Functions Part 1 of 3 Topics Using Predefined
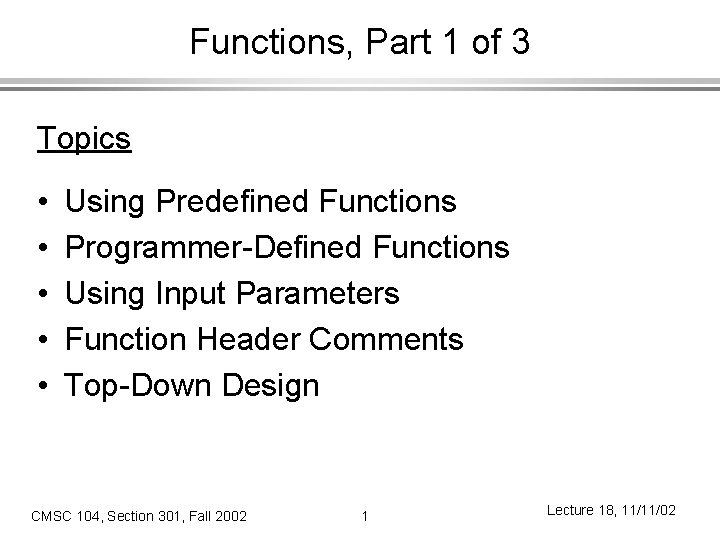
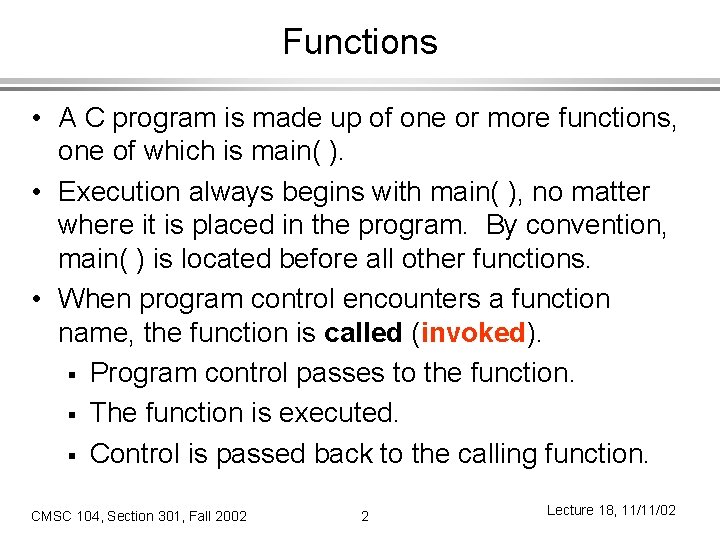
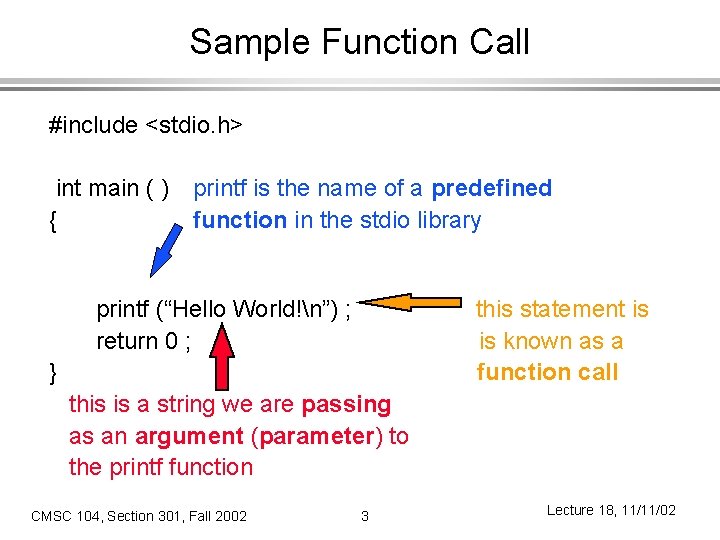
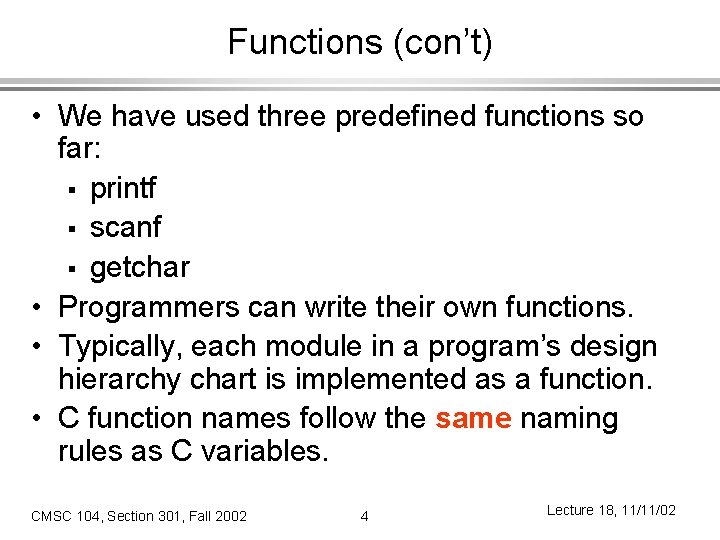
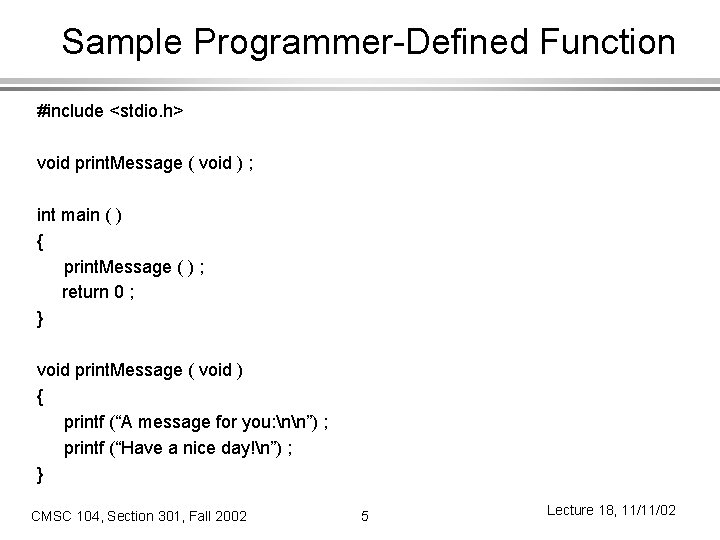
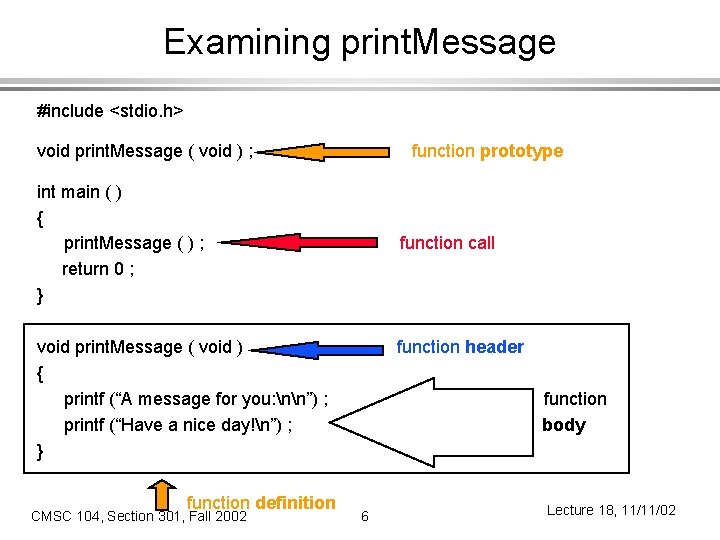
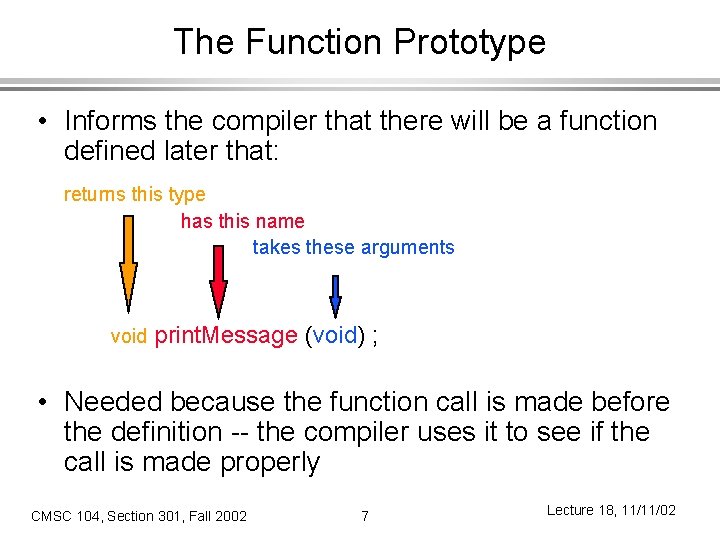
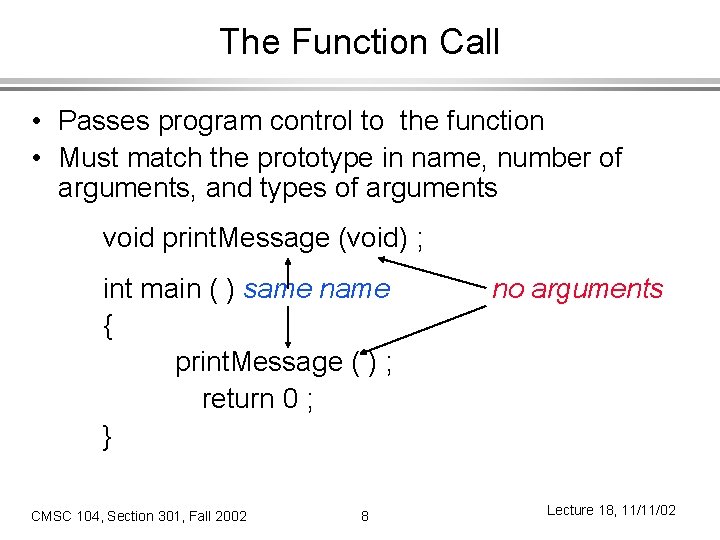
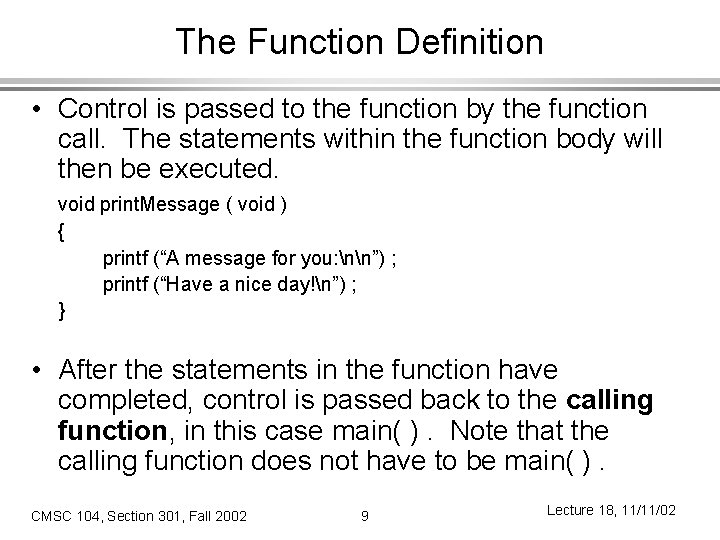
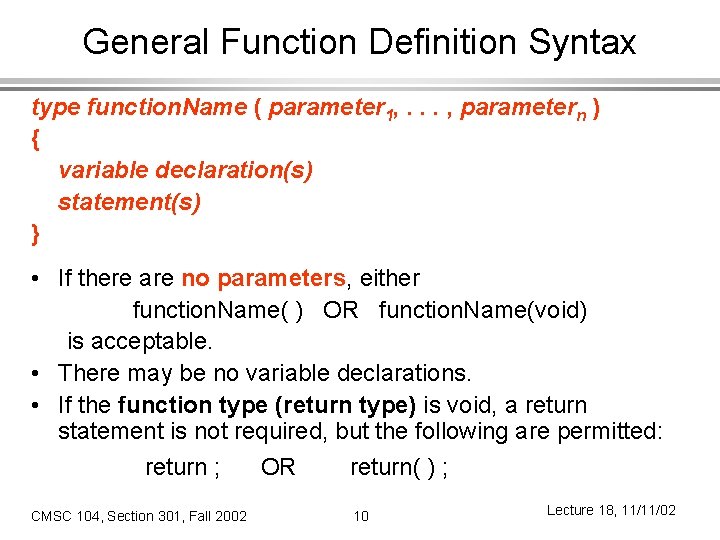
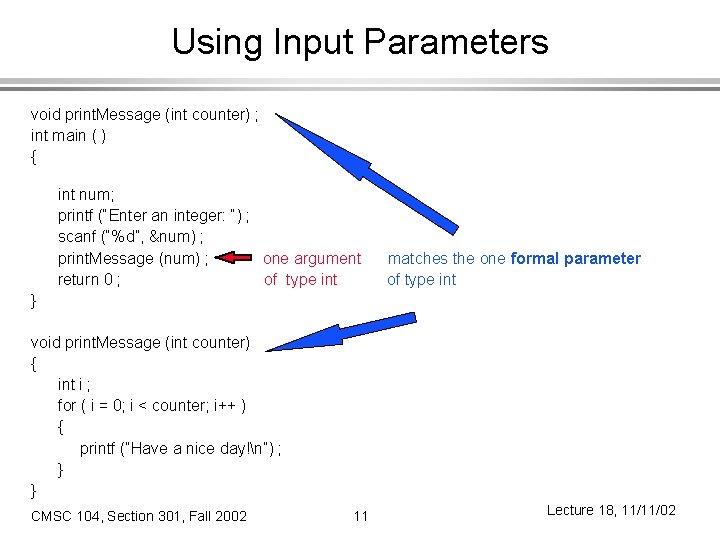
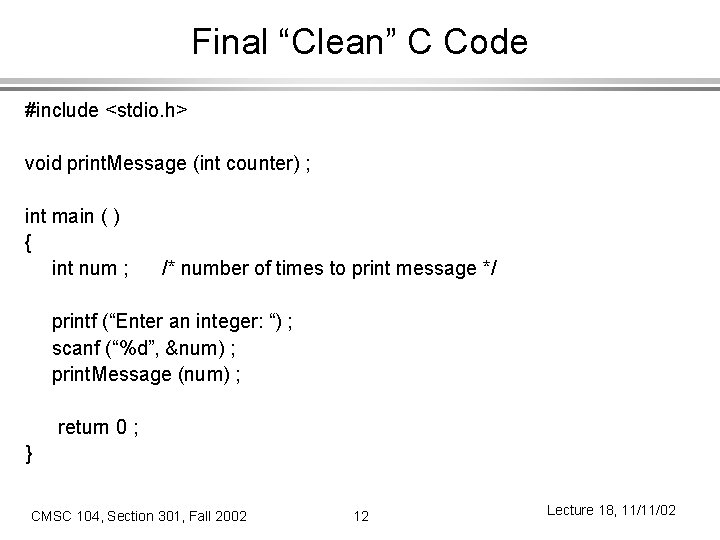
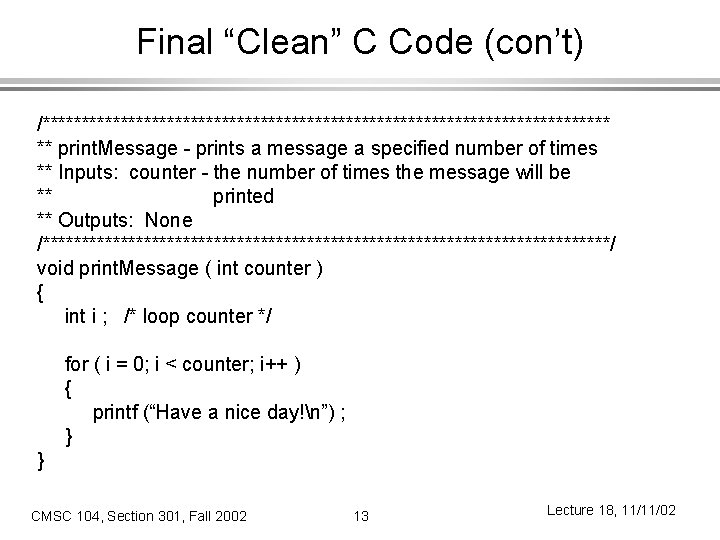
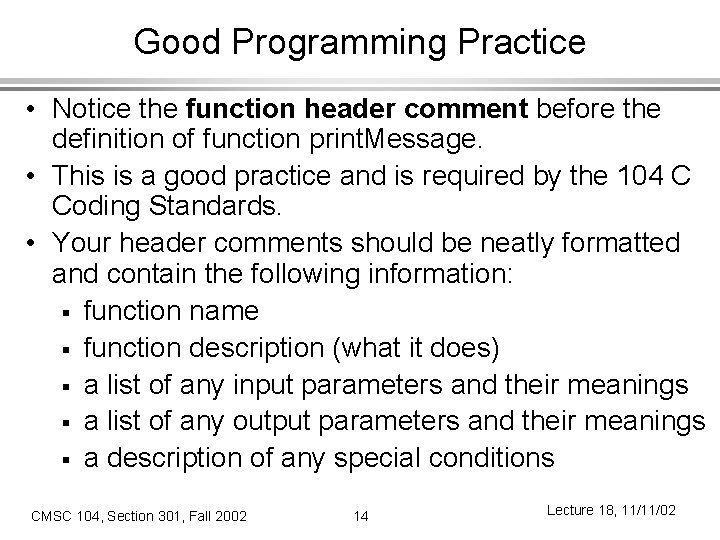
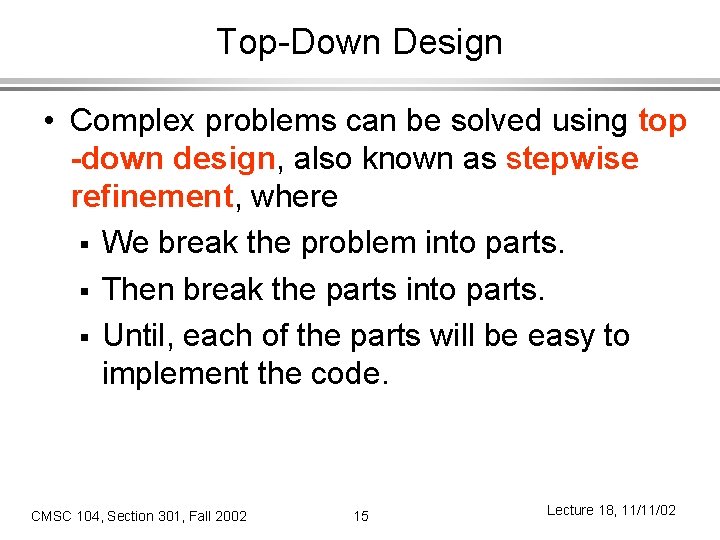
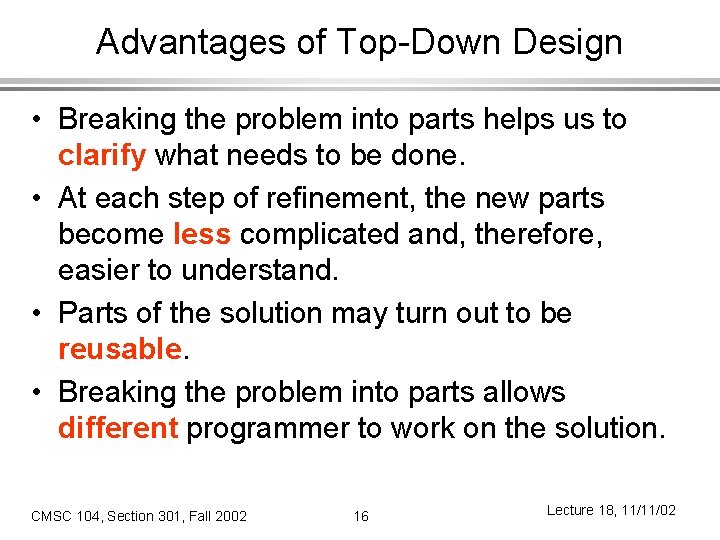
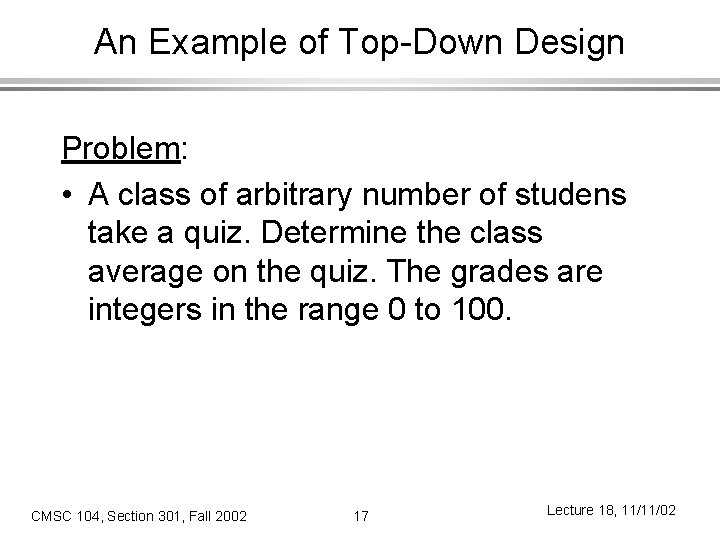
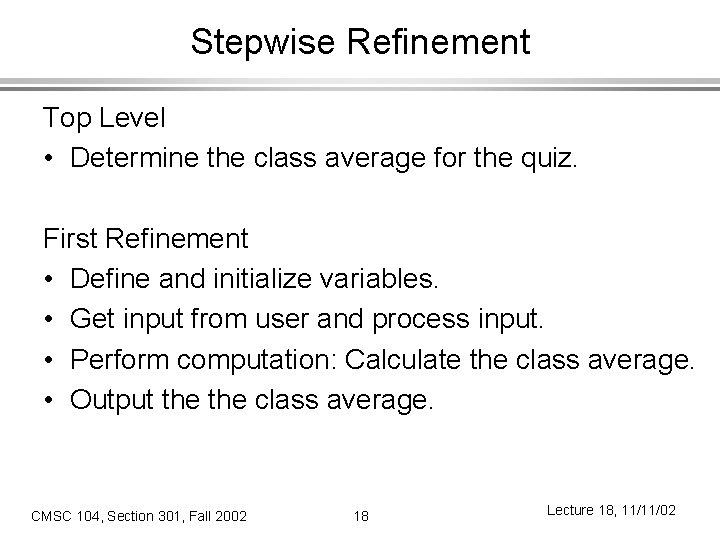
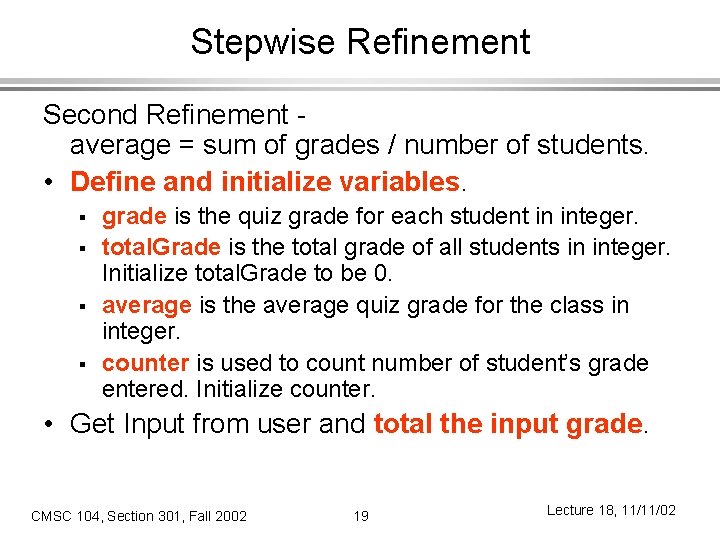
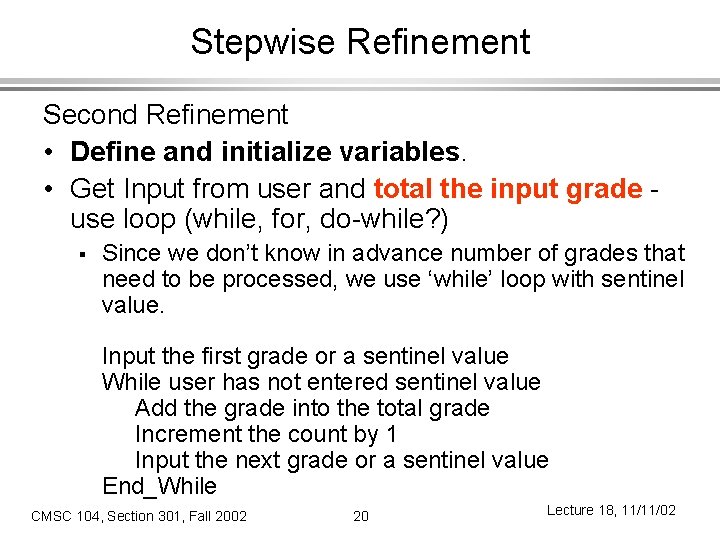
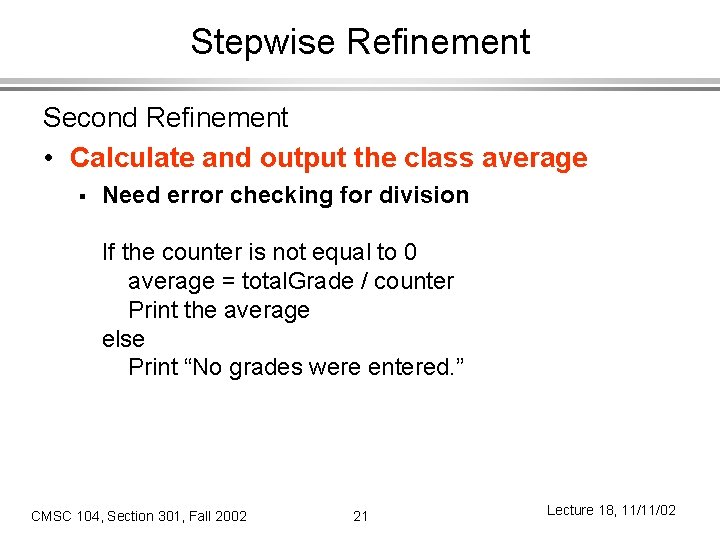
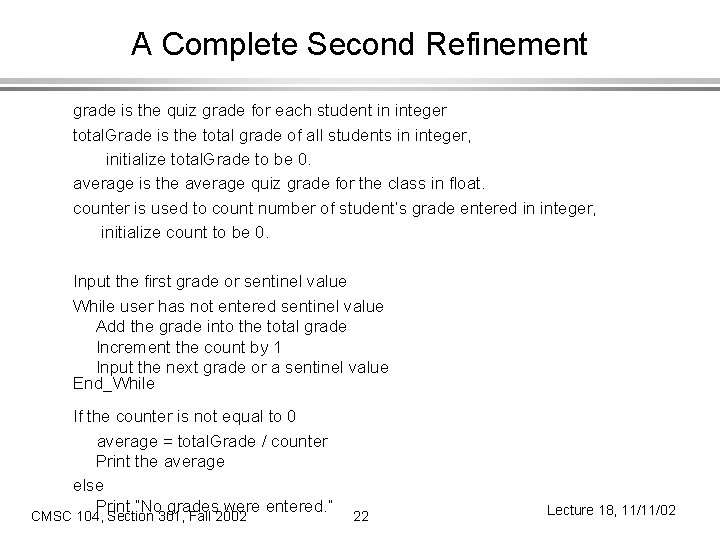
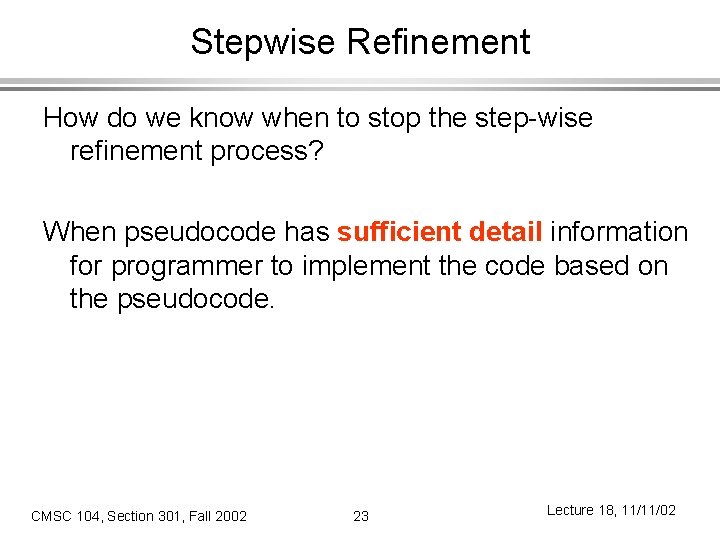
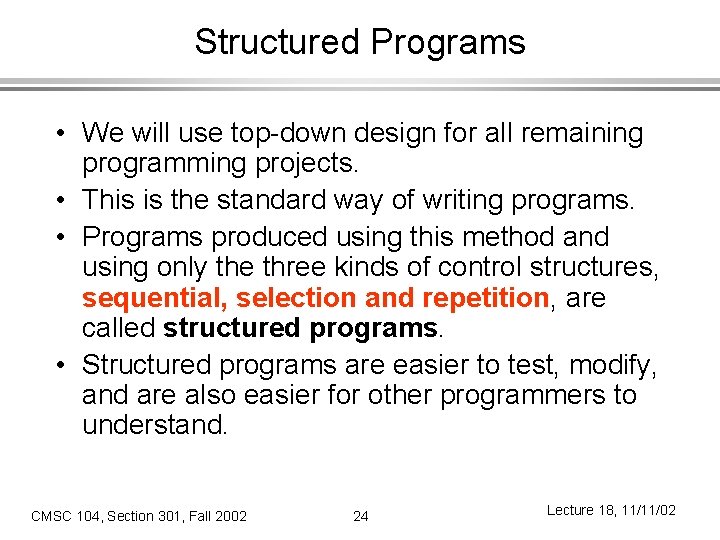
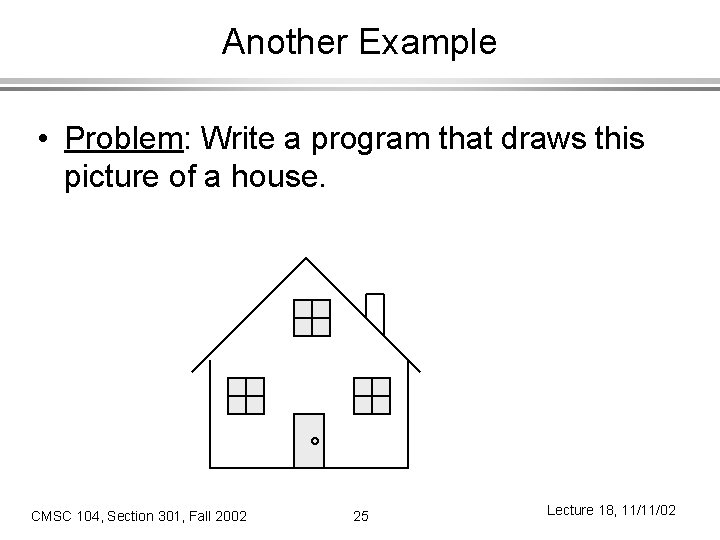
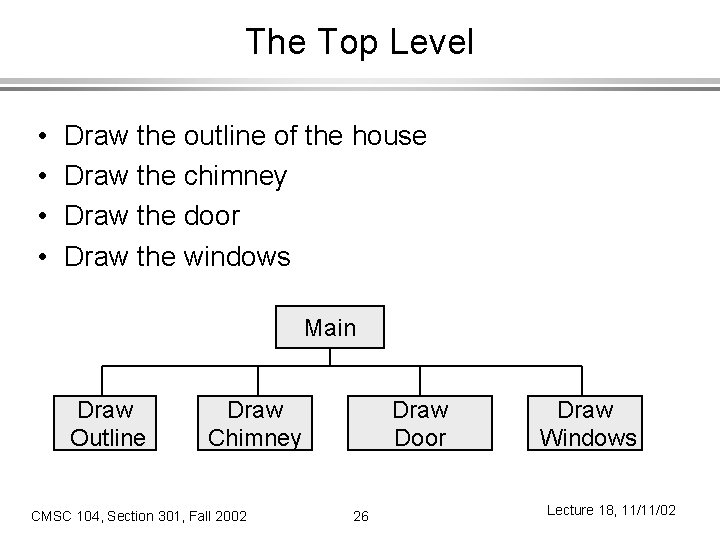
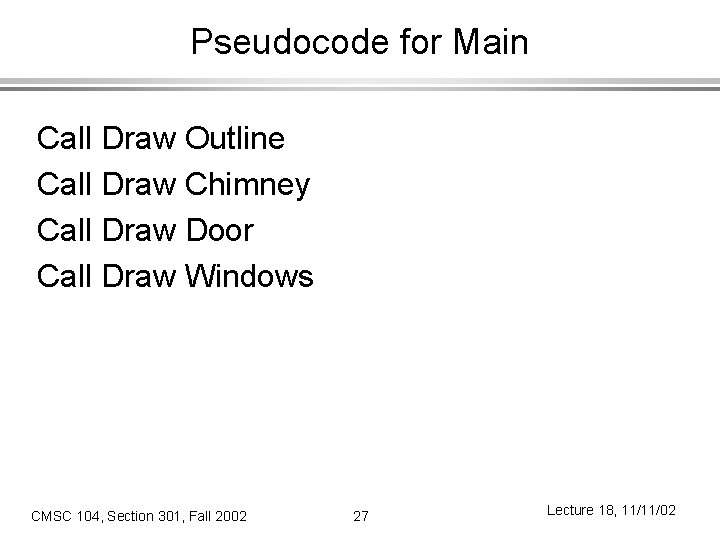
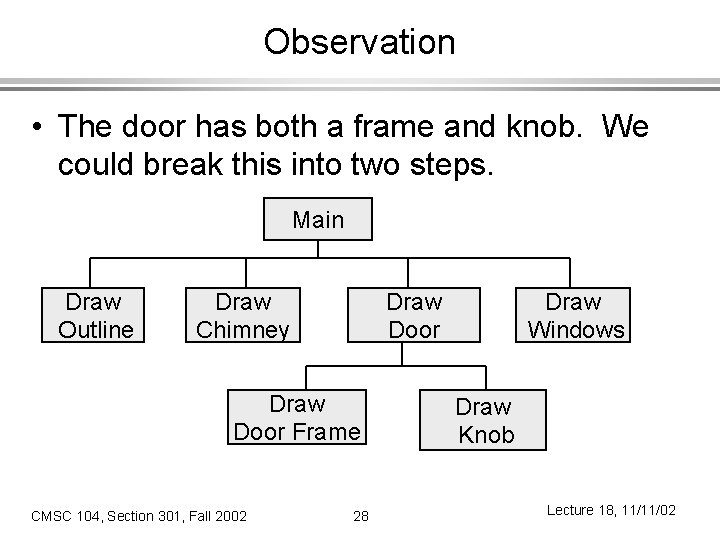
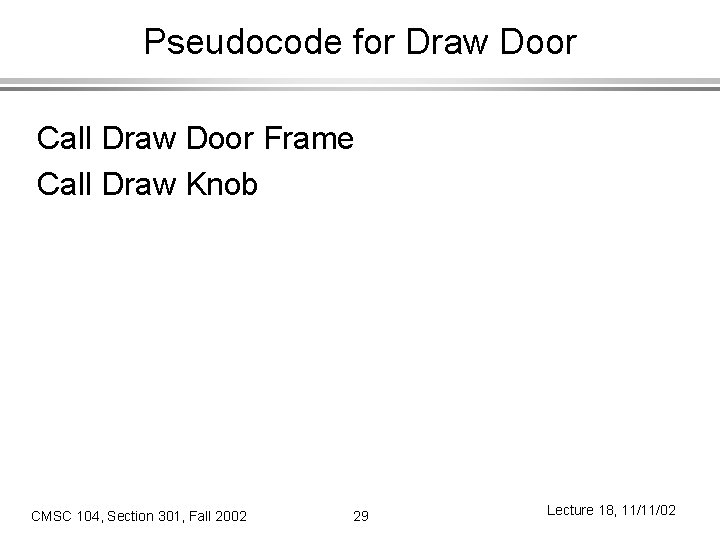
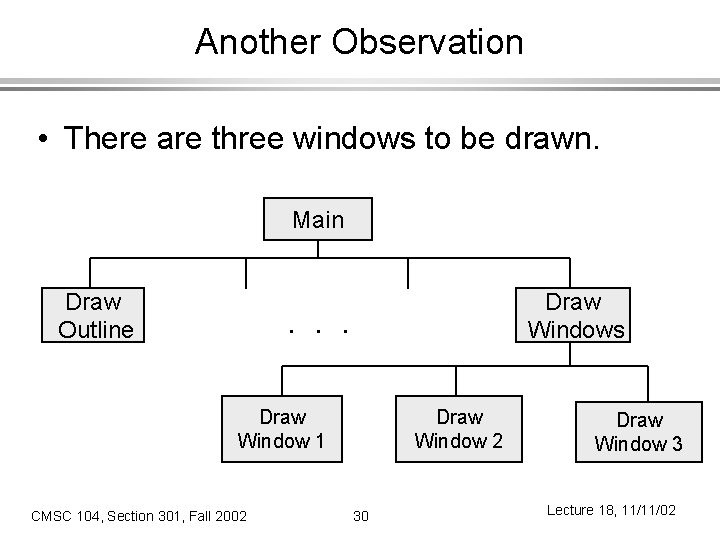
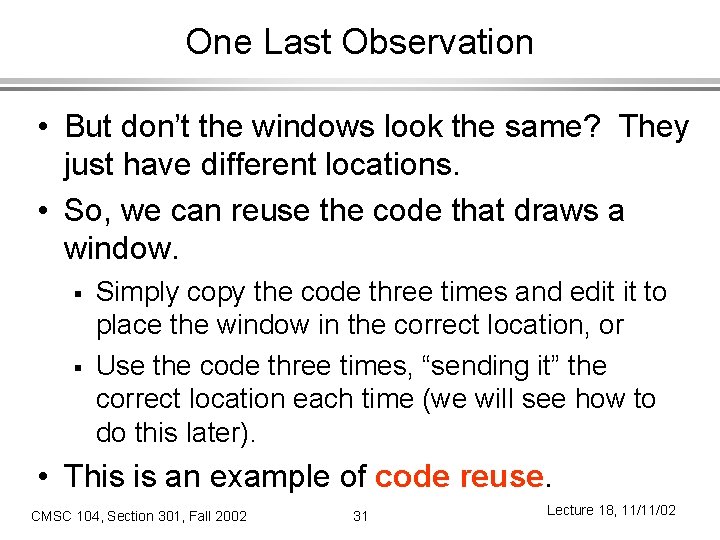
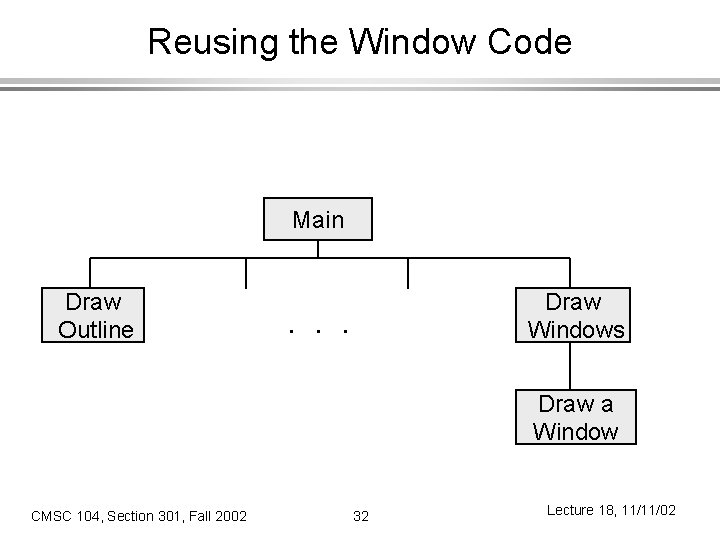
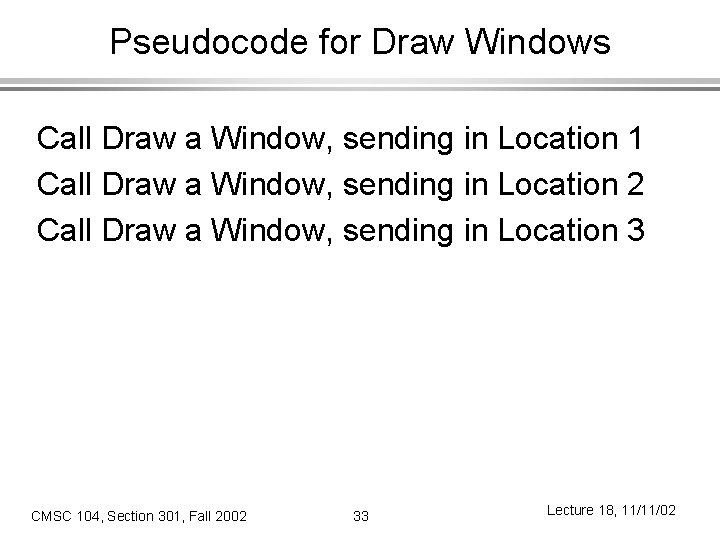
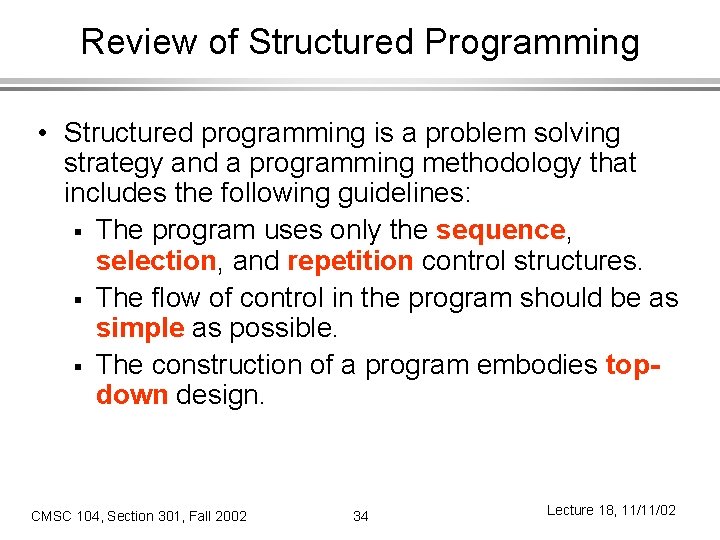
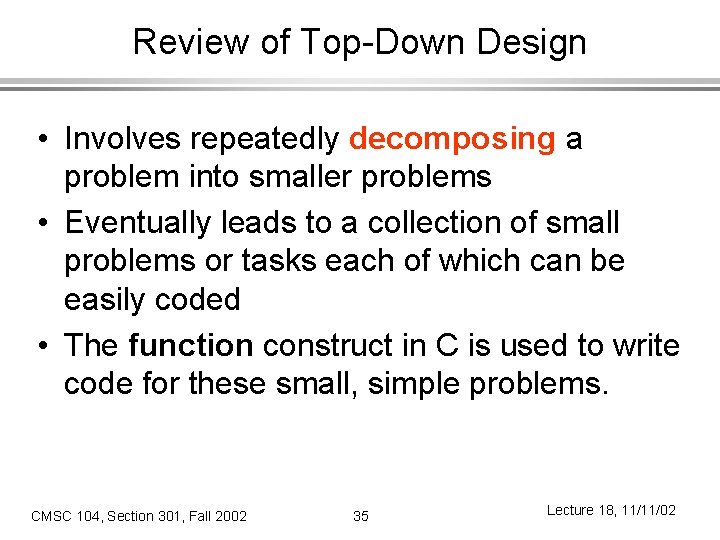
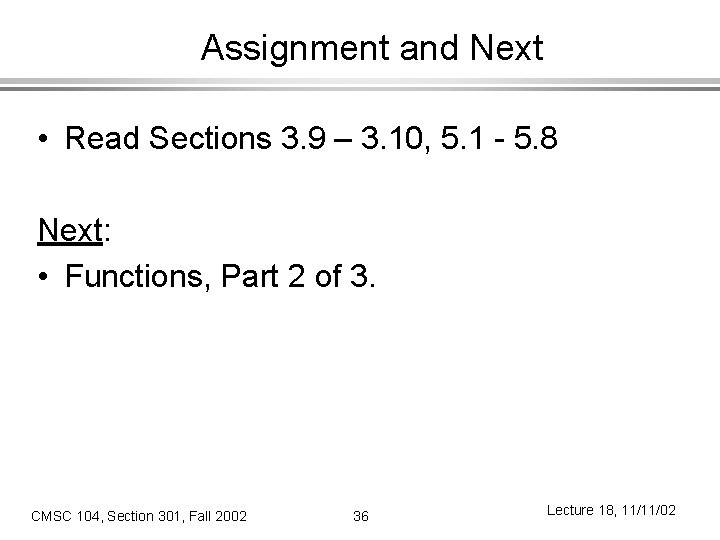
- Slides: 36
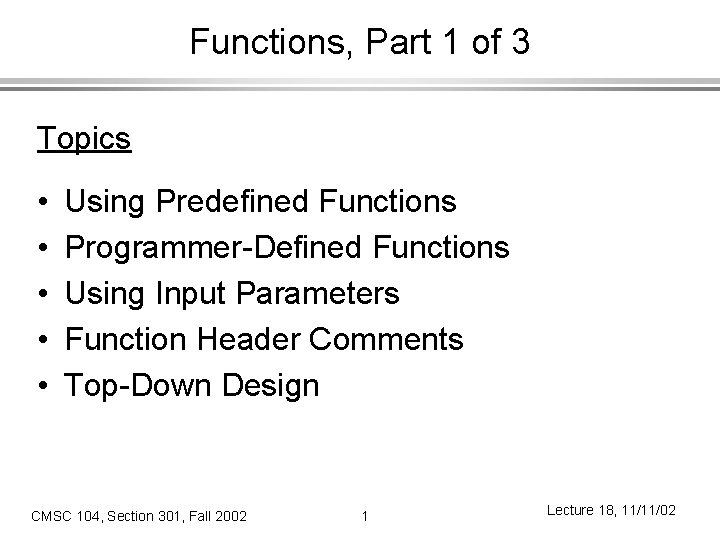
Functions, Part 1 of 3 Topics • • • Using Predefined Functions Programmer-Defined Functions Using Input Parameters Function Header Comments Top-Down Design CMSC 104, Section 301, Fall 2002 1 Lecture 18, 11/11/02
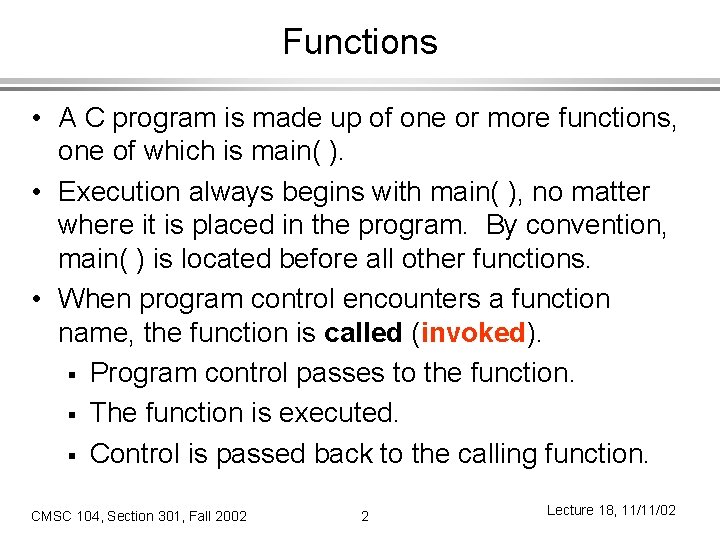
