Java Software Solutions Lewis and Loftus Java Language
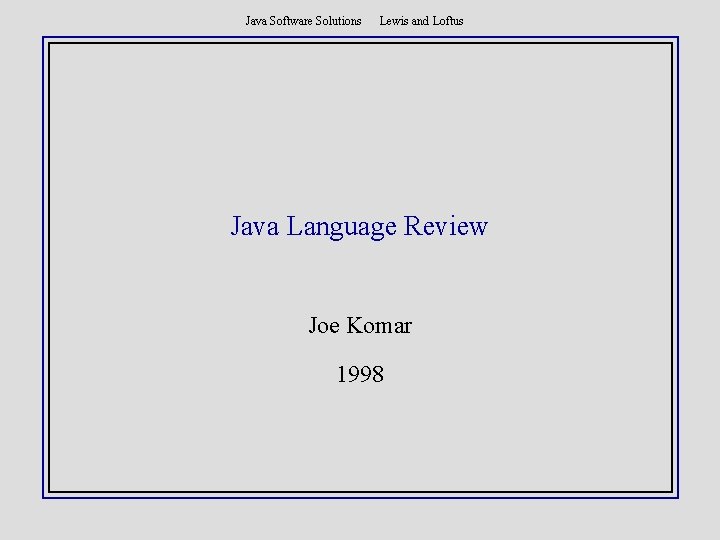
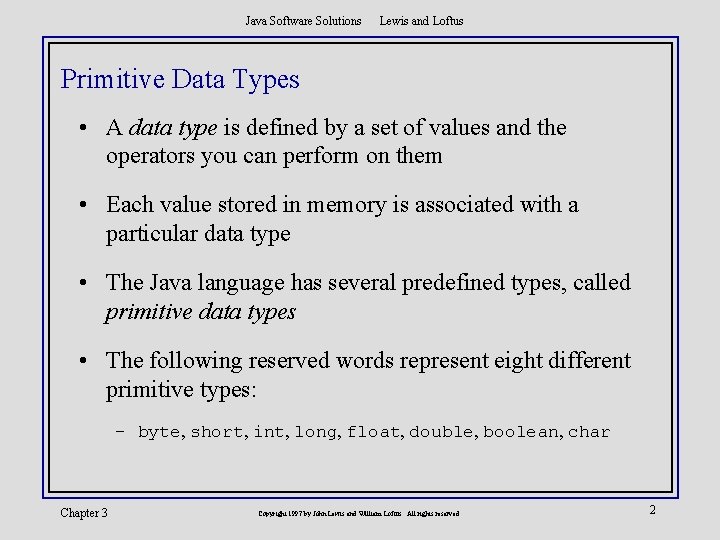
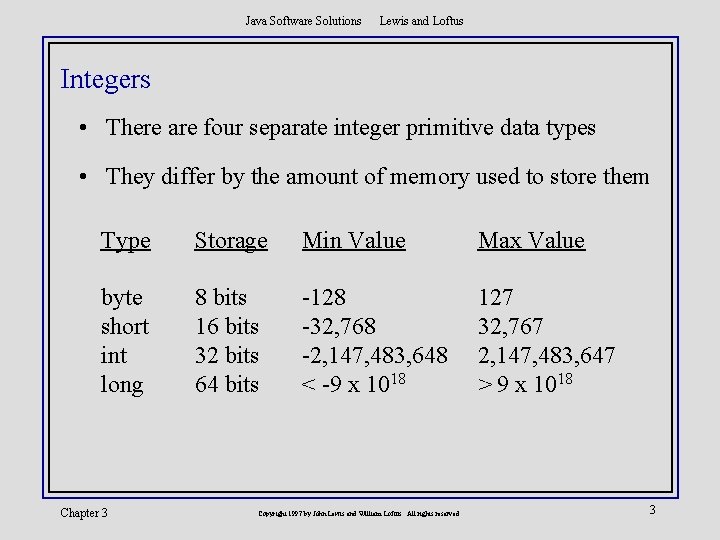
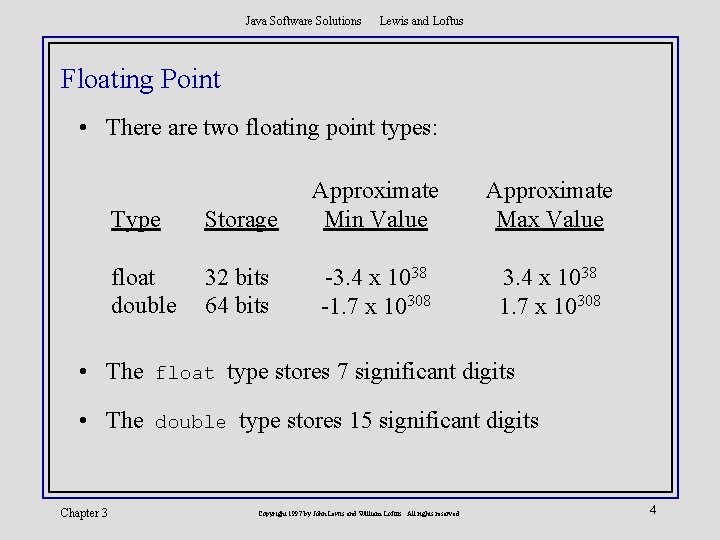
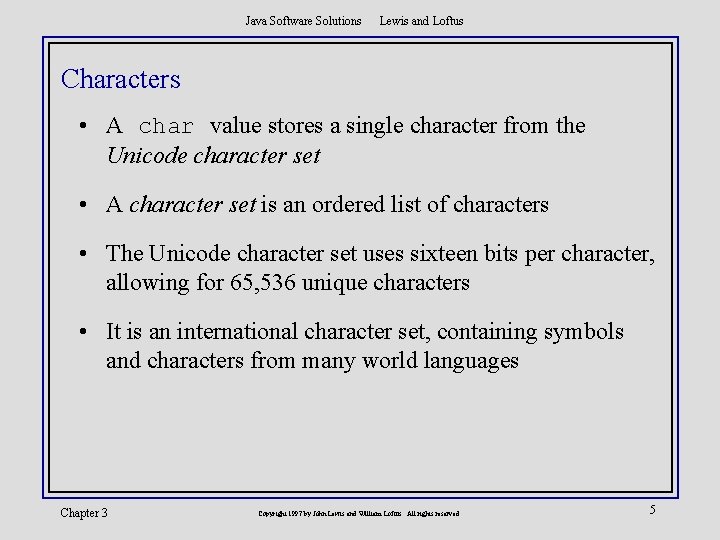
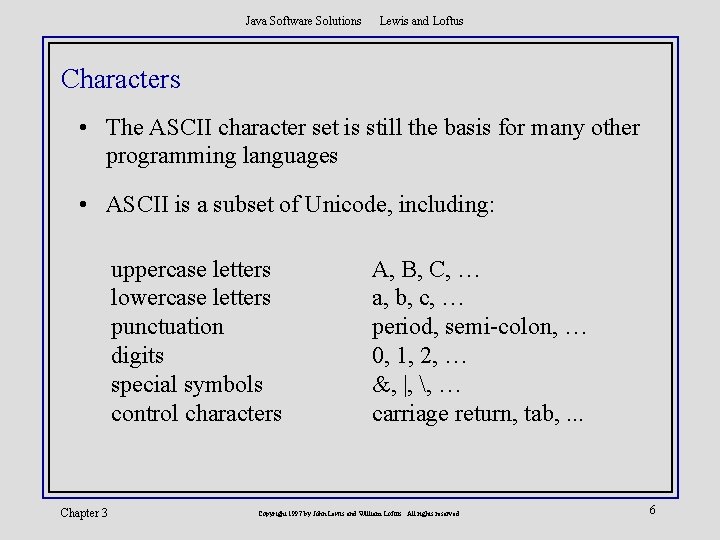
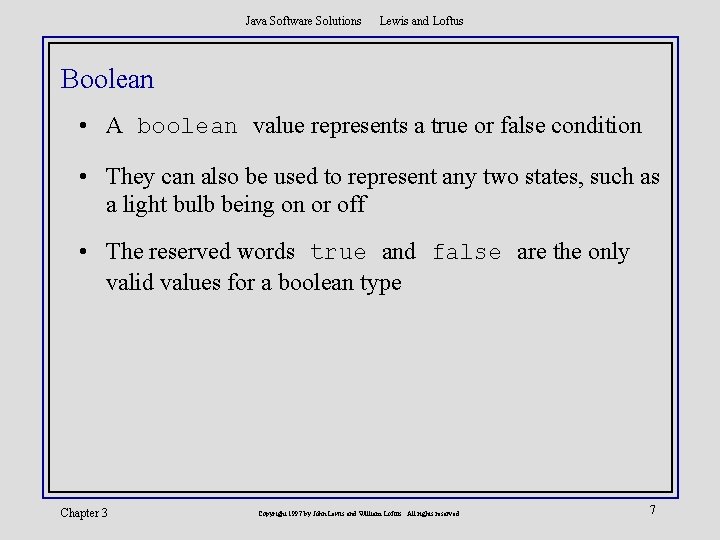
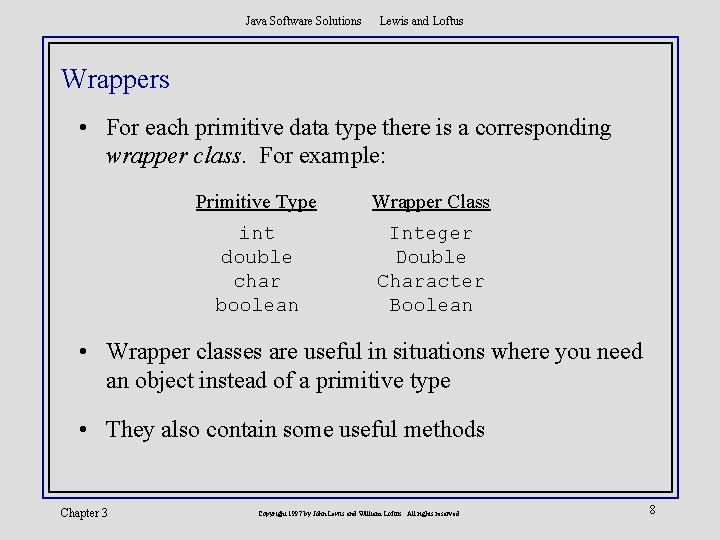
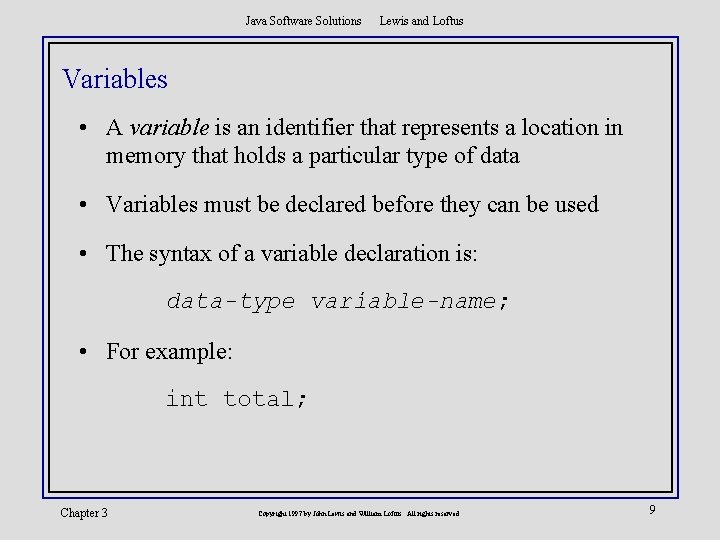
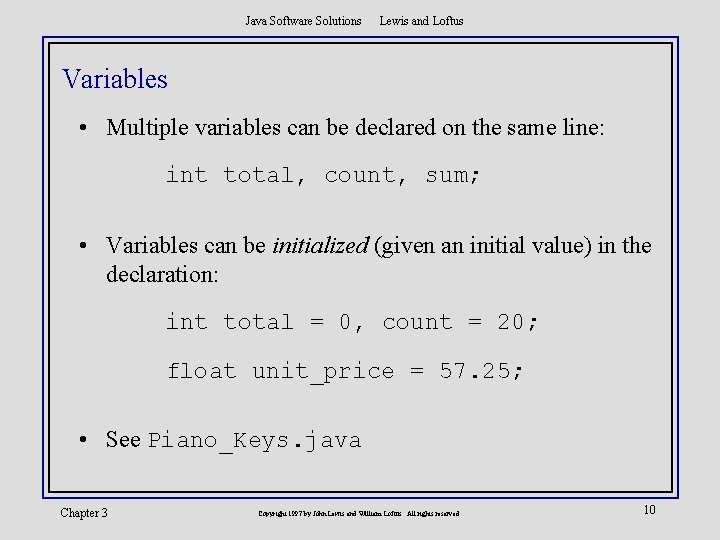
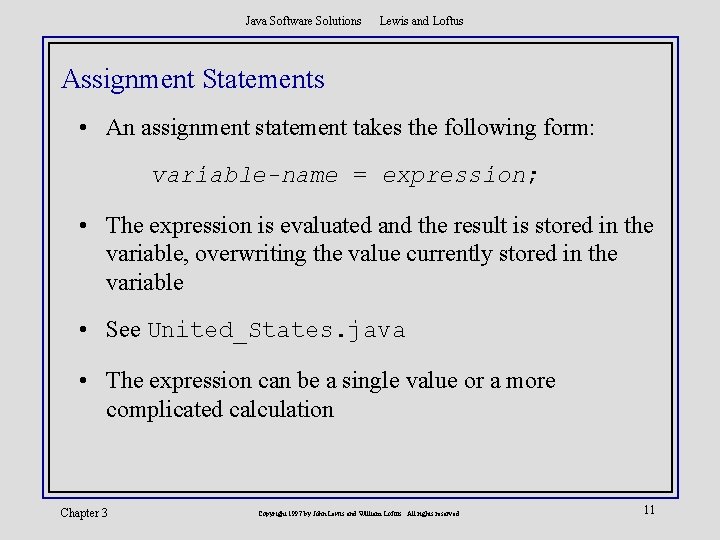
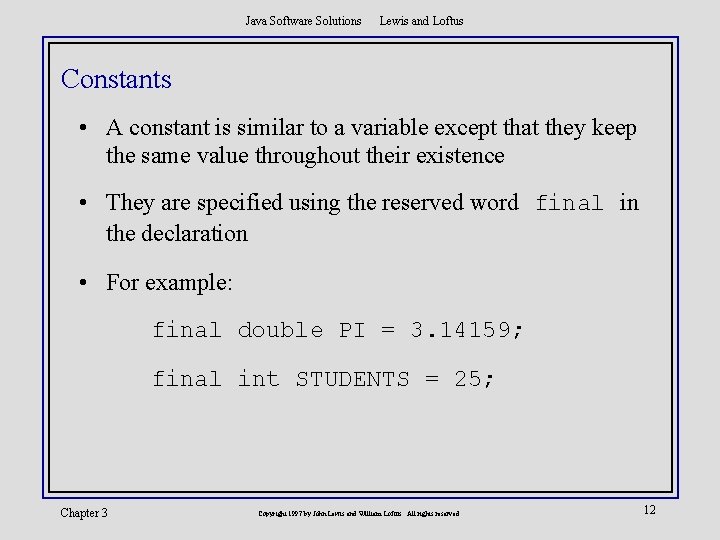
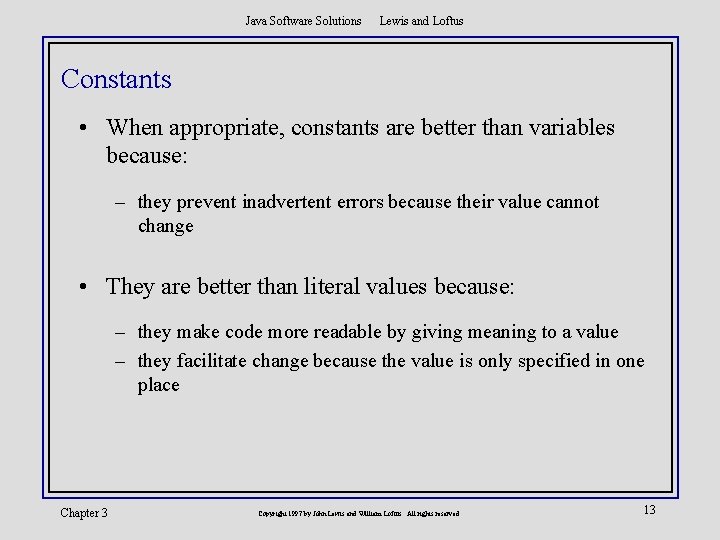
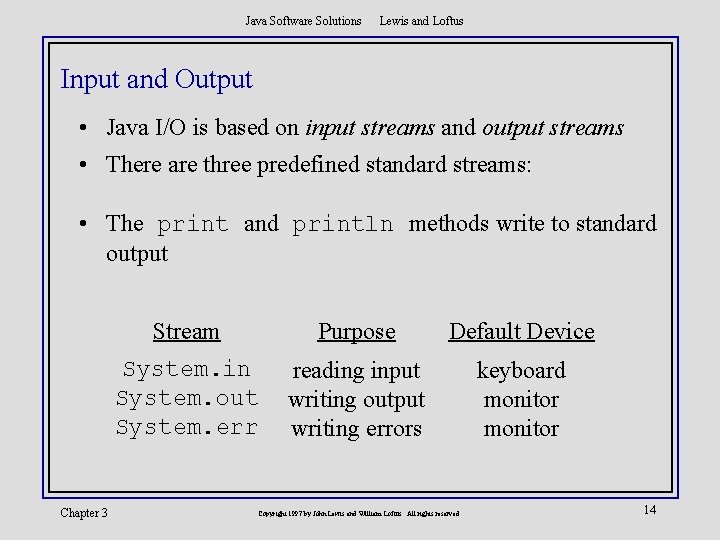
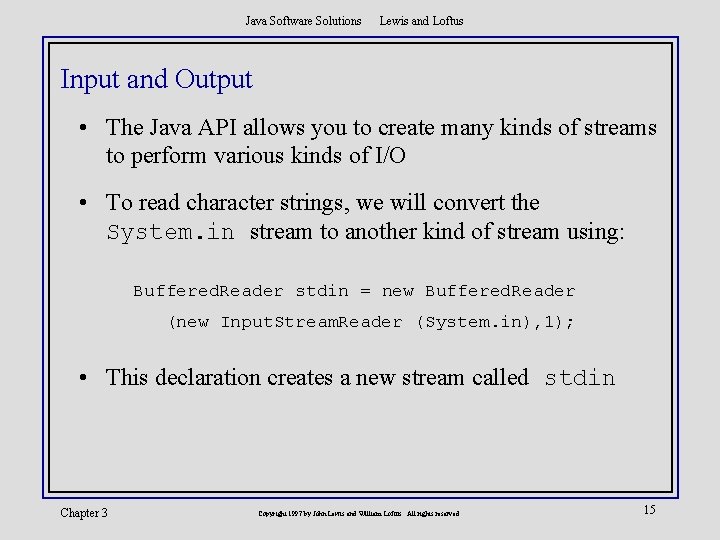
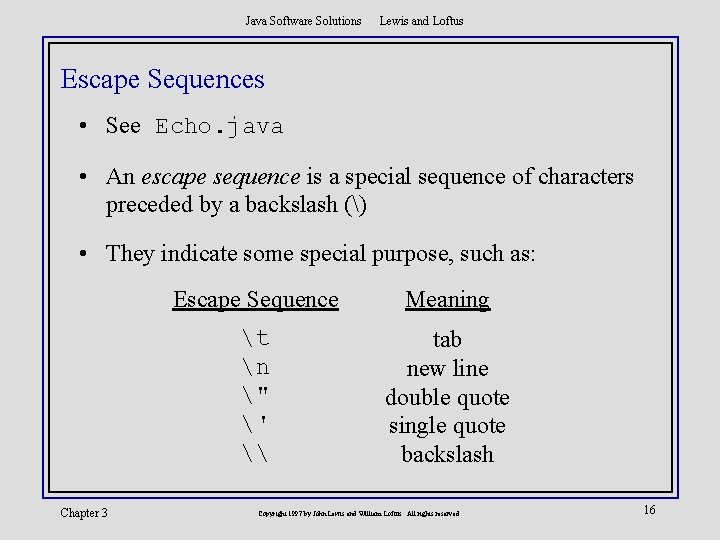
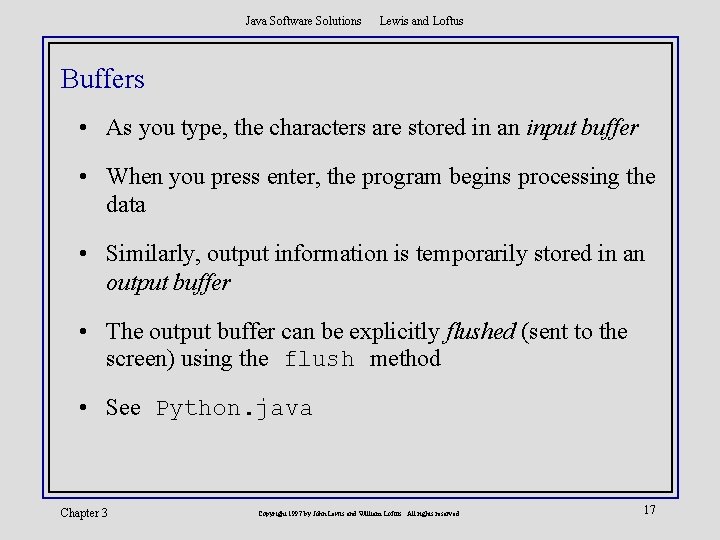
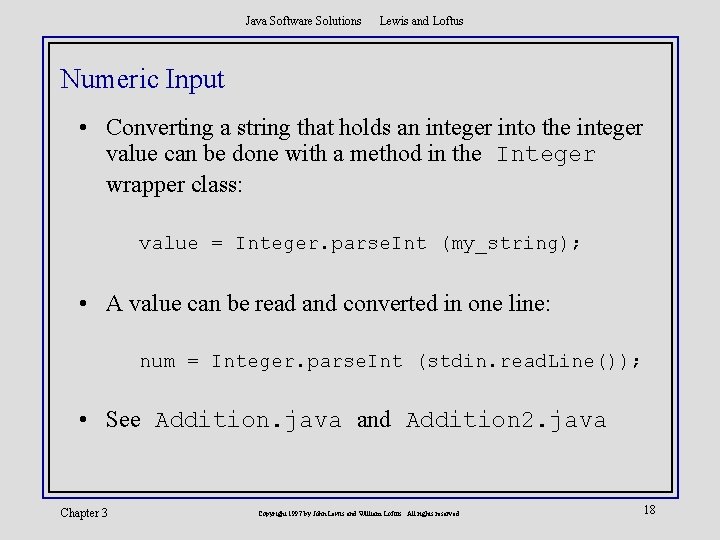
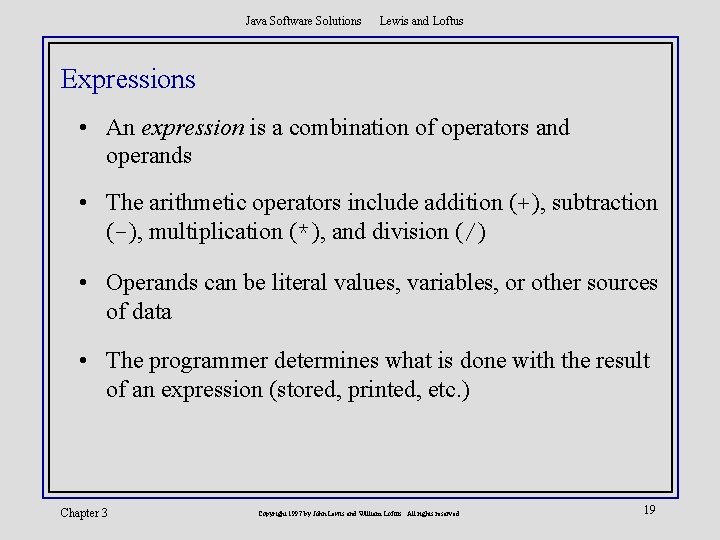
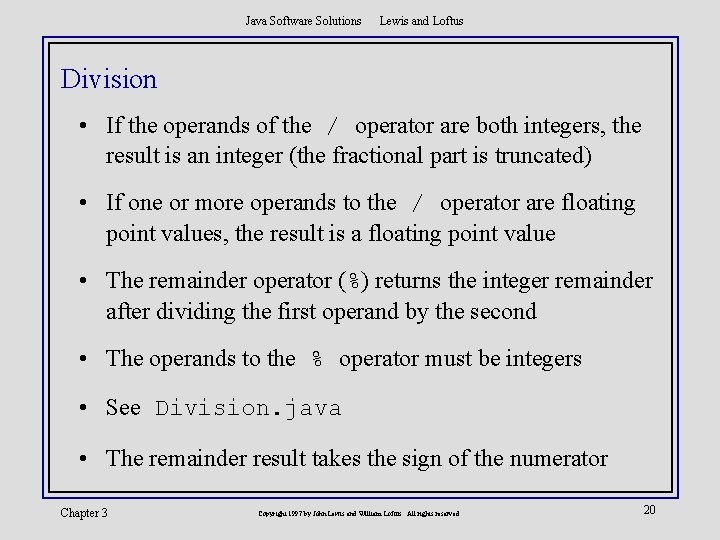
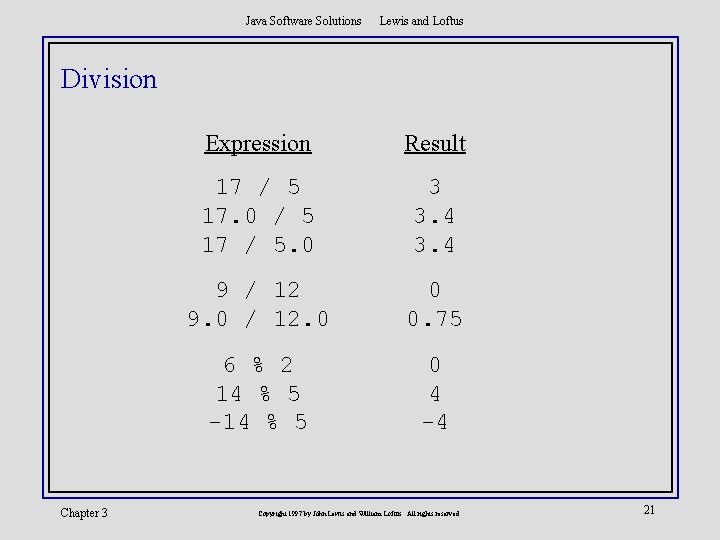
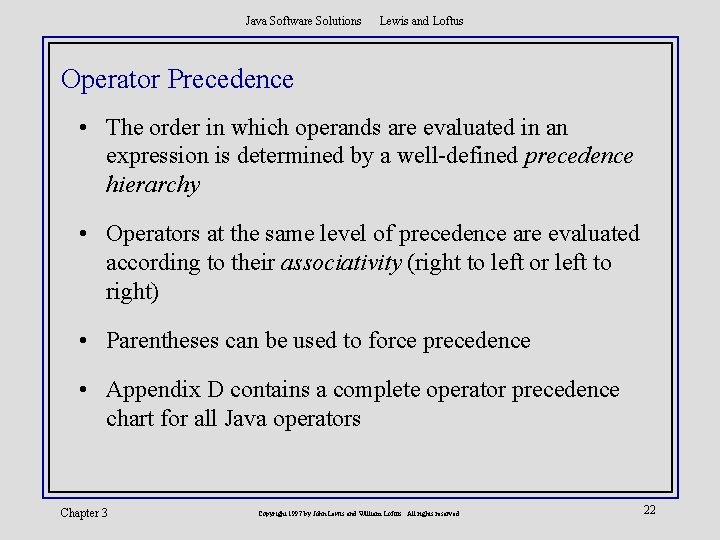
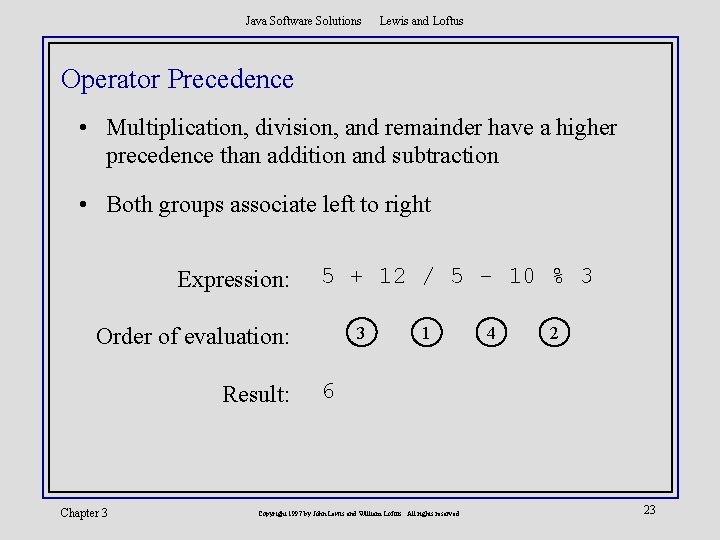
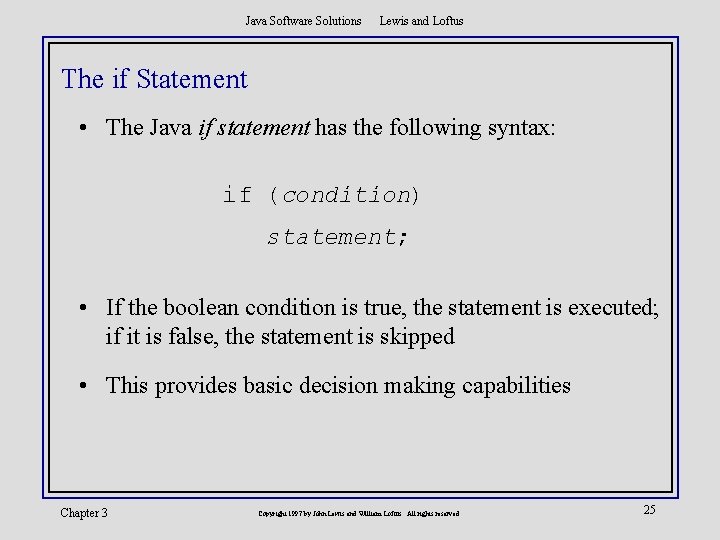
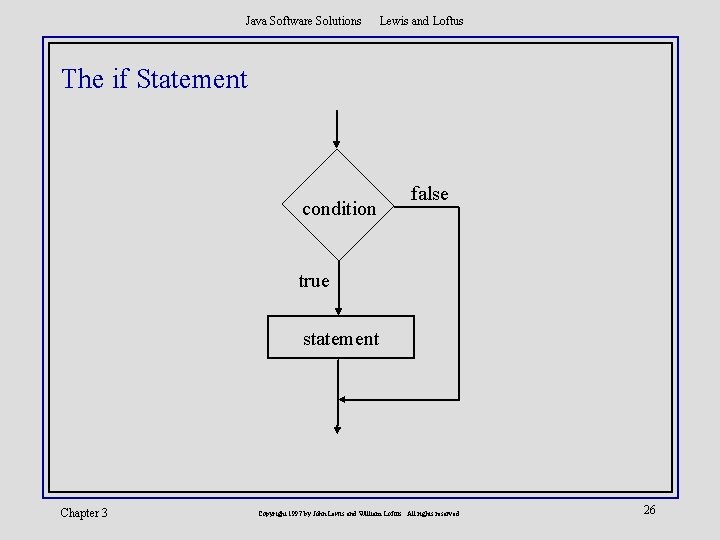
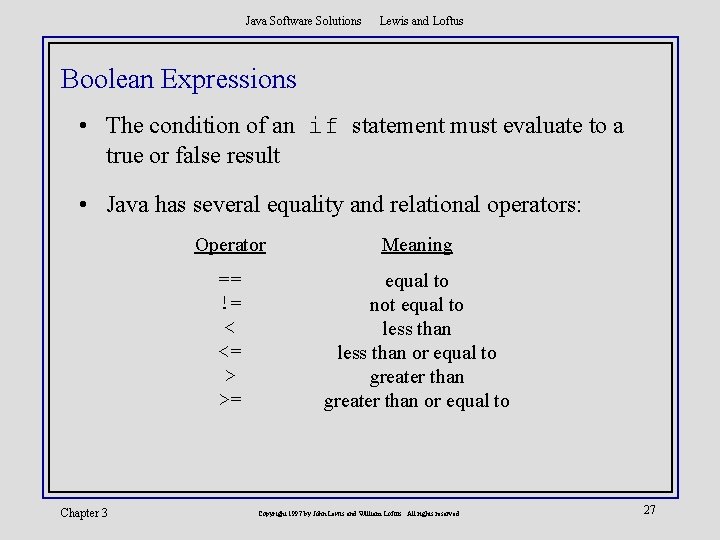
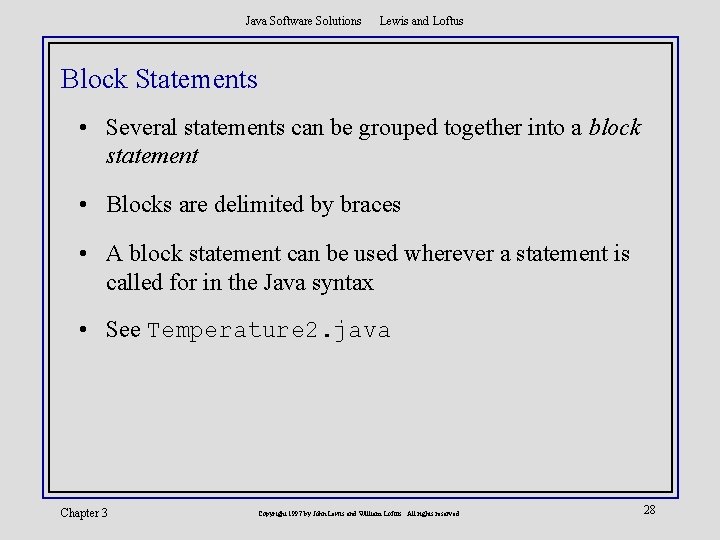
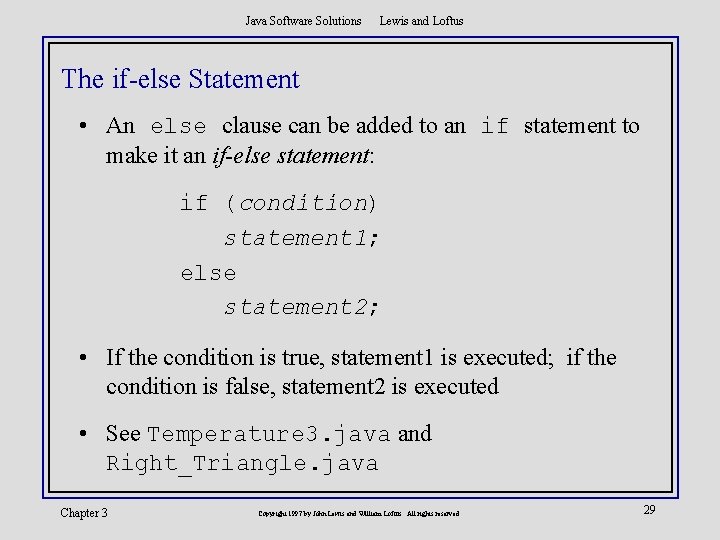
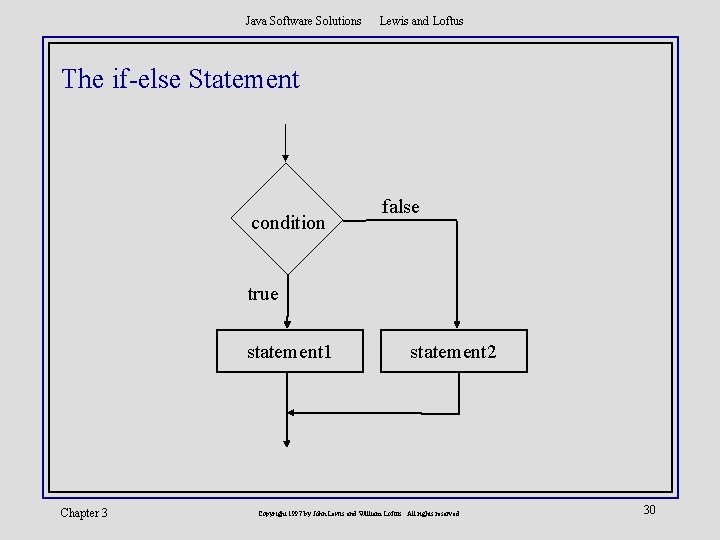
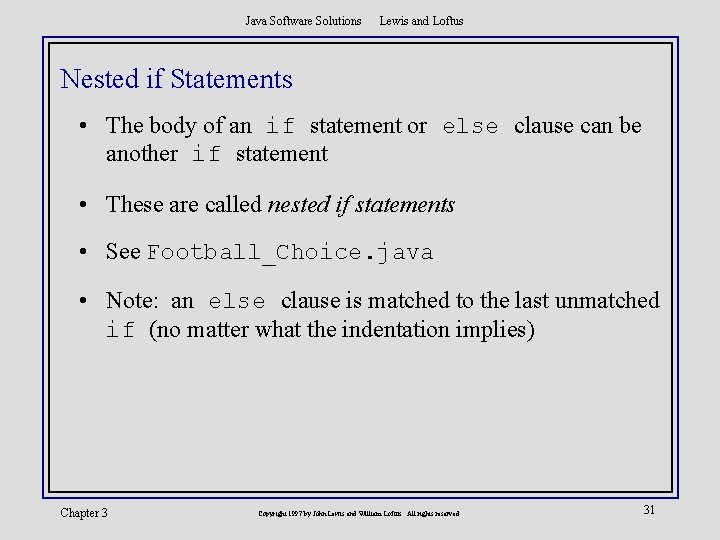
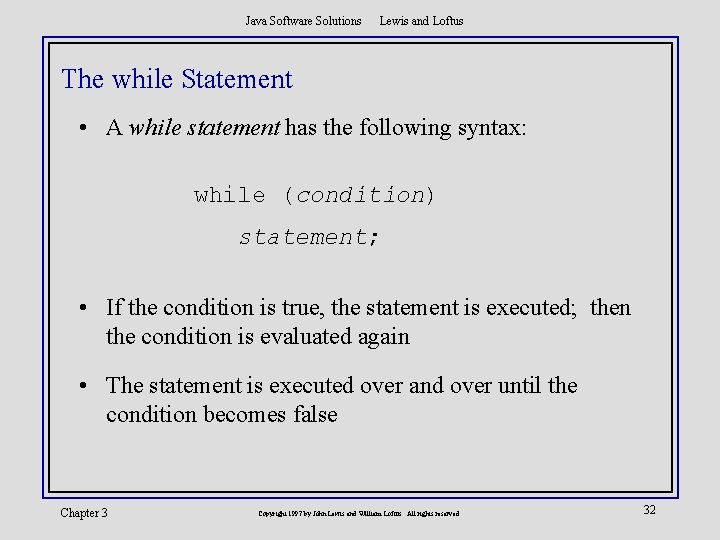
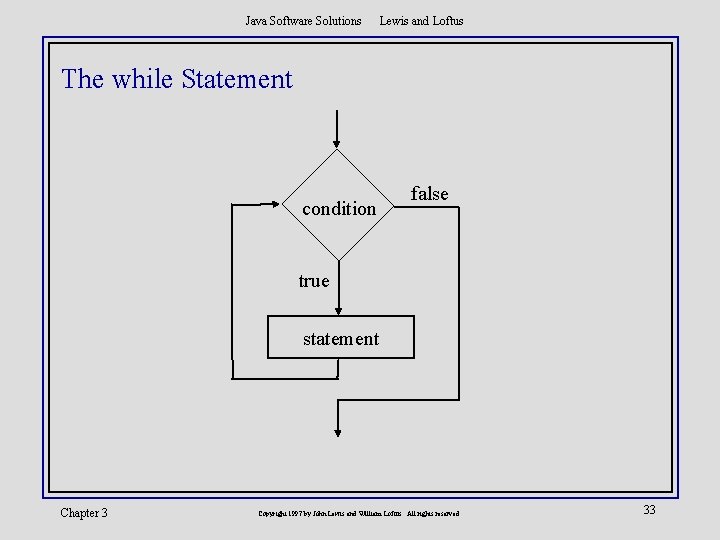
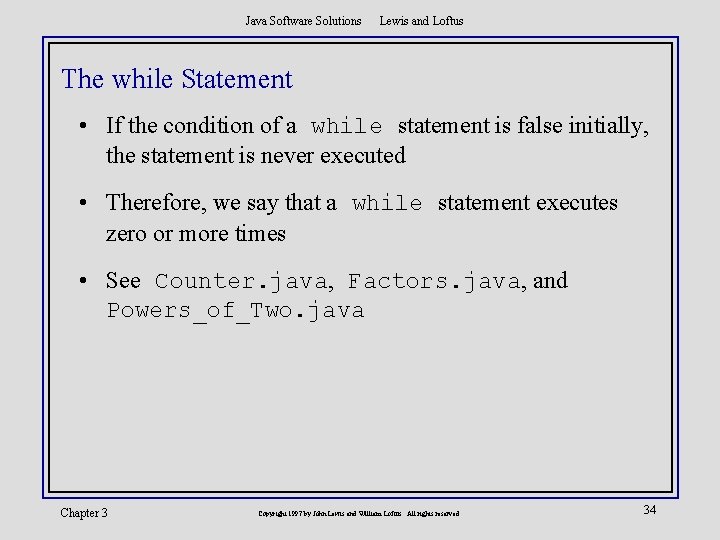
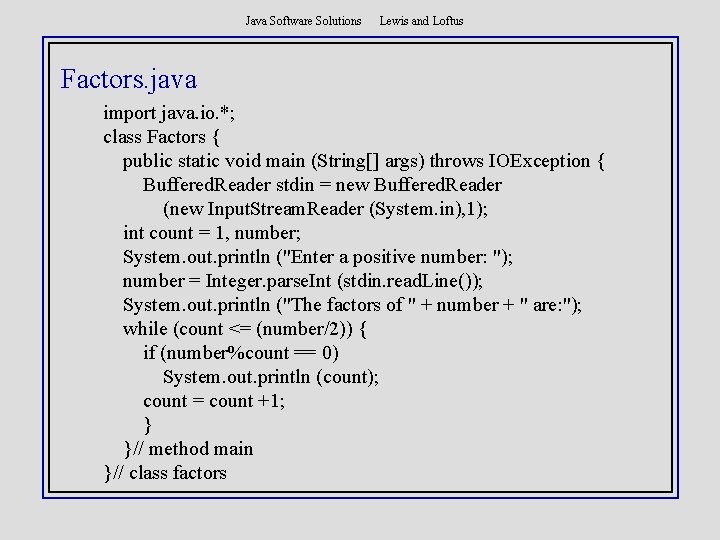
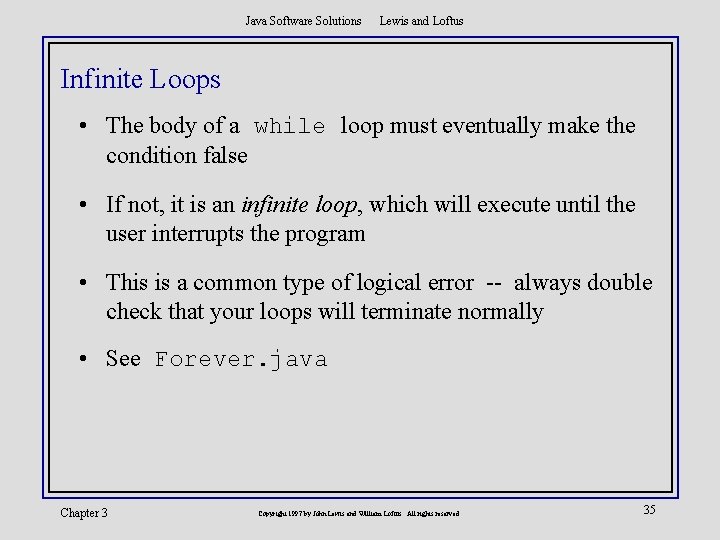
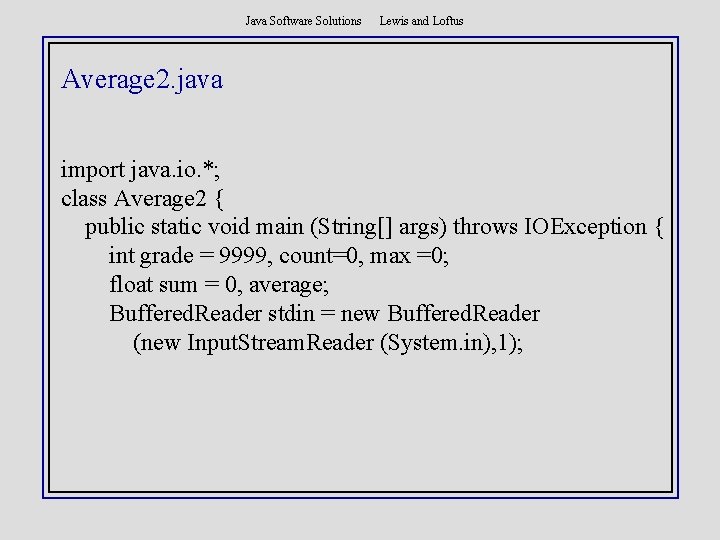
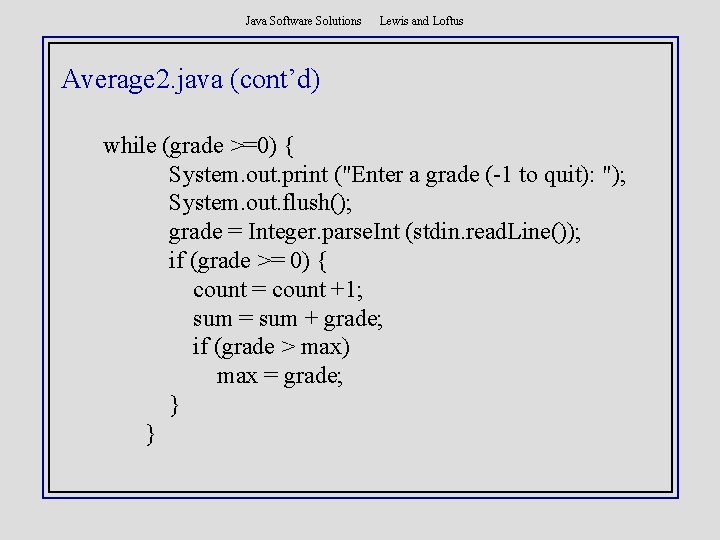
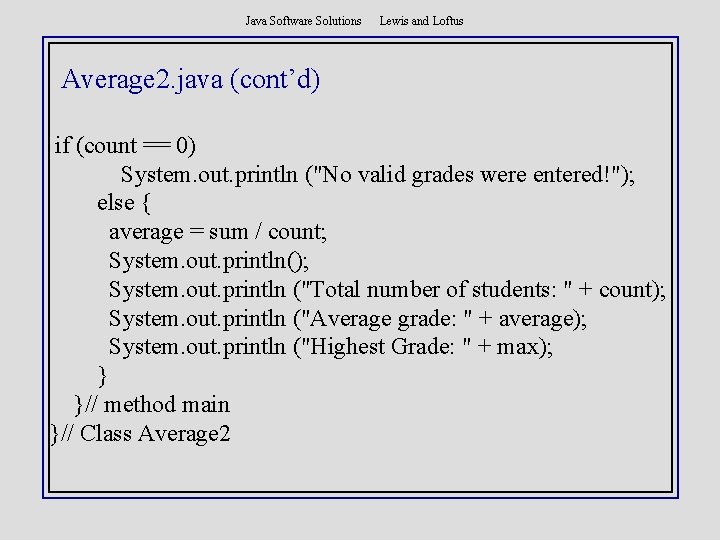
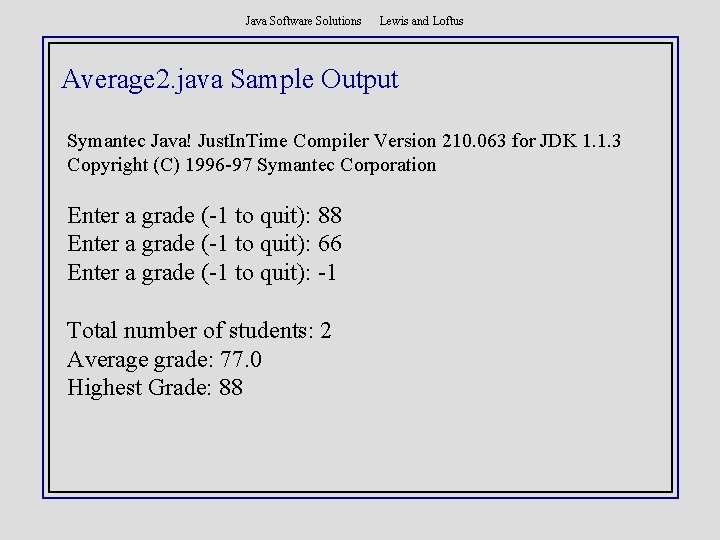
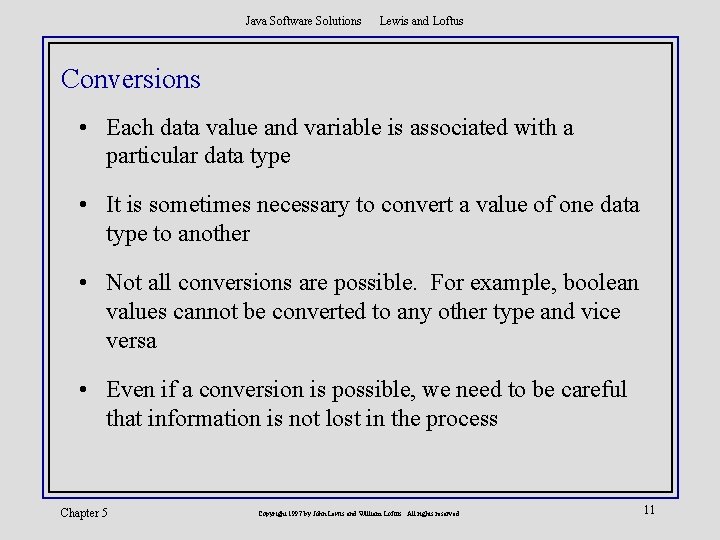
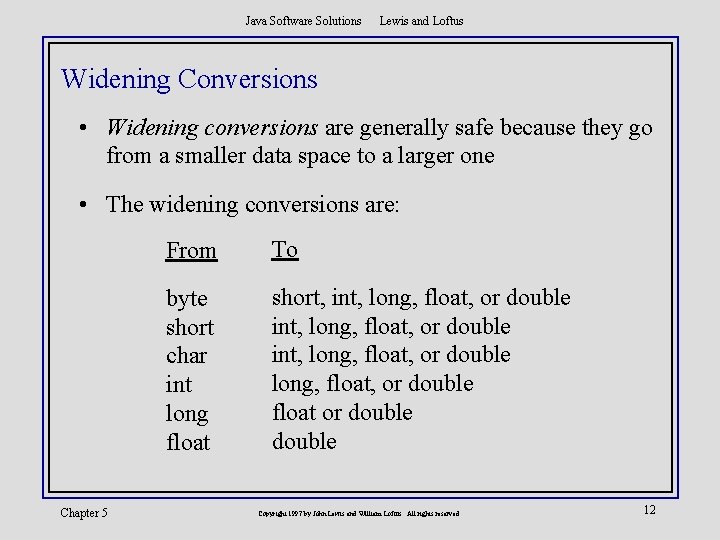
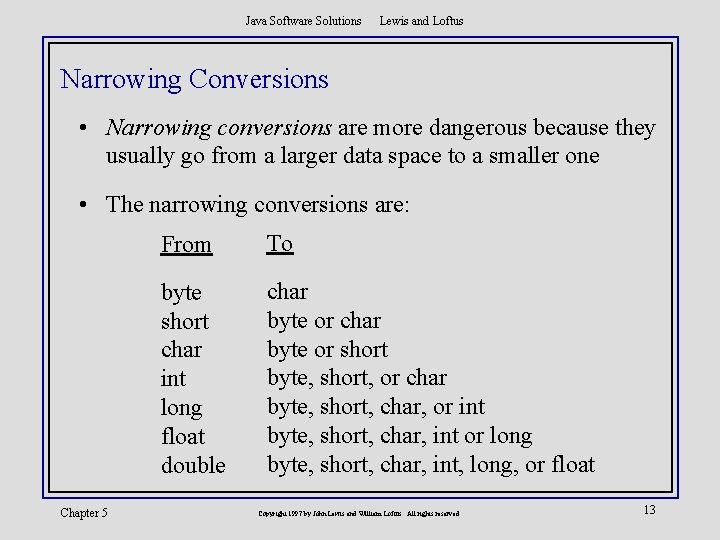
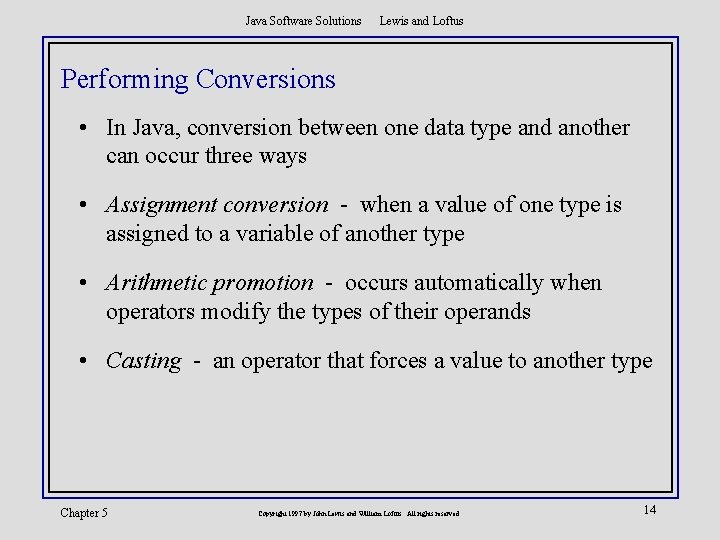
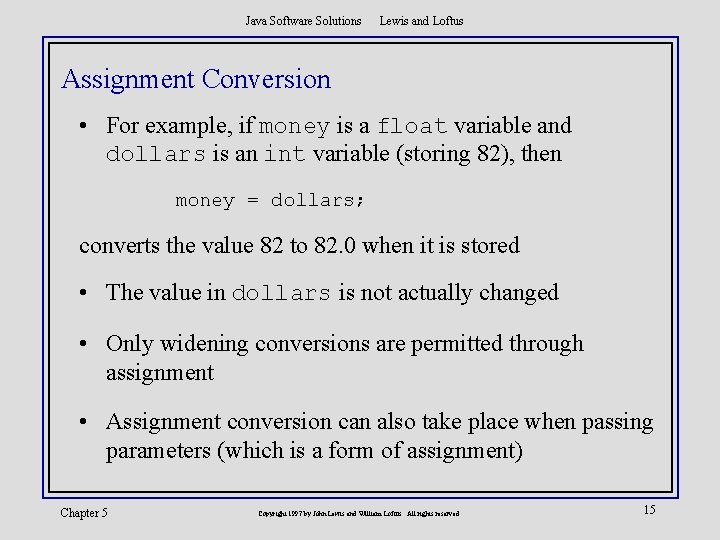
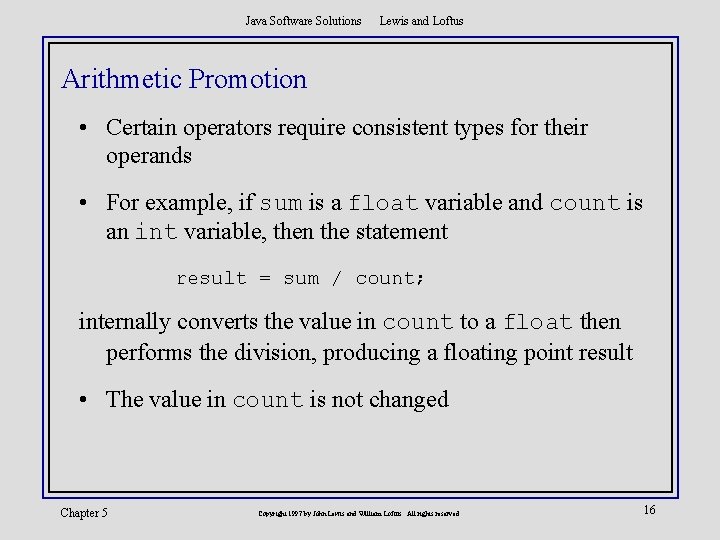
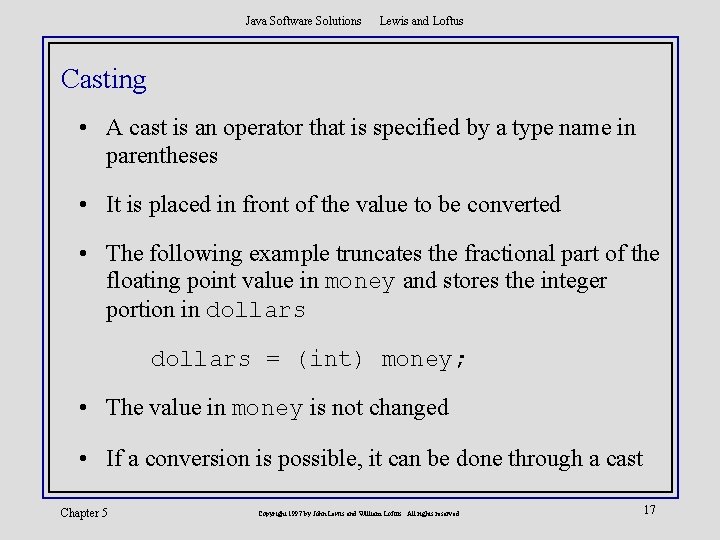
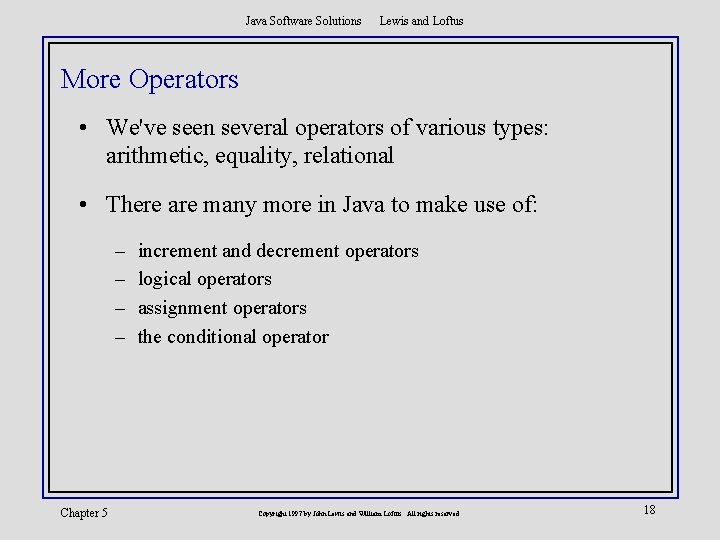
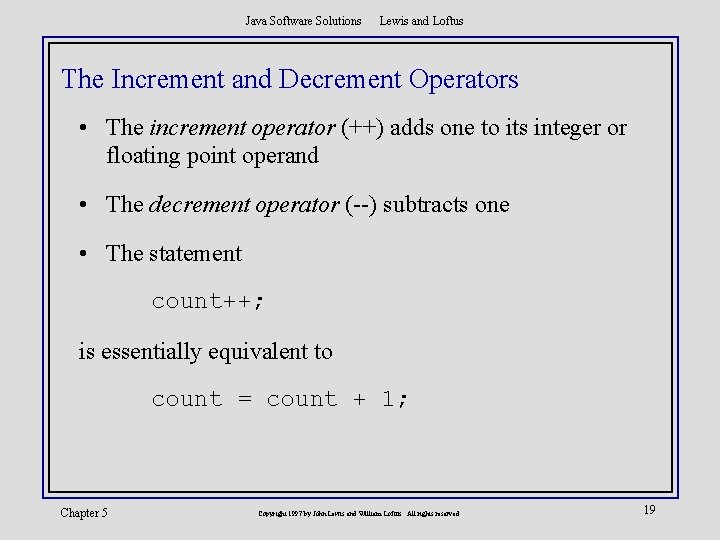
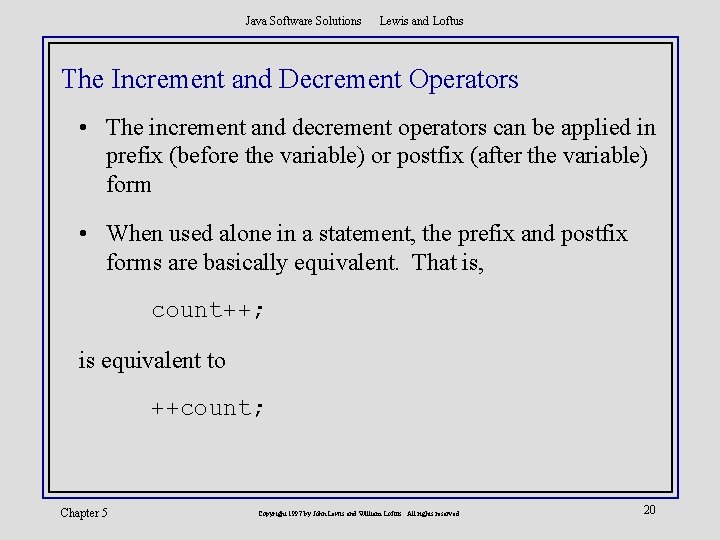
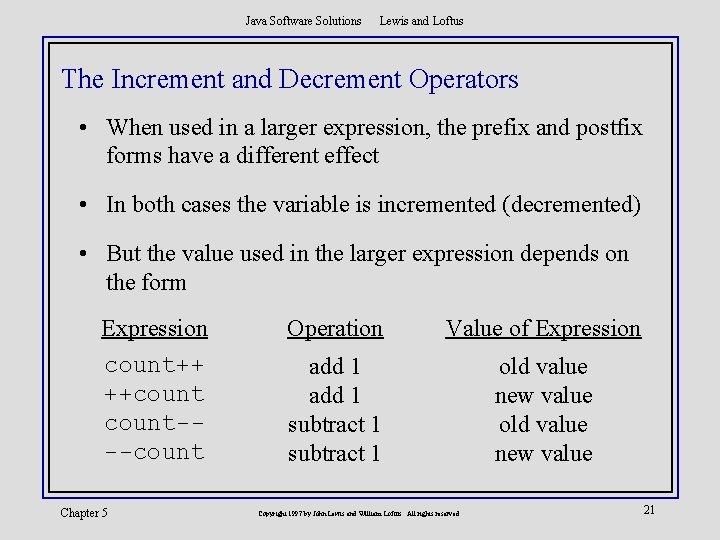
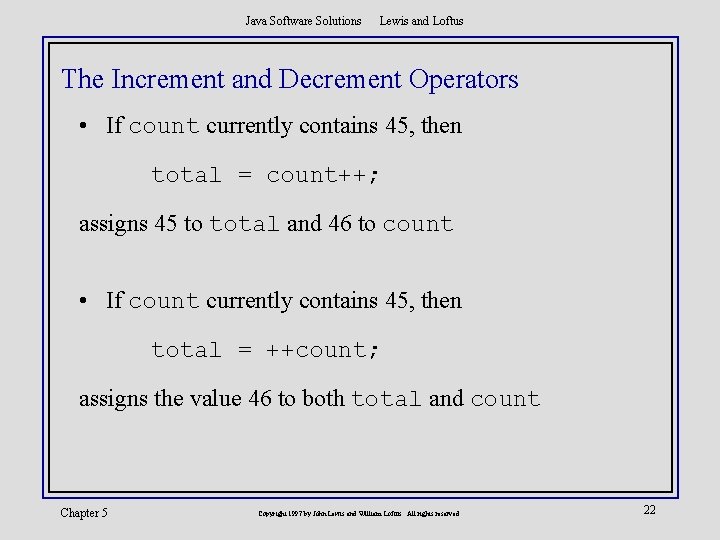
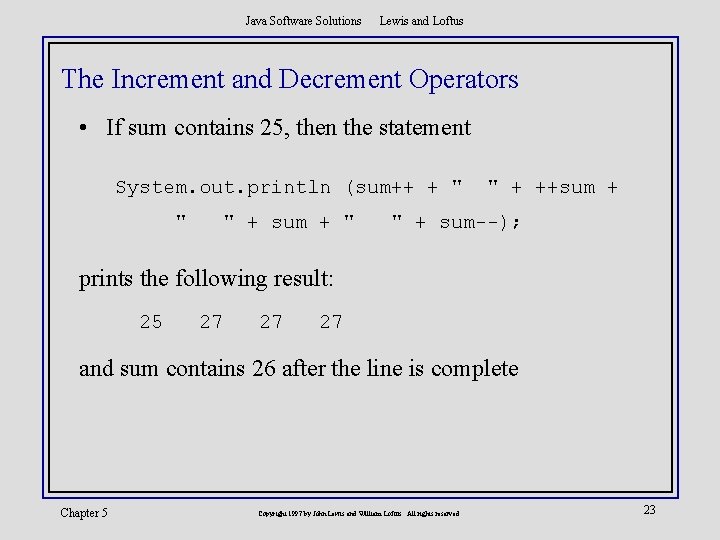
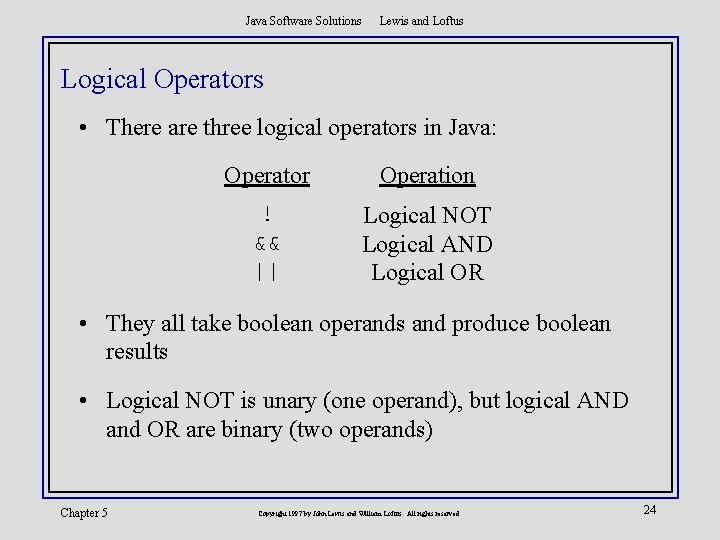
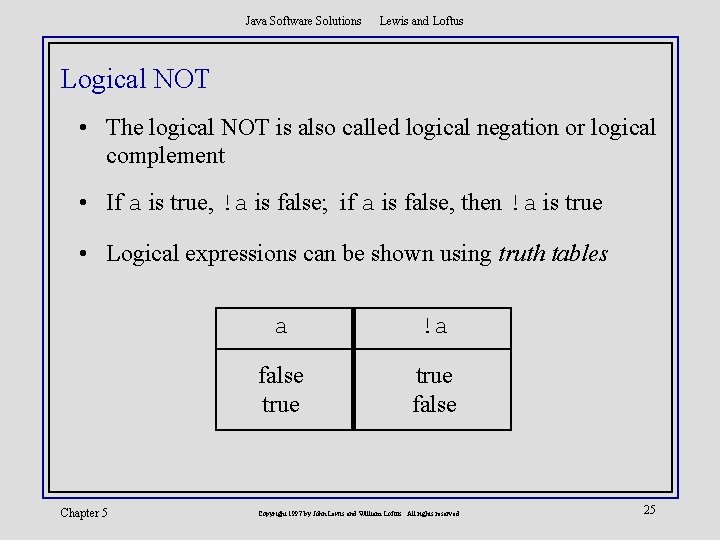
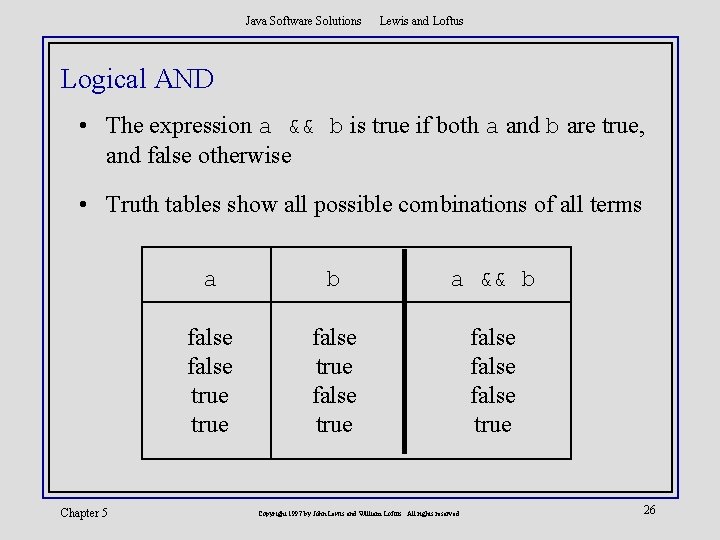
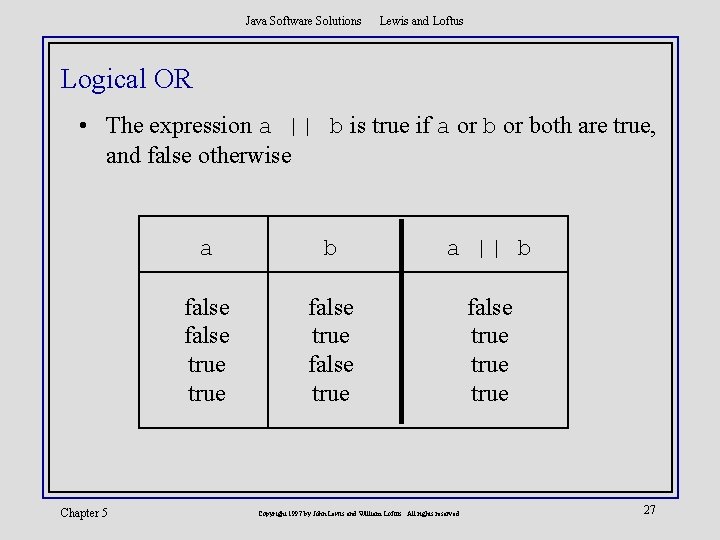
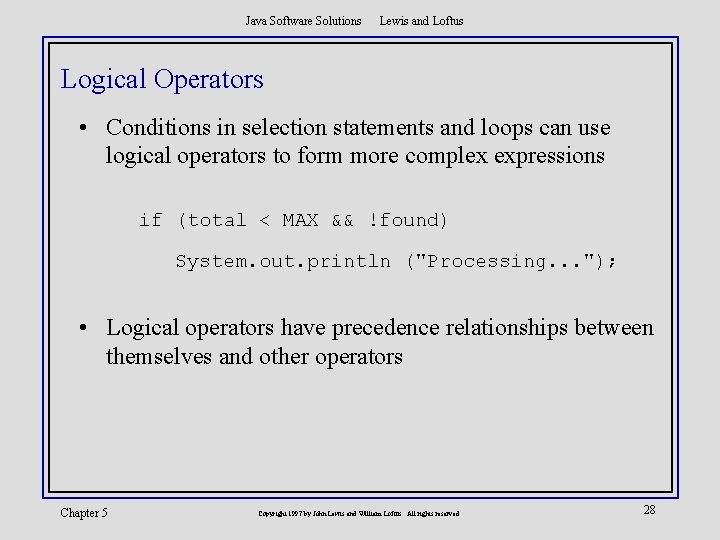
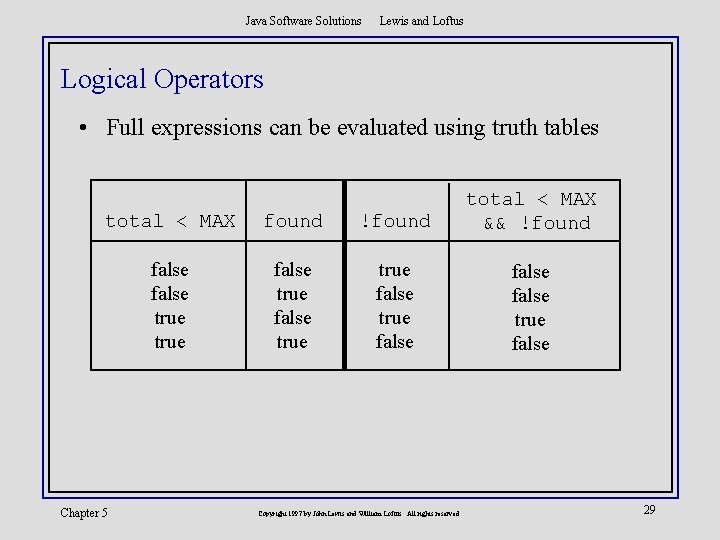
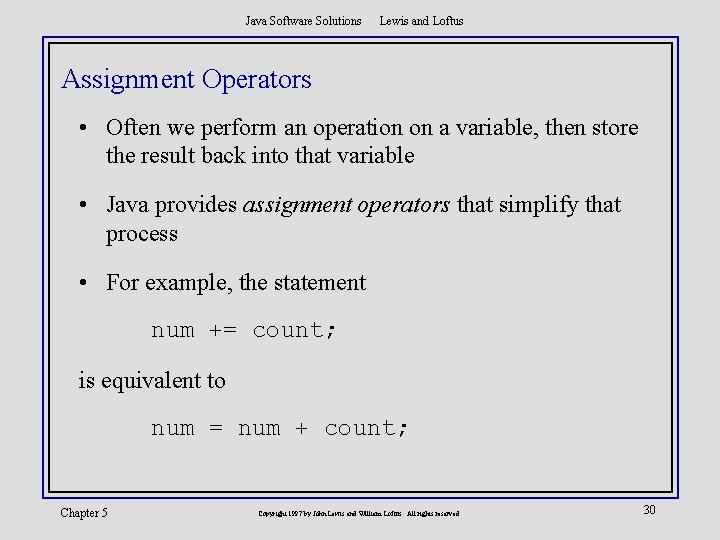
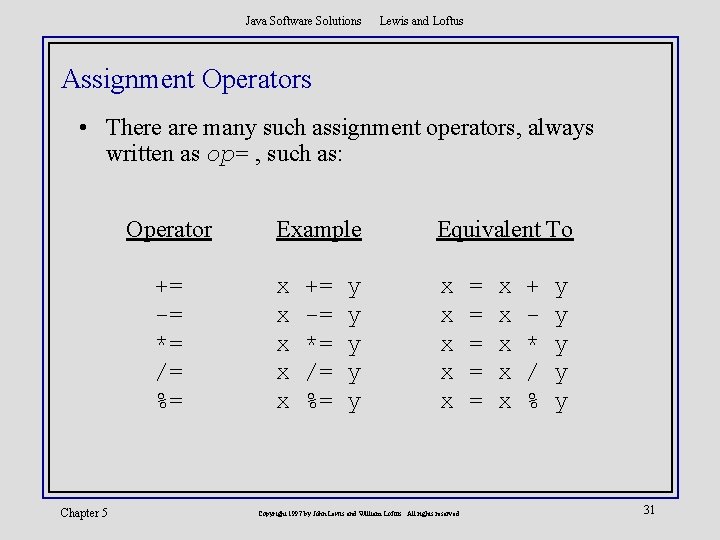
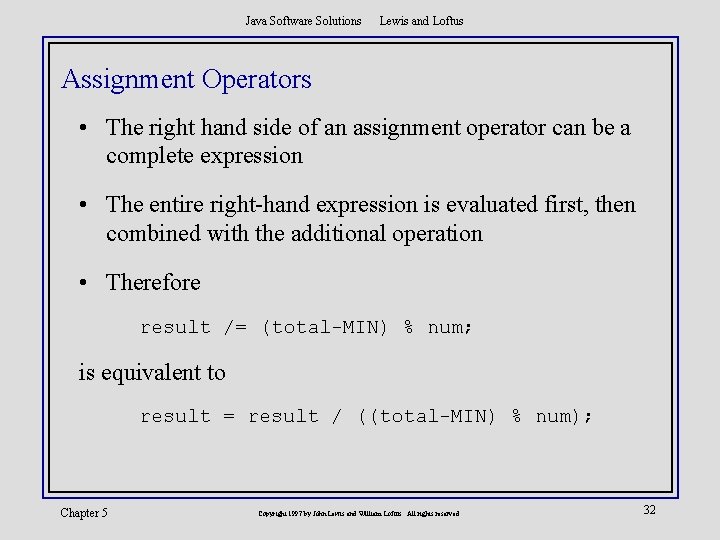
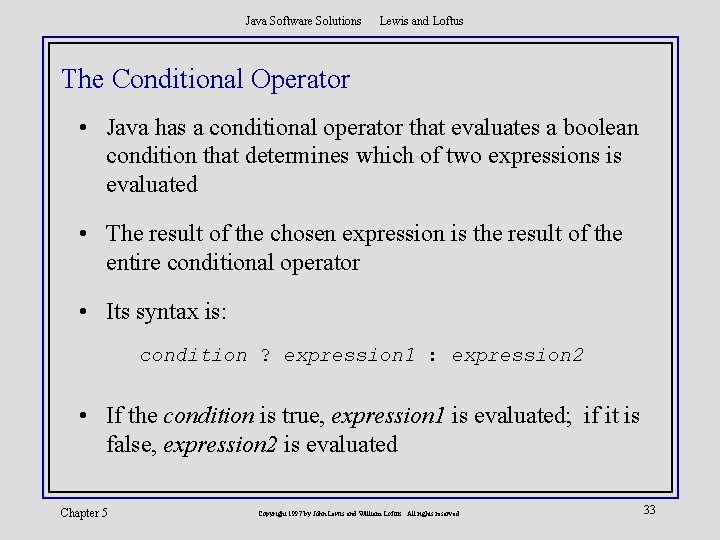
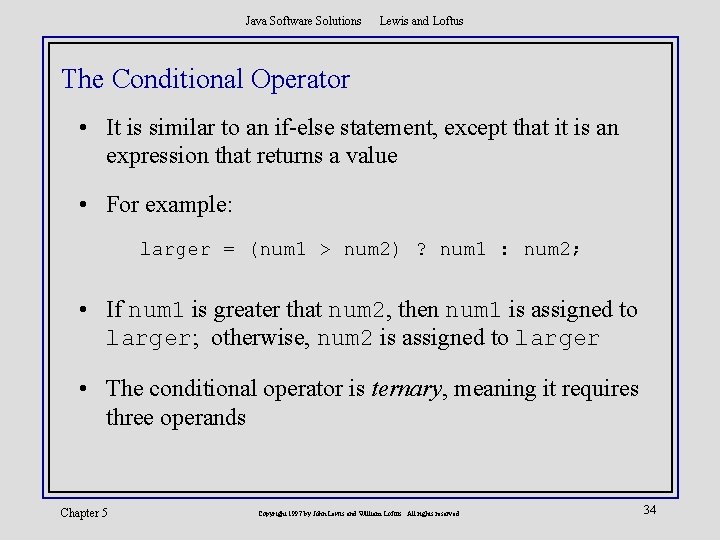
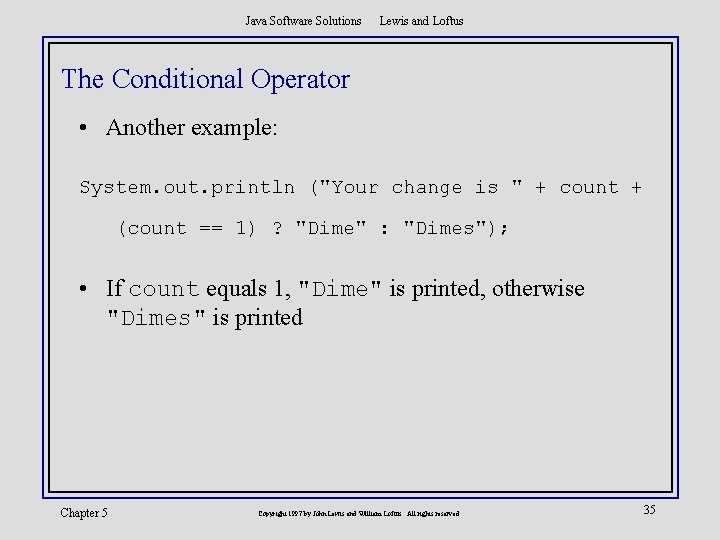
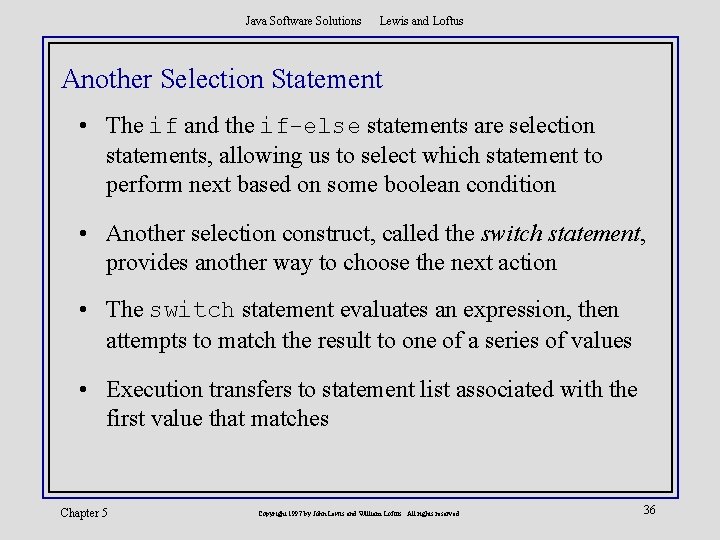
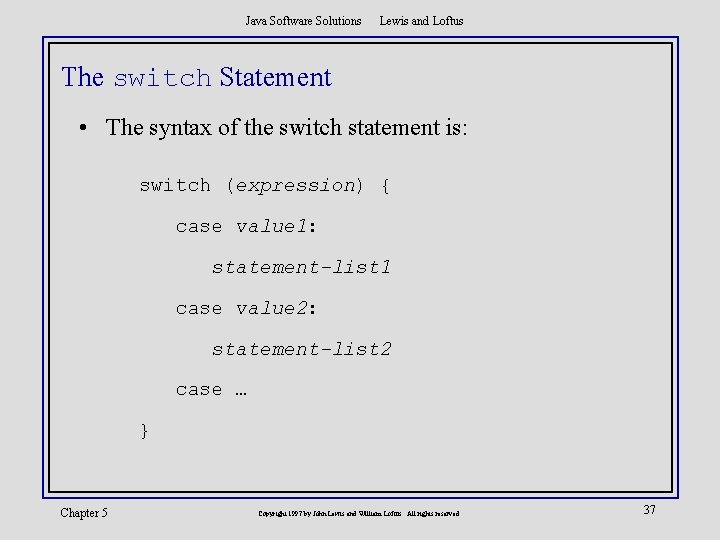
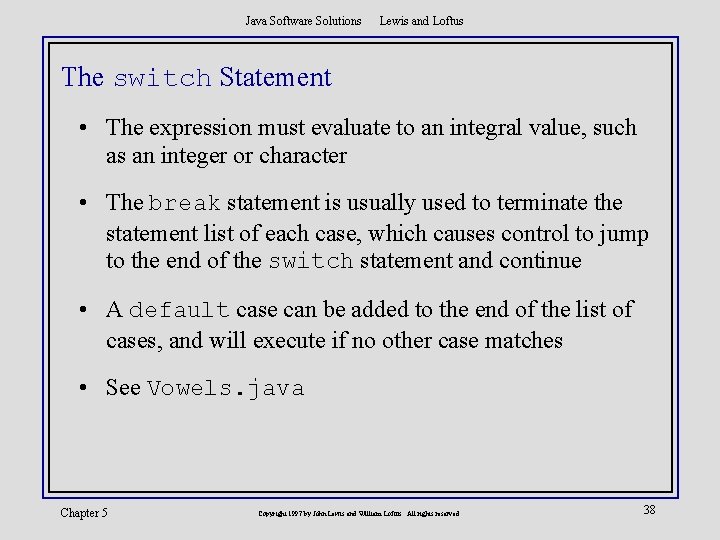
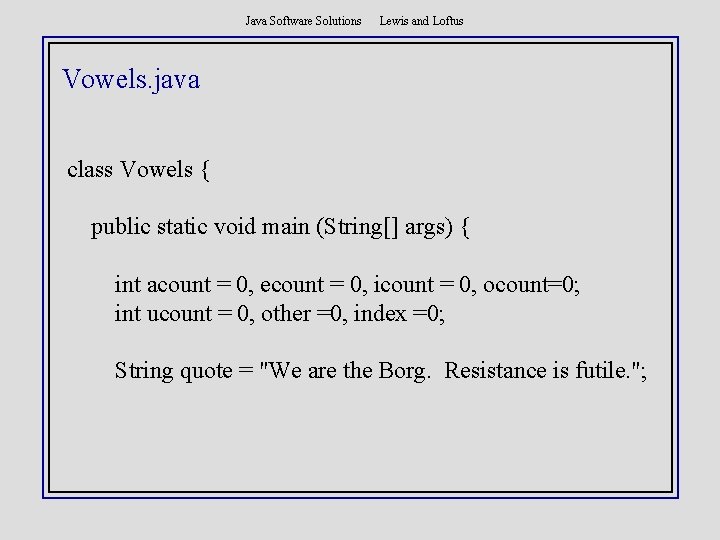
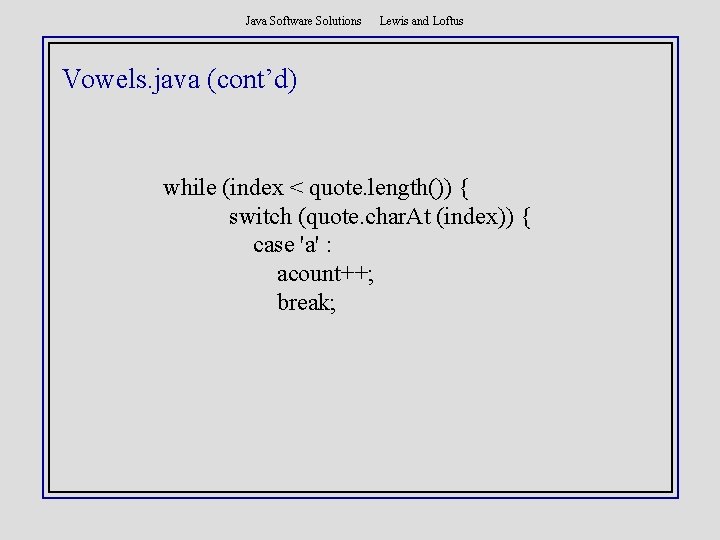
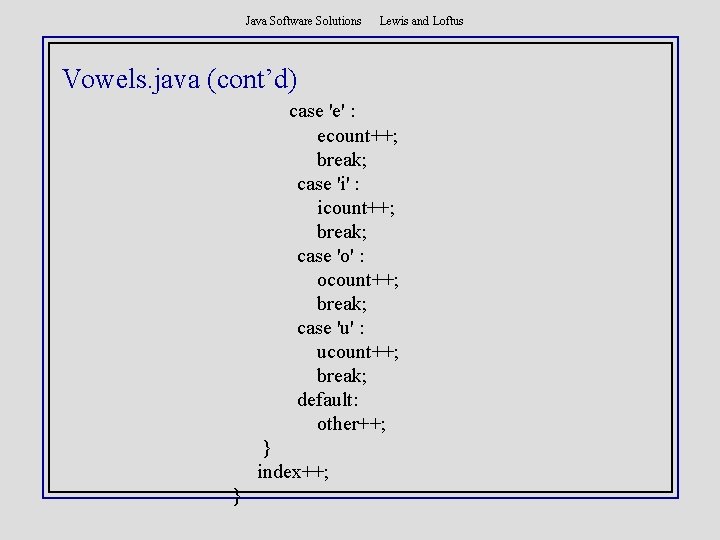
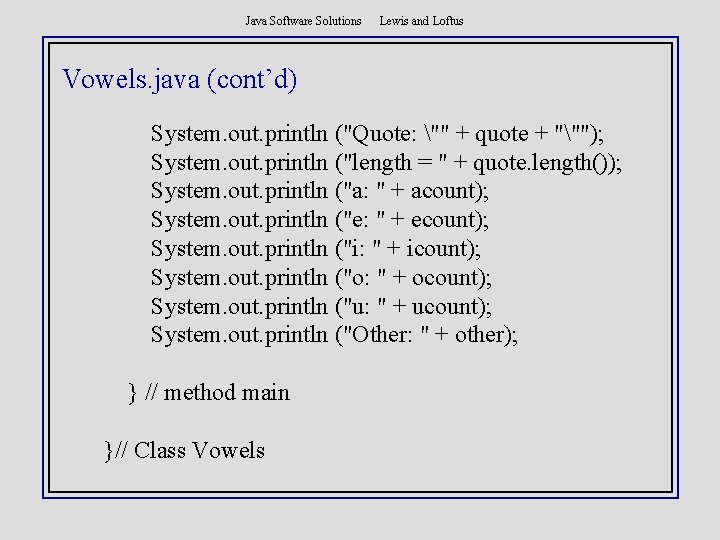
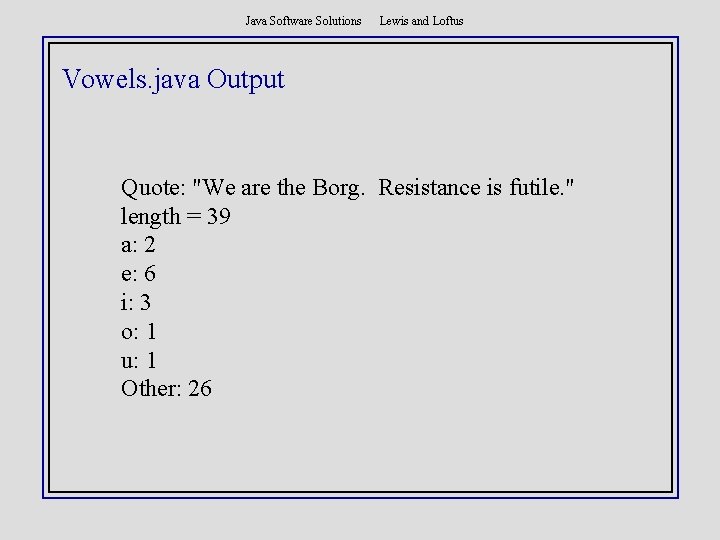
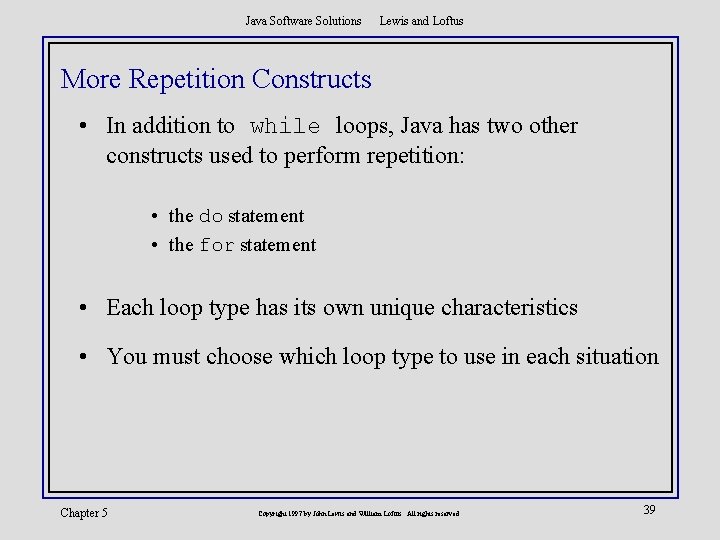
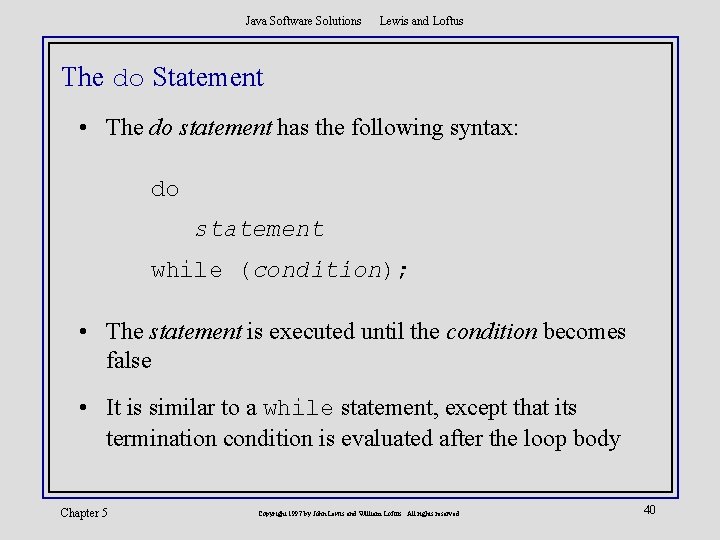
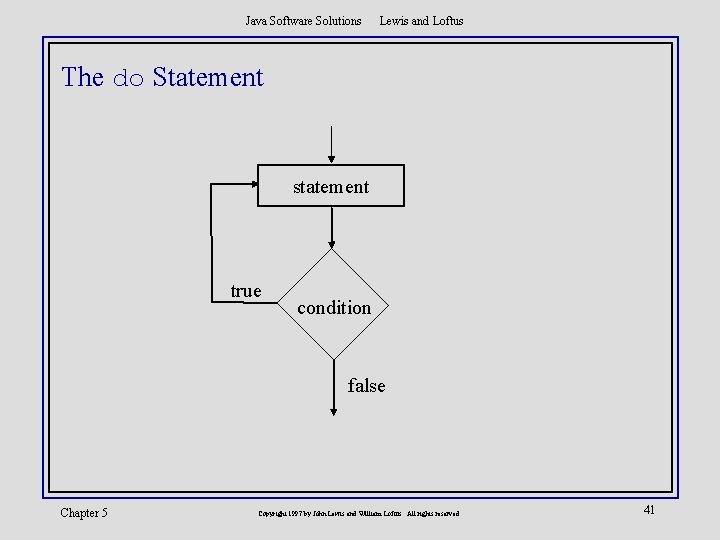
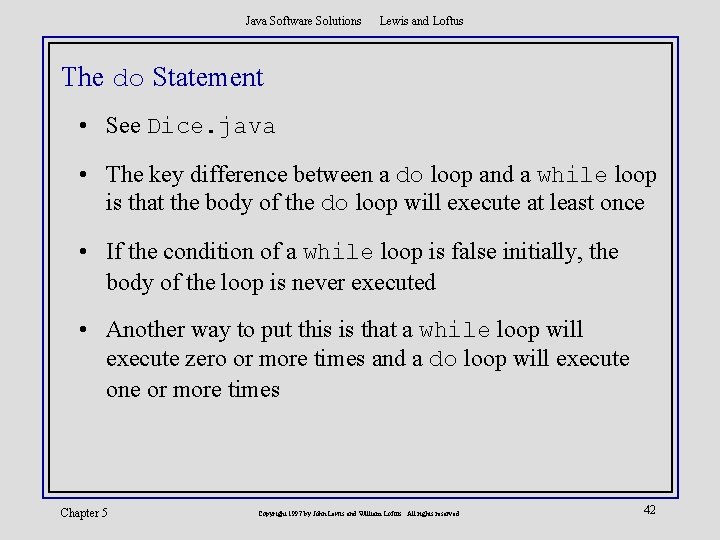
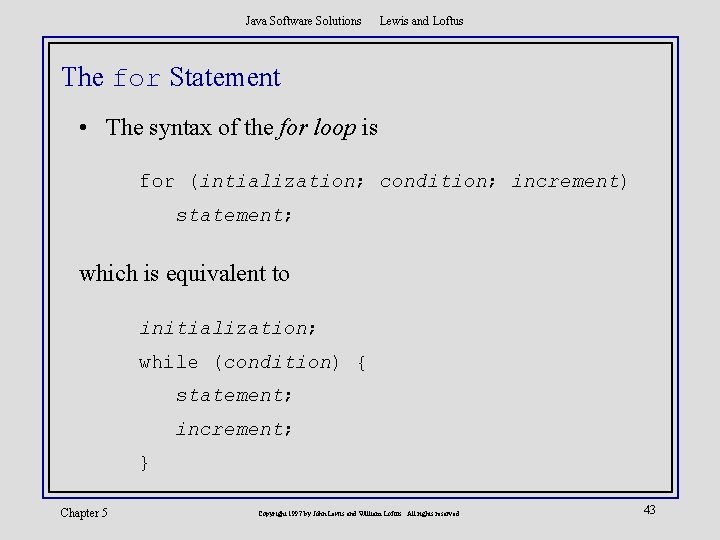
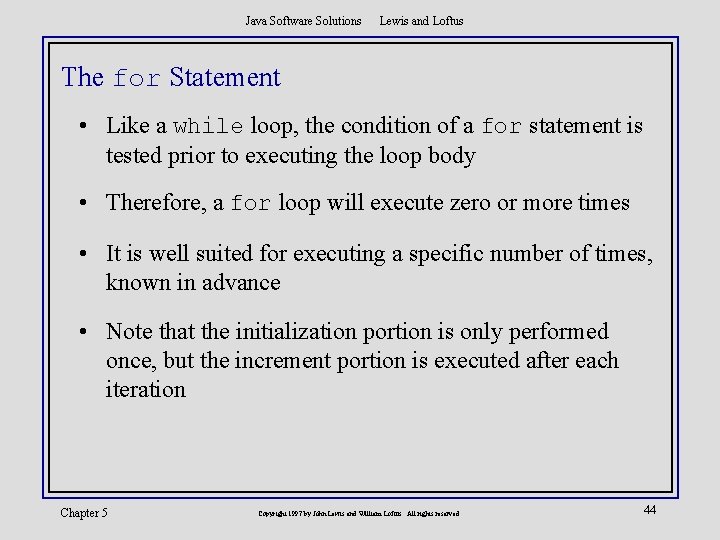
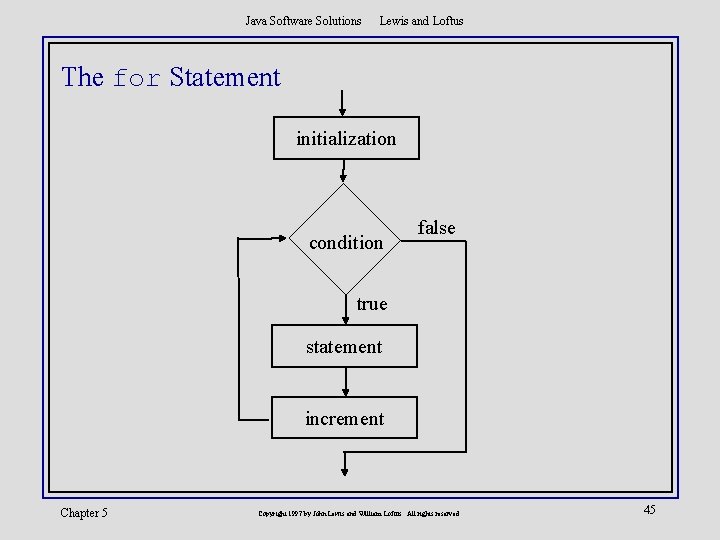
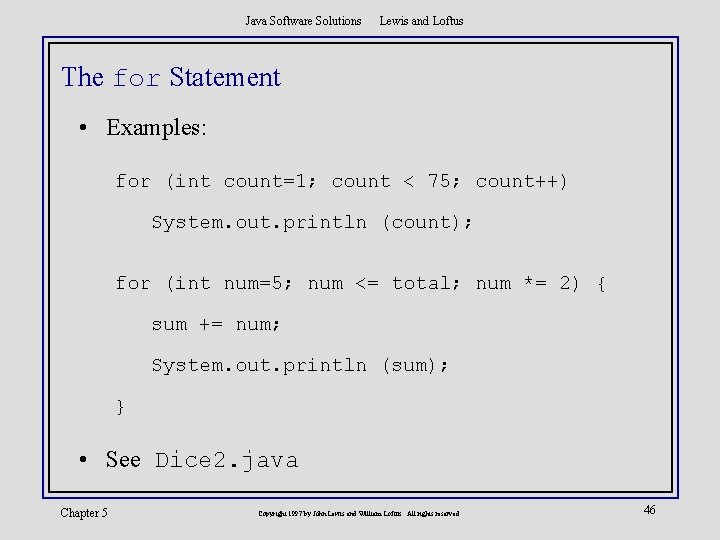
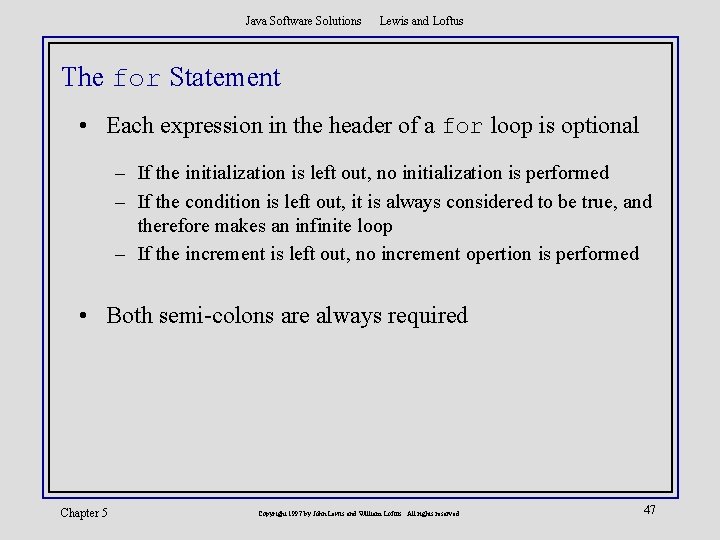
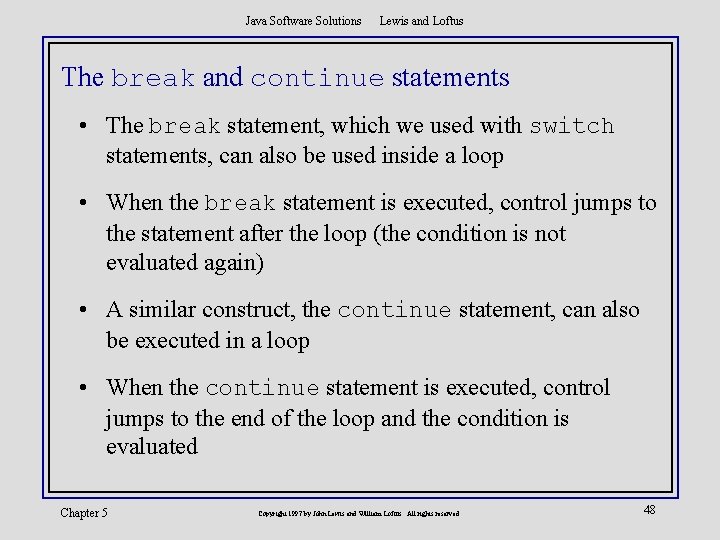
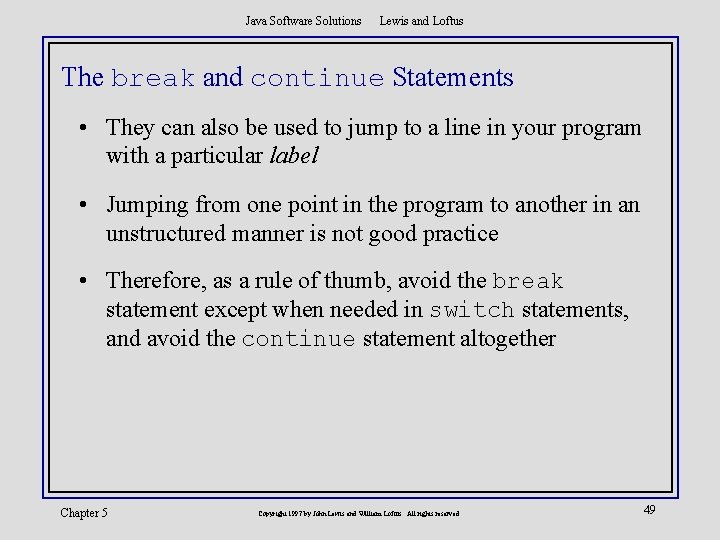
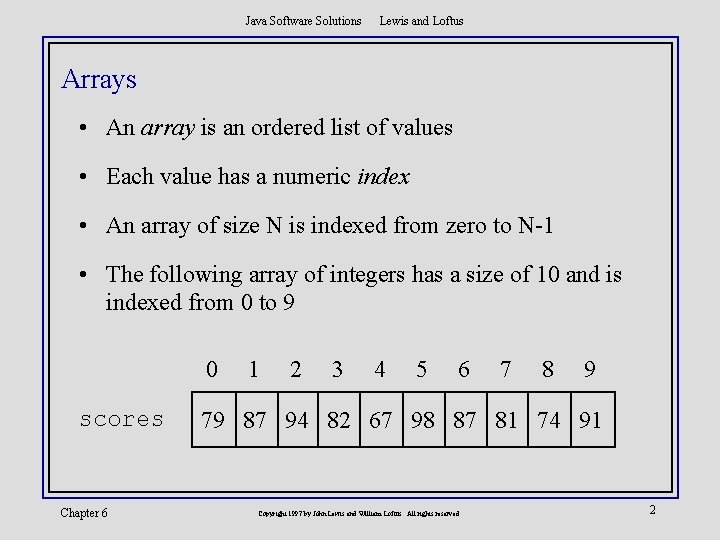
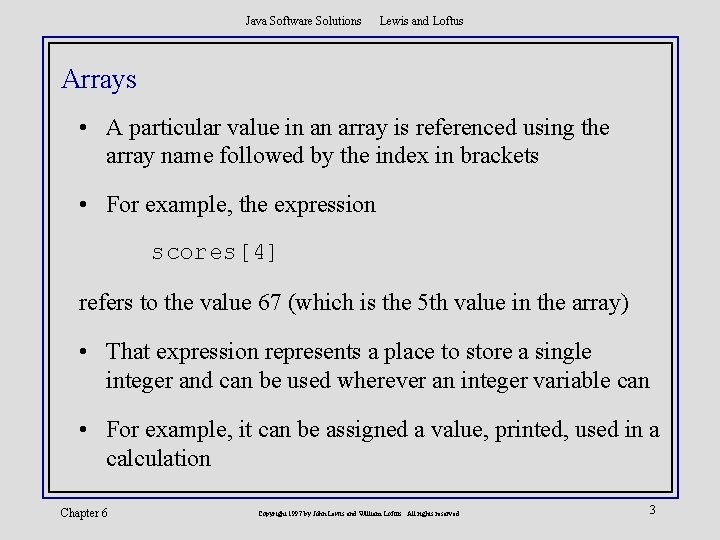
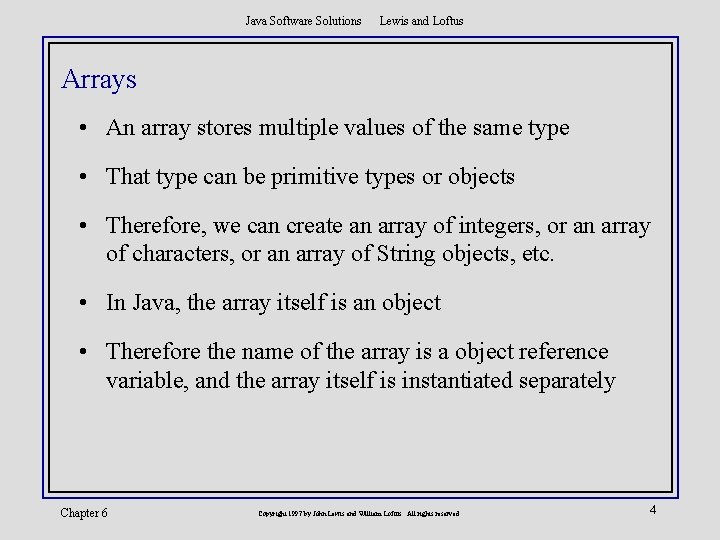
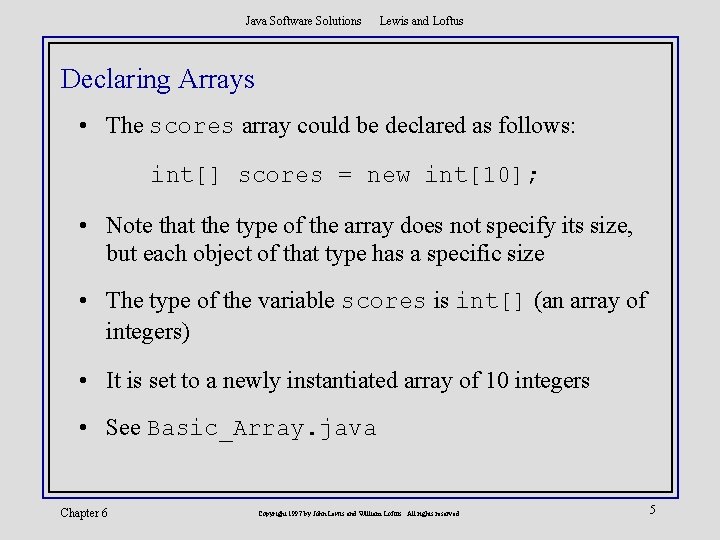
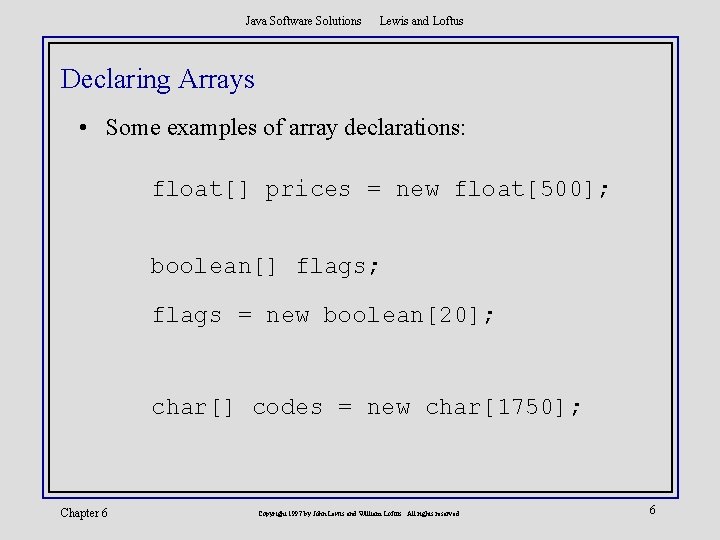
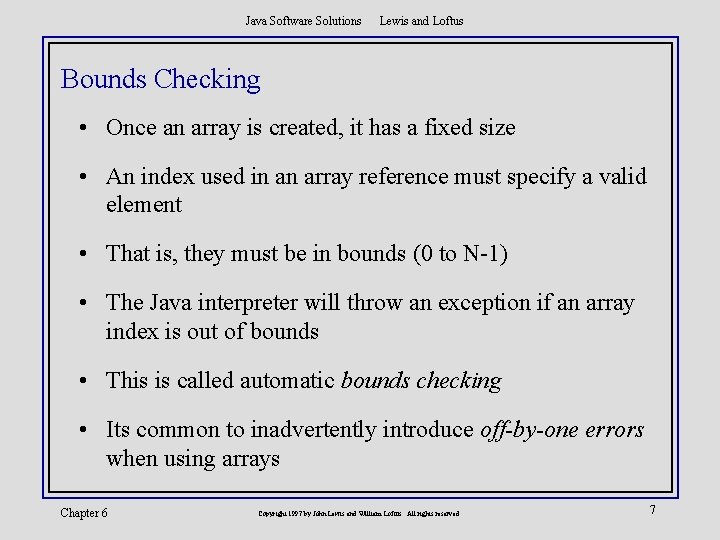
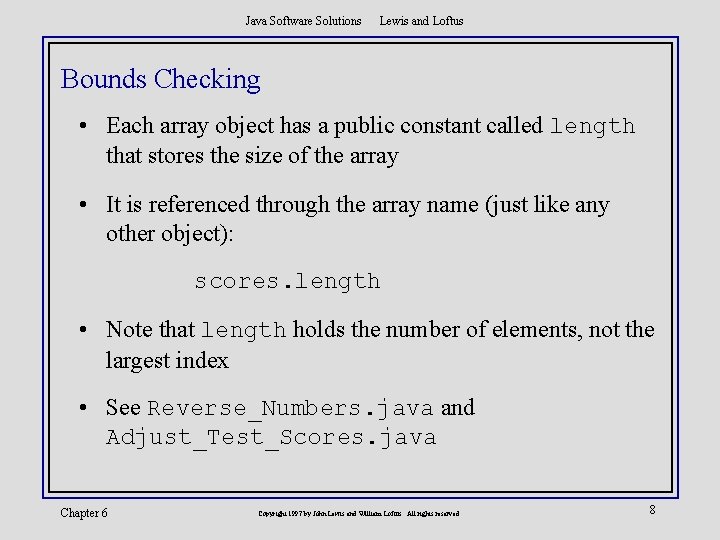
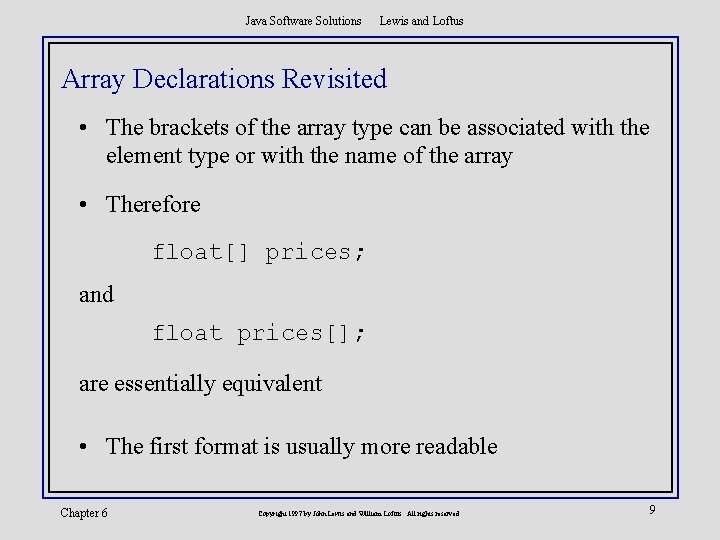
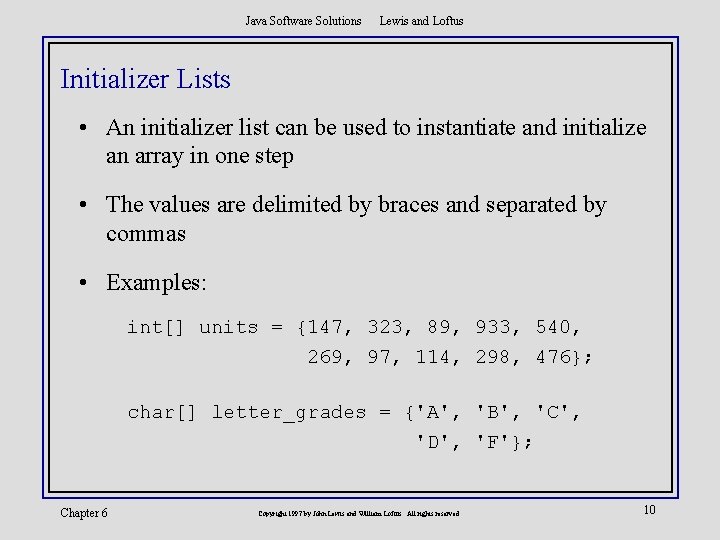
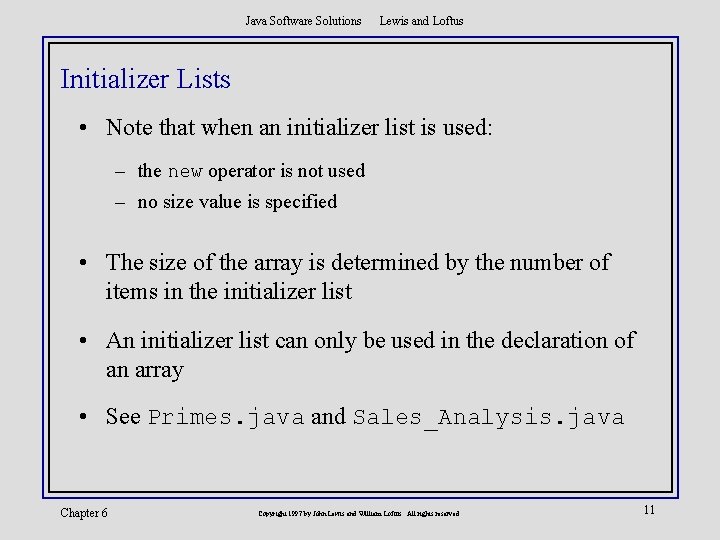
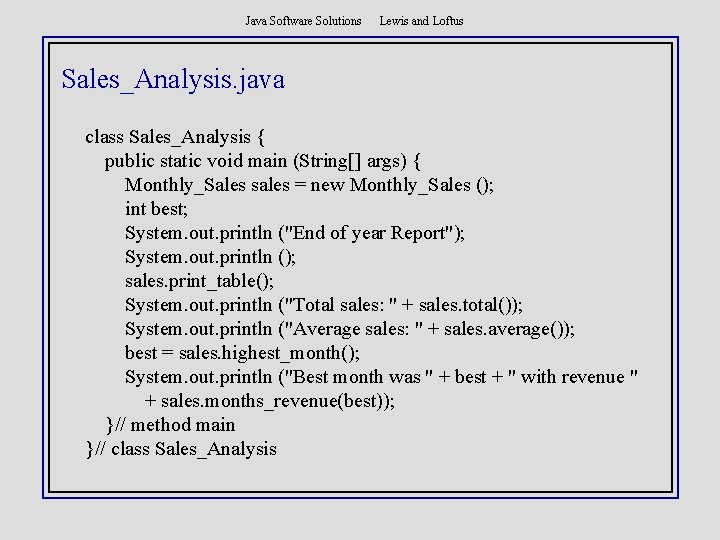
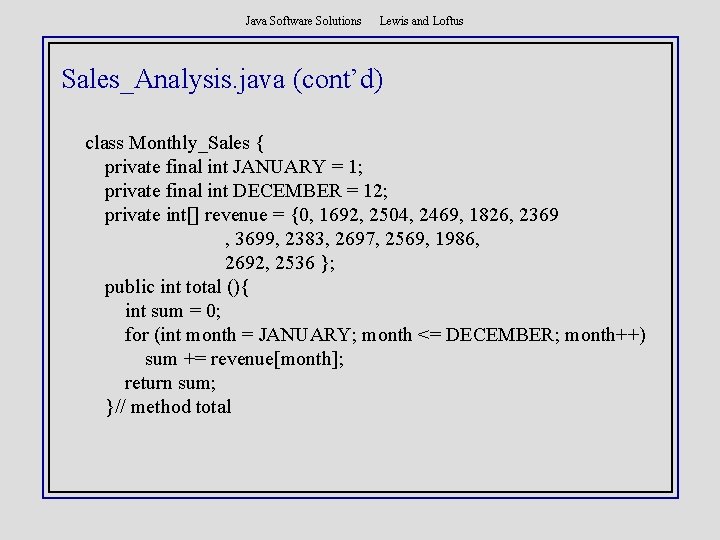
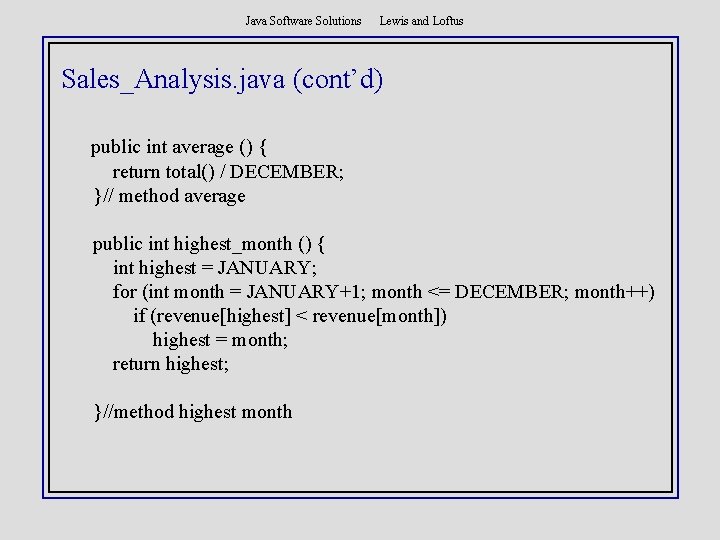
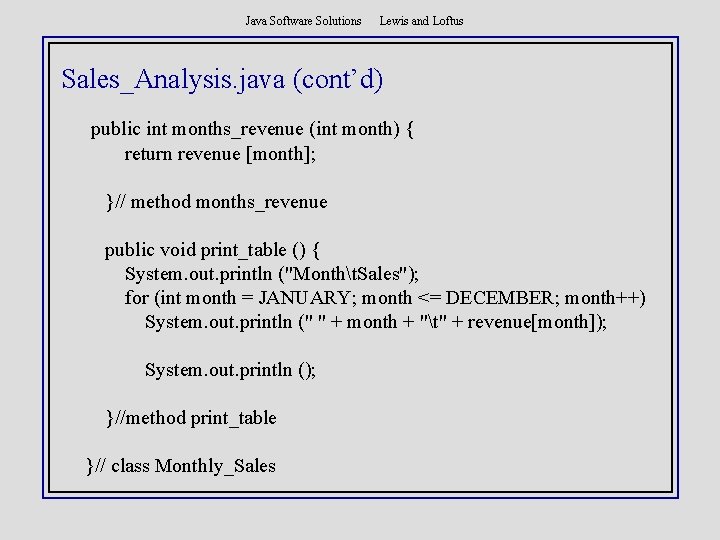
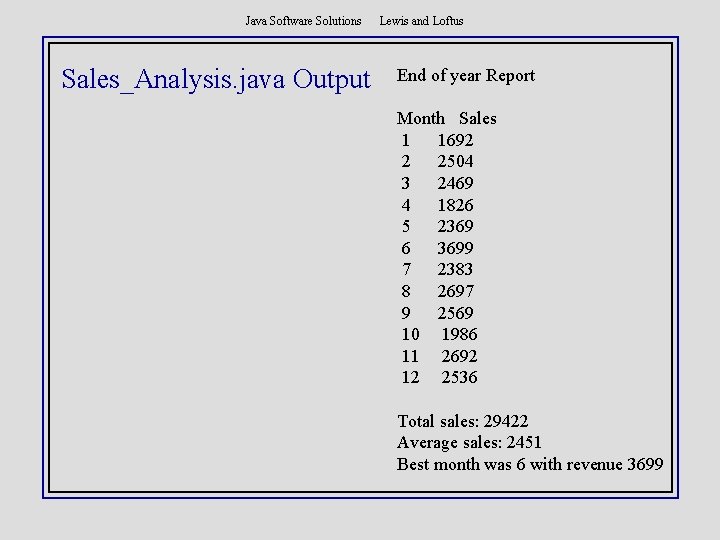
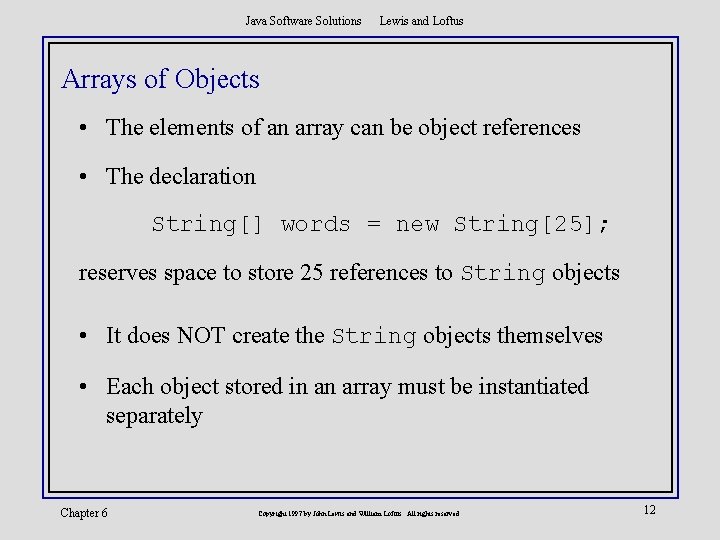
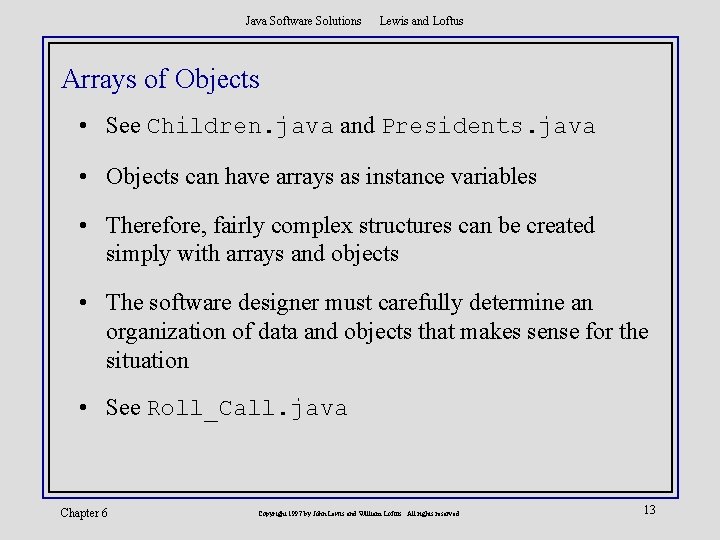
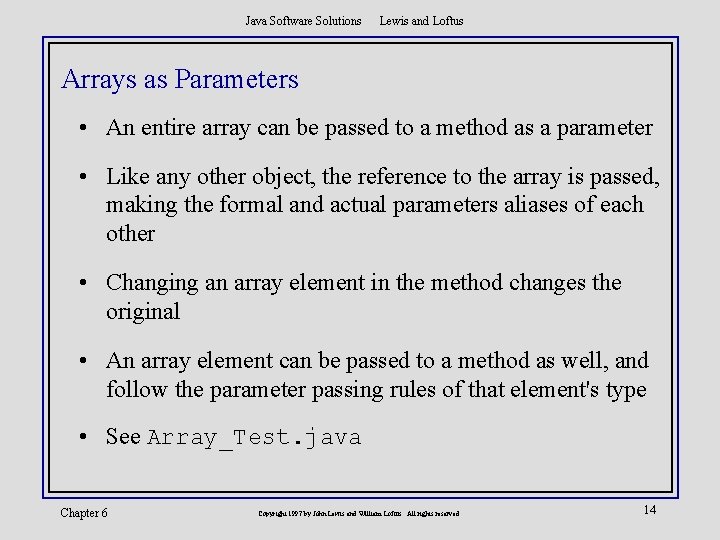
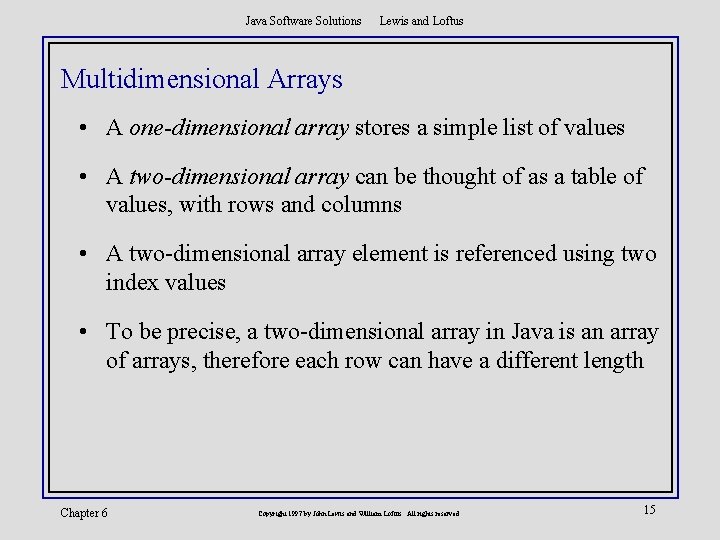
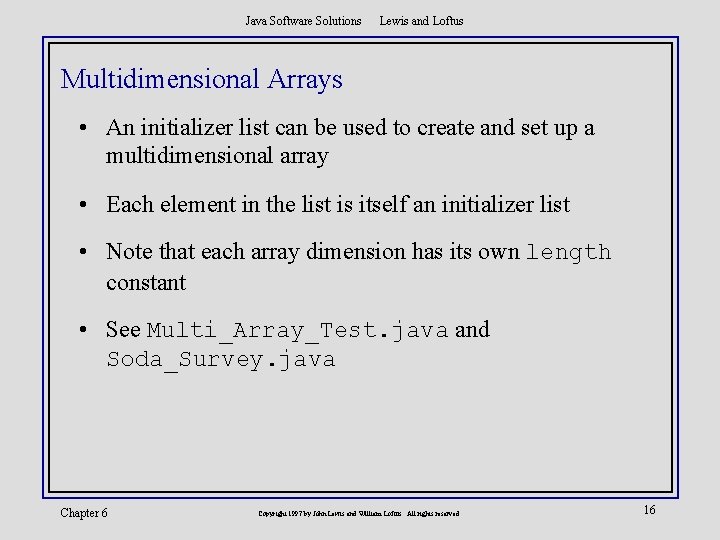
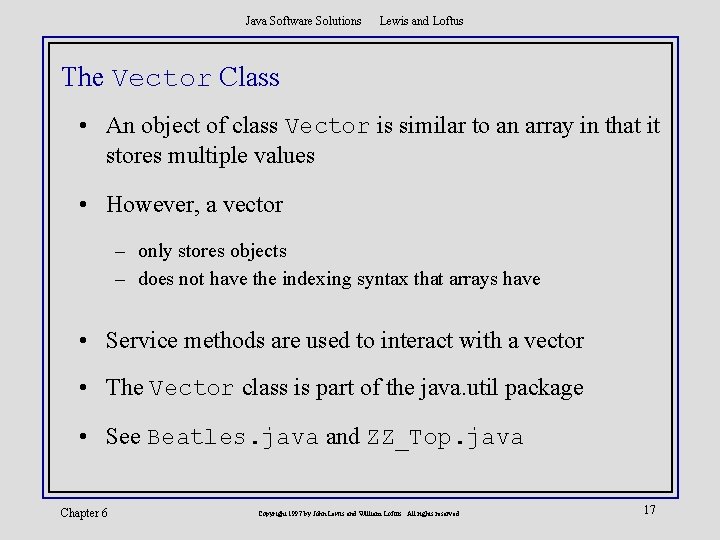
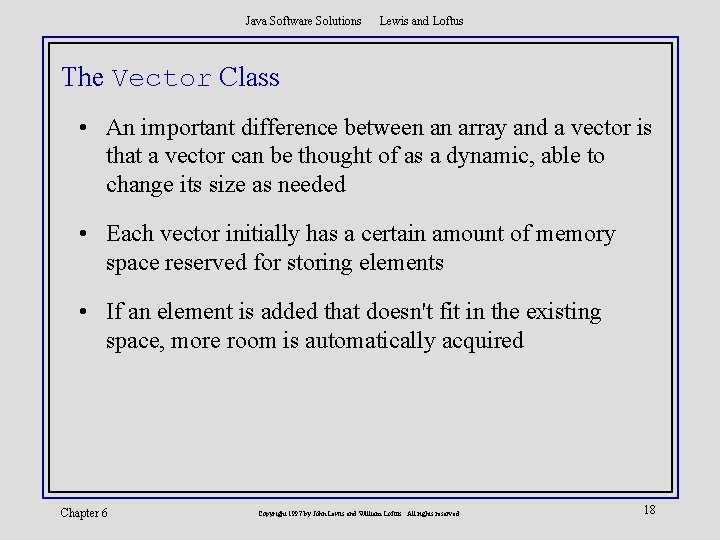
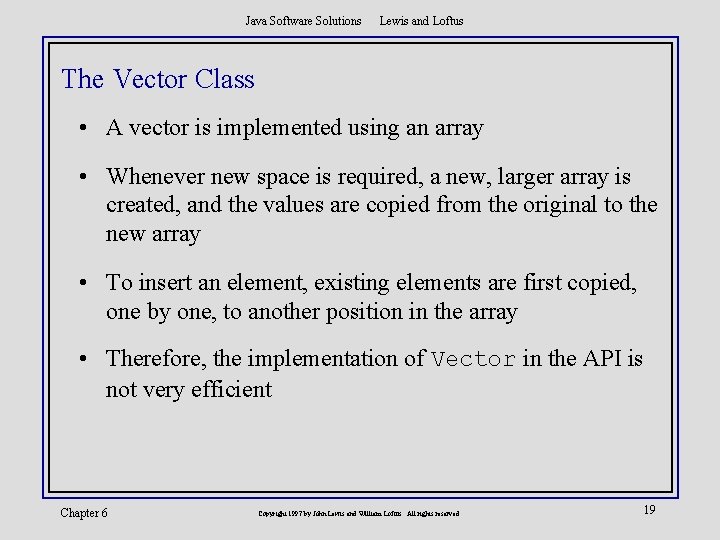
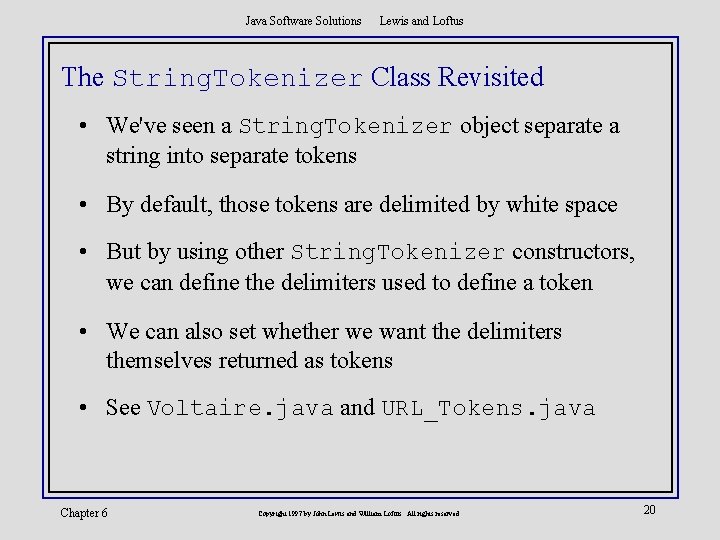
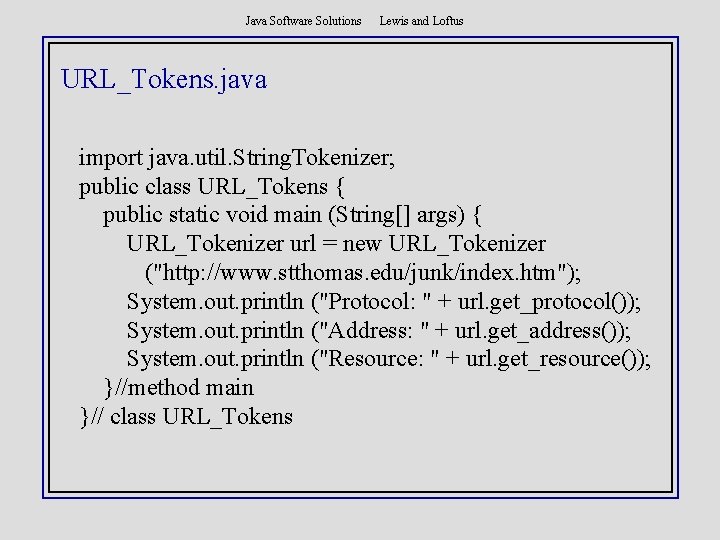
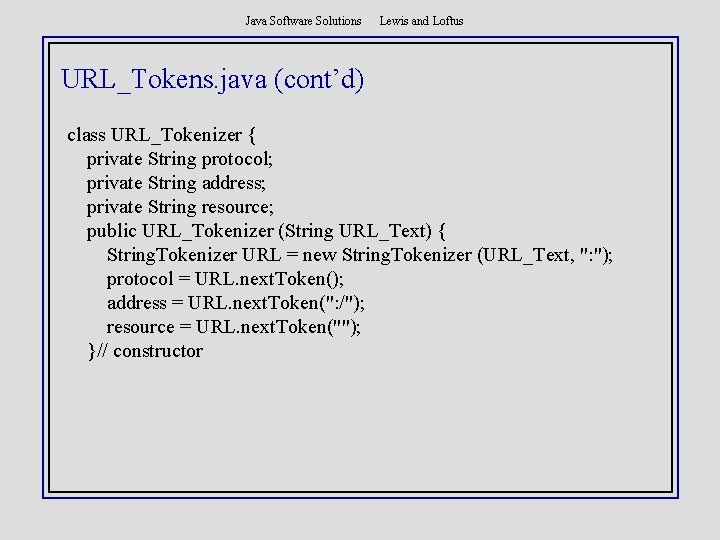
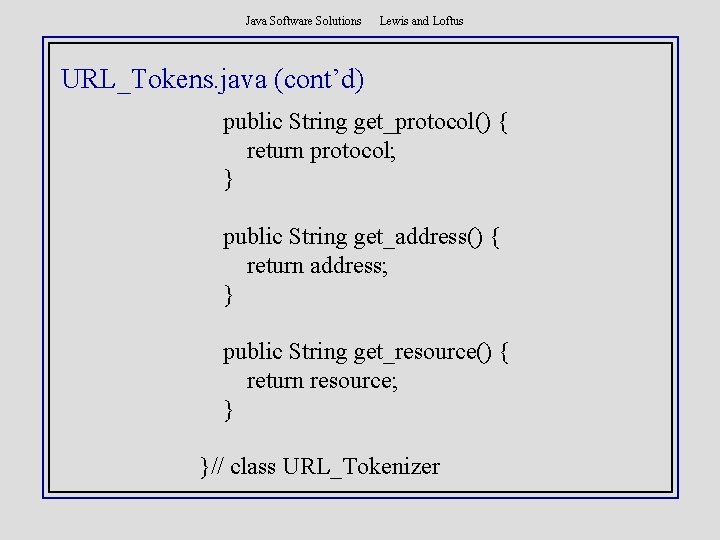
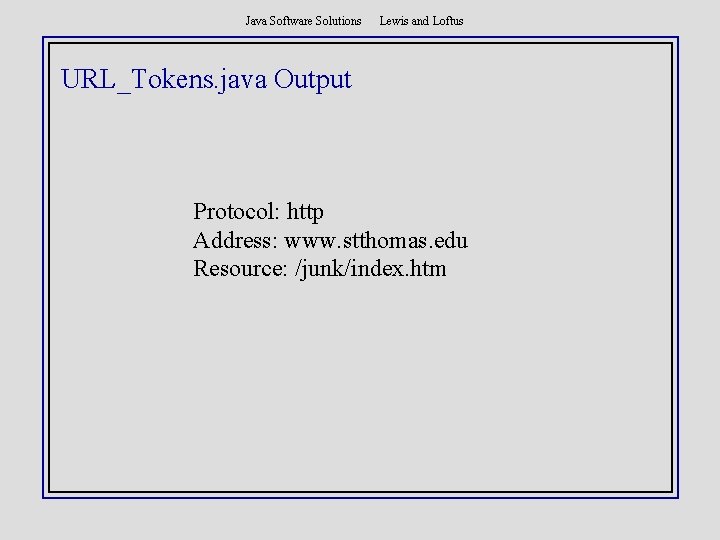
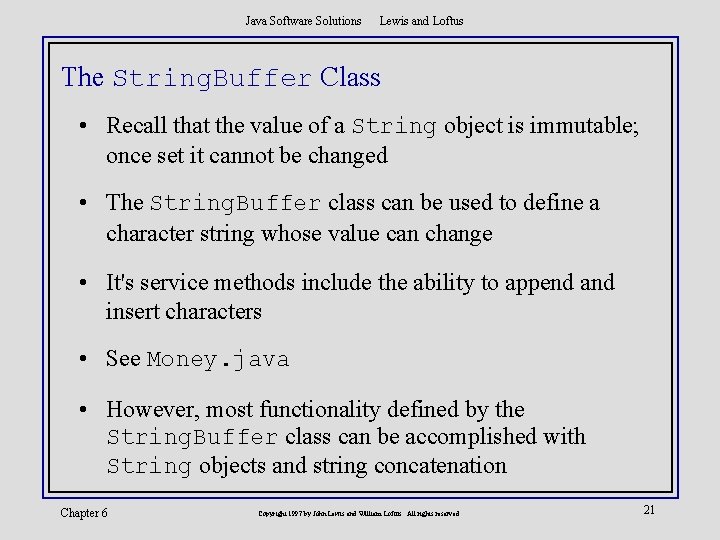
- Slides: 112
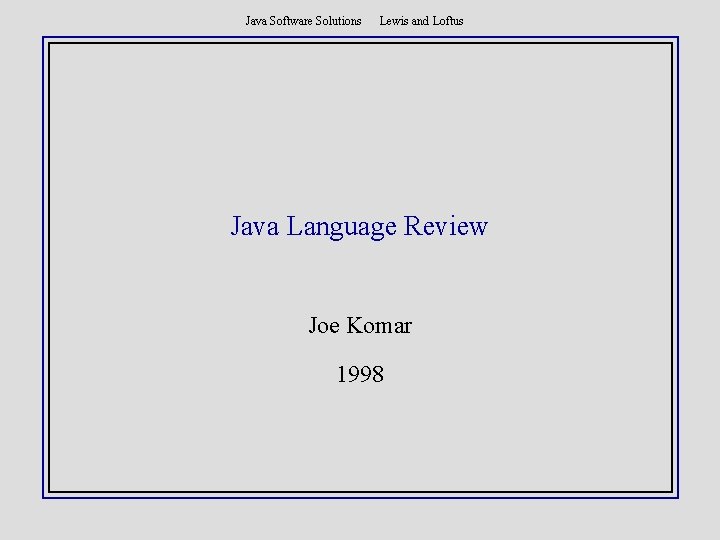
Java Software Solutions Lewis and Loftus Java Language Review Joe Komar 1998
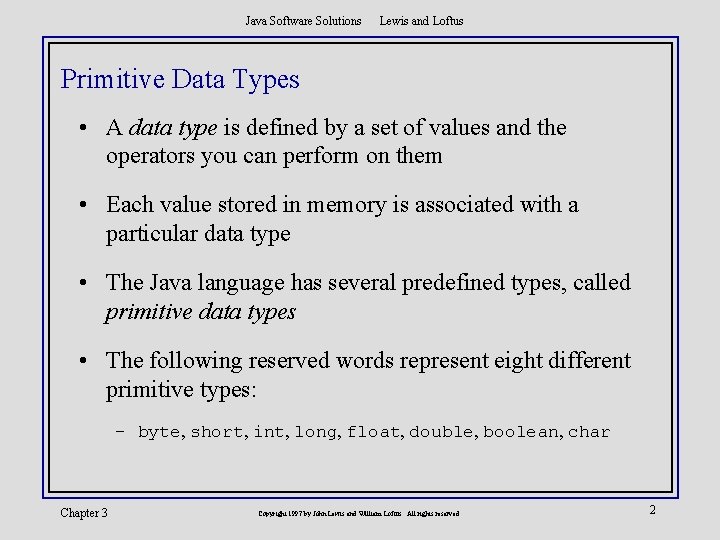
Java Software Solutions Lewis and Loftus Primitive Data Types • A data type is defined by a set of values and the operators you can perform on them • Each value stored in memory is associated with a particular data type • The Java language has several predefined types, called primitive data types • The following reserved words represent eight different primitive types: – byte, short, int, long, float, double, boolean, char Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 2
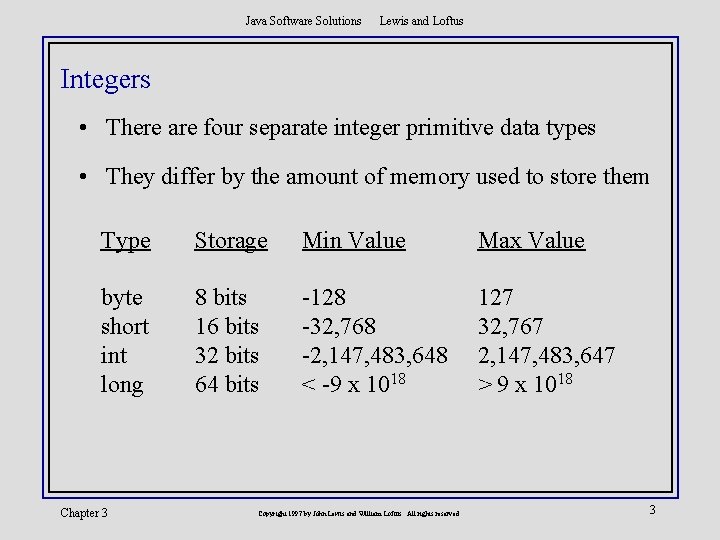
Java Software Solutions Lewis and Loftus Integers • There are four separate integer primitive data types • They differ by the amount of memory used to store them Type Storage Min Value Max Value byte short int long 8 bits 16 bits 32 bits 64 bits -128 -32, 768 -2, 147, 483, 648 < -9 x 1018 127 32, 767 2, 147, 483, 647 > 9 x 1018 Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 3
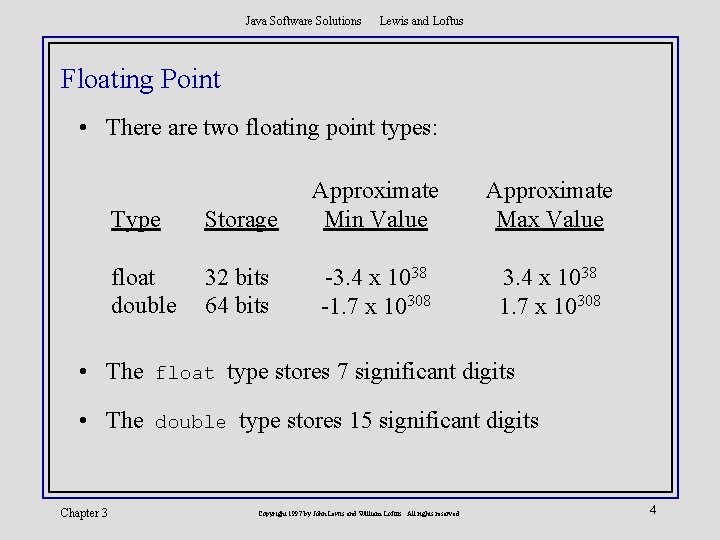
Java Software Solutions Lewis and Loftus Floating Point • There are two floating point types: Type Storage Approximate Min Value float double 32 bits 64 bits -3. 4 x 1038 -1. 7 x 10308 Approximate Max Value 3. 4 x 1038 1. 7 x 10308 • The float type stores 7 significant digits • The double type stores 15 significant digits Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 4
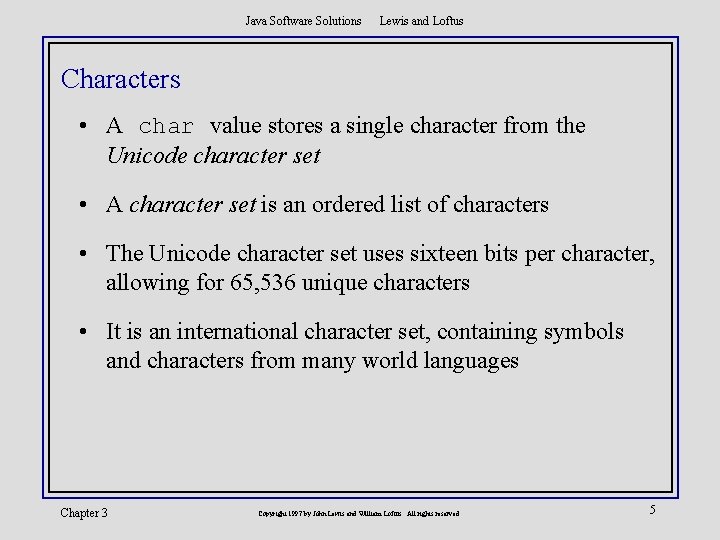
Java Software Solutions Lewis and Loftus Characters • A char value stores a single character from the Unicode character set • A character set is an ordered list of characters • The Unicode character set uses sixteen bits per character, allowing for 65, 536 unique characters • It is an international character set, containing symbols and characters from many world languages Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 5
