Java Software Solutions Lewis and Loftus Sorting and
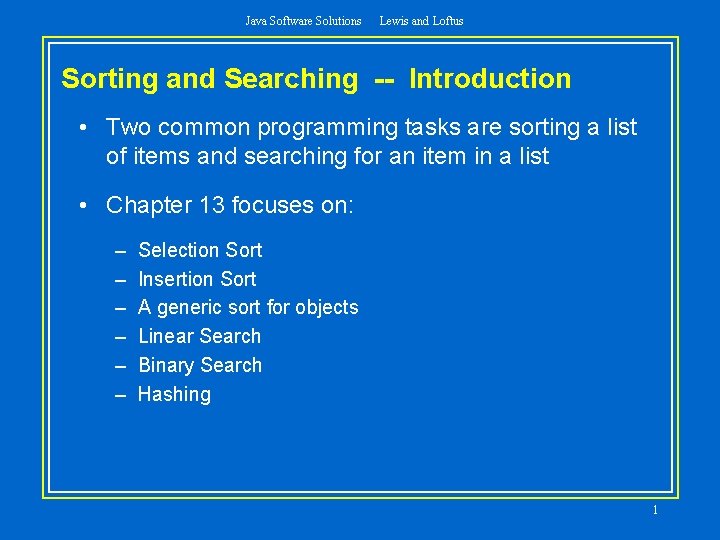
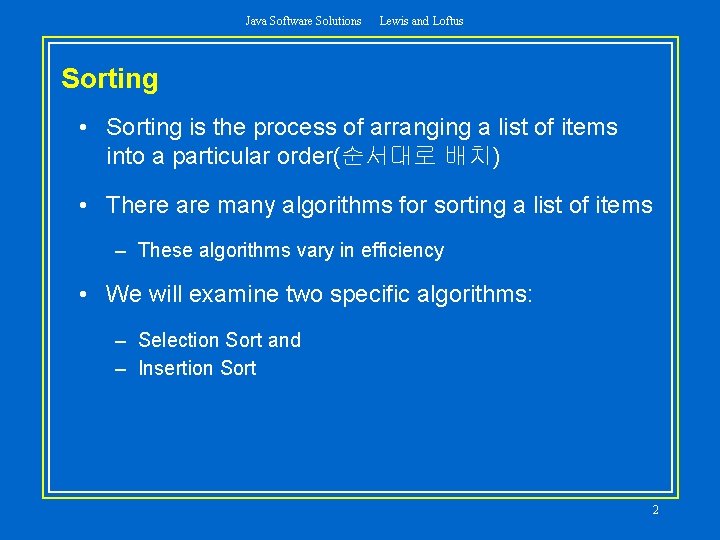
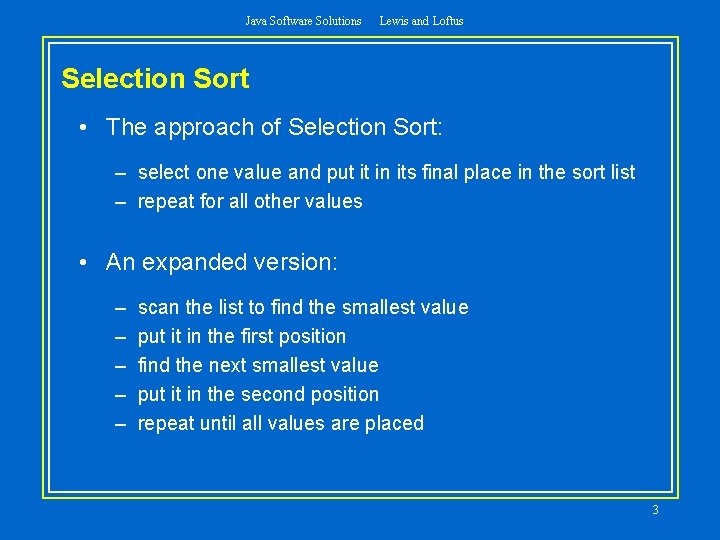
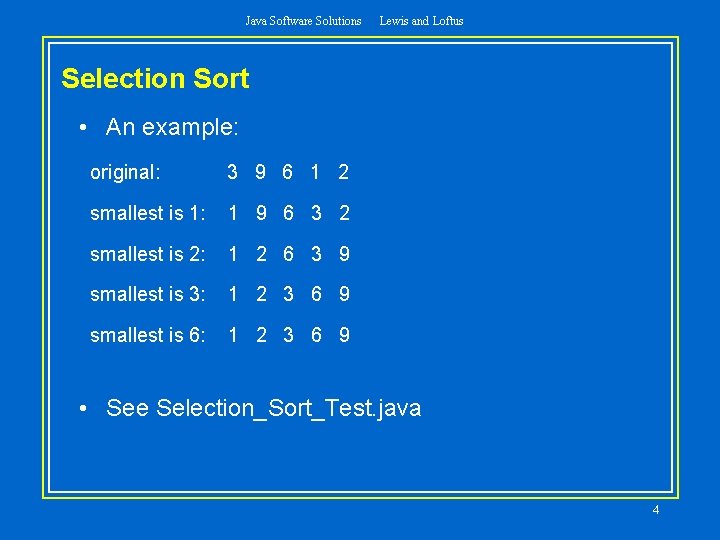
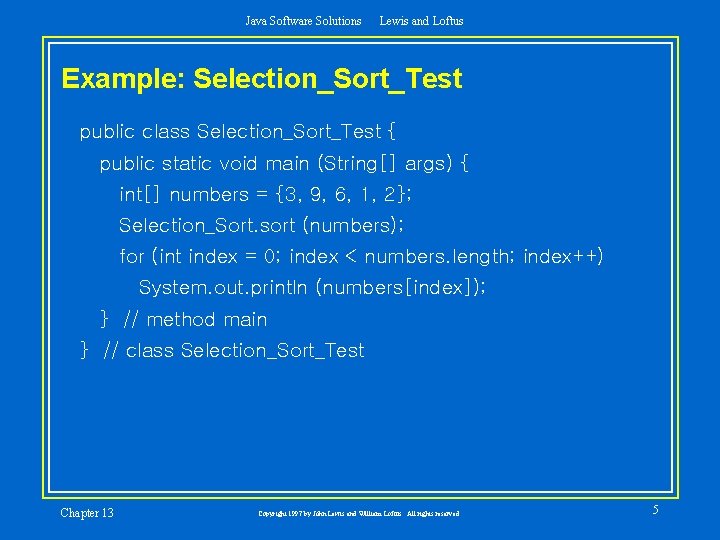
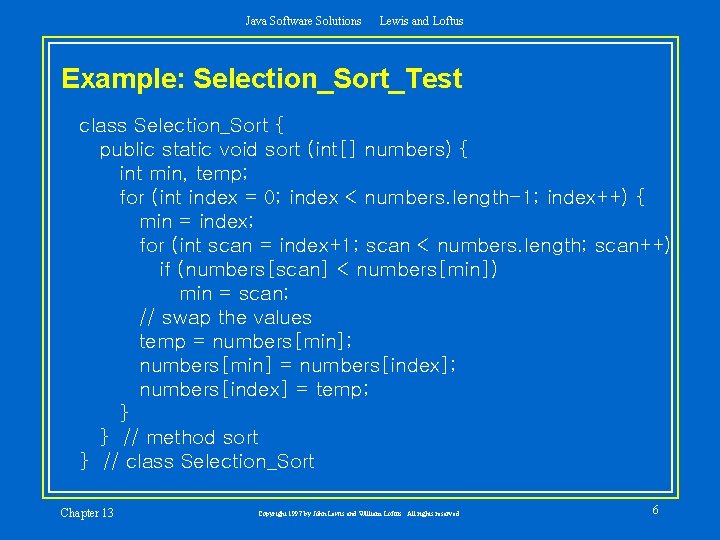
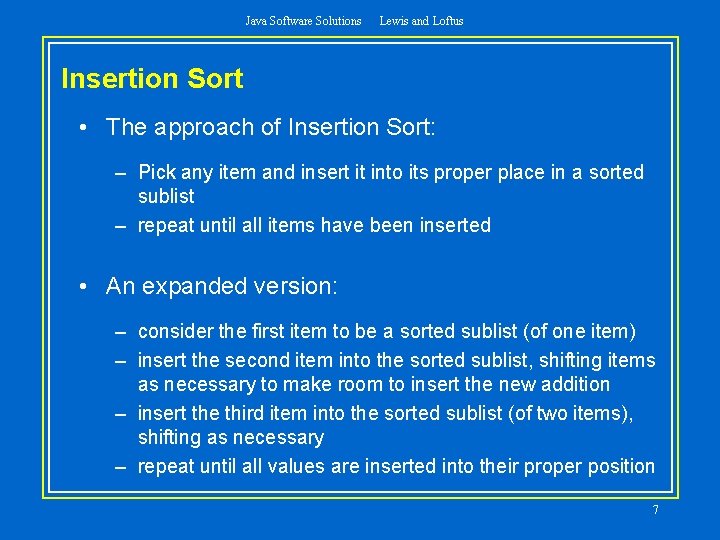
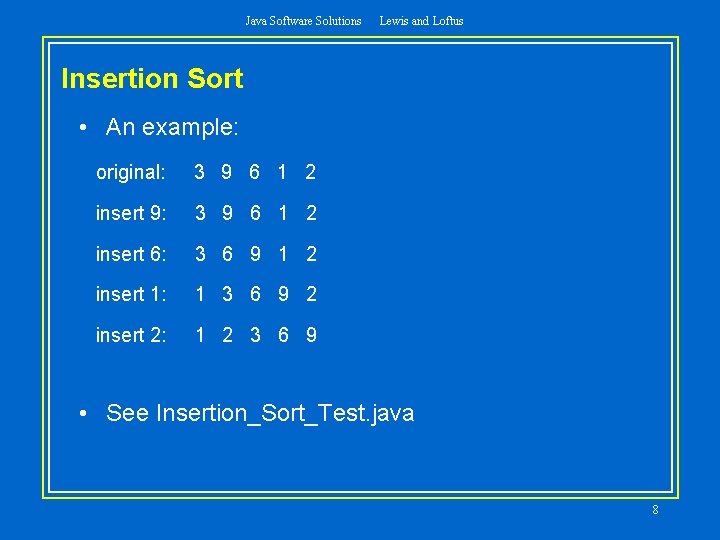
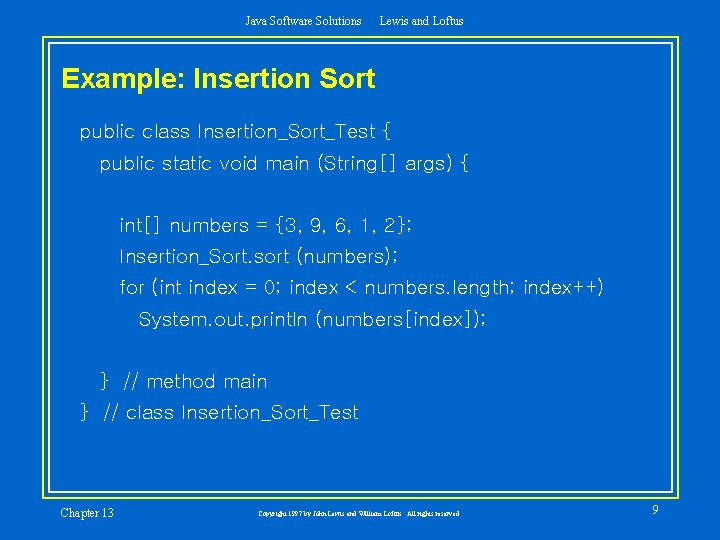
![Java Software Solutions Lewis and Loftus class Insertion_Sort { public static void sort (int[] Java Software Solutions Lewis and Loftus class Insertion_Sort { public static void sort (int[]](https://slidetodoc.com/presentation_image_h2/979f140e40379a9469950391d7909862/image-10.jpg)
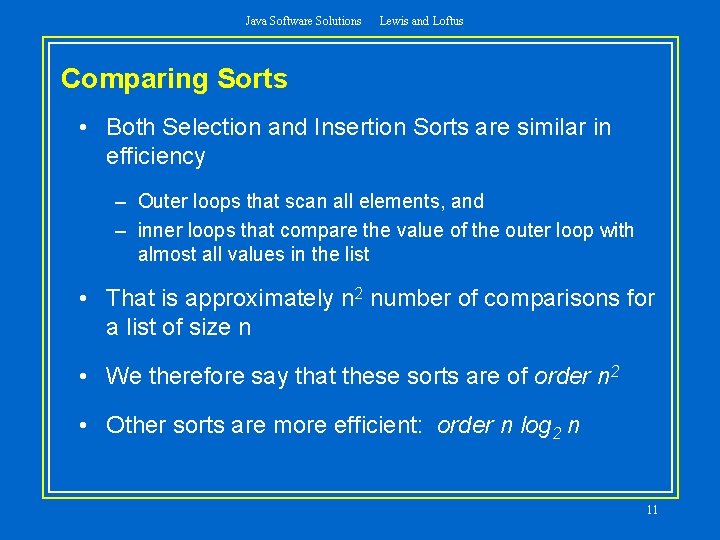
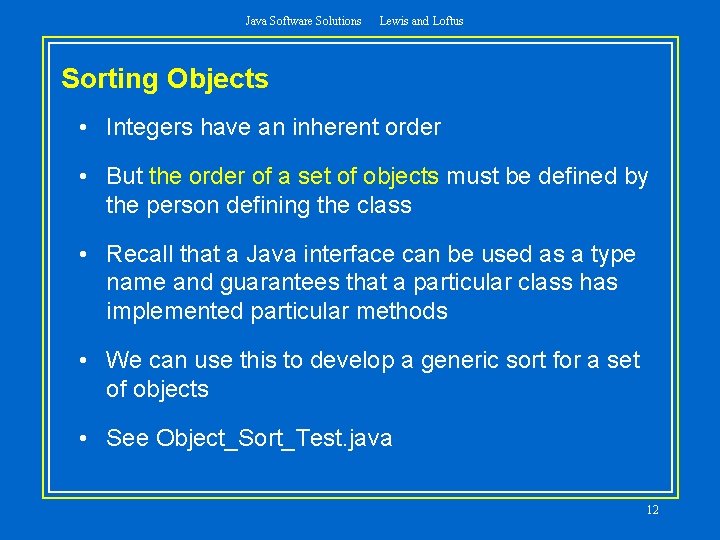
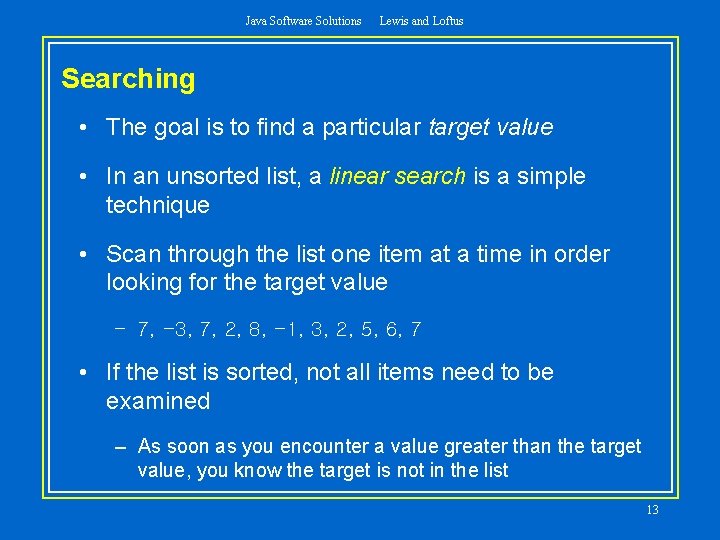
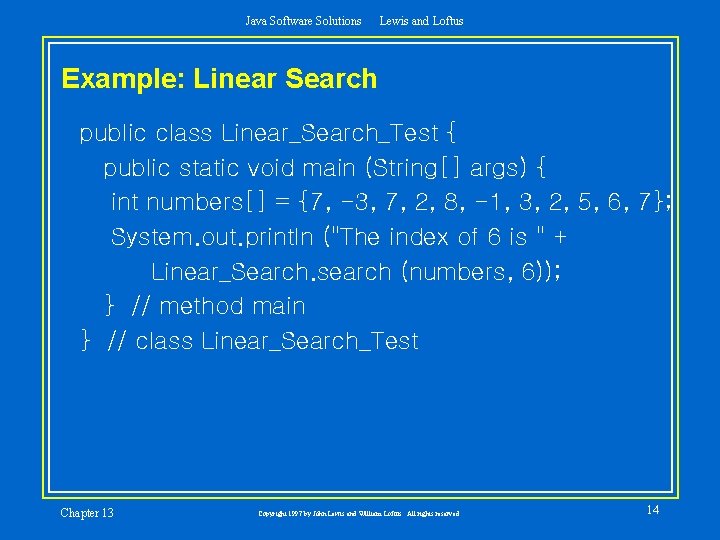
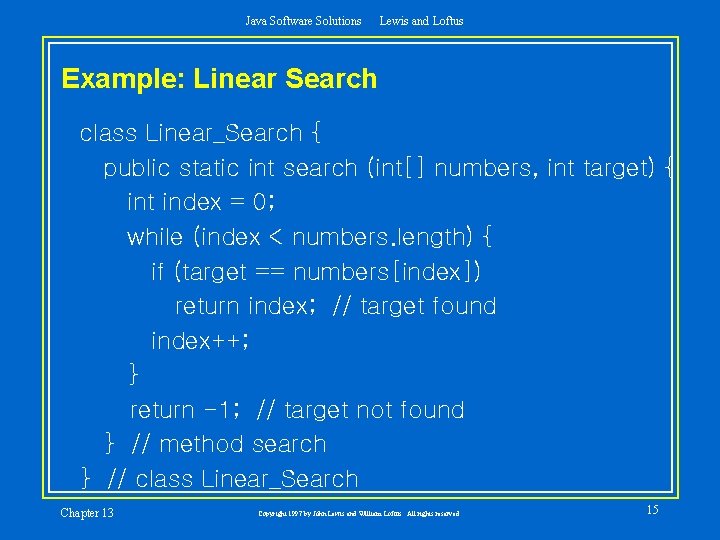
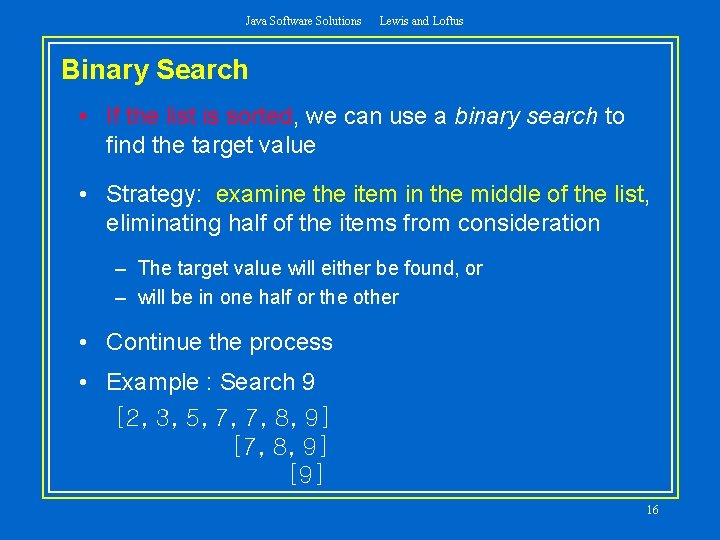
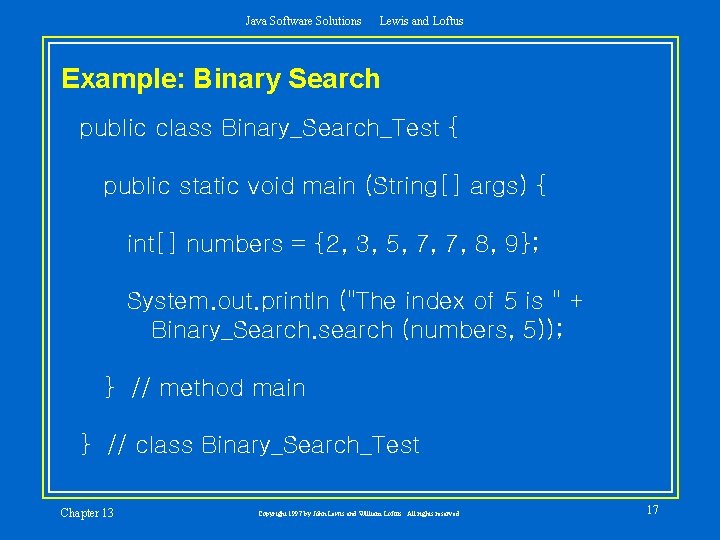
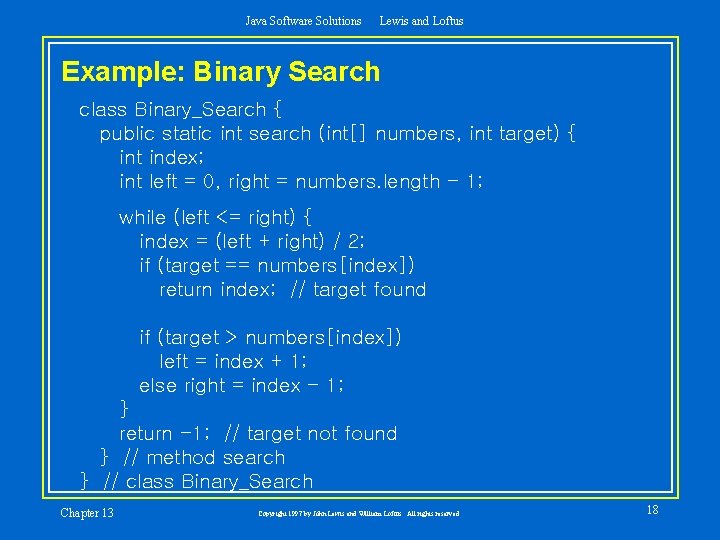
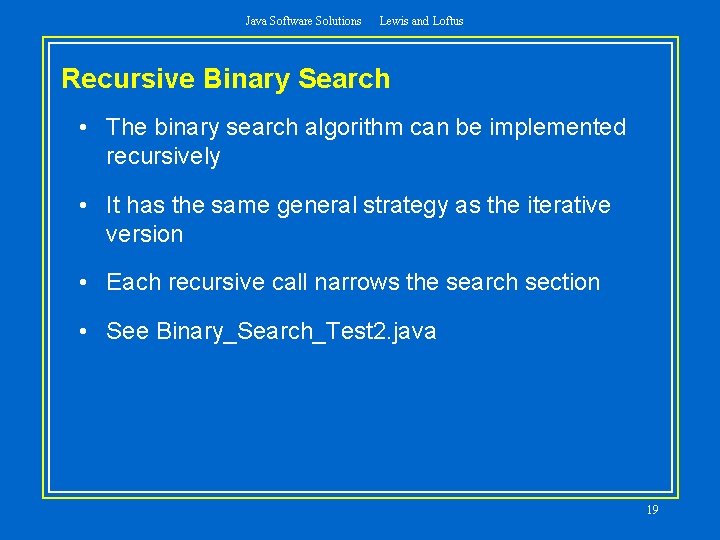
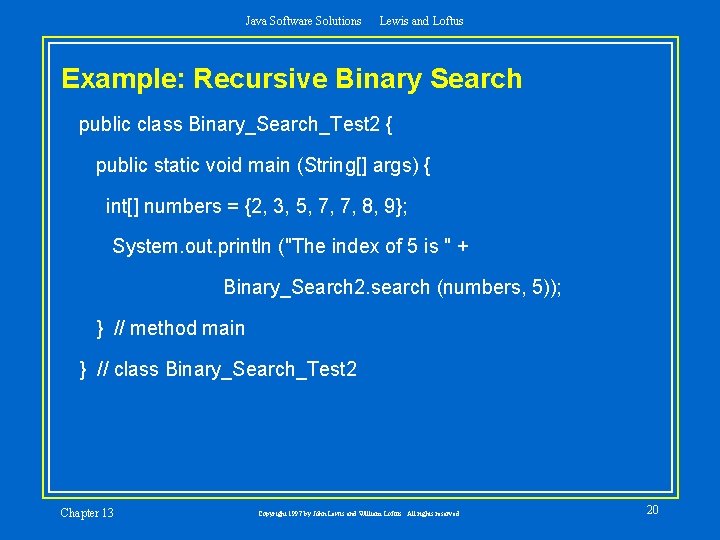
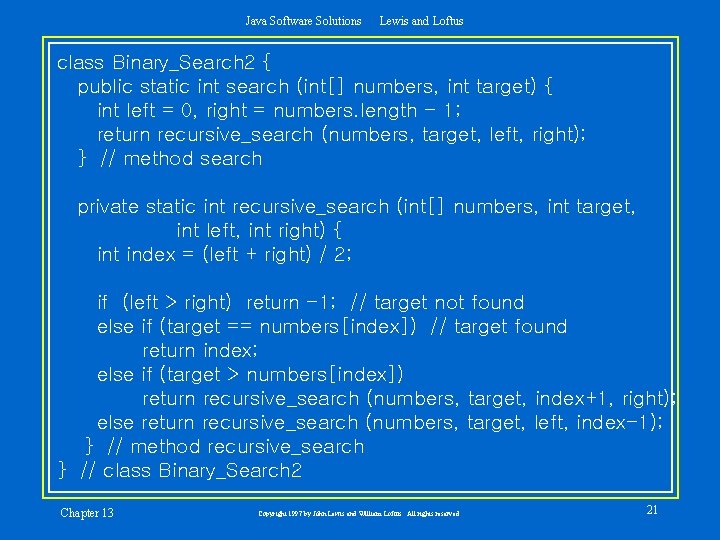
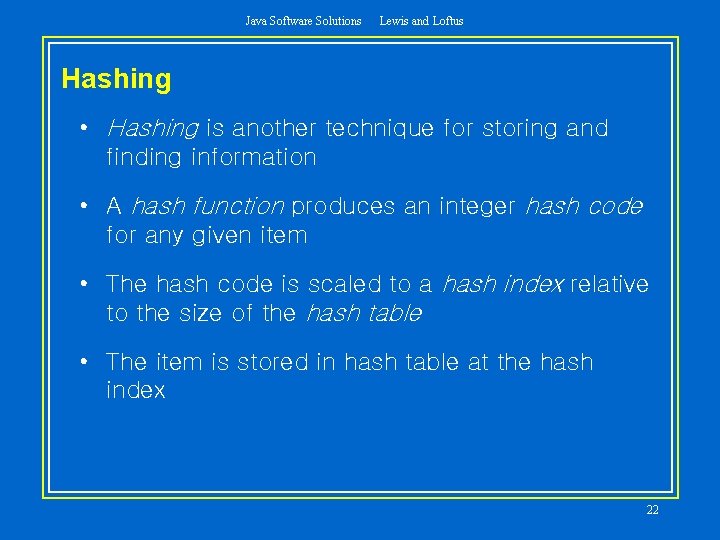
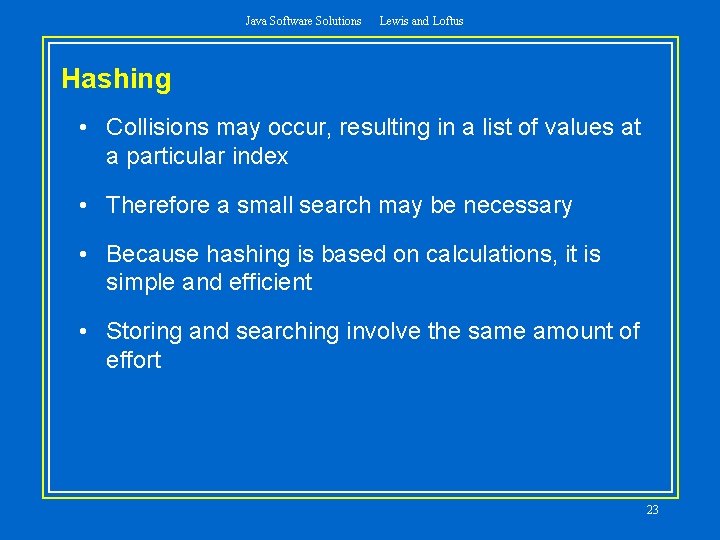
- Slides: 23
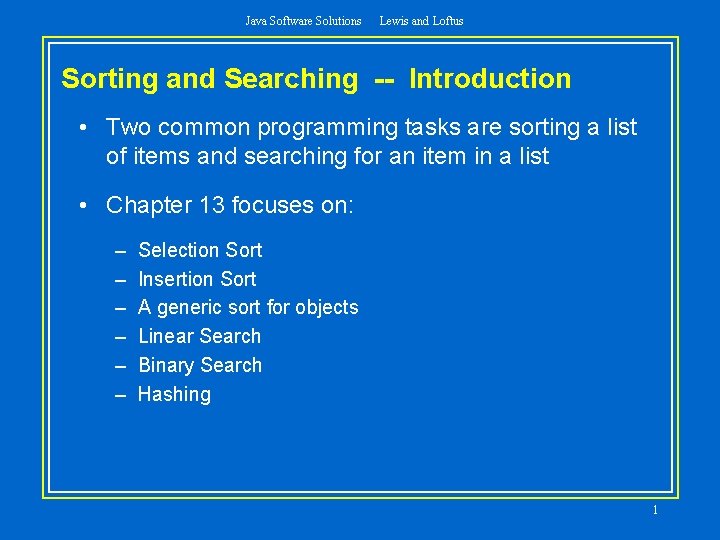
Java Software Solutions Lewis and Loftus Sorting and Searching -- Introduction • Two common programming tasks are sorting a list of items and searching for an item in a list • Chapter 13 focuses on: – – – Selection Sort Insertion Sort A generic sort for objects Linear Search Binary Search Hashing 1
