Java Collection Framework Introduction Java Collection Framework CollectionContainer
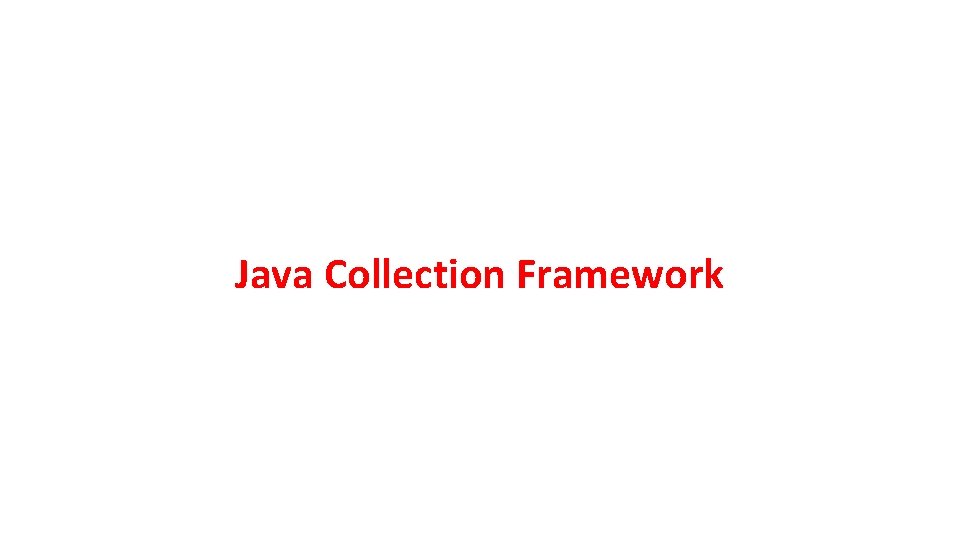
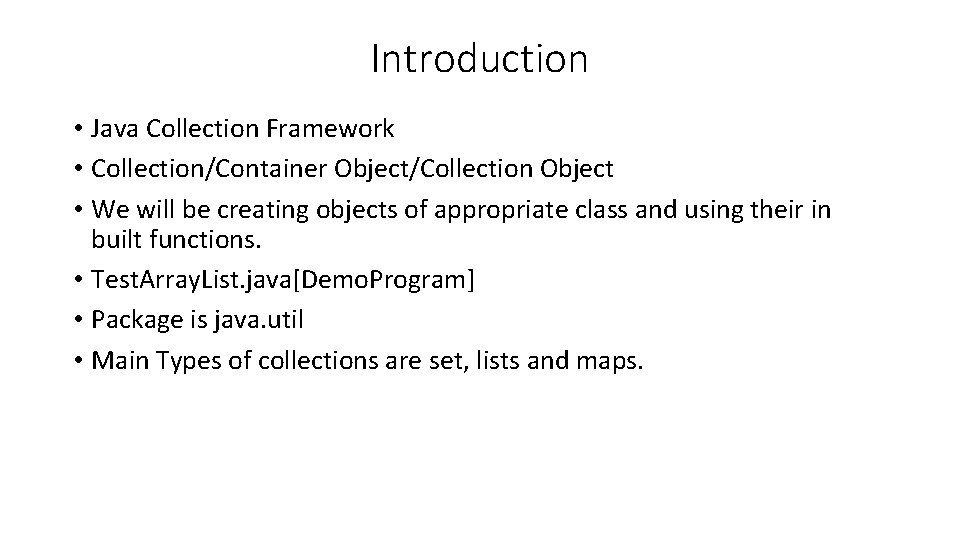
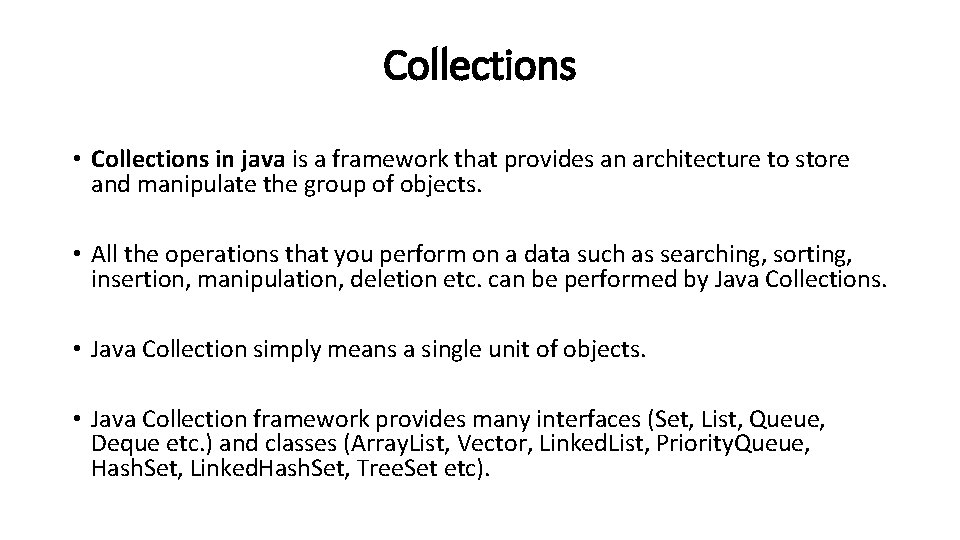
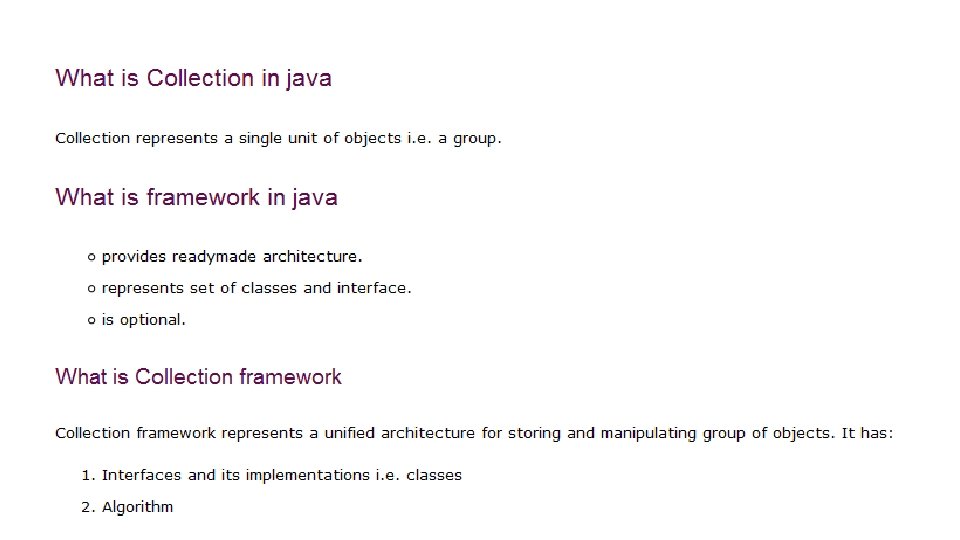
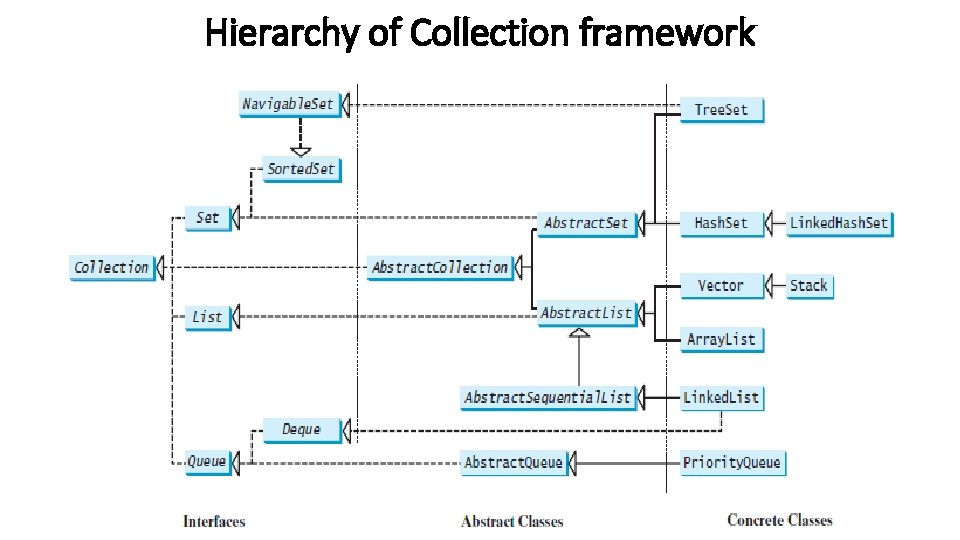
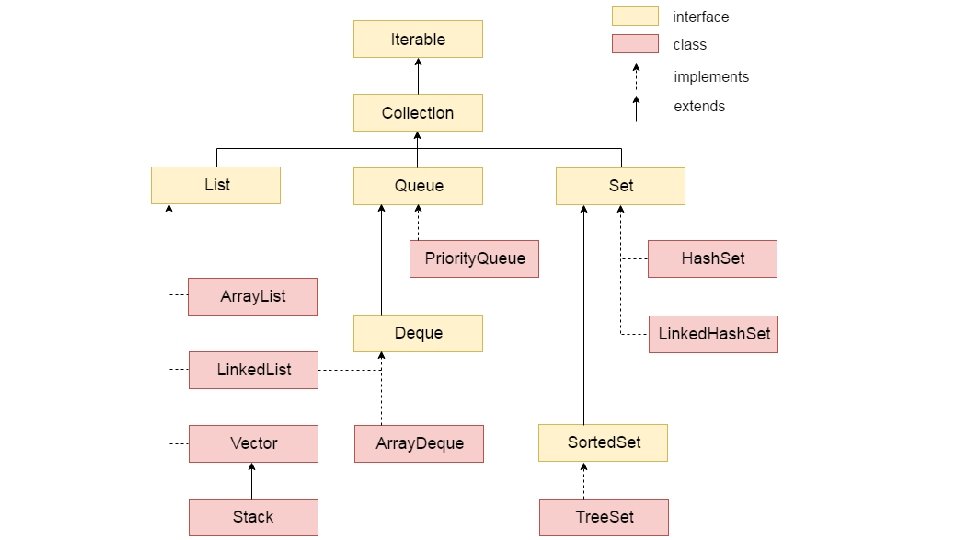
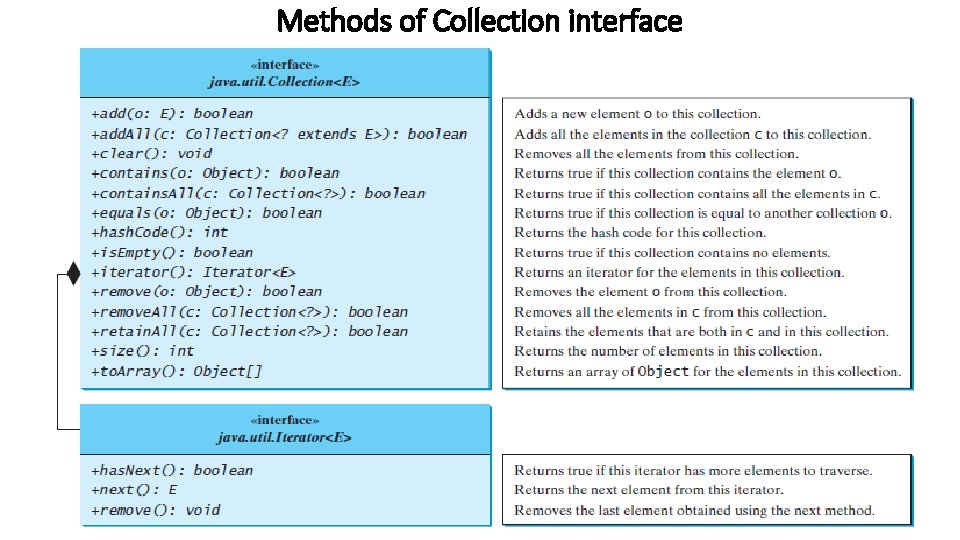
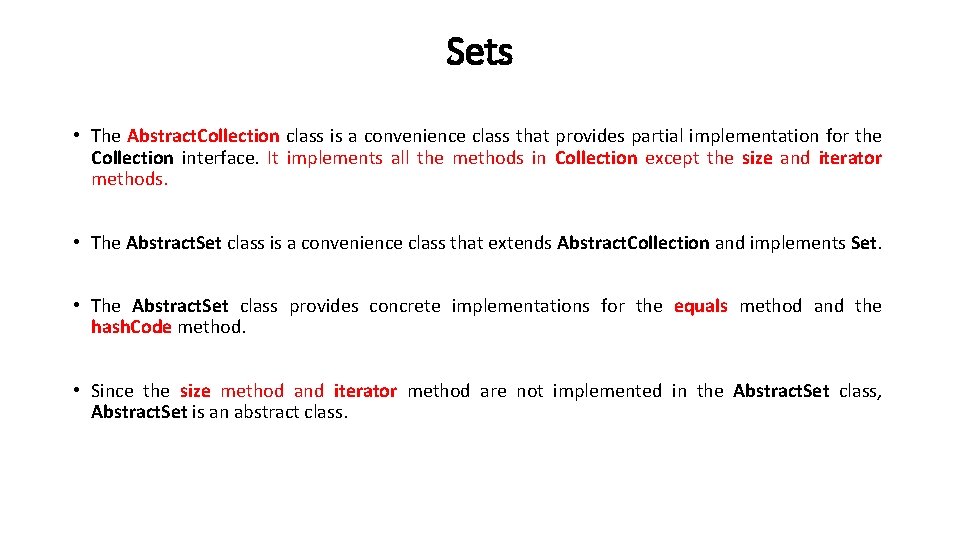
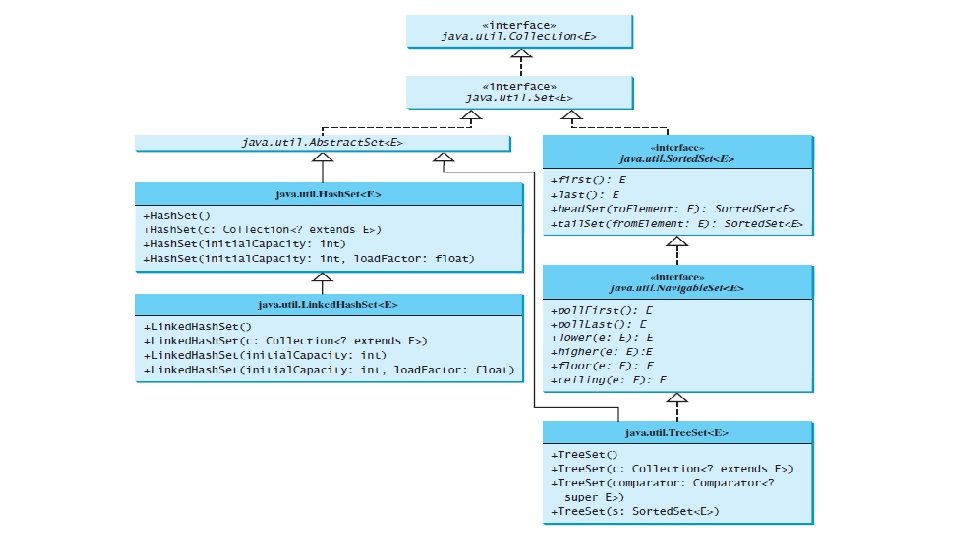
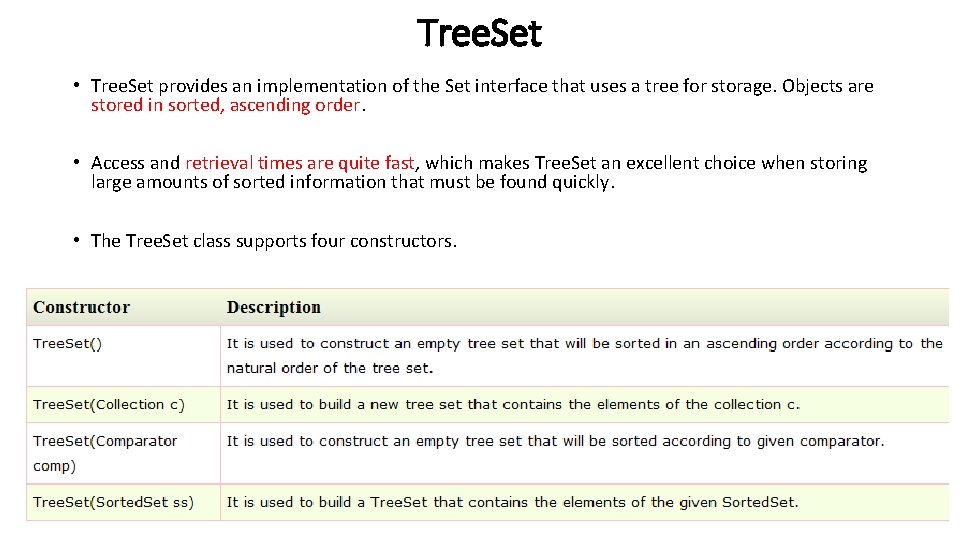
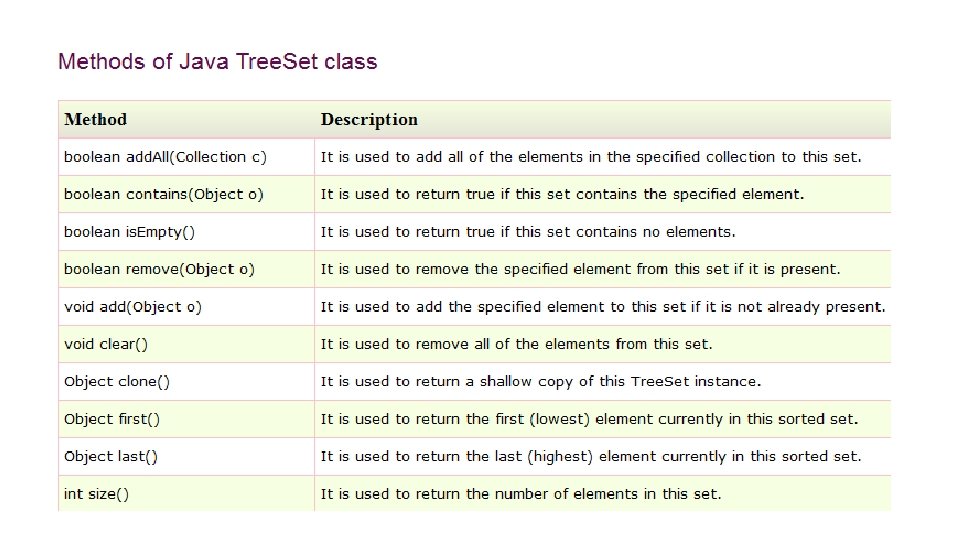
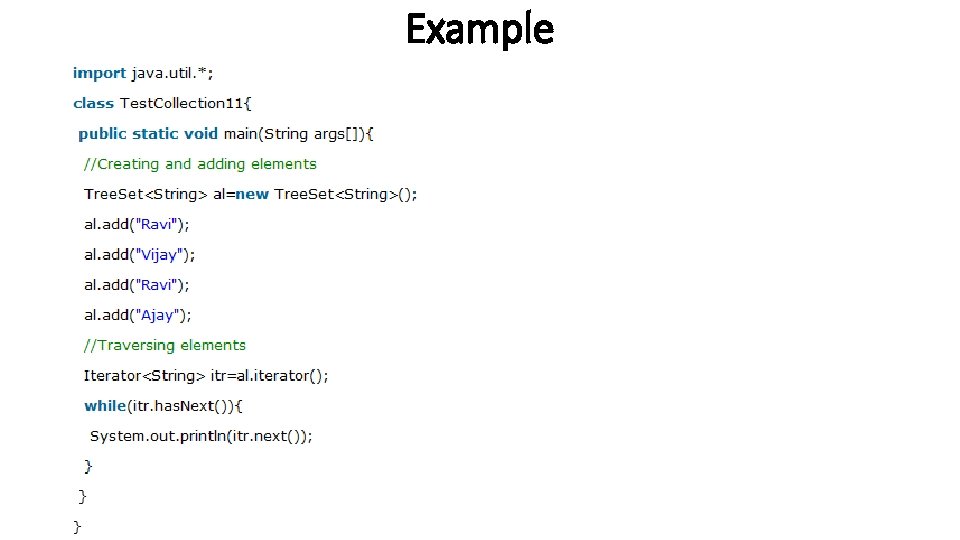
