Functions 2 Dr Khizar Hayat Associate Prof of
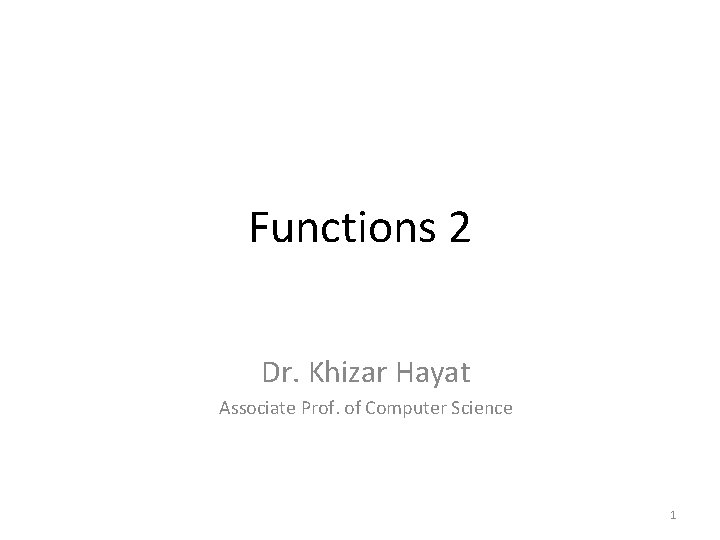
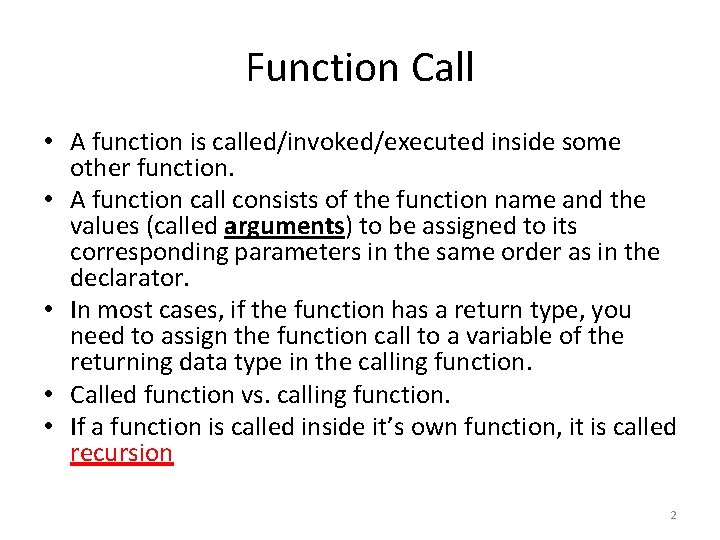
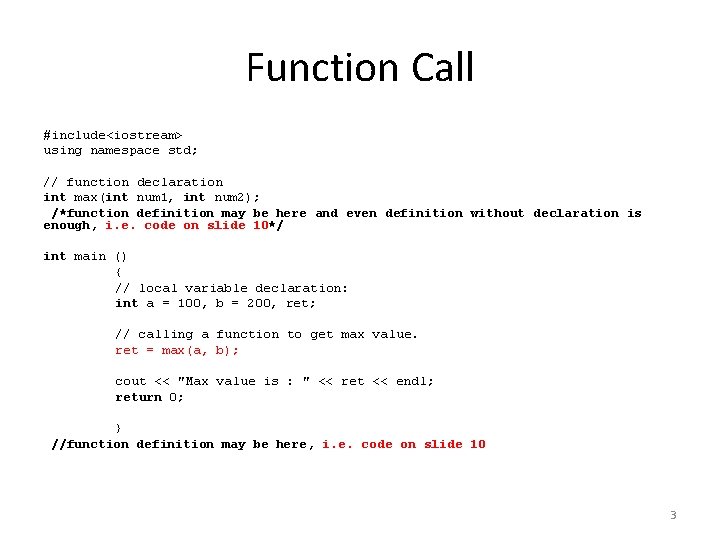
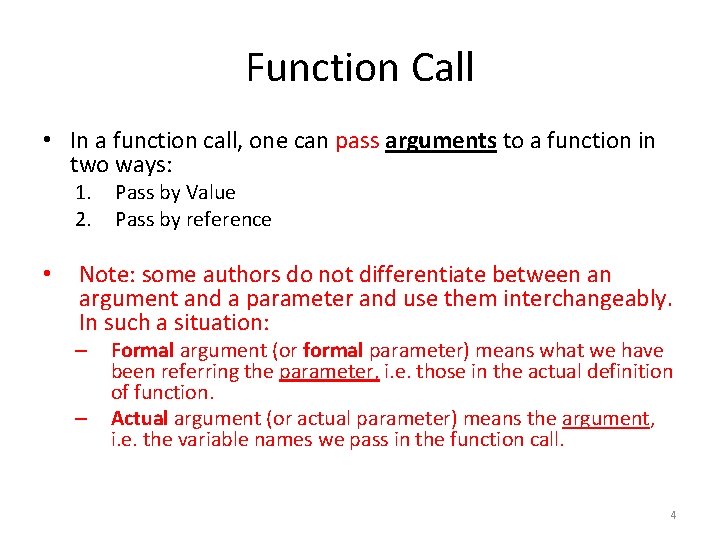
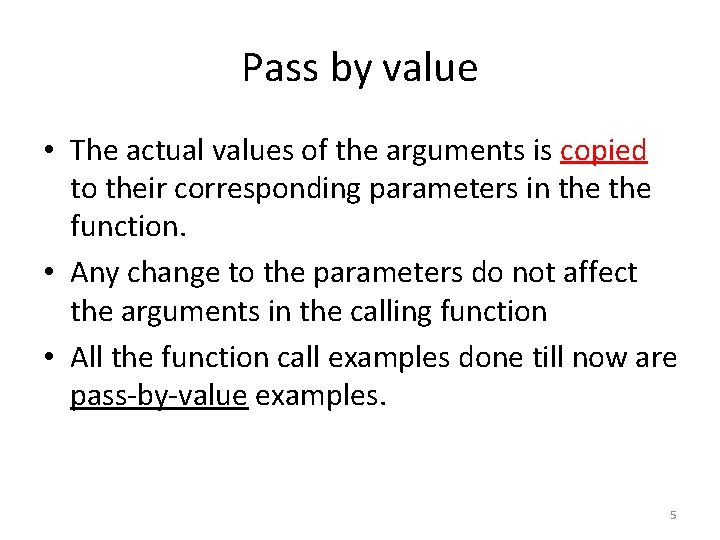
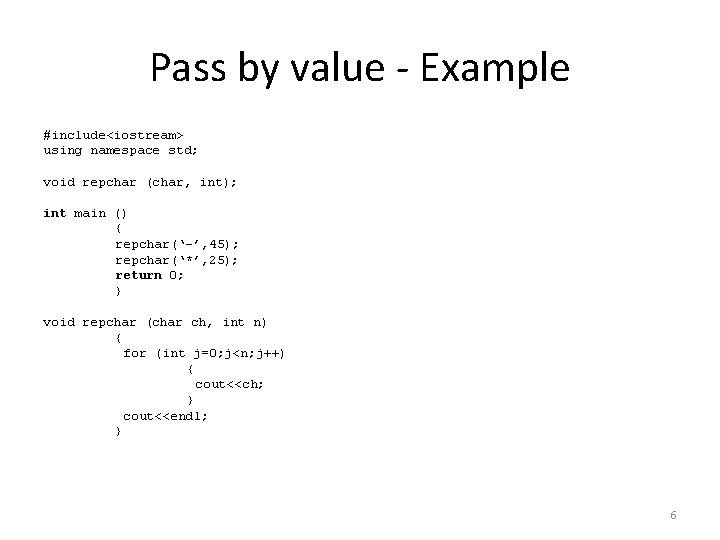
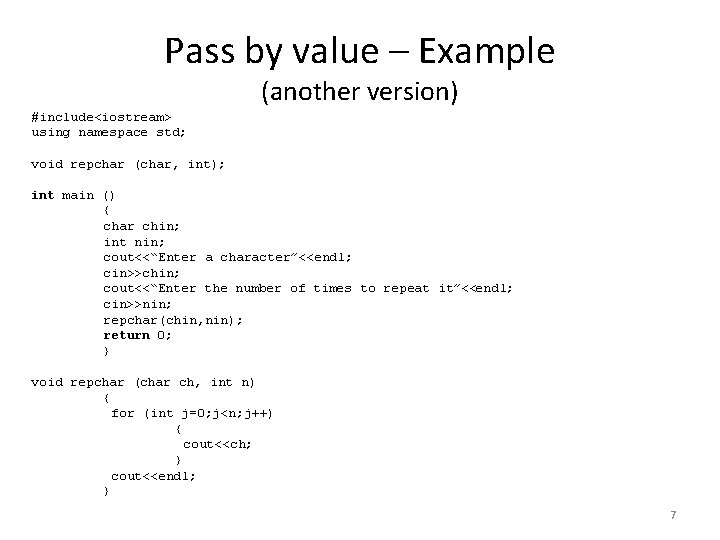
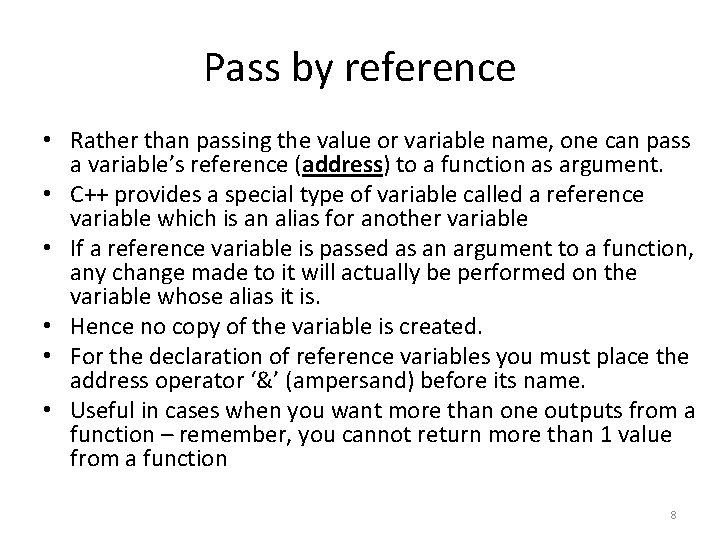
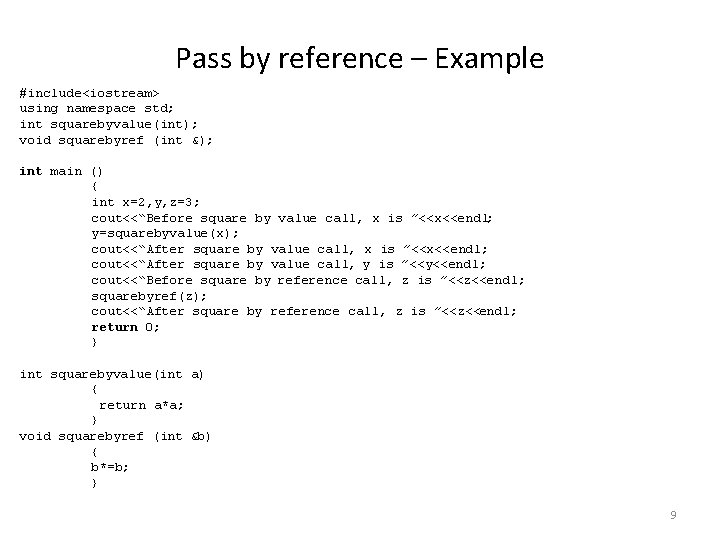
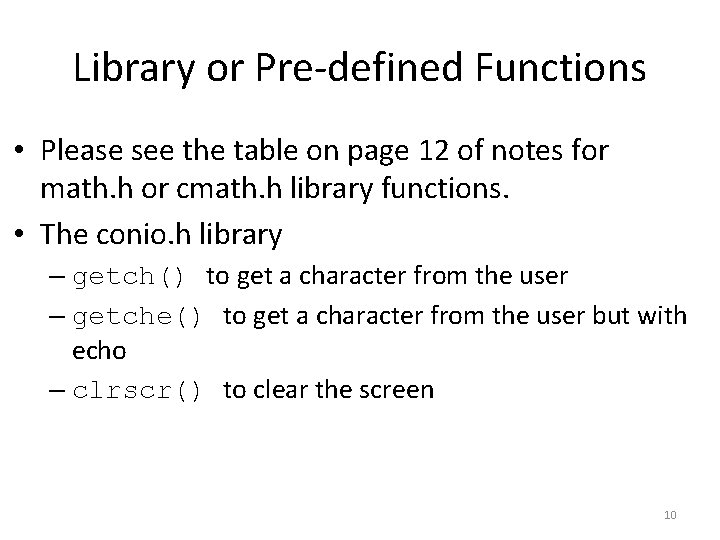
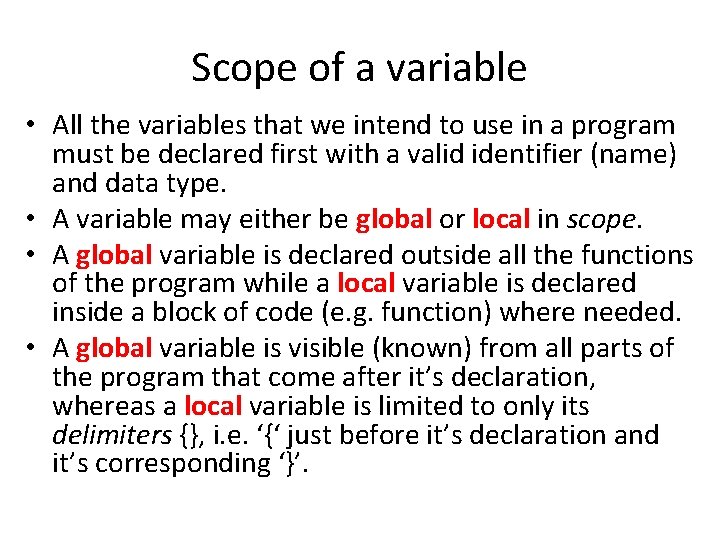
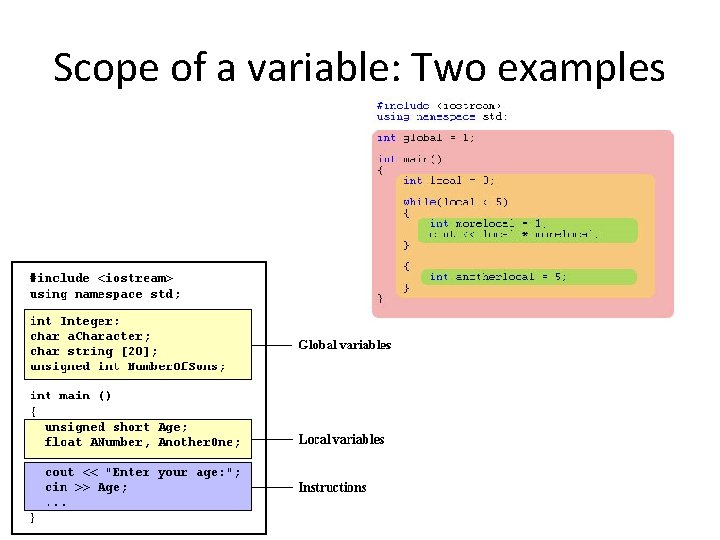
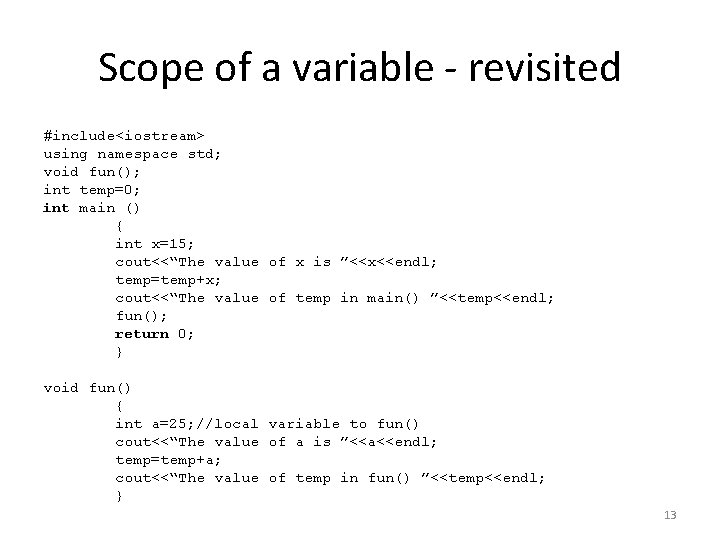
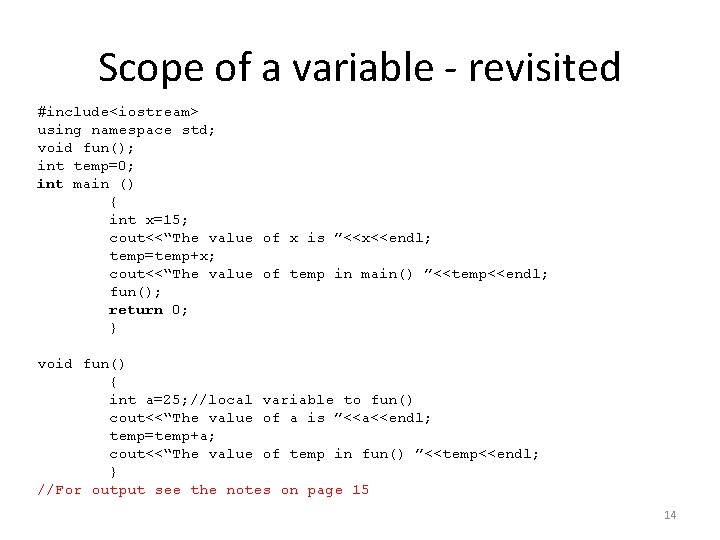
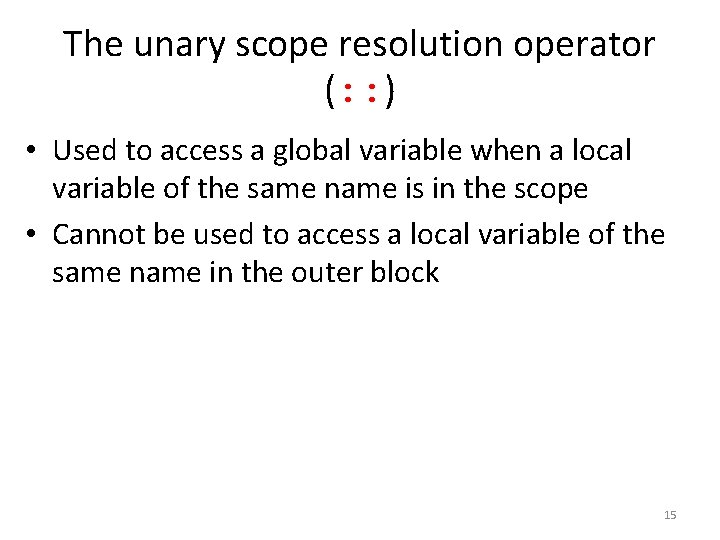
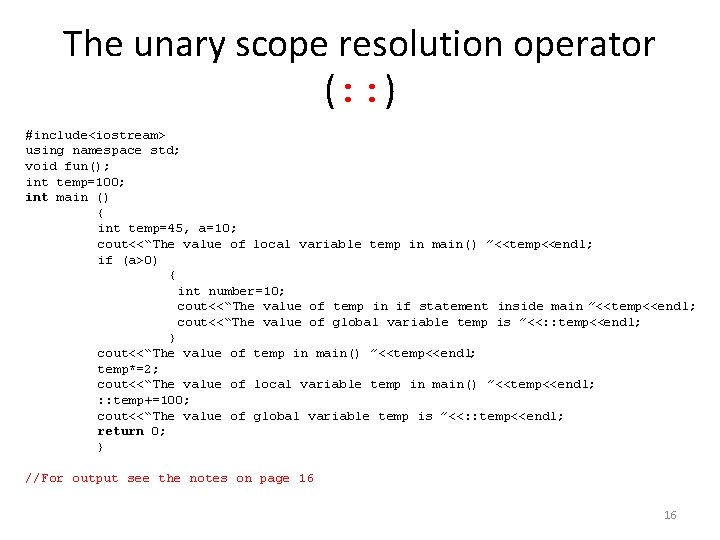
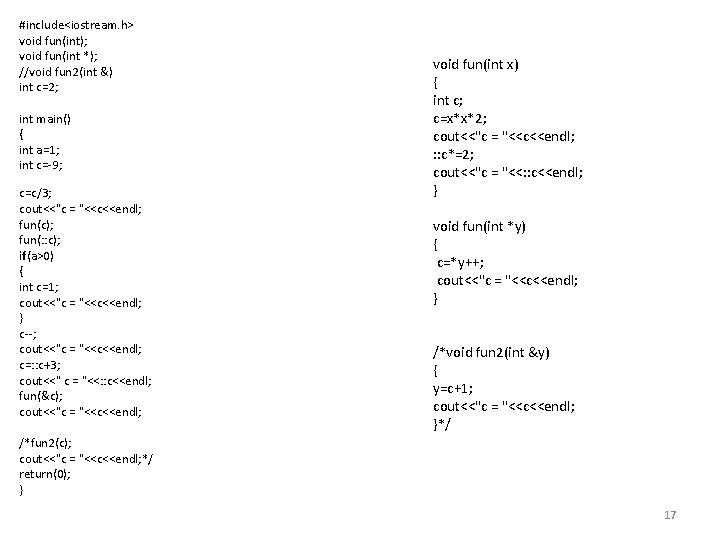
- Slides: 17
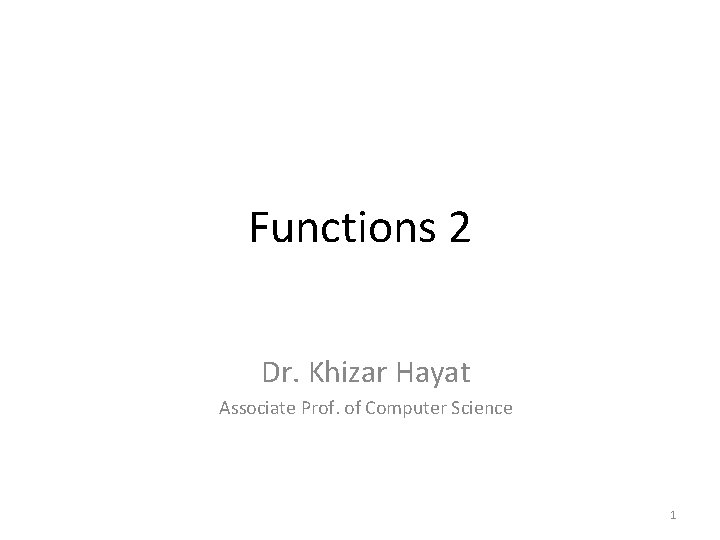
Functions 2 Dr. Khizar Hayat Associate Prof. of Computer Science 1
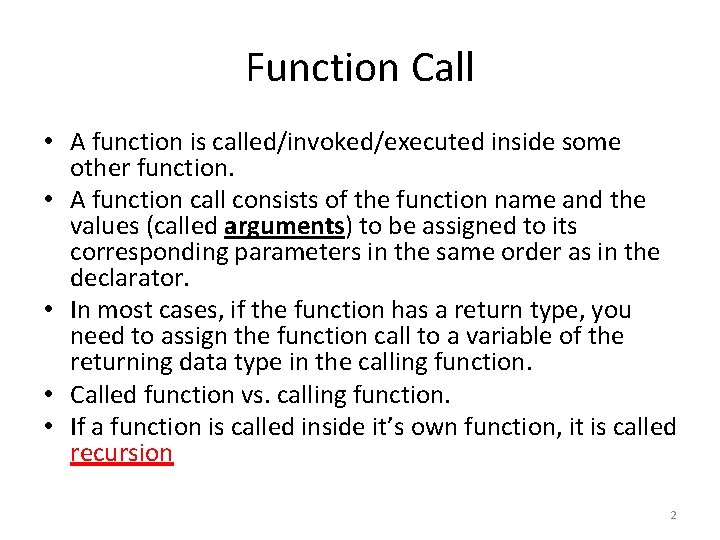
