Generic Programming and Inner classes generic 1 a
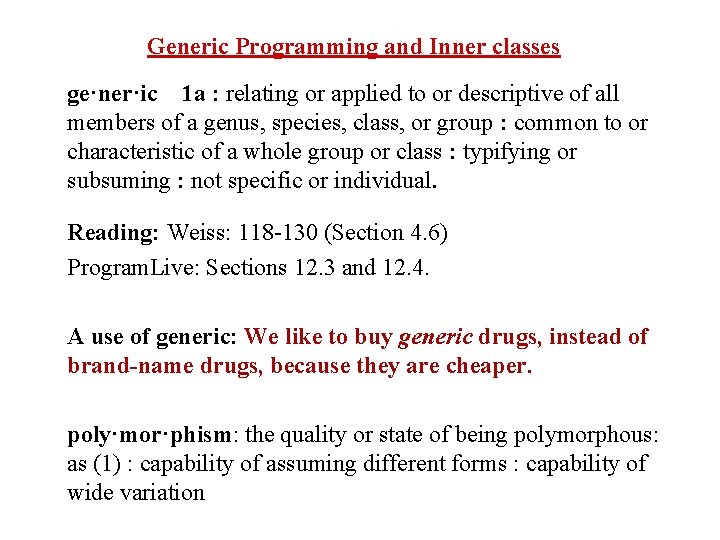
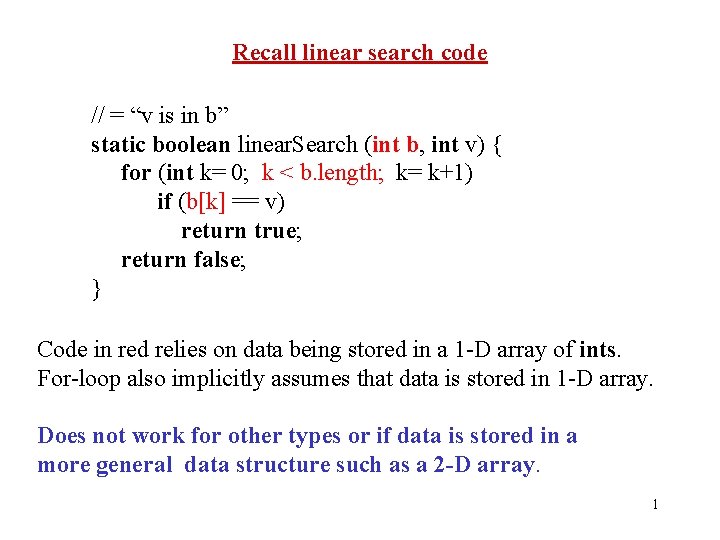
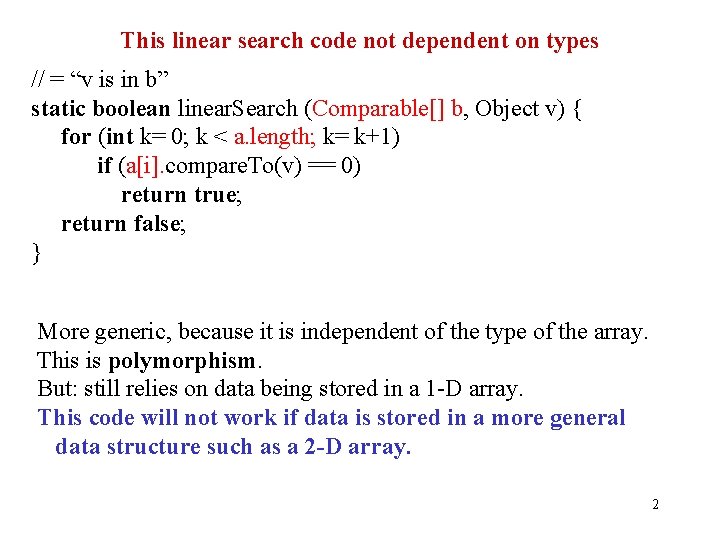
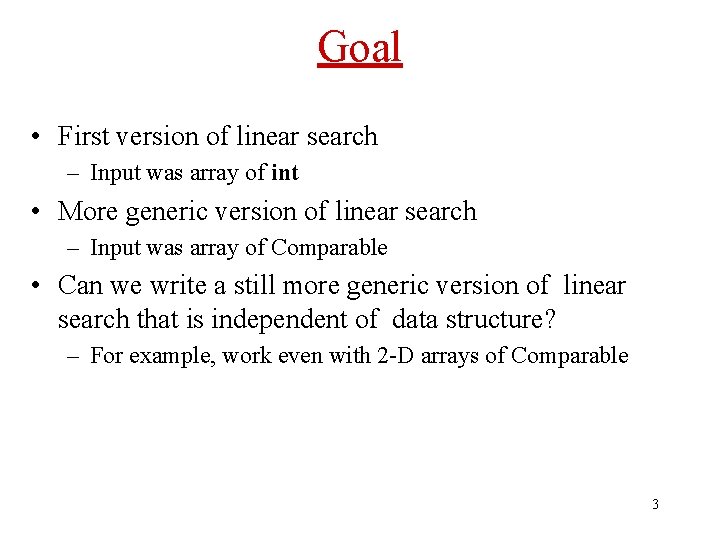
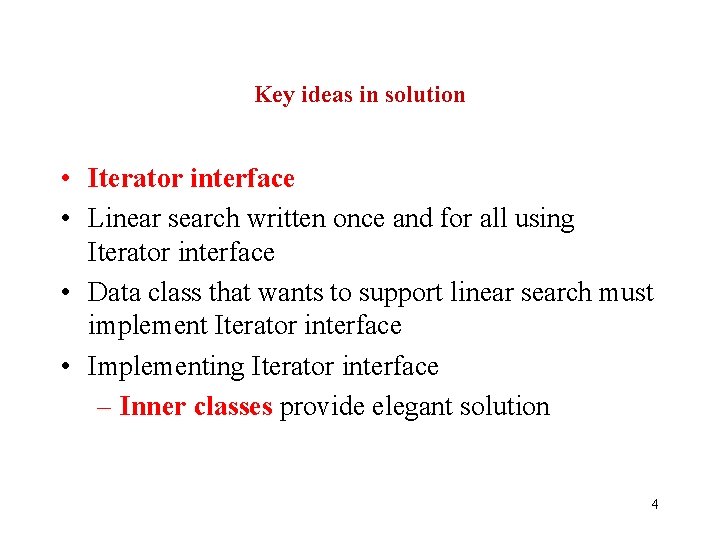
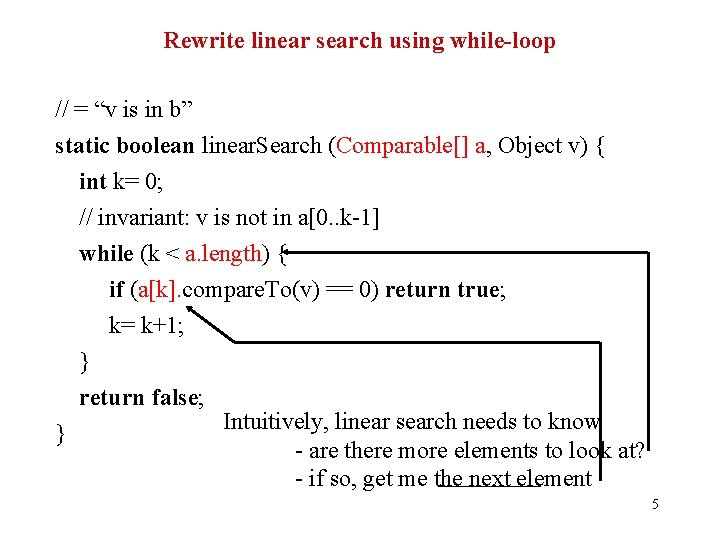
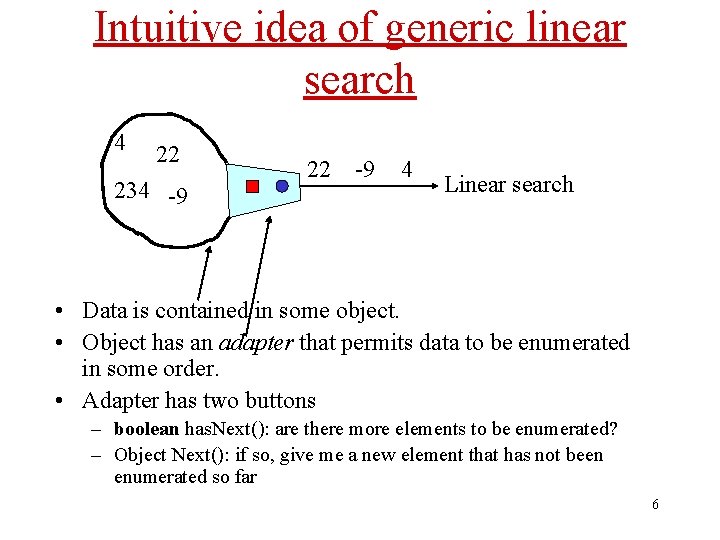
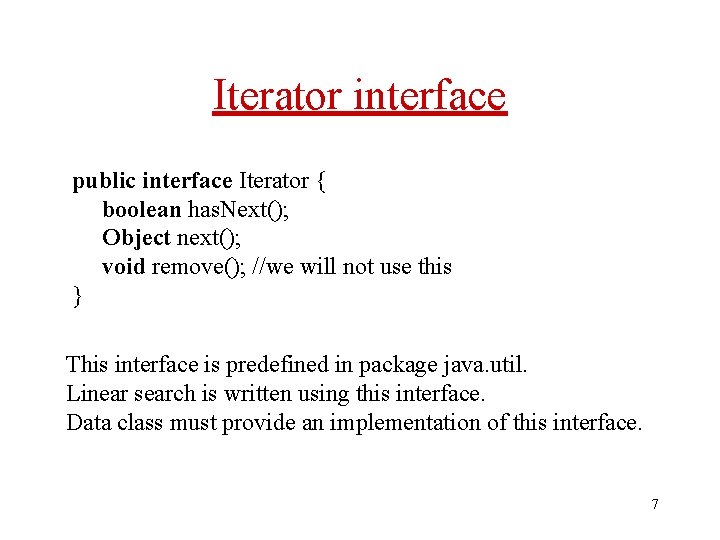
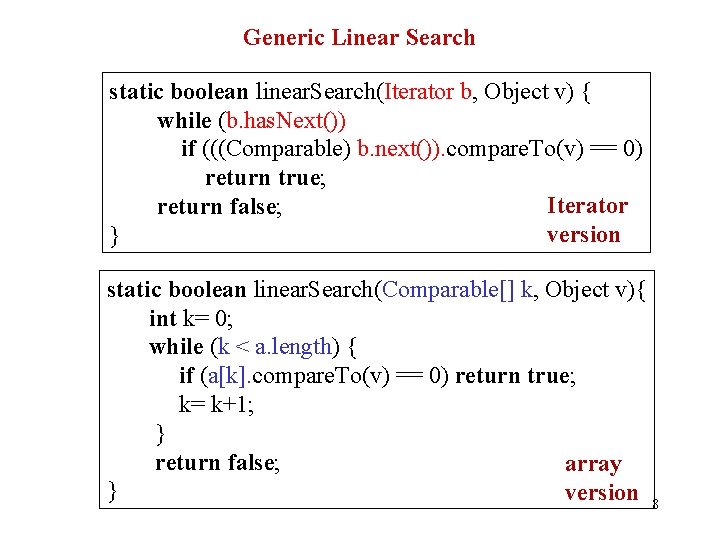
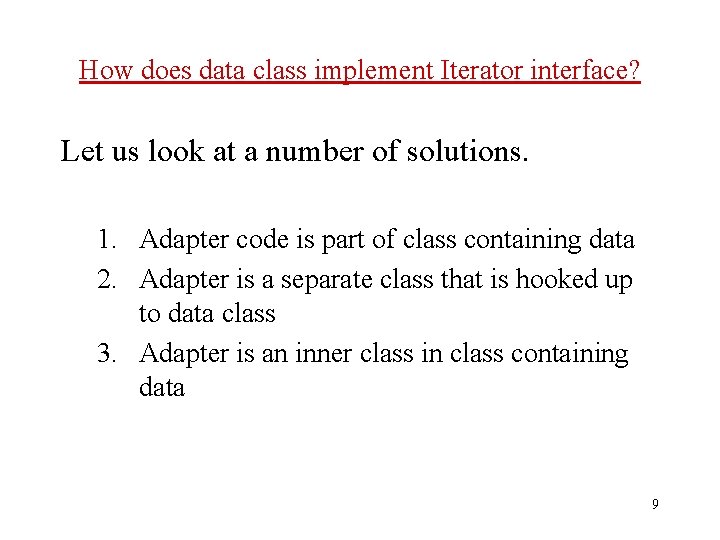
![Adapter (version 1) public class Crock 1 implements Iterator{ private Some. Class[] a; //Class Adapter (version 1) public class Crock 1 implements Iterator{ private Some. Class[] a; //Class](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-11.jpg)
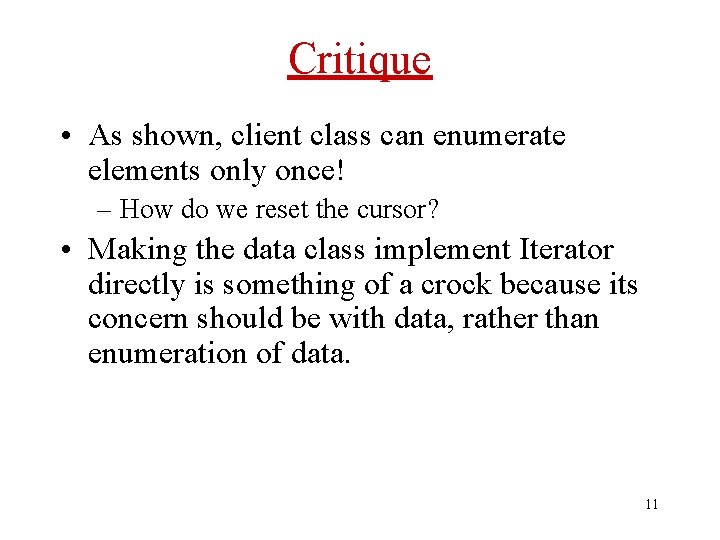
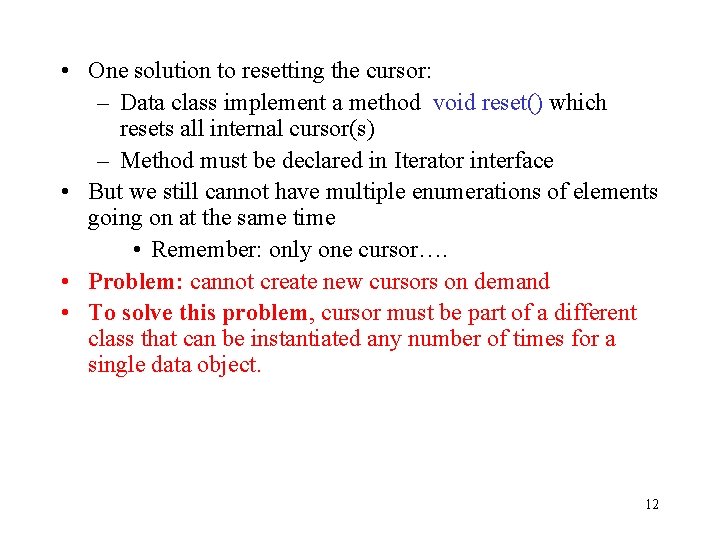
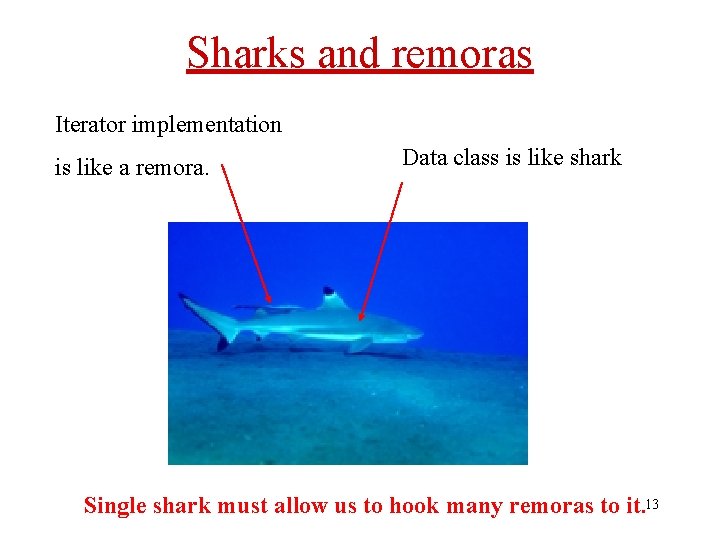
![Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-15.jpg)
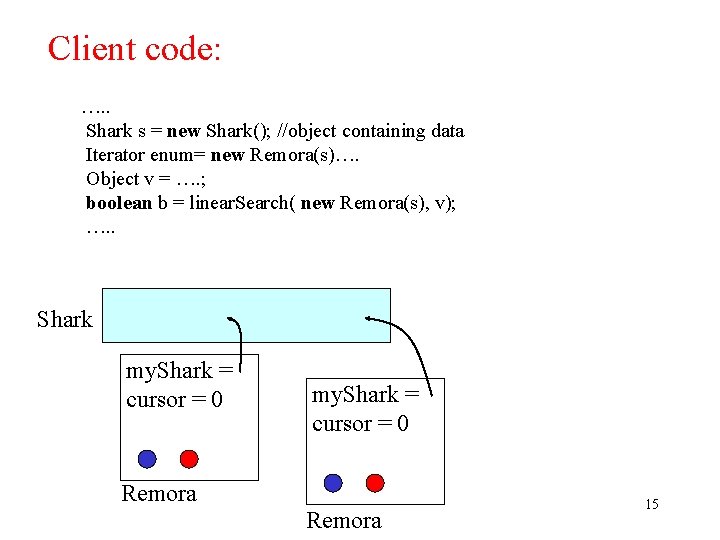
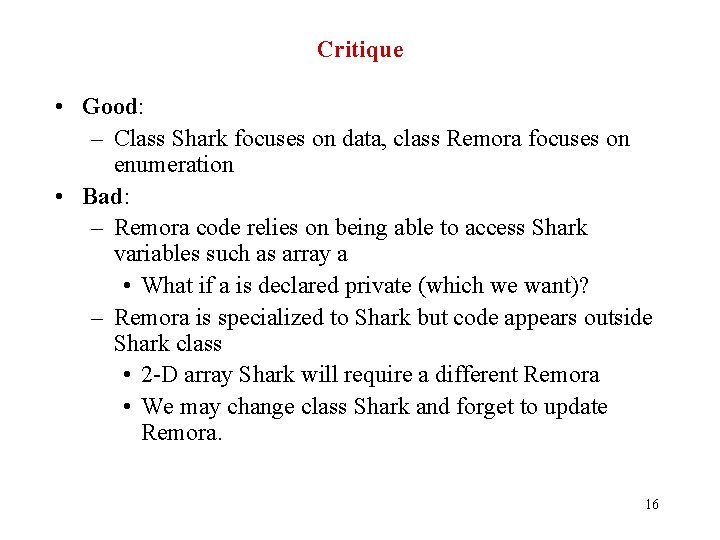
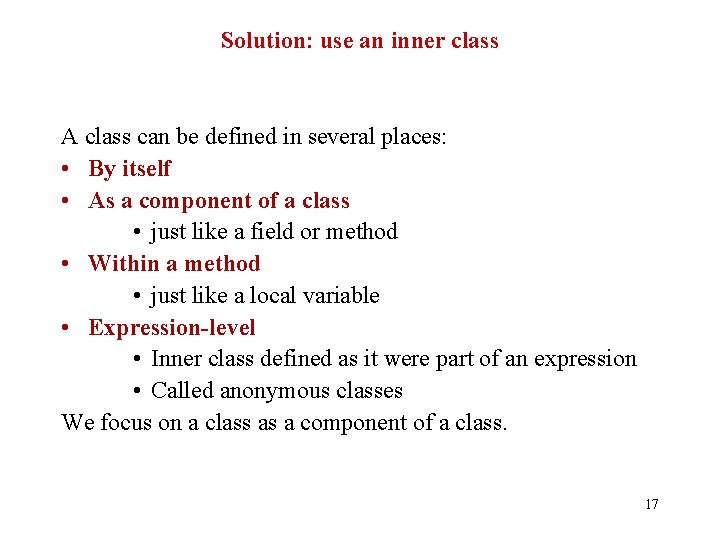
![Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-19.jpg)
![Adapter (version 3) class Shark{ private Comparable[] a; // Constructor: … public Shark() {…get Adapter (version 3) class Shark{ private Comparable[] a; // Constructor: … public Shark() {…get](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-20.jpg)
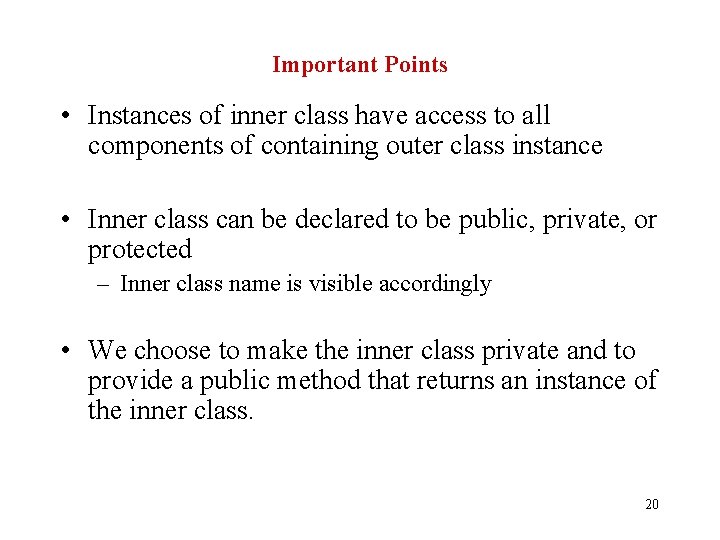
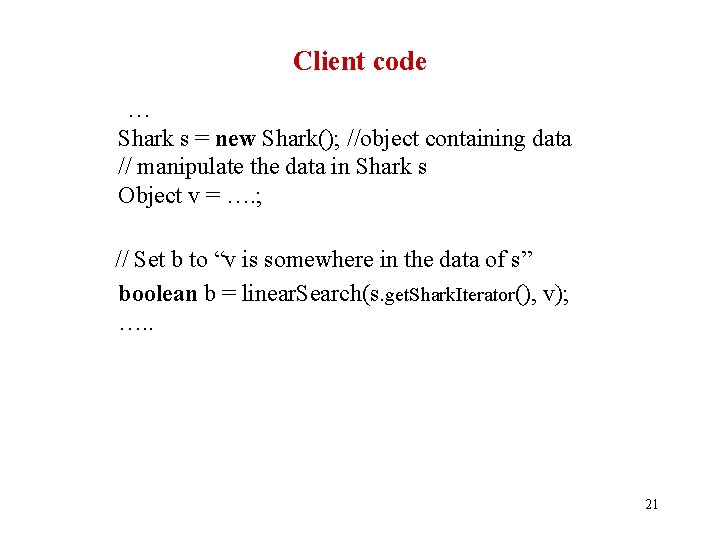
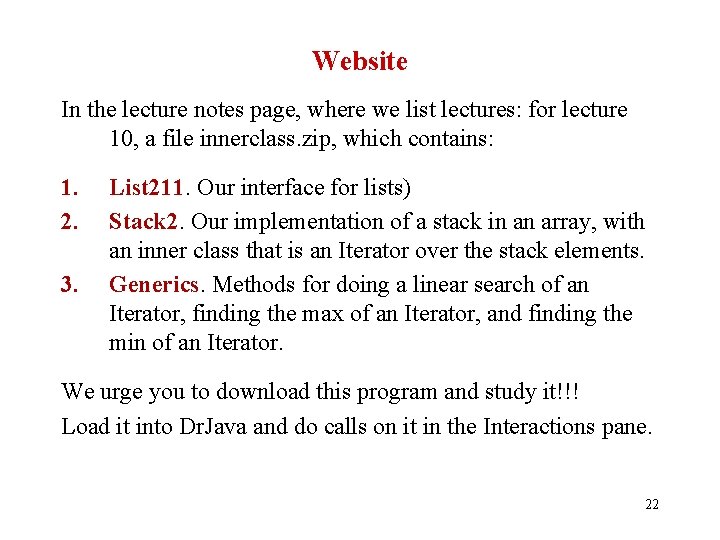
- Slides: 23
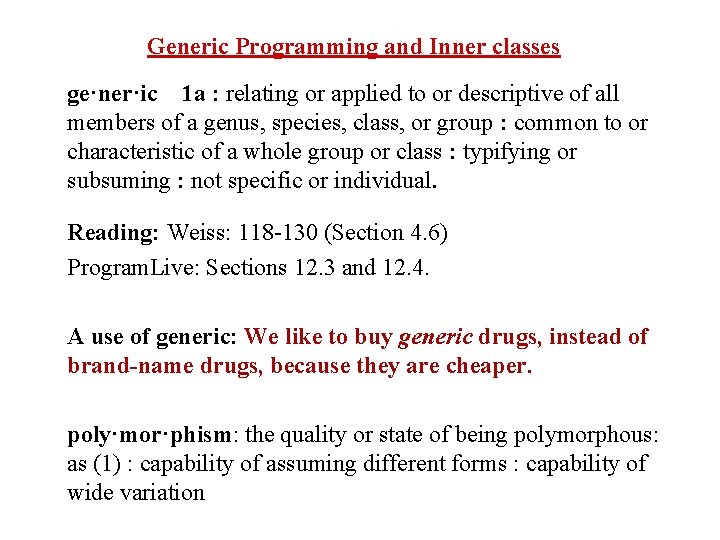