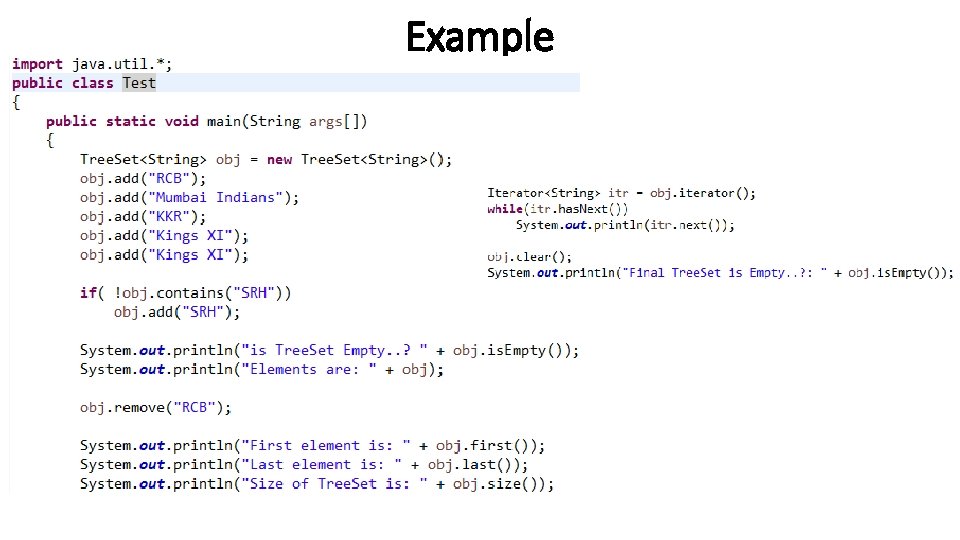
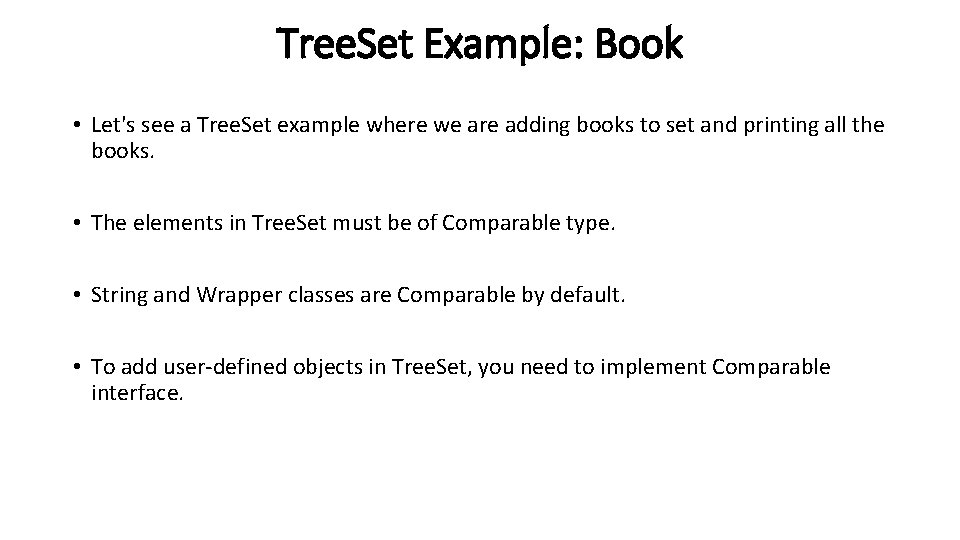
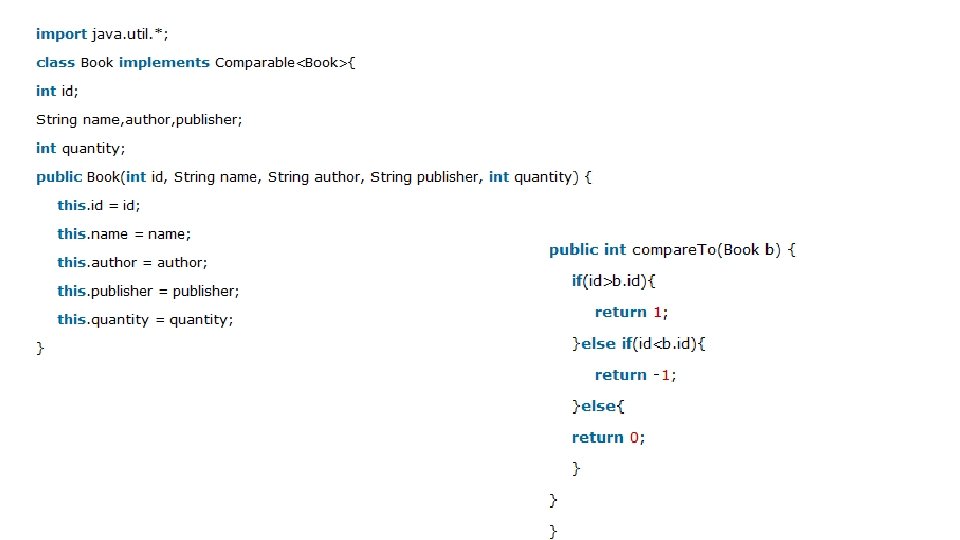
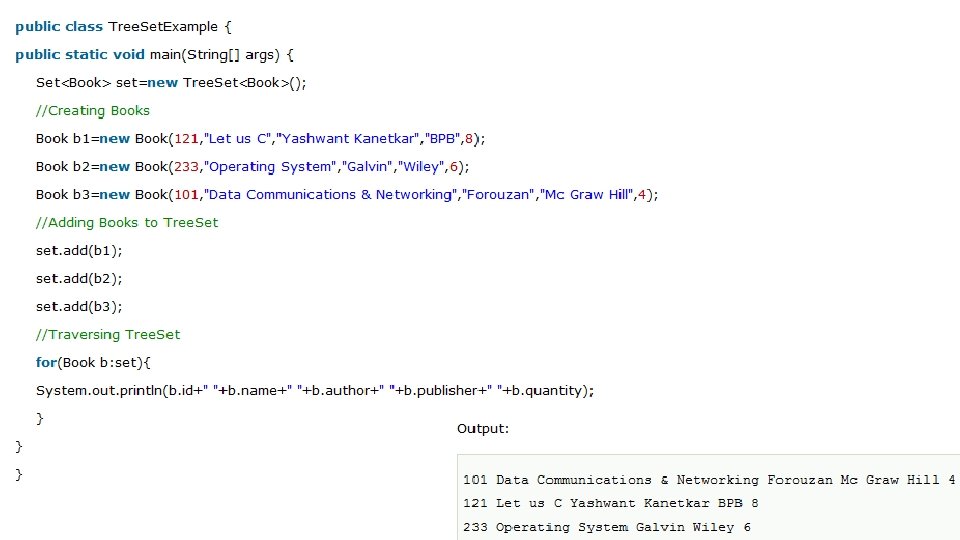
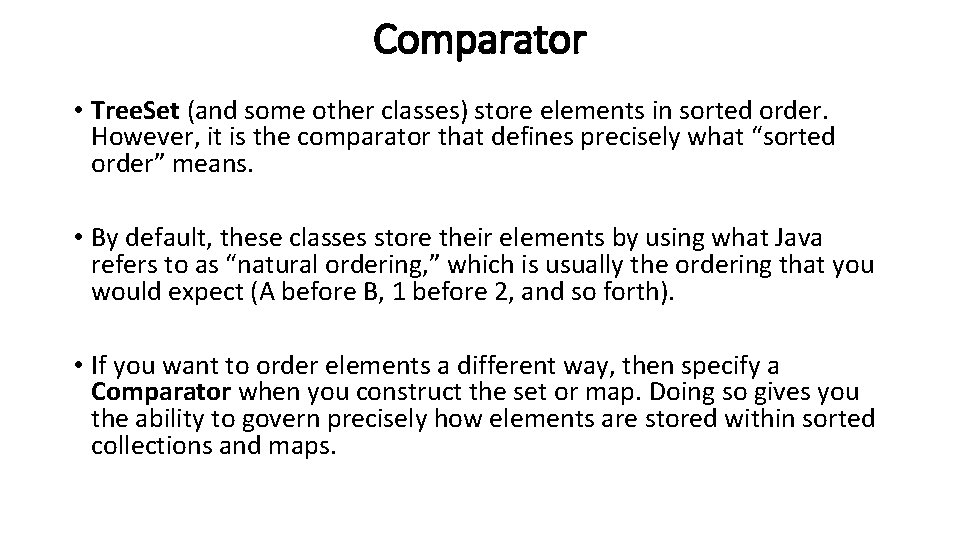
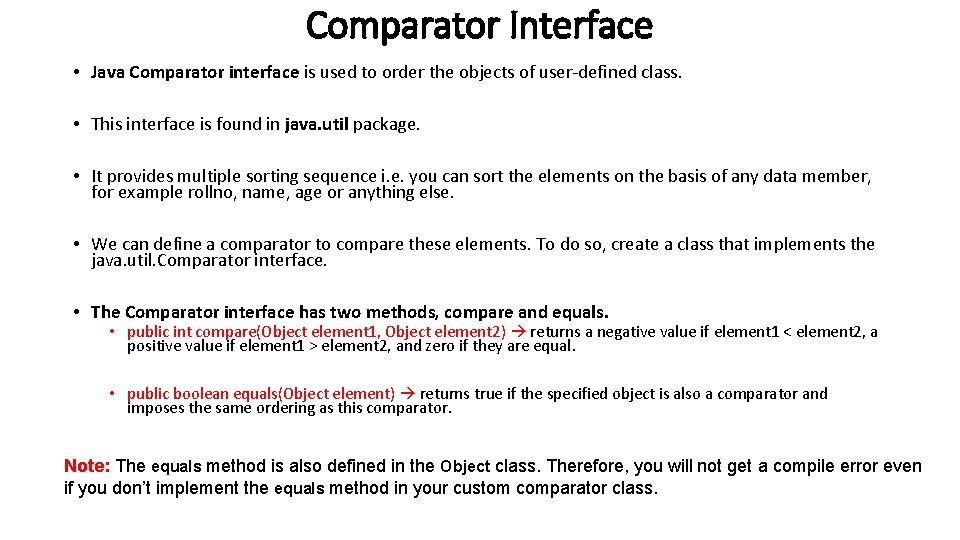
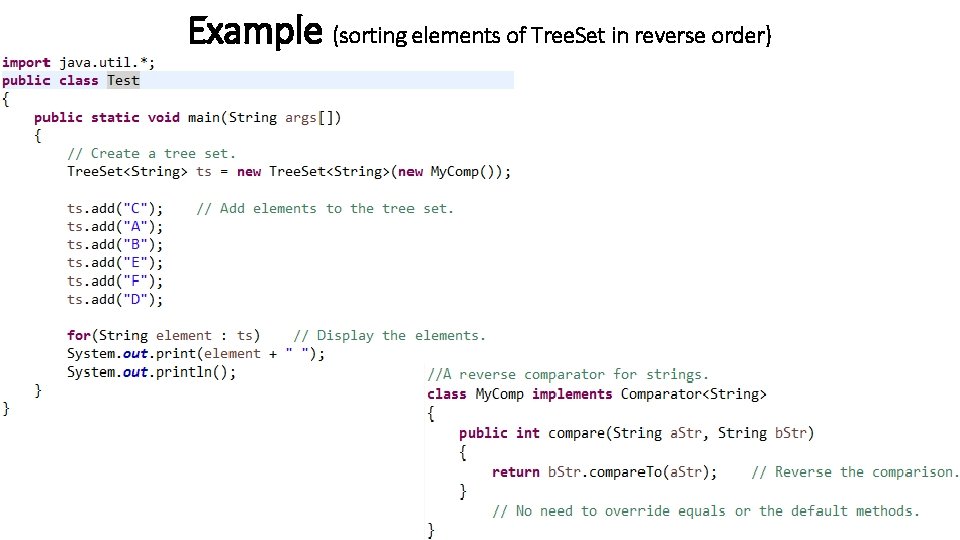
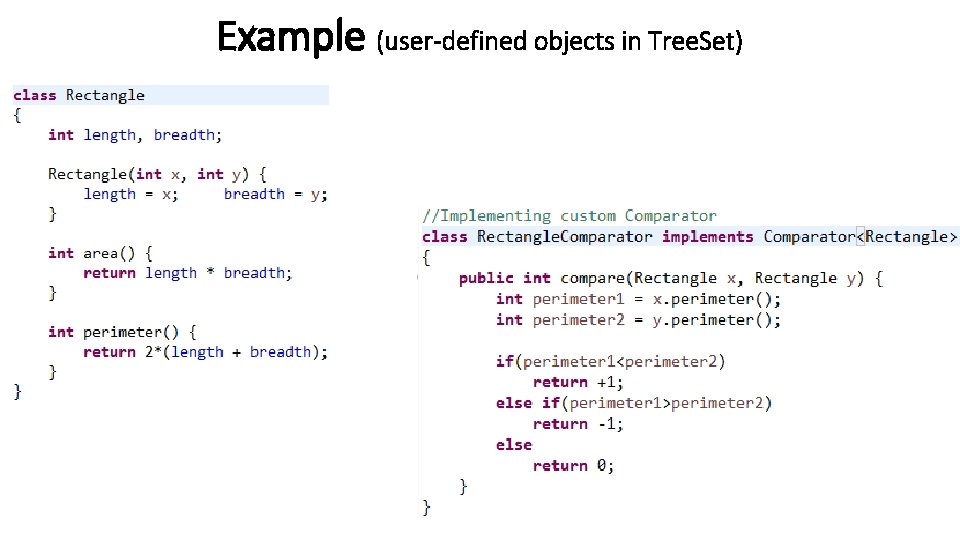
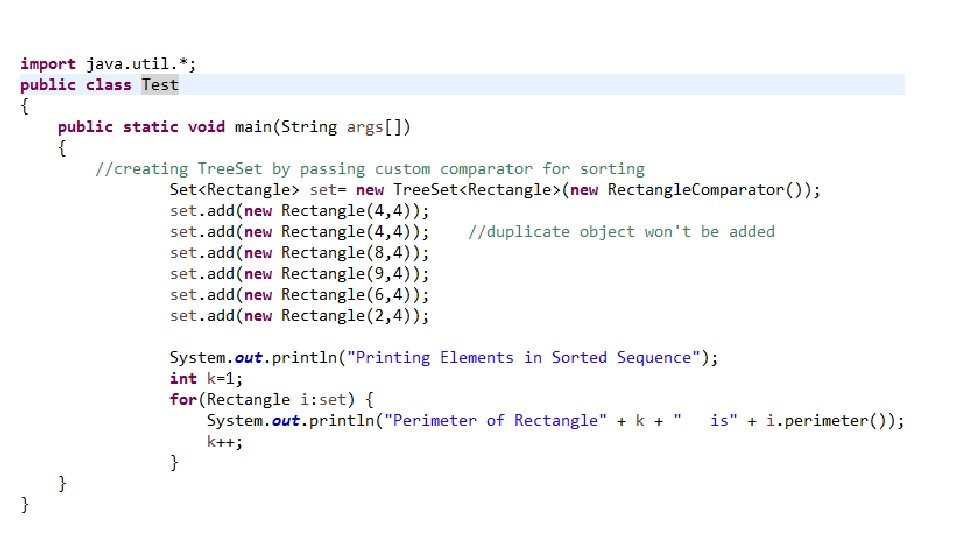
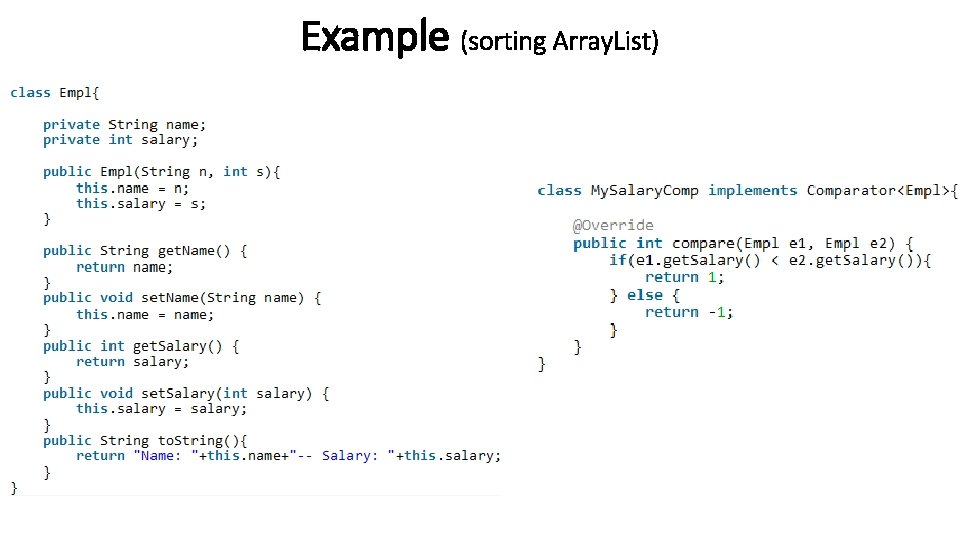
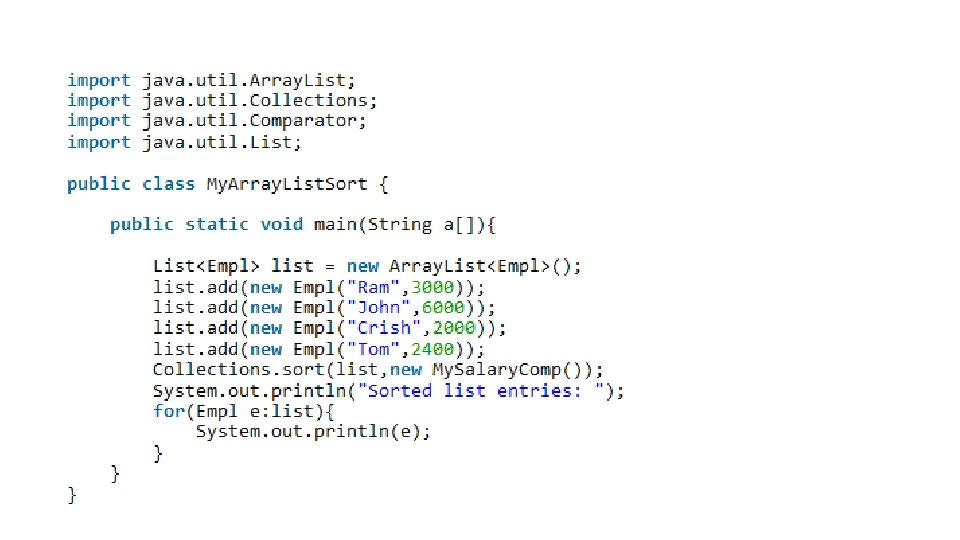
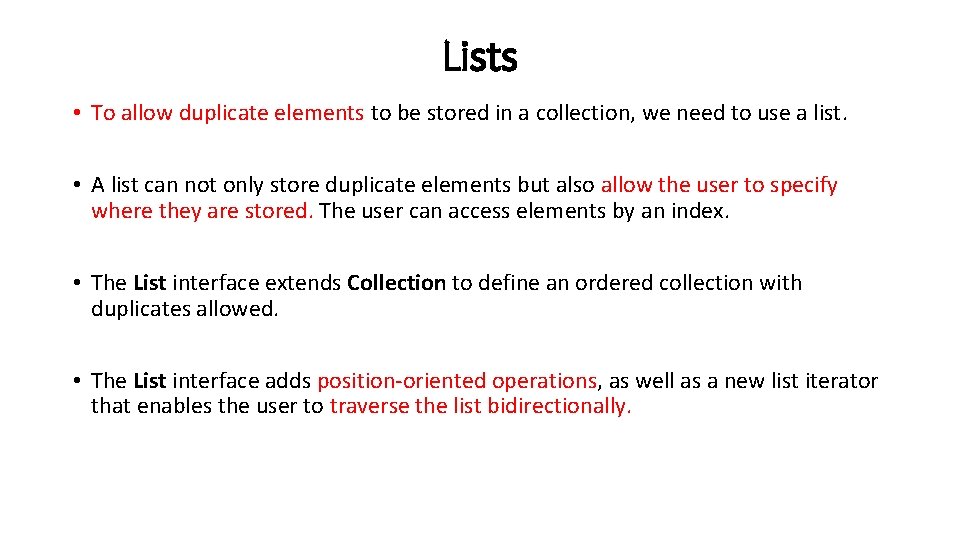
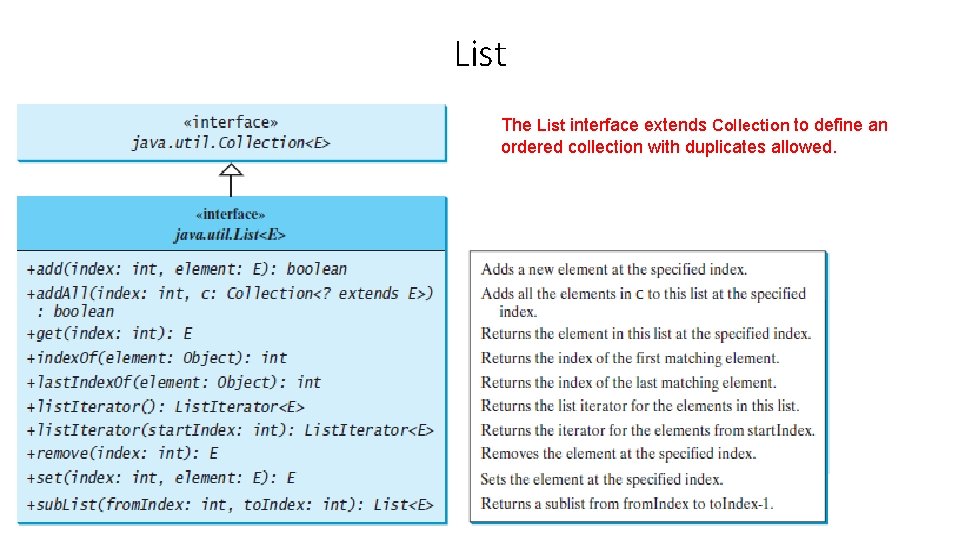
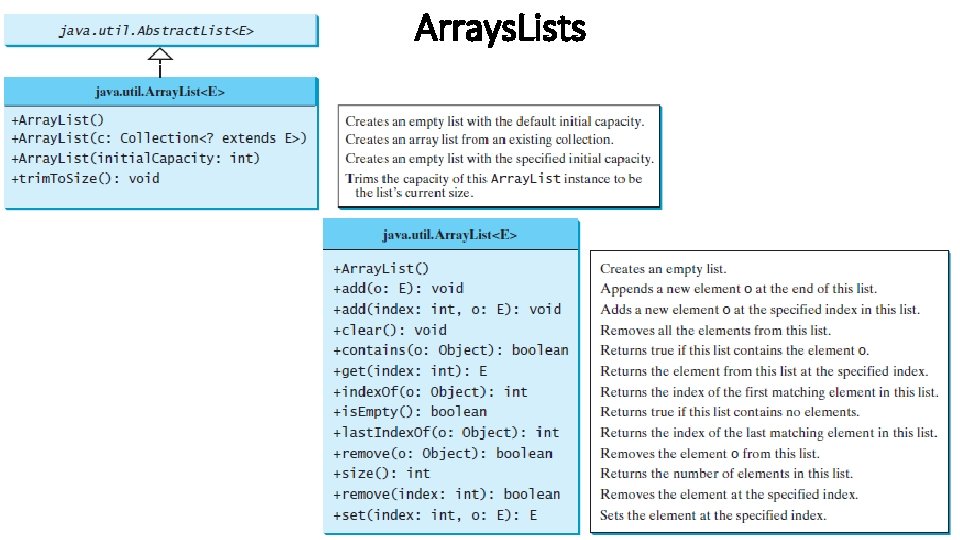