Functions • A C program is made up of one or more functions, one of which is main( ). • Execution always begins with main( ), no matter where it is placed in the program. By convention, main( ) is located before all other functions. • When program control encounters a function name, the function is called (invoked). § Program control passes to the function. § The function is executed. § Control is passed back to the calling function. CMSC 104, Section 301, Fall 2002 2 Lecture 18, 11/11/02
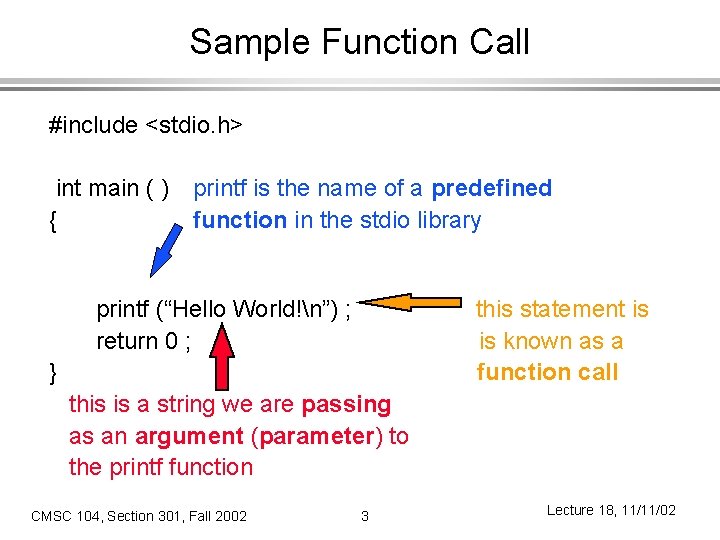
Sample Function Call #include <stdio. h> int main ( ) { printf is the name of a predefined function in the stdio library printf (“Hello World!n”) ; return 0 ; this statement is is known as a function call } this is a string we are passing as an argument (parameter) to the printf function CMSC 104, Section 301, Fall 2002 3 Lecture 18, 11/11/02
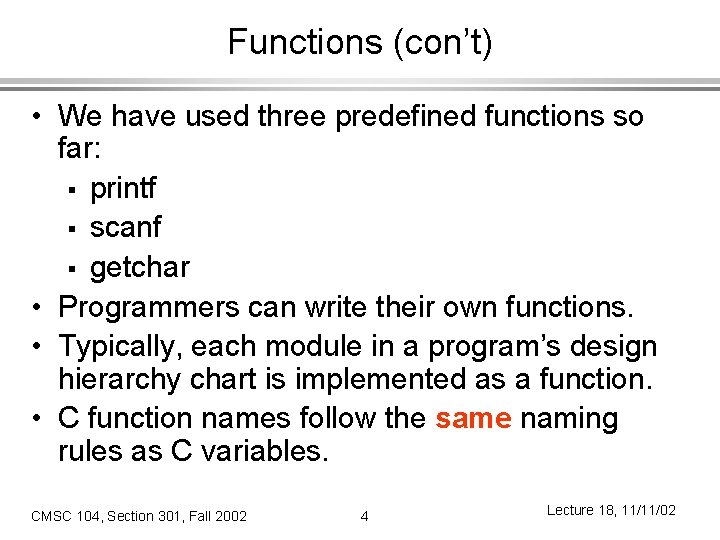
Functions (con’t) • We have used three predefined functions so far: § printf § scanf § getchar • Programmers can write their own functions. • Typically, each module in a program’s design hierarchy chart is implemented as a function. • C function names follow the same naming rules as C variables. CMSC 104, Section 301, Fall 2002 4 Lecture 18, 11/11/02
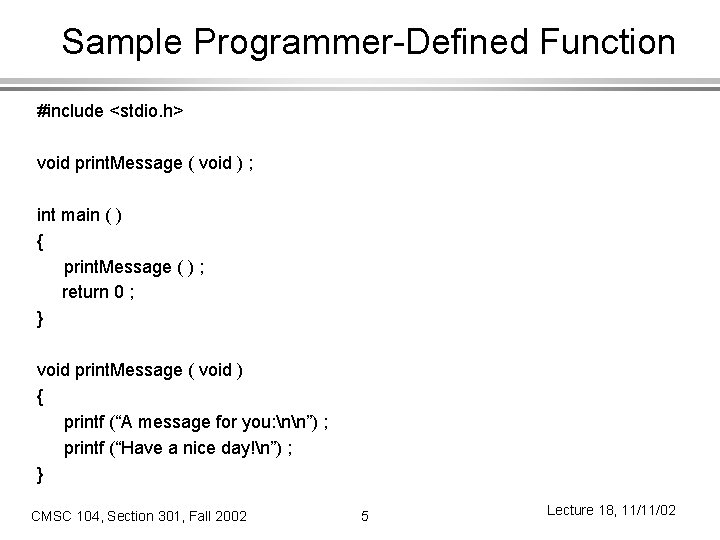
Sample Programmer-Defined Function #include <stdio. h> void print. Message ( void ) ; int main ( ) { print. Message ( ) ; return 0 ; } void print. Message ( void ) { printf (“A message for you: nn”) ; printf (“Have a nice day!n”) ; } CMSC 104, Section 301, Fall 2002 5 Lecture 18, 11/11/02
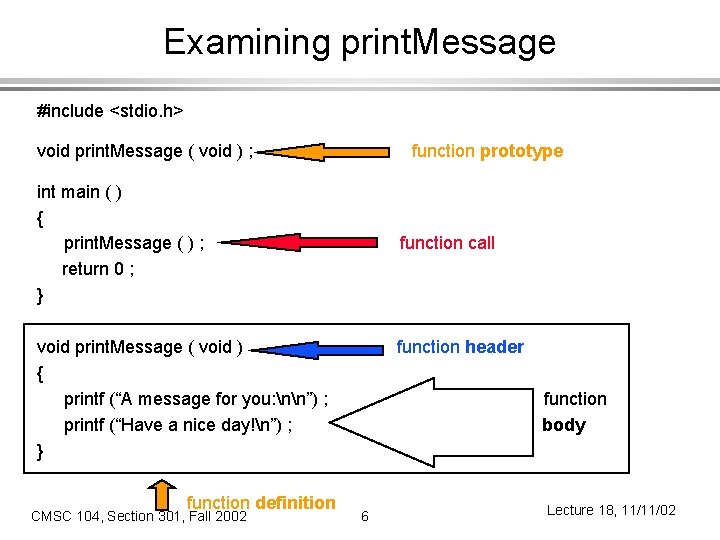
Examining print. Message #include <stdio. h> void print. Message ( void ) ; function prototype int main ( ) { print. Message ( ) ; return 0 ; } function call void print. Message ( void ) { printf (“A message for you: nn”) ; printf (“Have a nice day!n”) ; } function definition CMSC 104, Section 301, Fall 2002 function header function body 6 Lecture 18, 11/11/02
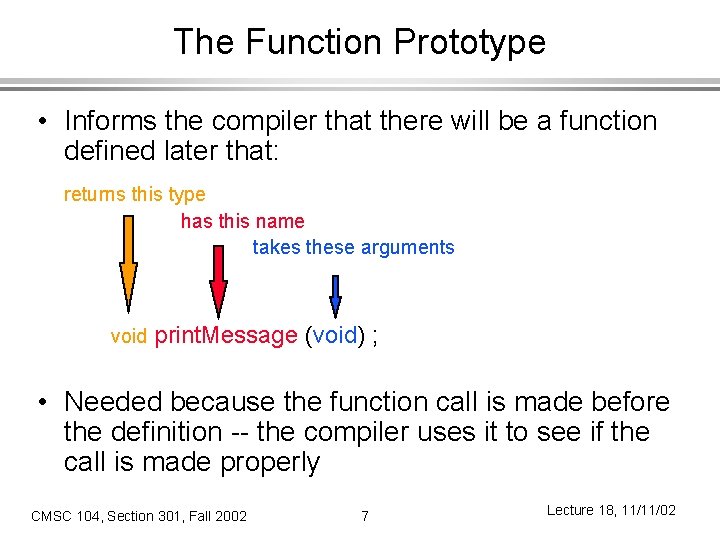
The Function Prototype • Informs the compiler that there will be a function defined later that: returns this type has this name takes these arguments void print. Message (void) ; • Needed because the function call is made before the definition -- the compiler uses it to see if the call is made properly CMSC 104, Section 301, Fall 2002 7 Lecture 18, 11/11/02
