Java Collection Framework Interface Collection addo clear containso
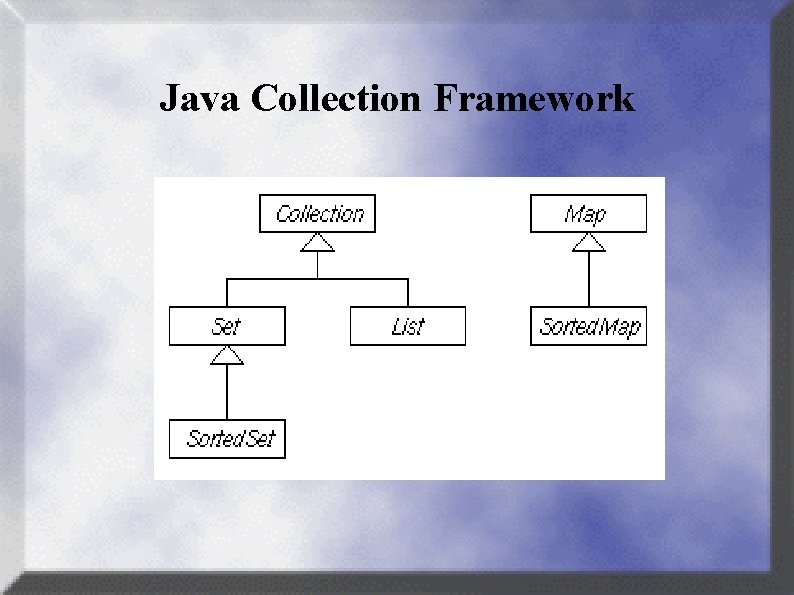
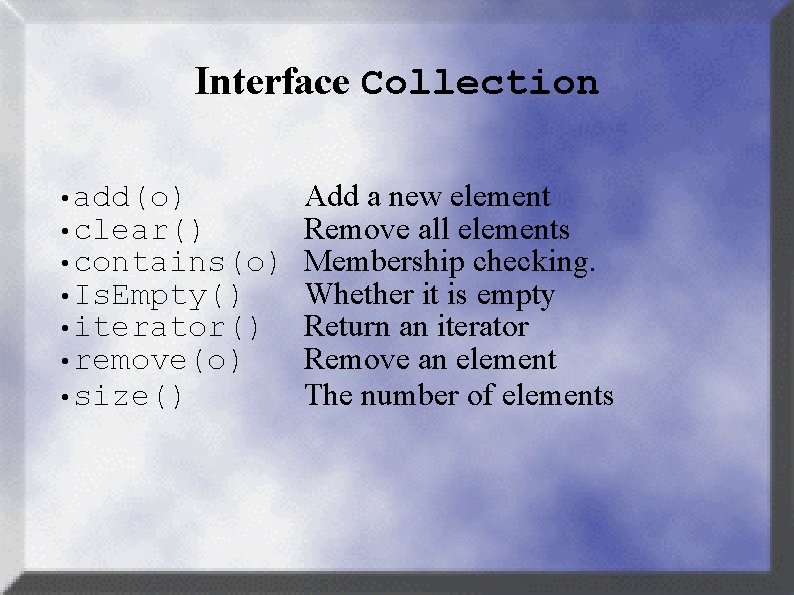
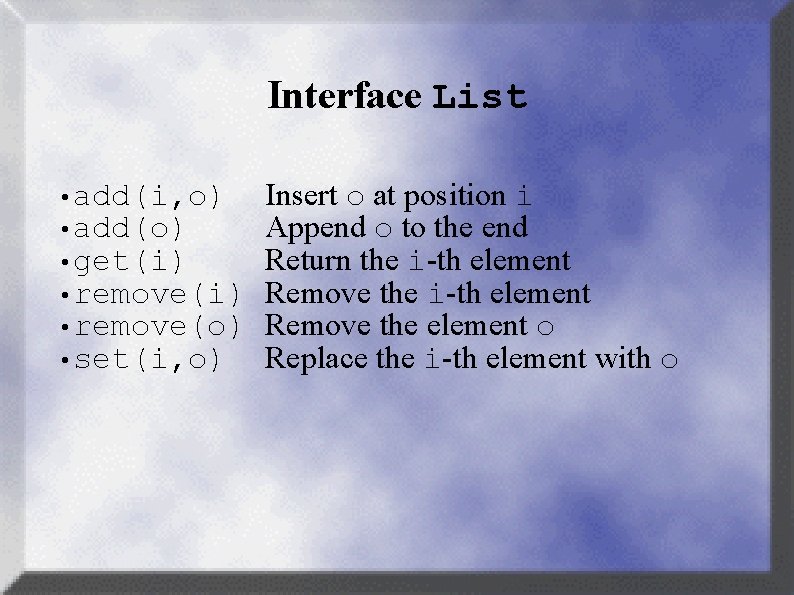
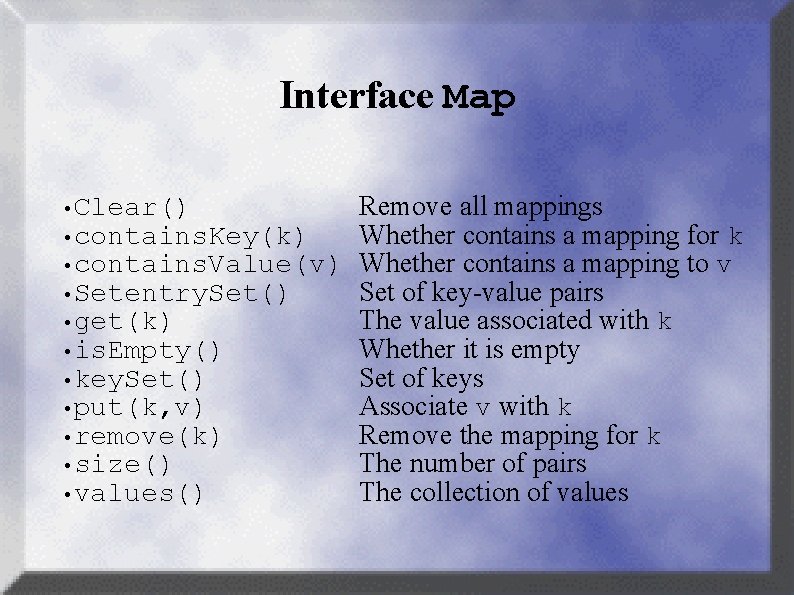
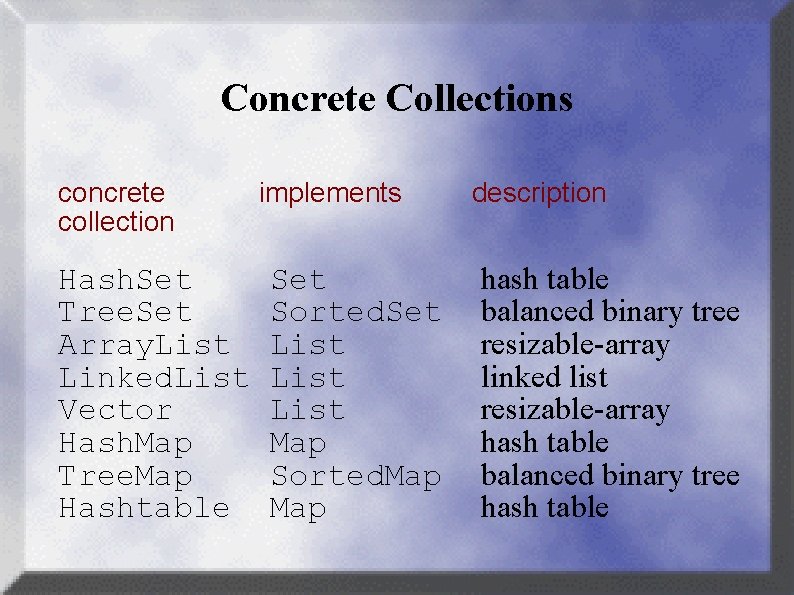
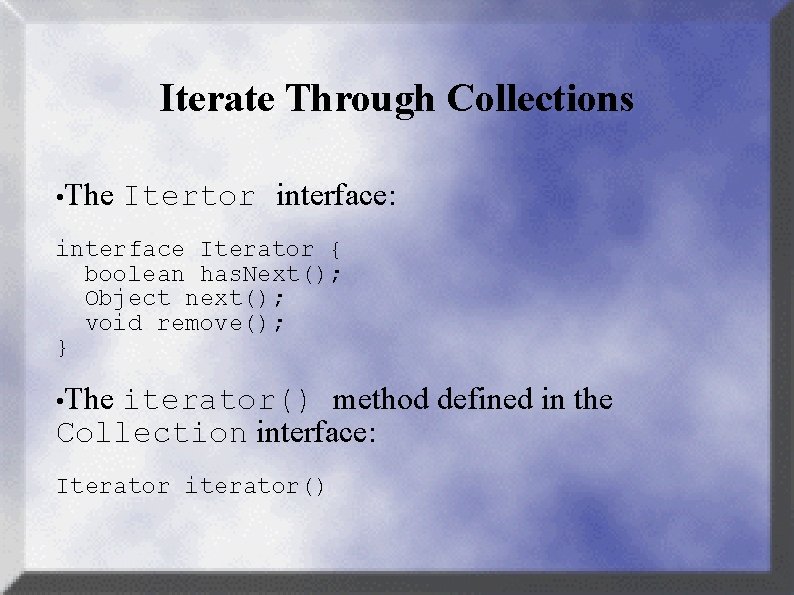
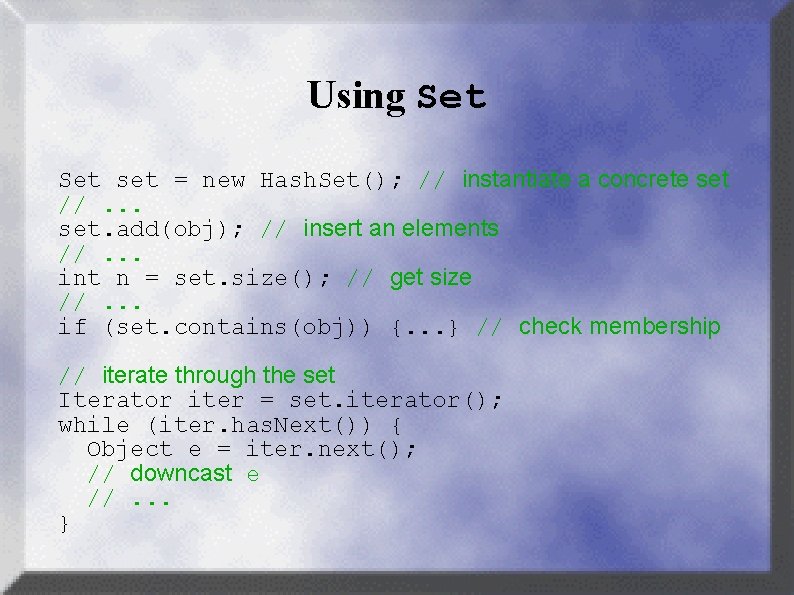
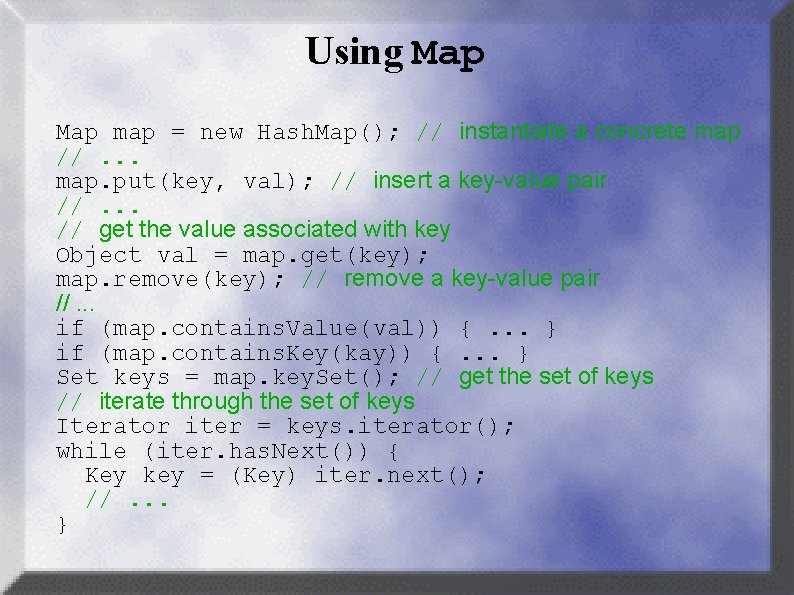
![Counting Different Words public class Count. Words { static public void main(String[] args) { Counting Different Words public class Count. Words { static public void main(String[] args) {](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-9.jpg)
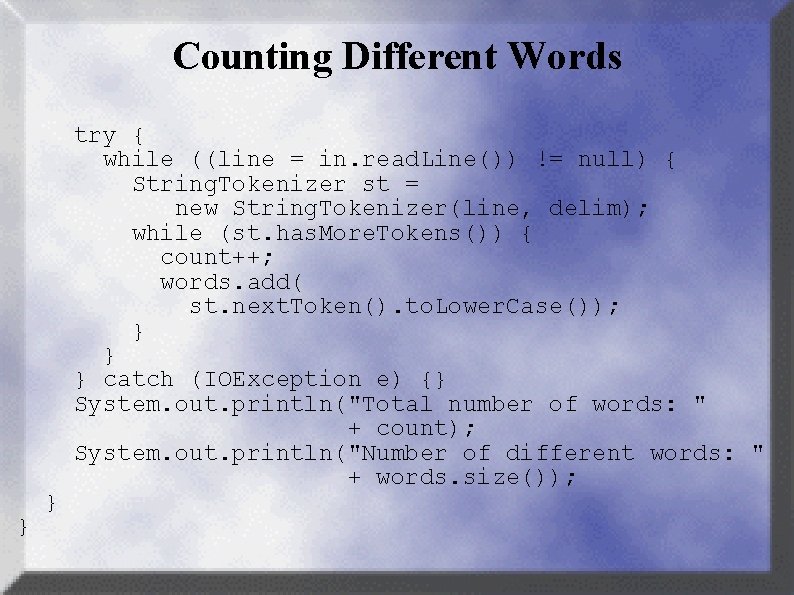
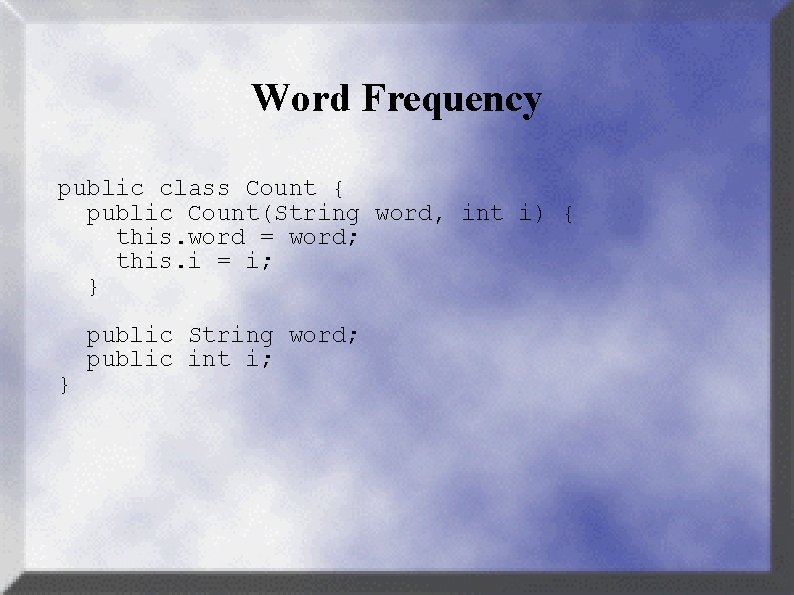
![Word Frequency (cont'd) public class Word. Frequency { static public void main(String[] args) { Word Frequency (cont'd) public class Word. Frequency { static public void main(String[] args) {](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-12.jpg)
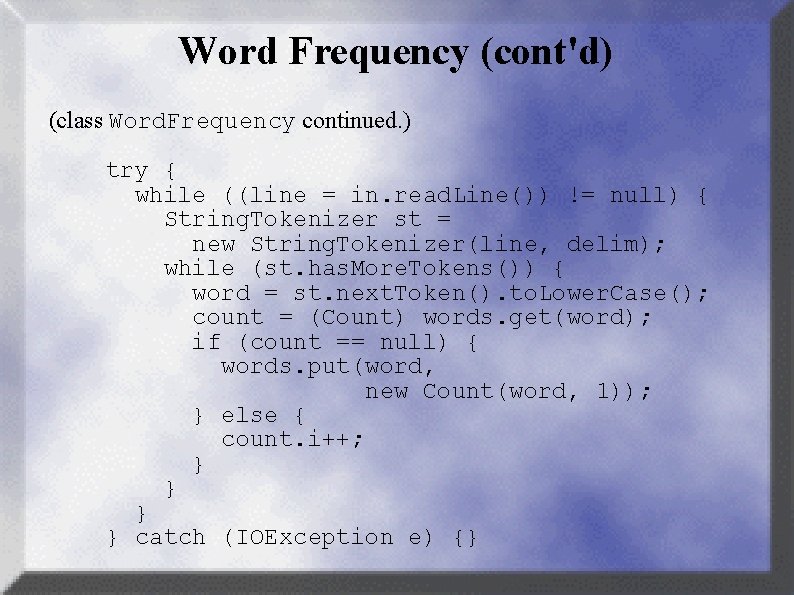
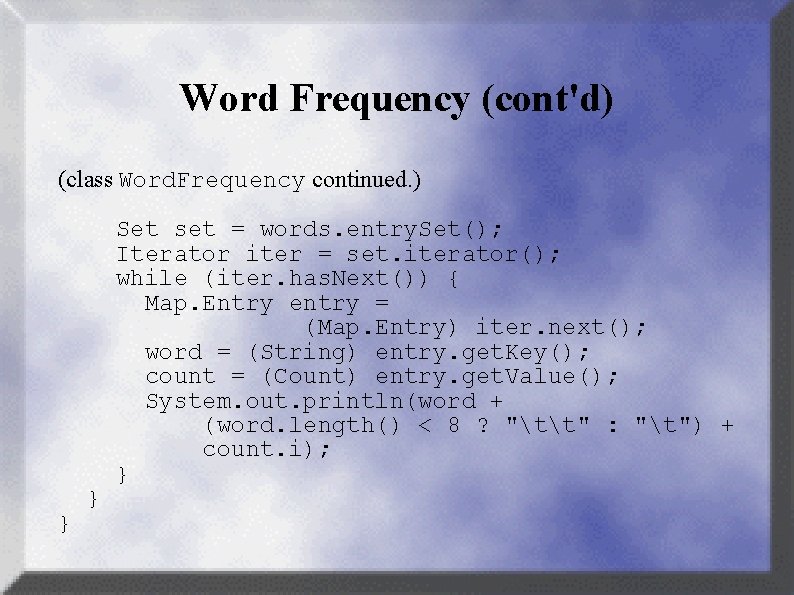
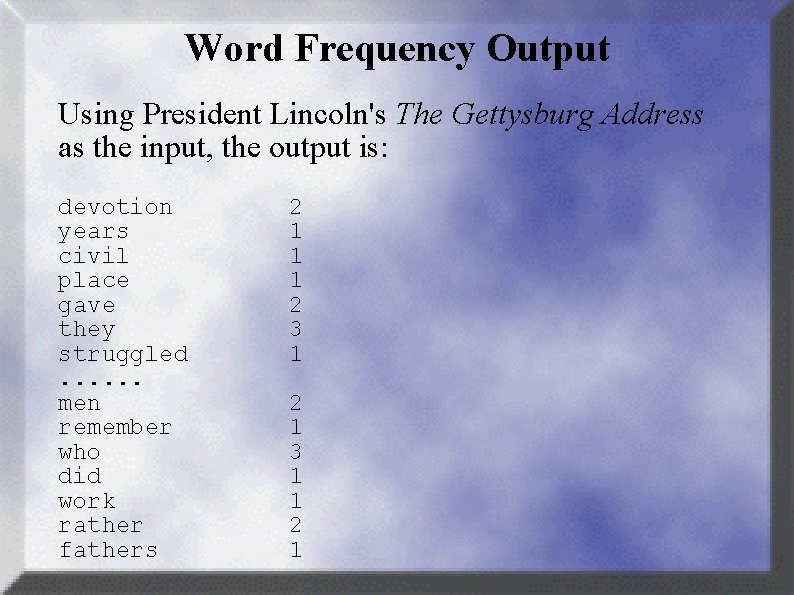
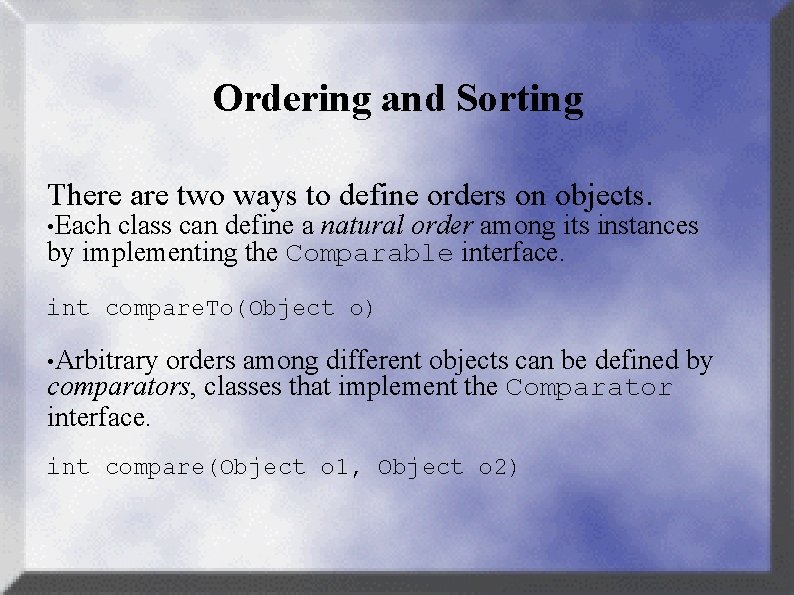
![Word Frequency II public class Word. Frequency 2 { static public void main(String[] args) Word Frequency II public class Word. Frequency 2 { static public void main(String[] args)](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-17.jpg)
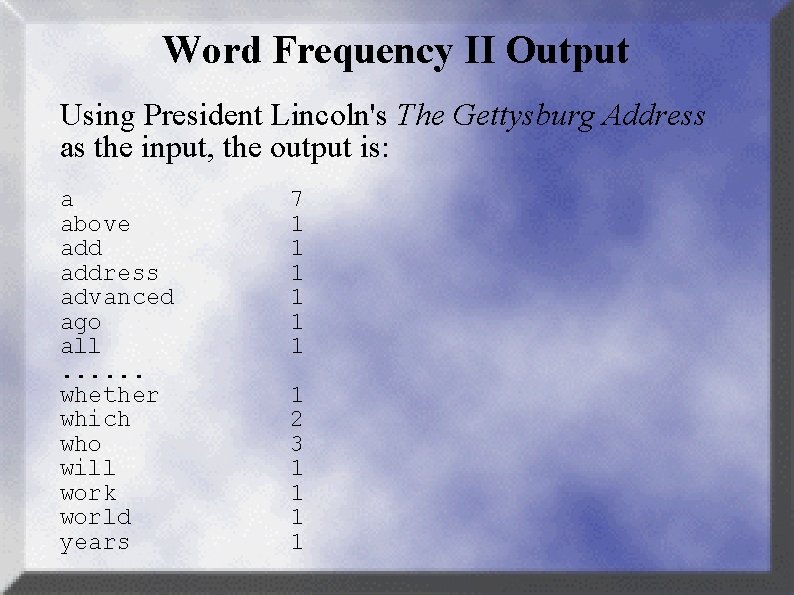