Generic Programming and Inner classes ge·ner·ic 1 a : relating or applied to or descriptive of all members of a genus, species, class, or group : common to or characteristic of a whole group or class : typifying or subsuming : not specific or individual. Reading: Weiss: 118 -130 (Section 4. 6) Program. Live: Sections 12. 3 and 12. 4. A use of generic: We like to buy generic drugs, instead of brand-name drugs, because they are cheaper. poly·mor·phism: the quality or state of being polymorphous: as (1) : capability of assuming different forms : capability of wide variation
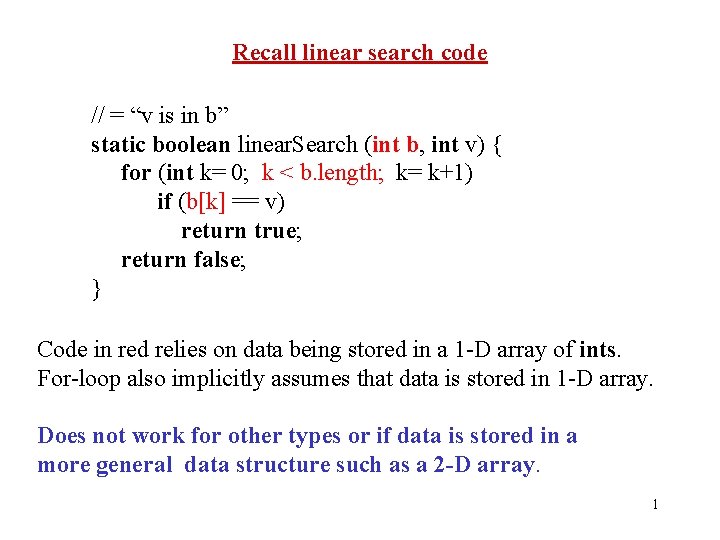
Recall linear search code // = “v is in b” static boolean linear. Search (int b, int v) { for (int k= 0; k < b. length; k= k+1) if (b[k] == v) return true; return false; } Code in red relies on data being stored in a 1 -D array of ints. For-loop also implicitly assumes that data is stored in 1 -D array. Does not work for other types or if data is stored in a more general data structure such as a 2 -D array. 1
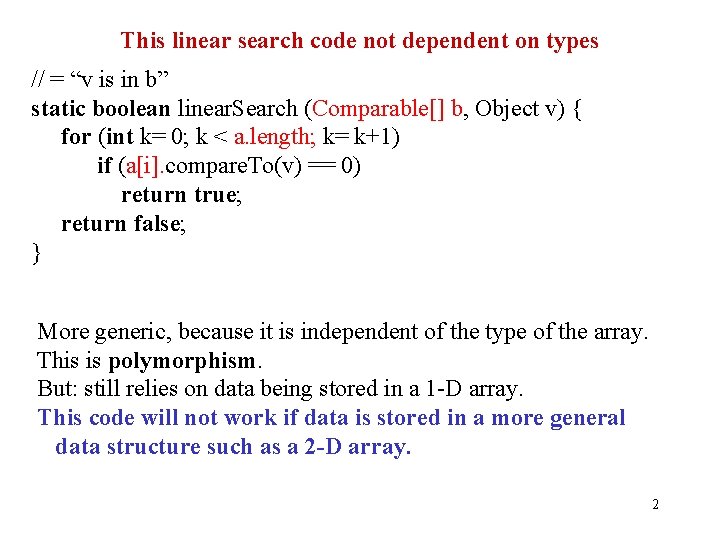
This linear search code not dependent on types // = “v is in b” static boolean linear. Search (Comparable[] b, Object v) { for (int k= 0; k < a. length; k= k+1) if (a[i]. compare. To(v) == 0) return true; return false; } More generic, because it is independent of the type of the array. This is polymorphism. But: still relies on data being stored in a 1 -D array. This code will not work if data is stored in a more general data structure such as a 2 -D array. 2
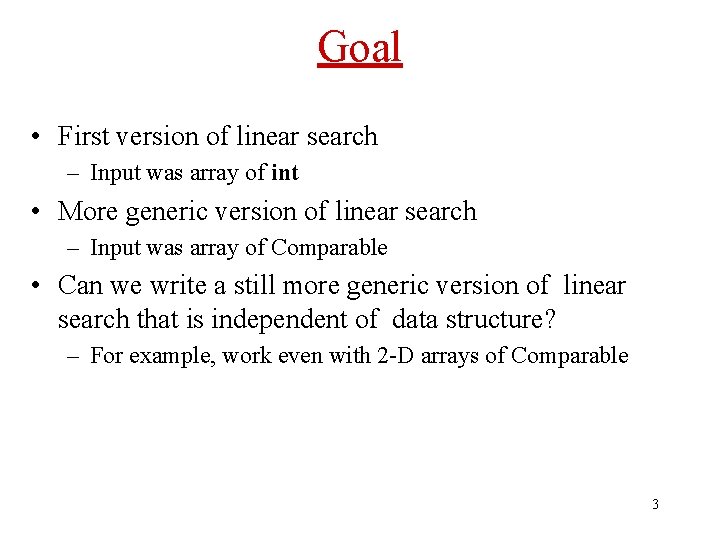