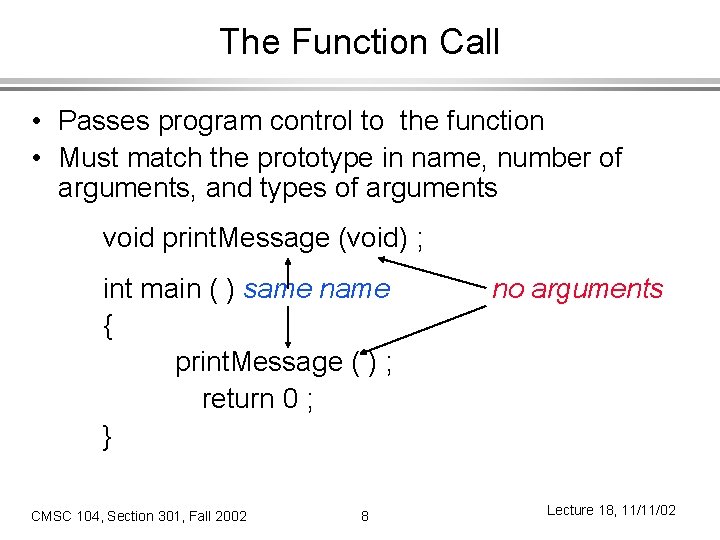
The Function Call • Passes program control to the function • Must match the prototype in name, number of arguments, and types of arguments void print. Message (void) ; int main ( ) same name { print. Message ( ) ; return 0 ; } CMSC 104, Section 301, Fall 2002 8 no arguments Lecture 18, 11/11/02
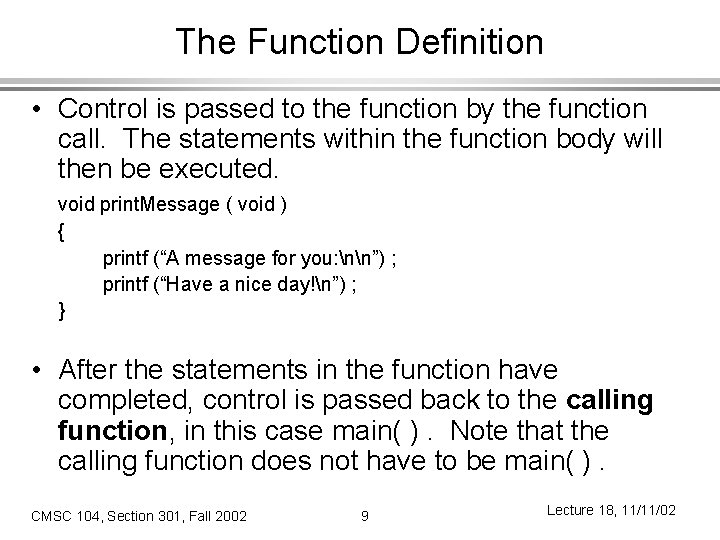
The Function Definition • Control is passed to the function by the function call. The statements within the function body will then be executed. void print. Message ( void ) { printf (“A message for you: nn”) ; printf (“Have a nice day!n”) ; } • After the statements in the function have completed, control is passed back to the calling function, in this case main( ). Note that the calling function does not have to be main( ). CMSC 104, Section 301, Fall 2002 9 Lecture 18, 11/11/02
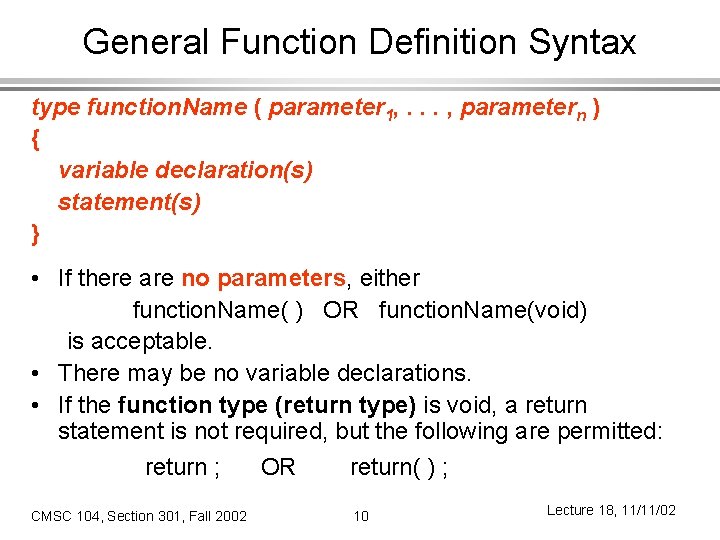
General Function Definition Syntax type function. Name ( parameter 1, . . . , parametern ) { variable declaration(s) statement(s) } • If there are no parameters, either function. Name( ) OR function. Name(void) is acceptable. • There may be no variable declarations. • If the function type (return type) is void, a return statement is not required, but the following are permitted: return ; CMSC 104, Section 301, Fall 2002 OR return( ) ; 10 Lecture 18, 11/11/02
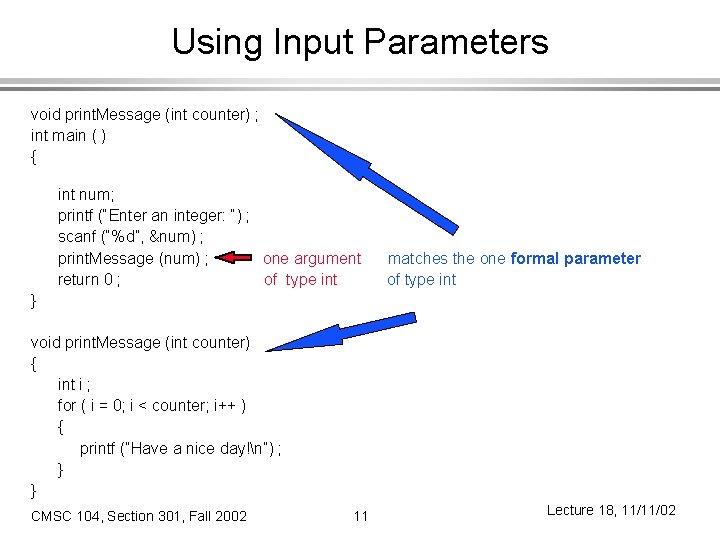
Using Input Parameters void print. Message (int counter) ; int main ( ) { int num; printf (“Enter an integer: “) ; scanf (“%d”, &num) ; print. Message (num) ; one argument return 0 ; of type int matches the one formal parameter of type int } void print. Message (int counter) { int i ; for ( i = 0; i < counter; i++ ) { printf (“Have a nice day!n”) ; } } CMSC 104, Section 301, Fall 2002 11 Lecture 18, 11/11/02
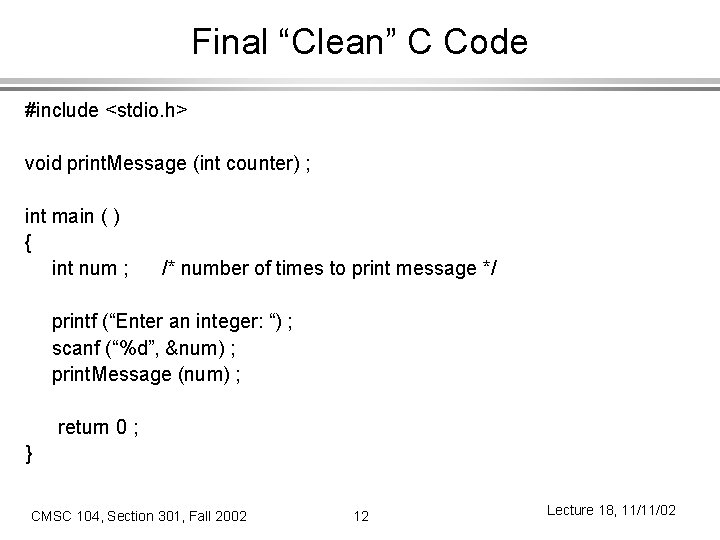