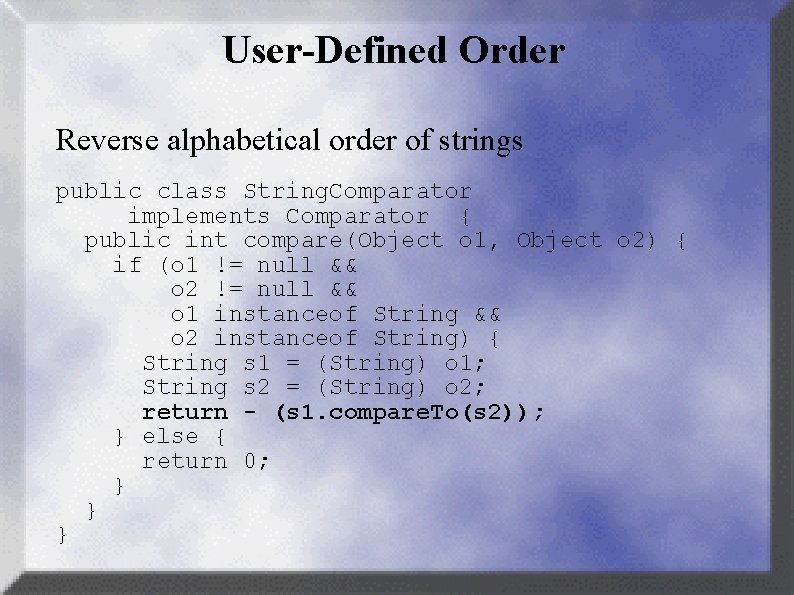
![Word Frequency III public class Word. Frequency 3 { static public void main(String[] args) Word Frequency III public class Word. Frequency 3 { static public void main(String[] args)](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-20.jpg)
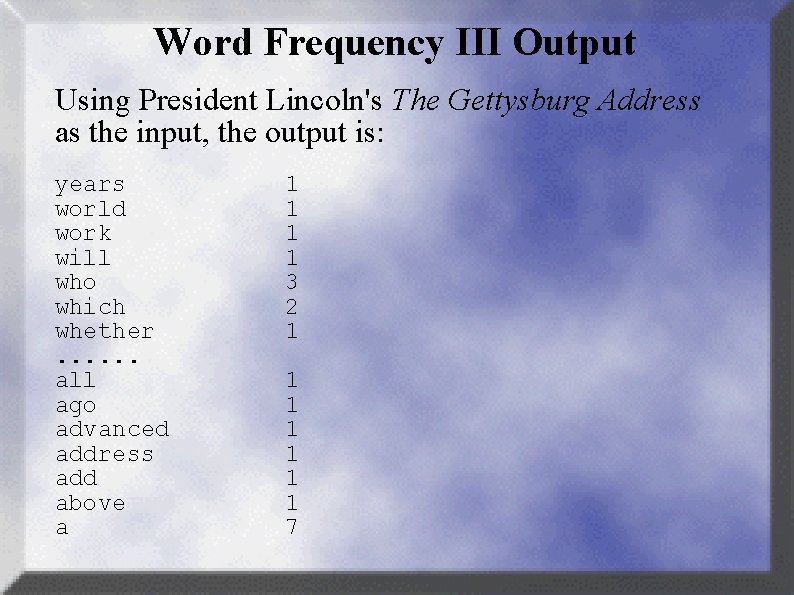
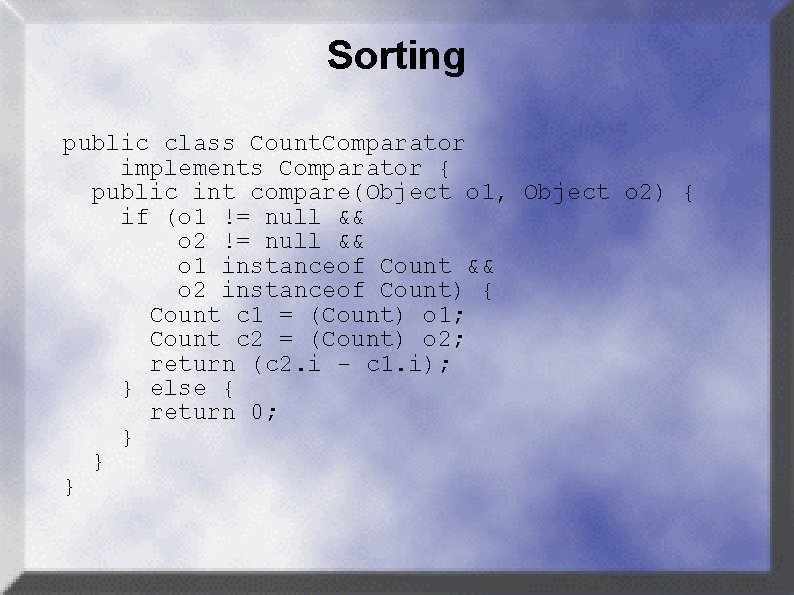
![Word Frequency IV public class Word. Frequency 4 { static public void main(String[] args) Word Frequency IV public class Word. Frequency 4 { static public void main(String[] args)](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-23.jpg)
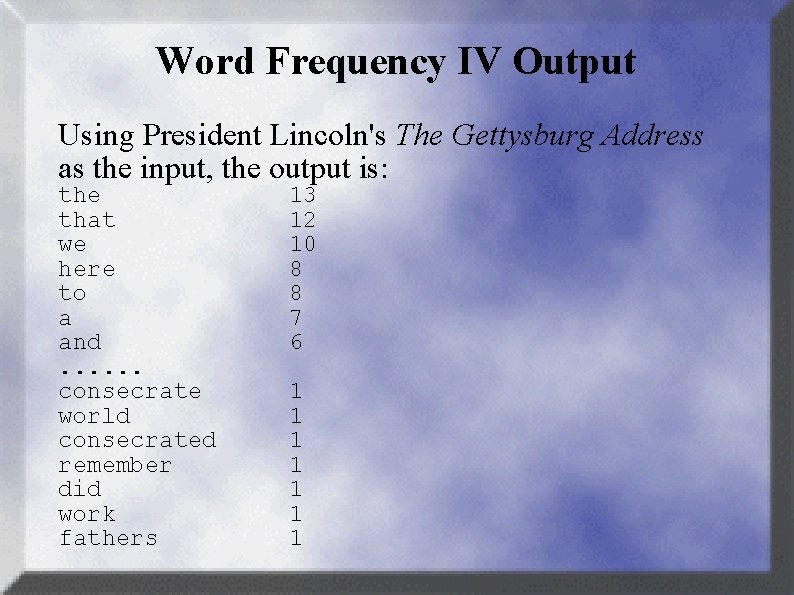
- Slides: 24
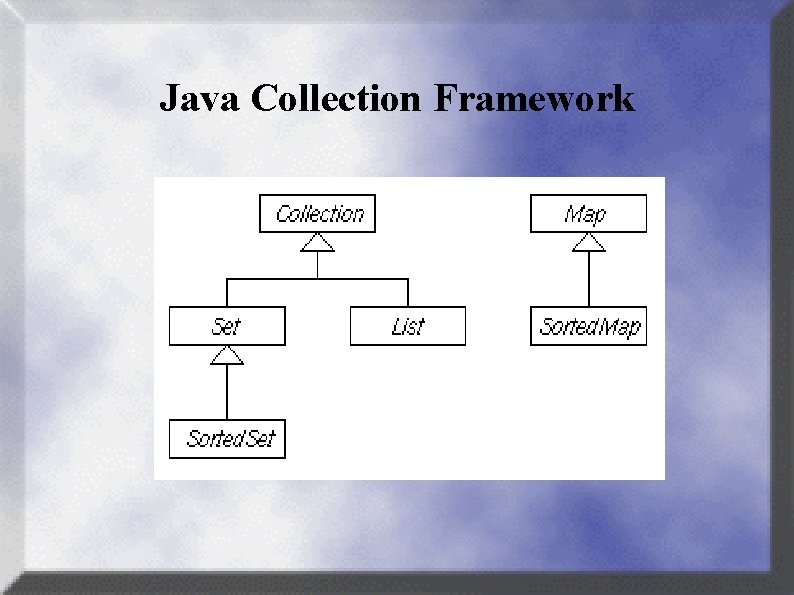
Java Collection Framework
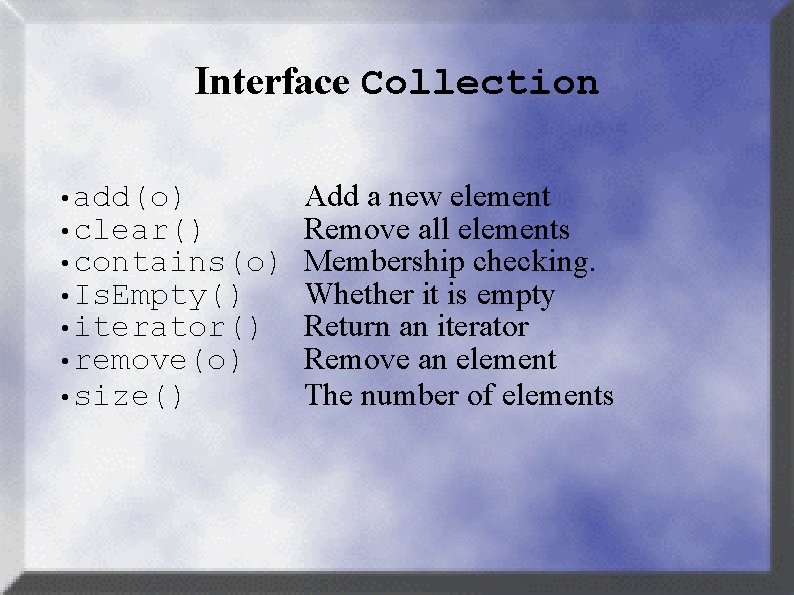
Interface Collection • add(o) • clear() • contains(o) • Is. Empty() • iterator() • remove(o) • size() Add a new element Remove all elements Membership checking. Whether it is empty Return an iterator Remove an element The number of elements
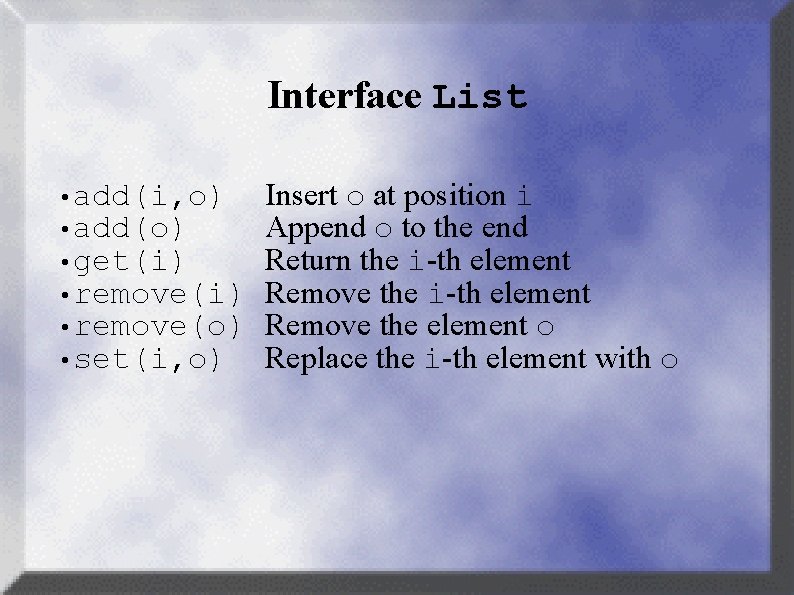
Interface List • add(i, o) • add(o) • get(i) • remove(o) • set(i, o) Insert o at position i Append o to the end Return the i-th element Remove the element o Replace the i-th element with o
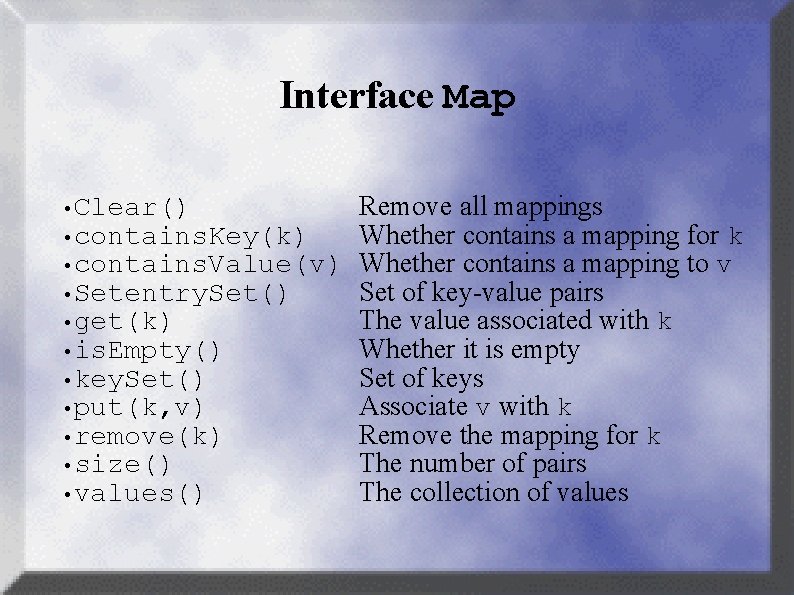
