Introduction to Programming 5 Operators2 Dr Khizar Hayat
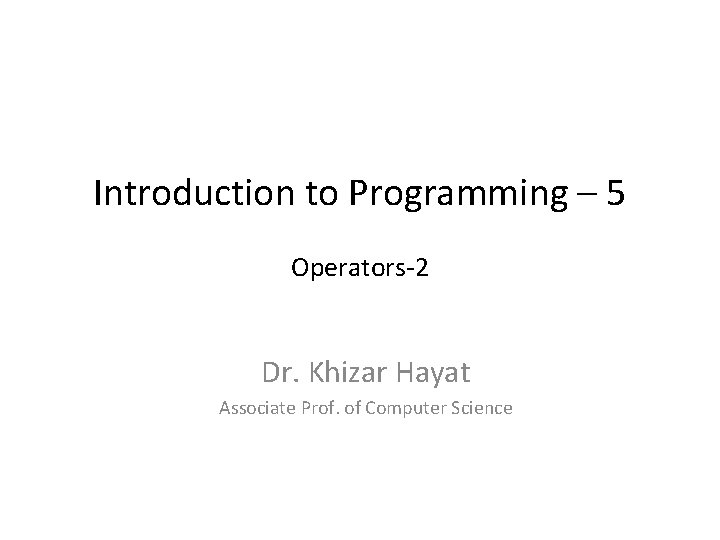
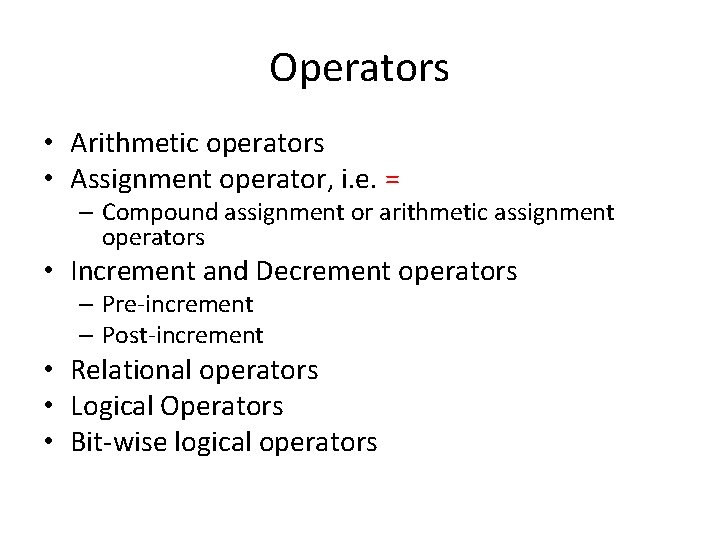
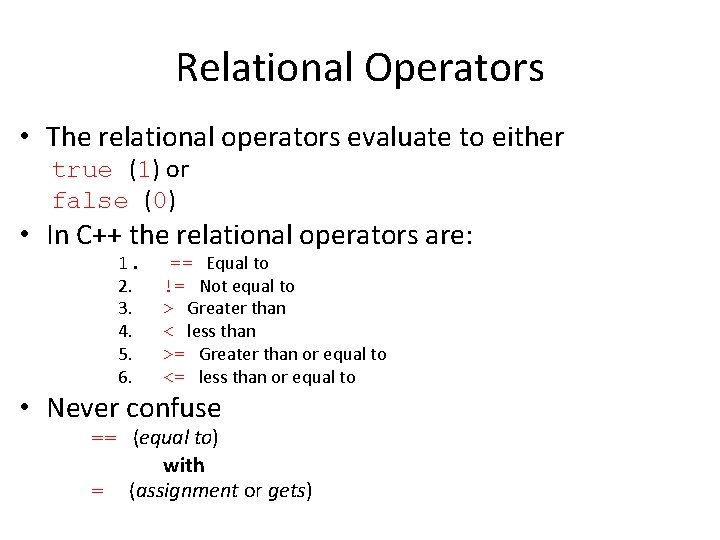
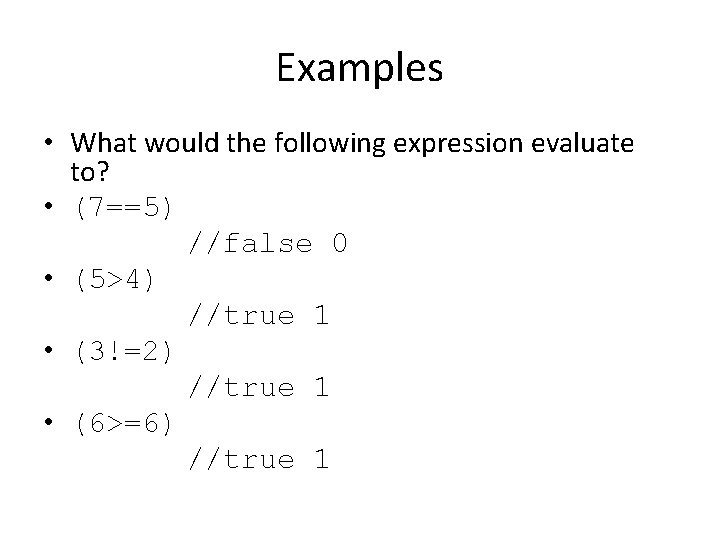
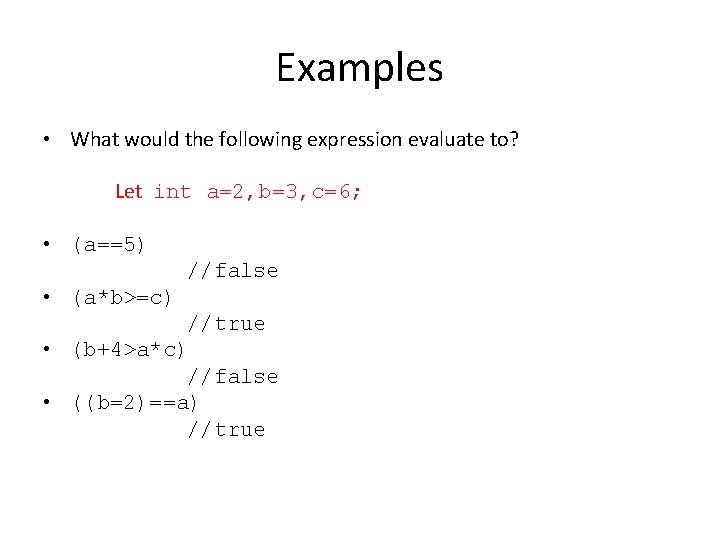
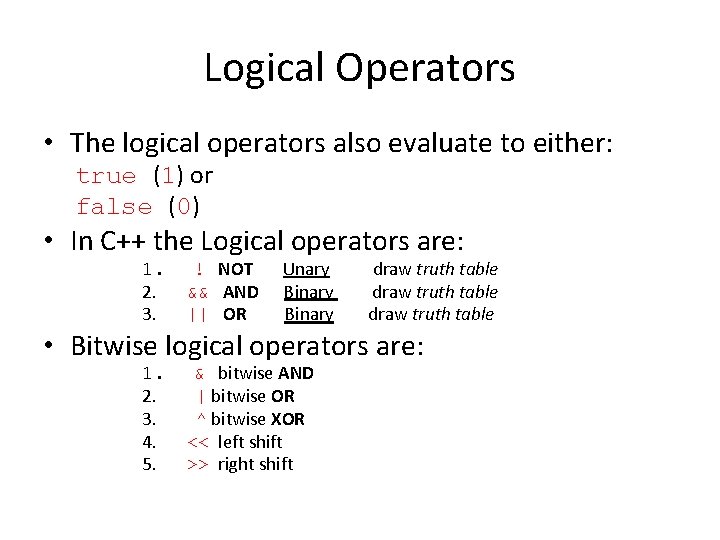
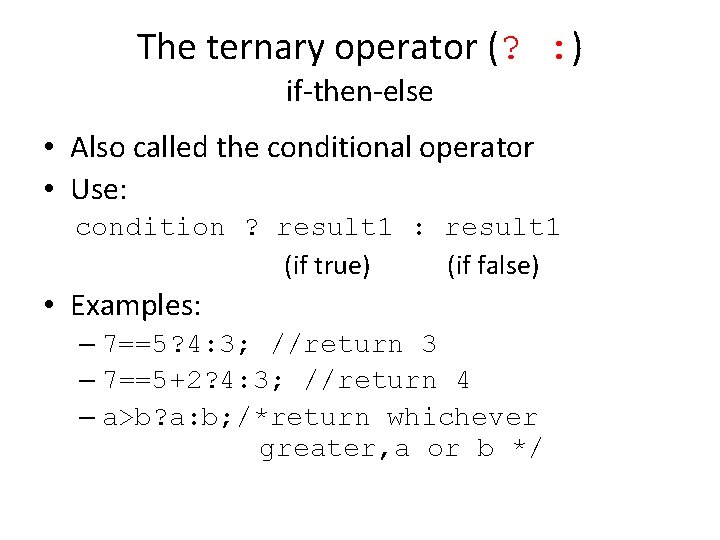
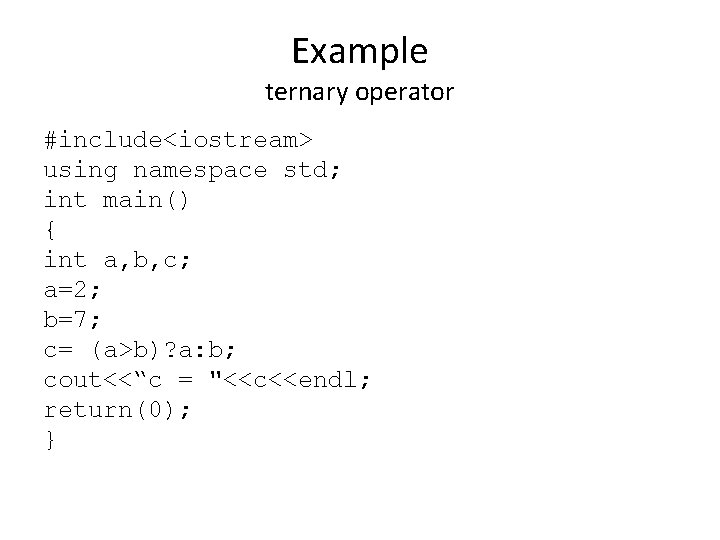
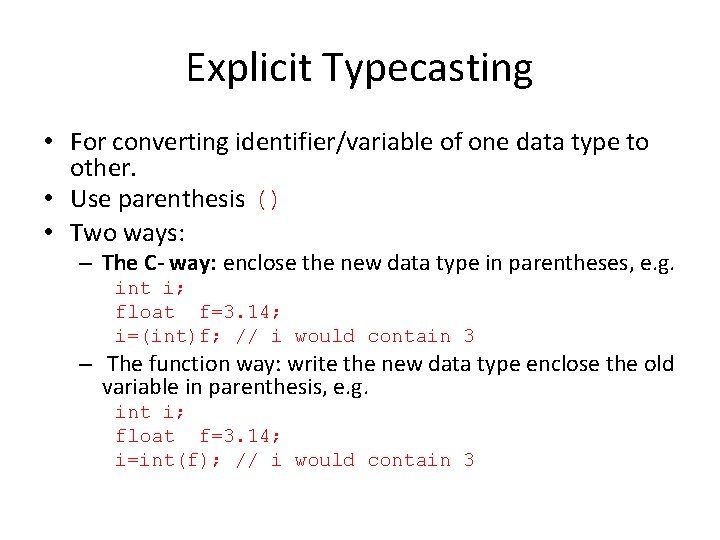
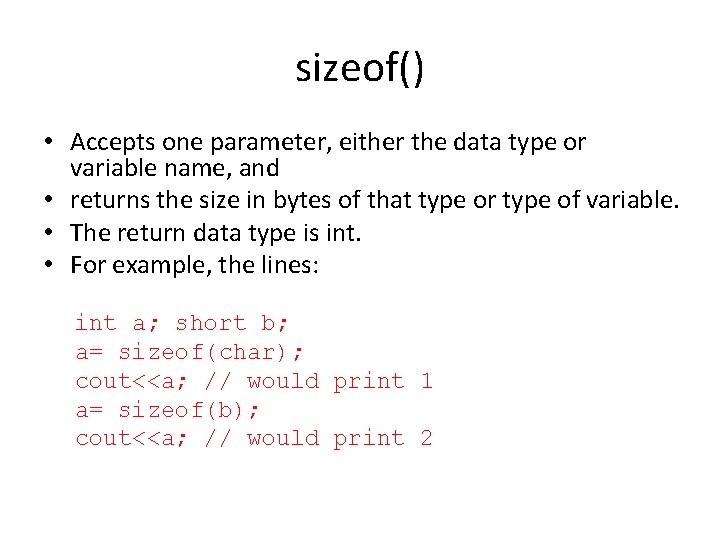
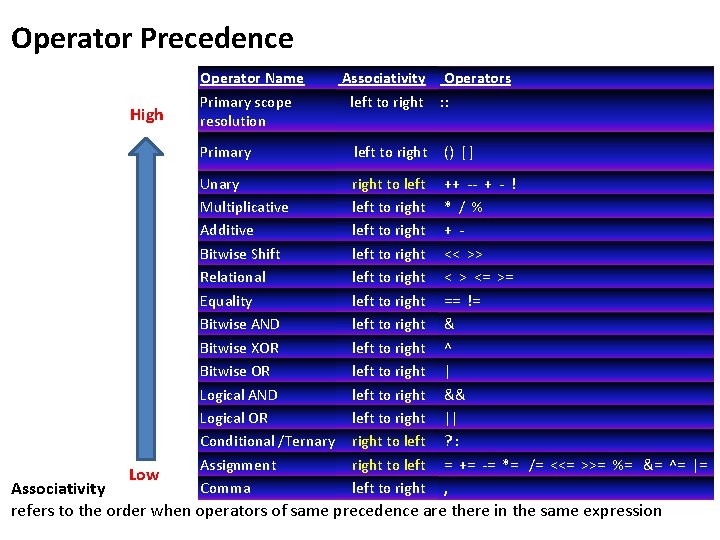
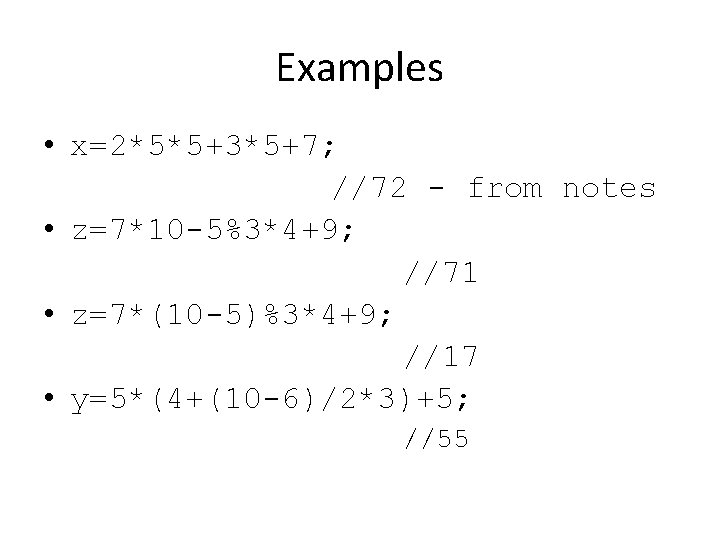
- Slides: 12
