MIPS Functions and the Runtime Stack COE 301
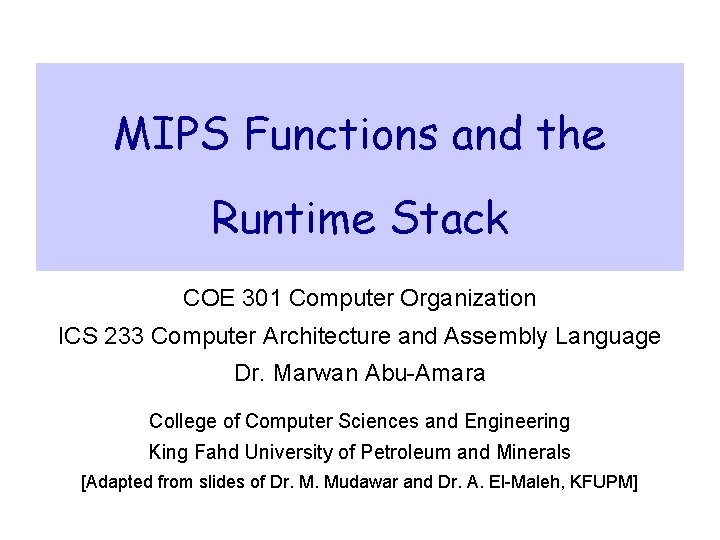
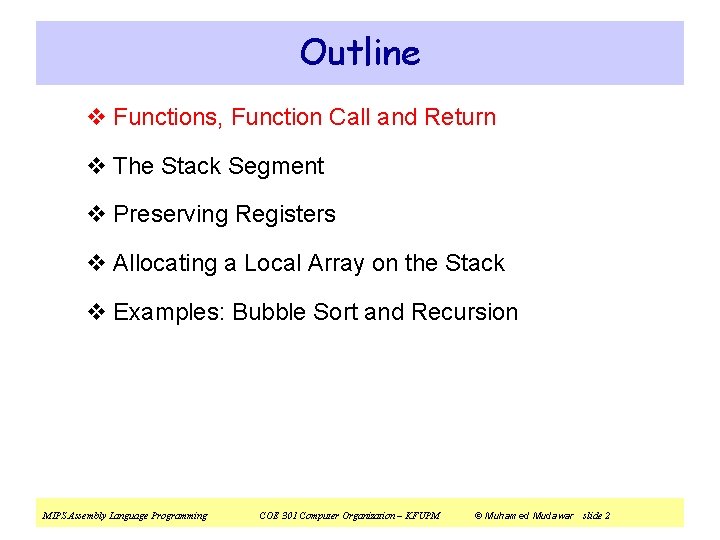
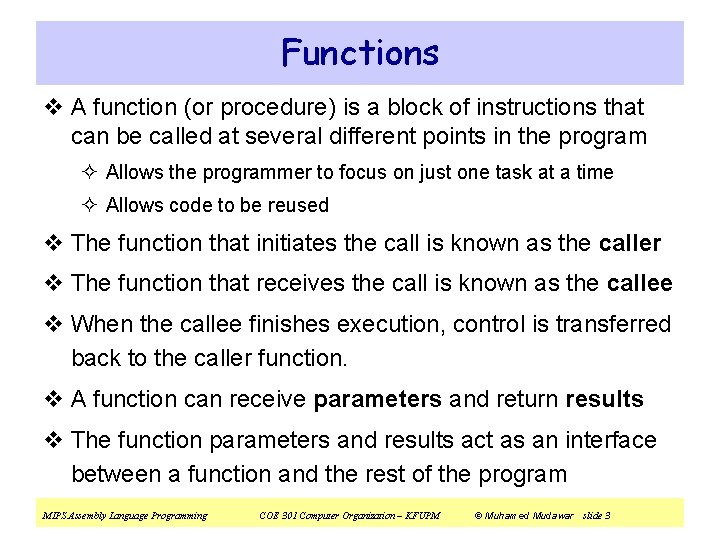
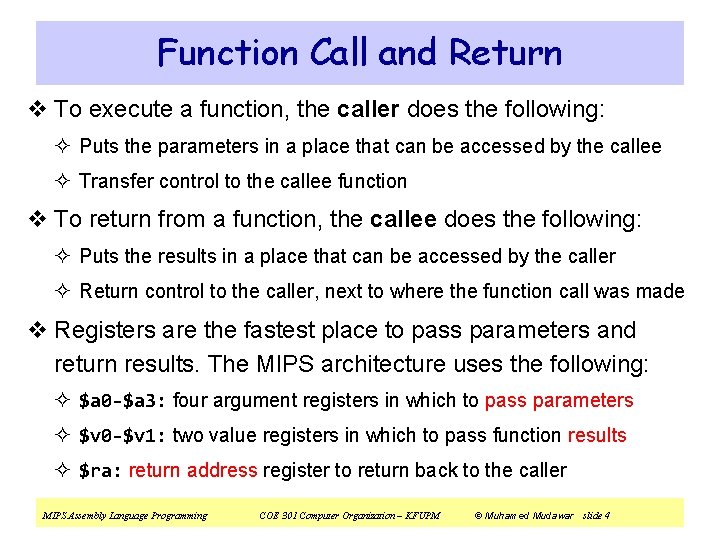
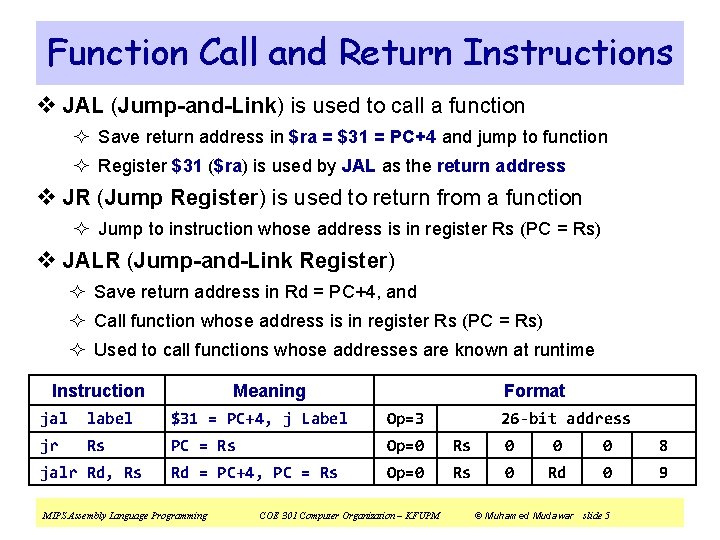
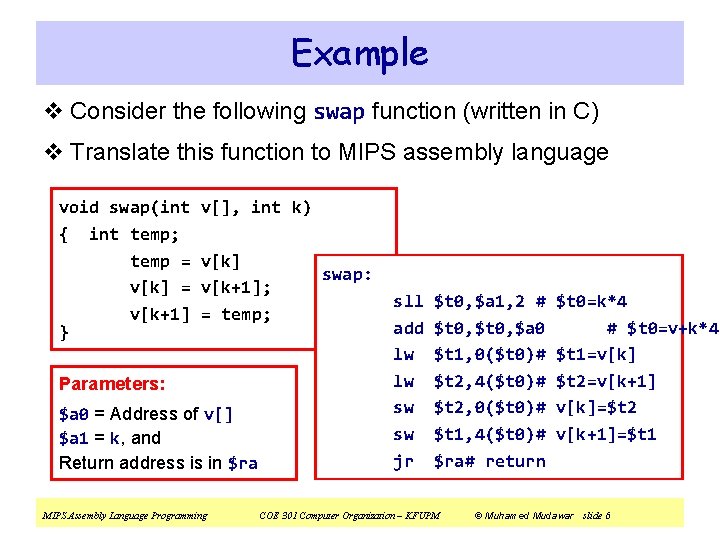
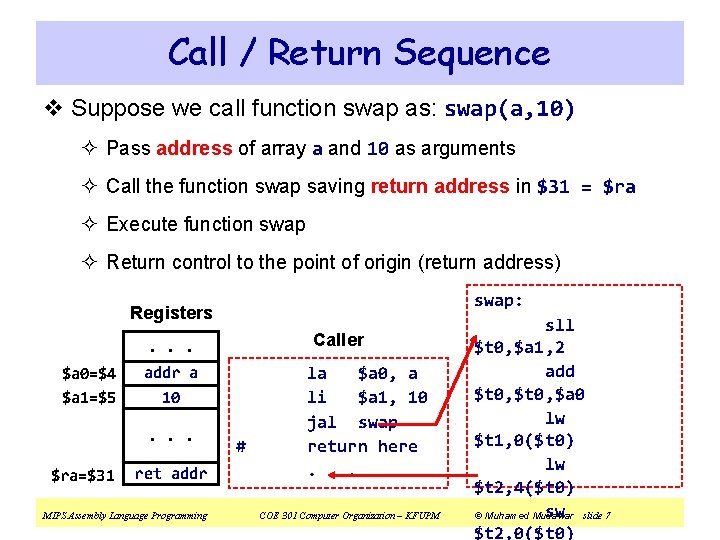
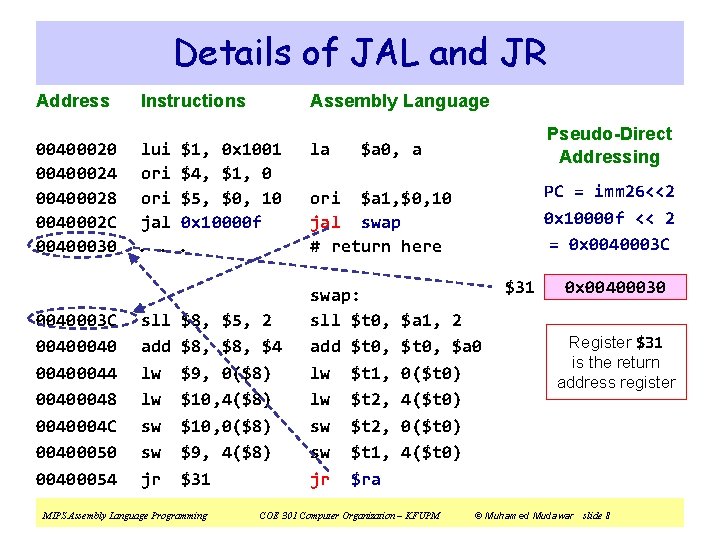
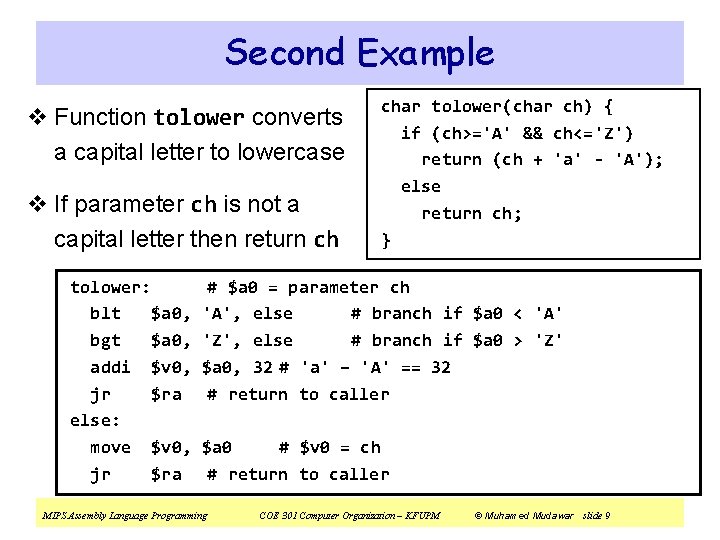
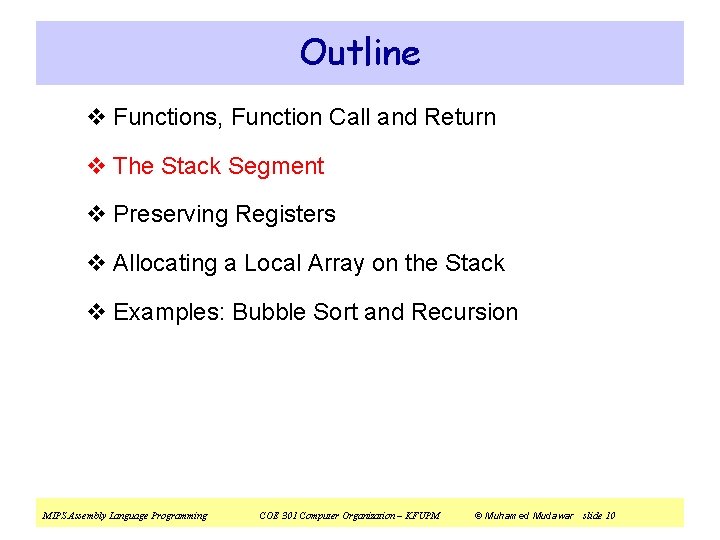
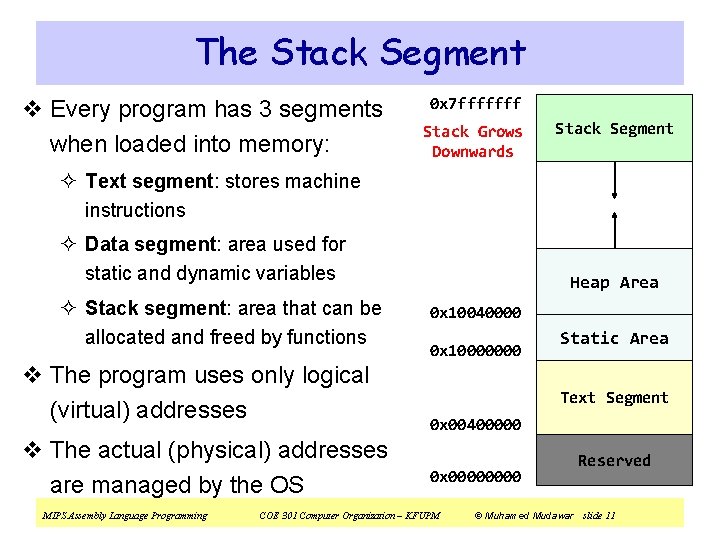
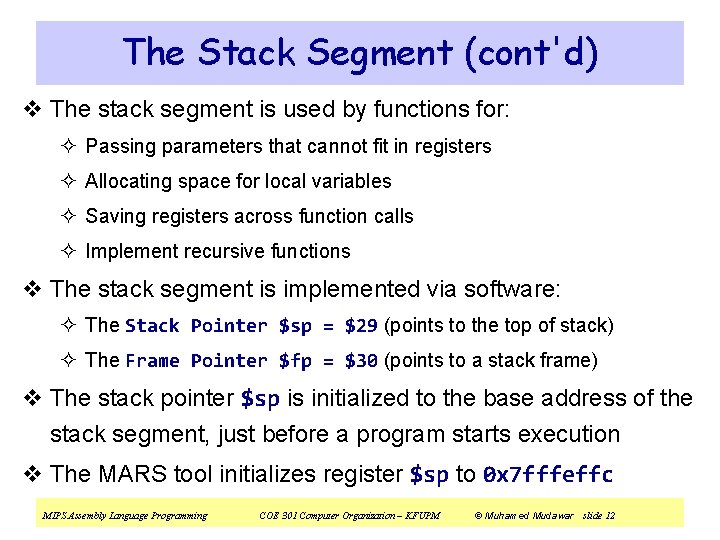
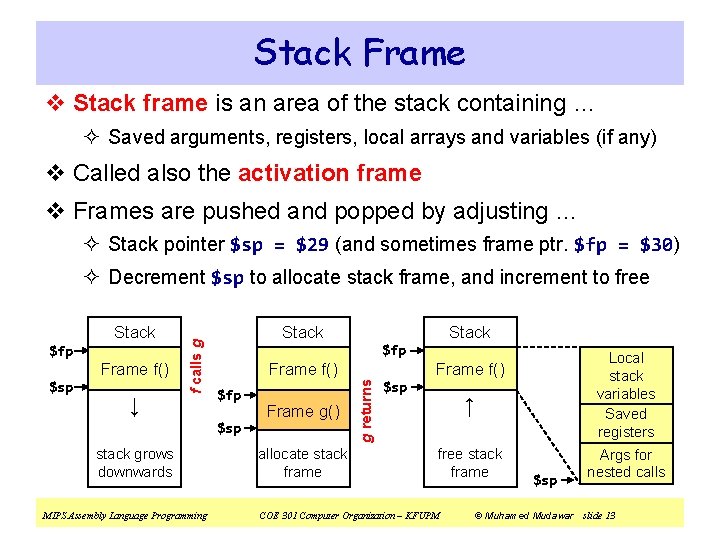
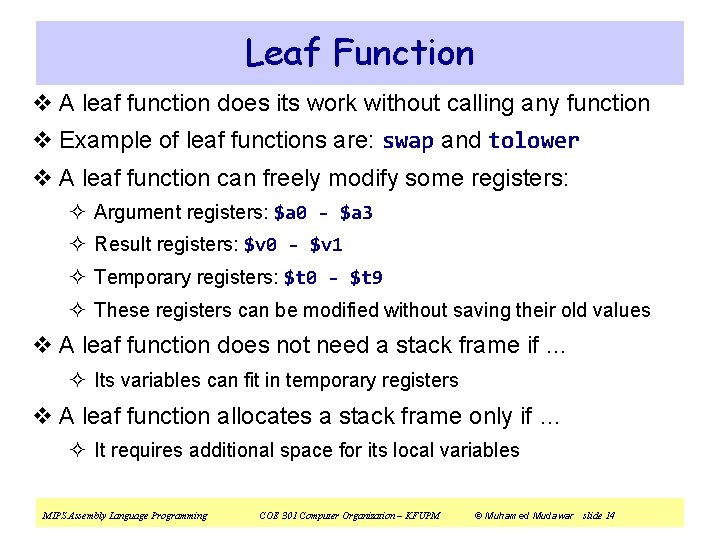
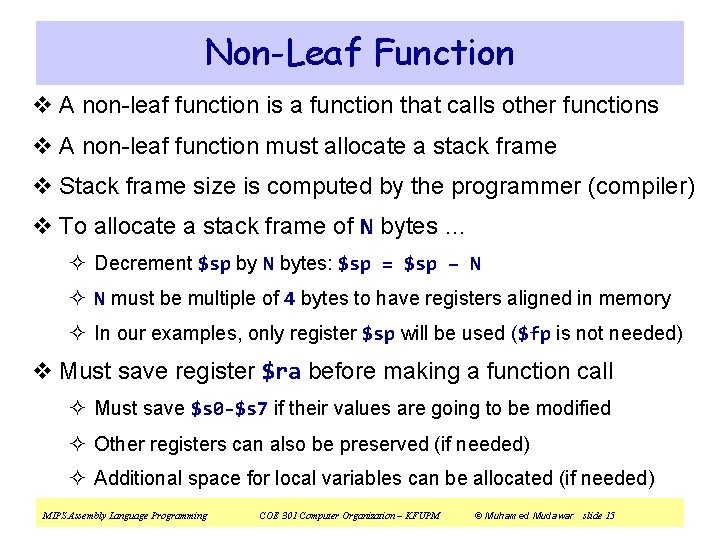
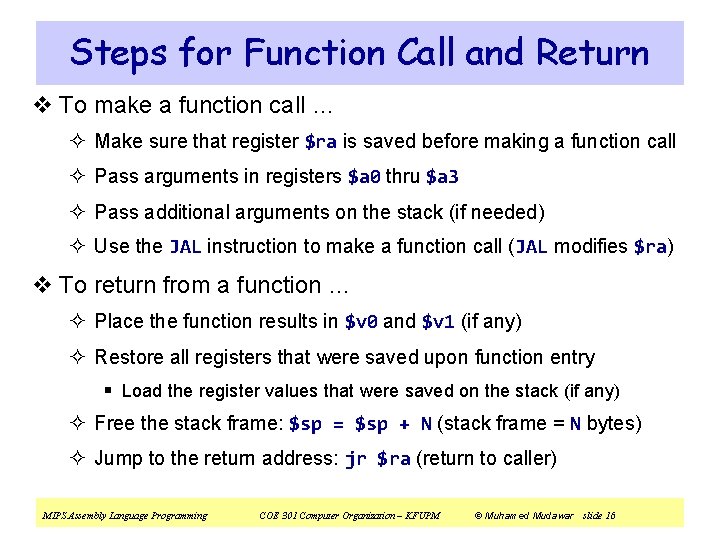
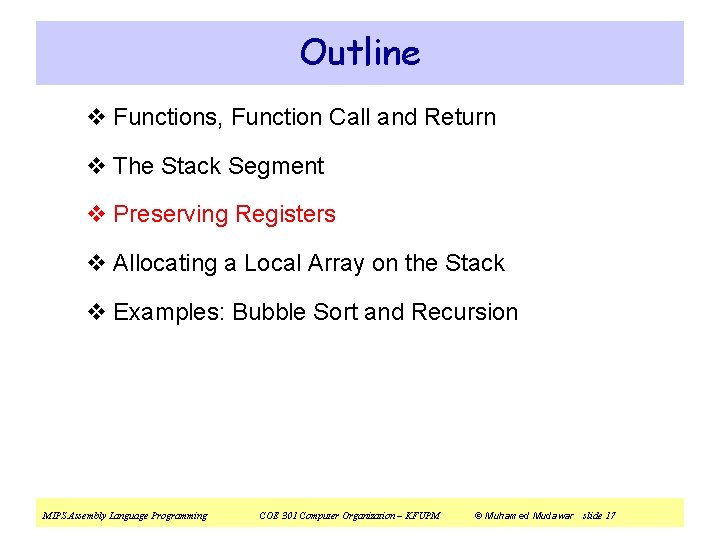
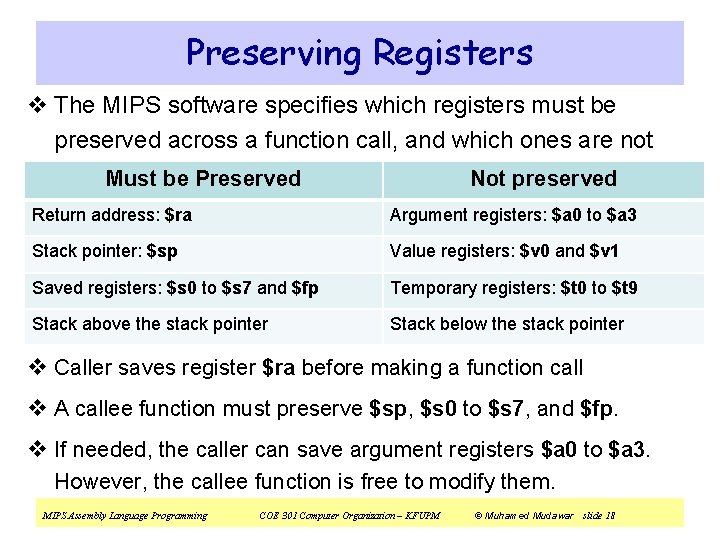
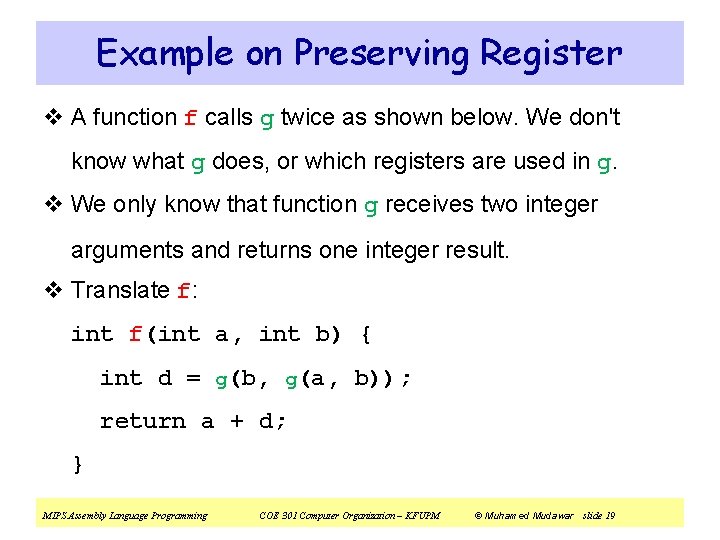
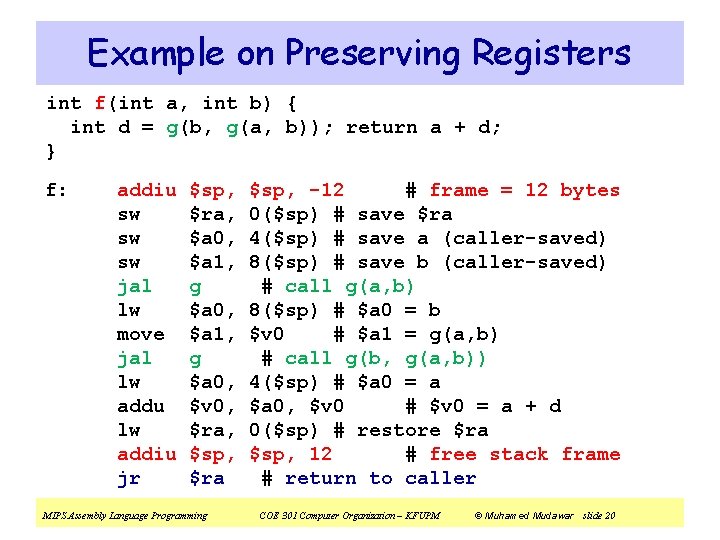
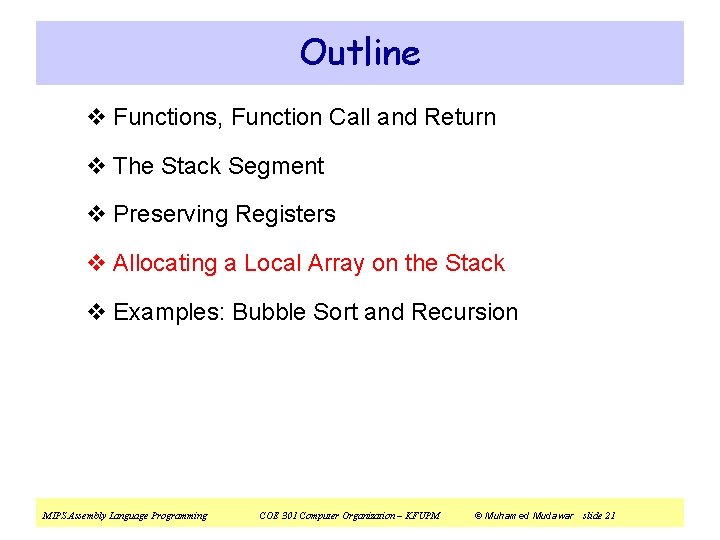
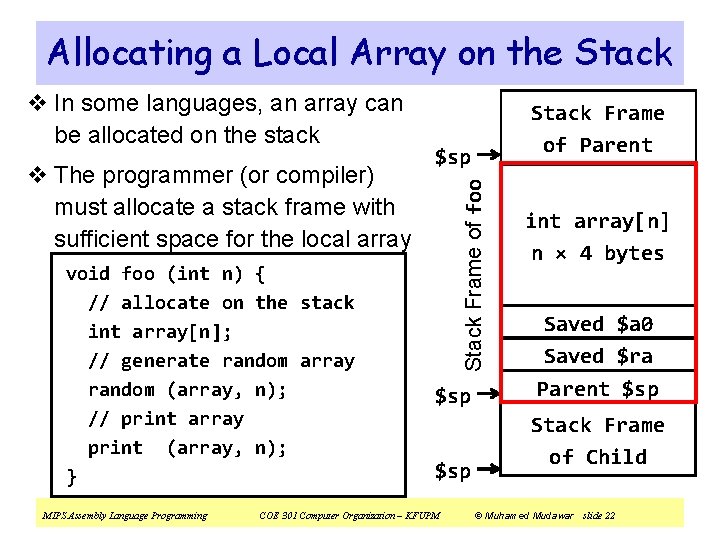
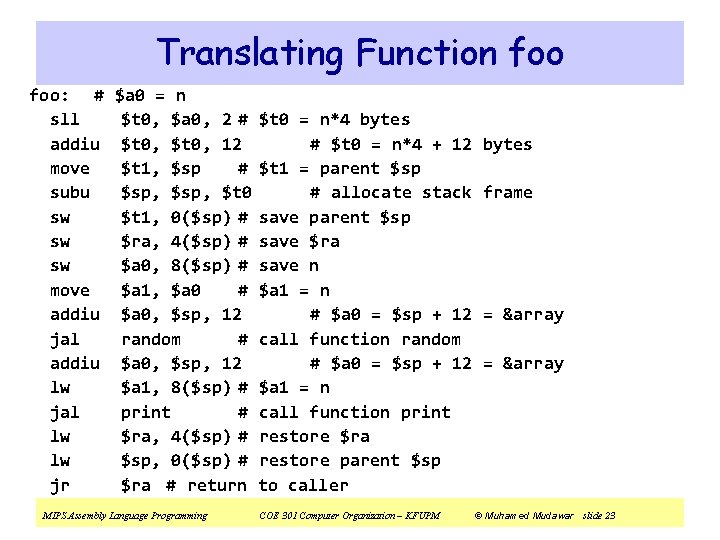
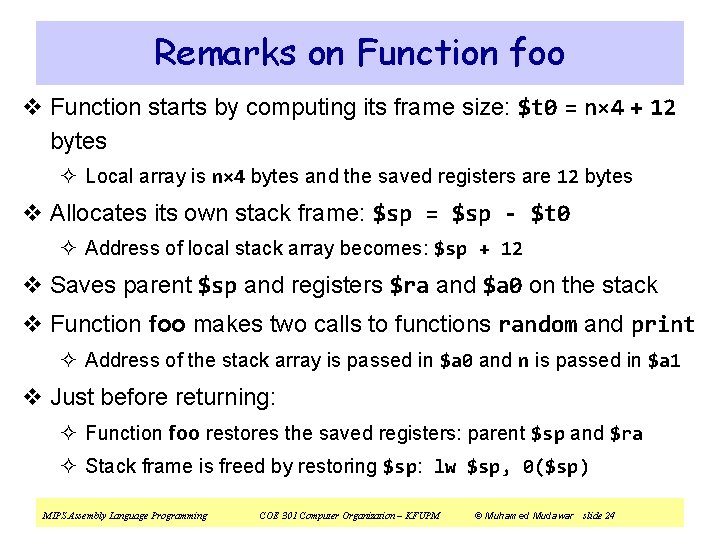
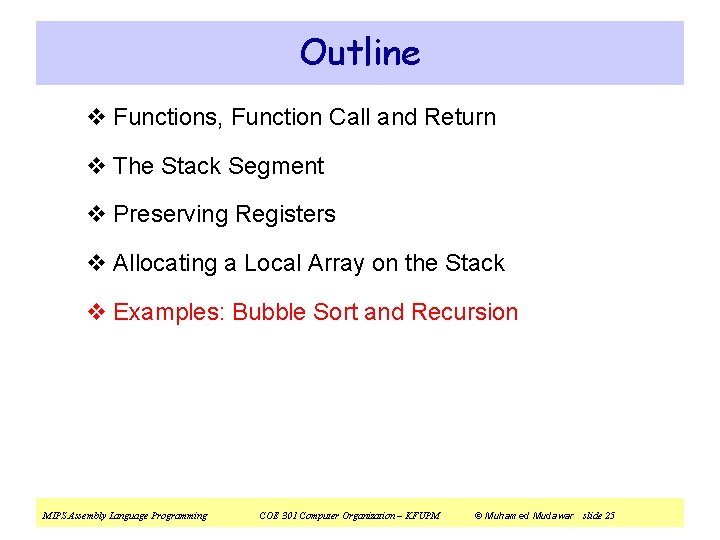
![Bubble Sort (Leaf Function) void bubble. Sort (int A[], int n) { int swapped, Bubble Sort (Leaf Function) void bubble. Sort (int A[], int n) { int swapped,](https://slidetodoc.com/presentation_image_h2/5badd13152ce6d6c1d9cdb1c7dfb552f/image-26.jpg)
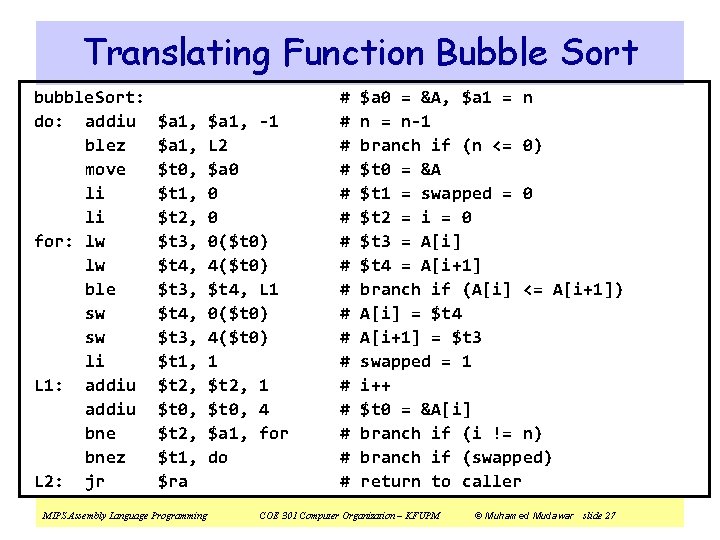
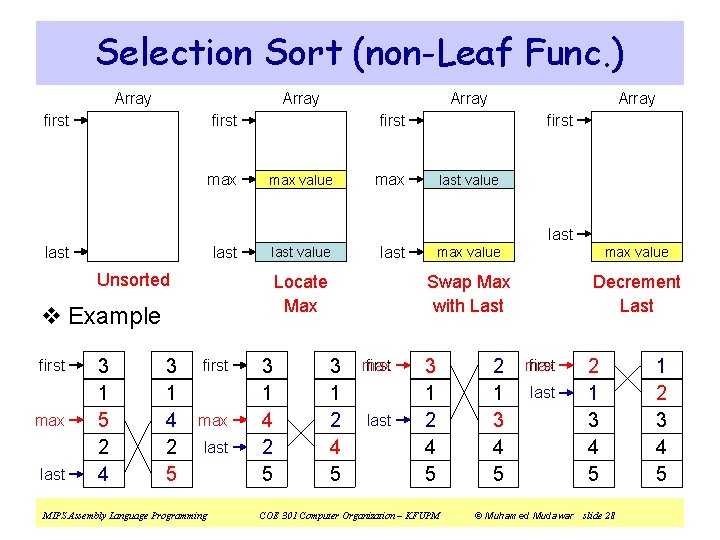
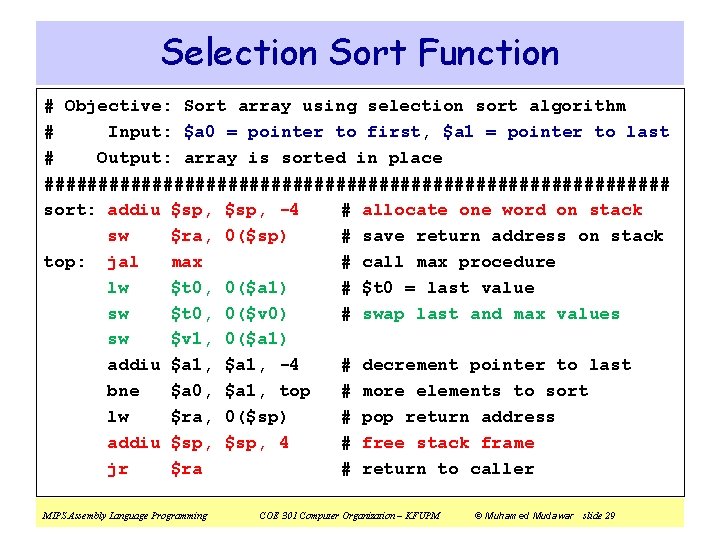
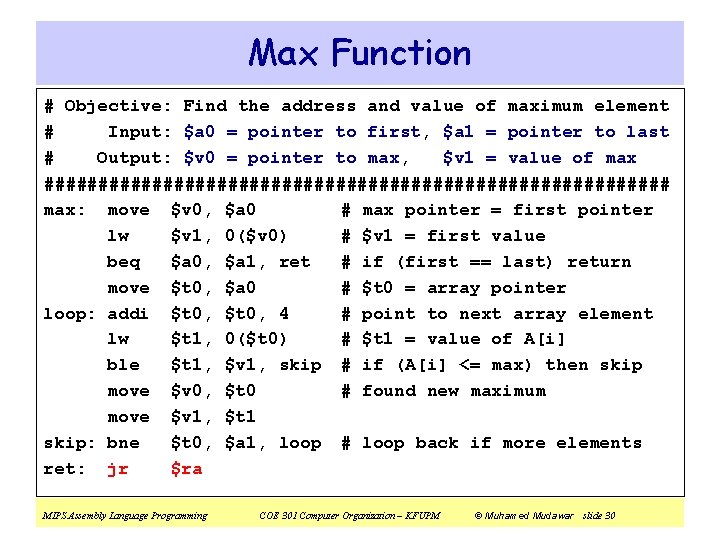
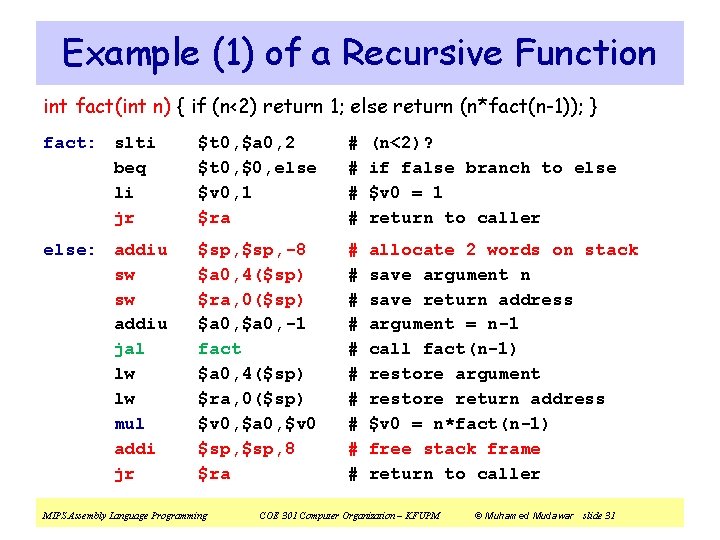
![Example (2) of a Recursive Function int recursive_sum (int A[], int n) { if Example (2) of a Recursive Function int recursive_sum (int A[], int n) { if](https://slidetodoc.com/presentation_image_h2/5badd13152ce6d6c1d9cdb1c7dfb552f/image-32.jpg)
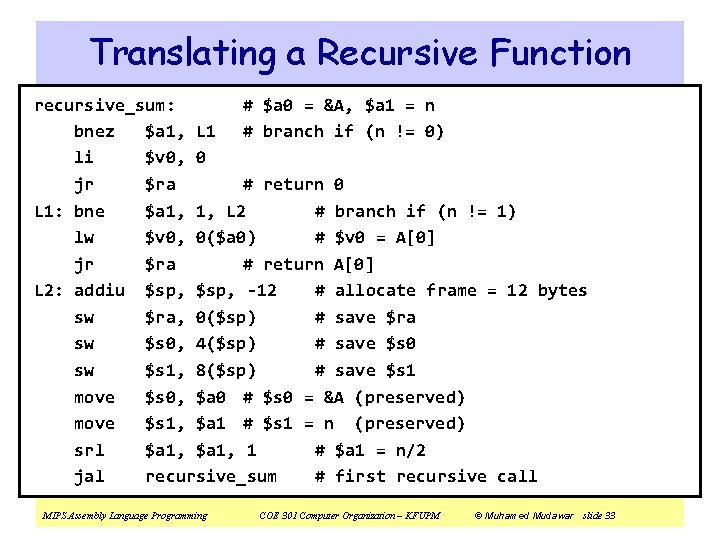
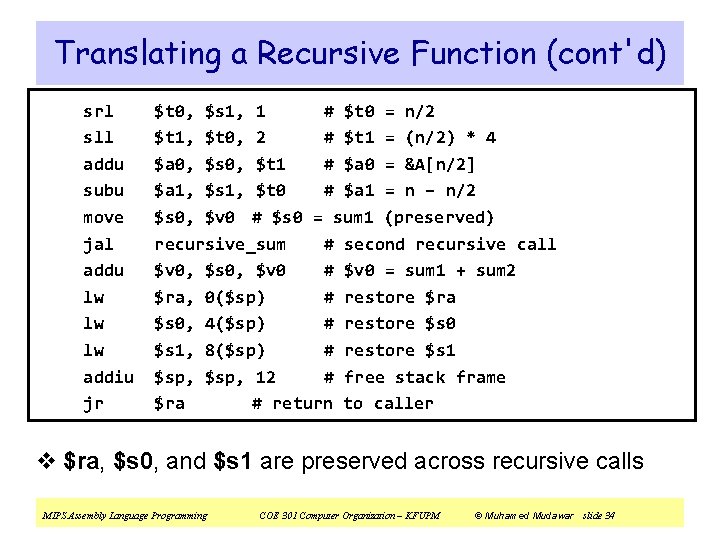
![Illustrating Recursive Calls $v 0 = A[0]+A[1]+A[2]+A[3]+A[4]+A[5] recursive_sum: $a 0 = &A[0], $a 1 Illustrating Recursive Calls $v 0 = A[0]+A[1]+A[2]+A[3]+A[4]+A[5] recursive_sum: $a 0 = &A[0], $a 1](https://slidetodoc.com/presentation_image_h2/5badd13152ce6d6c1d9cdb1c7dfb552f/image-35.jpg)
- Slides: 35
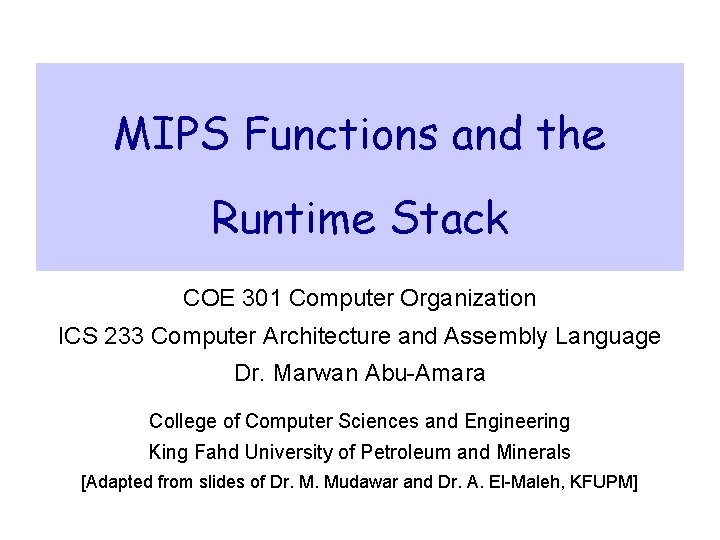
MIPS Functions and the Runtime Stack COE 301 Computer Organization ICS 233 Computer Architecture and Assembly Language Dr. Marwan Abu-Amara College of Computer Sciences and Engineering King Fahd University of Petroleum and Minerals [Adapted from slides of Dr. M. Mudawar and Dr. A. El-Maleh, KFUPM]
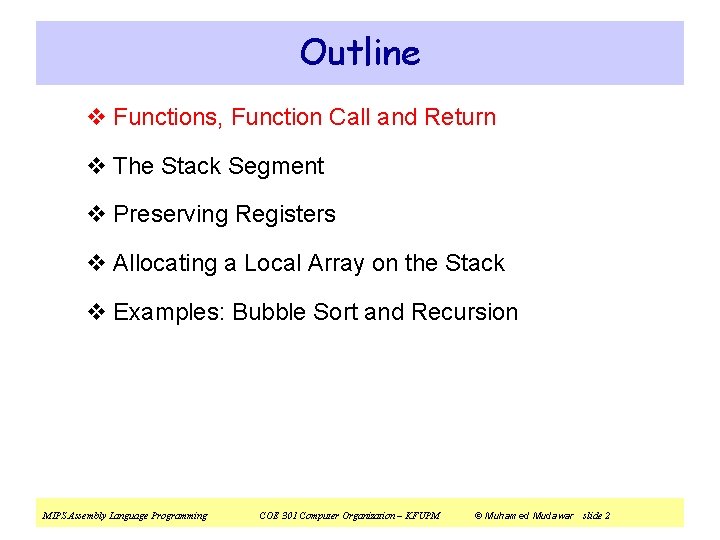
Outline v Functions, Function Call and Return v The Stack Segment v Preserving Registers v Allocating a Local Array on the Stack v Examples: Bubble Sort and Recursion MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 2
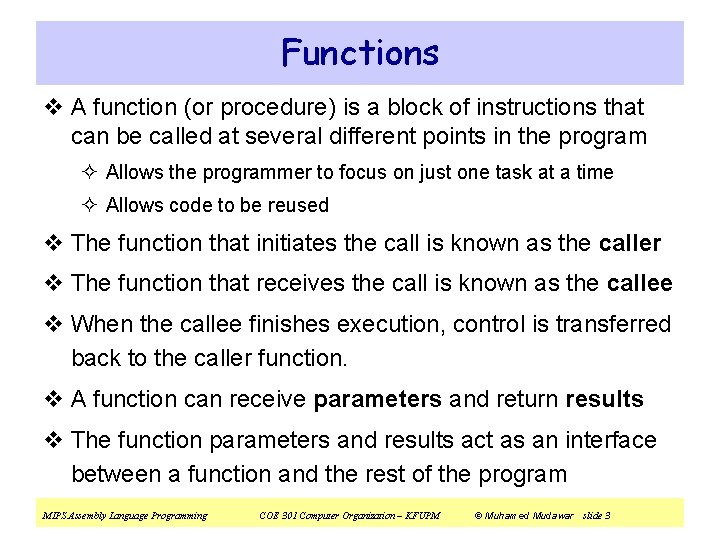
