For Loops Constant Value Role Accumulator Role Introduction
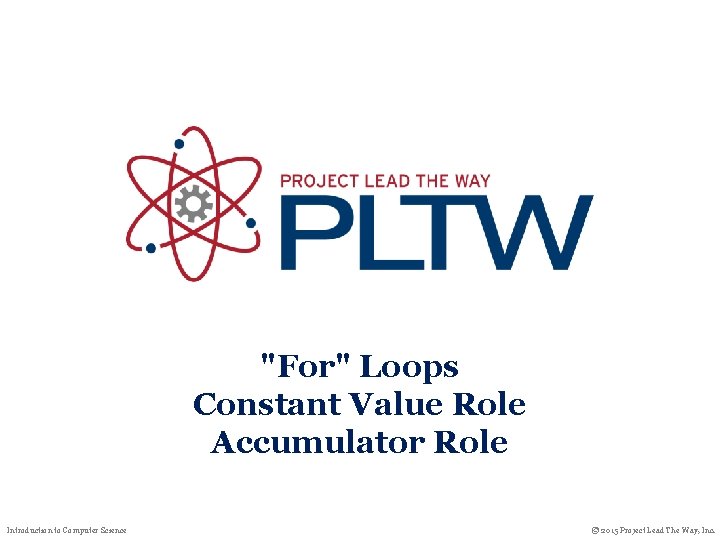
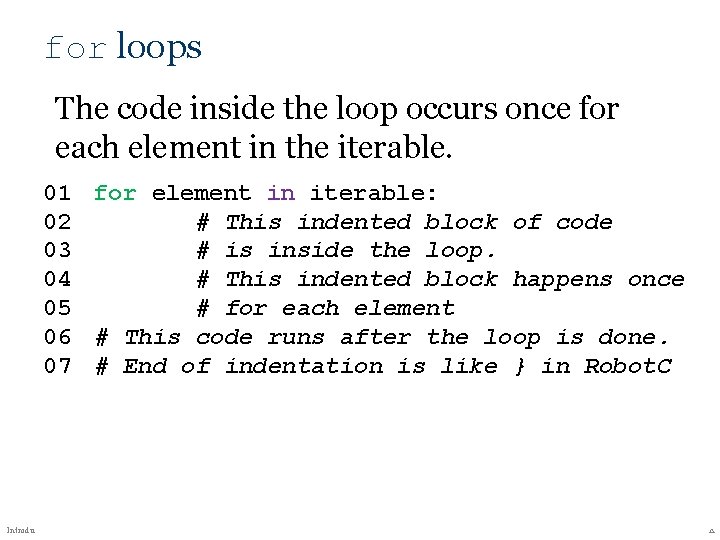
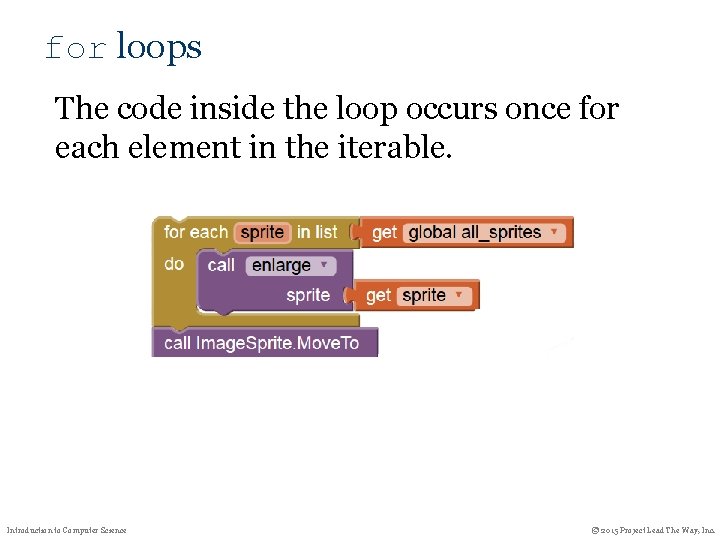
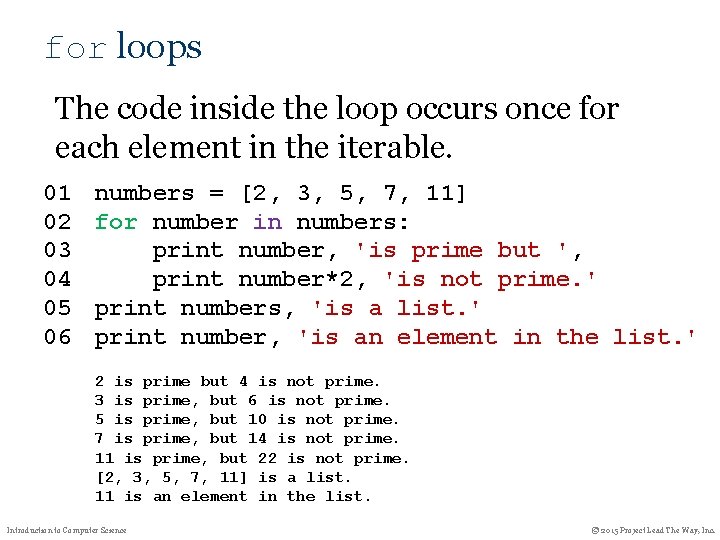
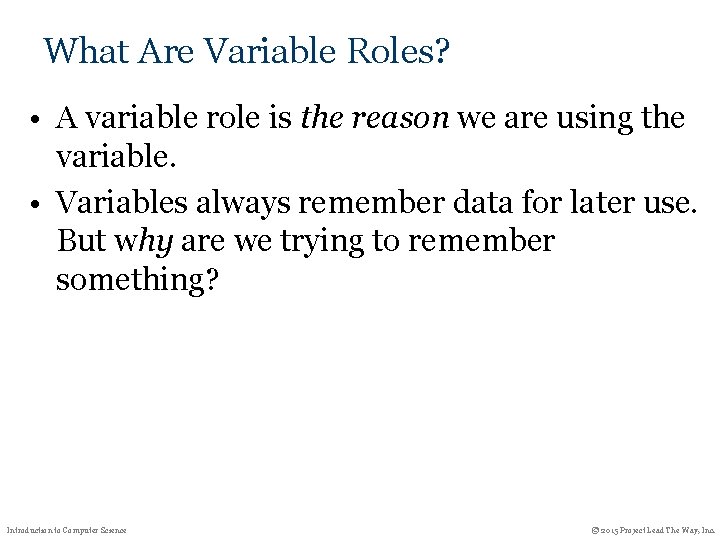
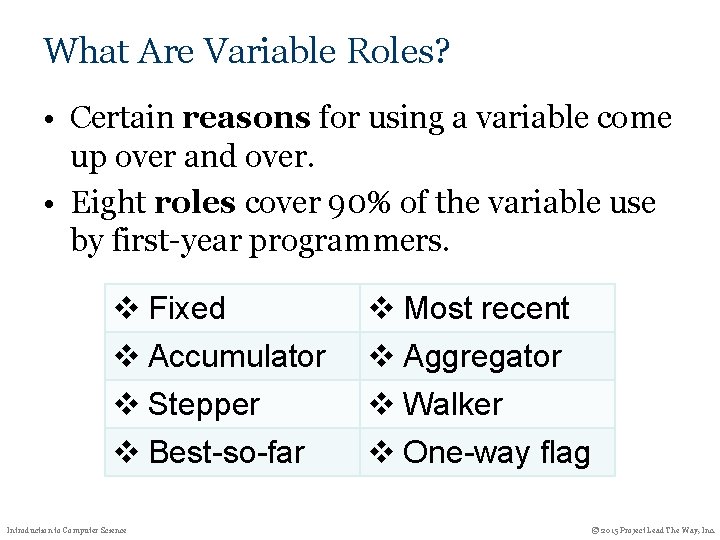
