Generic Java Classes Implementing your own generic classes
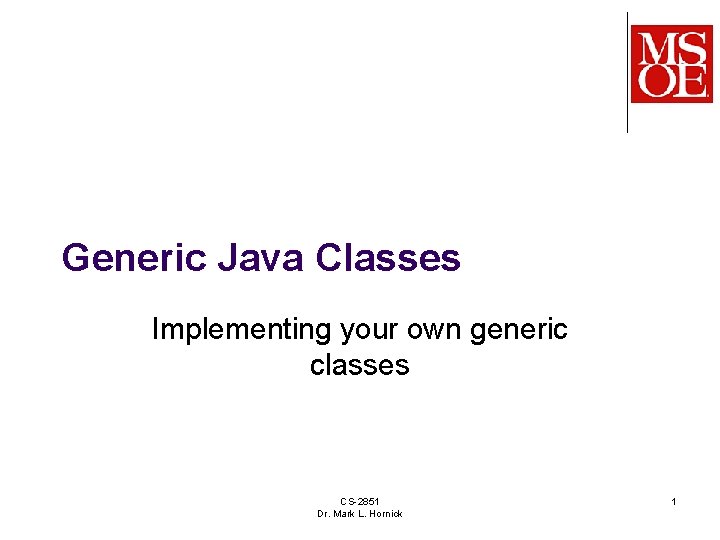
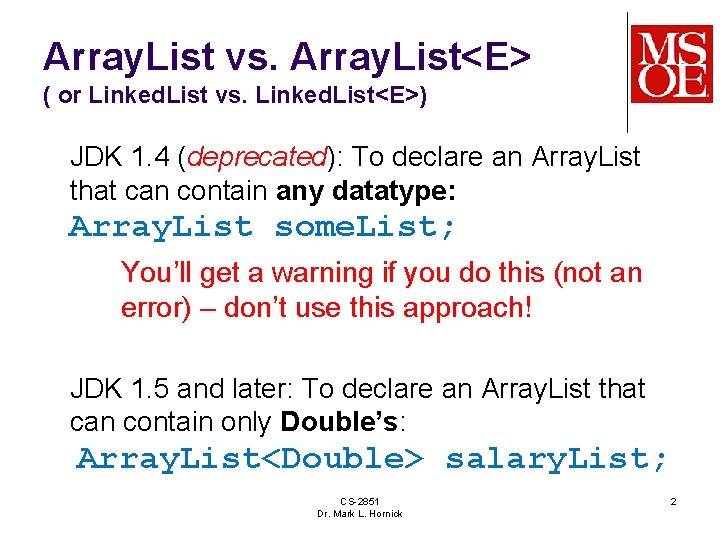
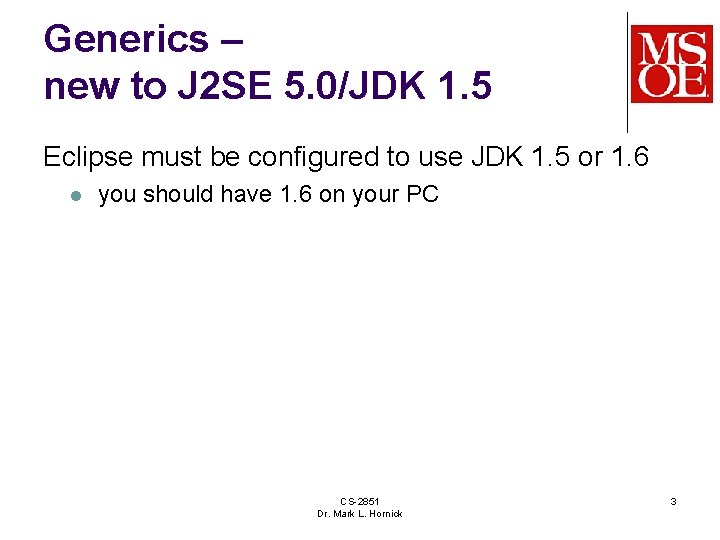
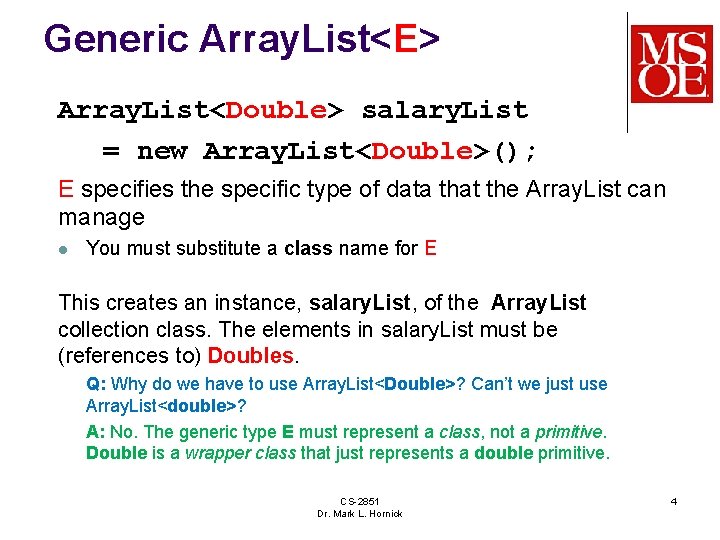
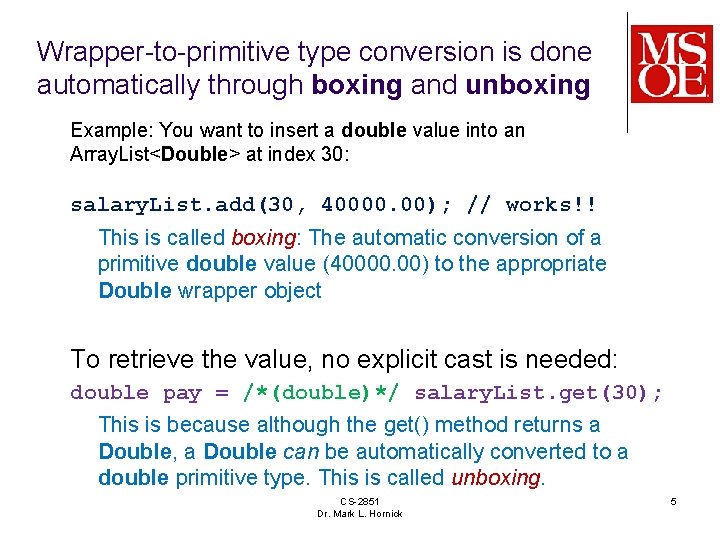
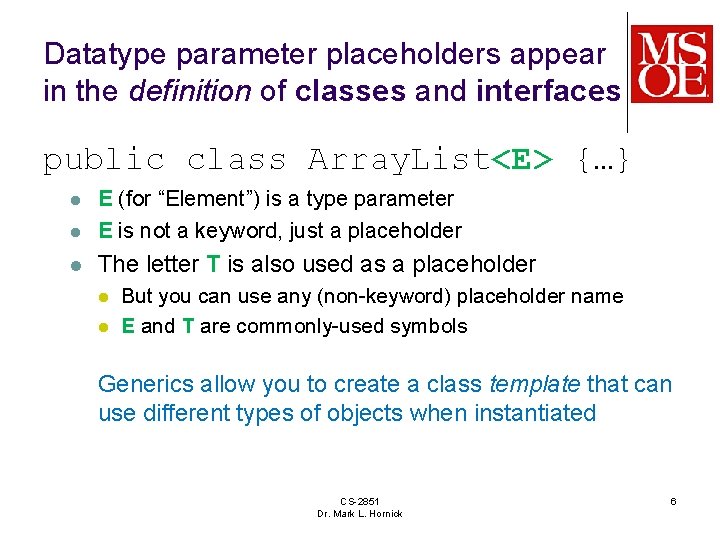
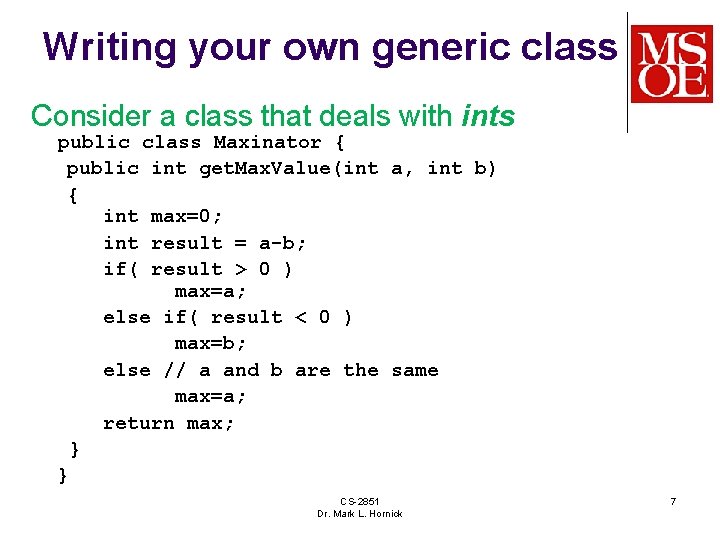
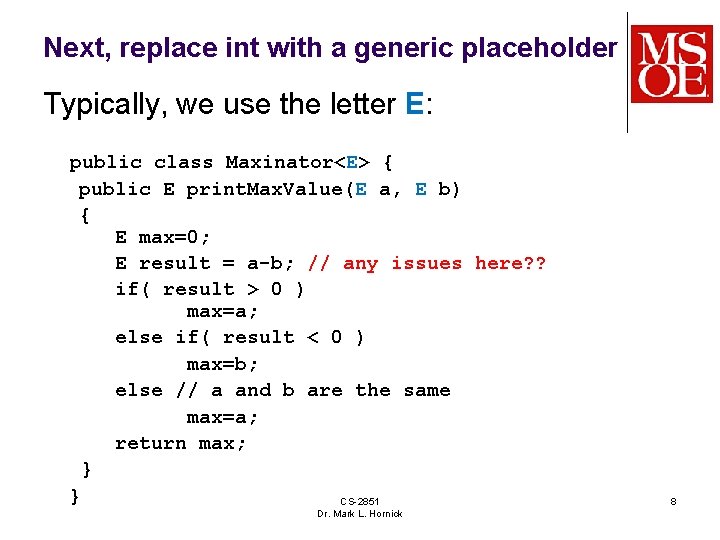
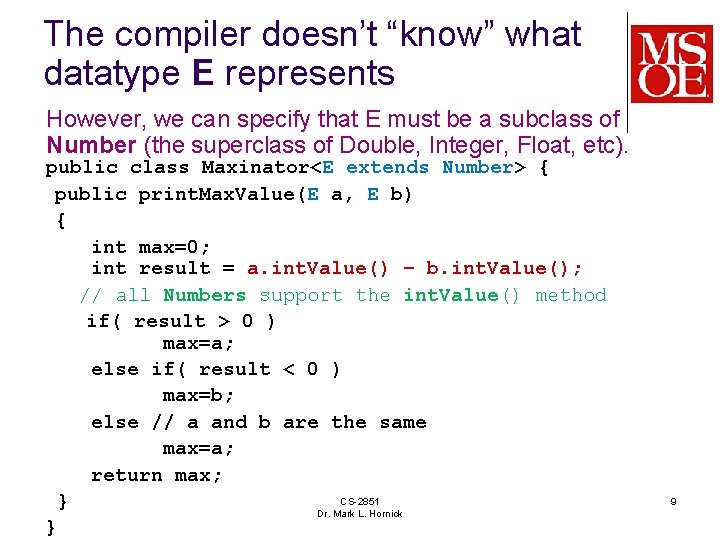
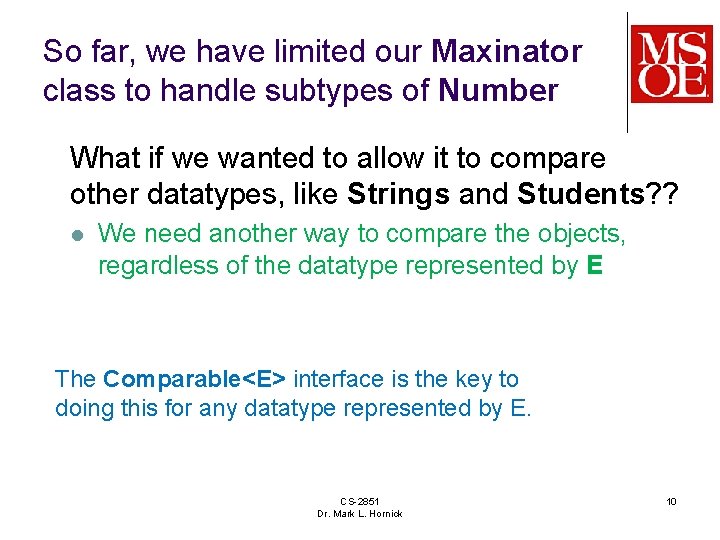
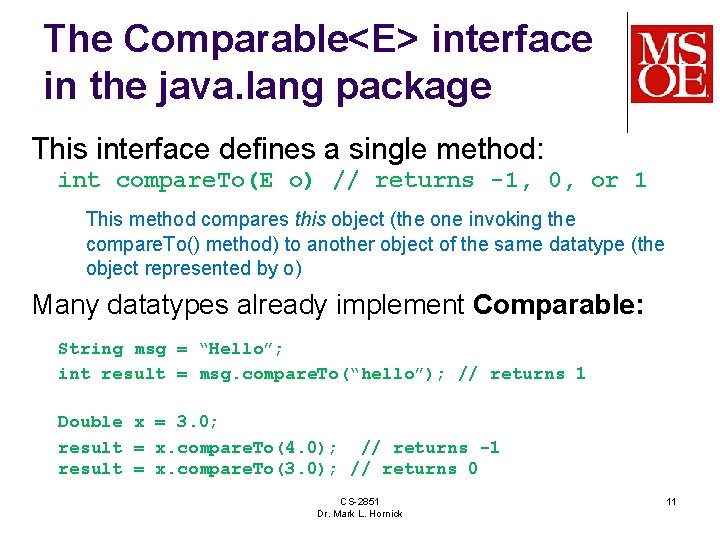
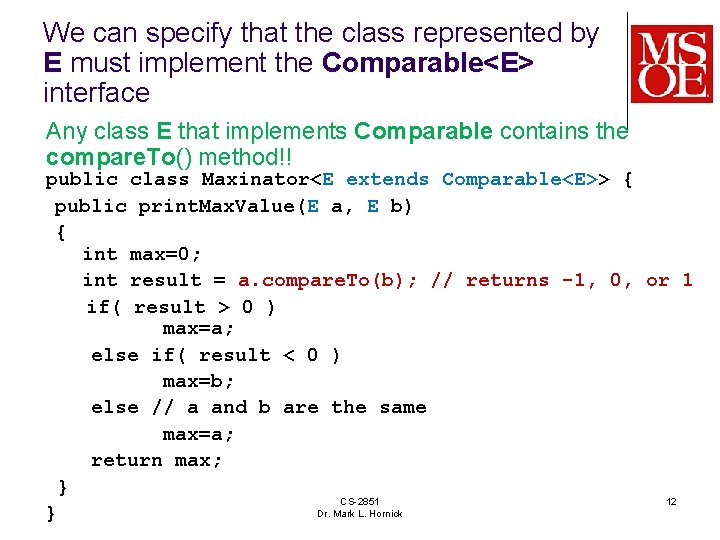
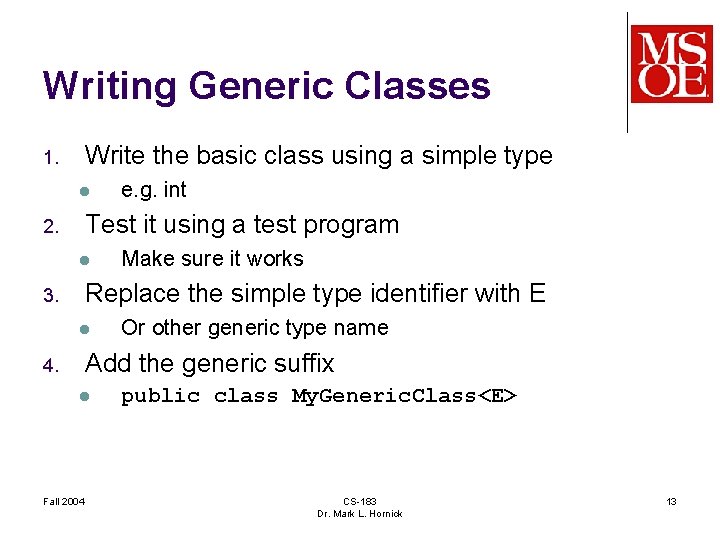
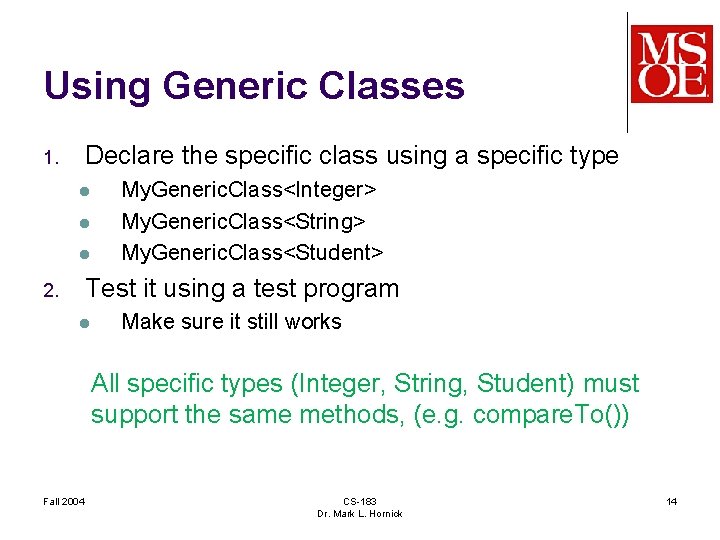
- Slides: 14
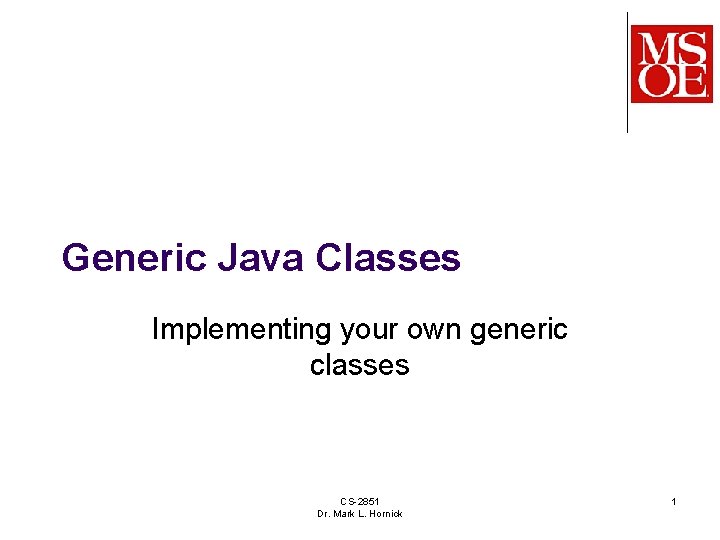
Generic Java Classes Implementing your own generic classes CS-2851 Dr. Mark L. Hornick 1
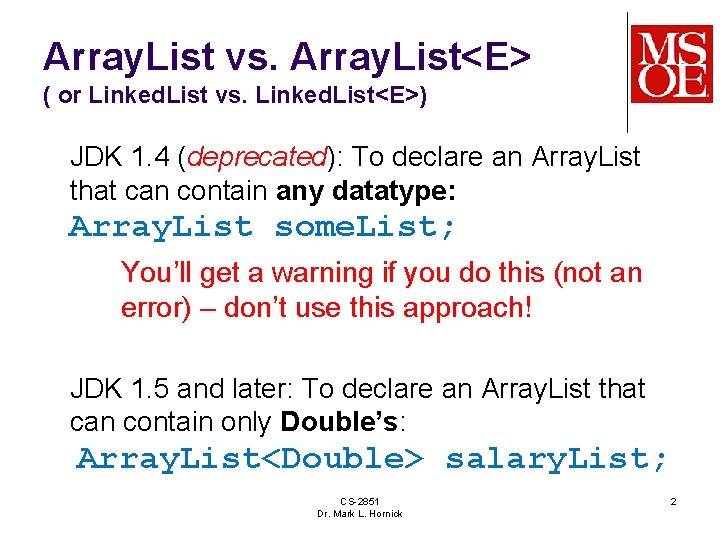