Interface Map • Clear() • contains. Key(k) • contains. Value(v) • Setentry. Set() • get(k) • is. Empty() • key. Set() • put(k, v) • remove(k) • size() • values() Remove all mappings Whether contains a mapping for k Whether contains a mapping to v Set of key-value pairs The value associated with k Whether it is empty Set of keys Associate v with k Remove the mapping for k The number of pairs The collection of values
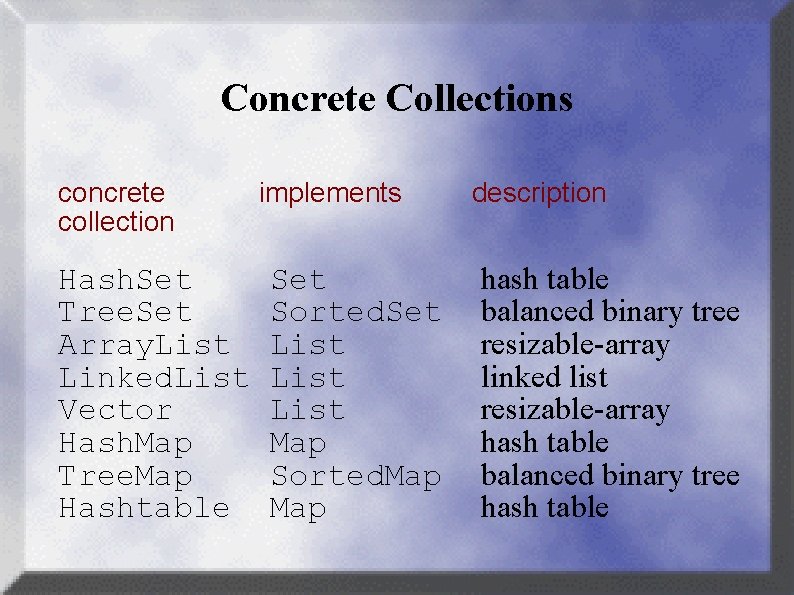
Concrete Collections concrete collection Hash. Set Tree. Set Array. List Linked. List Vector Hash. Map Tree. Map Hashtable implements Set Sorted. Set List Map Sorted. Map description hash table balanced binary tree resizable-array linked list resizable-array hash table balanced binary tree hash table
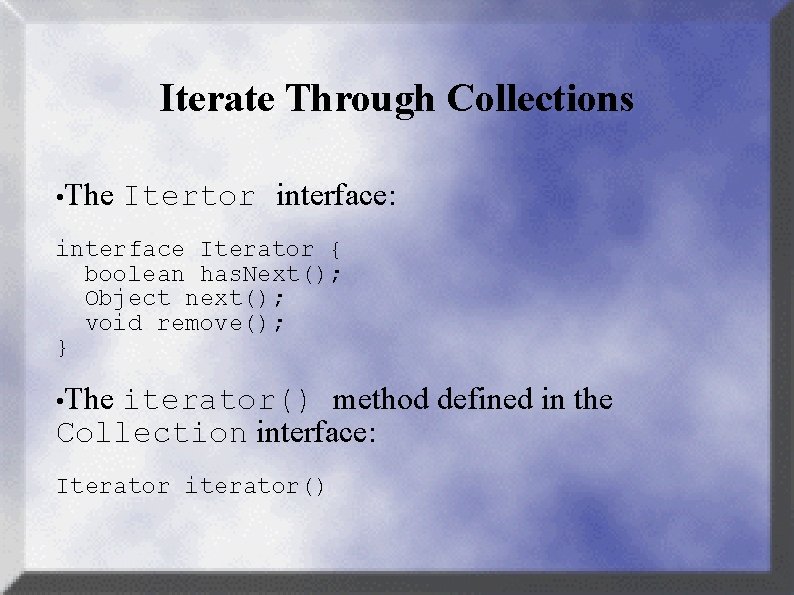
Iterate Through Collections • The Itertor interface: interface Iterator { boolean has. Next(); Object next(); void remove(); } • The iterator() method defined in the Collection interface: Iterator iterator()
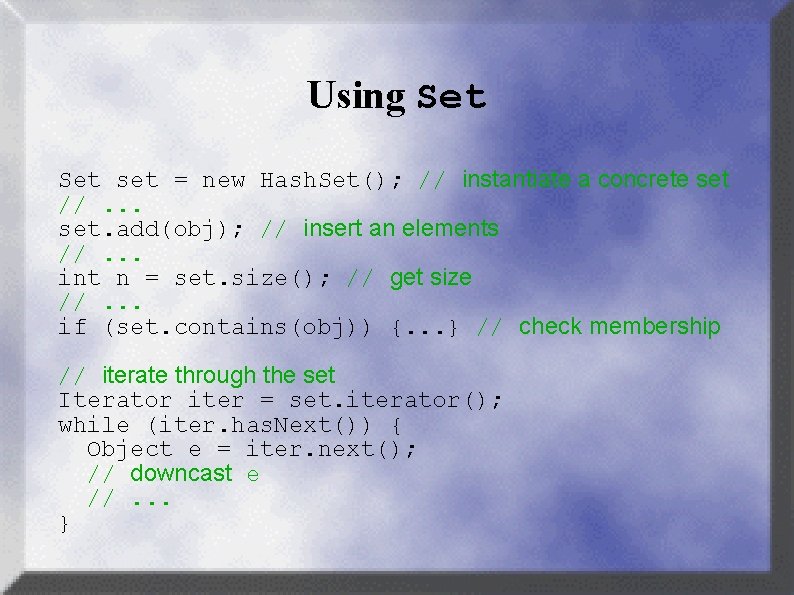
Using Set set = new Hash. Set(); // instantiate a concrete set //. . . set. add(obj); // insert an elements //. . . int n = set. size(); // get size //. . . if (set. contains(obj)) {. . . } // check membership // iterate through the set Iterator iter = set. iterator(); while (iter. has. Next()) { Object e = iter. next(); // downcast e //. . . }
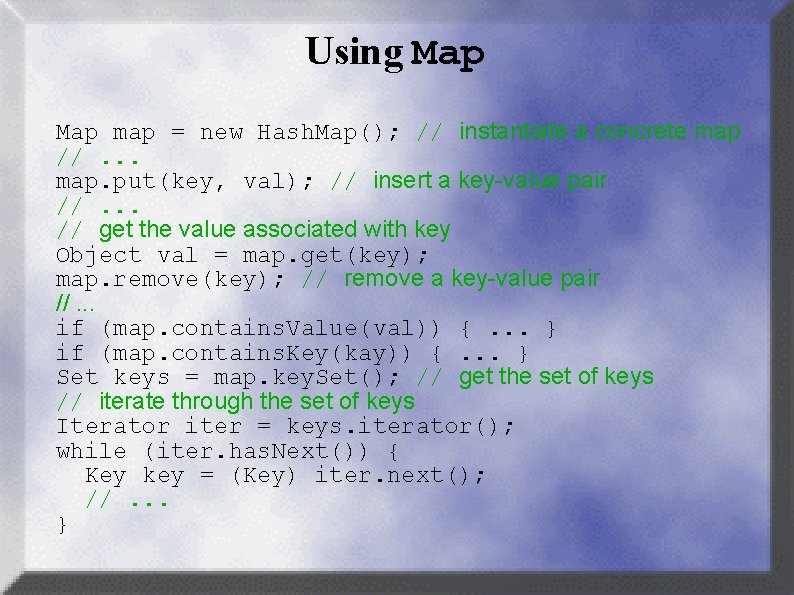