Final “Clean” C Code #include <stdio. h> void print. Message (int counter) ; int main ( ) { int num ; /* number of times to print message */ printf (“Enter an integer: “) ; scanf (“%d”, &num) ; print. Message (num) ; return 0 ; } CMSC 104, Section 301, Fall 2002 12 Lecture 18, 11/11/02
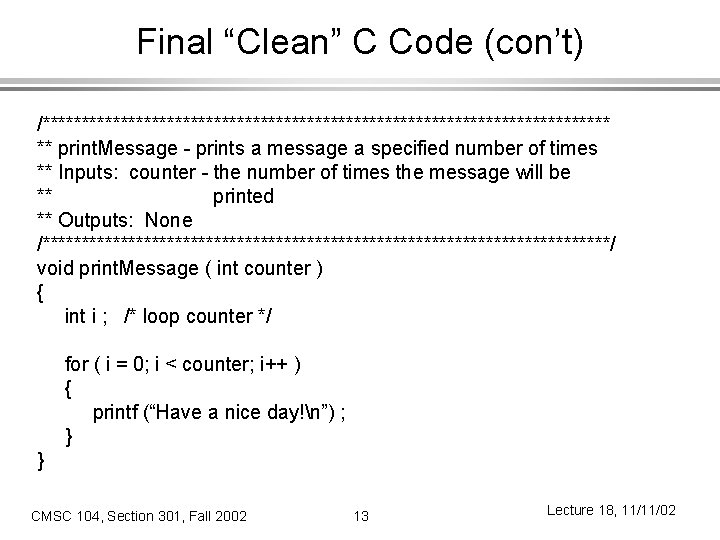
Final “Clean” C Code (con’t) /************************************* ** print. Message - prints a message a specified number of times ** Inputs: counter - the number of times the message will be ** printed ** Outputs: None /*************************************/ void print. Message ( int counter ) { int i ; /* loop counter */ for ( i = 0; i < counter; i++ ) { printf (“Have a nice day!n”) ; } } CMSC 104, Section 301, Fall 2002 13 Lecture 18, 11/11/02
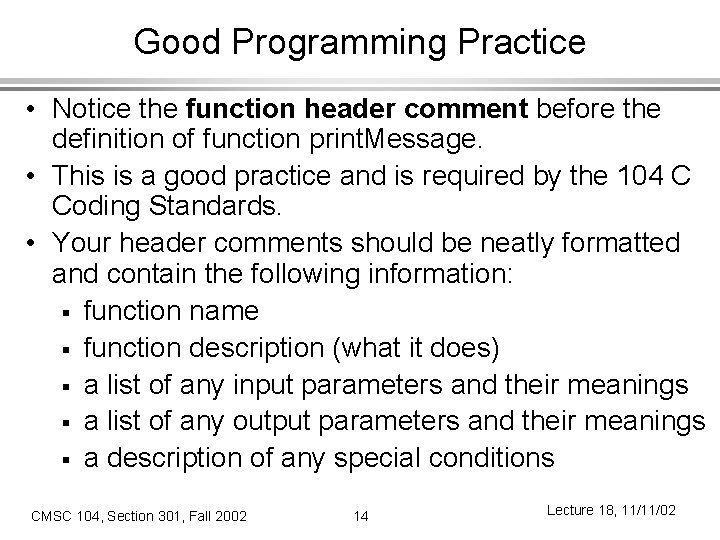
Good Programming Practice • Notice the function header comment before the definition of function print. Message. • This is a good practice and is required by the 104 C Coding Standards. • Your header comments should be neatly formatted and contain the following information: § function name § function description (what it does) § a list of any input parameters and their meanings § a list of any output parameters and their meanings § a description of any special conditions CMSC 104, Section 301, Fall 2002 14 Lecture 18, 11/11/02
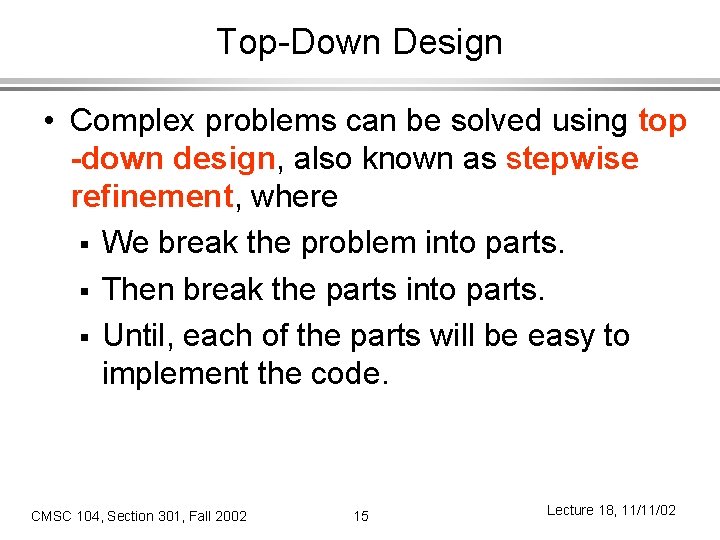
Top-Down Design • Complex problems can be solved using top -down design, also known as stepwise refinement, where § We break the problem into parts. § Then break the parts into parts. § Until, each of the parts will be easy to implement the code. CMSC 104, Section 301, Fall 2002 15 Lecture 18, 11/11/02
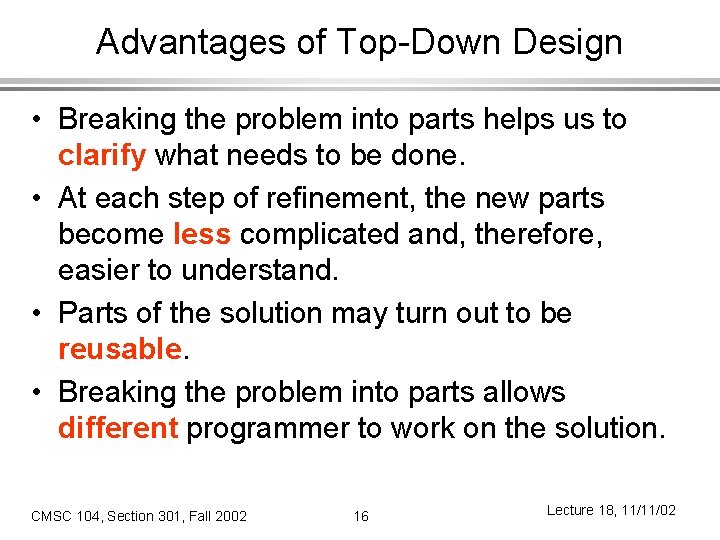
Advantages of Top-Down Design • Breaking the problem into parts helps us to clarify what needs to be done. • At each step of refinement, the new parts become less complicated and, therefore, easier to understand. • Parts of the solution may turn out to be reusable. • Breaking the problem into parts allows different programmer to work on the solution. CMSC 104, Section 301, Fall 2002 16 Lecture 18, 11/11/02