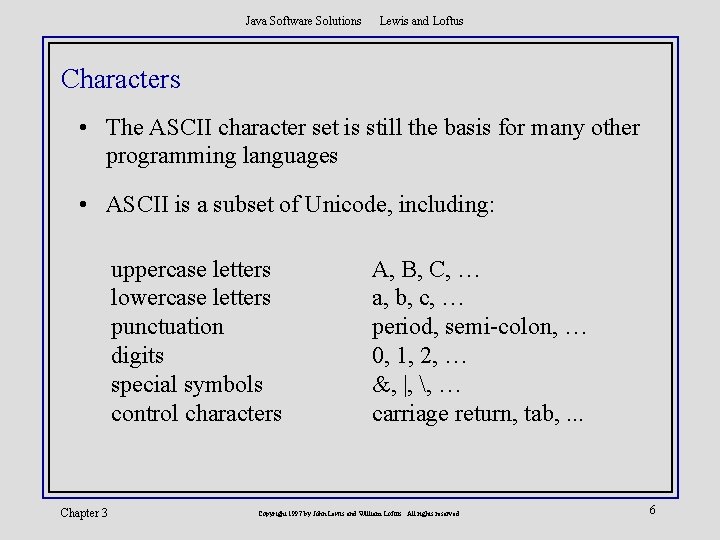
Java Software Solutions Lewis and Loftus Characters • The ASCII character set is still the basis for many other programming languages • ASCII is a subset of Unicode, including: uppercase letters lowercase letters punctuation digits special symbols control characters Chapter 3 A, B, C, … a, b, c, … period, semi-colon, … 0, 1, 2, … &, |, , … carriage return, tab, . . . Copyright 1997 by John Lewis and William Loftus. All rights reserved. 6
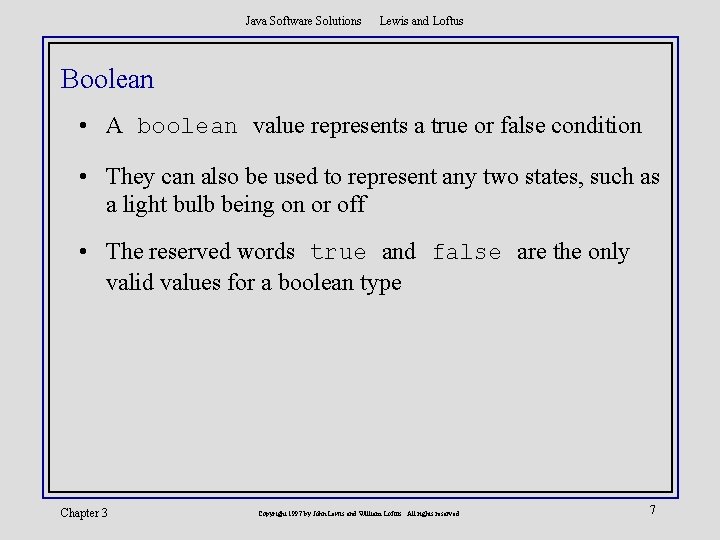
Java Software Solutions Lewis and Loftus Boolean • A boolean value represents a true or false condition • They can also be used to represent any two states, such as a light bulb being on or off • The reserved words true and false are the only valid values for a boolean type Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 7
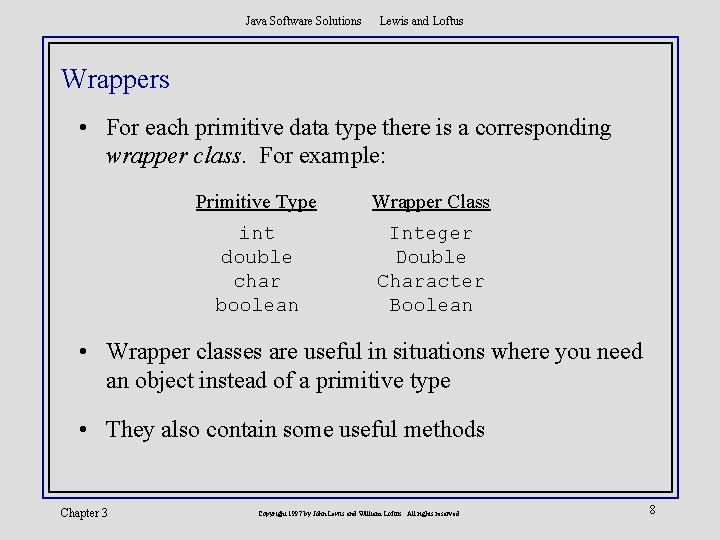
Java Software Solutions Lewis and Loftus Wrappers • For each primitive data type there is a corresponding wrapper class. For example: Primitive Type Wrapper Class int double char boolean Integer Double Character Boolean • Wrapper classes are useful in situations where you need an object instead of a primitive type • They also contain some useful methods Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 8
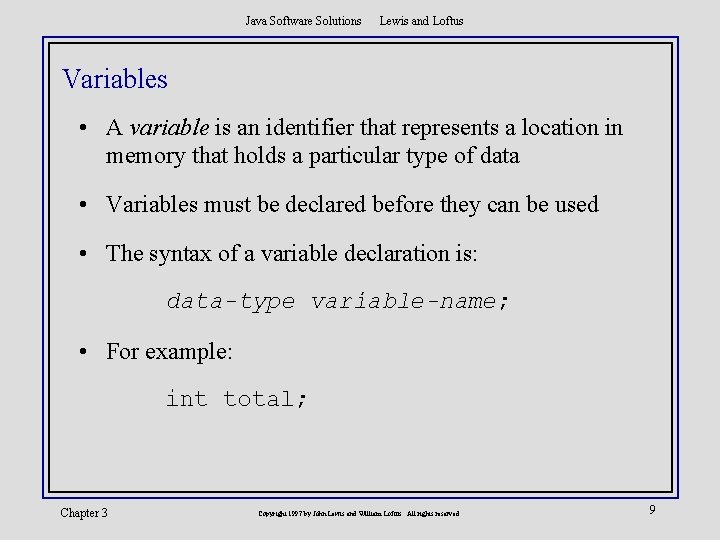
Java Software Solutions Lewis and Loftus Variables • A variable is an identifier that represents a location in memory that holds a particular type of data • Variables must be declared before they can be used • The syntax of a variable declaration is: data-type variable-name; • For example: int total; Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 9
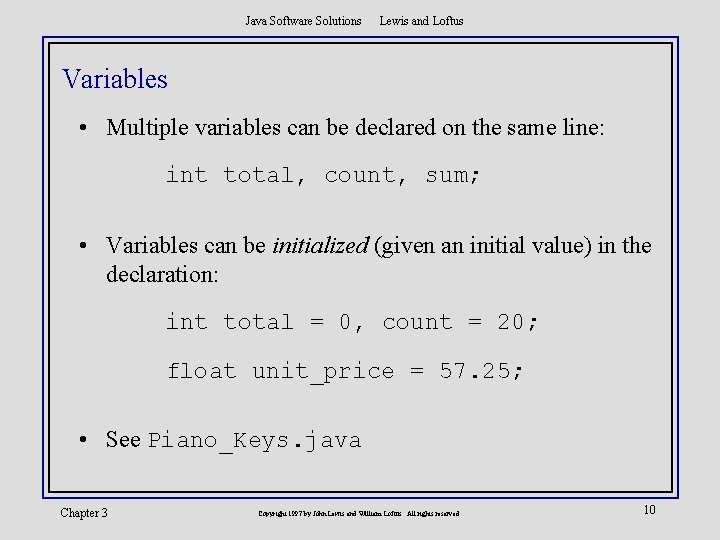
Java Software Solutions Lewis and Loftus Variables • Multiple variables can be declared on the same line: int total, count, sum; • Variables can be initialized (given an initial value) in the declaration: int total = 0, count = 20; float unit_price = 57. 25; • See Piano_Keys. java Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 10
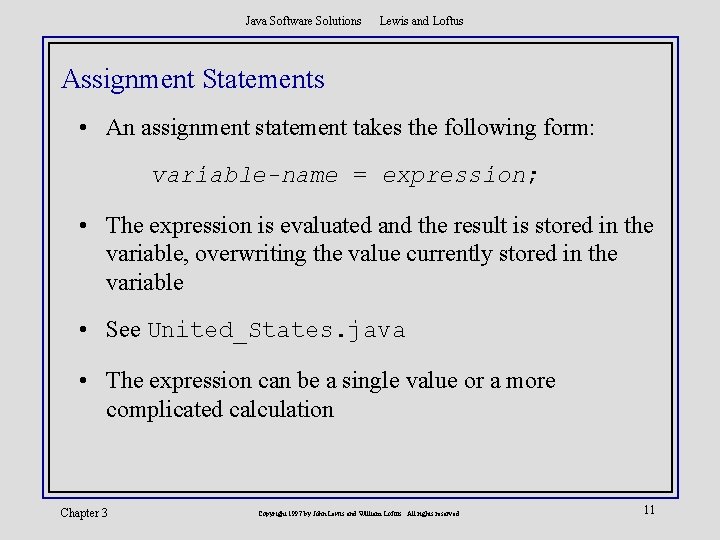
Java Software Solutions Lewis and Loftus Assignment Statements • An assignment statement takes the following form: variable-name = expression; • The expression is evaluated and the result is stored in the variable, overwriting the value currently stored in the variable • See United_States. java • The expression can be a single value or a more complicated calculation Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 11
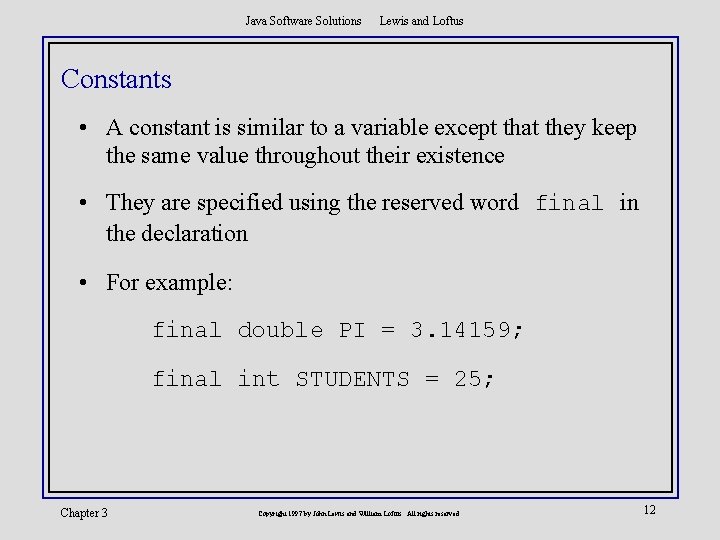
Java Software Solutions Lewis and Loftus Constants • A constant is similar to a variable except that they keep the same value throughout their existence • They are specified using the reserved word final in the declaration • For example: final double PI = 3. 14159; final int STUDENTS = 25; Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 12
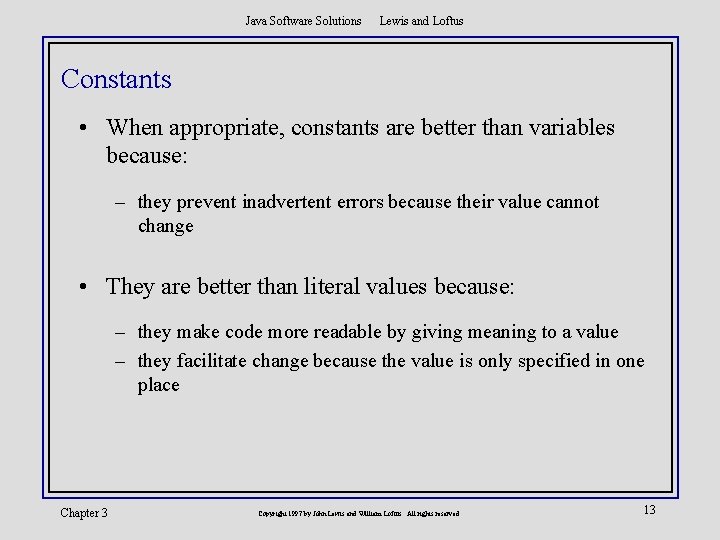
Java Software Solutions Lewis and Loftus Constants • When appropriate, constants are better than variables because: – they prevent inadvertent errors because their value cannot change • They are better than literal values because: – they make code more readable by giving meaning to a value – they facilitate change because the value is only specified in one place Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 13
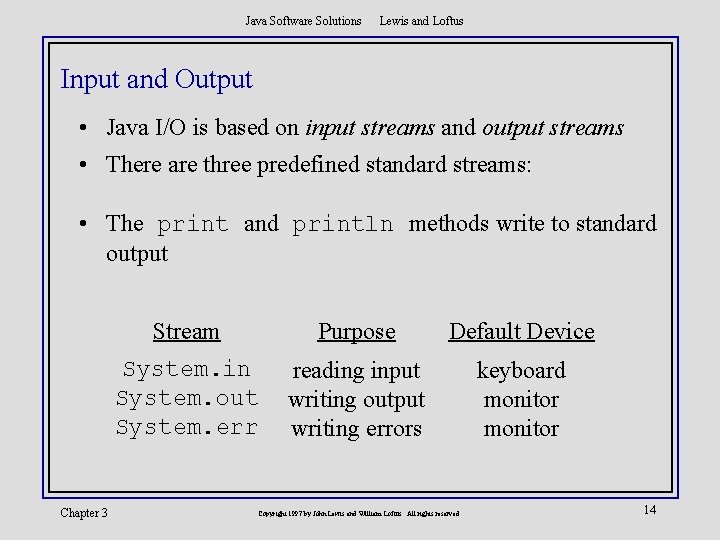
Java Software Solutions Lewis and Loftus Input and Output • Java I/O is based on input streams and output streams • There are three predefined standard streams: • The print and println methods write to standard output Chapter 3 Stream Purpose Default Device System. in System. out System. err reading input writing output writing errors keyboard monitor Copyright 1997 by John Lewis and William Loftus. All rights reserved. 14
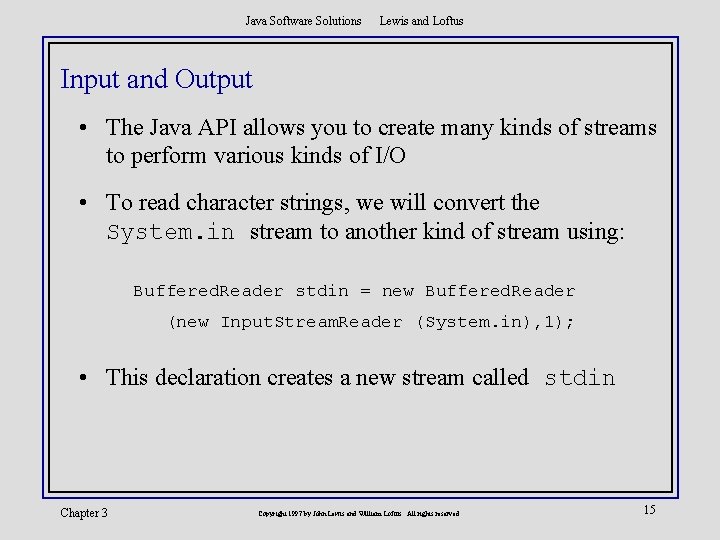
Java Software Solutions Lewis and Loftus Input and Output • The Java API allows you to create many kinds of streams to perform various kinds of I/O • To read character strings, we will convert the System. in stream to another kind of stream using: Buffered. Reader stdin = new Buffered. Reader (new Input. Stream. Reader (System. in), 1); • This declaration creates a new stream called stdin Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 15
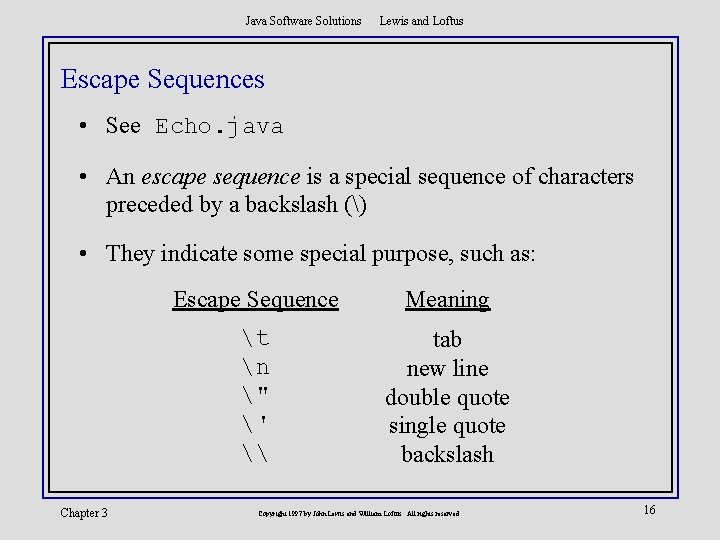
Java Software Solutions Lewis and Loftus Escape Sequences • See Echo. java • An escape sequence is a special sequence of characters preceded by a backslash () • They indicate some special purpose, such as: Chapter 3 Escape Sequence Meaning t n " ' \ tab new line double quote single quote backslash Copyright 1997 by John Lewis and William Loftus. All rights reserved. 16
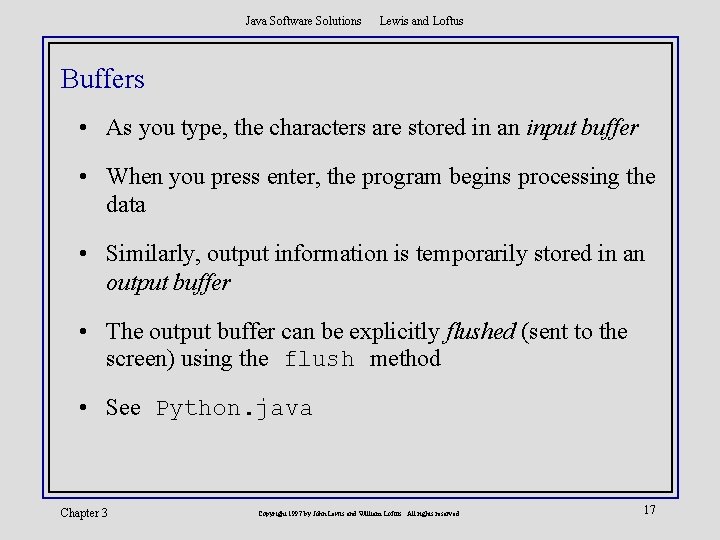
Java Software Solutions Lewis and Loftus Buffers • As you type, the characters are stored in an input buffer • When you press enter, the program begins processing the data • Similarly, output information is temporarily stored in an output buffer • The output buffer can be explicitly flushed (sent to the screen) using the flush method • See Python. java Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 17
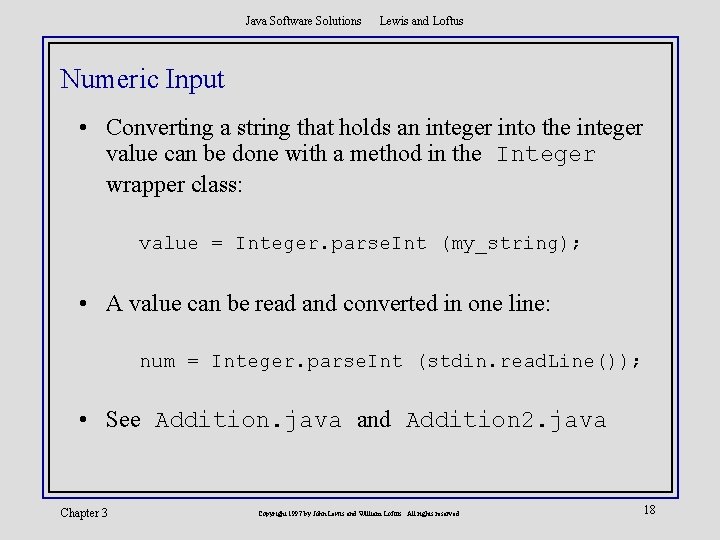
Java Software Solutions Lewis and Loftus Numeric Input • Converting a string that holds an integer into the integer value can be done with a method in the Integer wrapper class: value = Integer. parse. Int (my_string); • A value can be read and converted in one line: num = Integer. parse. Int (stdin. read. Line()); • See Addition. java and Addition 2. java Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 18
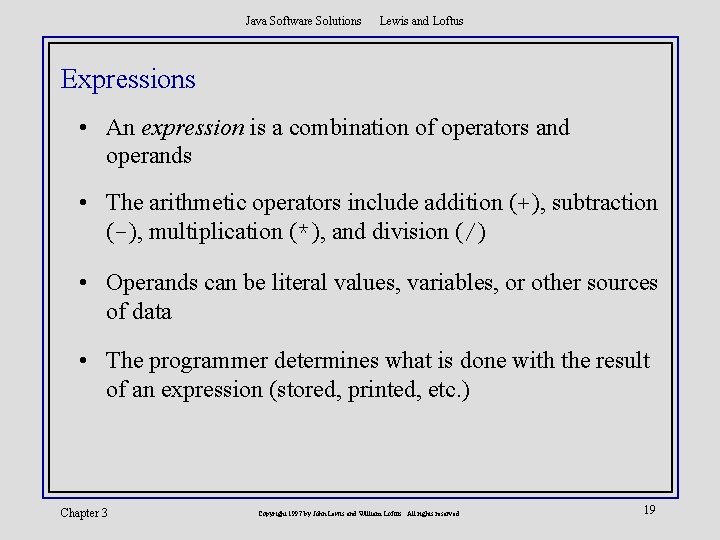
Java Software Solutions Lewis and Loftus Expressions • An expression is a combination of operators and operands • The arithmetic operators include addition (+), subtraction (-), multiplication (*), and division (/) • Operands can be literal values, variables, or other sources of data • The programmer determines what is done with the result of an expression (stored, printed, etc. ) Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 19
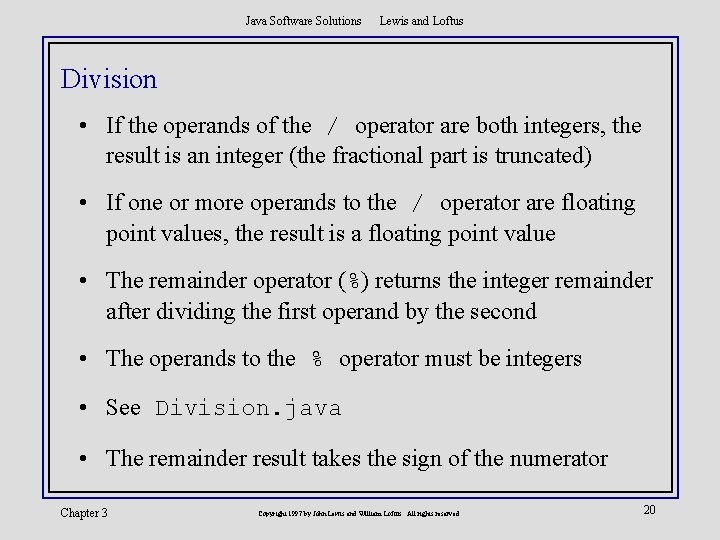