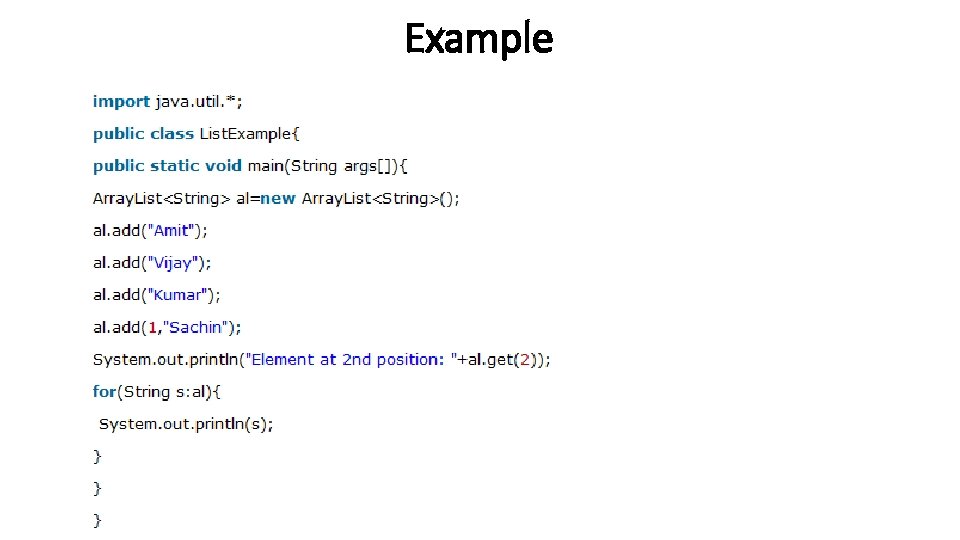
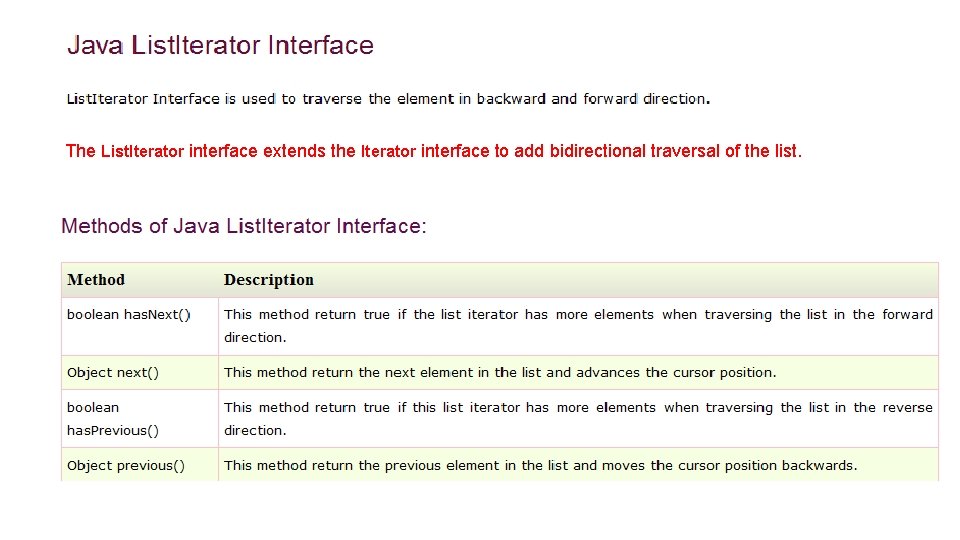
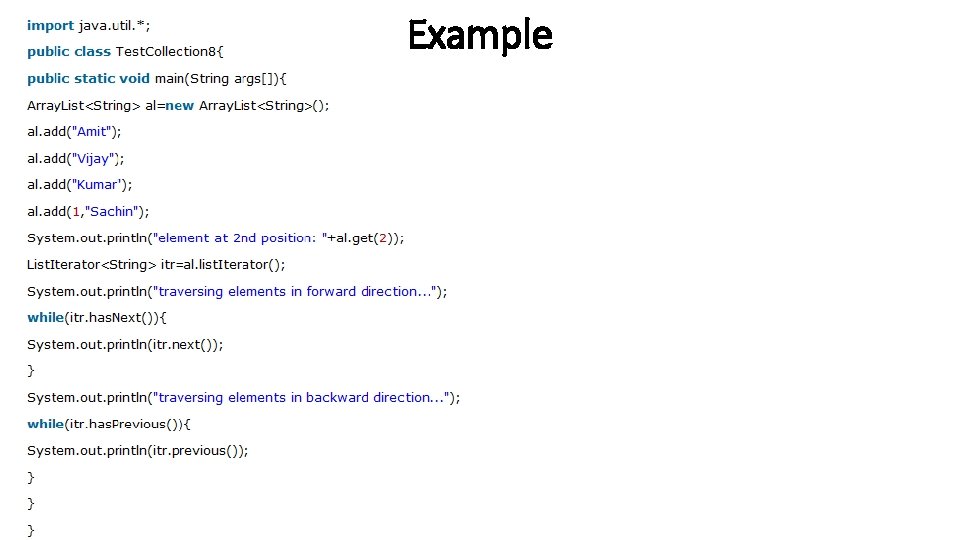
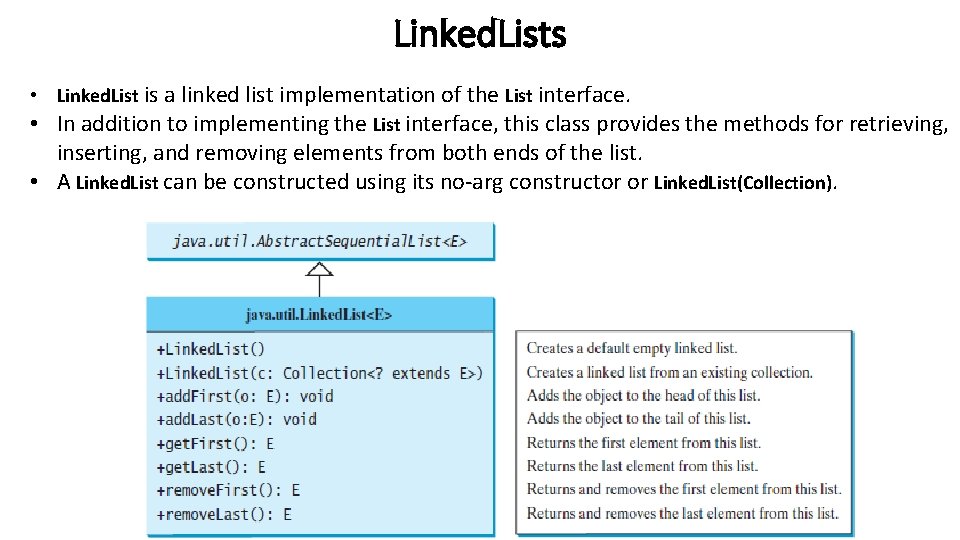
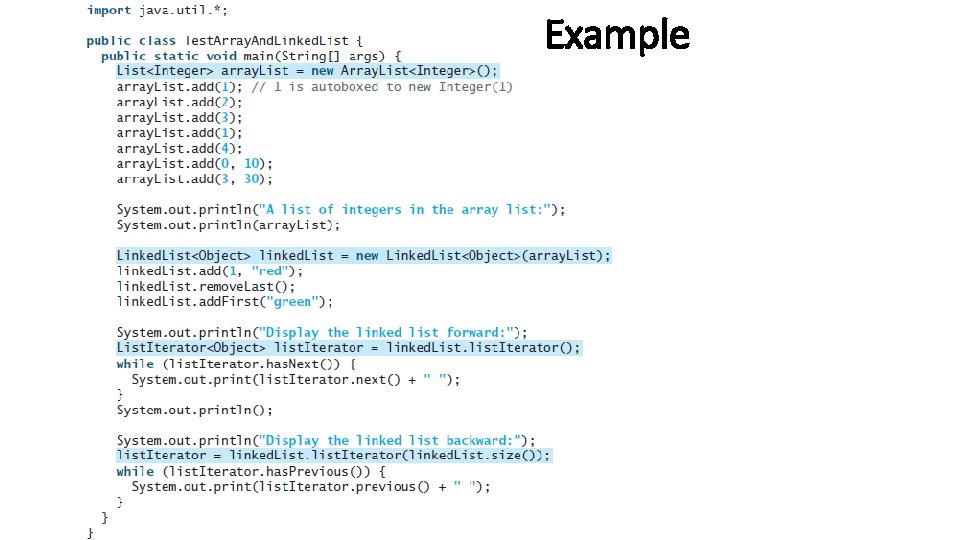
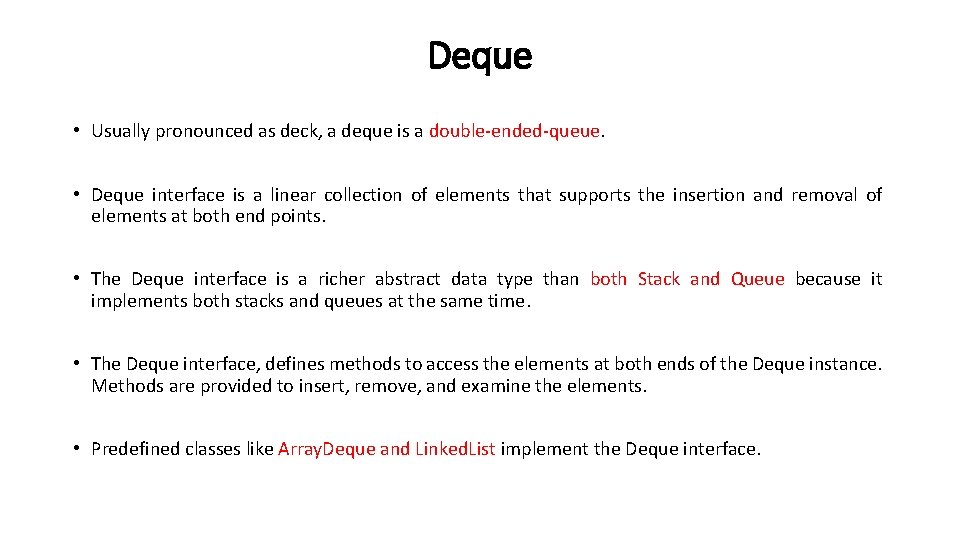
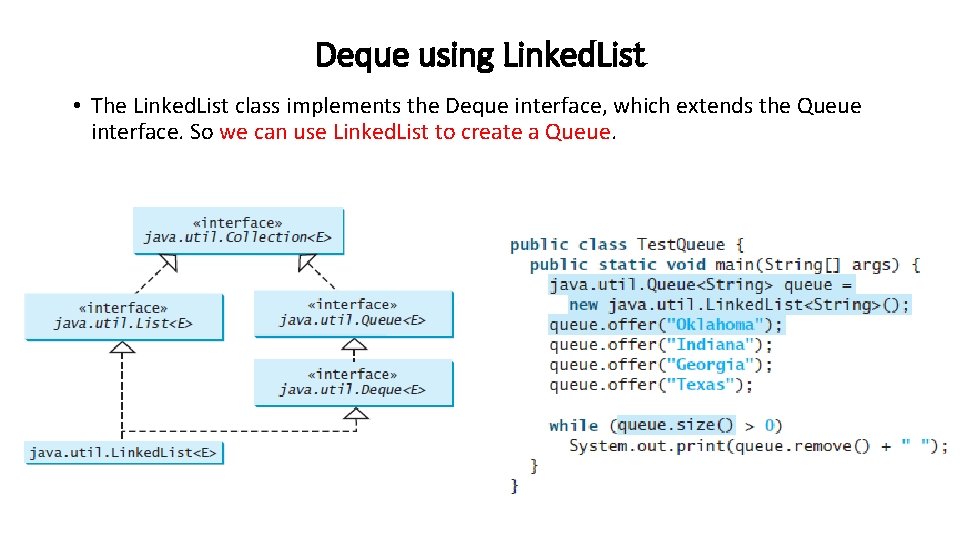
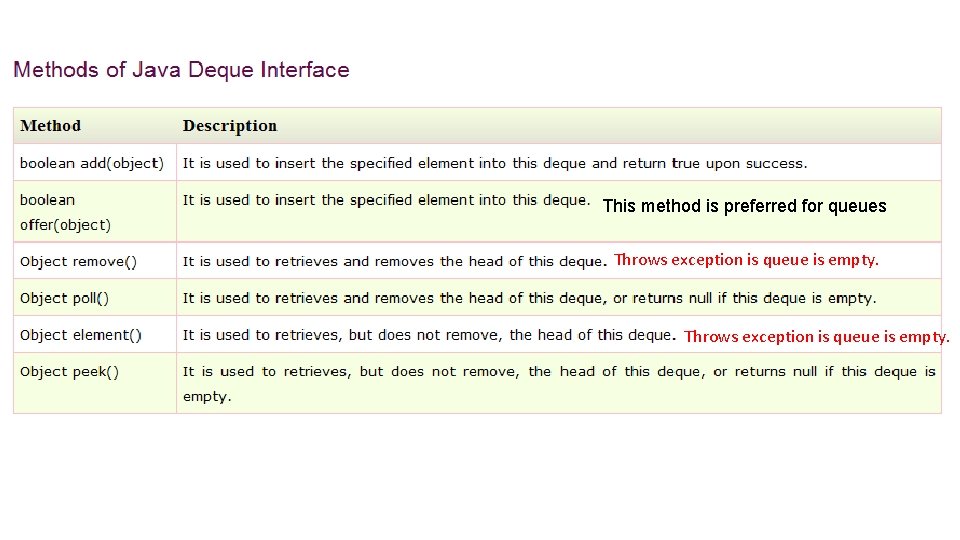
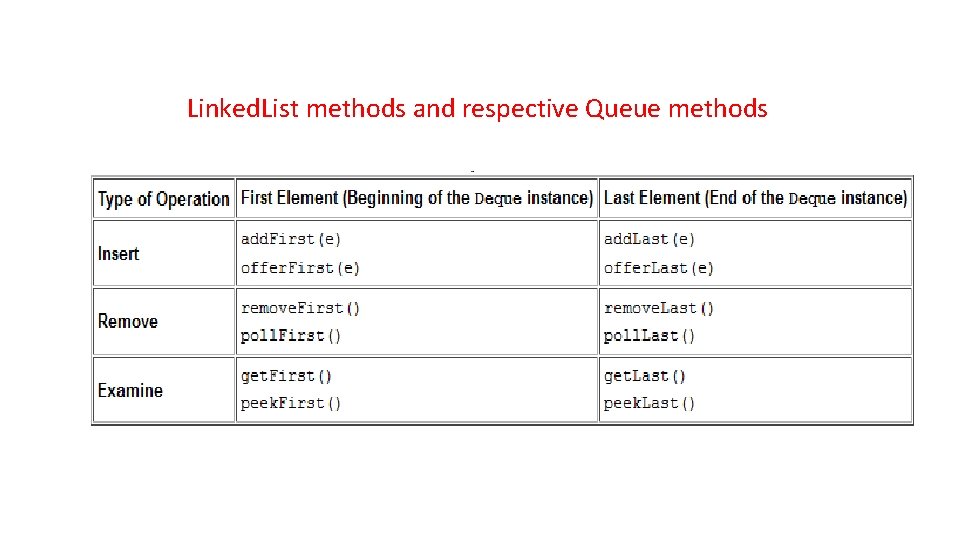
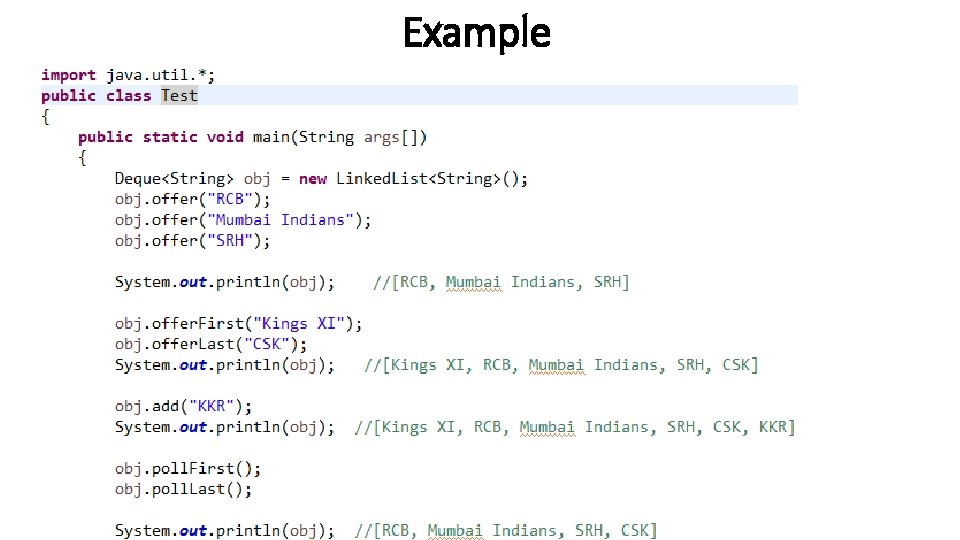
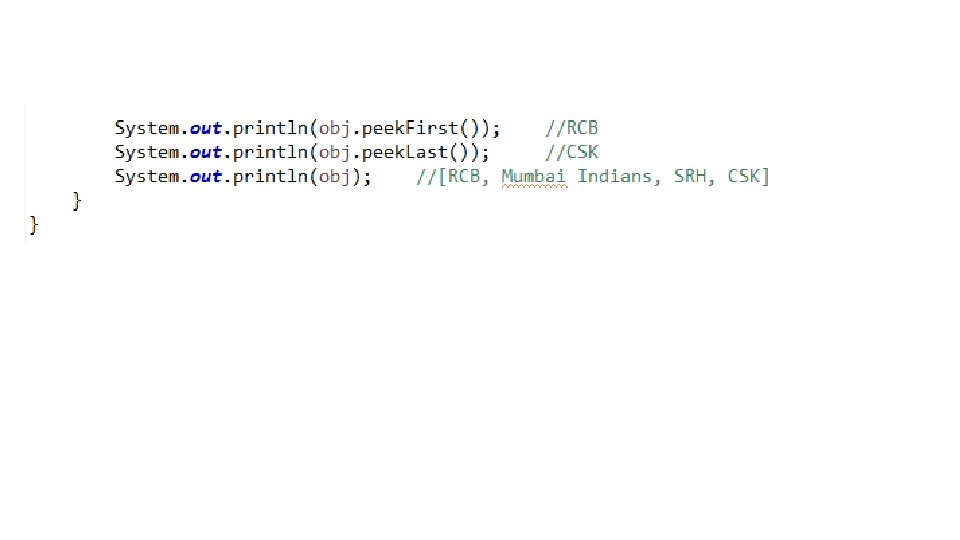
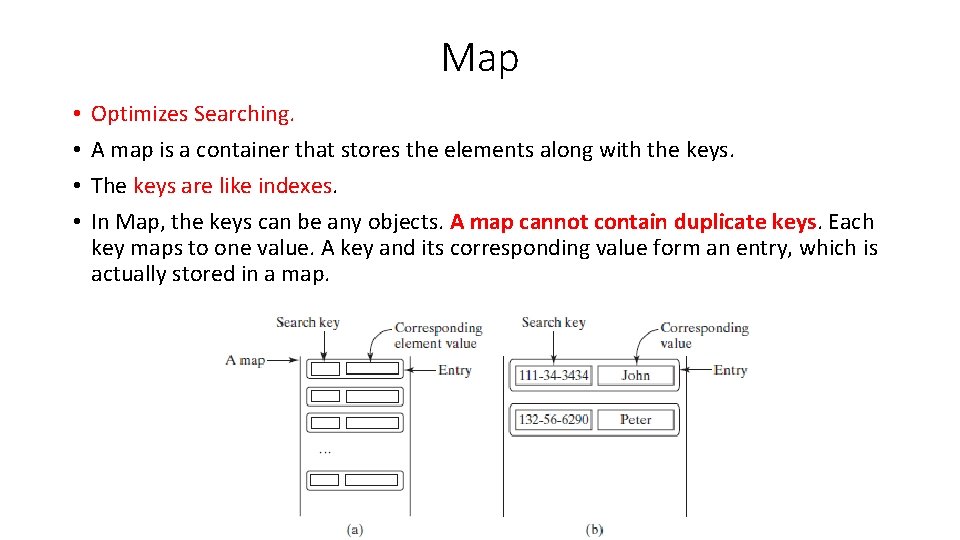
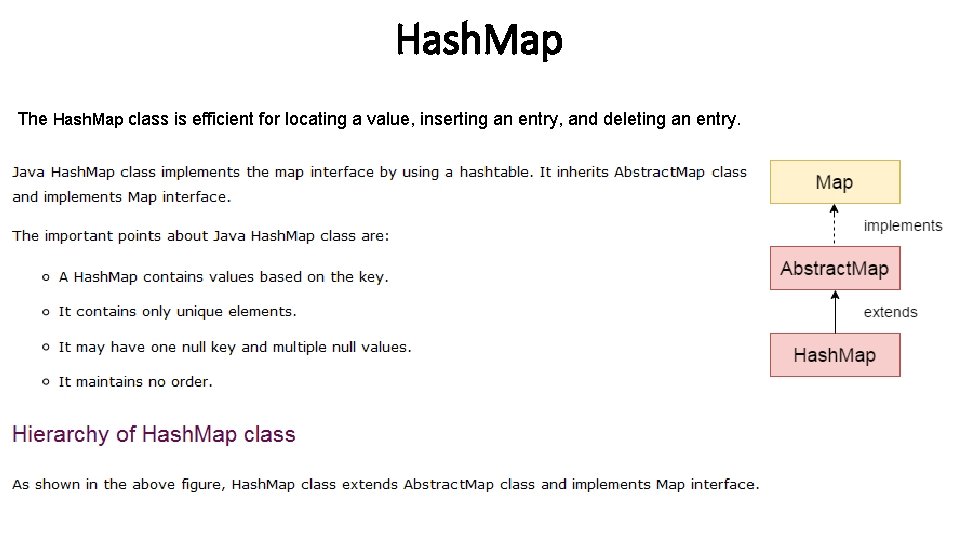
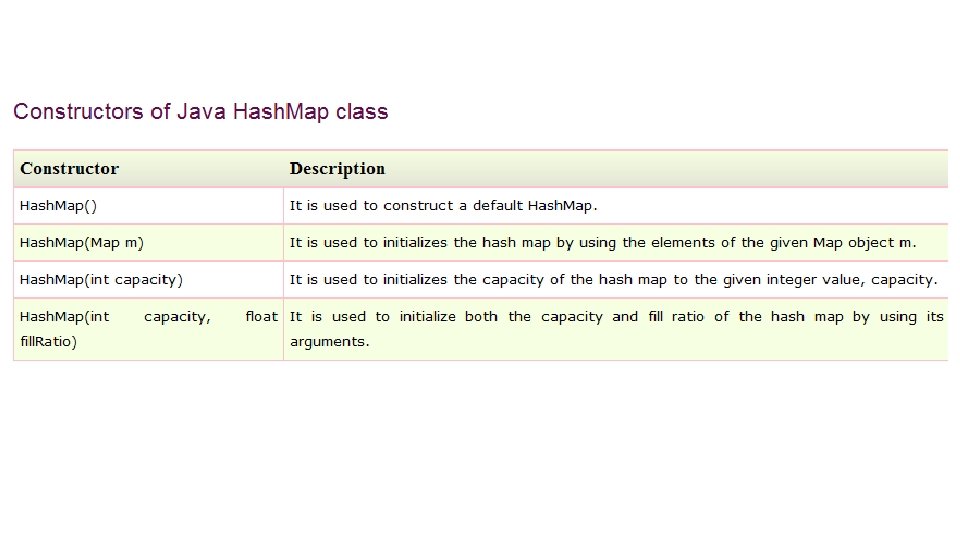