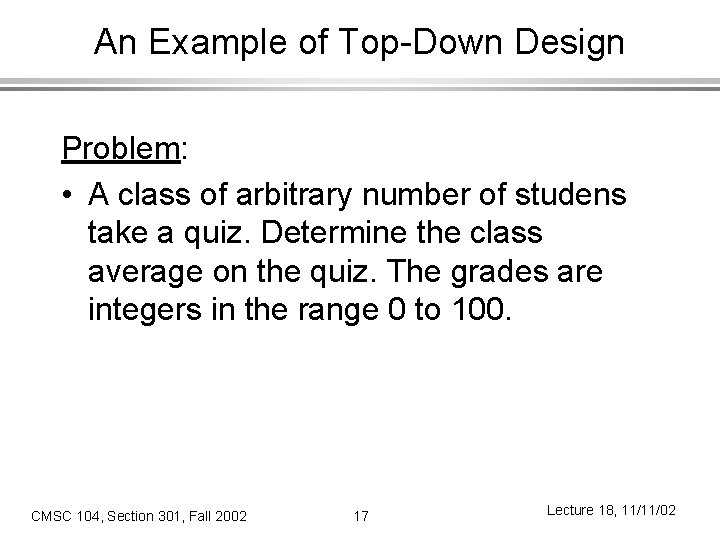
An Example of Top-Down Design Problem: • A class of arbitrary number of studens take a quiz. Determine the class average on the quiz. The grades are integers in the range 0 to 100. CMSC 104, Section 301, Fall 2002 17 Lecture 18, 11/11/02
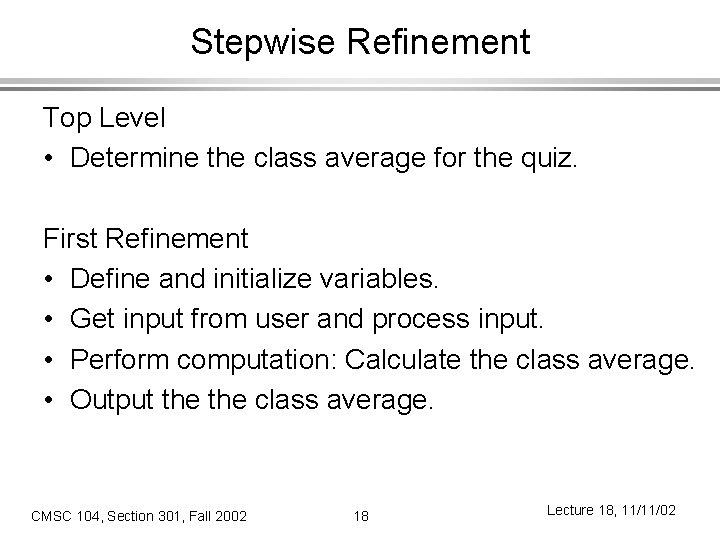
Stepwise Refinement Top Level • Determine the class average for the quiz. First Refinement • Define and initialize variables. • Get input from user and process input. • Perform computation: Calculate the class average. • Output the class average. CMSC 104, Section 301, Fall 2002 18 Lecture 18, 11/11/02
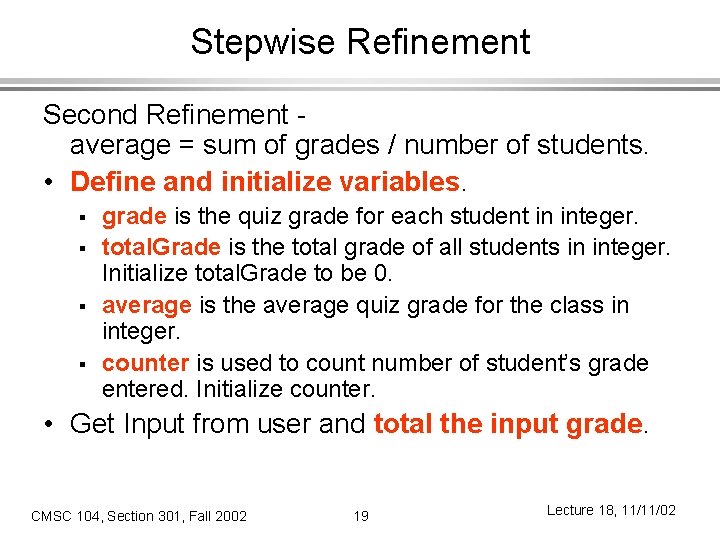
Stepwise Refinement Second Refinement average = sum of grades / number of students. • Define and initialize variables. § § grade is the quiz grade for each student in integer. total. Grade is the total grade of all students in integer. Initialize total. Grade to be 0. average is the average quiz grade for the class in integer. counter is used to count number of student’s grade entered. Initialize counter. • Get Input from user and total the input grade. CMSC 104, Section 301, Fall 2002 19 Lecture 18, 11/11/02
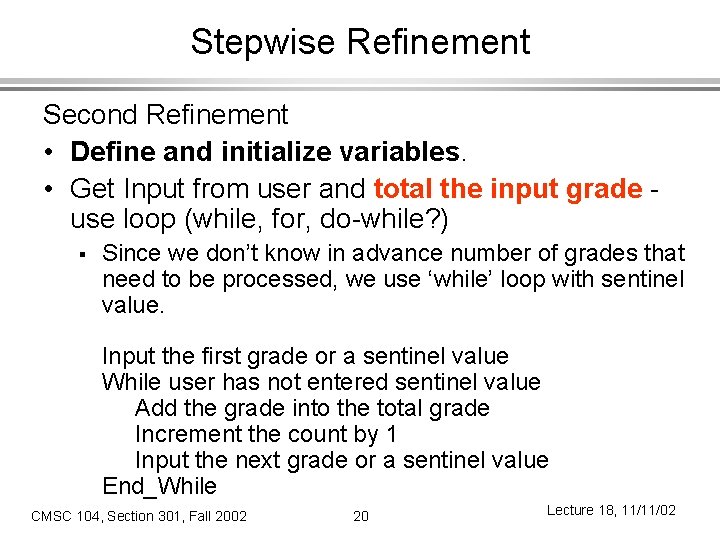
Stepwise Refinement Second Refinement • Define and initialize variables. • Get Input from user and total the input grade use loop (while, for, do-while? ) § Since we don’t know in advance number of grades that need to be processed, we use ‘while’ loop with sentinel value. Input the first grade or a sentinel value While user has not entered sentinel value Add the grade into the total grade Increment the count by 1 Input the next grade or a sentinel value End_While CMSC 104, Section 301, Fall 2002 20 Lecture 18, 11/11/02
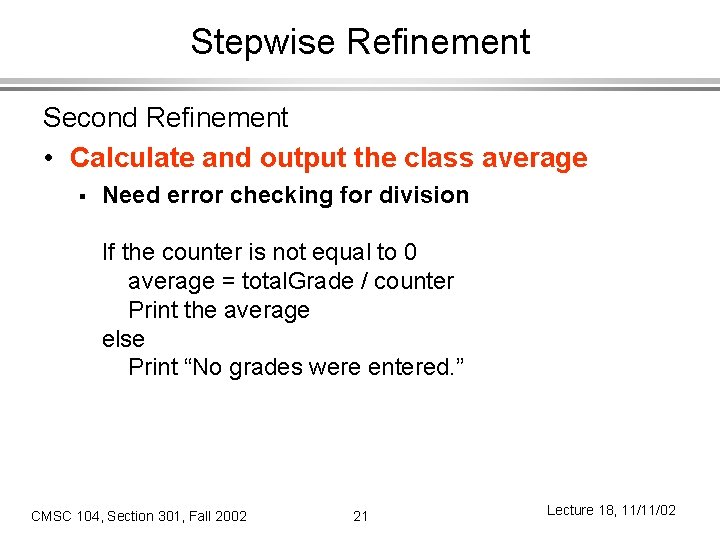
Stepwise Refinement Second Refinement • Calculate and output the class average § Need error checking for division If the counter is not equal to 0 average = total. Grade / counter Print the average else Print “No grades were entered. ” CMSC 104, Section 301, Fall 2002 21 Lecture 18, 11/11/02