Goal • First version of linear search – Input was array of int • More generic version of linear search – Input was array of Comparable • Can we write a still more generic version of linear search that is independent of data structure? – For example, work even with 2 -D arrays of Comparable 3
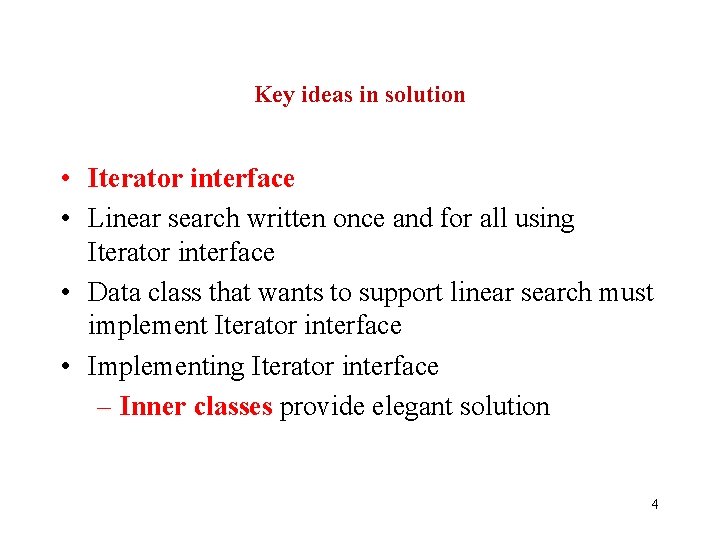
Key ideas in solution • Iterator interface • Linear search written once and for all using Iterator interface • Data class that wants to support linear search must implement Iterator interface • Implementing Iterator interface – Inner classes provide elegant solution 4
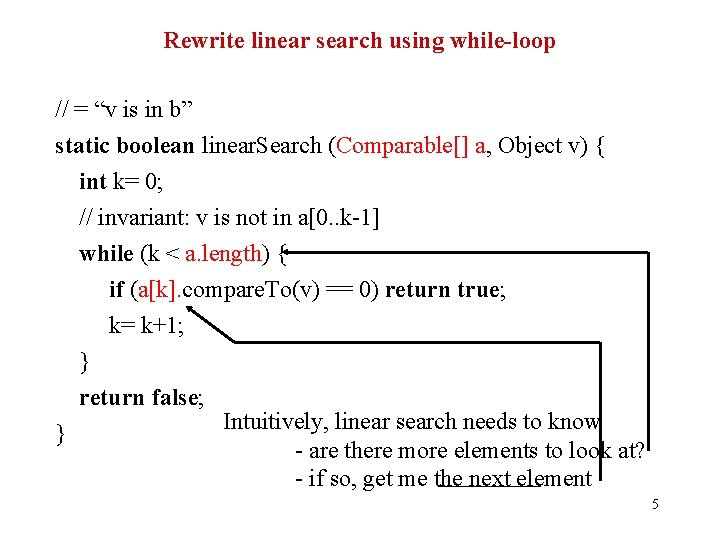
Rewrite linear search using while-loop // = “v is in b” static boolean linear. Search (Comparable[] a, Object v) { int k= 0; // invariant: v is not in a[0. . k-1] while (k < a. length) { if (a[k]. compare. To(v) == 0) return true; k= k+1; } return false; } Intuitively, linear search needs to know - are there more elements to look at? - if so, get me the next element 5
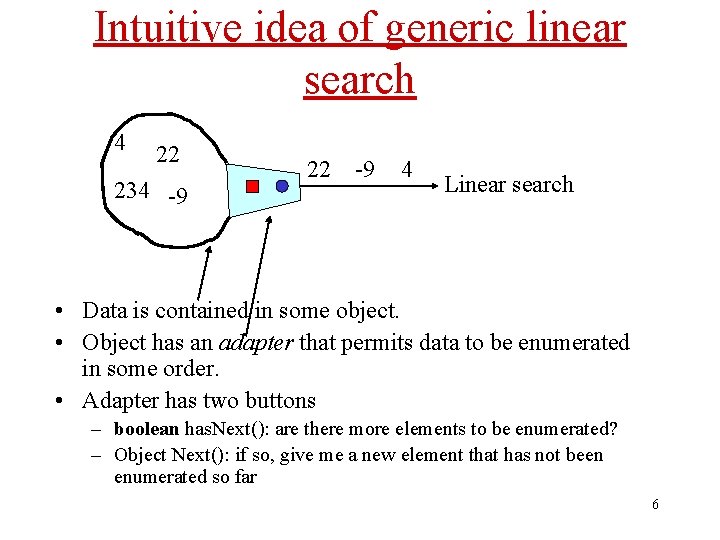
Intuitive idea of generic linear search 4 22 234 -9 22 -9 4 Linear search • Data is contained in some object. • Object has an adapter that permits data to be enumerated in some order. • Adapter has two buttons – boolean has. Next(): are there more elements to be enumerated? – Object Next(): if so, give me a new element that has not been enumerated so far 6
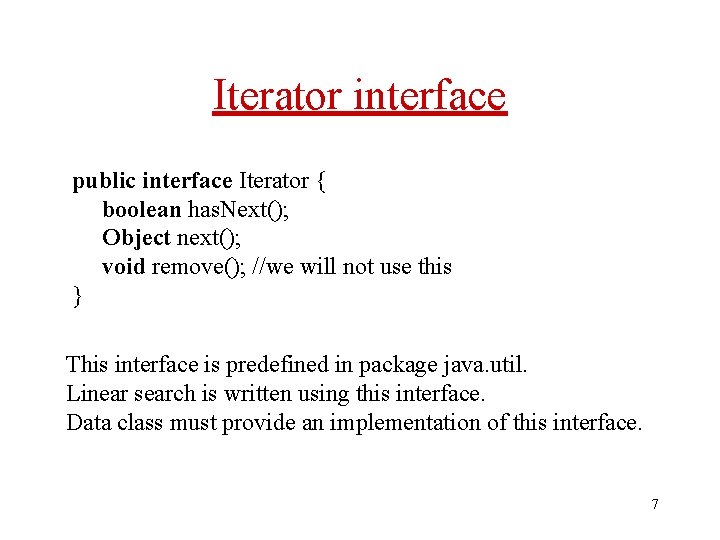
