Programming Microcontrollers in C Lecture L 7 1
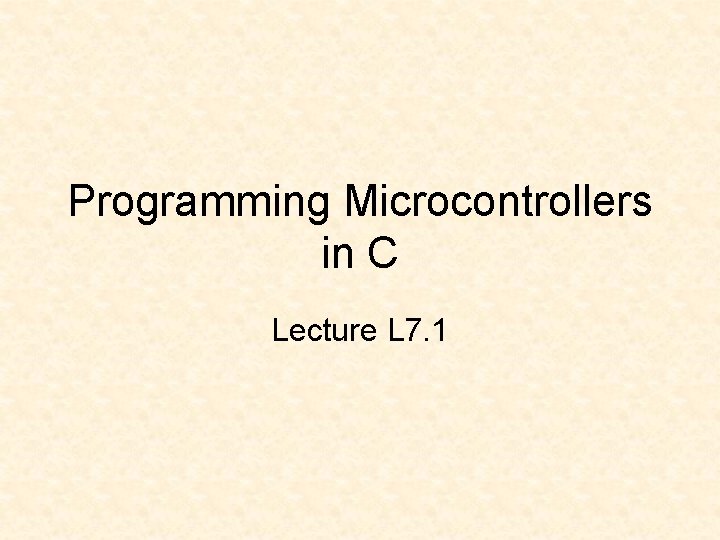
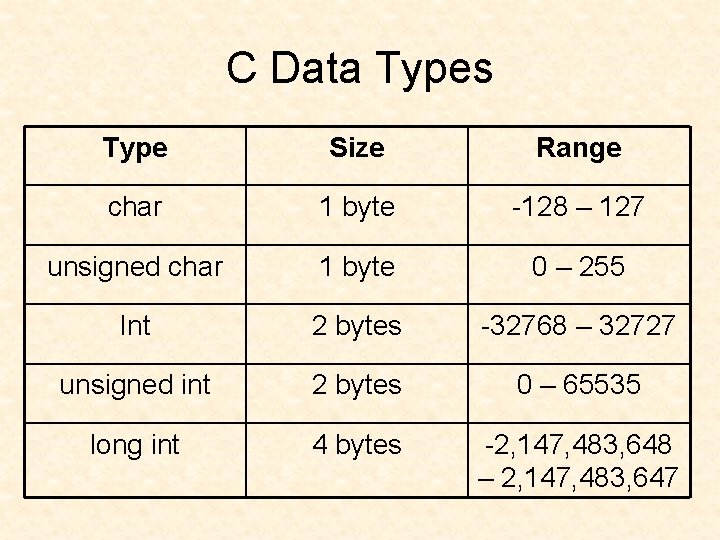
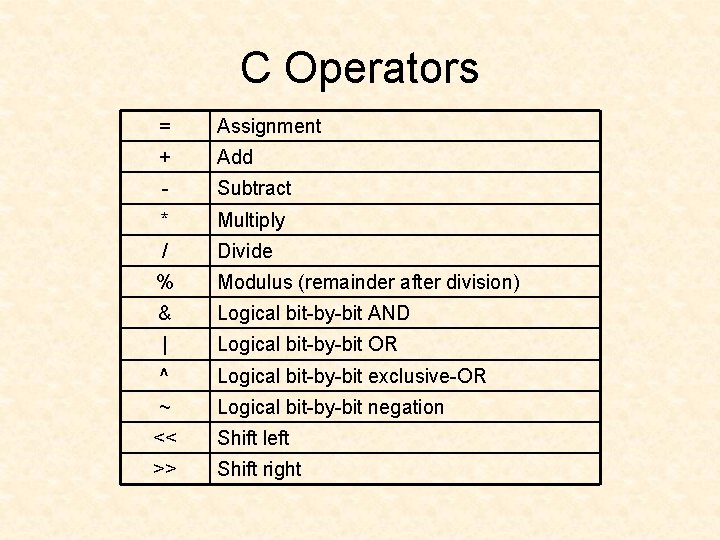
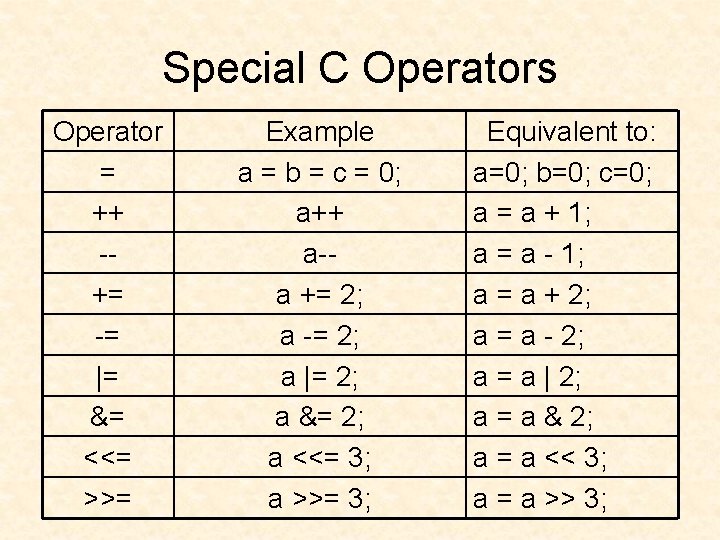
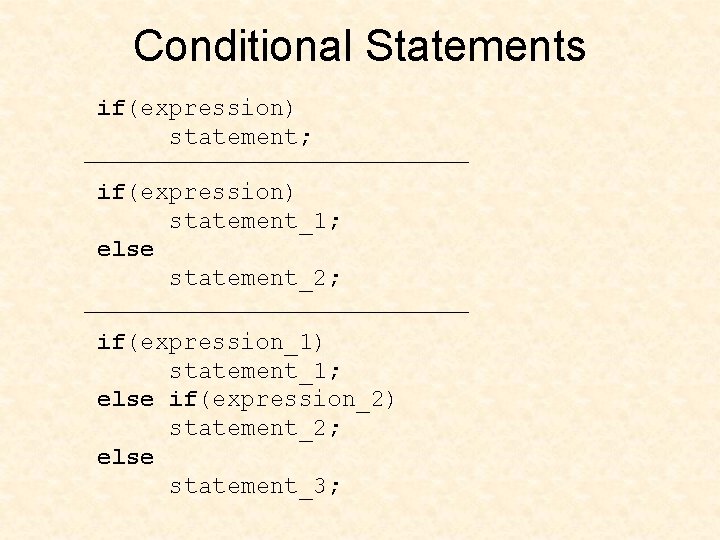
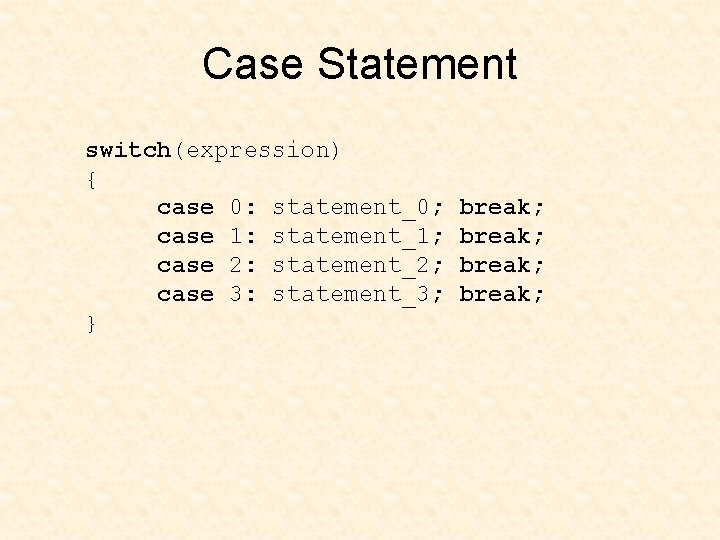
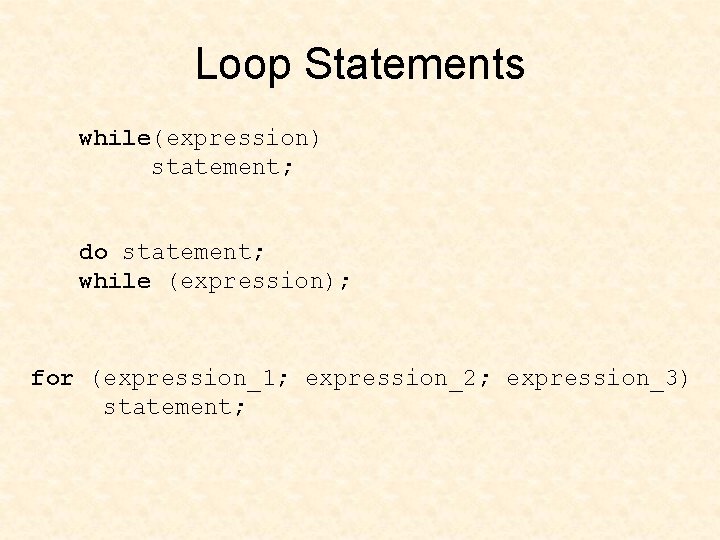
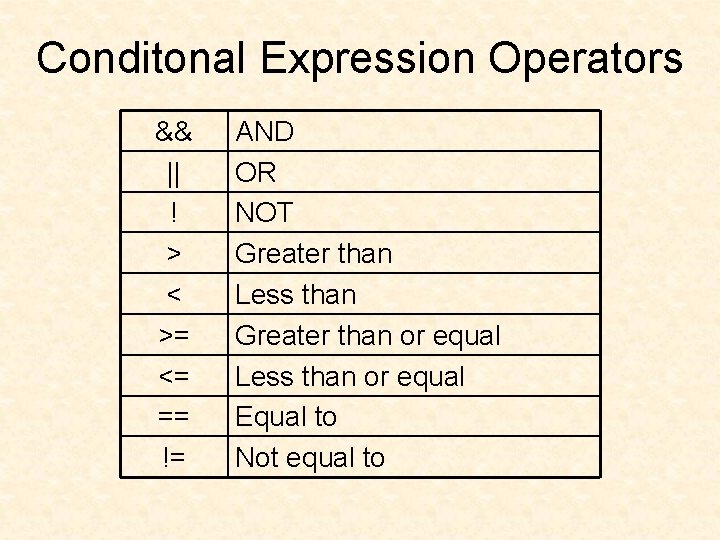
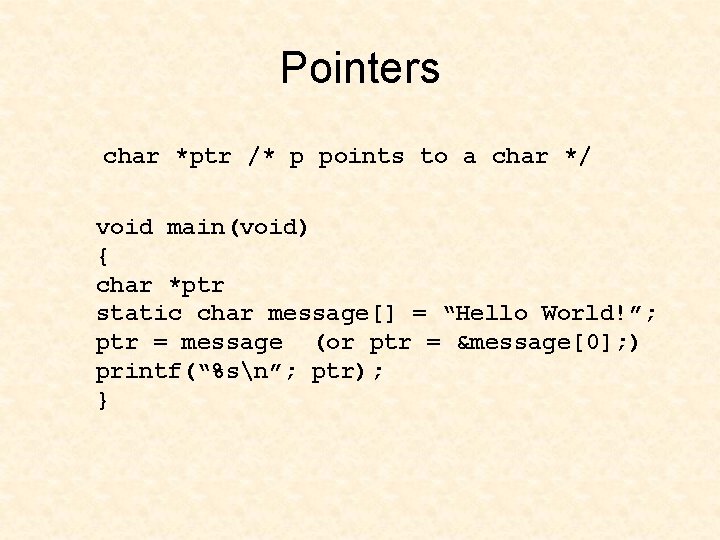
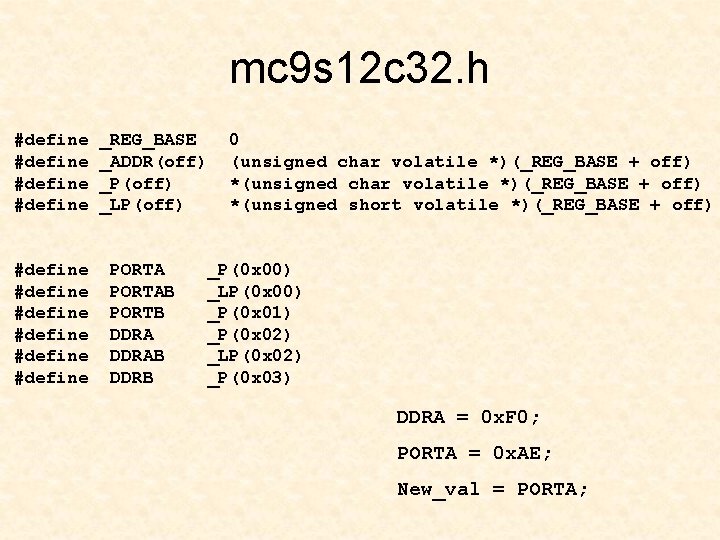
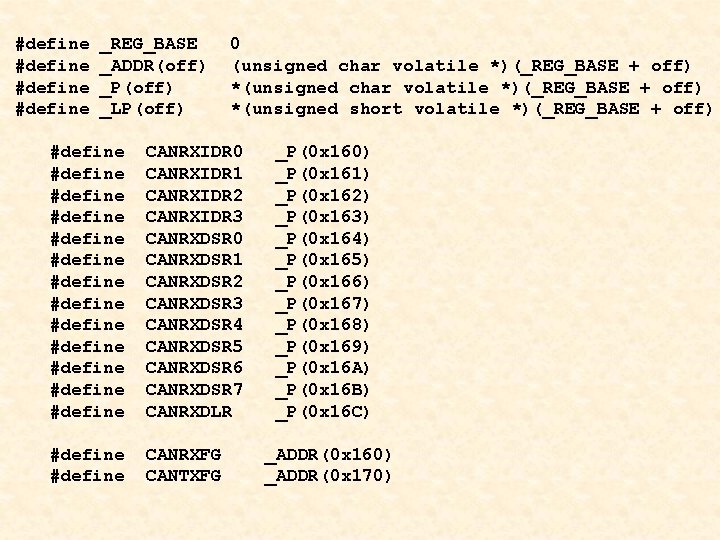
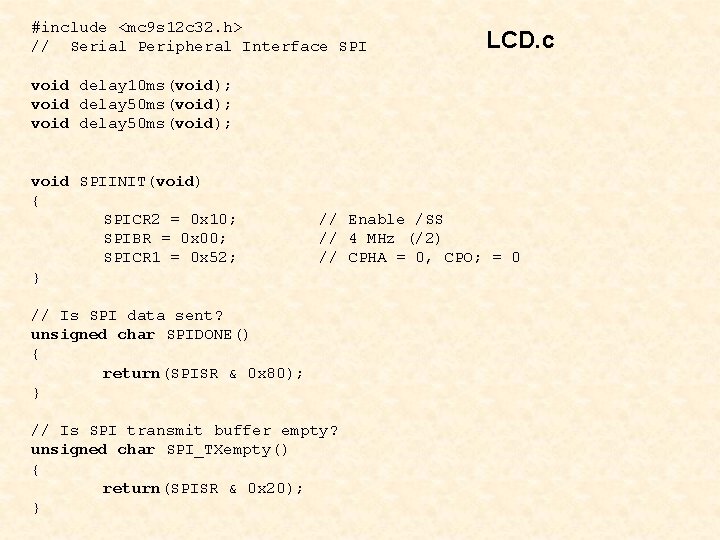
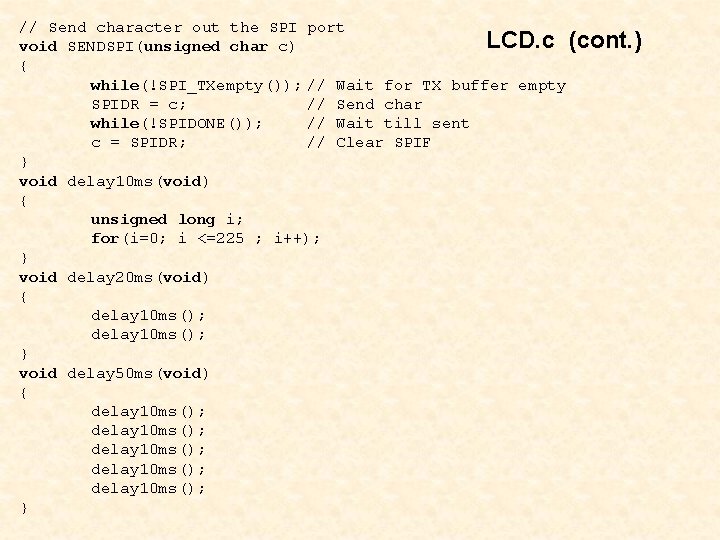
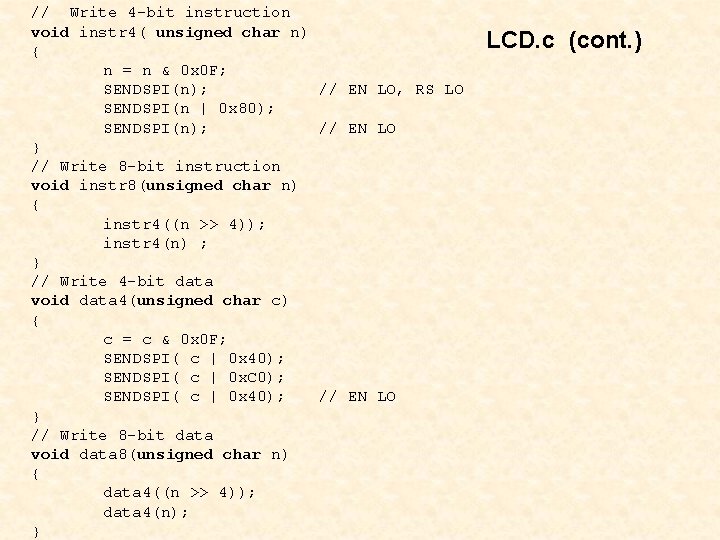
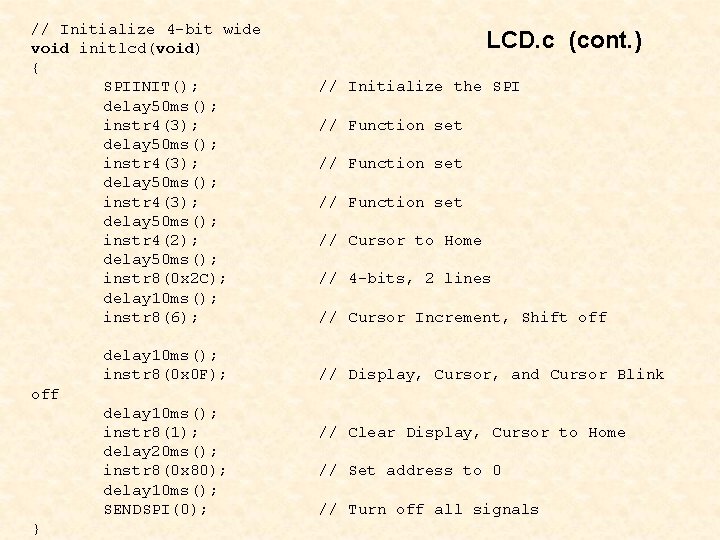
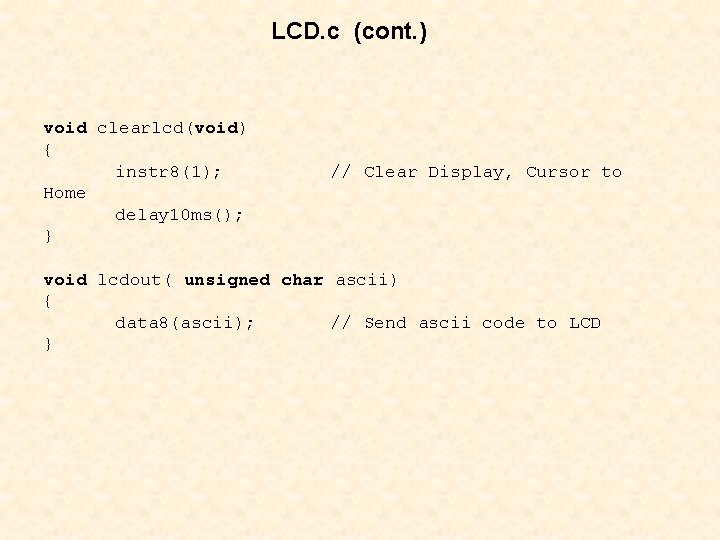
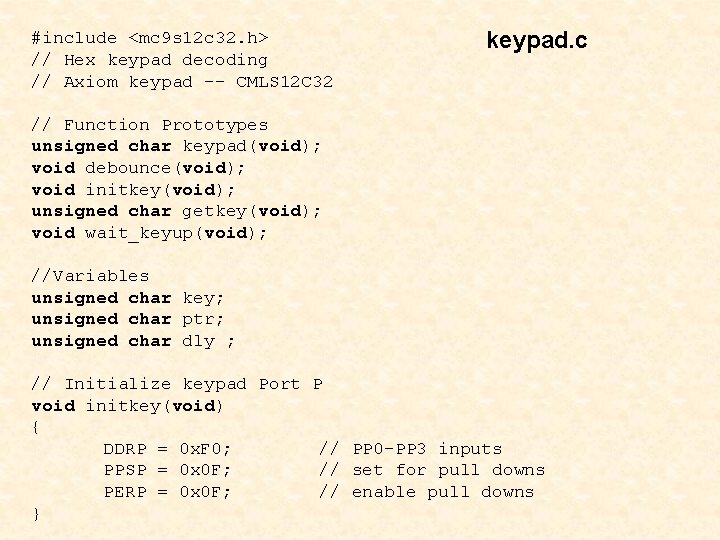
![keypad. c (cont. ) // Keycode table const unsigned char keycodes[16]={ /* key row keypad. c (cont. ) // Keycode table const unsigned char keycodes[16]={ /* key row](https://slidetodoc.com/presentation_image_h2/8a12b68f841f03110162ad7395ab5fcb/image-18.jpg)
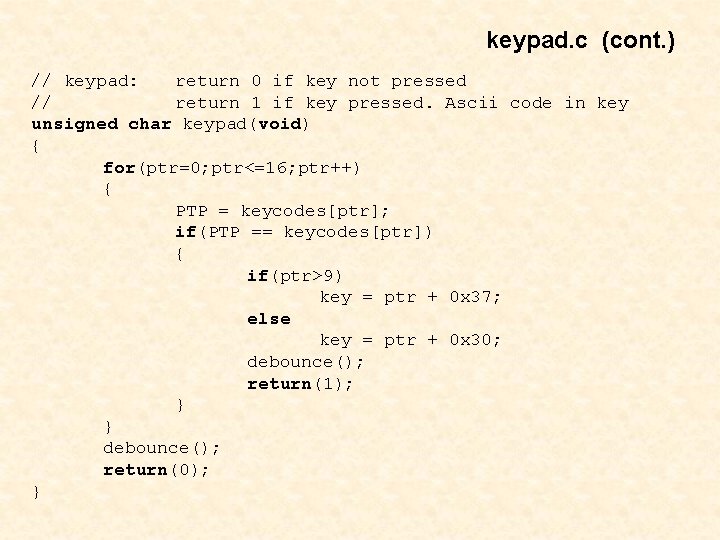
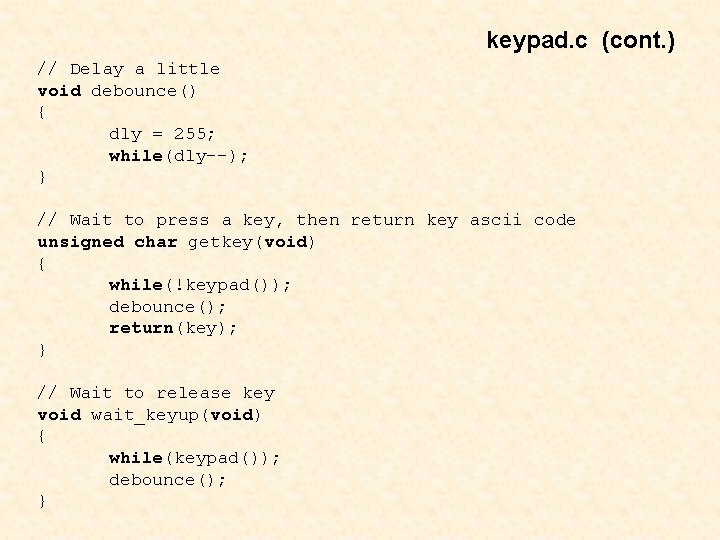
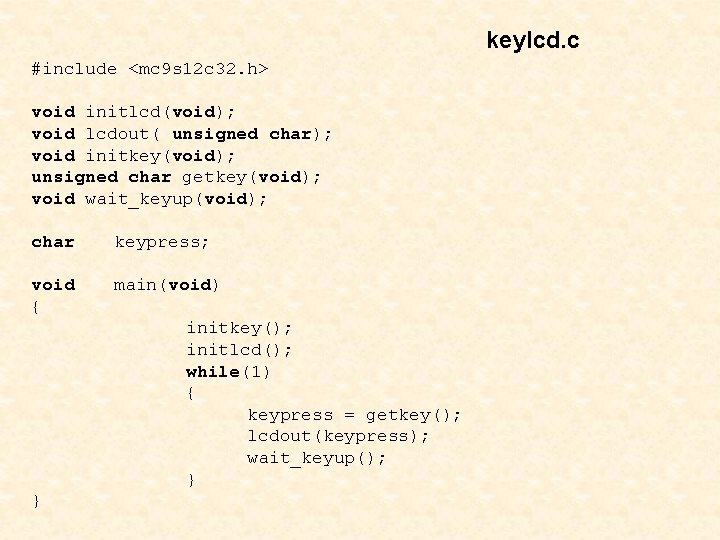