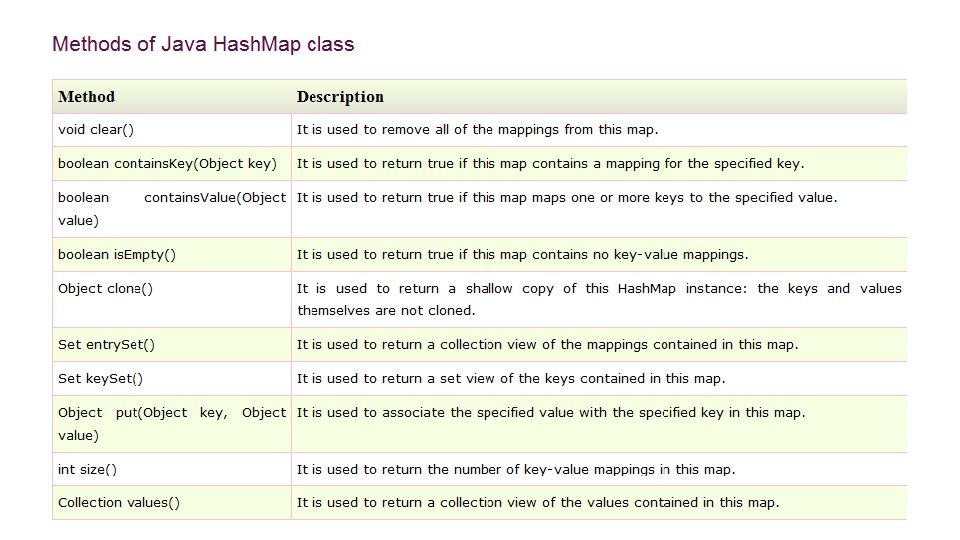
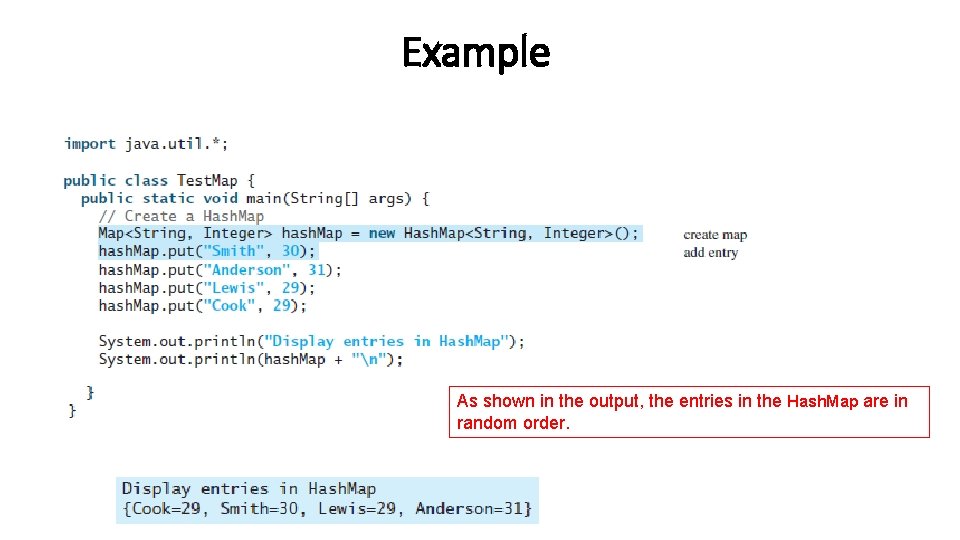
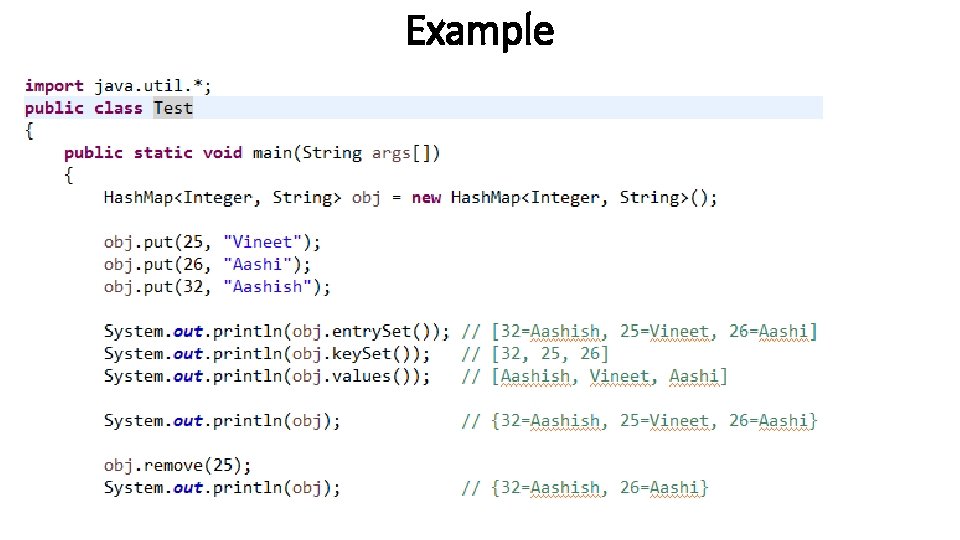
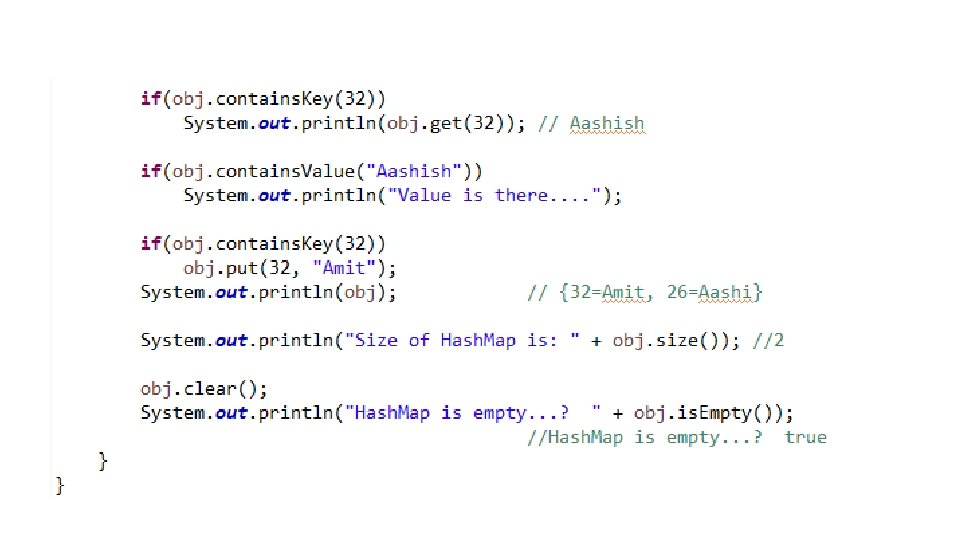
- Slides: 44
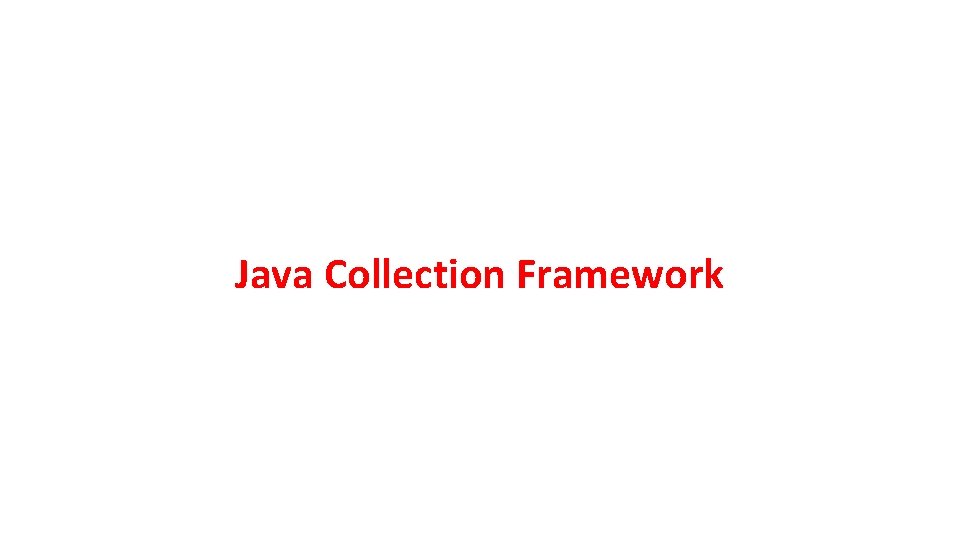
Java Collection Framework
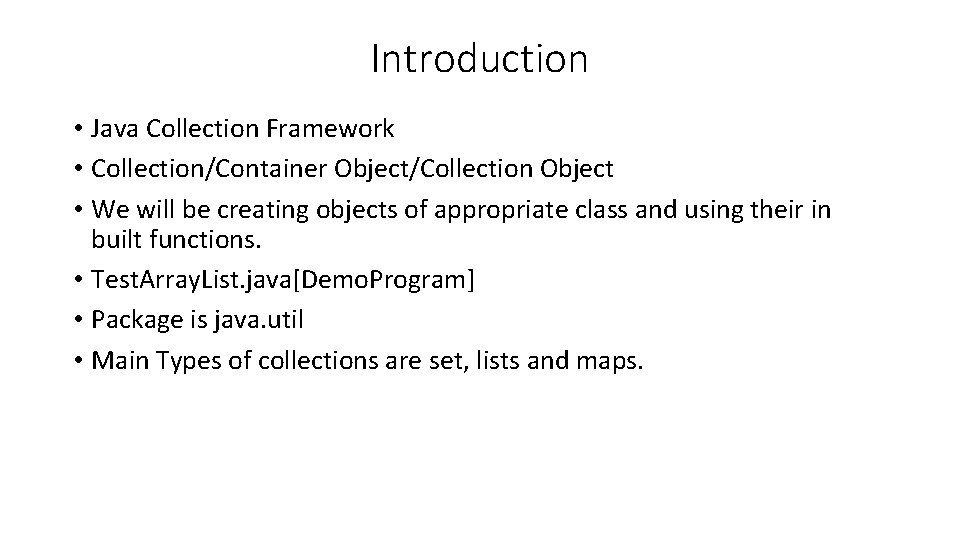
Introduction • Java Collection Framework • Collection/Container Object/Collection Object • We will be creating objects of appropriate class and using their in built functions. • Test. Array. List. java[Demo. Program] • Package is java. util • Main Types of collections are set, lists and maps.