Functions v A function (or procedure) is a block of instructions that can be called at several different points in the program ² Allows the programmer to focus on just one task at a time ² Allows code to be reused v The function that initiates the call is known as the caller v The function that receives the call is known as the callee v When the callee finishes execution, control is transferred back to the caller function. v A function can receive parameters and return results v The function parameters and results act as an interface between a function and the rest of the program MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 3
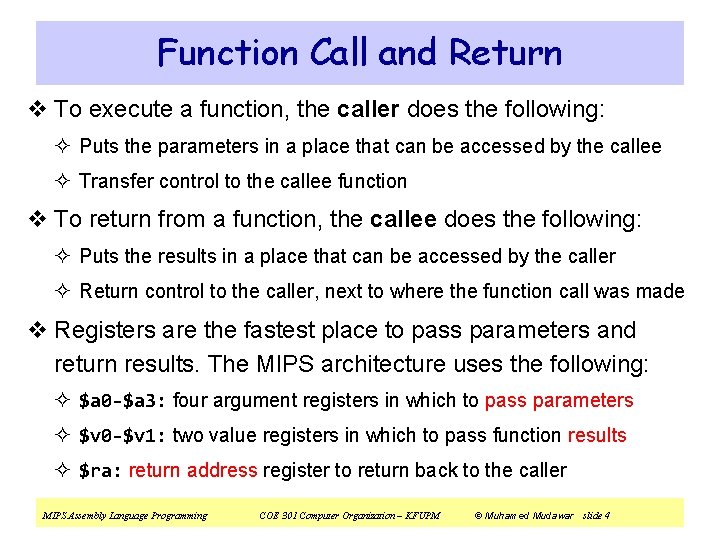
Function Call and Return v To execute a function, the caller does the following: ² Puts the parameters in a place that can be accessed by the callee ² Transfer control to the callee function v To return from a function, the callee does the following: ² Puts the results in a place that can be accessed by the caller ² Return control to the caller, next to where the function call was made v Registers are the fastest place to pass parameters and return results. The MIPS architecture uses the following: ² $a 0 -$a 3: four argument registers in which to pass parameters ² $v 0 -$v 1: two value registers in which to pass function results ² $ra: return address register to return back to the caller MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 4
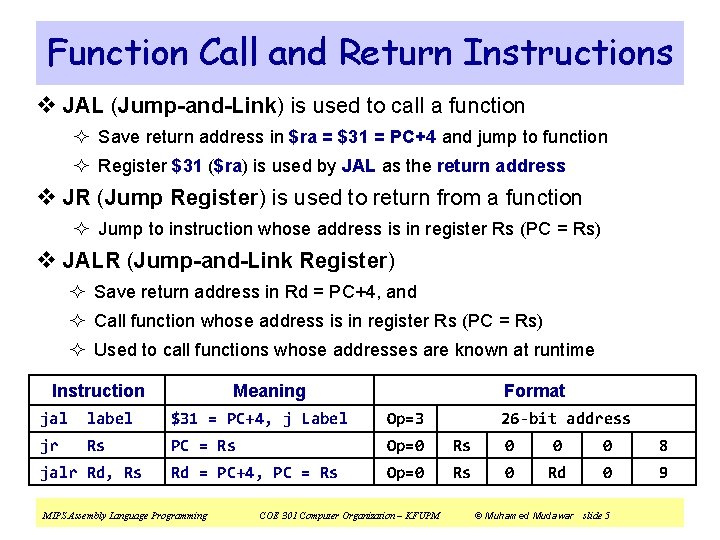
Function Call and Return Instructions v JAL (Jump-and-Link) is used to call a function ² Save return address in $ra = $31 = PC+4 and jump to function ² Register $31 ($ra) is used by JAL as the return address v JR (Jump Register) is used to return from a function ² Jump to instruction whose address is in register Rs (PC = Rs) v JALR (Jump-and-Link Register) ² Save return address in Rd = PC+4, and ² Call function whose address is in register Rs (PC = Rs) ² Used to call functions whose addresses are known at runtime Instruction Meaning Format jal label $31 = PC+4, j Label Op=3 jr Rs PC = Rs Op=0 Rs 0 0 0 8 Rd = PC+4, PC = Rs Op=0 Rs 0 Rd 0 9 jalr Rd, Rs MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM 26 -bit address © Muhamed Mudawar slide 5
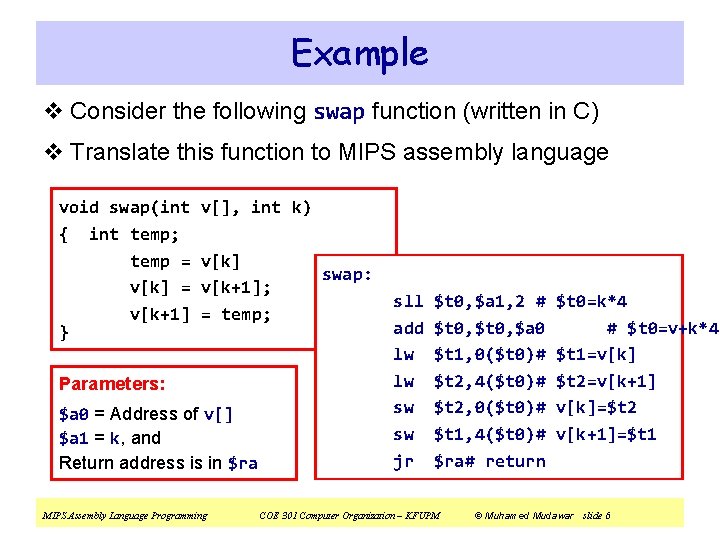
Example v Consider the following swap function (written in C) v Translate this function to MIPS assembly language void swap(int { int temp; temp = v[k] = v[k+1] } v[], int k) v[k] v[k+1]; = temp; Parameters: $a 0 = Address of v[] $a 1 = k, and Return address is in $ra MIPS Assembly Language Programming swap: sll add lw lw sw sw jr $t 0, $a 1, 2 # $t 0, $a 0 $t 1, 0($t 0)# $t 2, 4($t 0)# $t 2, 0($t 0)# $t 1, 4($t 0)# $ra# return COE 301 Computer Organization – KFUPM $t 0=k*4 # $t 0=v+k*4 $t 1=v[k] $t 2=v[k+1] v[k]=$t 2 v[k+1]=$t 1 © Muhamed Mudawar slide 6
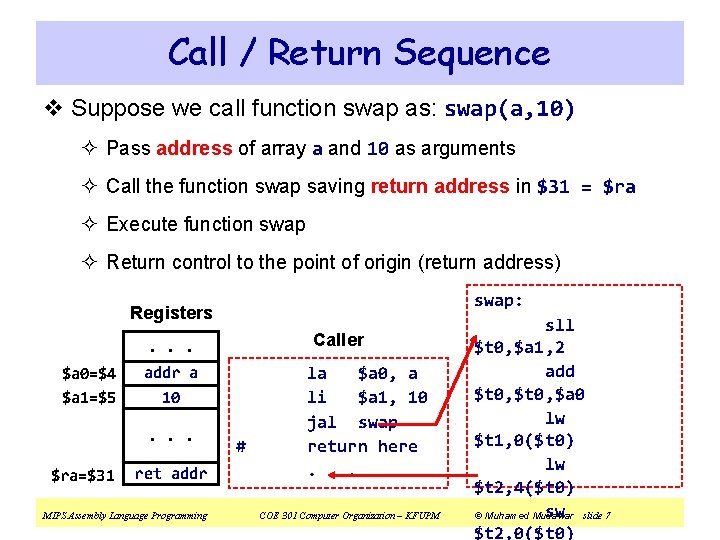
Call / Return Sequence v Suppose we call function swap as: swap(a, 10) ² Pass address of array a and 10 as arguments ² Call the function swap saving return address in $31 = $ra ² Execute function swap ² Return control to the point of origin (return address) swap: Registers Caller . . . $a 0=$4 $a 1=$5 addr a 10. . . $ra=$31 ret addr MIPS Assembly Language Programming # la $a 0, a li $a 1, 10 jal swap return here. . . COE 301 Computer Organization – KFUPM sll $t 0, $a 1, 2 add $t 0, $a 0 lw $t 1, 0($t 0) lw $t 2, 4($t 0) sw slide 7 © Muhamed Mudawar $t 2, 0($t 0)