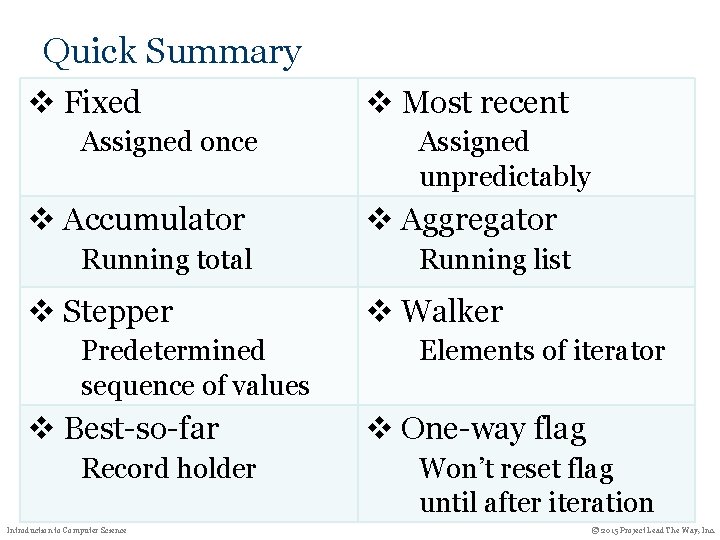
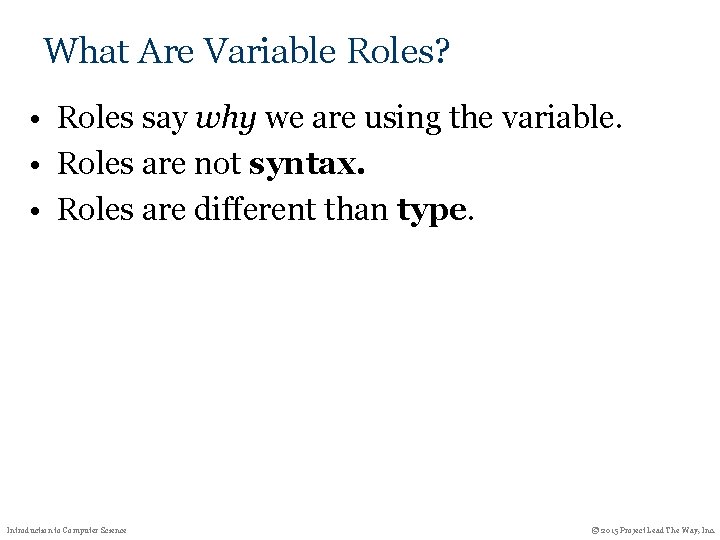
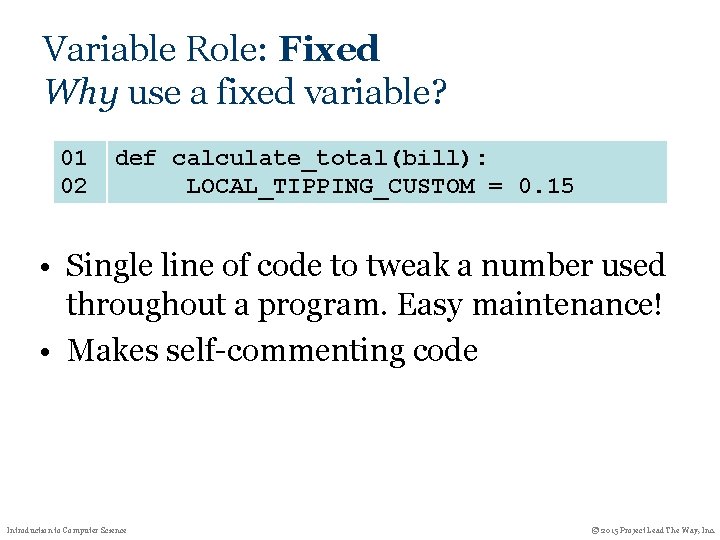
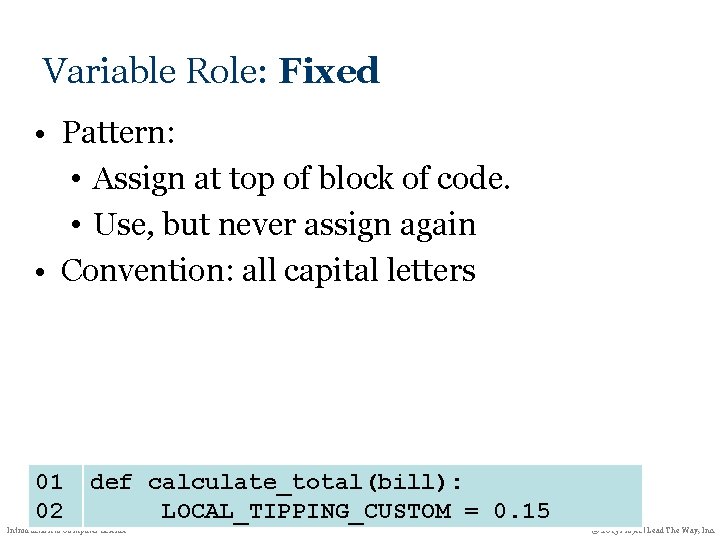
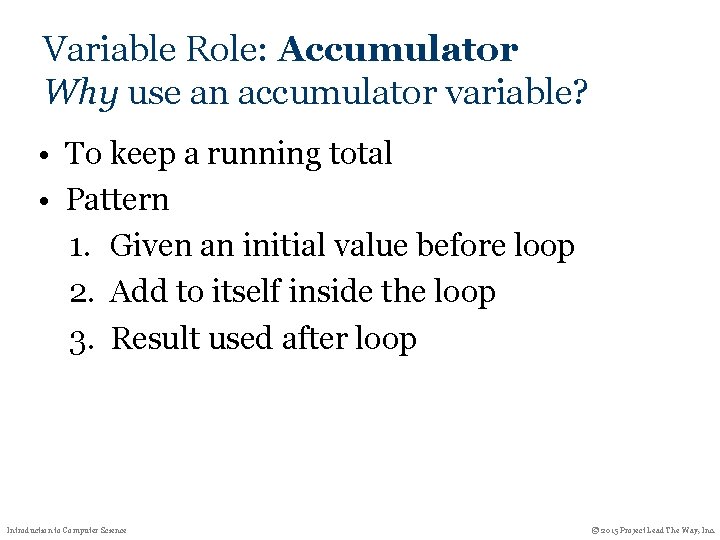
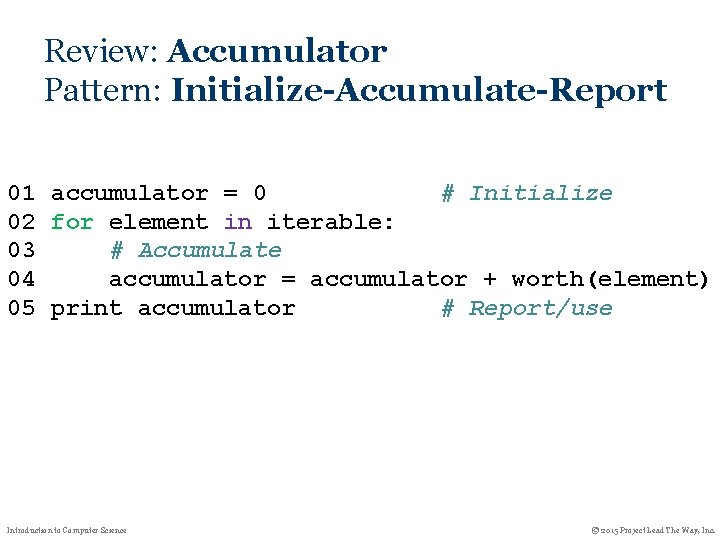
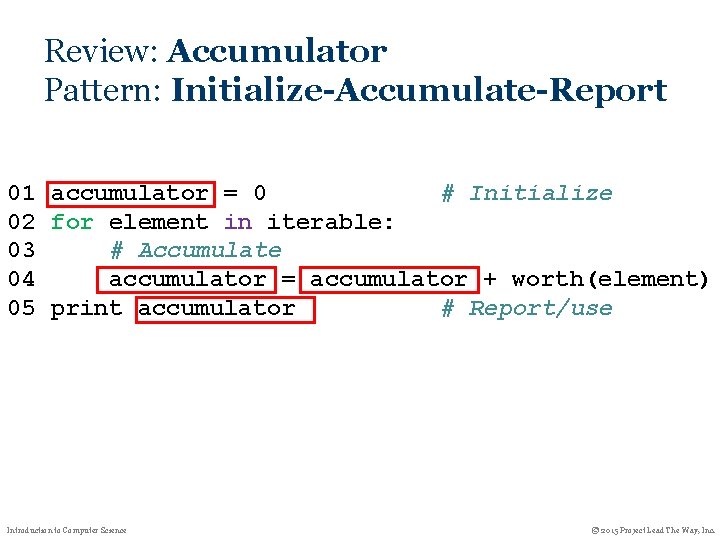
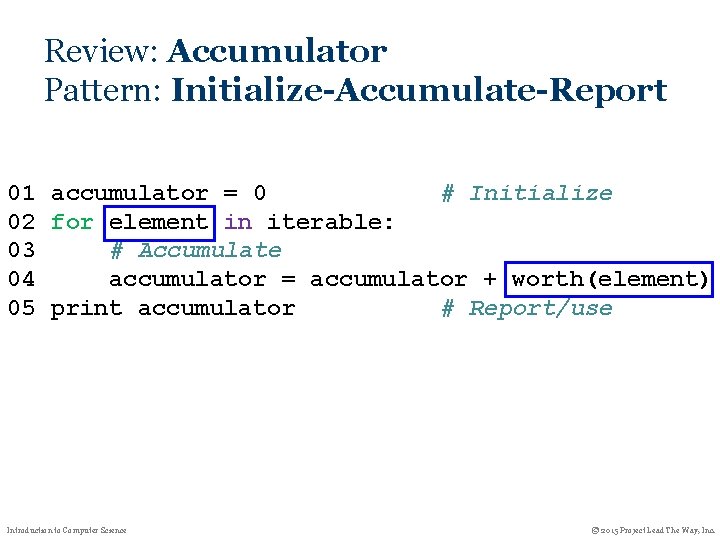