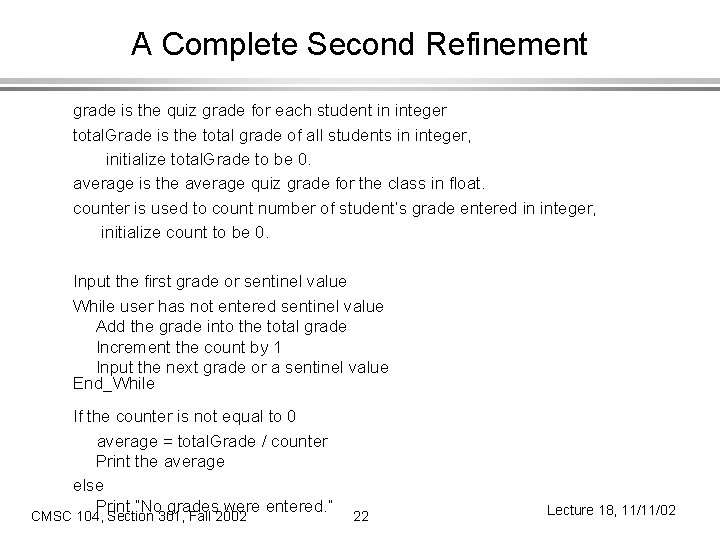
A Complete Second Refinement grade is the quiz grade for each student in integer total. Grade is the total grade of all students in integer, initialize total. Grade to be 0. average is the average quiz grade for the class in float. counter is used to count number of student’s grade entered in integer, initialize count to be 0. Input the first grade or sentinel value While user has not entered sentinel value Add the grade into the total grade Increment the count by 1 Input the next grade or a sentinel value End_While If the counter is not equal to 0 average = total. Grade / counter Print the average else Print “No grades were entered. ” CMSC 104, Section 301, Fall 2002 22 Lecture 18, 11/11/02
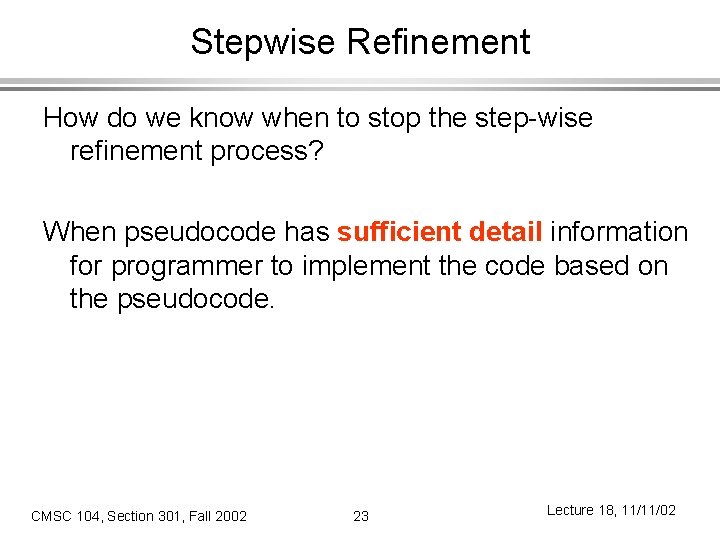
Stepwise Refinement How do we know when to stop the step-wise refinement process? When pseudocode has sufficient detail information for programmer to implement the code based on the pseudocode. CMSC 104, Section 301, Fall 2002 23 Lecture 18, 11/11/02
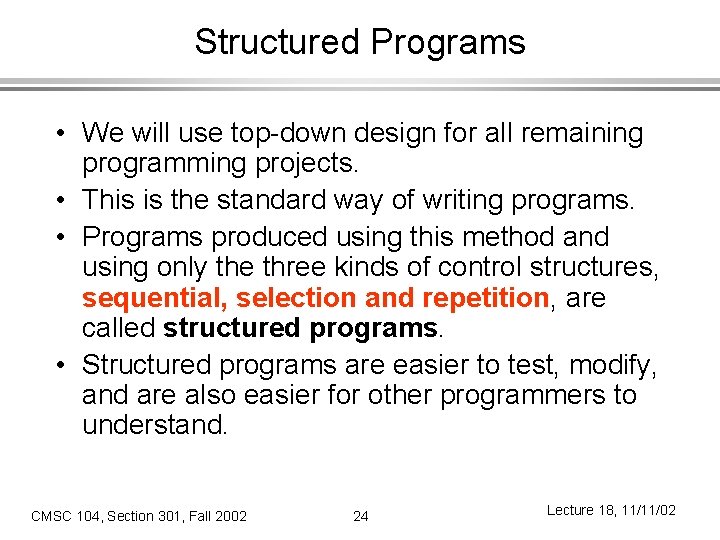
Structured Programs • We will use top-down design for all remaining programming projects. • This is the standard way of writing programs. • Programs produced using this method and using only the three kinds of control structures, sequential, selection and repetition, are called structured programs. • Structured programs are easier to test, modify, and are also easier for other programmers to understand. CMSC 104, Section 301, Fall 2002 24 Lecture 18, 11/11/02
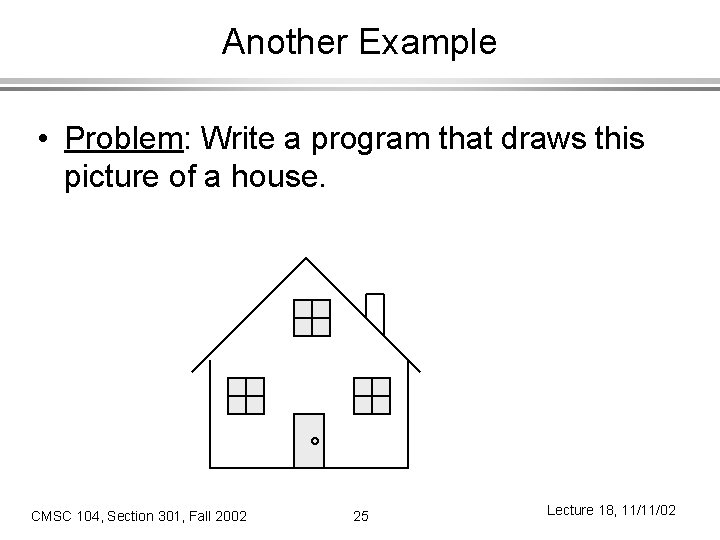
Another Example • Problem: Write a program that draws this picture of a house. CMSC 104, Section 301, Fall 2002 25 Lecture 18, 11/11/02
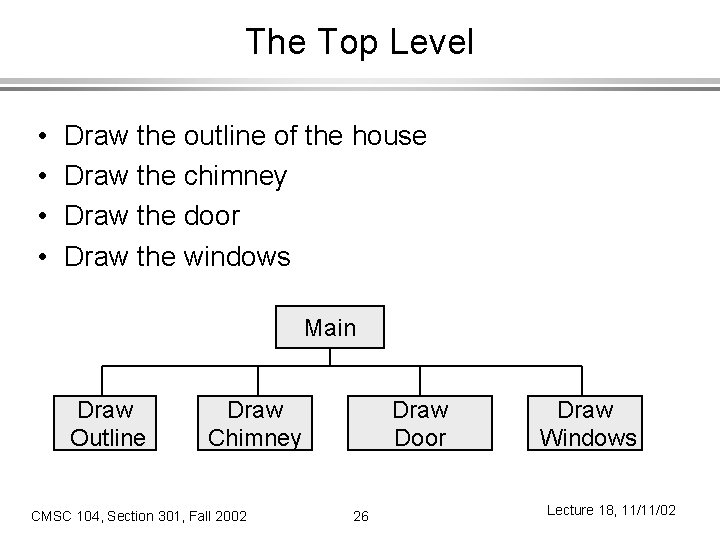
The Top Level • • Draw the outline of the house Draw the chimney Draw the door Draw the windows Main Draw Outline Draw Chimney CMSC 104, Section 301, Fall 2002 Draw Door 26 Draw Windows Lecture 18, 11/11/02
