Inheritance A simple class hierarchy A class for
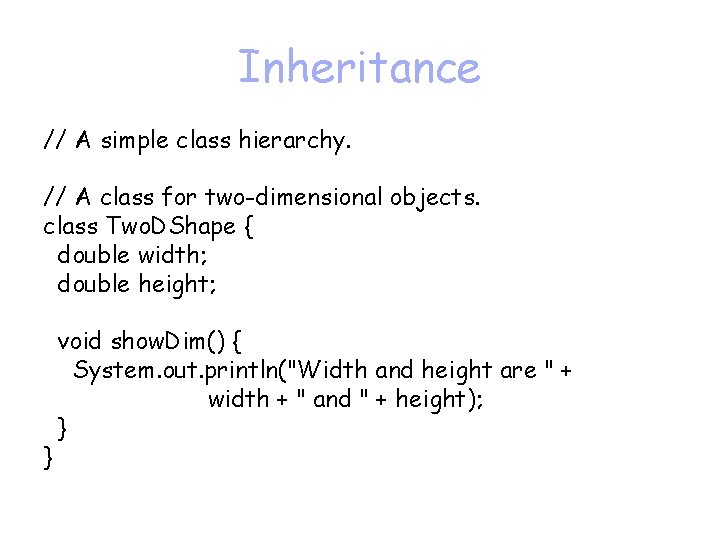
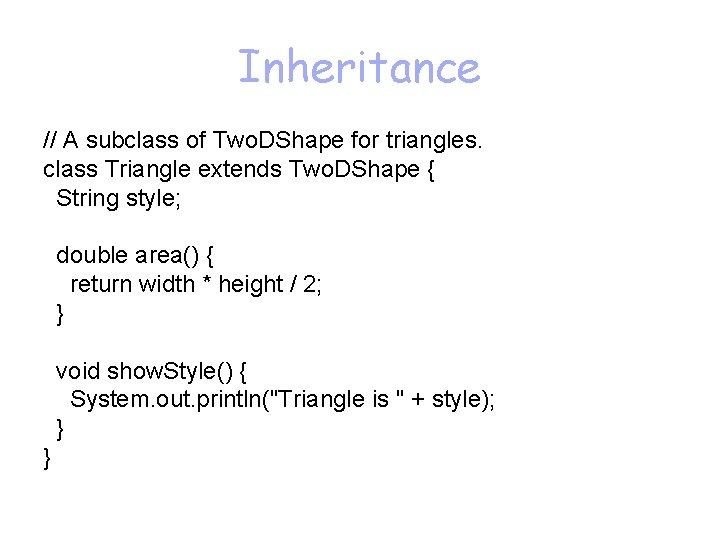
![Inheritance class Shapes { public static void main(String args[]) { Triangle t 1 = Inheritance class Shapes { public static void main(String args[]) { Triangle t 1 =](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-3.jpg)
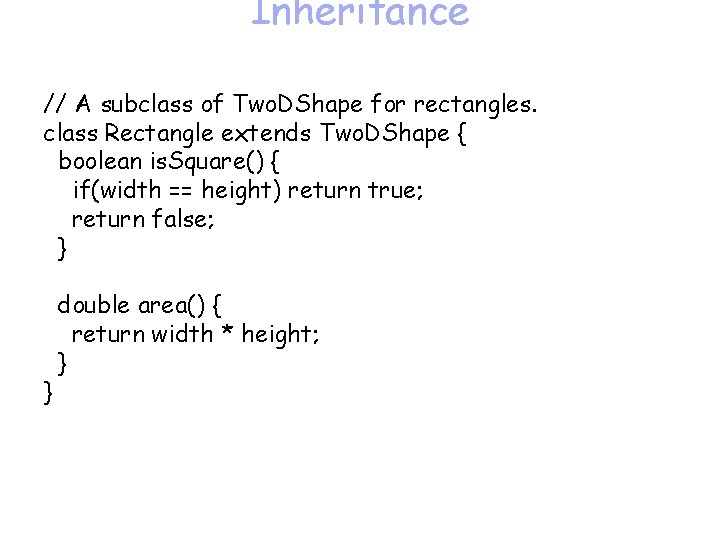
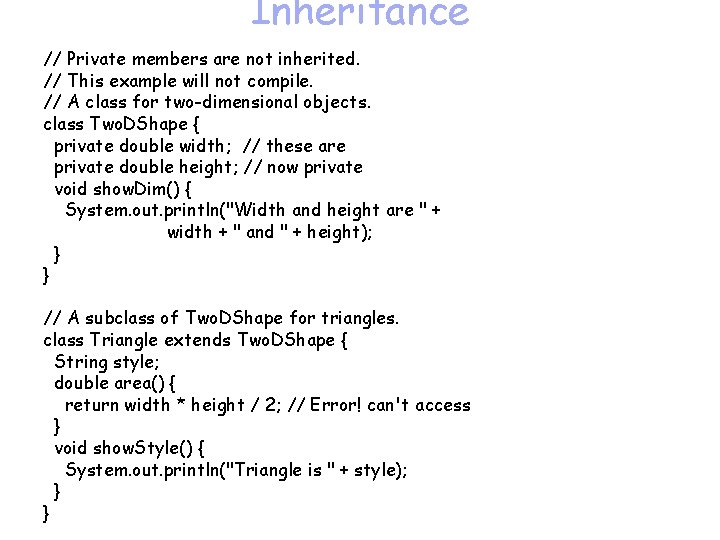
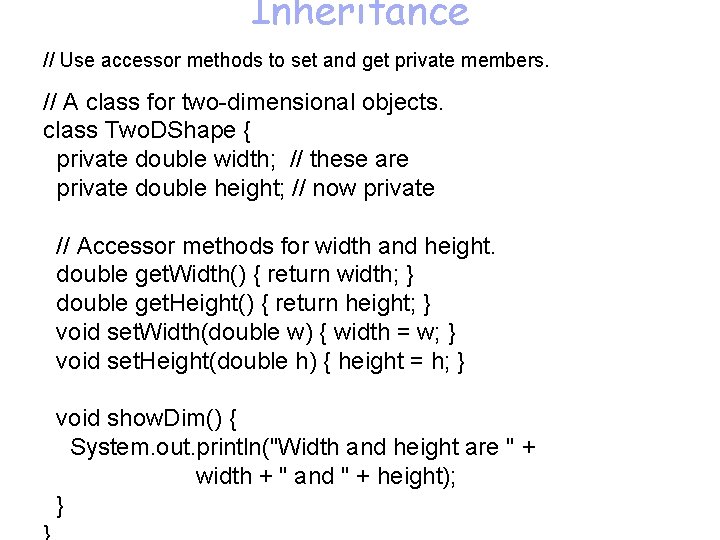
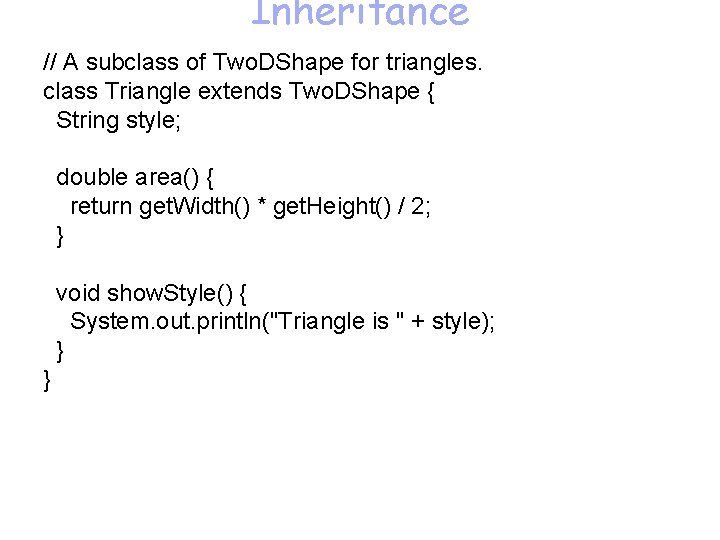
![Inheritance class Shapes 2 { public static void main(String args[]) { Triangle t 1 Inheritance class Shapes 2 { public static void main(String args[]) { Triangle t 1](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-8.jpg)
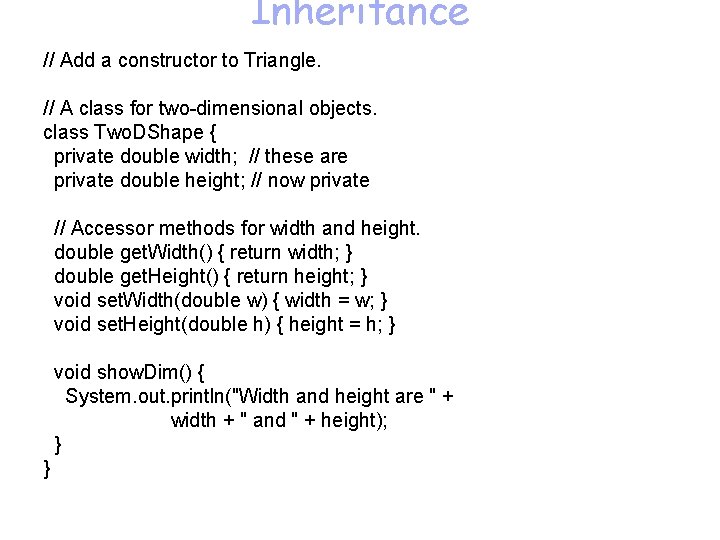
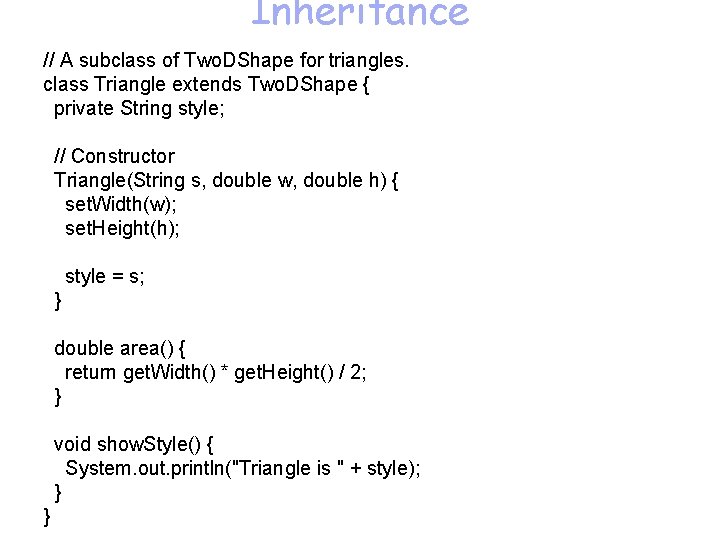
![Inheritance class Shapes 3 { public static void main(String args[]) { Triangle t 1 Inheritance class Shapes 3 { public static void main(String args[]) { Triangle t 1](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-11.jpg)
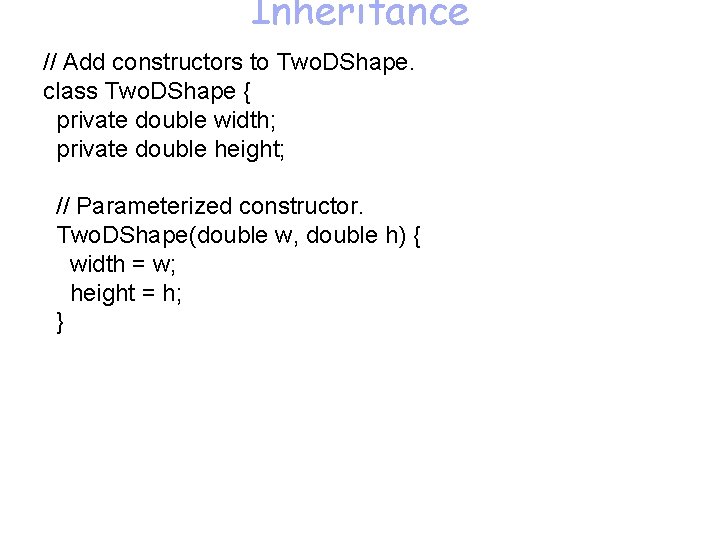
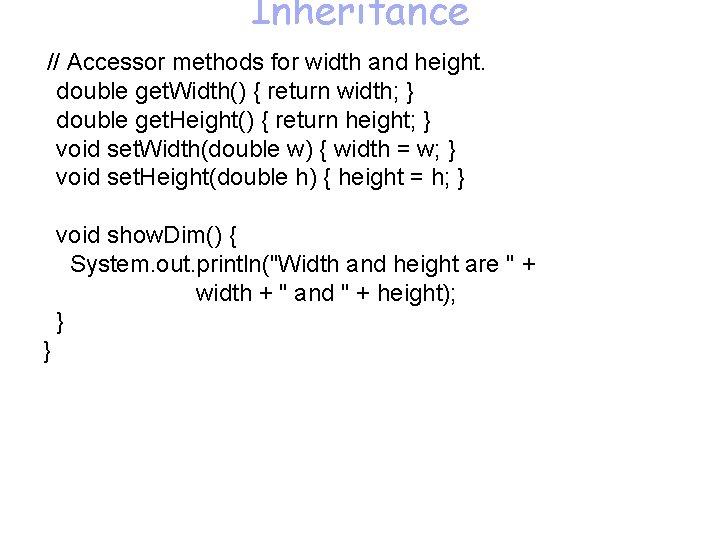
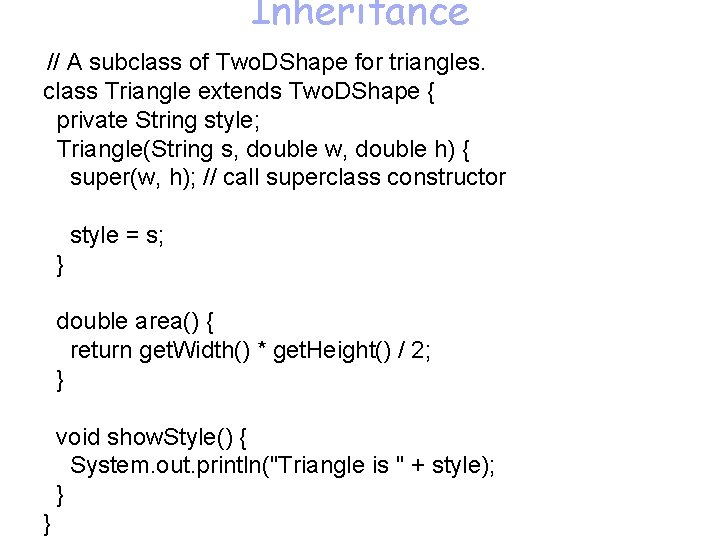
![Inheritance class Shapes 4 { public static void main(String args[]) { Triangle t 1 Inheritance class Shapes 4 { public static void main(String args[]) { Triangle t 1](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-15.jpg)
