Introduction to Open GL and GLUT What is
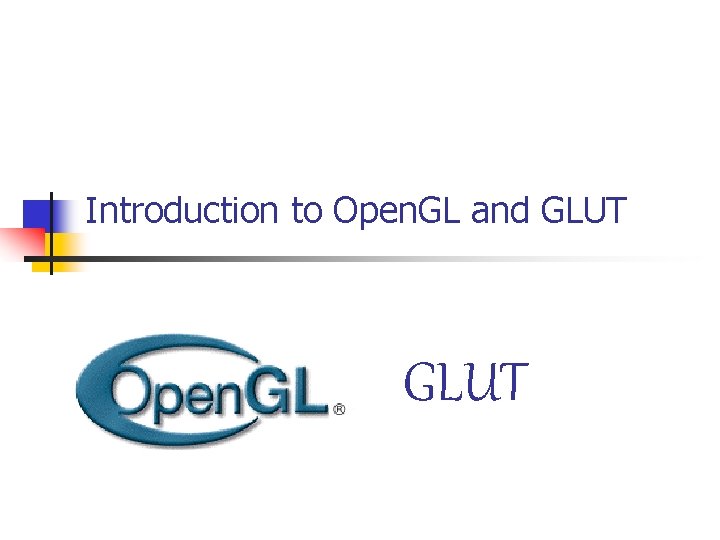
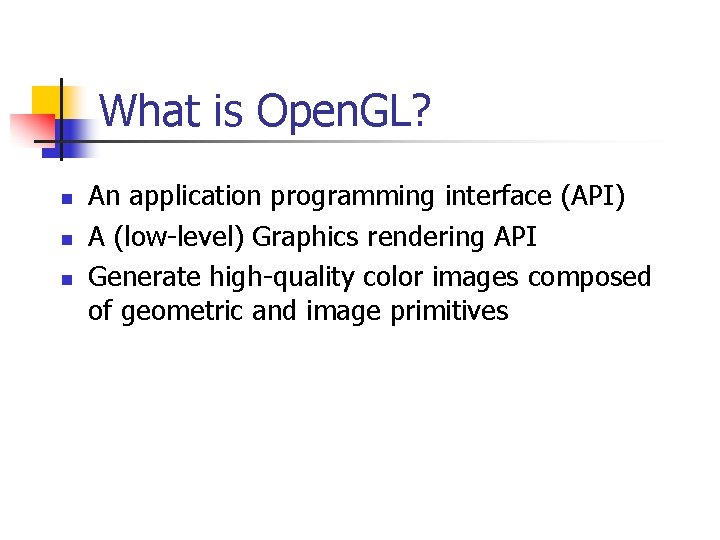
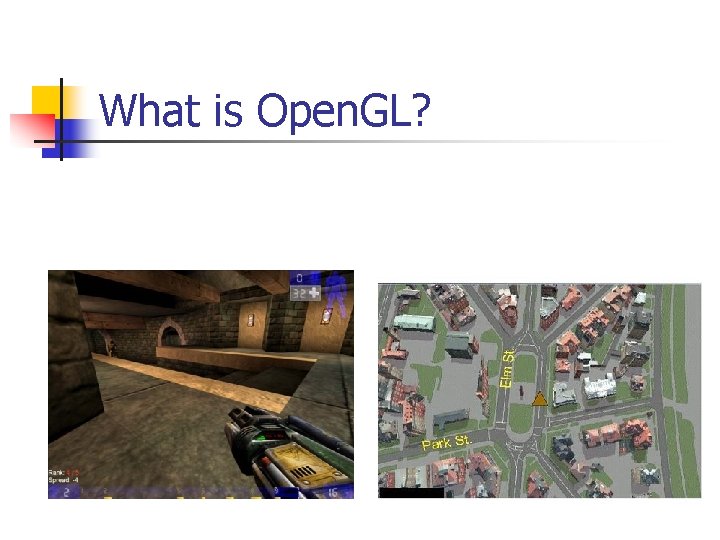
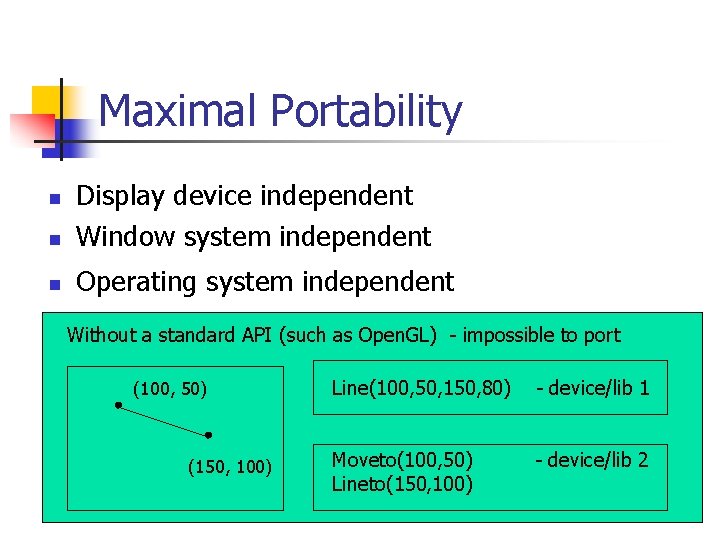
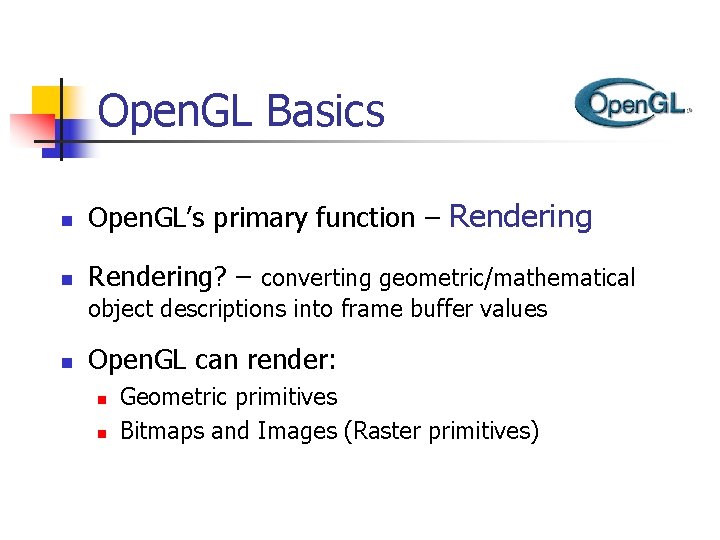
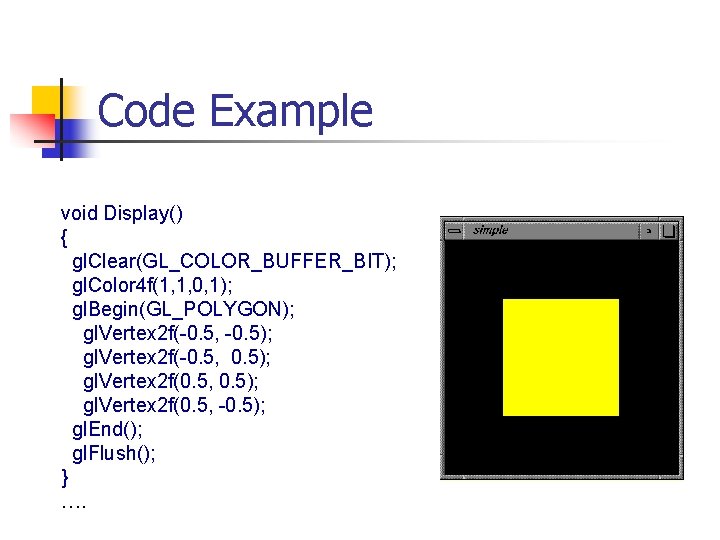
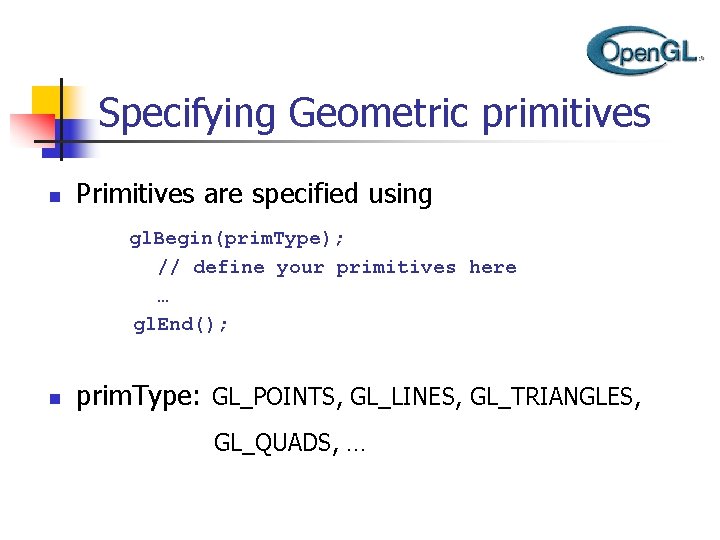
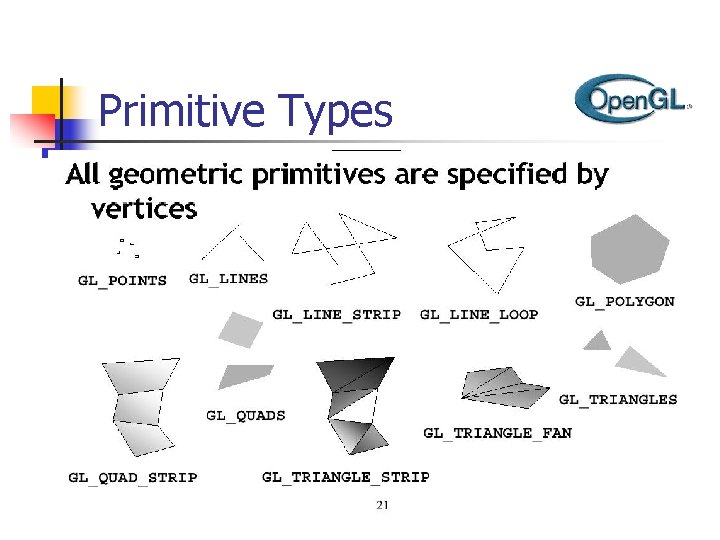