Java Software Solutions Lewis and Loftus Division • If the operands of the / operator are both integers, the result is an integer (the fractional part is truncated) • If one or more operands to the / operator are floating point values, the result is a floating point value • The remainder operator (%) returns the integer remainder after dividing the first operand by the second • The operands to the % operator must be integers • See Division. java • The remainder result takes the sign of the numerator Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 20
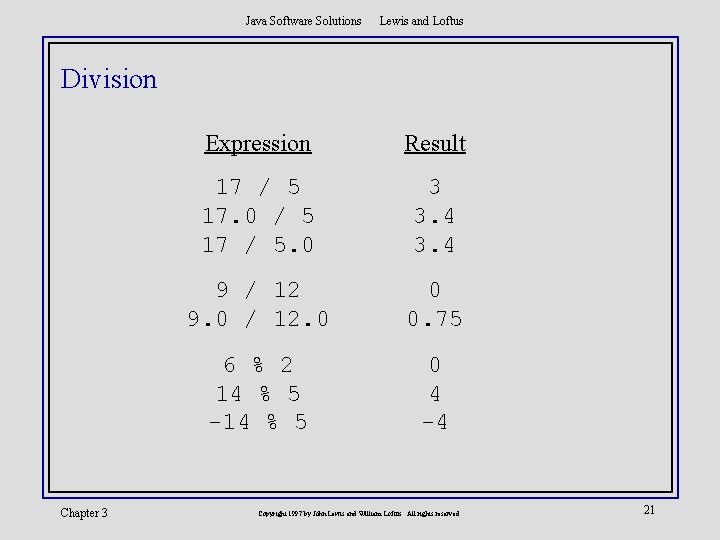
Java Software Solutions Lewis and Loftus Division Chapter 3 Expression Result 17 / 5 17. 0 / 5 17 / 5. 0 3 3. 4 9 / 12 9. 0 / 12. 0 0 0. 75 6 % 2 14 % 5 -14 % 5 0 4 -4 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 21
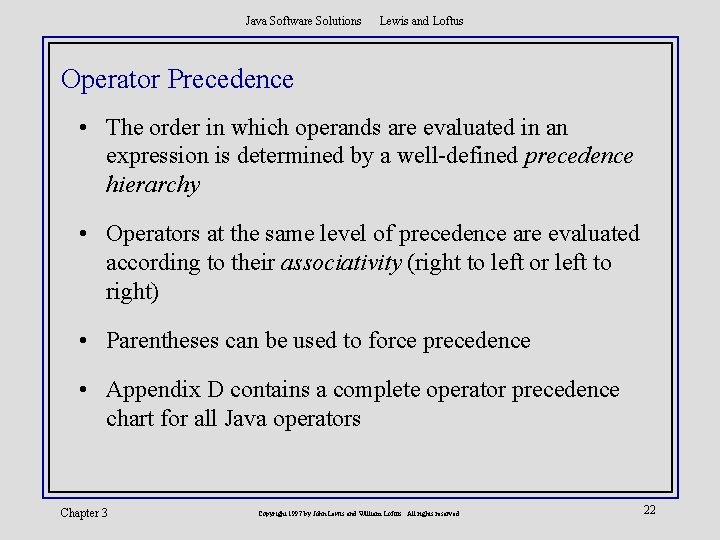
Java Software Solutions Lewis and Loftus Operator Precedence • The order in which operands are evaluated in an expression is determined by a well-defined precedence hierarchy • Operators at the same level of precedence are evaluated according to their associativity (right to left or left to right) • Parentheses can be used to force precedence • Appendix D contains a complete operator precedence chart for all Java operators Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 22
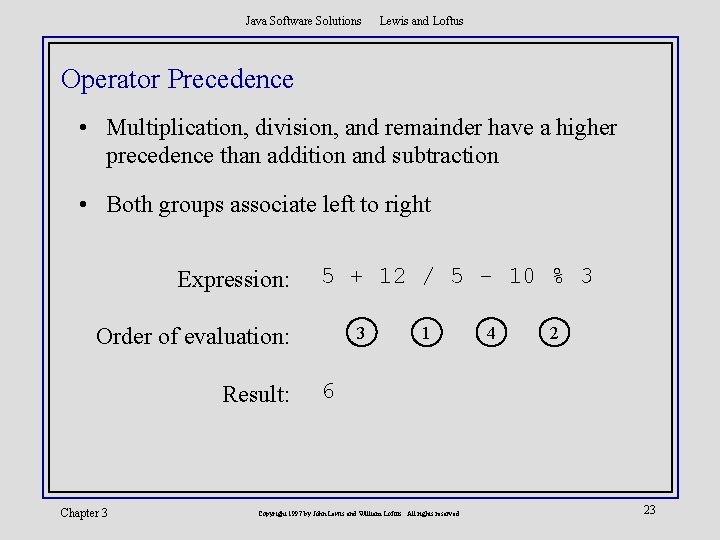
Java Software Solutions Lewis and Loftus Operator Precedence • Multiplication, division, and remainder have a higher precedence than addition and subtraction • Both groups associate left to right Expression: 5 + 12 / 5 - 10 % 3 3 Order of evaluation: Result: Chapter 3 1 4 2 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 23
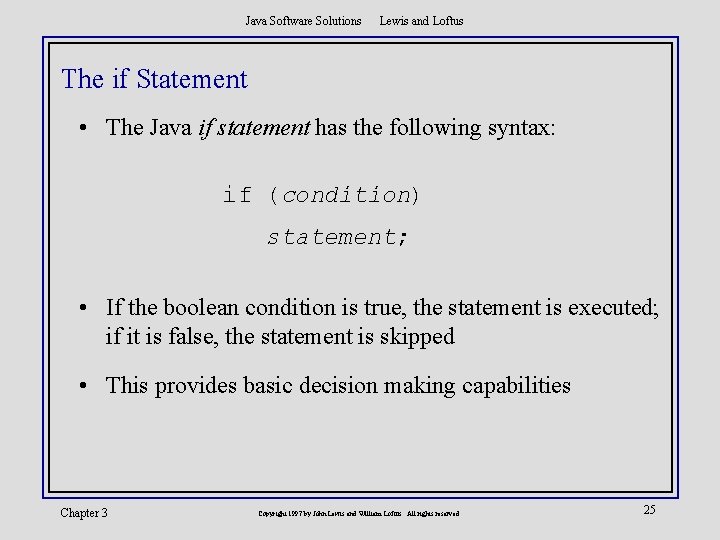
Java Software Solutions Lewis and Loftus The if Statement • The Java if statement has the following syntax: if (condition) statement; • If the boolean condition is true, the statement is executed; if it is false, the statement is skipped • This provides basic decision making capabilities Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 25
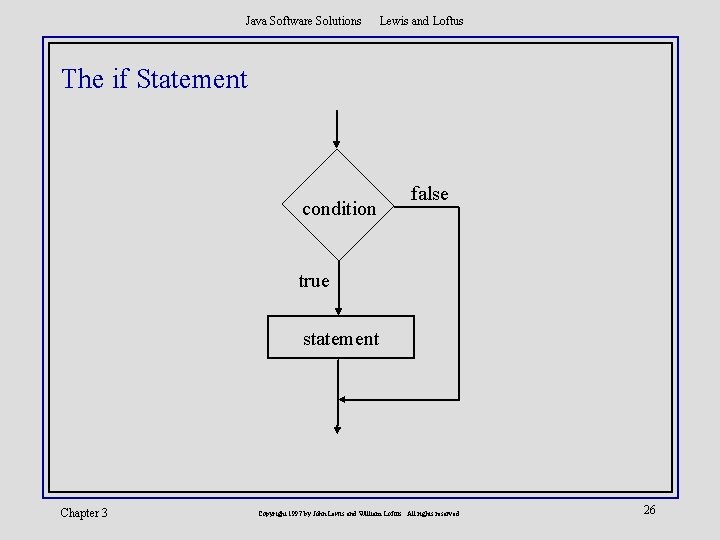
Java Software Solutions Lewis and Loftus The if Statement condition false true statement Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 26
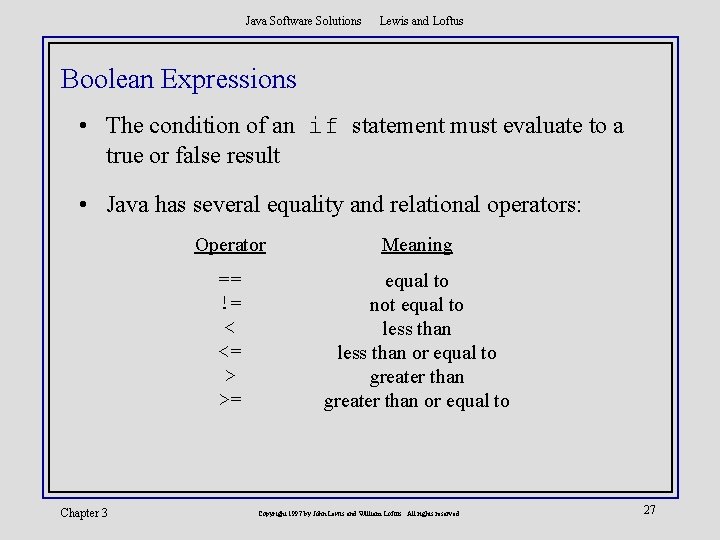
Java Software Solutions Lewis and Loftus Boolean Expressions • The condition of an if statement must evaluate to a true or false result • Java has several equality and relational operators: Chapter 3 Operator Meaning == != < <= > >= equal to not equal to less than or equal to greater than or equal to Copyright 1997 by John Lewis and William Loftus. All rights reserved. 27
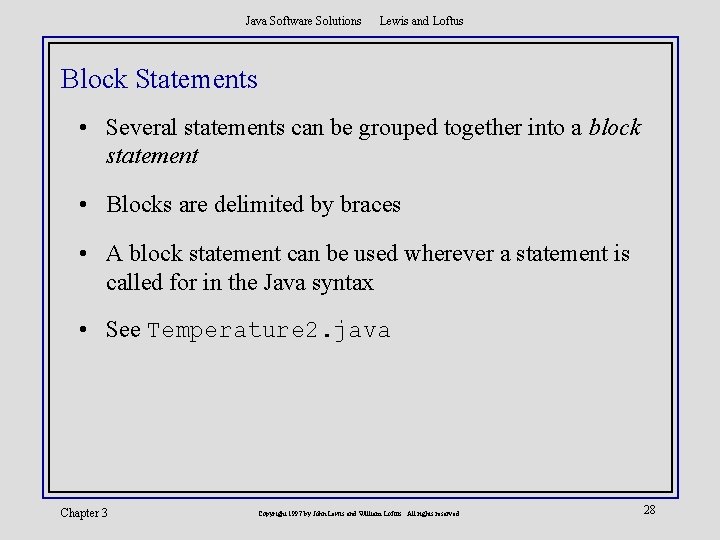
Java Software Solutions Lewis and Loftus Block Statements • Several statements can be grouped together into a block statement • Blocks are delimited by braces • A block statement can be used wherever a statement is called for in the Java syntax • See Temperature 2. java Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 28
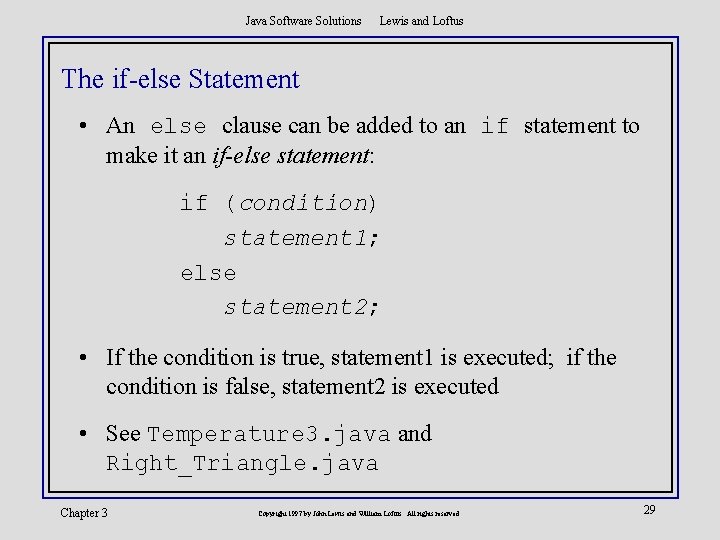
Java Software Solutions Lewis and Loftus The if-else Statement • An else clause can be added to an if statement to make it an if-else statement: if (condition) statement 1; else statement 2; • If the condition is true, statement 1 is executed; if the condition is false, statement 2 is executed • See Temperature 3. java and Right_Triangle. java Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 29
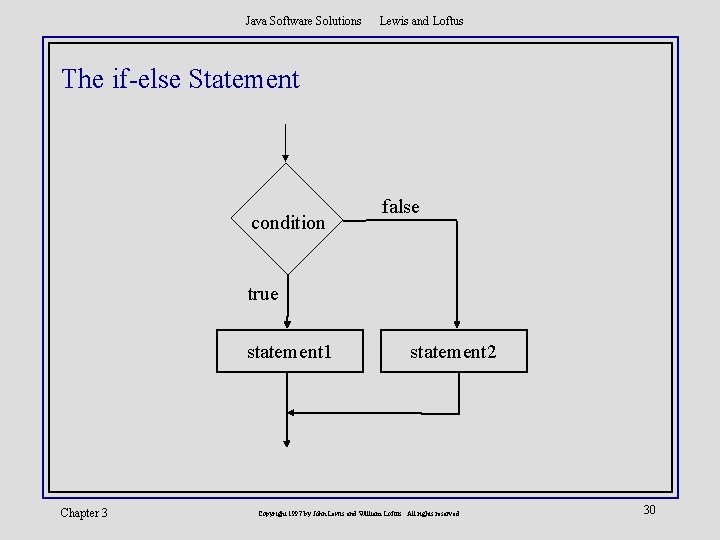
Java Software Solutions Lewis and Loftus The if-else Statement condition false true statement 1 Chapter 3 statement 2 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 30
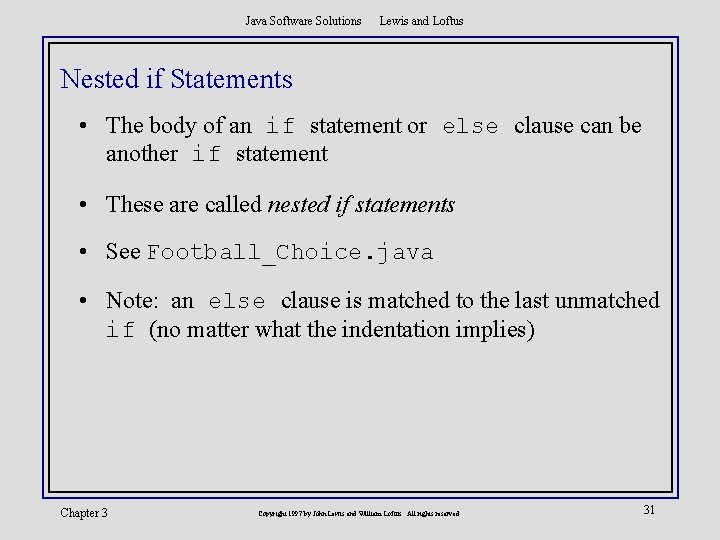
Java Software Solutions Lewis and Loftus Nested if Statements • The body of an if statement or else clause can be another if statement • These are called nested if statements • See Football_Choice. java • Note: an else clause is matched to the last unmatched if (no matter what the indentation implies) Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 31
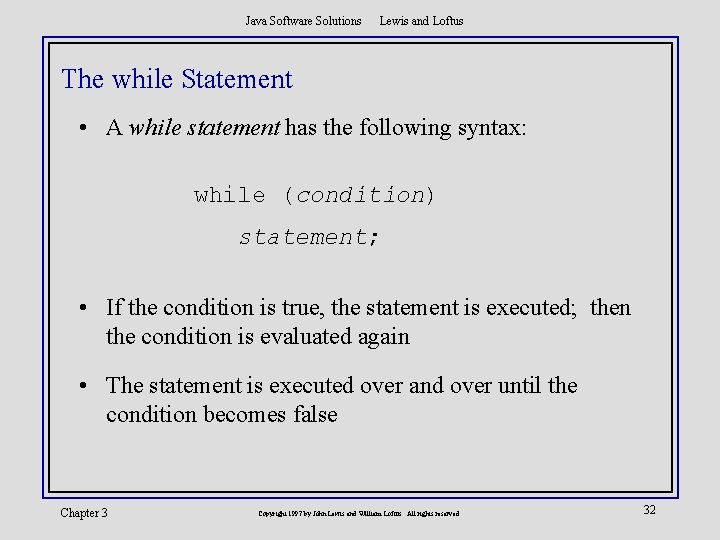
Java Software Solutions Lewis and Loftus The while Statement • A while statement has the following syntax: while (condition) statement; • If the condition is true, the statement is executed; then the condition is evaluated again • The statement is executed over and over until the condition becomes false Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 32
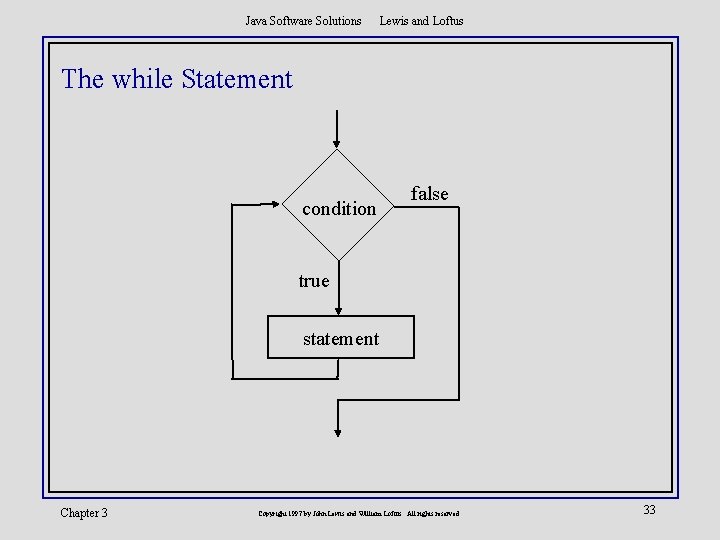
Java Software Solutions Lewis and Loftus The while Statement condition false true statement Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 33
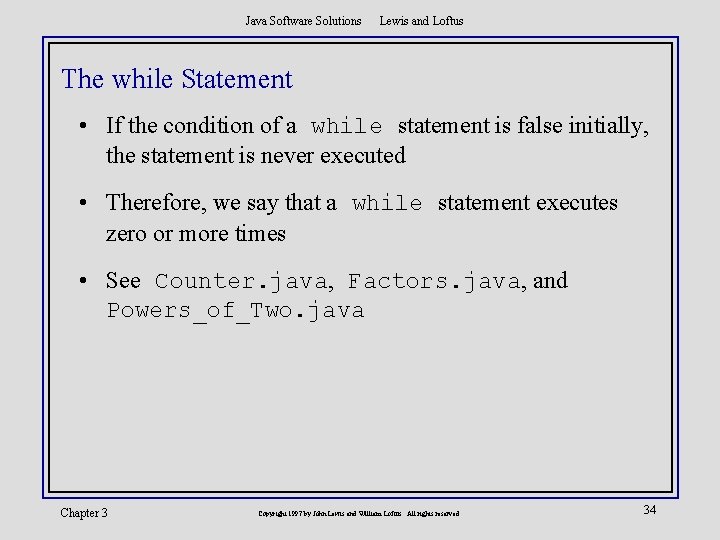
Java Software Solutions Lewis and Loftus The while Statement • If the condition of a while statement is false initially, the statement is never executed • Therefore, we say that a while statement executes zero or more times • See Counter. java, Factors. java, and Powers_of_Two. java Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 34
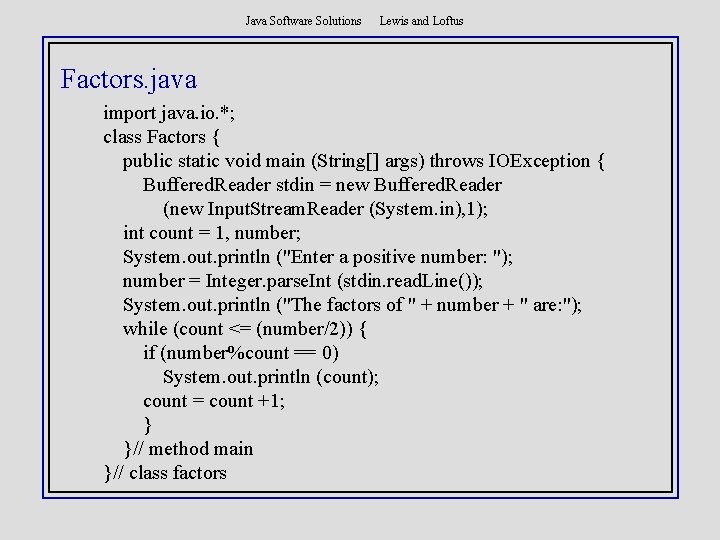
Java Software Solutions Lewis and Loftus Factors. java import java. io. *; class Factors { public static void main (String[] args) throws IOException { Buffered. Reader stdin = new Buffered. Reader (new Input. Stream. Reader (System. in), 1); int count = 1, number; System. out. println ("Enter a positive number: "); number = Integer. parse. Int (stdin. read. Line()); System. out. println ("The factors of " + number + " are: "); while (count <= (number/2)) { if (number%count == 0) System. out. println (count); count = count +1; } }// method main }// class factors
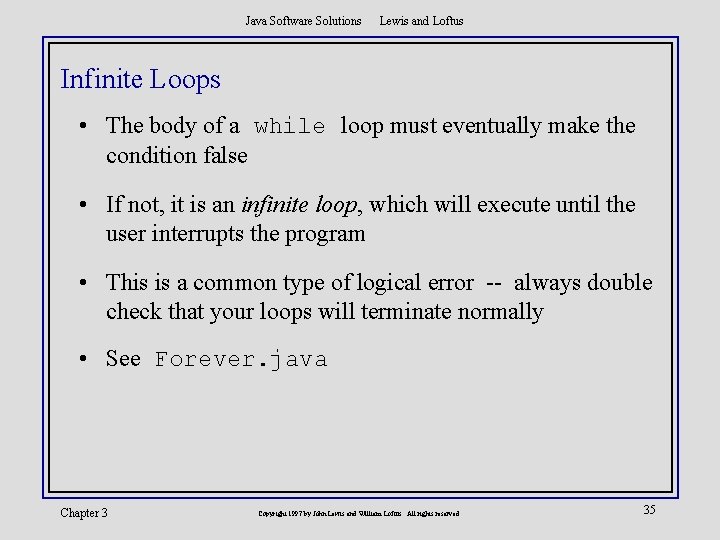
Java Software Solutions Lewis and Loftus Infinite Loops • The body of a while loop must eventually make the condition false • If not, it is an infinite loop, which will execute until the user interrupts the program • This is a common type of logical error -- always double check that your loops will terminate normally • See Forever. java Chapter 3 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 35
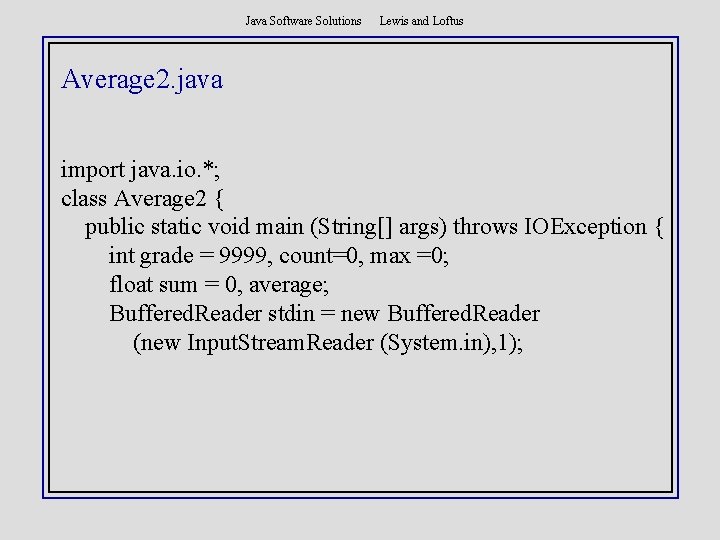
Java Software Solutions Lewis and Loftus Average 2. java import java. io. *; class Average 2 { public static void main (String[] args) throws IOException { int grade = 9999, count=0, max =0; float sum = 0, average; Buffered. Reader stdin = new Buffered. Reader (new Input. Stream. Reader (System. in), 1);