- Slides: 21
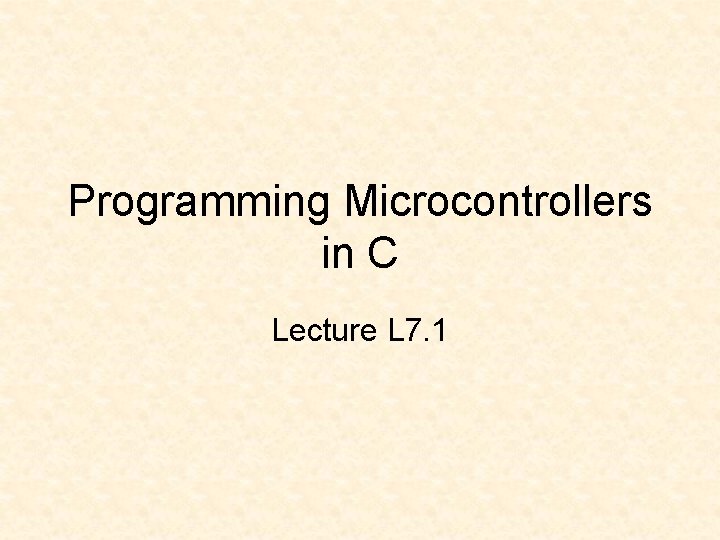
Programming Microcontrollers in C Lecture L 7. 1
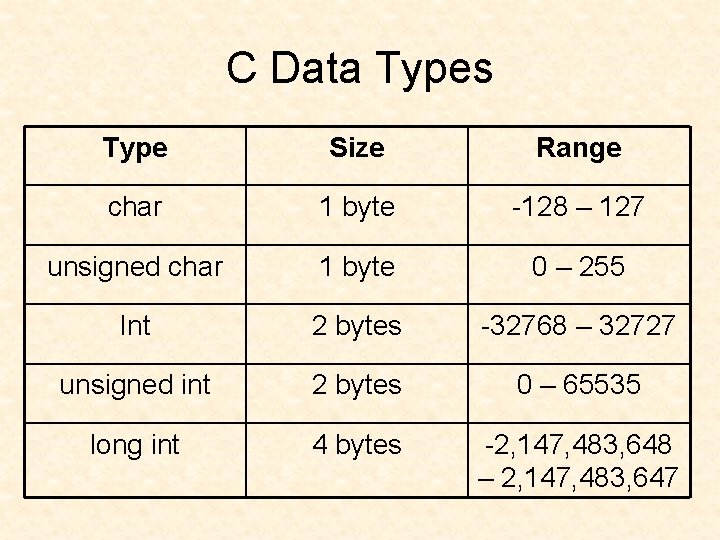
C Data Types Type Size Range char 1 byte -128 – 127 unsigned char 1 byte 0 – 255 Int 2 bytes -32768 – 32727 unsigned int 2 bytes 0 – 65535 long int 4 bytes -2, 147, 483, 648 – 2, 147, 483, 647
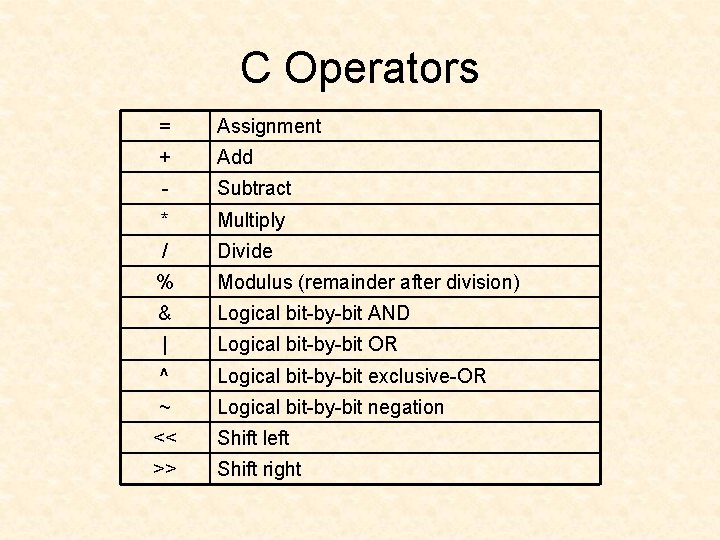
C Operators = Assignment + Add - Subtract * Multiply / Divide % Modulus (remainder after division) & Logical bit-by-bit AND | Logical bit-by-bit OR ^ Logical bit-by-bit exclusive-OR ~ Logical bit-by-bit negation << Shift left >> Shift right
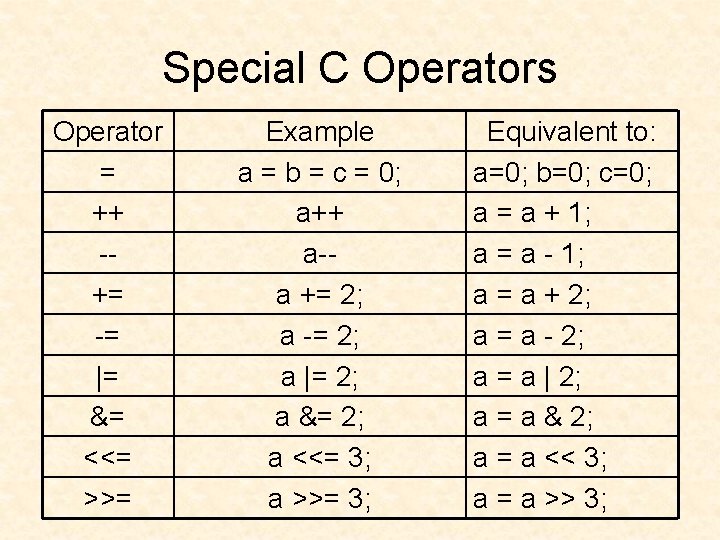
Special C Operators Operator = ++ -+= -= |= &= <<= >>= Example a = b = c = 0; a++ a-a += 2; a -= 2; a |= 2; a &= 2; a <<= 3; a >>= 3; Equivalent to: a=0; b=0; c=0; a = a + 1; a = a - 1; a = a + 2; a = a - 2; a = a | 2; a = a & 2; a = a << 3; a = a >> 3;
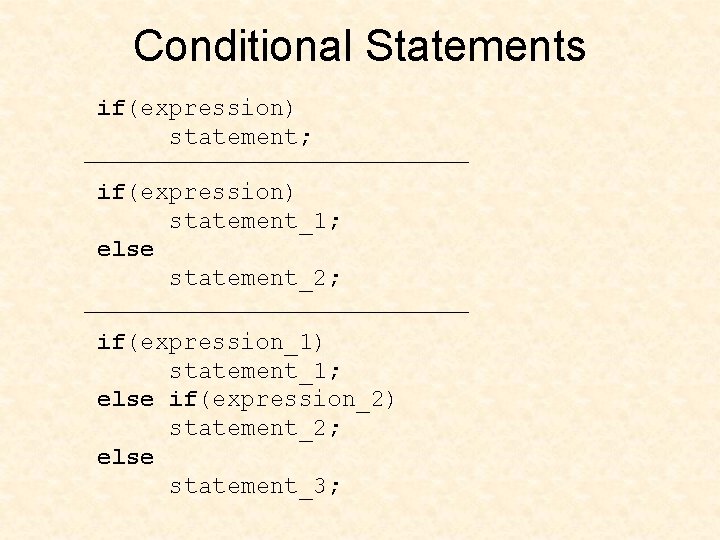
Conditional Statements if(expression) statement; if(expression) statement_1; else statement_2; if(expression_1) statement_1; else if(expression_2) statement_2; else statement_3;
