Lecture 19 Swing Control Programming Lecturer Prof Jim
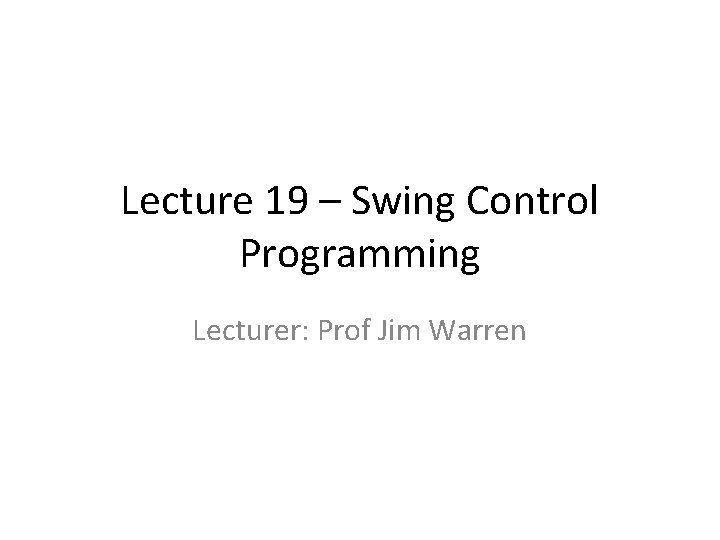
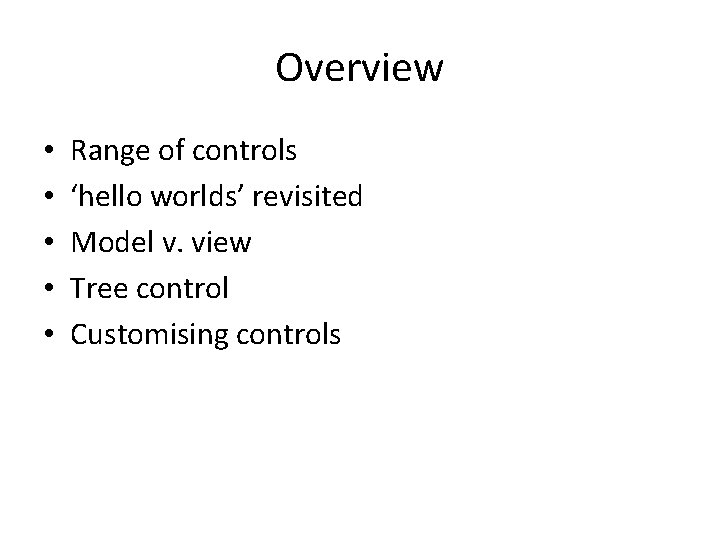
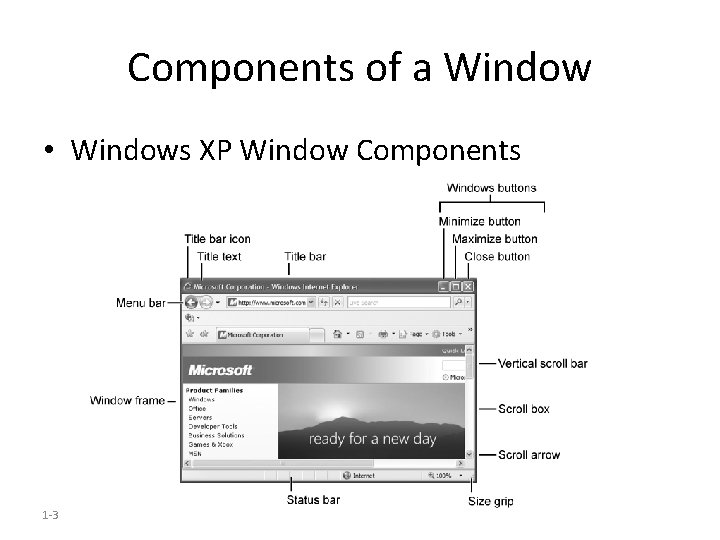
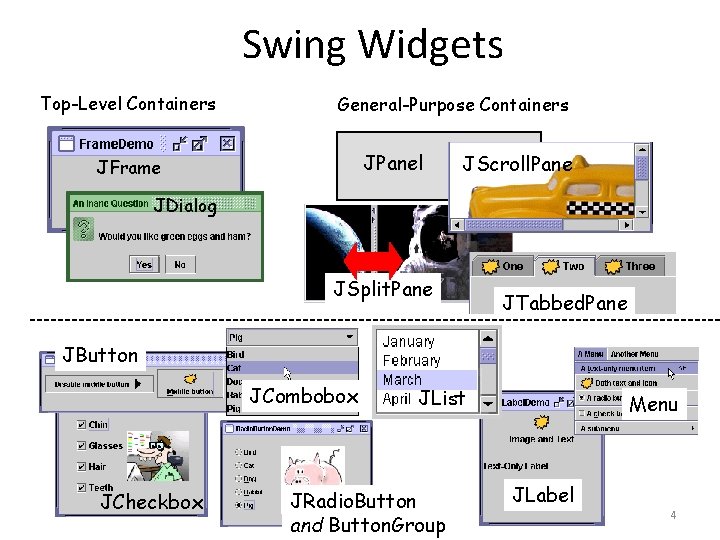
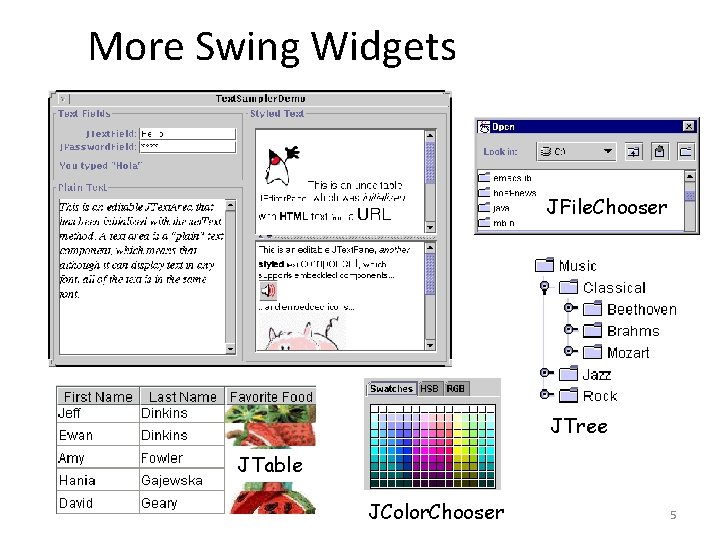
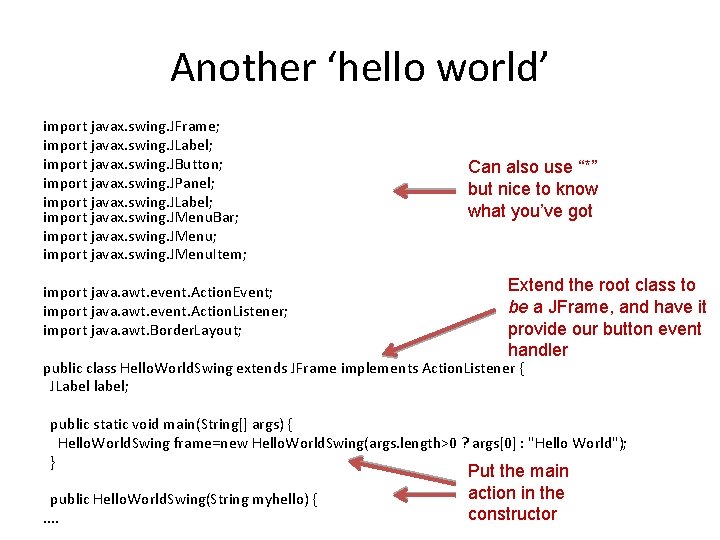
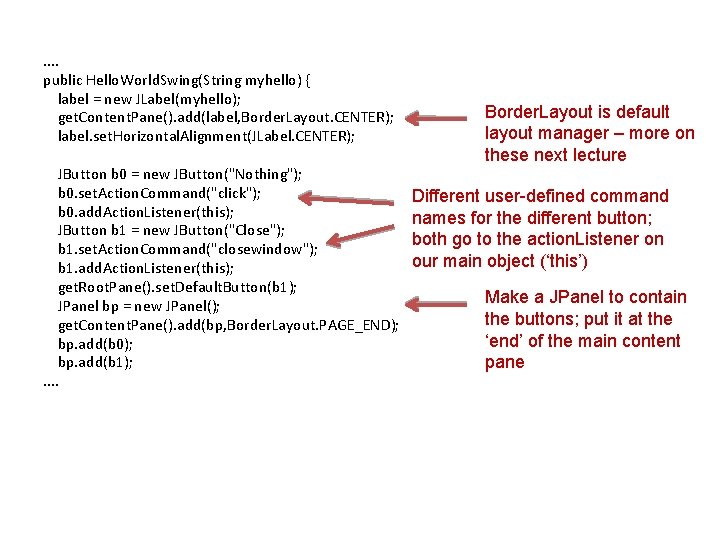
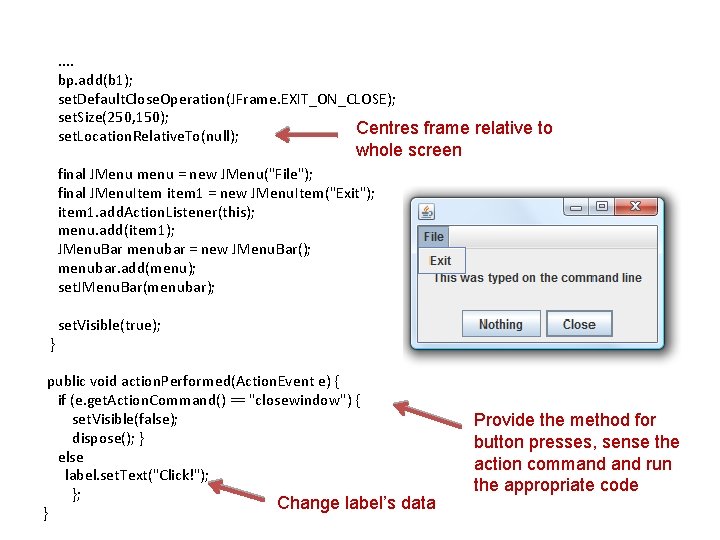
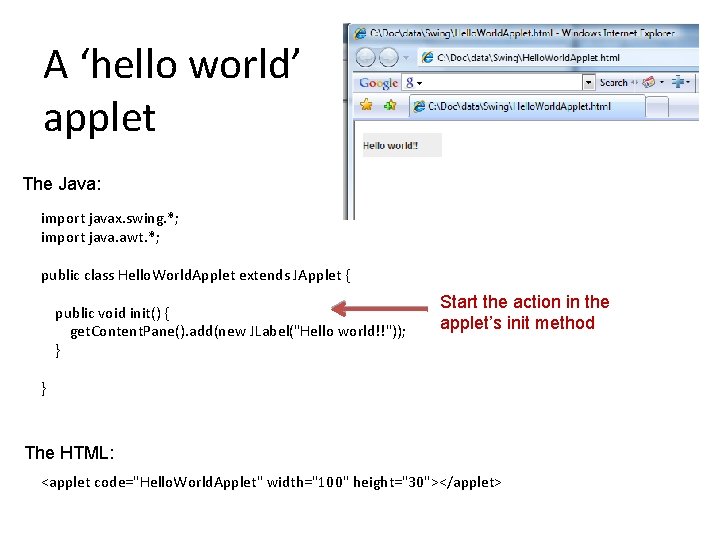
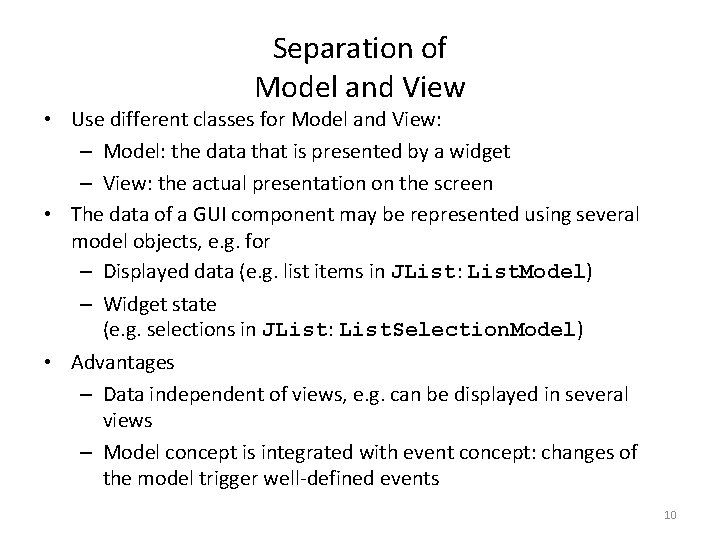
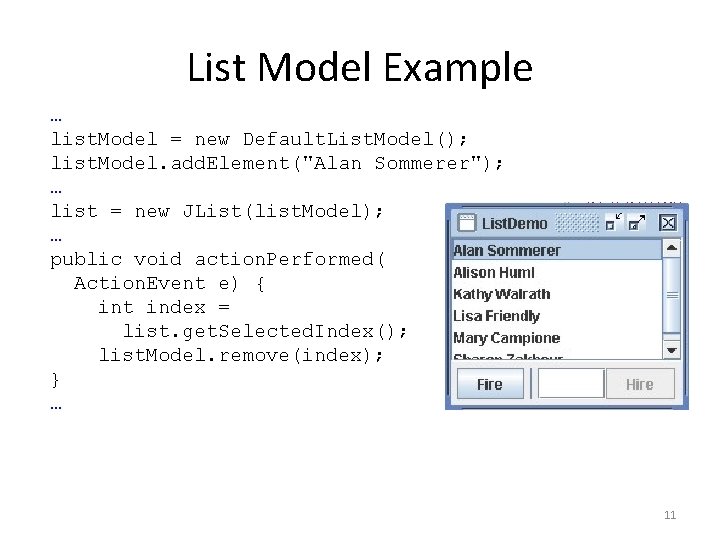
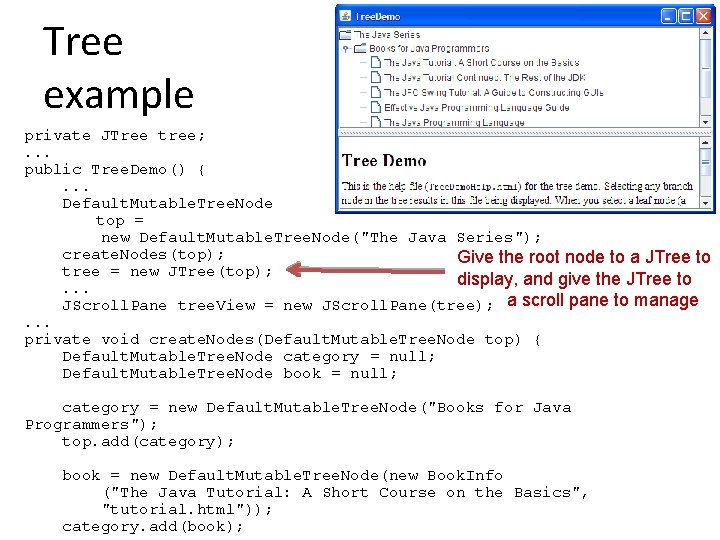
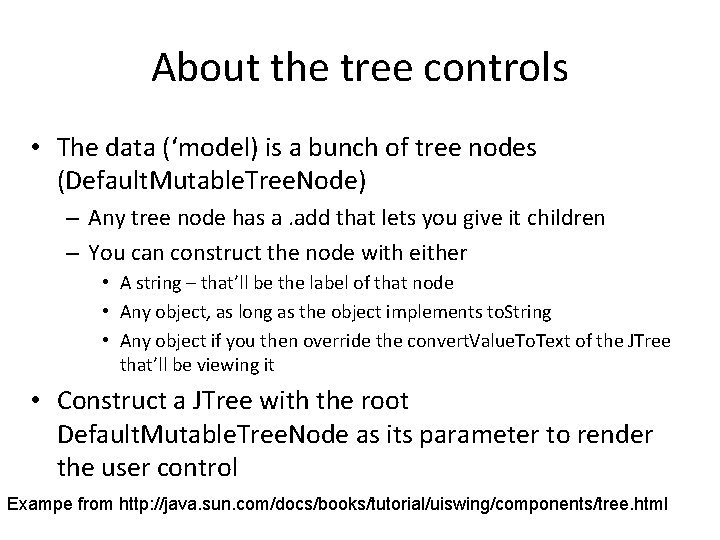
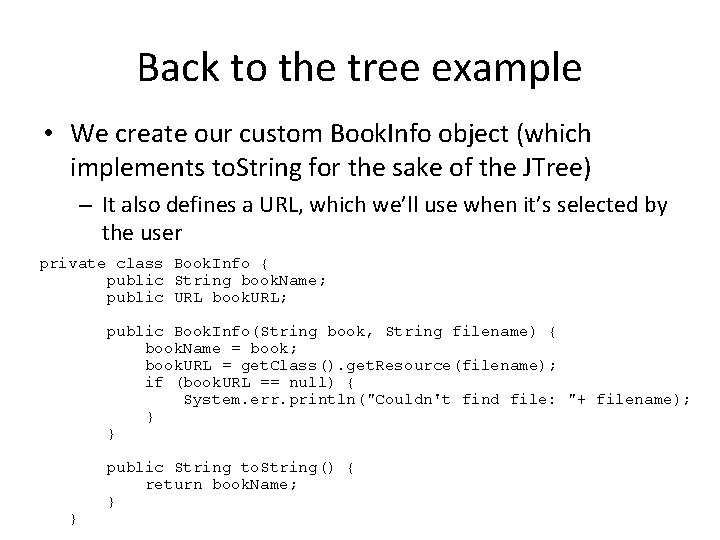
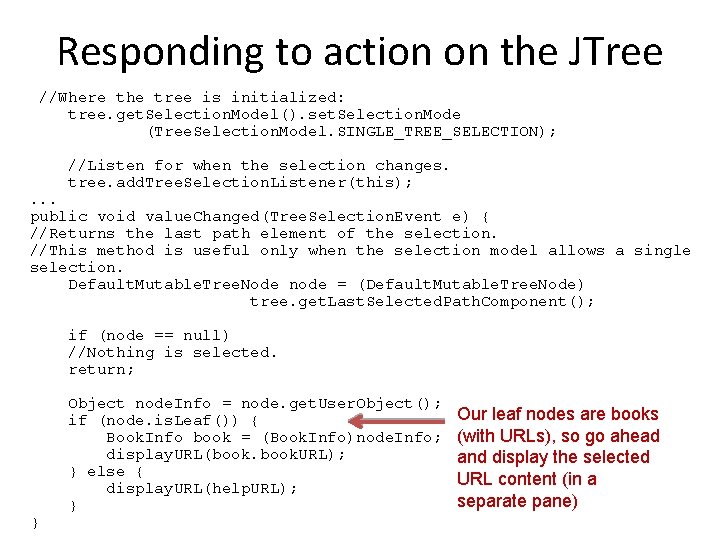
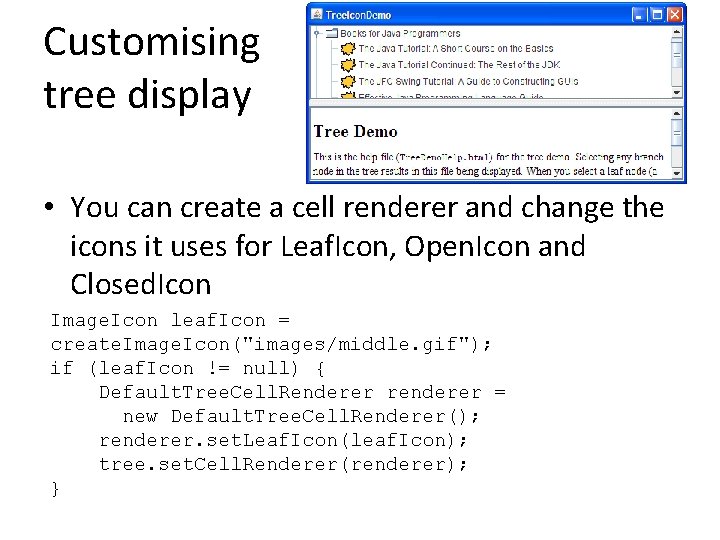
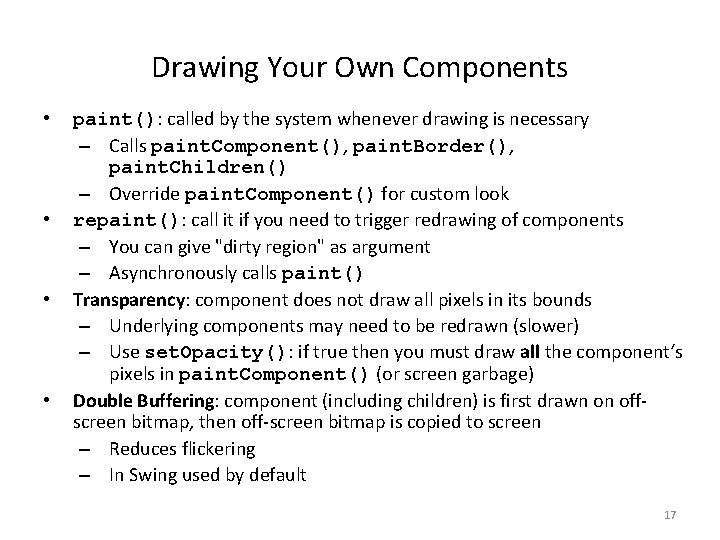
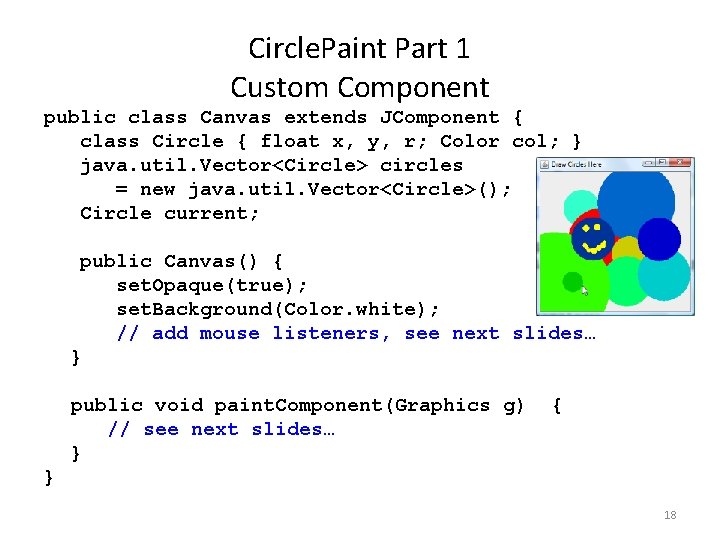
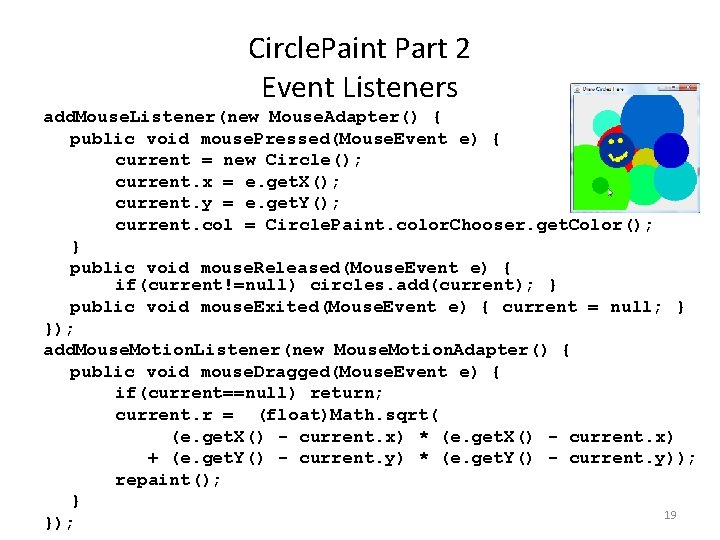
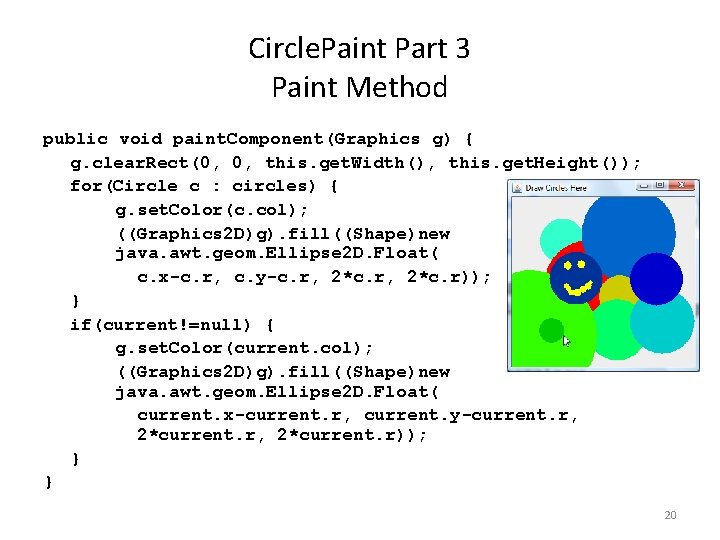
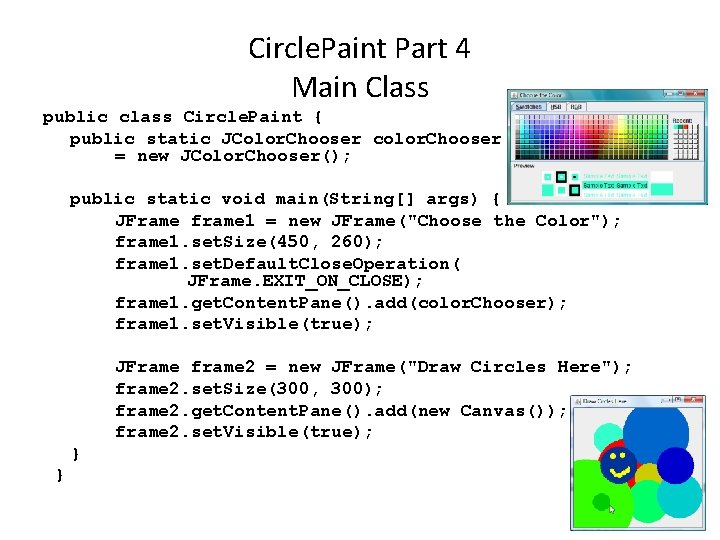
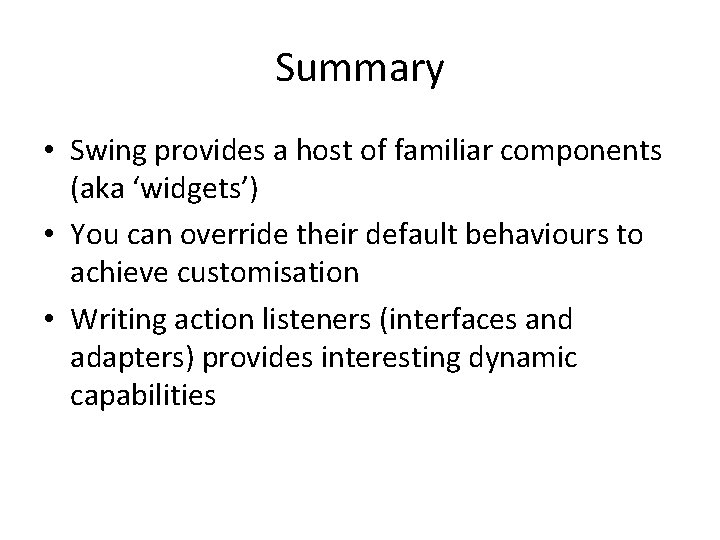
- Slides: 22
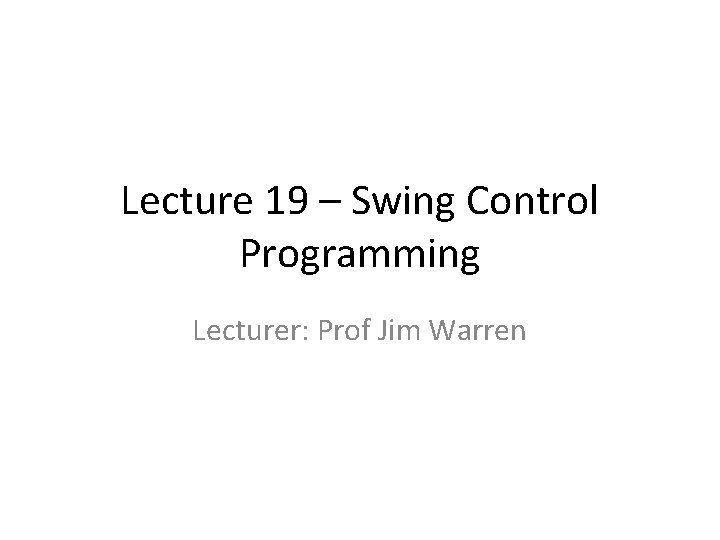
Lecture 19 – Swing Control Programming Lecturer: Prof Jim Warren
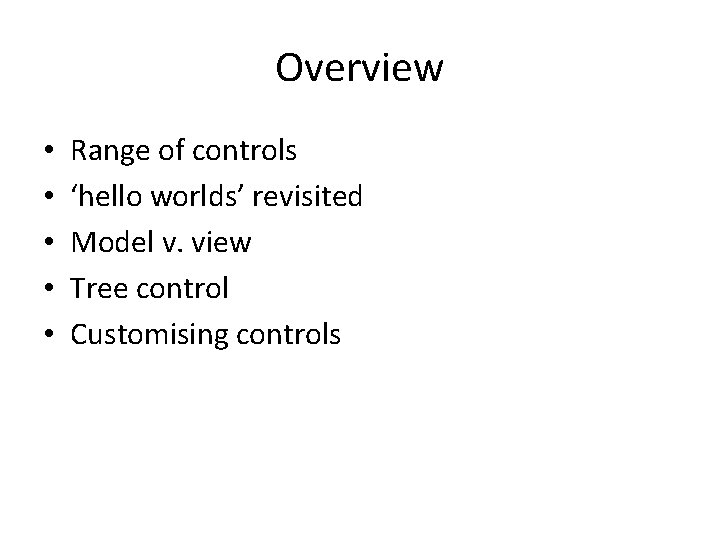
Overview • • • Range of controls ‘hello worlds’ revisited Model v. view Tree control Customising controls
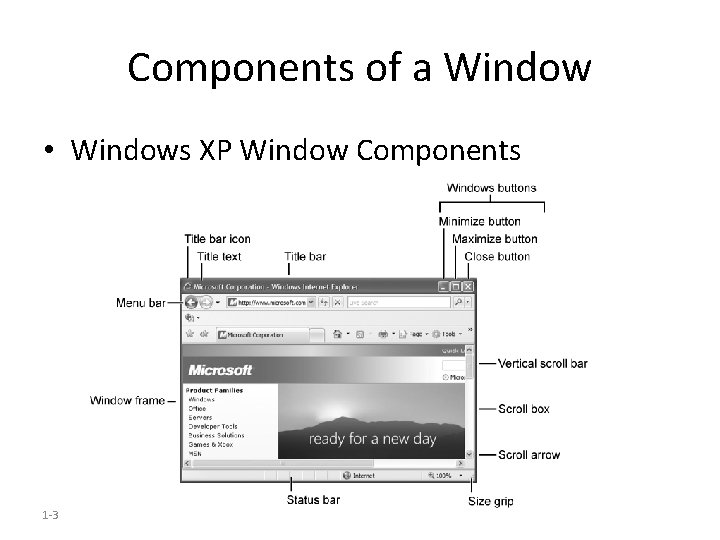
Components of a Window • Windows XP Window Components 1 -3
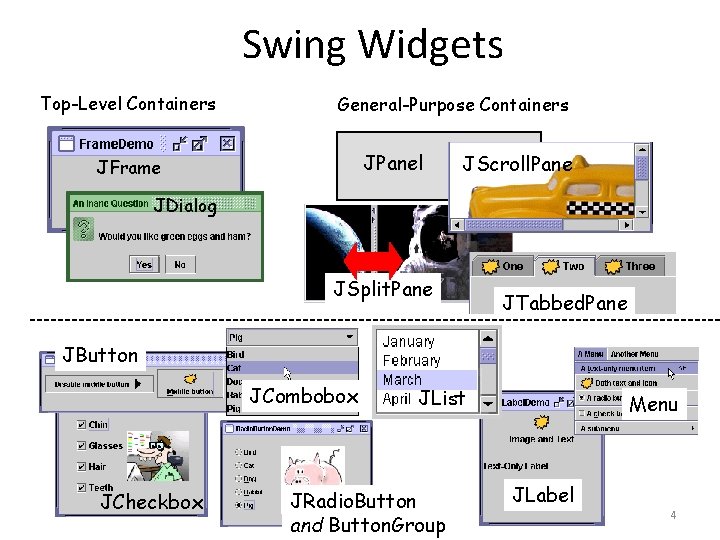
Swing Widgets Top-Level Containers General-Purpose Containers JPanel JFrame JScroll. Pane JDialog JSplit. Pane JTabbed. Pane JButton JCombobox JCheckbox JList JRadio. Button and Button. Group Menu JLabel 4
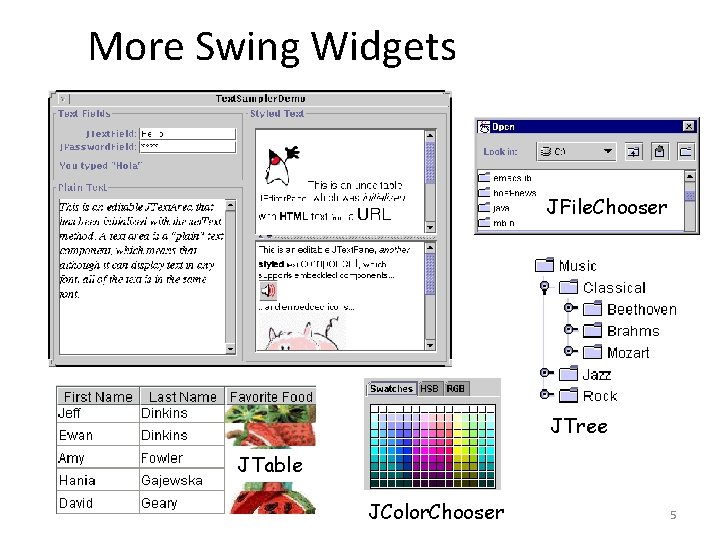
