Unit4 The collection framework A Collection represents a
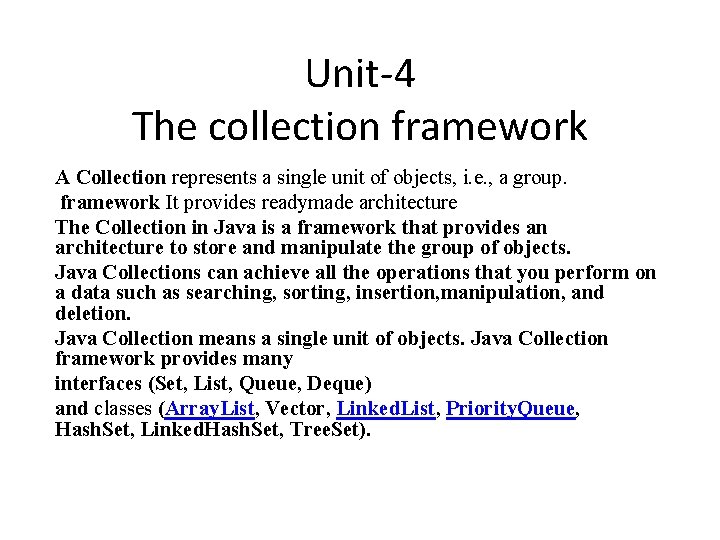
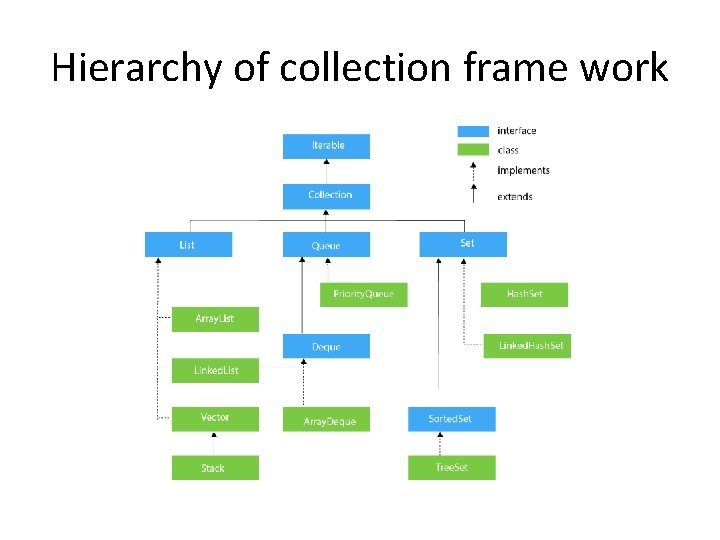
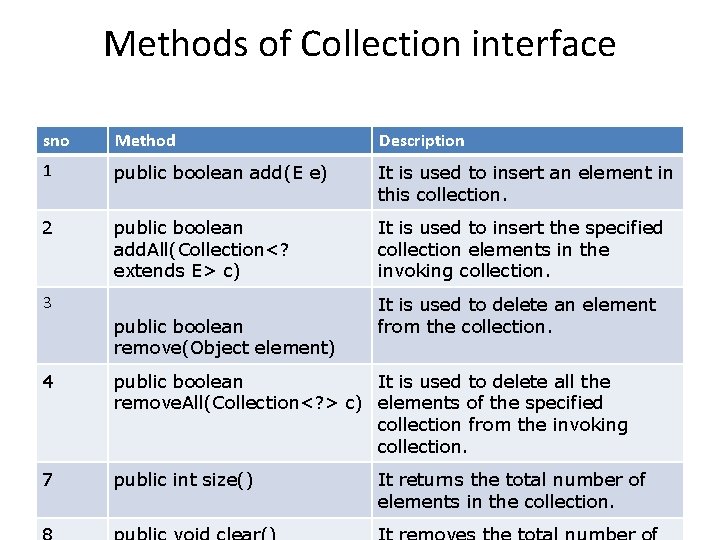
![9 public Iterator iterator() It returns an iterator. 10 public Object[] to. Array() It 9 public Iterator iterator() It returns an iterator. 10 public Object[] to. Array() It](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-4.jpg)
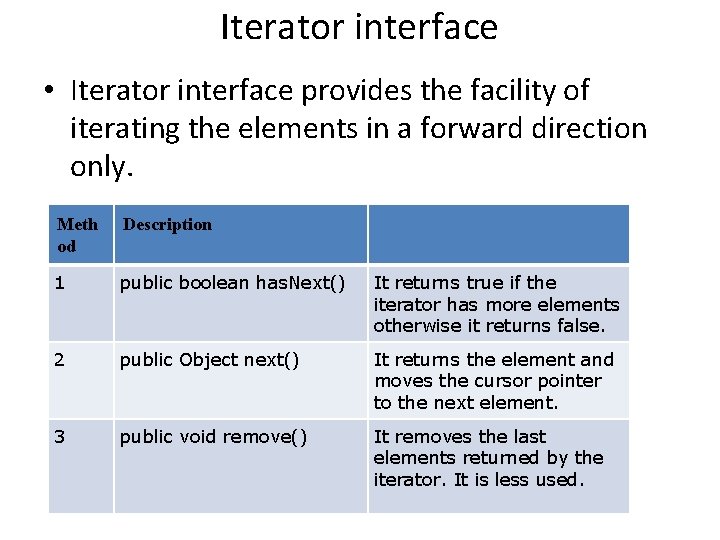
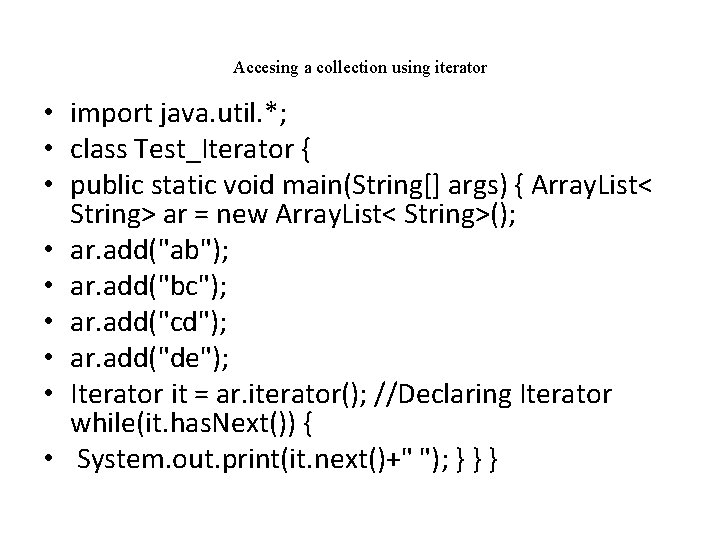
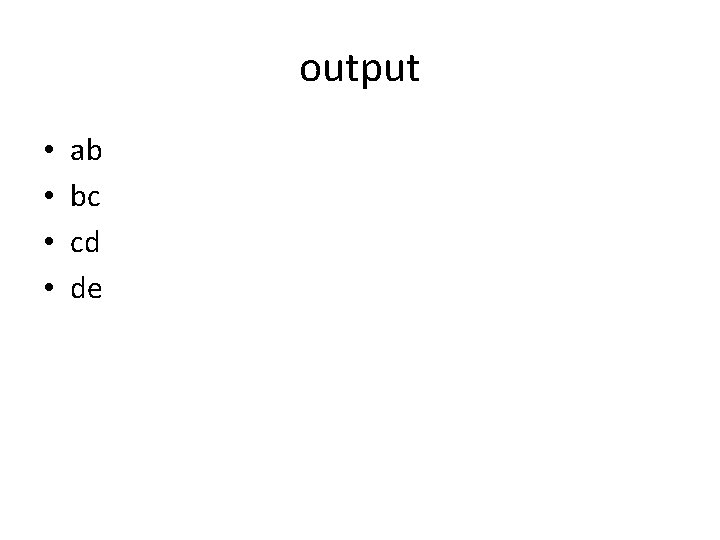
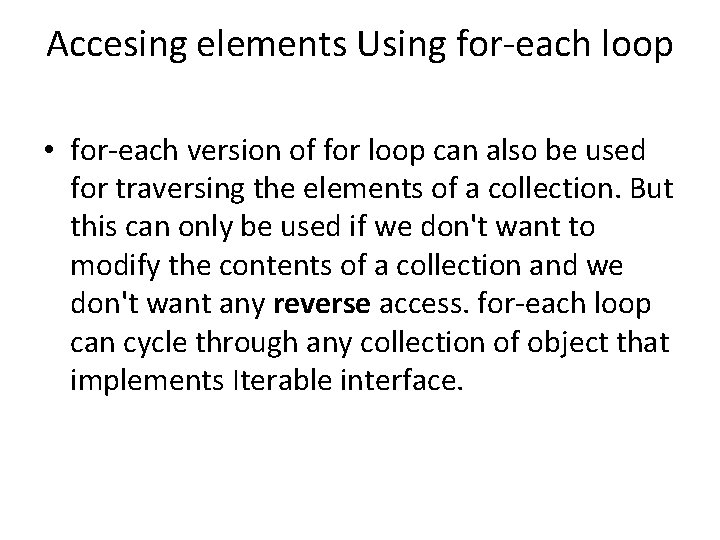
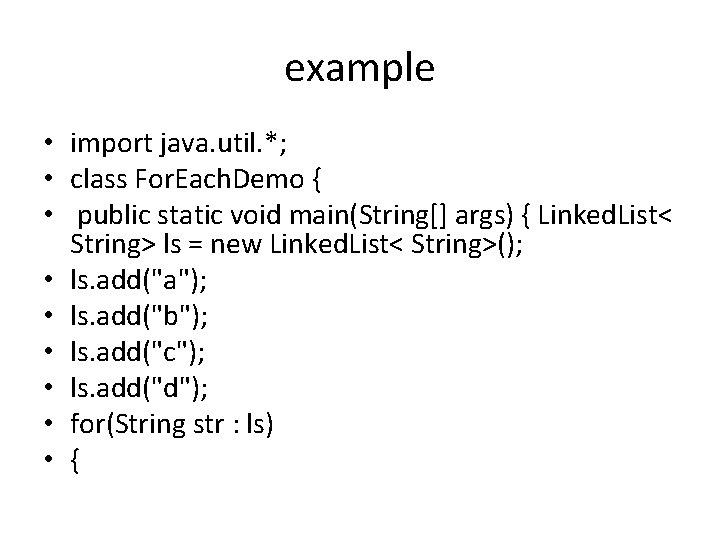
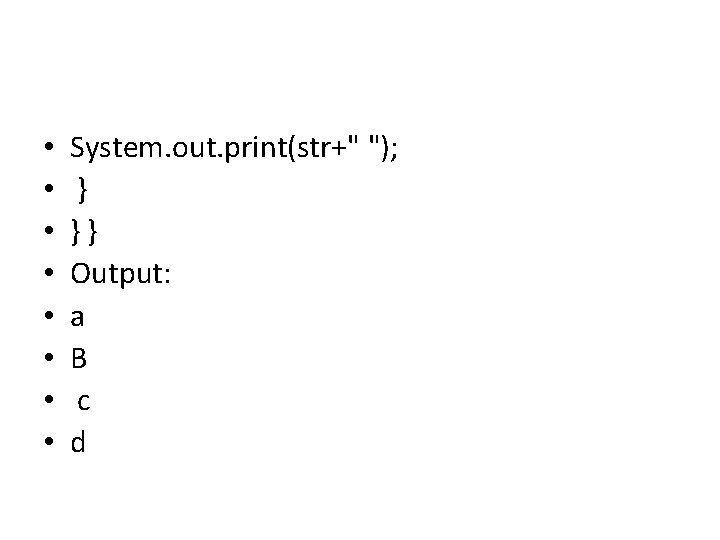
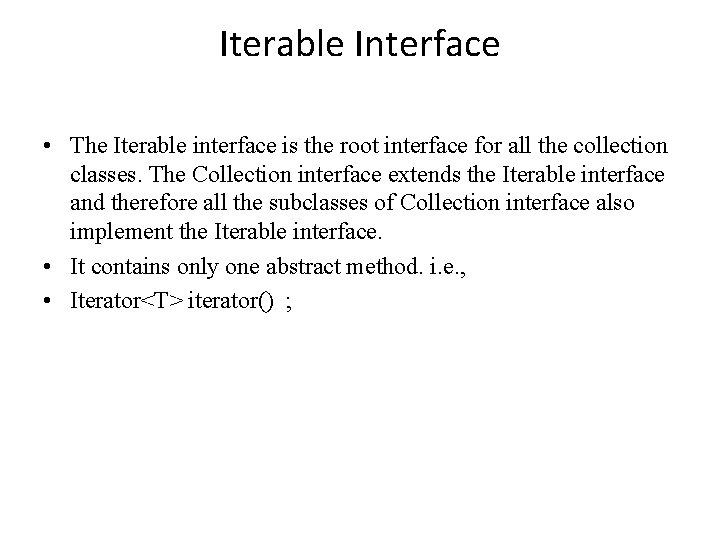
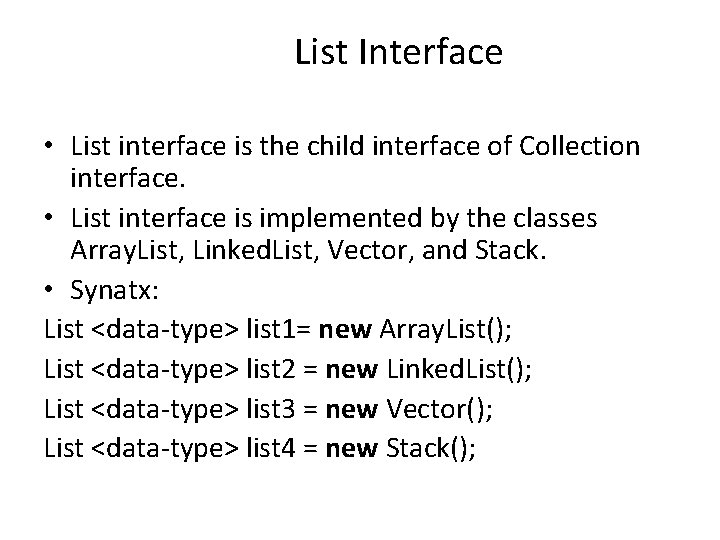
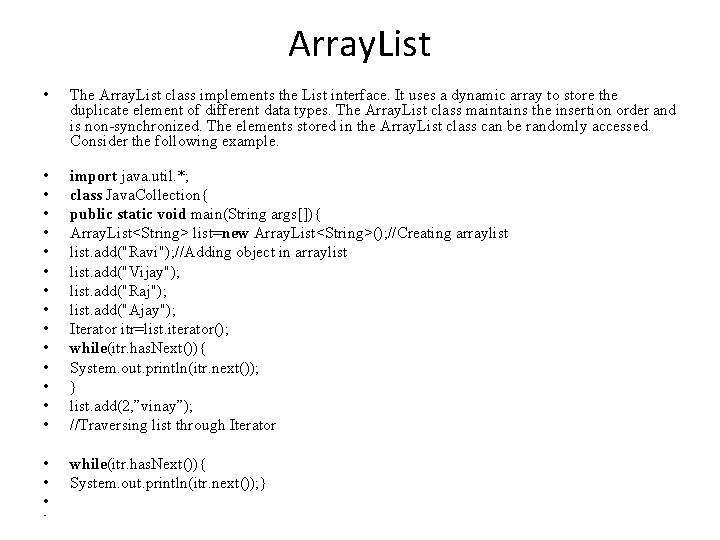
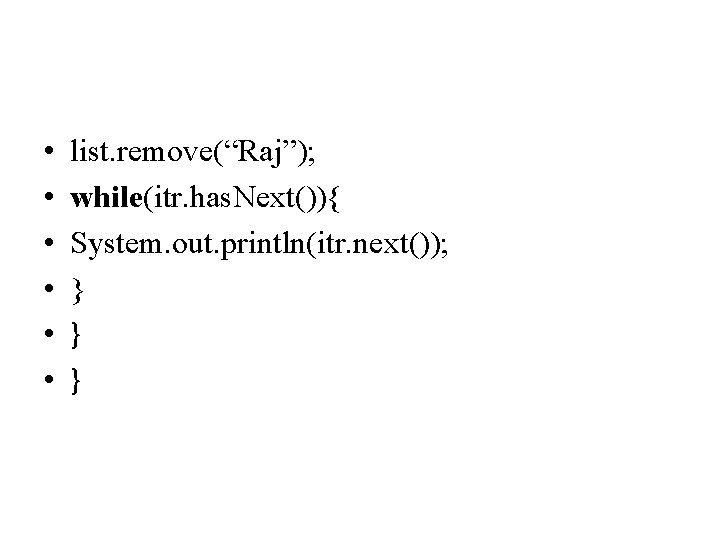
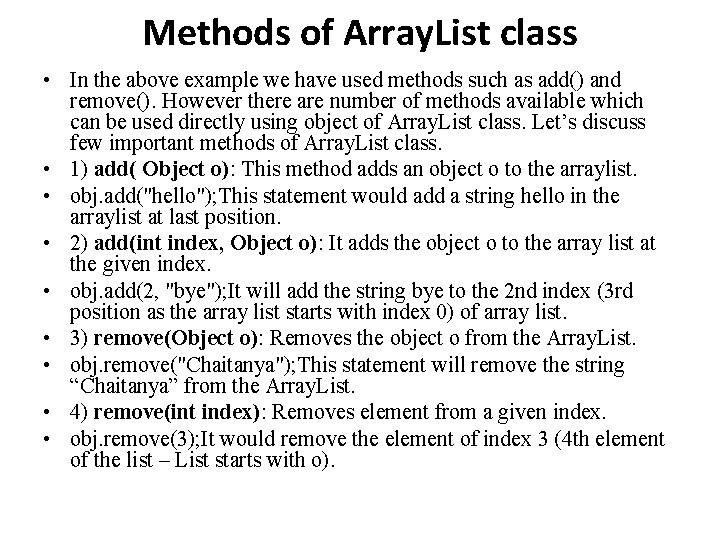
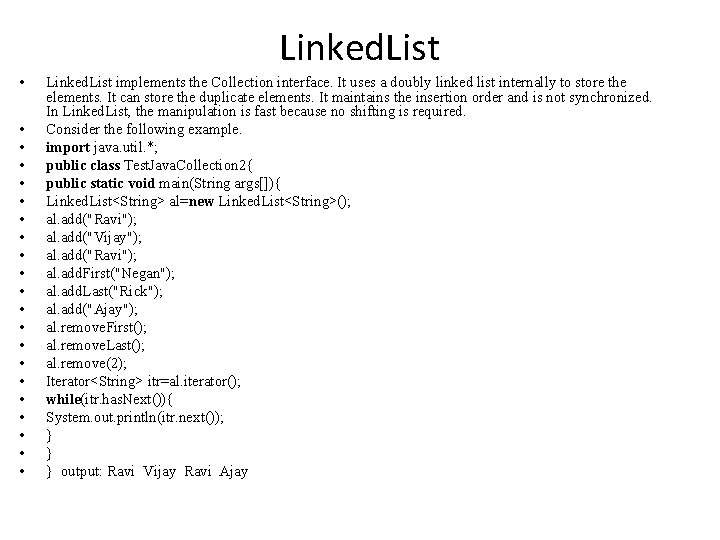
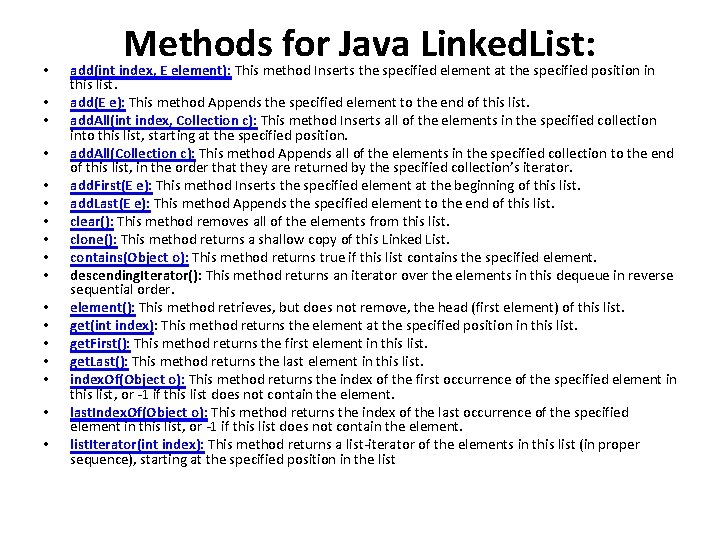
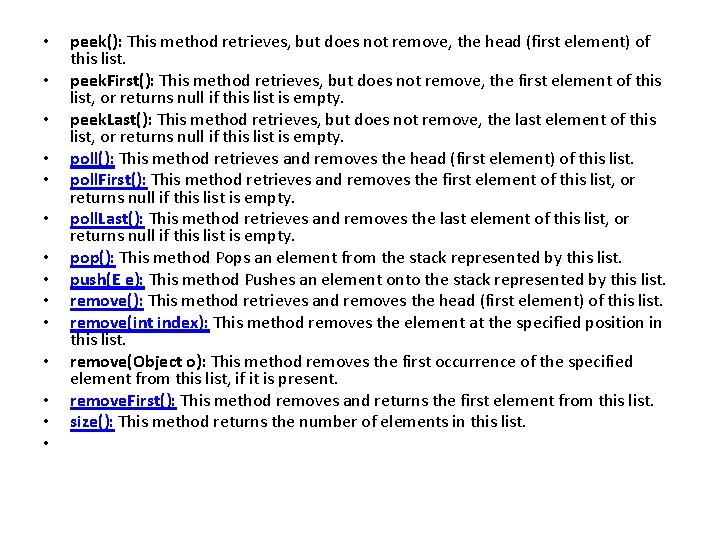
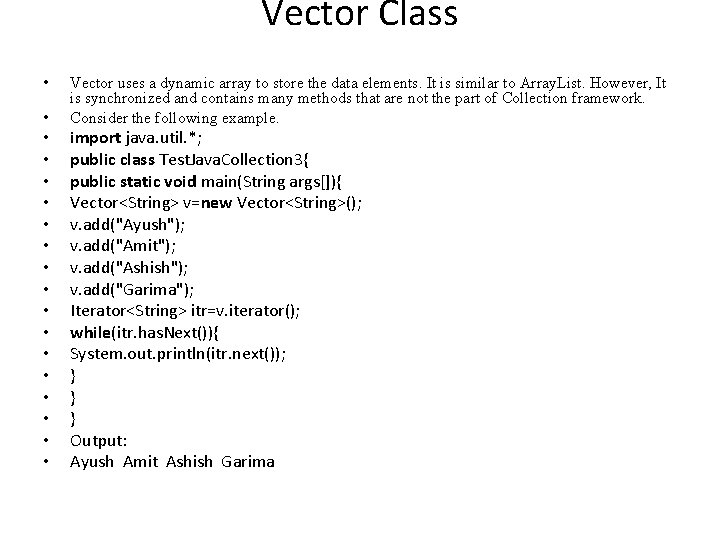
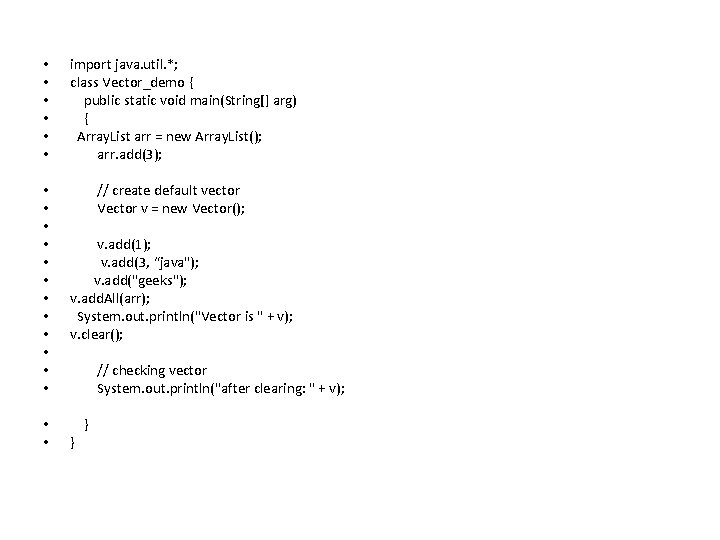
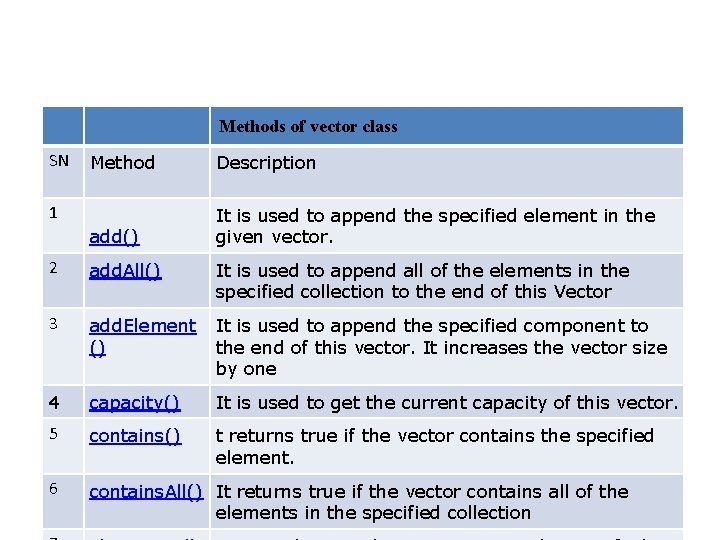
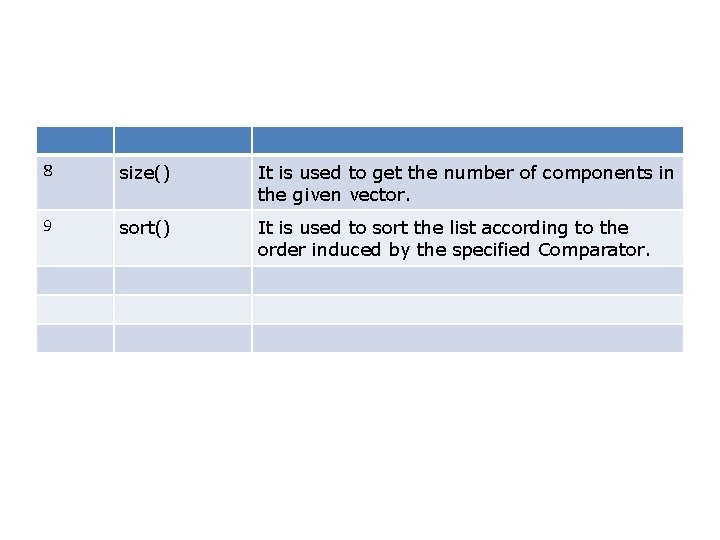
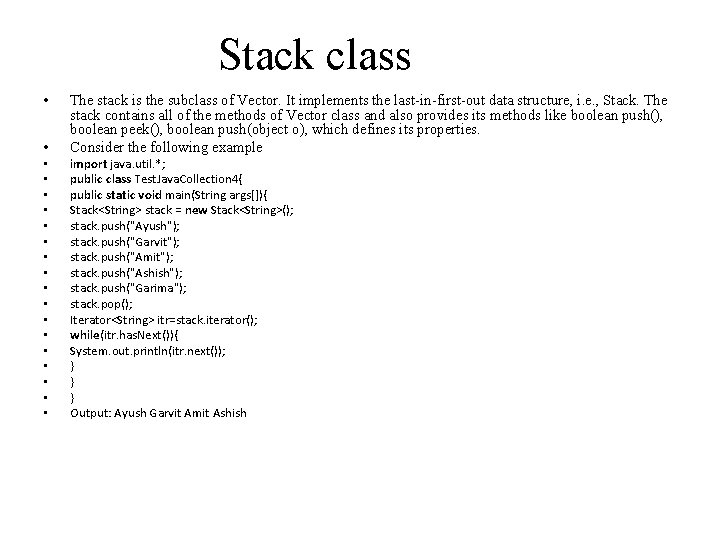
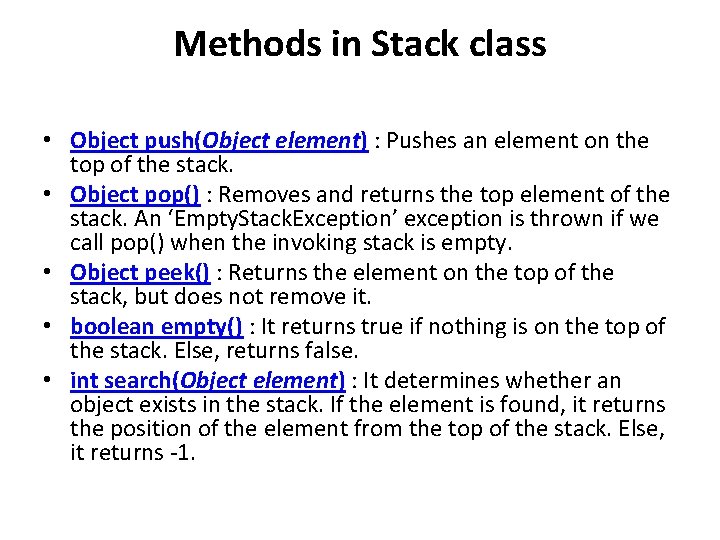
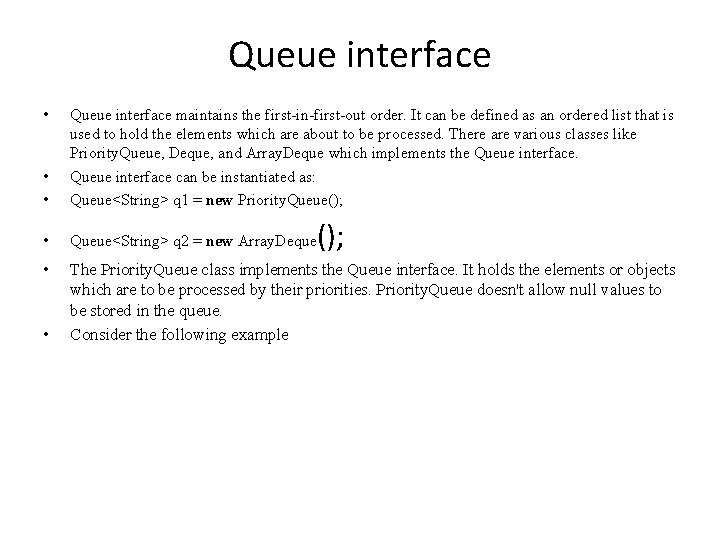
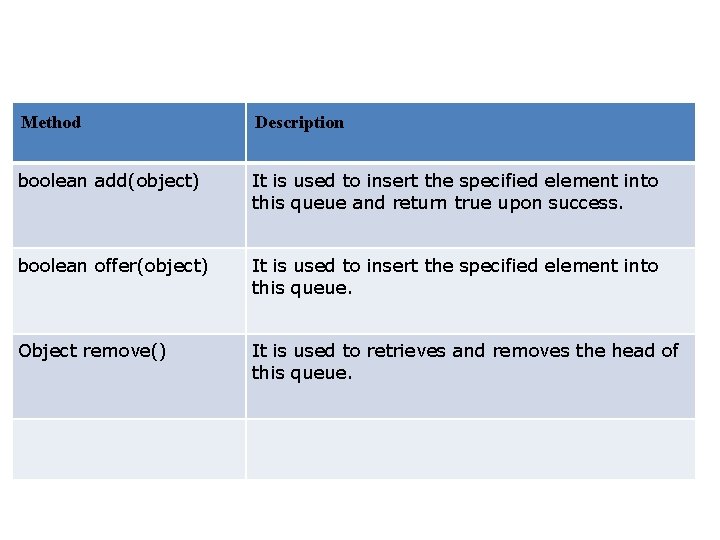
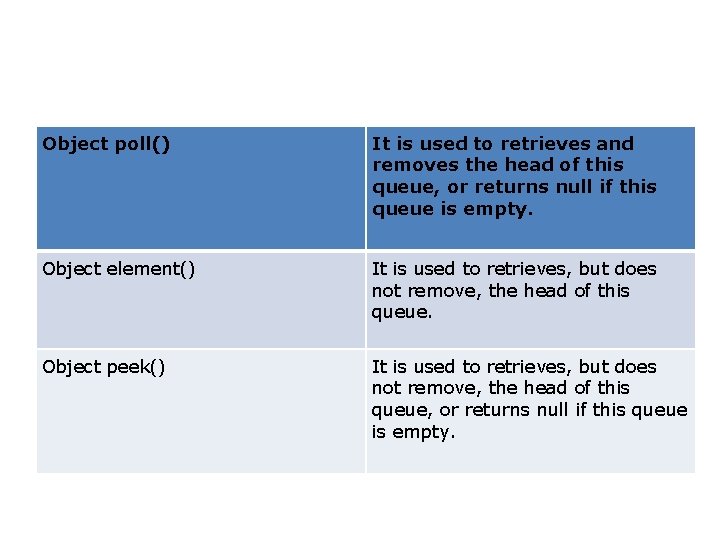
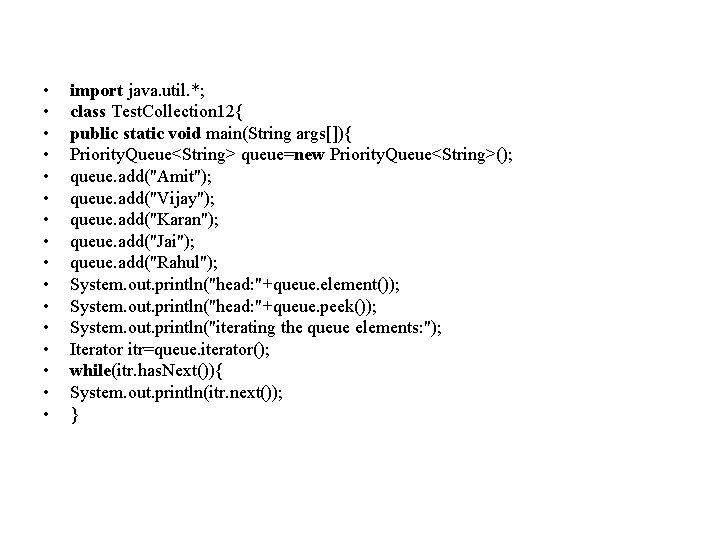
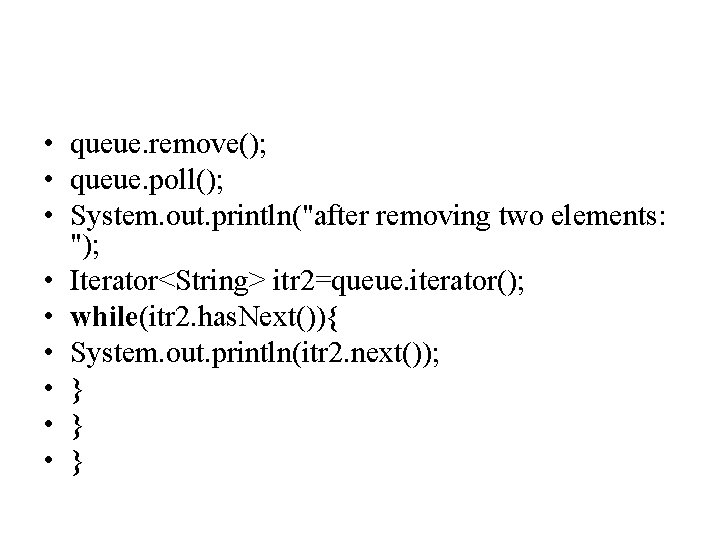
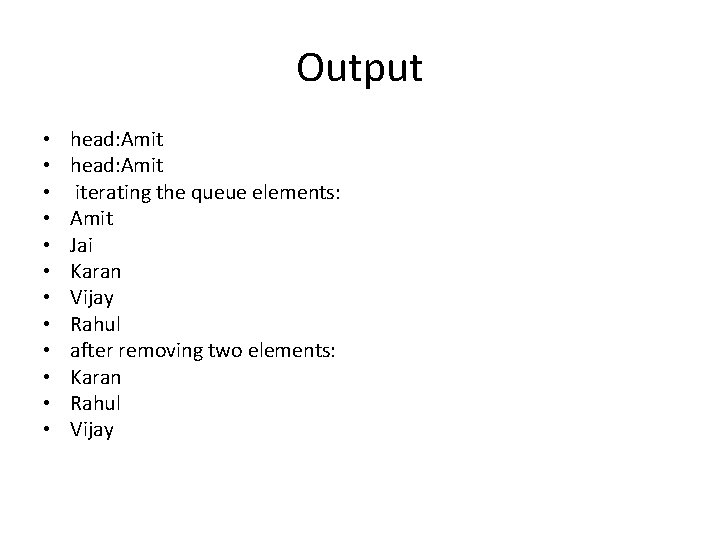
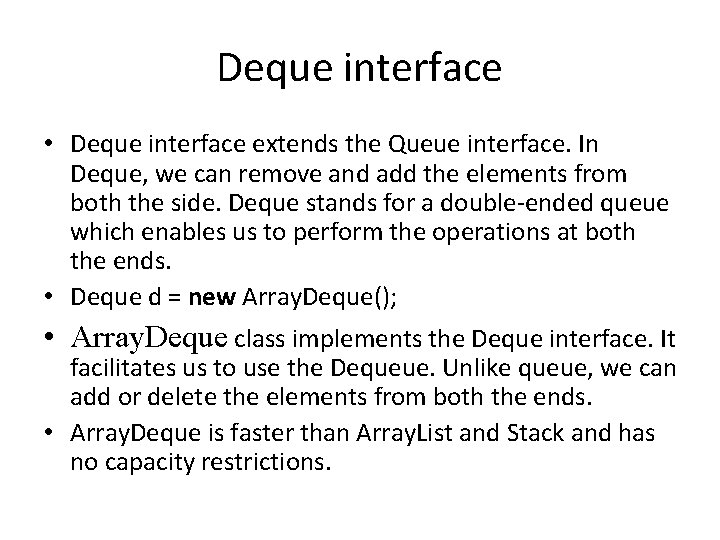
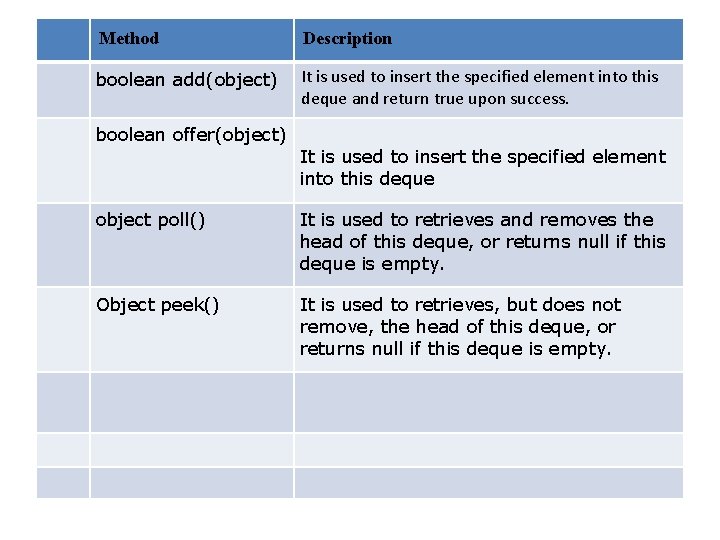
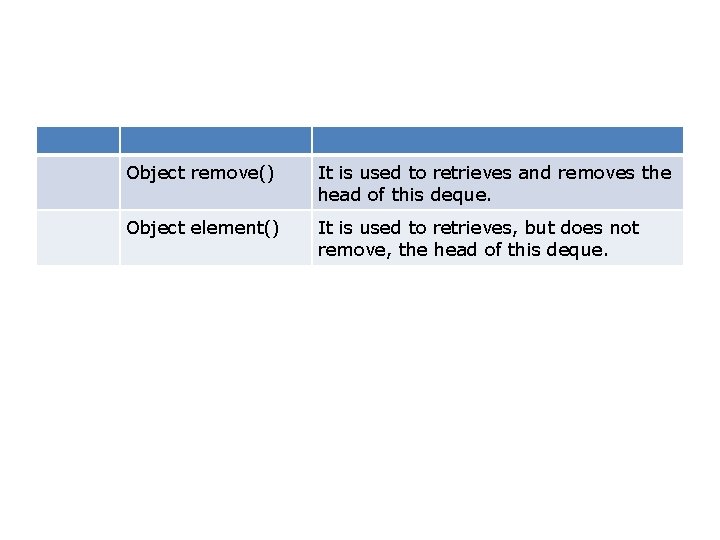
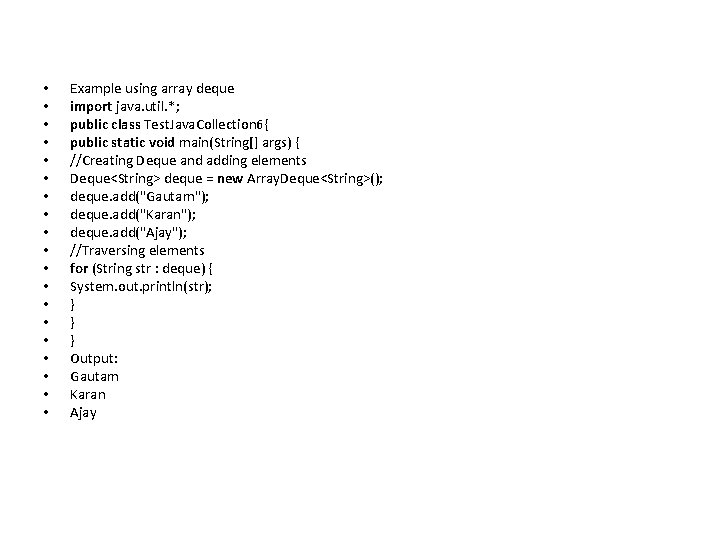
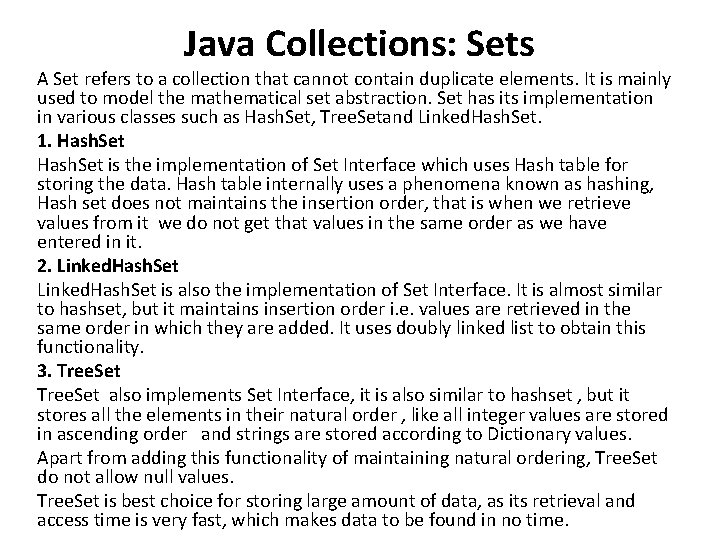
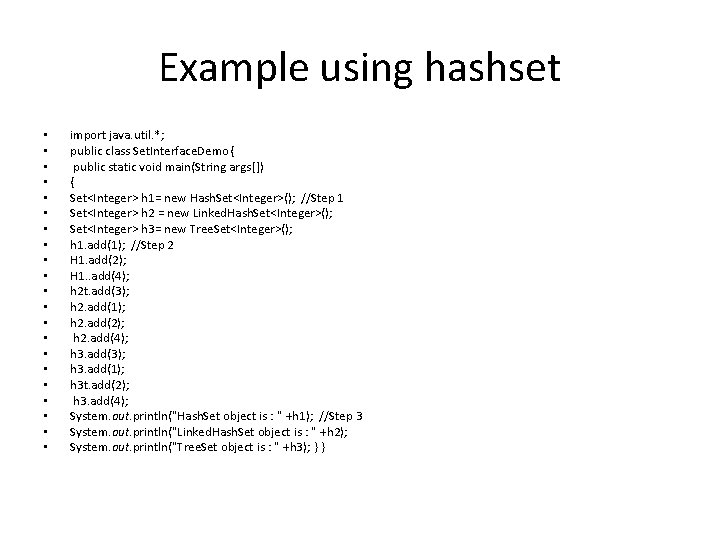
![Output: • Hash. Set object is : [1, 2, 4] • Linked. Hash. Set Output: • Hash. Set object is : [1, 2, 4] • Linked. Hash. Set](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-37.jpg)
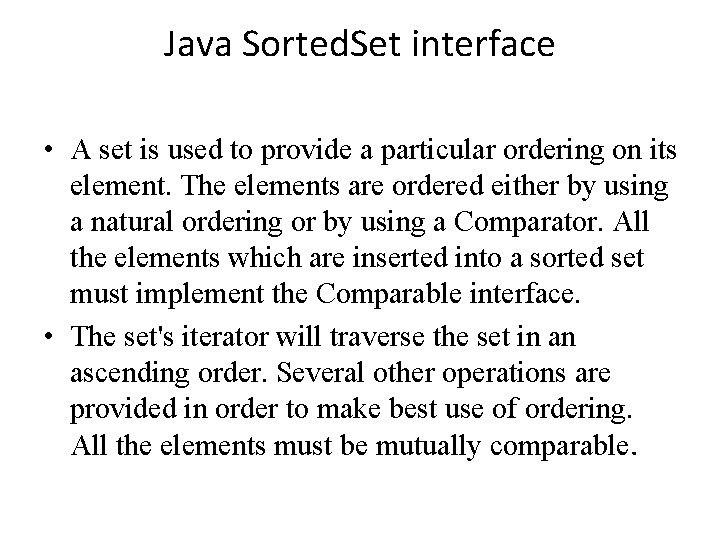
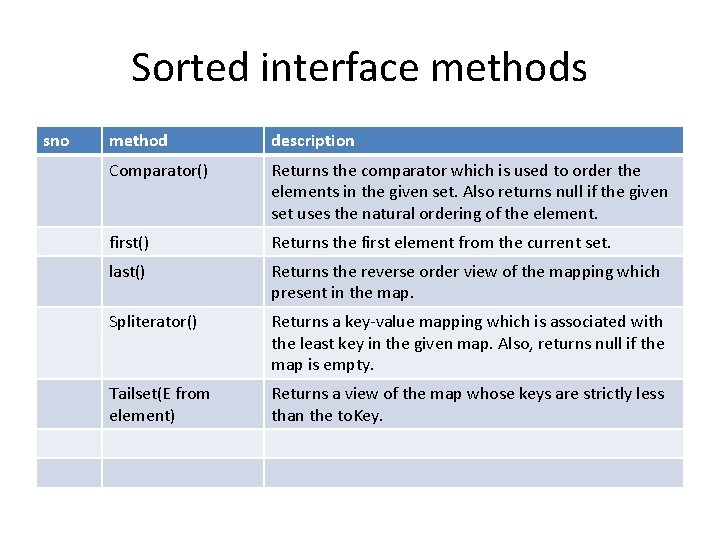
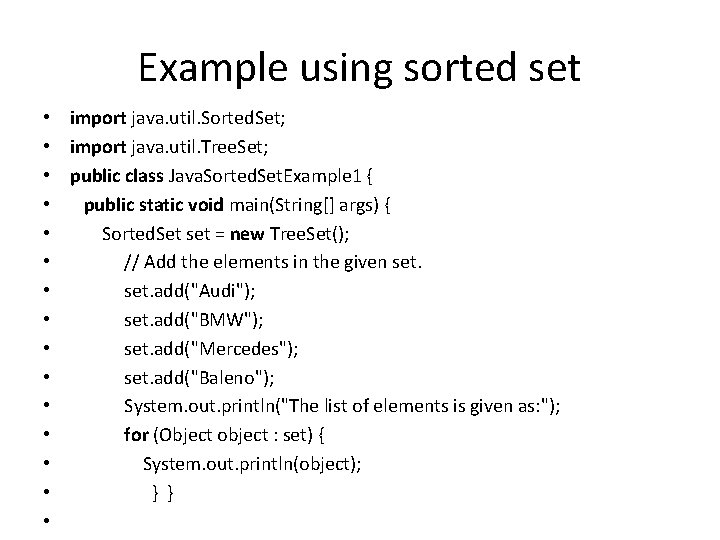
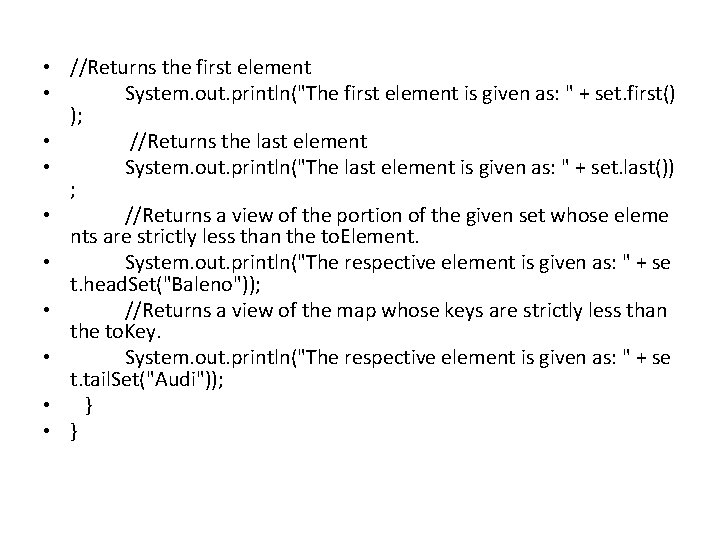
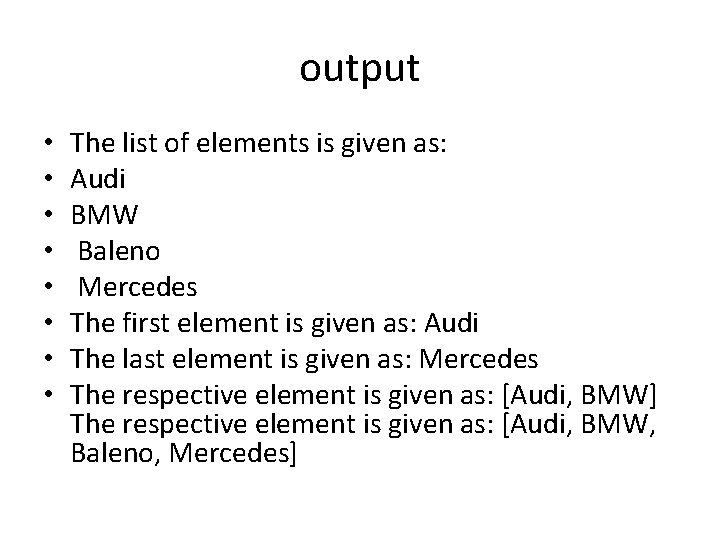
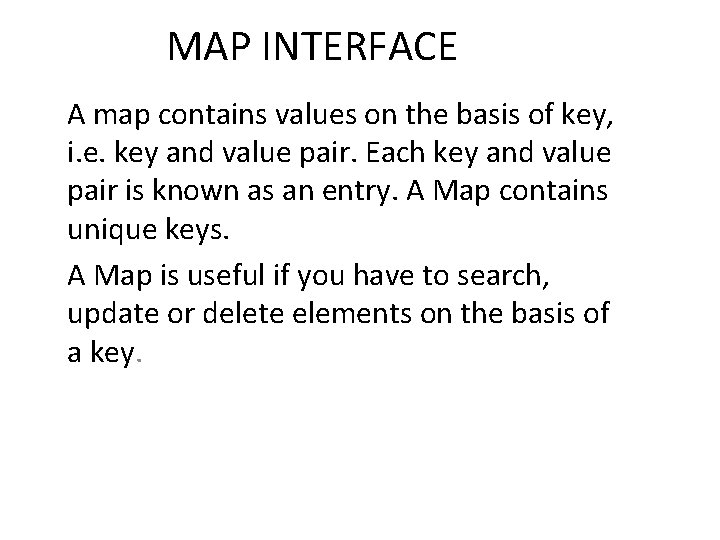
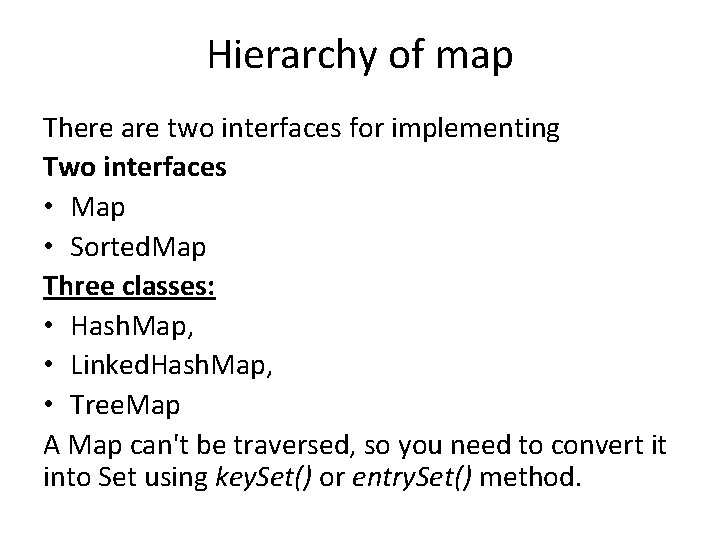
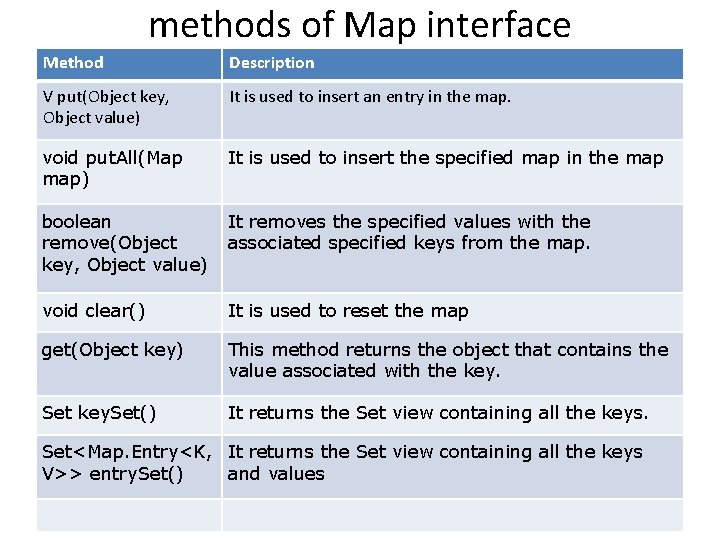
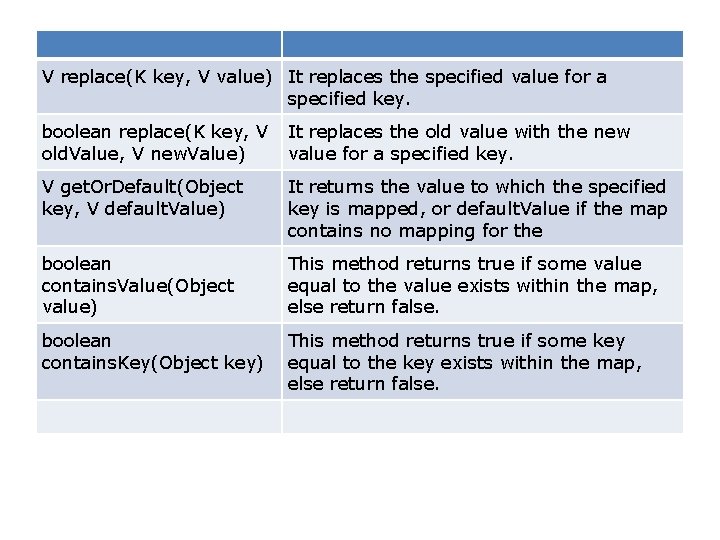
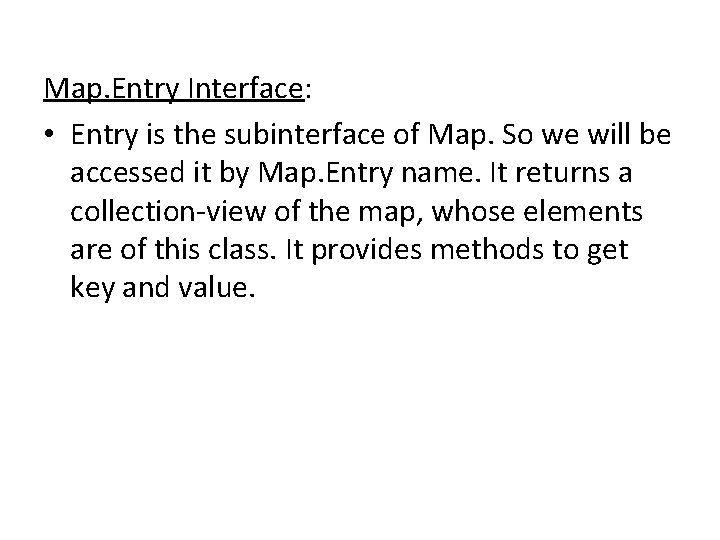
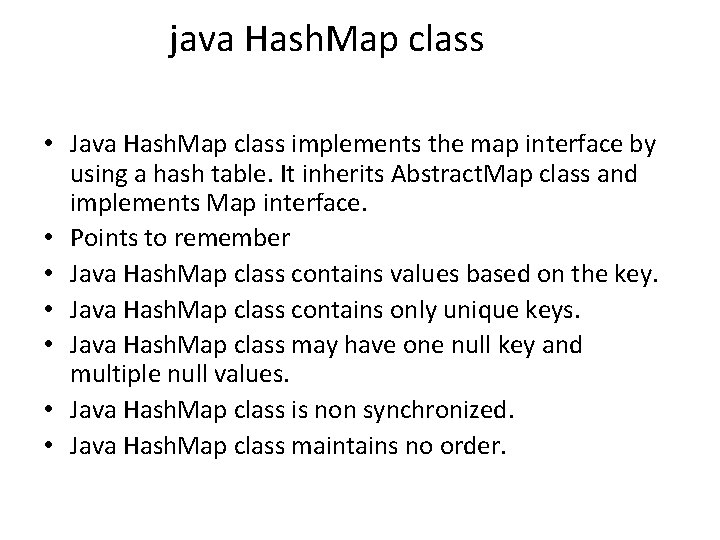
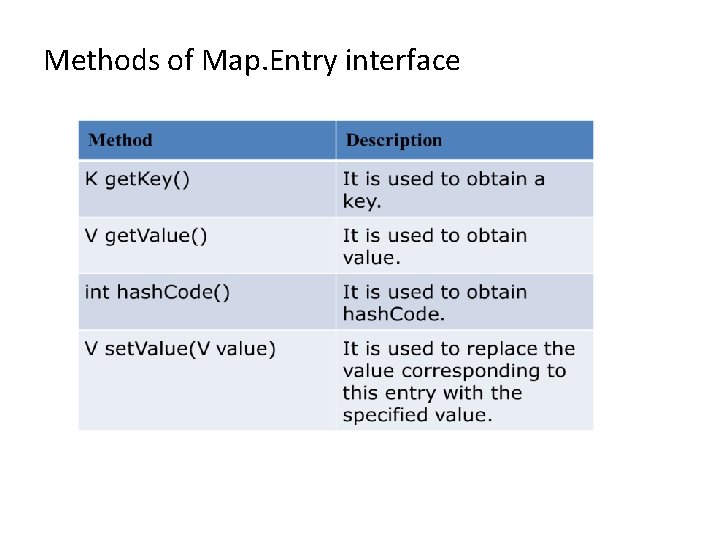
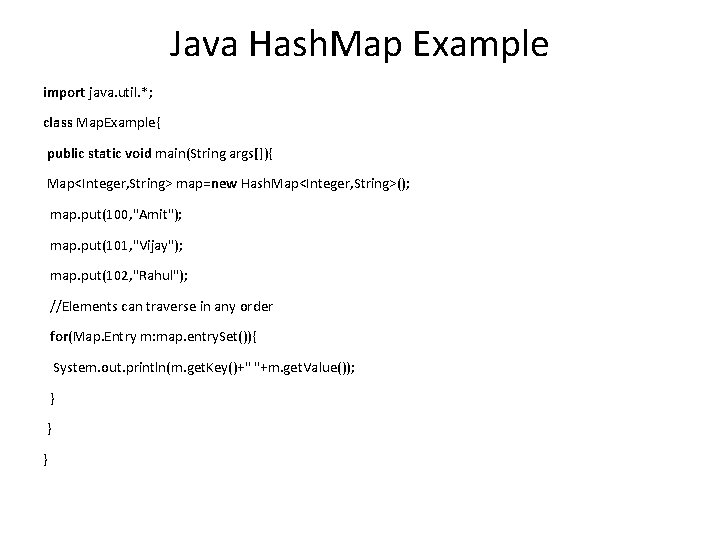
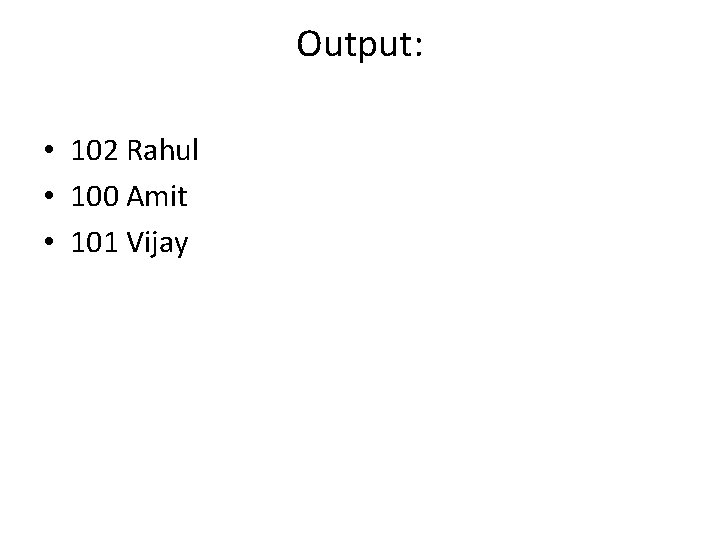
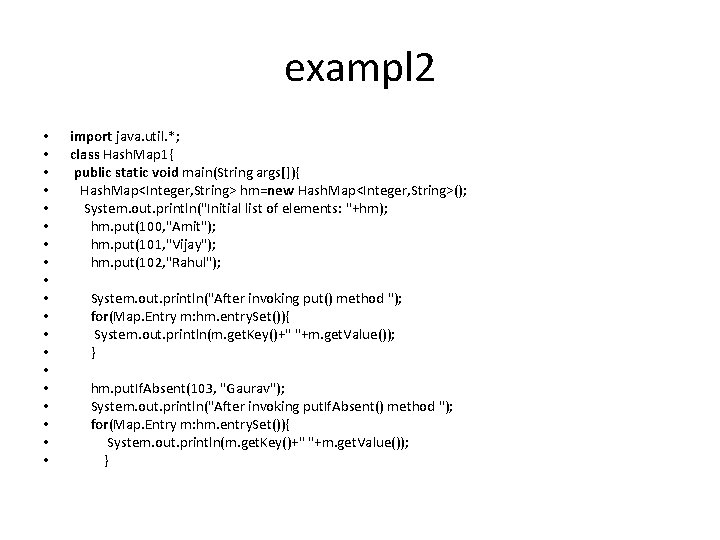
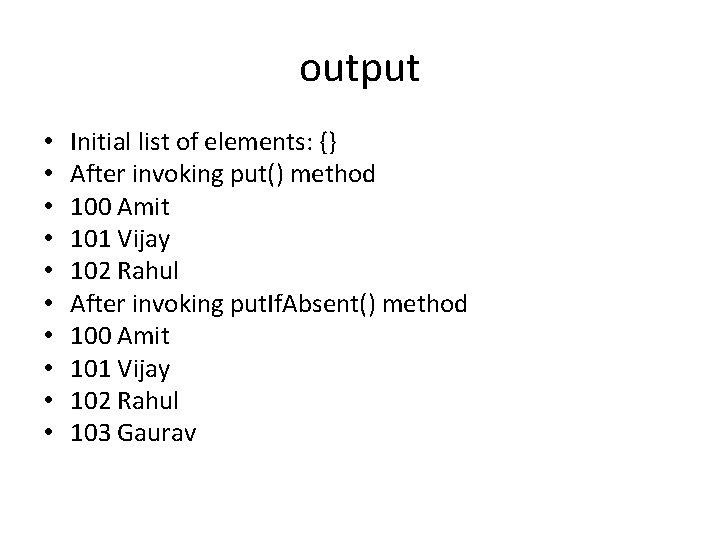
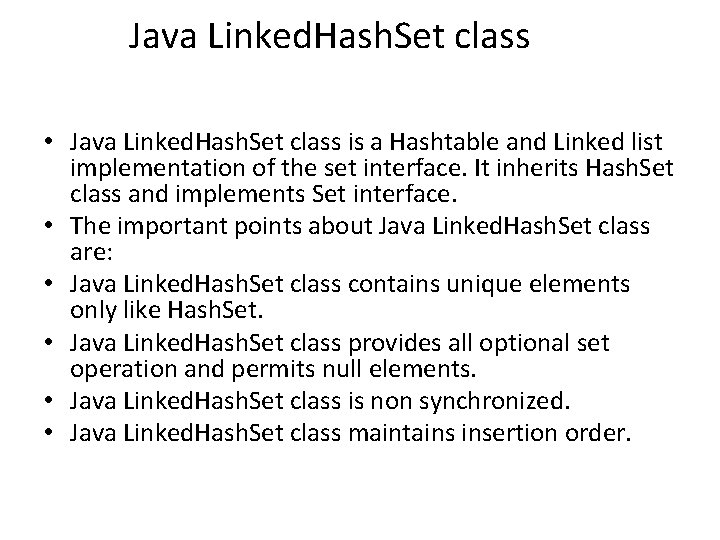
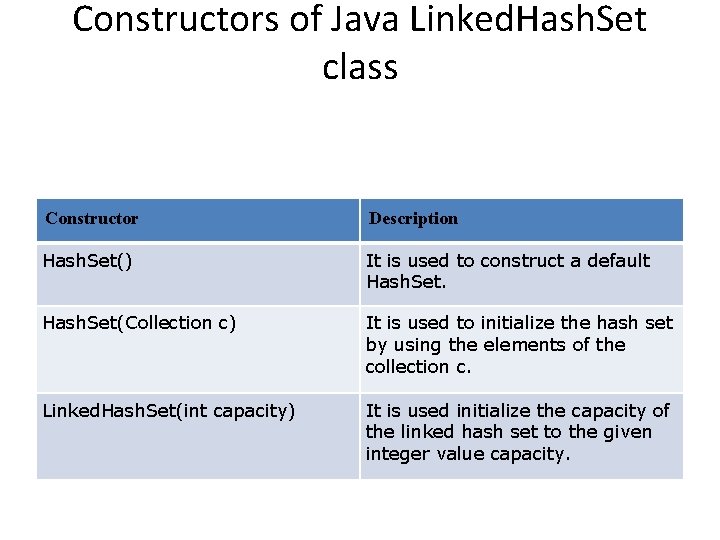
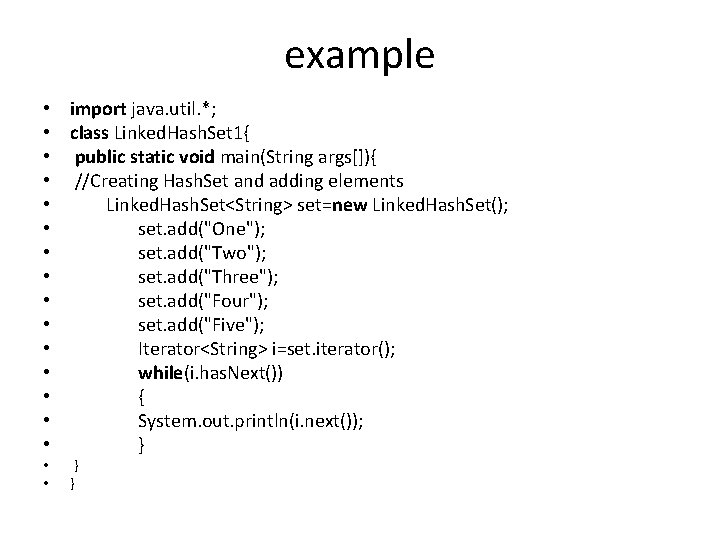
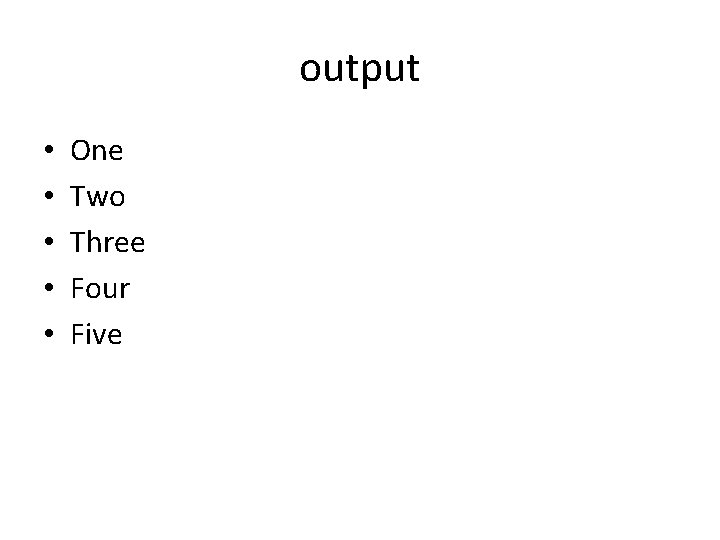
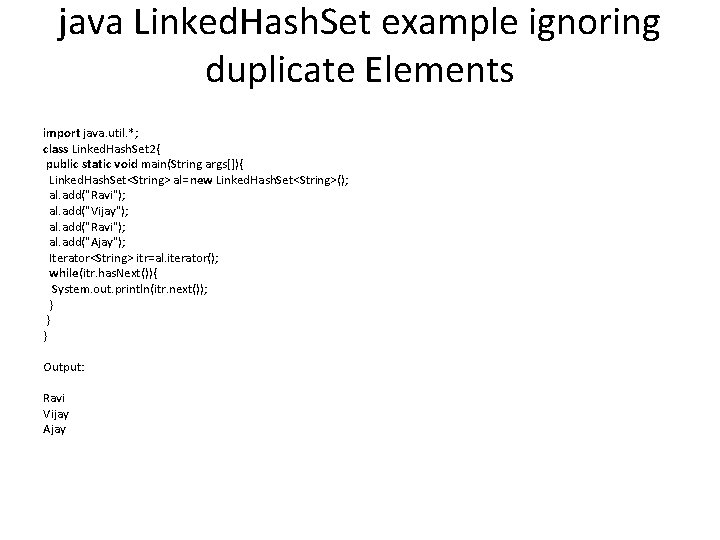
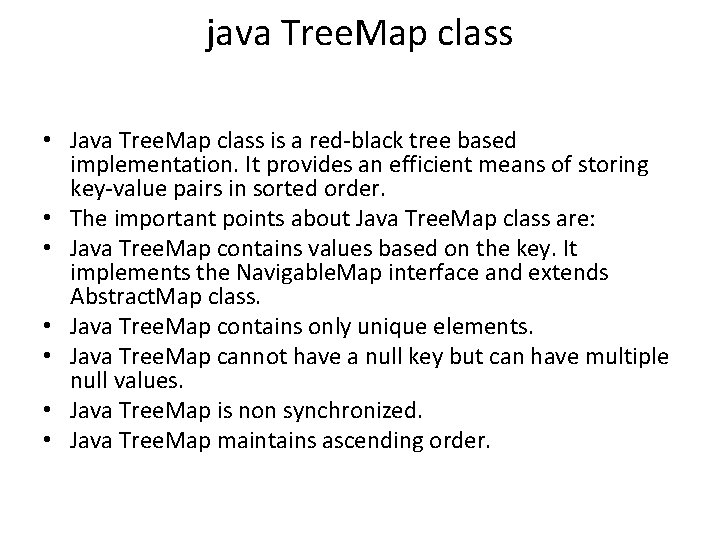
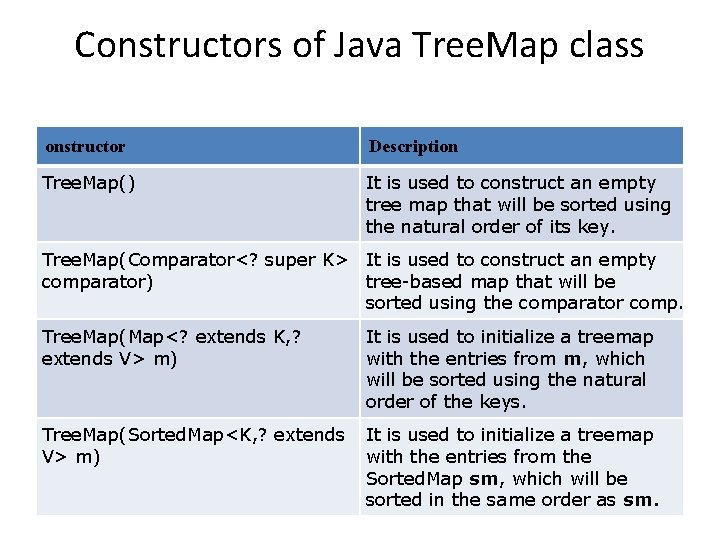
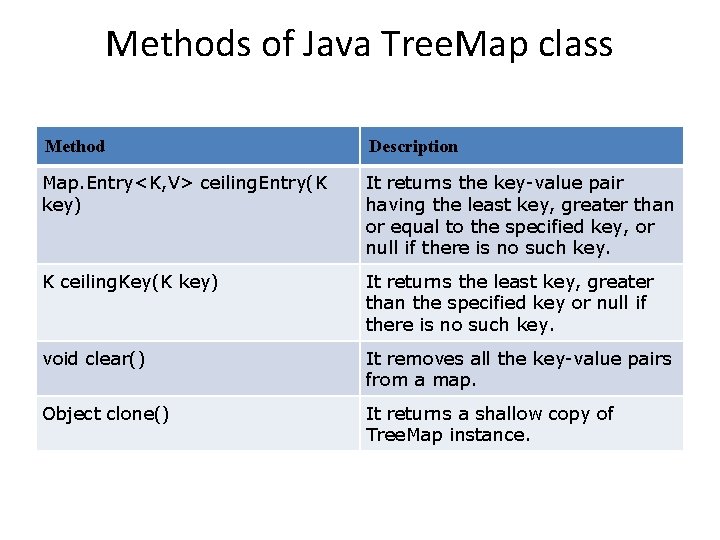
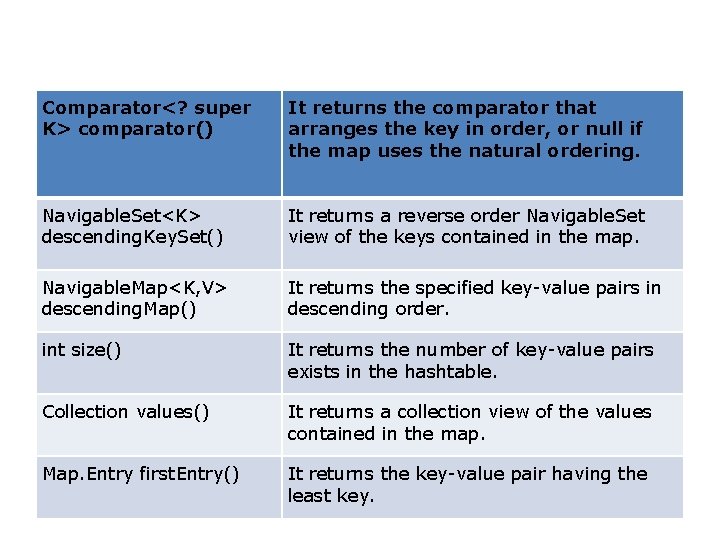
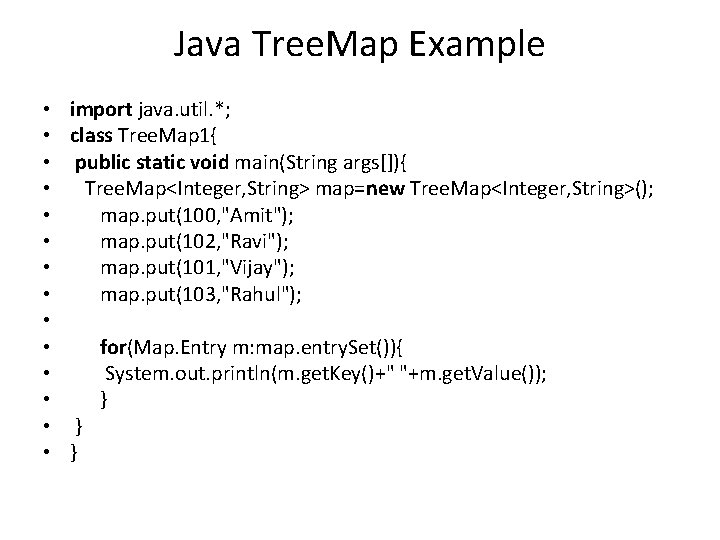
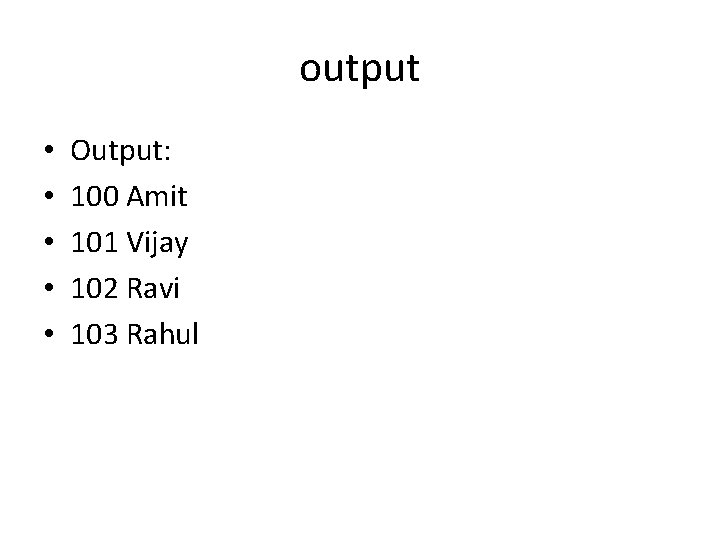
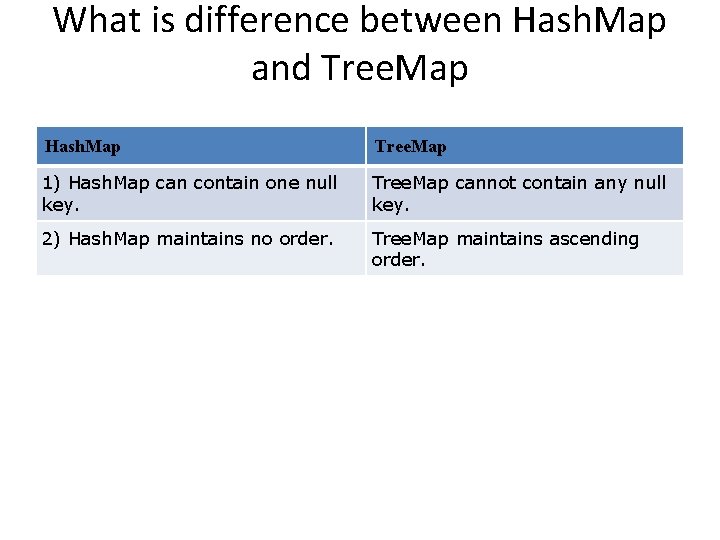
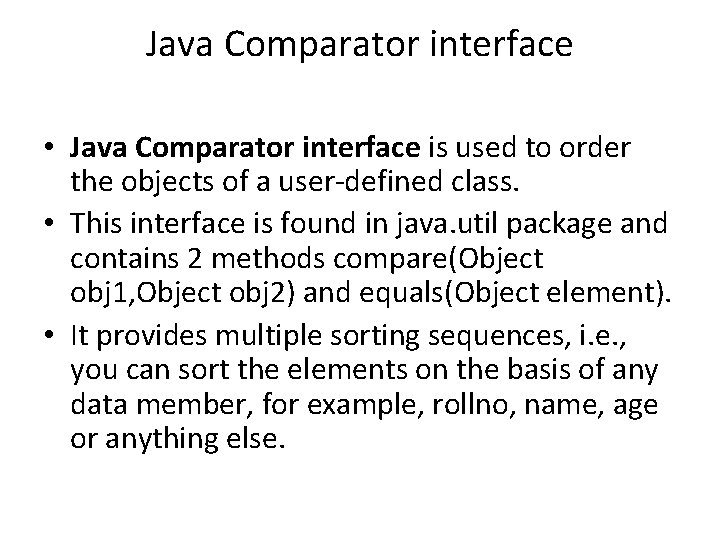
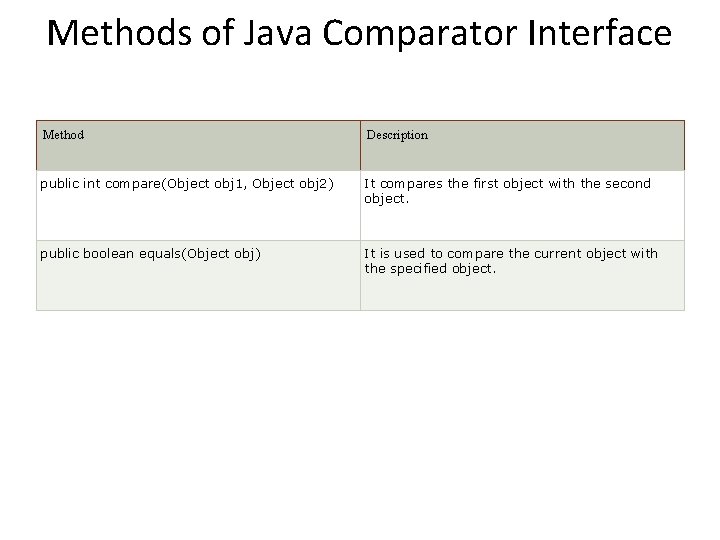
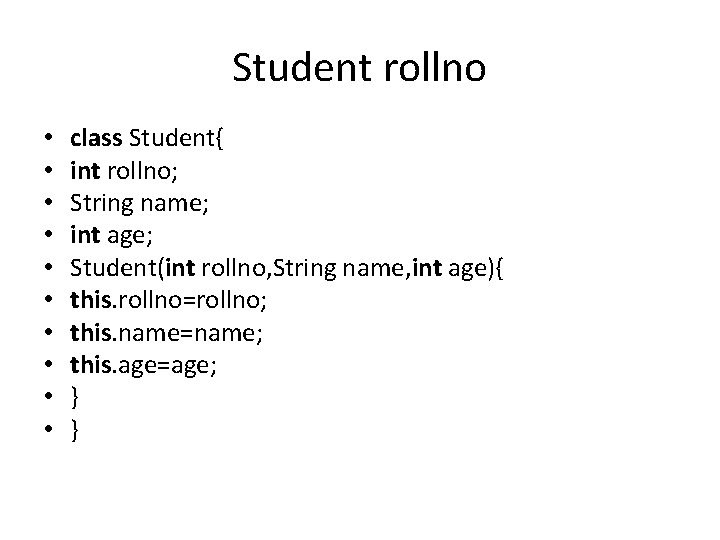
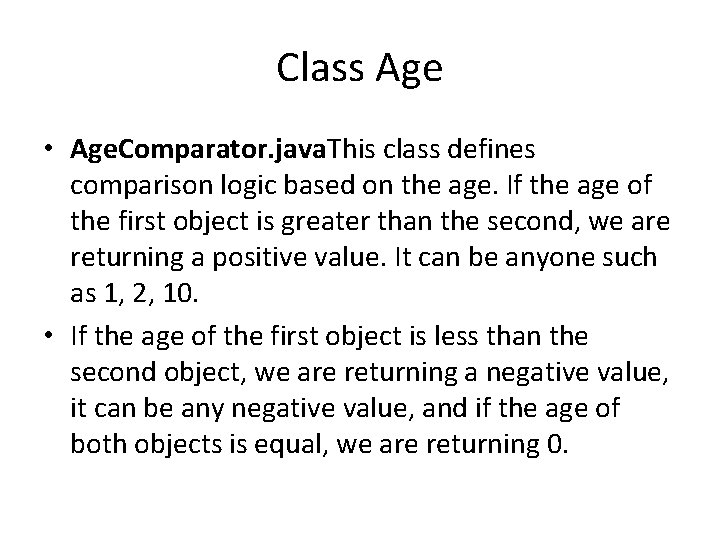
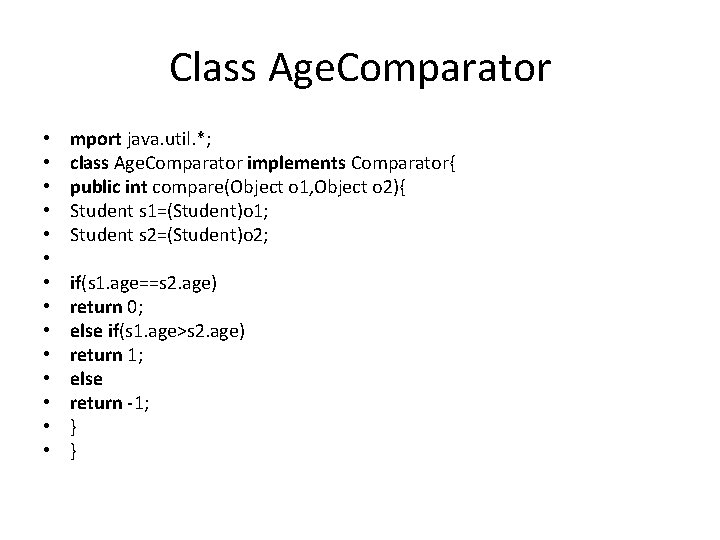