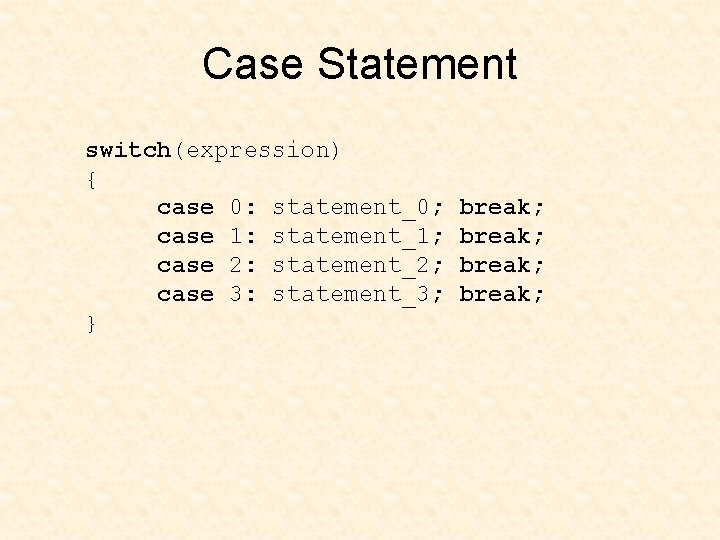
Case Statement switch(expression) { case 0: statement_0; case 1: statement_1; case 2: statement_2; case 3: statement_3; } break;
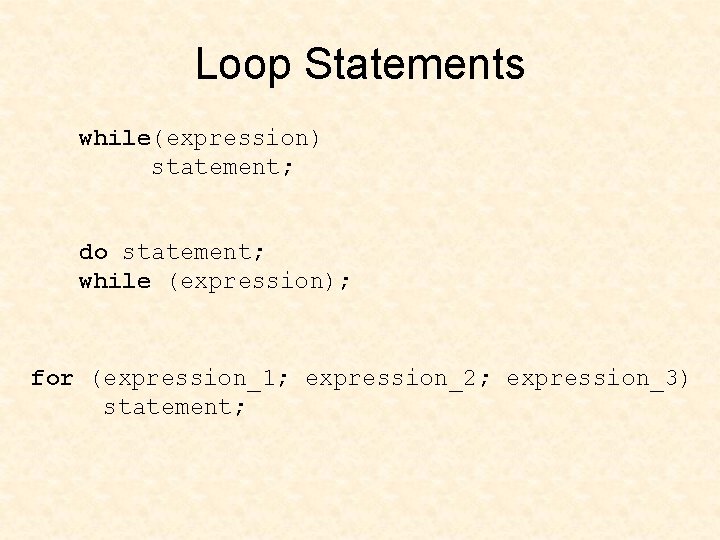
Loop Statements while(expression) statement; do statement; while (expression); for (expression_1; expression_2; expression_3) statement;
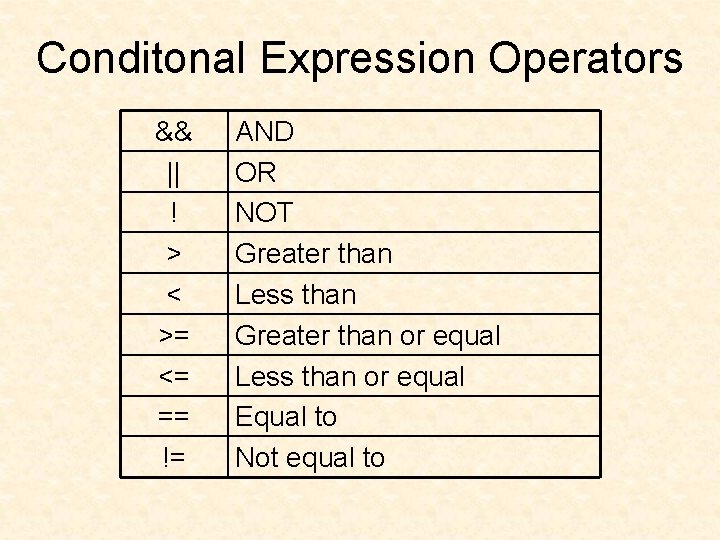
Conditonal Expression Operators && || ! > < >= <= == != AND OR NOT Greater than Less than Greater than or equal Less than or equal Equal to Not equal to
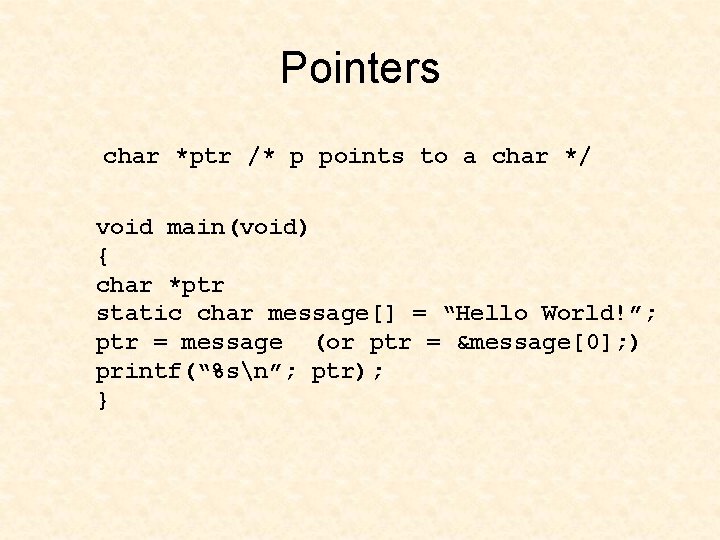
Pointers char *ptr /* p points to a char */ void main(void) { char *ptr static char message[] = “Hello World!”; ptr = message (or ptr = &message[0]; ) printf(“%sn”; ptr); }
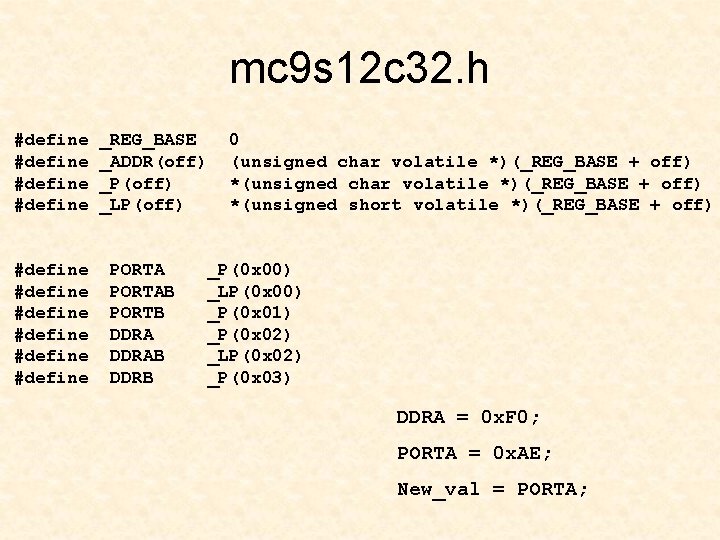
mc 9 s 12 c 32. h #define #define #define _REG_BASE _ADDR(off) _P(off) _LP(off) PORTAB PORTB DDRAB DDRB 0 (unsigned char volatile *)(_REG_BASE + off) *(unsigned short volatile *)(_REG_BASE + off) _P(0 x 00) _LP(0 x 00) _P(0 x 01) _P(0 x 02) _LP(0 x 02) _P(0 x 03) DDRA = 0 x. F 0; PORTA = 0 x. AE; New_val = PORTA;