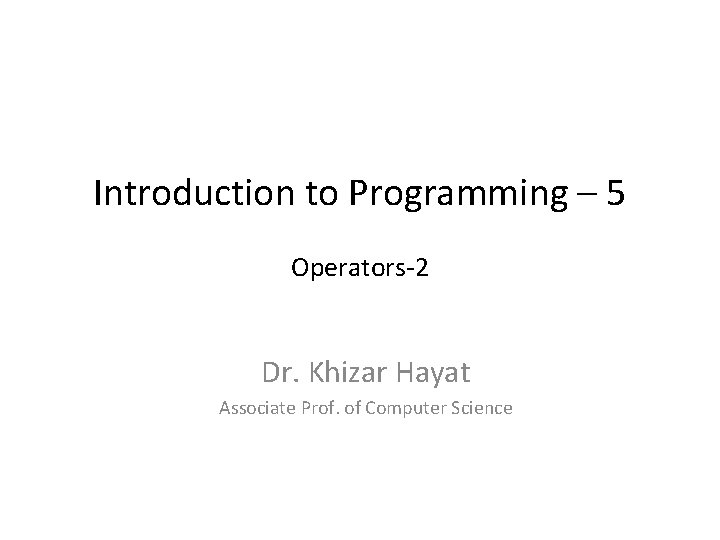
Introduction to Programming – 5 Operators-2 Dr. Khizar Hayat Associate Prof. of Computer Science
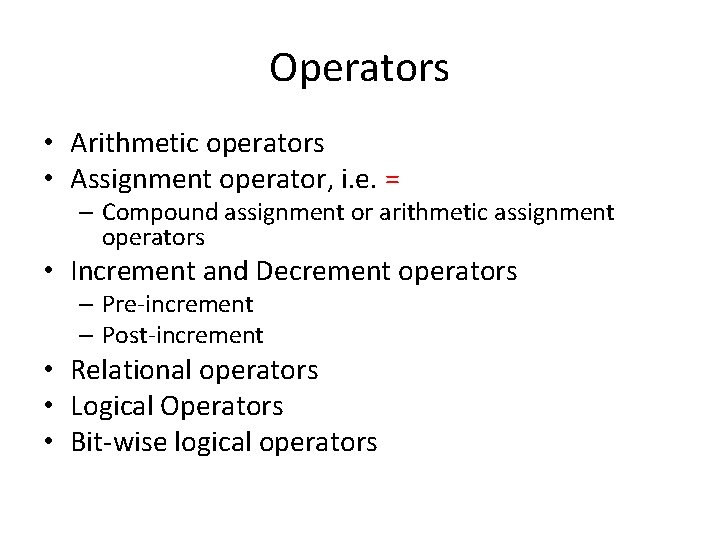
Operators • Arithmetic operators • Assignment operator, i. e. = – Compound assignment or arithmetic assignment operators • Increment and Decrement operators – Pre-increment – Post-increment • Relational operators • Logical Operators • Bit-wise logical operators
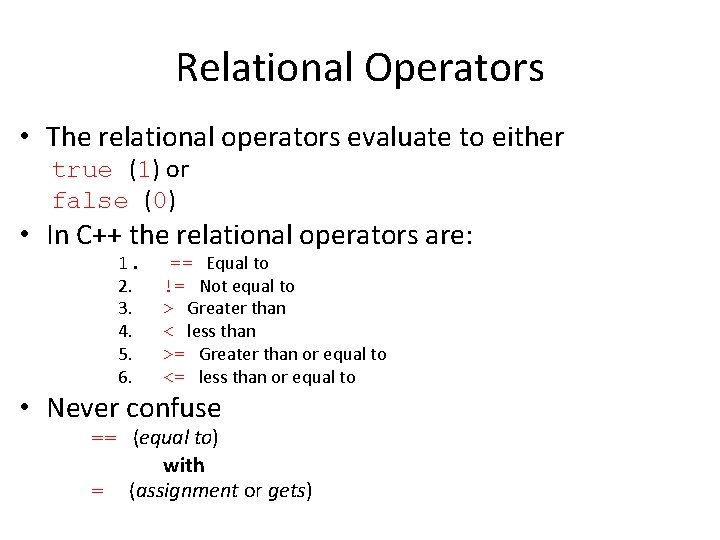