Function Call • A function is called/invoked/executed inside some other function. • A function call consists of the function name and the values (called arguments) to be assigned to its corresponding parameters in the same order as in the declarator. • In most cases, if the function has a return type, you need to assign the function call to a variable of the returning data type in the calling function. • Called function vs. calling function. • If a function is called inside it’s own function, it is called recursion 2
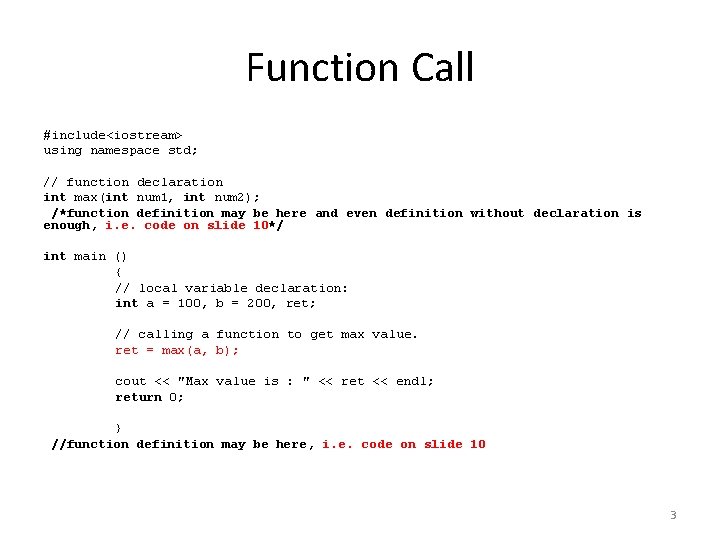
Function Call #include<iostream> using namespace std; // function declaration int max(int num 1, int num 2); /*function definition may be here and even definition without declaration is enough, i. e. code on slide 10*/ int main () { // local variable declaration: int a = 100, b = 200, ret; // calling a function to get max value. ret = max(a, b); cout << "Max value is : " << ret << endl; return 0; } //function definition may be here, i. e. code on slide 10 3
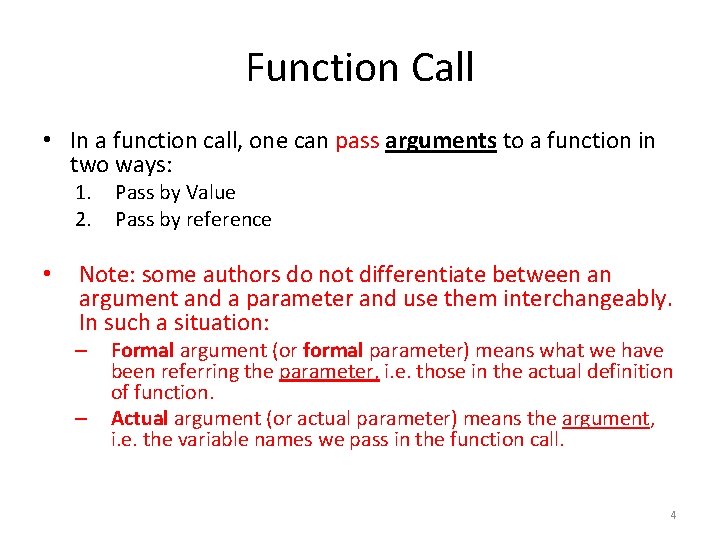