![Sample Example Void Draw. Quad(GLfloat color[]) { gl. Color 3 f(0, 0, 1); gl. Sample Example Void Draw. Quad(GLfloat color[]) { gl. Color 3 f(0, 0, 1); gl.](https://slidetodoc.com/presentation_image_h2/008615199f61dc361bb4f4d236901471/image-9.jpg)
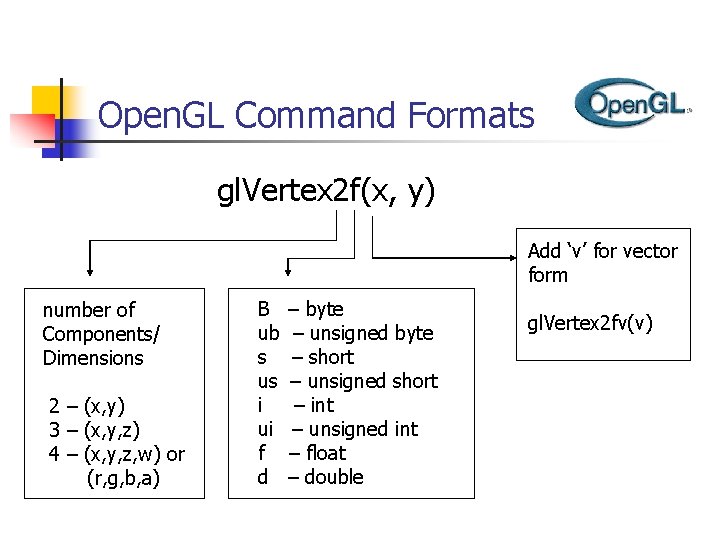
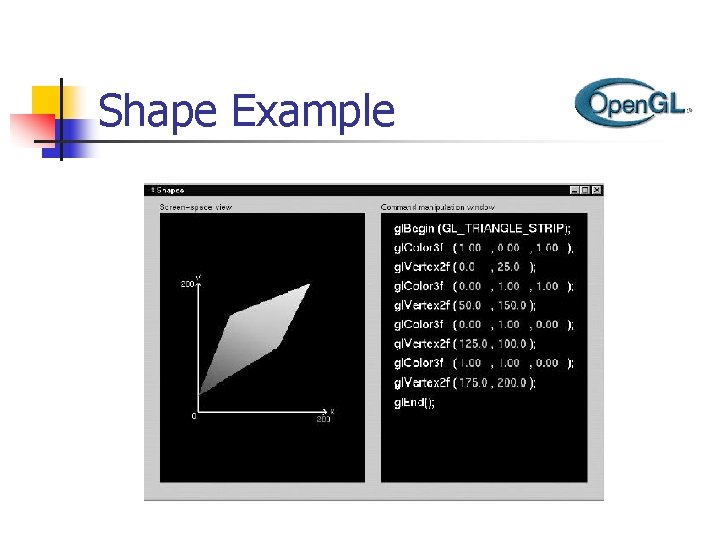
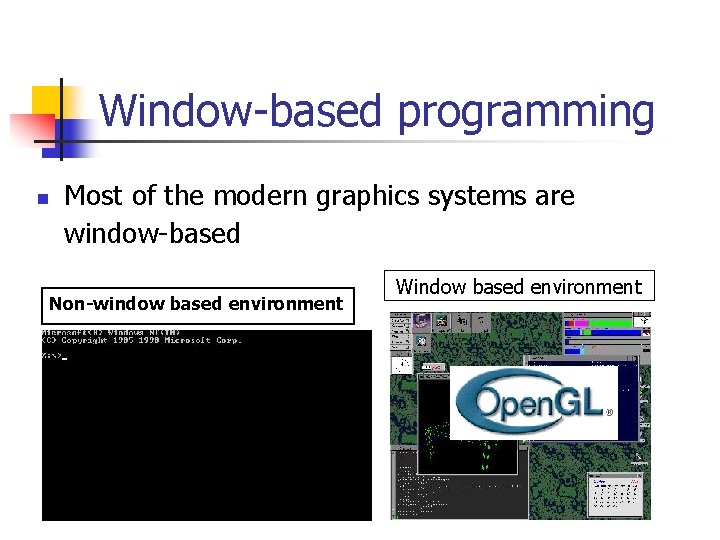
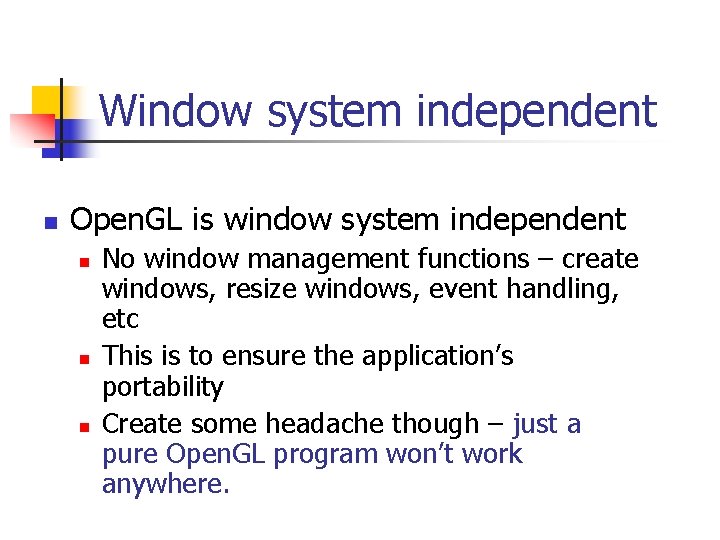
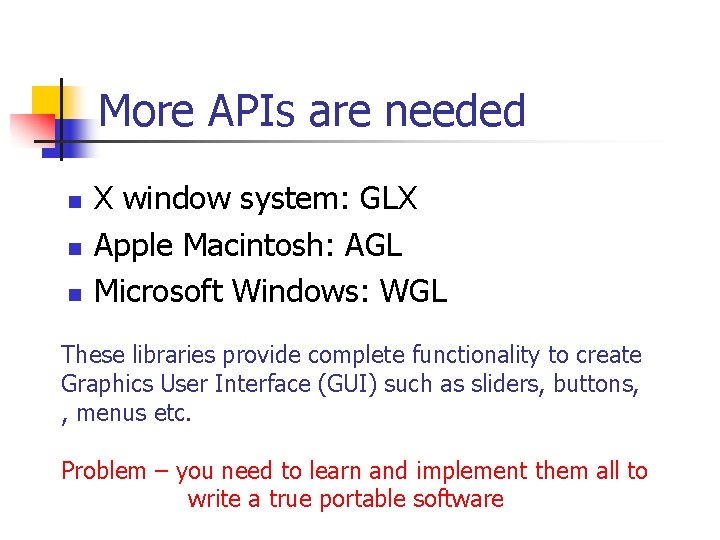