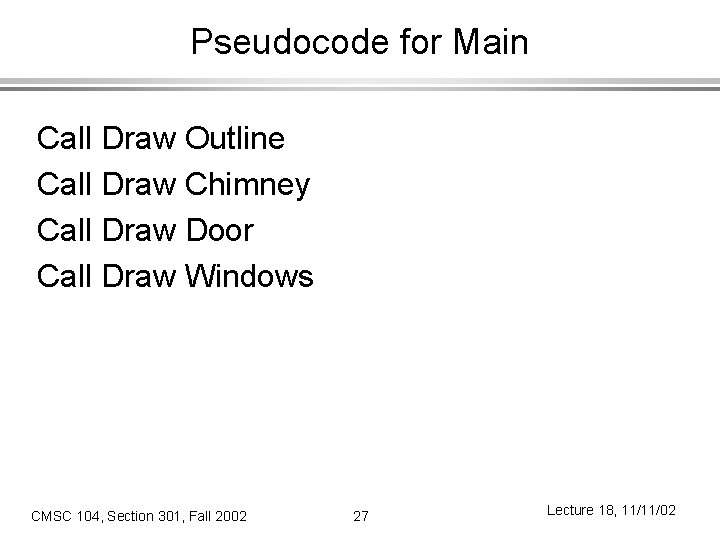
Pseudocode for Main Call Draw Outline Call Draw Chimney Call Draw Door Call Draw Windows CMSC 104, Section 301, Fall 2002 27 Lecture 18, 11/11/02
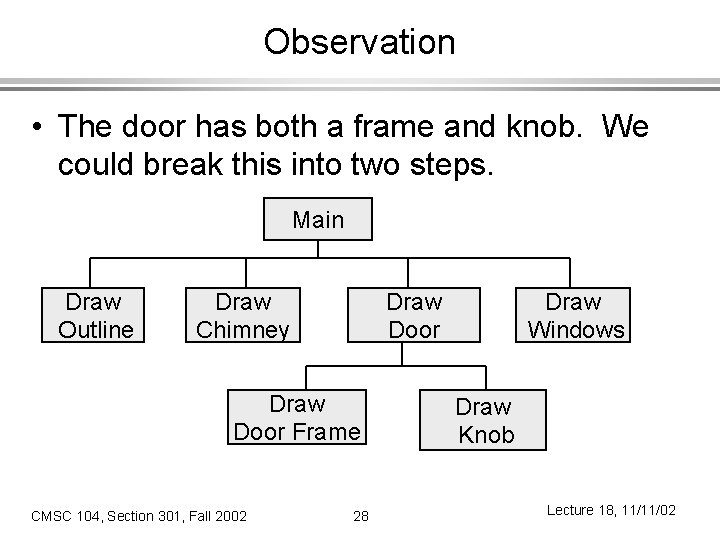
Observation • The door has both a frame and knob. We could break this into two steps. Main Draw Outline Draw Chimney Draw Door Frame CMSC 104, Section 301, Fall 2002 28 Draw Windows Draw Knob Lecture 18, 11/11/02
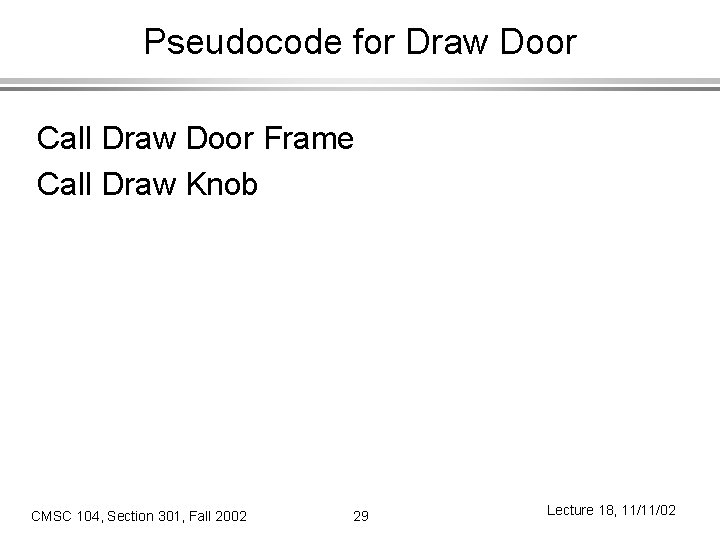
Pseudocode for Draw Door Call Draw Door Frame Call Draw Knob CMSC 104, Section 301, Fall 2002 29 Lecture 18, 11/11/02
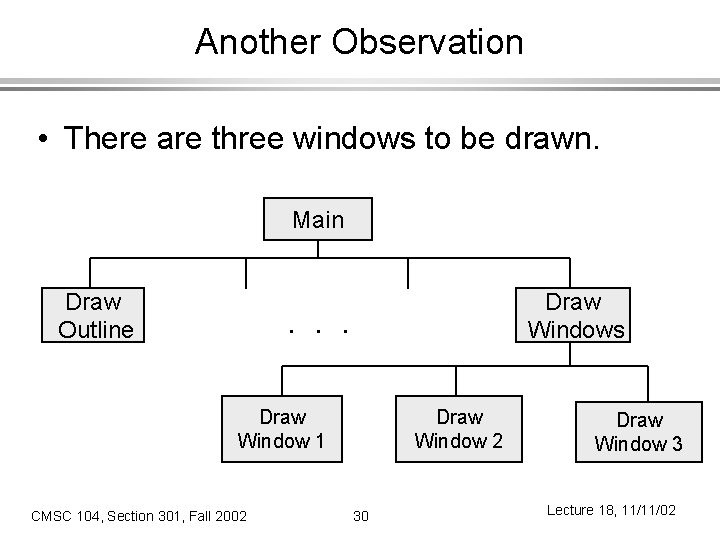
Another Observation • There are three windows to be drawn. Main Draw Outline Draw Windows . . . Draw Window 1 CMSC 104, Section 301, Fall 2002 Draw Window 2 30 Draw Window 3 Lecture 18, 11/11/02
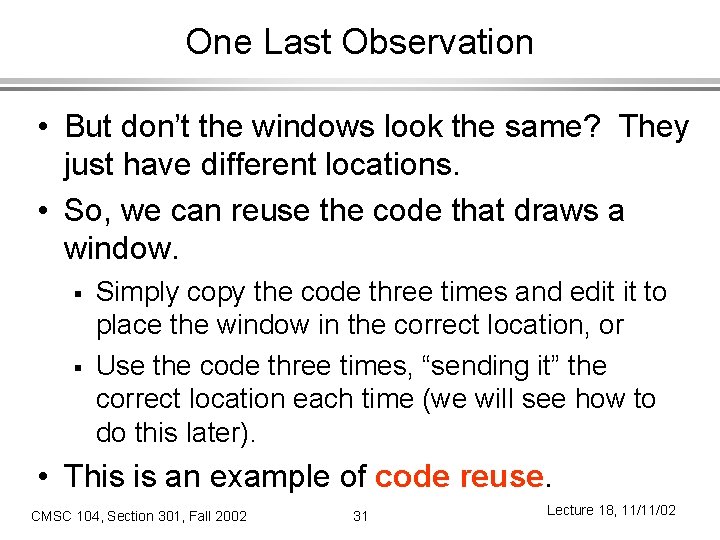
One Last Observation • But don’t the windows look the same? They just have different locations. • So, we can reuse the code that draws a window. § § Simply copy the code three times and edit it to place the window in the correct location, or Use the code three times, “sending it” the correct location each time (we will see how to do this later). • This is an example of code reuse. CMSC 104, Section 301, Fall 2002 31 Lecture 18, 11/11/02
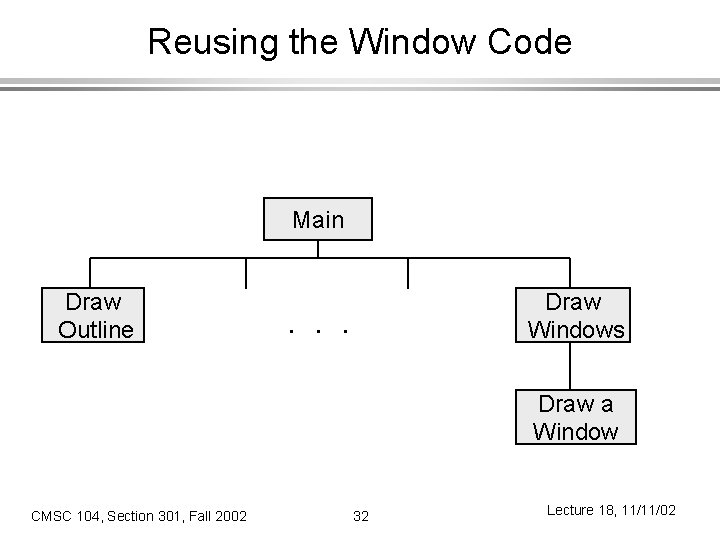
Reusing the Window Code Main Draw Outline Draw Windows . . . Draw a Window CMSC 104, Section 301, Fall 2002 32 Lecture 18, 11/11/02
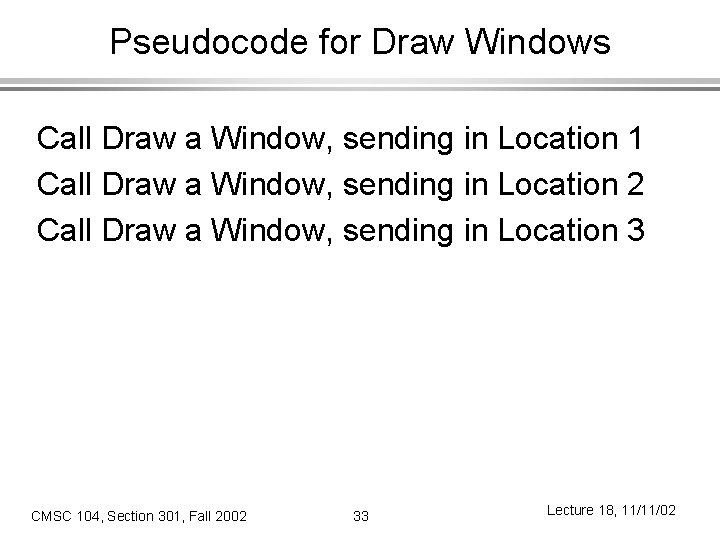
Pseudocode for Draw Windows Call Draw a Window, sending in Location 1 Call Draw a Window, sending in Location 2 Call Draw a Window, sending in Location 3 CMSC 104, Section 301, Fall 2002 33 Lecture 18, 11/11/02
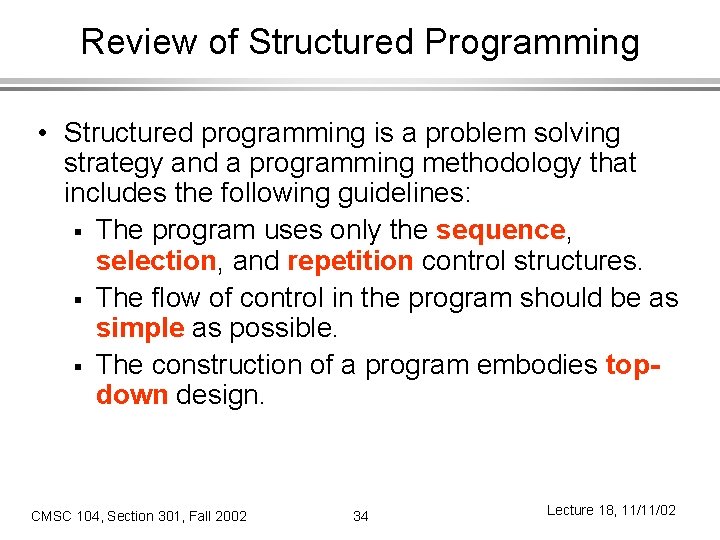
Review of Structured Programming • Structured programming is a problem solving strategy and a programming methodology that includes the following guidelines: § The program uses only the sequence, selection, and repetition control structures. § The flow of control in the program should be as simple as possible. § The construction of a program embodies topdown design. CMSC 104, Section 301, Fall 2002 34 Lecture 18, 11/11/02