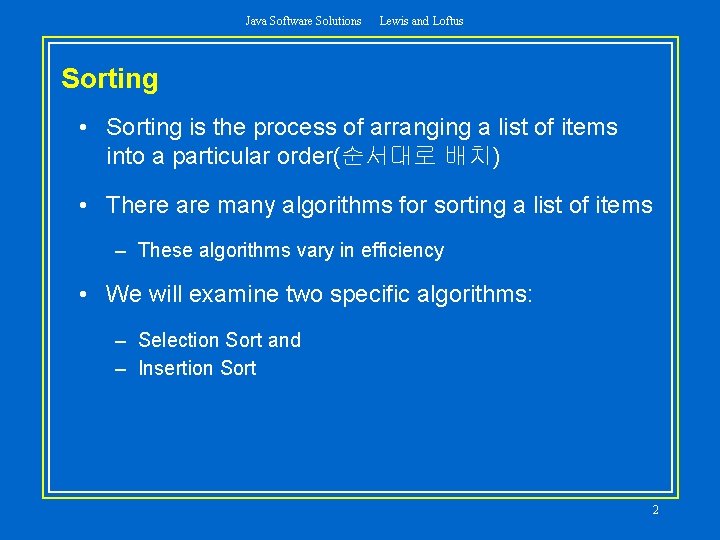
Java Software Solutions Lewis and Loftus Sorting • Sorting is the process of arranging a list of items into a particular order(순서대로 배치) • There are many algorithms for sorting a list of items – These algorithms vary in efficiency • We will examine two specific algorithms: – Selection Sort and – Insertion Sort 2
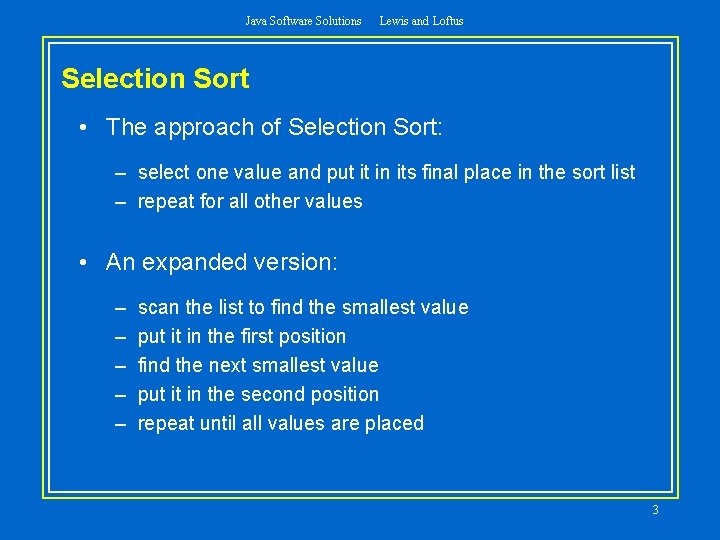
Java Software Solutions Lewis and Loftus Selection Sort • The approach of Selection Sort: – select one value and put it in its final place in the sort list – repeat for all other values • An expanded version: – – – scan the list to find the smallest value put it in the first position find the next smallest value put it in the second position repeat until all values are placed 3
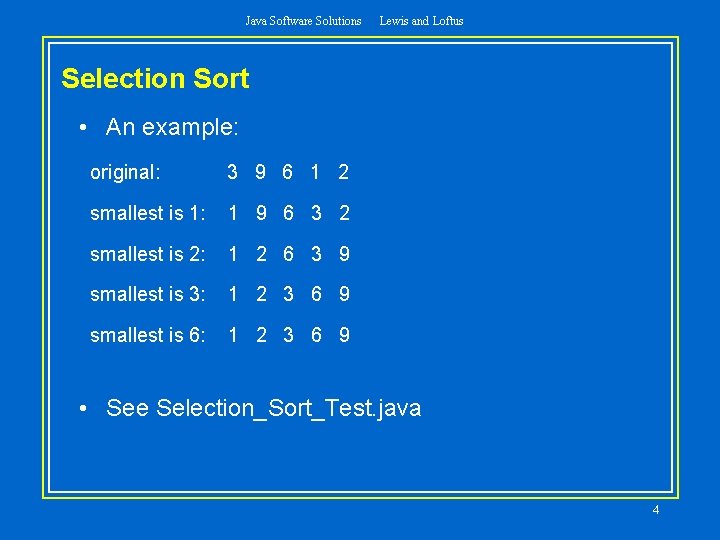
Java Software Solutions Lewis and Loftus Selection Sort • An example: original: 3 9 6 1 2 smallest is 1: 1 9 6 3 2 smallest is 2: 1 2 6 3 9 smallest is 3: 1 2 3 6 9 smallest is 6: 1 2 3 6 9 • See Selection_Sort_Test. java 4
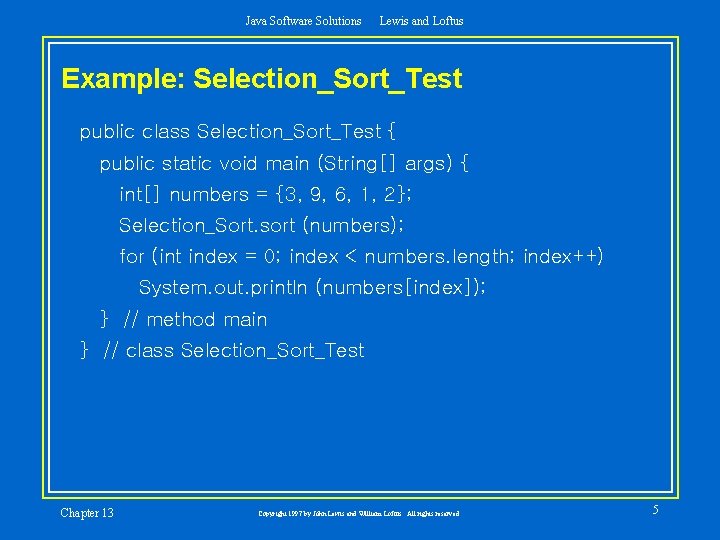
Java Software Solutions Lewis and Loftus Example: Selection_Sort_Test public class Selection_Sort_Test { public static void main (String[] args) { int[] numbers = {3, 9, 6, 1, 2}; Selection_Sort. sort (numbers); for (int index = 0; index < numbers. length; index++) System. out. println (numbers[index]); } // method main } // class Selection_Sort_Test Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 5