Using Map map = new Hash. Map(); // instantiate a concrete map //. . . map. put(key, val); // insert a key-value pair //. . . // get the value associated with key Object val = map. get(key); map. remove(key); // remove a key-value pair //. . . if (map. contains. Value(val)) {. . . } if (map. contains. Key(kay)) {. . . } Set keys = map. key. Set(); // get the set of keys // iterate through the set of keys Iterator iter = keys. iterator(); while (iter. has. Next()) { Key key = (Key) iter. next(); //. . . }
![Counting Different Words public class Count Words static public void mainString args Counting Different Words public class Count. Words { static public void main(String[] args) {](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-9.jpg)
Counting Different Words public class Count. Words { static public void main(String[] args) { Set words = new Hash. Set(); Buffered. Reader in = new Buffered. Reader( new Input. Stream. Reader(System. in)); String delim = " tn. , : ; ? !-/()[]"'"; String line; int count = 0;
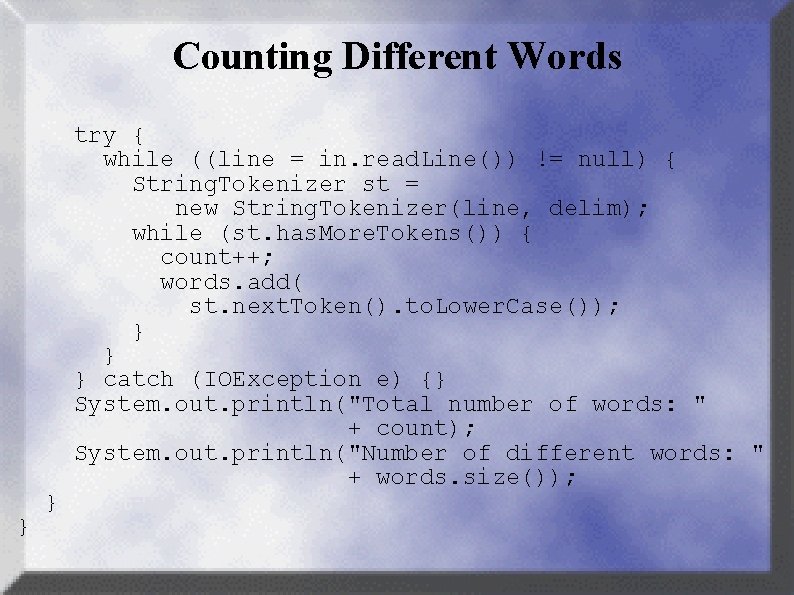
Counting Different Words } } try { while ((line = in. read. Line()) != null) { String. Tokenizer st = new String. Tokenizer(line, delim); while (st. has. More. Tokens()) { count++; words. add( st. next. Token(). to. Lower. Case()); } } } catch (IOException e) {} System. out. println("Total number of words: " + count); System. out. println("Number of different words: " + words. size());
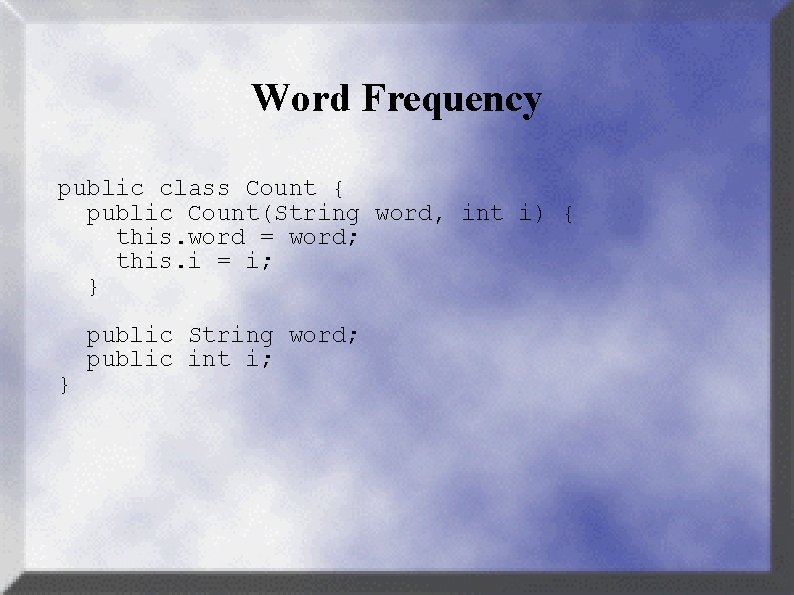