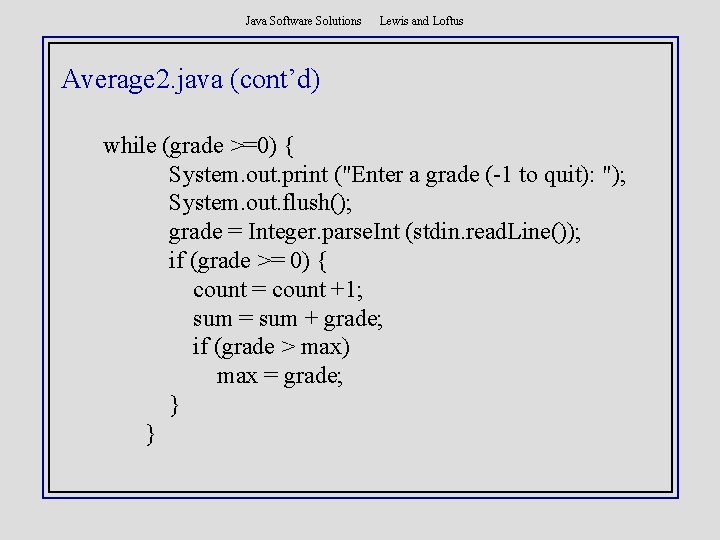
Java Software Solutions Lewis and Loftus Average 2. java (cont’d) while (grade >=0) { System. out. print ("Enter a grade (-1 to quit): "); System. out. flush(); grade = Integer. parse. Int (stdin. read. Line()); if (grade >= 0) { count = count +1; sum = sum + grade; if (grade > max) max = grade; } }
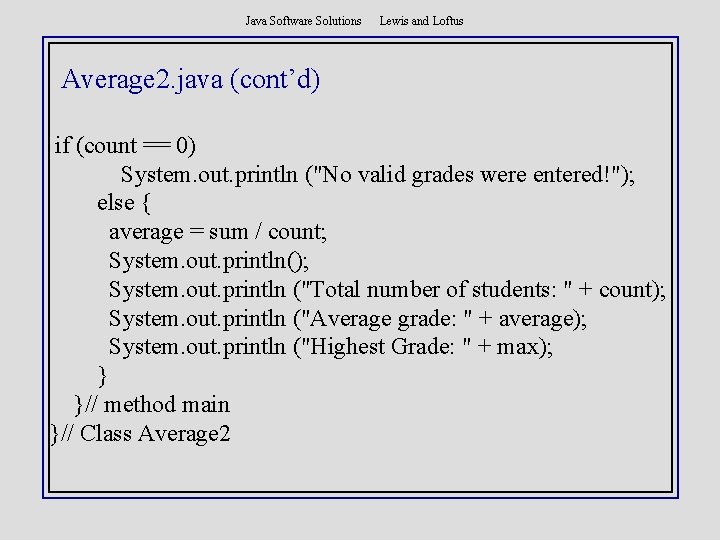
Java Software Solutions Lewis and Loftus Average 2. java (cont’d) if (count == 0) System. out. println ("No valid grades were entered!"); else { average = sum / count; System. out. println(); System. out. println ("Total number of students: " + count); System. out. println ("Average grade: " + average); System. out. println ("Highest Grade: " + max); } }// method main }// Class Average 2
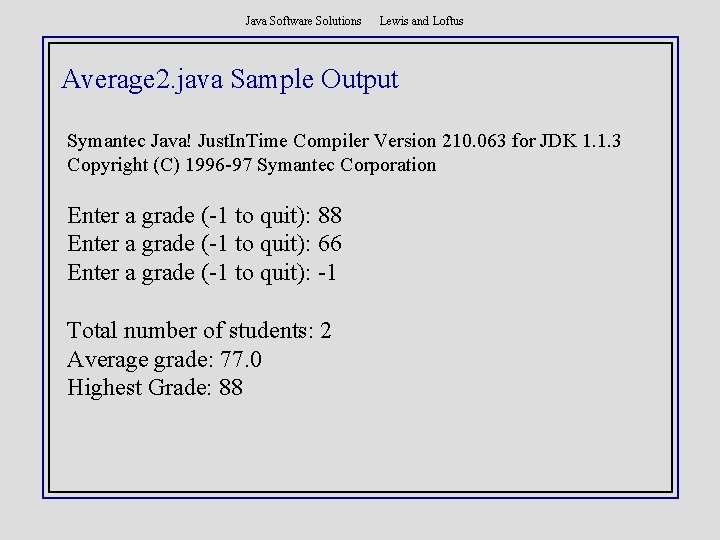
Java Software Solutions Lewis and Loftus Average 2. java Sample Output Symantec Java! Just. In. Time Compiler Version 210. 063 for JDK 1. 1. 3 Copyright (C) 1996 -97 Symantec Corporation Enter a grade (-1 to quit): 88 Enter a grade (-1 to quit): 66 Enter a grade (-1 to quit): -1 Total number of students: 2 Average grade: 77. 0 Highest Grade: 88
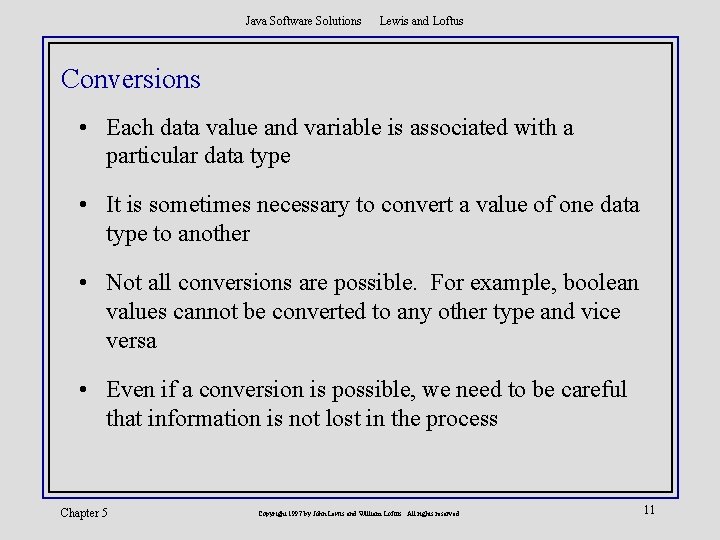
Java Software Solutions Lewis and Loftus Conversions • Each data value and variable is associated with a particular data type • It is sometimes necessary to convert a value of one data type to another • Not all conversions are possible. For example, boolean values cannot be converted to any other type and vice versa • Even if a conversion is possible, we need to be careful that information is not lost in the process Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 11
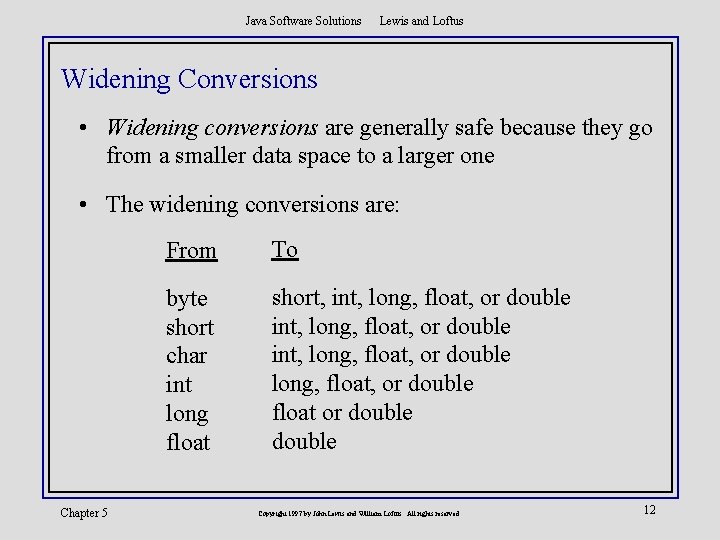
Java Software Solutions Lewis and Loftus Widening Conversions • Widening conversions are generally safe because they go from a smaller data space to a larger one • The widening conversions are: Chapter 5 From To byte short char int long float short, int, long, float, or double float or double Copyright 1997 by John Lewis and William Loftus. All rights reserved. 12
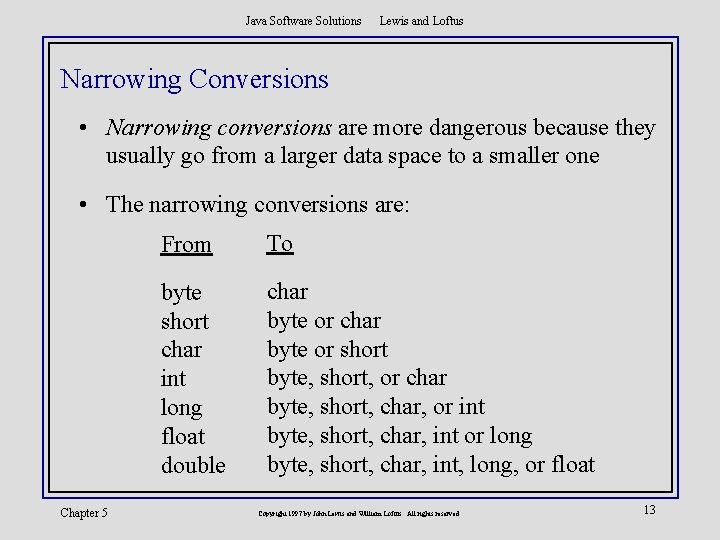
Java Software Solutions Lewis and Loftus Narrowing Conversions • Narrowing conversions are more dangerous because they usually go from a larger data space to a smaller one • The narrowing conversions are: Chapter 5 From To byte short char int long float double char byte or short byte, short, or char byte, short, char, or int byte, short, char, int or long byte, short, char, int, long, or float Copyright 1997 by John Lewis and William Loftus. All rights reserved. 13
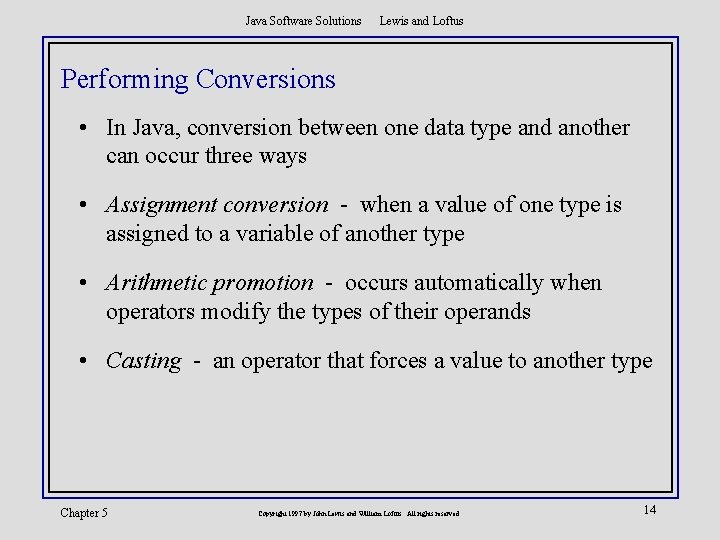
Java Software Solutions Lewis and Loftus Performing Conversions • In Java, conversion between one data type and another can occur three ways • Assignment conversion - when a value of one type is assigned to a variable of another type • Arithmetic promotion - occurs automatically when operators modify the types of their operands • Casting - an operator that forces a value to another type Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 14
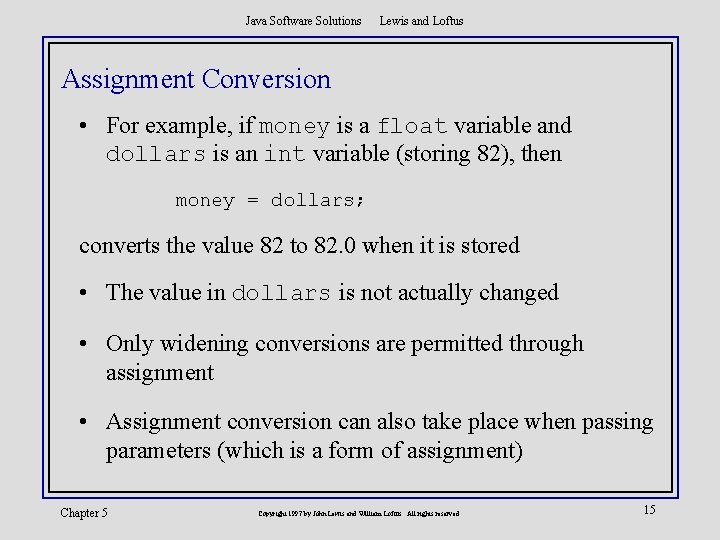
Java Software Solutions Lewis and Loftus Assignment Conversion • For example, if money is a float variable and dollars is an int variable (storing 82), then money = dollars; converts the value 82 to 82. 0 when it is stored • The value in dollars is not actually changed • Only widening conversions are permitted through assignment • Assignment conversion can also take place when passing parameters (which is a form of assignment) Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 15
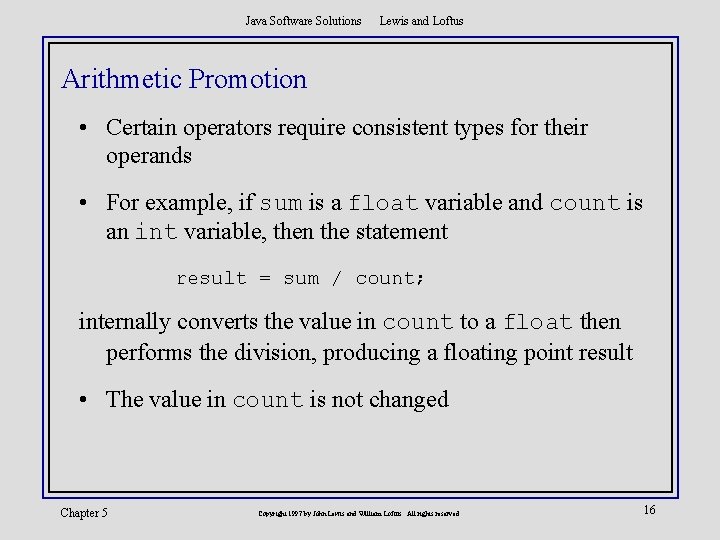
Java Software Solutions Lewis and Loftus Arithmetic Promotion • Certain operators require consistent types for their operands • For example, if sum is a float variable and count is an int variable, then the statement result = sum / count; internally converts the value in count to a float then performs the division, producing a floating point result • The value in count is not changed Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 16
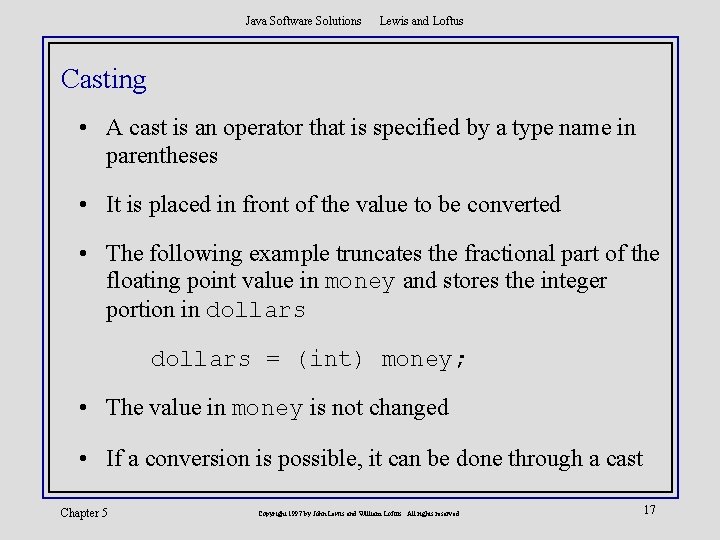
Java Software Solutions Lewis and Loftus Casting • A cast is an operator that is specified by a type name in parentheses • It is placed in front of the value to be converted • The following example truncates the fractional part of the floating point value in money and stores the integer portion in dollars = (int) money; • The value in money is not changed • If a conversion is possible, it can be done through a cast Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 17
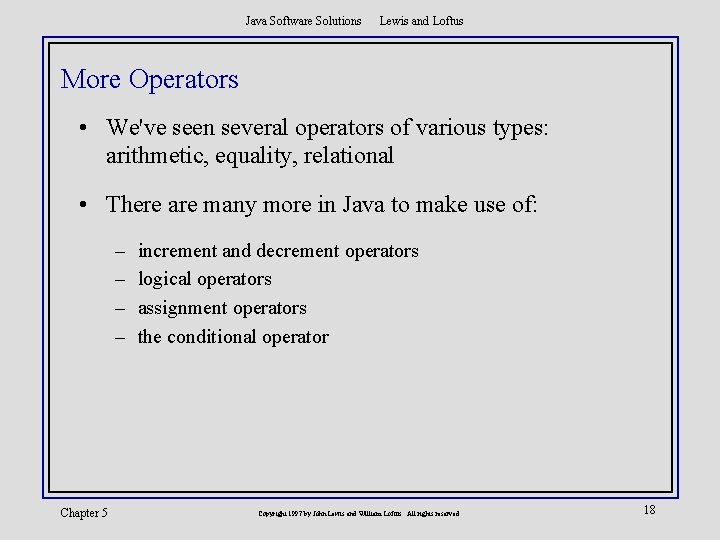
Java Software Solutions Lewis and Loftus More Operators • We've seen several operators of various types: arithmetic, equality, relational • There are many more in Java to make use of: – – Chapter 5 increment and decrement operators logical operators assignment operators the conditional operator Copyright 1997 by John Lewis and William Loftus. All rights reserved. 18
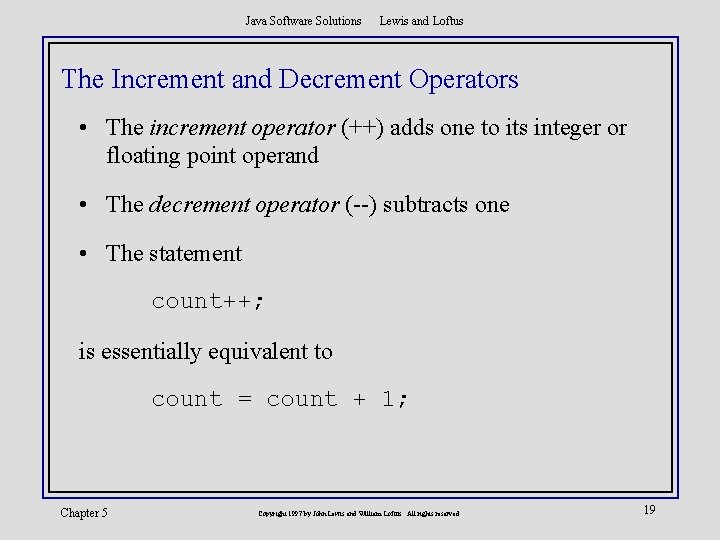
Java Software Solutions Lewis and Loftus The Increment and Decrement Operators • The increment operator (++) adds one to its integer or floating point operand • The decrement operator (--) subtracts one • The statement count++; is essentially equivalent to count = count + 1; Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 19
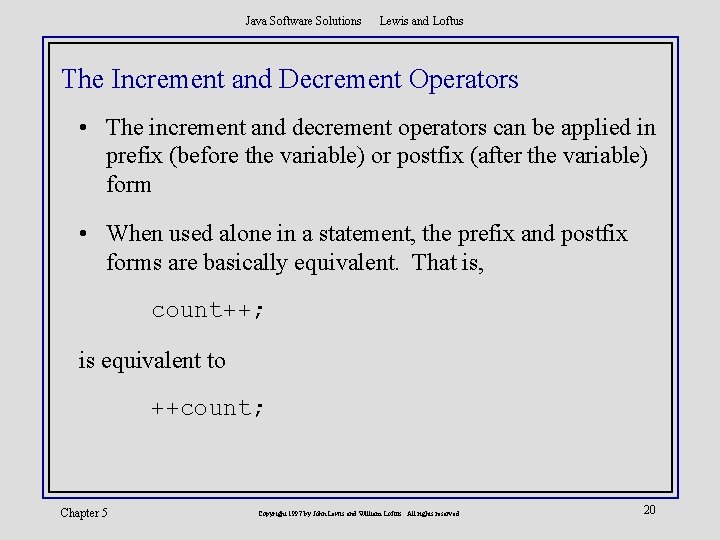
Java Software Solutions Lewis and Loftus The Increment and Decrement Operators • The increment and decrement operators can be applied in prefix (before the variable) or postfix (after the variable) form • When used alone in a statement, the prefix and postfix forms are basically equivalent. That is, count++; is equivalent to ++count; Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 20
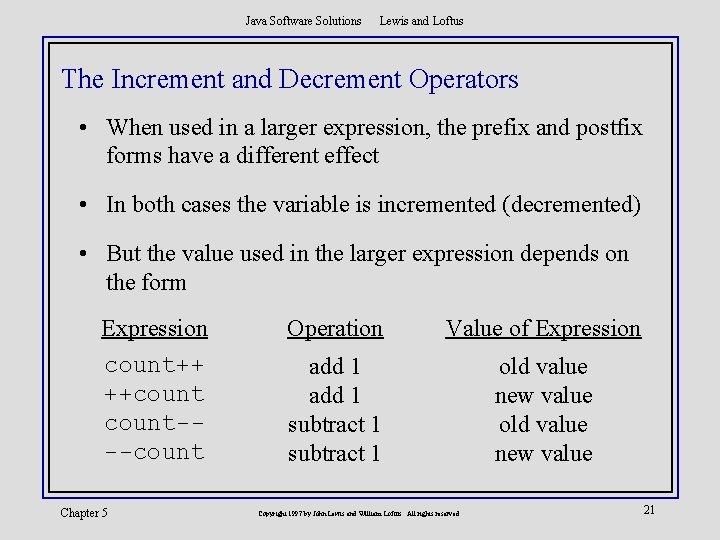
Java Software Solutions Lewis and Loftus The Increment and Decrement Operators • When used in a larger expression, the prefix and postfix forms have a different effect • In both cases the variable is incremented (decremented) • But the value used in the larger expression depends on the form Expression Operation Value of Expression count++ ++count---count add 1 subtract 1 old value new value Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 21
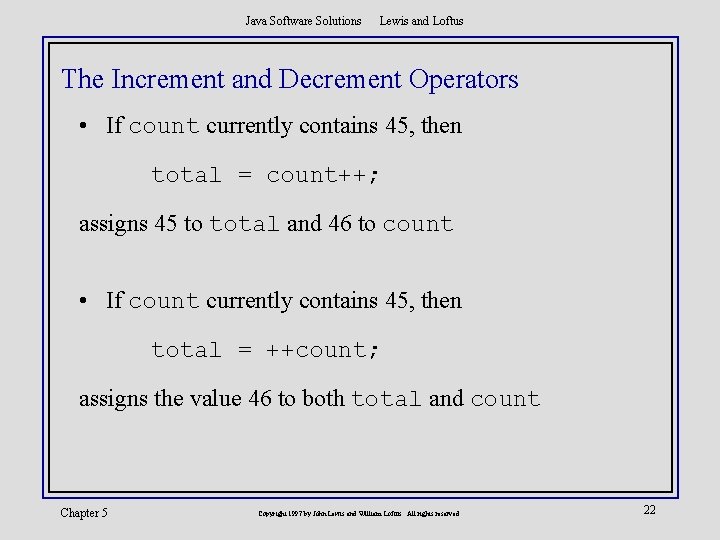