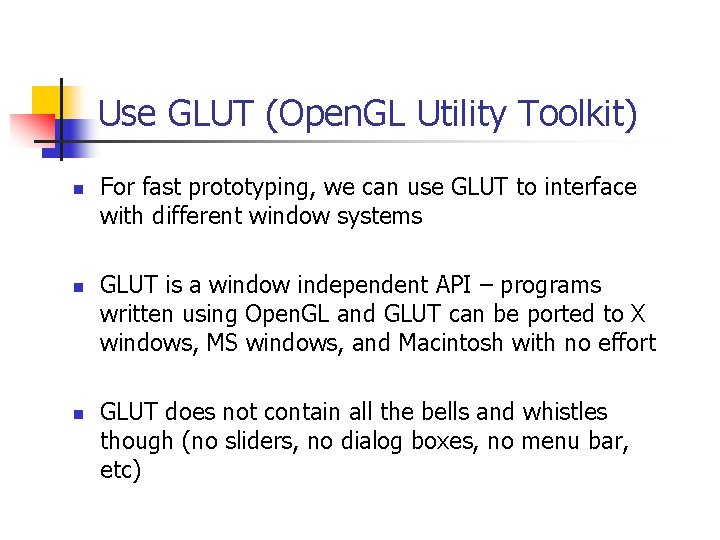
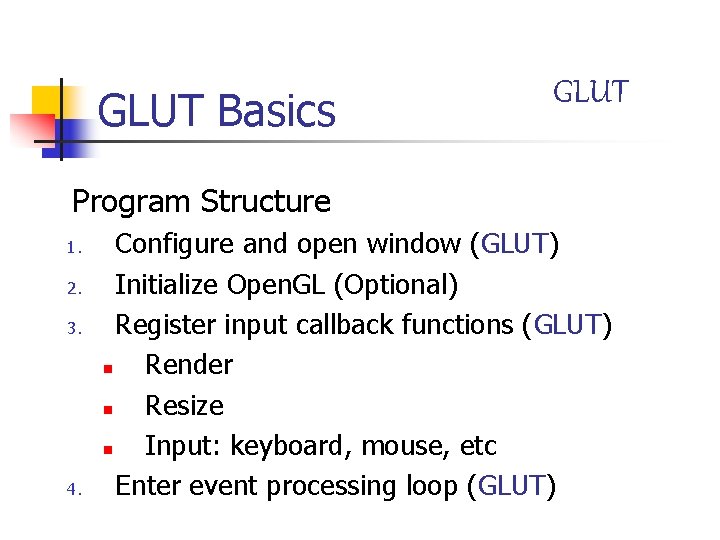
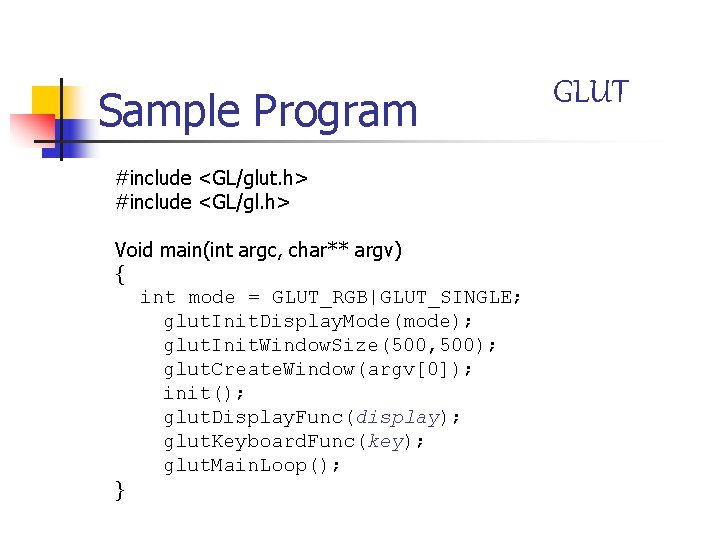
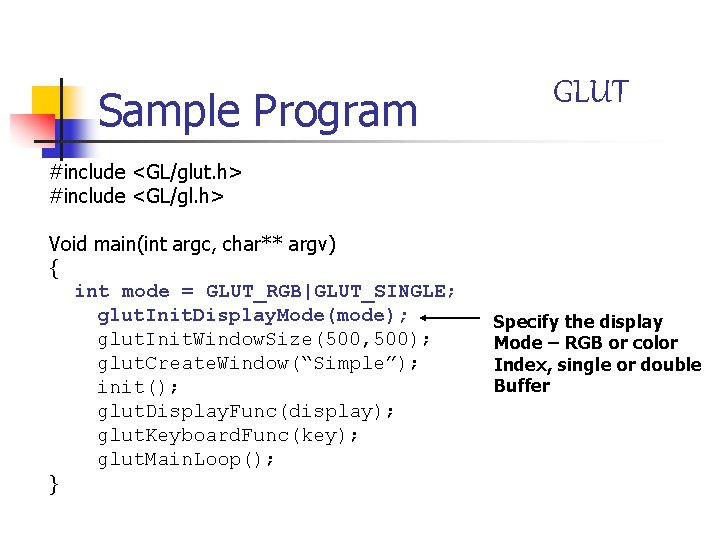
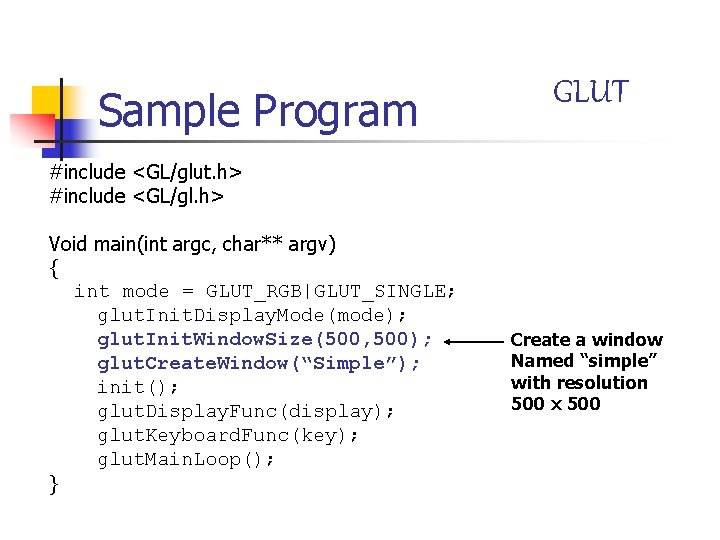
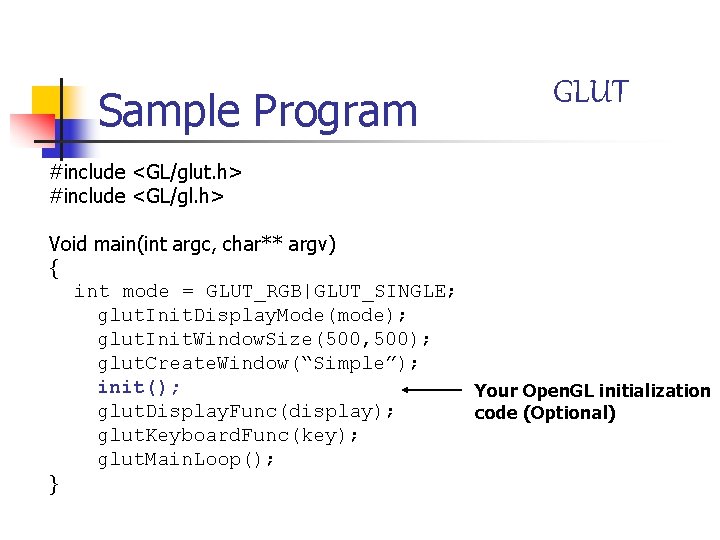
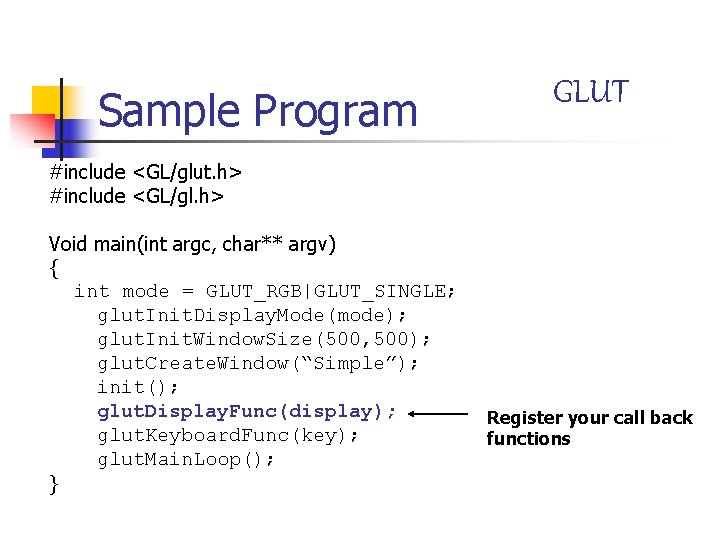
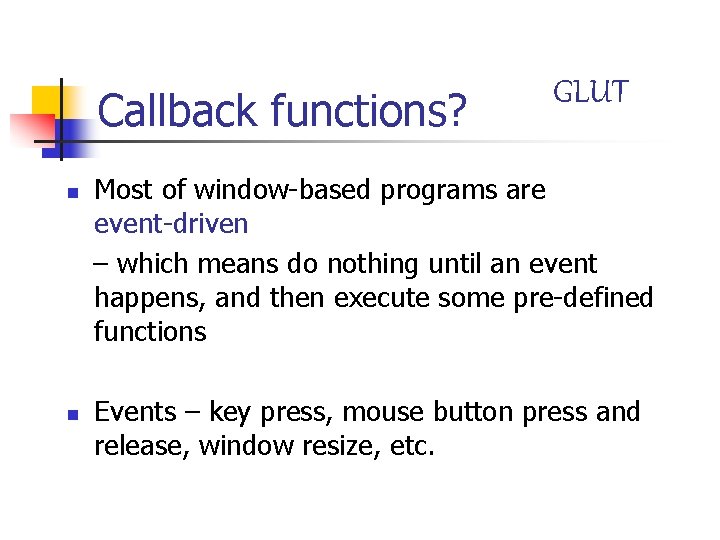
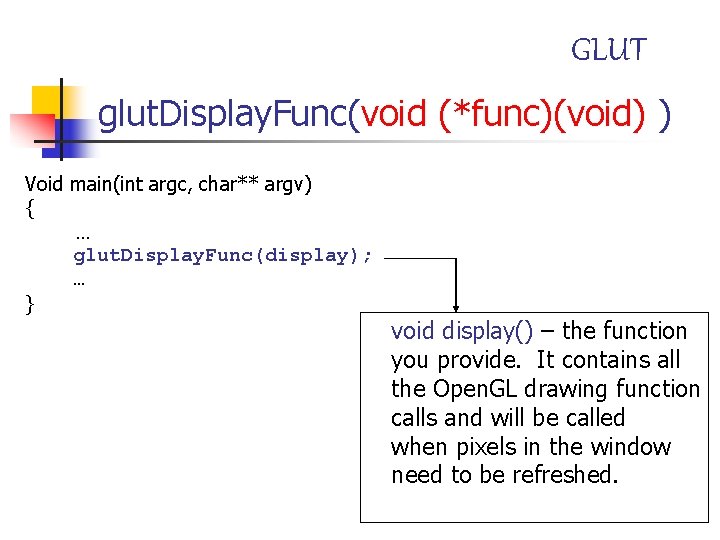
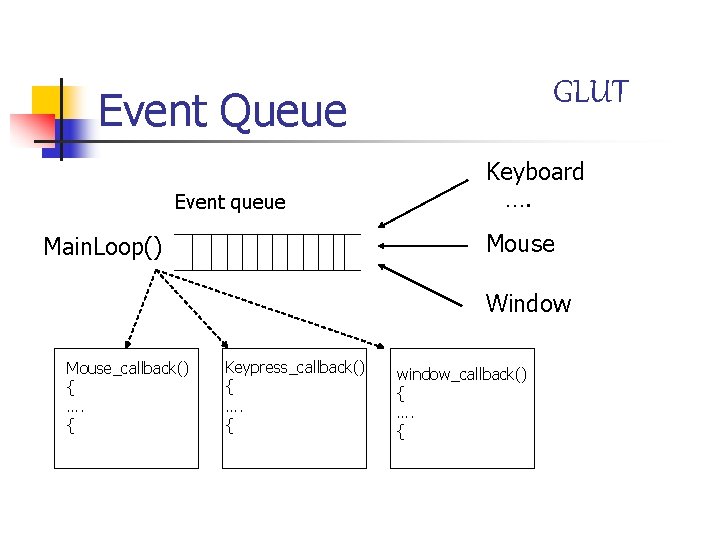
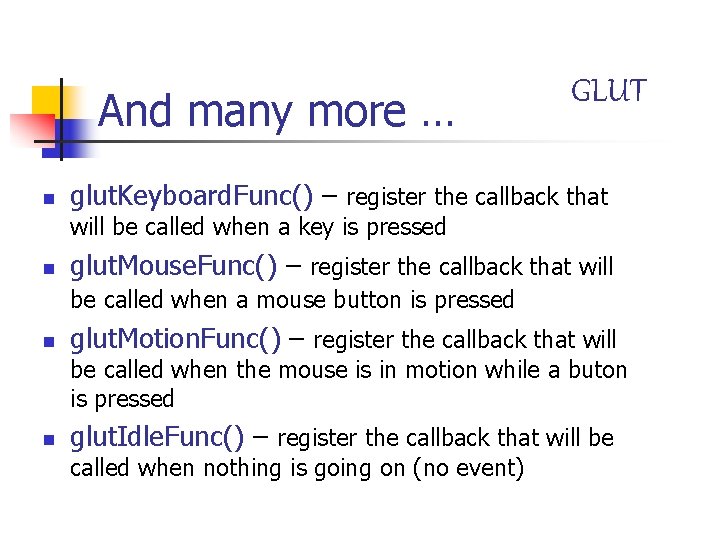