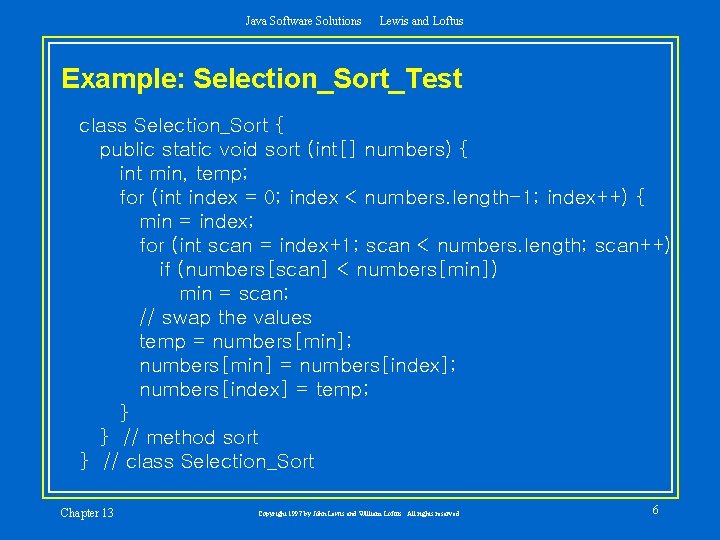
Java Software Solutions Lewis and Loftus Example: Selection_Sort_Test class Selection_Sort { public static void sort (int[] numbers) { int min, temp; for (int index = 0; index < numbers. length-1; index++) { min = index; for (int scan = index+1; scan < numbers. length; scan++) if (numbers[scan] < numbers[min]) min = scan; // swap the values temp = numbers[min]; numbers[min] = numbers[index]; numbers[index] = temp; } } // method sort } // class Selection_Sort Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 6
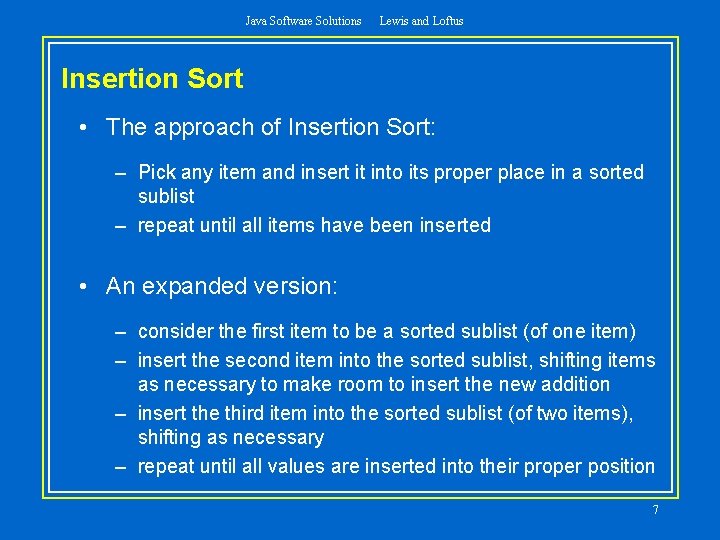
Java Software Solutions Lewis and Loftus Insertion Sort • The approach of Insertion Sort: – Pick any item and insert it into its proper place in a sorted sublist – repeat until all items have been inserted • An expanded version: – consider the first item to be a sorted sublist (of one item) – insert the second item into the sorted sublist, shifting items as necessary to make room to insert the new addition – insert the third item into the sorted sublist (of two items), shifting as necessary – repeat until all values are inserted into their proper position 7
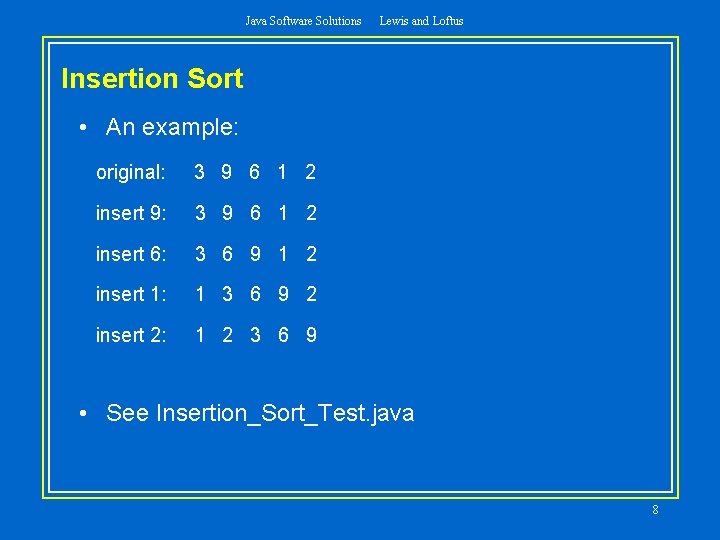
Java Software Solutions Lewis and Loftus Insertion Sort • An example: original: 3 9 6 1 2 insert 9: 3 9 6 1 2 insert 6: 3 6 9 1 2 insert 1: 1 3 6 9 2 insert 2: 1 2 3 6 9 • See Insertion_Sort_Test. java 8
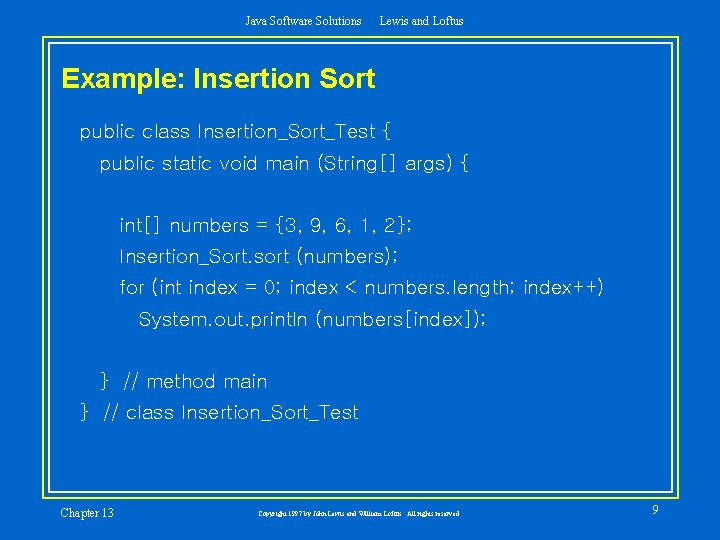
