Predefined Functions Revisited l l l l l
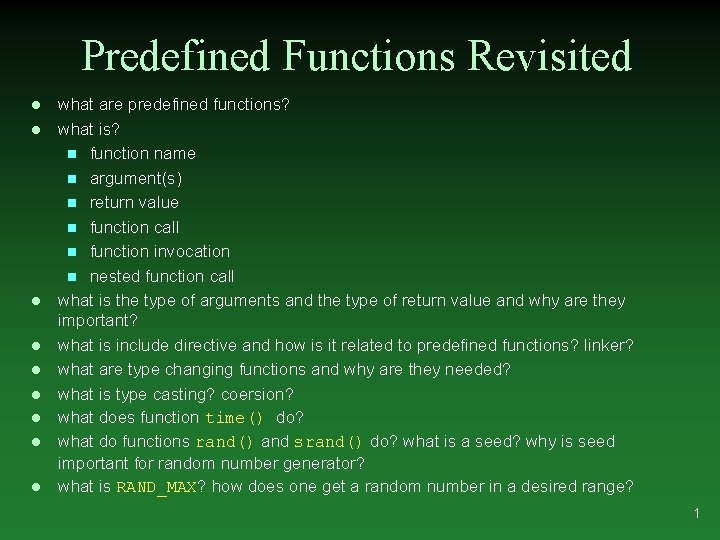
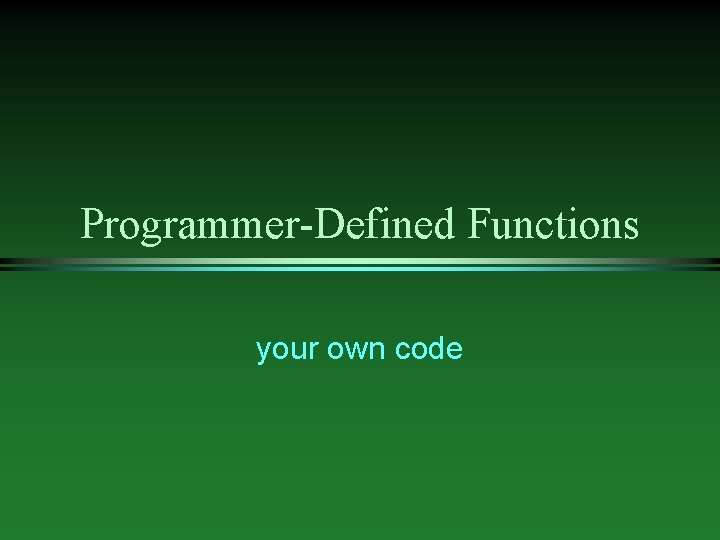
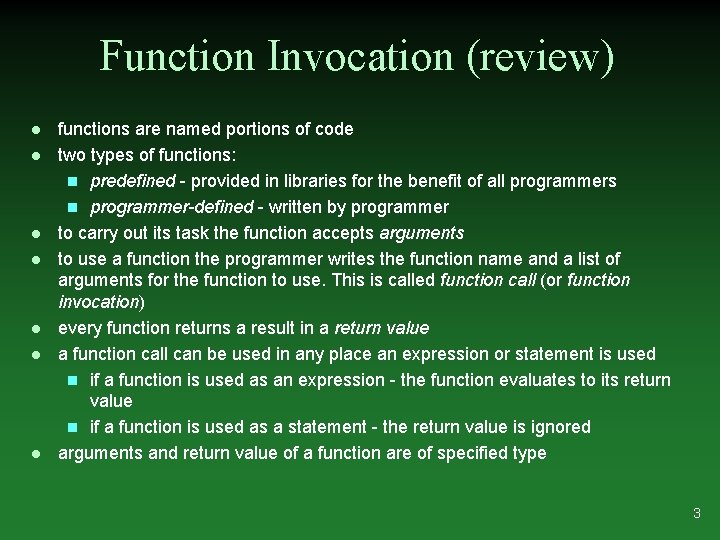
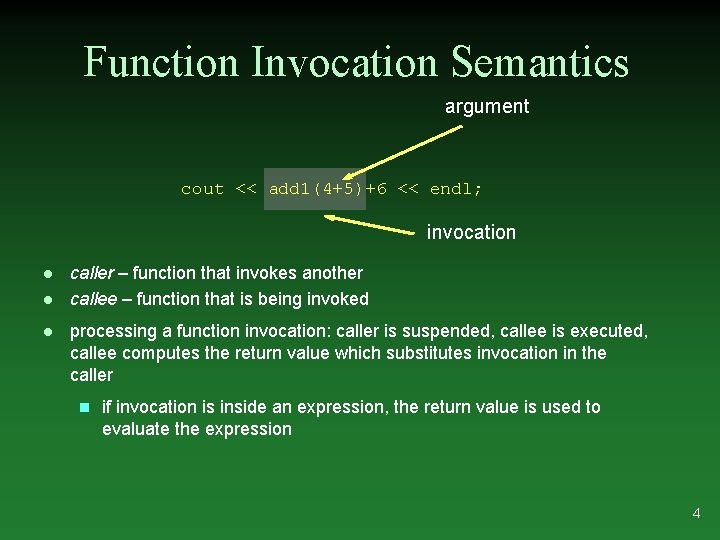
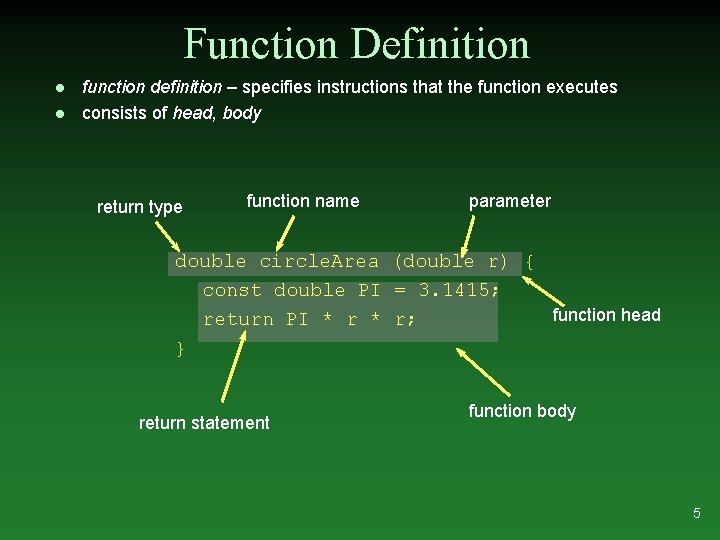
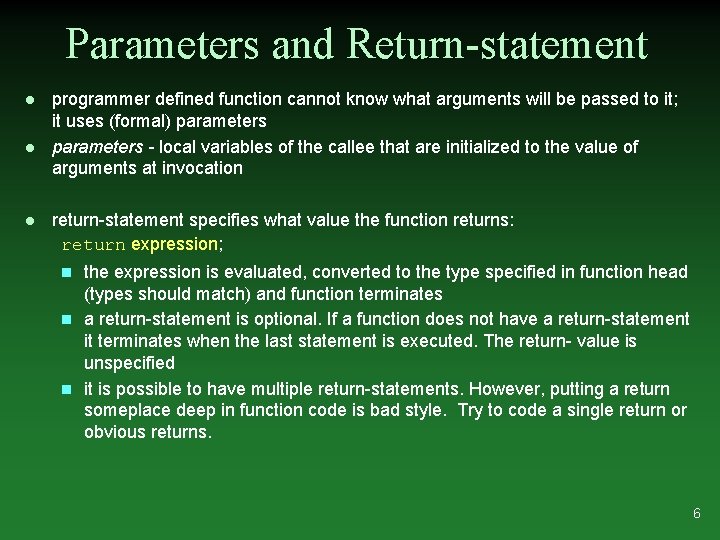
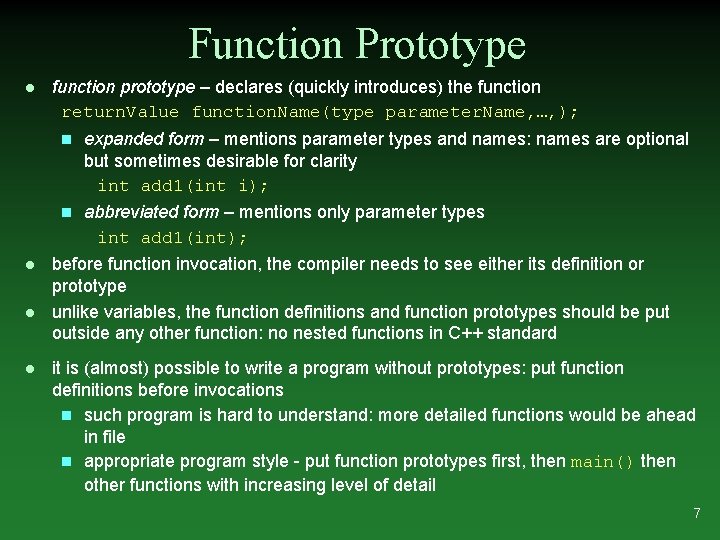
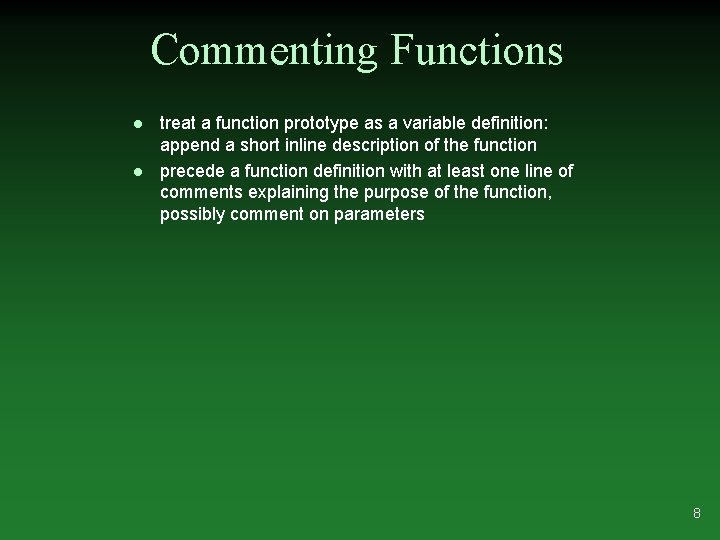
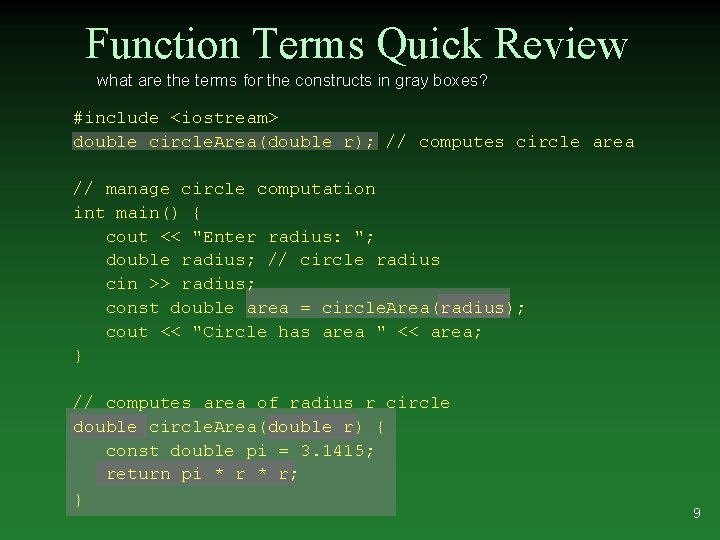
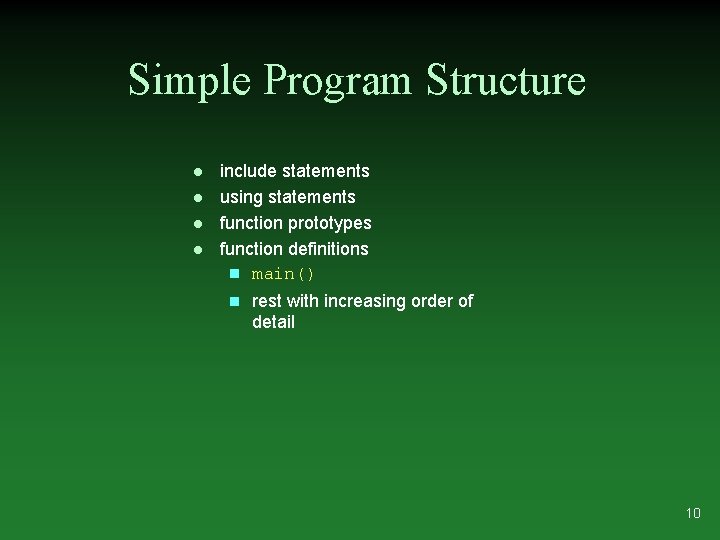
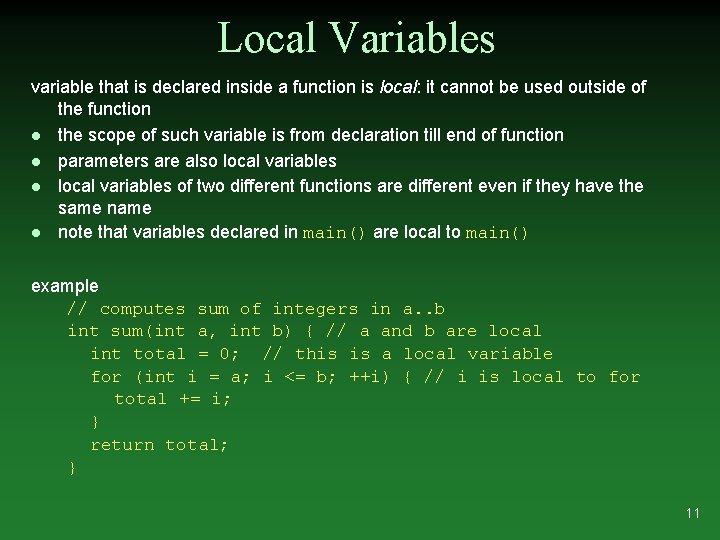
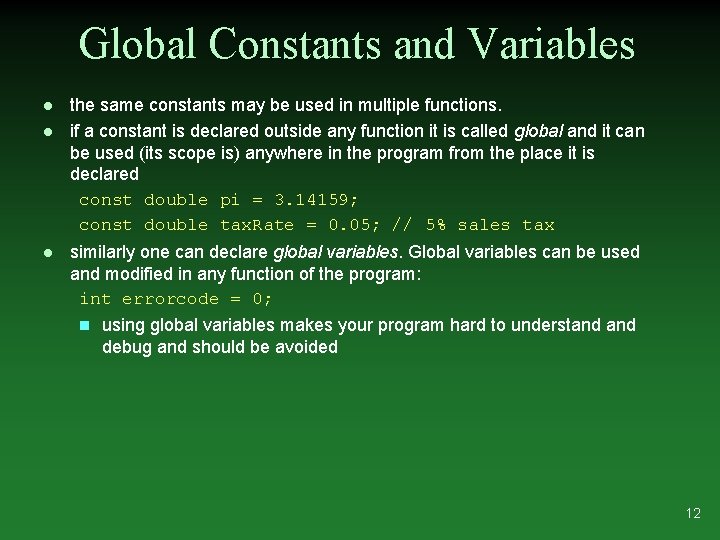
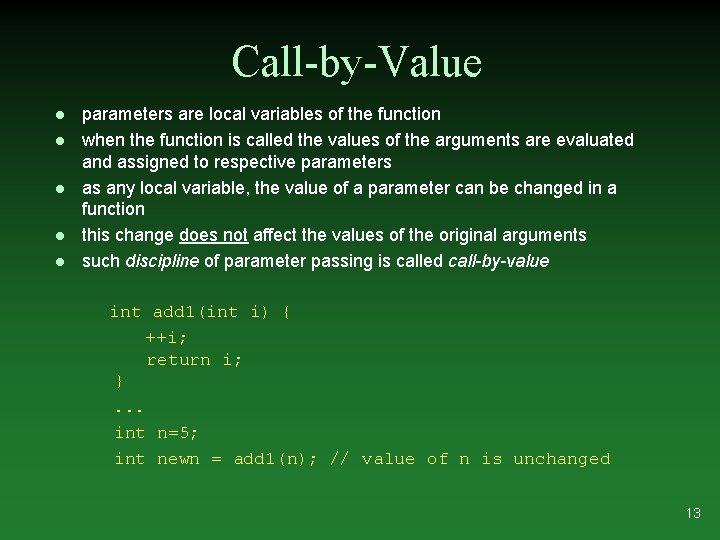
- Slides: 13
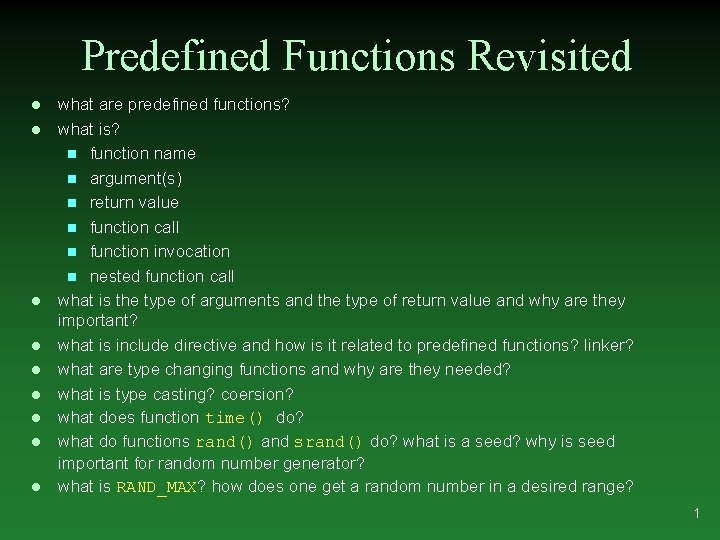
Predefined Functions Revisited l l l l l what are predefined functions? what is? n function name n argument(s) n return value n function call n function invocation n nested function call what is the type of arguments and the type of return value and why are they important? what is include directive and how is it related to predefined functions? linker? what