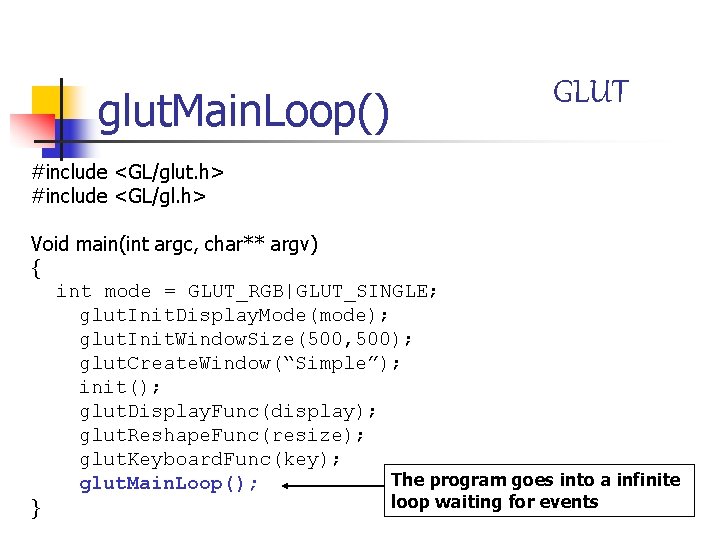
- Slides: 26
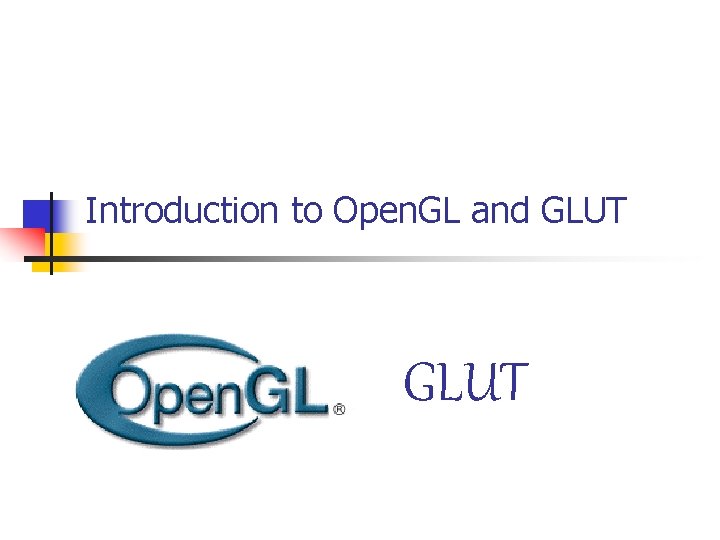
Introduction to Open. GL and GLUT
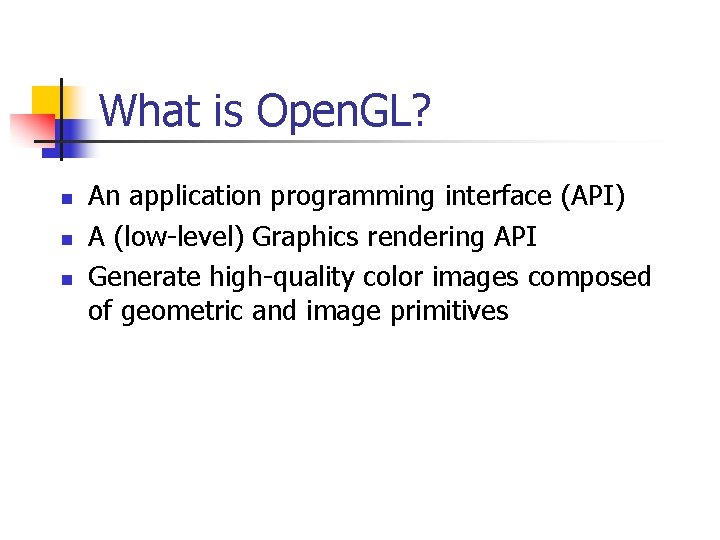
What is Open. GL? n n n An application programming interface (API) A (low-level) Graphics rendering API Generate high-quality color images composed of geometric and image primitives
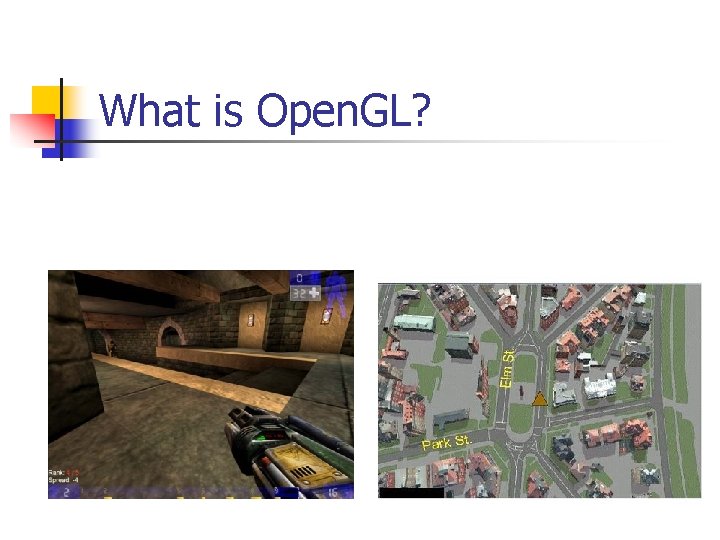
What is Open. GL?