Java Software Solutions Lewis and Loftus Example: Insertion Sort public class Insertion_Sort_Test { public static void main (String[] args) { int[] numbers = {3, 9, 6, 1, 2}; Insertion_Sort. sort (numbers); for (int index = 0; index < numbers. length; index++) System. out. println (numbers[index]); } // method main } // class Insertion_Sort_Test Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 9
![Java Software Solutions Lewis and Loftus class InsertionSort public static void sort int Java Software Solutions Lewis and Loftus class Insertion_Sort { public static void sort (int[]](https://slidetodoc.com/presentation_image_h2/979f140e40379a9469950391d7909862/image-10.jpg)
Java Software Solutions Lewis and Loftus class Insertion_Sort { public static void sort (int[] numbers) { for (int index = 1; index < numbers. length; index++) { int key = numbers[index]; int position = index; // shift larger values to the right while (position > 0 && numbers[position-1] > key) { numbers[position] = numbers[position-1]; position--; } numbers[position] = key; } } // method sort } // class Insertion_Sort Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 10
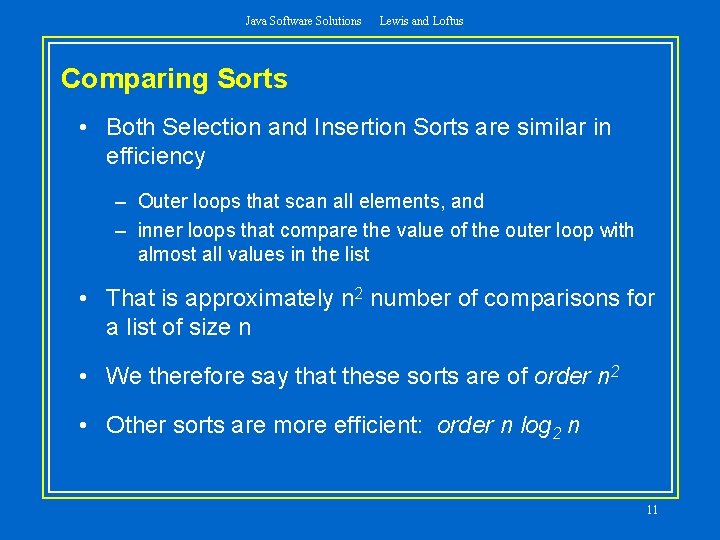
Java Software Solutions Lewis and Loftus Comparing Sorts • Both Selection and Insertion Sorts are similar in efficiency – Outer loops that scan all elements, and – inner loops that compare the value of the outer loop with almost all values in the list • That is approximately n 2 number of comparisons for a list of size n • We therefore say that these sorts are of order n 2 • Other sorts are more efficient: order n log 2 n 11