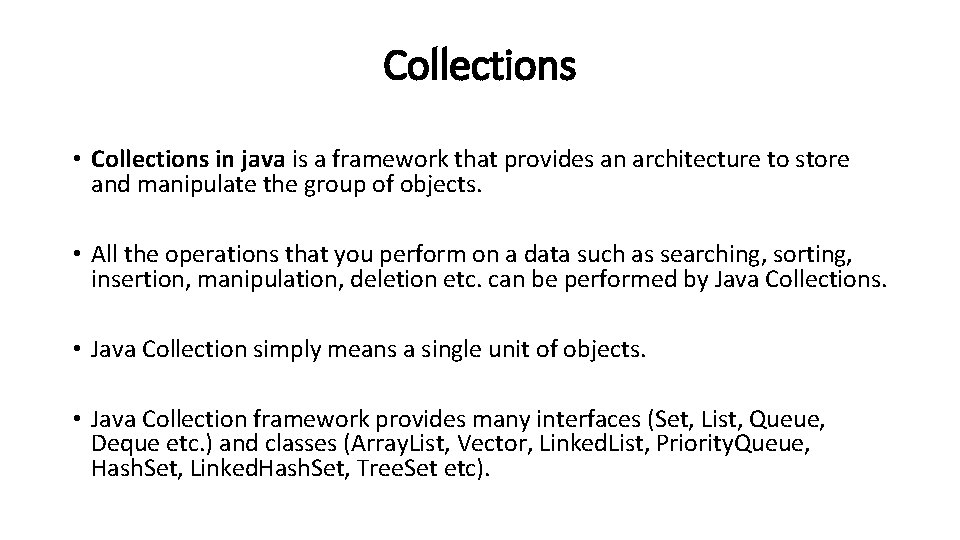
Collections • Collections in java is a framework that provides an architecture to store and manipulate the group of objects. • All the operations that you perform on a data such as searching, sorting, insertion, manipulation, deletion etc. can be performed by Java Collections. • Java Collection simply means a single unit of objects. • Java Collection framework provides many interfaces (Set, List, Queue, Deque etc. ) and classes (Array. List, Vector, Linked. List, Priority. Queue, Hash. Set, Linked. Hash. Set, Tree. Set etc).
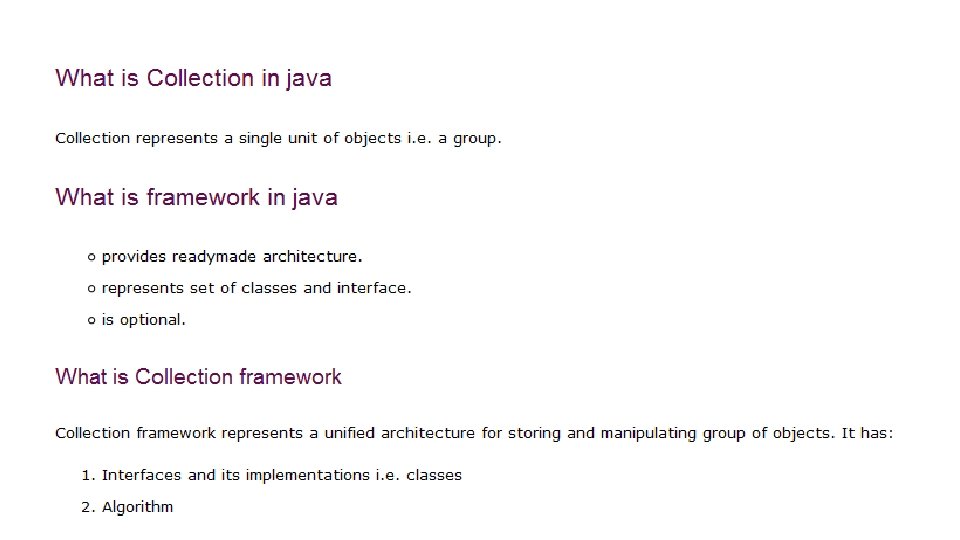
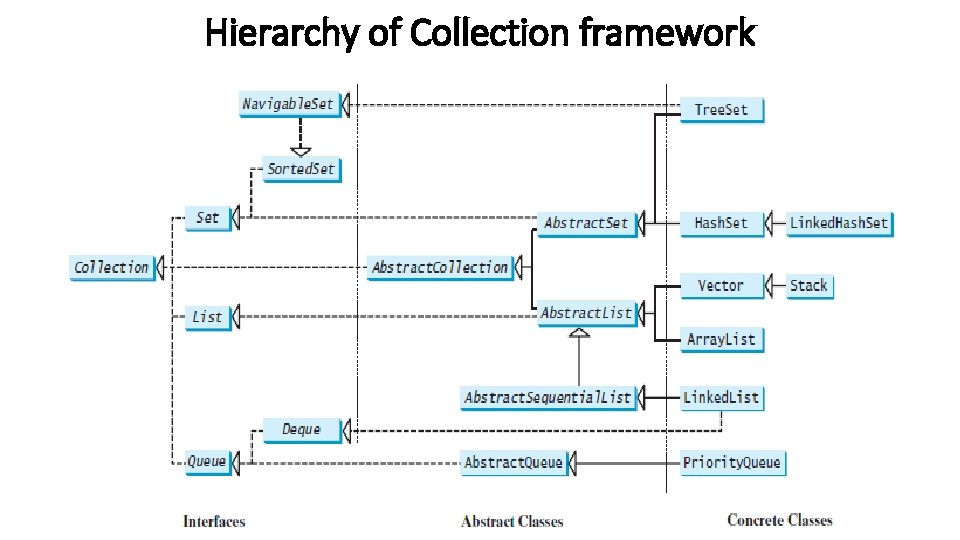
Hierarchy of Collection framework
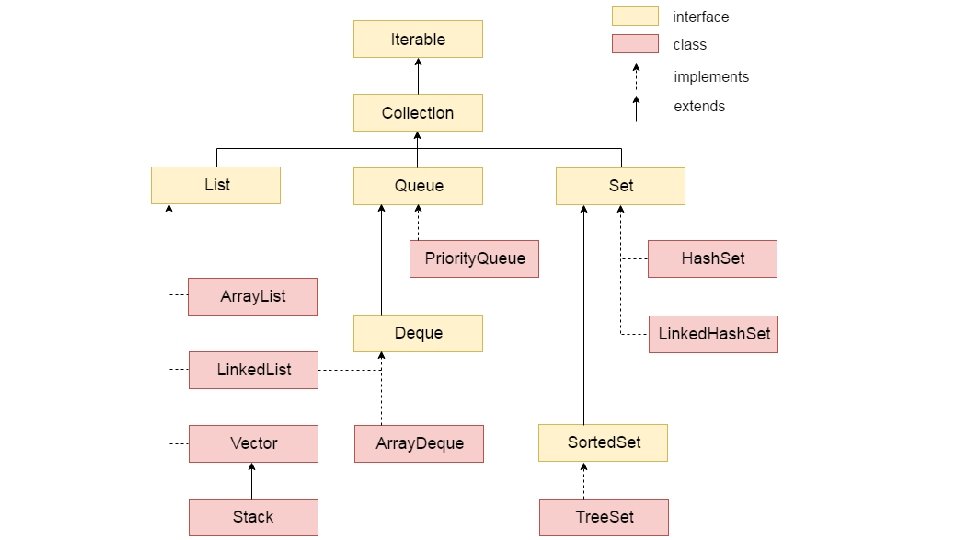
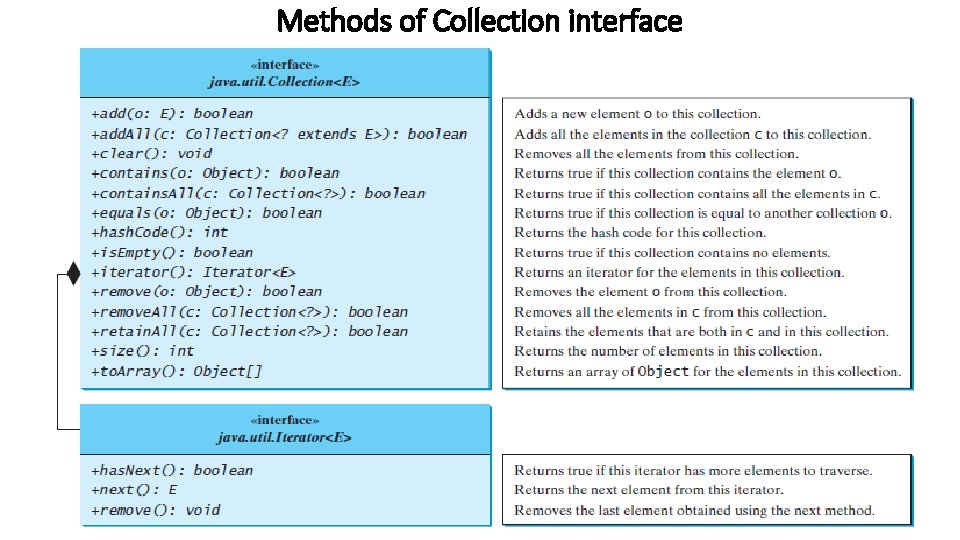
Methods of Collection interface
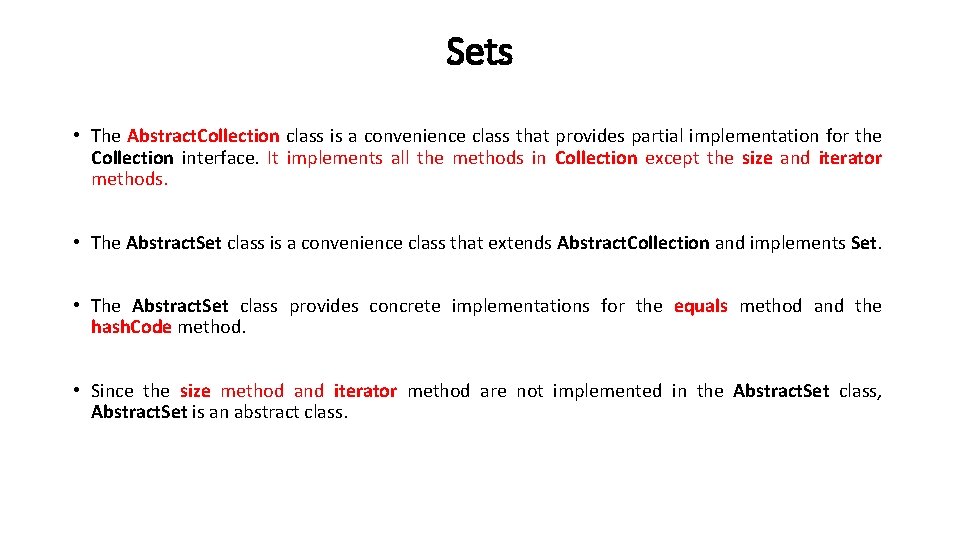
Sets • The Abstract. Collection class is a convenience class that provides partial implementation for the Collection interface. It implements all the methods in Collection except the size and iterator methods. • The Abstract. Set class is a convenience class that extends Abstract. Collection and implements Set. • The Abstract. Set class provides concrete implementations for the equals method and the hash. Code method. • Since the size method and iterator method are not implemented in the Abstract. Set class, Abstract. Set is an abstract class.
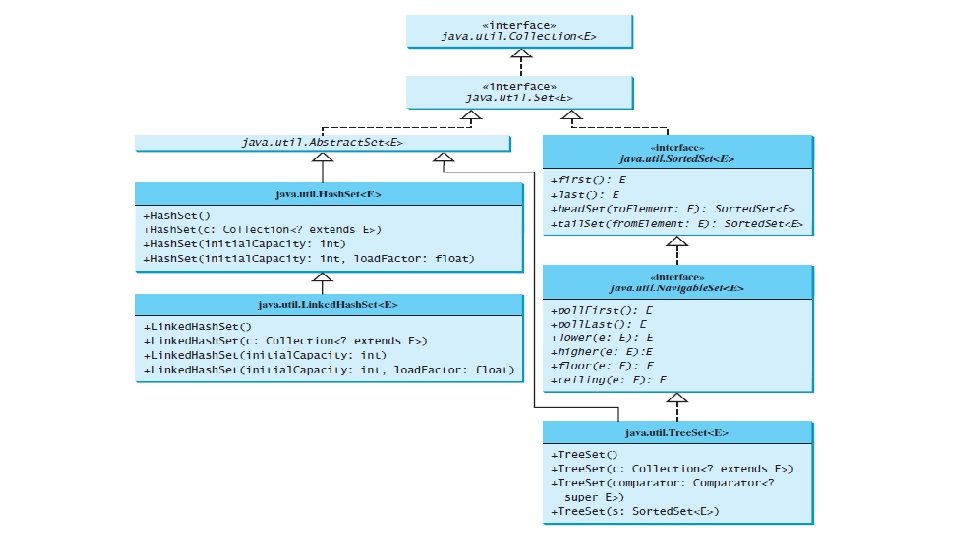
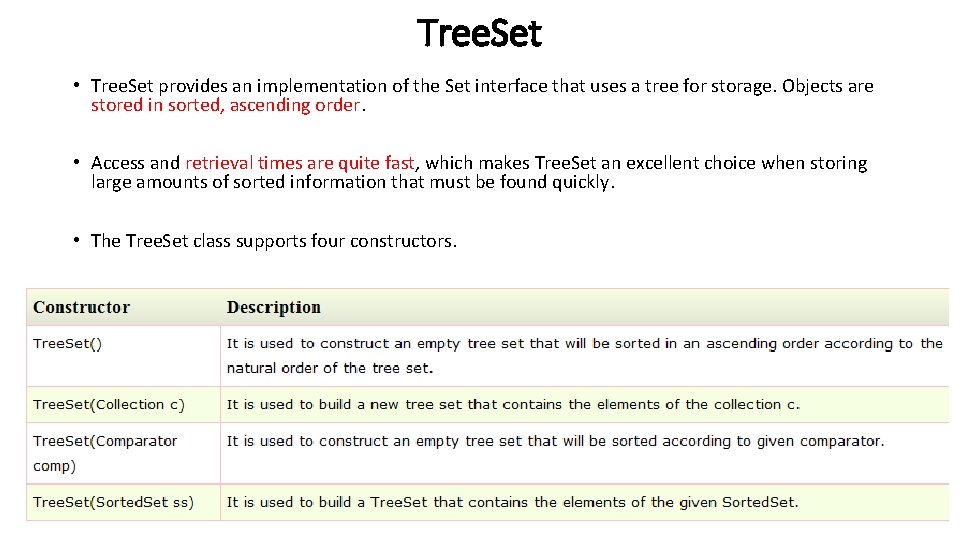
Tree. Set • Tree. Set provides an implementation of the Set interface that uses a tree for storage. Objects are stored in sorted, ascending order. • Access and retrieval times are quite fast, which makes Tree. Set an excellent choice when storing large amounts of sorted information that must be found quickly. • The Tree. Set class supports four constructors.
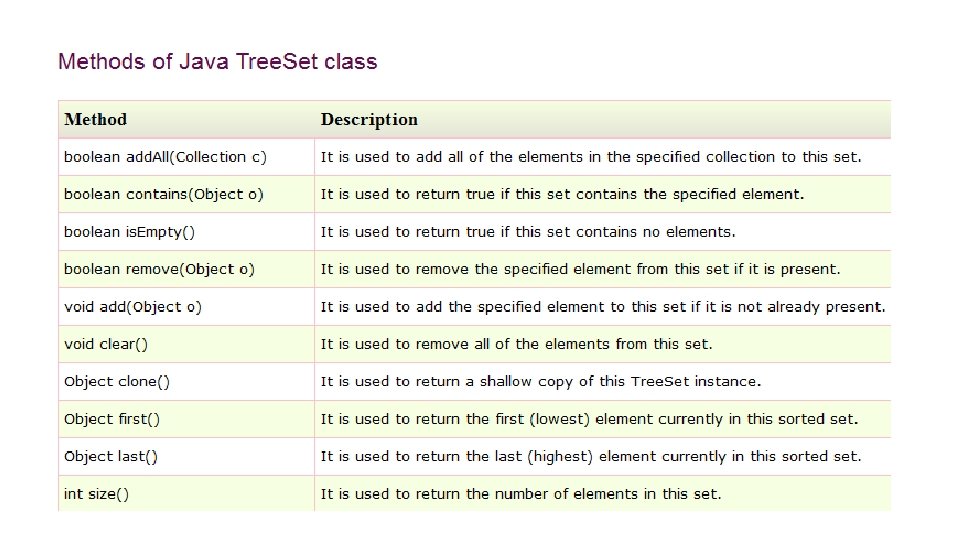
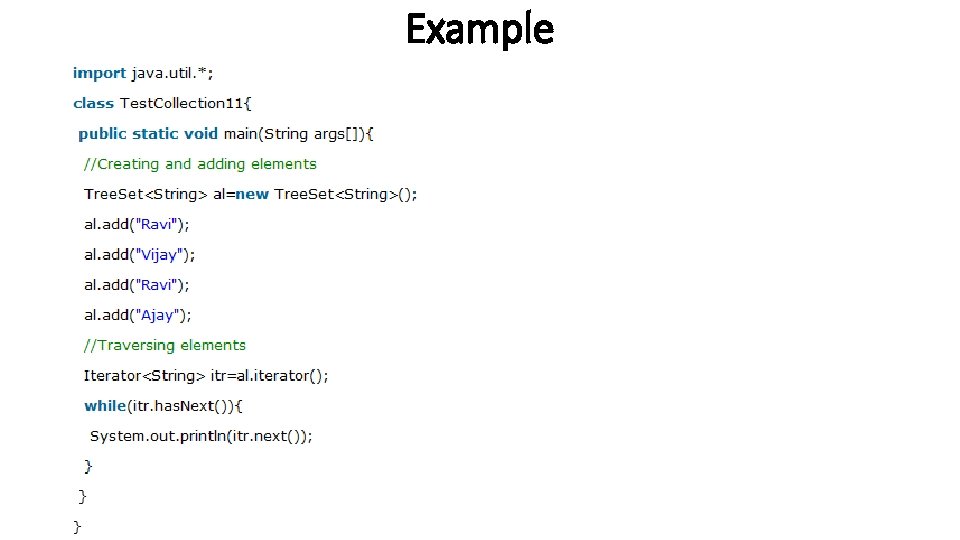
Example
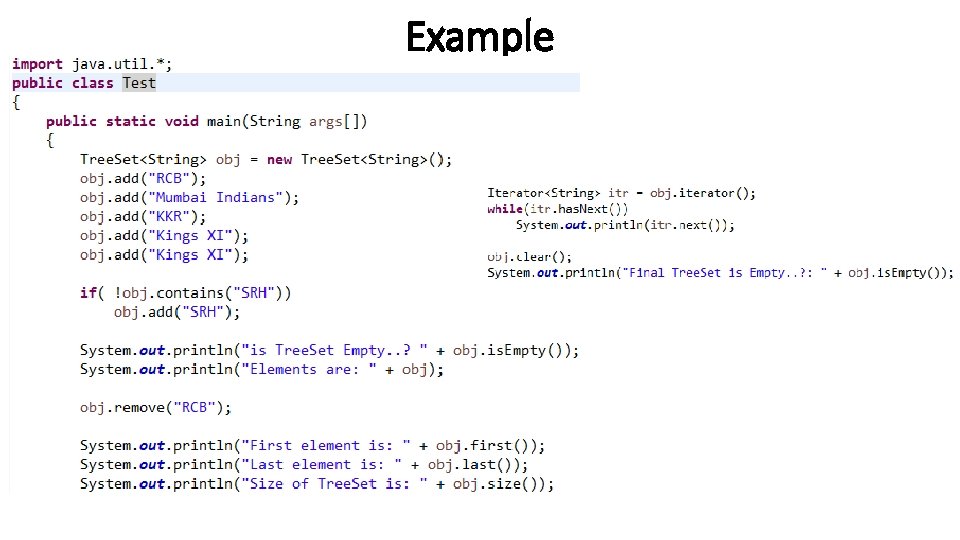
Example
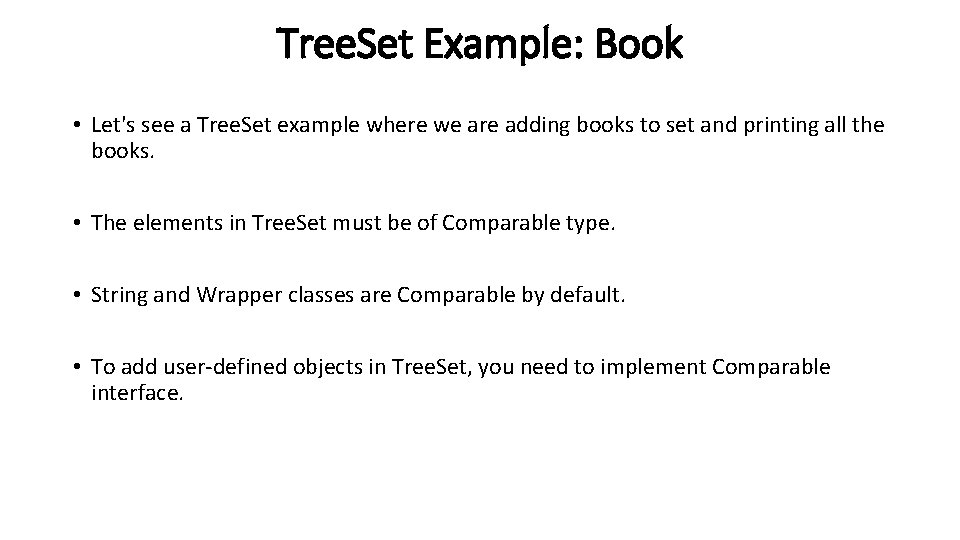
Tree. Set Example: Book • Let's see a Tree. Set example where we are adding books to set and printing all the books. • The elements in Tree. Set must be of Comparable type. • String and Wrapper classes are Comparable by default. • To add user-defined objects in Tree. Set, you need to implement Comparable interface.
