Introduction to Programming 1 Dr Khizar Hayat Associate
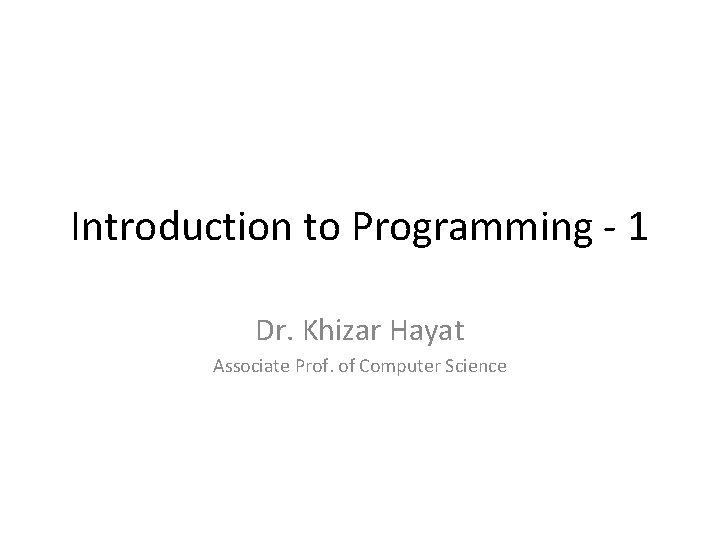
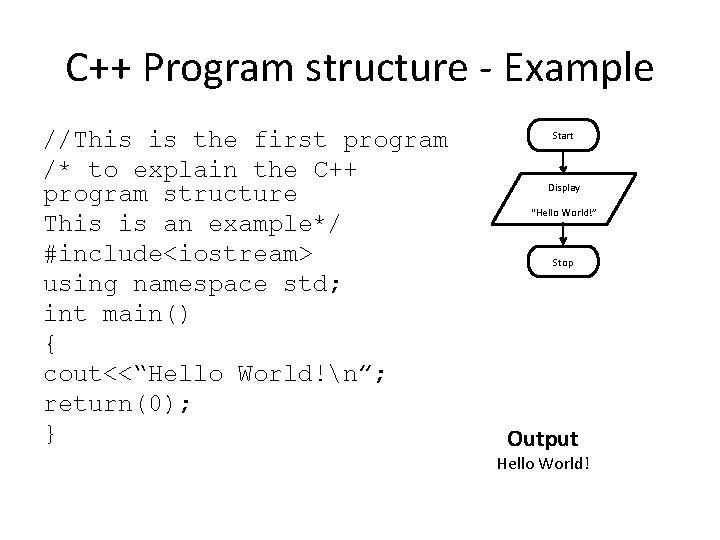
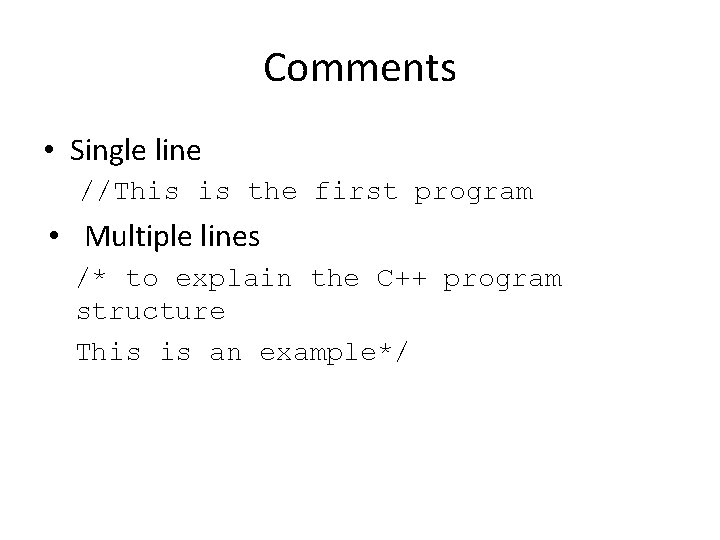
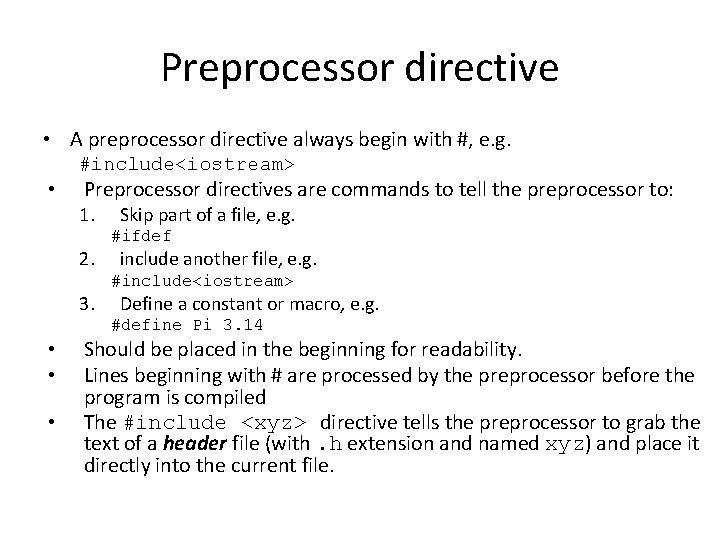