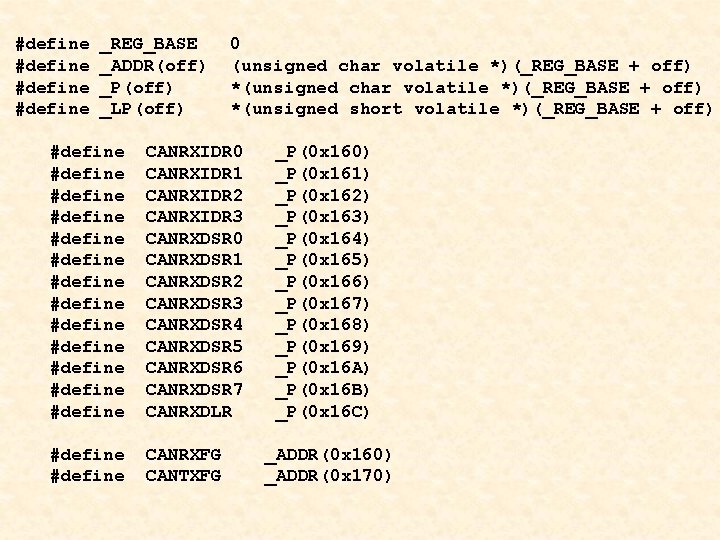
#define _REG_BASE _ADDR(off) _P(off) _LP(off) 0 (unsigned char volatile *)(_REG_BASE + off) *(unsigned short volatile *)(_REG_BASE + off) #define #define #define #define CANRXIDR 0 CANRXIDR 1 CANRXIDR 2 CANRXIDR 3 CANRXDSR 0 CANRXDSR 1 CANRXDSR 2 CANRXDSR 3 CANRXDSR 4 CANRXDSR 5 CANRXDSR 6 CANRXDSR 7 CANRXDLR #define CANRXFG CANTXFG _P(0 x 160) _P(0 x 161) _P(0 x 162) _P(0 x 163) _P(0 x 164) _P(0 x 165) _P(0 x 166) _P(0 x 167) _P(0 x 168) _P(0 x 169) _P(0 x 16 A) _P(0 x 16 B) _P(0 x 16 C) _ADDR(0 x 160) _ADDR(0 x 170)
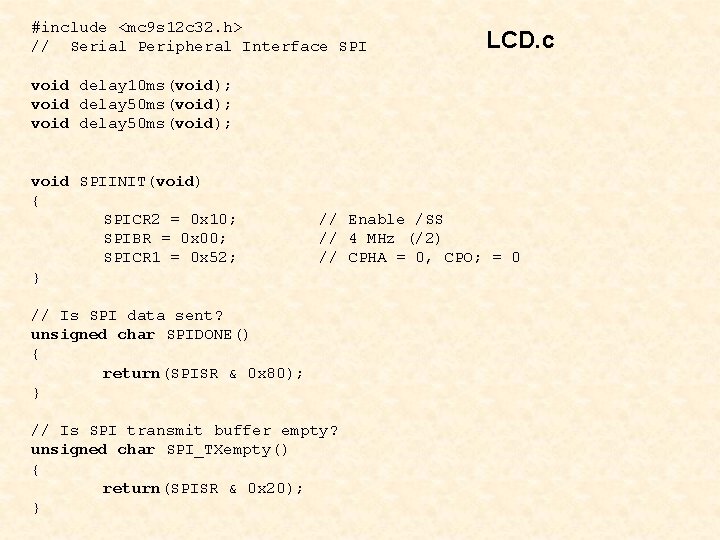
#include <mc 9 s 12 c 32. h> // Serial Peripheral Interface SPI LCD. c void delay 10 ms(void); void delay 50 ms(void); void SPIINIT(void) { SPICR 2 = 0 x 10; SPIBR = 0 x 00; SPICR 1 = 0 x 52; } // Enable /SS // 4 MHz (/2) // CPHA = 0, CPO; = 0 // Is SPI data sent? unsigned char SPIDONE() { return(SPISR & 0 x 80); } // Is SPI transmit buffer empty? unsigned char SPI_TXempty() { return(SPISR & 0 x 20); }
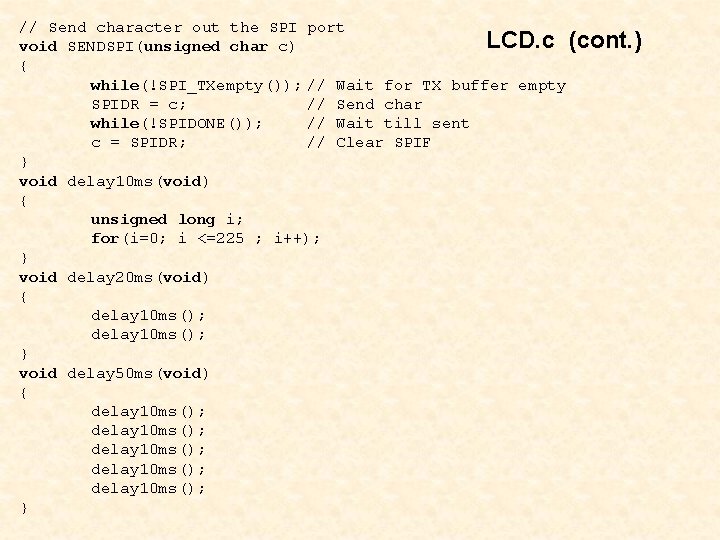