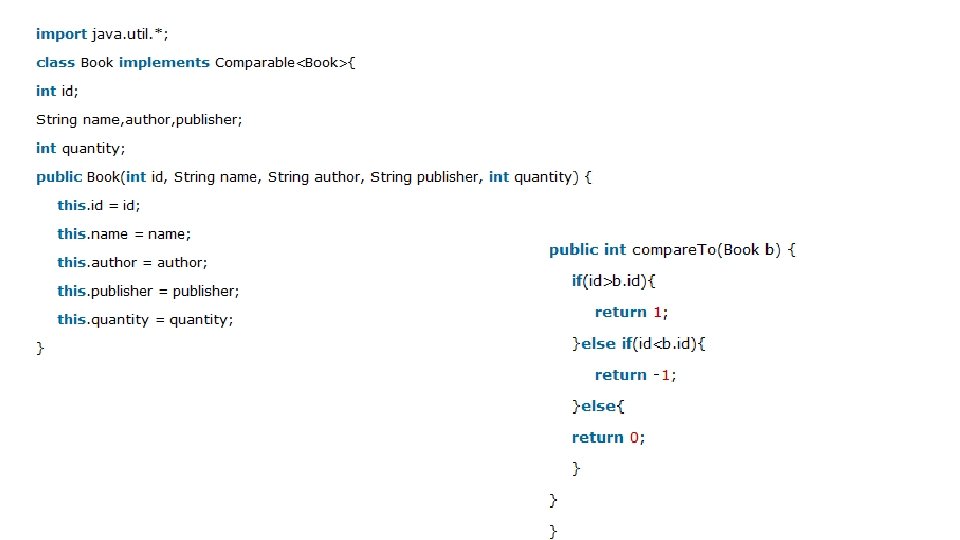
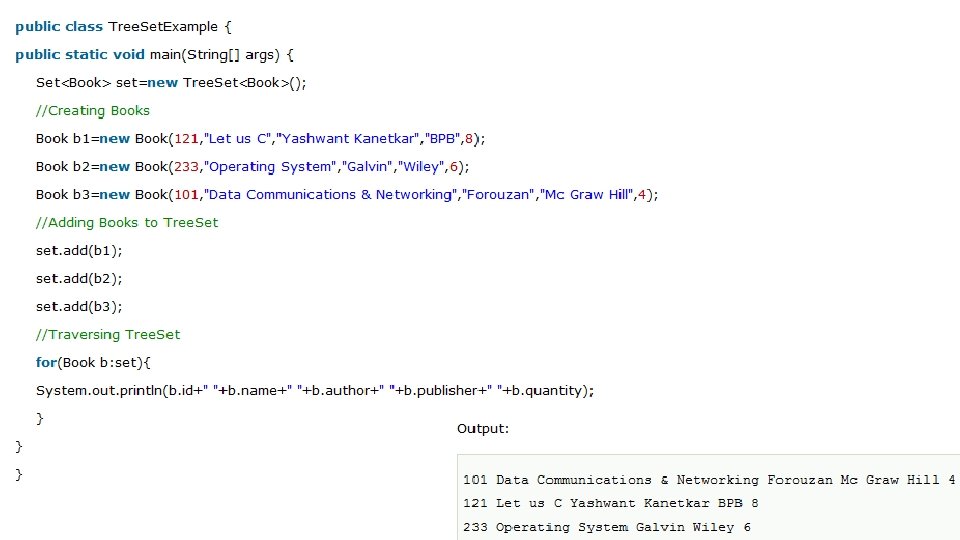
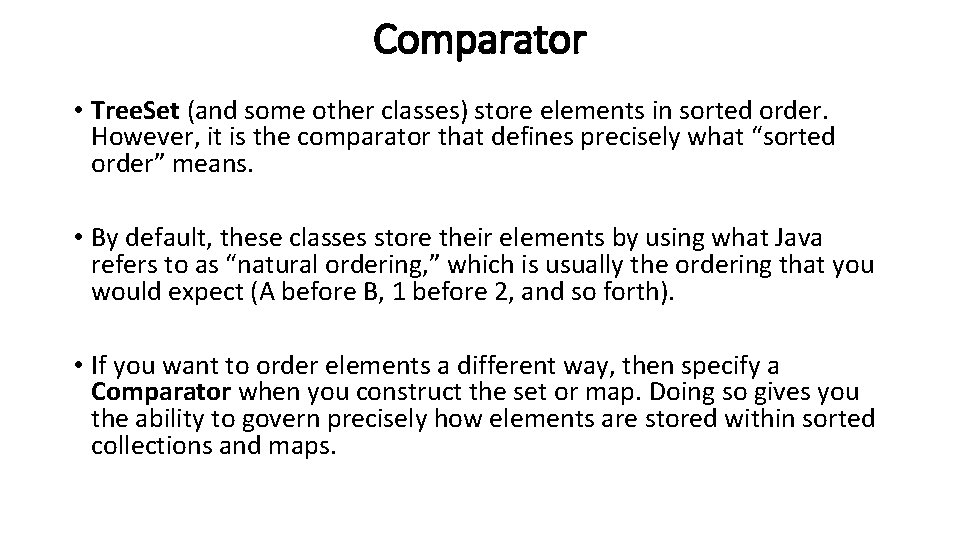
Comparator • Tree. Set (and some other classes) store elements in sorted order. However, it is the comparator that defines precisely what “sorted order” means. • By default, these classes store their elements by using what Java refers to as “natural ordering, ” which is usually the ordering that you would expect (A before B, 1 before 2, and so forth). • If you want to order elements a different way, then specify a Comparator when you construct the set or map. Doing so gives you the ability to govern precisely how elements are stored within sorted collections and maps.
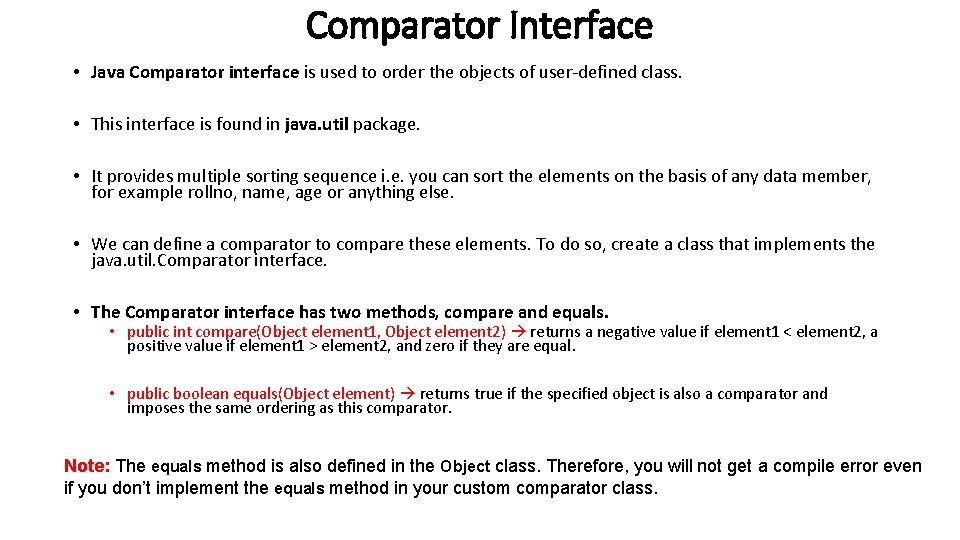
Comparator Interface • Java Comparator interface is used to order the objects of user-defined class. • This interface is found in java. util package. • It provides multiple sorting sequence i. e. you can sort the elements on the basis of any data member, for example rollno, name, age or anything else. • We can define a comparator to compare these elements. To do so, create a class that implements the java. util. Comparator interface. • The Comparator interface has two methods, compare and equals. • public int compare(Object element 1, Object element 2) returns a negative value if element 1 < element 2, a positive value if element 1 > element 2, and zero if they are equal. • public boolean equals(Object element) returns true if the specified object is also a comparator and imposes the same ordering as this comparator. Note: The equals method is also defined in the Object class. Therefore, you will not get a compile error even if you don’t implement the equals method in your custom comparator class.
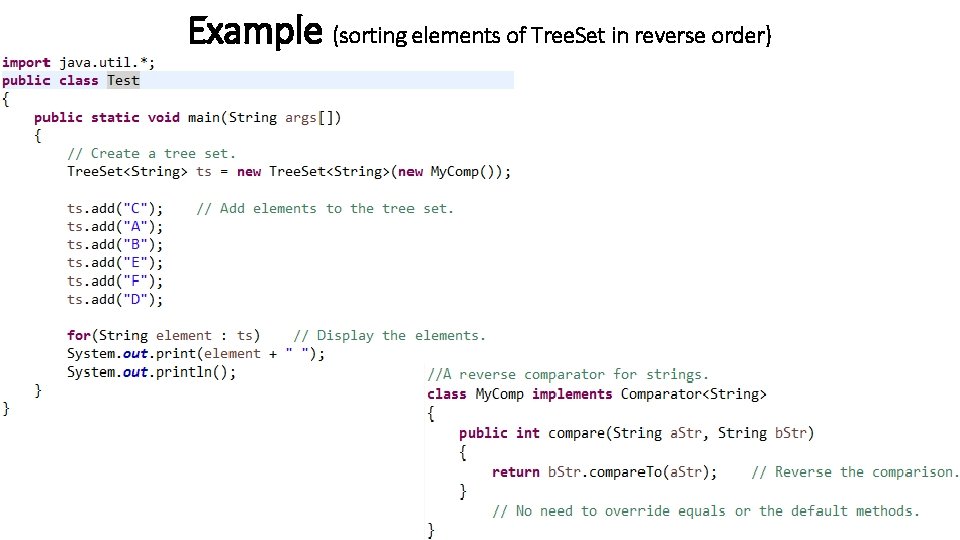
Example (sorting elements of Tree. Set in reverse order)
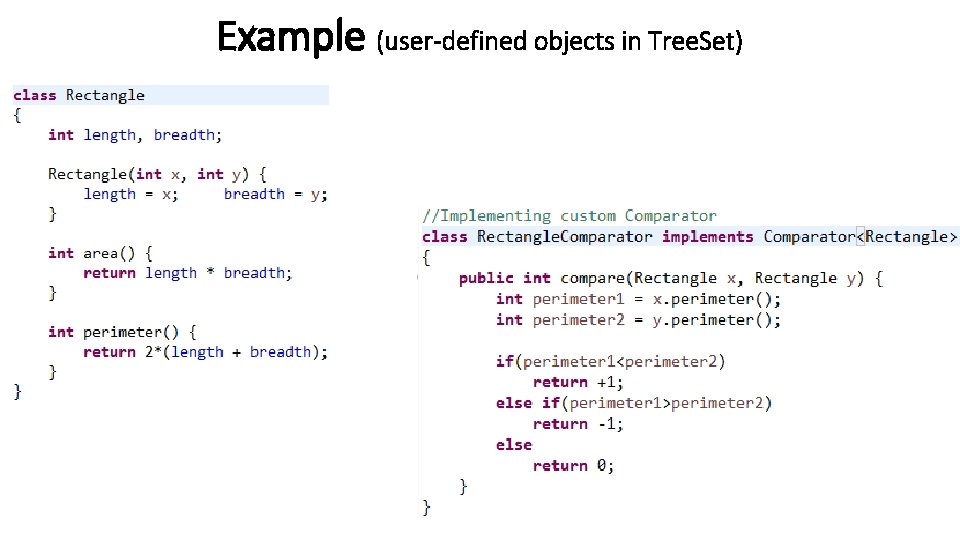
Example (user-defined objects in Tree. Set)
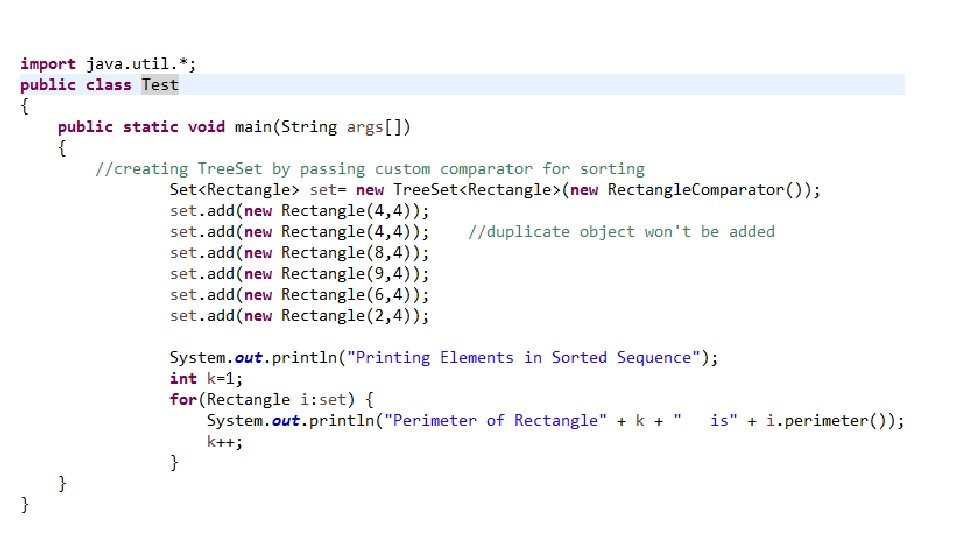
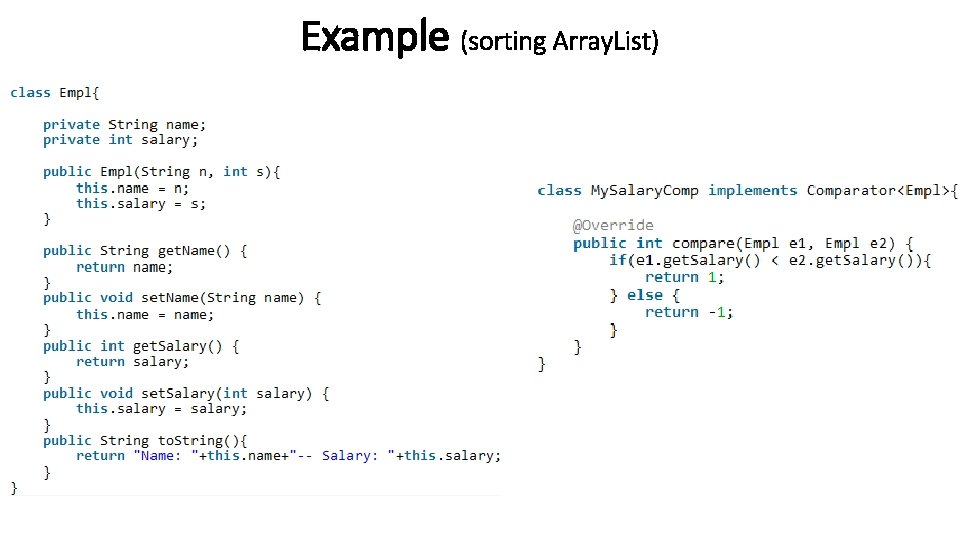
Example (sorting Array. List)
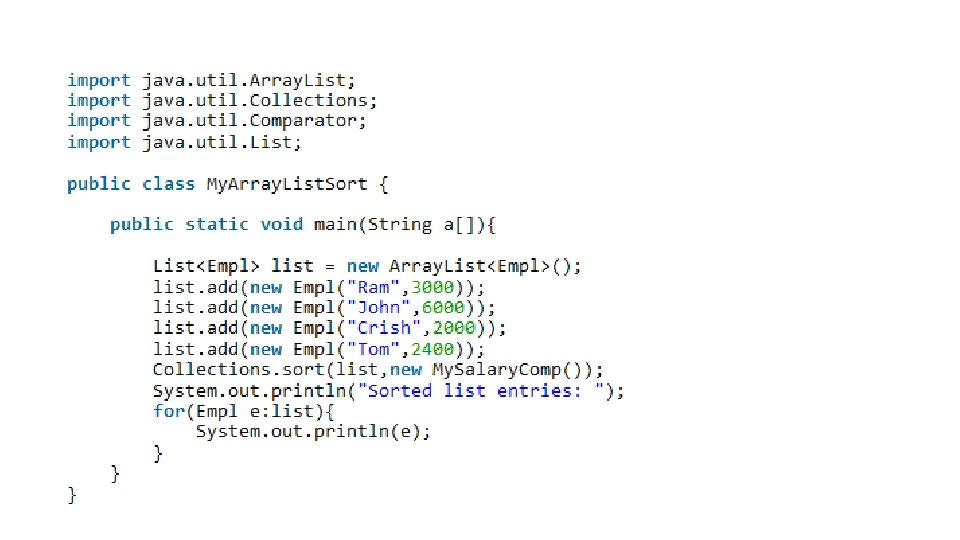
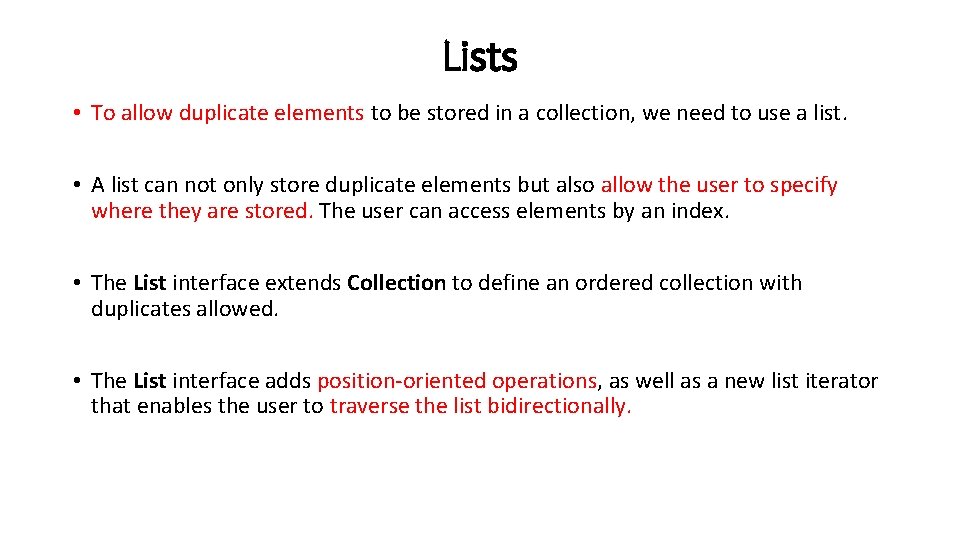
Lists • To allow duplicate elements to be stored in a collection, we need to use a list. • A list can not only store duplicate elements but also allow the user to specify where they are stored. The user can access elements by an index. • The List interface extends Collection to define an ordered collection with duplicates allowed. • The List interface adds position-oriented operations, as well as a new list iterator that enables the user to traverse the list bidirectionally.