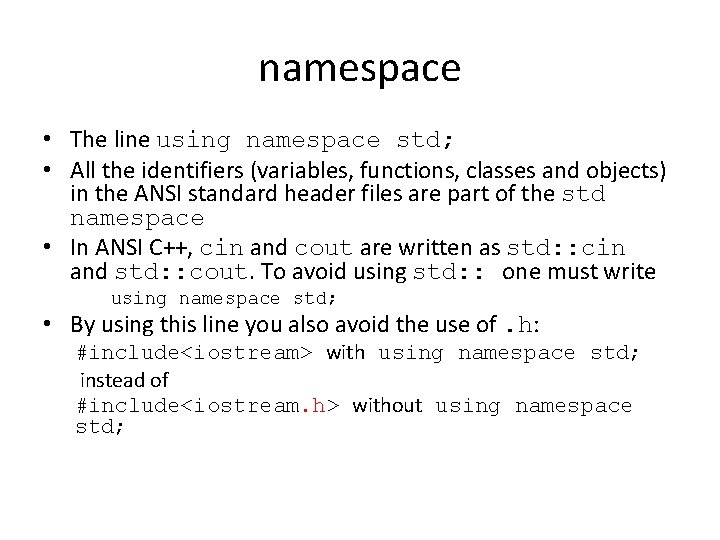
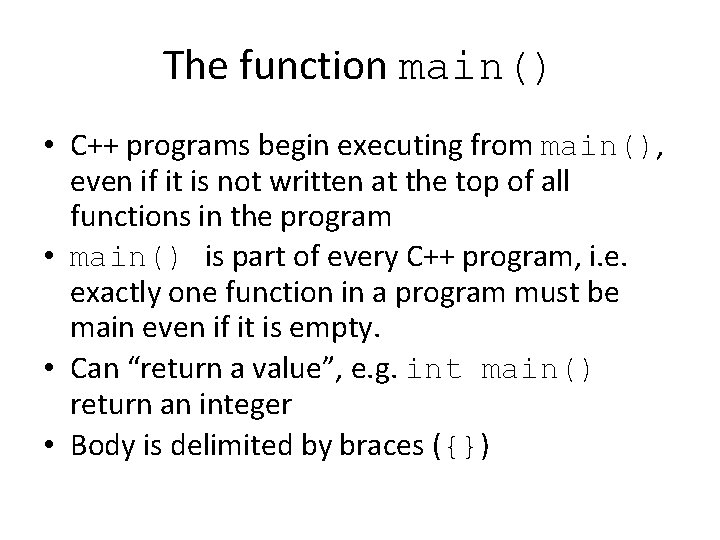
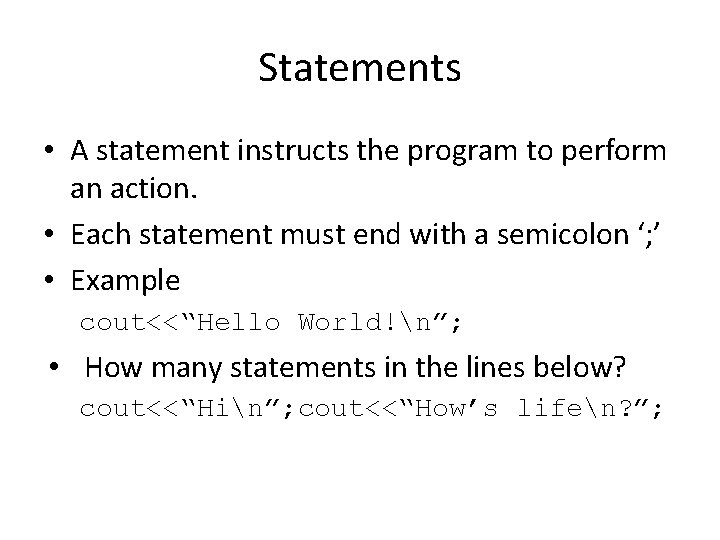
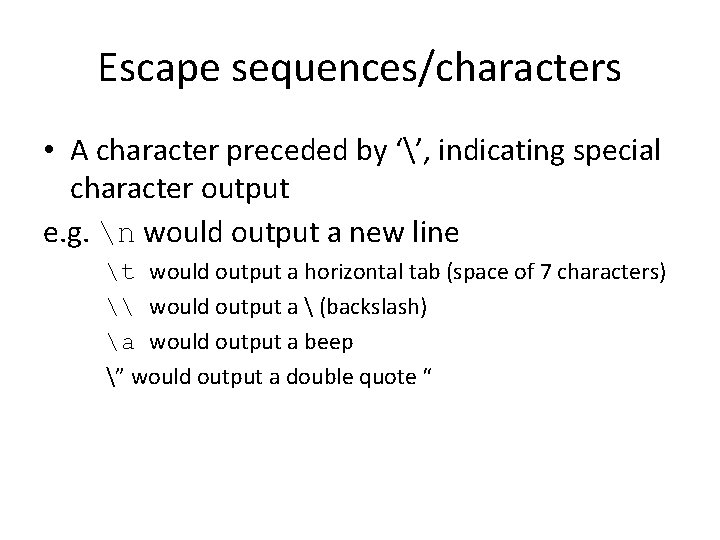
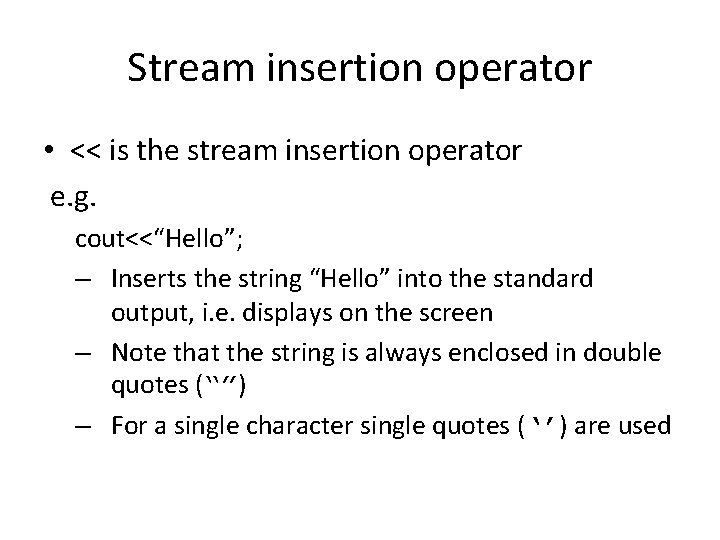
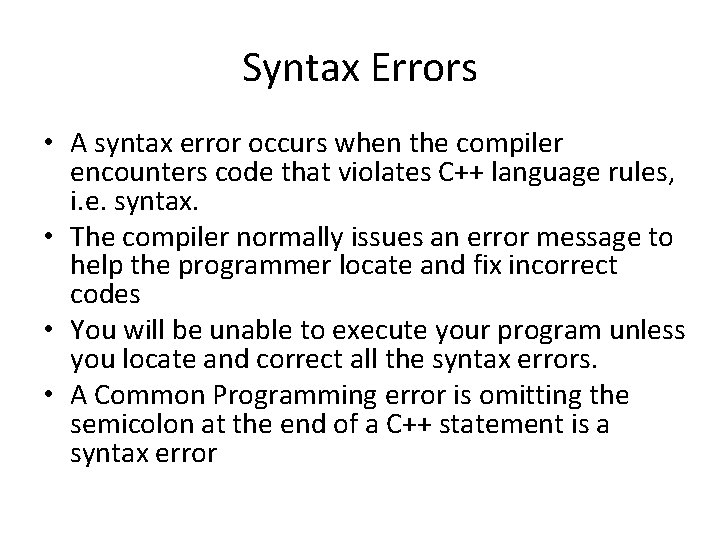