// Send character out the SPI port LCD. c (cont. ) void SENDSPI(unsigned char c) { while(!SPI_TXempty()); // Wait for TX buffer empty SPIDR = c; // Send char while(!SPIDONE()); // Wait till sent c = SPIDR; // Clear SPIF } void delay 10 ms(void) { unsigned long i; for(i=0; i <=225 ; i++); } void delay 20 ms(void) { delay 10 ms(); } void delay 50 ms(void) { delay 10 ms(); delay 10 ms(); }
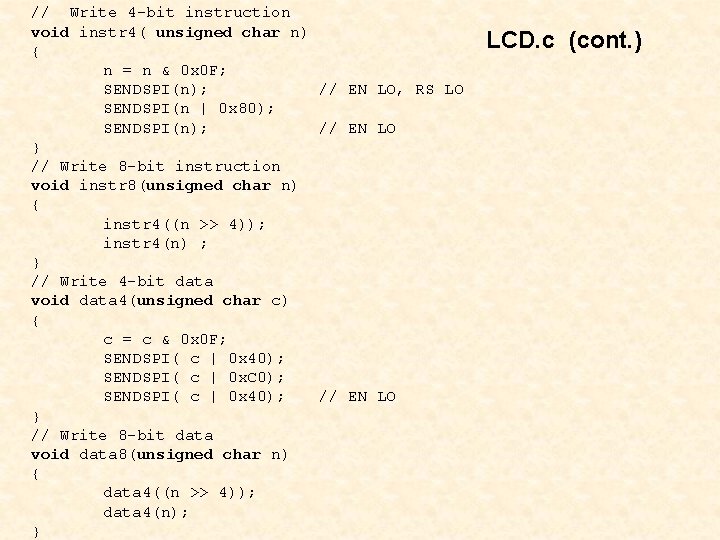
// Write 4 -bit instruction void instr 4( unsigned char n) { n = n & 0 x 0 F; SENDSPI(n); // EN LO, RS LO SENDSPI(n | 0 x 80); SENDSPI(n); // EN LO } // Write 8 -bit instruction void instr 8(unsigned char n) { instr 4((n >> 4)); instr 4(n) ; } // Write 4 -bit data void data 4(unsigned char c) { c = c & 0 x 0 F; SENDSPI( c | 0 x 40); SENDSPI( c | 0 x. C 0); SENDSPI( c | 0 x 40); // EN LO } // Write 8 -bit data void data 8(unsigned char n) { data 4((n >> 4)); data 4(n); } LCD. c (cont. )
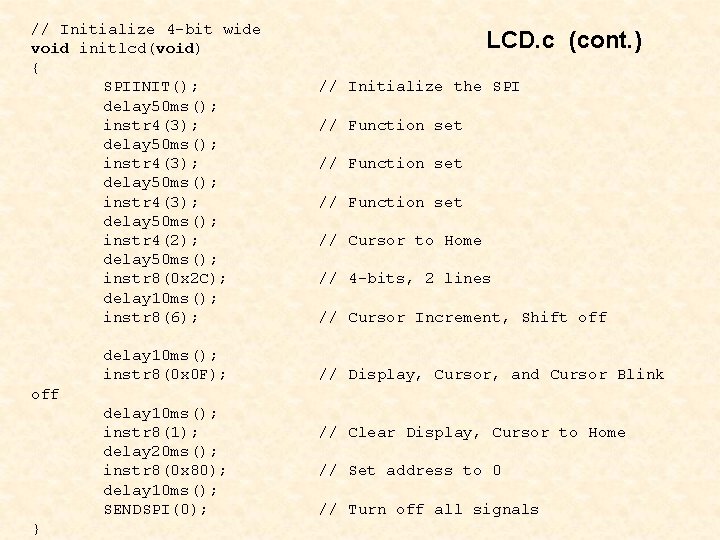
// Initialize 4 -bit wide void initlcd(void) { SPIINIT(); delay 50 ms(); instr 4(3); delay 50 ms(); instr 4(2); delay 50 ms(); instr 8(0 x 2 C); delay 10 ms(); instr 8(6); delay 10 