Function Call • In a function call, one can pass arguments to a function in two ways: 1. 2. • Pass by Value Pass by reference Note: some authors do not differentiate between an argument and a parameter and use them interchangeably. In such a situation: – – Formal argument (or formal parameter) means what we have been referring the parameter, i. e. those in the actual definition of function. Actual argument (or actual parameter) means the argument, i. e. the variable names we pass in the function call. 4
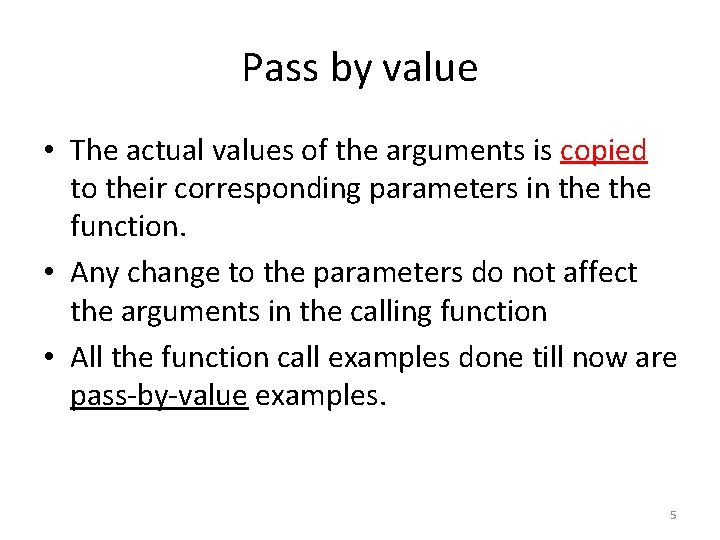
Pass by value • The actual values of the arguments is copied to their corresponding parameters in the function. • Any change to the parameters do not affect the arguments in the calling function • All the function call examples done till now are pass-by-value examples. 5
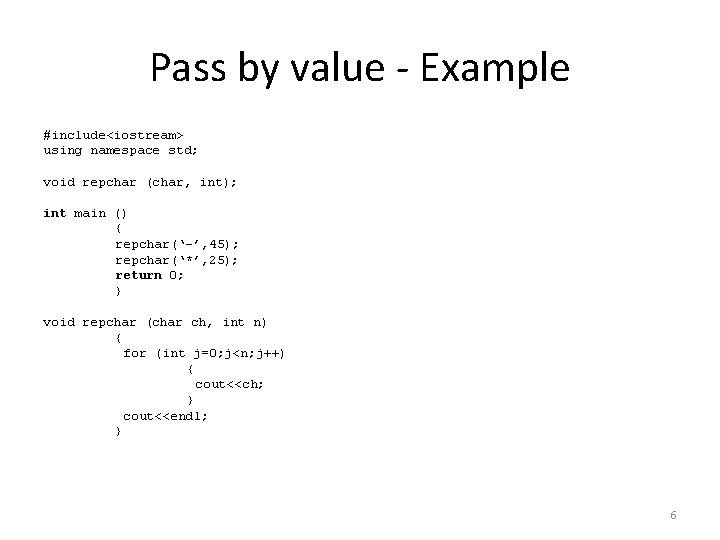
Pass by value - Example #include<iostream> using namespace std; void repchar (char, int); int main () { repchar(‘-’, 45); repchar(‘*’, 25); return 0; } void repchar (char ch, int n) { for (int j=0; j<n; j++) { cout<<ch; } cout<<endl; } 6
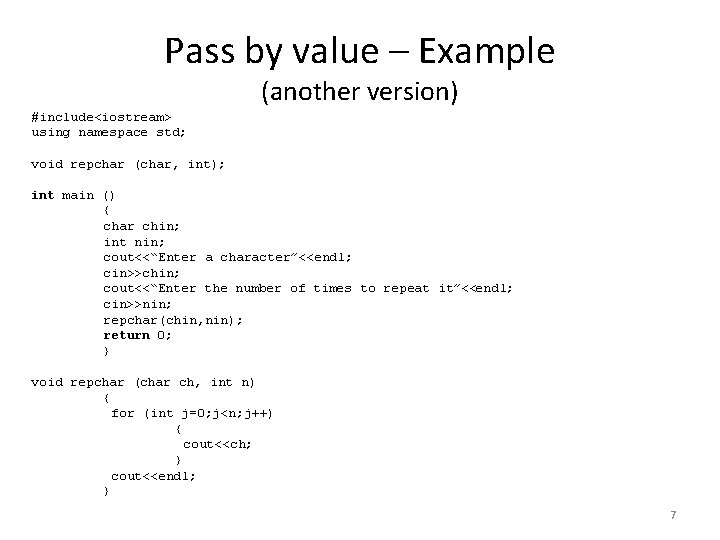