are type changing functions and why are they needed? what is type casting? coersion? what does function time() do? what do functions rand() and srand() do? what is a seed? why is seed important for random number generator? what is RAND_MAX? how does one get a random number in a desired range? 1
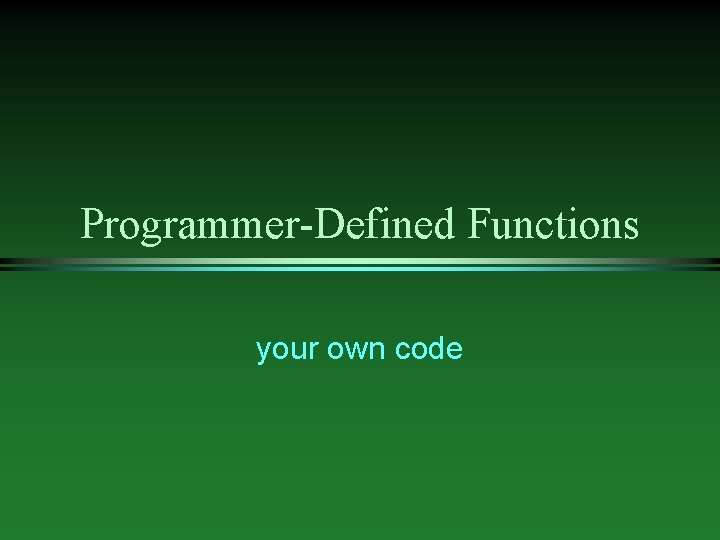
Programmer-Defined Functions your own code
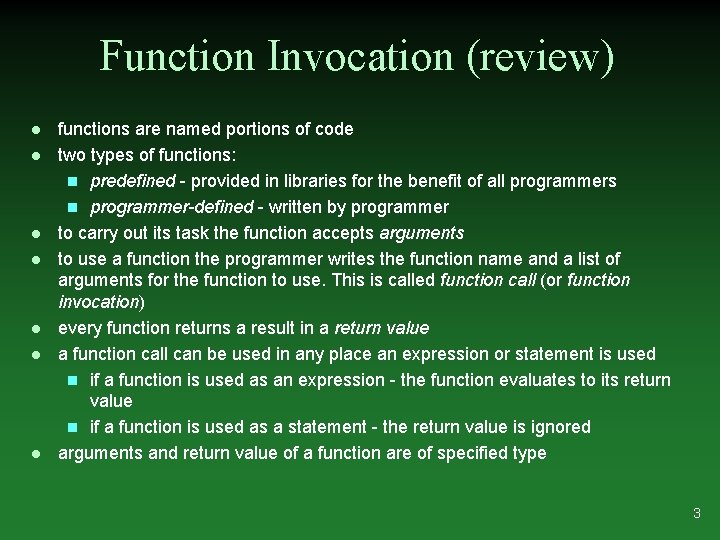
Function Invocation (review) l l l l functions are named portions of code two types of functions: n predefined - provided in libraries for the benefit of all programmers n programmer-defined - written by programmer to carry out its task the function accepts arguments to use a function the programmer writes the function name and a list of arguments for the function to use. This is called function call (or function invocation) every function returns a result in a return value a function call can be used in any place an expression or statement is used n if a