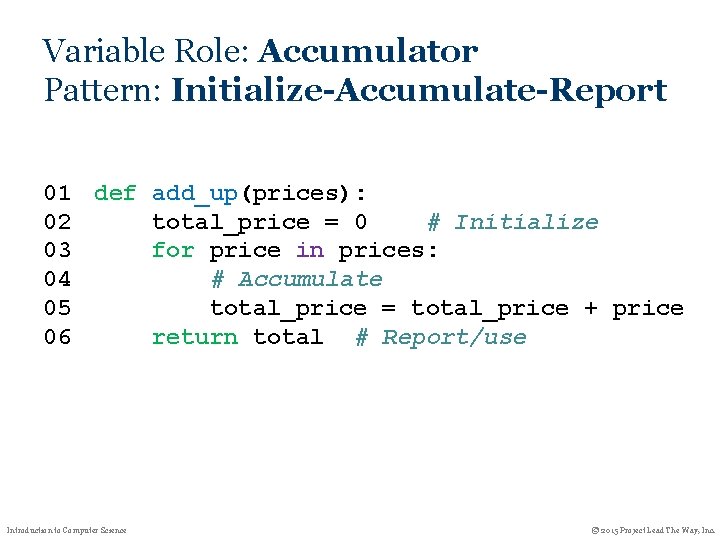
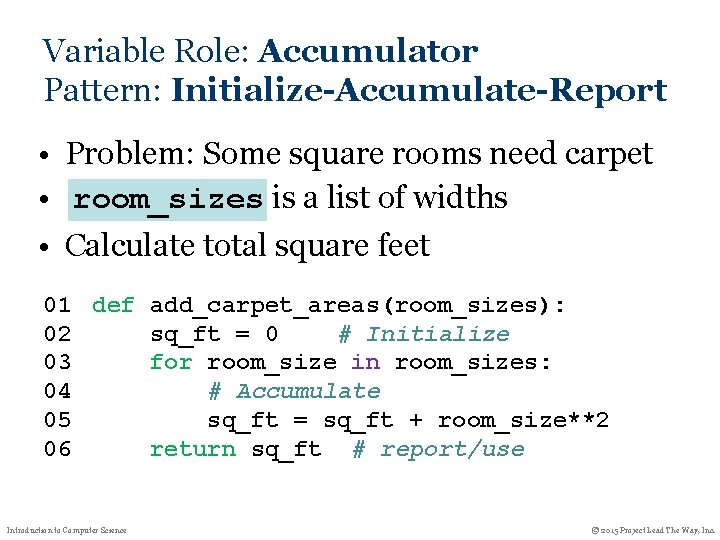
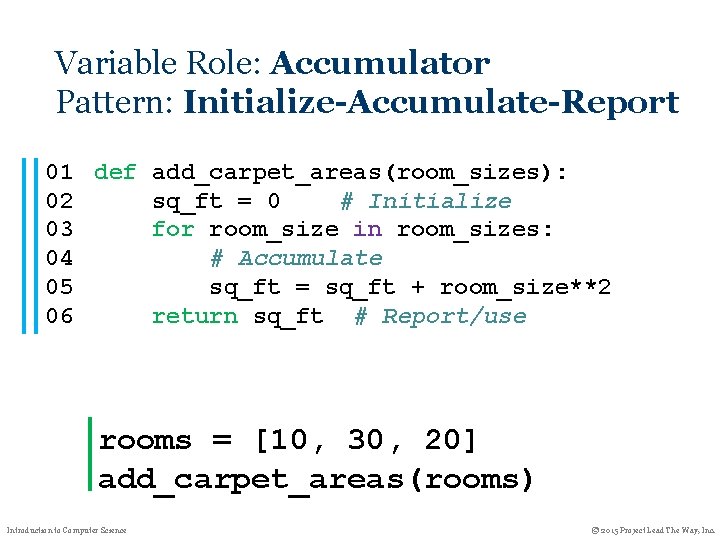
- Slides: 17
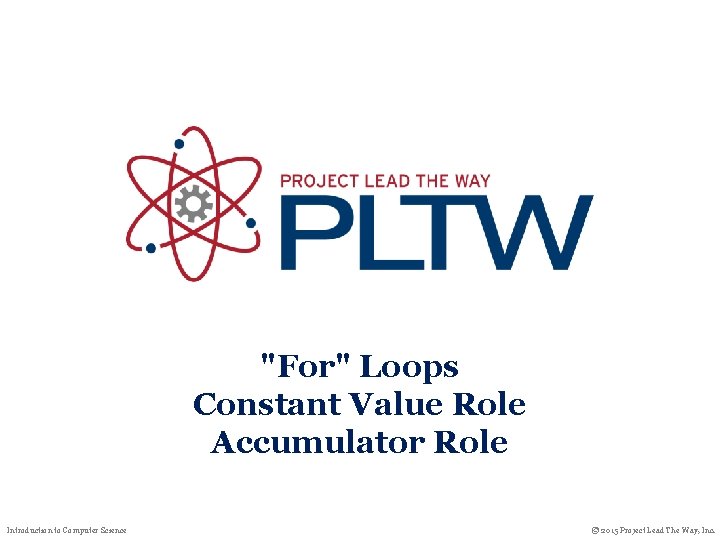
"For" Loops Constant Value Role Accumulator Role Introduction to Computer Science © 2015 Project Lead The Way, Inc.