Pass by value – Example (another version) #include<iostream> using namespace std; void repchar (char, int); int main () { char chin; int nin; cout<<“Enter a character”<<endl; cin>>chin; cout<<“Enter the number of times to repeat it”<<endl; cin>>nin; repchar(chin, nin); return 0; } void repchar (char ch, int n) { for (int j=0; j<n; j++) { cout<<ch; } cout<<endl; } 7
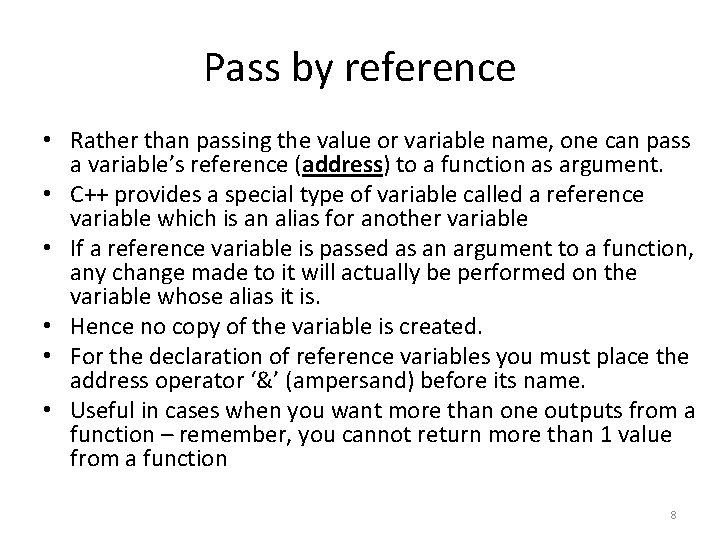
Pass by reference • Rather than passing the value or variable name, one can pass a variable’s reference (address) to a function as argument. • C++ provides a special type of variable called a reference variable which is an alias for another variable • If a reference variable is passed as an argument to a function, any change made to it will actually be performed on the variable whose alias it is. • Hence no copy of the variable is created. • For the declaration of reference variables you must place the address operator ‘&’ (ampersand) before its name. • Useful in cases when you want more than one outputs from a function – remember, you cannot return more than 1 value from a function 8