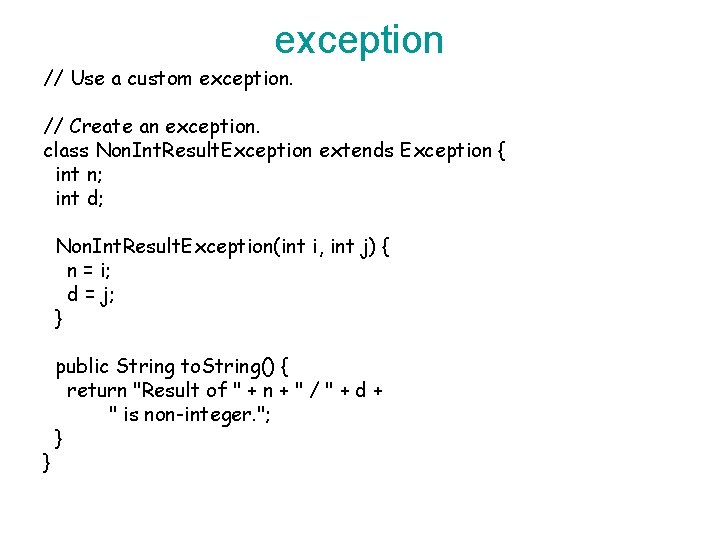
![exception class Custom. Except. Demo { public static void main(String args[]) { // Here, exception class Custom. Except. Demo { public static void main(String args[]) { // Here,](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-17.jpg)
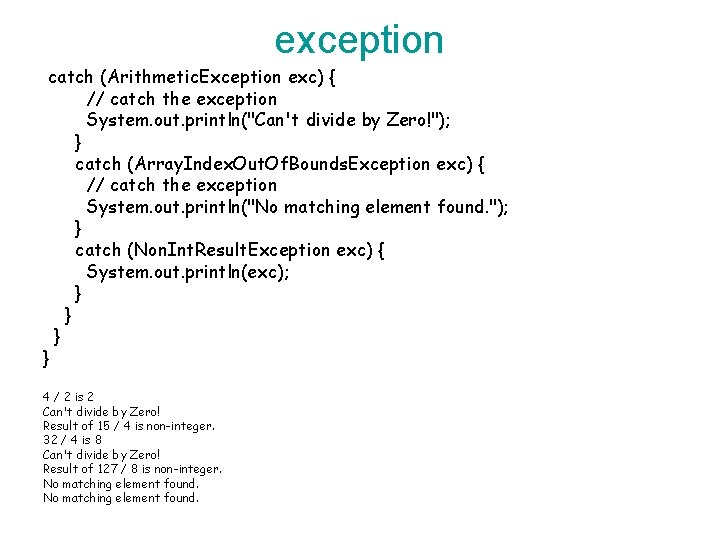
- Slides: 18
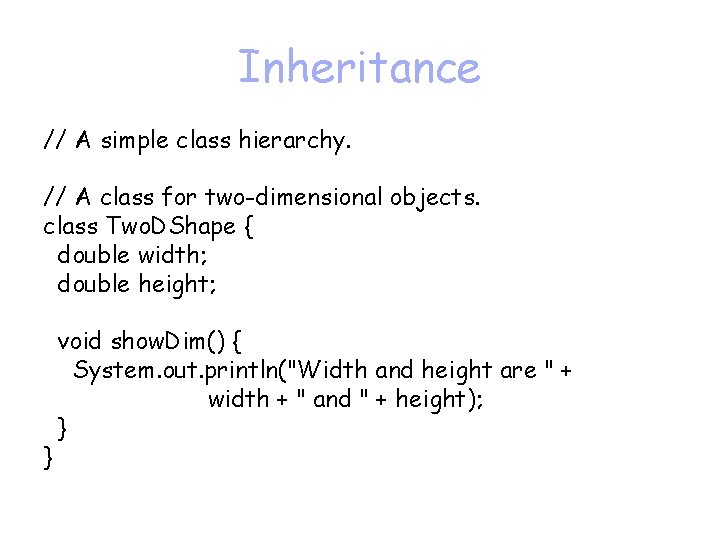
Inheritance // A simple class hierarchy. // A class for two-dimensional objects. class Two. DShape { double width; double height; } void show. Dim() { System. out. println("Width and height are " + width + " and " + height); }
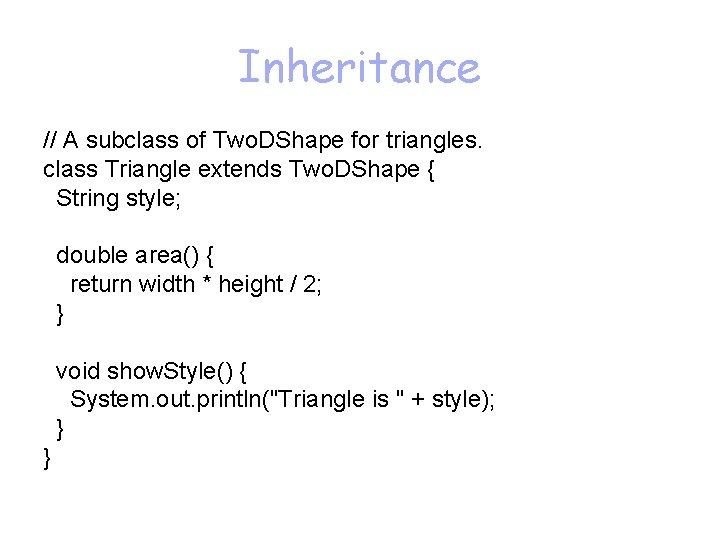
Inheritance // A subclass of Two. DShape for triangles. class Triangle extends Two. DShape { String style; double area() { return width * height / 2; } void show. Style() { System. out. println("Triangle is " + style); } }
![Inheritance class Shapes public static void mainString args Triangle t 1 Inheritance class Shapes { public static void main(String args[]) { Triangle t 1 =](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-3.jpg)