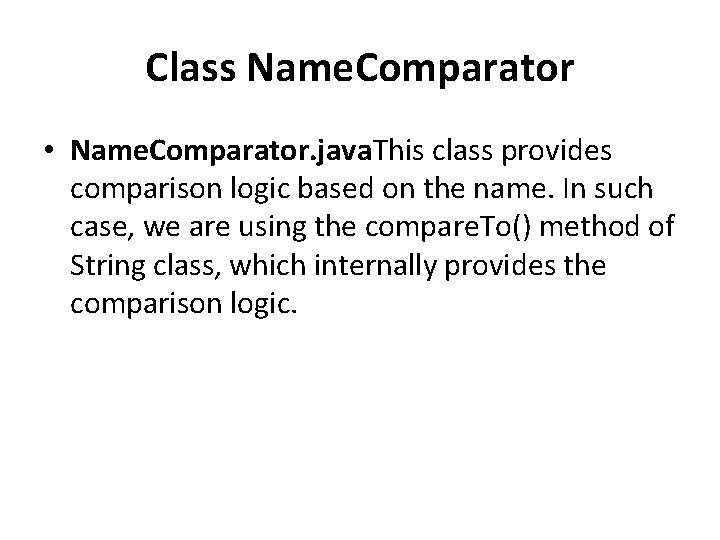
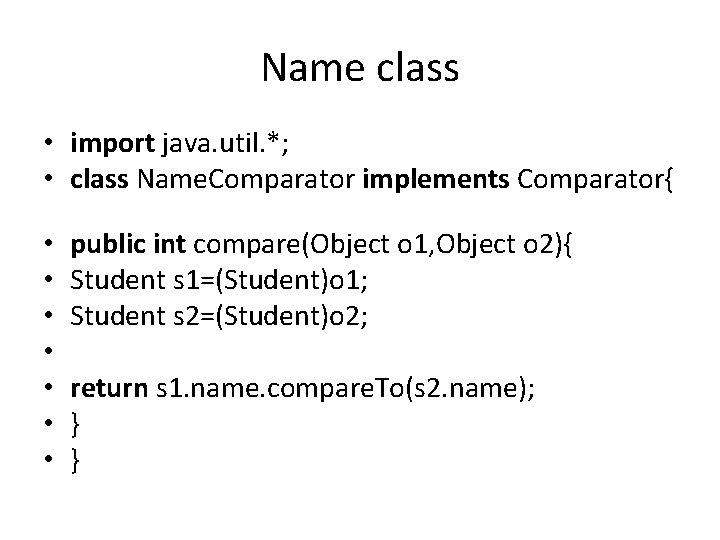
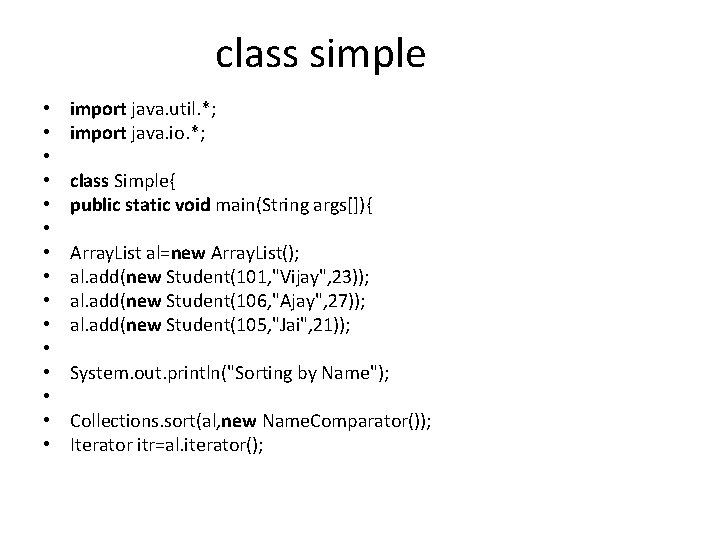
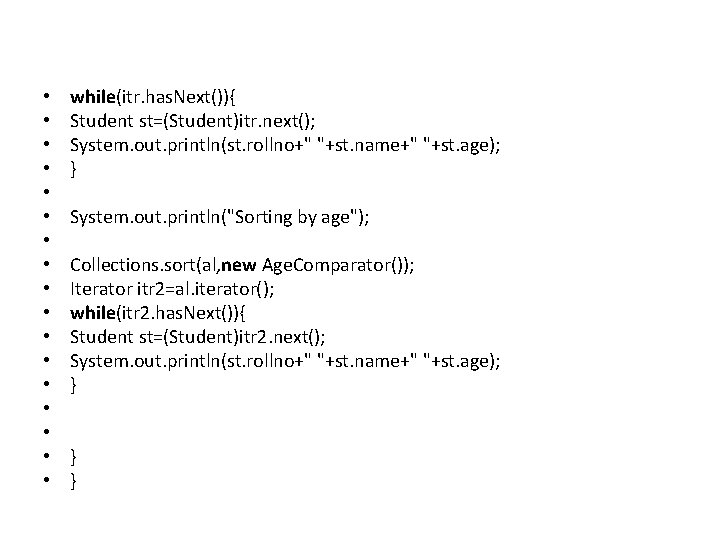
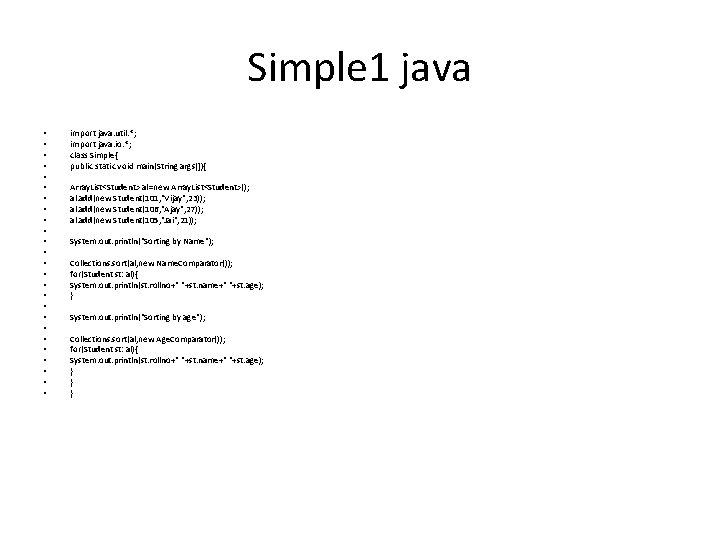
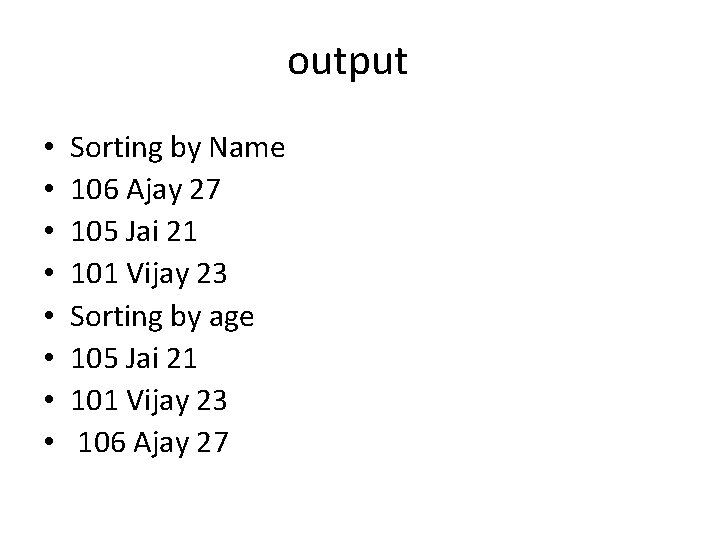
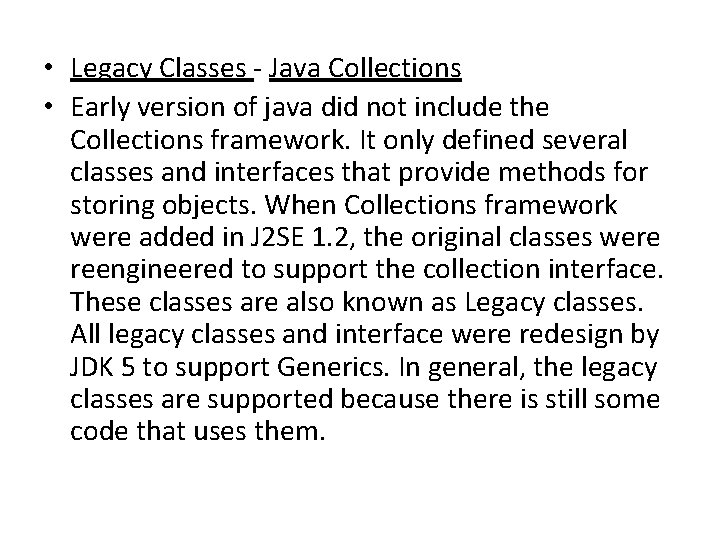
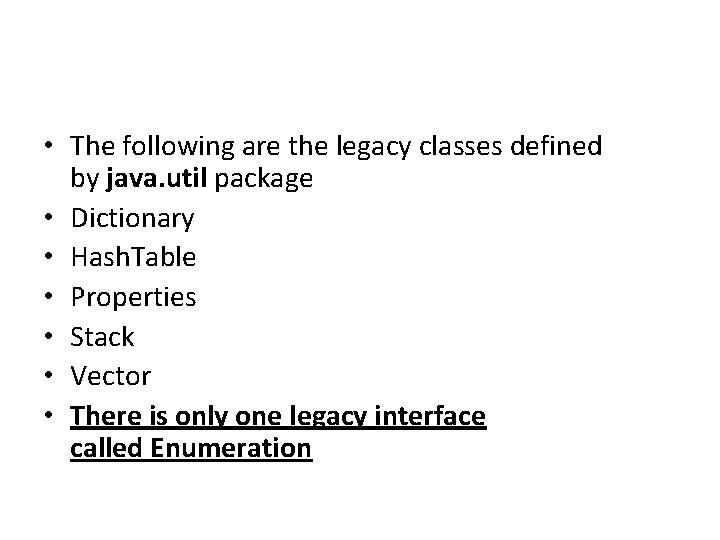
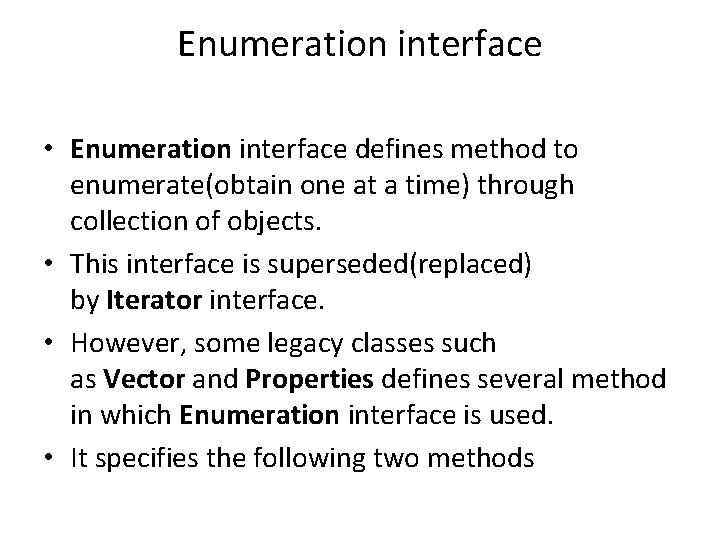
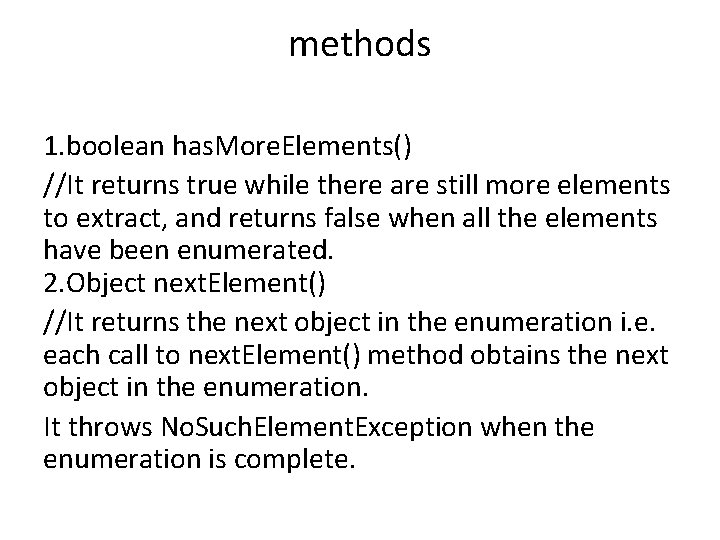
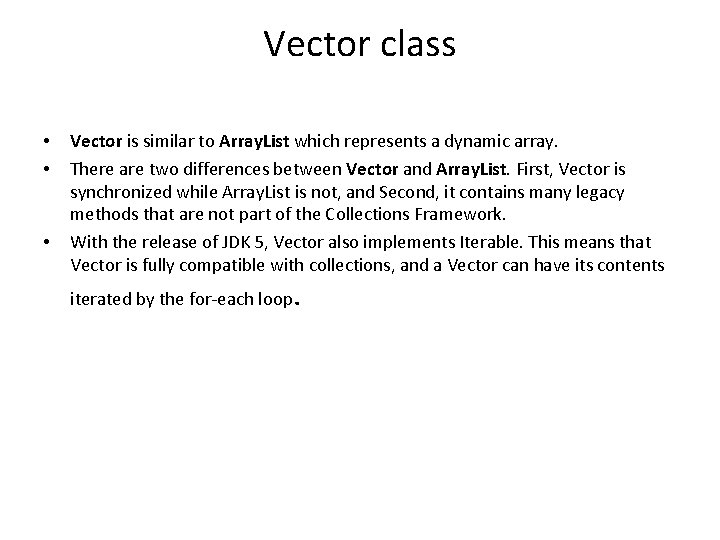
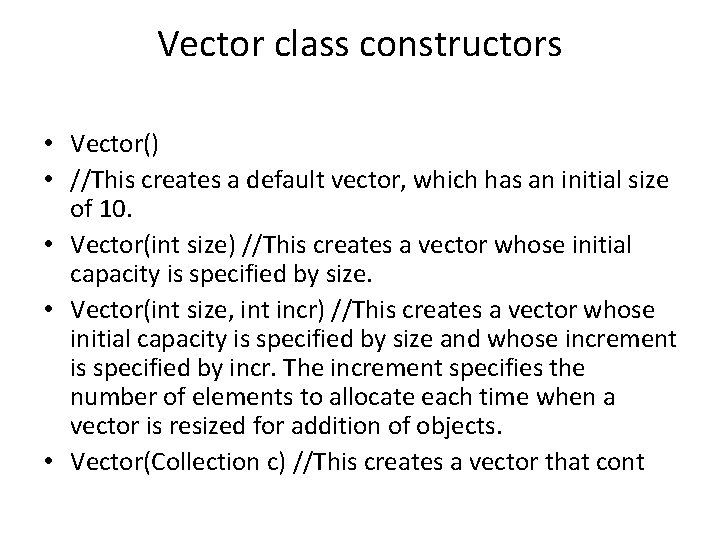
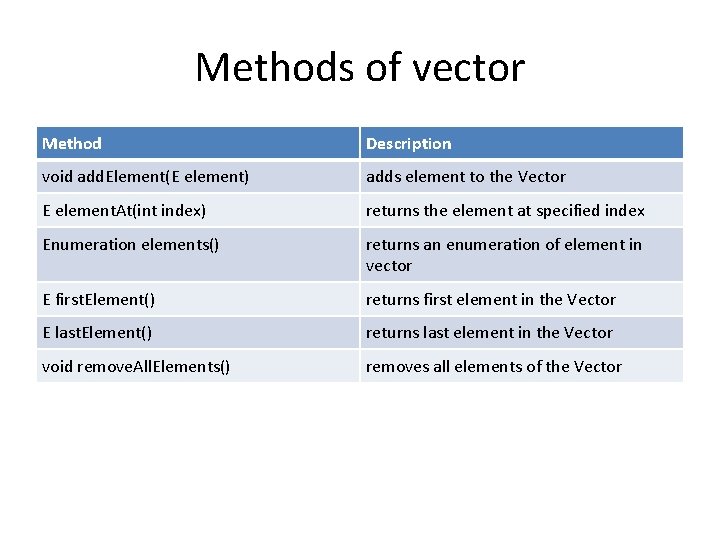
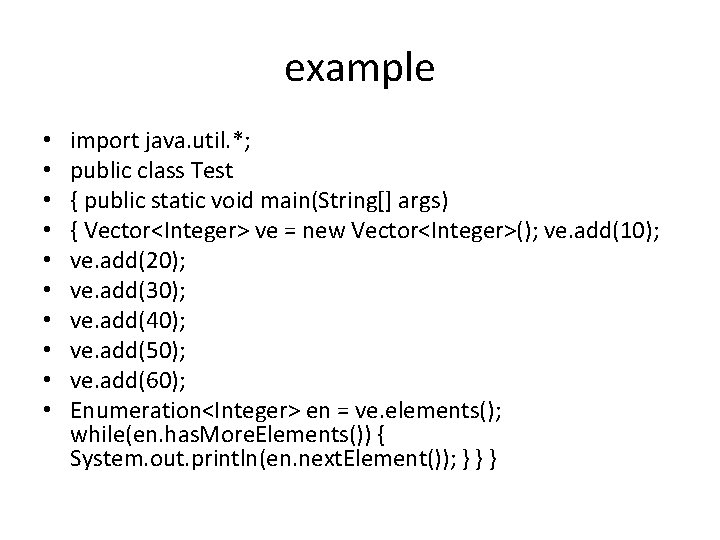
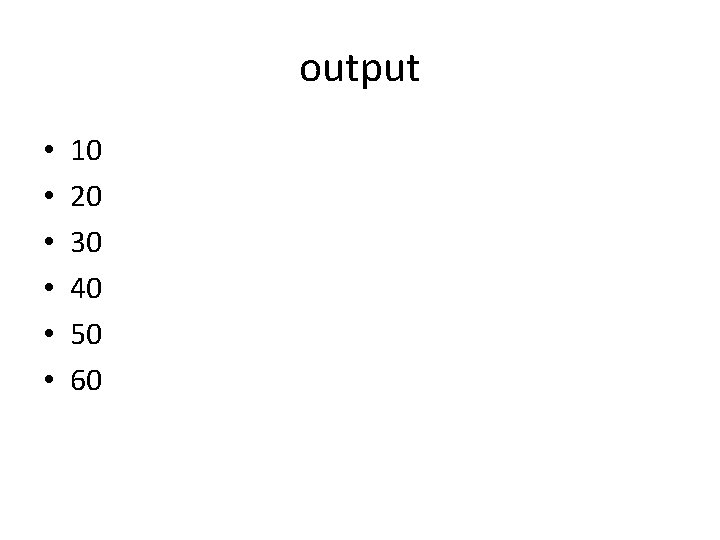
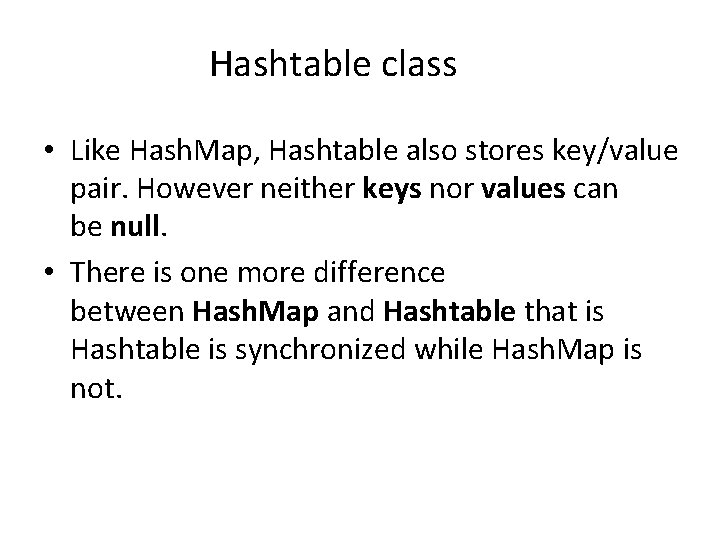
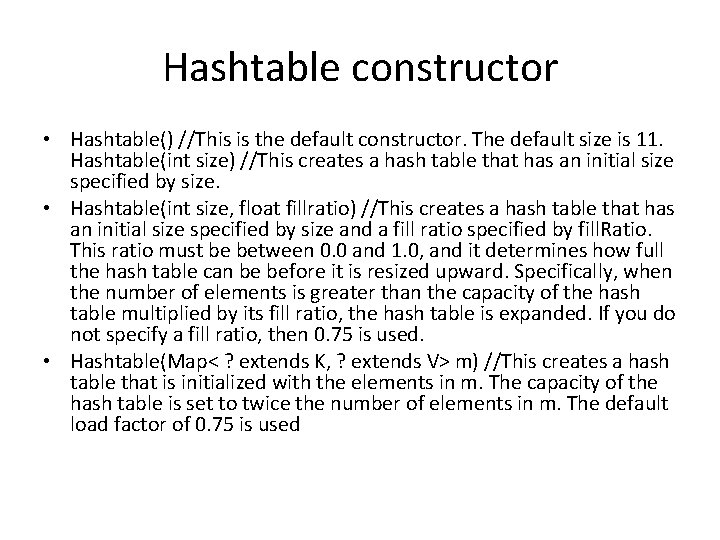
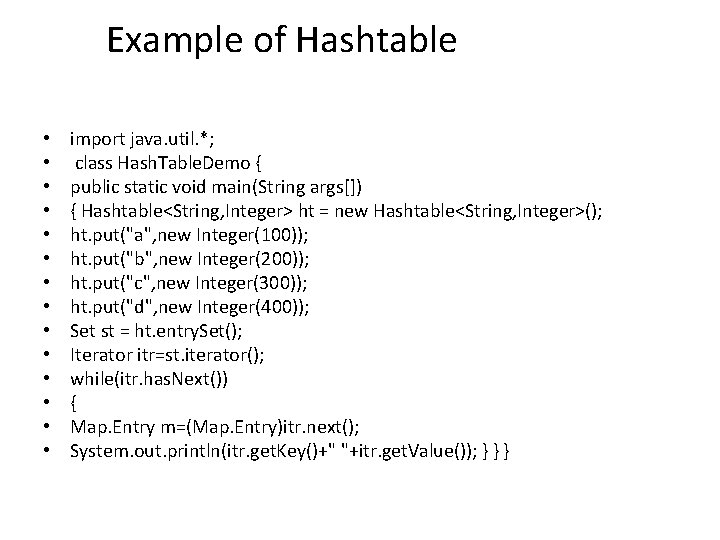
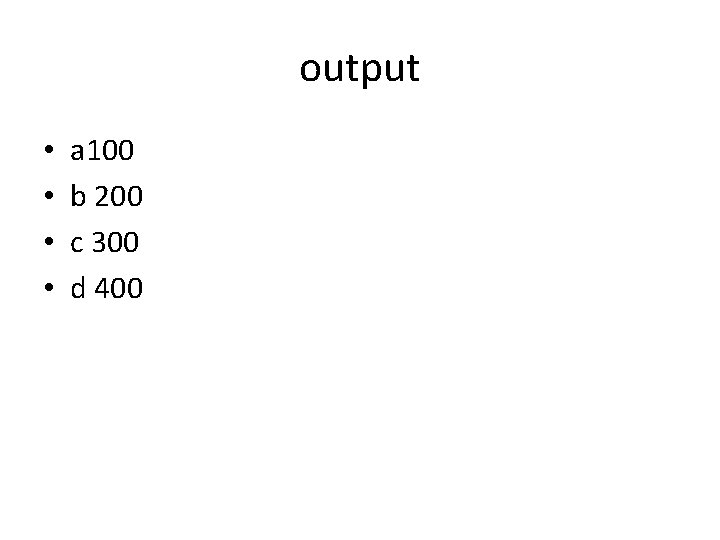
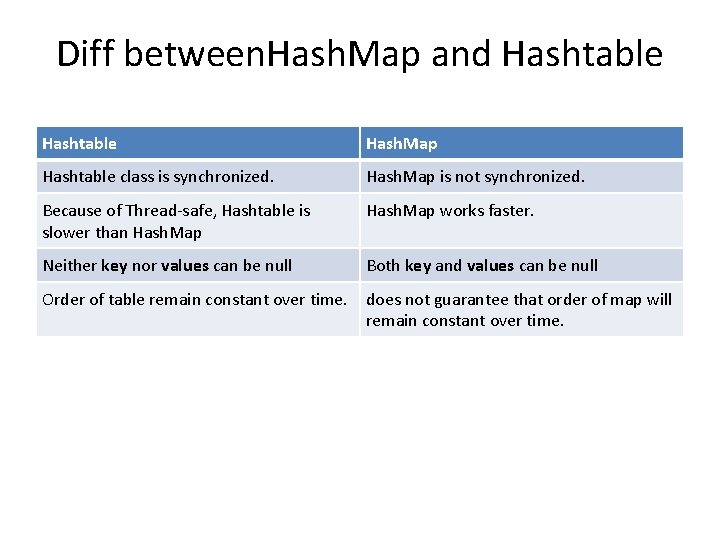
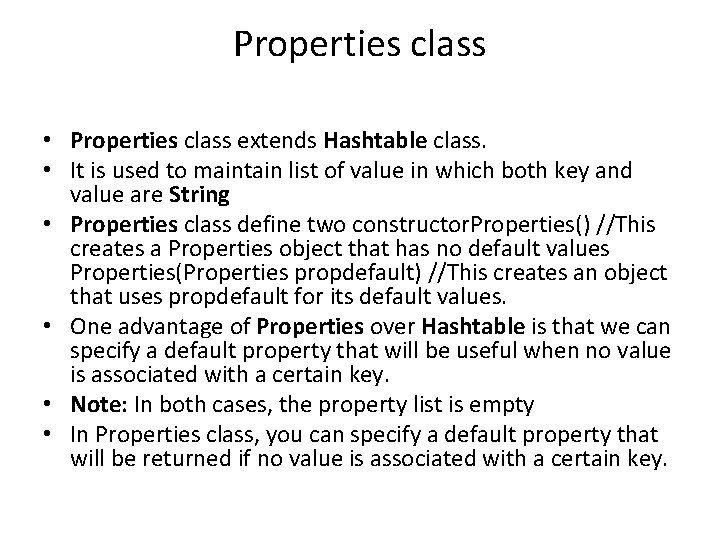
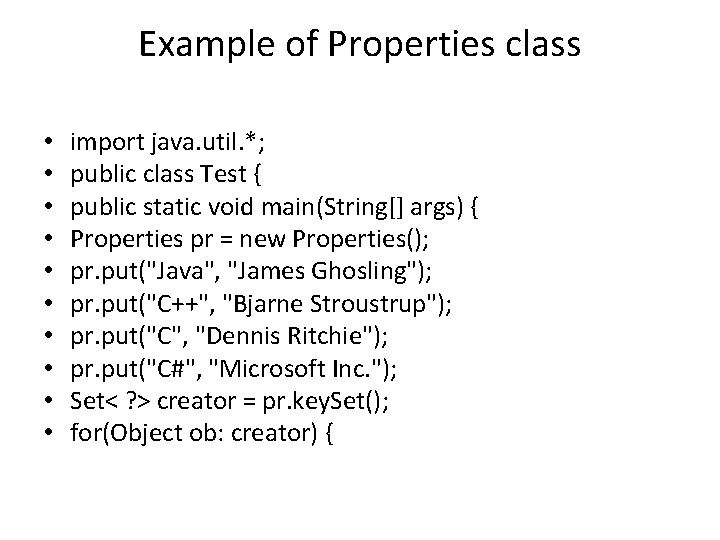
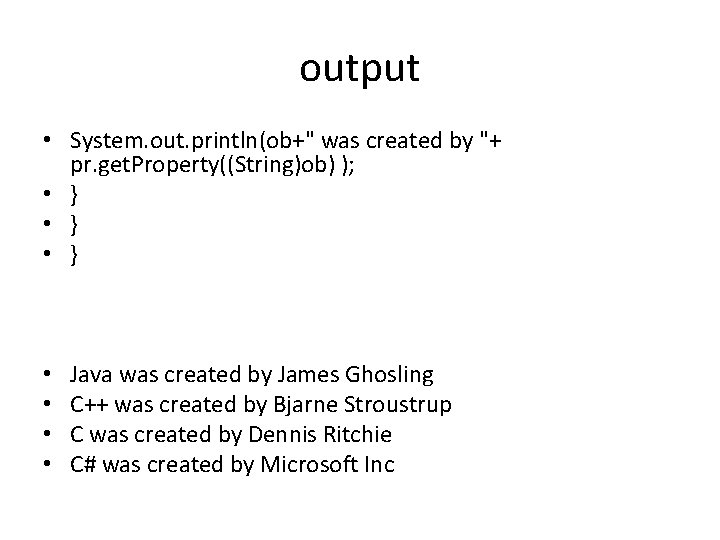
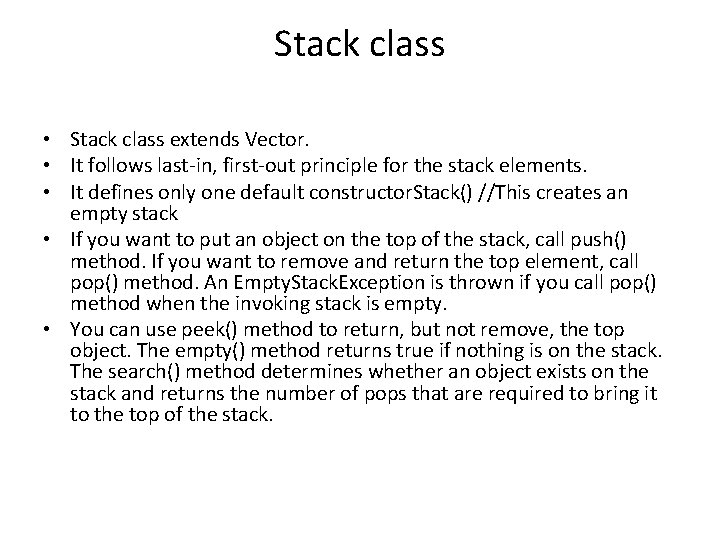
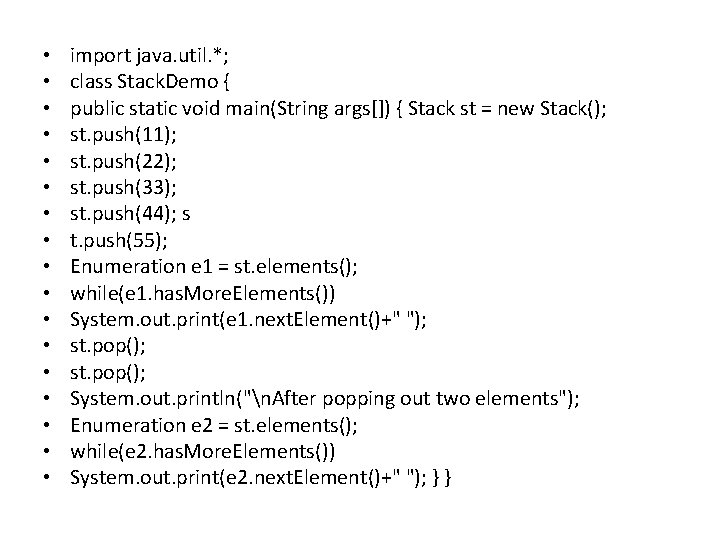
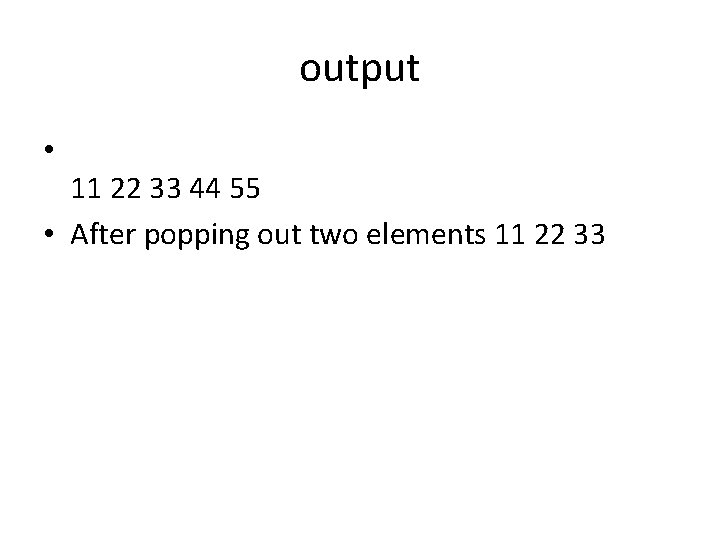
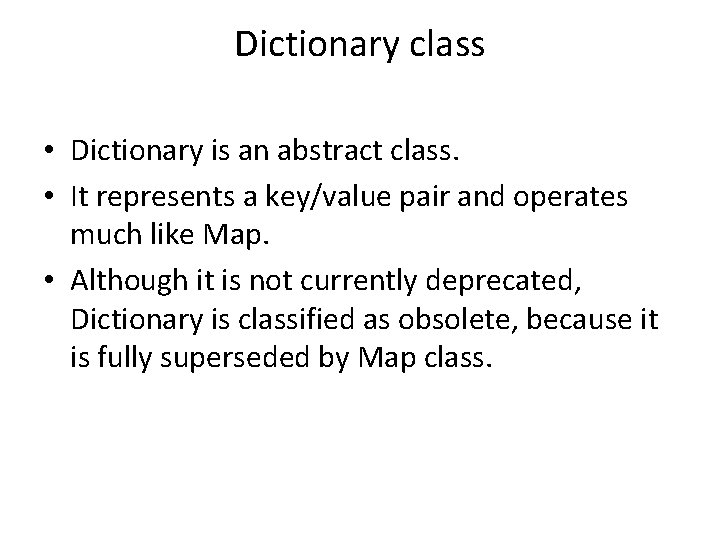
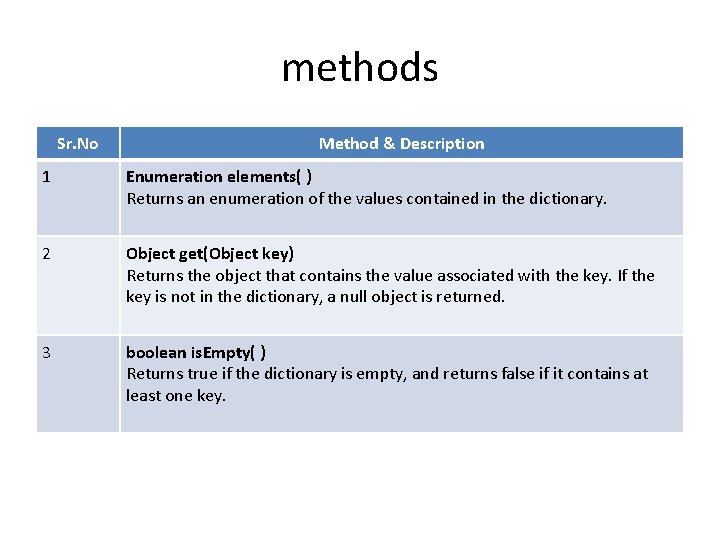
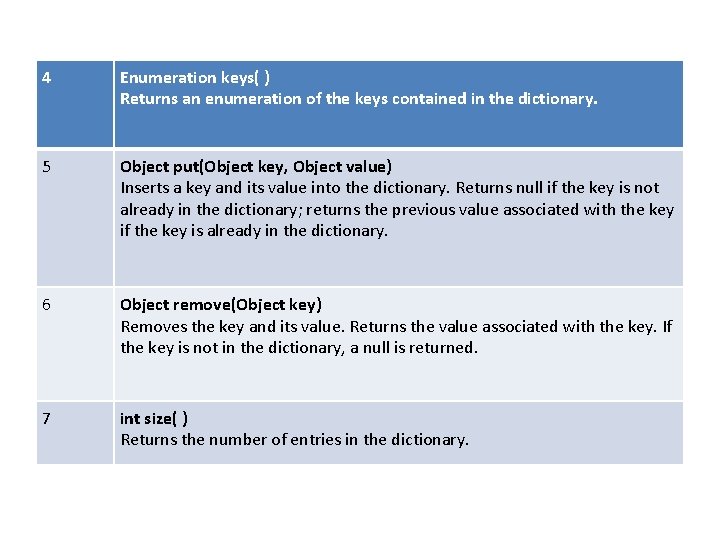
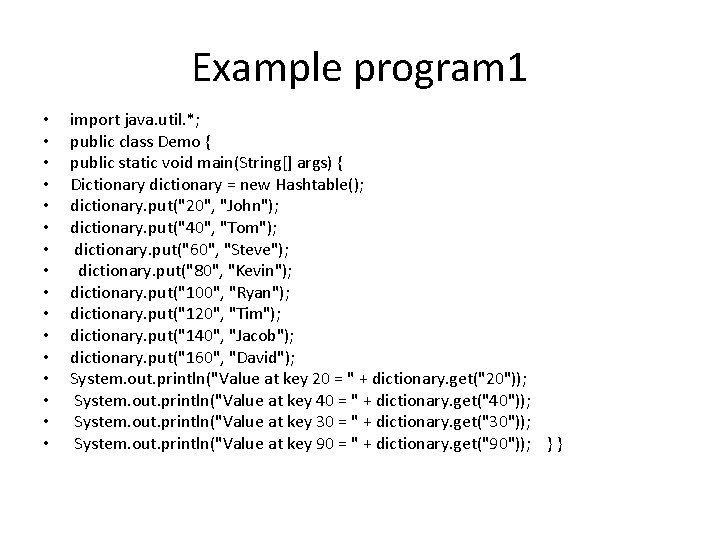
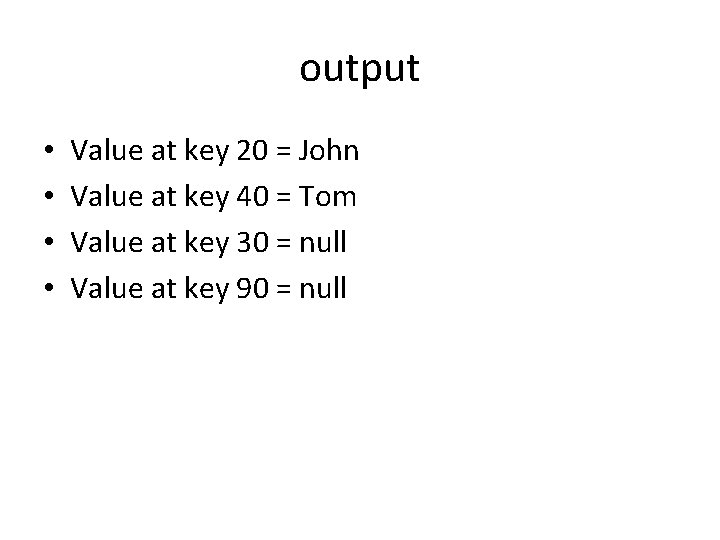
![example 2 import java. util. *; public class Demo { public static void main(String[] example 2 import java. util. *; public class Demo { public static void main(String[]](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-102.jpg)
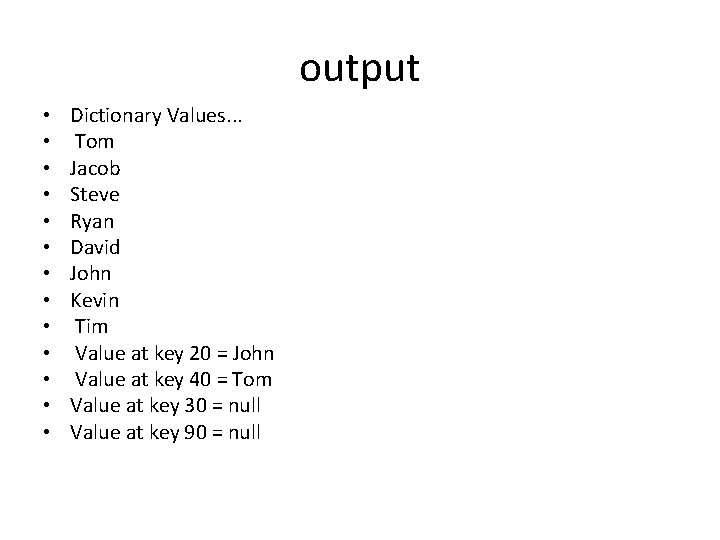
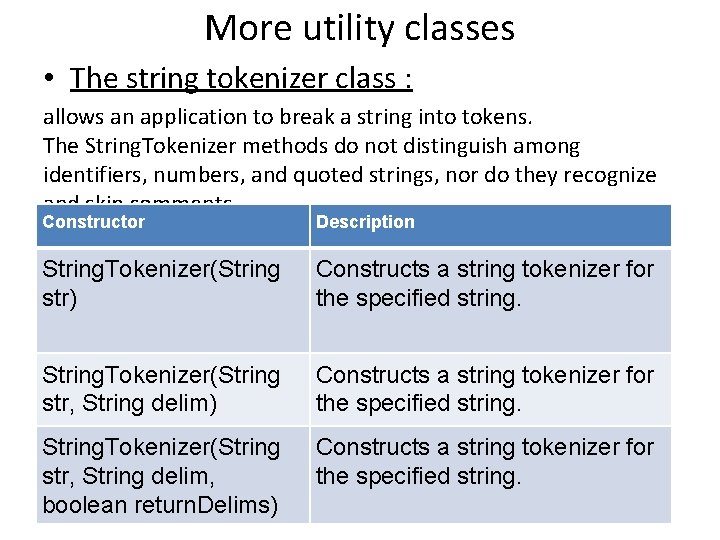
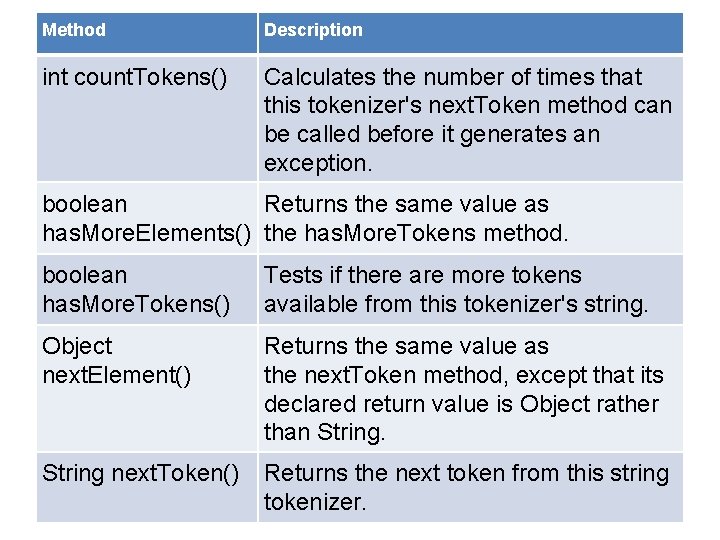
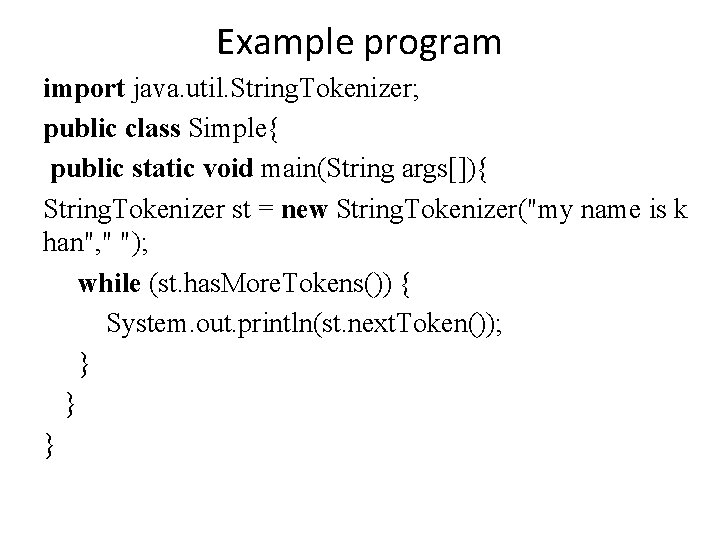
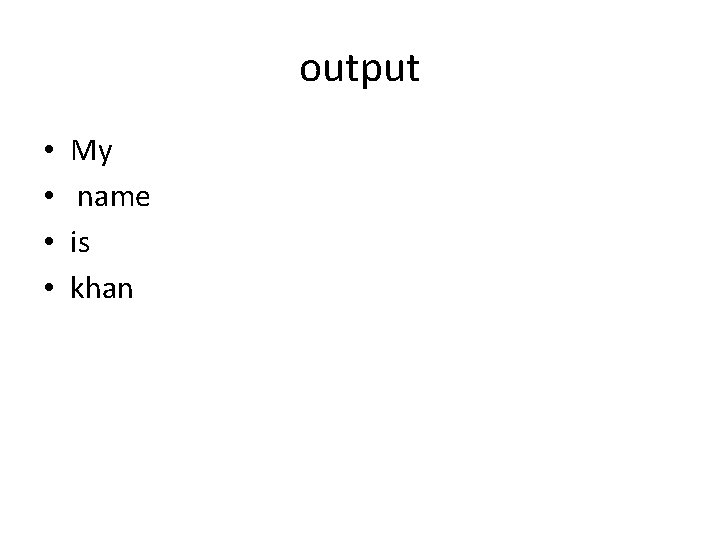
![import java. util. *; public class Test { public static void main(String[] args) { import java. util. *; public class Test { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-108.jpg)
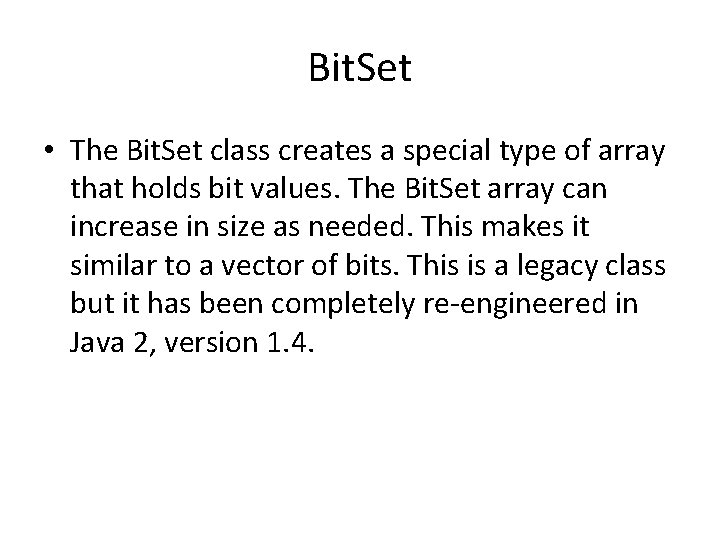
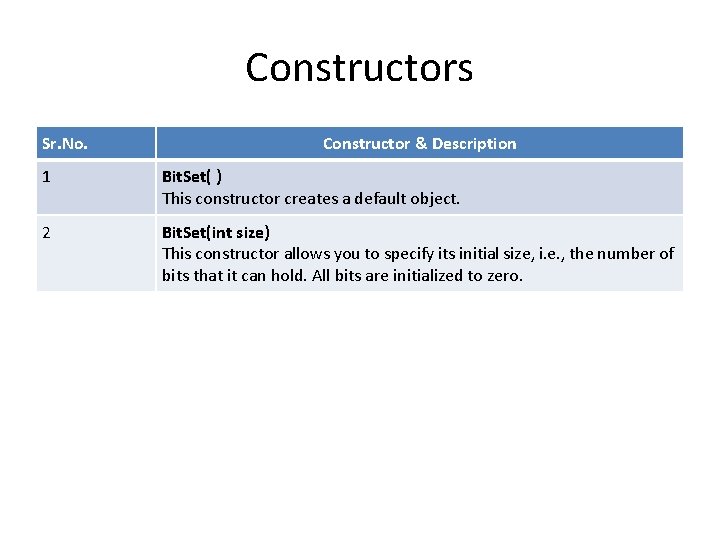
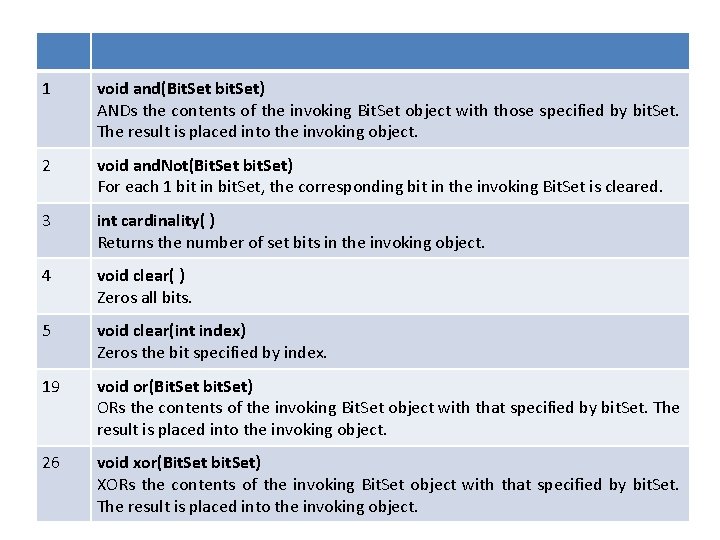
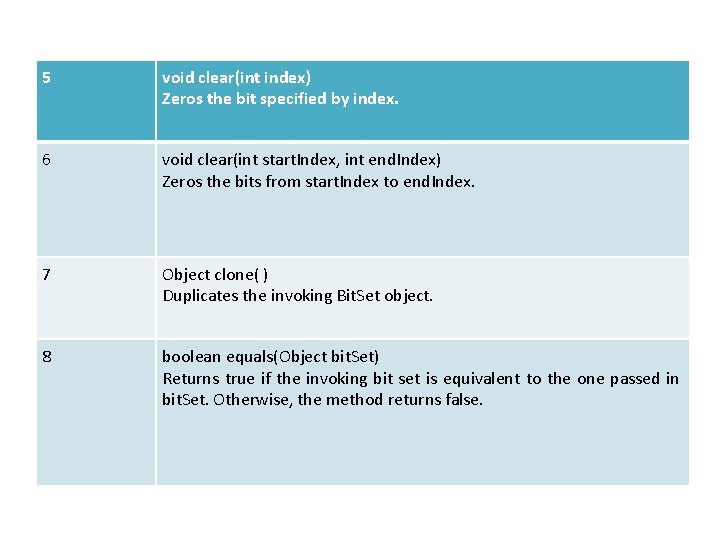
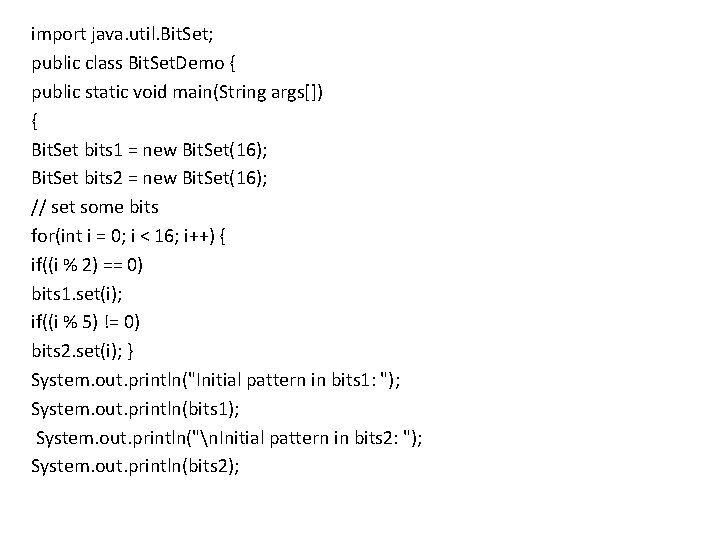
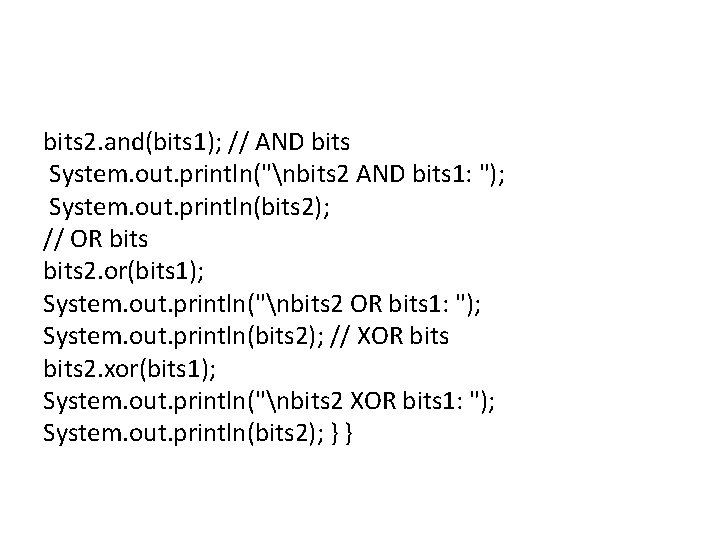
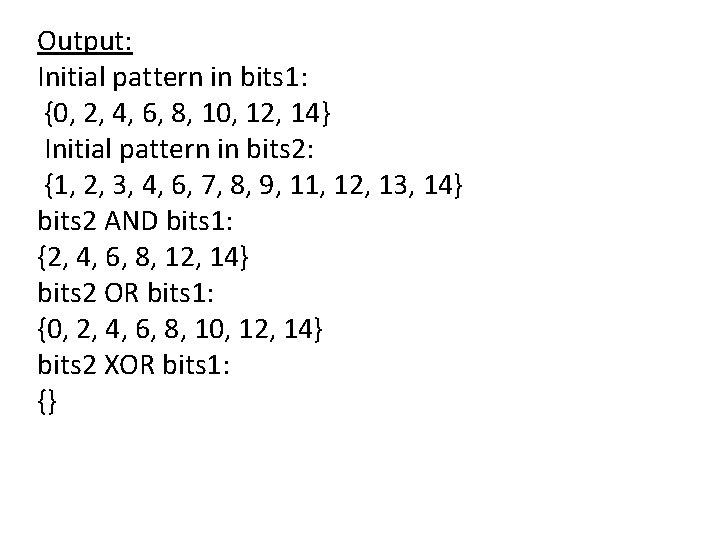
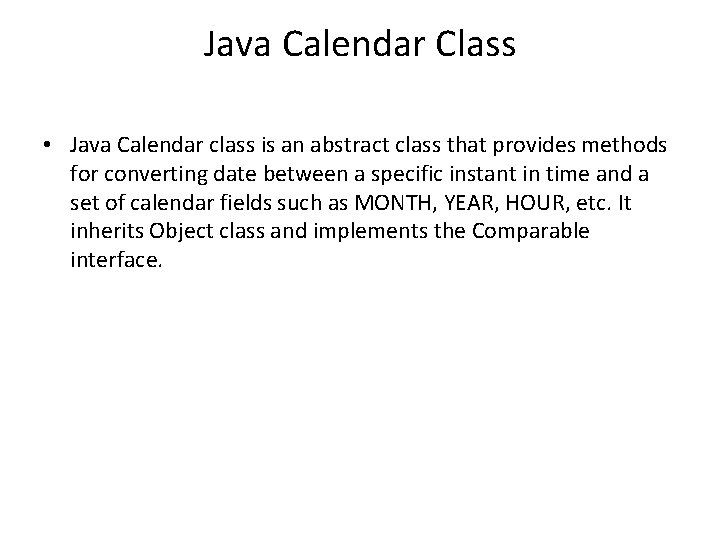
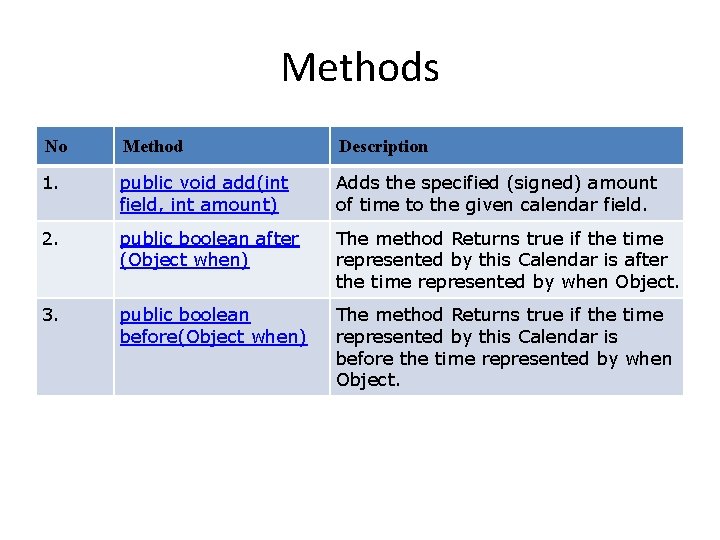
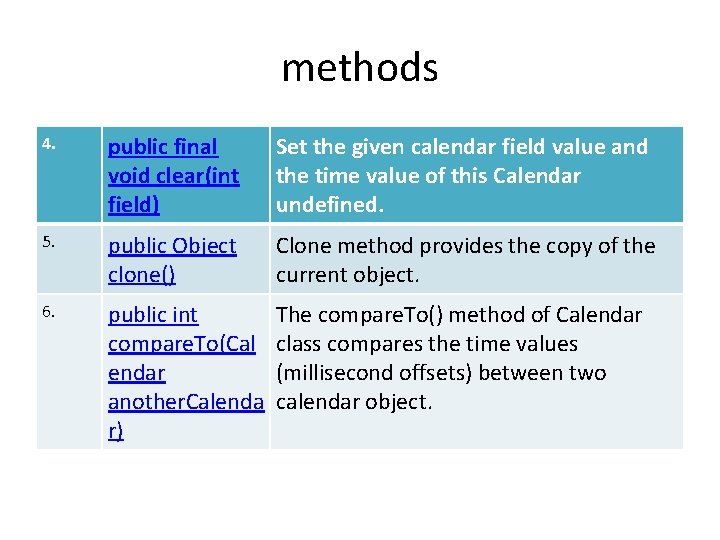
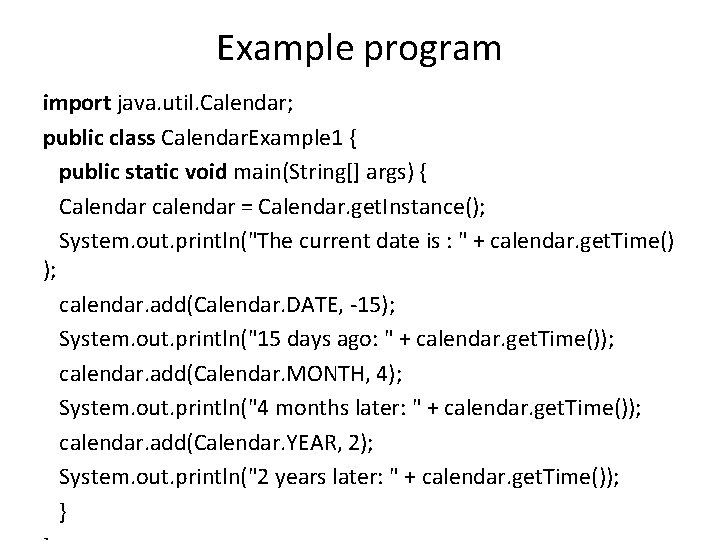
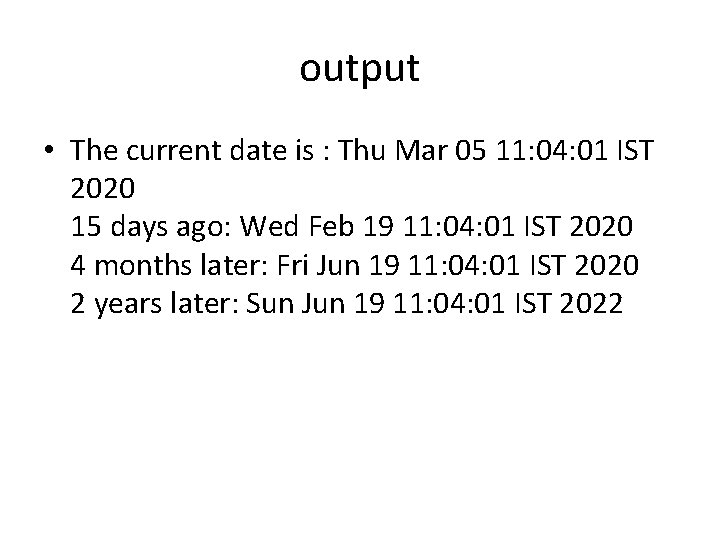
![import java. util. *; public class Calendar. Example 2{ public static void main(String[] args) import java. util. *; public class Calendar. Example 2{ public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-121.jpg)
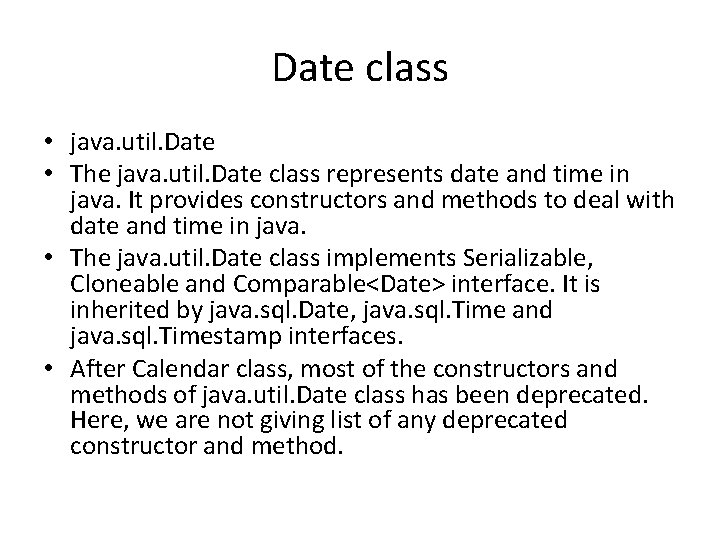
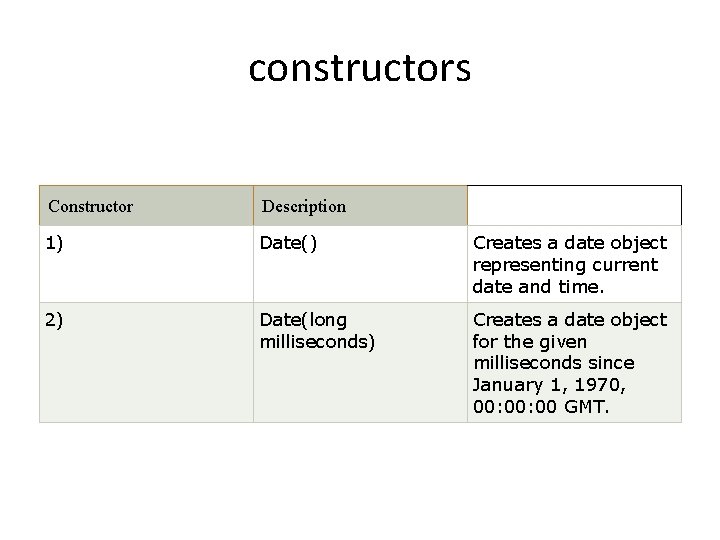
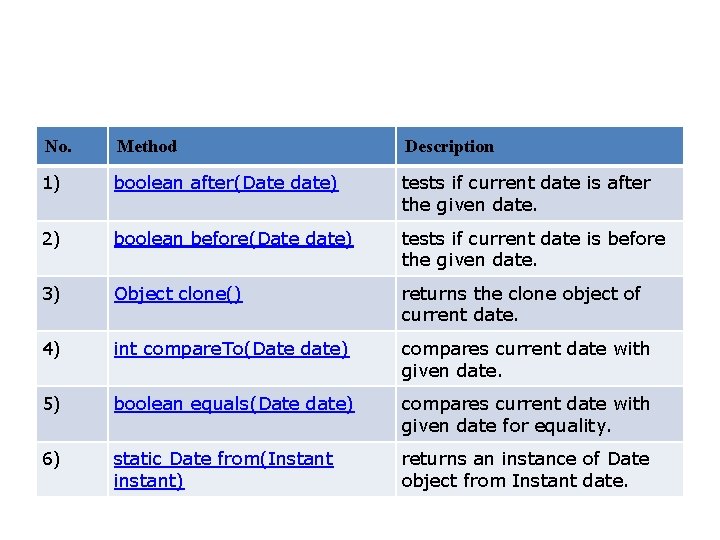
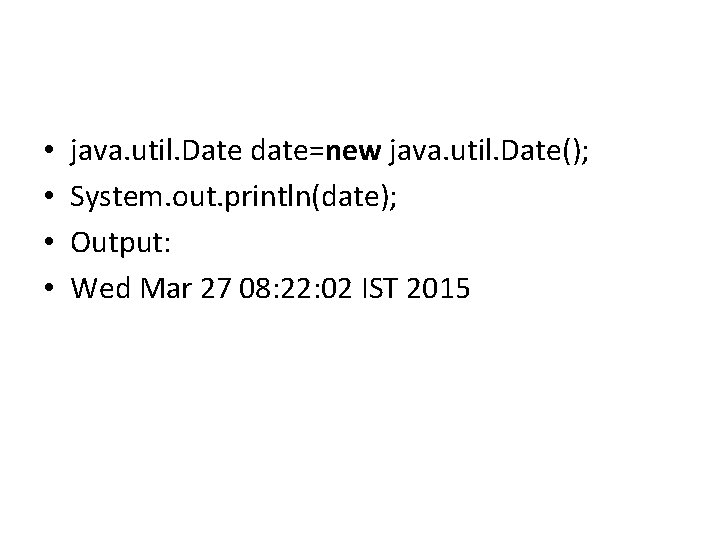
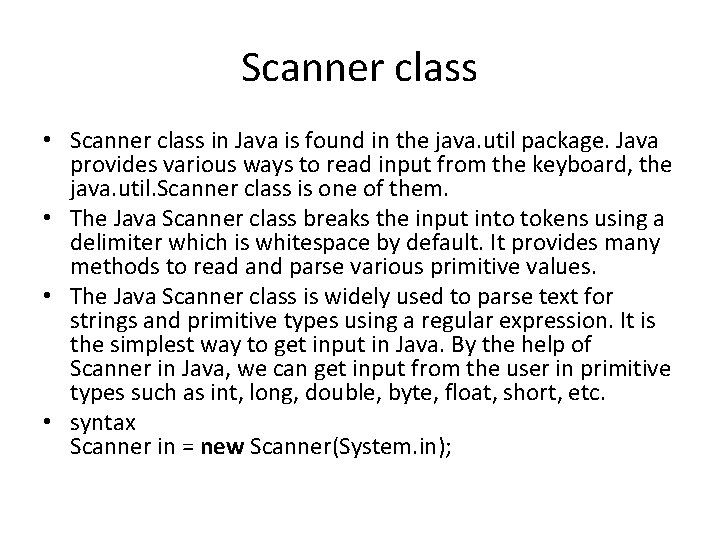
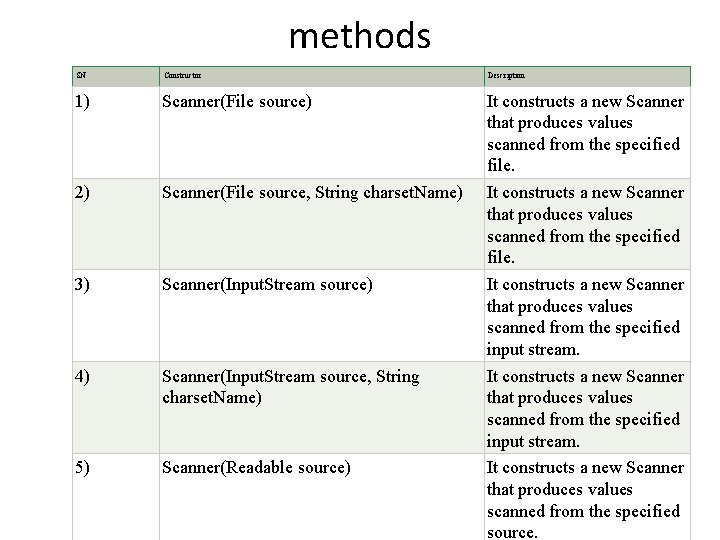
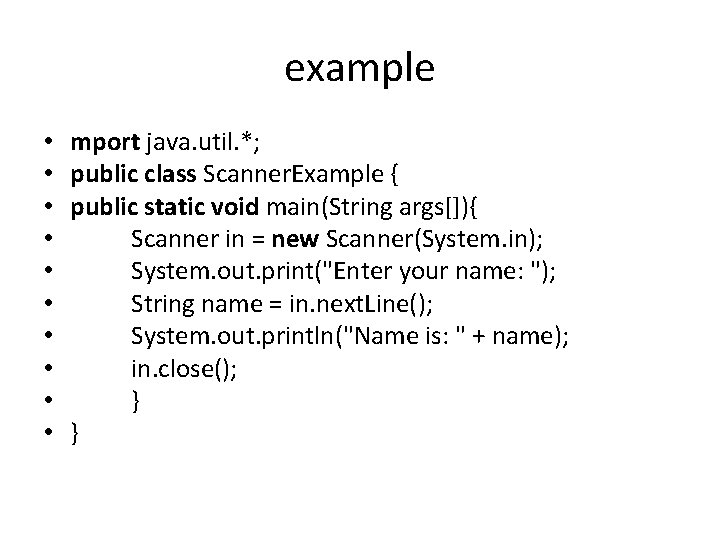
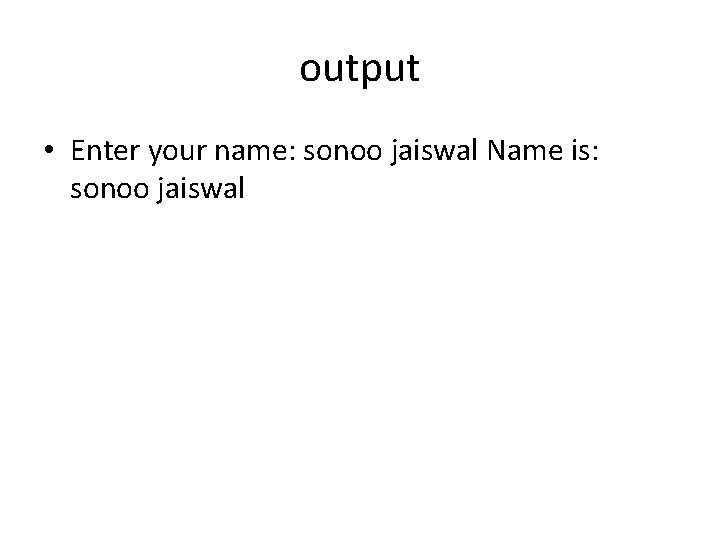
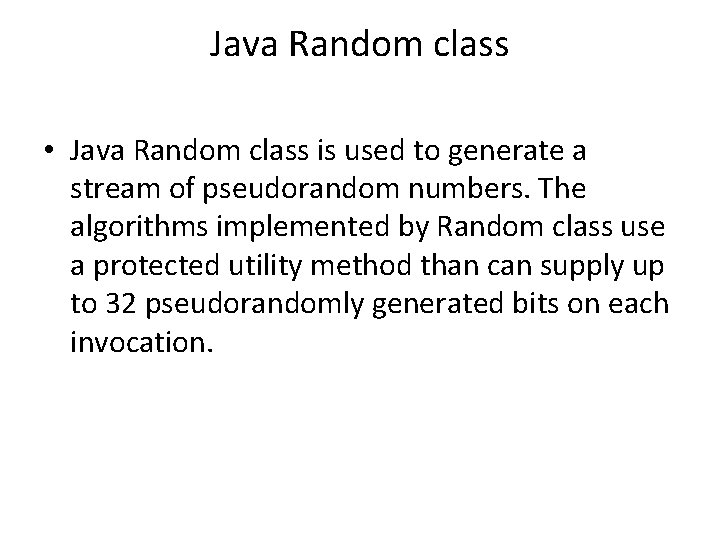
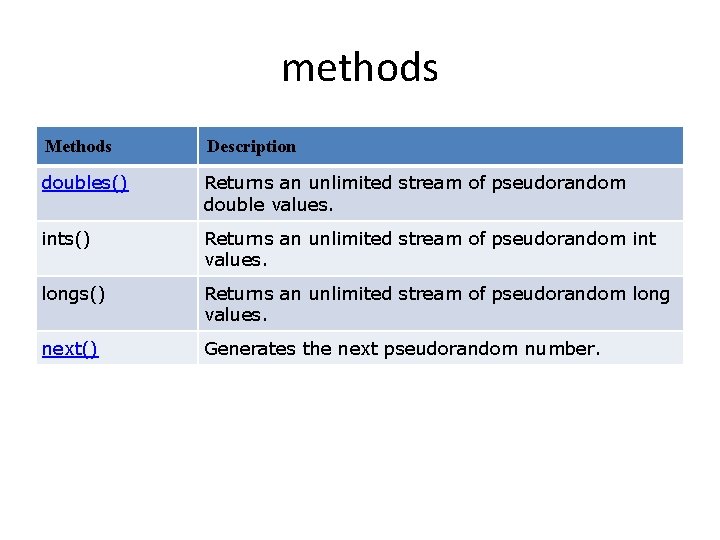
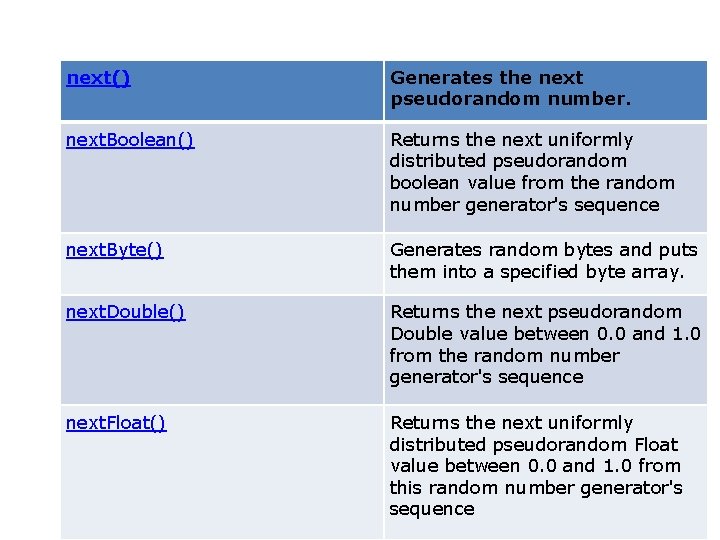
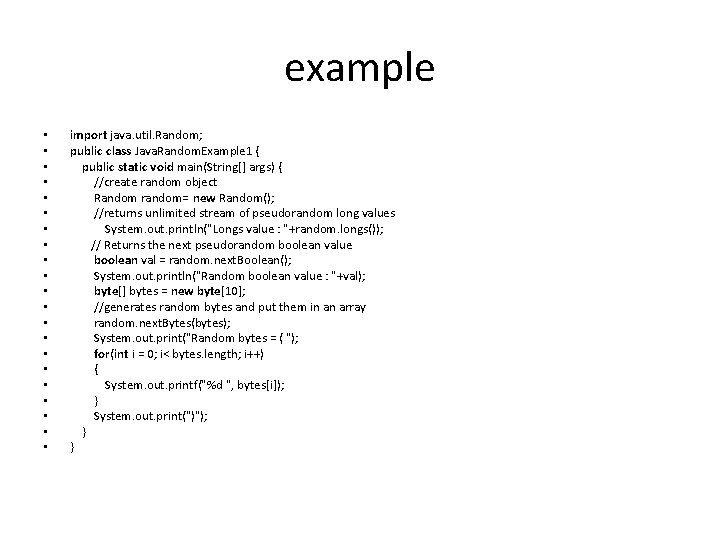
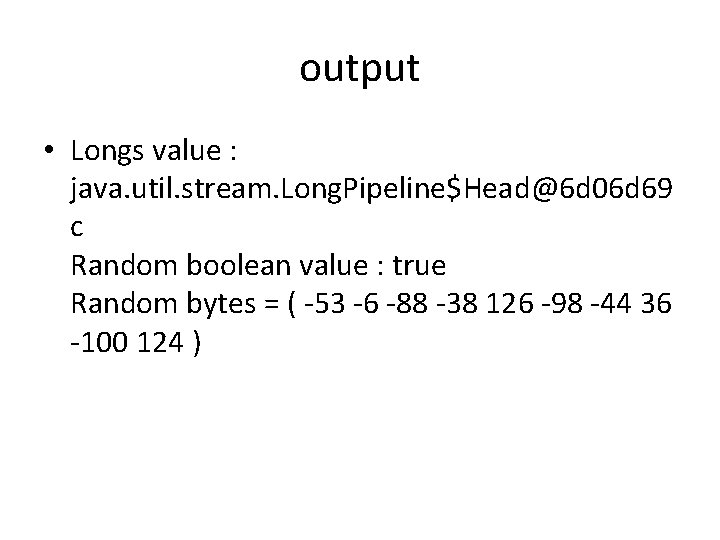
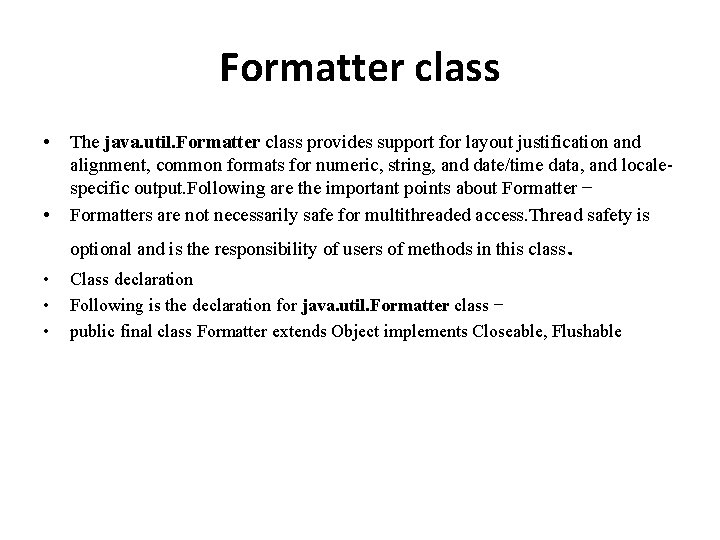
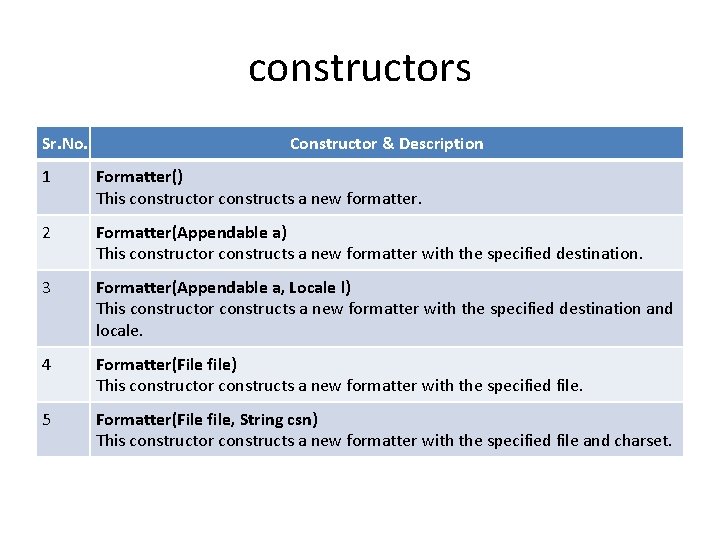