More Swing Widgets JFile. Chooser JTree JTable JColor. Chooser 5
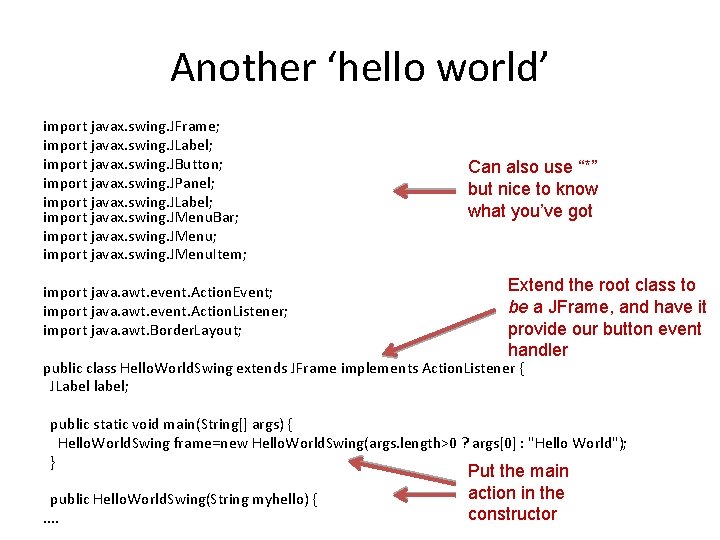
Another ‘hello world’ import javax. swing. JFrame; import javax. swing. JLabel; import javax. swing. JButton; import javax. swing. JPanel; import javax. swing. JLabel; import javax. swing. JMenu. Bar; import javax. swing. JMenu. Item; import java. awt. event. Action. Event; import java. awt. event. Action. Listener; import java. awt. Border. Layout; Can also use “*” but nice to know what you’ve got Extend the root class to be a JFrame, and have it provide our button event handler public class Hello. World. Swing extends JFrame implements Action. Listener { JLabel label; public static void main(String[] args) { Hello. World. Swing frame=new Hello. World. Swing(args. length>0 ? args[0] : "Hello World"); } public Hello. World. Swing(String myhello) {. . Put the main action in the constructor
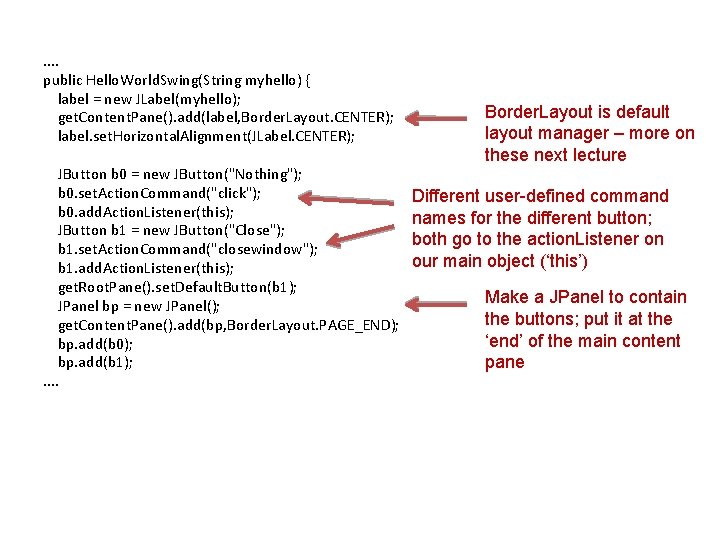
. . public Hello. World. Swing(String myhello) { label = new JLabel(myhello); get. Content. Pane(). add(label, Border. Layout. CENTER); label. set. Horizontal. Alignment(JLabel. CENTER); JButton b 0 = new JButton("Nothing"); b 0. set. Action. Command("click"); b 0. add. Action. Listener(this); JButton b 1 = new JButton("Close"); b 1. set. Action. Command("closewindow"); b 1. add. Action. Listener(this); get. Root. Pane(). set. Default. Button(b 1); JPanel bp = new JPanel(); get. Content. Pane(). add(bp, Border. Layout. PAGE_END); bp. add(b 0); bp. add(b 1); . . Border. Layout is default layout manager – more on these next lecture Different user-defined command names for the different button; both go to the action. Listener on our main object (‘this’) Make a JPanel to contain the buttons; put it at the ‘end’ of the main content pane