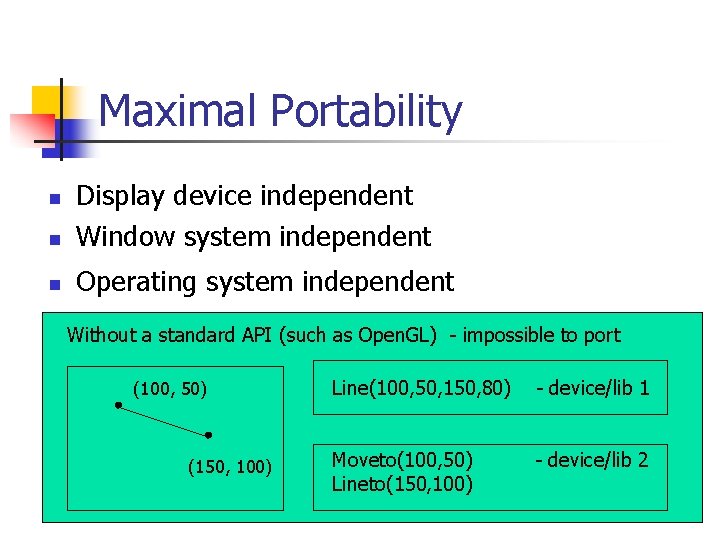
Maximal Portability n Display device independent Window system independent n Operating system independent n Without a standard API (such as Open. GL) - impossible to port (100, 50) (150, 100) Line(100, 50, 150, 80) - device/lib 1 Moveto(100, 50) Lineto(150, 100) - device/lib 2
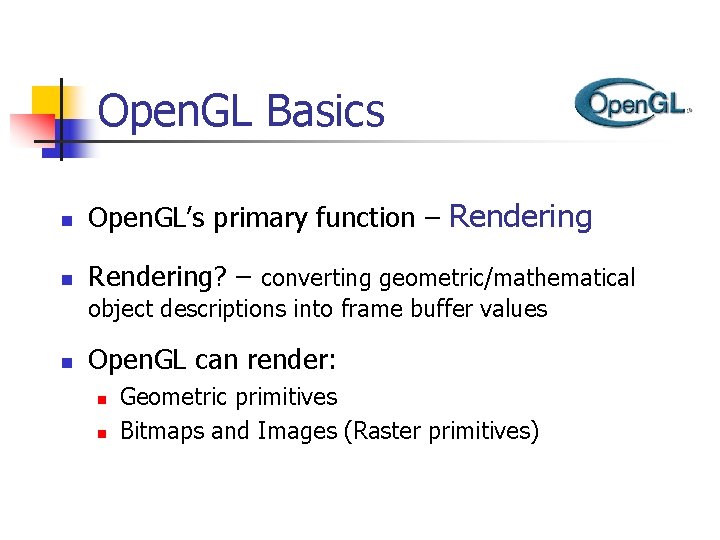
Open. GL Basics n Open. GL’s primary function – Rendering n Rendering? – converting geometric/mathematical object descriptions into frame buffer values n Open. GL can render: n n Geometric primitives Bitmaps and Images (Raster primitives)
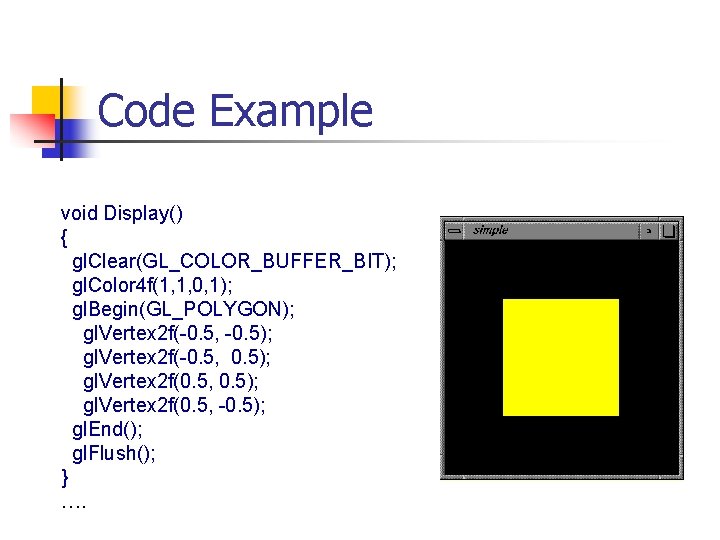
Code Example void Display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Color 4 f(1, 1, 0, 1); gl. Begin(GL_POLYGON); gl. Vertex 2 f(-0. 5, -0. 5); gl. Vertex 2 f(-0. 5, 0. 5); gl. Vertex 2 f(0. 5, -0. 5); gl. End(); gl. Flush(); } ….
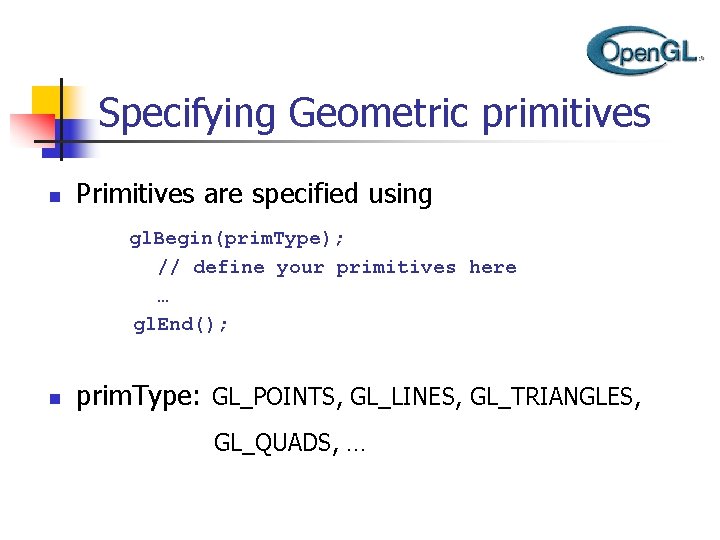
Specifying Geometric primitives n Primitives are specified using gl. Begin(prim. Type); // define your primitives here … gl. End(); n prim. Type: GL_POINTS, GL_LINES, GL_TRIANGLES, GL_QUADS, …
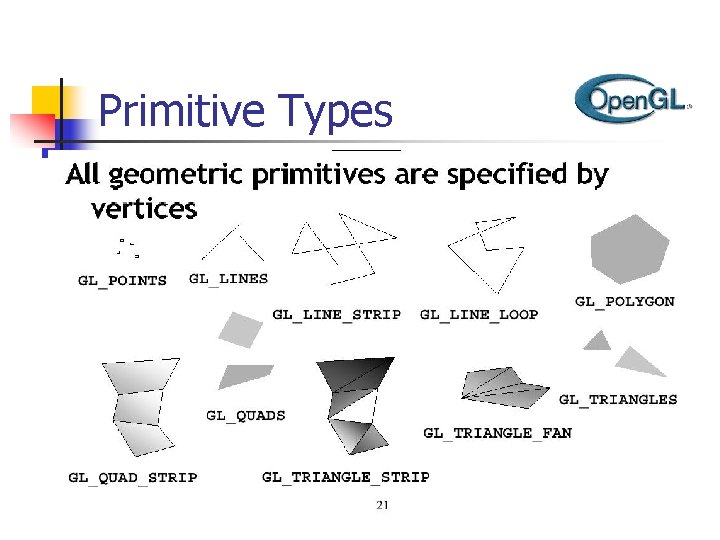
Primitive Types
![Sample Example Void Draw QuadGLfloat color gl Color 3 f0 0 1 gl Sample Example Void Draw. Quad(GLfloat color[]) { gl. Color 3 f(0, 0, 1); gl.](https://slidetodoc.com/presentation_image_h2/008615199f61dc361bb4f4d236901471/image-9.jpg)
Sample Example Void Draw. Quad(GLfloat color[]) { gl. Color 3 f(0, 0, 1); gl. Begin(GL_QUADS); gl. Vertex 2 f(0, 0); gl. Vertex 2 f(1. 0, 1. 0); gl. Vertex 2 f(0. 0, 1. 0); gl. End(); }
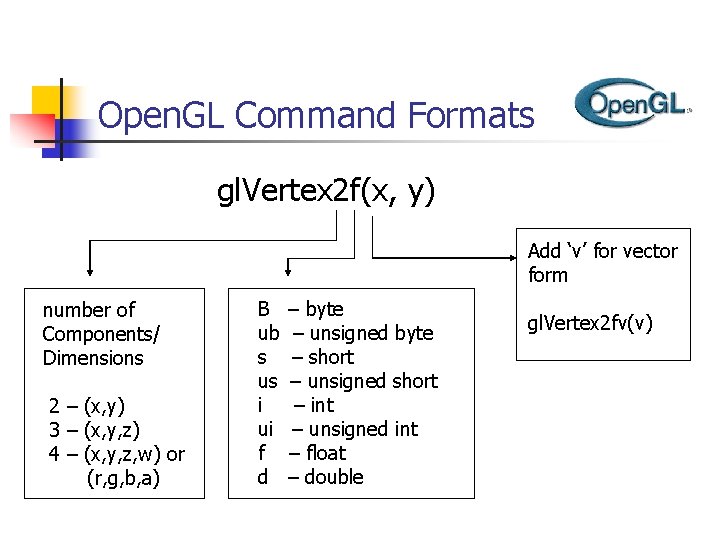
Open. GL Command Formats gl. Vertex 2 f(x, y) Add ‘v’ for vector form number of Components/ Dimensions 2 – (x, y) 3 – (x, y, z) 4 – (x, y, z, w) or (r, g, b, a) B ub s us i ui f d – byte – unsigned byte – short – unsigned short – int – unsigned int – float – double gl. Vertex 2 fv(v)