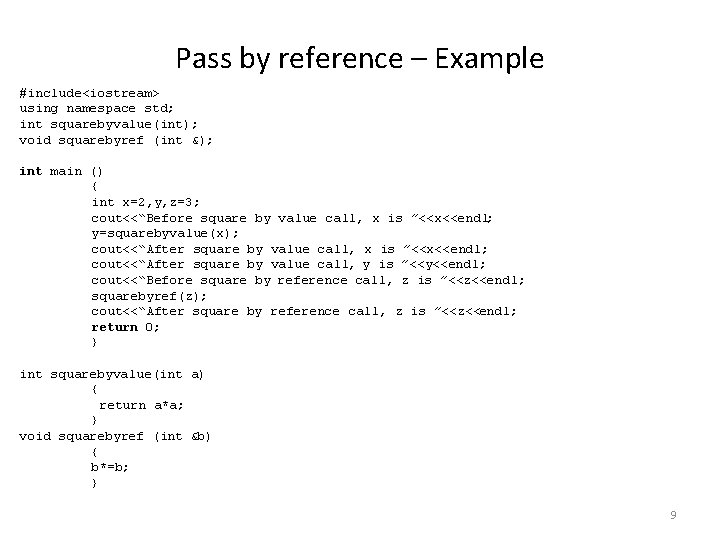
Pass by reference – Example #include<iostream> using namespace std; int squarebyvalue(int); void squarebyref (int &); int main () { int x=2, y, z=3; cout<<“Before square by value call, x is ”<<x<<endl; y=squarebyvalue(x); cout<<“After square by value call, x is ”<<x<<endl; cout<<“After square by value call, y is ”<<y<<endl; cout<<“Before square by reference call, z is ”<<z<<endl; squarebyref(z); cout<<“After square by reference call, z is ”<<z<<endl; return 0; } int squarebyvalue(int a) { return a*a; } void squarebyref (int &b) { b*=b; } 9
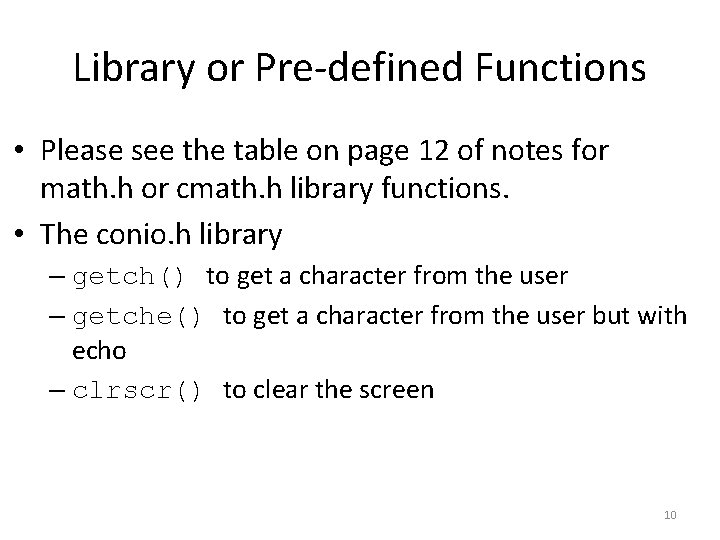
Library or Pre-defined Functions • Please see the table on page 12 of notes for math. h or cmath. h library functions. • The conio. h library – getch() to get a character from the user – getche() to get a character from the user but with echo – clrscr() to clear the screen 10
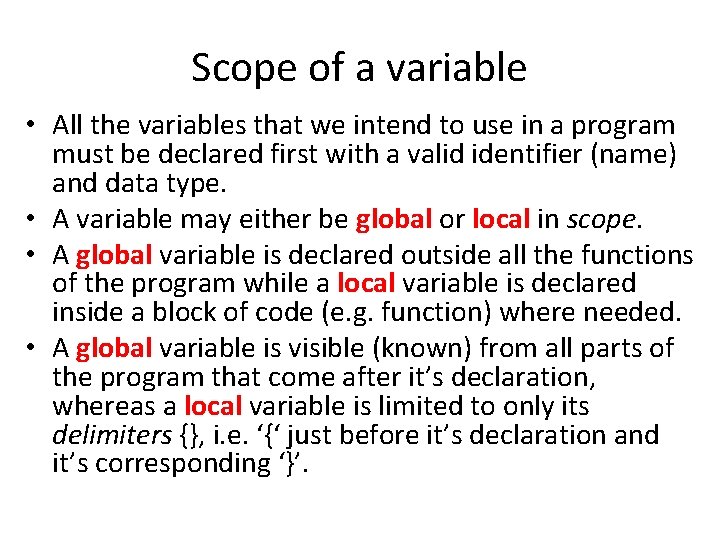