Inheritance class Shapes { public static void main(String args[]) { Triangle t 1 = new Triangle(); Triangle t 2 = new Triangle(); t 1. width = 4. 0; t 1. height = 4. 0; t 1. style = "isosceles"; t 2. width = 8. 0; t 2. height = 12. 0; t 2. style = "right"; System. out. println("Info for t 1: "); t 1. show. Style(); t 1. show. Dim(); System. out. println("Area is " + t 1. area()); System. out. println(); } } System. out. println("Info for t 2: "); t 2. show. Style(); t 2. show. Dim(); System. out. println("Area is " + t 2. area());
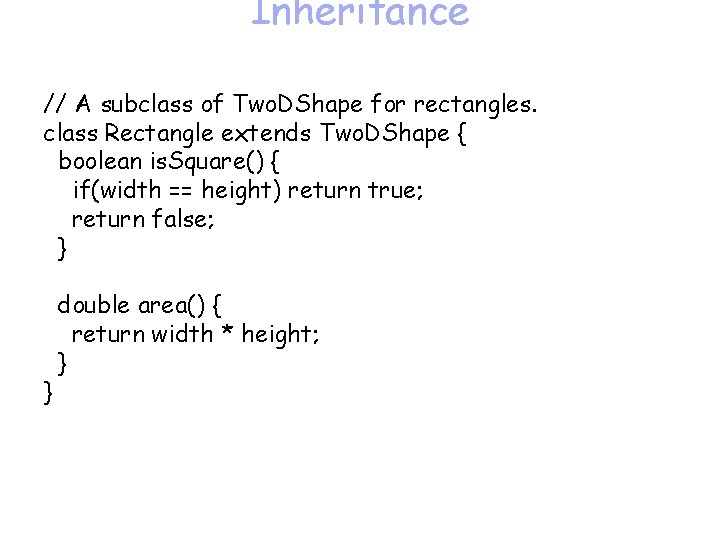
Inheritance // A subclass of Two. DShape for rectangles. class Rectangle extends Two. DShape { boolean is. Square() { if(width == height) return true; return false; } } double area() { return width * height; }
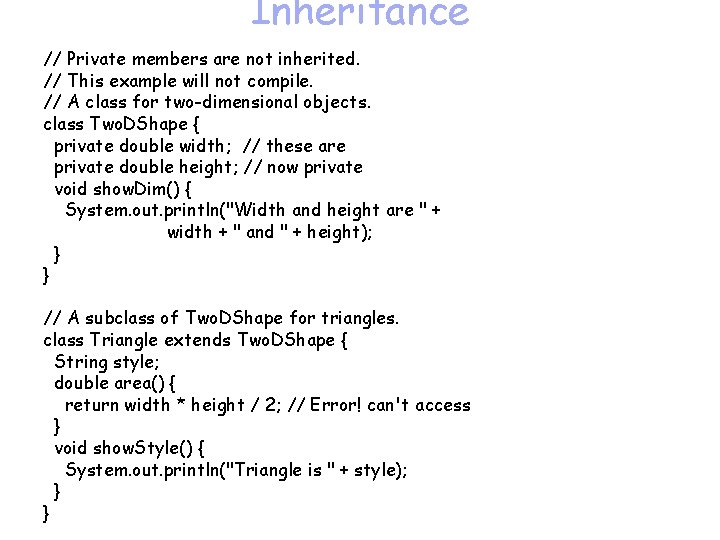
Inheritance // Private members are not inherited. // This example will not compile. // A class for two-dimensional objects. class Two. DShape { private double width; // these are private double height; // now private void show. Dim() { System. out. println("Width and height are " + width + " and " + height); } } // A subclass of Two. DShape for triangles. class Triangle extends Two. DShape { String style; double area() { return width * height / 2; // Error! can't access } void show. Style() { System. out. println("Triangle is " + style); } }
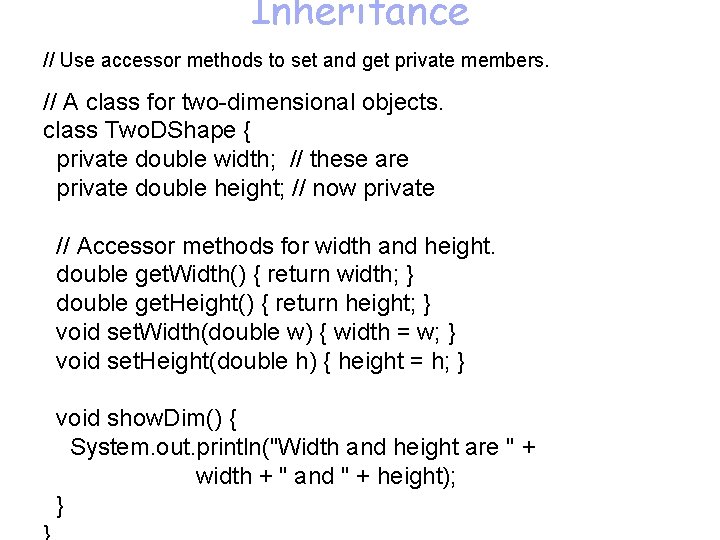