function is used as an expression - the function evaluates to its return value n if a function is used as a statement - the return value is ignored arguments and return value of a function are of specified type 3
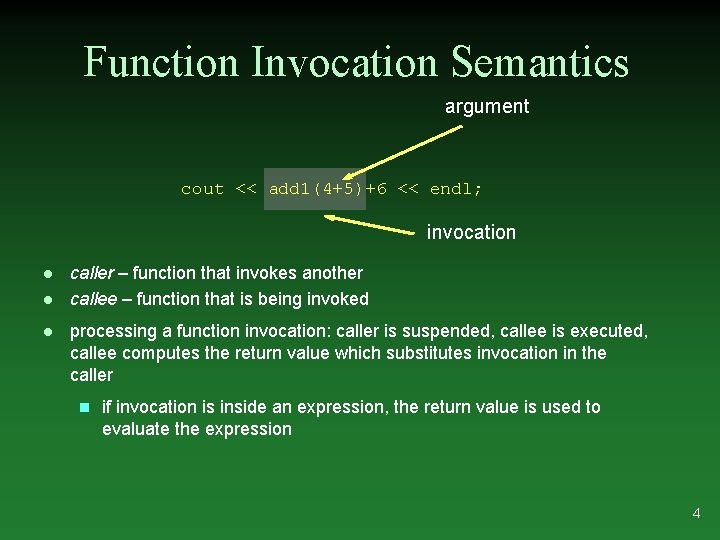
Function Invocation Semantics argument cout << add 1(4+5)+6 << endl; invocation l l l caller – function that invokes another callee – function that is being invoked processing a function invocation: caller is suspended, callee is executed, callee computes the return value which substitutes invocation in the caller n if invocation is inside an expression, the return value is used to evaluate the expression 4
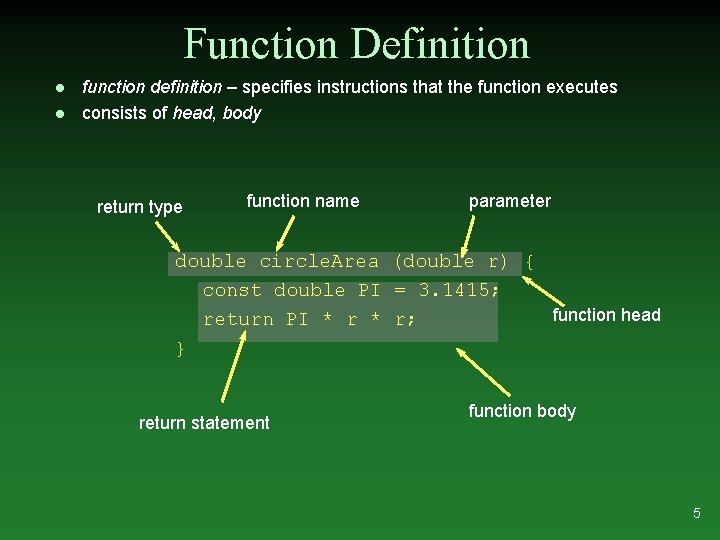
Function Definition l l function definition – specifies instructions that the function executes consists of head, body return type function name parameter double circle. Area (double r) { const double PI = 3. 1415; function head return PI * r; } return statement function body 5