Word Frequency public class Count { public Count(String word, int i) { this. word = word; this. i = i; } } public String word; public int i;
![Word Frequency contd public class Word Frequency static public void mainString args Word Frequency (cont'd) public class Word. Frequency { static public void main(String[] args) {](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-12.jpg)
Word Frequency (cont'd) public class Word. Frequency { static public void main(String[] args) { Map words = new Hash. Map(); String delim = " tn. , : ; ? !-/()[]"'"; Buffered. Reader in = new Buffered. Reader( new Input. Stream. Reader(System. in)); String line, word; Count count;
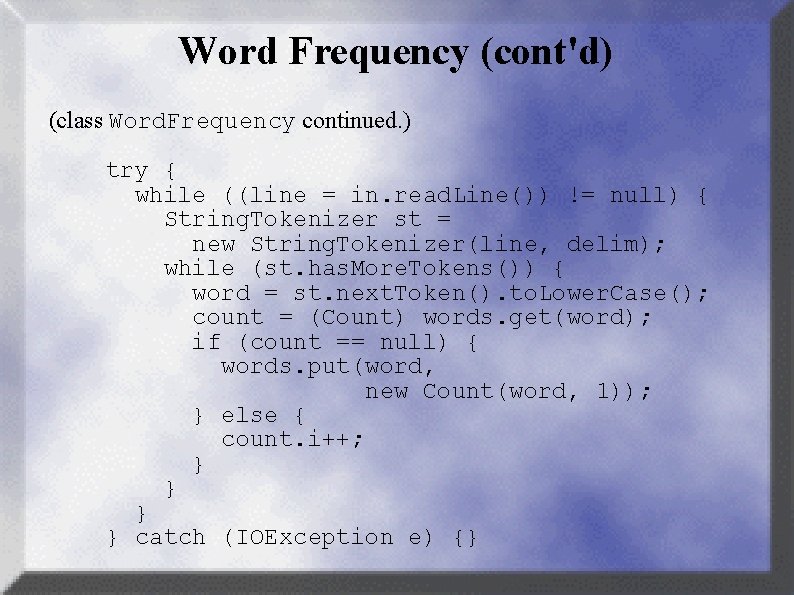
Word Frequency (cont'd) (class Word. Frequency continued. ) try { while ((line = in. read. Line()) != null) { String. Tokenizer st = new String. Tokenizer(line, delim); while (st. has. More. Tokens()) { word = st. next. Token(). to. Lower. Case(); count = (Count) words. get(word); if (count == null) { words. put(word, new Count(word, 1)); } else { count. i++; } } catch (IOException e) {}
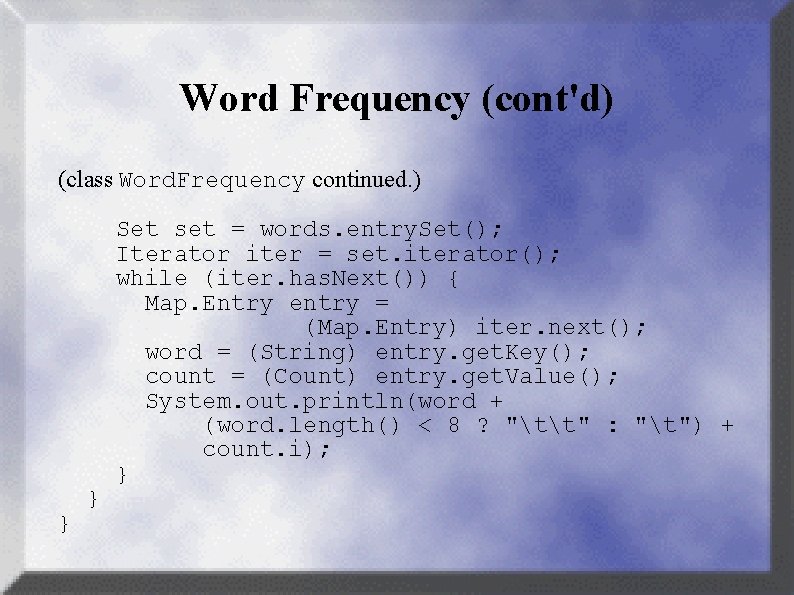
Word Frequency (cont'd) (class Word. Frequency continued. ) } } Set set = words. entry. Set(); Iterator iter = set. iterator(); while (iter. has. Next()) { Map. Entry entry = (Map. Entry) iter. next(); word = (String) entry. get. Key(); count = (Count) entry. get. Value(); System. out. println(word + (word. length() < 8 ? "tt" : "t") + count. i); }