Iterator interface public interface Iterator { boolean has. Next(); Object next(); void remove(); //we will not use this } This interface is predefined in package java. util. Linear search is written using this interface. Data class must provide an implementation of this interface. 7
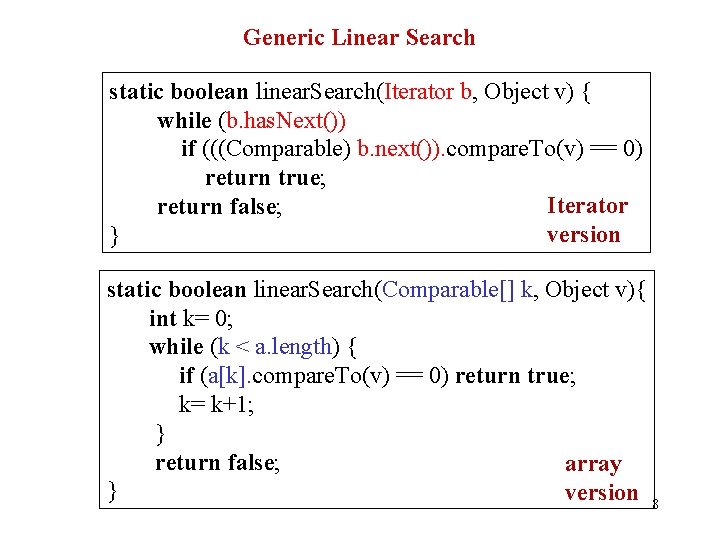
Generic Linear Search static boolean linear. Search(Iterator b, Object v) { while (b. has. Next()) if (((Comparable) b. next()). compare. To(v) == 0) return true; Iterator return false; version } static boolean linear. Search(Comparable[] k, Object v){ int k= 0; while (k < a. length) { if (a[k]. compare. To(v) == 0) return true; k= k+1; } return false; array } version 8
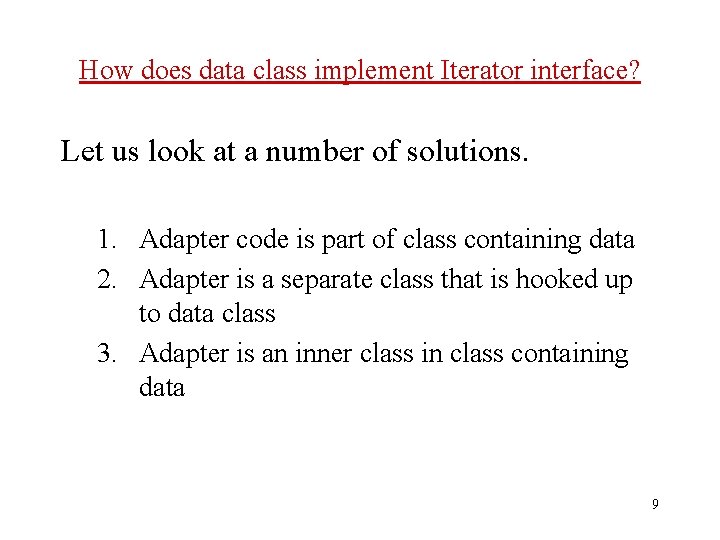
How does data class implement Iterator interface? Let us look at a number of solutions. 1. Adapter code is part of class containing data 2. Adapter is a separate class that is hooked up to data class 3. Adapter is an inner class in class containing data 9
![Adapter version 1 public class Crock 1 implements Iterator private Some Class a Class Adapter (version 1) public class Crock 1 implements Iterator{ private Some. Class[] a; //Class](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-11.jpg)
Adapter (version 1) public class Crock 1 implements Iterator{ private Some. Class[] a; //Class Some. Class must implement // Comparable private int cursor= 0; //index of next element to be enumerated /** Constructor: an instance with … public Crock 1() { …store data in array a… } public boolean has. Next() { return (cursor < a. length); } public Object next() { cursor= cursor + 1; return a[cursor-1]; } public void remove() {}//unimplementated } 10