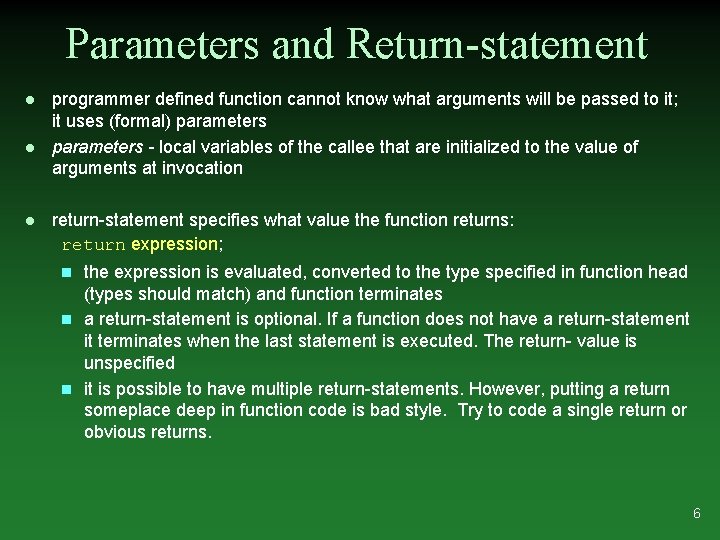
Parameters and Return-statement l l l programmer defined function cannot know what arguments will be passed to it; it uses (formal) parameters - local variables of the callee that are initialized to the value of arguments at invocation return-statement specifies what value the function returns: return expression; the expression is evaluated, converted to the type specified in function head (types should match) and function terminates n a return-statement is optional. If a function does not have a return-statement it terminates when the last statement is executed. The return- value is unspecified n it is possible to have multiple return-statements. However, putting a return someplace deep in function code is bad style. Try to code a single return or obvious returns. n 6
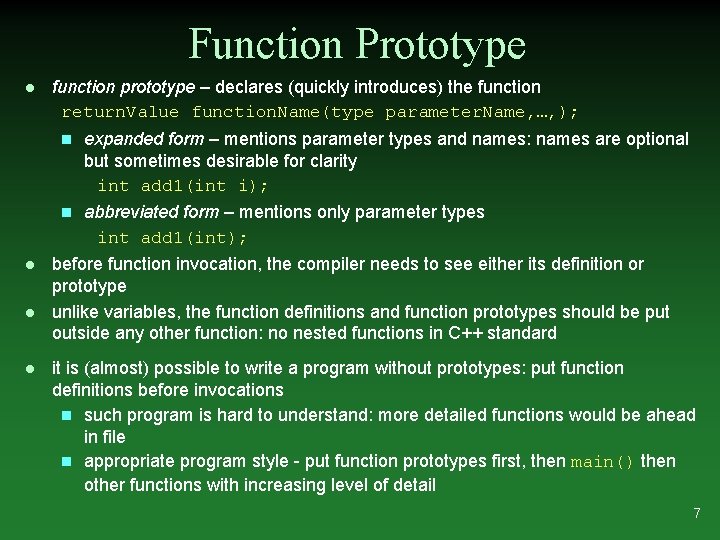