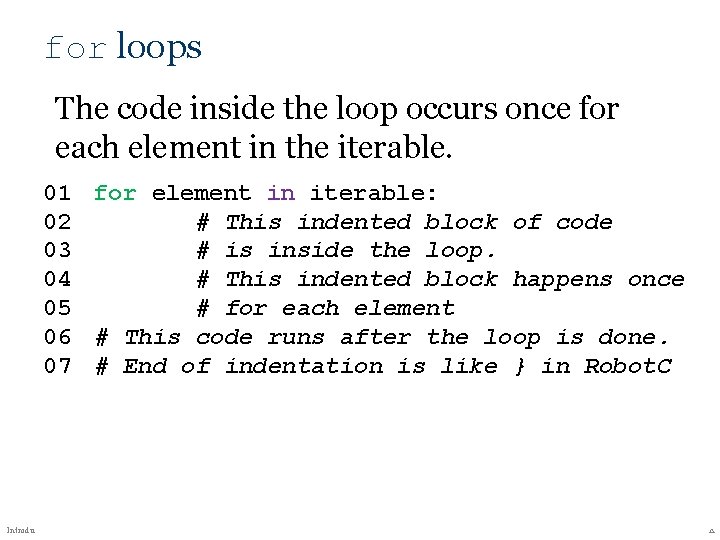
for loops The code inside the loop occurs once for each element in the iterable. 01 for element in iterable: 02 # This indented block of code 03 # is inside the loop. 04 # This indented block happens once 05 # for each element 06 # This code runs after the loop is