- Slides: 10
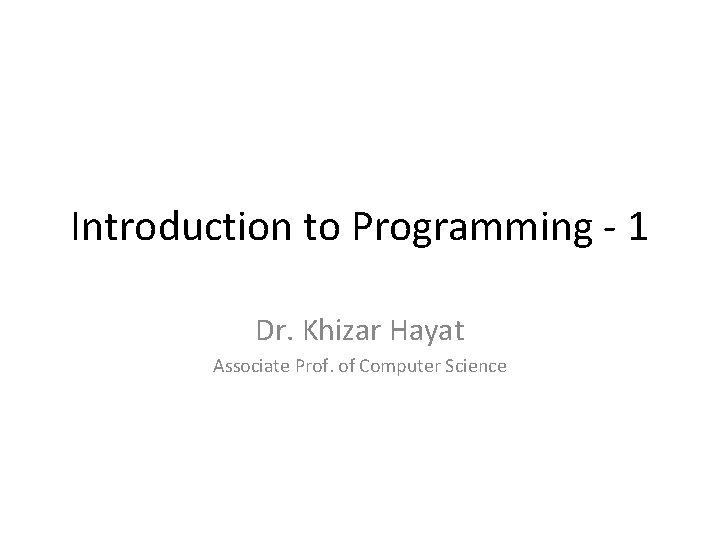
Introduction to Programming - 1 Dr. Khizar Hayat Associate Prof. of Computer Science
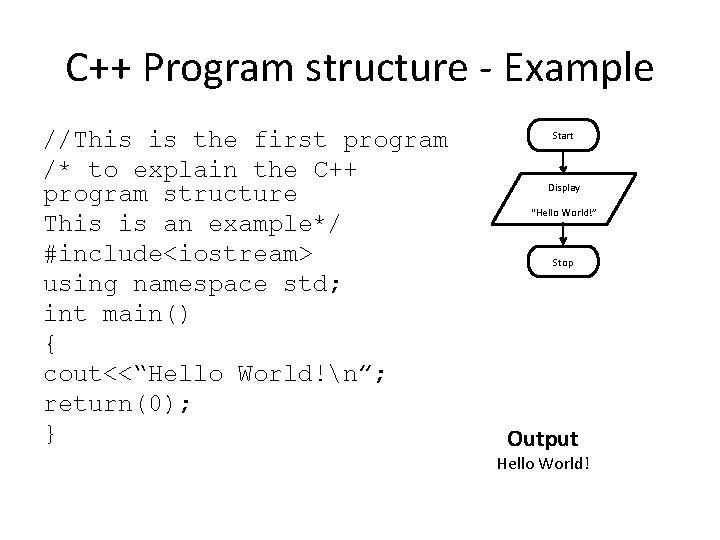
C++ Program structure - Example //This is the first program /* to explain the C++ program structure This is an example*/ #include<iostream> using namespace std; int main() { cout<<“Hello World!n”; return(0); } Start Display “Hello World!” Stop Output Hello World!