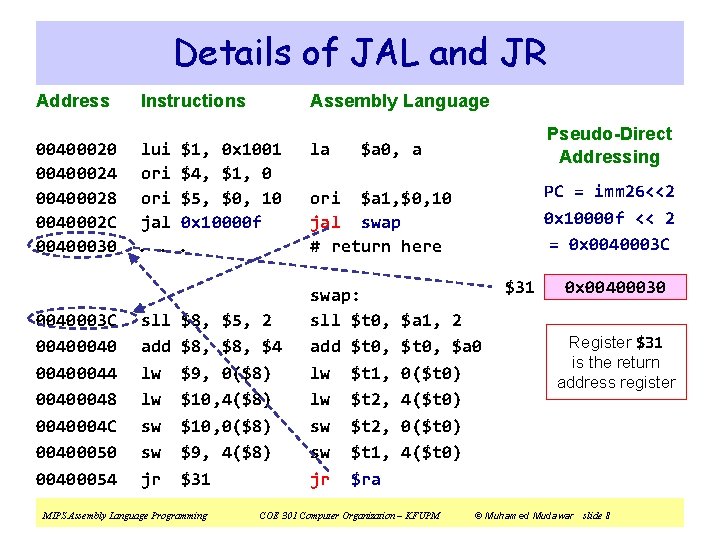
Details of JAL and JR Address Instructions Assembly Language 00400020 00400024 00400028 0040002 C 00400030 lui ori jal. . la 0040003 C 00400044 00400048 0040004 C 00400050 00400054 sll add lw lw sw sw jr $1, 0 x 1001 $4, $1, 0 $5, $0, 10 0 x 10000 f. $8, $5, 2 $8, $4 $9, 0($8) $10, 4($8) $10, 0($8) $9, 4($8) $31 MIPS Assembly Language Programming Pseudo-Direct Addressing $a 0, a PC = imm 26<<2 0 x 10000 f << 2 = 0 x 0040003 C ori $a 1, $0, 10 jal swap # return here swap: sll $t 0, add $t 0, lw $t 1, lw $t 2, sw $t 1, jr $ra $31 $a 1, 2 $t 0, $a 0 0($t 0) 4($t 0) COE 301 Computer Organization – KFUPM 0 x 00400030 Register $31 is the return address register © Muhamed Mudawar slide 8
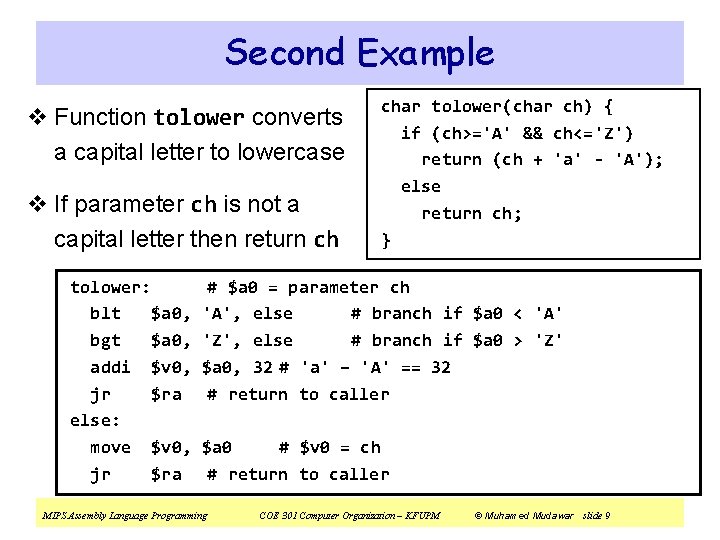
Second Example v Function tolower converts a capital letter to lowercase v If parameter ch is not a capital letter then return ch tolower: blt $a 0, bgt $a 0, addi $v 0, jr $ra else: move $v 0, jr $ra char tolower(char ch) { if (ch>='A' && ch<='Z') return (ch + 'a' - 'A'); else return ch; } # $a 0 = parameter ch 'A', else # branch if $a 0 < 'A' 'Z', else # branch if $a 0 > 'Z' $a 0, 32 # 'a' – 'A' == 32 # return to caller $a 0 # $v 0 = ch # return to caller MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 9
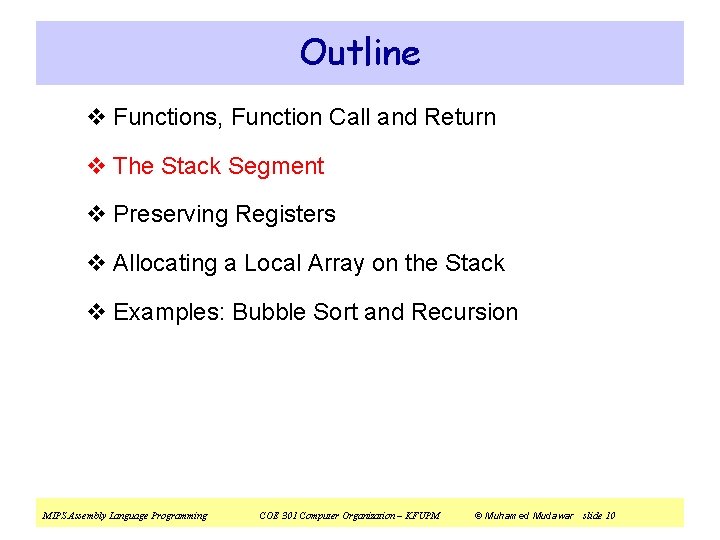
Outline v Functions, Function Call and Return v The Stack Segment v Preserving Registers v Allocating a Local Array on the Stack v Examples: Bubble Sort and Recursion MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 10
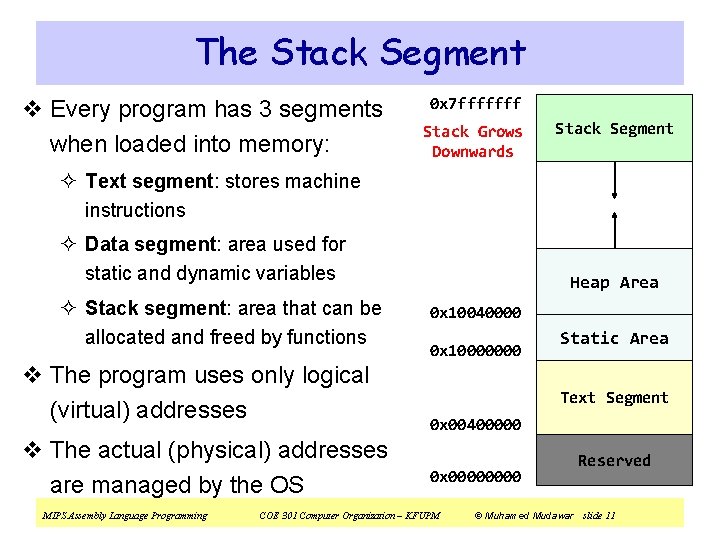
The Stack Segment v Every program has 3 segments when loaded into memory: 0 x 7 fffffff Stack Grows Downwards Stack Segment ² Text segment: stores machine instructions ² Data segment: area used for static and dynamic variables ² Stack segment: area that can be allocated and freed by functions v The program uses only logical (virtual) addresses v The actual (physical) addresses are managed by the OS MIPS Assembly Language Programming Heap Area 0 x 10040000 0 x 10000000 Static Area Text Segment 0 x 00400000 0 x 0000 COE 301 Computer Organization – KFUPM Reserved © Muhamed Mudawar slide 11
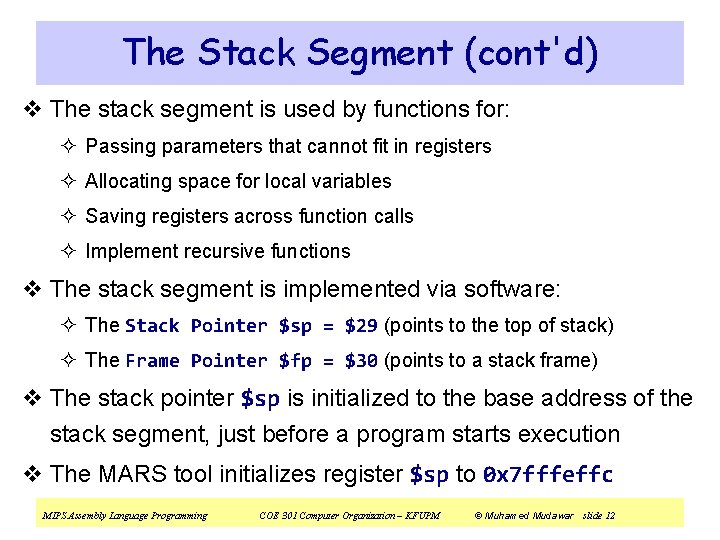
The Stack Segment (cont'd) v The stack segment is used by functions for: ² Passing parameters that cannot fit in registers ² Allocating space for local variables ² Saving registers across function calls ² Implement recursive functions v The stack segment is implemented via software: ² The Stack Pointer $sp = $29 (points to the top of stack) ² The Frame Pointer $fp = $30 (points to a stack frame) v The stack pointer $sp is initialized to the base address of the stack segment, just before a program starts execution v The MARS tool initializes register $sp to 0 x 7 fffeffc MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 12
