new Vector(); Fred container. add. Element(“Fred”); Fred container. add. Element(“Barney”); Barney iterator = container. elements(); Barney while (iterator. has. More. Elements()) { System. out. println(iterator. value()); System. out. println(iterator. next. Element()); } } ©Duane Szafron 2000 code based on Bailey pg. 158
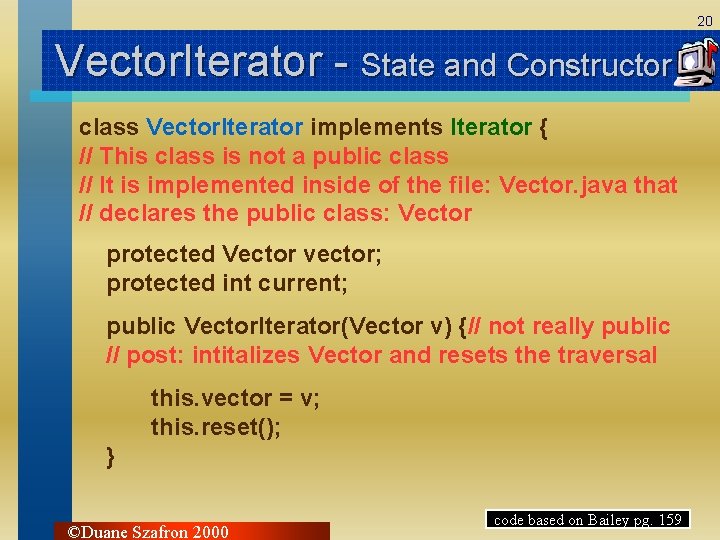
20 Vector. Iterator - State and Constructor class Vector. Iterator implements Iterator { // This class is not a public class // It is implemented inside of the file: Vector. java that // declares the public class: Vector protected Vector vector; protected int current; public Vector. Iterator(Vector v) {// not really public // post: intitalizes Vector and resets the traversal this. vector = v; this. reset(); } ©Duane Szafron 2000 code based on Bailey pg. 159
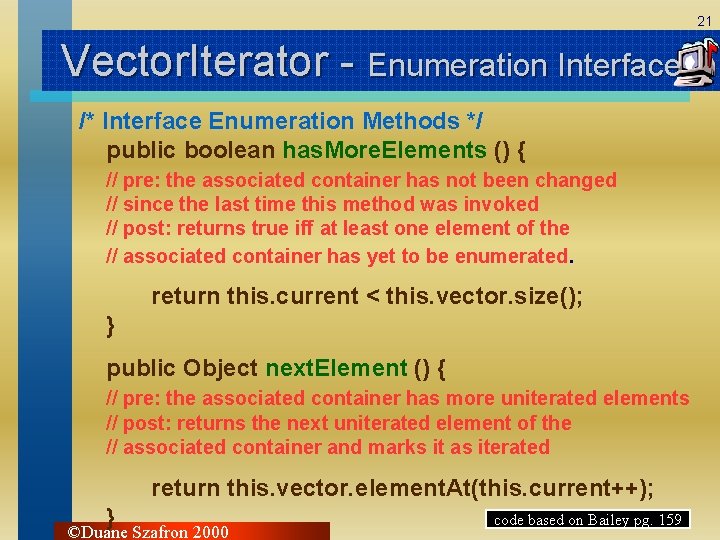
21 Vector. Iterator - Enumeration Interface /* Interface Enumeration Methods */ public boolean has. More. Elements () { // pre: the associated container has not been changed // since the last time this method was invoked // post: returns true iff at least one element of the // associated container has yet to be enumerated. return this. current < this. vector. size(); } public