Array. List vs. Array. List<E> ( or Linked. List vs. Linked. List<E>) JDK 1. 4 (deprecated): To declare an Array. List that can contain any datatype: Array. List some. List; You’ll get a warning if you do this (not an error) – don’t use this approach! JDK 1. 5 and later: To declare an Array. List that can contain only Double’s: Array. List<Double> salary. List; CS-2851 Dr. Mark L. Hornick 2
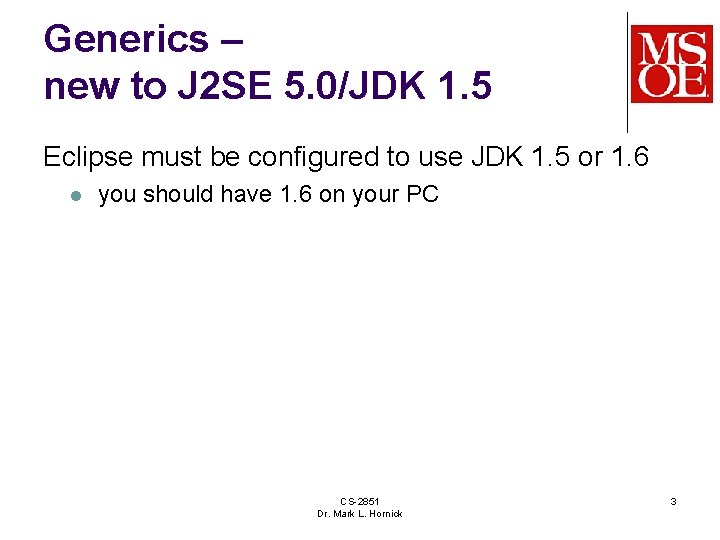
Generics – new to J 2 SE 5. 0/JDK 1. 5 Eclipse must be configured to use JDK 1. 5 or 1. 6 l you should have 1. 6 on your PC CS-2851 Dr. Mark L. Hornick 3
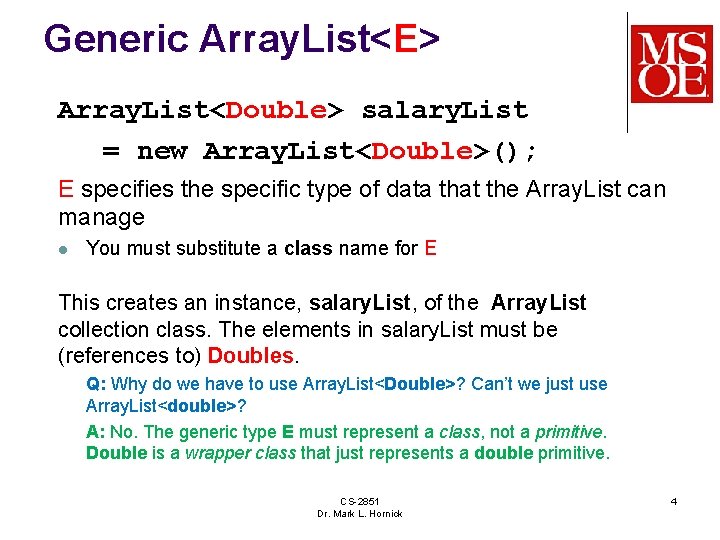
Generic Array. List<E> Array. List<Double> salary. List = new Array. List<Double>(); E specifies the specific type of data that the Array. List can manage l You must substitute a class name for E This creates an instance, salary. List, of the Array. List collection class. The elements in salary. List must be (references to) Doubles. Q: Why do we have to use Array. List<Double>? Can’t we just use Array. List<double>? A: No. The generic type E must represent a class, not a primitive. Double is a wrapper class that just represents a double primitive. CS-2851 Dr. Mark L. Hornick 4
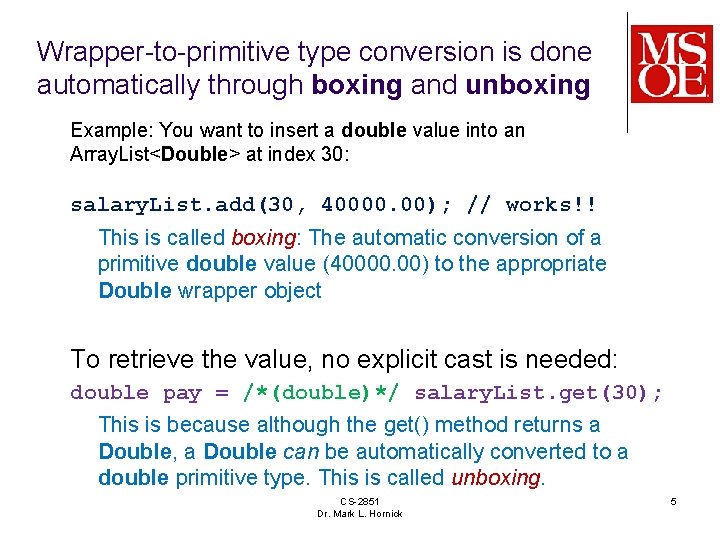
Wrapper-to-primitive type conversion is done automatically through boxing and unboxing Example: You want to insert a double value into an Array. List<Double> at index 30: salary. List. add(30, 40000. 00); // works!! This is called boxing: The automatic conversion of a primitive double value (40000. 00) to the appropriate Double wrapper object To retrieve the value, no explicit cast is needed: double pay = /*(double)*/ salary. List. get(30); This is because although the get() method returns a Double, a Double can be automatically converted to a double primitive type. This is called unboxing. CS-2851 Dr. Mark L. Hornick 5