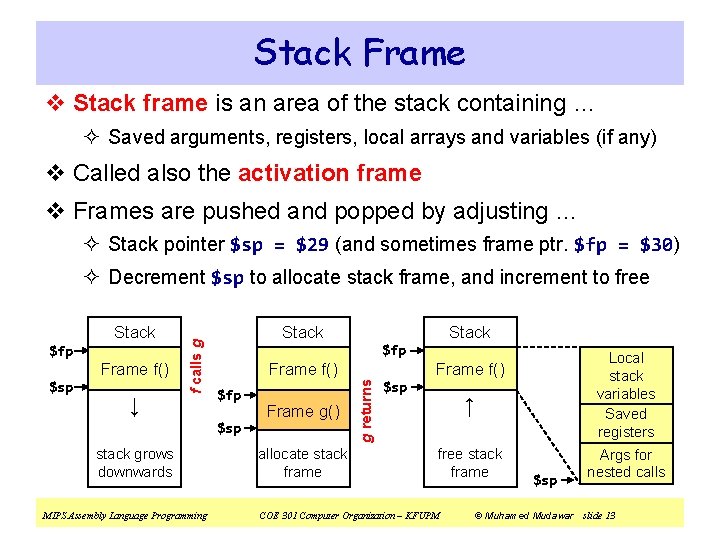
Stack Frame v Stack frame is an area of the stack containing … ² Saved arguments, registers, local arrays and variables (if any) v Called also the activation frame v Frames are pushed and popped by adjusting … ² Stack pointer $sp = $29 (and sometimes frame ptr. $fp = $30) $fp Frame f() $sp ↓ Stack Frame f() $fp $sp stack grows downwards MIPS Assembly Language Programming Frame g() allocate stack frame Stack $fp g returns Stack f calls g ² Decrement $sp to allocate stack frame, and increment to free $sp Local stack variables Saved registers Frame f() ↑ free stack frame COE 301 Computer Organization – KFUPM $sp Args for nested calls © Muhamed Mudawar slide 13
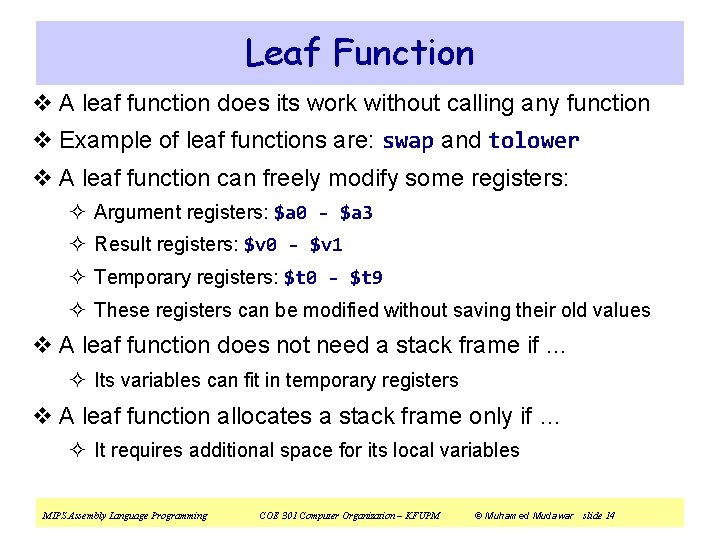
Leaf Function v A leaf function does its work without calling any function v Example of leaf functions are: swap and tolower v A leaf function can freely modify some registers: ² Argument registers: $a 0 - $a 3 ² Result registers: $v 0 - $v 1 ² Temporary registers: $t 0 - $t 9 ² These registers can be modified without saving their old values v A leaf function does not need a stack frame if … ² Its variables can fit in temporary registers v A leaf function allocates a stack frame only if … ² It requires additional space for its local variables MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 14
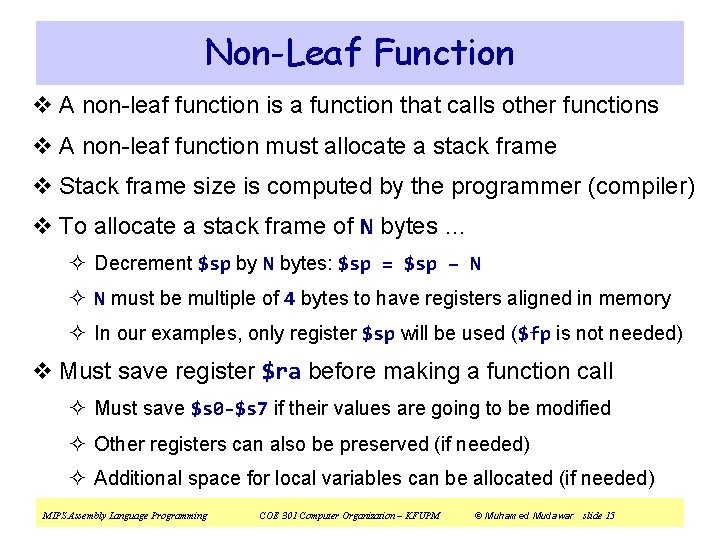
Non-Leaf Function v A non-leaf function is a function that calls other functions v A non-leaf function must allocate a stack frame v Stack frame size is computed by the programmer (compiler) v To allocate a stack frame of N bytes … ² Decrement $sp by N bytes: $sp = $sp – N ² N must be multiple of 4 bytes to have registers aligned in memory ² In our examples, only register $sp will be used ($fp is not needed) v Must save register $ra before making a function call ² Must save $s 0 -$s 7 if their values are going to be modified ² Other registers can also be preserved (if needed) ² Additional space for local variables can be allocated (if needed) MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 15
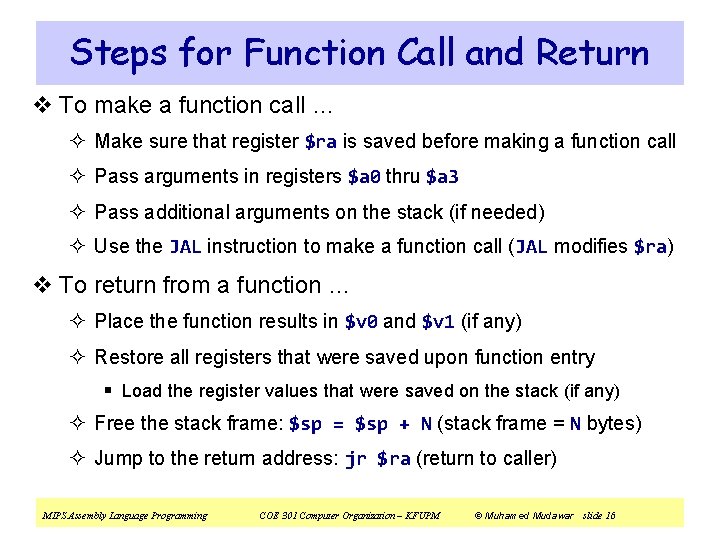
Steps for Function Call and Return v To make a function call … ² Make sure that register $ra is saved before making a function call ² Pass arguments in registers $a 0 thru $a 3 ² Pass additional arguments on the stack (if needed) ² Use the JAL instruction to make a function call (JAL modifies $ra) v To return from a function … ² Place the function results in $v 0 and $v 1 (if any) ² Restore all registers that were saved upon function entry § Load the register values that were saved on the stack (if any) ² Free the stack frame: $sp = $sp + N (stack frame = N bytes) ² Jump to the return address: jr $ra (return to caller) MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 16
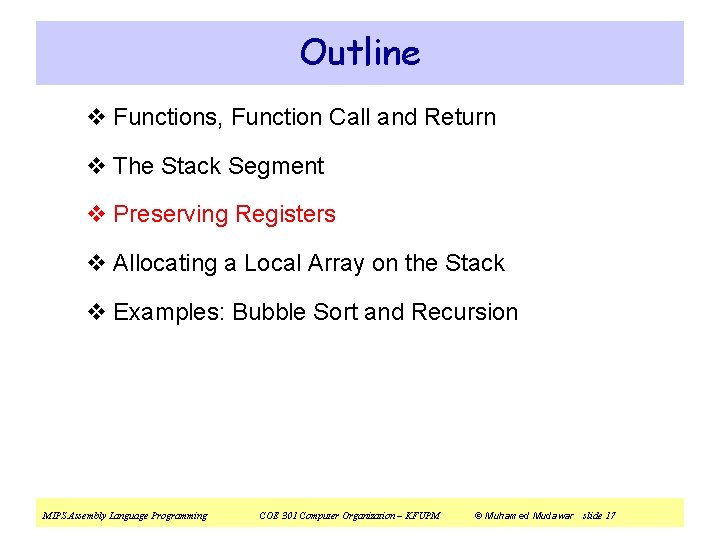