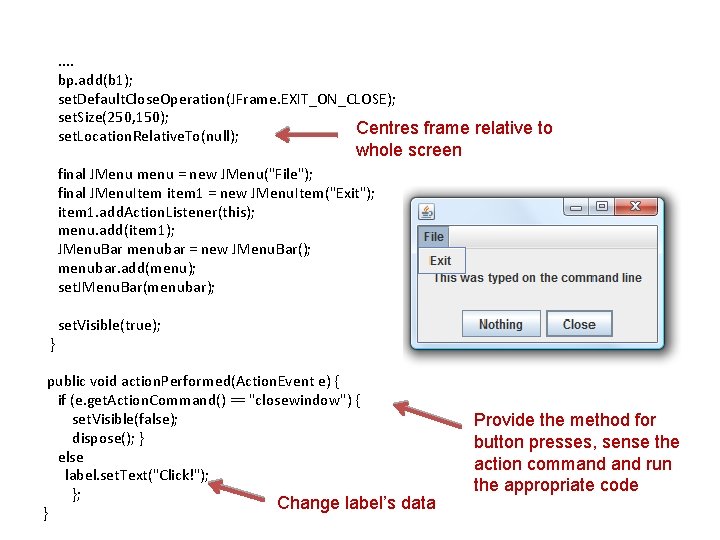
. . bp. add(b 1); set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); set. Size(250, 150); Centres frame relative to set. Location. Relative. To(null); whole screen final JMenu menu = new JMenu("File"); final JMenu. Item item 1 = new JMenu. Item("Exit"); item 1. add. Action. Listener(this); menu. add(item 1); JMenu. Bar menubar = new JMenu. Bar(); menubar. add(menu); set. JMenu. Bar(menubar); } set. Visible(true); public void action. Performed(Action. Event e) { if (e. get. Action. Command() == "closewindow") { set. Visible(false); dispose(); } else label. set. Text("Click!"); }; Change label’s data } Provide the method for button presses, sense the action command run the appropriate code
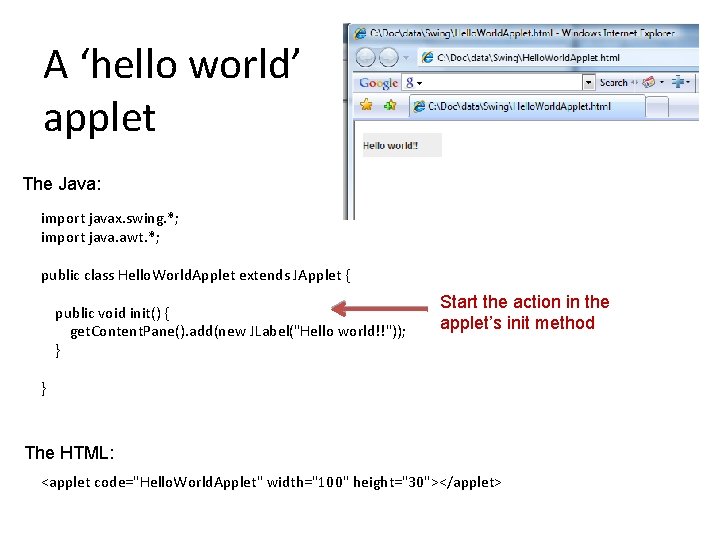
A ‘hello world’ applet The Java: import javax. swing. *; import java. awt. *; public class Hello. World. Applet extends JApplet { public void init() { get. Content. Pane(). add(new JLabel("Hello world!!")); } Start the action in the applet’s init method } The HTML: <applet code="Hello. World. Applet" width="100" height="30"></applet>
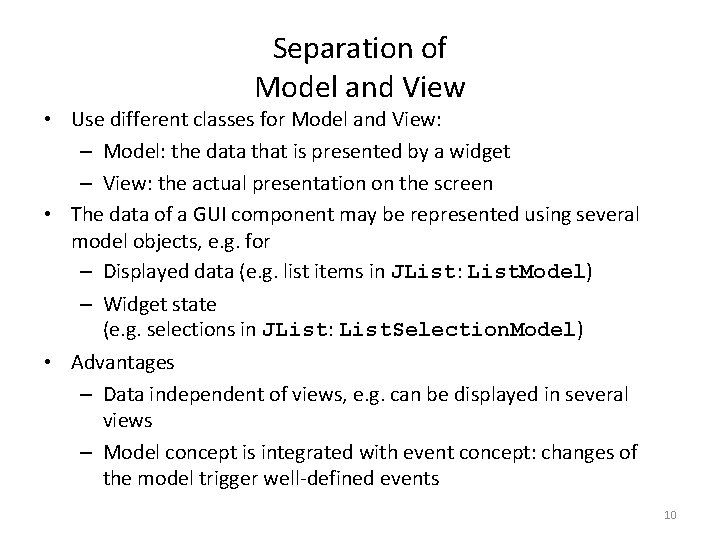