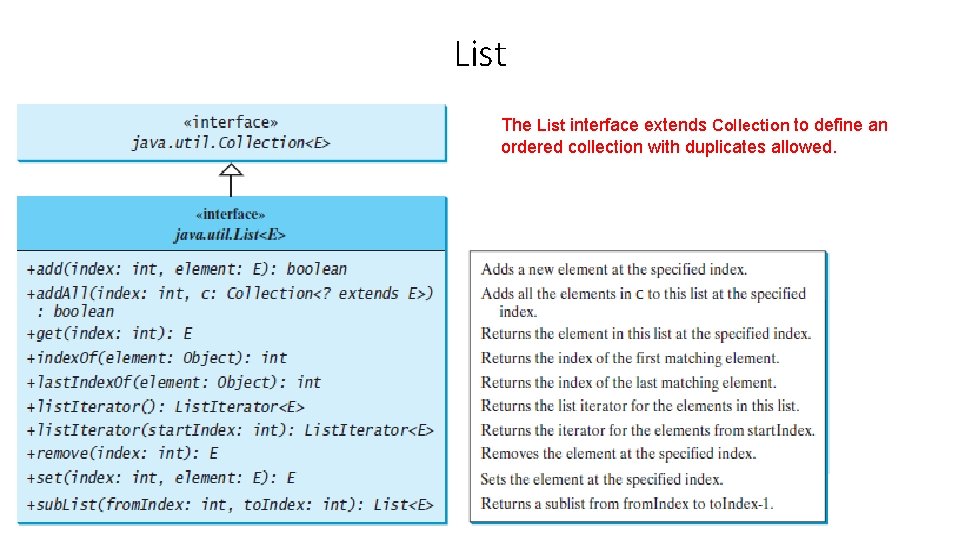
List The List interface extends Collection to define an ordered collection with duplicates allowed.
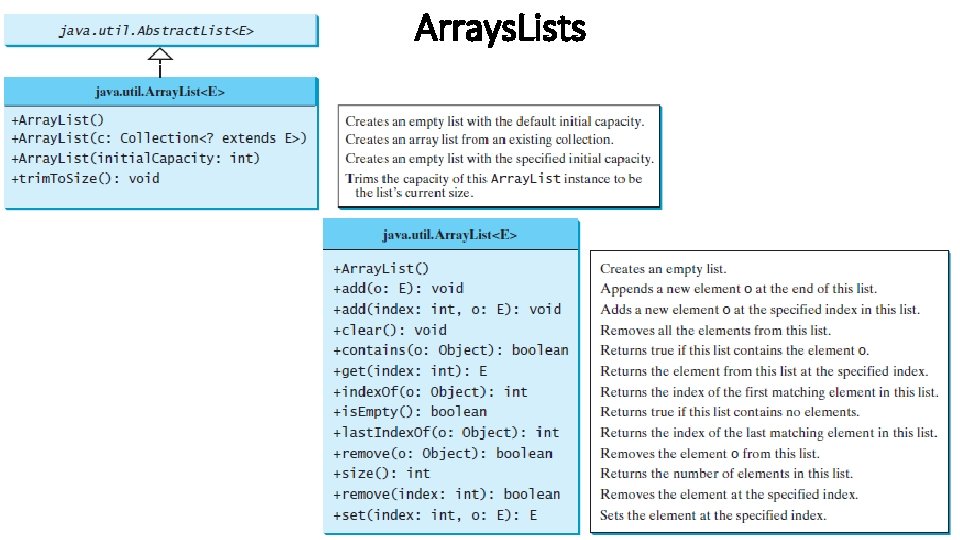
Arrays. Lists
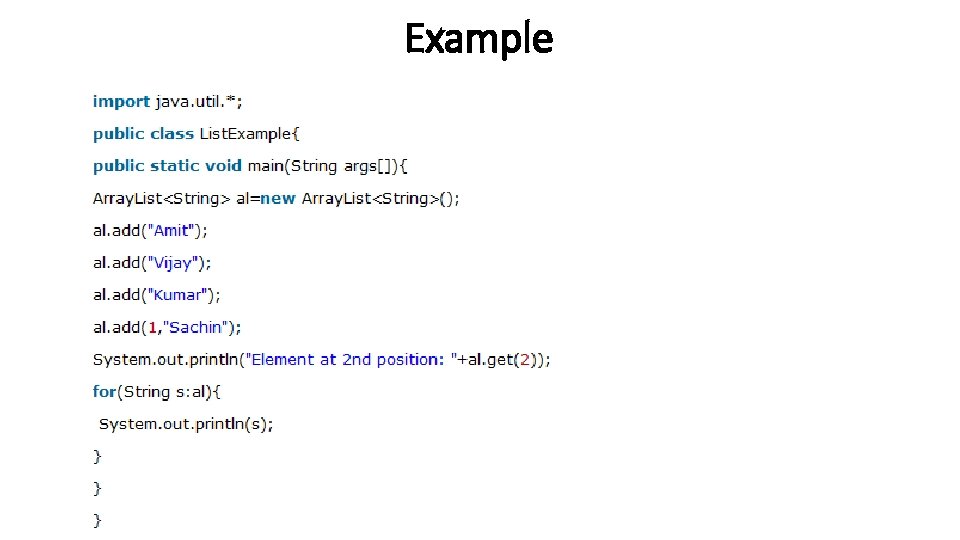
Example
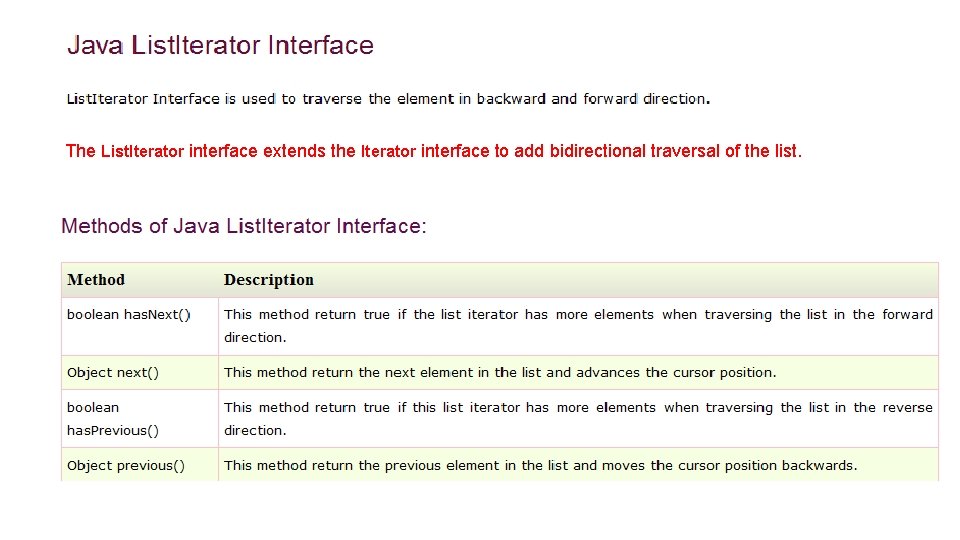
The List. Iterator interface extends the Iterator interface to add bidirectional traversal of the list.
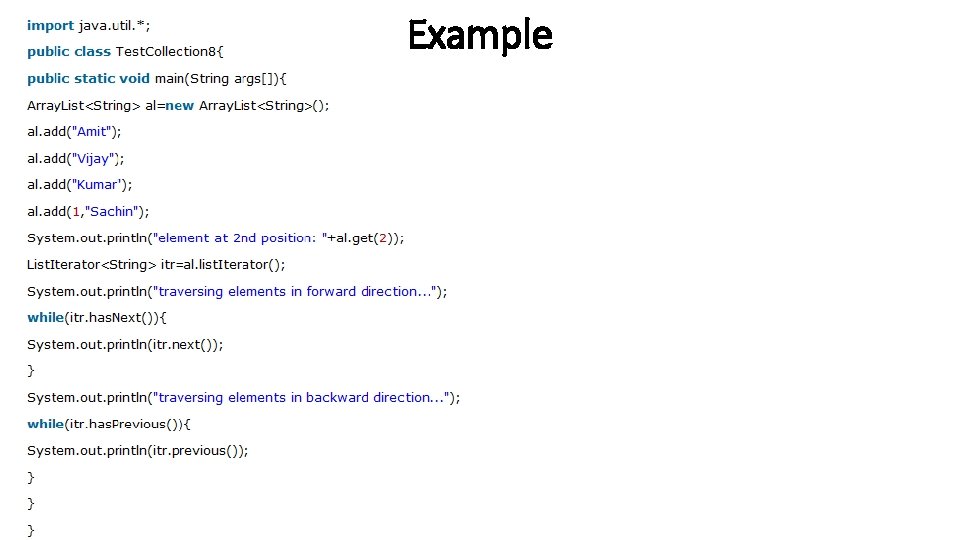
Example
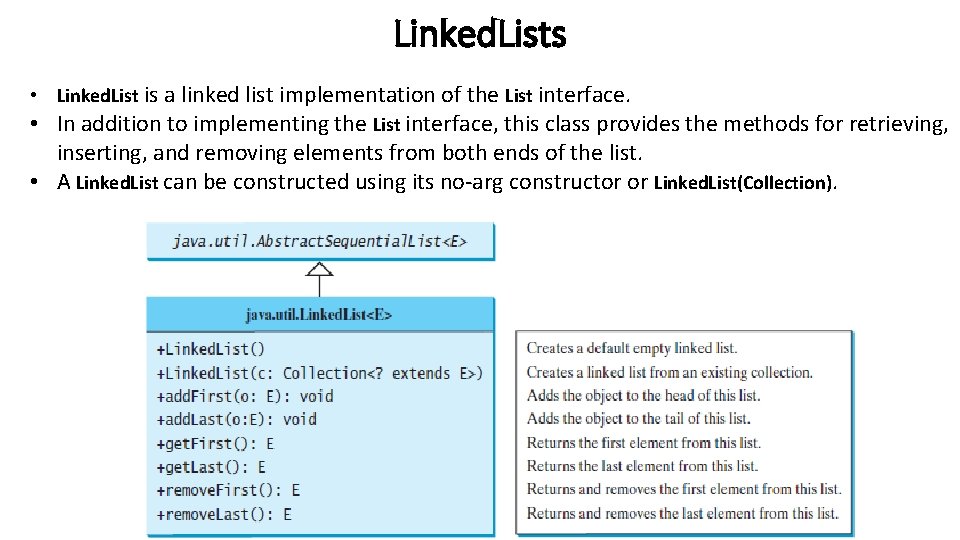
Linked. Lists • Linked. List is a linked list implementation of the List interface. • In addition to implementing the List interface, this class provides the methods for retrieving, inserting, and removing elements from both ends of the list. • A Linked. List can be constructed using its no-arg constructor or Linked. List(Collection).
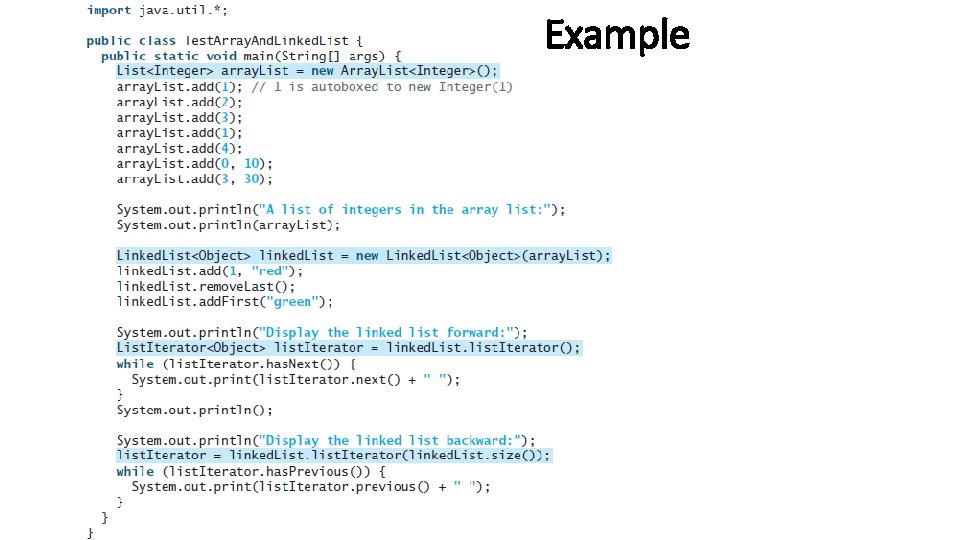
Example
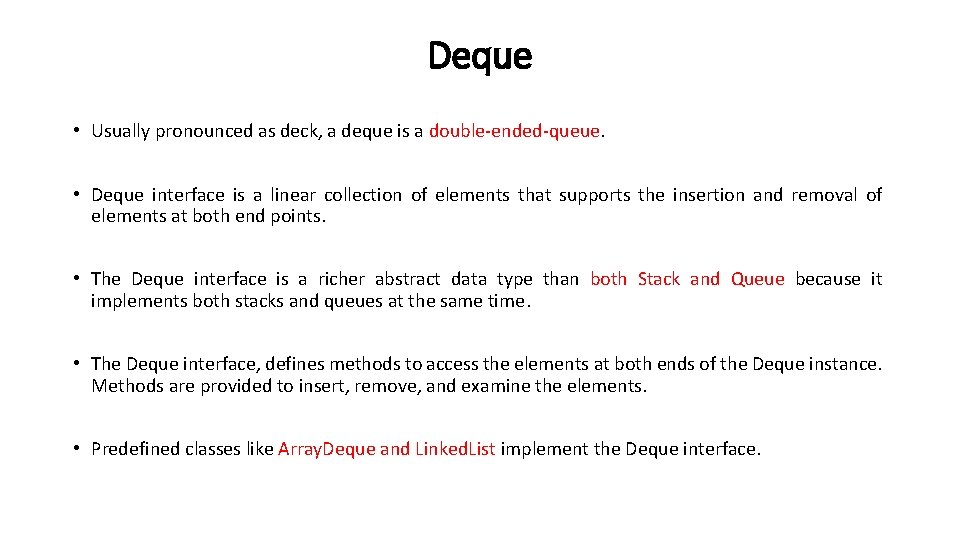
Deque • Usually pronounced as deck, a deque is a double-ended-queue. • Deque interface is a linear collection of elements that supports the insertion and removal of elements at both end points. • The Deque interface is a richer abstract data type than both Stack and Queue because it implements both stacks and queues at the same time. • The Deque interface, defines methods to access the elements at both ends of the Deque instance. Methods are provided to insert, remove, and examine the elements. • Predefined classes like Array. Deque and Linked. List implement the Deque interface.
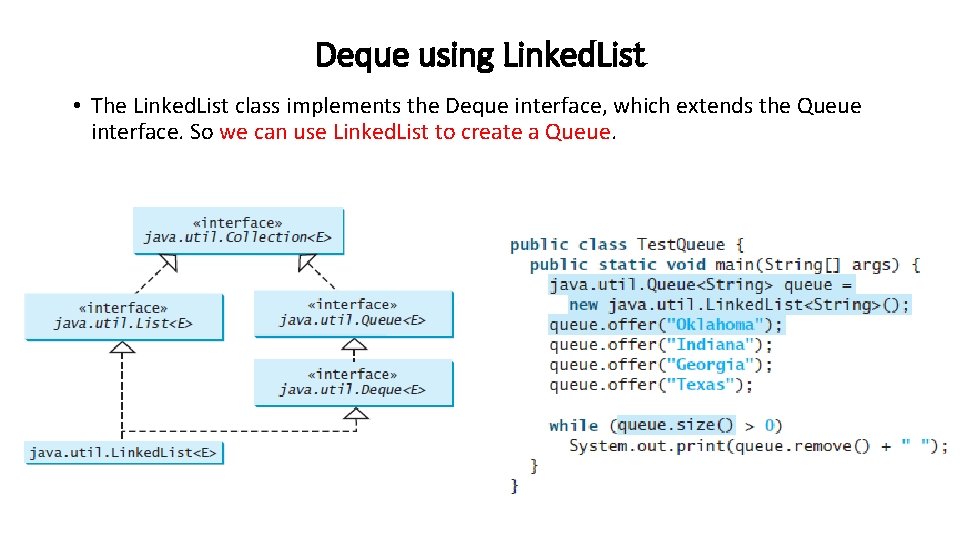
Deque using Linked. List • The Linked. List class implements the Deque interface, which extends the Queue interface. So we can use Linked. List to create a Queue.