Outline v Functions, Function Call and Return v The Stack Segment v Preserving Registers v Allocating a Local Array on the Stack v Examples: Bubble Sort and Recursion MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 17
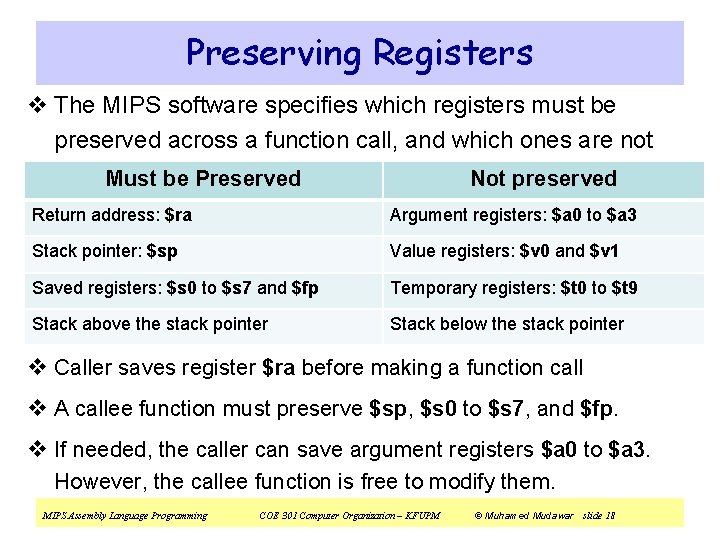
Preserving Registers v The MIPS software specifies which registers must be preserved across a function call, and which ones are not Must be Preserved Not preserved Return address: $ra Argument registers: $a 0 to $a 3 Stack pointer: $sp Value registers: $v 0 and $v 1 Saved registers: $s 0 to $s 7 and $fp Temporary registers: $t 0 to $t 9 Stack above the stack pointer Stack below the stack pointer v Caller saves register $ra before making a function call v A callee function must preserve $sp, $s 0 to $s 7, and $fp. v If needed, the caller can save argument registers $a 0 to $a 3. However, the callee function is free to modify them. MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 18
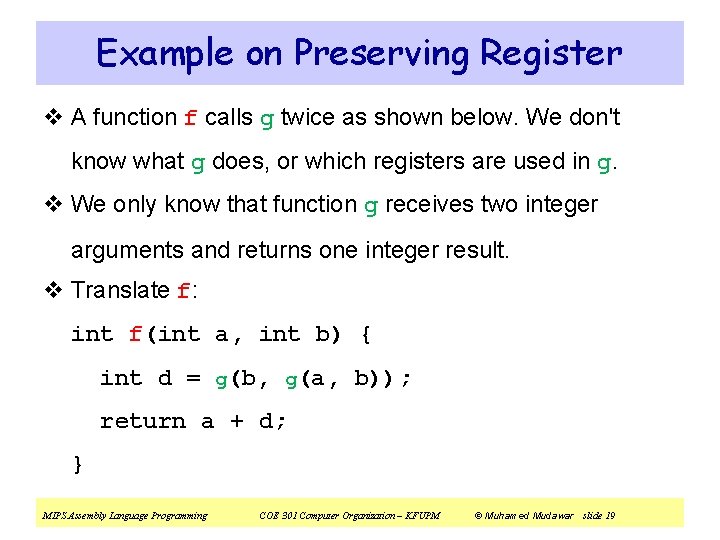
Example on Preserving Register v A function f calls g twice as shown below. We don't know what g does, or which registers are used in g. v We only know that function g receives two integer arguments and returns one integer result. v Translate f: int f(int a, int b) { int d = g(b, g(a, b)); return a + d; } MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 19
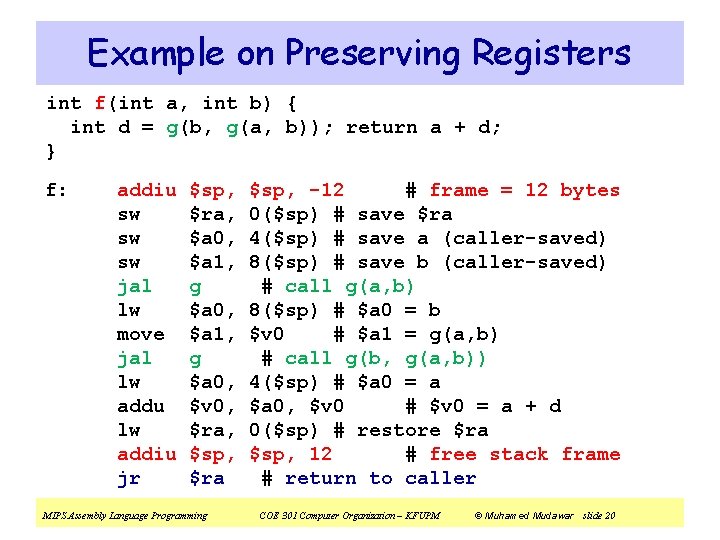
Example on Preserving Registers int f(int a, int b) { int d = g(b, g(a, b)); return a + d; } f: addiu sw sw sw jal lw move jal lw addu lw addiu jr $sp, $ra, $a 0, $a 1, g $a 0, $v 0, $ra, $sp, $ra MIPS Assembly Language Programming $sp, -12 # frame = 12 bytes 0($sp) # save $ra 4($sp) # save a (caller-saved) 8($sp) # save b (caller-saved) # call g(a, b) 8($sp) # $a 0 = b $v 0 # $a 1 = g(a, b) # call g(b, g(a, b)) 4($sp) # $a 0 = a $a 0, $v 0 # $v 0 = a + d 0($sp) # restore $ra $sp, 12 # free stack frame # return to caller COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 20
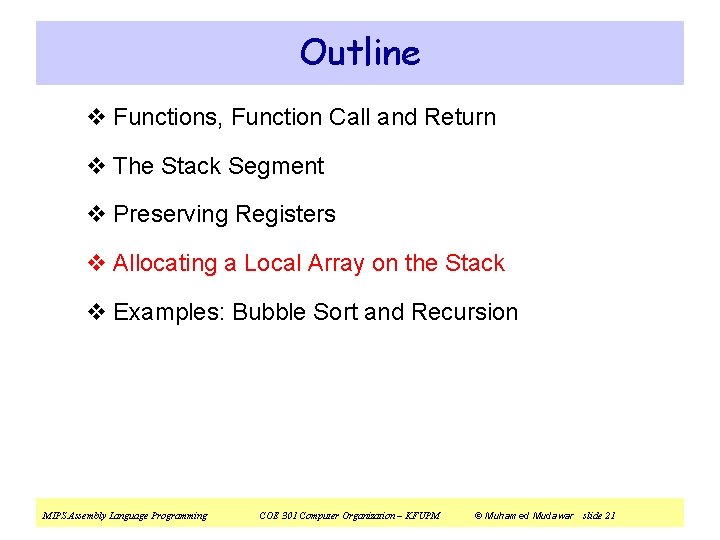
Outline v Functions, Function Call and Return v The Stack Segment v Preserving Registers v Allocating a Local Array on the Stack v Examples: Bubble Sort and Recursion MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 21
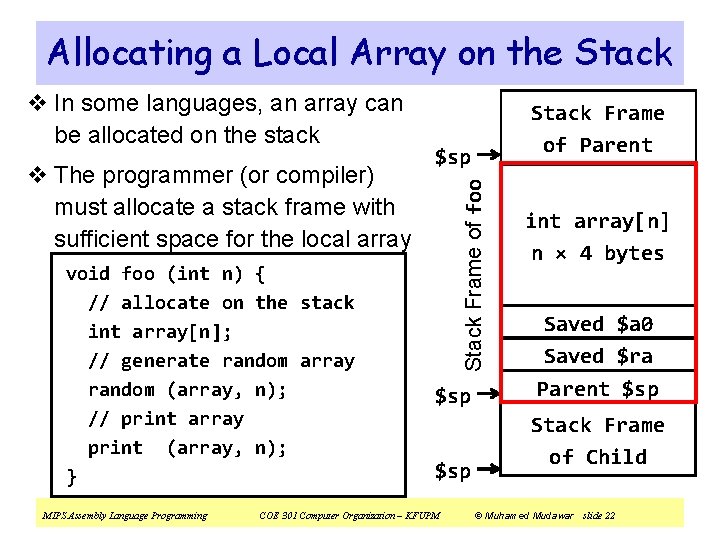
Allocating a Local Array on the Stack v The programmer (or compiler) must allocate a stack frame with sufficient space for the local array void foo (int n) { // allocate on the stack int array[n]; // generate random array random (array, n); // print array print (array, n); } MIPS Assembly Language Programming Stack Frame of Parent $sp Stack Frame of foo v In some languages, an array can be allocated on the stack $sp COE 301 Computer Organization – KFUPM int array[n] n × 4 bytes Saved $a 0 Saved $ra Parent $sp Stack Frame of Child © Muhamed Mudawar slide 22
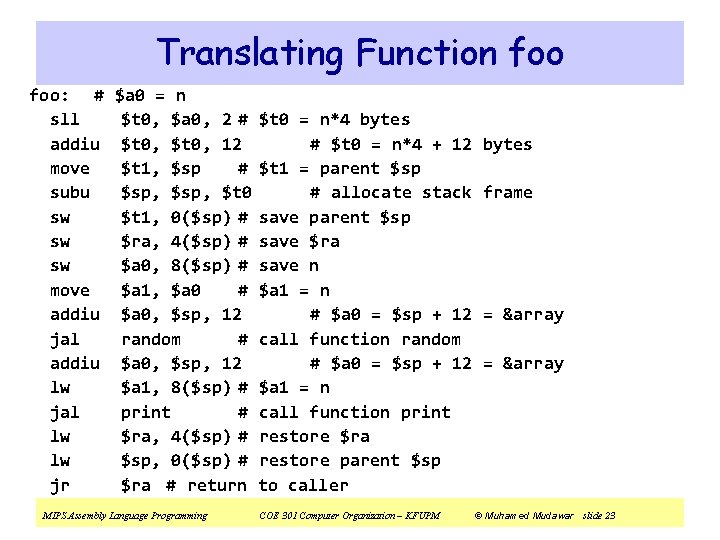