Separation of Model and View • Use different classes for Model and View: – Model: the data that is presented by a widget – View: the actual presentation on the screen • The data of a GUI component may be represented using several model objects, e. g. for – Displayed data (e. g. list items in JList: List. Model) – Widget state (e. g. selections in JList: List. Selection. Model) • Advantages – Data independent of views, e. g. can be displayed in several views – Model concept is integrated with event concept: changes of the model trigger well-defined events 10
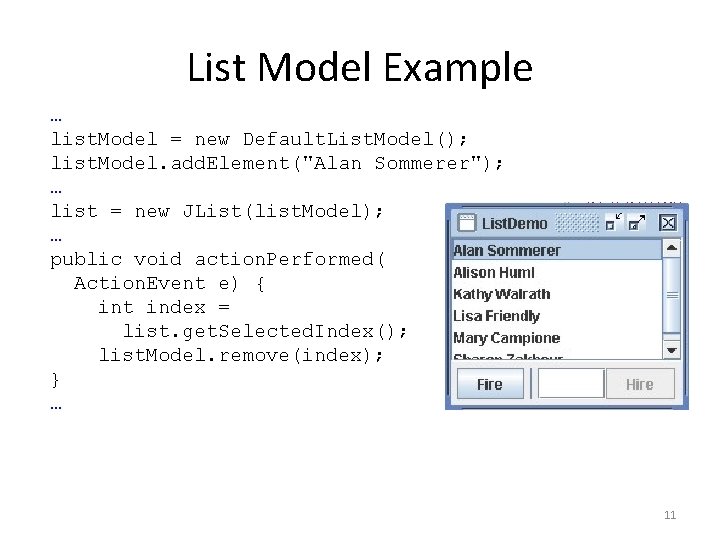
List Model Example … list. Model = new Default. List. Model(); list. Model. add. Element("Alan Sommerer"); … list = new JList(list. Model); … public void action. Performed( Action. Event e) { int index = list. get. Selected. Index(); list. Model. remove(index); } … 11
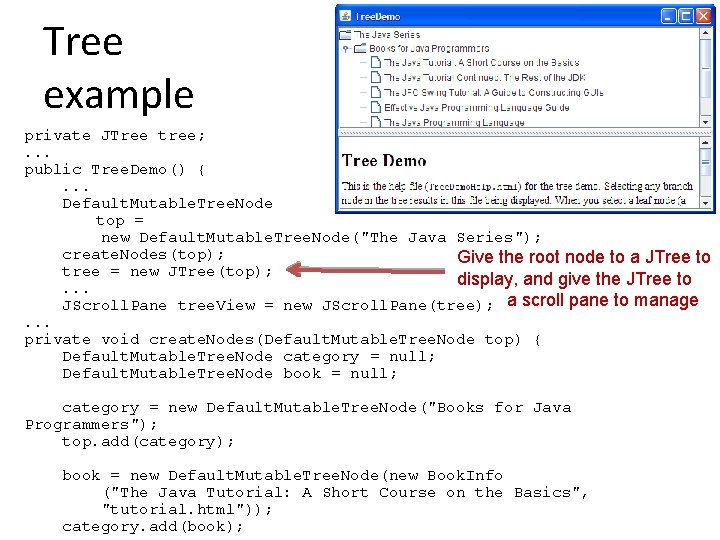
Tree example private JTree tree; . . . public Tree. Demo() {. . . Default. Mutable. Tree. Node top = new Default. Mutable. Tree. Node("The Java Series"); create. Nodes(top); Give the root node to a JTree to tree = new JTree(top); display, and give the JTree to. . . JScroll. Pane tree. View = new JScroll. Pane(tree); a scroll pane to manage. . . private void create. Nodes(Default. Mutable. Tree. Node top) { Default. Mutable. Tree. Node category = null; Default. Mutable. Tree. Node book = null; category = new Default. Mutable. Tree. Node("Books for Java Programmers"); top. add(category); book = new Default. Mutable. Tree. Node(new Book. Info ("The Java Tutorial: A Short Course on the Basics", "tutorial. html")); category. add(book);
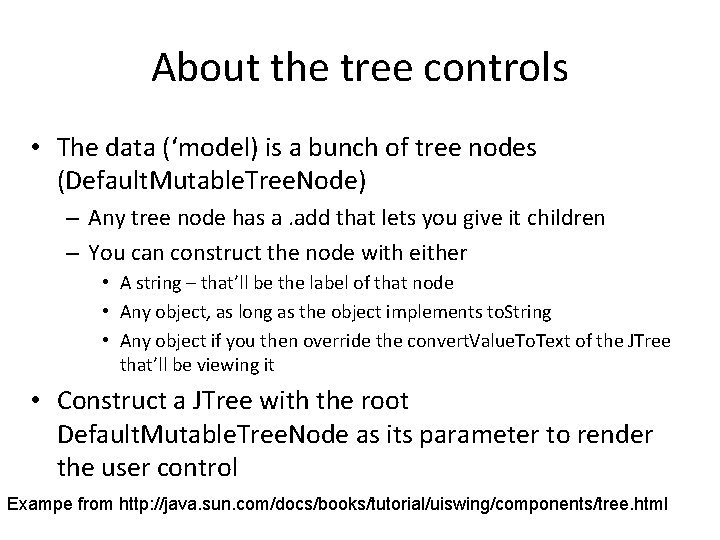