Java Software Solutions Lewis and Loftus The Increment and Decrement Operators • If count currently contains 45, then total = count++; assigns 45 to total and 46 to count • If count currently contains 45, then total = ++count; assigns the value 46 to both total and count Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 22
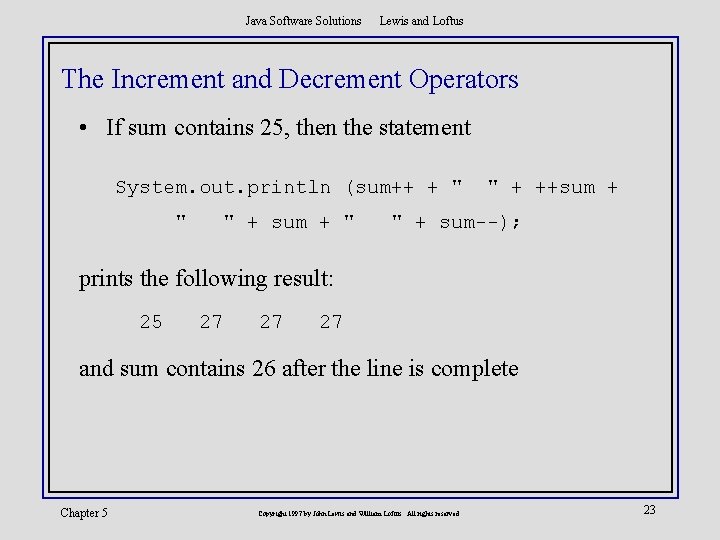
Java Software Solutions Lewis and Loftus The Increment and Decrement Operators • If sum contains 25, then the statement System. out. println (sum++ + " " " + sum + " " + ++sum + " + sum--); prints the following result: 25 27 27 27 and sum contains 26 after the line is complete Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 23
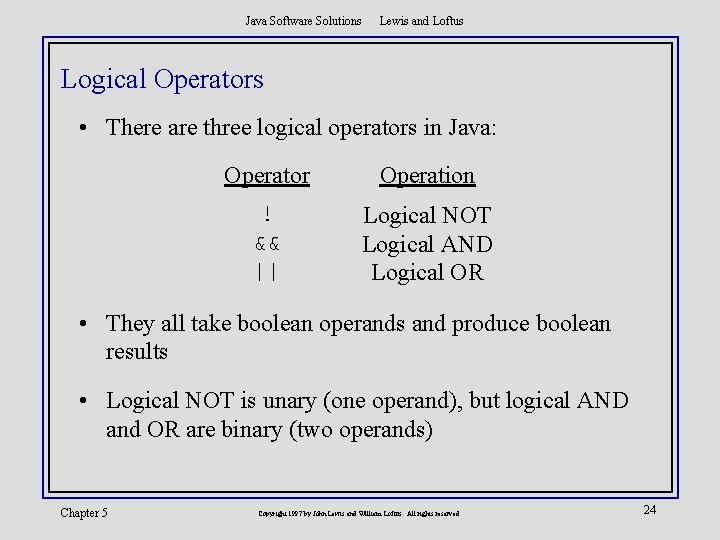
Java Software Solutions Lewis and Loftus Logical Operators • There are three logical operators in Java: Operator Operation ! && || Logical NOT Logical AND Logical OR • They all take boolean operands and produce boolean results • Logical NOT is unary (one operand), but logical AND and OR are binary (two operands) Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 24
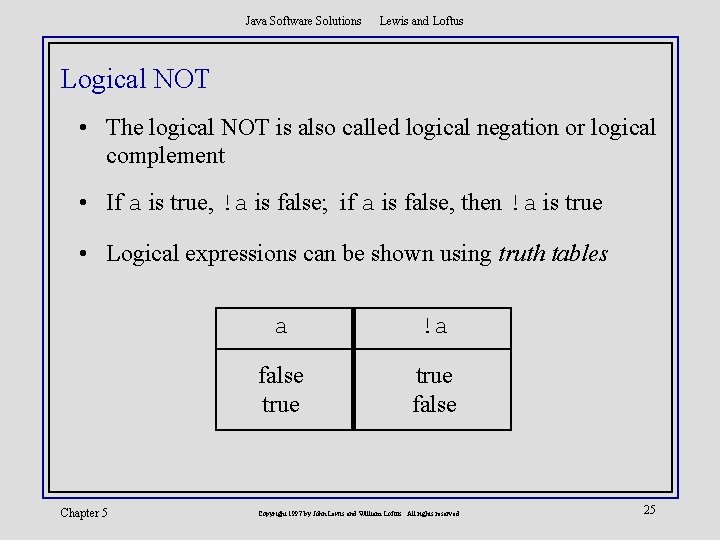
Java Software Solutions Lewis and Loftus Logical NOT • The logical NOT is also called logical negation or logical complement • If a is true, !a is false; if a is false, then !a is true • Logical expressions can be shown using truth tables Chapter 5 a !a false true false Copyright 1997 by John Lewis and William Loftus. All rights reserved. 25
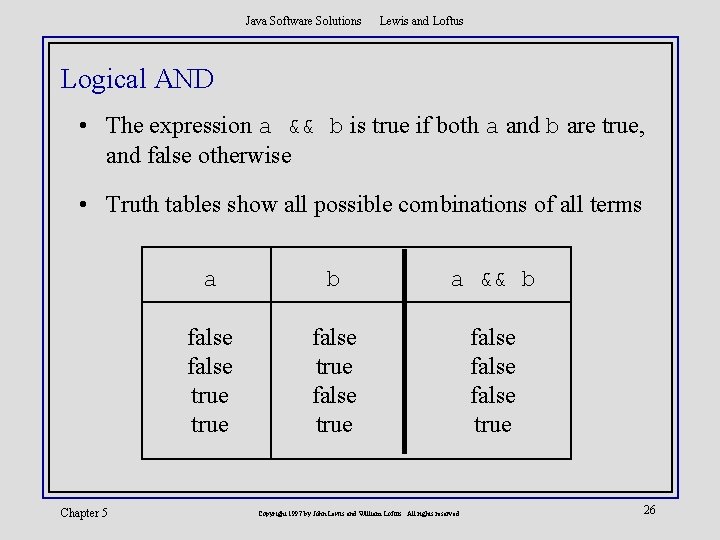
Java Software Solutions Lewis and Loftus Logical AND • The expression a && b is true if both a and b are true, and false otherwise • Truth tables show all possible combinations of all terms Chapter 5 a b a && b false true false true Copyright 1997 by John Lewis and William Loftus. All rights reserved. 26
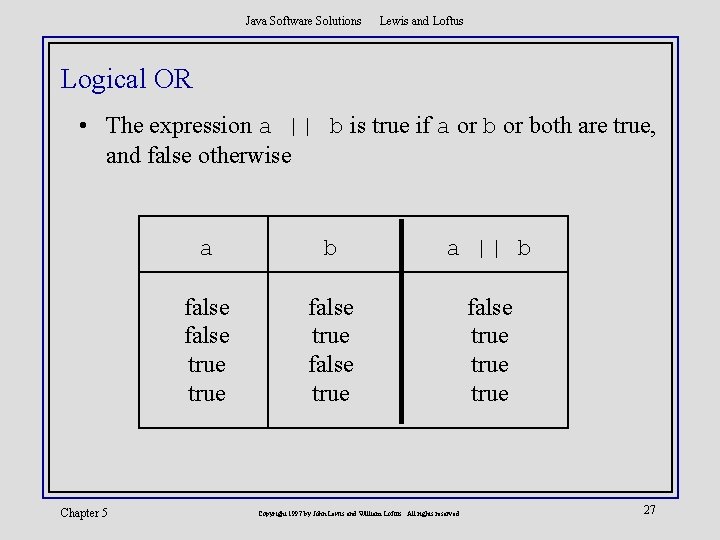
Java Software Solutions Lewis and Loftus Logical OR • The expression a || b is true if a or both are true, and false otherwise Chapter 5 a b a || b false true false true Copyright 1997 by John Lewis and William Loftus. All rights reserved. 27
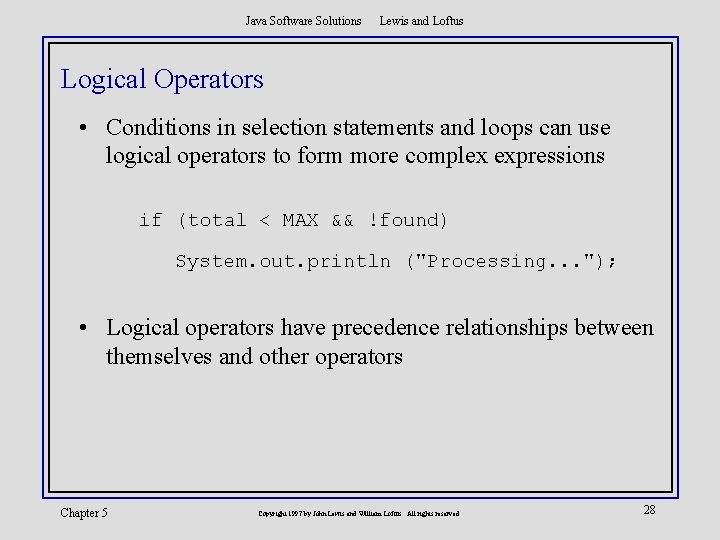
Java Software Solutions Lewis and Loftus Logical Operators • Conditions in selection statements and loops can use logical operators to form more complex expressions if (total < MAX && !found) System. out. println ("Processing. . . "); • Logical operators have precedence relationships between themselves and other operators Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 28
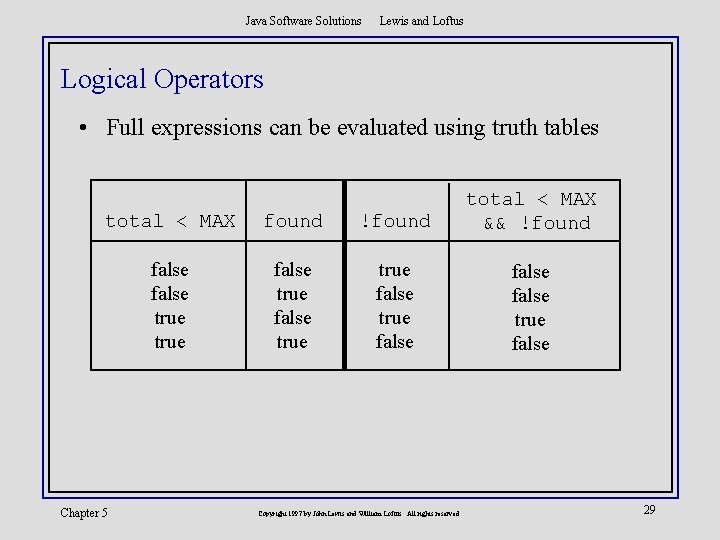
Java Software Solutions Lewis and Loftus Logical Operators • Full expressions can be evaluated using truth tables total < MAX found !found total < MAX && !found false true false true false Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 29
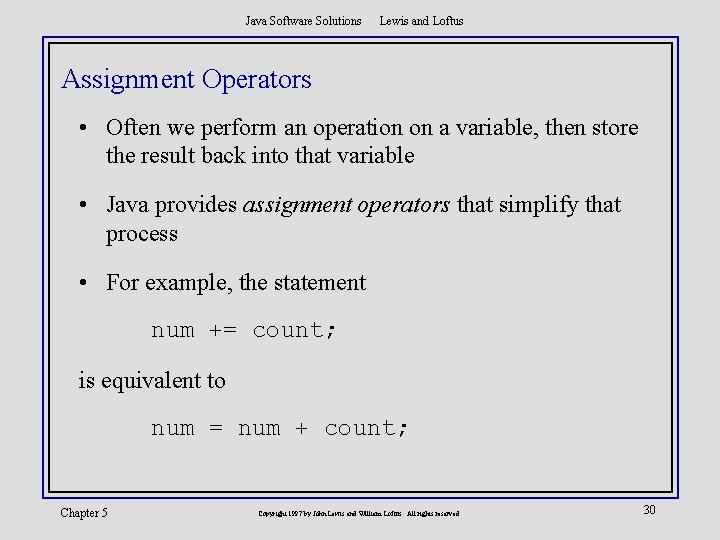
Java Software Solutions Lewis and Loftus Assignment Operators • Often we perform an operation on a variable, then store the result back into that variable • Java provides assignment operators that simplify that process • For example, the statement num += count; is equivalent to num = num + count; Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 30
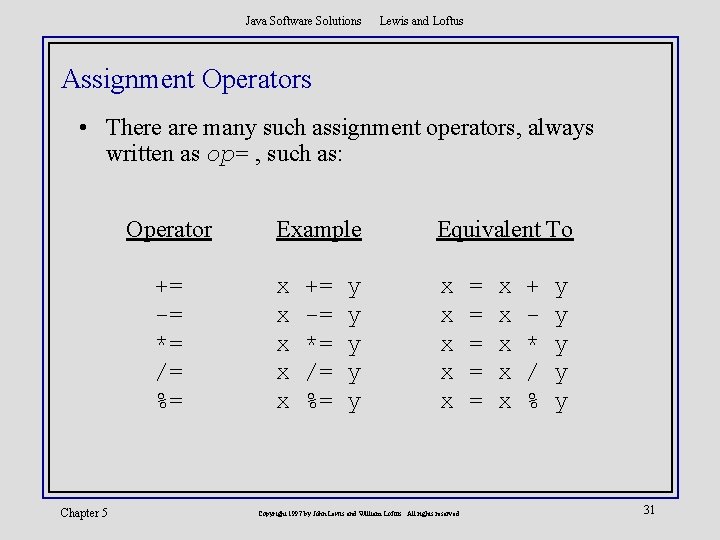
Java Software Solutions Lewis and Loftus Assignment Operators • There are many such assignment operators, always written as op= , such as: Operator += -= *= /= %= Chapter 5 Example Equivalent To x x x x x += -= *= /= %= y y y Copyright 1997 by John Lewis and William Loftus. All rights reserved. = = = x x x + * / % y y y 31
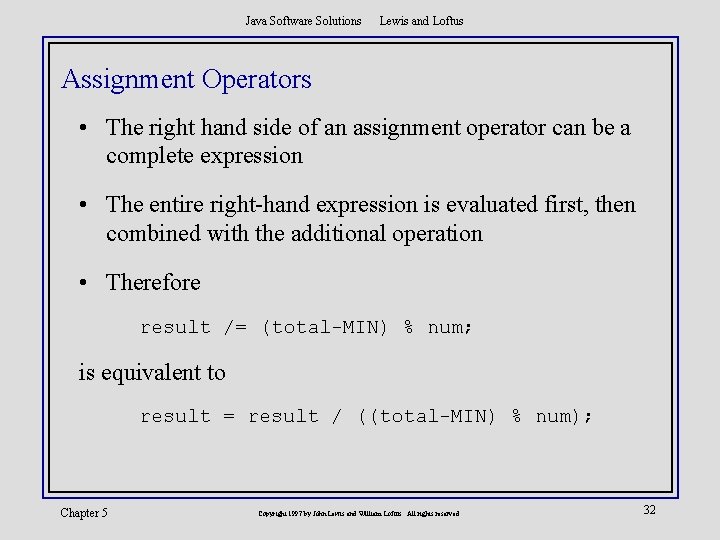
Java Software Solutions Lewis and Loftus Assignment Operators • The right hand side of an assignment operator can be a complete expression • The entire right-hand expression is evaluated first, then combined with the additional operation • Therefore result /= (total-MIN) % num; is equivalent to result = result / ((total-MIN) % num); Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 32
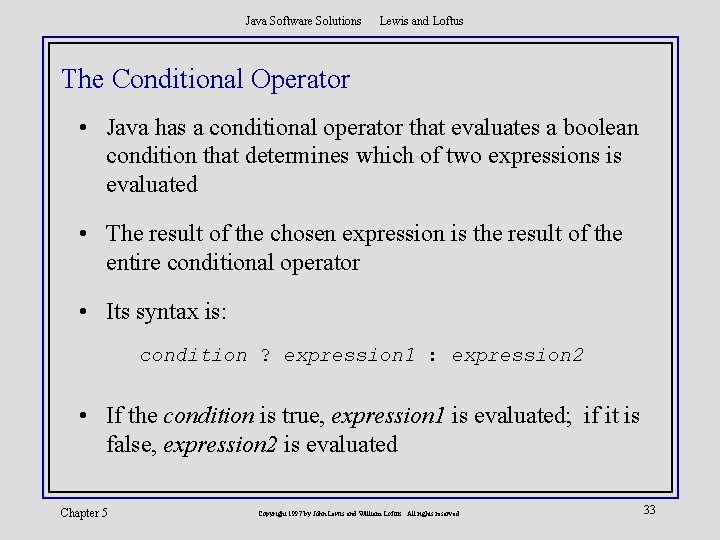
Java Software Solutions Lewis and Loftus The Conditional Operator • Java has a conditional operator that evaluates a boolean condition that determines which of two expressions is evaluated • The result of the chosen expression is the result of the entire conditional operator • Its syntax is: condition ? expression 1 : expression 2 • If the condition is true, expression 1 is evaluated; if it is false, expression 2 is evaluated Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 33
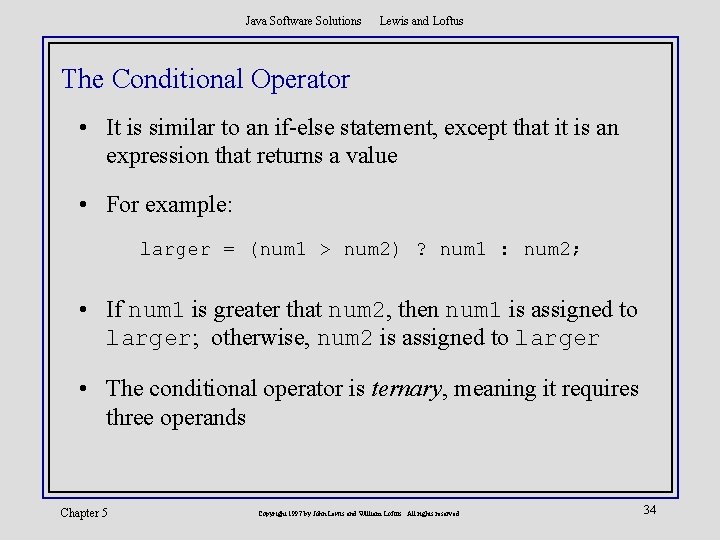
Java Software Solutions Lewis and Loftus The Conditional Operator • It is similar to an if-else statement, except that it is an expression that returns a value • For example: larger = (num 1 > num 2) ? num 1 : num 2; • If num 1 is greater that num 2, then num 1 is assigned to larger; otherwise, num 2 is assigned to larger • The conditional operator is ternary, meaning it requires three operands Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 34
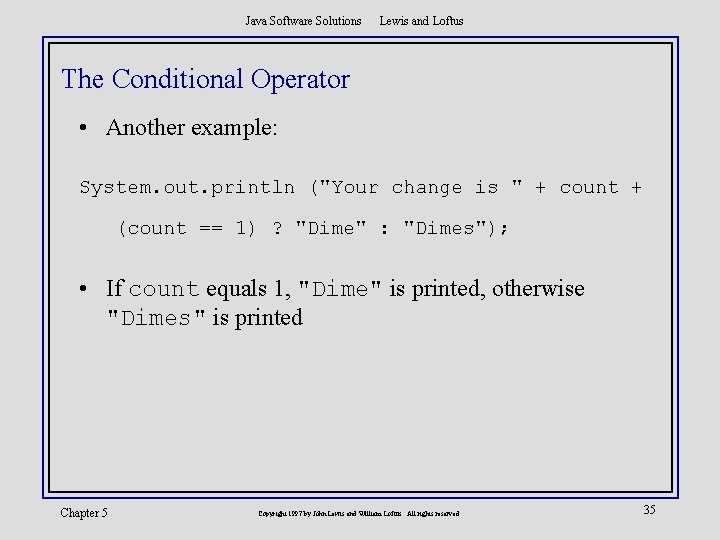
Java Software Solutions Lewis and Loftus The Conditional Operator • Another example: System. out. println ("Your change is " + count + (count == 1) ? "Dime" : "Dimes"); • If count equals 1, "Dime" is printed, otherwise "Dimes" is printed Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 35
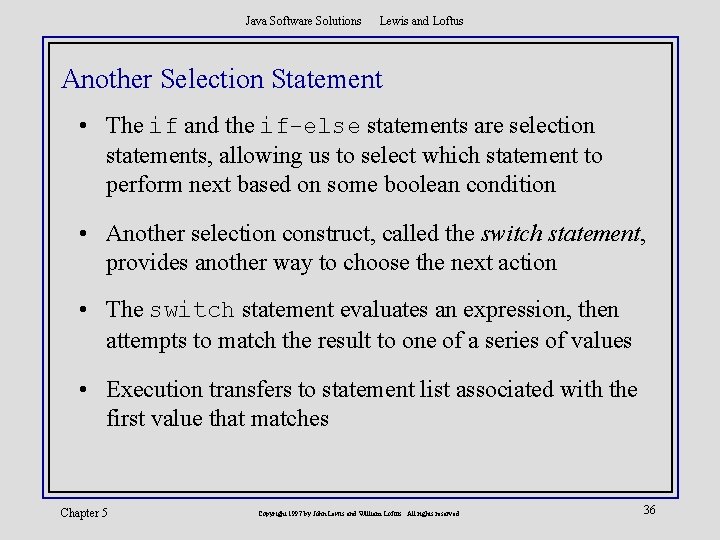
Java Software Solutions Lewis and Loftus Another Selection Statement • The if and the if-else statements are selection statements, allowing us to select which statement to perform next based on some boolean condition • Another selection construct, called the switch statement, provides another way to choose the next action • The switch statement evaluates an expression, then attempts to match the result to one of a series of values • Execution transfers to statement list associated with the first value that matches Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 36
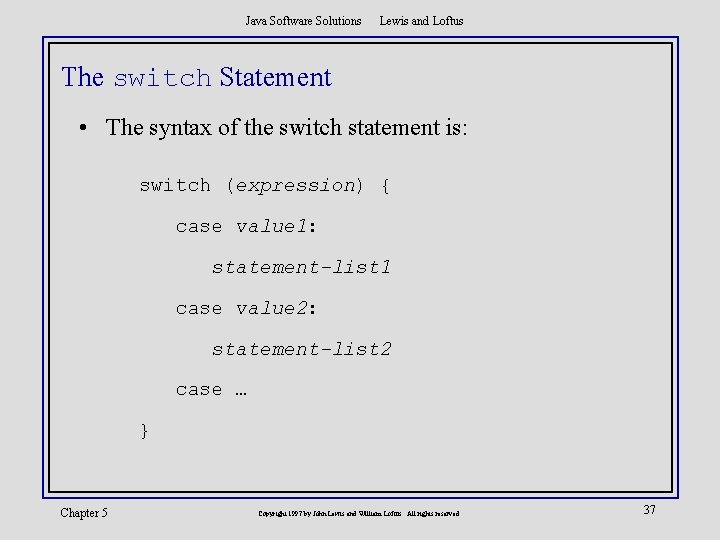
Java Software Solutions Lewis and Loftus The switch Statement • The syntax of the switch statement is: switch (expression) { case value 1: statement-list 1 case value 2: statement-list 2 case … } Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 37
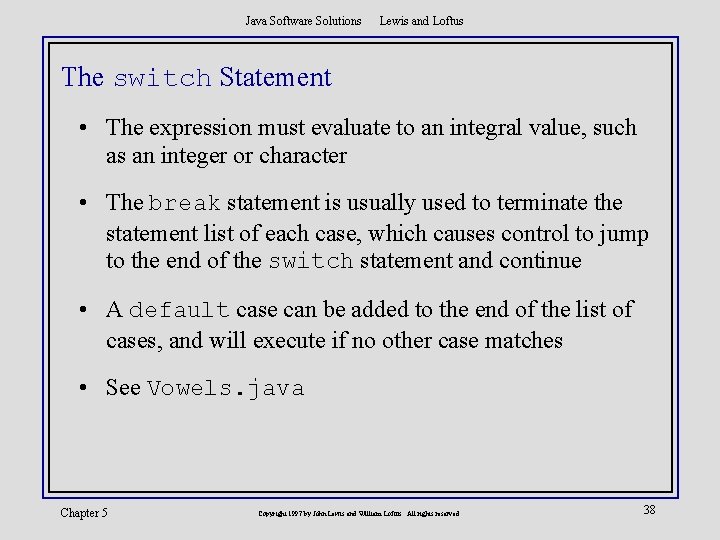