Inheritance // Use accessor methods to set and get private members. // A class for two-dimensional objects. class Two. DShape { private double width; // these are private double height; // now private // Accessor methods for width and height. double get. Width() { return width; } double get. Height() { return height; } void set. Width(double w) { width = w; } void set. Height(double h) { height = h; } void show. Dim() { System. out. println("Width and height are " + width + " and " + height); }
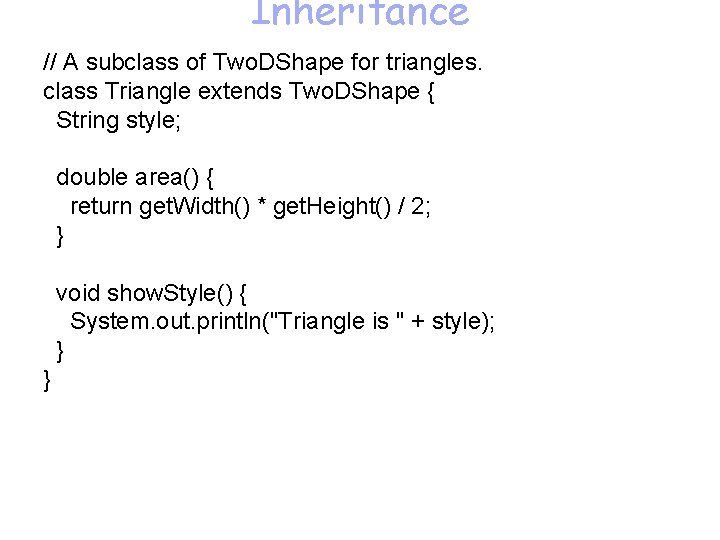
Inheritance // A subclass of Two. DShape for triangles. class Triangle extends Two. DShape { String style; double area() { return get. Width() * get. Height() / 2; } void show. Style() { System. out. println("Triangle is " + style); } }
![Inheritance class Shapes 2 public static void mainString args Triangle t 1 Inheritance class Shapes 2 { public static void main(String args[]) { Triangle t 1](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-8.jpg)
Inheritance class Shapes 2 { public static void main(String args[]) { Triangle t 1 = new Triangle(); Triangle t 2 = new Triangle(); t 1. set. Width(4. 0); t 1. set. Height(4. 0); t 1. style = "isosceles"; t 2. set. Width(8. 0); t 2. set. Height(12. 0); t 2. style = "right"; System. out. println("Info for t 1: "); t 1. show. Style(); t 1. show. Dim(); System. out. println("Area is " + t 1. area()); System. out. println("Info for t 2: "); t 2. show. Style(); t 2. show. Dim(); System. out. println("Area is " + t 2. area()); }