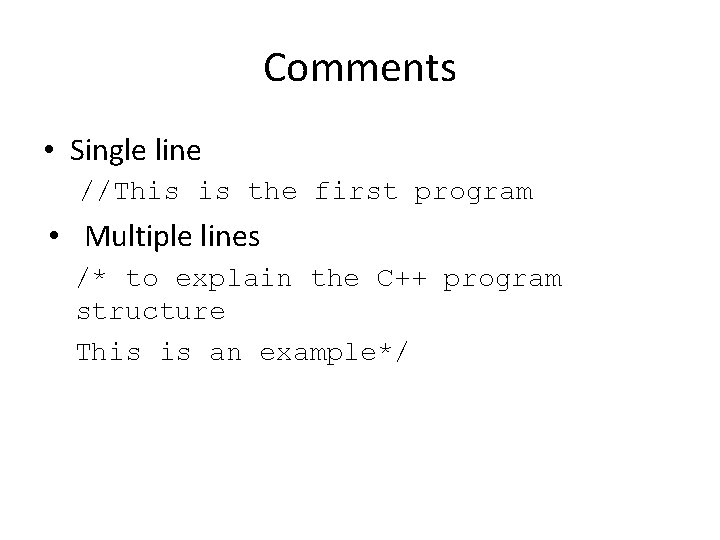
Comments • Single line //This is the first program • Multiple lines /* to explain the C++ program structure This is an example*/
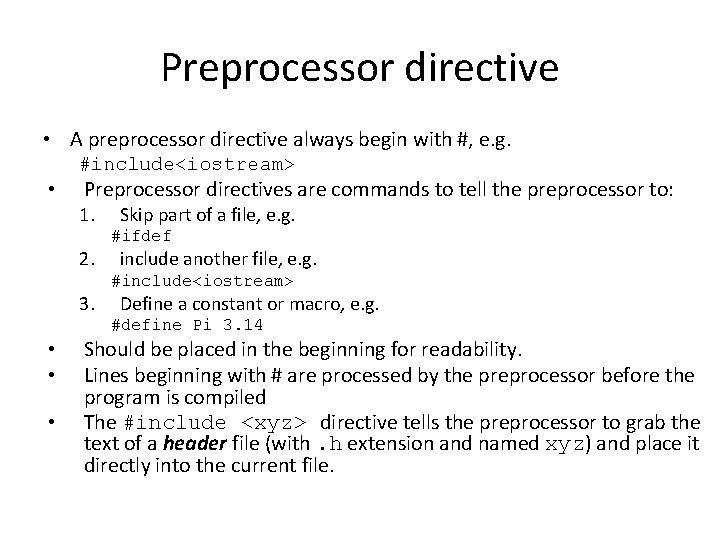
Preprocessor directive • A preprocessor directive always begin with #, e. g. #include<iostream> • Preprocessor directives are commands to tell the preprocessor to: 1. 2. 3. Skip part of a file, e. g. #ifdef include another file, e. g. #include<iostream> Define a constant or macro, e. g. #define Pi 3. 14 • • • Should be placed in the beginning for readability. Lines beginning with # are processed by the preprocessor before the program is compiled The #include <xyz> directive tells the preprocessor to grab the text of a header file (with. h extension and named xyz) and place it directly into the current file.
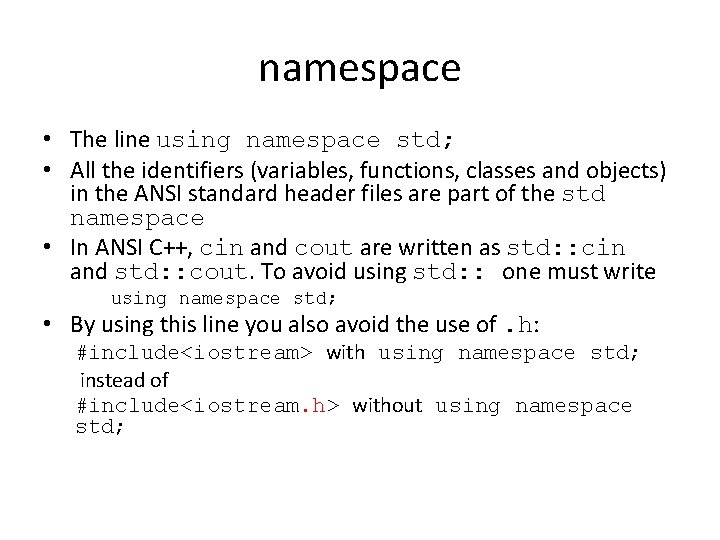
namespace • The line using namespace std; • All the identifiers (variables, functions, classes and objects) in the ANSI standard header files are part of the