done. 07 # End of indentation is like } in Robot. C Introduction to Computer Science © 2015 Project Lead The Way, Inc.
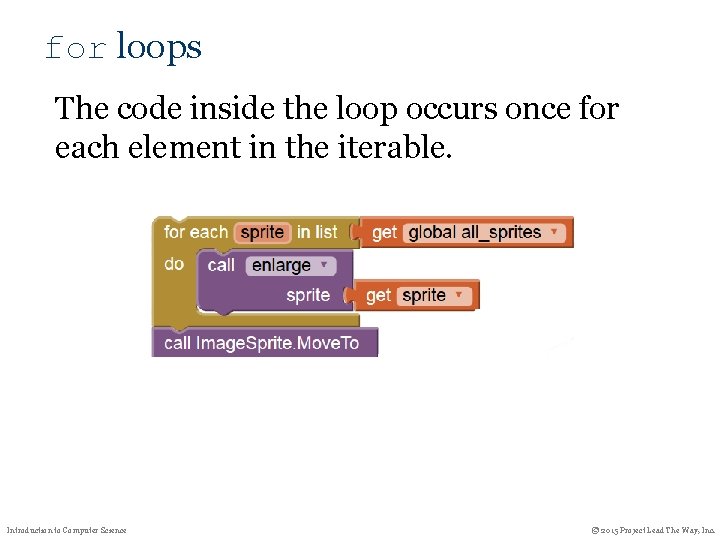
for loops The code inside the loop occurs once for each element in the iterable. Introduction to Computer Science © 2015 Project Lead The Way, Inc.