About the tree controls • The data (‘model) is a bunch of tree nodes (Default. Mutable. Tree. Node) – Any tree node has a. add that lets you give it children – You can construct the node with either • A string – that’ll be the label of that node • Any object, as long as the object implements to. String • Any object if you then override the convert. Value. To. Text of the JTree that’ll be viewing it • Construct a JTree with the root Default. Mutable. Tree. Node as its parameter to render the user control Exampe from http: //java. sun. com/docs/books/tutorial/uiswing/components/tree. html
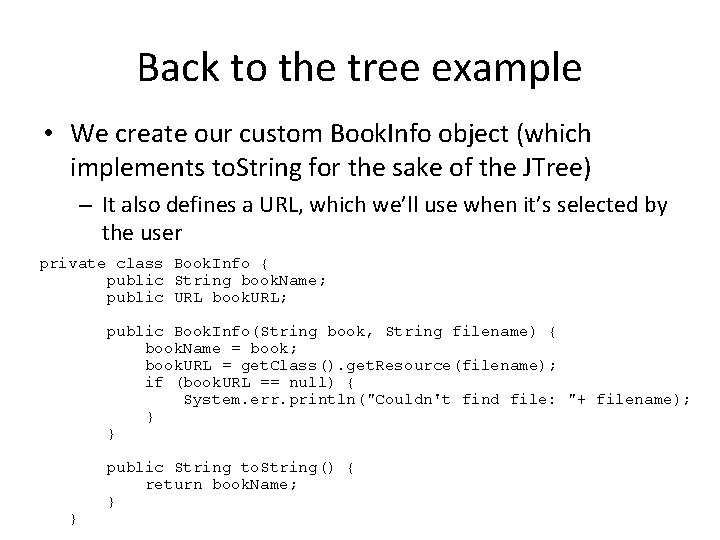
Back to the tree example • We create our custom Book. Info object (which implements to. String for the sake of the JTree) – It also defines a URL, which we’ll use when it’s selected by the user private class Book. Info { public String book. Name; public URL book. URL; public Book. Info(String book, String filename) { book. Name = book; book. URL = get. Class(). get. Resource(filename); if (book. URL == null) { System. err. println("Couldn't find file: "+ filename); } } } public String to. String() { return book. Name; }
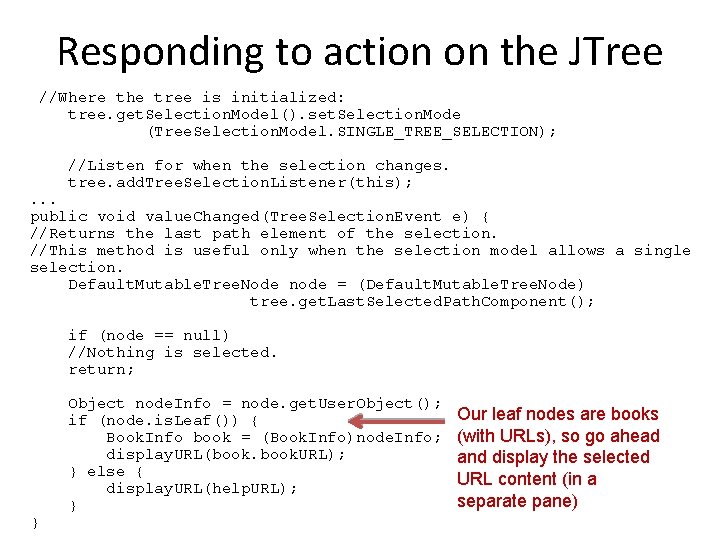
Responding to action on the JTree //Where the tree is initialized: tree. get. Selection. Model(). set. Selection. Mode (Tree. Selection. Model. SINGLE_TREE_SELECTION); //Listen for when the selection changes. tree. add. Tree. Selection. Listener(this); . . . public void value. Changed(Tree. Selection. Event e) { //Returns the last path element of the selection. //This method is useful only when the selection model allows a single selection. Default. Mutable. Tree. Node node = (Default. Mutable. Tree. Node) tree. get. Last. Selected. Path. Component(); if (node == null) //Nothing is selected. return; } Object node. Info = node. get. User. Object(); if (node. is. Leaf()) { Book. Info book = (Book. Info)node. Info; display. URL(book. URL); } else { display. URL(help. URL); } Our leaf nodes are books (with URLs), so go ahead and display the selected URL content (in a separate pane)
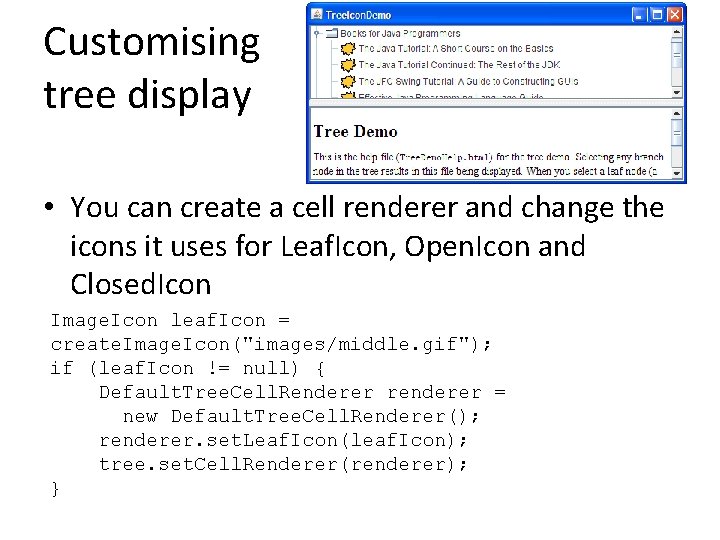
Customising tree display • You can create a cell renderer and change the icons it uses for Leaf. Icon, Open. Icon and Closed. Icon Image. Icon leaf. Icon = create. Image. Icon("images/middle. gif"); if (leaf. Icon != null) { Default. Tree. Cell. Renderer renderer = new Default. Tree. Cell. Renderer(); renderer. set. Leaf. Icon(leaf. Icon); tree. set. Cell. Renderer(renderer); }
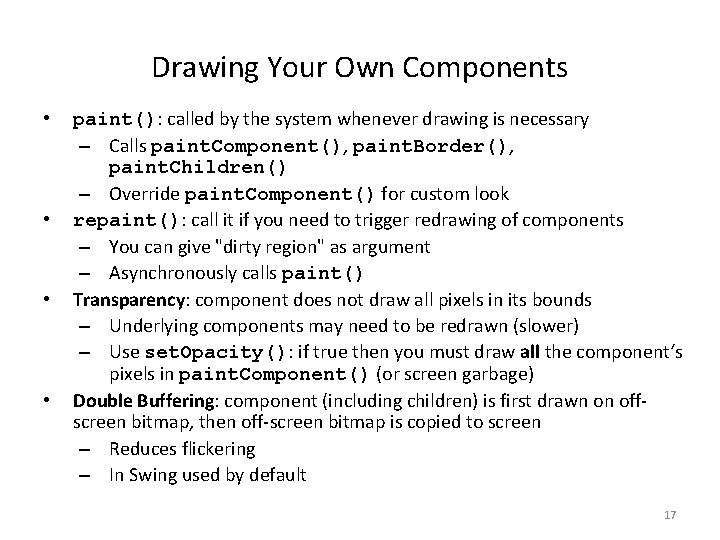
Drawing Your Own Components • • paint(): called by the system whenever drawing is necessary – Calls paint. Component(), paint. Border(), paint. Children() – Override paint. Component() for custom look repaint(): call it if you need to trigger redrawing of components – You can give "dirty region" as argument – Asynchronously calls paint() Transparency: component does not draw all pixels in its bounds – Underlying components may need to be redrawn (slower) – Use set. Opacity(): if true then you must draw all the component‘s pixels in paint. Component() (or screen garbage) Double Buffering: component (including children) is first drawn on offscreen bitmap, then off-screen bitmap is copied to screen – Reduces flickering – In Swing used by default 17