Java Software Solutions Lewis and Loftus The switch Statement • The expression must evaluate to an integral value, such as an integer or character • The break statement is usually used to terminate the statement list of each case, which causes control to jump to the end of the switch statement and continue • A default case can be added to the end of the list of cases, and will execute if no other case matches • See Vowels. java Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 38
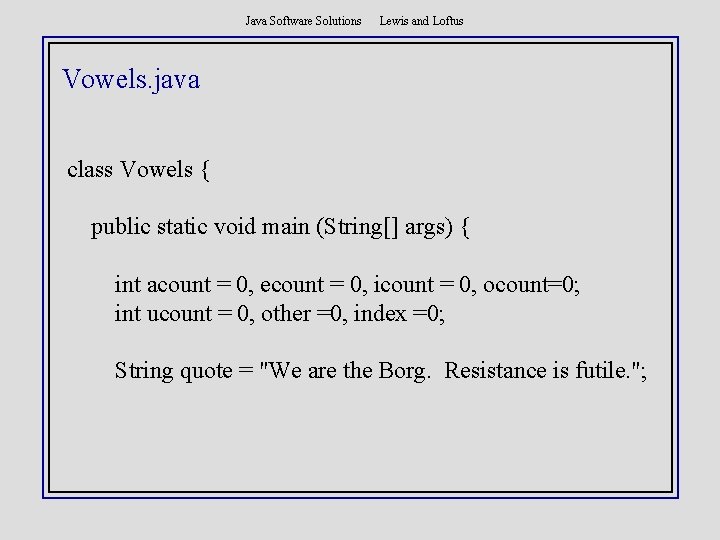
Java Software Solutions Lewis and Loftus Vowels. java class Vowels { public static void main (String[] args) { int acount = 0, ecount = 0, icount = 0, ocount=0; int ucount = 0, other =0, index =0; String quote = "We are the Borg. Resistance is futile. ";
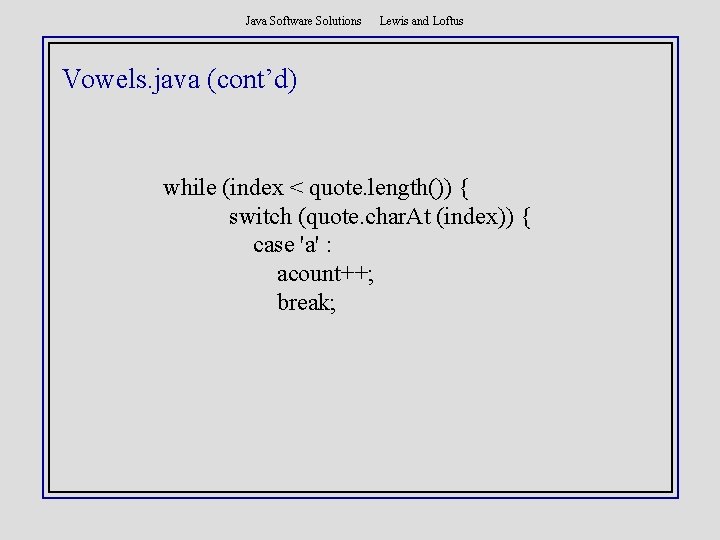
Java Software Solutions Lewis and Loftus Vowels. java (cont’d) while (index < quote. length()) { switch (quote. char. At (index)) { case 'a' : acount++; break;
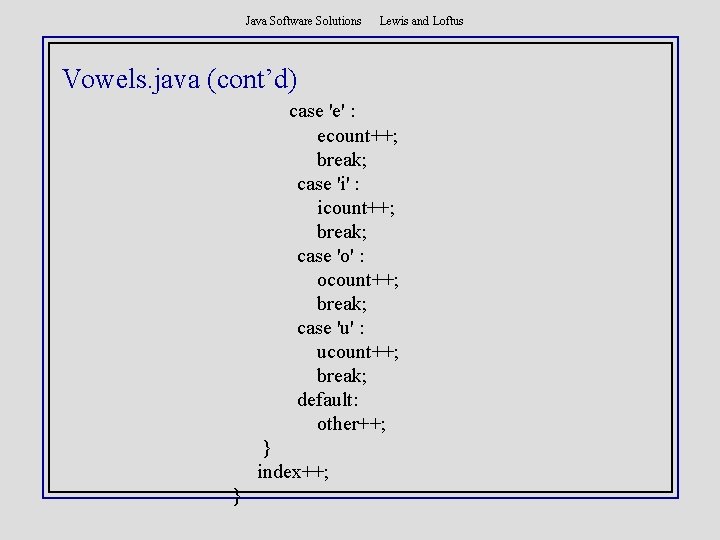
Java Software Solutions Lewis and Loftus Vowels. java (cont’d) case 'e' : ecount++; break; case 'i' : icount++; break; case 'o' : ocount++; break; case 'u' : ucount++; break; default: other++; } index++; }
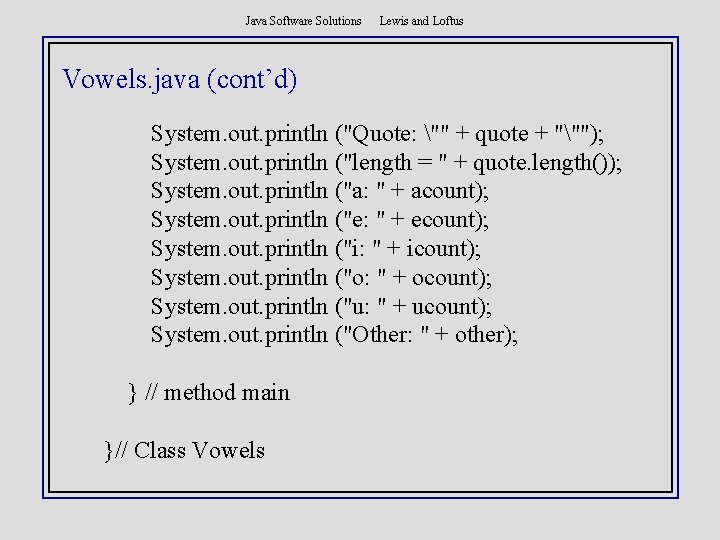
Java Software Solutions Lewis and Loftus Vowels. java (cont’d) System. out. println ("Quote: "" + quote + """); System. out. println ("length = " + quote. length()); System. out. println ("a: " + acount); System. out. println ("e: " + ecount); System. out. println ("i: " + icount); System. out. println ("o: " + ocount); System. out. println ("u: " + ucount); System. out. println ("Other: " + other); } // method main }// Class Vowels
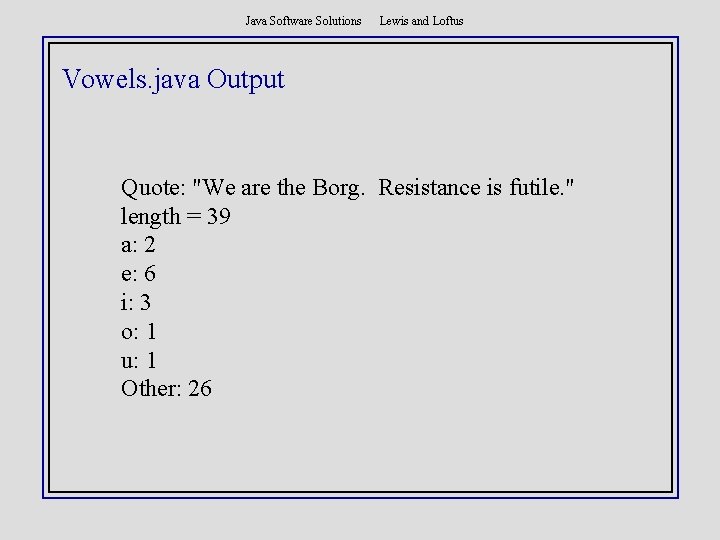
Java Software Solutions Lewis and Loftus Vowels. java Output Quote: "We are the Borg. Resistance is futile. " length = 39 a: 2 e: 6 i: 3 o: 1 u: 1 Other: 26
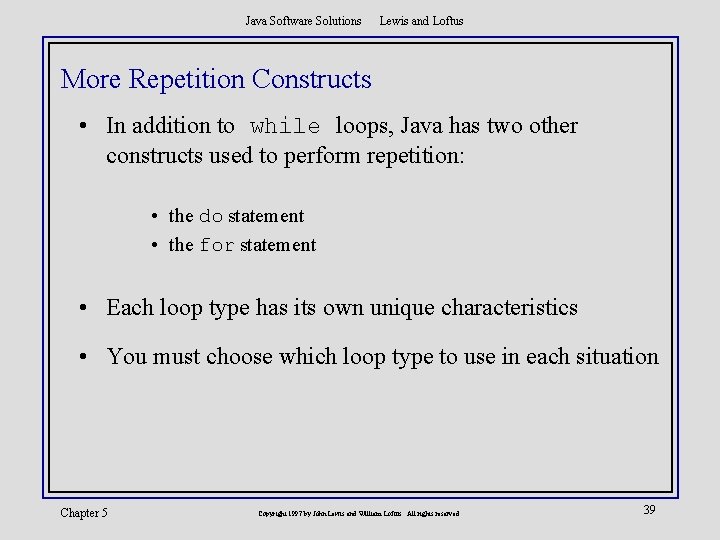
Java Software Solutions Lewis and Loftus More Repetition Constructs • In addition to while loops, Java has two other constructs used to perform repetition: • the do statement • the for statement • Each loop type has its own unique characteristics • You must choose which loop type to use in each situation Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 39
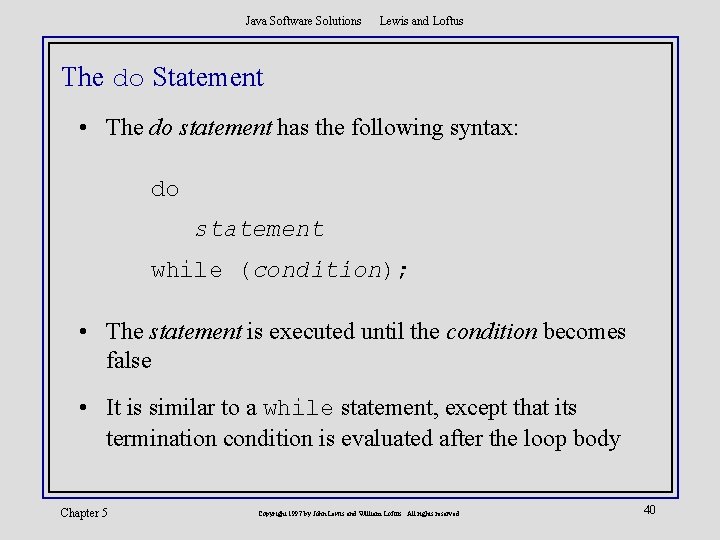
Java Software Solutions Lewis and Loftus The do Statement • The do statement has the following syntax: do statement while (condition); • The statement is executed until the condition becomes false • It is similar to a while statement, except that its termination condition is evaluated after the loop body Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 40
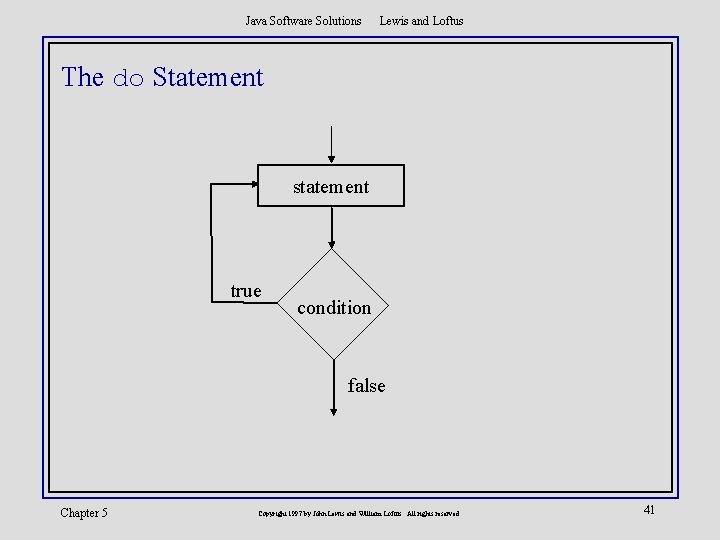
Java Software Solutions Lewis and Loftus The do Statement statement true condition false Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 41
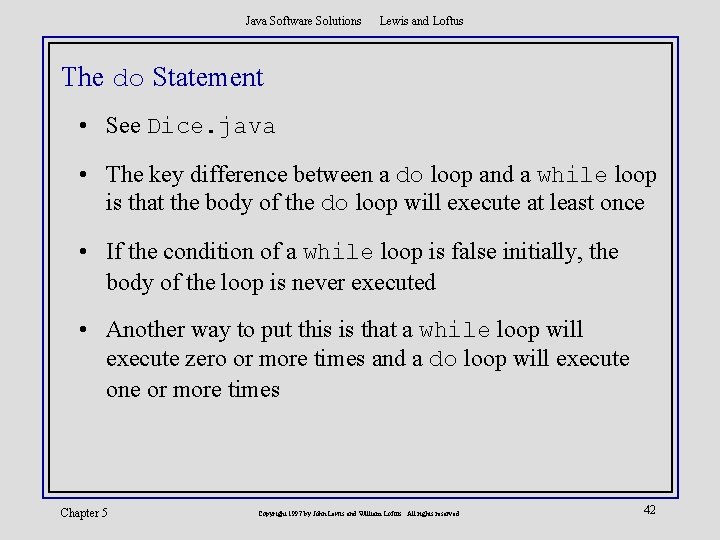
Java Software Solutions Lewis and Loftus The do Statement • See Dice. java • The key difference between a do loop and a while loop is that the body of the do loop will execute at least once • If the condition of a while loop is false initially, the body of the loop is never executed • Another way to put this is that a while loop will execute zero or more times and a do loop will execute one or more times Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 42
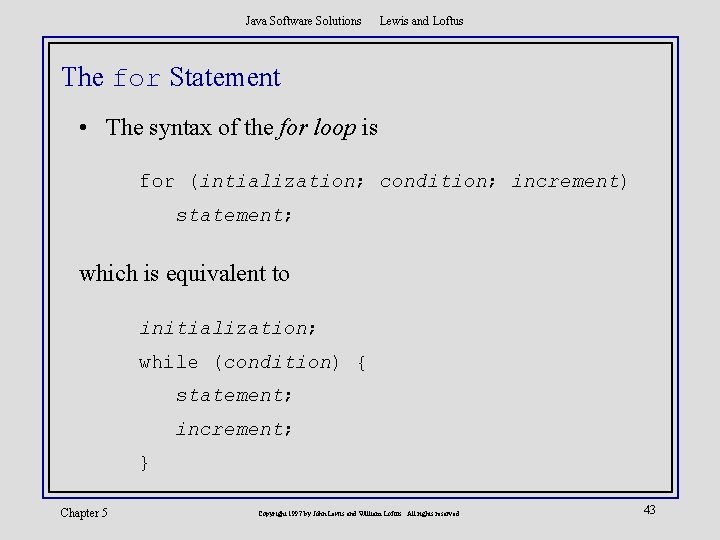
Java Software Solutions Lewis and Loftus The for Statement • The syntax of the for loop is for (intialization; condition; increment) statement; which is equivalent to initialization; while (condition) { statement; increment; } Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 43
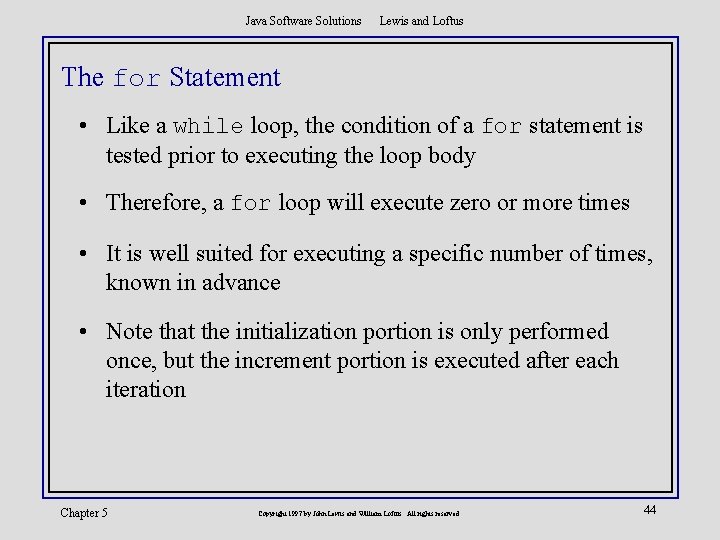
Java Software Solutions Lewis and Loftus The for Statement • Like a while loop, the condition of a for statement is tested prior to executing the loop body • Therefore, a for loop will execute zero or more times • It is well suited for executing a specific number of times, known in advance • Note that the initialization portion is only performed once, but the increment portion is executed after each iteration Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 44
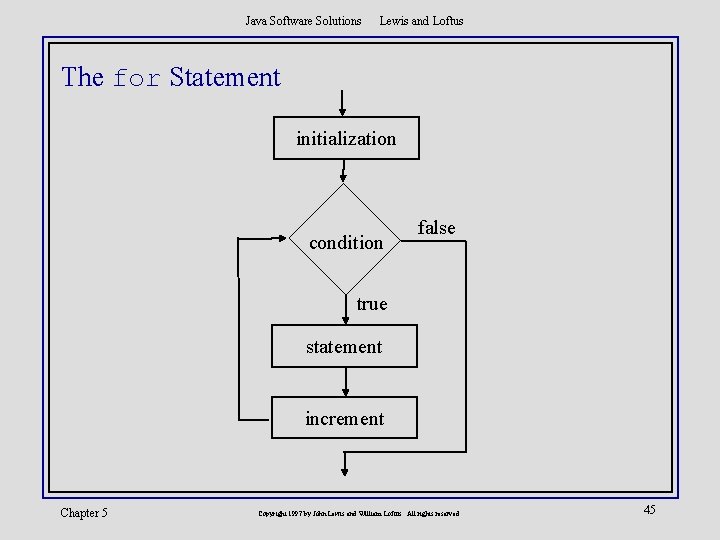
Java Software Solutions Lewis and Loftus The for Statement initialization condition false true statement increment Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 45
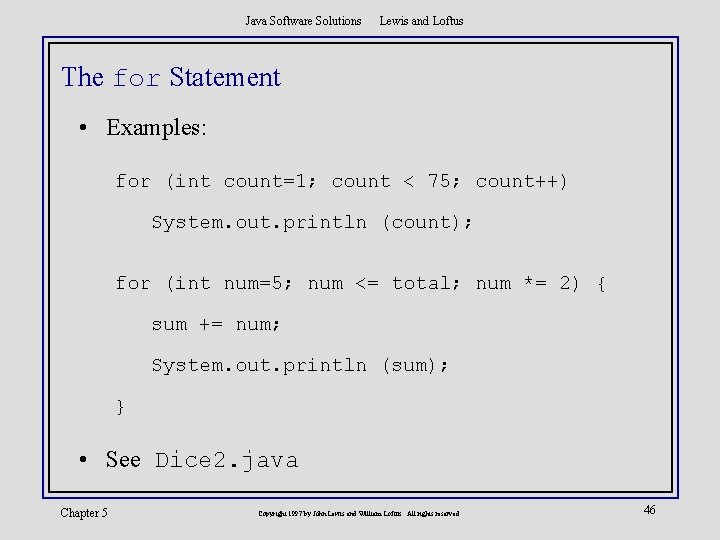
Java Software Solutions Lewis and Loftus The for Statement • Examples: for (int count=1; count < 75; count++) System. out. println (count); for (int num=5; num <= total; num *= 2) { sum += num; System. out. println (sum); } • See Dice 2. java Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 46
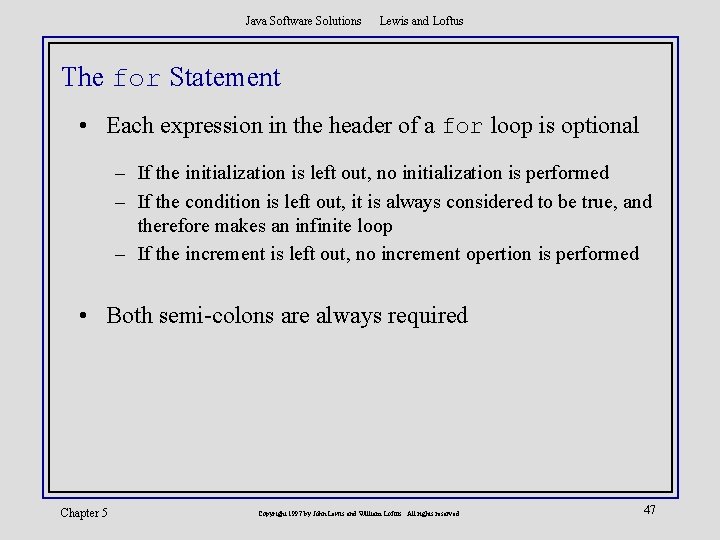
Java Software Solutions Lewis and Loftus The for Statement • Each expression in the header of a for loop is optional – If the initialization is left out, no initialization is performed – If the condition is left out, it is always considered to be true, and therefore makes an infinite loop – If the increment is left out, no increment opertion is performed • Both semi-colons are always required Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 47
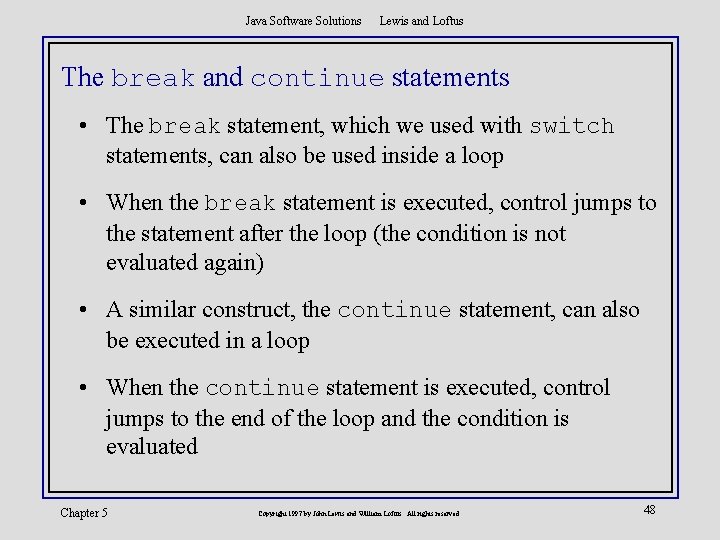
Java Software Solutions Lewis and Loftus The break and continue statements • The break statement, which we used with switch statements, can also be used inside a loop • When the break statement is executed, control jumps to the statement after the loop (the condition is not evaluated again) • A similar construct, the continue statement, can also be executed in a loop • When the continue statement is executed, control jumps to the end of the loop and the condition is evaluated Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 48
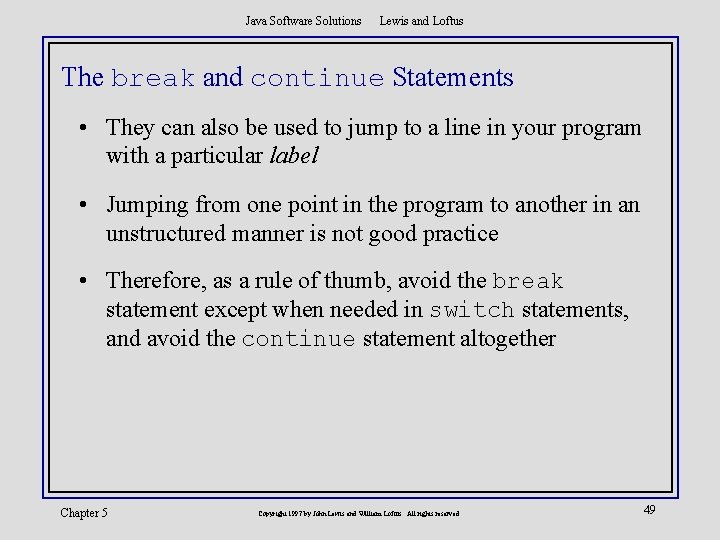
Java Software Solutions Lewis and Loftus The break and continue Statements • They can also be used to jump to a line in your program with a particular label • Jumping from one point in the program to another in an unstructured manner is not good practice • Therefore, as a rule of thumb, avoid the break statement except when needed in switch statements, and avoid the continue statement altogether Chapter 5 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 49
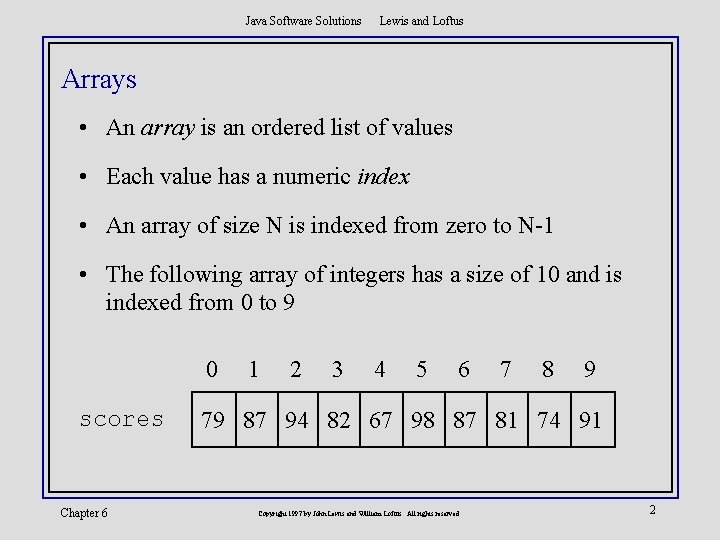