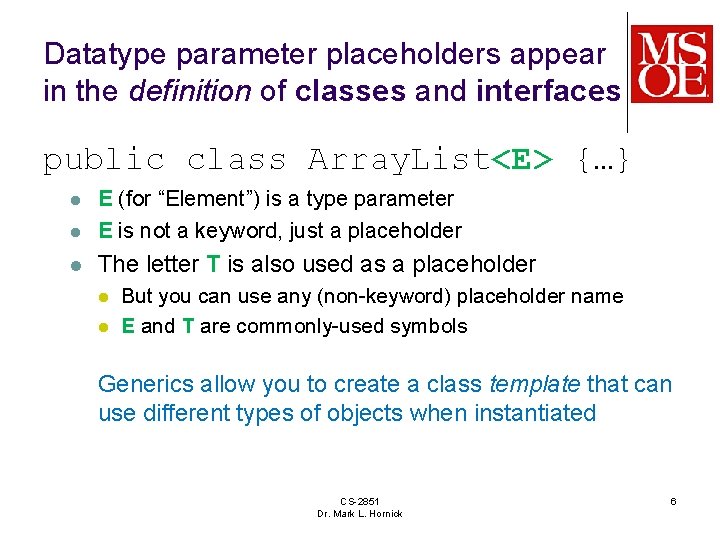
Datatype parameter placeholders appear in the definition of classes and interfaces public class Array. List<E> {…} l E (for “Element”) is a type parameter E is not a keyword, just a placeholder l The letter T is also used as a placeholder l l l But you can use any (non-keyword) placeholder name E and T are commonly-used symbols Generics allow you to create a class template that can use different types of objects when instantiated CS-2851 Dr. Mark L. Hornick 6
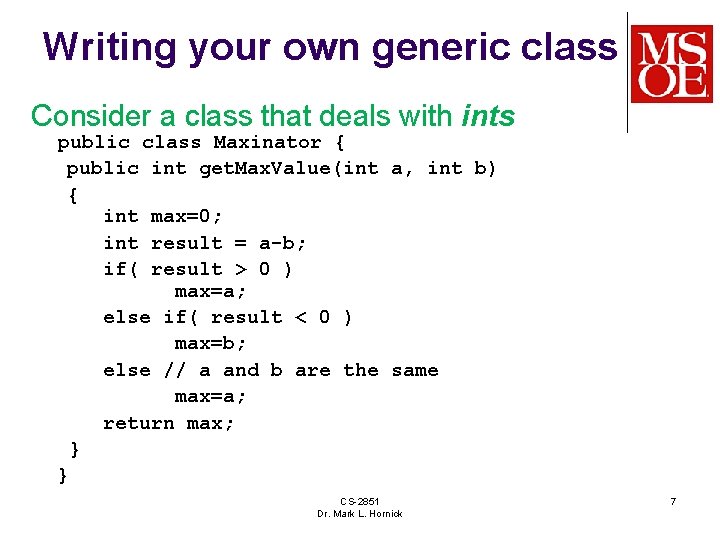
Writing your own generic class Consider a class that deals with ints public class Maxinator { public int get. Max. Value(int a, int b) { int max=0; int result = a-b; if( result > 0 ) max=a; else if( result < 0 ) max=b; else // a and b are the same max=a; return max; } } CS-2851 Dr. Mark L. Hornick 7