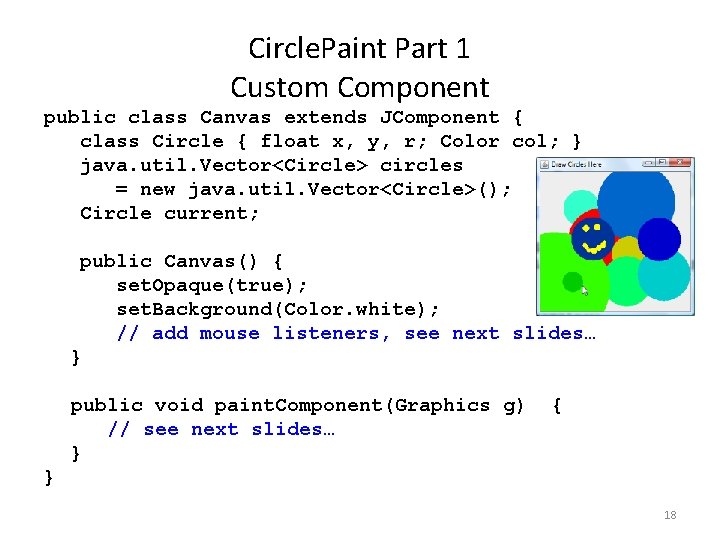
Circle. Paint Part 1 Custom Component public class Canvas extends JComponent { class Circle { float x, y, r; Color col; } java. util. Vector<Circle> circles = new java. util. Vector<Circle>(); Circle current; public Canvas() { set. Opaque(true); set. Background(Color. white); // add mouse listeners, see next slides… } public void paint. Component(Graphics g) // see next slides… } { } 18
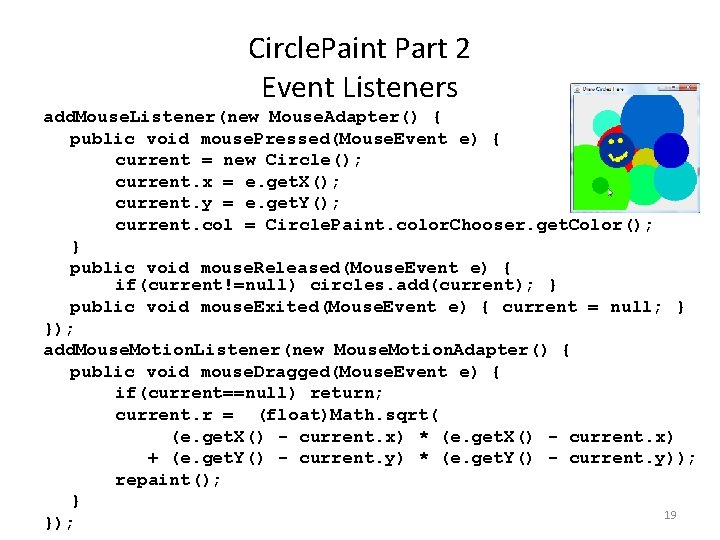
Circle. Paint Part 2 Event Listeners add. Mouse. Listener(new Mouse. Adapter() { public void mouse. Pressed(Mouse. Event e) { current = new Circle(); current. x = e. get. X(); current. y = e. get. Y(); current. col = Circle. Paint. color. Chooser. get. Color(); } public void mouse. Released(Mouse. Event e) { if(current!=null) circles. add(current); } public void mouse. Exited(Mouse. Event e) { current = null; } }); add. Mouse. Motion. Listener(new Mouse. Motion. Adapter() { public void mouse. Dragged(Mouse. Event e) { if(current==null) return; current. r = (float)Math. sqrt( (e. get. X() - current. x) * (e. get. X() - current. x) + (e. get. Y() - current. y) * (e. get. Y() - current. y)); repaint(); } 19 });
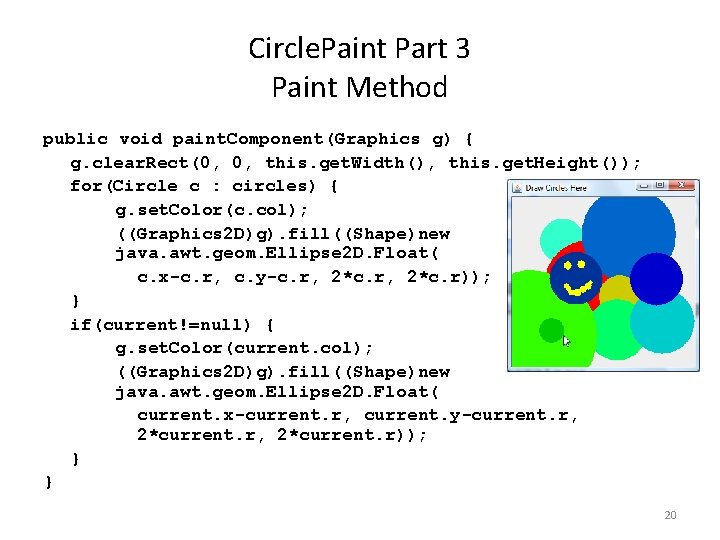
Circle. Paint Part 3 Paint Method public void paint. Component(Graphics g) { g. clear. Rect(0, 0, this. get. Width(), this. get. Height()); for(Circle c : circles) { g. set. Color(c. col); ((Graphics 2 D)g). fill((Shape)new java. awt. geom. Ellipse 2 D. Float( c. x-c. r, c. y-c. r, 2*c. r)); } if(current!=null) { g. set. Color(current. col); ((Graphics 2 D)g). fill((Shape)new java. awt. geom. Ellipse 2 D. Float( current. x-current. r, current. y-current. r, 2*current. r)); } } 20