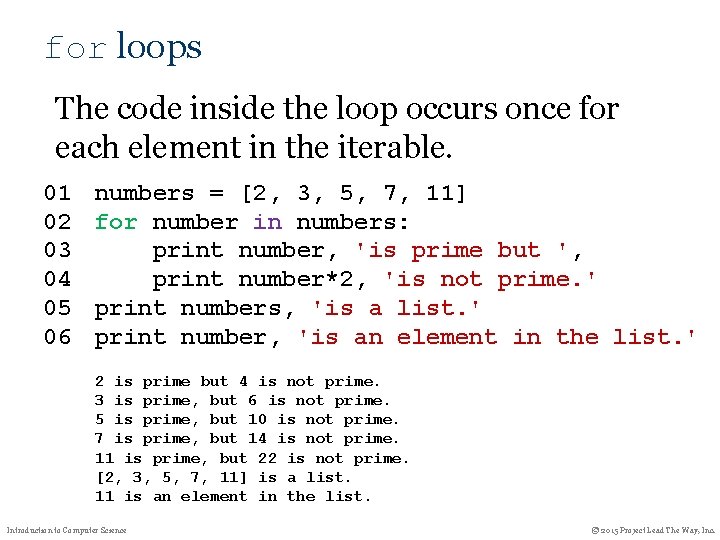
for loops The code inside the loop occurs once for each element in the iterable. 01 02 03 04 05 06 numbers = [2, 3, 5, 7, 11] for number in numbers: print number, 'is prime but ', print number*2, 'is not prime. ' print numbers, 'is a list. ' print number, 'is an element in the list. ' 2 is prime but 4 is not prime. 3 is prime, but 6 is not prime. 5 is prime, but 10 is not prime. 7 is prime, but 14 is not prime. 11 is prime, but 22 is not prime. [2, 3, 5, 7, 11] is a list. 11 is an element in the list. Introduction to Computer Science © 2015 Project Lead The Way, Inc.
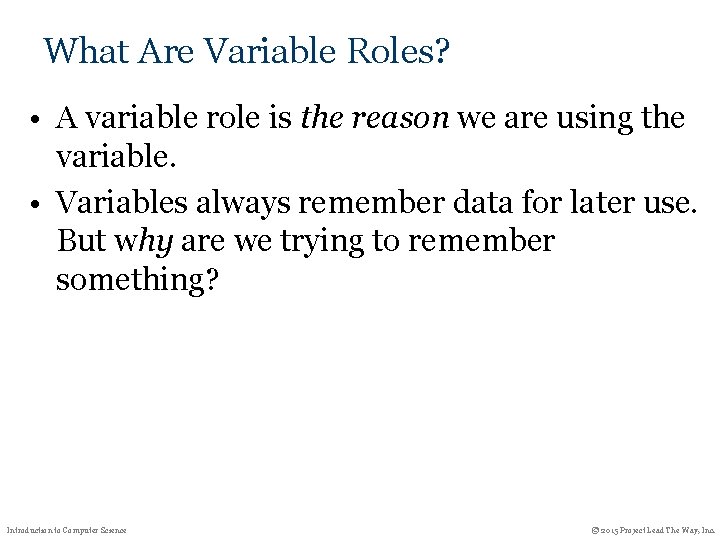
What Are Variable Roles? • A variable role is the reason we are using the variable. • Variables always remember data for later use. But why are we trying to remember something? Introduction to Computer Science © 2015 Project Lead The Way, Inc.
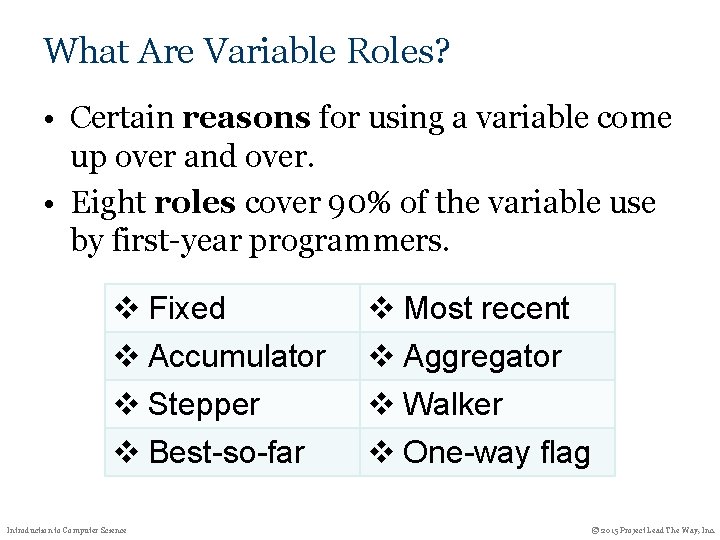
What Are Variable Roles? • Certain reasons for using a variable come up over and over. • Eight roles cover 90% of the variable use by first-year programmers. v Fixed v Accumulator v Stepper v Best-so-far Introduction to Computer Science v Most recent v Aggregator v Walker v One-way flag © 2015 Project Lead The Way, Inc.