Function Prototype l l function prototype – declares (quickly introduces) the function return. Value function. Name(type parameter. Name, …, ); n expanded form – mentions parameter types and names: names are optional but sometimes desirable for clarity int add 1(int i); n abbreviated form – mentions only parameter types int add 1(int); before function invocation, the compiler needs to see either its definition or prototype unlike variables, the function definitions and function prototypes should be put outside any other function: no nested functions in C++ standard it is (almost) possible to write a program without prototypes: put function definitions before invocations n such program is hard to understand: more detailed functions would be ahead in file n appropriate program style - put function prototypes first, then main() then other functions with increasing level of detail 7
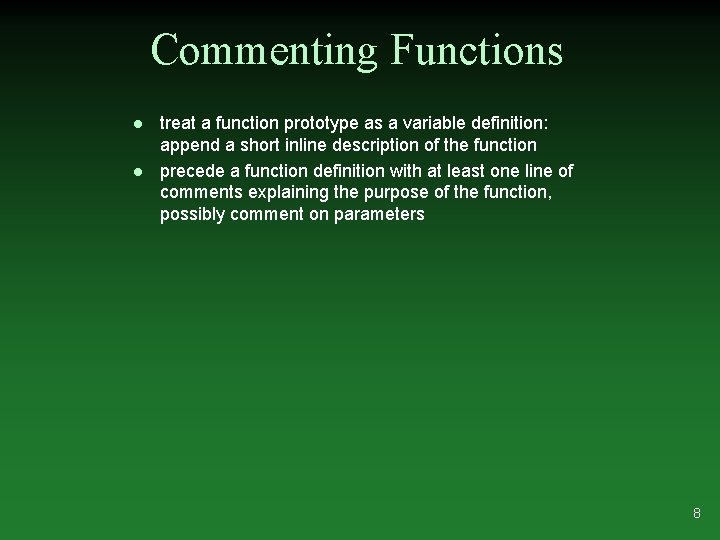
Commenting Functions l l treat a function prototype as a variable definition: append a short inline description of the function precede a function definition with at least one line of comments explaining the purpose of the function, possibly comment on parameters 8