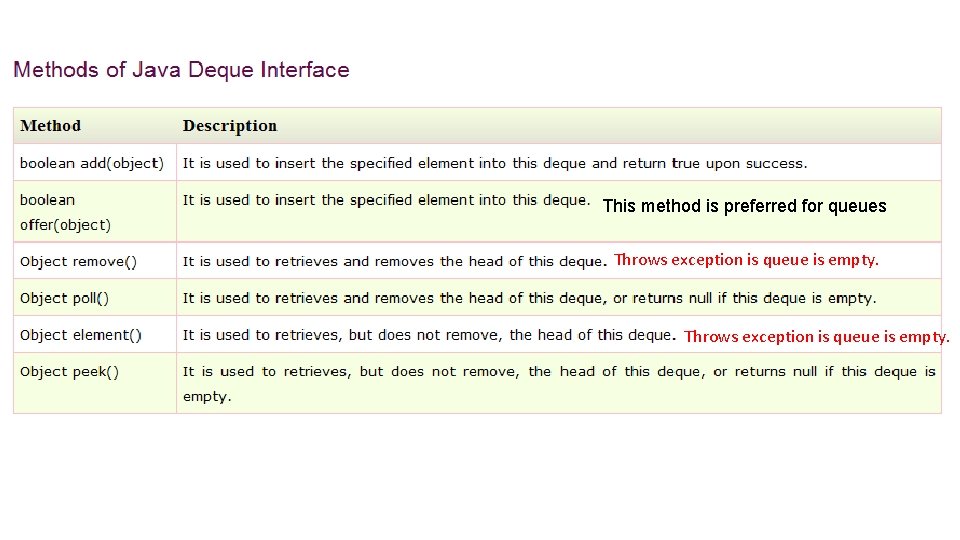
This method is preferred for queues Throws exception is queue is empty.
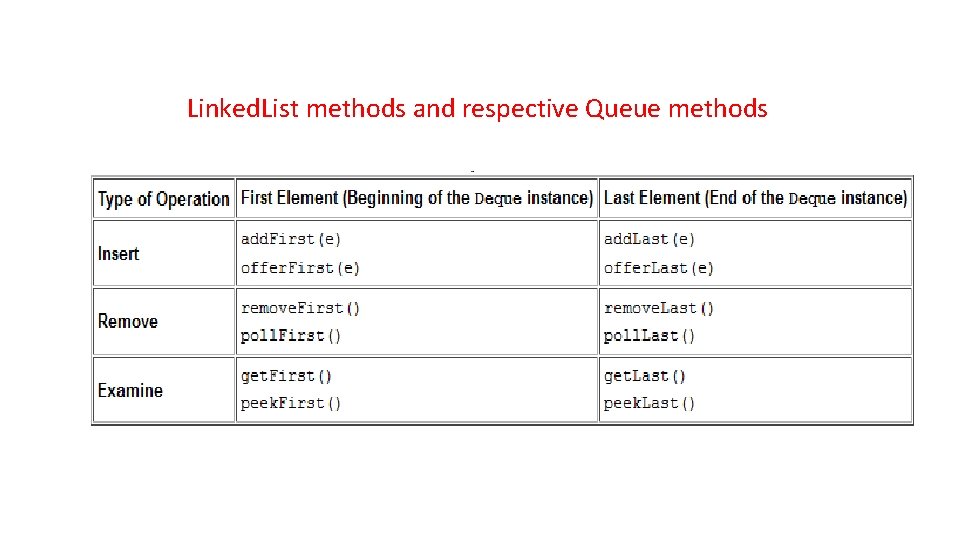
Linked. List methods and respective Queue methods
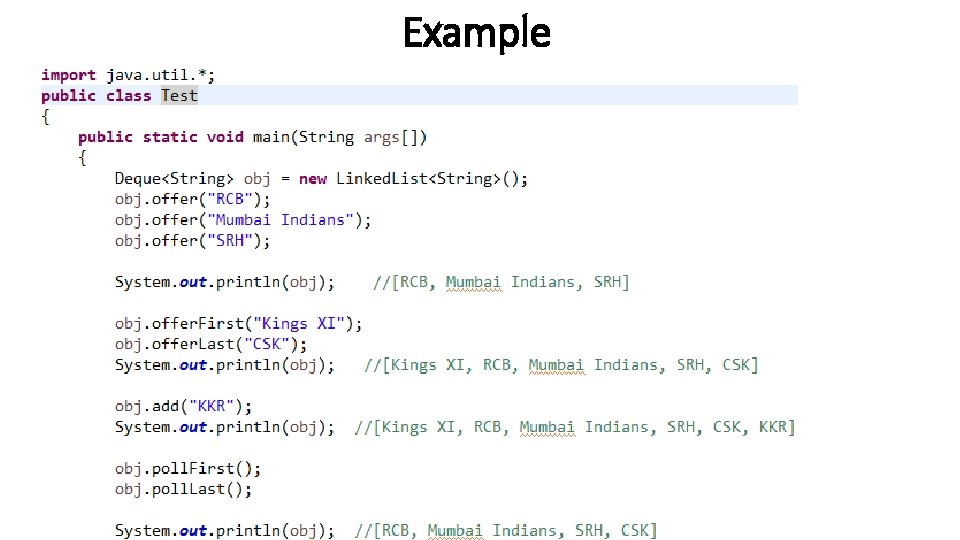
Example
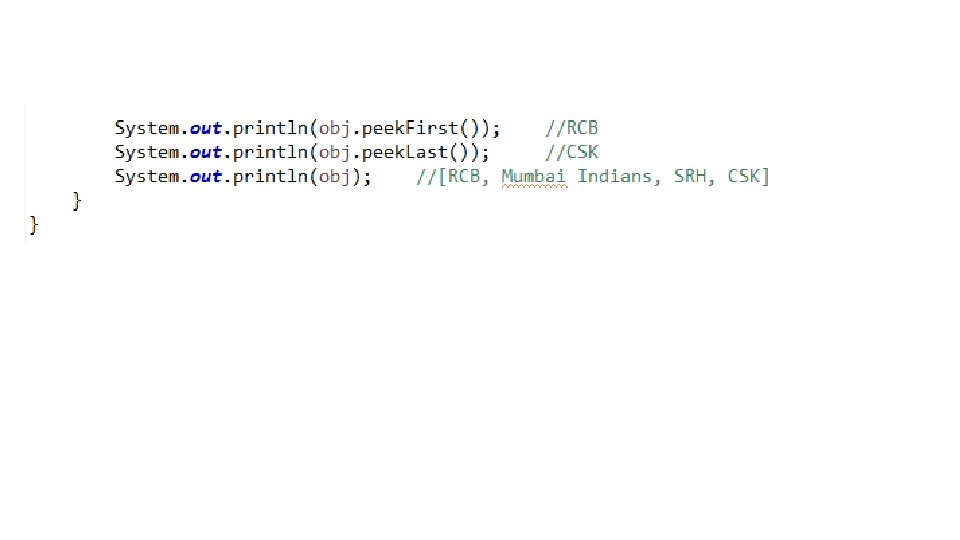
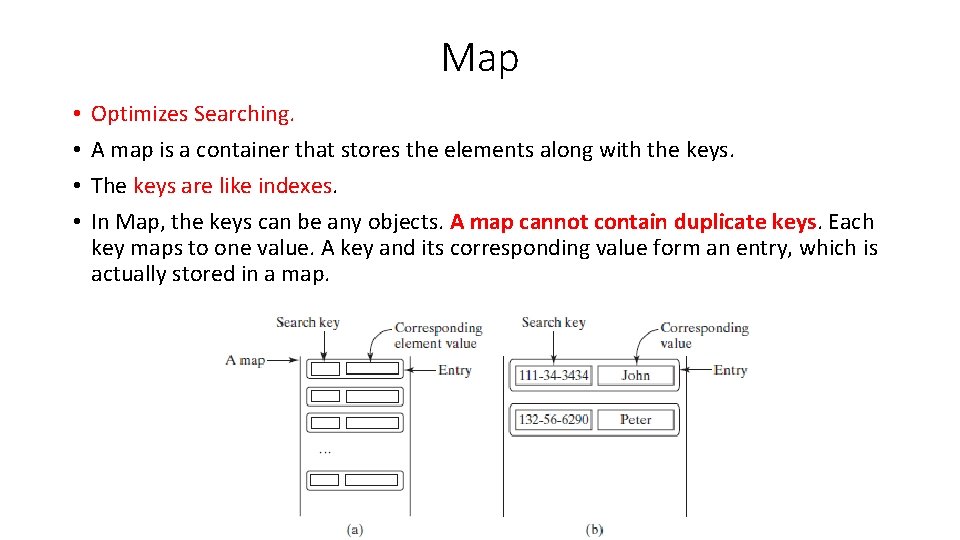
Map • • Optimizes Searching. A map is a container that stores the elements along with the keys. The keys are like indexes. In Map, the keys can be any objects. A map cannot contain duplicate keys. Each key maps to one value. A key and its corresponding value form an entry, which is actually stored in a map.
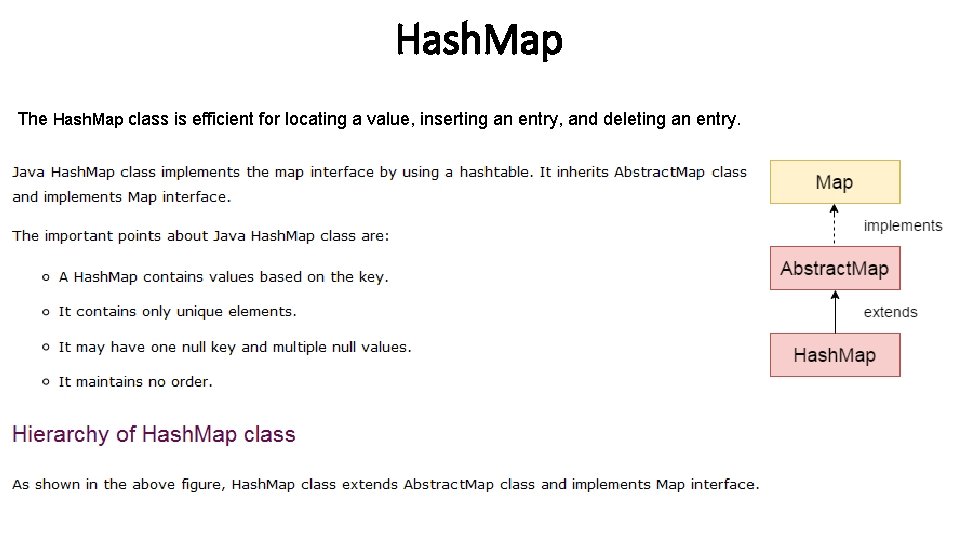
Hash. Map The Hash. Map class is efficient for locating a value, inserting an entry, and deleting an entry.
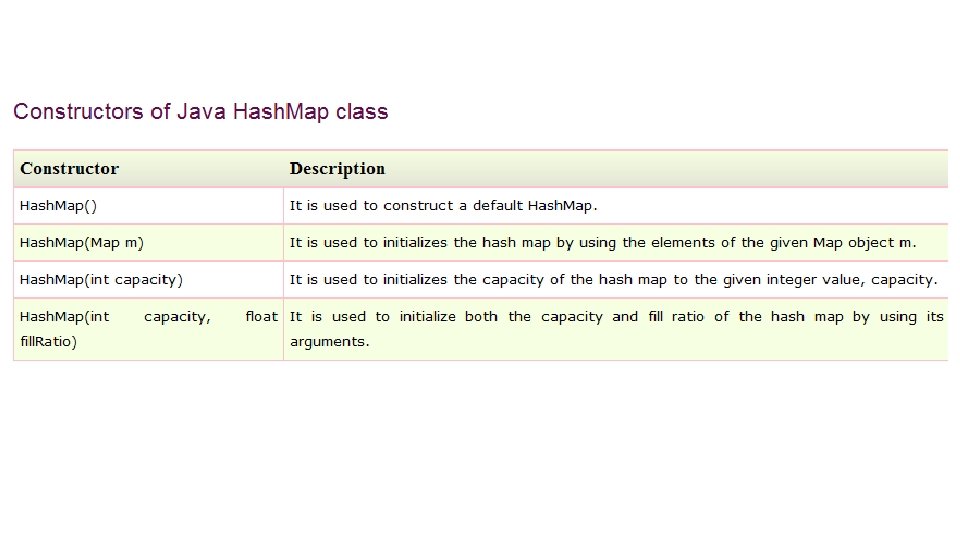
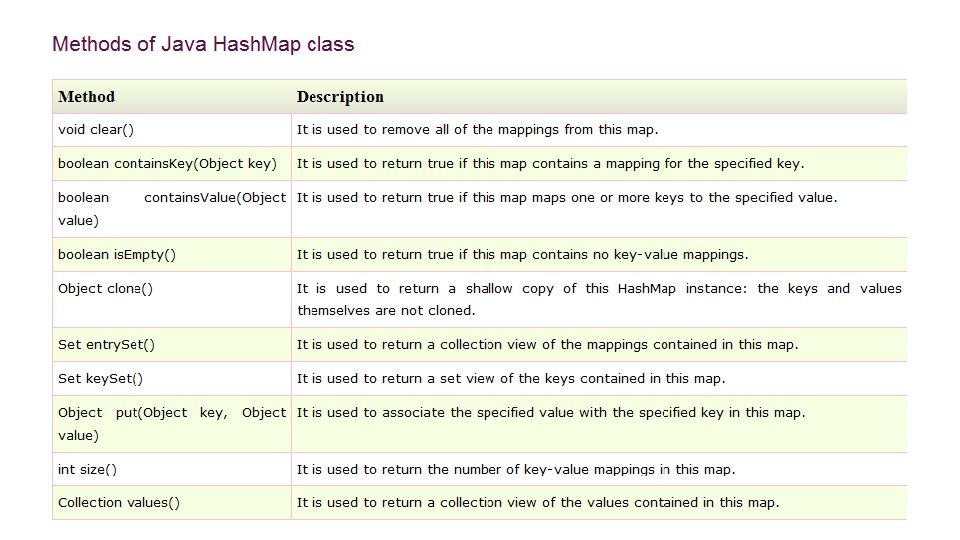
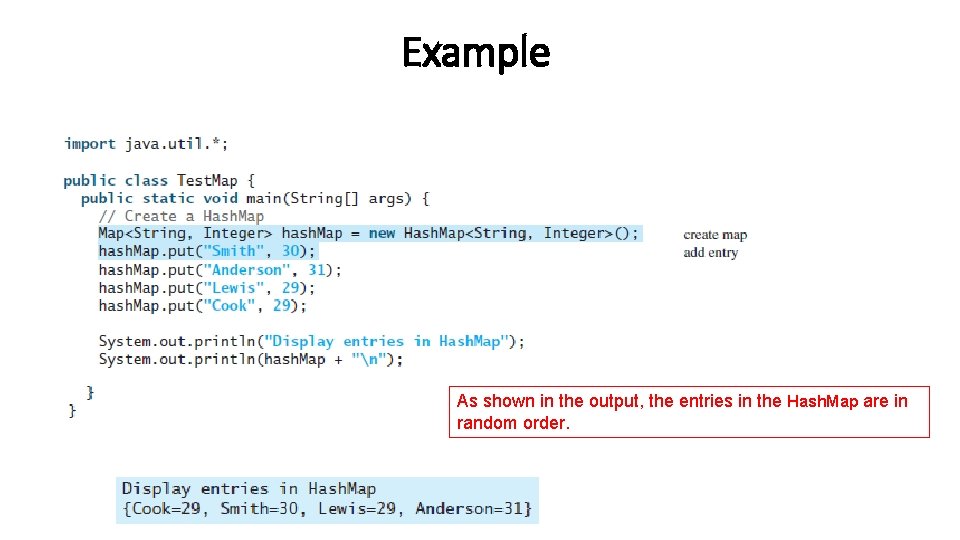
Example As shown in the output, the entries in the Hash. Map are in random order.
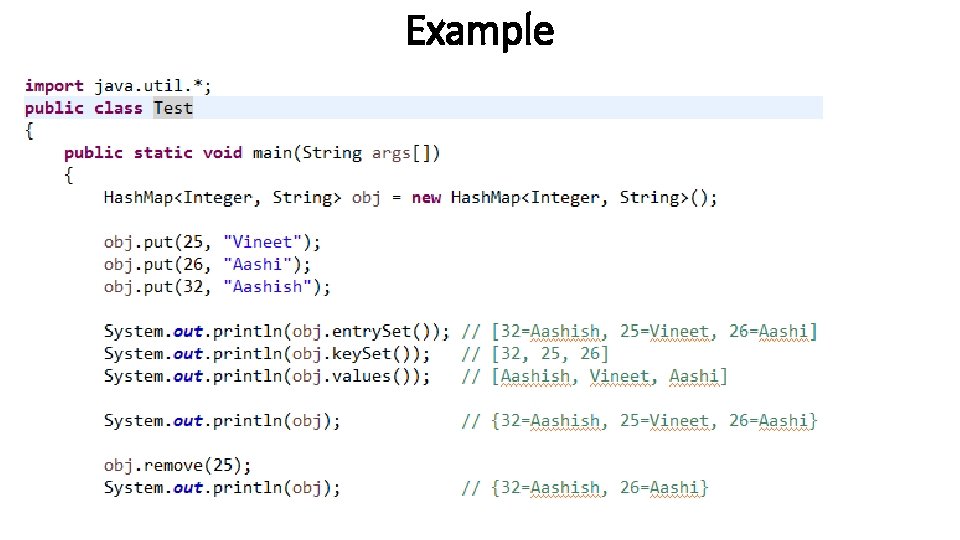
Example
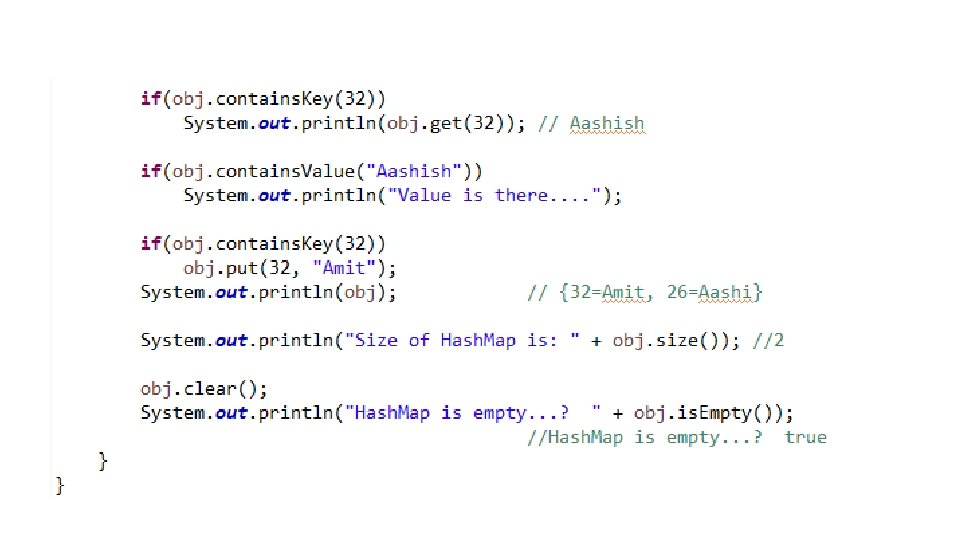
Landsat collection 1 vs collection 2
D/a 30 days
Collection hierarchy
Stop and copy garbage collection
Import java.util.* public class test
иерархия коллекций
Lesson 3.1 introduction to data collection
Rodak
Specimen collection introduction
Dispositional framework vs regulatory framework
Example of theoretical and conceptual framework
Conceptual framework maker
Theoretical framework
Dispositional framework vs regulatory framework
Theoretical framework example
Jade java
Java ecommerce framework
Java collections framework diagram
Java media framework
Java agent development framework tutorial
Ssh lee
Birt scripting
Java component framework
Java
En el framework de colecciones de java un set es:
Java plugin framework
Ajax framework
Java import java.util.*
Java import java.util.*
Import java swing
Import.java.util.scanner
Import java.util.*
Gcd java
Java random
Java import java.io.*
Import java
Java thread import
Awt adalah
Import java.awt.event.*
Language
Java bean vs enterprise java bean
Spring introduction
Relentless improvement safe
How to install apache struts
Problem solving