Java Software Solutions Lewis and Loftus Arrays • An array is an ordered list of values • Each value has a numeric index • An array of size N is indexed from zero to N-1 • The following array of integers has a size of 10 and is indexed from 0 to 9 0 scores Chapter 6 1 2 3 4 5 6 7 8 9 79 87 94 82 67 98 87 81 74 91 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 2
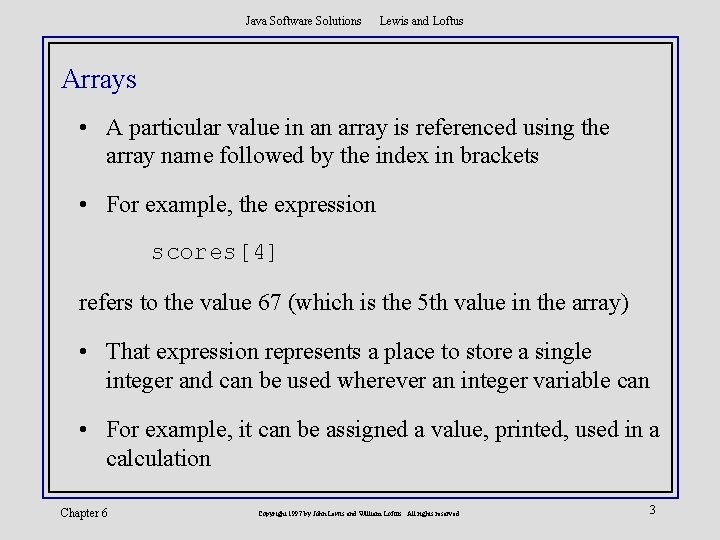
Java Software Solutions Lewis and Loftus Arrays • A particular value in an array is referenced using the array name followed by the index in brackets • For example, the expression scores[4] refers to the value 67 (which is the 5 th value in the array) • That expression represents a place to store a single integer and can be used wherever an integer variable can • For example, it can be assigned a value, printed, used in a calculation Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 3
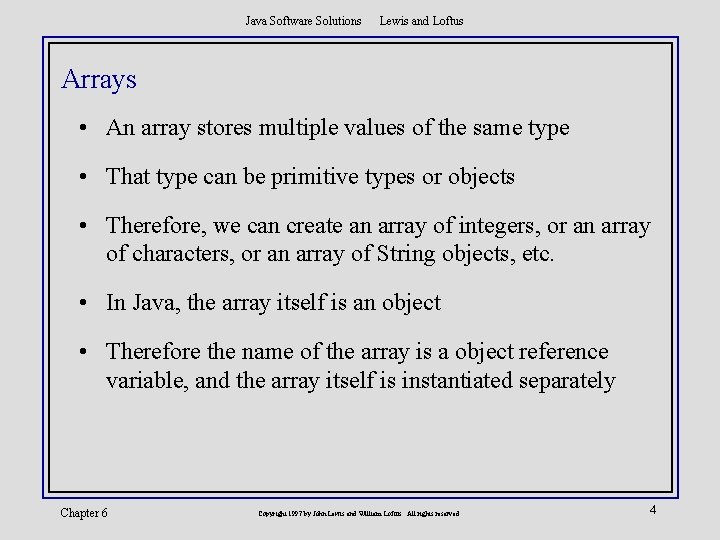
Java Software Solutions Lewis and Loftus Arrays • An array stores multiple values of the same type • That type can be primitive types or objects • Therefore, we can create an array of integers, or an array of characters, or an array of String objects, etc. • In Java, the array itself is an object • Therefore the name of the array is a object reference variable, and the array itself is instantiated separately Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 4
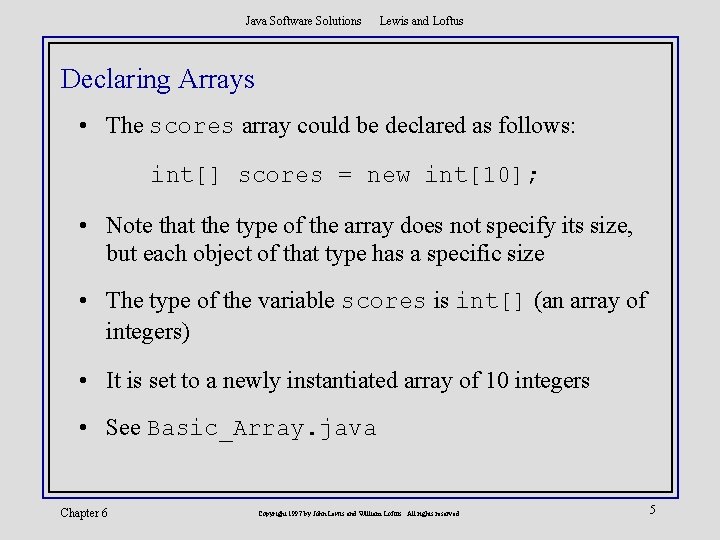
Java Software Solutions Lewis and Loftus Declaring Arrays • The scores array could be declared as follows: int[] scores = new int[10]; • Note that the type of the array does not specify its size, but each object of that type has a specific size • The type of the variable scores is int[] (an array of integers) • It is set to a newly instantiated array of 10 integers • See Basic_Array. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 5
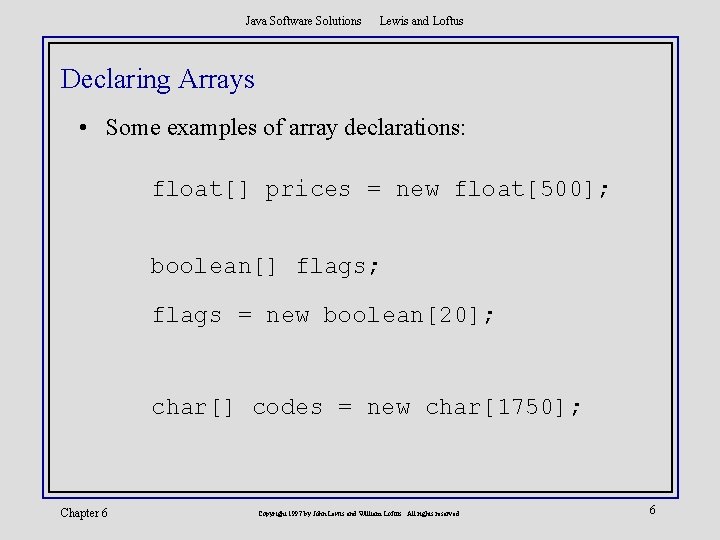
Java Software Solutions Lewis and Loftus Declaring Arrays • Some examples of array declarations: float[] prices = new float[500]; boolean[] flags; flags = new boolean[20]; char[] codes = new char[1750]; Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 6
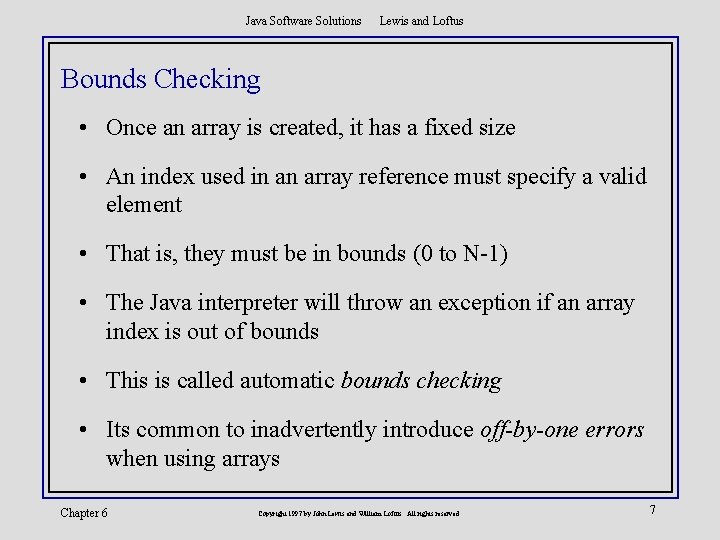
Java Software Solutions Lewis and Loftus Bounds Checking • Once an array is created, it has a fixed size • An index used in an array reference must specify a valid element • That is, they must be in bounds (0 to N-1) • The Java interpreter will throw an exception if an array index is out of bounds • This is called automatic bounds checking • Its common to inadvertently introduce off-by-one errors when using arrays Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 7
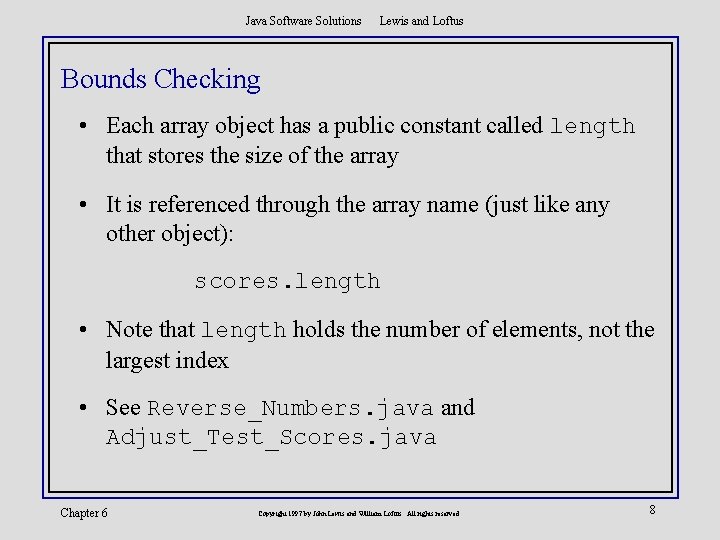
Java Software Solutions Lewis and Loftus Bounds Checking • Each array object has a public constant called length that stores the size of the array • It is referenced through the array name (just like any other object): scores. length • Note that length holds the number of elements, not the largest index • See Reverse_Numbers. java and Adjust_Test_Scores. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 8
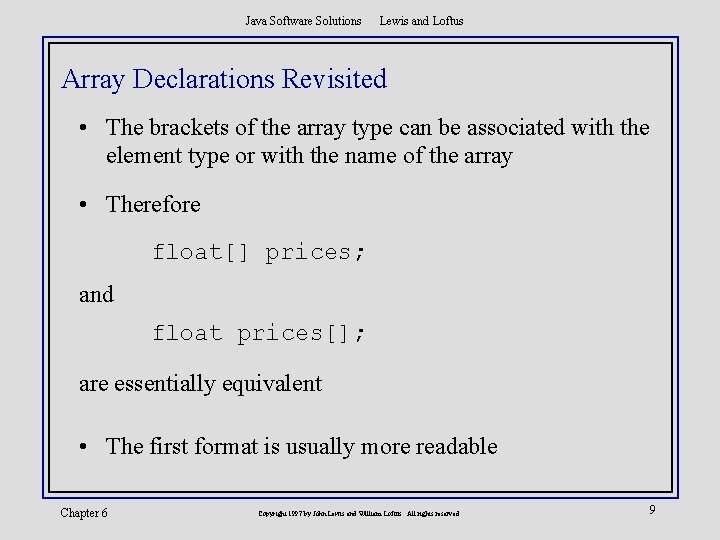
Java Software Solutions Lewis and Loftus Array Declarations Revisited • The brackets of the array type can be associated with the element type or with the name of the array • Therefore float[] prices; and float prices[]; are essentially equivalent • The first format is usually more readable Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 9
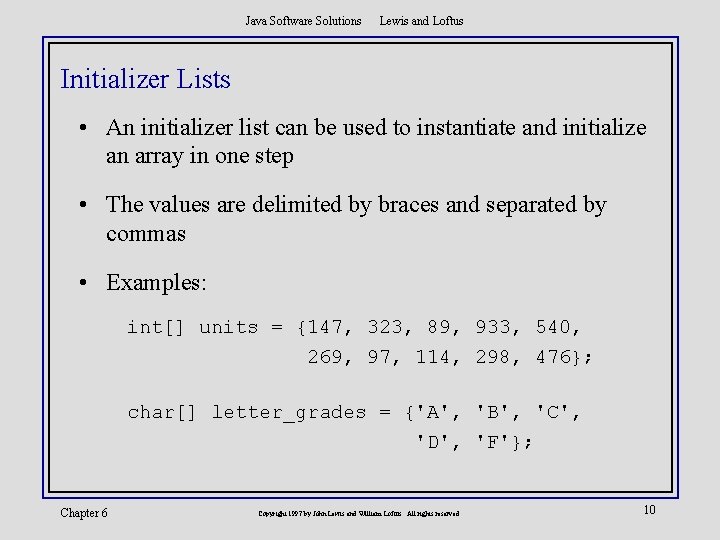
Java Software Solutions Lewis and Loftus Initializer Lists • An initializer list can be used to instantiate and initialize an array in one step • The values are delimited by braces and separated by commas • Examples: int[] units = {147, 323, 89, 933, 540, 269, 97, 114, 298, 476}; char[] letter_grades = {'A', 'B', 'C', 'D', 'F'}; Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 10
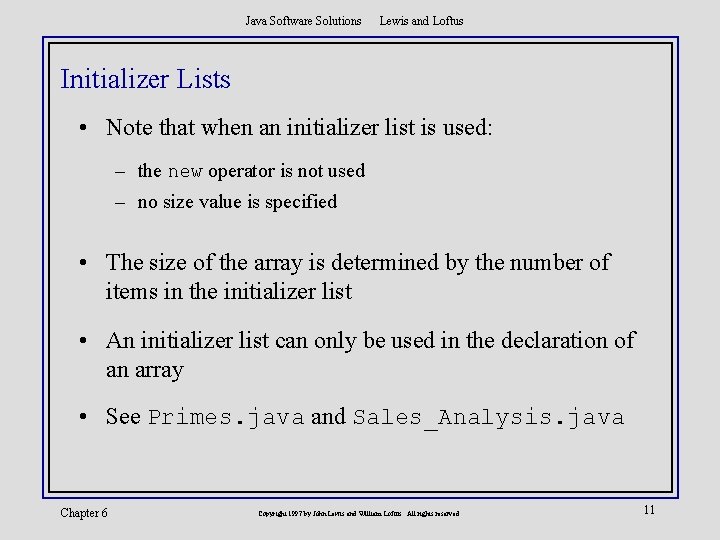
Java Software Solutions Lewis and Loftus Initializer Lists • Note that when an initializer list is used: – the new operator is not used – no size value is specified • The size of the array is determined by the number of items in the initializer list • An initializer list can only be used in the declaration of an array • See Primes. java and Sales_Analysis. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 11
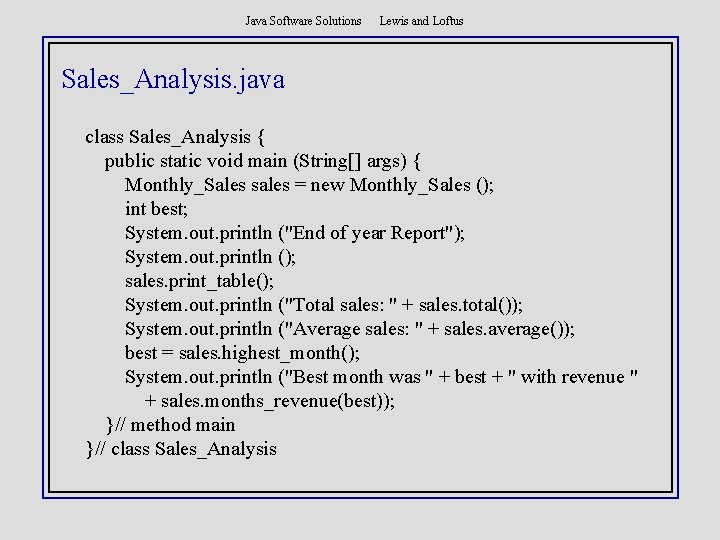
Java Software Solutions Lewis and Loftus Sales_Analysis. java class Sales_Analysis { public static void main (String[] args) { Monthly_Sales sales = new Monthly_Sales (); int best; System. out. println ("End of year Report"); System. out. println (); sales. print_table(); System. out. println ("Total sales: " + sales. total()); System. out. println ("Average sales: " + sales. average()); best = sales. highest_month(); System. out. println ("Best month was " + best + " with revenue " + sales. months_revenue(best)); }// method main }// class Sales_Analysis
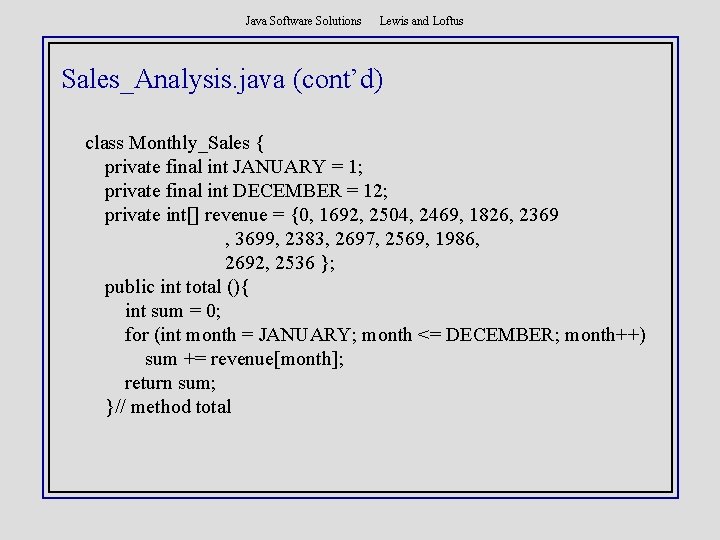
Java Software Solutions Lewis and Loftus Sales_Analysis. java (cont’d) class Monthly_Sales { private final int JANUARY = 1; private final int DECEMBER = 12; private int[] revenue = {0, 1692, 2504, 2469, 1826, 2369 , 3699, 2383, 2697, 2569, 1986, 2692, 2536 }; public int total (){ int sum = 0; for (int month = JANUARY; month <= DECEMBER; month++) sum += revenue[month]; return sum; }// method total
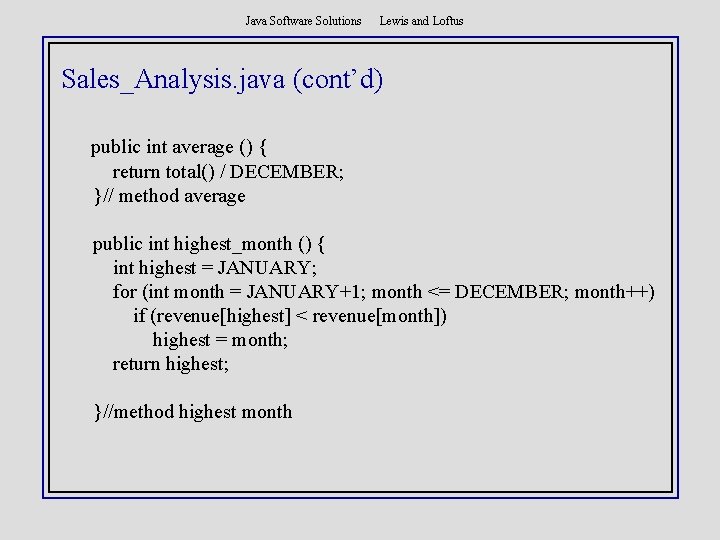
Java Software Solutions Lewis and Loftus Sales_Analysis. java (cont’d) public int average () { return total() / DECEMBER; }// method average public int highest_month () { int highest = JANUARY; for (int month = JANUARY+1; month <= DECEMBER; month++) if (revenue[highest] < revenue[month]) highest = month; return highest; }//method highest month
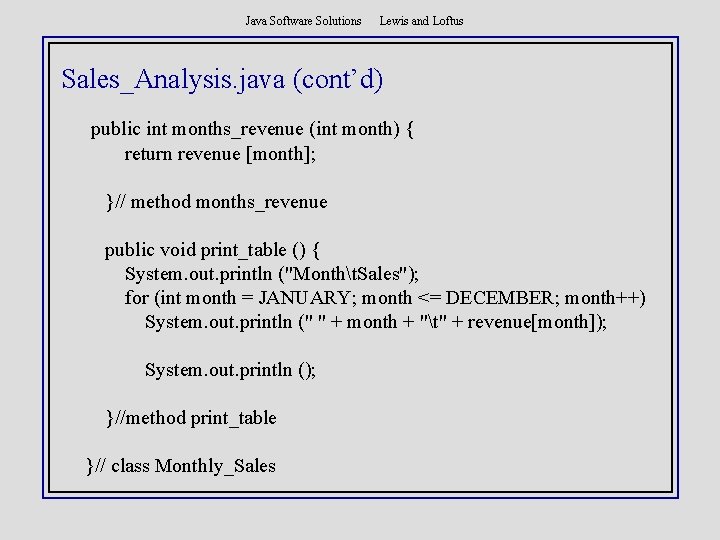
Java Software Solutions Lewis and Loftus Sales_Analysis. java (cont’d) public int months_revenue (int month) { return revenue [month]; }// method months_revenue public void print_table () { System. out. println ("Montht. Sales"); for (int month = JANUARY; month <= DECEMBER; month++) System. out. println (" " + month + "t" + revenue[month]); System. out. println (); }//method print_table }// class Monthly_Sales
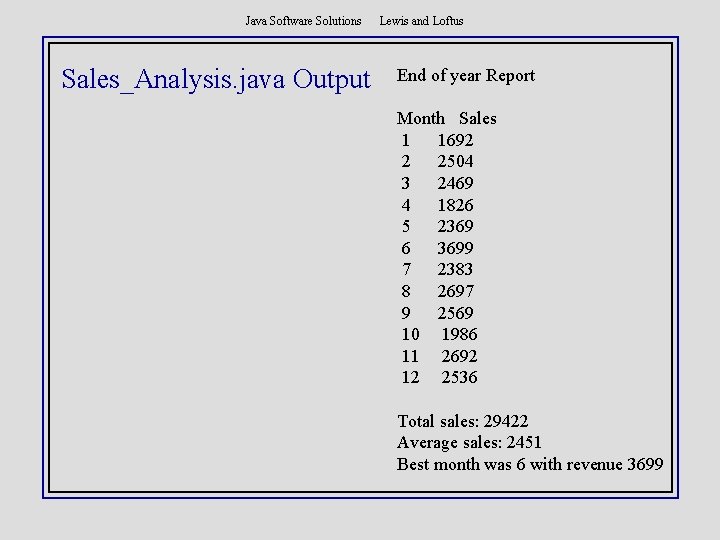
Java Software Solutions Sales_Analysis. java Output Lewis and Loftus End of year Report Month Sales 1 1692 2 2504 3 2469 4 1826 5 2369 6 3699 7 2383 8 2697 9 2569 10 1986 11 2692 12 2536 Total sales: 29422 Average sales: 2451 Best month was 6 with revenue 3699