Relational Operators • The relational operators evaluate to either true (1) or false (0) • In C++ the relational operators are: 1. 2. 3. 4. 5. 6. == Equal to != Not equal to > Greater than < less than >= Greater than or equal to <= less than or equal to • Never confuse == (equal to) with = (assignment or gets)
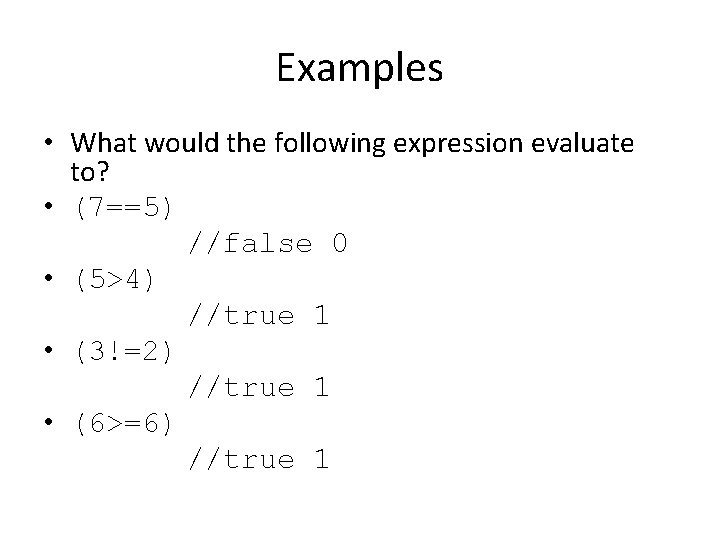
Examples • What would the following expression evaluate to? • (7==5) //false 0 • (5>4) //true 1 • (3!=2) //true 1 • (6>=6) //true 1
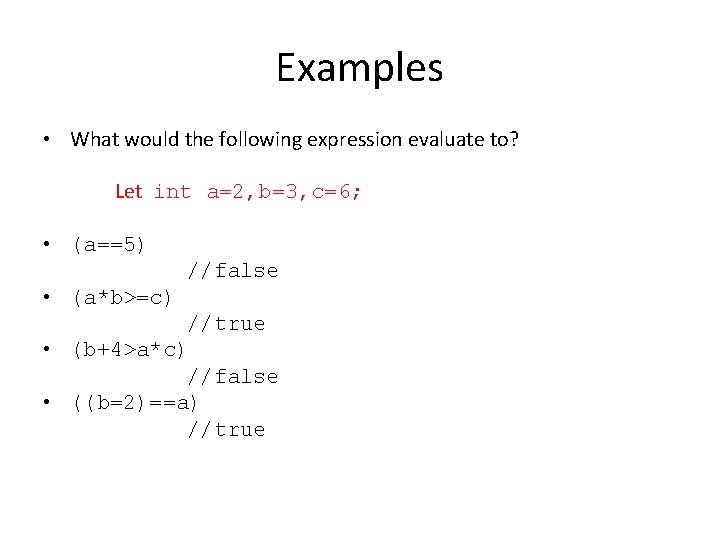
Examples • What would the following expression evaluate to? Let int a=2, b=3, c=6; • (a==5) • (a*b>=c) //false //true • (b+4>a*c) //false • ((b=2)==a) //true