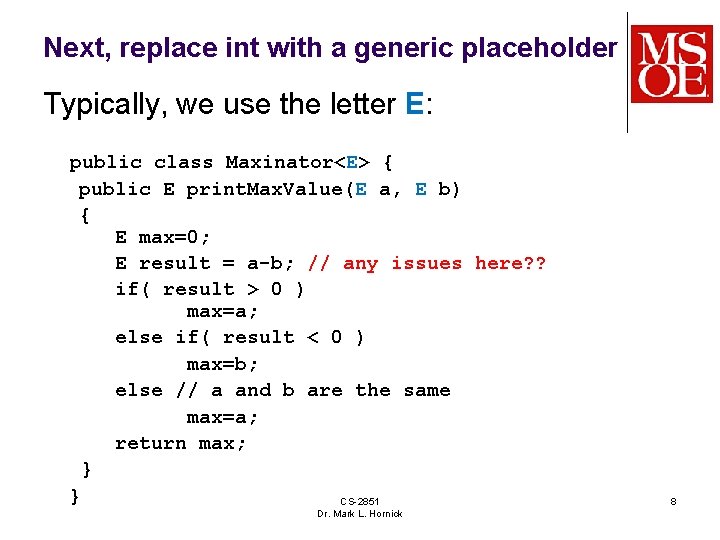
Next, replace int with a generic placeholder Typically, we use the letter E: public class Maxinator<E> { public E print. Max. Value(E a, E b) { E max=0; E result = a-b; // any issues here? ? if( result > 0 ) max=a; else if( result < 0 ) max=b; else // a and b are the same max=a; return max; } } CS-2851 Dr. Mark L. Hornick 8
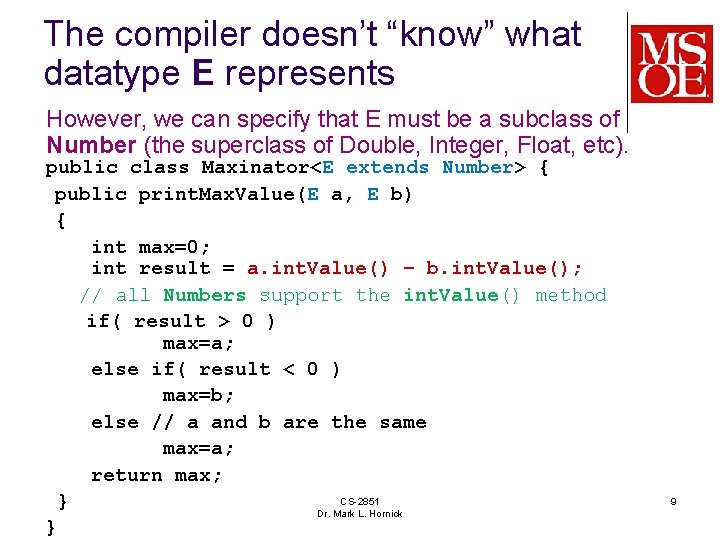
The compiler doesn’t “know” what datatype E represents However, we can specify that E must be a subclass of Number (the superclass of Double, Integer, Float, etc). public class Maxinator<E extends Number> { public print. Max. Value(E a, E b) { int max=0; int result = a. int. Value() – b. int. Value(); // all Numbers support the int. Value() method if( result > 0 ) max=a; else if( result < 0 ) max=b; else // a and b are the same max=a; return max; CS-2851 } Dr. Mark L. Hornick } 9