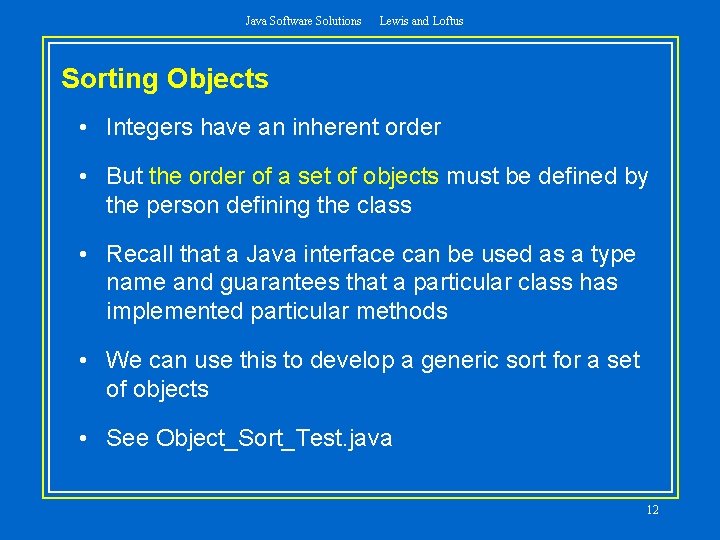
Java Software Solutions Lewis and Loftus Sorting Objects • Integers have an inherent order • But the order of a set of objects must be defined by the person defining the class • Recall that a Java interface can be used as a type name and guarantees that a particular class has implemented particular methods • We can use this to develop a generic sort for a set of objects • See Object_Sort_Test. java 12
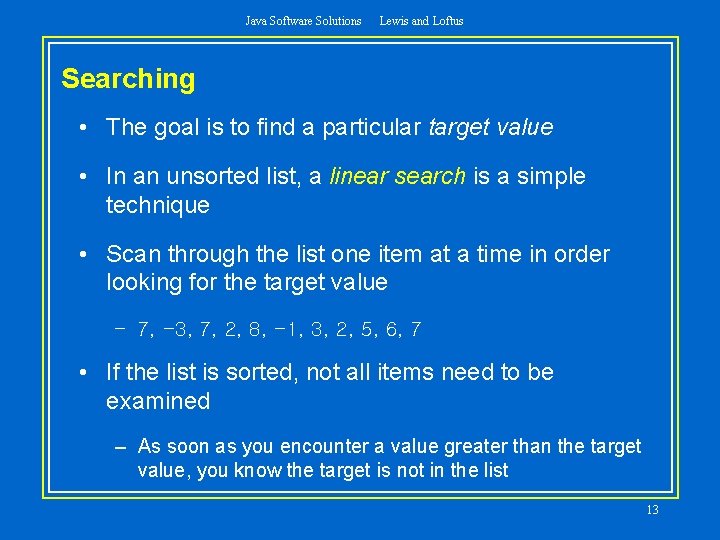
Java Software Solutions Lewis and Loftus Searching • The goal is to find a particular target value • In an unsorted list, a linear search is a simple technique • Scan through the list one item at a time in order looking for the target value – 7, -3, 7, 2, 8, -1, 3, 2, 5, 6, 7 • If the list is sorted, not all items need to be examined – As soon as you encounter a value greater than the target value, you know the target is not in the list 13
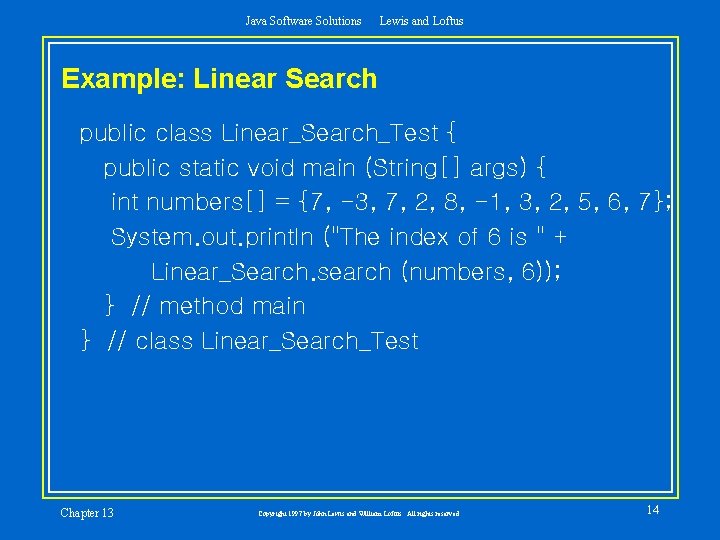
Java Software Solutions Lewis and Loftus Example: Linear Search public class Linear_Search_Test { public static void main (String[] args) { int numbers[] = {7, -3, 7, 2, 8, -1, 3, 2, 5, 6, 7}; System. out. println ("The index of 6 is " + Linear_Search. search (numbers, 6)); } // method main } // class Linear_Search_Test Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 14
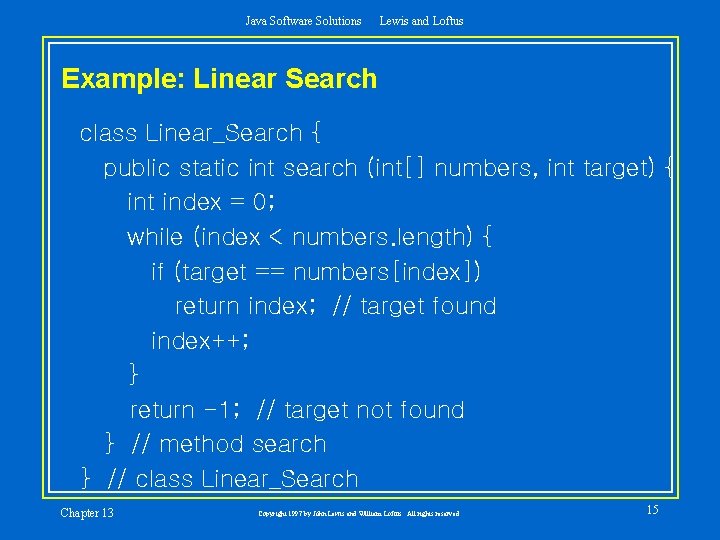