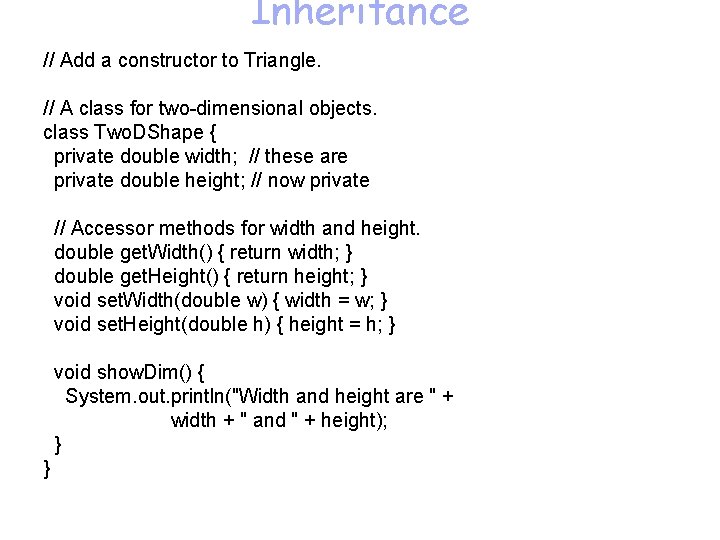
Inheritance // Add a constructor to Triangle. // A class for two-dimensional objects. class Two. DShape { private double width; // these are private double height; // now private // Accessor methods for width and height. double get. Width() { return width; } double get. Height() { return height; } void set. Width(double w) { width = w; } void set. Height(double h) { height = h; } void show. Dim() { System. out. println("Width and height are " + width + " and " + height); } }
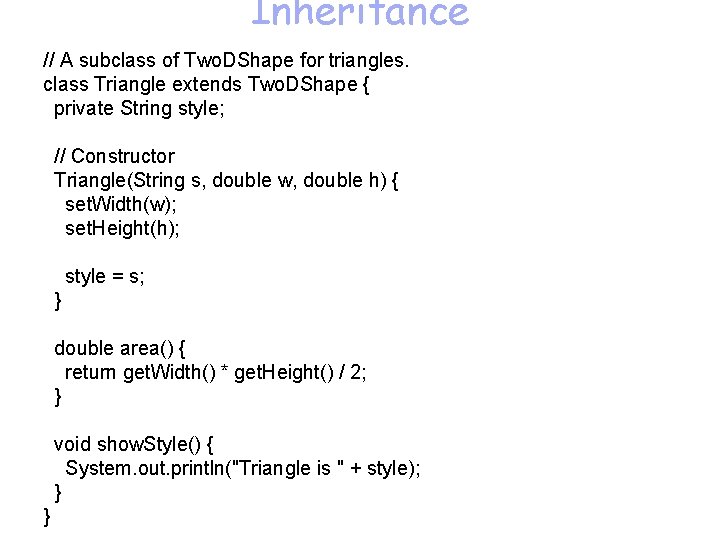
Inheritance // A subclass of Two. DShape for triangles. class Triangle extends Two. DShape { private String style; // Constructor Triangle(String s, double w, double h) { set. Width(w); set. Height(h); style = s; } double area() { return get. Width() * get. Height() / 2; } void show. Style() { System. out. println("Triangle is " + style); } }
![Inheritance class Shapes 3 public static void mainString args Triangle t 1 Inheritance class Shapes 3 { public static void main(String args[]) { Triangle t 1](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-11.jpg)