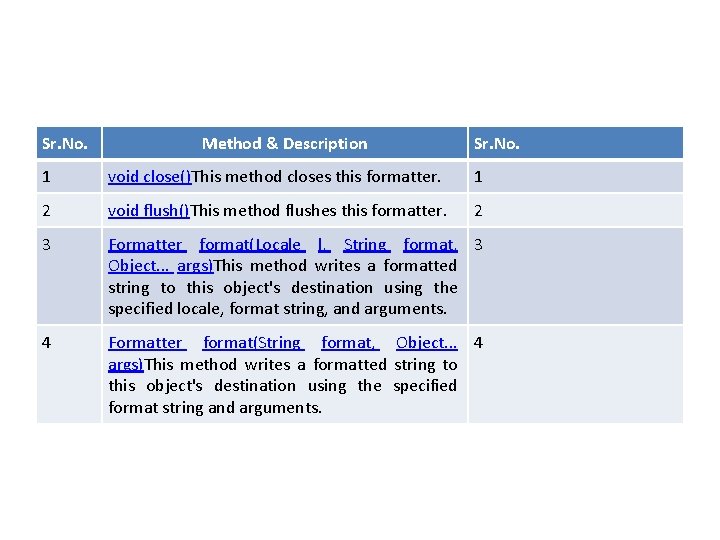
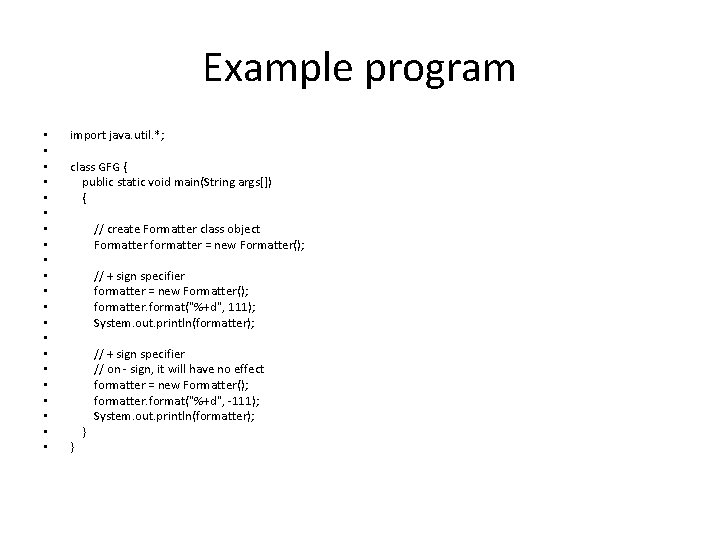
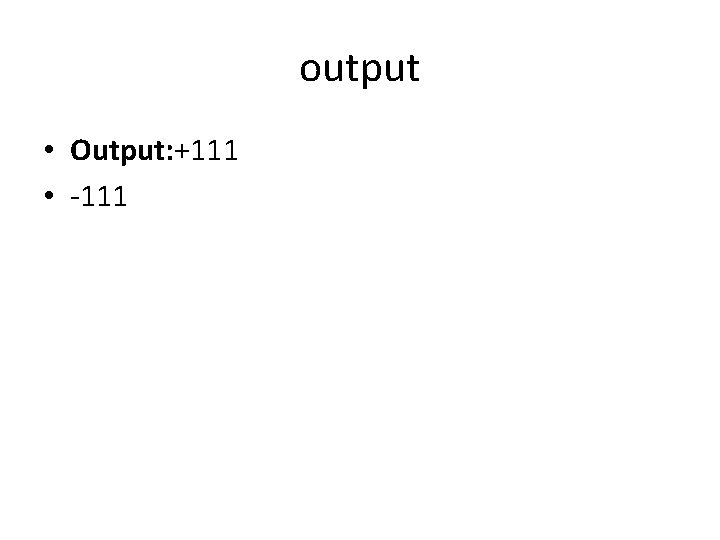
- Slides: 139
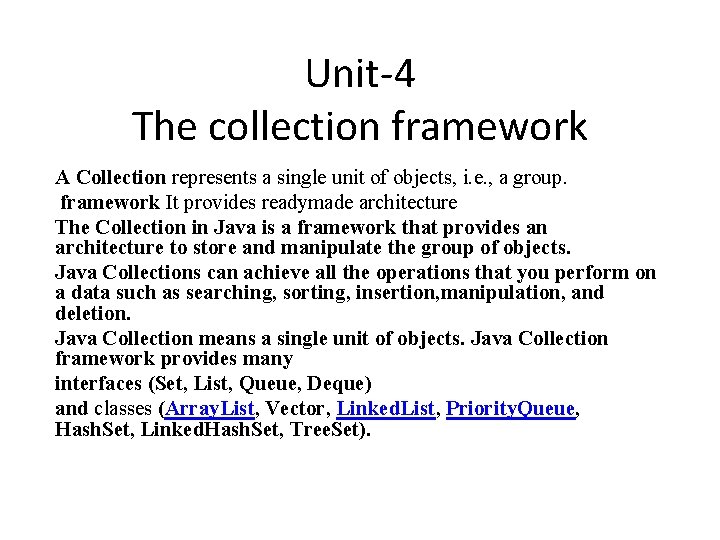
Unit-4 The collection framework A Collection represents a single unit of objects, i. e. , a group. framework It provides readymade architecture The Collection in Java is a framework that provides an architecture to store and manipulate the group of objects. Java Collections can achieve all the operations that you perform on a data such as searching, sorting, insertion, manipulation, and deletion. Java Collection means a single unit of objects. Java Collection framework provides many interfaces (Set, List, Queue, Deque) and classes (Array. List, Vector, Linked. List, Priority. Queue, Hash. Set, Linked. Hash. Set, Tree. Set).
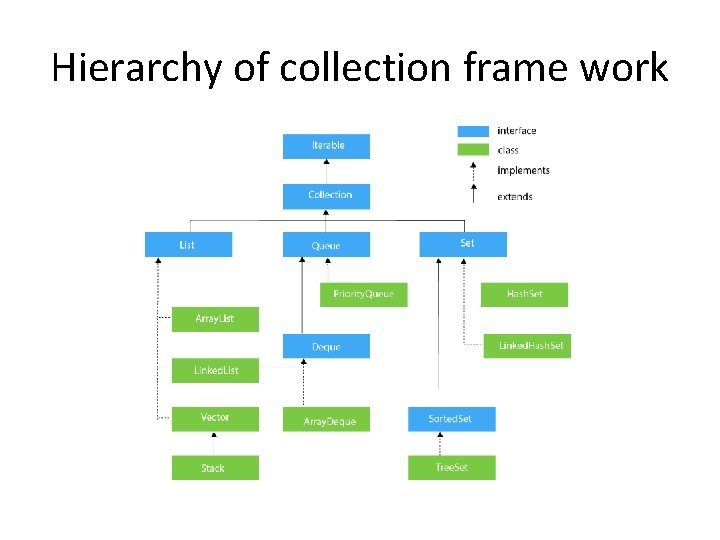
Hierarchy of collection frame work
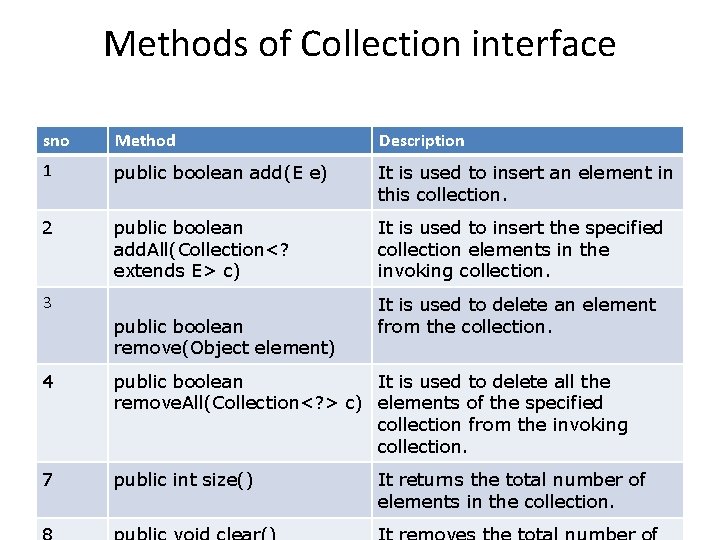
Methods of Collection interface sno Method Description 1 public boolean add(E e) It is used to insert an element in this collection. 2 public boolean add. All(Collection<? extends E> c) It is used to insert the specified collection elements in the invoking collection. 3 public boolean remove(Object element) It is used to delete an element from the collection. 4 public boolean It is used to delete all the remove. All(Collection<? > c) elements of the specified collection from the invoking collection. 7 public int size() It returns the total number of elements in the collection.
![9 public Iterator iterator It returns an iterator 10 public Object to Array It 9 public Iterator iterator() It returns an iterator. 10 public Object[] to. Array() It](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-4.jpg)
9 public Iterator iterator() It returns an iterator. 10 public Object[] to. Array() It converts collection into array. 11 public boolean is. Empty() It checks if collection is empty. 12 public Iterator iterator() It returns an iterator. public int hash. Code() It returns the hash code number of the collection. 13
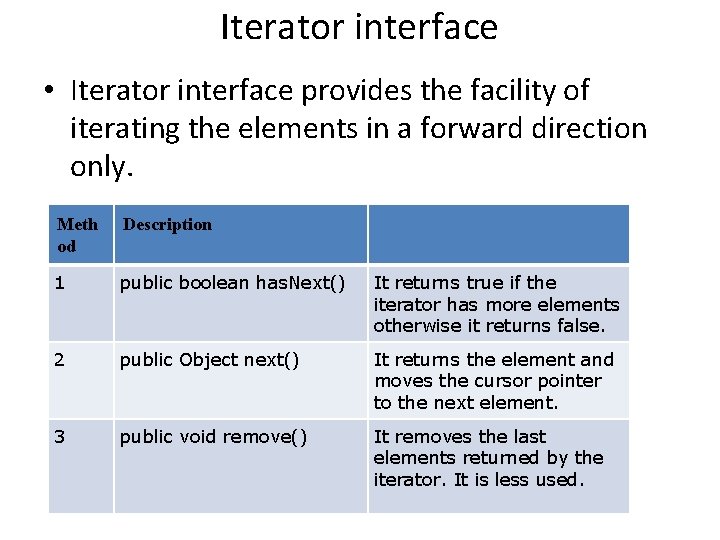
Iterator interface • Iterator interface provides the facility of iterating the elements in a forward direction only. Meth od Description 1 public boolean has. Next() It returns true if the iterator has more elements otherwise it returns false. 2 public Object next() It returns the element and moves the cursor pointer to the next element. 3 public void remove() It removes the last elements returned by the iterator. It is less used.
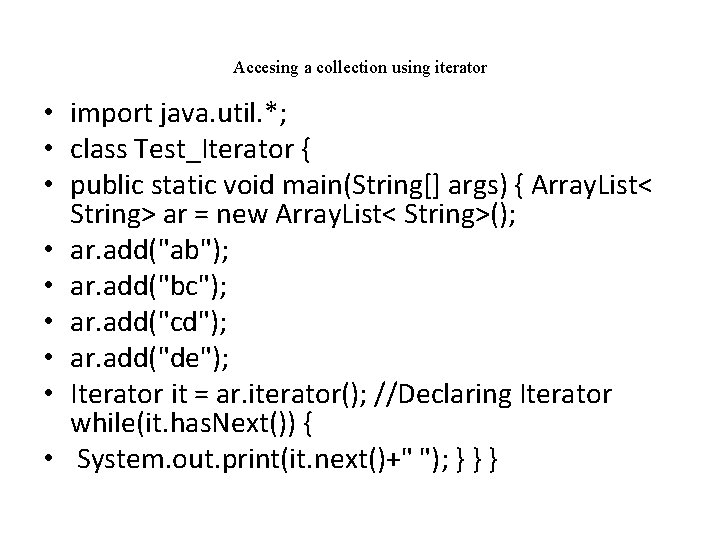
Accesing a collection using iterator • import java. util. *; • class Test_Iterator { • public static void main(String[] args) { Array. List< String> ar = new Array. List< String>(); • ar. add("ab"); • ar. add("bc"); • ar. add("cd"); • ar. add("de"); • Iterator it = ar. iterator(); //Declaring Iterator while(it. has. Next()) { • System. out. print(it. next()+" "); } } }
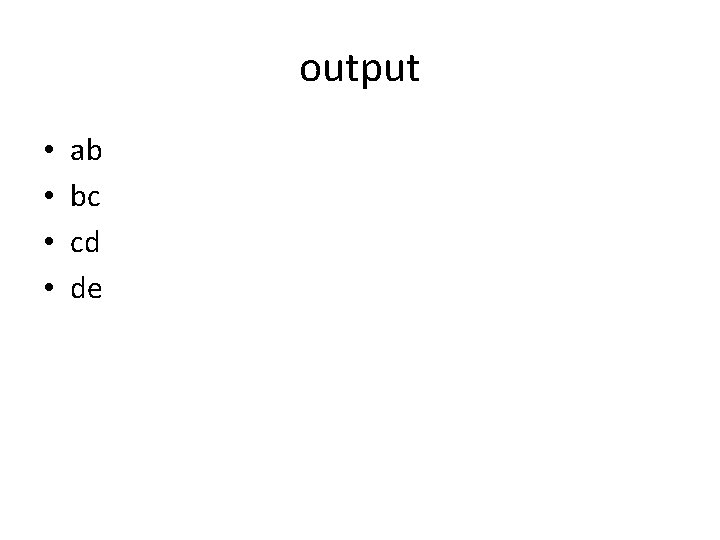
output • • ab bc cd de
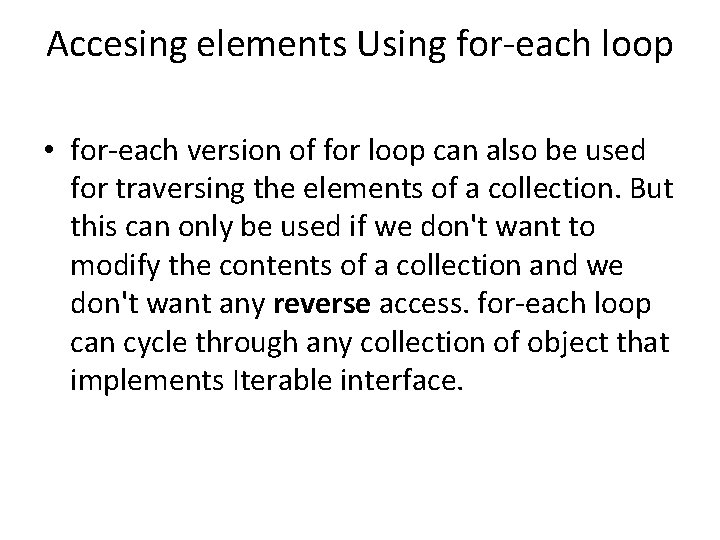
Accesing elements Using for-each loop • for-each version of for loop can also be used for traversing the elements of a collection. But this can only be used if we don't want to modify the contents of a collection and we don't want any reverse access. for-each loop can cycle through any collection of object that implements Iterable interface.