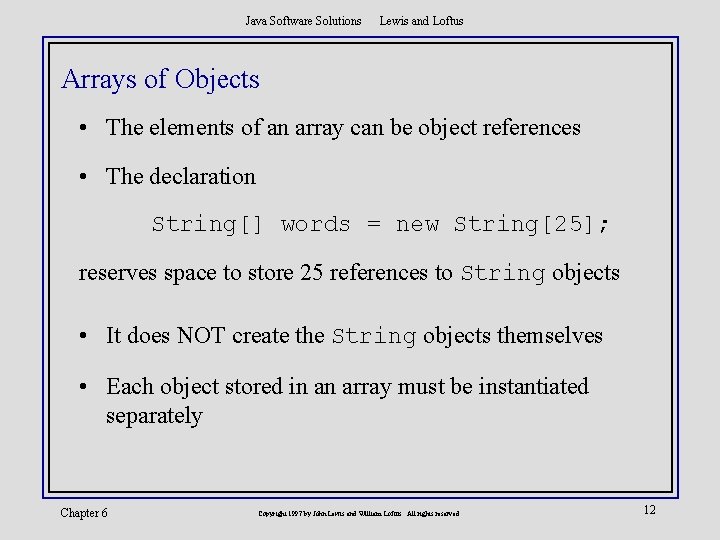
Java Software Solutions Lewis and Loftus Arrays of Objects • The elements of an array can be object references • The declaration String[] words = new String[25]; reserves space to store 25 references to String objects • It does NOT create the String objects themselves • Each object stored in an array must be instantiated separately Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 12
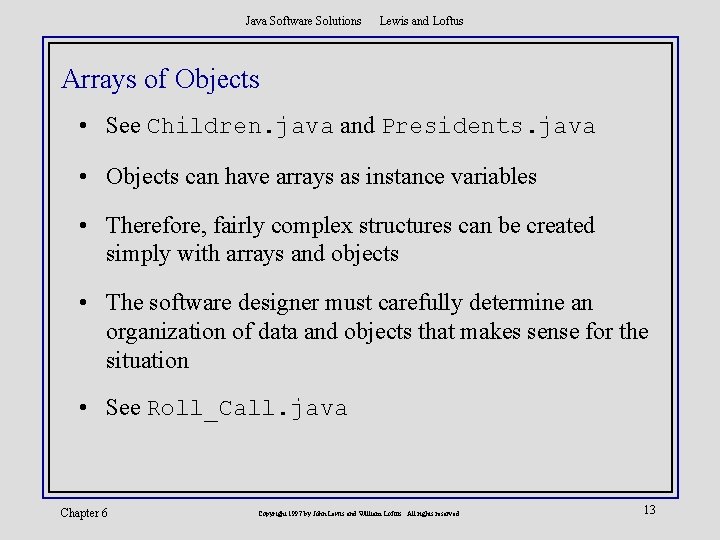
Java Software Solutions Lewis and Loftus Arrays of Objects • See Children. java and Presidents. java • Objects can have arrays as instance variables • Therefore, fairly complex structures can be created simply with arrays and objects • The software designer must carefully determine an organization of data and objects that makes sense for the situation • See Roll_Call. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 13
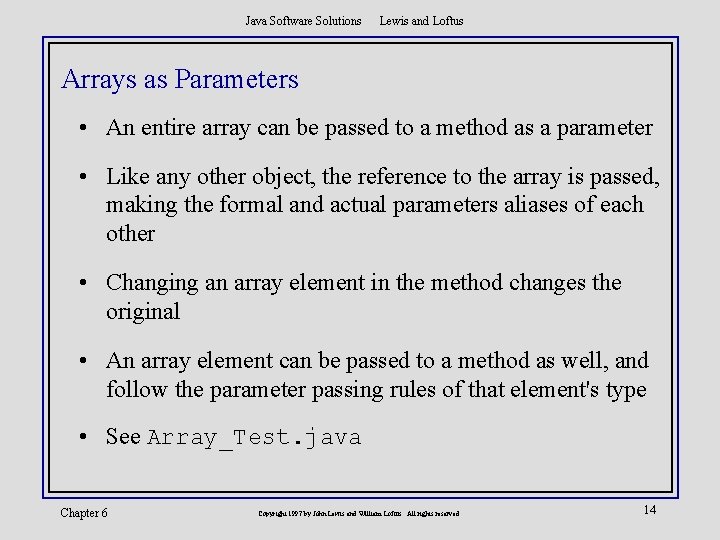
Java Software Solutions Lewis and Loftus Arrays as Parameters • An entire array can be passed to a method as a parameter • Like any other object, the reference to the array is passed, making the formal and actual parameters aliases of each other • Changing an array element in the method changes the original • An array element can be passed to a method as well, and follow the parameter passing rules of that element's type • See Array_Test. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 14
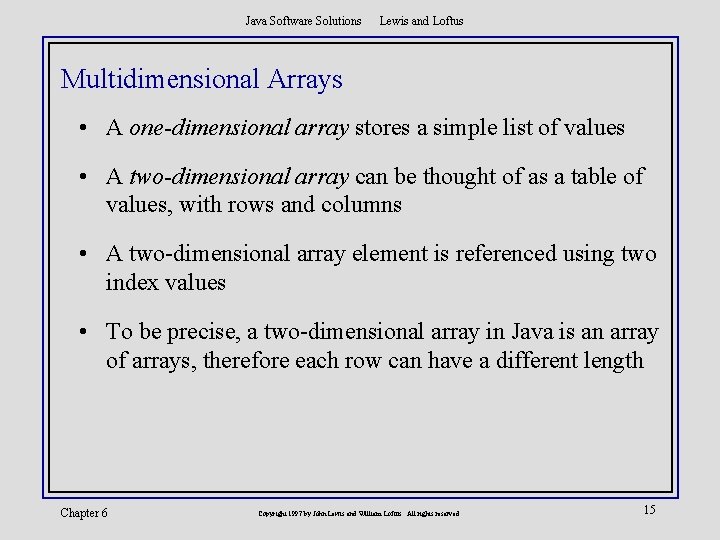
Java Software Solutions Lewis and Loftus Multidimensional Arrays • A one-dimensional array stores a simple list of values • A two-dimensional array can be thought of as a table of values, with rows and columns • A two-dimensional array element is referenced using two index values • To be precise, a two-dimensional array in Java is an array of arrays, therefore each row can have a different length Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 15
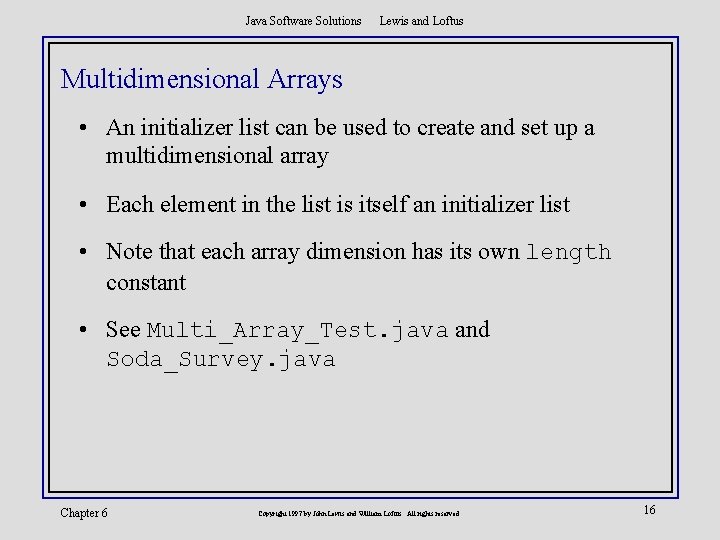
Java Software Solutions Lewis and Loftus Multidimensional Arrays • An initializer list can be used to create and set up a multidimensional array • Each element in the list is itself an initializer list • Note that each array dimension has its own length constant • See Multi_Array_Test. java and Soda_Survey. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 16
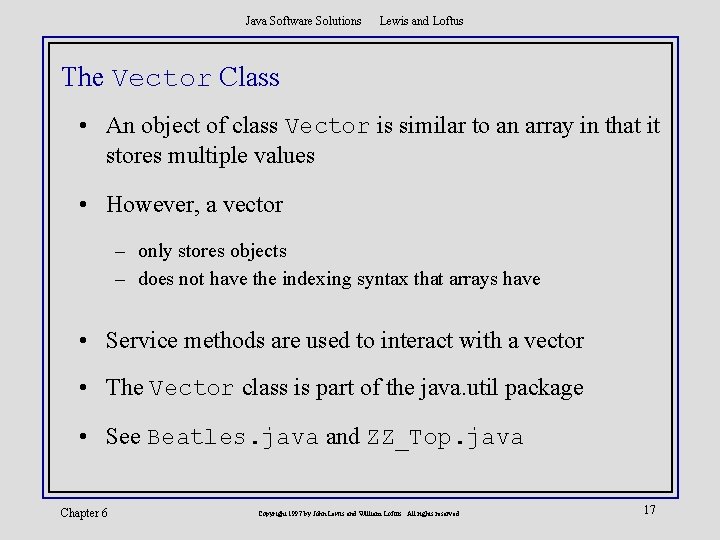
Java Software Solutions Lewis and Loftus The Vector Class • An object of class Vector is similar to an array in that it stores multiple values • However, a vector – only stores objects – does not have the indexing syntax that arrays have • Service methods are used to interact with a vector • The Vector class is part of the java. util package • See Beatles. java and ZZ_Top. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 17
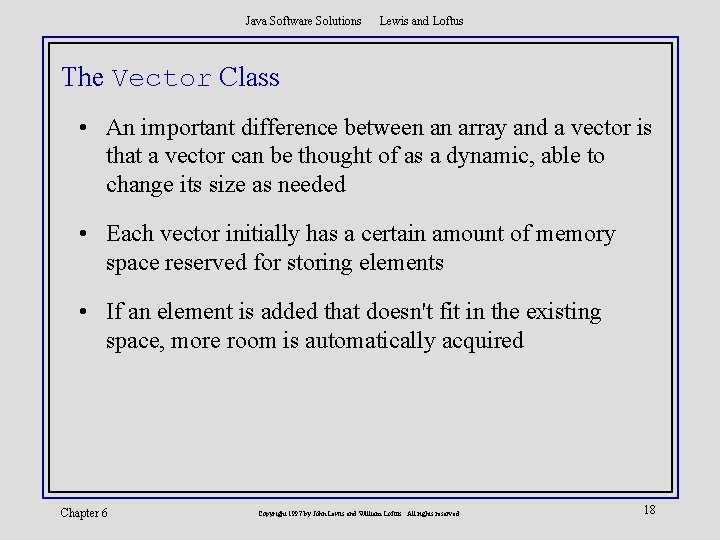
Java Software Solutions Lewis and Loftus The Vector Class • An important difference between an array and a vector is that a vector can be thought of as a dynamic, able to change its size as needed • Each vector initially has a certain amount of memory space reserved for storing elements • If an element is added that doesn't fit in the existing space, more room is automatically acquired Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 18
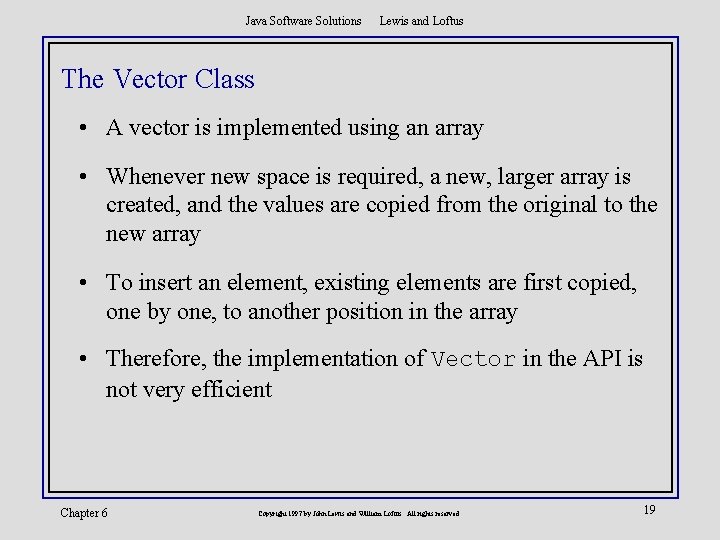
Java Software Solutions Lewis and Loftus The Vector Class • A vector is implemented using an array • Whenever new space is required, a new, larger array is created, and the values are copied from the original to the new array • To insert an element, existing elements are first copied, one by one, to another position in the array • Therefore, the implementation of Vector in the API is not very efficient Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 19
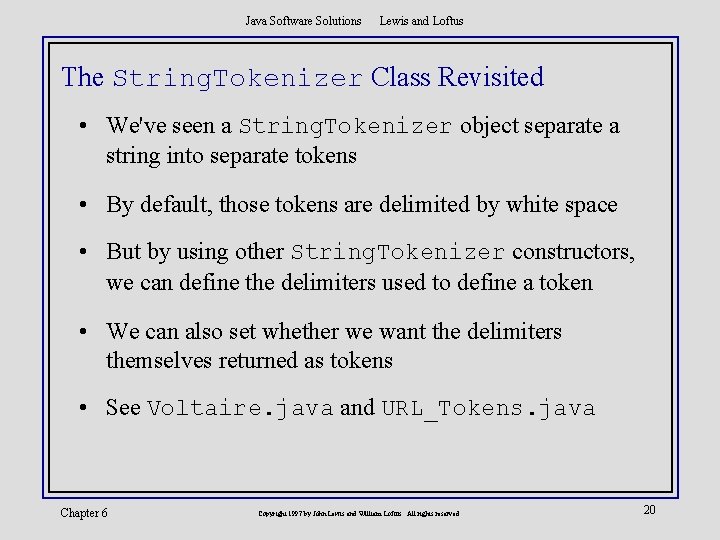
Java Software Solutions Lewis and Loftus The String. Tokenizer Class Revisited • We've seen a String. Tokenizer object separate a string into separate tokens • By default, those tokens are delimited by white space • But by using other String. Tokenizer constructors, we can define the delimiters used to define a token • We can also set whether we want the delimiters themselves returned as tokens • See Voltaire. java and URL_Tokens. java Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 20
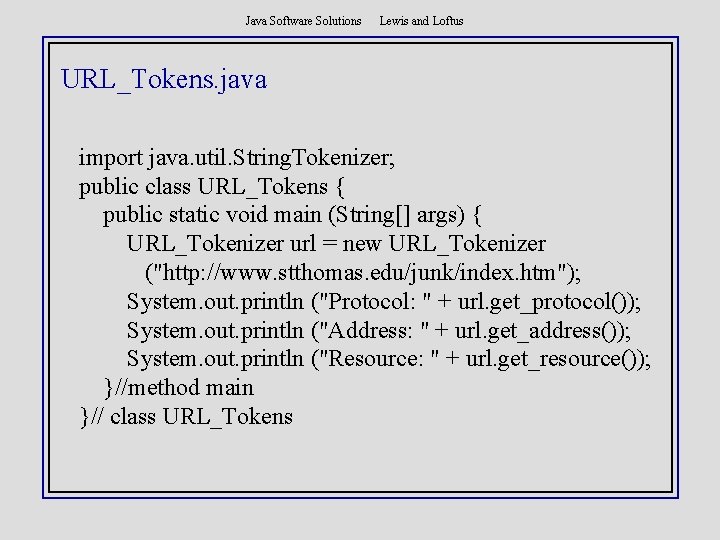
Java Software Solutions Lewis and Loftus URL_Tokens. java import java. util. String. Tokenizer; public class URL_Tokens { public static void main (String[] args) { URL_Tokenizer url = new URL_Tokenizer ("http: //www. stthomas. edu/junk/index. htm"); System. out. println ("Protocol: " + url. get_protocol()); System. out. println ("Address: " + url. get_address()); System. out. println ("Resource: " + url. get_resource()); }//method main }// class URL_Tokens
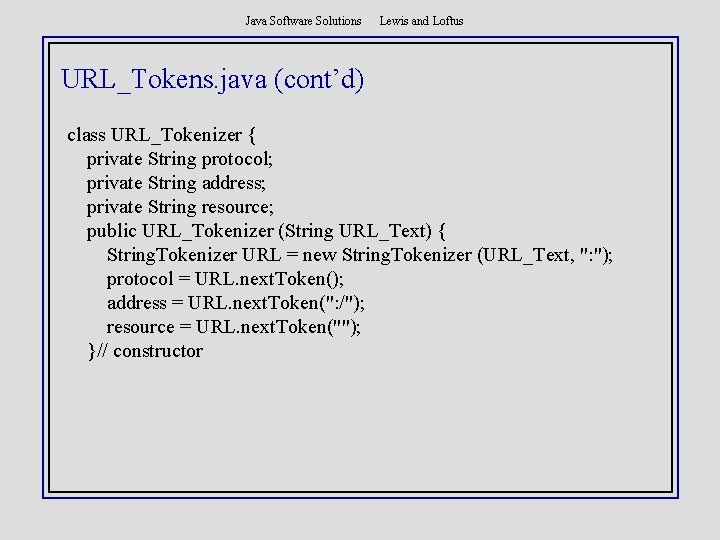
Java Software Solutions Lewis and Loftus URL_Tokens. java (cont’d) class URL_Tokenizer { private String protocol; private String address; private String resource; public URL_Tokenizer (String URL_Text) { String. Tokenizer URL = new String. Tokenizer (URL_Text, ": "); protocol = URL. next. Token(); address = URL. next. Token(": /"); resource = URL. next. Token(""); }// constructor
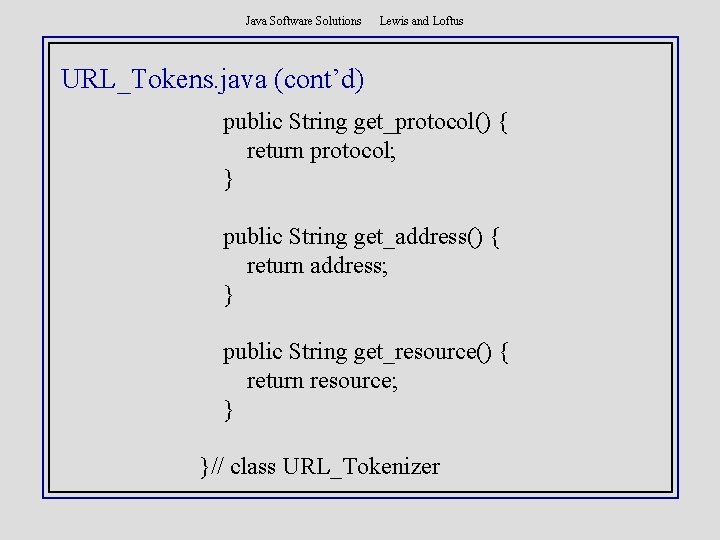
Java Software Solutions Lewis and Loftus URL_Tokens. java (cont’d) public String get_protocol() { return protocol; } public String get_address() { return address; } public String get_resource() { return resource; } }// class URL_Tokenizer
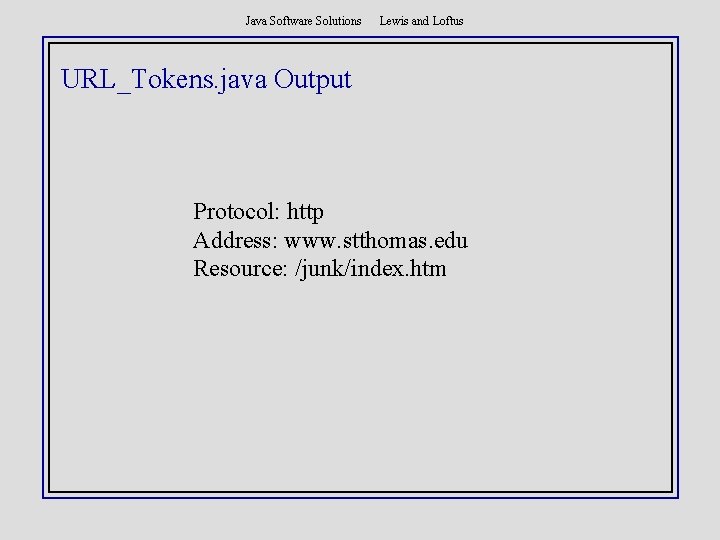
Java Software Solutions Lewis and Loftus URL_Tokens. java Output Protocol: http Address: www. stthomas. edu Resource: /junk/index. htm
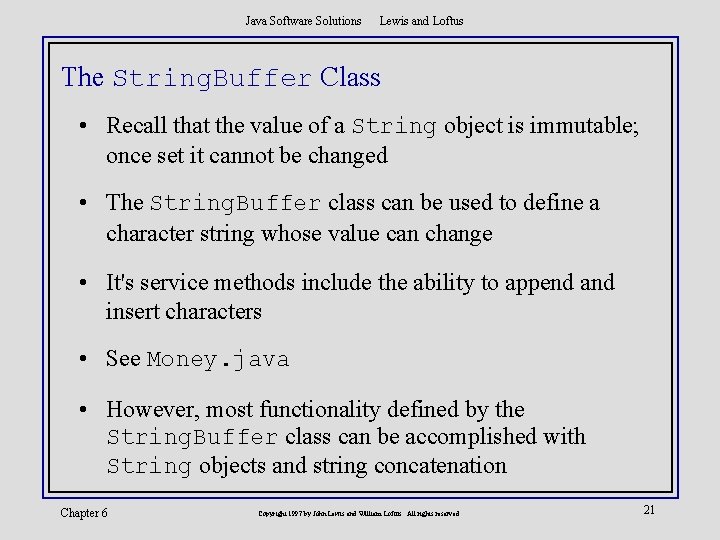
Java Software Solutions Lewis and Loftus The String. Buffer Class • Recall that the value of a String object is immutable; once set it cannot be changed • The String. Buffer class can be used to define a character string whose value can change • It's service methods include the ability to append and insert characters • See Money. java • However, most functionality defined by the String. Buffer class can be accomplished with String objects and string concatenation Chapter 6 Copyright 1997 by John Lewis and William Loftus. All rights reserved. 21