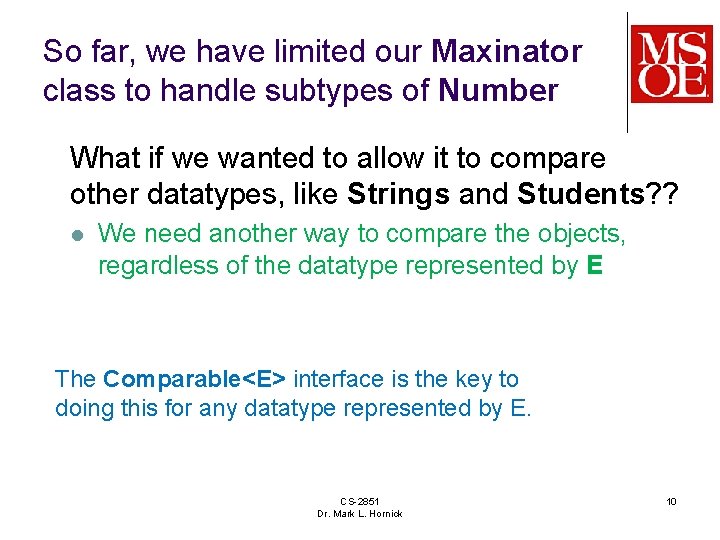
So far, we have limited our Maxinator class to handle subtypes of Number What if we wanted to allow it to compare other datatypes, like Strings and Students? ? l We need another way to compare the objects, regardless of the datatype represented by E The Comparable<E> interface is the key to doing this for any datatype represented by E. CS-2851 Dr. Mark L. Hornick 10
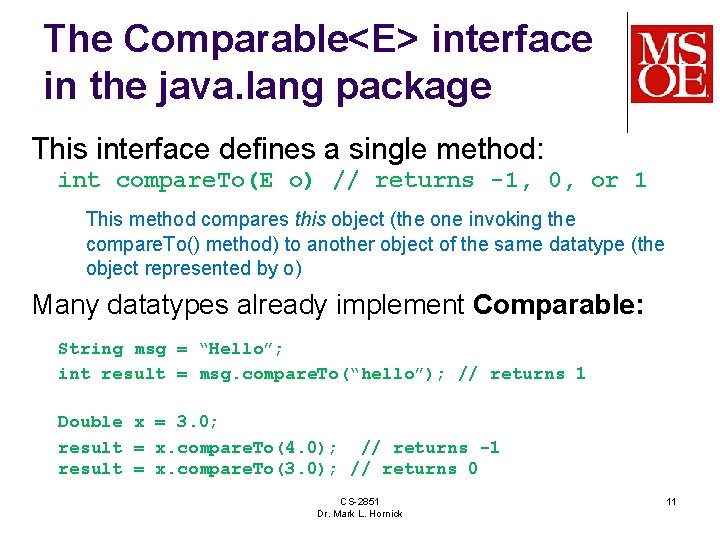
The Comparable<E> interface in the java. lang package This interface defines a single method: int compare. To(E o) // returns -1, 0, or 1 This method compares this object (the one invoking the compare. To() method) to another object of the same datatype (the object represented by o) Many datatypes already implement Comparable: String msg = “Hello”; int result = msg. compare. To(“hello”); // returns 1 Double x = 3. 0; result = x. compare. To(4. 0); // returns -1 result = x. compare. To(3. 0); // returns 0 CS-2851 Dr. Mark L. Hornick 11