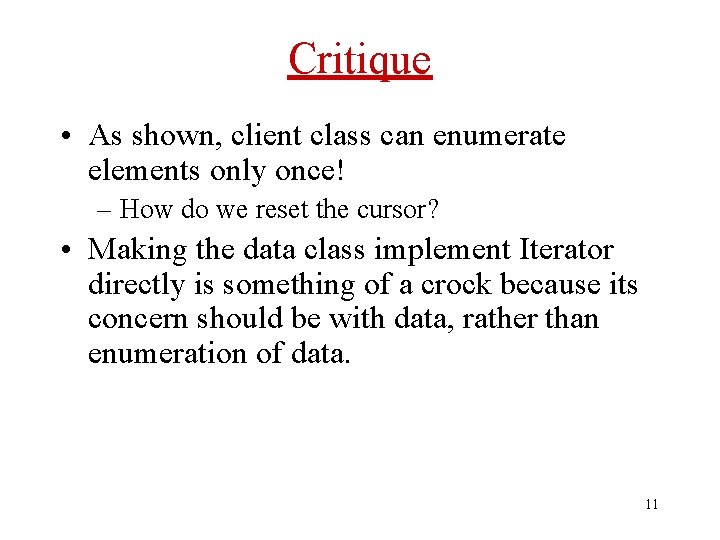
Critique • As shown, client class can enumerate elements only once! – How do we reset the cursor? • Making the data class implement Iterator directly is something of a crock because its concern should be with data, rather than enumeration of data. 11
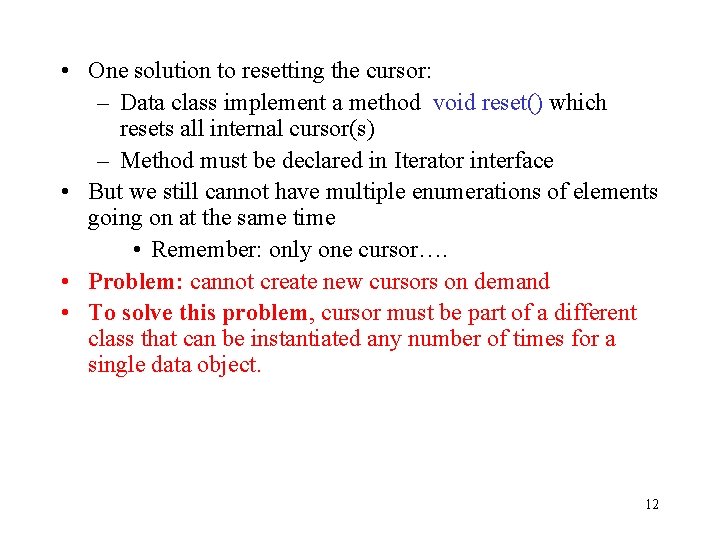
• One solution to resetting the cursor: – Data class implement a method void reset() which resets all internal cursor(s) – Method must be declared in Iterator interface • But we still cannot have multiple enumerations of elements going on at the same time • Remember: only one cursor…. • Problem: cannot create new cursors on demand • To solve this problem, cursor must be part of a different class that can be instantiated any number of times for a single data object. 12
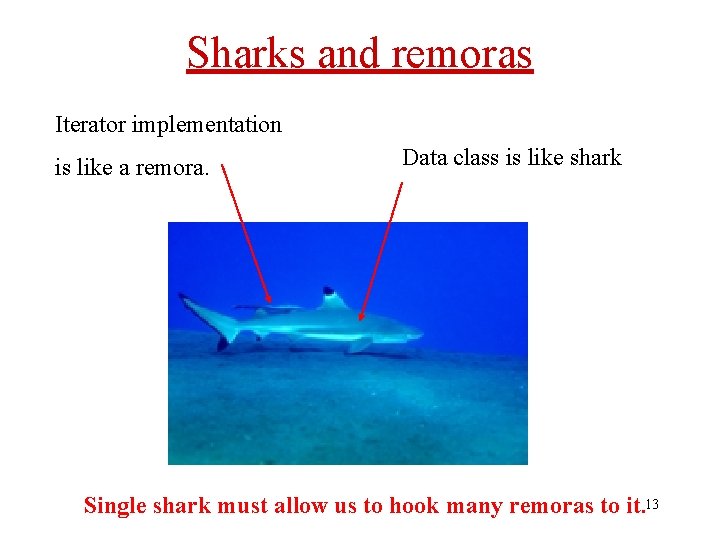
Sharks and remoras Iterator implementation is like a remora. Data class is like shark Single shark must allow us to hook many remoras to it. 13
![Adapter version 2 class Shark public Comparable a Constructor public Shark get Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-15.jpg)
Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get data into a…} public class Remora implements Iterator{ int cursor; Shark my. Shark; public Remora(Shark s) { { my. Shark= s; cursor= 0; } public boolean has. Next() { return (cursor < my. Shark. a. length); } public Object next() { cursor= cursor+1; return my. Shark. a[cursor-1]; } public void remove() {} //unimplemented … } } The client, using Shark: Shark s= new Shark(…); … Iterator enum= new Remora(s); while (enum. has. Next()) {… } Remora teeth 14