Scope of a variable • All the variables that we intend to use in a program must be declared first with a valid identifier (name) and data type. • A variable may either be global or local in scope. • A global variable is declared outside all the functions of the program while a local variable is declared inside a block of code (e. g. function) where needed. • A global variable is visible (known) from all parts of the program that come after it’s declaration, whereas a local variable is limited to only its delimiters {}, i. e. ‘{‘ just before it’s declaration and it’s corresponding ‘}’.
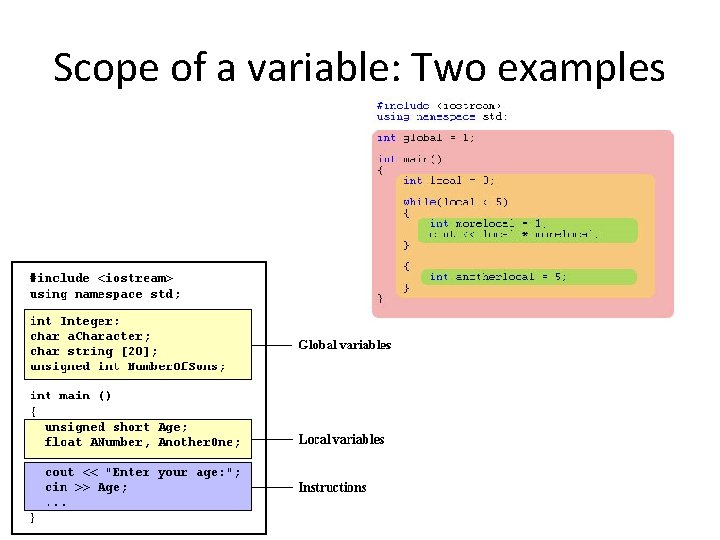
Scope of a variable: Two examples
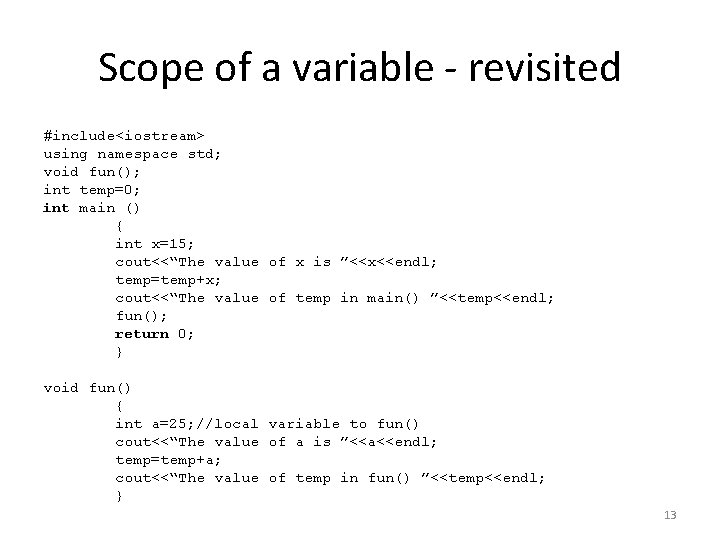
Scope of a variable - revisited #include<iostream> using namespace std; void fun(); int temp=0; int main () { int x=15; cout<<“The value of x is ”<<x<<endl; temp=temp+x; cout<<“The value of temp in main() ”<<temp<<endl; fun(); return 0; } void fun() { int a=25; //local variable to fun() cout<<“The value of a is ”<<a<<endl; temp=temp+a; cout<<“The value of temp in fun() ”<<temp<<endl; } 13