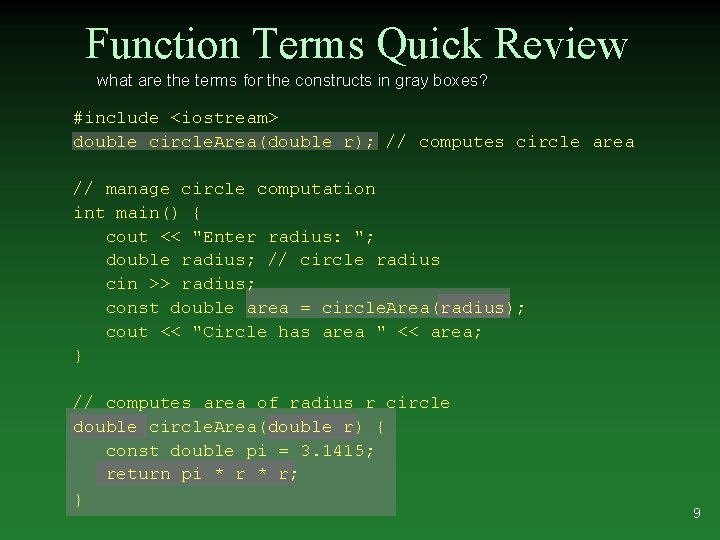
Function Terms Quick Review what are the terms for the constructs in gray boxes? #include <iostream> double circle. Area(double r); // computes circle area // manage circle computation int main() { cout << "Enter radius: "; double radius; // circle radius cin >> radius; const double area = circle. Area(radius); cout << "Circle has area " << area; } // computes area of radius r circle double circle. Area(double r) { const double pi = 3. 1415; return pi * r; } 9
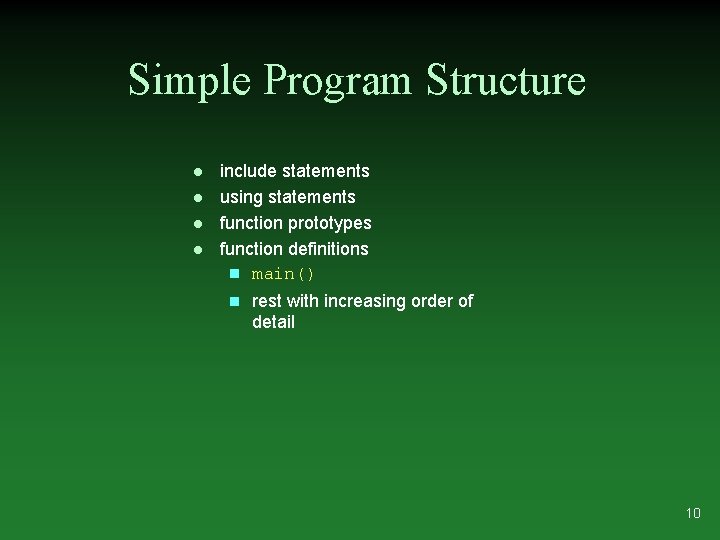
Simple Program Structure l l include statements using statements function prototypes function definitions n main() n rest with increasing order of detail 10
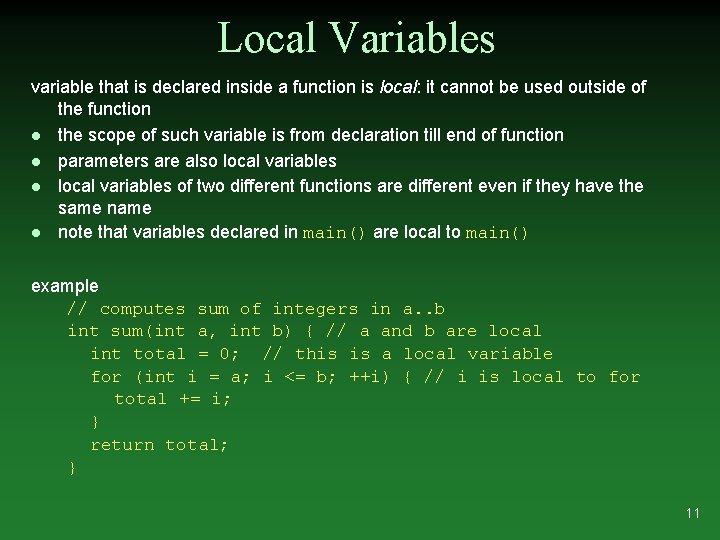
Local Variables variable that is declared inside a function is local: it cannot be used outside of the function l the scope of such variable is from