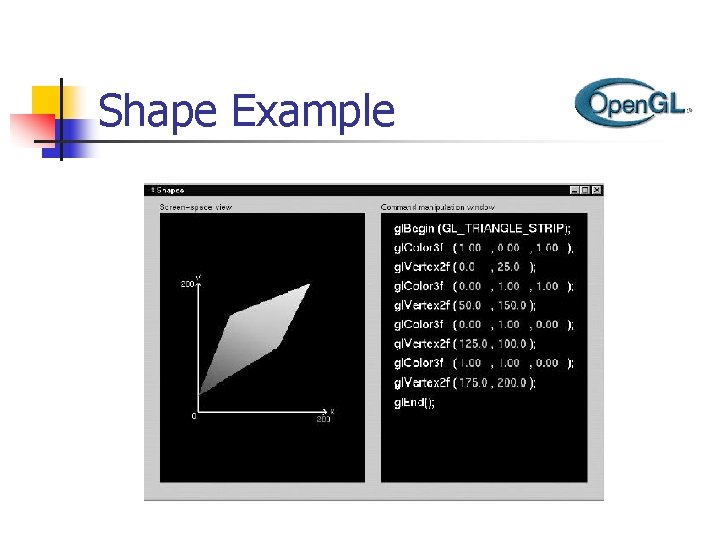
Shape Example
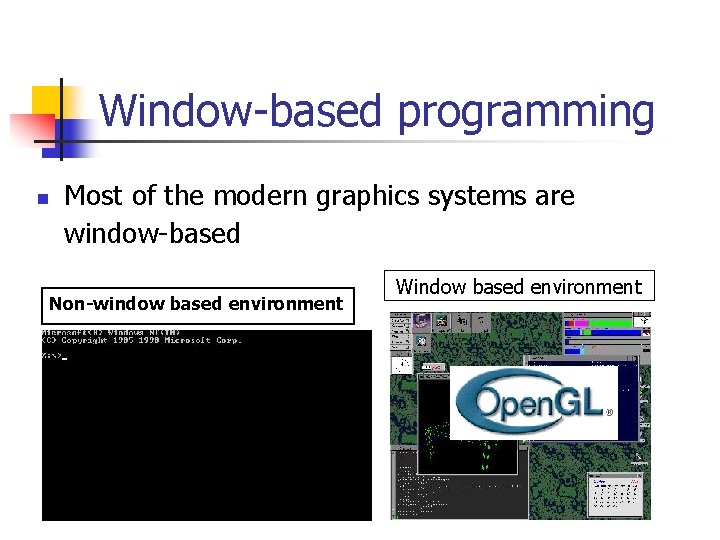
Window-based programming n Most of the modern graphics systems are window-based Non-window based environment Window based environment
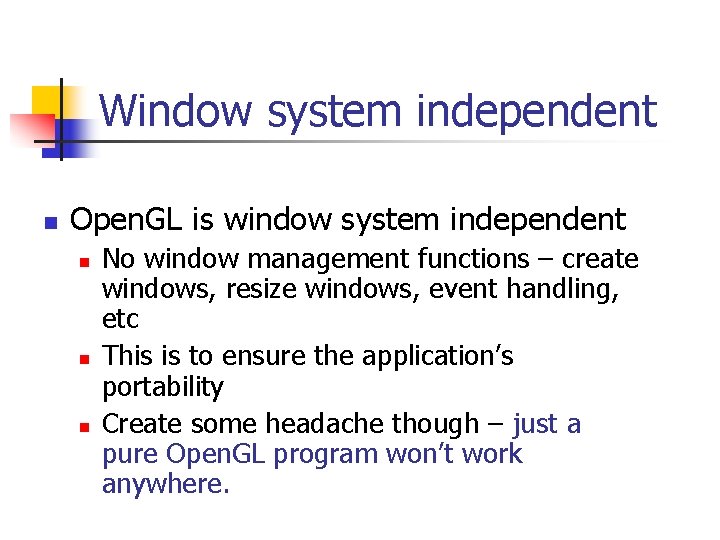
Window system independent n Open. GL is window system independent n n n No window management functions – create windows, resize windows, event handling, etc This is to ensure the application’s portability Create some headache though – just a pure Open. GL program won’t work anywhere.
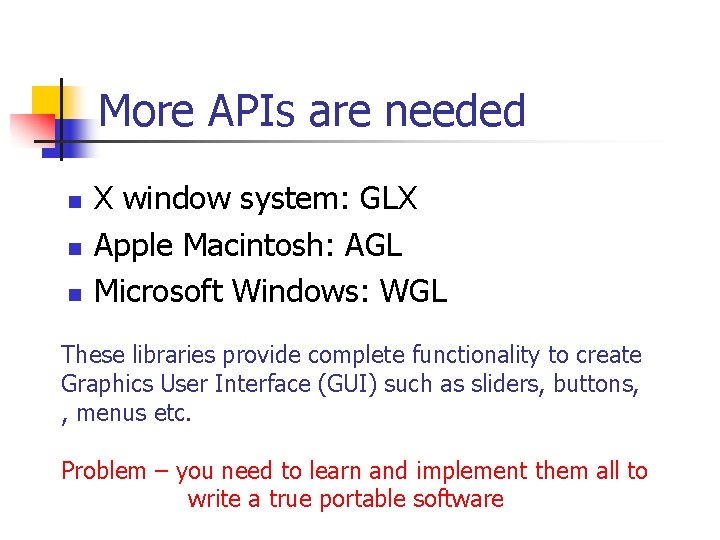
More APIs are needed n n n X window system: GLX Apple Macintosh: AGL Microsoft Windows: WGL These libraries provide complete functionality to create Graphics User Interface (GUI) such as sliders, buttons, , menus etc. Problem – you need to learn and implement them all to write a true portable software
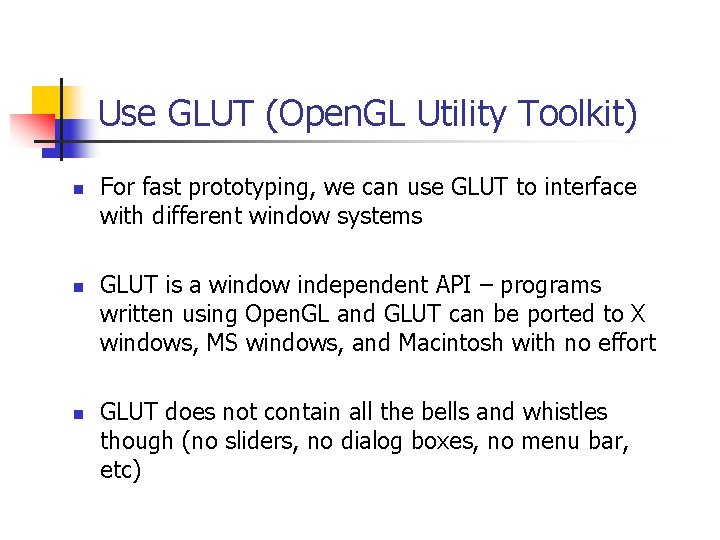
Use GLUT (Open. GL Utility Toolkit) n n n For fast prototyping, we can use GLUT to interface with different window systems GLUT is a window independent API – programs written using Open. GL and GLUT can be ported to X windows, MS windows, and Macintosh with no effort GLUT does not contain all the bells and whistles though (no sliders, no dialog boxes, no menu bar, etc)
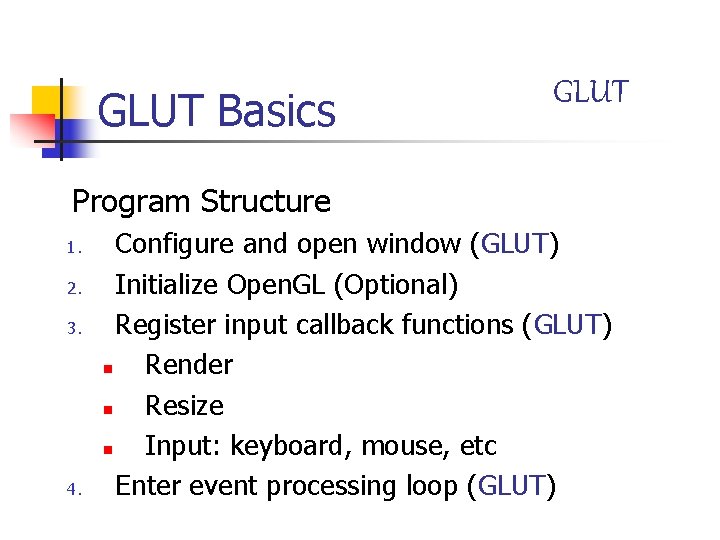
GLUT Basics GLUT Program Structure 1. 2. 3. 4. Configure and open window (GLUT) Initialize Open. GL (Optional) Register input callback functions (GLUT) n Render n Resize n Input: keyboard, mouse, etc Enter event processing loop (GLUT)
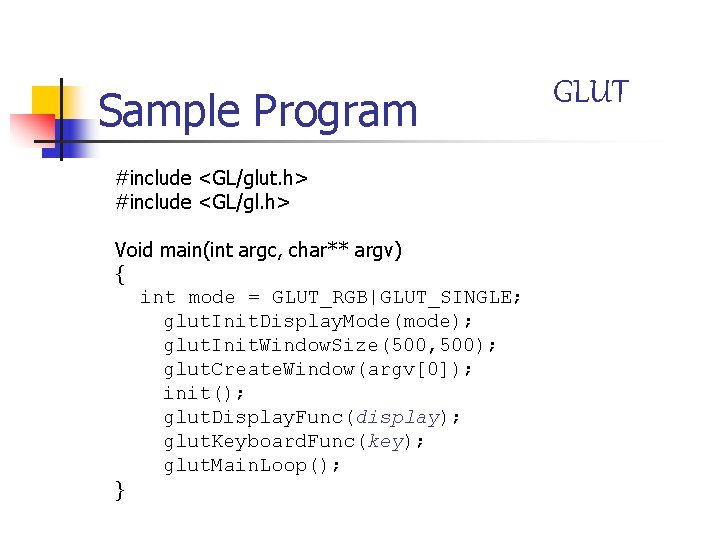
Sample Program #include <GL/glut. h> #include <GL/gl. h> Void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_SINGLE; glut. Init. Display. Mode(mode); glut. Init. Window. Size(500, 500); glut. Create. Window(argv[0]); init(); glut. Display. Func(display); glut. Keyboard. Func(key); glut. Main. Loop(); } GLUT