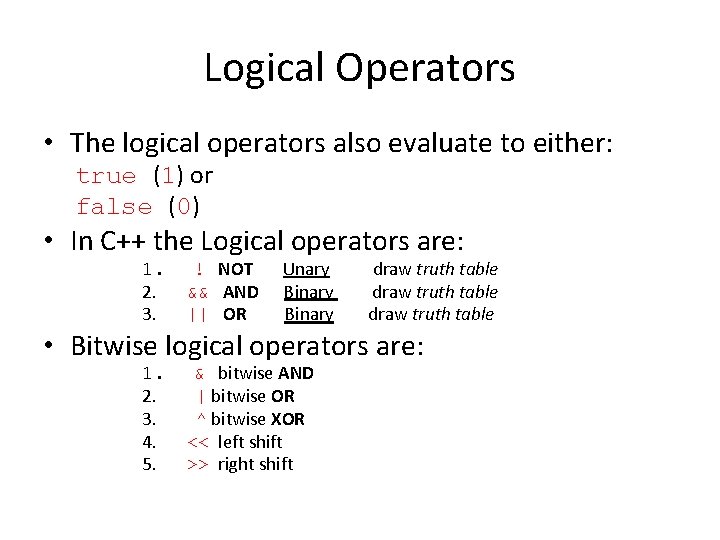
Logical Operators • The logical operators also evaluate to either: true (1) or false (0) • In C++ the Logical operators are: 1. 2. 3. ! NOT && AND || OR Unary Binary draw truth table • Bitwise logical operators are: 1. 2. 3. 4. 5. & bitwise AND | bitwise OR ^ bitwise XOR << left shift >> right shift
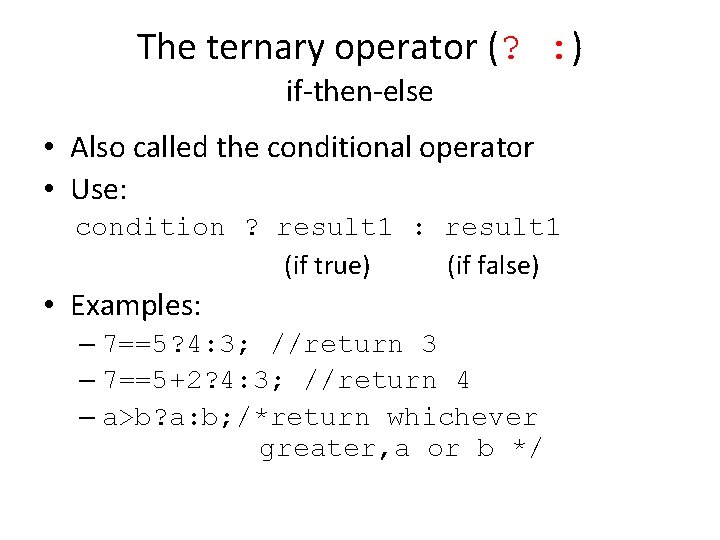
The ternary operator (? : ) if-then-else • Also called the conditional operator • Use: condition ? result 1 : result 1 (if true) (if false) • Examples: – 7==5? 