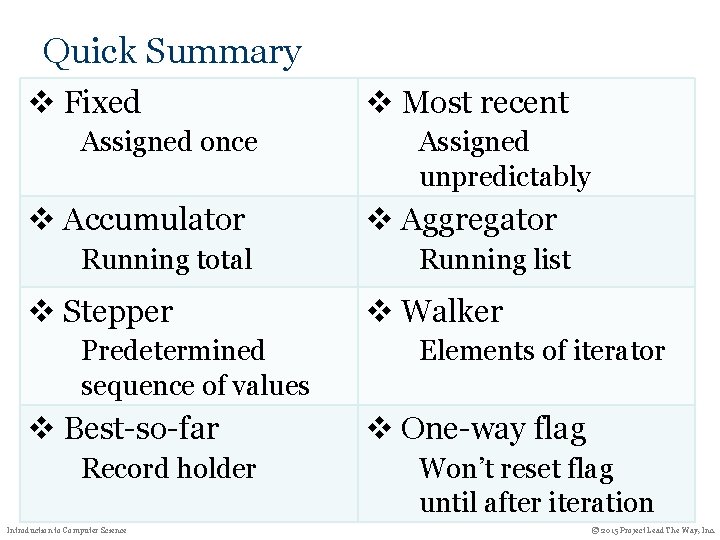
Quick Summary v Fixed Assigned once v Accumulator Running total v Stepper Predetermined sequence of values v Best-so-far Record holder Introduction to Computer Science v Most recent Assigned unpredictably v Aggregator Running list v Walker Elements of iterator v One-way flag Won’t reset flag until after iteration © 2015 Project Lead The Way, Inc.
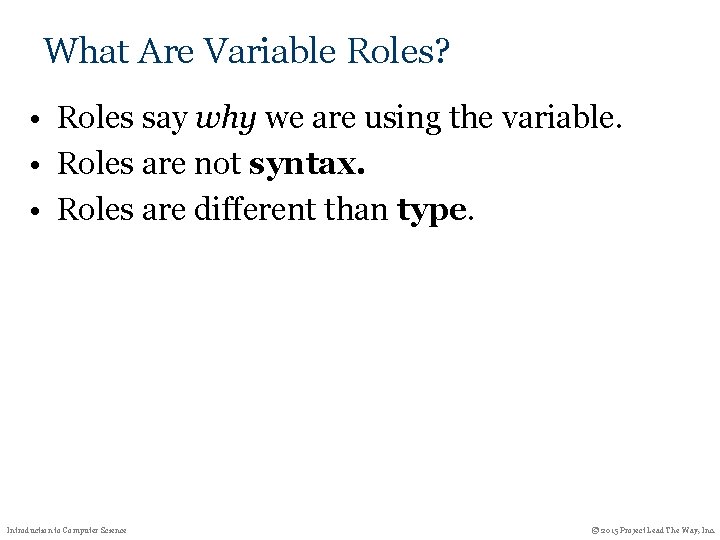
What Are Variable Roles? • Roles say why we are using the variable. • Roles are not syntax. • Roles are different than type. Introduction to Computer Science © 2015 Project Lead The Way, Inc.
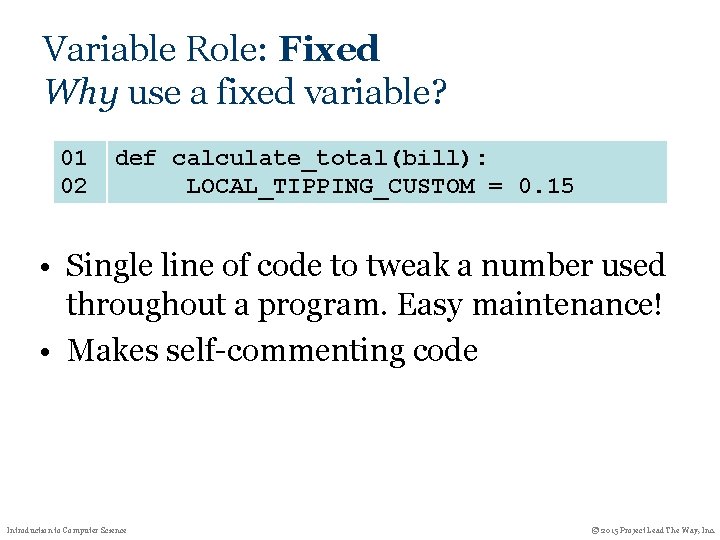
Variable Role: Fixed Why use a fixed variable? 01 02 def calculate_total(bill): LOCAL_TIPPING_CUSTOM = 0. 15 • Single line of code to tweak a number used throughout a program. Easy maintenance! • Makes self-commenting code Introduction to Computer Science © 2015 Project Lead The Way, Inc.
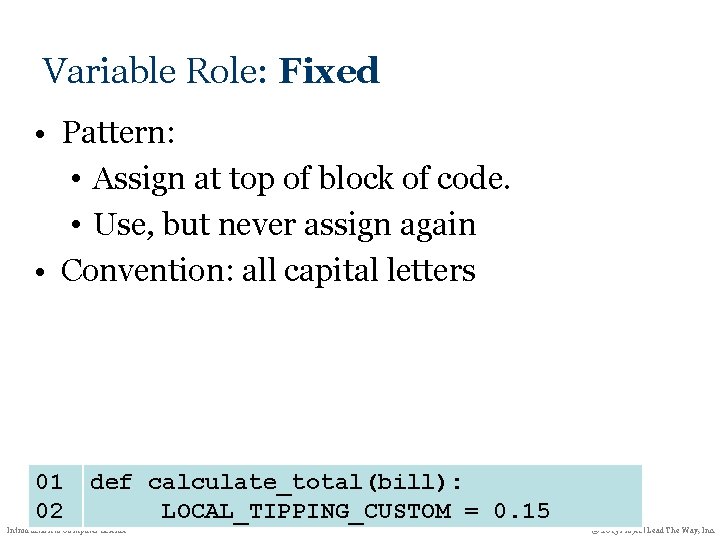
Variable Role: Fixed • Pattern: • Assign at top of block of code. • Use, but never assign again • Convention: all capital letters 01 02 def calculate_total(bill): LOCAL_TIPPING_CUSTOM = 0. 15 Introduction to Computer Science © 2015 Project Lead The Way, Inc.