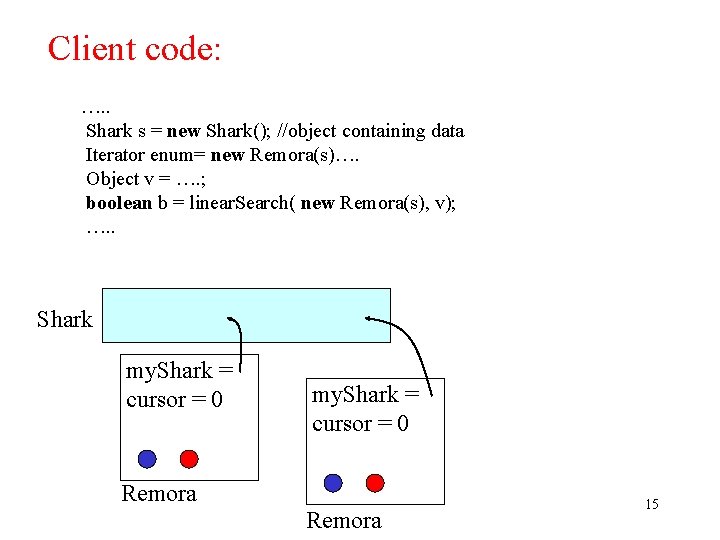
Client code: …. . Shark s = new Shark(); //object containing data Iterator enum= new Remora(s)…. Object v = …. ; boolean b = linear. Search( new Remora(s), v); …. . Shark my. Shark = cursor = 0 Remora 15
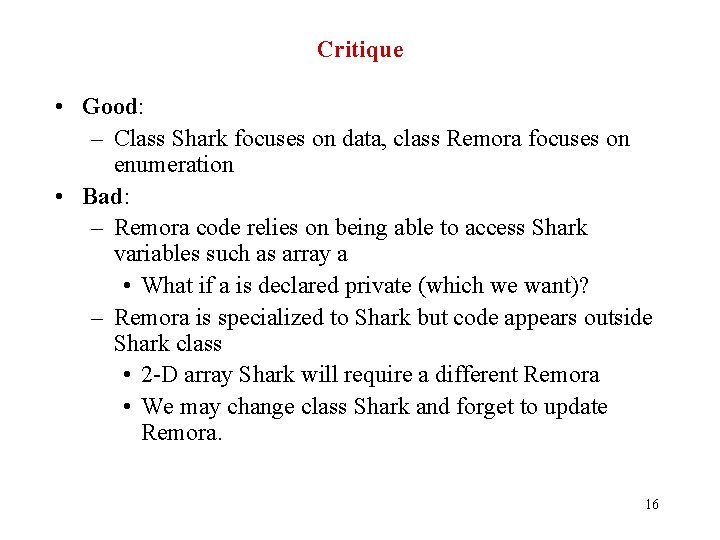
Critique • Good: – Class Shark focuses on data, class Remora focuses on enumeration • Bad: – Remora code relies on being able to access Shark variables such as array a • What if a is declared private (which we want)? – Remora is specialized to Shark but code appears outside Shark class • 2 -D array Shark will require a different Remora • We may change class Shark and forget to update Remora. 16
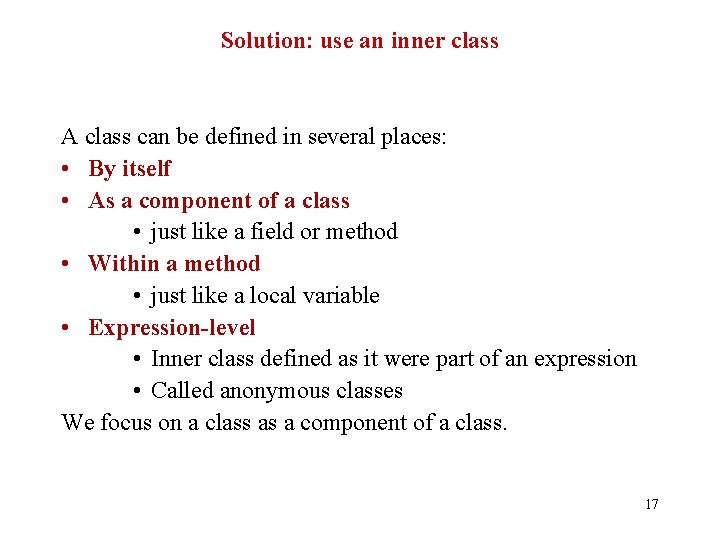
Solution: use an inner class A class can be defined in several places: • By itself • As a component of a class • just like a field or method • Within a method • just like a local variable • Expression-level • Inner class defined as it were part of an expression • Called anonymous classes We focus on a class as a component of a class. 17
![Adapter version 2 class Shark public Comparable a Constructor public Shark get Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-19.jpg)
Adapter (version 2) class Shark{ public Comparable[] a; // Constructor: … public Shark() {…get data into a…} … } public class Remora implements Iterator{ int cursor; Shark my. Shark; public Remora(Shark s) { my. Shark= s; cursor= 0; } public