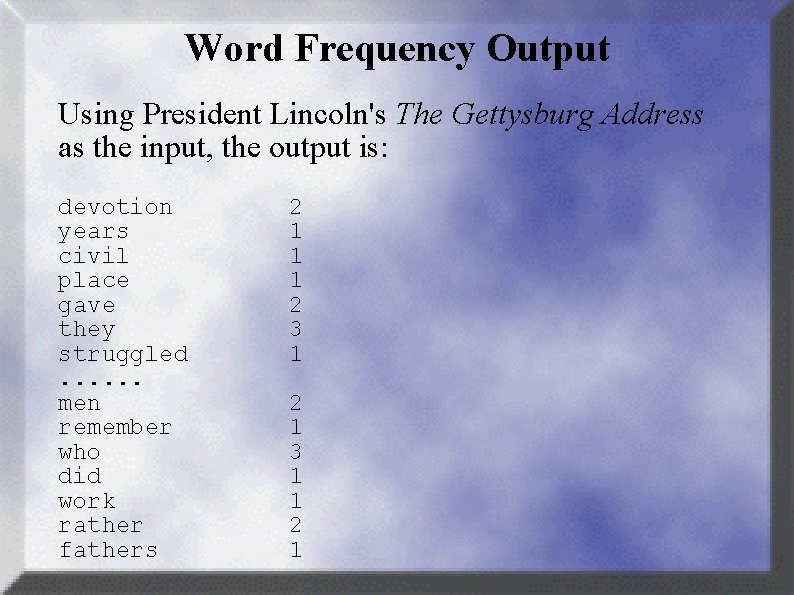
Word Frequency Output Using President Lincoln's The Gettysburg Address as the input, the output is: devotion years civil place gave they struggled. . . men remember who did work rather fathers 2 1 1 1 2 3 1 2 1 3 1 1 2 1
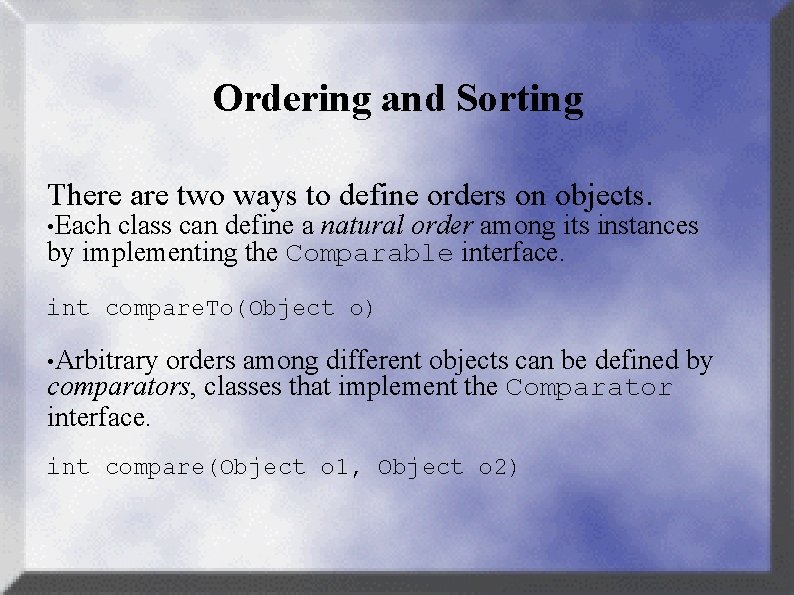
Ordering and Sorting There are two ways to define orders on objects. • Each class can define a natural order among its instances by implementing the Comparable interface. int compare. To(Object o) • Arbitrary orders among different objects can be defined by comparators, classes that implement the Comparator interface. int compare(Object o 1, Object o 2)
![Word Frequency II public class Word Frequency 2 static public void mainString args Word Frequency II public class Word. Frequency 2 { static public void main(String[] args)](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-17.jpg)
Word Frequency II public class Word. Frequency 2 { static public void main(String[] args) { Map words = new Tree. Map(); <smae as Word. Frequency> } }
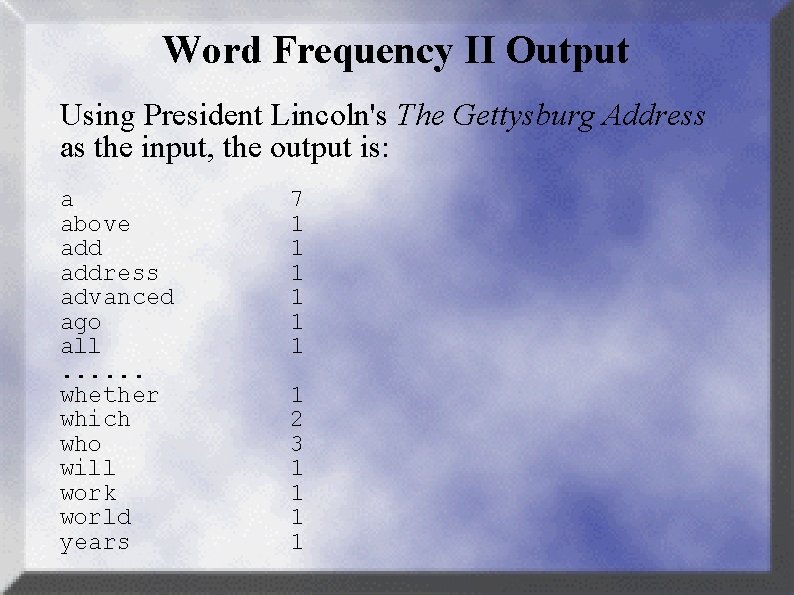
Word Frequency II Output Using President Lincoln's The Gettysburg Address as the input, the output is: a above address advanced ago all. . . whether which who will work world years 7 1 1 1 1 2 3 1 1
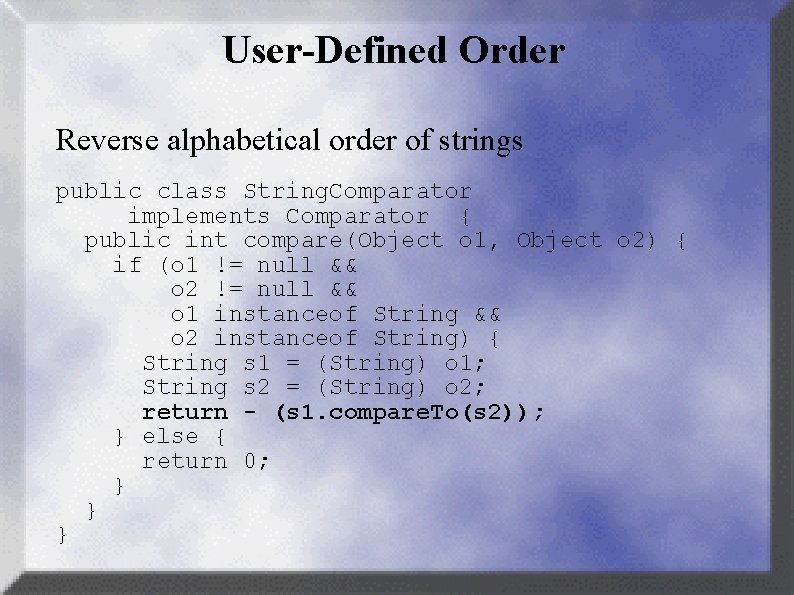