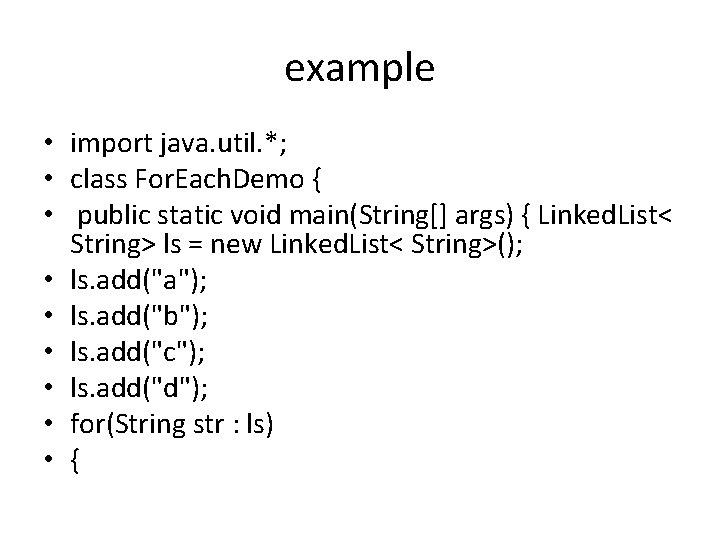
example • import java. util. *; • class For. Each. Demo { • public static void main(String[] args) { Linked. List< String> ls = new Linked. List< String>(); • ls. add("a"); • ls. add("b"); • ls. add("c"); • ls. add("d"); • for(String str : ls) • {
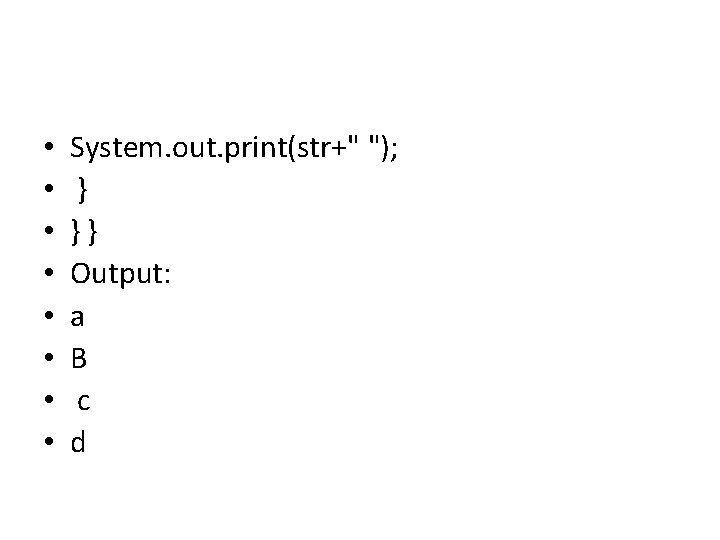
• • System. out. print(str+" "); } } } Output: a B c d
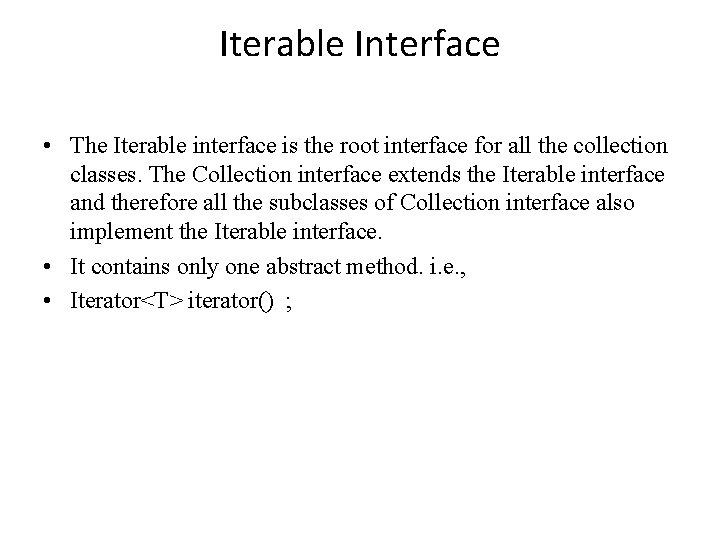
Iterable Interface • The Iterable interface is the root interface for all the collection classes. The Collection interface extends the Iterable interface and therefore all the subclasses of Collection interface also implement the Iterable interface. • It contains only one abstract method. i. e. , • Iterator<T> iterator() ;
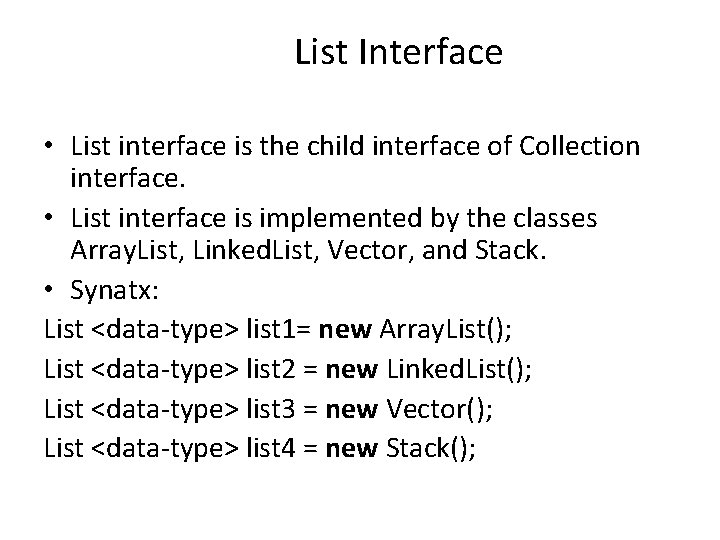
List Interface • List interface is the child interface of Collection interface. • List interface is implemented by the classes Array. List, Linked. List, Vector, and Stack. • Synatx: List <data-type> list 1= new Array. List(); List <data-type> list 2 = new Linked. List(); List <data-type> list 3 = new Vector(); List <data-type> list 4 = new Stack();
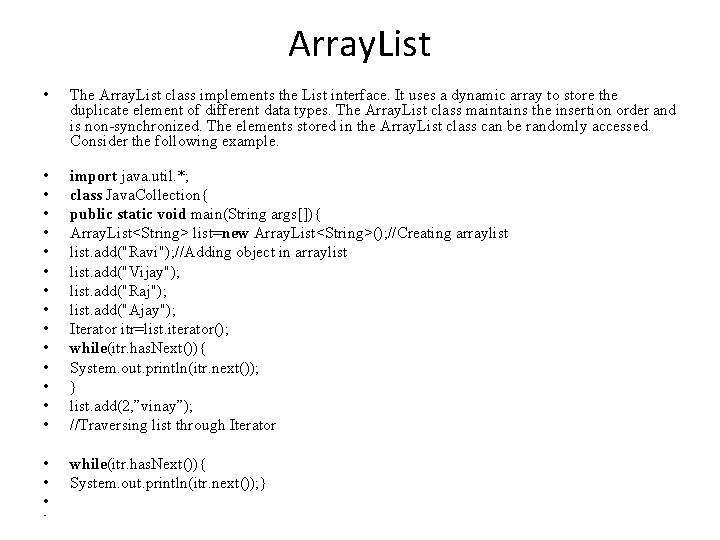
Array. List • The Array. List class implements the List interface. It uses a dynamic array to store the duplicate element of different data types. The Array. List class maintains the insertion order and is non-synchronized. The elements stored in the Array. List class can be randomly accessed. Consider the following example. • • • • import java. util. *; class Java. Collection{ public static void main(String args[]){ Array. List<String> list=new Array. List<String>(); //Creating arraylist list. add("Ravi"); //Adding object in arraylist list. add("Vijay"); list. add("Raj"); list. add("Ajay"); Iterator itr=list. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } list. add(2, ”vinay”); //Traversing list through Iterator • • • while(itr. has. Next()){ System. out. println(itr. next()); } •
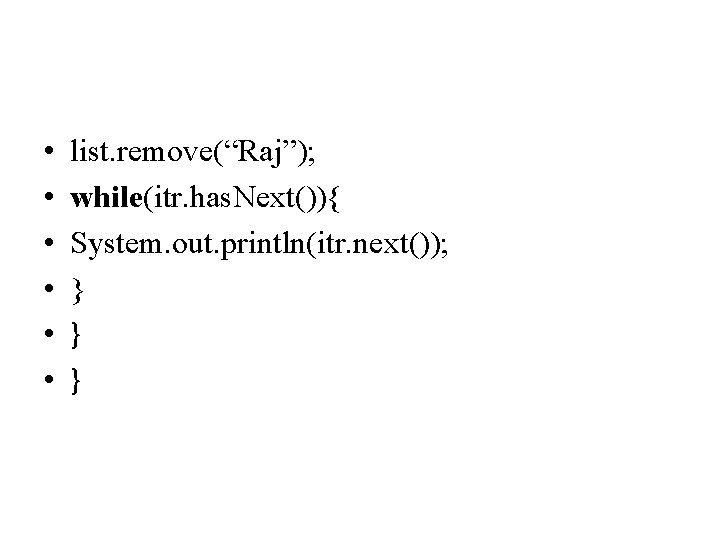
• • • list. remove(“Raj”); while(itr. has. Next()){ System. out. println(itr. next()); } } }
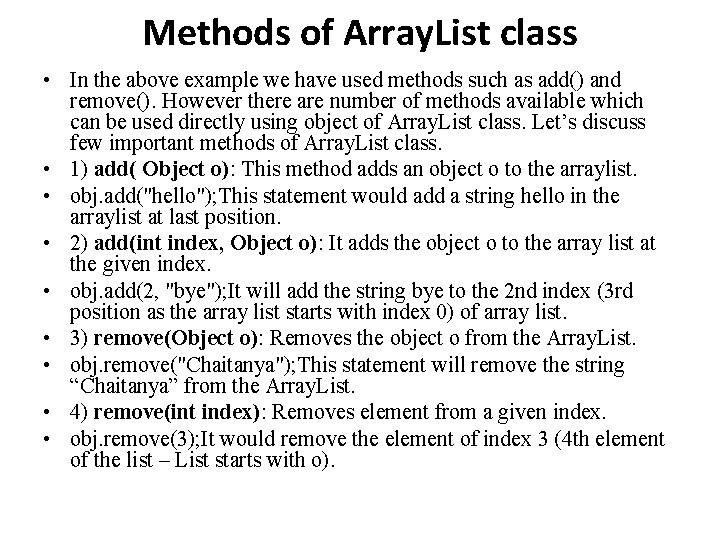
Methods of Array. List class • In the above example we have used methods such as add() and remove(). However there are number of methods available which can be used directly using object of Array. List class. Let’s discuss few important methods of Array. List class. • 1) add( Object o): This method adds an object o to the arraylist. • obj. add("hello"); This statement would add a string hello in the arraylist at last position. • 2) add(int index, Object o): It adds the object o to the array list at the given index. • obj. add(2, "bye"); It will add the string bye to the 2 nd index (3 rd position as the array list starts with index 0) of array list. • 3) remove(Object o): Removes the object o from the Array. List. • obj. remove("Chaitanya"); This statement will remove the string “Chaitanya” from the Array. List. • 4) remove(int index): Removes element from a given index. • obj. remove(3); It would remove the element of index 3 (4 th element of the list – List starts with o).