Inheritance class Shapes 3 { public static void main(String args[]) { Triangle t 1 = new Triangle("isosceles", 4. 0); Triangle t 2 = new Triangle("right", 8. 0, 12. 0); System. out. println("Info for t 1: "); t 1. show. Style(); t 1. show. Dim(); System. out. println("Area is " + t 1. area()); System. out. println("Info for t 2: "); t 2. show. Style(); t 2. show. Dim(); System. out. println("Area is " + t 2. area()); } }
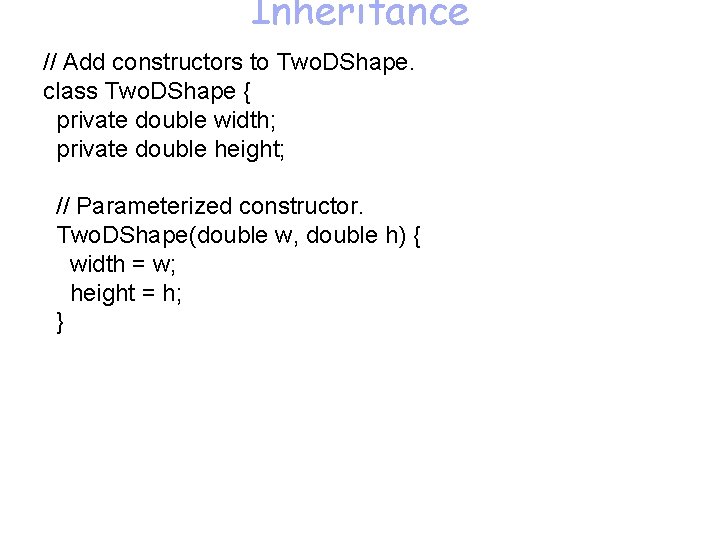
Inheritance // Add constructors to Two. DShape. class Two. DShape { private double width; private double height; // Parameterized constructor. Two. DShape(double w, double h) { width = w; height = h; }
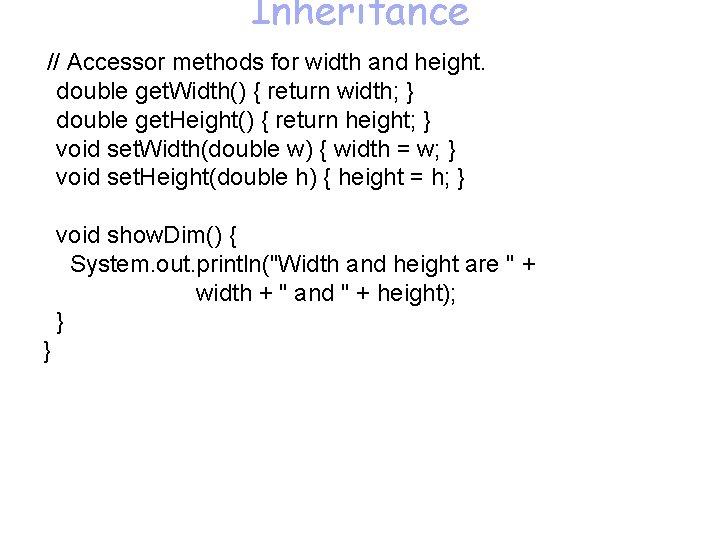
Inheritance // Accessor methods for width and height. double get. Width() { return width; } double get. Height() { return height; } void set. Width(double w) { width = w; } void set. Height(double h) { height = h; } void show. Dim() { System. out. println("Width and height are " + width + " and " + height); } }
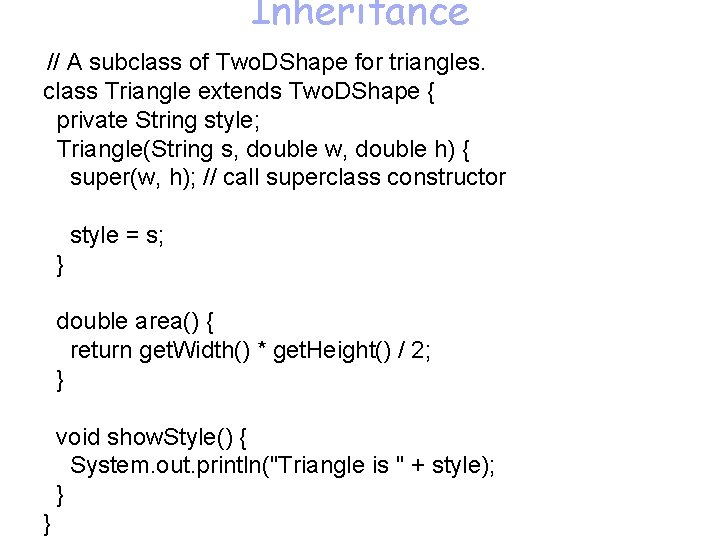
Inheritance // A subclass of Two. DShape for triangles. class Triangle extends Two. DShape { private String style; Triangle(String s, double w, double h) { super(w, h); // call superclass constructor style = s; } double area() { return get. Width() * get. Height() / 2; } void show. Style() { System. out. println("Triangle is " + style); } }