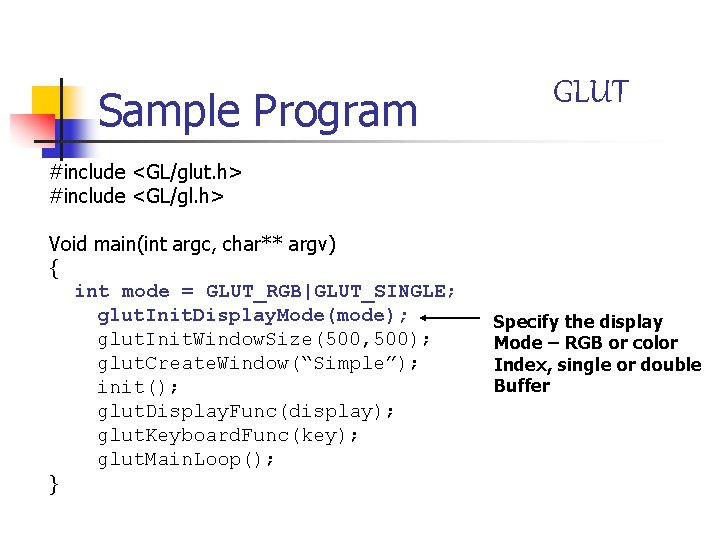
Sample Program GLUT #include <GL/glut. h> #include <GL/gl. h> Void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_SINGLE; glut. Init. Display. Mode(mode); glut. Init. Window. Size(500, 500); glut. Create. Window(“Simple”); init(); glut. Display. Func(display); glut. Keyboard. Func(key); glut. Main. Loop(); } Specify the display Mode – RGB or color Index, single or double Buffer
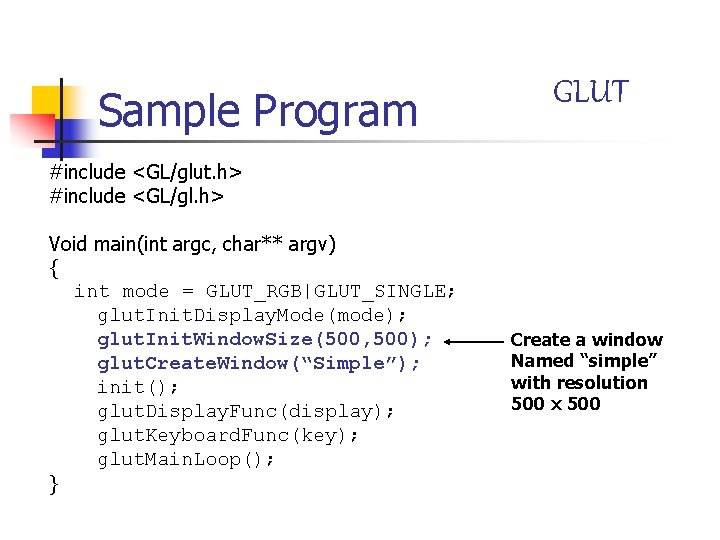
Sample Program GLUT #include <GL/glut. h> #include <GL/gl. h> Void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_SINGLE; glut. Init. Display. Mode(mode); glut. Init. Window. Size(500, 500); glut. Create. Window(“Simple”); init(); glut. Display. Func(display); glut. Keyboard. Func(key); glut. Main. Loop(); } Create a window Named “simple” with resolution 500 x 500
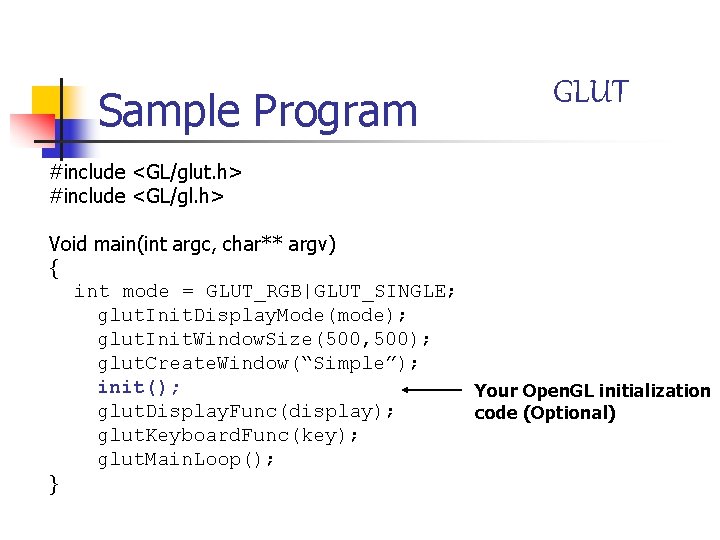
Sample Program GLUT #include <GL/glut. h> #include <GL/gl. h> Void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_SINGLE; glut. Init. Display. Mode(mode); glut. Init. Window. Size(500, 500); glut. Create. Window(“Simple”); init(); Your Open. GL initialization glut. Display. Func(display); code (Optional) glut. Keyboard. Func(key); glut. Main. Loop(); }
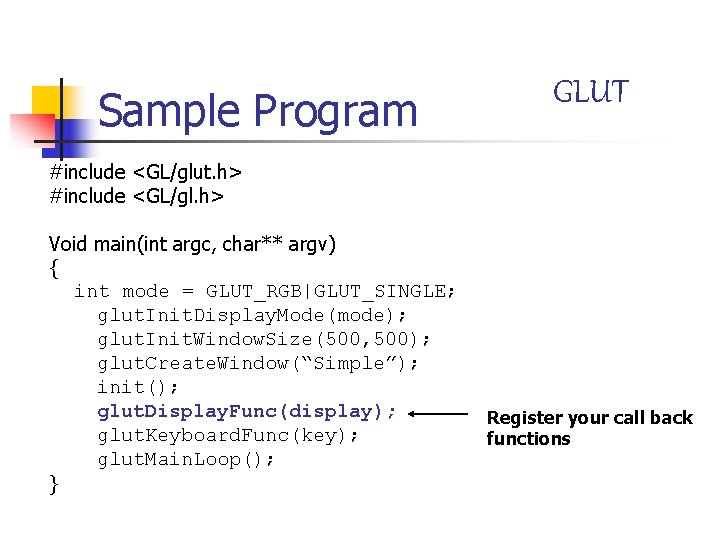
Sample Program GLUT #include <GL/glut. h> #include <GL/gl. h> Void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_SINGLE; glut. Init. Display. Mode(mode); glut. Init. Window. Size(500, 500); glut. Create. Window(“Simple”); init(); glut. Display. Func(display); glut. Keyboard. Func(key); glut. Main. Loop(); } Register your call back functions
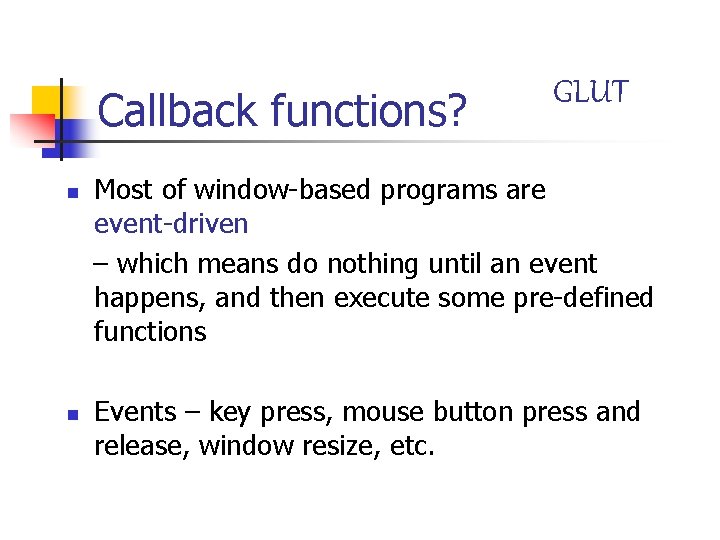
Callback functions? n n GLUT Most of window-based programs are event-driven – which means do nothing until an event happens, and then execute some pre-defined functions Events – key press, mouse button press and release, window resize, etc.