Java Software Solutions Lewis and Loftus Example: Linear Search class Linear_Search { public static int search (int[] numbers, int target) { int index = 0; while (index < numbers. length) { if (target == numbers[index]) return index; // target found index++; } return -1; // target not found } // method search } // class Linear_Search Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 15
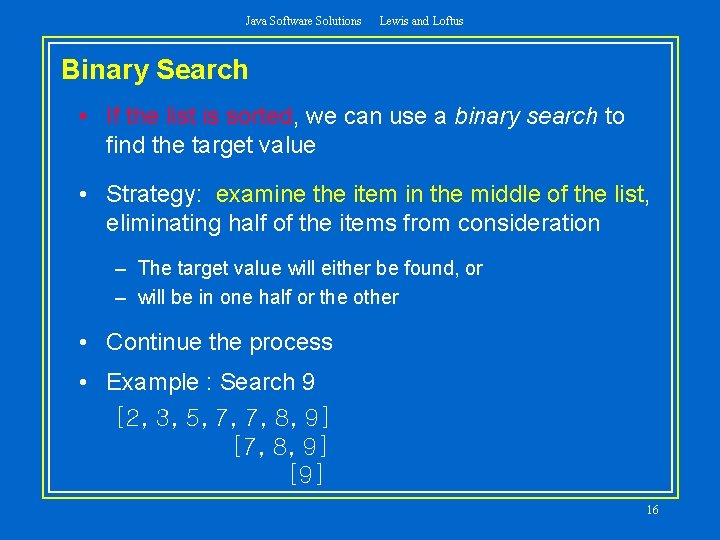
Java Software Solutions Lewis and Loftus Binary Search • If the list is sorted, we can use a binary search to find the target value • Strategy: examine the item in the middle of the list, eliminating half of the items from consideration – The target value will either be found, or – will be in one half or the other • Continue the process • Example : Search 9 [2, 3, 5, 7, 7, 8, 9] [9] 16
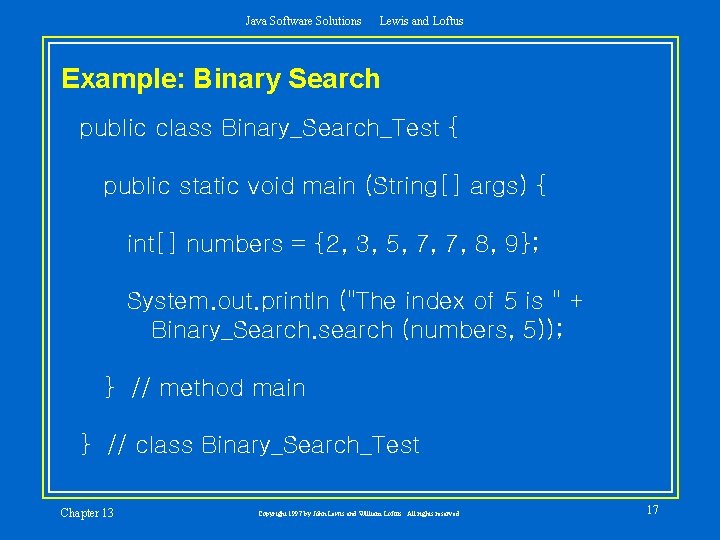
Java Software Solutions Lewis and Loftus Example: Binary Search public class Binary_Search_Test { public static void main (String[] args) { int[] numbers = {2, 3, 5, 7, 7, 8, 9}; System. out. println ("The index of 5 is " + Binary_Search. search (numbers, 5)); } // method main } // class Binary_Search_Test Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 17
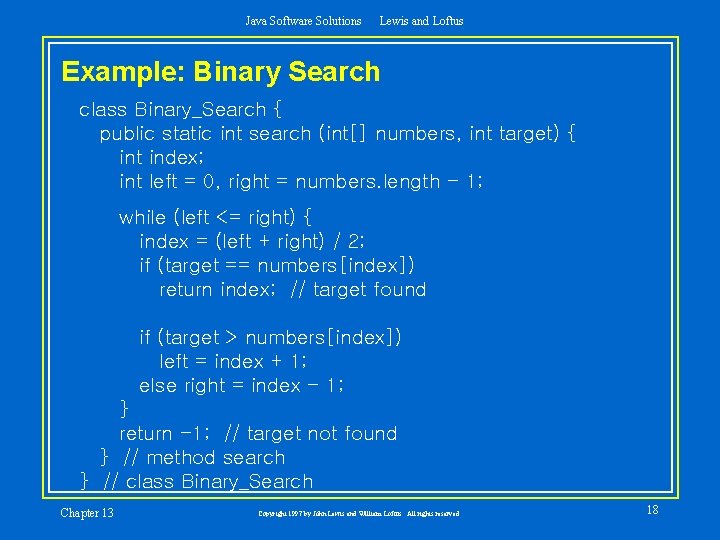
Java Software Solutions Lewis and Loftus Example: Binary Search class Binary_Search { public static int search (int[] numbers, int target) { int index; int left = 0, right = numbers. length - 1; while (left <= right) { index = (left + right) / 2; if (target == numbers[index]) return index; // target found if (target > numbers[index]) left = index + 1; else right = index - 1; } return -1; // target not found } // method search } // class Binary_Search Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 18