User-Defined Order Reverse alphabetical order of strings public class String. Comparator implements Comparator { public int compare(Object o 1, Object o 2) { if (o 1 != null && o 2 != null && o 1 instanceof String && o 2 instanceof String) { String s 1 = (String) o 1; String s 2 = (String) o 2; return - (s 1. compare. To(s 2)); } else { return 0; } } }
![Word Frequency III public class Word Frequency 3 static public void mainString args Word Frequency III public class Word. Frequency 3 { static public void main(String[] args)](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-20.jpg)
Word Frequency III public class Word. Frequency 3 { static public void main(String[] args) { Map words = new Tree. Map(new String. Comparator()); <smae as Word. Frequency> } }
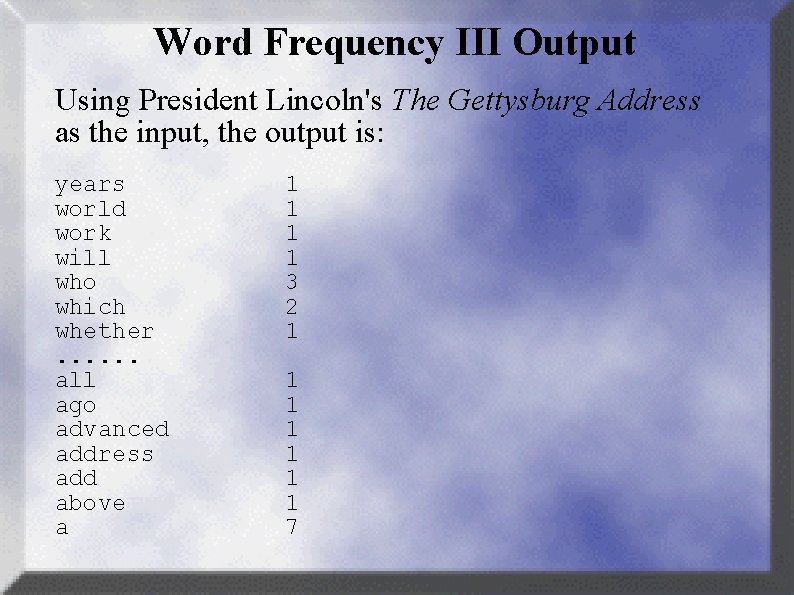
Word Frequency III Output Using President Lincoln's The Gettysburg Address as the input, the output is: years world work will who which whether. . . all ago advanced address add above a 1 1 3 2 1 1 1 1 7
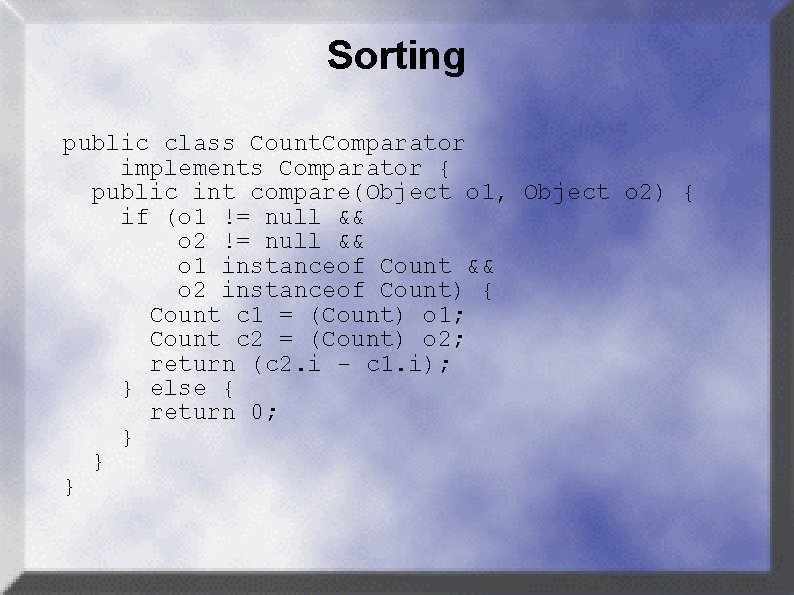
Sorting public class Count. Comparator implements Comparator { public int compare(Object o 1, Object o 2) { if (o 1 != null && o 2 != null && o 1 instanceof Count && o 2 instanceof Count) { Count c 1 = (Count) o 1; Count c 2 = (Count) o 2; return (c 2. i - c 1. i); } else { return 0; } } }
![Word Frequency IV public class Word Frequency 4 static public void mainString args Word Frequency IV public class Word. Frequency 4 { static public void main(String[] args)](https://slidetodoc.com/presentation_image_h/c94d9039a6ec032165a4b7b4d13e1b1c/image-23.jpg)