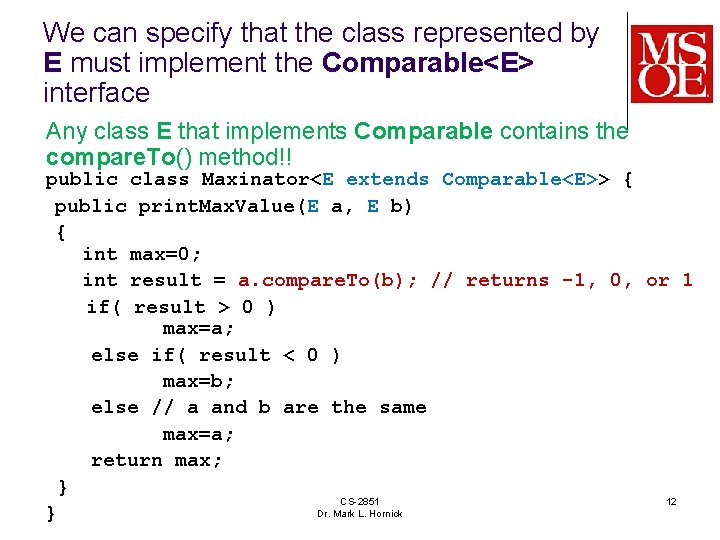
We can specify that the class represented by E must implement the Comparable<E> interface Any class E that implements Comparable contains the compare. To() method!! public class Maxinator<E extends Comparable<E>> { public print. Max. Value(E a, E b) { int max=0; int result = a. compare. To(b); // returns -1, 0, or 1 if( result > 0 ) max=a; else if( result < 0 ) max=b; else // a and b are the same max=a; return max; } CS-2851 12 Dr. Mark L. Hornick }
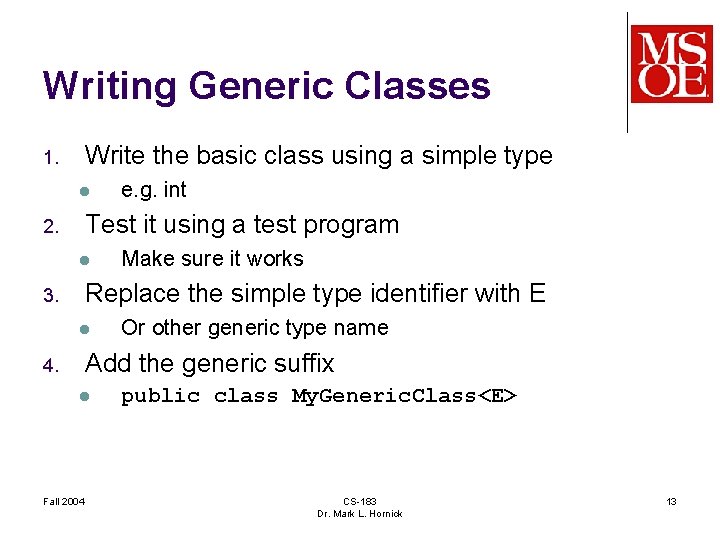
Writing Generic Classes Write the basic class using a simple type 1. l e. g. int Test it using a test program 2. l Make sure it works Replace the simple type identifier with E 3. l Or other generic type name Add the generic suffix 4. l Fall 2004 public class My. Generic. Class<E> CS-183 Dr. Mark L. Hornick 13