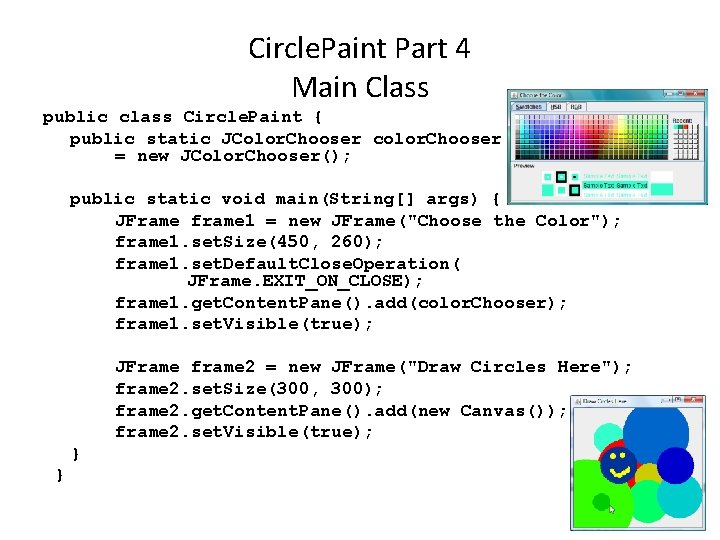
Circle. Paint Part 4 Main Class public class Circle. Paint { public static JColor. Chooser color. Chooser = new JColor. Chooser(); public static void main(String[] args) { JFrame frame 1 = new JFrame("Choose the Color"); frame 1. set. Size(450, 260); frame 1. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE); frame 1. get. Content. Pane(). add(color. Chooser); frame 1. set. Visible(true); JFrame frame 2 = new JFrame("Draw Circles Here"); frame 2. set. Size(300, 300); frame 2. get. Content. Pane(). add(new Canvas()); frame 2. set. Visible(true); } } 21
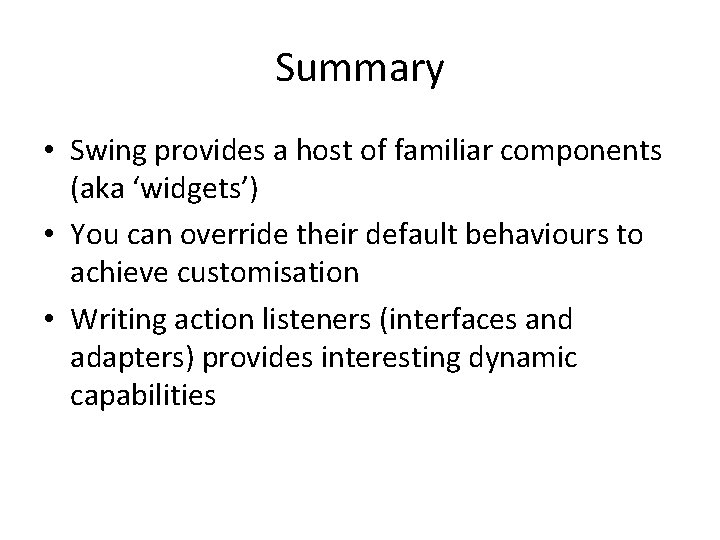
Summary • Swing provides a host of familiar components (aka ‘widgets’) • You can override their default behaviours to achieve customisation • Writing action listeners (interfaces and adapters) provides interesting dynamic capabilities
Lecturer's name or lecturer name
Prof jim inc
Swing 4
01:640:244 lecture notes - lecture 15: plat, idah, farad
Jeannie watkins
Spe distinguished lecturer
Teacher good morning students
Photography lecturer
Lecturer in charge
Designation lecturer
Designation of lecturer
Why himalayan rivers are pernnial in nature
Lecturer name
Pearson lecturer resources
140000/120
Lector vs lecturer
Lecturer in charge
Cfa lecturer handbook
Lecturer asad ali
C data types with examples
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Windows 10 system programming, part 1