4: 3; //return 3 – 7==5+2? 4: 3; //return 4 – a>b? a: b; /*return whichever greater, a or b */
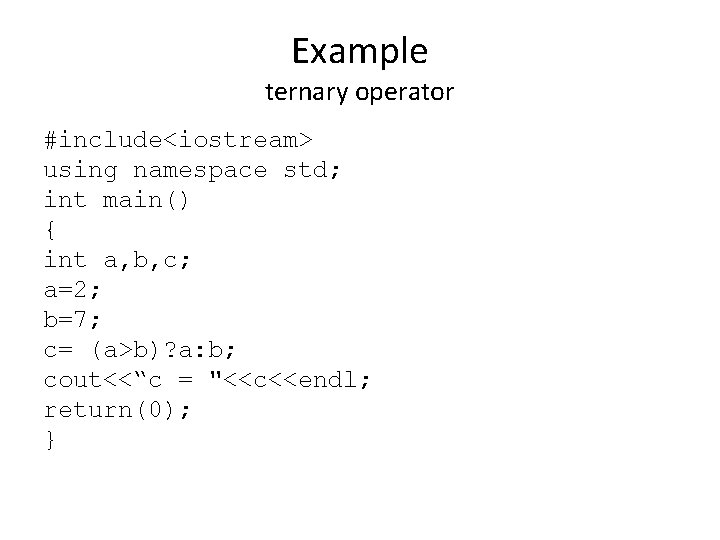
Example ternary operator #include<iostream> using namespace std; int main() { int a, b, c; a=2; b=7; c= (a>b)? a: b; cout<<“c = "<<c<<endl; return(0); }
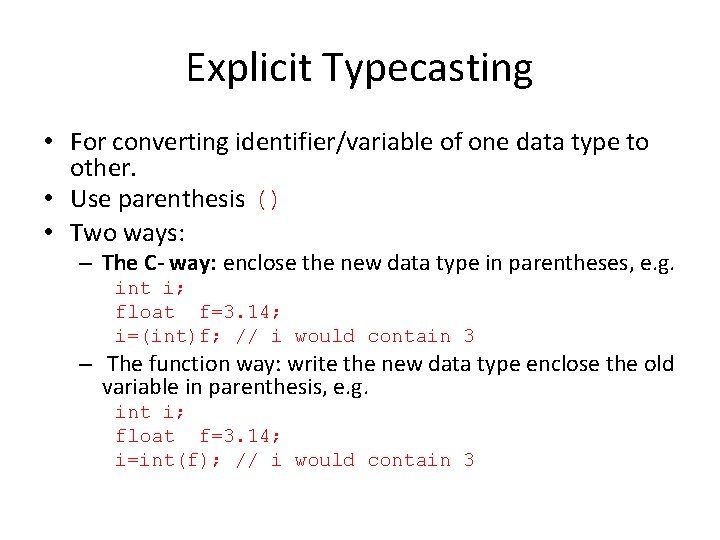
Explicit Typecasting • For converting identifier/variable of one data type to other. • Use parenthesis () • Two ways: – The C- way: enclose the new data type in parentheses, e. g. int i; float f=3. 