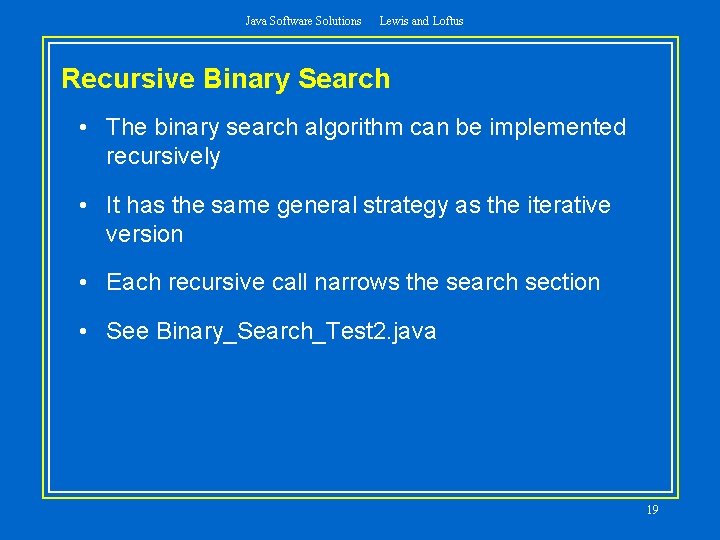
Java Software Solutions Lewis and Loftus Recursive Binary Search • The binary search algorithm can be implemented recursively • It has the same general strategy as the iterative version • Each recursive call narrows the search section • See Binary_Search_Test 2. java 19
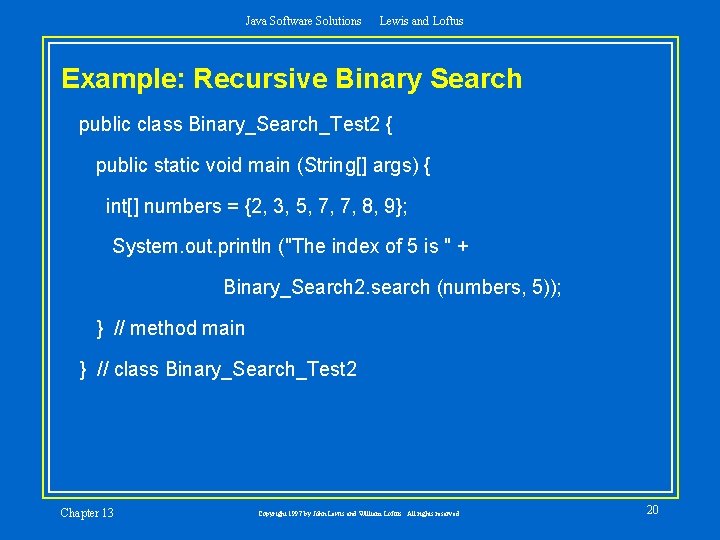
Java Software Solutions Lewis and Loftus Example: Recursive Binary Search public class Binary_Search_Test 2 { public static void main (String[] args) { int[] numbers = {2, 3, 5, 7, 7, 8, 9}; System. out. println ("The index of 5 is " + Binary_Search 2. search (numbers, 5)); } // method main } // class Binary_Search_Test 2 Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 20
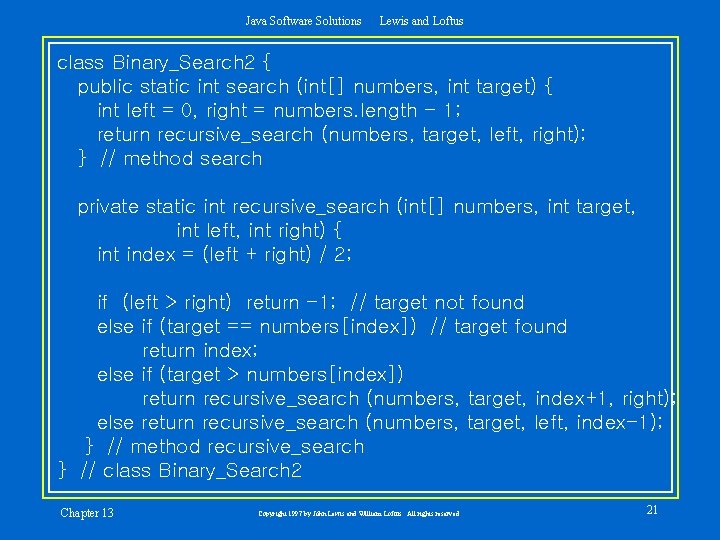
Java Software Solutions Lewis and Loftus class Binary_Search 2 { public static int search (int[] numbers, int target) { int left = 0, right = numbers. length - 1; return recursive_search (numbers, target, left, right); } // method search private static int recursive_search (int[] numbers, int target, int left, int right) { int index = (left + right) / 2; if (left > right) return -1; // target not found else if (target == numbers[index]) // target found return index; else if (target > numbers[index]) return recursive_search (numbers, target, index+1, right); else return recursive_search (numbers, target, left, index-1); } // method recursive_search } // class Binary_Search 2 Chapter 13 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 21