Object next. Element () { // pre: the associated container has more uniterated elements // post: returns the next uniterated element of the // associated container and marks it as iterated return this. vector. element. At(this. current++); } ©Duane Szafron 2000 code based on Bailey pg. 159
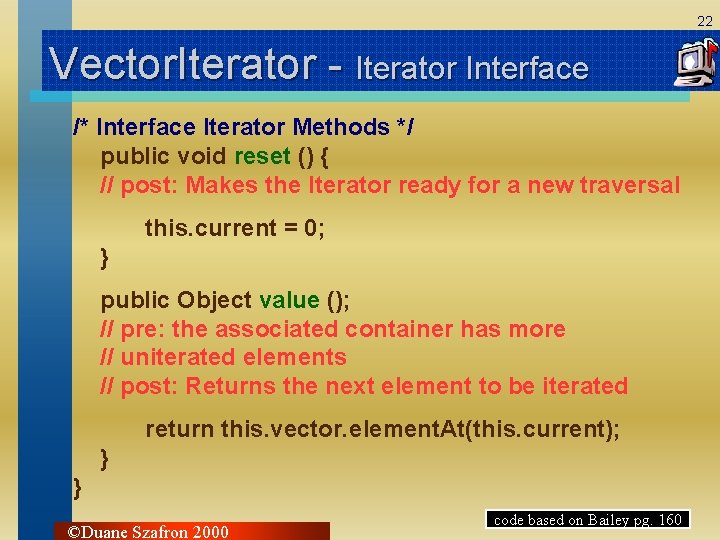
22 Vector. Iterator - Iterator Interface /* Interface Iterator Methods */ public void reset () { // post: Makes the Iterator ready for a new traversal this. current = 0; } public Object value (); // pre: the associated container has more // uniterated elements // post: Returns the next element to be iterated return this. vector. element. At(this. current); } } ©Duane Szafron 2000 code based on Bailey pg. 160
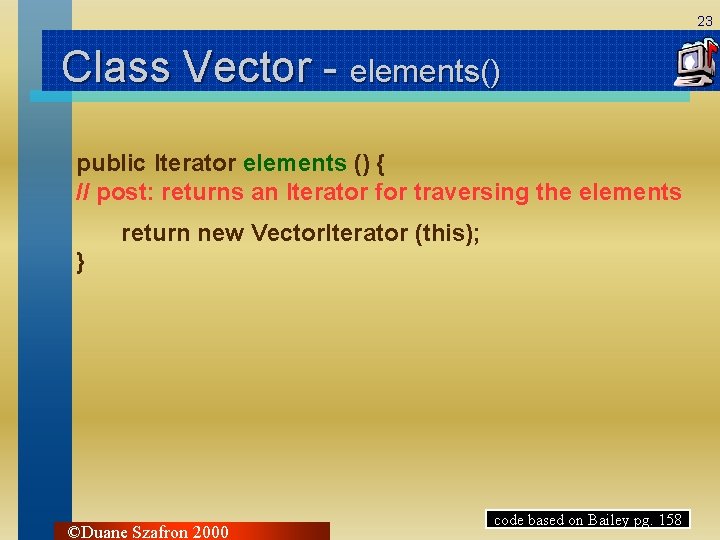
23 Class Vector - elements() public Iterator elements () { // post: returns an Iterator for traversing the elements return new Vector. Iterator (this); } ©Duane Szafron 2000 code based on Bailey pg. 158
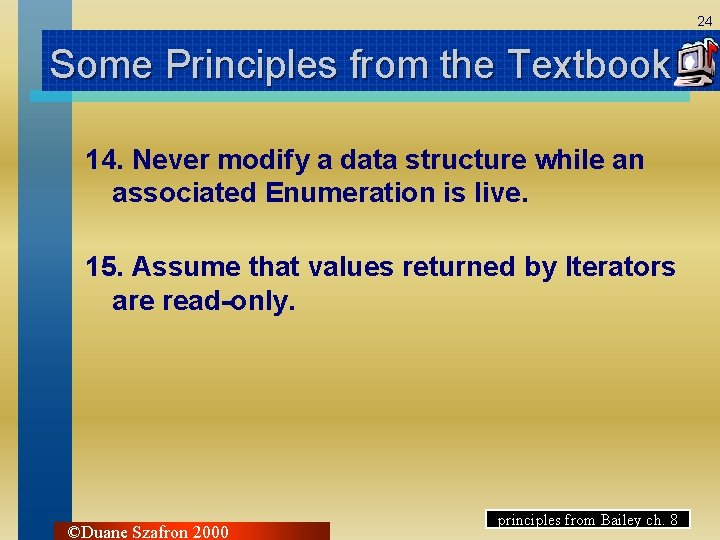
24 Some Principles from the Textbook 14. Never modify a data structure while an associated Enumeration is live. 15. Assume that values returned by Iterators are read-only. ©Duane Szafron 2000 principles from Bailey ch. 8