ms(); instr 8(0 x 0 F); LCD. c (cont. ) // Initialize the SPI // Function set // Cursor to Home // 4 -bits, 2 lines // Cursor Increment, Shift off // Display, Cursor, and Cursor Blink off delay 10 ms(); instr 8(1); delay 20 ms(); instr 8(0 x 80); delay 10 ms(); SENDSPI(0); } // Clear Display, Cursor to Home // Set address to 0 // Turn off all signals
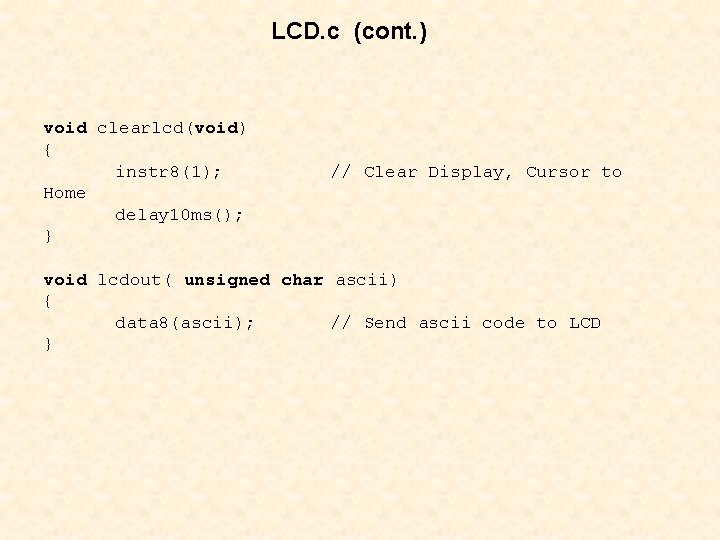
LCD. c (cont. ) void clearlcd(void) { instr 8(1); Home delay 10 ms(); } // Clear Display, Cursor to void lcdout( unsigned char ascii) { data 8(ascii); // Send ascii code to LCD }
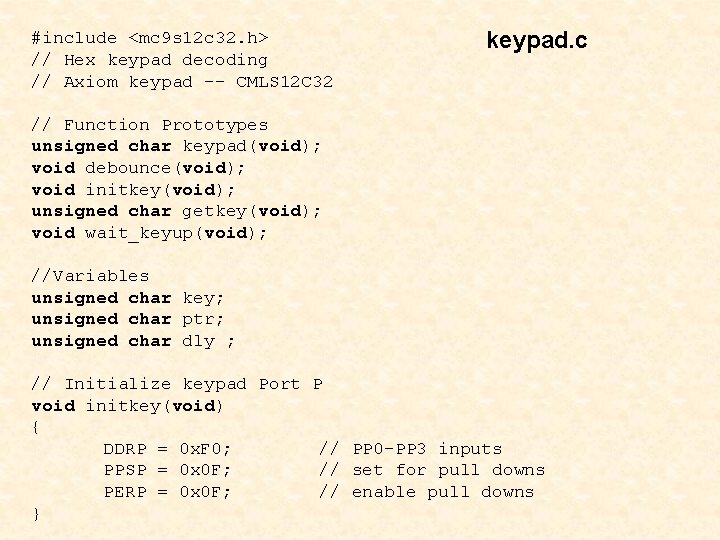
#include <mc 9 s 12 c 32. h> // Hex keypad decoding // Axiom keypad -- CMLS 12 C 32 keypad. c // Function Prototypes unsigned char keypad(void); void debounce(void); void initkey(void); unsigned char getkey(void); void wait_keyup(void); //Variables unsigned char key; unsigned char ptr; unsigned char dly ; // Initialize keypad Port P void initkey(void) { DDRP = 0 x. F 0; // PP 0 -PP 3 inputs PPSP = 0 x 0 F; // set for pull downs PERP = 0 x 0 F; // enable pull downs }