14; i=(int)f; // i would contain 3 – The function way: write the new data type enclose the old variable in parenthesis, e. g. int i; float f=3. 14; i=int(f); // i would contain 3
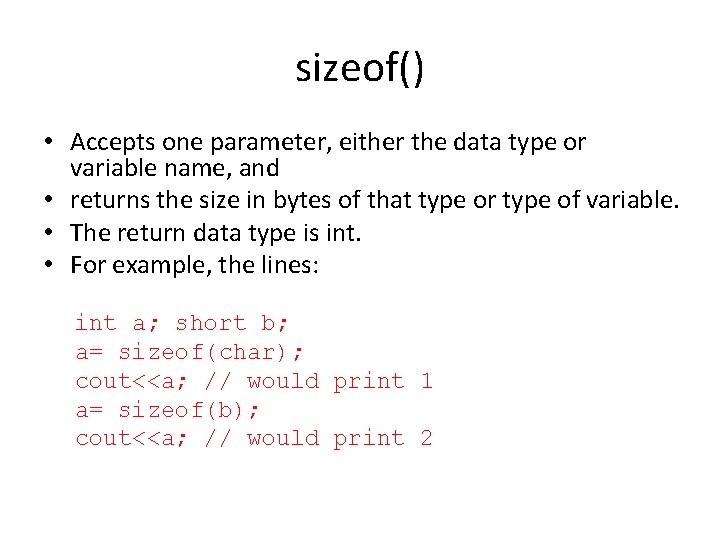
sizeof() • Accepts one parameter, either the data type or variable name, and • returns the size in bytes of that type or type of variable. • The return data type is int. • For example, the lines: int a; short b; a= sizeof(char); cout<<a; // would print 1 a= sizeof(b); cout<<a; // would print 2
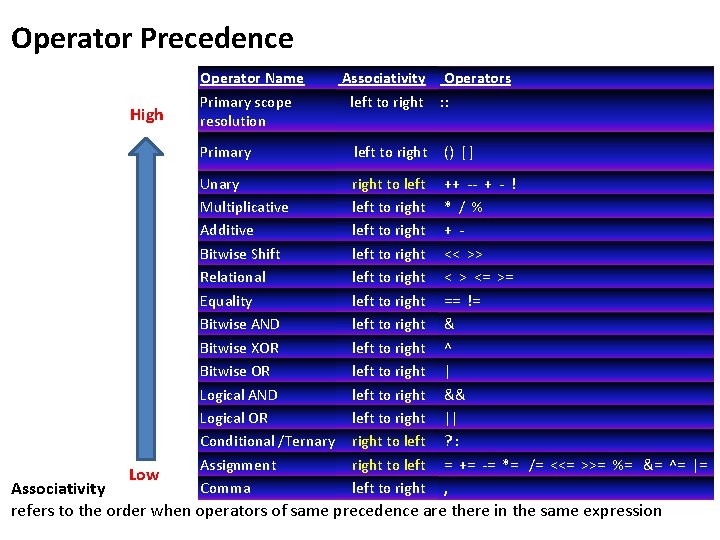