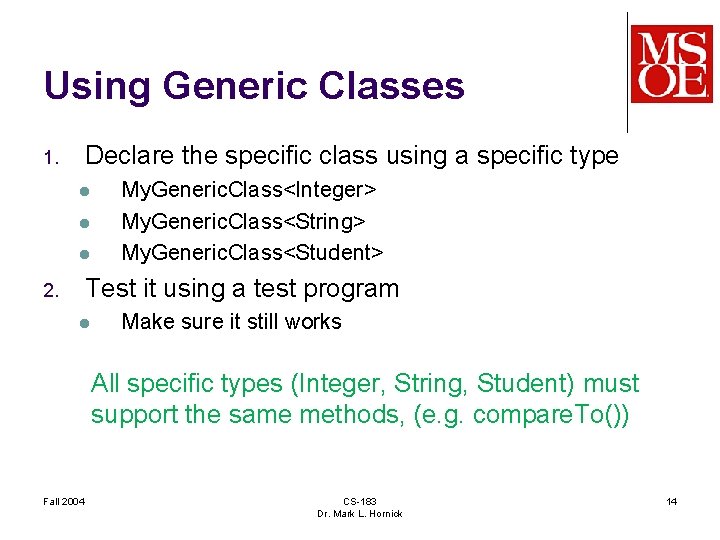
Using Generic Classes Declare the specific class using a specific type 1. l l l My. Generic. Class<Integer> My. Generic. Class<String> My. Generic. Class<Student> Test it using a test program 2. l Make sure it still works All specific types (Integer, String, Student) must support the same methods, (e. g. compare. To()) Fall 2004 CS-183 Dr. Mark L. Hornick 14
What type of sae is when you own your own business
Choose your own adventure java
In your notebook define the following terms
Your conscious awareness of your own name
Java generics array
Generic rest api java
Generic linked list in c
Classes e subclasses palavras
Pre ap classes vs regular classes
Give us your hungry your tired your poor
List button
Mouse adapter class in java
Thirosine
Abstract classes in java
Writing classes java