Translating Function foo: # sll addiu move subu sw sw sw move addiu jal addiu lw jal lw lw jr $a 0 = n $t 0, $a 0, 2 # $t 0 = n*4 bytes $t 0, 12 # $t 0 = n*4 + 12 $t 1, $sp # $t 1 = parent $sp, $t 0 # allocate stack $t 1, 0($sp) # save parent $sp $ra, 4($sp) # save $ra $a 0, 8($sp) # save n $a 1, $a 0 # $a 1 = n $a 0, $sp, 12 # $a 0 = $sp + 12 random # call function random $a 0, $sp, 12 # $a 0 = $sp + 12 $a 1, 8($sp) # $a 1 = n print # call function print $ra, 4($sp) # restore $ra $sp, 0($sp) # restore parent $sp $ra # return to caller MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM bytes frame = &array © Muhamed Mudawar slide 23
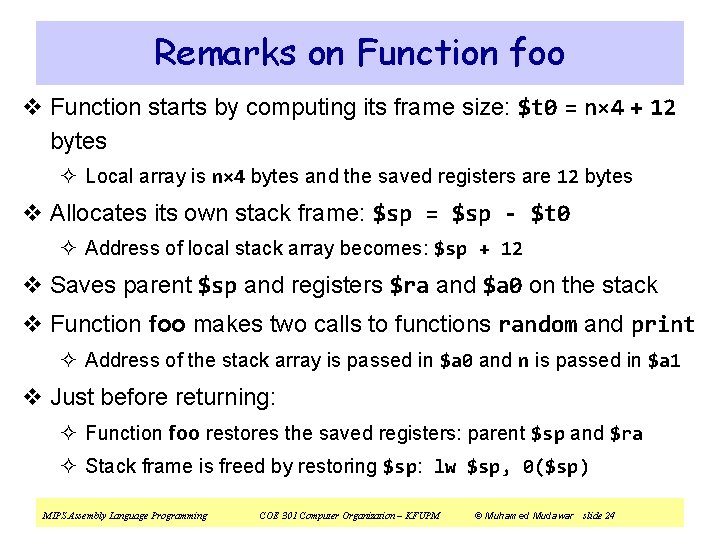
Remarks on Function foo v Function starts by computing its frame size: $t 0 = n× 4 + 12 bytes ² Local array is n× 4 bytes and the saved registers are 12 bytes v Allocates its own stack frame: $sp = $sp - $t 0 ² Address of local stack array becomes: $sp + 12 v Saves parent $sp and registers $ra and $a 0 on the stack v Function foo makes two calls to functions random and print ² Address of the stack array is passed in $a 0 and n is passed in $a 1 v Just before returning: ² Function foo restores the saved registers: parent $sp and $ra ² Stack frame is freed by restoring $sp: lw $sp, 0($sp) MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 24
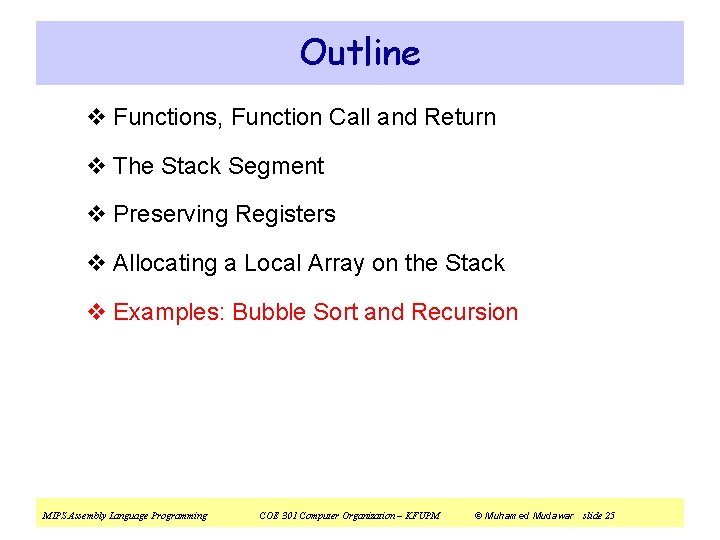
Outline v Functions, Function Call and Return v The Stack Segment v Preserving Registers v Allocating a Local Array on the Stack v Examples: Bubble Sort and Recursion MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 25
![Bubble Sort Leaf Function void bubble Sort int A int n int swapped Bubble Sort (Leaf Function) void bubble. Sort (int A[], int n) { int swapped,](https://slidetodoc.com/presentation_image_h2/5badd13152ce6d6c1d9cdb1c7dfb552f/image-26.jpg)
Bubble Sort (Leaf Function) void bubble. Sort (int A[], int n) { int swapped, i, temp; do { n = n-1; swapped = 0; // false for (i=0; i<n; i++) { if (A[i] > A[i+1]) { temp = A[i]; // swap A[i] = A[i+1]; // with A[i+1] = temp; swapped = 1; // true } Worst case Performance } } while (swapped); Best case Performance O(n) } MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 26 O(n 2)
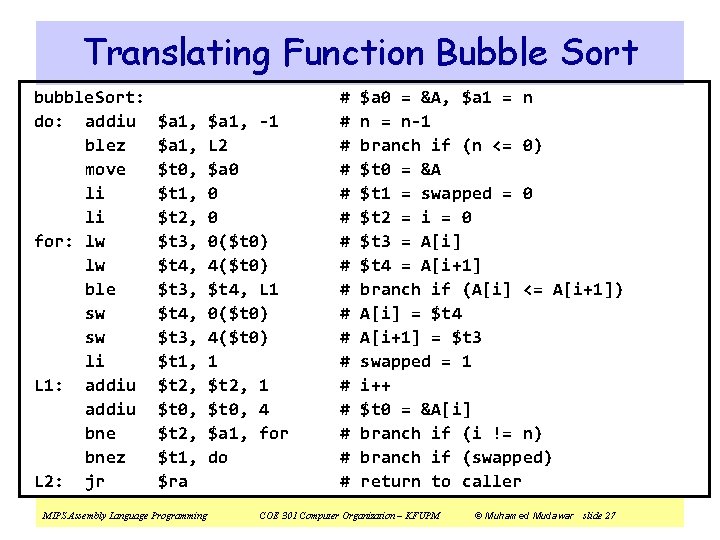
Translating Function Bubble Sort bubble. Sort: do: addiu blez move li li for: lw lw ble sw sw li L 1: addiu bnez L 2: jr $a 1, $t 0, $t 1, $t 2, $t 3, $t 4, $t 3, $t 1, $t 2, $t 0, $t 2, $t 1, $ra MIPS Assembly Language Programming $a 1, -1 L 2 $a 0 0($t 0) 4($t 0) $t 4, L 1 0($t 0) 4($t 0) 1 $t 2, 1 $t 0, 4 $a 1, for do # # # # # $a 0 = &A, $a 1 = n n = n-1 branch if (n <= 0) $t 0 = &A $t 1 = swapped = 0 $t 2 = i = 0 $t 3 = A[i] $t 4 = A[i+1] branch if (A[i] <= A[i+1]) A[i] = $t 4 A[i+1] = $t 3 swapped = 1 i++ $t 0 = &A[i] branch if (i != n) branch if (swapped) return to caller COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 27
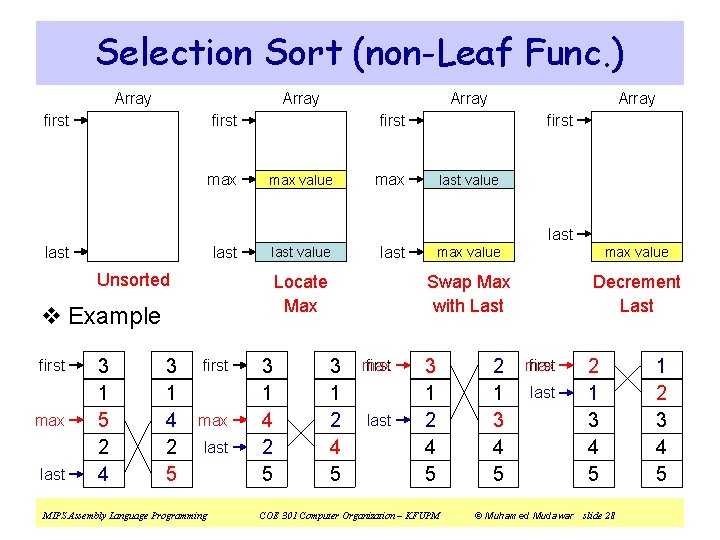