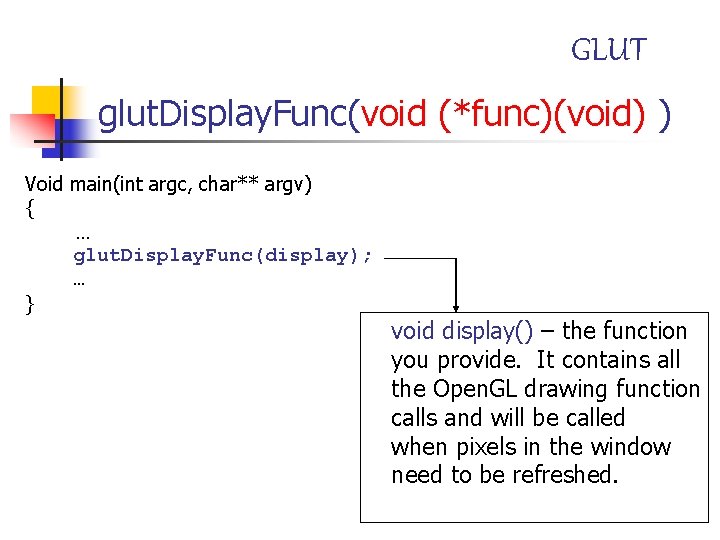
GLUT glut. Display. Func(void (*func)(void) ) Void main(int argc, char** argv) { … glut. Display. Func(display); … } void display() – the function you provide. It contains all the Open. GL drawing function calls and will be called when pixels in the window need to be refreshed.
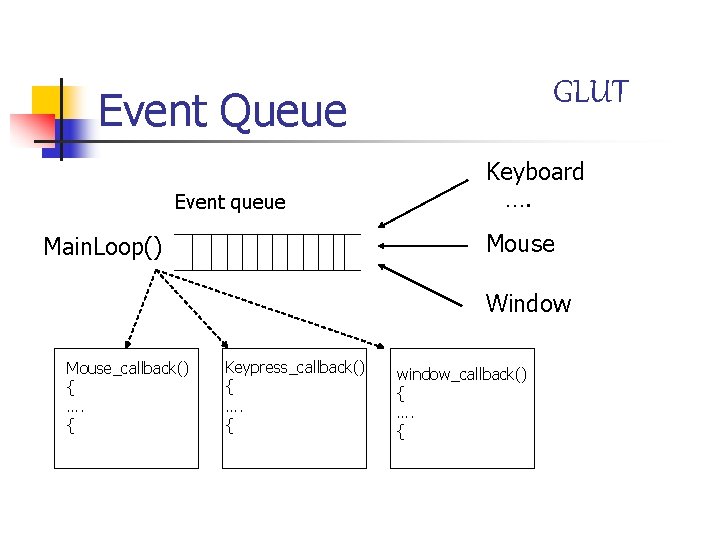
GLUT Event Queue Event queue Keyboard …. Mouse Main. Loop() Window Mouse_callback() { …. { Keypress_callback() { …. { window_callback() { …. {
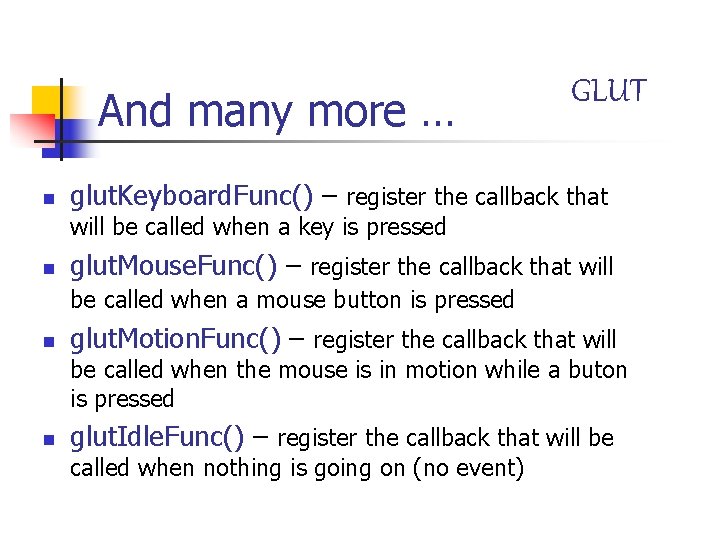
And many more … n GLUT glut. Keyboard. Func() – register the callback that will be called when a key is pressed n glut. Mouse. Func() – register the callback that will be called when a mouse button is pressed n glut. Motion. Func() – register the callback that will be called when the mouse is in motion while a buton is pressed n glut. Idle. Func() – register the callback that will be called when nothing is going on (no event)
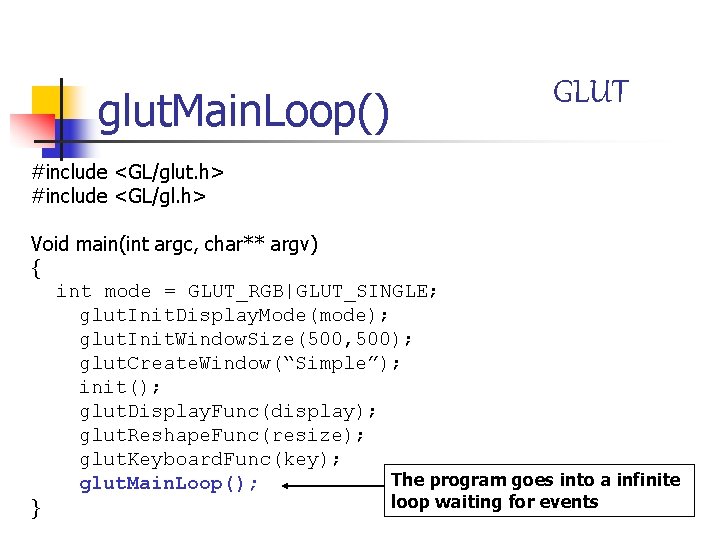
glut. Main. Loop() GLUT #include <GL/glut. h> #include <GL/gl. h> Void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_SINGLE; glut. Init. Display. Mode(mode); glut. Init. Window. Size(500, 500); glut. Create. Window(“Simple”); init(); glut. Display. Func(display); glut. Reshape. Func(resize); glut. Keyboard. Func(key); The program goes into a infinite glut. Main. Loop(); loop waiting for events }
영국 beis
Gl
Marketing information and customer insights
糖化血色素6
Glutsolid
Glutwire
Glut functions
Glut draw text
Glutattachmenu
Glutwire
Ornitin ciklus
Glut torus
Gl resources
Gl/glut.h
Glut solid torus example
Glut init
Glut is ours
Glut idle function
Insulina
Familia glut
Glut
Glut receptors
Glut_key_left
Metabolismo
Whats a glut
Glut transportery
Glut menu example