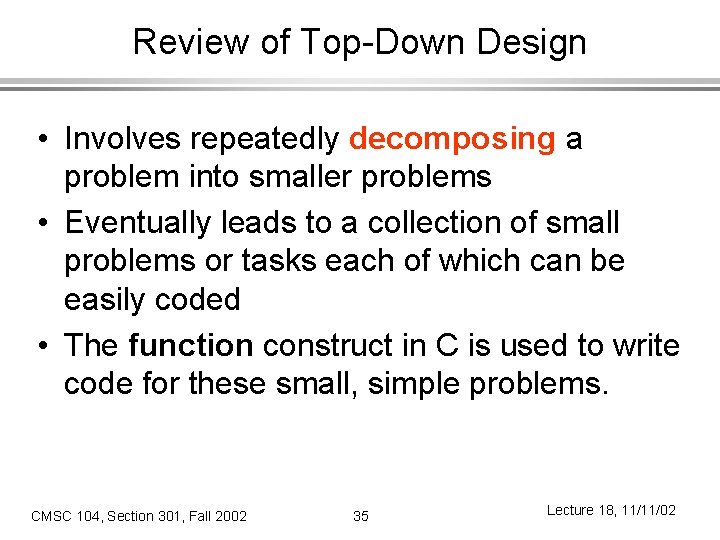
Review of Top-Down Design • Involves repeatedly decomposing a problem into smaller problems • Eventually leads to a collection of small problems or tasks each of which can be easily coded • The function construct in C is used to write code for these small, simple problems. CMSC 104, Section 301, Fall 2002 35 Lecture 18, 11/11/02
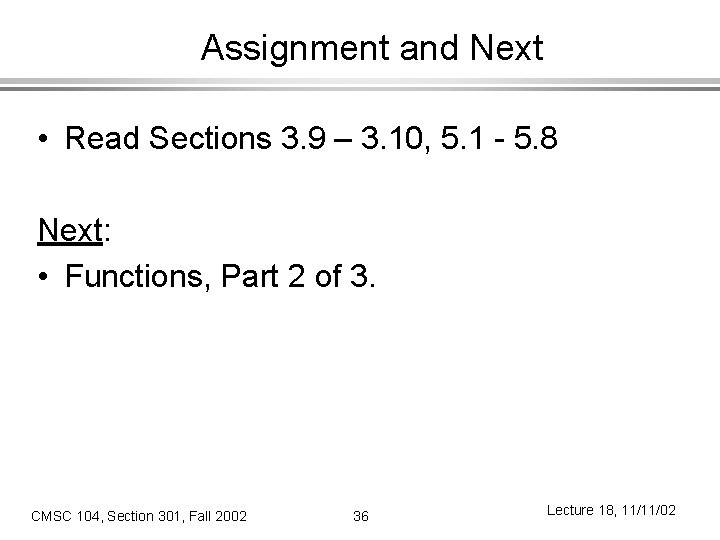
Assignment and Next • Read Sections 3. 9 – 3. 10, 5. 1 - 5. 8 Next: • Functions, Part 2 of 3. CMSC 104, Section 301, Fall 2002 36 Lecture 18, 11/11/02
Non predefined exceptions in oracle
Predefined sets of different shapes group
Predefined process flowchart meaning
Powerpoint lesson 4
Php global variable
Flowchart logic
Predefined objects in javascript
What is predefined function
Java programs perform i/o through ……….. *
An hidps can monitor system logs for predefined events.
Predefined function adalah
Make automatic variables
Addition symbol
Part to part ratio definition
Part part whole
Technical descriptions
Different parts of bar
The part of a shadow surrounding the darkest part
Two way anova minitab 17
8-7 factoring special products
8-2 characteristics of quadratic functions
Transformations of polynomial functions
8-2 quadratic functions
Graphing radical functions quiz part 2
Parts of a flowering plant and their functions
What is the function of the male part of the flower
Using system.collections.generic
Defrost using internal heat is accomplished using
Transformations of quadratic functions
Using functions in models and decision making
Reporting aggregated data using the group functions
Using transformations to graph quadratic functions
Transformation of quadratic functions
Proving inverse functions using composition
Evaluating composite functions using tables
Reporting aggregated data using the group functions
Reporting aggregated data using the group functions