Selection Sort (non-Leaf Func. ) Array first max Array first max value first max last value last value Unsorted Locate Max v Example first max last 3 1 5 2 4 3 1 4 2 5 last first max last MIPS Assembly Language Programming 3 1 4 2 5 3 1 2 4 5 max first last max value Swap Max with Last Decrement Last 3 1 2 4 5 COE 301 Computer Organization – KFUPM 2 1 3 4 5 max first last 2 1 3 4 5 © Muhamed Mudawar slide 28 1 2 3 4 5
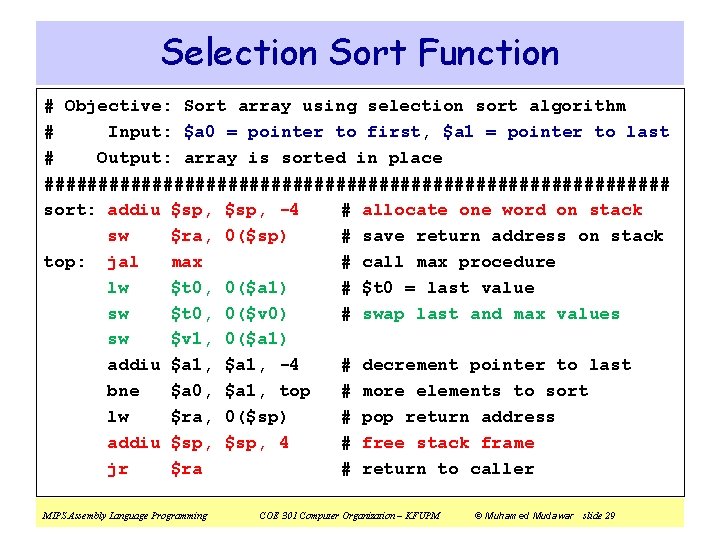
Selection Sort Function # Objective: Sort array using selection sort algorithm # Input: $a 0 = pointer to first, $a 1 = pointer to last # Output: array is sorted in place ############################# sort: addiu $sp, -4 # allocate one word on stack sw $ra, 0($sp) # save return address on stack top: jal max # call max procedure lw $t 0, 0($a 1) # $t 0 = last value sw $t 0, 0($v 0) # swap last and max values sw $v 1, 0($a 1) addiu $a 1, -4 # decrement pointer to last bne $a 0, $a 1, top # more elements to sort lw $ra, 0($sp) # pop return address addiu $sp, 4 # free stack frame jr $ra # return to caller MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 29
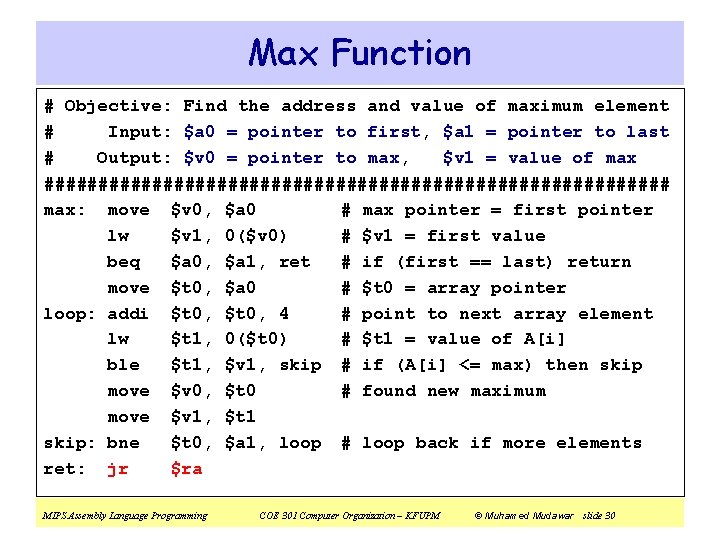
Max Function # Objective: Find the address and value of maximum element # Input: $a 0 = pointer to first, $a 1 = pointer to last # Output: $v 0 = pointer to max, $v 1 = value of max ############################# max: move $v 0, $a 0 # max pointer = first pointer lw $v 1, 0($v 0) # $v 1 = first value beq $a 0, $a 1, ret # if (first == last) return move $t 0, $a 0 # $t 0 = array pointer loop: addi $t 0, 4 # point to next array element lw $t 1, 0($t 0) # $t 1 = value of A[i] ble $t 1, $v 1, skip # if (A[i] <= max) then skip move $v 0, $t 0 # found new maximum move $v 1, $t 1 skip: bne $t 0, $a 1, loop # loop back if more elements ret: jr $ra MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 30
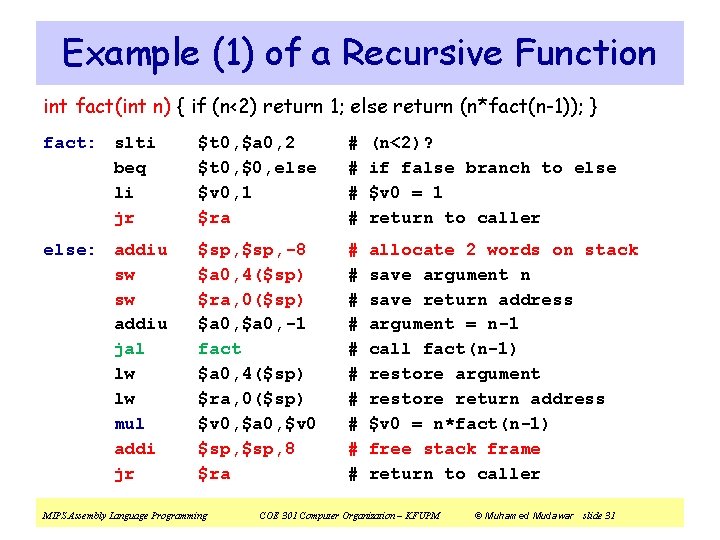
Example (1) of a Recursive Function int fact(int n) { if (n<2) return 1; else return (n*fact(n-1)); } fact: slti beq li jr $t 0, $a 0, 2 $t 0, $0, else $v 0, 1 $ra # # (n<2)? if false branch to else $v 0 = 1 return to caller else: addiu sw sw addiu jal lw lw mul addi jr $sp, -8 $a 0, 4($sp) $ra, 0($sp) $a 0, -1 fact $a 0, 4($sp) $ra, 0($sp) $v 0, $a 0, $v 0 $sp, 8 $ra # # # # # allocate 2 words on stack save argument n save return address argument = n-1 call fact(n-1) restore argument restore return address $v 0 = n*fact(n-1) free stack frame return to caller MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 31
![Example 2 of a Recursive Function int recursivesum int A int n if Example (2) of a Recursive Function int recursive_sum (int A[], int n) { if](https://slidetodoc.com/presentation_image_h2/5badd13152ce6d6c1d9cdb1c7dfb552f/image-32.jpg)
Example (2) of a Recursive Function int recursive_sum (int A[], int n) { if (n == 0) return 0; if (n == 1) return A[0]; int sum 1 = recursive_sum (&A[0], n/2); int sum 2 = recursive_sum (&A[n/2], n – n/2); return sum 1 + sum 2; } v Two recursive calls ² First call computes the sum of the first half of the array elements ² Second call computes the sum of the 2 nd half of the array elements MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 32