std namespace • In ANSI C++, cin and cout are written as std: : cin and std: : cout. To avoid using std: : one must write using namespace std; • By using this line you also avoid the use of. h: #include<iostream> with using namespace std; instead of #include<iostream. h> without using namespace std;
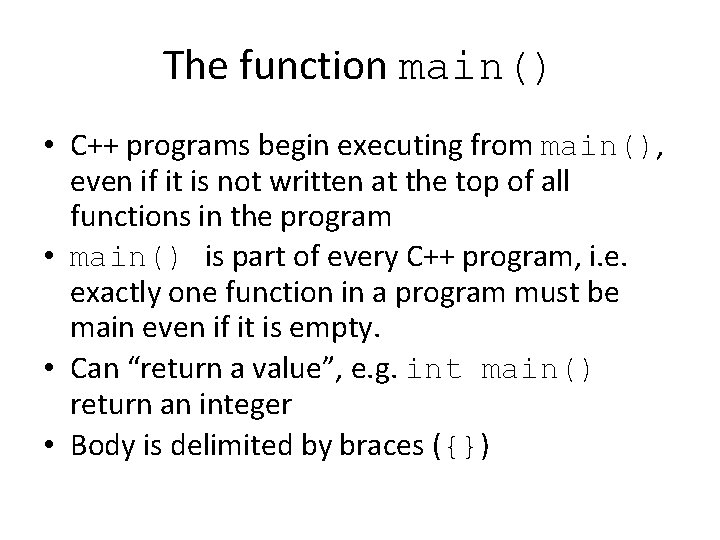
The function main() • C++ programs begin executing from main(), even if it is not written at the top of all functions in the program • main() is part of every C++ program, i. e. exactly one function in a program must be main even if it is empty. • Can “return a value”, e. g. int main() return an integer • Body is delimited by braces ({})
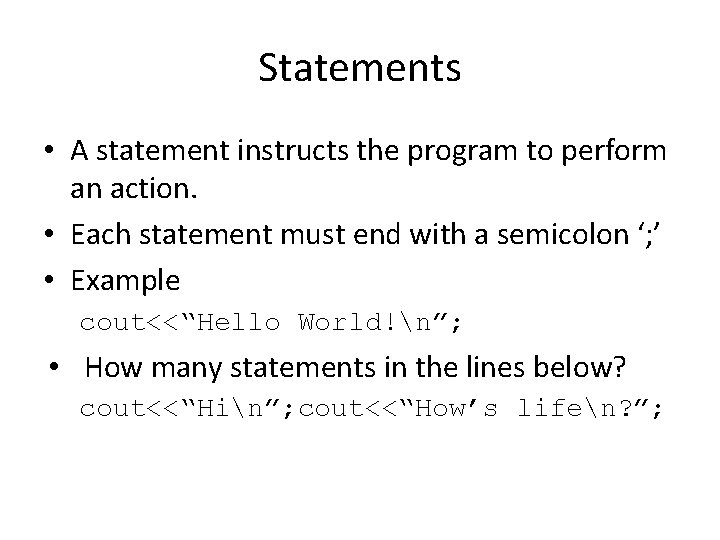
Statements • A statement instructs the program to perform an action. • Each statement must end with a semicolon ‘; ’ • Example cout<<“Hello World!n”; • How many statements in the lines below? cout<<“Hin”; cout<<“How’s lifen? ”;
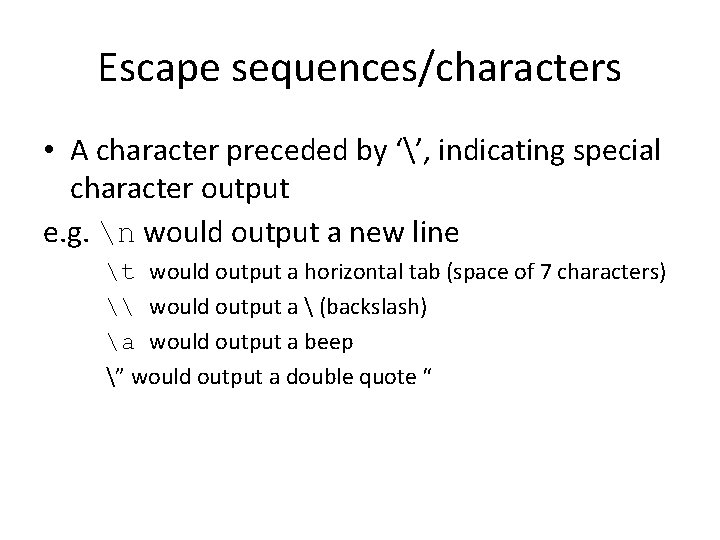