![keypad c cont Keycode table const unsigned char keycodes16 key row keypad. c (cont. ) // Keycode table const unsigned char keycodes[16]={ /* key row](https://slidetodoc.com/presentation_image_h2/8a12b68f841f03110162ad7395ab5fcb/image-18.jpg)
keypad. c (cont. ) // Keycode table const unsigned char keycodes[16]={ /* key row 0 x 14, /* 0 1 0 x 88, /* 1 4 0 x 84, /* 2 4 0 x 82, /* 3 4 0 x 48, /* 4 3 0 x 44, /* 5 3 0 x 42, /* 6 3 0 x 28, /* 7 2 0 x 24, /* 8 2 0 x 22, /* 9 2 0 x 81, /* A 4 0 x 41, /* B 3 0 x 21, /* C 2 0 x 11, /* D 1 0 x 18, /* E/* 1 0 x 12, /* F/# 1 }; col 2 1 2 3 4 4 1 3 */ */ */ */ */
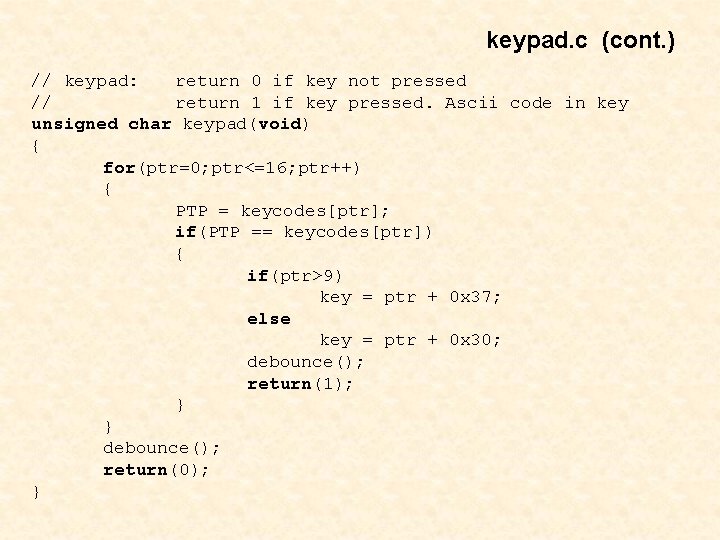
keypad. c (cont. ) // keypad: return 0 if key not pressed // return 1 if key pressed. Ascii code in key unsigned char keypad(void) { for(ptr=0; ptr<=16; ptr++) { PTP = keycodes[ptr]; if(PTP == keycodes[ptr]) { if(ptr>9) key = ptr + 0 x 37; else key = ptr + 0 x 30; debounce(); return(1); } } debounce(); return(0); }
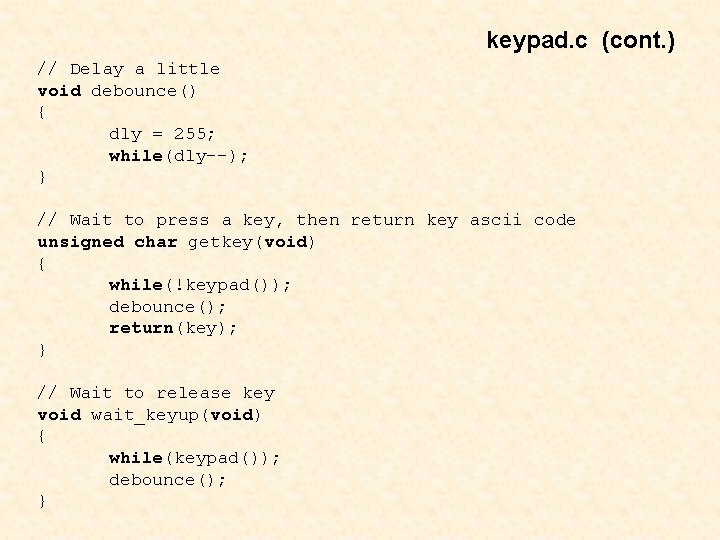