declaration till end of function l parameters are also local variables l local variables of two different functions are different even if they have the same name l note that variables declared in main() are local to main() example // computes sum of integers in a. . b int sum(int a, int b) { // a and b are local int total = 0; // this is a local variable for (int i = a; i <= b; ++i) { // i is local to for total += i; } return total; } 11
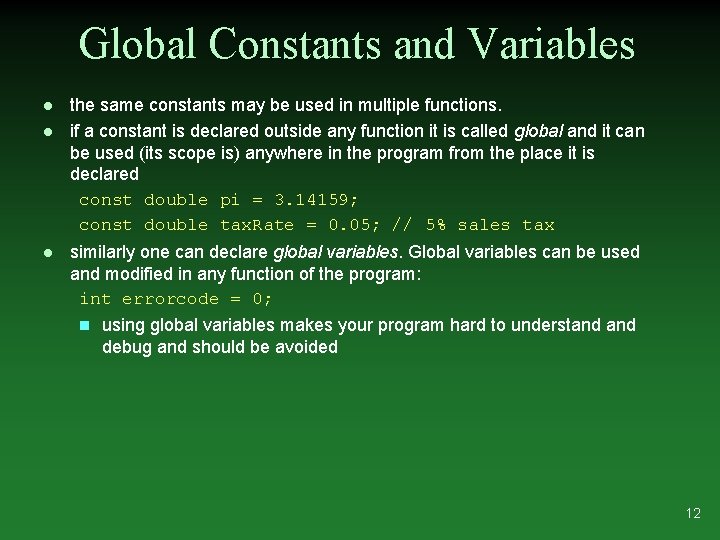
Global Constants and Variables l l l the same constants may be used in multiple functions. if a constant is declared outside any function it is called global and it can be used (its scope is) anywhere in the program from the place it is declared const double pi = 3. 14159; const double tax. Rate = 0. 05; // 5% sales tax similarly one can declare global variables. Global variables can be used and modified in any function of the program: int errorcode = 0; n using global variables makes your program hard to understand debug and should be avoided 12