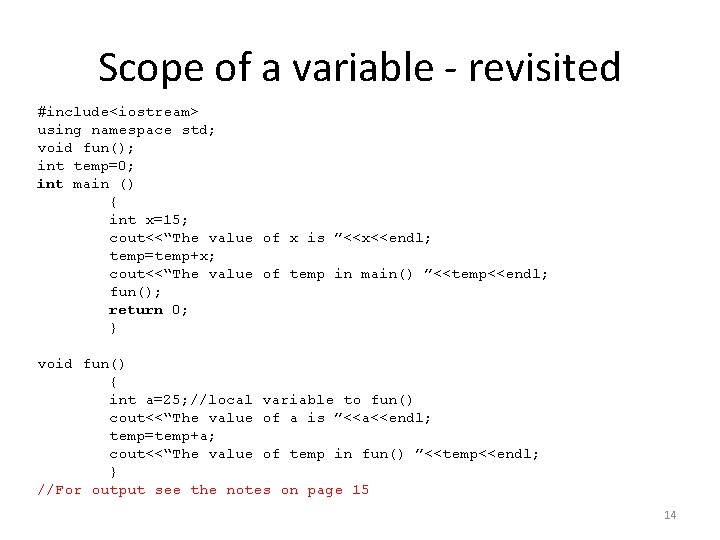
Scope of a variable - revisited #include<iostream> using namespace std; void fun(); int temp=0; int main () { int x=15; cout<<“The value of x is ”<<x<<endl; temp=temp+x; cout<<“The value of temp in main() ”<<temp<<endl; fun(); return 0; } void fun() { int a=25; //local variable to fun() cout<<“The value of a is ”<<a<<endl; temp=temp+a; cout<<“The value of temp in fun() ”<<temp<<endl; } //For output see the notes on page 15 14
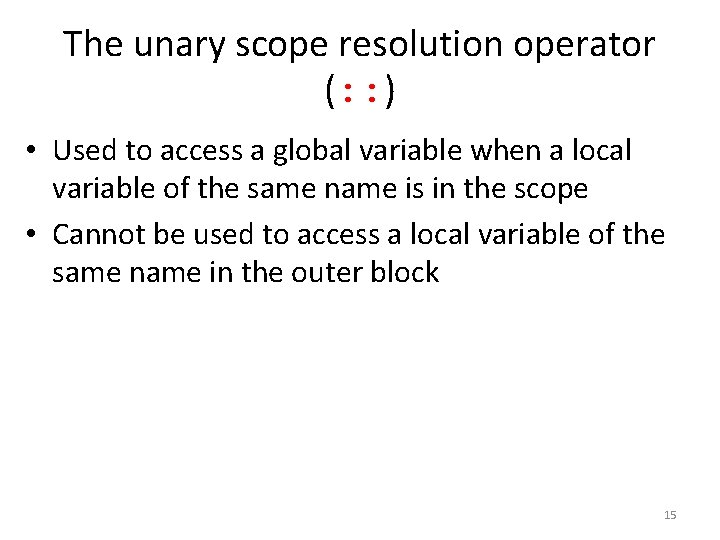
The unary scope resolution operator (: : ) • Used to access a global variable when a local variable of the same name is in the scope • Cannot be used to access a local variable of the same name in the outer block 15
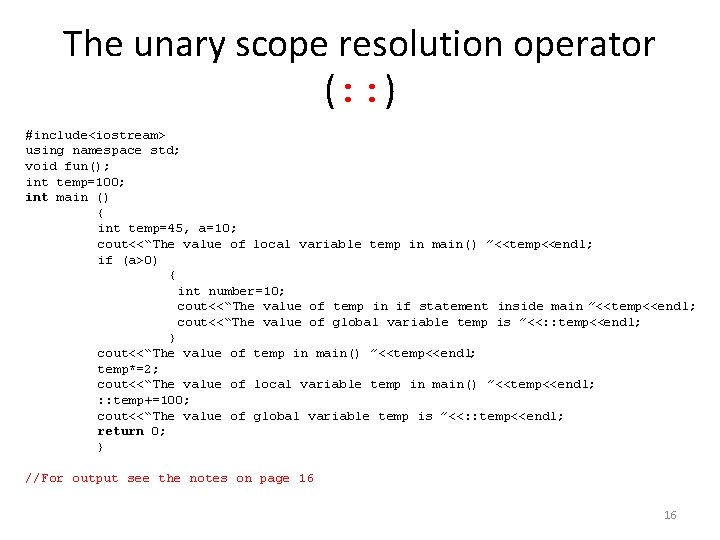
The unary scope resolution operator (: : ) #include<iostream> using namespace std; void fun(); int temp=100; int main () { int temp=45, a=10; cout<<“The value of local variable temp in