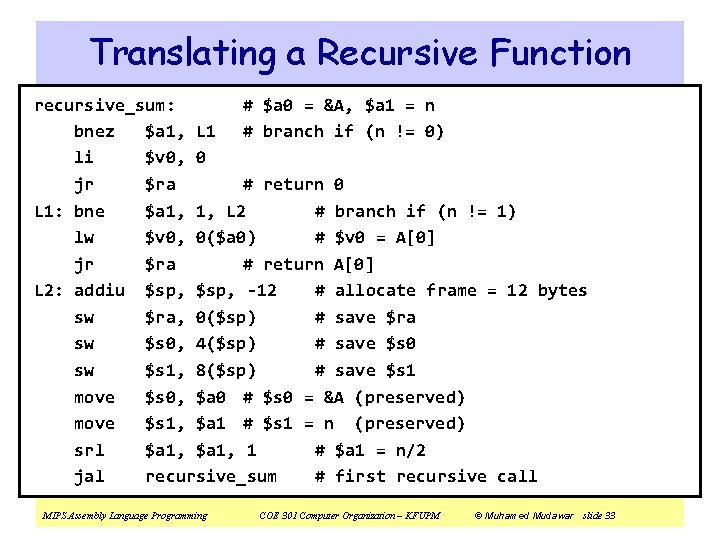
Translating a Recursive Function recursive_sum: # $a 0 = &A, $a 1 = n bnez $a 1, L 1 # branch if (n != 0) li $v 0, 0 jr $ra # return 0 L 1: bne $a 1, 1, L 2 # branch if (n != 1) lw $v 0, 0($a 0) # $v 0 = A[0] jr $ra # return A[0] L 2: addiu $sp, -12 # allocate frame = 12 bytes sw $ra, 0($sp) # save $ra sw $s 0, 4($sp) # save $s 0 sw $s 1, 8($sp) # save $s 1 move $s 0, $a 0 # $s 0 = &A (preserved) move $s 1, $a 1 # $s 1 = n (preserved) srl $a 1, 1 # $a 1 = n/2 jal recursive_sum # first recursive call MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 33
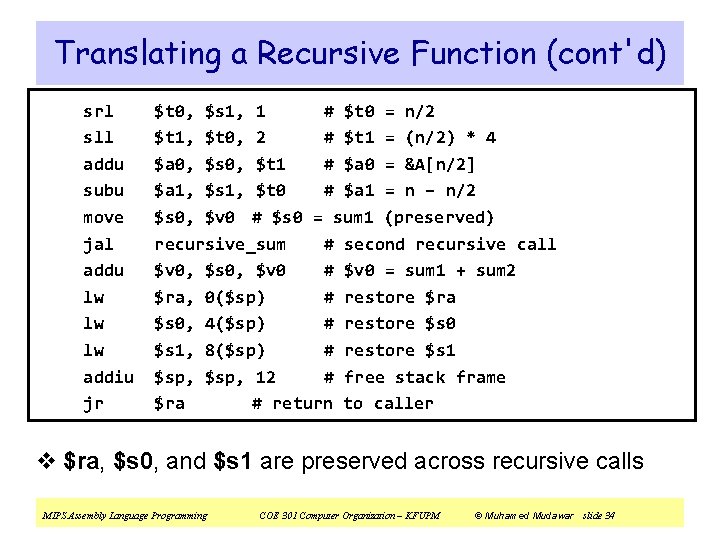
Translating a Recursive Function (cont'd) srl sll addu subu move jal addu lw lw lw addiu jr $t 0, $s 1, 1 # $t 0 = n/2 $t 1, $t 0, 2 # $t 1 = (n/2) * 4 $a 0, $s 0, $t 1 # $a 0 = &A[n/2] $a 1, $s 1, $t 0 # $a 1 = n – n/2 $s 0, $v 0 # $s 0 = sum 1 (preserved) recursive_sum # second recursive call $v 0, $s 0, $v 0 # $v 0 = sum 1 + sum 2 $ra, 0($sp) # restore $ra $s 0, 4($sp) # restore $s 0 $s 1, 8($sp) # restore $s 1 $sp, 12 # free stack frame $ra # return to caller v $ra, $s 0, and $s 1 are preserved across recursive calls MIPS Assembly Language Programming COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 34
![Illustrating Recursive Calls v 0 A0A1A2A3A4A5 recursivesum a 0 A0 a 1 Illustrating Recursive Calls $v 0 = A[0]+A[1]+A[2]+A[3]+A[4]+A[5] recursive_sum: $a 0 = &A[0], $a 1](https://slidetodoc.com/presentation_image_h2/5badd13152ce6d6c1d9cdb1c7dfb552f/image-35.jpg)
Illustrating Recursive Calls $v 0 = A[0]+A[1]+A[2]+A[3]+A[4]+A[5] recursive_sum: $a 0 = &A[0], $a 1 = 6 A[0]+A[1]+A[2] A[3]+A[4]+A[5] recursive_sum: $a 0 = &A[0], $a 1 = 3 A[0] recursive_sum: $a 0 = &A[3], $a 1 = 3 A[3] A[1]+A[2] recursive_sum: $a 0 = &A[0] $a 0 = &A[1] $a 1 = 1 $a 1 = 2 A[1] recursive_sum: $a 0 = &A[3] $a 0 = &A[4] $a 1 = 1 $a 1 = 2 A[4] A[2] recursive_sum: $a 0 = &A[2] $a 0 = &A[1] $a 1 = 1 MIPS Assembly Language Programming A[4]+A[5] recursive_sum: $a 0 = &A[5] $a 0 = &A[4] $a 1 = 1 COE 301 Computer Organization – KFUPM © Muhamed Mudawar slide 35
Coe 301 kfupm
Access memory
Coe 301
Coe 301 kfupm
Mult mips
Mudawar
Conditional move assembly
Stack grows downwards
Stack smashing vs stack overflow
Contoh soal stack dan jawabannya
Compile time polymorphism java
What are constructors in java
Mips functions
Recursive functions in mips
Mips nested functions
List and explain different subdivisions of run-time memory
System programming
Arduino scadabr
Kinect for windows speech recognition language pack
Heapify runtime
Matrix multiplication runtime
Runtime error index out of bounds
Java runtime code generation
Activation tree for quicksort
Ford fulkerson runtime
Prims algorithm runtime
Basic runtime checks
Logical error in python
Microsoft common language runtime native compiler
Heapify runtime
Control flow guard
Kernel mode driver framework runtime
Runtime error 9
Java runtime code generation
Blackberry
Compile time vs run time