Escape sequences/characters • A character preceded by ‘’, indicating special character output e. g. n would output a new line t would output a horizontal tab (space of 7 characters) \ would output a (backslash) a would output a beep ” would output a double quote “
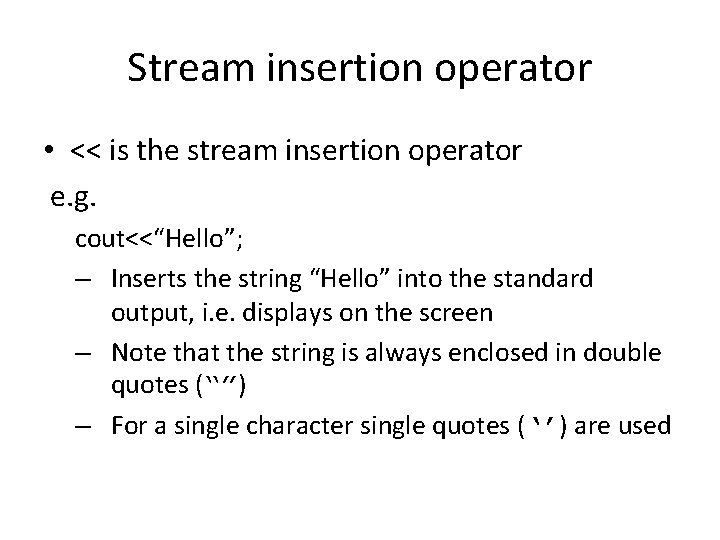
Stream insertion operator • << is the stream insertion operator e. g. cout<<“Hello”; – Inserts the string “Hello” into the standard output, i. e. displays on the screen – Note that the string is always enclosed in double quotes (“”) – For a single character single quotes (‘’) are used
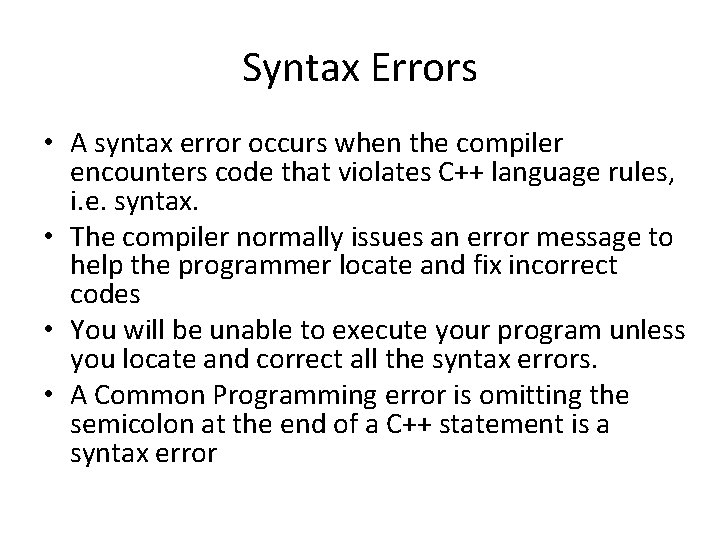
Syntax Errors • A syntax error occurs when the compiler encounters code that violates C++ language rules, i. e. syntax. • The compiler normally issues an error message to help the programmer locate and fix incorrect codes • You will be unable to execute your program unless you locate and correct all the syntax errors. • A Common Programming error is omitting the semicolon at the end of a C++ statement is a syntax error