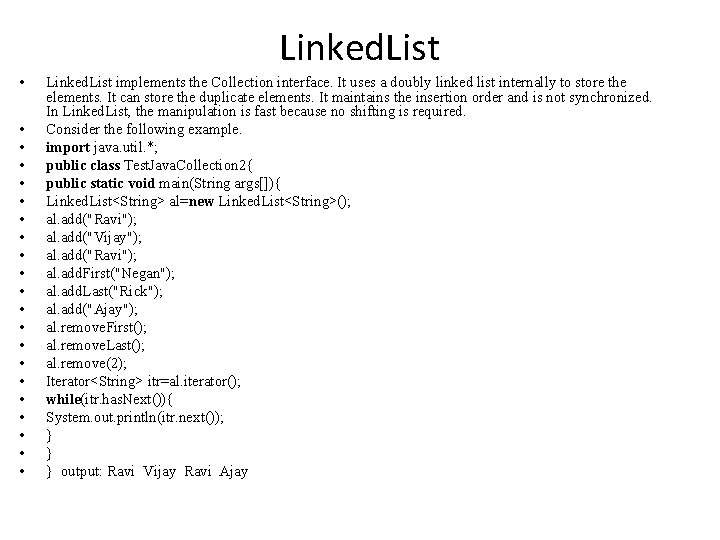
Linked. List • • • • • • Linked. List implements the Collection interface. It uses a doubly linked list internally to store the elements. It can store the duplicate elements. It maintains the insertion order and is not synchronized. In Linked. List, the manipulation is fast because no shifting is required. Consider the following example. import java. util. *; public class Test. Java. Collection 2{ public static void main(String args[]){ Linked. List<String> al=new Linked. List<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ravi"); al. add. First("Negan"); al. add. Last("Rick"); al. add("Ajay"); al. remove. First(); al. remove. Last(); al. remove(2); Iterator<String> itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } output: Ravi Vijay Ravi Ajay
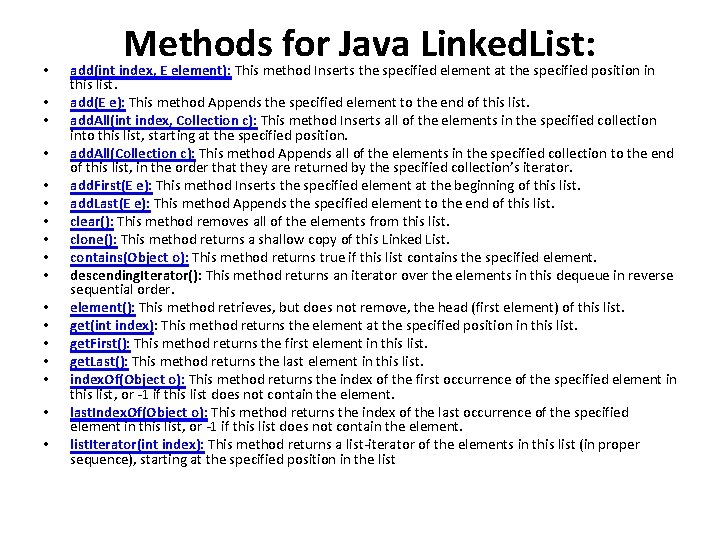
• • • • • Methods for Java Linked. List: add(int index, E element): This method Inserts the specified element at the specified position in this list. add(E e): This method Appends the specified element to the end of this list. add. All(int index, Collection c): This method Inserts all of the elements in the specified collection into this list, starting at the specified position. add. All(Collection c): This method Appends all of the elements in the specified collection to the end of this list, in the order that they are returned by the specified collection’s iterator. add. First(E e): This method Inserts the specified element at the beginning of this list. add. Last(E e): This method Appends the specified element to the end of this list. clear(): This method removes all of the elements from this list. clone(): This method returns a shallow copy of this Linked List. contains(Object o): This method returns true if this list contains the specified element. descending. Iterator(): This method returns an iterator over the elements in this dequeue in reverse sequential order. element(): This method retrieves, but does not remove, the head (first element) of this list. get(int index): This method returns the element at the specified position in this list. get. First(): This method returns the first element in this list. get. Last(): This method returns the last element in this list. index. Of(Object o): This method returns the index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element. last. Index. Of(Object o): This method returns the index of the last occurrence of the specified element in this list, or -1 if this list does not contain the element. list. Iterator(int index): This method returns a list-iterator of the elements in this list (in proper sequence), starting at the specified position in the list
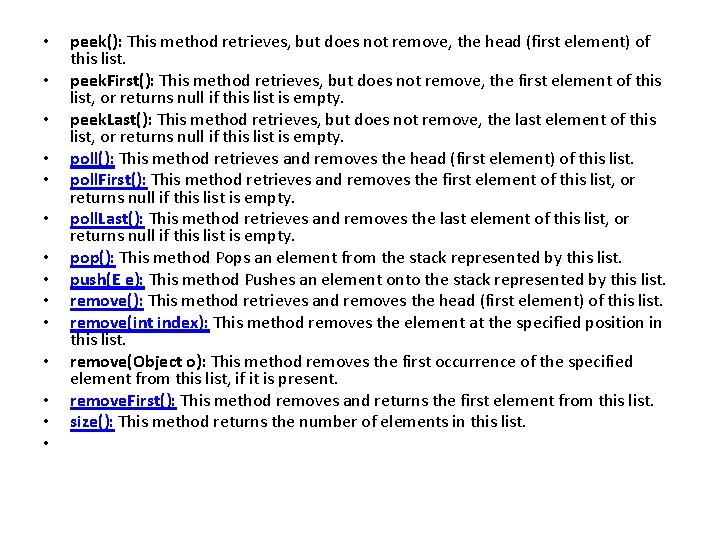
• • • • peek(): This method retrieves, but does not remove, the head (first element) of this list. peek. First(): This method retrieves, but does not remove, the first element of this list, or returns null if this list is empty. peek. Last(): This method retrieves, but does not remove, the last element of this list, or returns null if this list is empty. poll(): This method retrieves and removes the head (first element) of this list. poll. First(): This method retrieves and removes the first element of this list, or returns null if this list is empty. poll. Last(): This method retrieves and removes the last element of this list, or returns null if this list is empty. pop(): This method Pops an element from the stack represented by this list. push(E e): This method Pushes an element onto the stack represented by this list. remove(): This method retrieves and removes the head (first element) of this list. remove(int index): This method removes the element at the specified position in this list. remove(Object o): This method removes the first occurrence of the specified element from this list, if it is present. remove. First(): This method removes and returns the first element from this list. size(): This method returns the number of elements in this list.
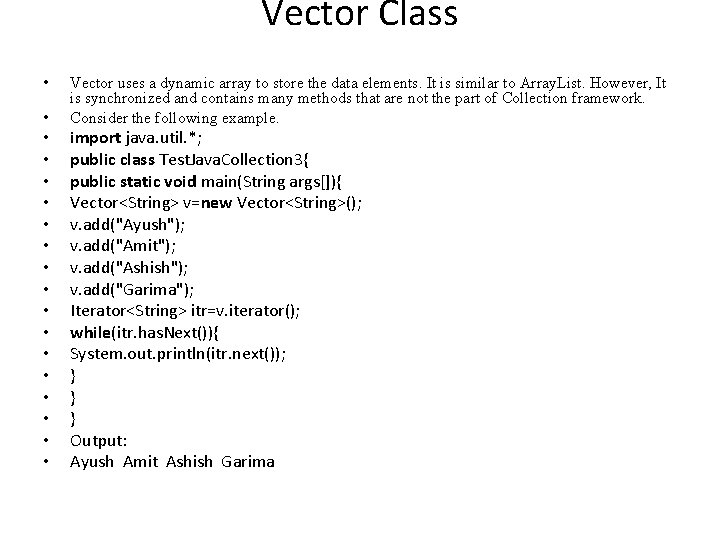
Vector Class • • Vector uses a dynamic array to store the data elements. It is similar to Array. List. However, It is synchronized and contains many methods that are not the part of Collection framework. Consider the following example. • • • • import java. util. *; public class Test. Java. Collection 3{ public static void main(String args[]){ Vector<String> v=new Vector<String>(); v. add("Ayush"); v. add("Amit"); v. add("Ashish"); v. add("Garima"); Iterator<String> itr=v. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } Output: Ayush Amit Ashish Garima
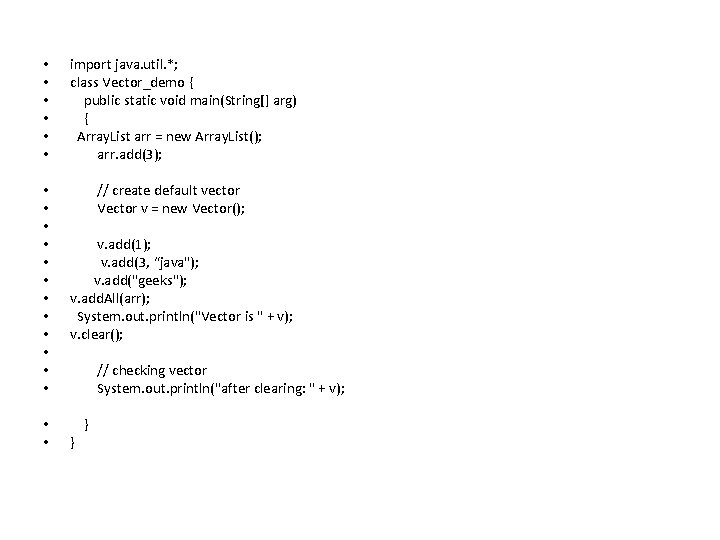
• • • import java. util. *; class Vector_demo { public static void main(String[] arg) { Array. List arr = new Array. List(); arr. add(3); • • • // create default vector Vector v = new Vector(); v. add(1); v. add(3, “java"); v. add("geeks"); v. add. All(arr); System. out. println("Vector is " + v); v. clear(); // checking vector System. out. println("after clearing: " + v); • • } }
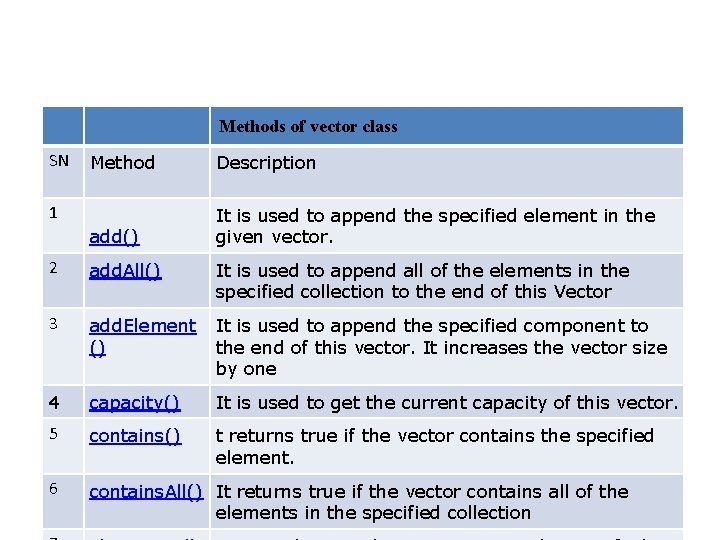
Methods of vector class SN Method Description add() It is used to append the specified element in the given vector. 1 2 add. All() It is used to append all of the elements in the specified collection to the end of this Vector 3 add. Element () It is used to append the specified component to the end of this vector. It increases the vector size by one 4 capacity() It is used to get the current capacity of this vector. 5 contains() t returns true if the vector contains the specified element. 6 contains. All() It returns true if the vector contains all of the elements in the specified collection
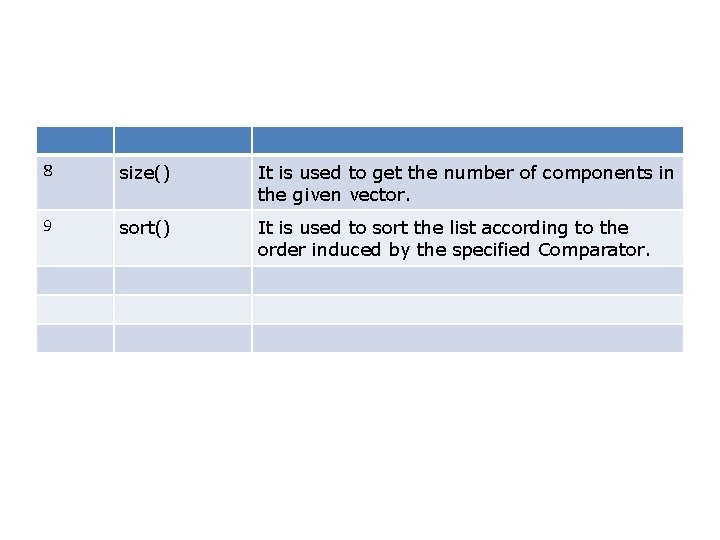
8 size() It is used to get the number of components in the given vector. 9 sort() It is used to sort the list according to the order induced by the specified Comparator.
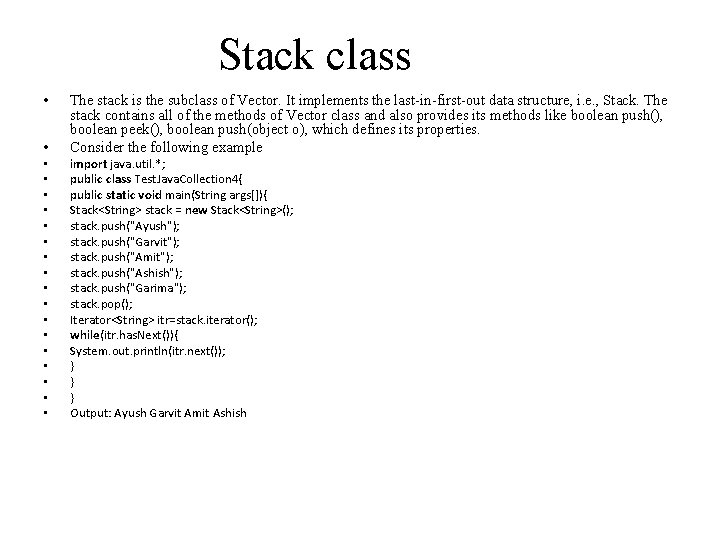
Stack class • • The stack is the subclass of Vector. It implements the last-in-first-out data structure, i. e. , Stack. The stack contains all of the methods of Vector class and also provides its methods like boolean push(), boolean peek(), boolean push(object o), which defines its properties. Consider the following example • • • • • import java. util. *; public class Test. Java. Collection 4{ public static void main(String args[]){ Stack<String> stack = new Stack<String>(); stack. push("Ayush"); stack. push("Garvit"); stack. push("Amit"); stack. push("Ashish"); stack. push("Garima"); stack. pop(); Iterator<String> itr=stack. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } Output: Ayush Garvit Amit Ashish
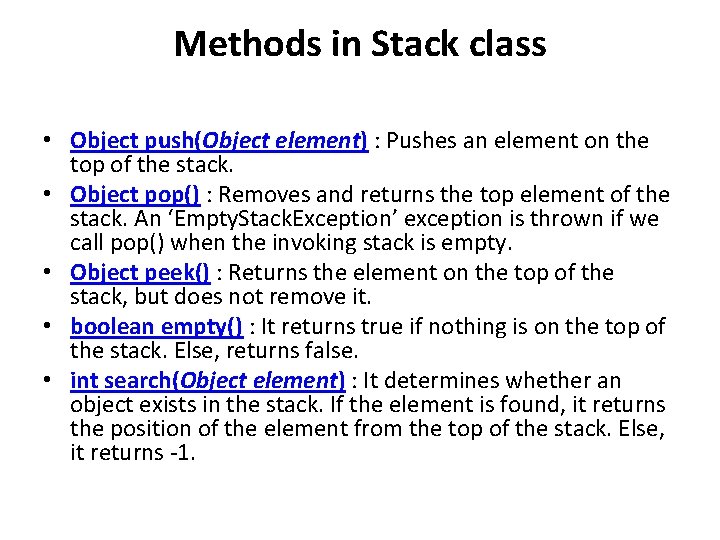
Methods in Stack class • Object push(Object element) : Pushes an element on the top of the stack. • Object pop() : Removes and returns the top element of the stack. An ‘Empty. Stack. Exception’ exception is thrown if we call pop() when the invoking stack is empty. • Object peek() : Returns the element on the top of the stack, but does not remove it. • boolean empty() : It returns true if nothing is on the top of the stack. Else, returns false. • int search(Object element) : It determines whether an object exists in the stack. If the element is found, it returns the position of the element from the top of the stack. Else, it returns -1.
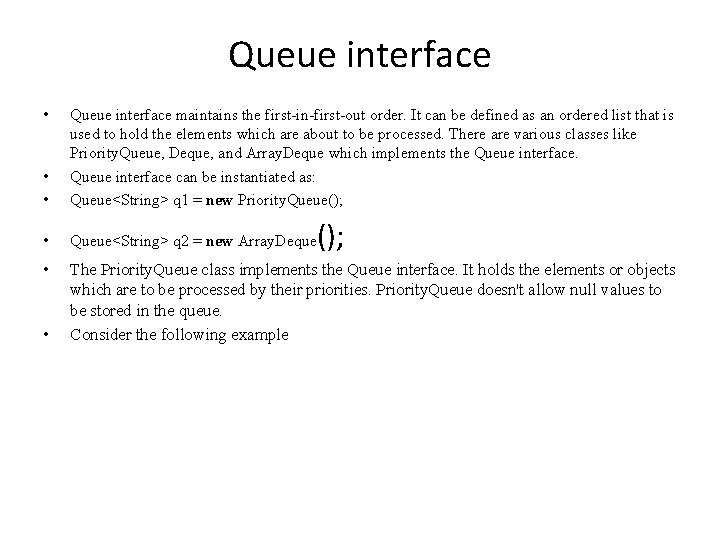
Queue interface • • • Queue interface maintains the first-in-first-out order. It can be defined as an ordered list that is used to hold the elements which are about to be processed. There are various classes like Priority. Queue, Deque, and Array. Deque which implements the Queue interface can be instantiated as: Queue<String> q 1 = new Priority. Queue(); • Queue<String> q 2 = new Array. Deque • The Priority. Queue class implements the Queue interface. It holds the elements or objects which are to be processed by their priorities. Priority. Queue doesn't allow null values to be stored in the queue. Consider the following example • ();
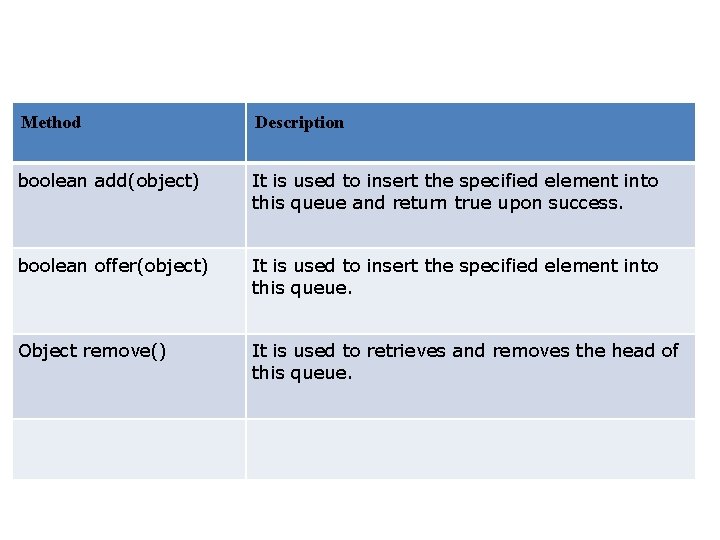
Method Description boolean add(object) It is used to insert the specified element into this queue and return true upon success. boolean offer(object) It is used to insert the specified element into this queue. Object remove() It is used to retrieves and removes the head of this queue.
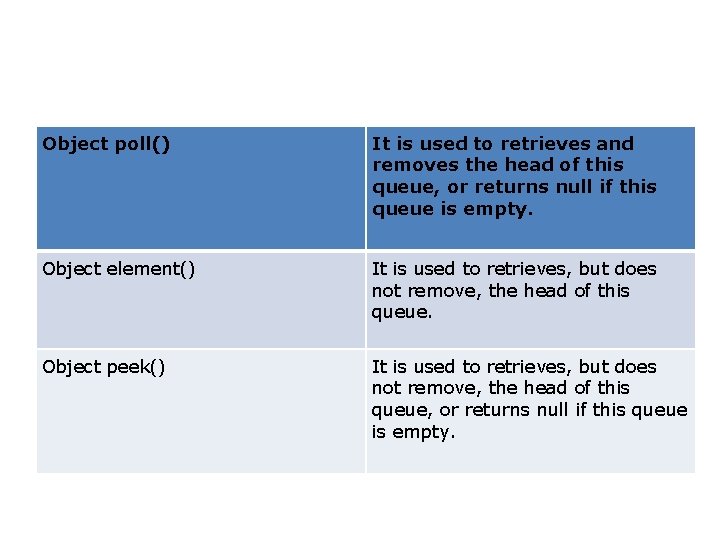
Object poll() It is used to retrieves and removes the head of this queue, or returns null if this queue is empty. Object element() It is used to retrieves, but does not remove, the head of this queue. Object peek() It is used to retrieves, but does not remove, the head of this queue, or returns null if this queue is empty.
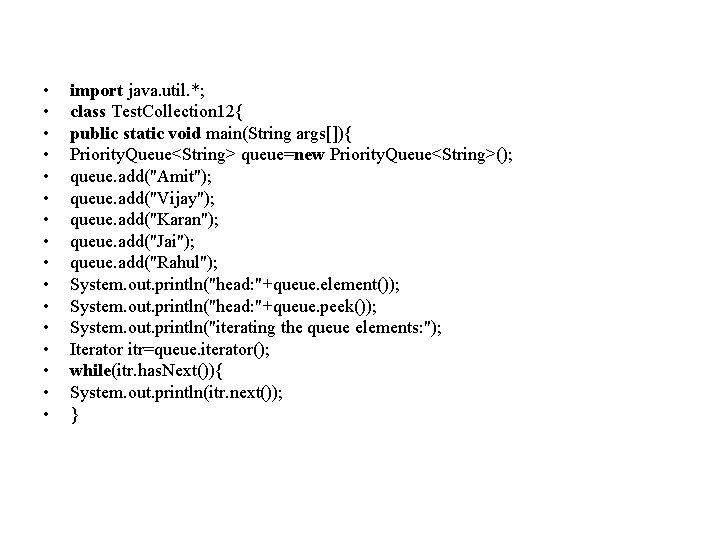
• • • • import java. util. *; class Test. Collection 12{ public static void main(String args[]){ Priority. Queue<String> queue=new Priority. Queue<String>(); queue. add("Amit"); queue. add("Vijay"); queue. add("Karan"); queue. add("Jai"); queue. add("Rahul"); System. out. println("head: "+queue. element()); System. out. println("head: "+queue. peek()); System. out. println("iterating the queue elements: "); Iterator itr=queue. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); }
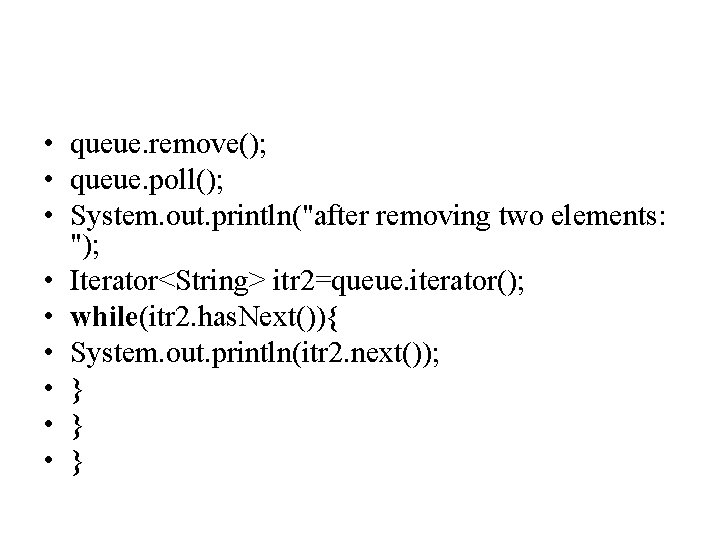
• queue. remove(); • queue. poll(); • System. out. println("after removing two elements: "); • Iterator<String> itr 2=queue. iterator(); • while(itr 2. has. Next()){ • System. out. println(itr 2. next()); • }
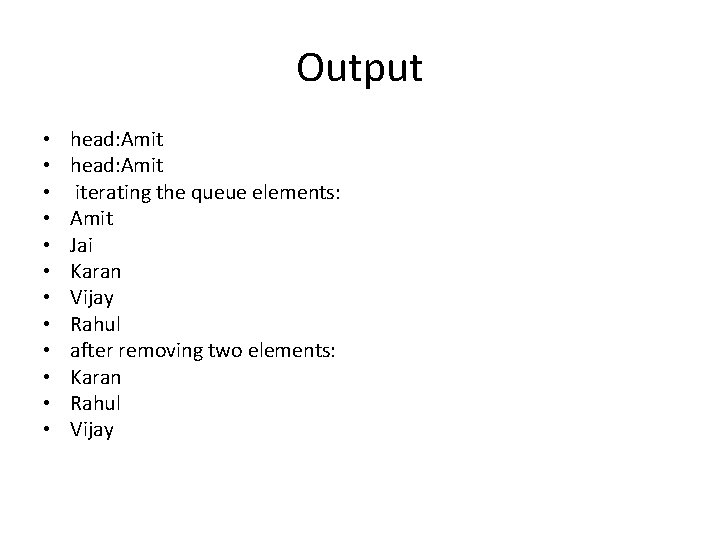
Output • • • head: Amit iterating the queue elements: Amit Jai Karan Vijay Rahul after removing two elements: Karan Rahul Vijay
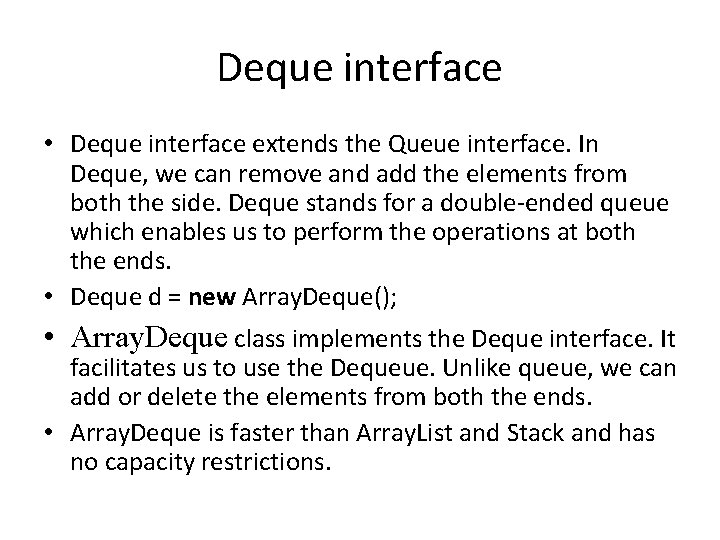
Deque interface • Deque interface extends the Queue interface. In Deque, we can remove and add the elements from both the side. Deque stands for a double-ended queue which enables us to perform the operations at both the ends. • Deque d = new Array. Deque(); • Array. Deque class implements the Deque interface. It facilitates us to use the Dequeue. Unlike queue, we can add or delete the elements from both the ends. • Array. Deque is faster than Array. List and Stack and has no capacity restrictions.
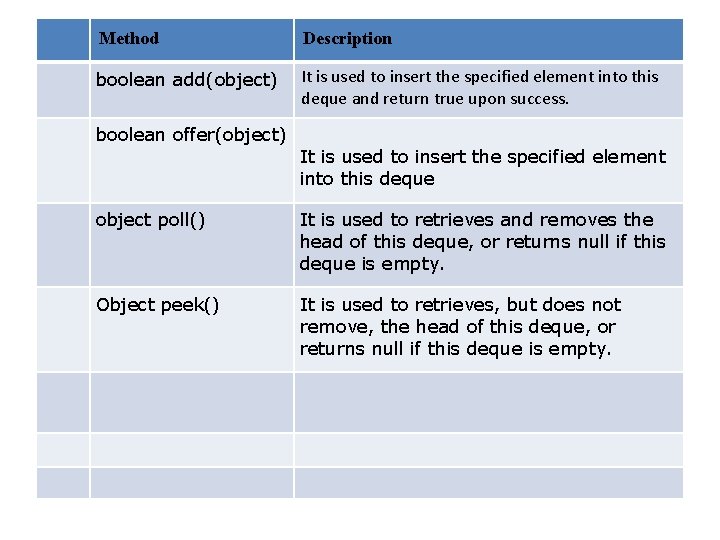
Method Array. Deque methods It is used to insert the specified element into this boolean add(object) boolean offer(object) Description deque and return true upon success. It is used to insert the specified element into this deque object poll() It is used to retrieves and removes the head of this deque, or returns null if this deque is empty. Object peek() It is used to retrieves, but does not remove, the head of this deque, or returns null if this deque is empty.
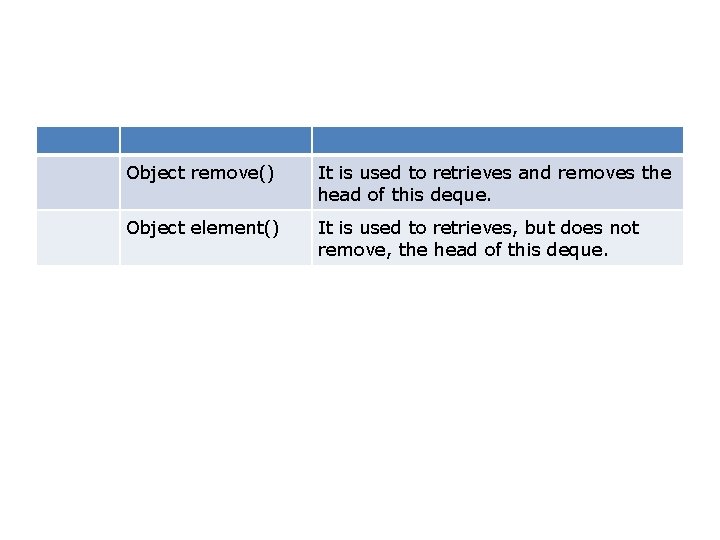
Object remove() It is used to retrieves and removes the head of this deque. Object element() It is used to retrieves, but does not remove, the head of this deque.
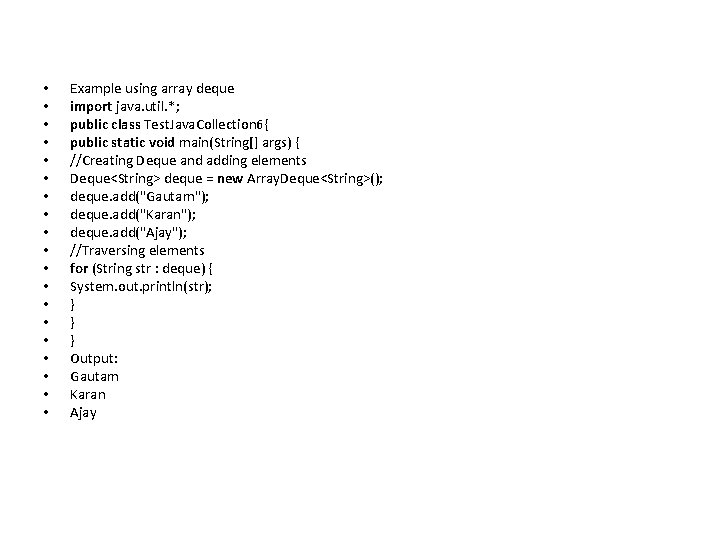
• • • • • Example using array deque import java. util. *; public class Test. Java. Collection 6{ public static void main(String[] args) { //Creating Deque and adding elements Deque<String> deque = new Array. Deque<String>(); deque. add("Gautam"); deque. add("Karan"); deque. add("Ajay"); //Traversing elements for (String str : deque) { System. out. println(str); } } } Output: Gautam Karan Ajay
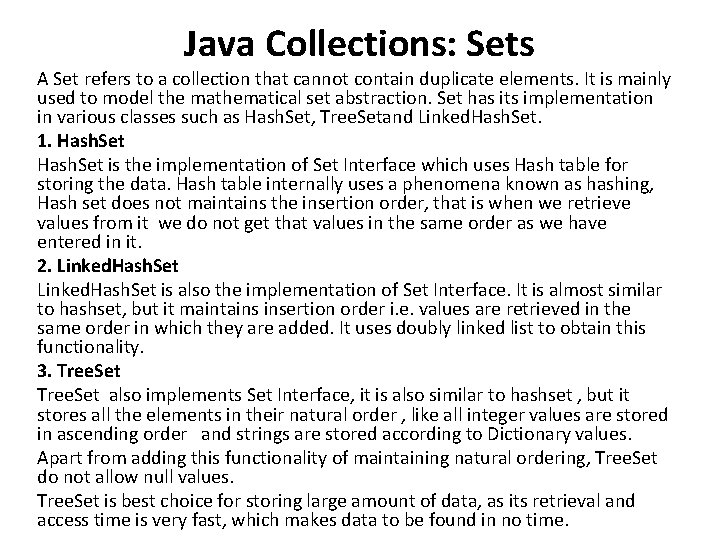
Java Collections: Sets A Set refers to a collection that cannot contain duplicate elements. It is mainly used to model the mathematical set abstraction. Set has its implementation in various classes such as Hash. Set, Tree. Setand Linked. Hash. Set. 1. Hash. Set is the implementation of Set Interface which uses Hash table for storing the data. Hash table internally uses a phenomena known as hashing, Hash set does not maintains the insertion order, that is when we retrieve values from it we do not get that values in the same order as we have entered in it. 2. Linked. Hash. Set is also the implementation of Set Interface. It is almost similar to hashset, but it maintains insertion order i. e. values are retrieved in the same order in which they are added. It uses doubly linked list to obtain this functionality. 3. Tree. Set also implements Set Interface, it is also similar to hashset , but it stores all the elements in their natural order , like all integer values are stored in ascending order and strings are stored according to Dictionary values. Apart from adding this functionality of maintaining natural ordering, Tree. Set do not allow null values. Tree. Set is best choice for storing large amount of data, as its retrieval and access time is very fast, which makes data to be found in no time.
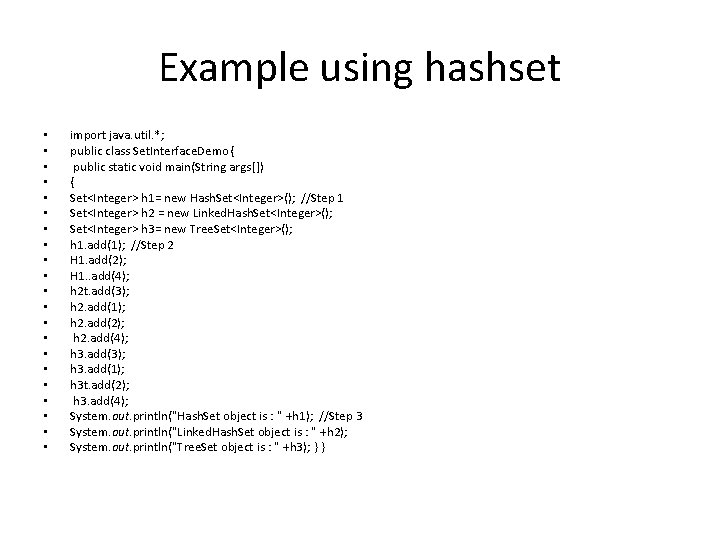
Example using hashset • • • • • • import java. util. *; public class Set. Interface. Demo{ public static void main(String args[]) { Set<Integer> h 1= new Hash. Set<Integer>(); //Step 1 Set<Integer> h 2 = new Linked. Hash. Set<Integer>(); Set<Integer> h 3= new Tree. Set<Integer>(); h 1. add(1); //Step 2 H 1. add(2); H 1. . add(4); h 2 t. add(3); h 2. add(1); h 2. add(2); h 2. add(4); h 3. add(3); h 3. add(1); h 3 t. add(2); h 3. add(4); System. out. println("Hash. Set object is : " +h 1); //Step 3 System. out. println("Linked. Hash. Set object is : " +h 2); System. out. println("Tree. Set object is : " +h 3); } }
![Output Hash Set object is 1 2 4 Linked Hash Set Output: • Hash. Set object is : [1, 2, 4] • Linked. Hash. Set](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-37.jpg)
Output: • Hash. Set object is : [1, 2, 4] • Linked. Hash. Set object is : [3, 1, 2, 4] • Tree. Set object is : [1, 2, 3, 4]
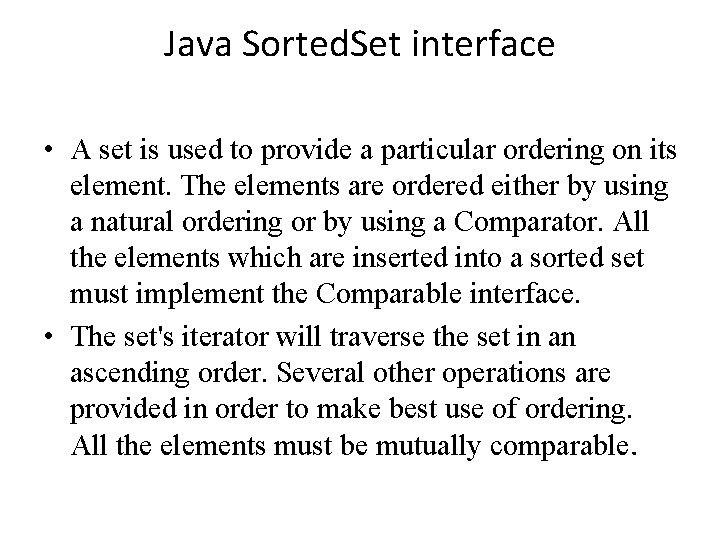
Java Sorted. Set interface • A set is used to provide a particular ordering on its element. The elements are ordered either by using a natural ordering or by using a Comparator. All the elements which are inserted into a sorted set must implement the Comparable interface. • The set's iterator will traverse the set in an ascending order. Several other operations are provided in order to make best use of ordering. All the elements must be mutually comparable.
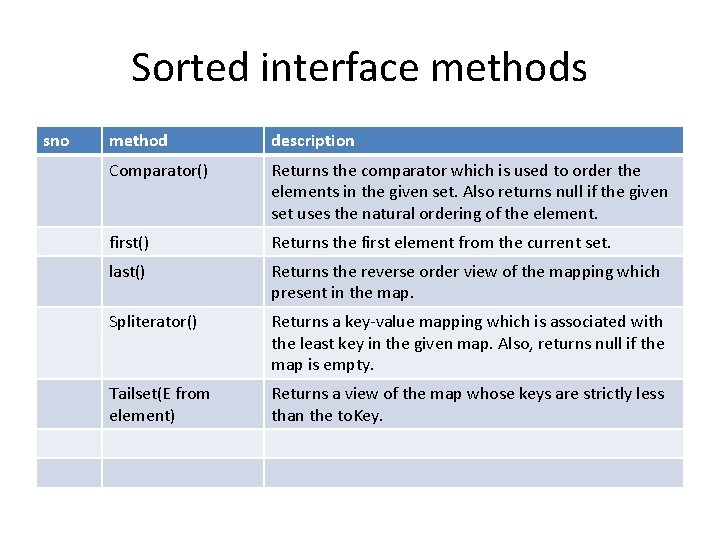
Sorted interface methods sno method description Comparator() Returns the comparator which is used to order the elements in the given set. Also returns null if the given set uses the natural ordering of the element. first() Returns the first element from the current set. last() Returns the reverse order view of the mapping which present in the map. Spliterator() Returns a key-value mapping which is associated with the least key in the given map. Also, returns null if the map is empty. Tailset(E from element) Returns a view of the map whose keys are strictly less than the to. Key.
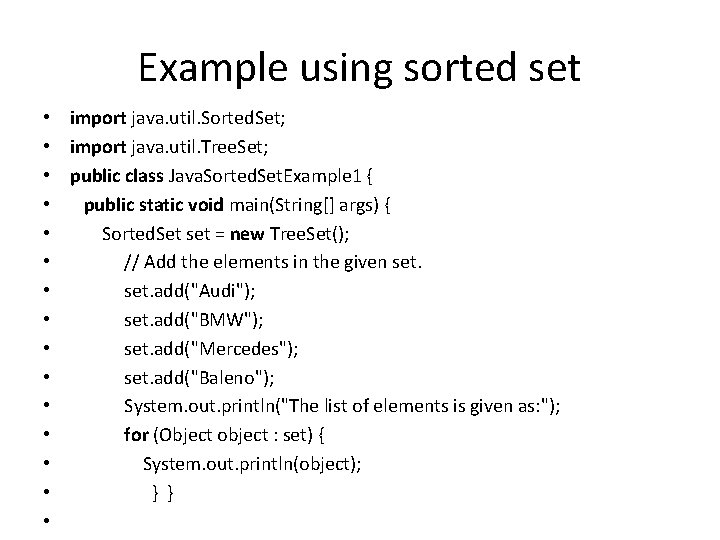
Example using sorted set • • • • import java. util. Sorted. Set; import java. util. Tree. Set; public class Java. Sorted. Set. Example 1 { public static void main(String[] args) { Sorted. Set set = new Tree. Set(); // Add the elements in the given set. set. add("Audi"); set. add("BMW"); set. add("Mercedes"); set. add("Baleno"); System. out. println("The list of elements is given as: "); for (Object object : set) { System. out. println(object); } }
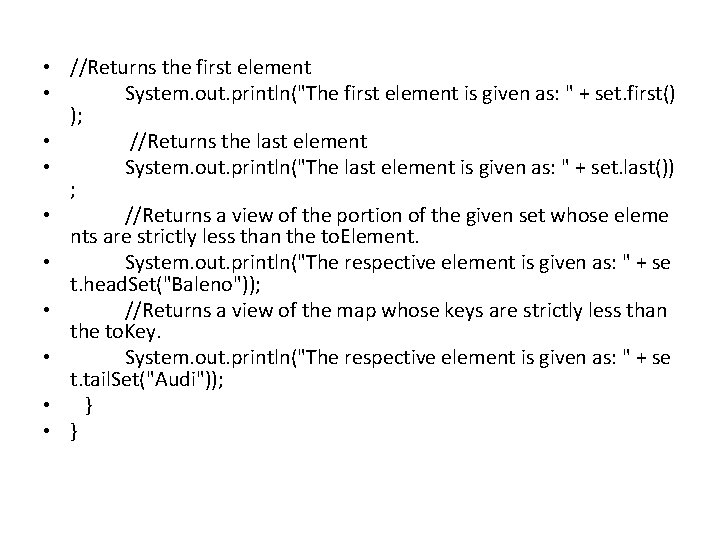
• //Returns the first element • System. out. println("The first element is given as: " + set. first() ); • //Returns the last element • System. out. println("The last element is given as: " + set. last()) ; • //Returns a view of the portion of the given set whose eleme nts are strictly less than the to. Element. • System. out. println("The respective element is given as: " + se t. head. Set("Baleno")); • //Returns a view of the map whose keys are strictly less than the to. Key. • System. out. println("The respective element is given as: " + se t. tail. Set("Audi")); • }
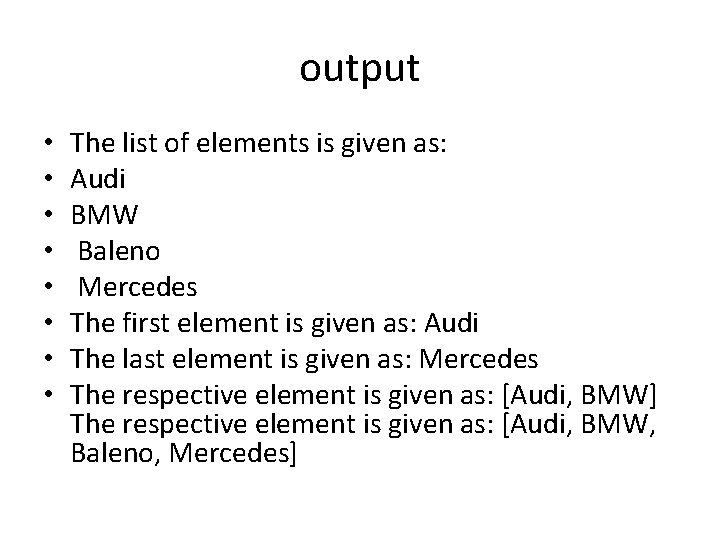
output • • The list of elements is given as: Audi BMW Baleno Mercedes The first element is given as: Audi The last element is given as: Mercedes The respective element is given as: [Audi, BMW] The respective element is given as: [Audi, BMW, Baleno, Mercedes]
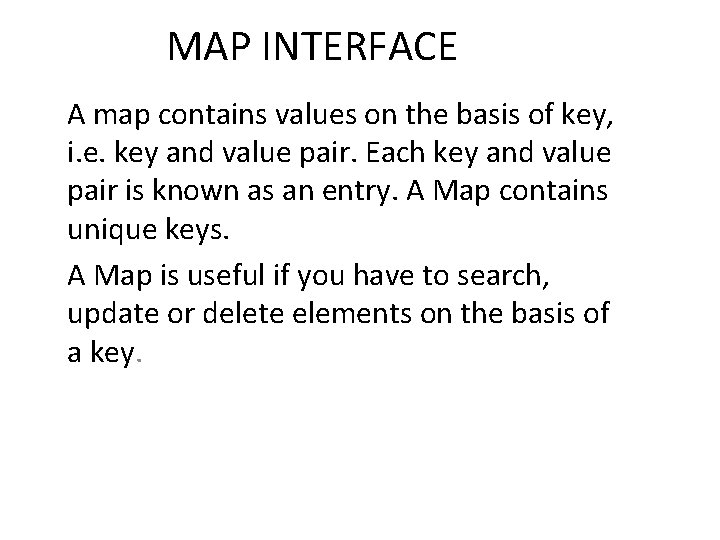
MAP INTERFACE A map contains values on the basis of key, i. e. key and value pair. Each key and value pair is known as an entry. A Map contains unique keys. A Map is useful if you have to search, update or delete elements on the basis of a key.
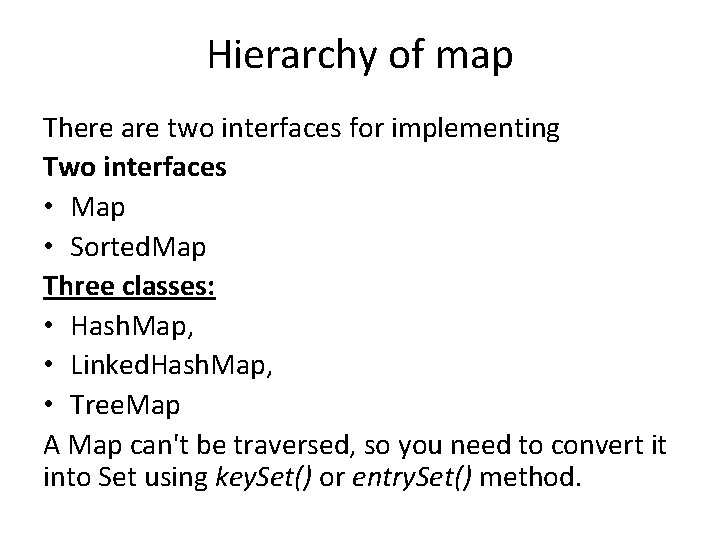
Hierarchy of map There are two interfaces for implementing Two interfaces • Map • Sorted. Map Three classes: • Hash. Map, • Linked. Hash. Map, • Tree. Map A Map can't be traversed, so you need to convert it into Set using key. Set() or entry. Set() method.
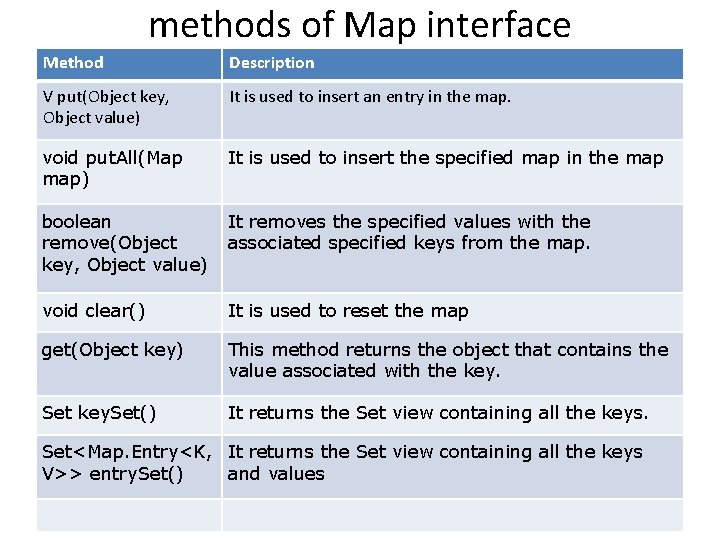
methods of Map interface Method Description V put(Object key, Object value) It is used to insert an entry in the map. void put. All(Map map) It is used to insert the specified map in the map boolean remove(Object key, Object value) It removes the specified values with the associated specified keys from the map. void clear() It is used to reset the map get(Object key) This method returns the object that contains the value associated with the key. Set key. Set() It returns the Set view containing all the keys. Set<Map. Entry<K, It returns the Set view containing all the keys V>> entry. Set() and values
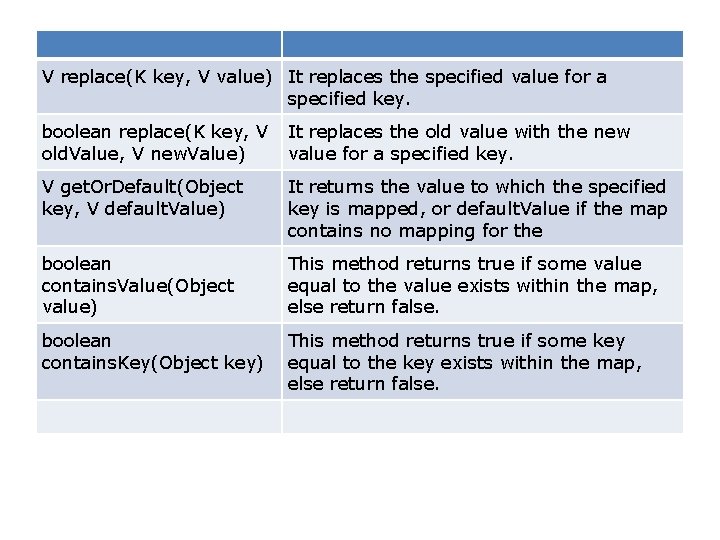
V replace(K key, V value) It replaces the specified value for a specified key. boolean replace(K key, V It replaces the old value with the new old. Value, V new. Value) value for a specified key. V get. Or. Default(Object key, V default. Value) It returns the value to which the specified key is mapped, or default. Value if the map contains no mapping for the boolean contains. Value(Object value) This method returns true if some value equal to the value exists within the map, else return false. boolean contains. Key(Object key) This method returns true if some key equal to the key exists within the map, else return false.