keypad. c (cont. ) // Delay a little void debounce() { dly = 255; while(dly--); } // Wait to press a key, then return key ascii code unsigned char getkey(void) { while(!keypad()); debounce(); return(key); } // Wait to release key void wait_keyup(void) { while(keypad()); debounce(); }
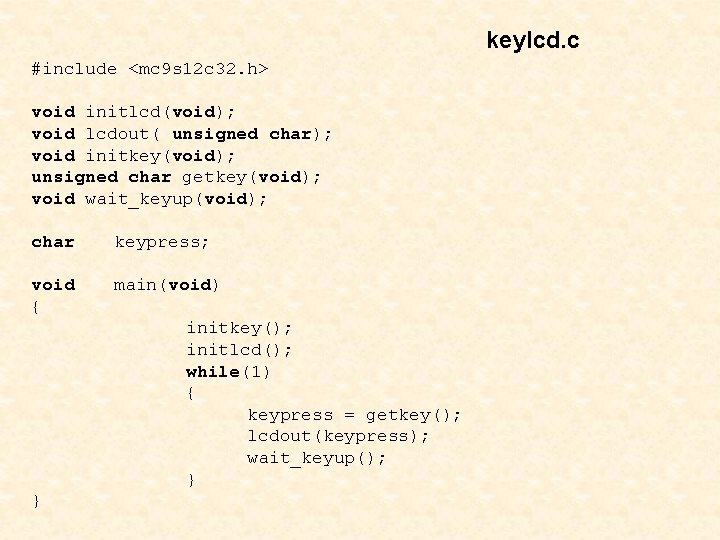
keylcd. c #include <mc 9 s 12 c 32. h> void initlcd(void); void lcdout( unsigned char); void initkey(void); unsigned char getkey(void); void wait_keyup(void); char keypress; void { main(void) initkey(); initlcd(); while(1) { keypress = getkey(); lcdout(keypress); wait_keyup(); } }
01:640:244 lecture notes - lecture 15: plat, idah, farad
Introduction to microcontrollers
Arm based microcontrollers
Pic architecture diagram
Single-board microcontrollers
Data memory
What is a microcontroller?
Sbuf in 8051
History of microcontrollers
Microcontrollers and embedded processors
Microprocessor presentation
Hello edge
Evolution of microcontrollers
Designing embedded systems with pic microcontrollers
C programming lecture
What is system programing
Linear vs integer programming
Perbedaan linear programming dan integer programming
Definisi linear
Greedy algorithm vs dynamic programming
Microcontroller system design
Lecture title