boolean has. Next() { return (cursor < my. Shark. a. length); } public Object next() { cursor= cursor+1; return my. Shark. a[cursor-1]; } public void remove() {} //unimplemented } Shark s= new Shark(…); … Iterator enum= new Remora(s); while (enum. has. Next()) { … } Remora teeth 18
![Adapter version 3 class Shark private Comparable a Constructor public Shark get Adapter (version 3) class Shark{ private Comparable[] a; // Constructor: … public Shark() {…get](https://slidetodoc.com/presentation_image_h2/40be8e2c68fb7b6ee22308e695e8c06a/image-20.jpg)
Adapter (version 3) class Shark{ private Comparable[] a; // Constructor: … public Shark() {…get data into a…} … private class Remora implements Iterator{ int cursor; Shark my. Shark; public Remora(Shark s) { my. Shark= s; cursor= 0; } public boolean has. Next() { return (cursor < my. Shark. a. length); } public Object next() { cursor= cursor+1; return my. Shark. a[cursor-1]; } public void remove() {} //unimpl. } // end Remora // = an iterator over the data of this Shark public Iterator get. Shark. Iterator() { return new Remora(); } } // end Shark 19
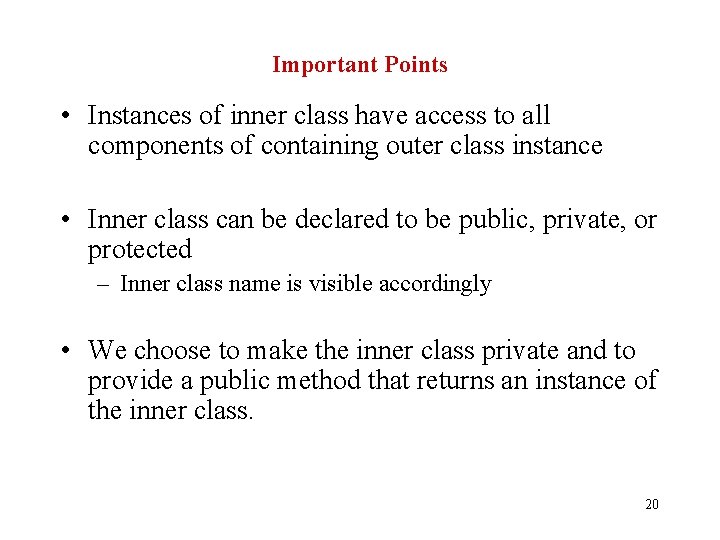
Important Points • Instances of inner class have access to all components of containing outer class instance • Inner class can be declared to be public, private, or protected – Inner class name is visible accordingly • We choose to make the inner class private and to provide a public method that returns an instance of the inner class. 20
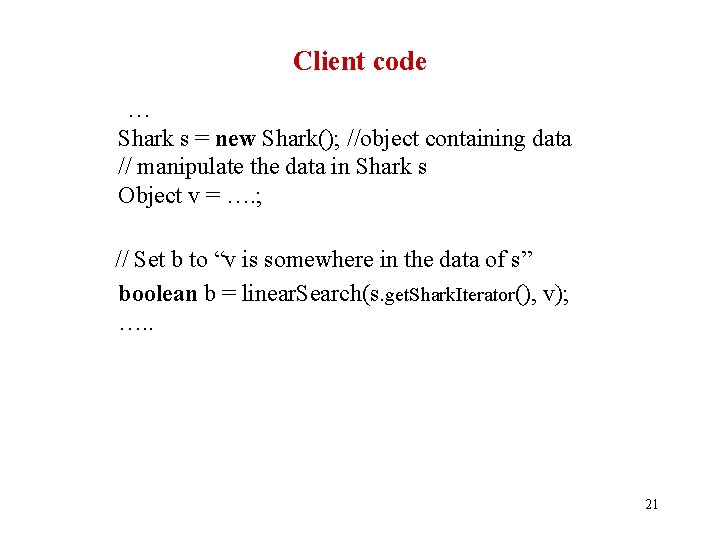