Operator Precedence High Low Operator Name Primary scope resolution Associativity Operators left to right : : Primary left to right () [ ] Unary Multiplicative Additive Bitwise Shift Relational Equality Bitwise AND Bitwise XOR Bitwise OR Logical AND Logical OR Conditional /Ternary Assignment Comma right to left to right left to right left to right to left right to left to right ++ -- + - ! * / % + << >> < > <= >= == != & ^ | && || ? : = += -= *= /= <<= >>= %= &= ^= |= , Associativity refers to the order when operators of same precedence are there in the same expression
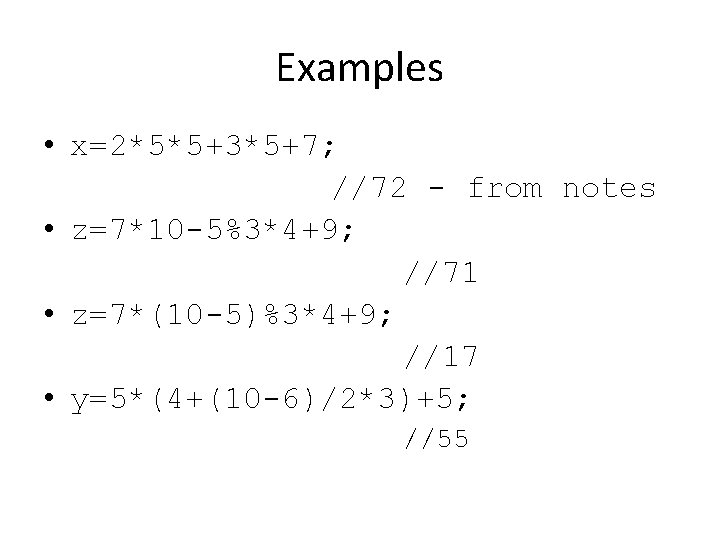
Examples • x=2*5*5+3*5+7; //72 - from notes • z=7*10 -5%3*4+9; //71 • z=7*(10 -5)%3*4+9; //17 • y=5*(4+(10 -6)/2*3)+5; //55
Hayat ve hayat dışı sigortalar arasındaki farklar
Raunkiaer hayat formları
Insurans am tingkatan 5
Maksud hak cipta terpelihara
Adli olgu nedir
Engelsiz hayat teknolojileri 7. sınıf sunum
Jumlah fasa yang terlibat dalam pembangunan atur cara
Osmancılık nedir
Karamand
Mamülün hayat seyri
Kitaran hayat projek
Hayat bilgisi dersinin kurucusu
Allah'ın koymuş olduğu sınırları gözetenler ile