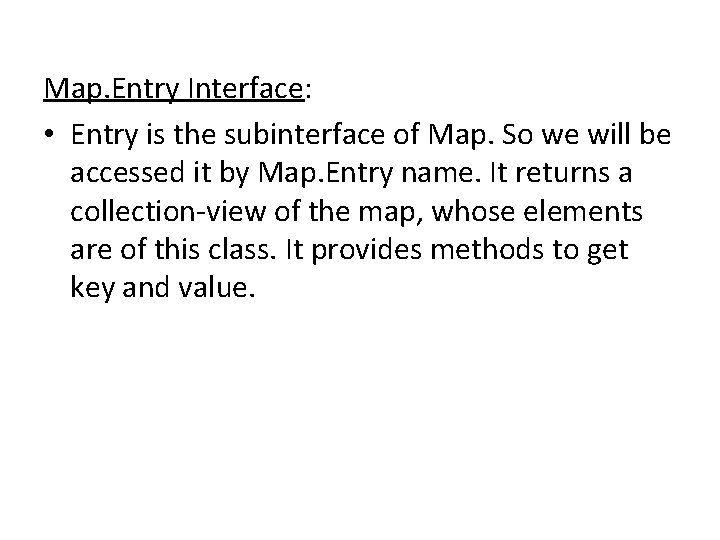
Map. Entry Interface: • Entry is the subinterface of Map. So we will be accessed it by Map. Entry name. It returns a collection-view of the map, whose elements are of this class. It provides methods to get key and value.
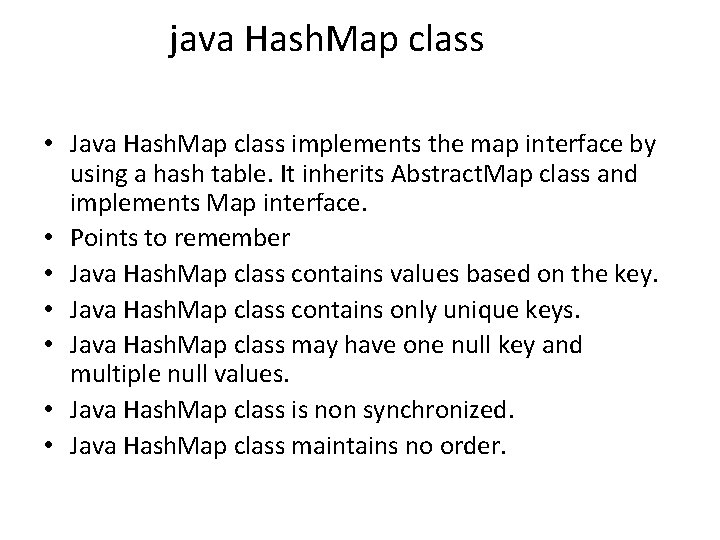
java Hash. Map class • Java Hash. Map class implements the map interface by using a hash table. It inherits Abstract. Map class and implements Map interface. • Points to remember • Java Hash. Map class contains values based on the key. • Java Hash. Map class contains only unique keys. • Java Hash. Map class may have one null key and multiple null values. • Java Hash. Map class is non synchronized. • Java Hash. Map class maintains no order.
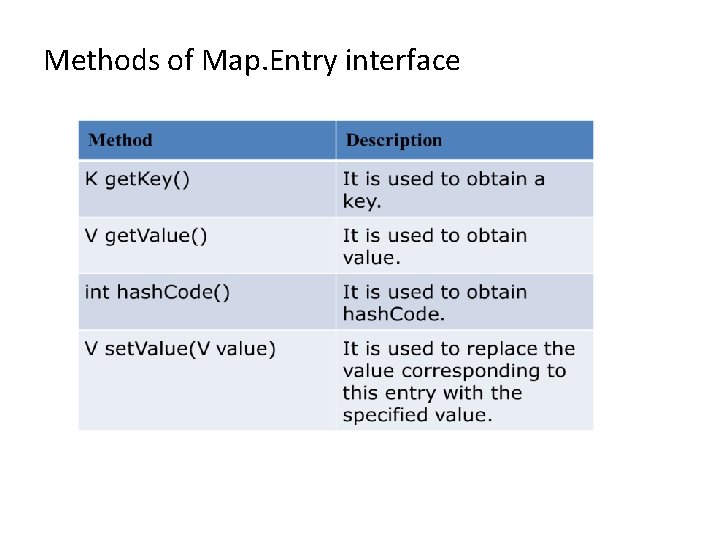
Methods of Map. Entry interface
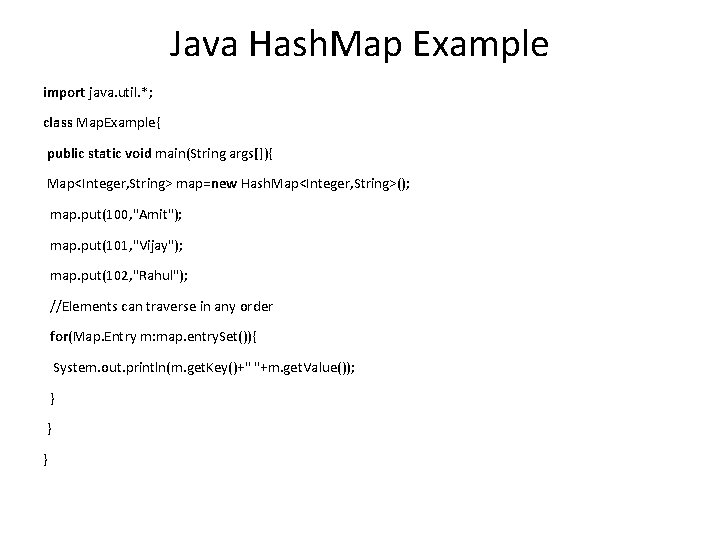
Java Hash. Map Example import java. util. *; class Map. Example{ public static void main(String args[]){ Map<Integer, String> map=new Hash. Map<Integer, String>(); map. put(100, "Amit"); map. put(101, "Vijay"); map. put(102, "Rahul"); //Elements can traverse in any order for(Map. Entry m: map. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); } } }
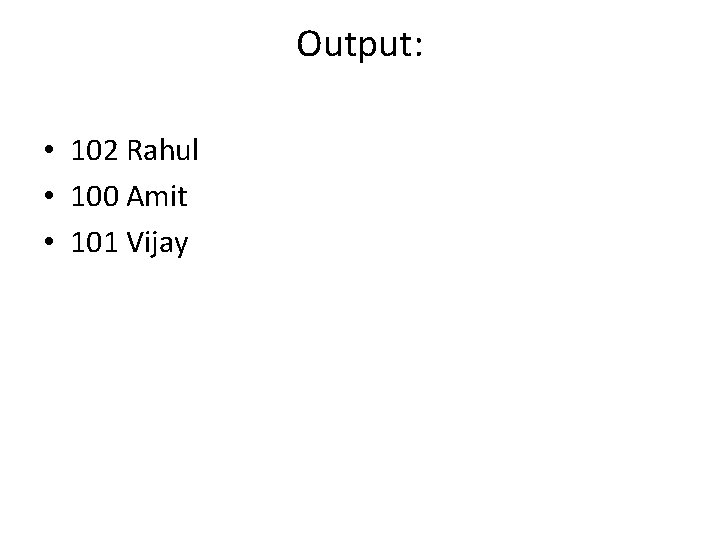
Output: • 102 Rahul • 100 Amit • 101 Vijay
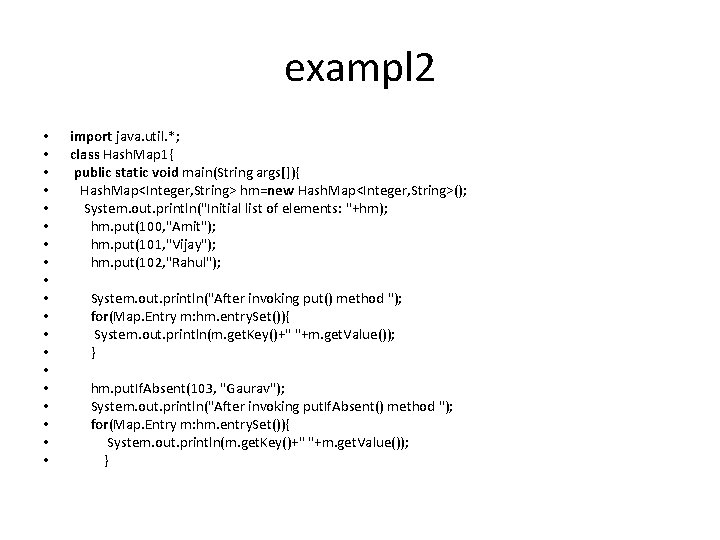
exampl 2 • • • • • import java. util. *; class Hash. Map 1{ public static void main(String args[]){ Hash. Map<Integer, String> hm=new Hash. Map<Integer, String>(); System. out. println("Initial list of elements: "+hm); hm. put(100, "Amit"); hm. put(101, "Vijay"); hm. put(102, "Rahul"); System. out. println("After invoking put() method "); for(Map. Entry m: hm. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); } hm. put. If. Absent(103, "Gaurav"); System. out. println("After invoking put. If. Absent() method "); for(Map. Entry m: hm. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); }
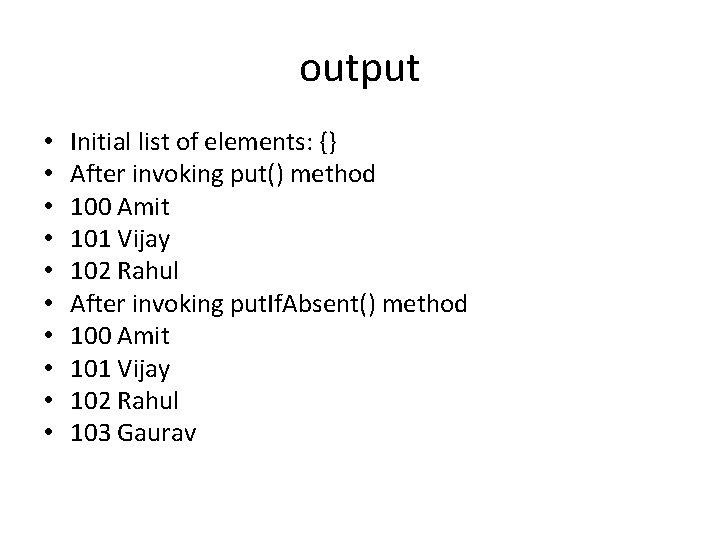
output • • • Initial list of elements: {} After invoking put() method 100 Amit 101 Vijay 102 Rahul After invoking put. If. Absent() method 100 Amit 101 Vijay 102 Rahul 103 Gaurav
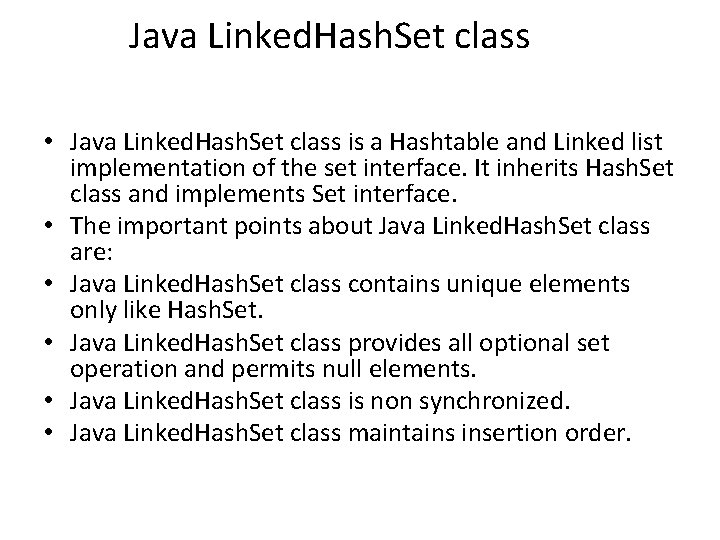
Java Linked. Hash. Set class • Java Linked. Hash. Set class is a Hashtable and Linked list implementation of the set interface. It inherits Hash. Set class and implements Set interface. • The important points about Java Linked. Hash. Set class are: • Java Linked. Hash. Set class contains unique elements only like Hash. Set. • Java Linked. Hash. Set class provides all optional set operation and permits null elements. • Java Linked. Hash. Set class is non synchronized. • Java Linked. Hash. Set class maintains insertion order.
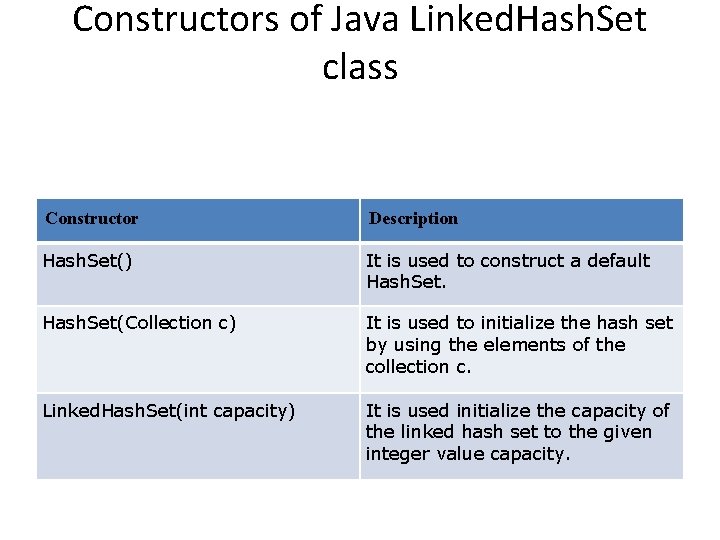
Constructors of Java Linked. Hash. Set class Constructor Description Hash. Set() It is used to construct a default Hash. Set(Collection c) It is used to initialize the hash set by using the elements of the collection c. Linked. Hash. Set(int capacity) It is used initialize the capacity of the linked hash set to the given integer value capacity.
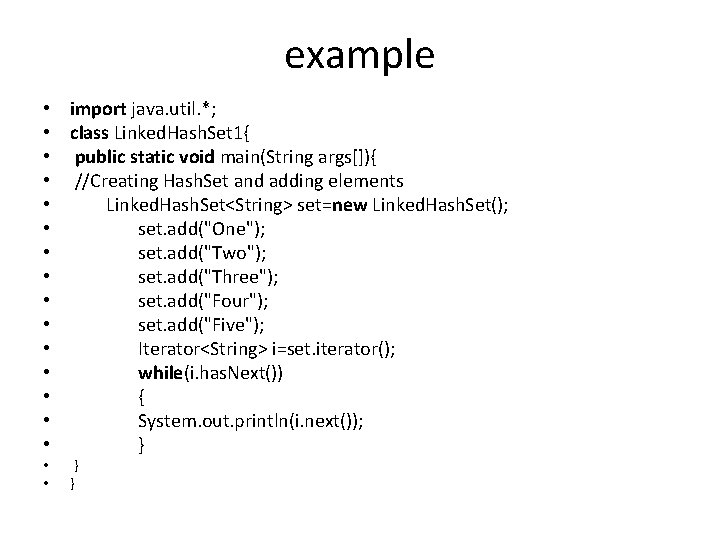
example • • • • • import java. util. *; class Linked. Hash. Set 1{ public static void main(String args[]){ //Creating Hash. Set and adding elements Linked. Hash. Set<String> set=new Linked. Hash. Set(); set. add("One"); set. add("Two"); set. add("Three"); set. add("Four"); set. add("Five"); Iterator<String> i=set. iterator(); while(i. has. Next()) { System. out. println(i. next()); } } }
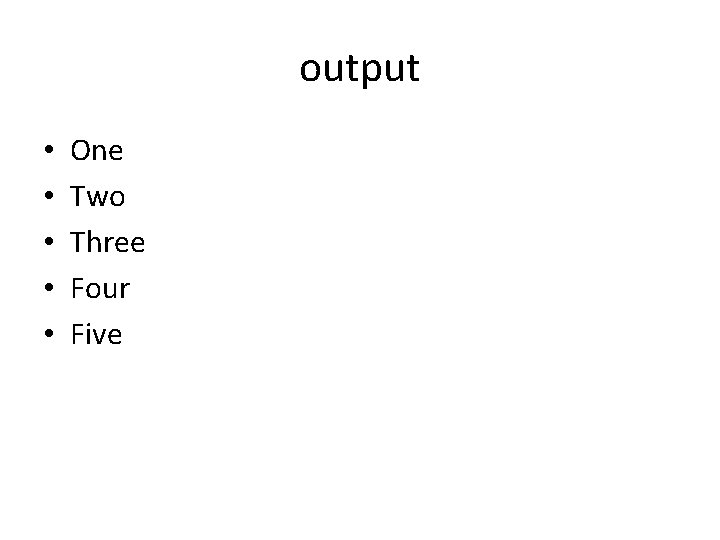
output • • • One Two Three Four Five
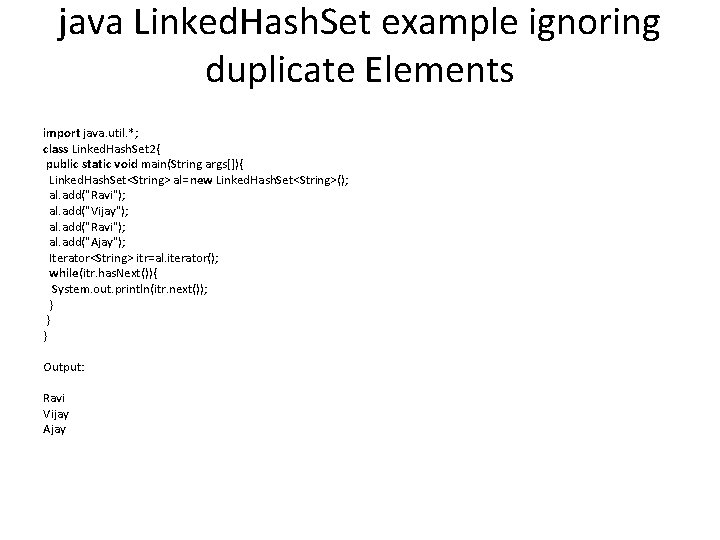
java Linked. Hash. Set example ignoring duplicate Elements import java. util. *; class Linked. Hash. Set 2{ public static void main(String args[]){ Linked. Hash. Set<String> al=new Linked. Hash. Set<String>(); al. add("Ravi"); al. add("Vijay"); al. add("Ravi"); al. add("Ajay"); Iterator<String> itr=al. iterator(); while(itr. has. Next()){ System. out. println(itr. next()); } } } Output: Ravi Vijay Ajay
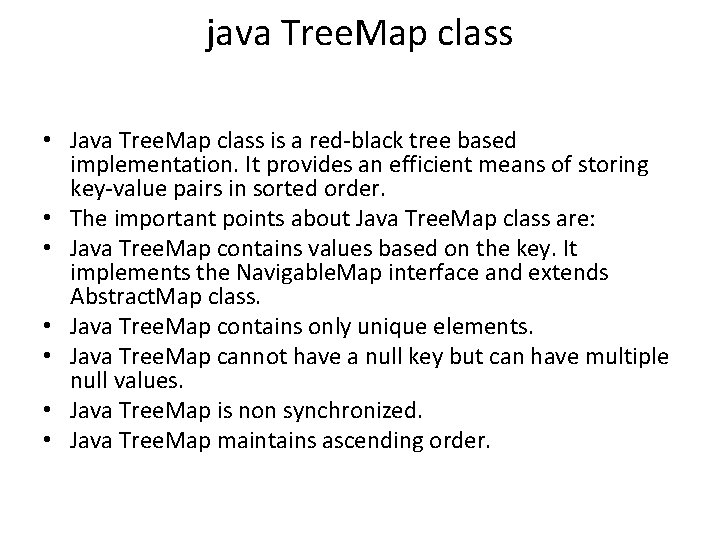
java Tree. Map class • Java Tree. Map class is a red-black tree based implementation. It provides an efficient means of storing key-value pairs in sorted order. • The important points about Java Tree. Map class are: • Java Tree. Map contains values based on the key. It implements the Navigable. Map interface and extends Abstract. Map class. • Java Tree. Map contains only unique elements. • Java Tree. Map cannot have a null key but can have multiple null values. • Java Tree. Map is non synchronized. • Java Tree. Map maintains ascending order.
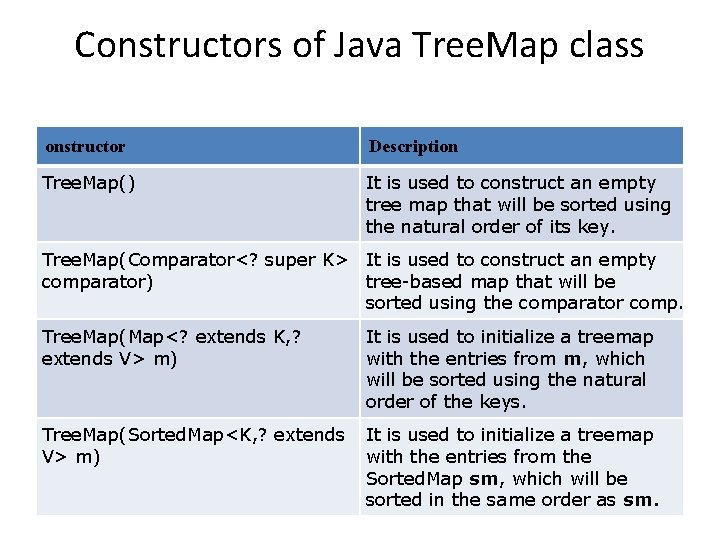
Constructors of Java Tree. Map class onstructor Description Tree. Map() It is used to construct an empty tree map that will be sorted using the natural order of its key. Tree. Map(Comparator<? super K> It is used to construct an empty comparator) tree-based map that will be sorted using the comparator comp. Tree. Map(Map<? extends K, ? extends V> m) It is used to initialize a treemap with the entries from m, which will be sorted using the natural order of the keys. Tree. Map(Sorted. Map<K, ? extends It is used to initialize a treemap V> m) with the entries from the Sorted. Map sm, which will be sorted in the same order as sm.
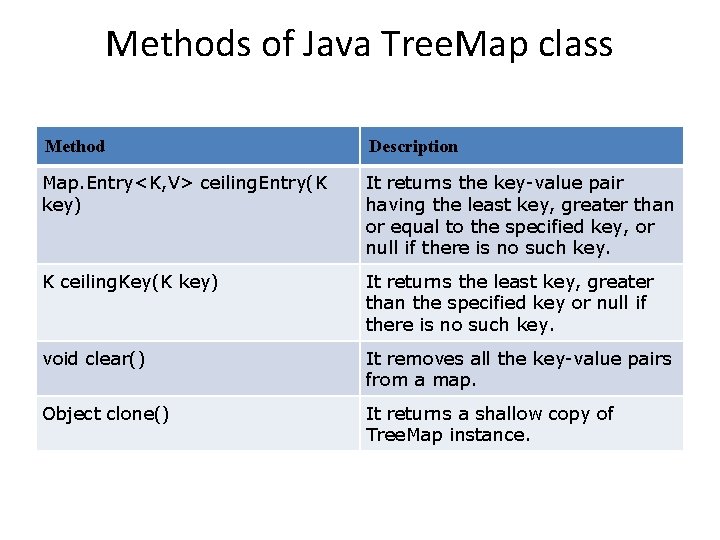
Methods of Java Tree. Map class Method Description Map. Entry<K, V> ceiling. Entry(K key) It returns the key-value pair having the least key, greater than or equal to the specified key, or null if there is no such key. K ceiling. Key(K key) It returns the least key, greater than the specified key or null if there is no such key. void clear() It removes all the key-value pairs from a map. Object clone() It returns a shallow copy of Tree. Map instance.
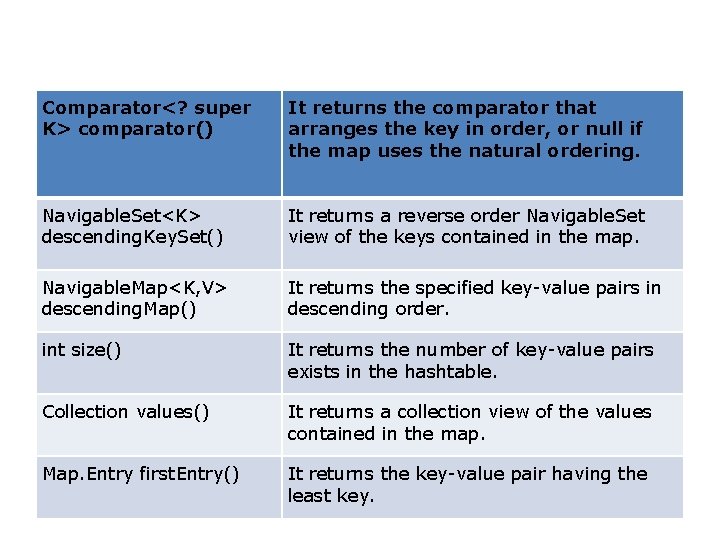
Comparator<? super K> comparator() It returns the comparator that arranges the key in order, or null if the map uses the natural ordering. Navigable. Set<K> descending. Key. Set() It returns a reverse order Navigable. Set view of the keys contained in the map. Navigable. Map<K, V> descending. Map() It returns the specified key-value pairs in descending order. int size() It returns the number of key-value pairs exists in the hashtable. Collection values() It returns a collection view of the values contained in the map. Map. Entry first. Entry() It returns the key-value pair having the least key.
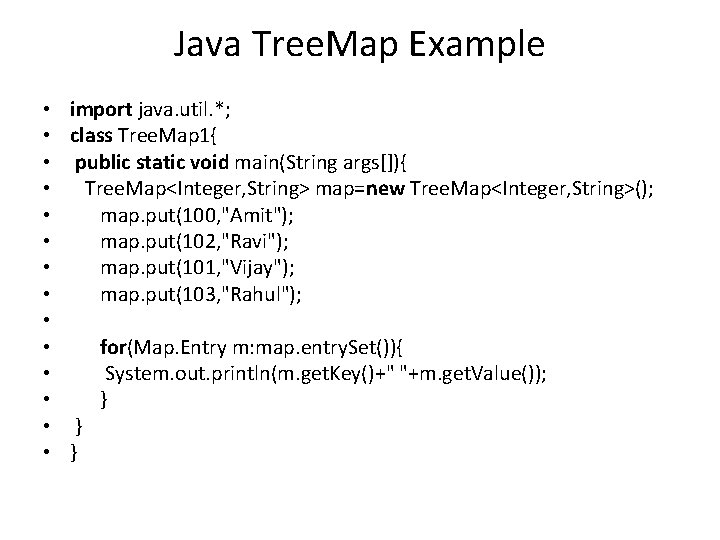
Java Tree. Map Example • • • • import java. util. *; class Tree. Map 1{ public static void main(String args[]){ Tree. Map<Integer, String> map=new Tree. Map<Integer, String>(); map. put(100, "Amit"); map. put(102, "Ravi"); map. put(101, "Vijay"); map. put(103, "Rahul"); for(Map. Entry m: map. entry. Set()){ System. out. println(m. get. Key()+" "+m. get. Value()); } } }
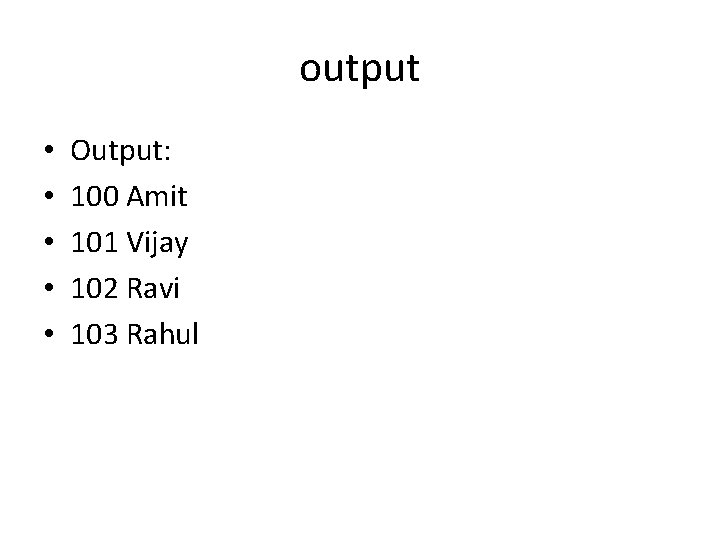
output • • • Output: 100 Amit 101 Vijay 102 Ravi 103 Rahul
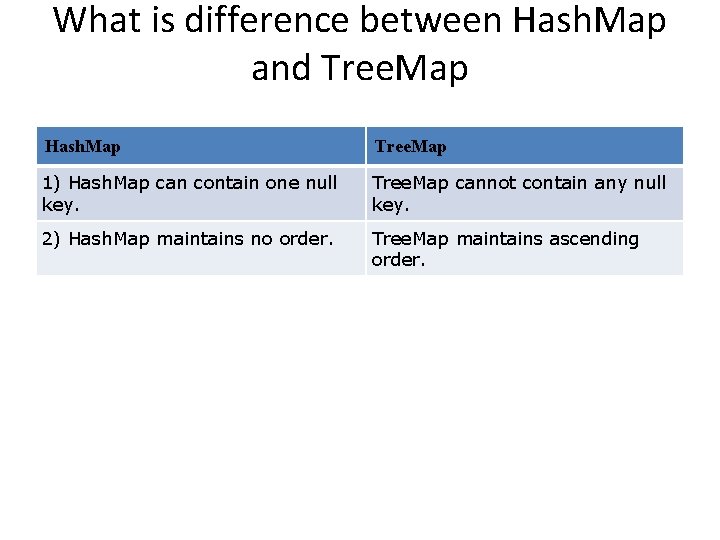
What is difference between Hash. Map and Tree. Map Hash. Map Tree. Map 1) Hash. Map can contain one null key. Tree. Map cannot contain any null key. 2) Hash. Map maintains no order. Tree. Map maintains ascending order.
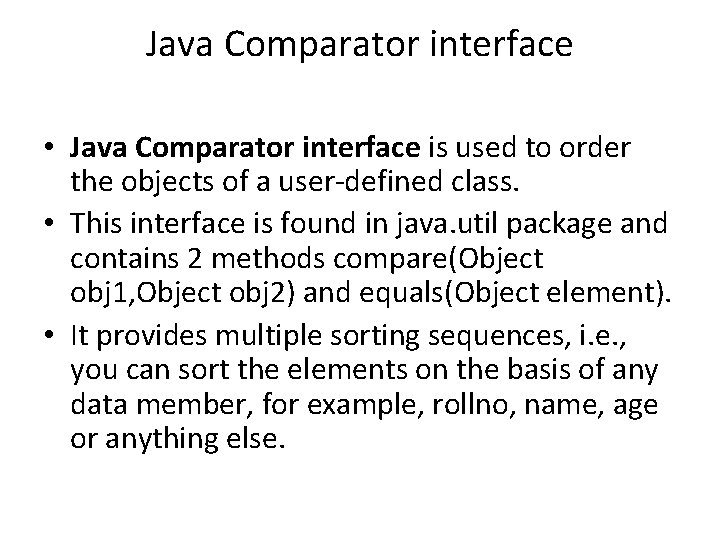
Java Comparator interface • Java Comparator interface is used to order the objects of a user-defined class. • This interface is found in java. util package and contains 2 methods compare(Object obj 1, Object obj 2) and equals(Object element). • It provides multiple sorting sequences, i. e. , you can sort the elements on the basis of any data member, for example, rollno, name, age or anything else.
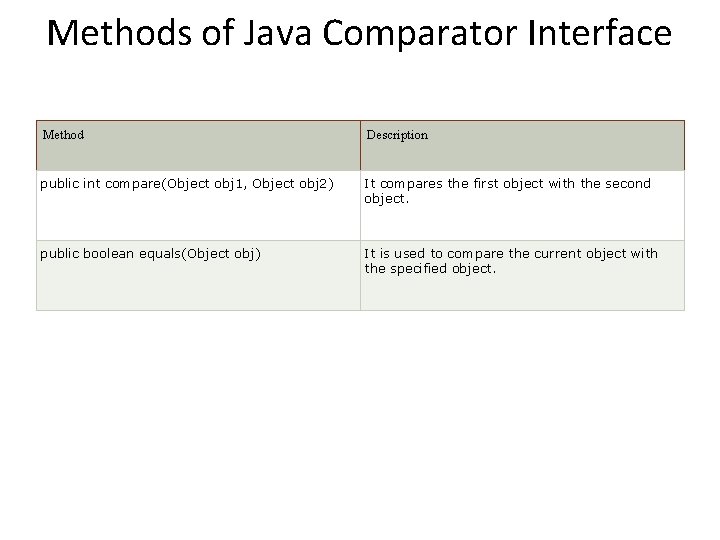
Methods of Java Comparator Interface Method Description public int compare(Object obj 1, Object obj 2) It compares the first object with the second object. public boolean equals(Object obj) It is used to compare the current object with the specified object.
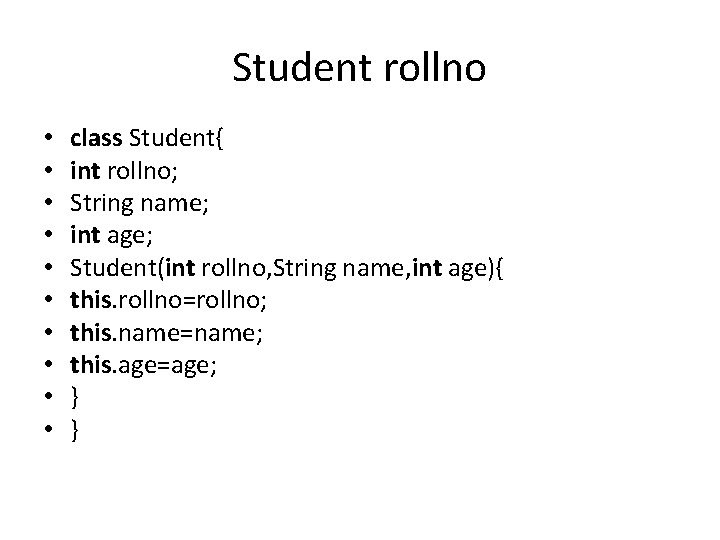
Student rollno • • • class Student{ int rollno; String name; int age; Student(int rollno, String name, int age){ this. rollno=rollno; this. name=name; this. age=age; } }
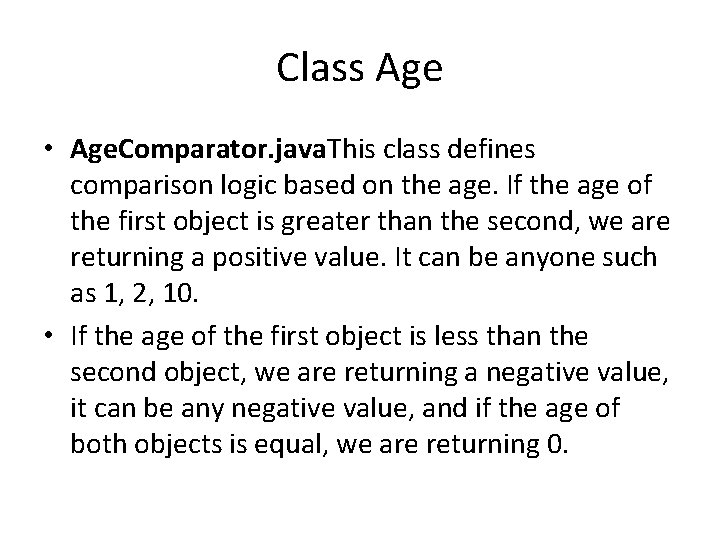
Class Age • Age. Comparator. java. This class defines comparison logic based on the age. If the age of the first object is greater than the second, we are returning a positive value. It can be anyone such as 1, 2, 10. • If the age of the first object is less than the second object, we are returning a negative value, it can be any negative value, and if the age of both objects is equal, we are returning 0.
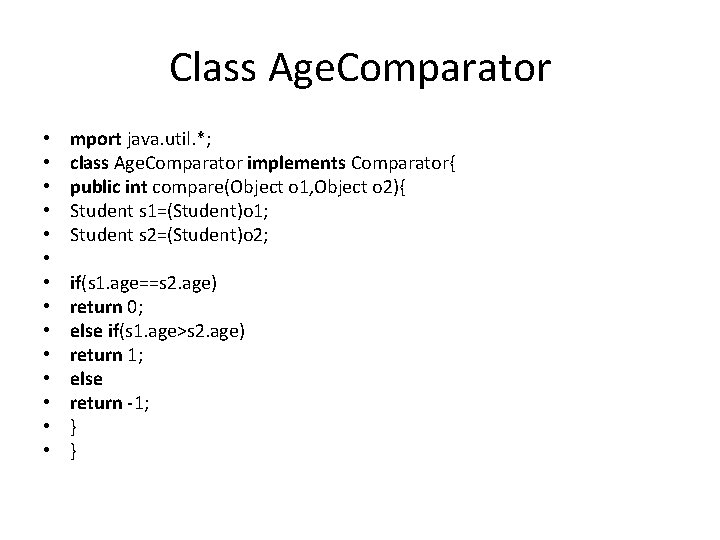
Class Age. Comparator • • • • mport java. util. *; class Age. Comparator implements Comparator{ public int compare(Object o 1, Object o 2){ Student s 1=(Student)o 1; Student s 2=(Student)o 2; if(s 1. age==s 2. age) return 0; else if(s 1. age>s 2. age) return 1; else return -1; } }
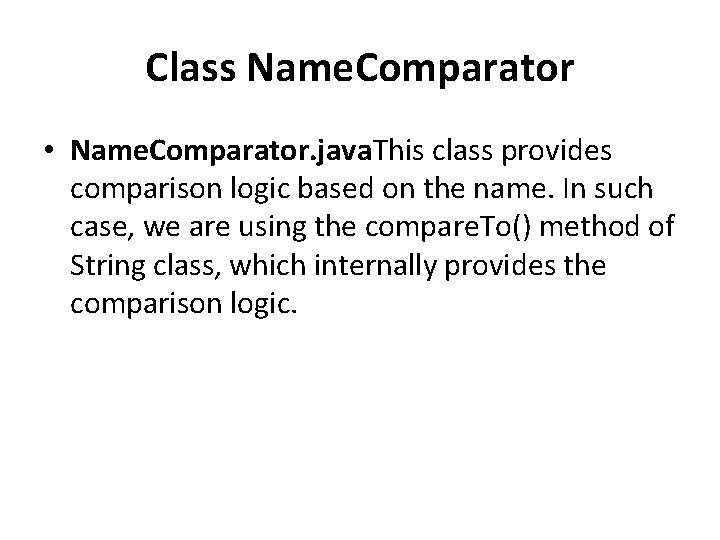
Class Name. Comparator • Name. Comparator. java. This class provides comparison logic based on the name. In such case, we are using the compare. To() method of String class, which internally provides the comparison logic.
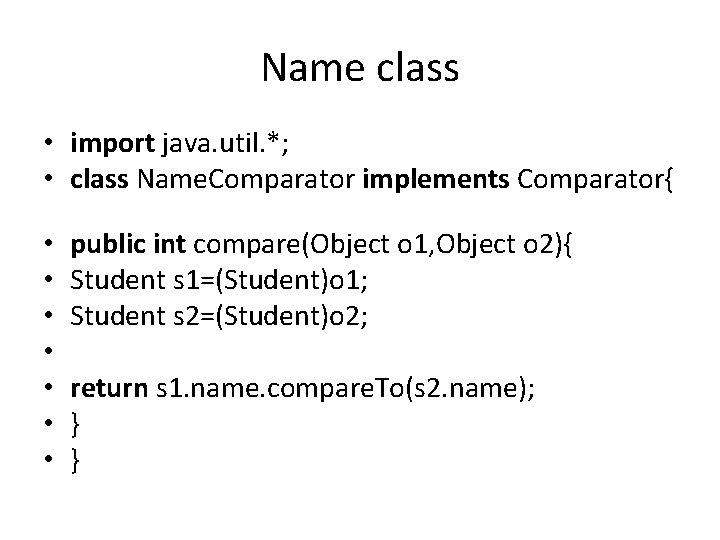
Name class • import java. util. *; • class Name. Comparator implements Comparator{ • public int compare(Object o 1, Object o 2){ • Student s 1=(Student)o 1; • Student s 2=(Student)o 2; • • return s 1. name. compare. To(s 2. name); • }
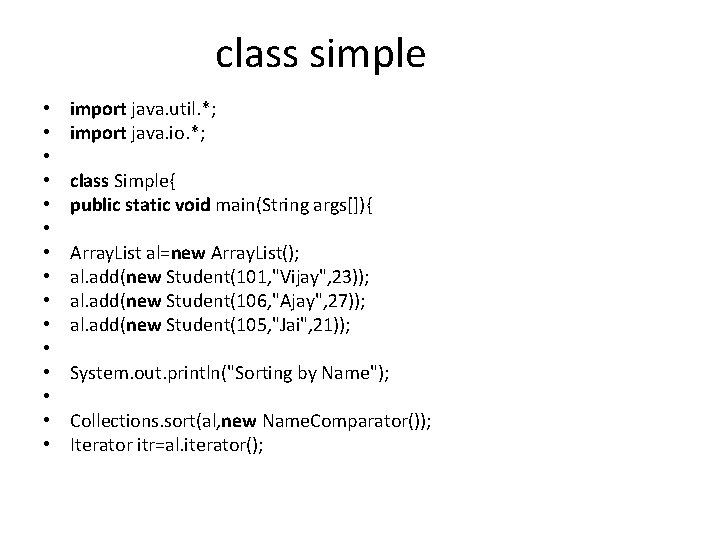
class simple • • • • import java. util. *; import java. io. *; class Simple{ public static void main(String args[]){ Array. List al=new Array. List(); al. add(new Student(101, "Vijay", 23)); al. add(new Student(106, "Ajay", 27)); al. add(new Student(105, "Jai", 21)); System. out. println("Sorting by Name"); Collections. sort(al, new Name. Comparator()); Iterator itr=al. iterator();
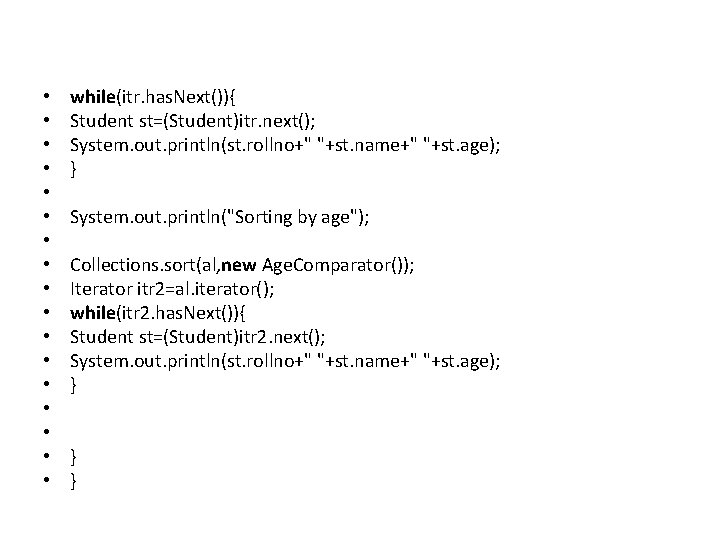
• • • • • while(itr. has. Next()){ Student st=(Student)itr. next(); System. out. println(st. rollno+" "+st. name+" "+st. age); } System. out. println("Sorting by age"); Collections. sort(al, new Age. Comparator()); Iterator itr 2=al. iterator(); while(itr 2. has. Next()){ Student st=(Student)itr 2. next(); System. out. println(st. rollno+" "+st. name+" "+st. age); } }
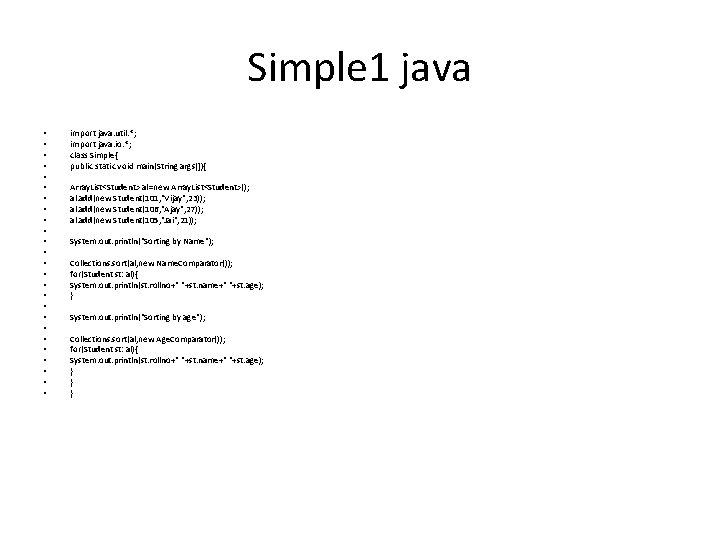
Simple 1 java • • • • • • • import java. util. *; import java. io. *; class Simple{ public static void main(String args[]){ Array. List<Student> al=new Array. List<Student>(); al. add(new Student(101, "Vijay", 23)); al. add(new Student(106, "Ajay", 27)); al. add(new Student(105, "Jai", 21)); System. out. println("Sorting by Name"); Collections. sort(al, new Name. Comparator()); for(Student st: al){ System. out. println(st. rollno+" "+st. name+" "+st. age); } System. out. println("Sorting by age"); Collections. sort(al, new Age. Comparator()); for(Student st: al){ System. out. println(st. rollno+" "+st. name+" "+st. age); } } }
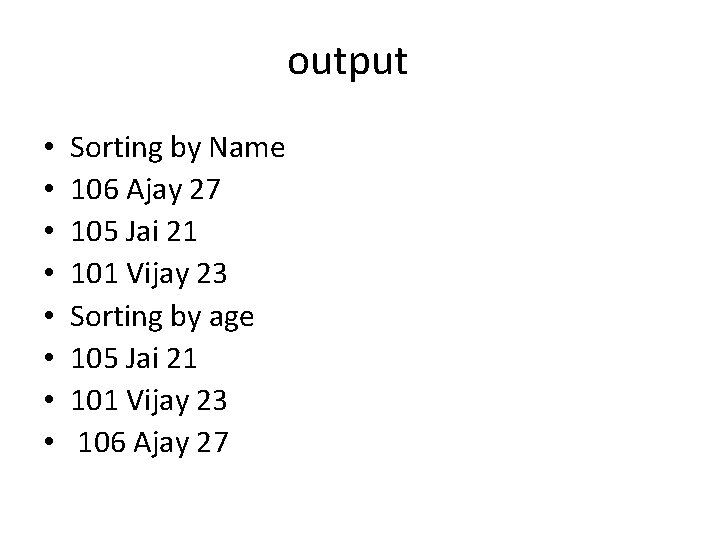
output • • Sorting by Name 106 Ajay 27 105 Jai 21 101 Vijay 23 Sorting by age 105 Jai 21 101 Vijay 23 106 Ajay 27
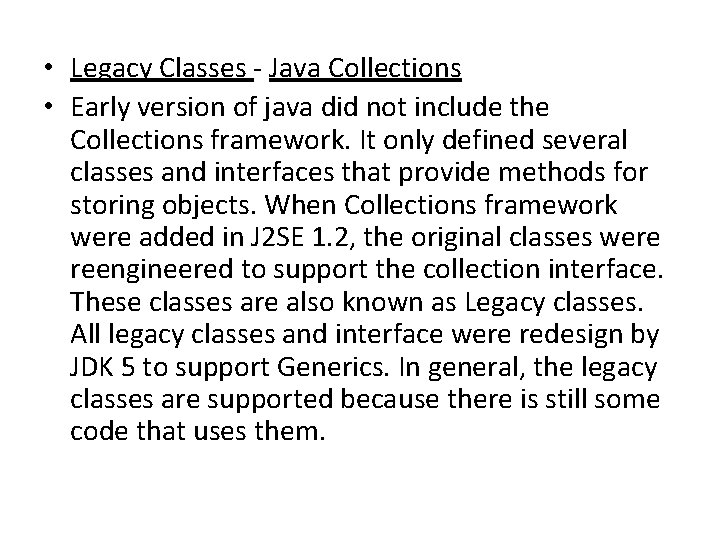
• Legacy Classes - Java Collections • Early version of java did not include the Collections framework. It only defined several classes and interfaces that provide methods for storing objects. When Collections framework were added in J 2 SE 1. 2, the original classes were reengineered to support the collection interface. These classes are also known as Legacy classes. All legacy classes and interface were redesign by JDK 5 to support Generics. In general, the legacy classes are supported because there is still some code that uses them.