![Inheritance class Shapes 4 public static void mainString args Triangle t 1 Inheritance class Shapes 4 { public static void main(String args[]) { Triangle t 1](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-15.jpg)
Inheritance class Shapes 4 { public static void main(String args[]) { Triangle t 1 = new Triangle("isosceles", 4. 0); Triangle t 2 = new Triangle("right", 8. 0, 12. 0); System. out. println("Info for t 1: "); t 1. show. Style(); t 1. show. Dim(); System. out. println("Area is " + t 1. area()); System. out. println("Info for t 2: "); t 2. show. Style(); t 2. show. Dim(); System. out. println("Area is " + t 2. area()); } }
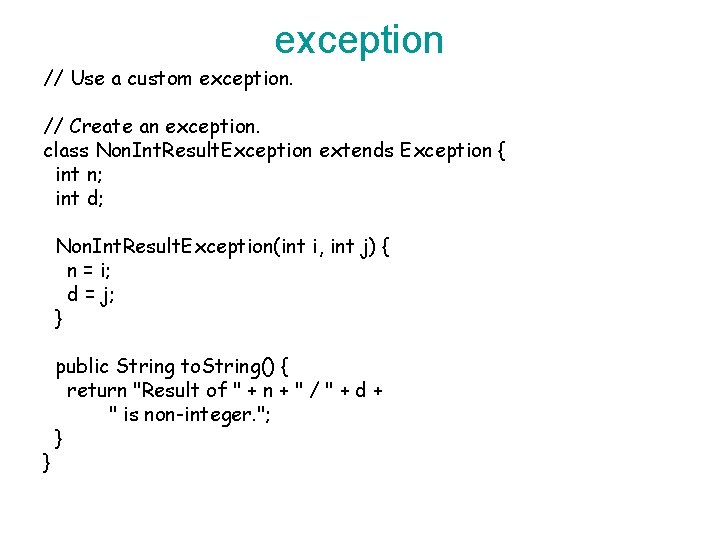
exception // Use a custom exception. // Create an exception. class Non. Int. Result. Exception extends Exception { int n; int d; Non. Int. Result. Exception(int i, int j) { n = i; d = j; } } public String to. String() { return "Result of " + n + " / " + d + " is non-integer. "; }
![exception class Custom Except Demo public static void mainString args Here exception class Custom. Except. Demo { public static void main(String args[]) { // Here,](https://slidetodoc.com/presentation_image_h2/ecbc124bf1360e0d3398e3f99ab70b7b/image-17.jpg)