Word Frequency IV public class Word. Frequency 4 { static public void main(String[] args) { <smae as Word. Frequency> List list = new Array. List(words. values()); Collections. sort(list, new Count. Comparator()); Iterator iter = list. iterator(); while (iter. has. Next()) { count = (Count) iter. next(); word = count. word; System. out. println(word + (word. length() < 8 ? "tt" : "t") + count. i); } } }
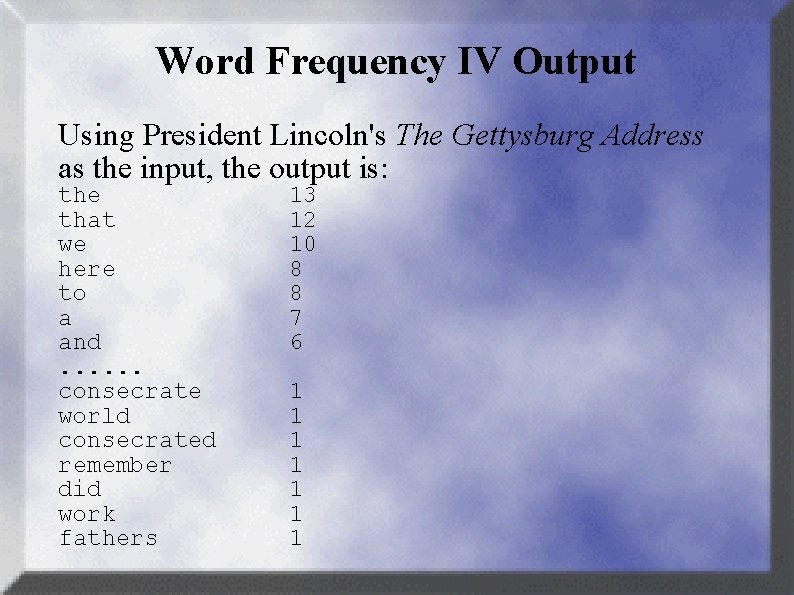
Word Frequency IV Output Using President Lincoln's The Gettysburg Address as the input, the output is: the that we here to a and. . . consecrate world consecrated remember did work fathers 13 12 10 8 8 7 6 1 1 1 1
Interface in java
List ya dawa za dldm
Addo course in tanzania
Dr addo quaye
Office interface vs industrial interface
Office interface vs industrial interface
Interface------------ an interface *
Java extend interface
Public interface java
Interface graphique java
Java uml interface
Java graphical user interface
Collections.iterable
Jndi name
Java interface polymorphism
Interface graphique java eclipse
профайлер java
Java comparable interface
Interfaces are syntactically similar to
Ift 1025
Inheritance
Java measurable interface
What is difference between abstract class and interface
Landsat collection 1 vs collection 2
Documentary payment