main() ”<<temp<<endl; if (a>0) { int number=10; cout<<“The value of temp in if statement inside main ”<<temp<<endl; cout<<“The value of global variable temp is ”<<: : temp<<endl; } cout<<“The value of temp in main() ”<<temp<<endl; temp*=2; cout<<“The value of local variable temp in main() ”<<temp<<endl; : : temp+=100; cout<<“The value of global variable temp is ”<<: : temp<<endl; return 0; } //For output see the notes on page 16 16
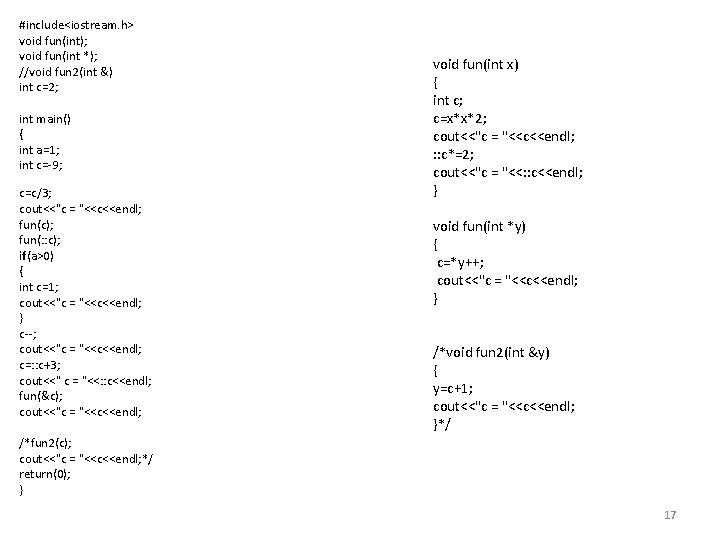
#include<iostream. h> void fun(int); void fun(int *); //void fun 2(int &) int c=2; int main() { int a=1; int c=-9; c=c/3; cout<<"c = "<<c<<endl; fun(c); fun(: : c); if(a>0) { int c=1; cout<<"c = "<<c<<endl; } c--; cout<<"c = "<<c<<endl; c=: : c+3; cout<<" c = "<<: : c<<endl; fun(&c); cout<<"c = "<<c<<endl; void fun(int x) { int c; c=x*x*2; cout<<"c = "<<c<<endl; : : c*=2; cout<<"c = "<<: : c<<endl; } void fun(int *y) { c=*y++; cout<<"c = "<<c<<endl; } /*void fun 2(int &y) { y=c+1; cout<<"c = "<<c<<endl; }*/ /*fun 2(c); cout<<"c = "<<c<<endl; */ return(0); } 17