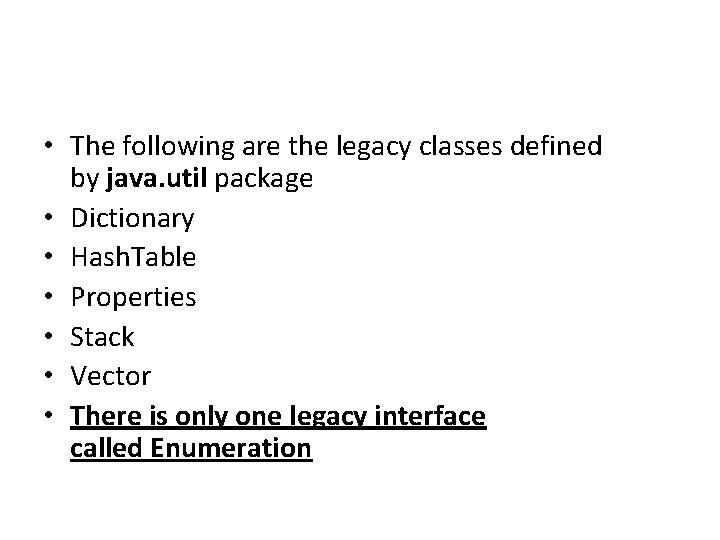
• The following are the legacy classes defined by java. util package • Dictionary • Hash. Table • Properties • Stack • Vector • There is only one legacy interface called Enumeration
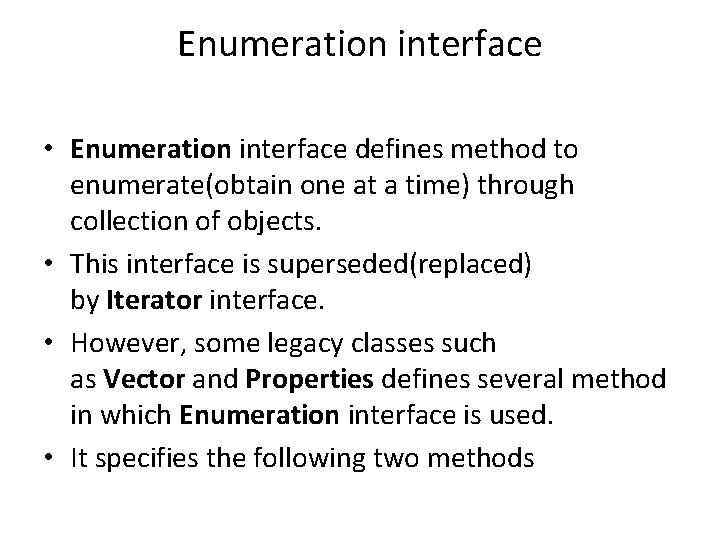
Enumeration interface • Enumeration interface defines method to enumerate(obtain one at a time) through collection of objects. • This interface is superseded(replaced) by Iterator interface. • However, some legacy classes such as Vector and Properties defines several method in which Enumeration interface is used. • It specifies the following two methods
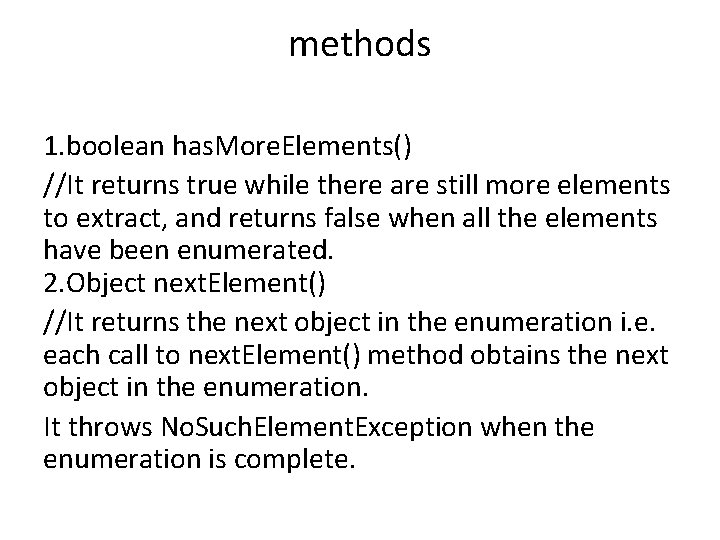
methods 1. boolean has. More. Elements() //It returns true while there are still more elements to extract, and returns false when all the elements have been enumerated. 2. Object next. Element() //It returns the next object in the enumeration i. e. each call to next. Element() method obtains the next object in the enumeration. It throws No. Such. Element. Exception when the enumeration is complete.
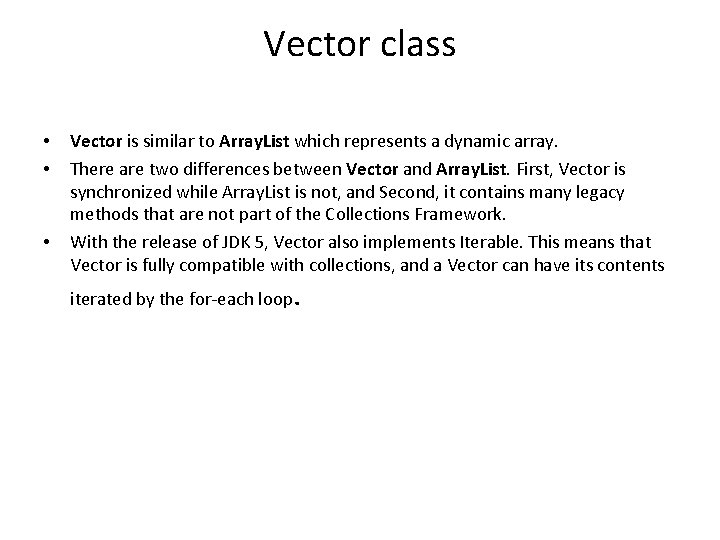
Vector class • • • Vector is similar to Array. List which represents a dynamic array. There are two differences between Vector and Array. List. First, Vector is synchronized while Array. List is not, and Second, it contains many legacy methods that are not part of the Collections Framework. With the release of JDK 5, Vector also implements Iterable. This means that Vector is fully compatible with collections, and a Vector can have its contents iterated by the for-each loop .
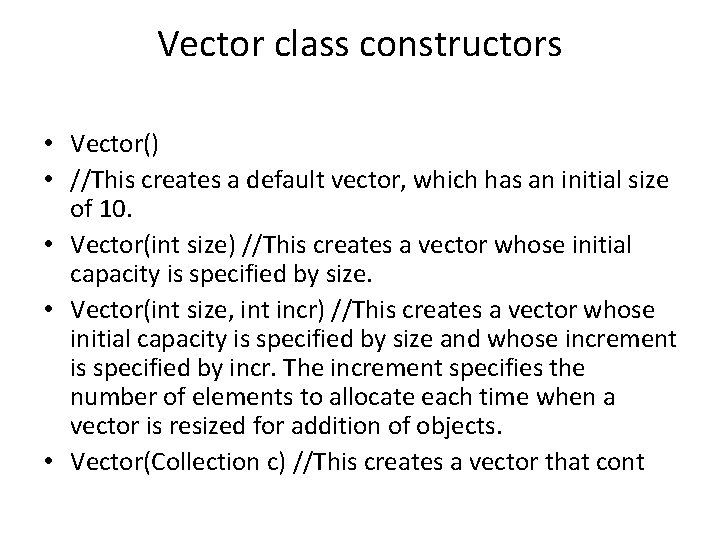
Vector class constructors • Vector() • //This creates a default vector, which has an initial size of 10. • Vector(int size) //This creates a vector whose initial capacity is specified by size. • Vector(int size, int incr) //This creates a vector whose initial capacity is specified by size and whose increment is specified by incr. The increment specifies the number of elements to allocate each time when a vector is resized for addition of objects. • Vector(Collection c) //This creates a vector that cont
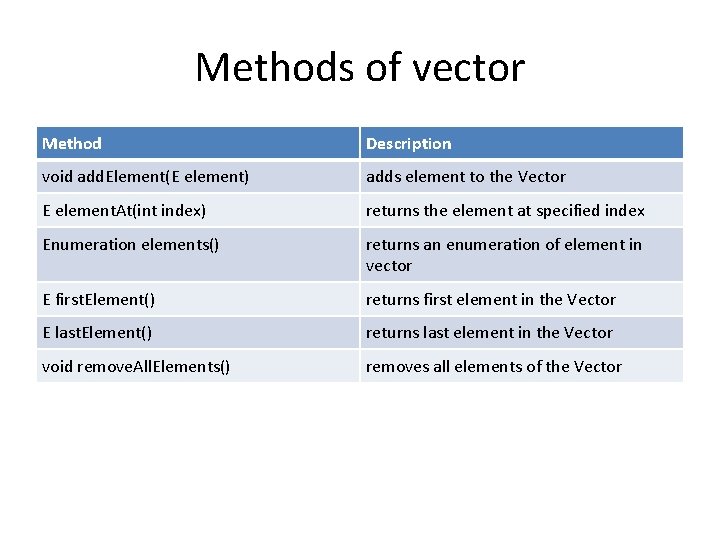
Methods of vector Method Description void add. Element(E element) adds element to the Vector E element. At(int index) returns the element at specified index Enumeration elements() returns an enumeration of element in vector E first. Element() returns first element in the Vector E last. Element() returns last element in the Vector void remove. All. Elements() removes all elements of the Vector
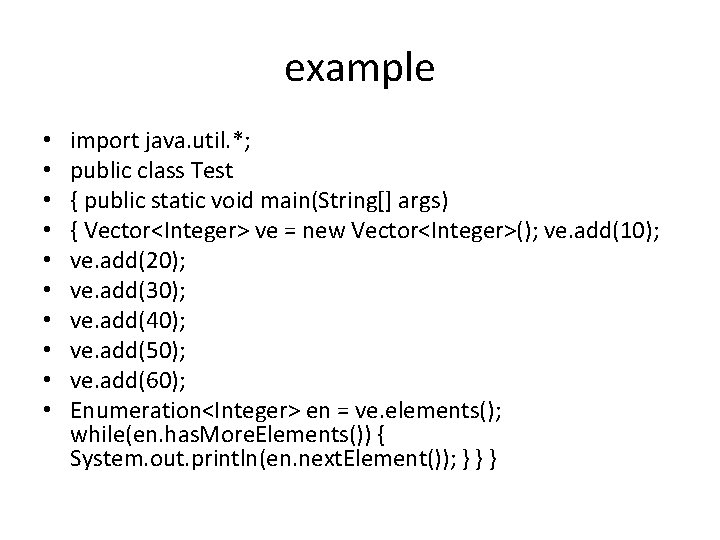
example • • • import java. util. *; public class Test { public static void main(String[] args) { Vector<Integer> ve = new Vector<Integer>(); ve. add(10); ve. add(20); ve. add(30); ve. add(40); ve. add(50); ve. add(60); Enumeration<Integer> en = ve. elements(); while(en. has. More. Elements()) { System. out. println(en. next. Element()); } } }
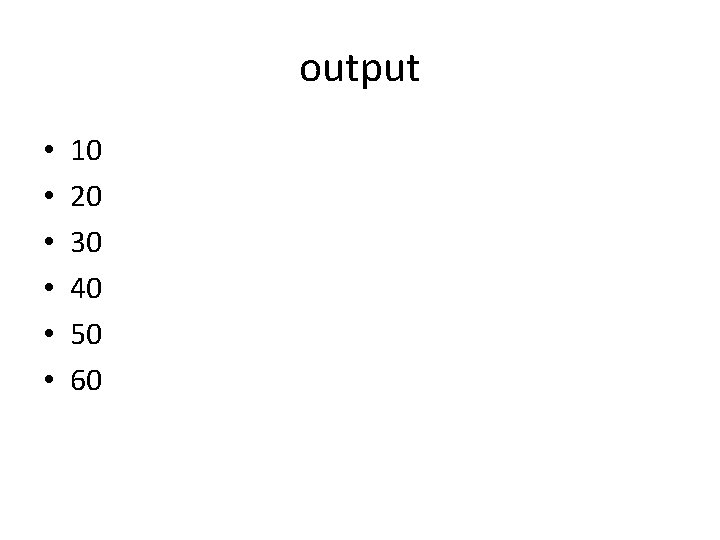
output • • • 10 20 30 40 50 60
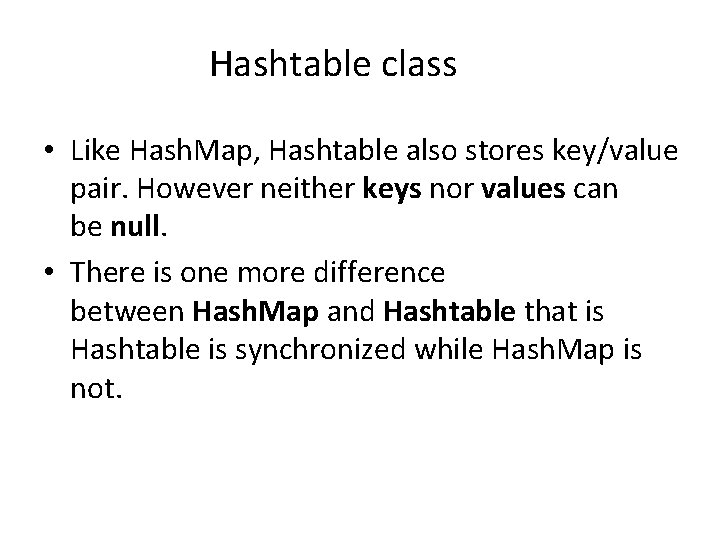
Hashtable class • Like Hash. Map, Hashtable also stores key/value pair. However neither keys nor values can be null. • There is one more difference between Hash. Map and Hashtable that is Hashtable is synchronized while Hash. Map is not.
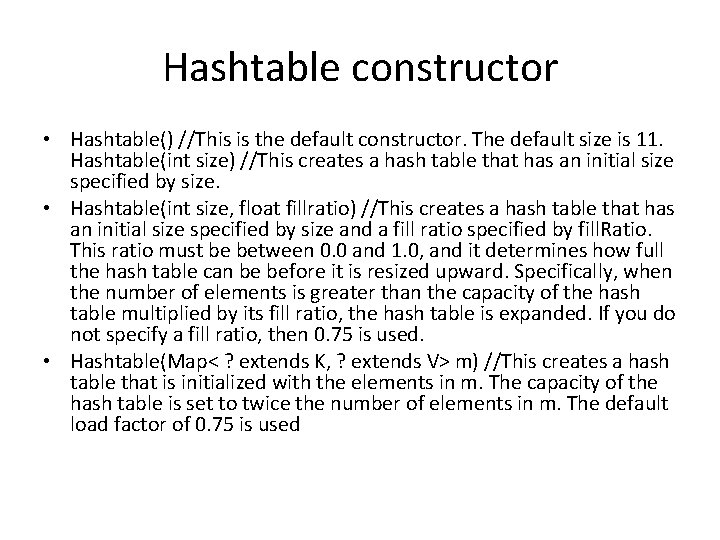
Hashtable constructor • Hashtable() //This is the default constructor. The default size is 11. Hashtable(int size) //This creates a hash table that has an initial size specified by size. • Hashtable(int size, float fillratio) //This creates a hash table that has an initial size specified by size and a fill ratio specified by fill. Ratio. This ratio must be between 0. 0 and 1. 0, and it determines how full the hash table can be before it is resized upward. Specifically, when the number of elements is greater than the capacity of the hash table multiplied by its fill ratio, the hash table is expanded. If you do not specify a fill ratio, then 0. 75 is used. • Hashtable(Map< ? extends K, ? extends V> m) //This creates a hash table that is initialized with the elements in m. The capacity of the hash table is set to twice the number of elements in m. The default load factor of 0. 75 is used
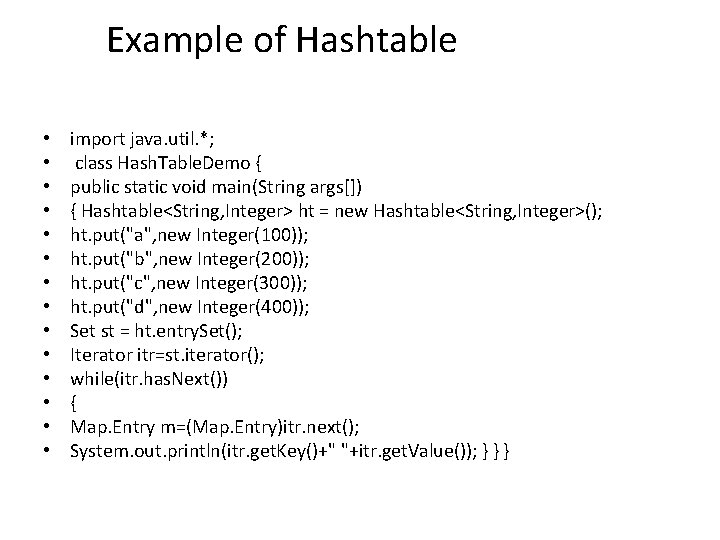
Example of Hashtable • • • • import java. util. *; class Hash. Table. Demo { public static void main(String args[]) { Hashtable<String, Integer> ht = new Hashtable<String, Integer>(); ht. put("a", new Integer(100)); ht. put("b", new Integer(200)); ht. put("c", new Integer(300)); ht. put("d", new Integer(400)); Set st = ht. entry. Set(); Iterator itr=st. iterator(); while(itr. has. Next()) { Map. Entry m=(Map. Entry)itr. next(); System. out. println(itr. get. Key()+" "+itr. get. Value()); } } }
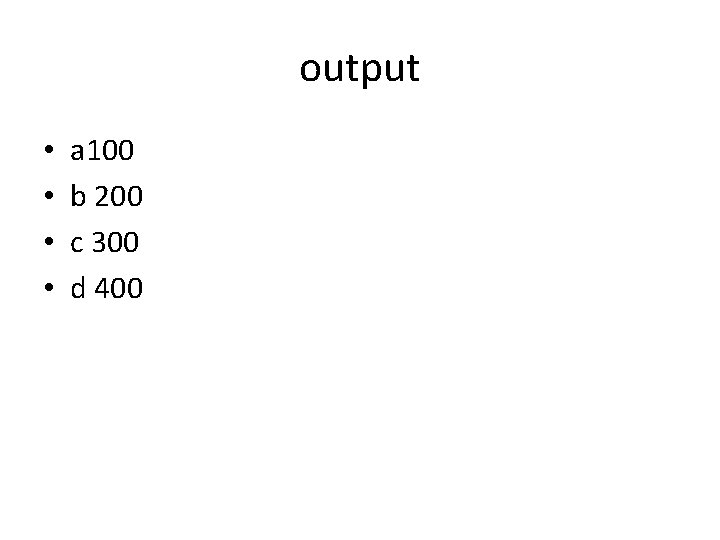
output • • a 100 b 200 c 300 d 400
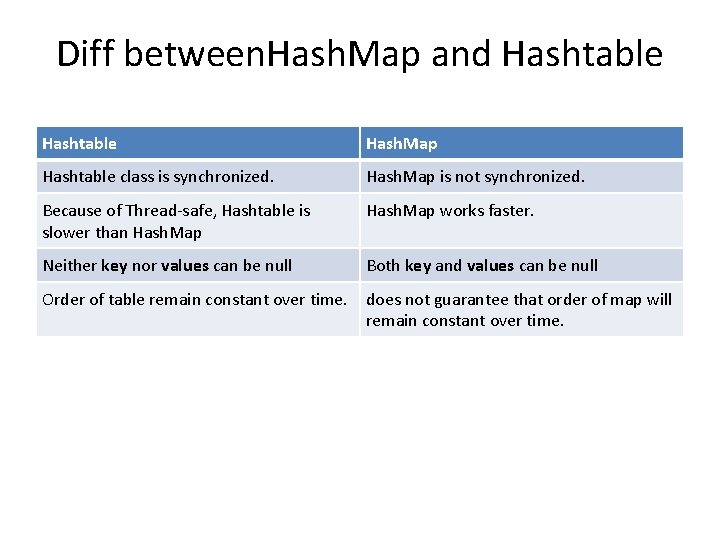
Diff between. Hash. Map and Hashtable Hash. Map Hashtable class is synchronized. Hash. Map is not synchronized. Because of Thread-safe, Hashtable is slower than Hash. Map works faster. Neither key nor values can be null Both key and values can be null Order of table remain constant over time. does not guarantee that order of map will remain constant over time.
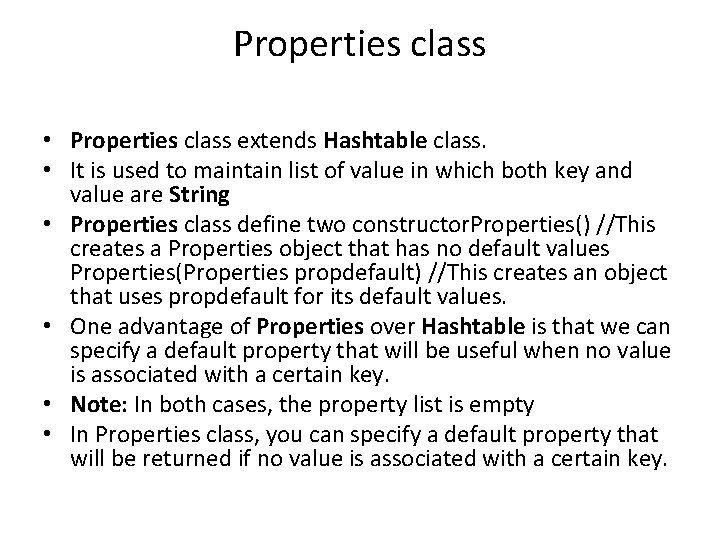
Properties class • Properties class extends Hashtable class. • It is used to maintain list of value in which both key and value are String • Properties class define two constructor. Properties() //This creates a Properties object that has no default values Properties(Properties propdefault) //This creates an object that uses propdefault for its default values. • One advantage of Properties over Hashtable is that we can specify a default property that will be useful when no value is associated with a certain key. • Note: In both cases, the property list is empty • In Properties class, you can specify a default property that will be returned if no value is associated with a certain key.
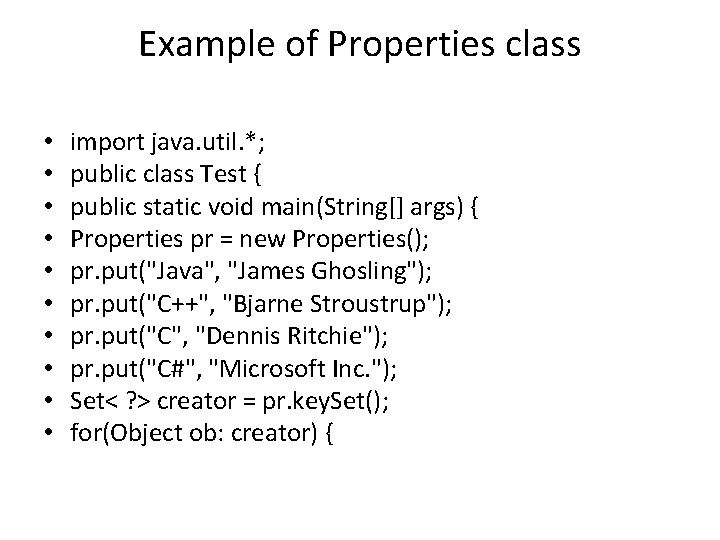
Example of Properties class • • • import java. util. *; public class Test { public static void main(String[] args) { Properties pr = new Properties(); pr. put("Java", "James Ghosling"); pr. put("C++", "Bjarne Stroustrup"); pr. put("C", "Dennis Ritchie"); pr. put("C#", "Microsoft Inc. "); Set< ? > creator = pr. key. Set(); for(Object ob: creator) {
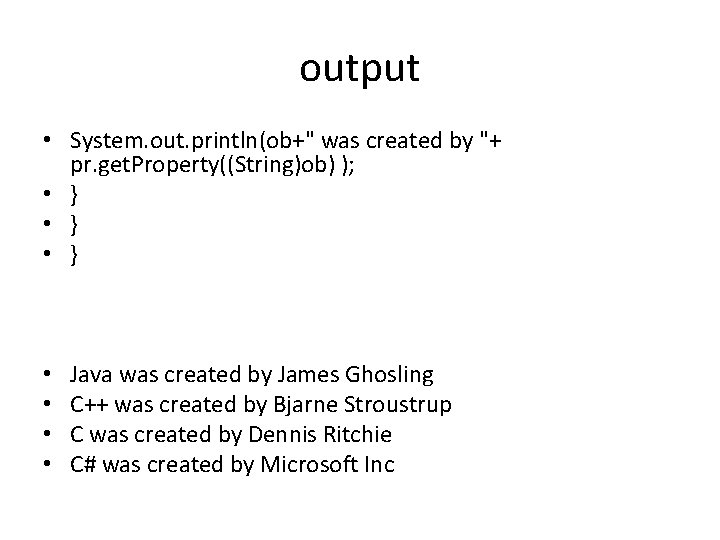
output • System. out. println(ob+" was created by "+ pr. get. Property((String)ob) ); • } • • Java was created by James Ghosling C++ was created by Bjarne Stroustrup C was created by Dennis Ritchie C# was created by Microsoft Inc
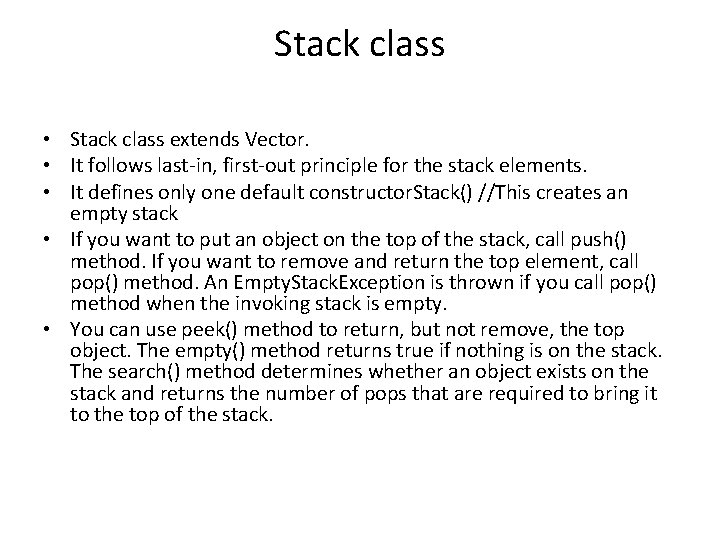
Stack class • Stack class extends Vector. • It follows last-in, first-out principle for the stack elements. • It defines only one default constructor. Stack() //This creates an empty stack • If you want to put an object on the top of the stack, call push() method. If you want to remove and return the top element, call pop() method. An Empty. Stack. Exception is thrown if you call pop() method when the invoking stack is empty. • You can use peek() method to return, but not remove, the top object. The empty() method returns true if nothing is on the stack. The search() method determines whether an object exists on the stack and returns the number of pops that are required to bring it to the top of the stack.
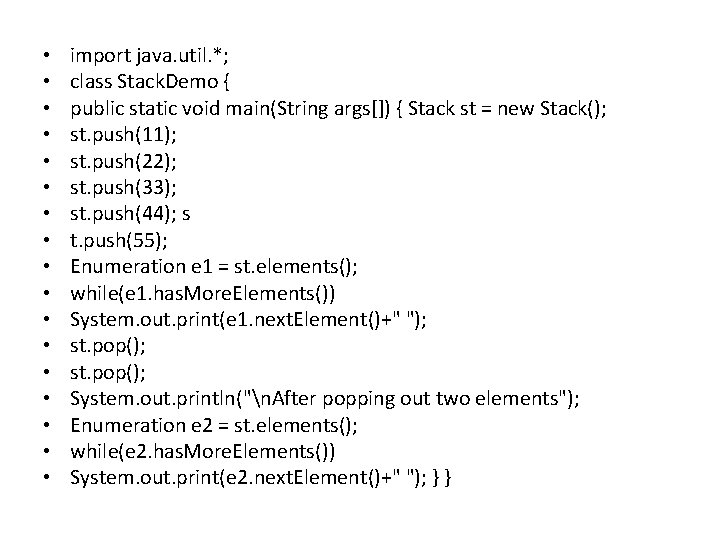
• • • • • import java. util. *; class Stack. Demo { public static void main(String args[]) { Stack st = new Stack(); st. push(11); st. push(22); st. push(33); st. push(44); s t. push(55); Enumeration e 1 = st. elements(); while(e 1. has. More. Elements()) System. out. print(e 1. next. Element()+" "); st. pop(); System. out. println("n. After popping out two elements"); Enumeration e 2 = st. elements(); while(e 2. has. More. Elements()) System. out. print(e 2. next. Element()+" "); } }
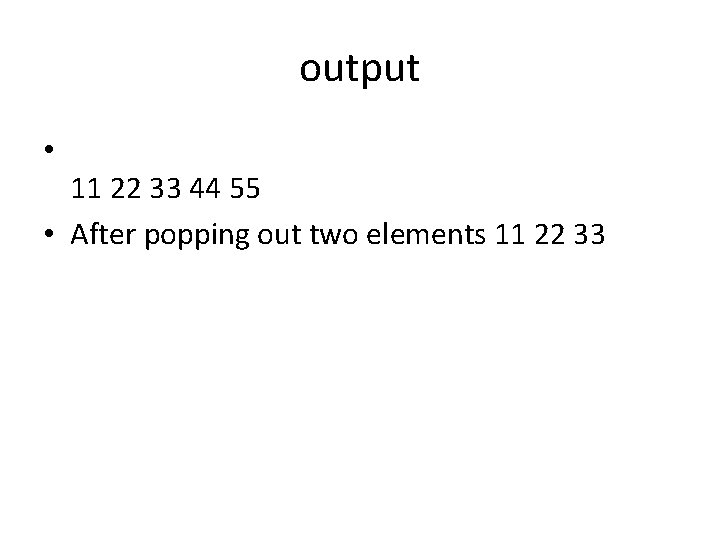
output • 11 22 33 44 55 • After popping out two elements 11 22 33
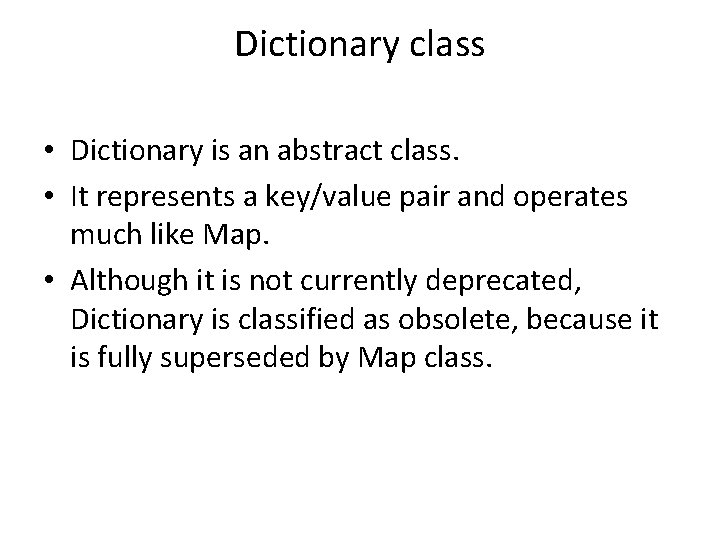
Dictionary class • Dictionary is an abstract class. • It represents a key/value pair and operates much like Map. • Although it is not currently deprecated, Dictionary is classified as obsolete, because it is fully superseded by Map class.
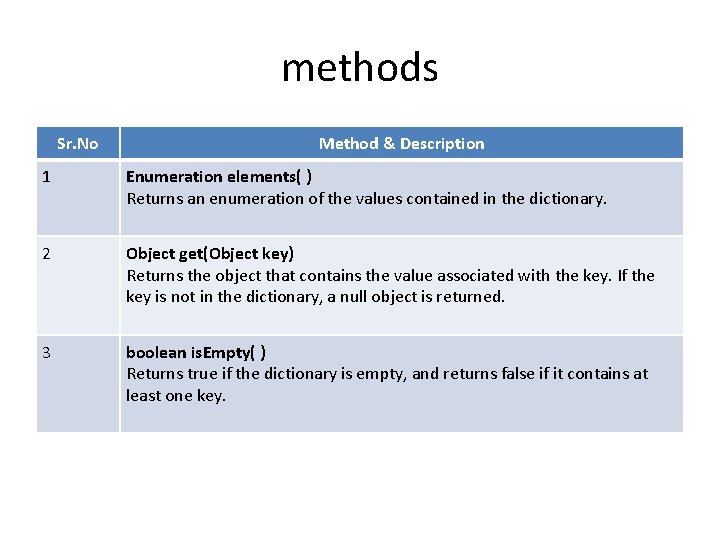
methods Sr. No Method & Description 1 Enumeration elements( ) Returns an enumeration of the values contained in the dictionary. 2 Object get(Object key) Returns the object that contains the value associated with the key. If the key is not in the dictionary, a null object is returned. 3 boolean is. Empty( ) Returns true if the dictionary is empty, and returns false if it contains at least one key.
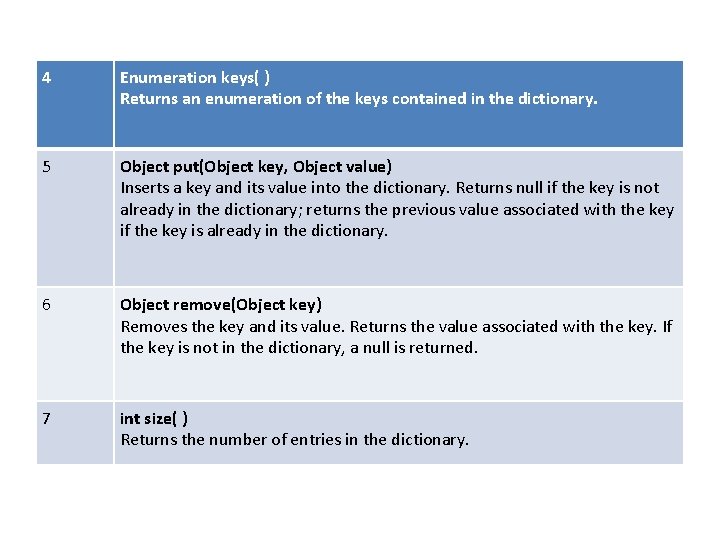
4 Enumeration keys( ) Returns an enumeration of the keys contained in the dictionary. 5 Object put(Object key, Object value) Inserts a key and its value into the dictionary. Returns null if the key is not already in the dictionary; returns the previous value associated with the key if the key is already in the dictionary. 6 Object remove(Object key) Removes the key and its value. Returns the value associated with the key. If the key is not in the dictionary, a null is returned. 7 int size( ) Returns the number of entries in the dictionary.
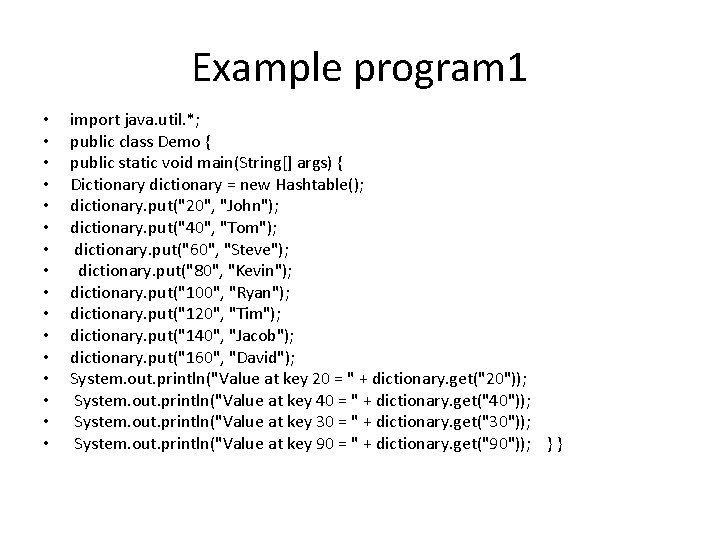
Example program 1 • • • • import java. util. *; public class Demo { public static void main(String[] args) { Dictionary dictionary = new Hashtable(); dictionary. put("20", "John"); dictionary. put("40", "Tom"); dictionary. put("60", "Steve"); dictionary. put("80", "Kevin"); dictionary. put("100", "Ryan"); dictionary. put("120", "Tim"); dictionary. put("140", "Jacob"); dictionary. put("160", "David"); System. out. println("Value at key 20 = " + dictionary. get("20")); System. out. println("Value at key 40 = " + dictionary. get("40")); System. out. println("Value at key 30 = " + dictionary. get("30")); System. out. println("Value at key 90 = " + dictionary. get("90")); } }
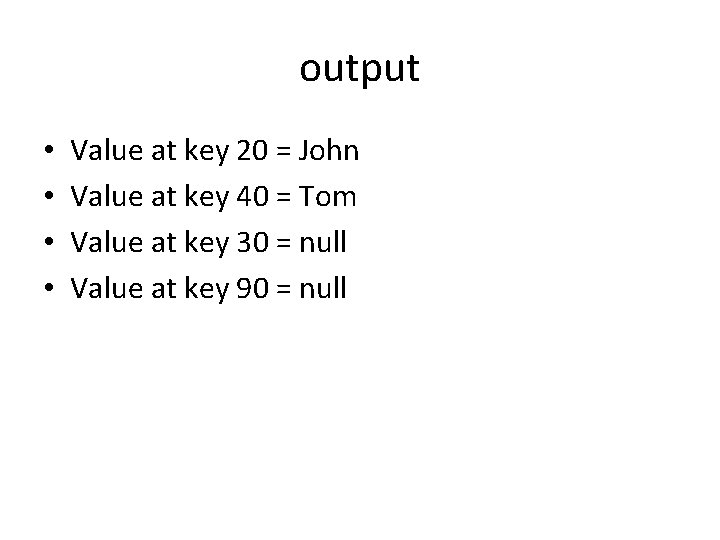
output • • Value at key 20 = John Value at key 40 = Tom Value at key 30 = null Value at key 90 = null
![example 2 import java util public class Demo public static void mainString example 2 import java. util. *; public class Demo { public static void main(String[]](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-102.jpg)
example 2 import java. util. *; public class Demo { public static void main(String[] args) { Dictionary dictionary = new Hashtable(); dictionary. put("20", "John"); dictionary. put("40", "Tom"); dictionary. put("60", "Steve"); dictionary. put("80", "Kevin"); dictionary. put("100", "Ryan"); dictionary. put("120", "Tim"); dictionary. put("140", "Jacob"); dictionary. put("160", "David"); System. out. println("Dictionary Values. . . "); for (Enumeration i = dictionary. elements(); i. has. More. Elements(); ) { System. out. println(i. next. Element()); } System. out. println("Value at key 20 = " + dictionary. get("20")); System. out. println("Value at key 40 = " + dictionary. get("40")); System. out. println("Value at key 30 = " + dictionary. get("30"));
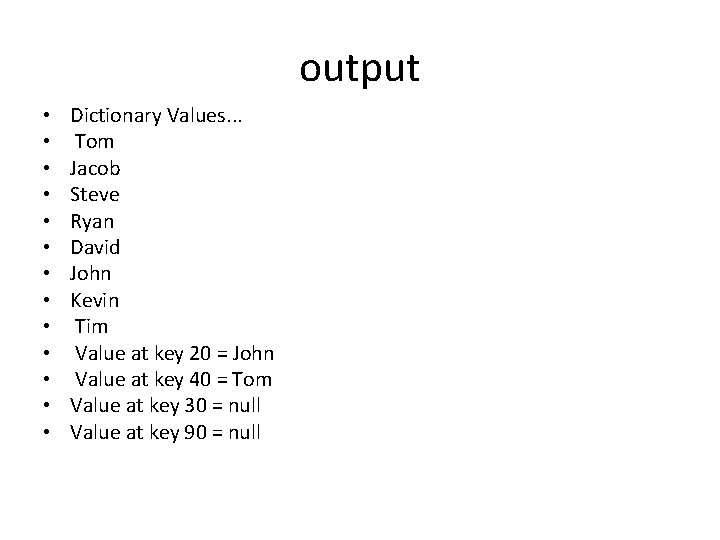
output • • • • Dictionary Values. . . Tom Jacob Steve Ryan David John Kevin Tim Value at key 20 = John Value at key 40 = Tom Value at key 30 = null Value at key 90 = null
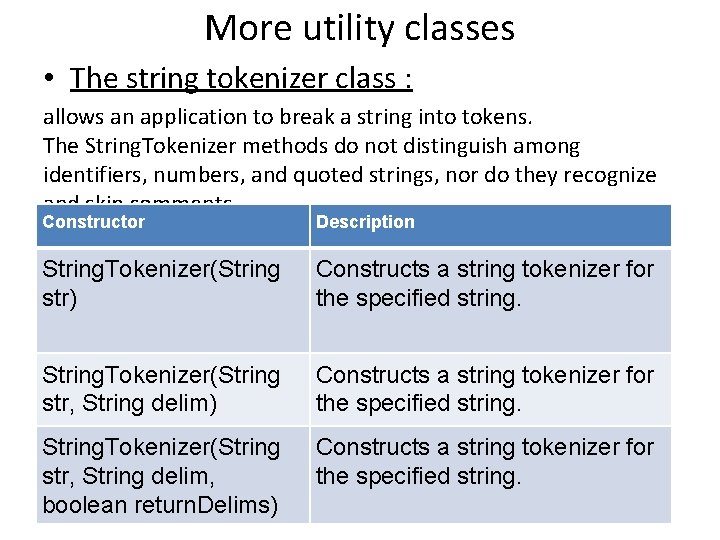
More utility classes • The string tokenizer class : allows an application to break a string into tokens. The String. Tokenizer methods do not distinguish among identifiers, numbers, and quoted strings, nor do they recognize and skip comments. Constructor Description The set of delimiters (the characters that separate tokens) may String. Tokenizer(String Constructs a string tokenizer for be specified either at creation time or on a per-token basis. str) the specified string. String. Tokenizer(String str, String delim) Constructs a string tokenizer for the specified string. String. Tokenizer(String str, String delim, boolean return. Delims) Constructs a string tokenizer for the specified string.
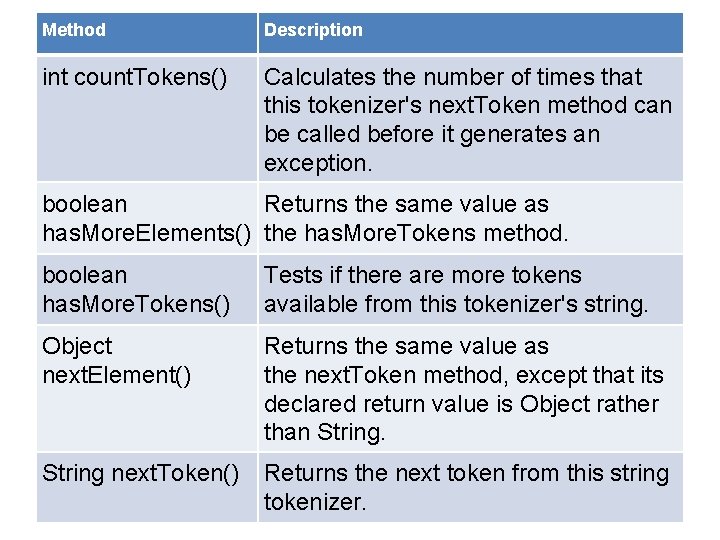
Method Description int count. Tokens() Calculates the number of times that this tokenizer's next. Token method can be called before it generates an exception. boolean Returns the same value as has. More. Elements() the has. More. Tokens method. boolean has. More. Tokens() Tests if there are more tokens available from this tokenizer's string. Object next. Element() Returns the same value as the next. Token method, except that its declared return value is Object rather than String next. Token() Returns the next token from this string tokenizer.
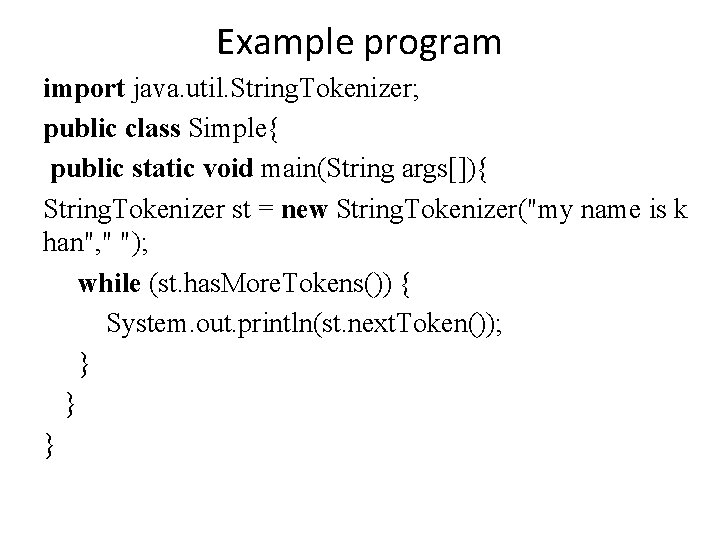
Example program import java. util. String. Tokenizer; public class Simple{ public static void main(String args[]){ String. Tokenizer st = new String. Tokenizer("my name is k han", " "); while (st. has. More. Tokens()) { System. out. println(st. next. Token()); } } }
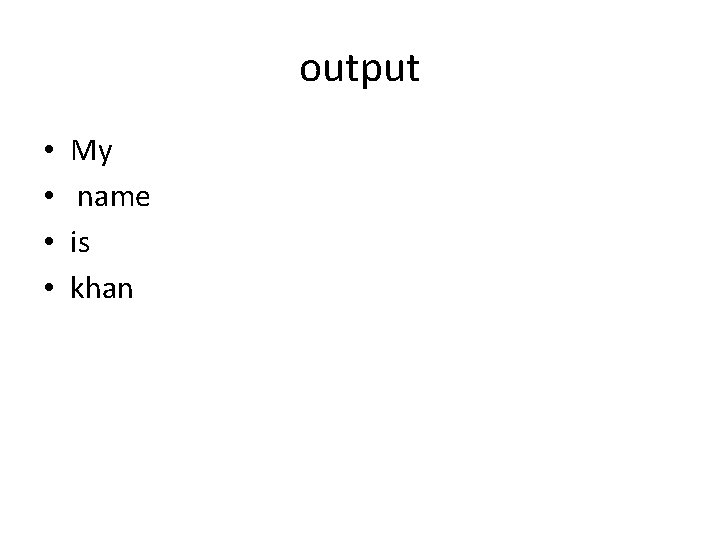
output • • My name is khan
![import java util public class Test public static void mainString args import java. util. *; public class Test { public static void main(String[] args) {](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-108.jpg)
import java. util. *; public class Test { public static void main(String[] args) { String. Tokenizer st = new String. Tokenizer("my, name, is, khan"); // printing next token System. out. println("Next token is : " + st. next. Token(", ")); } }
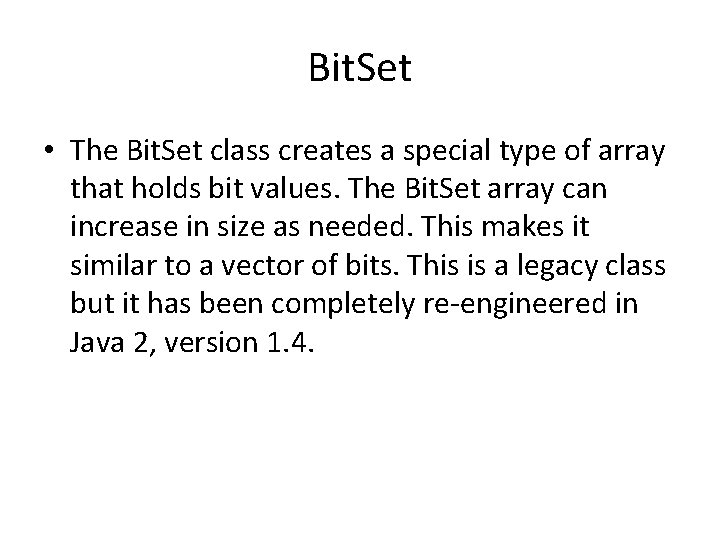
Bit. Set • The Bit. Set class creates a special type of array that holds bit values. The Bit. Set array can increase in size as needed. This makes it similar to a vector of bits. This is a legacy class but it has been completely re-engineered in Java 2, version 1. 4.