Client code … Shark s = new Shark(); //object containing data // manipulate the data in Shark s Object v = …. ; // Set b to “v is somewhere in the data of s” boolean b = linear. Search(s. get. Shark. Iterator(), v); …. . 21
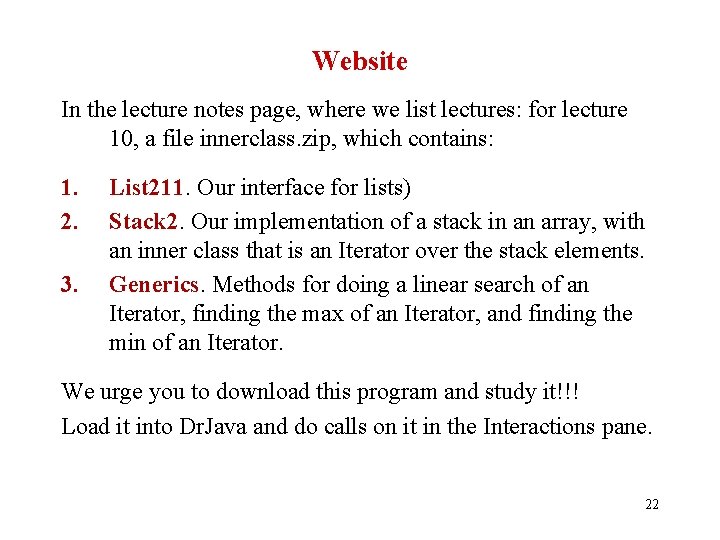
Website In the lecture notes page, where we list lectures: for lecture 10, a file innerclass. zip, which contains: 1. 2. 3. List 211. Our interface for lists) Stack 2. Our implementation of a stack in an array, with an inner class that is an Iterator over the stack elements. Generics. Methods for doing a linear search of an Iterator, finding the max of an Iterator, and finding the min of an Iterator. We urge you to download this program and study it!!! Load it into Dr. Java and do calls on it in the Interactions pane. 22
Strategies for creating success in college and in life
Inner defender examples
Primeiro classe e subclasse
Pre ap classes vs regular classes
Generic programming and the stl
Generic subroutine
Generic programming
Templates in c++
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
Runtime programming
Integer programming vs linear programming
Perbedaan linear programming dan integer programming
What are the inner planets
Inner core and outer core
Earth mantle definition
Inner and outer controls work against deviance
Which type of wave can penetrate the outer and inner core *
Inner and outer sphere mechanism
Inner and outer forces
To study inner sensations images and feelings
What separates the inner and outer planets
My very excited mother just
What separates inner and outer planets