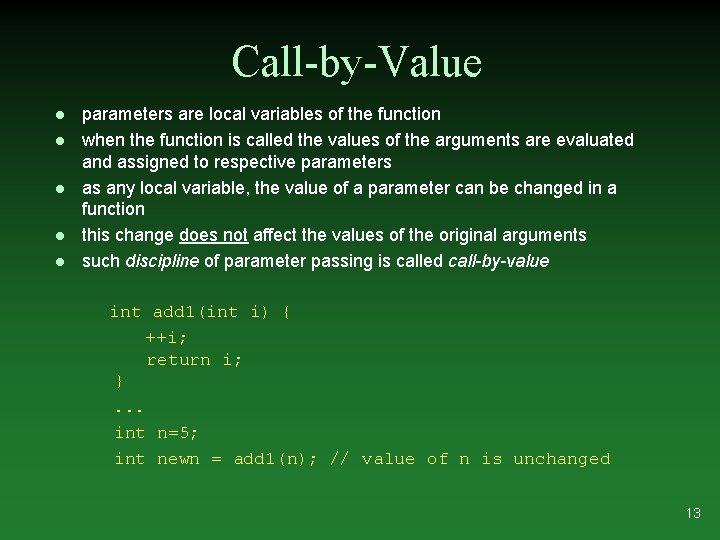
Call-by-Value l l l parameters are local variables of the function when the function is called the values of the arguments are evaluated and assigned to respective parameters as any local variable, the value of a parameter can be changed in a function this change does not affect the values of the original arguments such discipline of parameter passing is called call-by-value int add 1(int i) { ++i; return i; }. . . int n=5; int newn = add 1(n); // value of n is unchanged 13
Predefined and non predefined exceptions are raised
Straight line motion revisited homework
Zhuoyue zhao
Predefined process flowchart meaning
Dom predefined objects
Predefined function adalah
A predefined arrangement of placeholders
What is predefined function
Gnu make predefined variables
Php global variable
Predefined streams in java
It creates a blank form document that simulates an envelope
Flowchart logic
Hidps