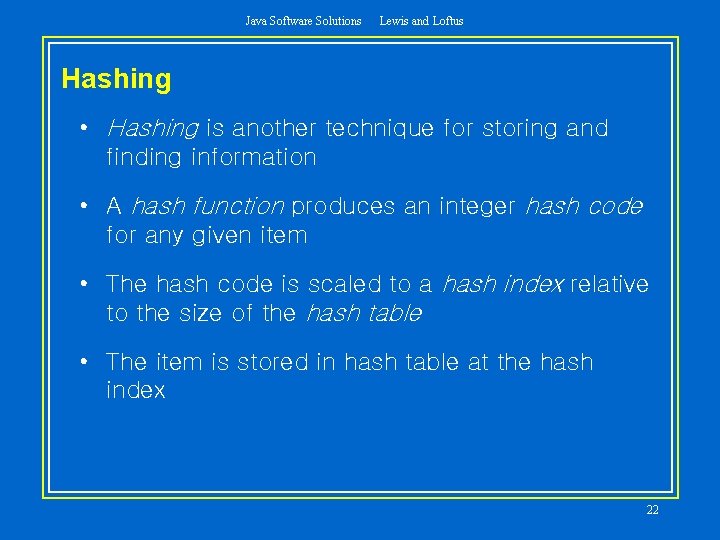
Java Software Solutions Lewis and Loftus Hashing • Hashing is another technique for storing and finding information • A hash function produces an integer hash code for any given item • The hash code is scaled to a hash index relative to the size of the hash table • The item is stored in hash table at the hash index 22
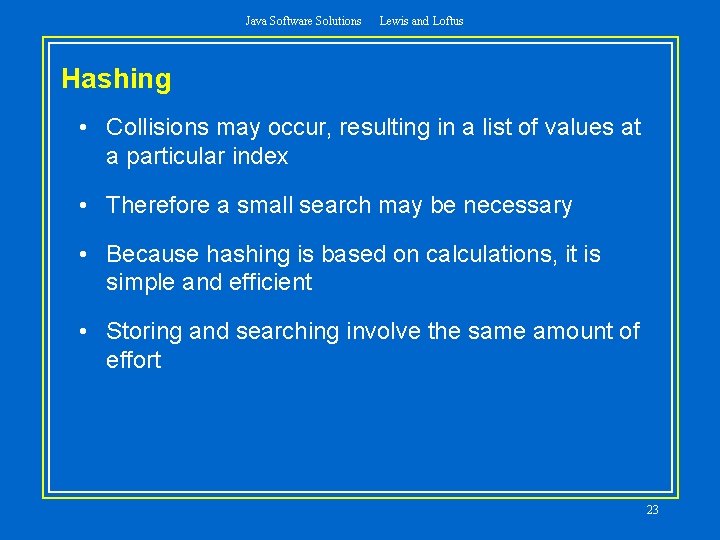
Java Software Solutions Lewis and Loftus Hashing • Collisions may occur, resulting in a list of values at a particular index • Therefore a small search may be necessary • Because hashing is based on calculations, it is simple and efficient • Storing and searching involve the same amount of effort 23
Difference between external and internal sorting
Loftus and palmer (1974)
Searching and sorting in java
Searching and sorting in java
Searching and sorting in java
Searching and sorting in java
Elizabeth loftus biography
Elizabeth loftus
Loftus experiment
Misattribution psychology
Java
Loftus
Judith loftus huck finn
Sorting and grading difference
Intracellular compartments and protein sorting
Selection sort vs bubble sort
Searching and sorting arrays in c++
Restricting and sorting data in oracle
Grading of food
Lesson 2 assignment a sort of sorts answers
Physical and chemical properties sorting activity
Java swing form example
Import java.util.*
Import java.awt