exception class Custom. Except. Demo { public static void main(String args[]) { // Here, numer contains some odd values. int numer[] = { 4, 8, 15, 32, 64, 127, 256, 512 }; int denom[] = { 2, 0, 4, 4, 0, 8 }; for(int i=0; i<numer. length; i++) { try { if((numer[i]%2) != 0) throw new Non. Int. Result. Exception(numer[i], denom[i]); } System. out. println(numer[i] + " / " + denom[i] + " is " + numer[i]/denom[i]);
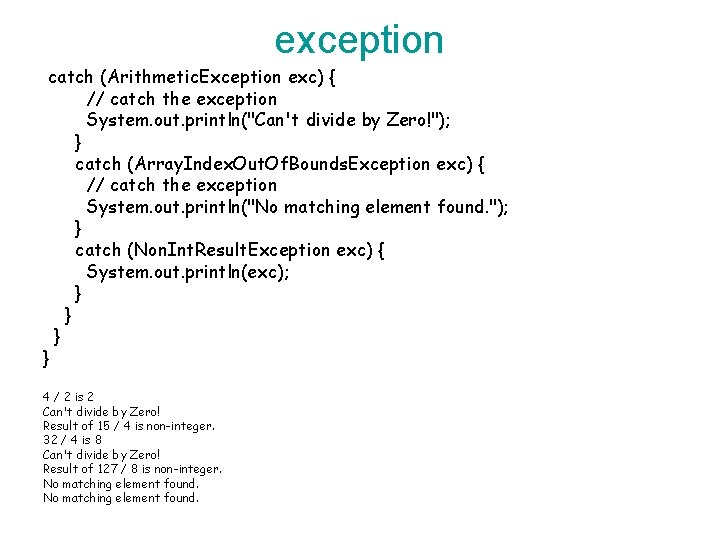
exception catch (Arithmetic. Exception exc) { // catch the exception System. out. println("Can't divide by Zero!"); } catch (Array. Index. Out. Of. Bounds. Exception exc) { // catch the exception System. out. println("No matching element found. "); } catch (Non. Int. Result. Exception exc) { System. out. println(exc); } } 4 / 2 is 2 Can't divide by Zero! Result of 15 / 4 is non-integer. 32 / 4 is 8 Can't divide by Zero! Result of 127 / 8 is non-integer. No matching element found.
Simple inheritance
Fspos
Typiska novell drag
Nationell inriktning för artificiell intelligens
Returpilarna
Shingelfrisyren
En lathund för arbete med kontinuitetshantering
Personalliggare bygg undantag
Personlig tidbok för yrkesförare
A gastrica
Förklara densitet för barn
Datorkunskap för nybörjare
Boverket ka
Hur skriver man en tes
Autokratiskt ledarskap
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Arkimedes princip formel
Offentlig förvaltning