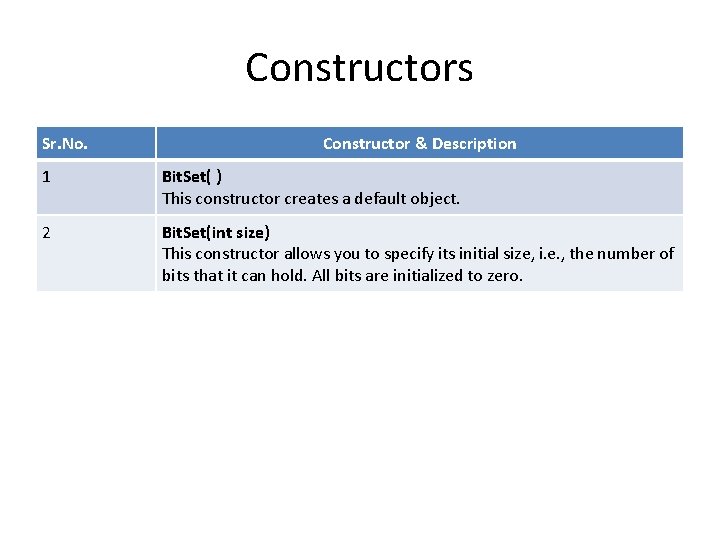
Constructors Sr. No. Constructor & Description 1 Bit. Set( ) This constructor creates a default object. 2 Bit. Set(int size) This constructor allows you to specify its initial size, i. e. , the number of bits that it can hold. All bits are initialized to zero.
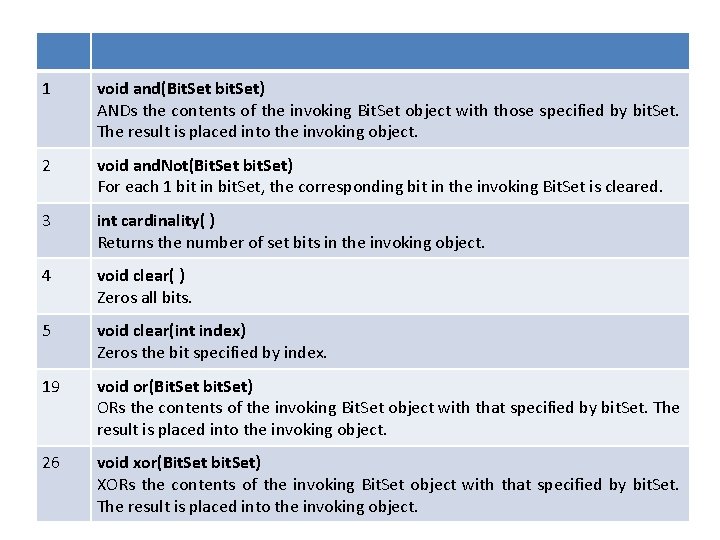
1 void and(Bit. Set bit. Set) ANDs the contents of the invoking Bit. Set object with those specified by bit. Set. The result is placed into the invoking object. 2 void and. Not(Bit. Set bit. Set) For each 1 bit in bit. Set, the corresponding bit in the invoking Bit. Set is cleared. 3 int cardinality( ) Returns the number of set bits in the invoking object. 4 void clear( ) Zeros all bits. 5 void clear(int index) Zeros the bit specified by index. 19 void or(Bit. Set bit. Set) ORs the contents of the invoking Bit. Set object with that specified by bit. Set. The result is placed into the invoking object. 26 void xor(Bit. Set bit. Set) XORs the contents of the invoking Bit. Set object with that specified by bit. Set. The result is placed into the invoking object.
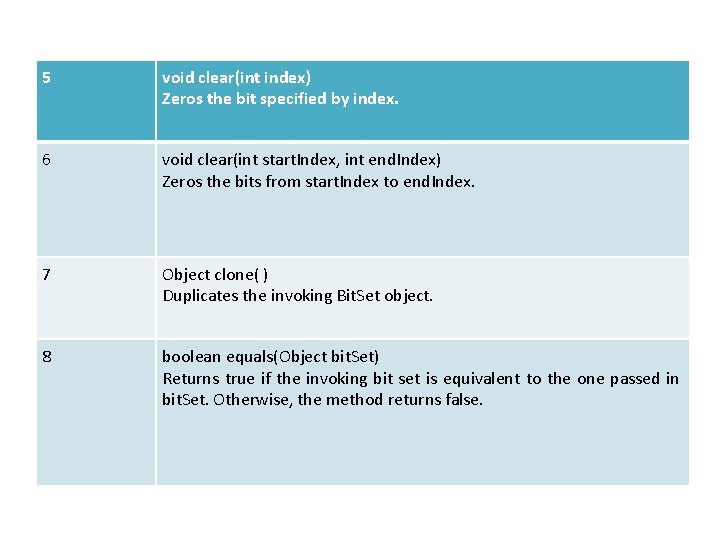
5 void clear(int index) Zeros the bit specified by index. 6 void clear(int start. Index, int end. Index) Zeros the bits from start. Index to end. Index. 7 Object clone( ) Duplicates the invoking Bit. Set object. 8 boolean equals(Object bit. Set) Returns true if the invoking bit set is equivalent to the one passed in bit. Set. Otherwise, the method returns false.
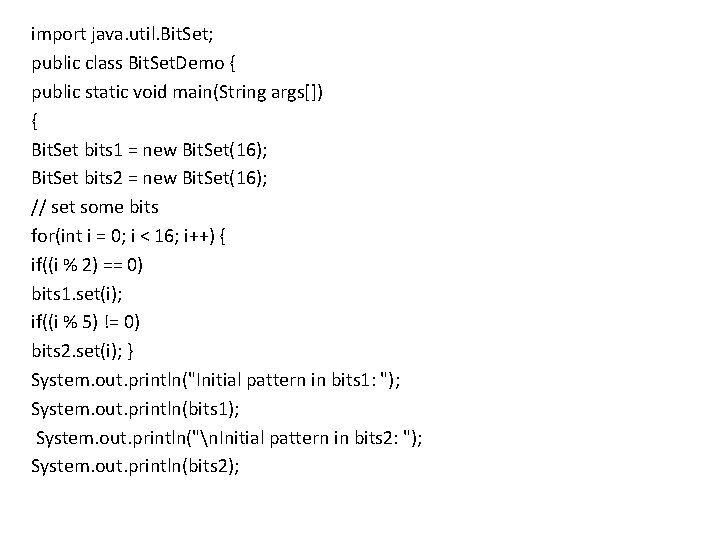
import java. util. Bit. Set; public class Bit. Set. Demo { public static void main(String args[]) { Bit. Set bits 1 = new Bit. Set(16); Bit. Set bits 2 = new Bit. Set(16); // set some bits for(int i = 0; i < 16; i++) { if((i % 2) == 0) bits 1. set(i); if((i % 5) != 0) bits 2. set(i); } System. out. println("Initial pattern in bits 1: "); System. out. println(bits 1); System. out. println("n. Initial pattern in bits 2: "); System. out. println(bits 2);
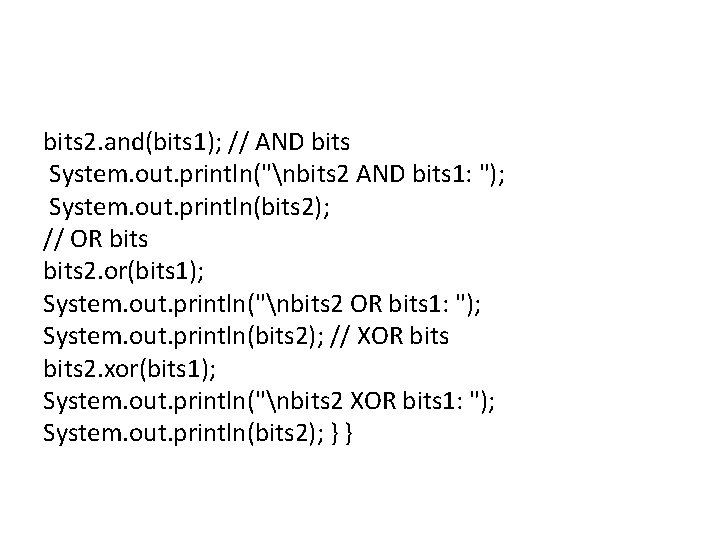
bits 2. and(bits 1); // AND bits System. out. println("nbits 2 AND bits 1: "); System. out. println(bits 2); // OR bits 2. or(bits 1); System. out. println("nbits 2 OR bits 1: "); System. out. println(bits 2); // XOR bits 2. xor(bits 1); System. out. println("nbits 2 XOR bits 1: "); System. out. println(bits 2); } }
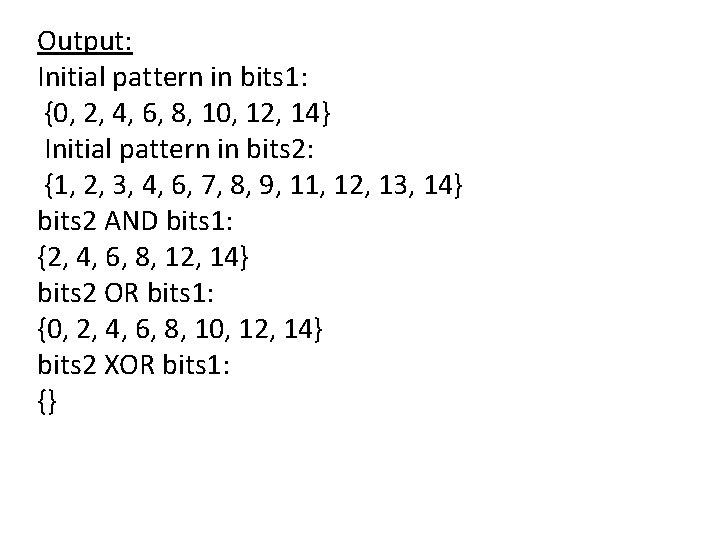
Output: Initial pattern in bits 1: {0, 2, 4, 6, 8, 10, 12, 14} Initial pattern in bits 2: {1, 2, 3, 4, 6, 7, 8, 9, 11, 12, 13, 14} bits 2 AND bits 1: {2, 4, 6, 8, 12, 14} bits 2 OR bits 1: {0, 2, 4, 6, 8, 10, 12, 14} bits 2 XOR bits 1: {}
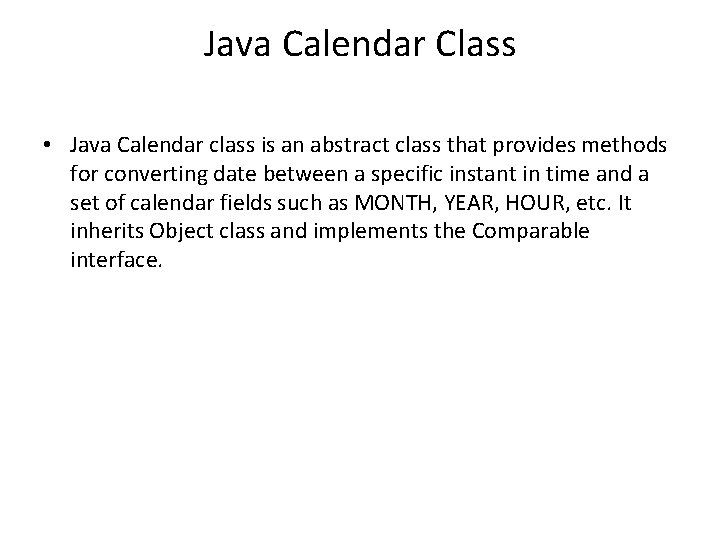
Java Calendar Class • Java Calendar class is an abstract class that provides methods for converting date between a specific instant in time and a set of calendar fields such as MONTH, YEAR, HOUR, etc. It inherits Object class and implements the Comparable interface.
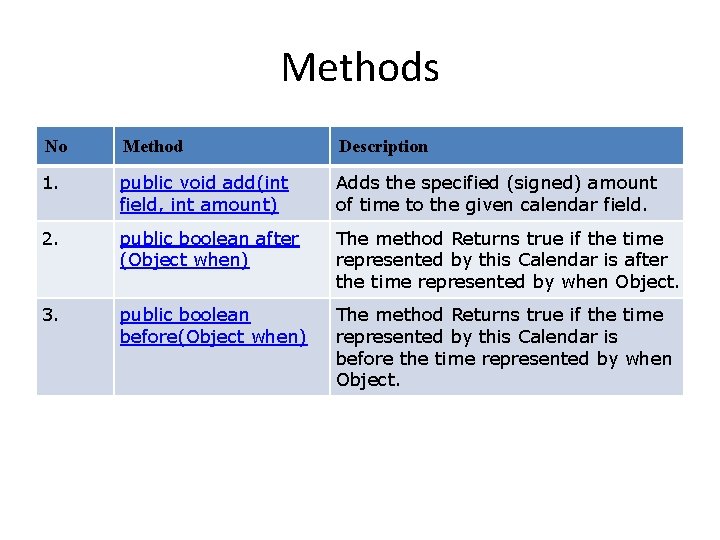
Methods No Method Description 1. public void add(int field, int amount) Adds the specified (signed) amount of time to the given calendar field. 2. public boolean after (Object when) The method Returns true if the time represented by this Calendar is after the time represented by when Object. 3. public boolean before(Object when) The method Returns true if the time represented by this Calendar is before the time represented by when Object.
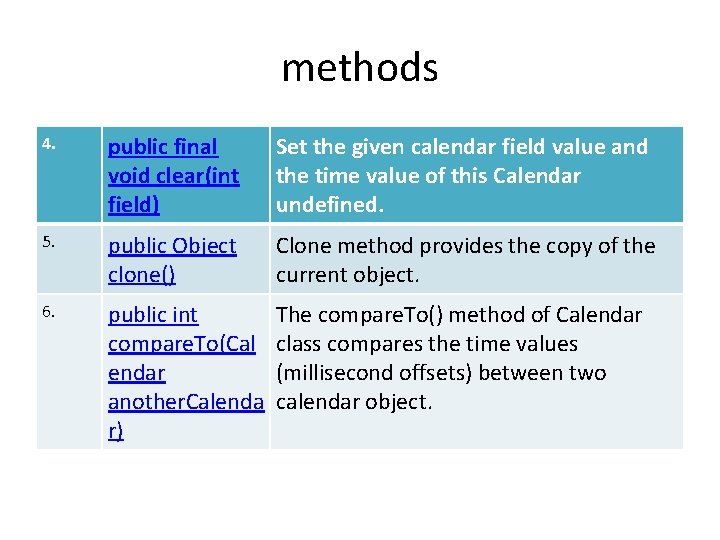
methods 4. public final void clear(int field) Set the given calendar field value and the time value of this Calendar undefined. 5. public Object clone() Clone method provides the copy of the current object. 6. public int compare. To(Cal endar another. Calenda r) The compare. To() method of Calendar class compares the time values (millisecond offsets) between two calendar object.
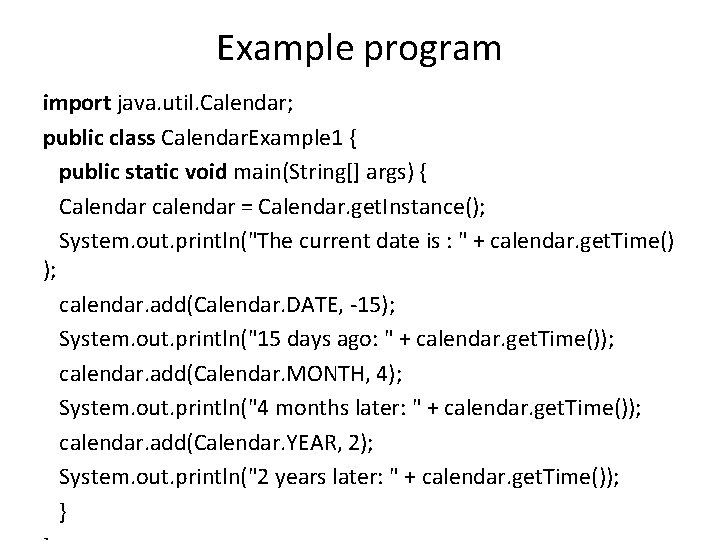
Example program import java. util. Calendar; public class Calendar. Example 1 { public static void main(String[] args) { Calendar calendar = Calendar. get. Instance(); System. out. println("The current date is : " + calendar. get. Time() ); calendar. add(Calendar. DATE, -15); System. out. println("15 days ago: " + calendar. get. Time()); calendar. add(Calendar. MONTH, 4); System. out. println("4 months later: " + calendar. get. Time()); calendar. add(Calendar. YEAR, 2); System. out. println("2 years later: " + calendar. get. Time()); }
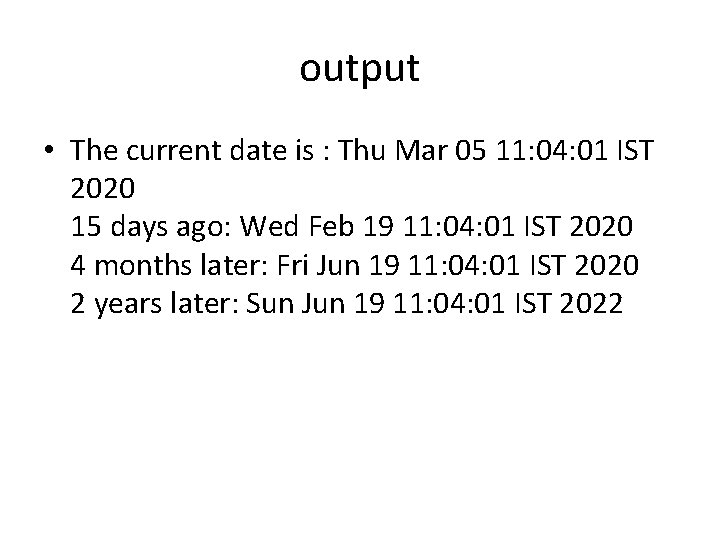
output • The current date is : Thu Mar 05 11: 04: 01 IST 2020 15 days ago: Wed Feb 19 11: 04: 01 IST 2020 4 months later: Fri Jun 19 11: 04: 01 IST 2020 2 years later: Sun Jun 19 11: 04: 01 IST 2022
![import java util public class Calendar Example 2 public static void mainString args import java. util. *; public class Calendar. Example 2{ public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/145e052fe11afa00f1487bd03a4f5edf/image-121.jpg)
import java. util. *; public class Calendar. Example 2{ public static void main(String[] args) { Calendar calendar = Calendar. get. Instance(); System. out. println("At present Calendar's Year: " + calendar. get(Calendar. YEAR)); • System. out. println("At present Calendar's Day: " + calendar. get(Calendar. DATE)); • } output: • • •
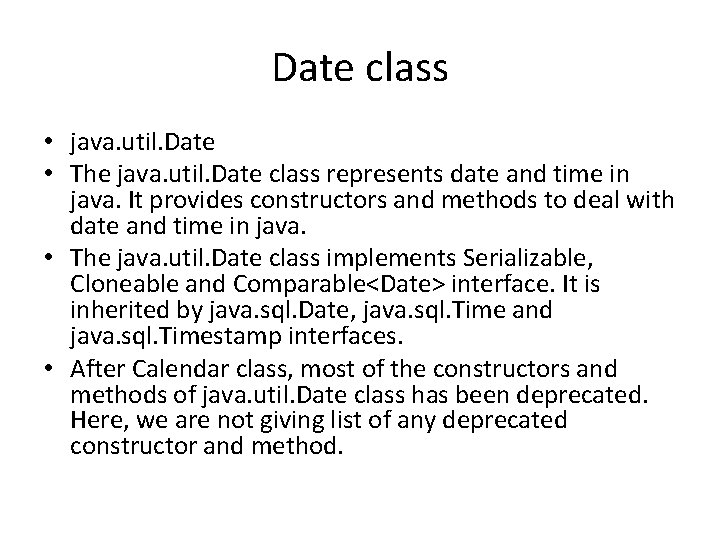
Date class • java. util. Date • The java. util. Date class represents date and time in java. It provides constructors and methods to deal with date and time in java. • The java. util. Date class implements Serializable, Cloneable and Comparable<Date> interface. It is inherited by java. sql. Date, java. sql. Time and java. sql. Timestamp interfaces. • After Calendar class, most of the constructors and methods of java. util. Date class has been deprecated. Here, we are not giving list of any deprecated constructor and method.
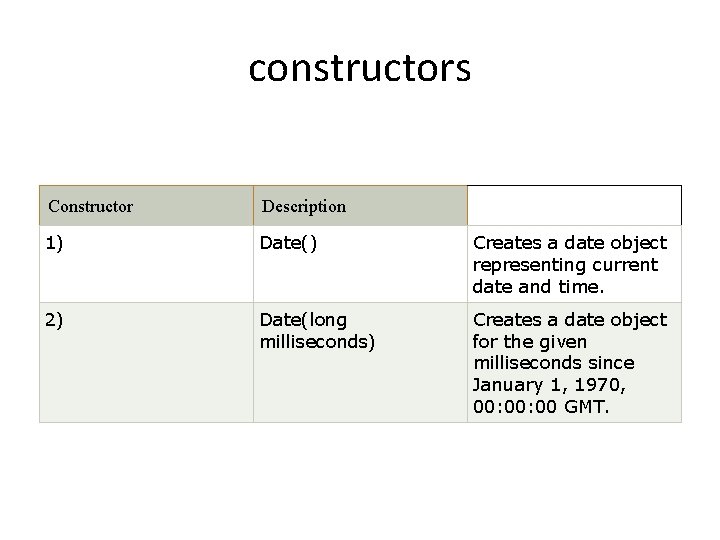
constructors Constructor Description 1) Date() Creates a date object representing current date and time. 2) Date(long milliseconds) Creates a date object for the given milliseconds since January 1, 1970, 00: 00 GMT.
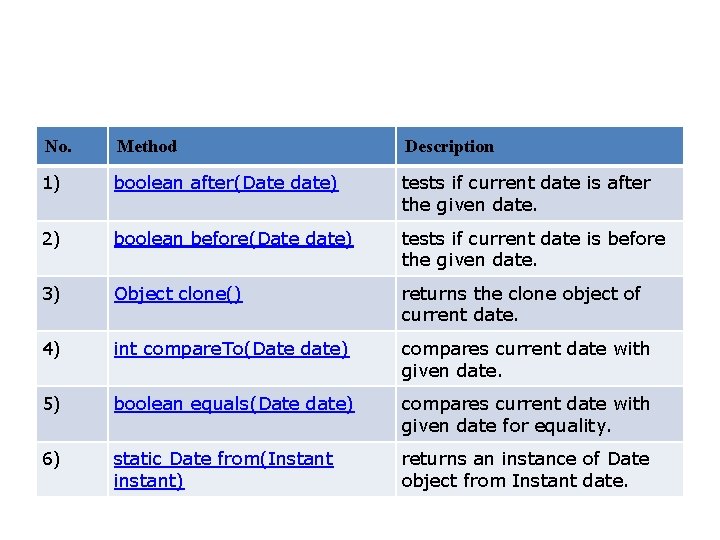
No. Method Description 1) boolean after(Date date) tests if current date is after the given date. 2) boolean before(Date date) tests if current date is before the given date. 3) Object clone() returns the clone object of current date. 4) int compare. To(Date date) compares current date with given date. 5) boolean equals(Date date) compares current date with given date for equality. 6) static Date from(Instant instant) returns an instance of Date object from Instant date.
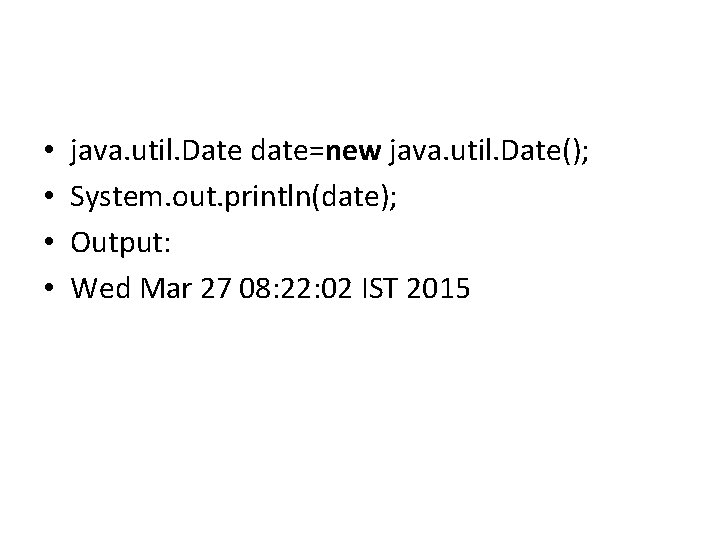
• • java. util. Date date=new java. util. Date(); System. out. println(date); Output: Wed Mar 27 08: 22: 02 IST 2015
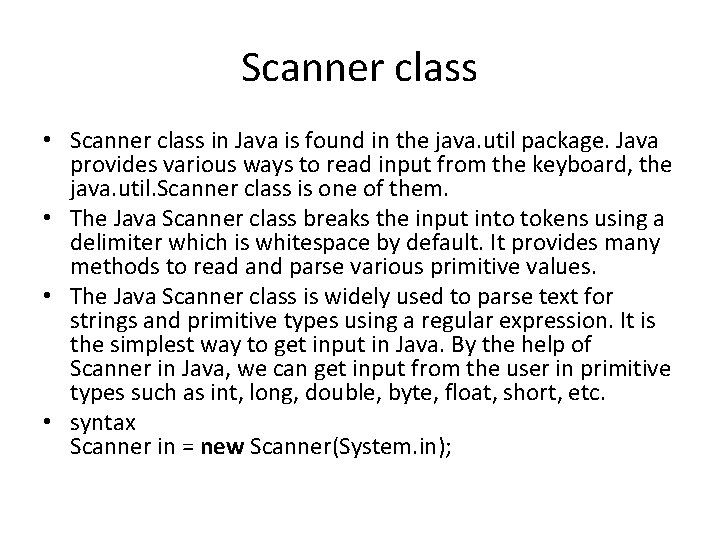
Scanner class • Scanner class in Java is found in the java. util package. Java provides various ways to read input from the keyboard, the java. util. Scanner class is one of them. • The Java Scanner class breaks the input into tokens using a delimiter which is whitespace by default. It provides many methods to read and parse various primitive values. • The Java Scanner class is widely used to parse text for strings and primitive types using a regular expression. It is the simplest way to get input in Java. By the help of Scanner in Java, we can get input from the user in primitive types such as int, long, double, byte, float, short, etc. • syntax Scanner in = new Scanner(System. in);
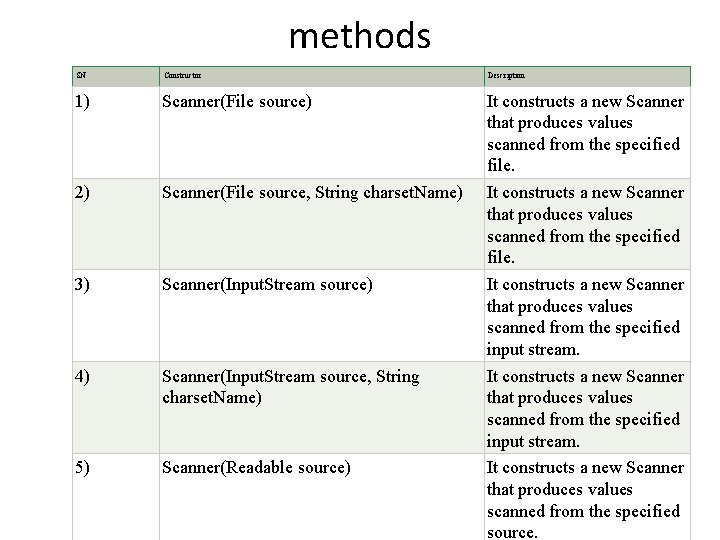
methods SN Constructor Description 1) Scanner(File source) 2) Scanner(File source, String charset. Name) 3) Scanner(Input. Stream source) 4) Scanner(Input. Stream source, String charset. Name) 5) Scanner(Readable source) It constructs a new Scanner that produces values scanned from the specified file. It constructs a new Scanner that produces values scanned from the specified input stream. It constructs a new Scanner that produces values scanned from the specified source.
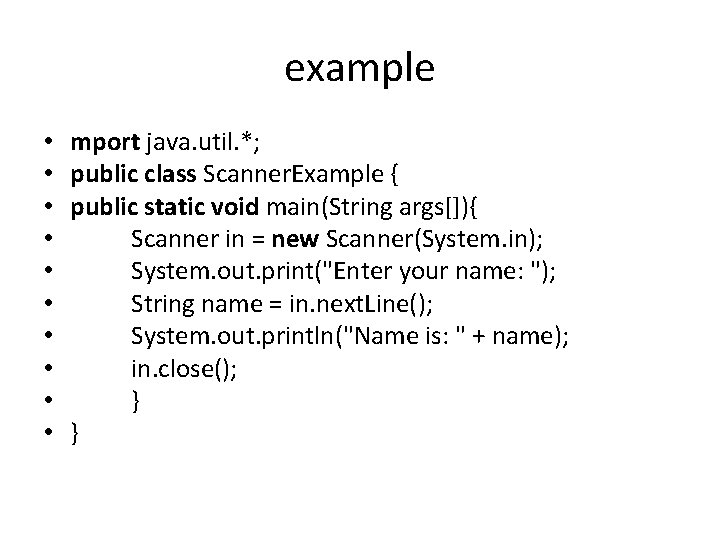
example • • • mport java. util. *; public class Scanner. Example { public static void main(String args[]){ Scanner in = new Scanner(System. in); System. out. print("Enter your name: "); String name = in. next. Line(); System. out. println("Name is: " + name); in. close(); } }
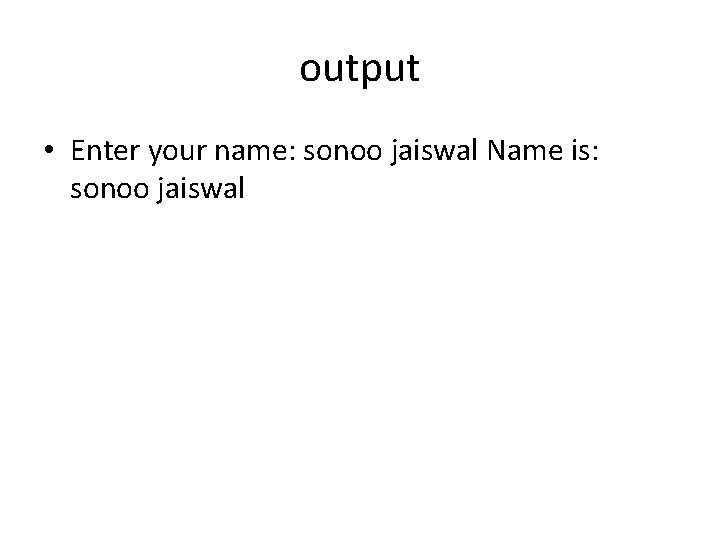
output • Enter your name: sonoo jaiswal Name is: sonoo jaiswal
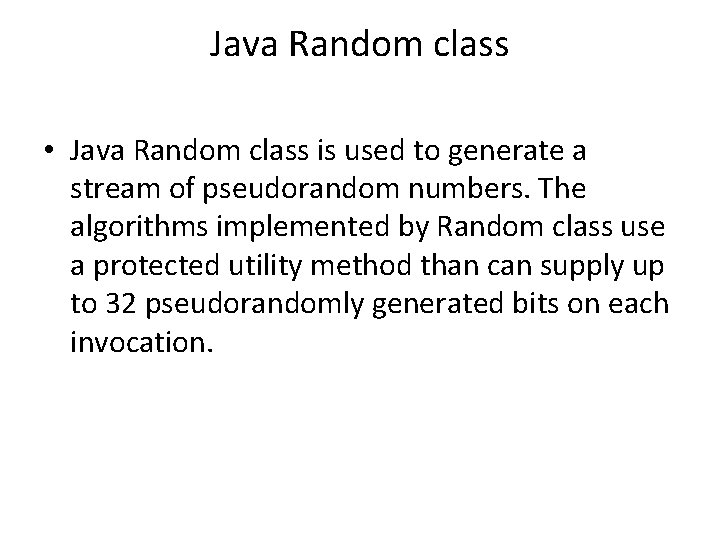
Java Random class • Java Random class is used to generate a stream of pseudorandom numbers. The algorithms implemented by Random class use a protected utility method than can supply up to 32 pseudorandomly generated bits on each invocation.
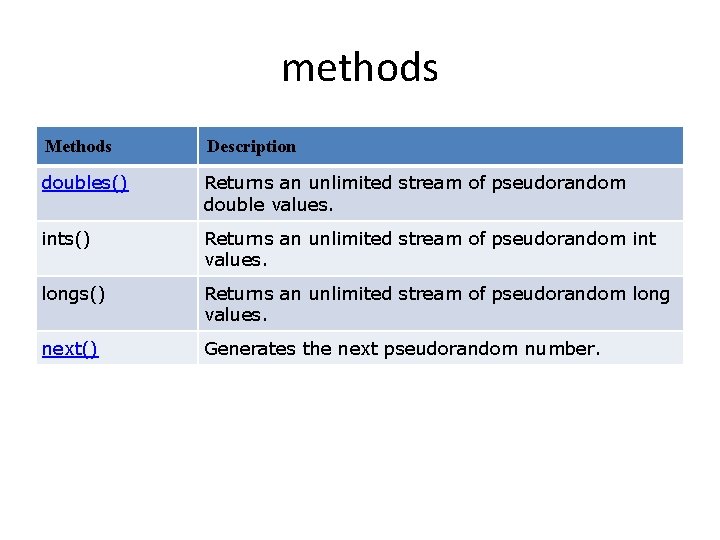
methods Methods Description doubles() Returns an unlimited stream of pseudorandom double values. ints() Returns an unlimited stream of pseudorandom int values. longs() Returns an unlimited stream of pseudorandom long values. next() Generates the next pseudorandom number.
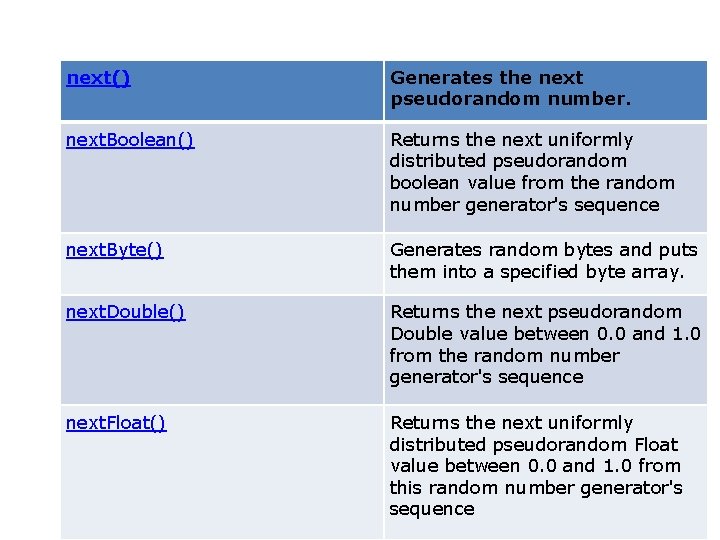
next() Generates the next pseudorandom number. next. Boolean() Returns the next uniformly distributed pseudorandom boolean value from the random number generator's sequence next. Byte() Generates random bytes and puts them into a specified byte array. next. Double() Returns the next pseudorandom Double value between 0. 0 and 1. 0 from the random number generator's sequence next. Float() Returns the next uniformly distributed pseudorandom Float value between 0. 0 and 1. 0 from this random number generator's sequence
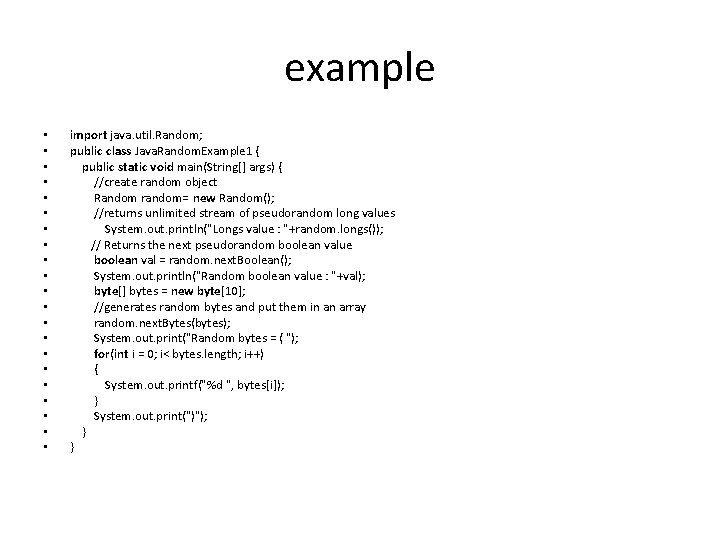
example • • • • • • import java. util. Random; public class Java. Random. Example 1 { public static void main(String[] args) { //create random object Random random= new Random(); //returns unlimited stream of pseudorandom long values System. out. println("Longs value : "+random. longs()); // Returns the next pseudorandom boolean value boolean val = random. next. Boolean(); System. out. println("Random boolean value : "+val); byte[] bytes = new byte[10]; //generates random bytes and put them in an array random. next. Bytes(bytes); System. out. print("Random bytes = ( "); for(int i = 0; i< bytes. length; i++) { System. out. printf("%d ", bytes[i]); } System. out. print(")"); } }
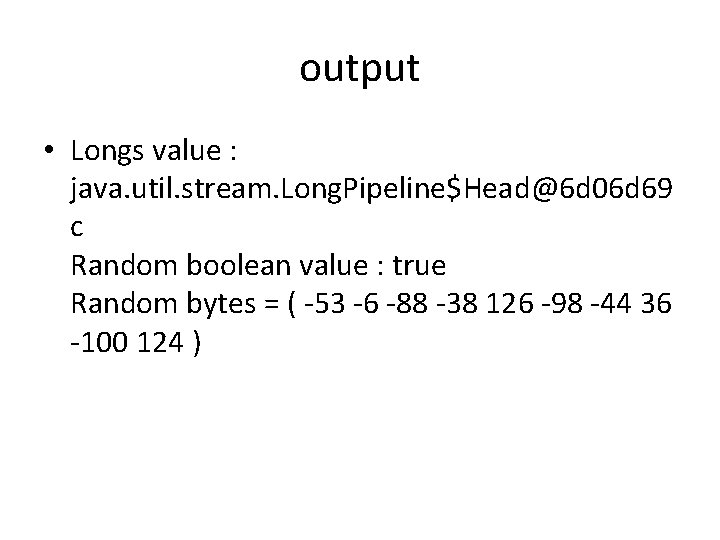
output • Longs value : java. util. stream. Long. Pipeline$Head@6 d 06 d 69 c Random boolean value : true Random bytes = ( -53 -6 -88 -38 126 -98 -44 36 -100 124 )
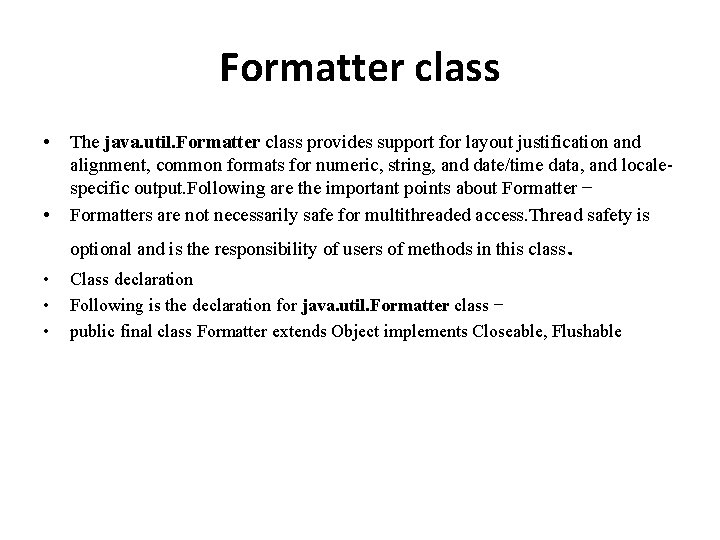
Formatter class • • The java. util. Formatter class provides support for layout justification and alignment, common formats for numeric, string, and date/time data, and localespecific output. Following are the important points about Formatter − Formatters are not necessarily safe for multithreaded access. Thread safety is optional and is the responsibility of users of methods in this class • • • . Class declaration Following is the declaration for java. util. Formatter class − public final class Formatter extends Object implements Closeable, Flushable
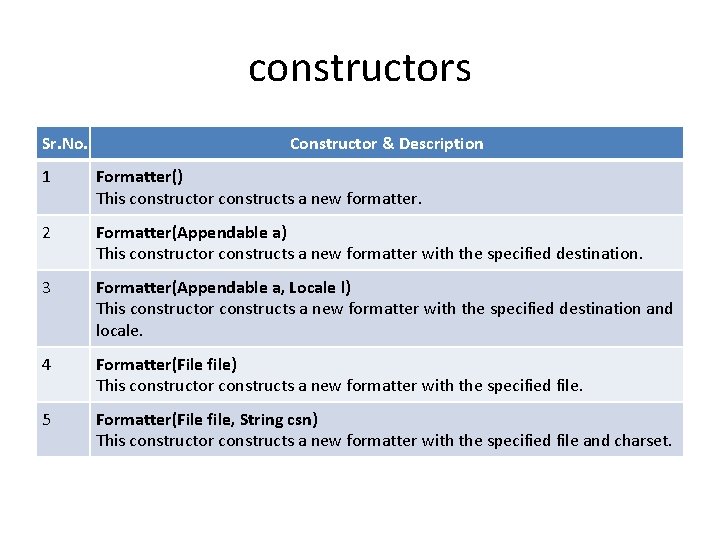
constructors Sr. No. Constructor & Description 1 Formatter() This constructor constructs a new formatter. 2 Formatter(Appendable a) This constructor constructs a new formatter with the specified destination. 3 Formatter(Appendable a, Locale l) This constructor constructs a new formatter with the specified destination and locale. 4 Formatter(File file) This constructor constructs a new formatter with the specified file. 5 Formatter(File file, String csn) This constructor constructs a new formatter with the specified file and charset.
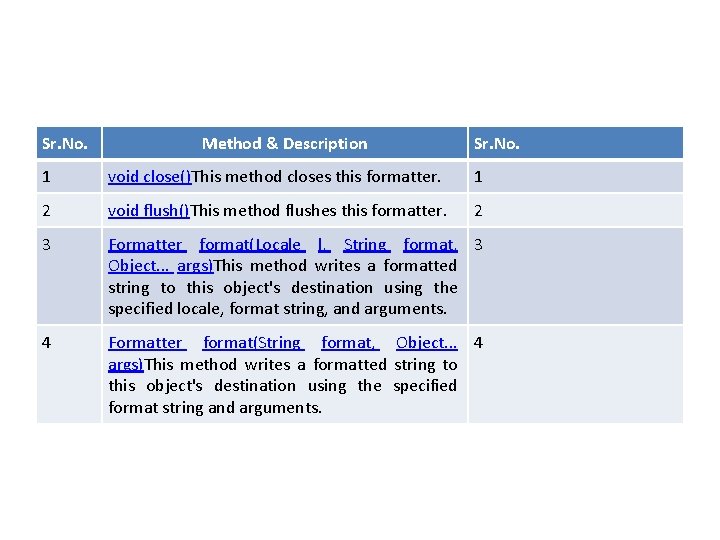
Sr. No. Method & Description Sr. No. 1 void close()This method closes this formatter. 1 2 void flush()This method flushes this formatter. 2 3 Formatter format(Locale l, String format, 3 Object. . . args)This method writes a formatted string to this object's destination using the specified locale, format string, and arguments. 4 Formatter format(String format, Object. . . 4 args)This method writes a formatted string to this object's destination using the specified format string and arguments.
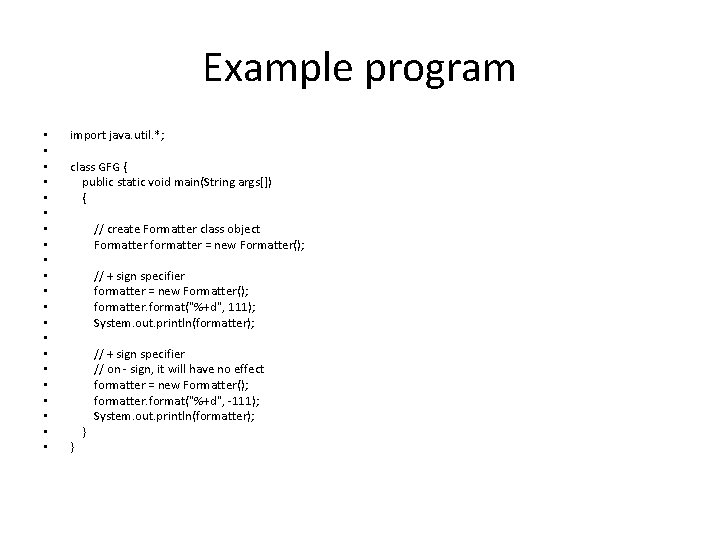
Example program • • • • • • import java. util. *; class GFG { public static void main(String args[]) { // create Formatter class object Formatter formatter = new Formatter(); // + sign specifier formatter = new Formatter(); formatter. format("%+d", 111); System. out. println(formatter); // + sign specifier // on - sign, it will have no effect formatter = new Formatter(); formatter. format("%+d", -111); System. out. println(formatter); } }
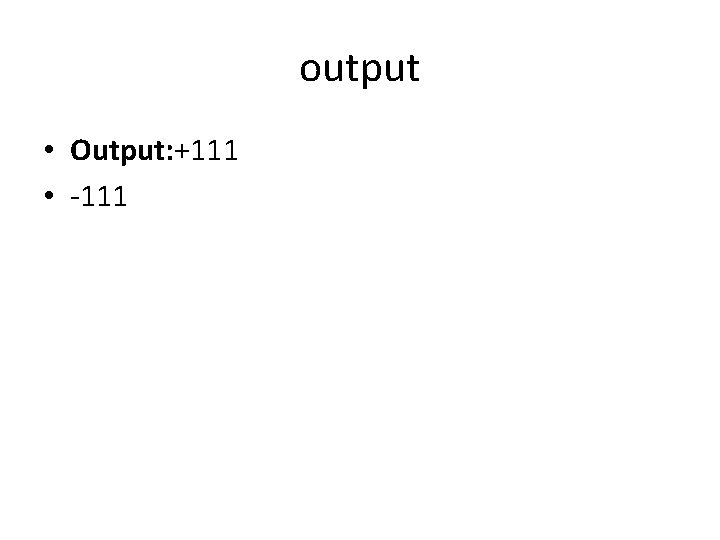
output • Output: +111 • -111