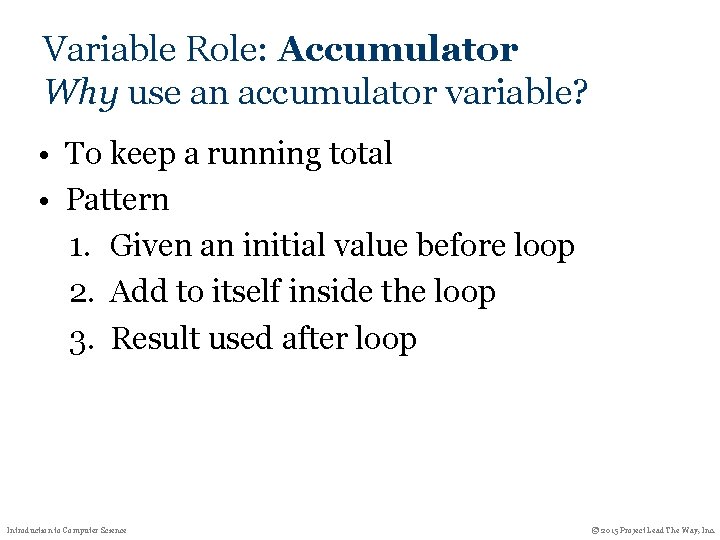
Variable Role: Accumulator Why use an accumulator variable? • To keep a running total • Pattern 1. Given an initial value before loop 2. Add to itself inside the loop 3. Result used after loop Introduction to Computer Science © 2015 Project Lead The Way, Inc.
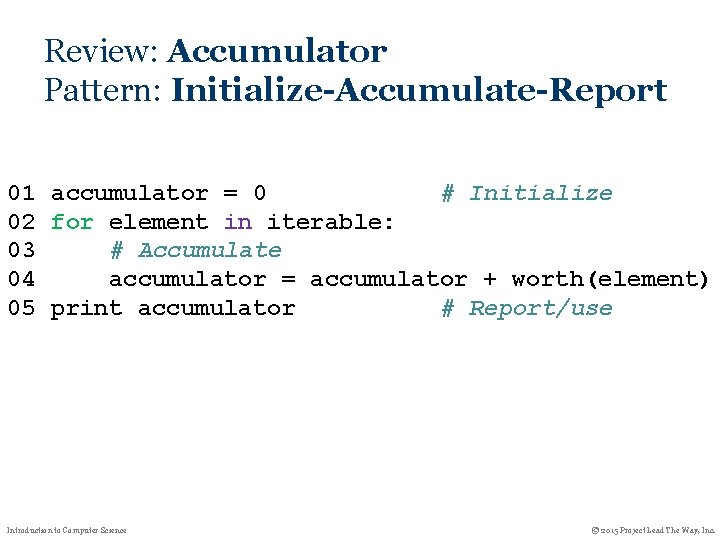
Review: Accumulator Pattern: Initialize-Accumulate-Report 01 accumulator = 0 # Initialize 02 for element in iterable: 03 # Accumulate 04 accumulator = accumulator + worth(element) 05 print accumulator # Report/use Introduction to Computer Science © 2015 Project Lead The Way, Inc.
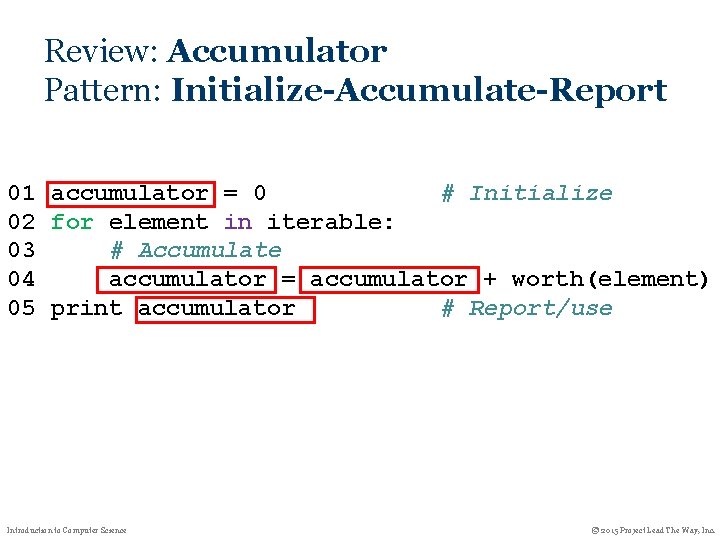
Review: Accumulator Pattern: Initialize-Accumulate-Report 01 accumulator = 0 # Initialize 02 for element in iterable: 03 # Accumulate 04 accumulator = accumulator + worth(element) 05 print accumulator # Report/use Introduction to Computer Science © 2015 Project Lead The Way, Inc.
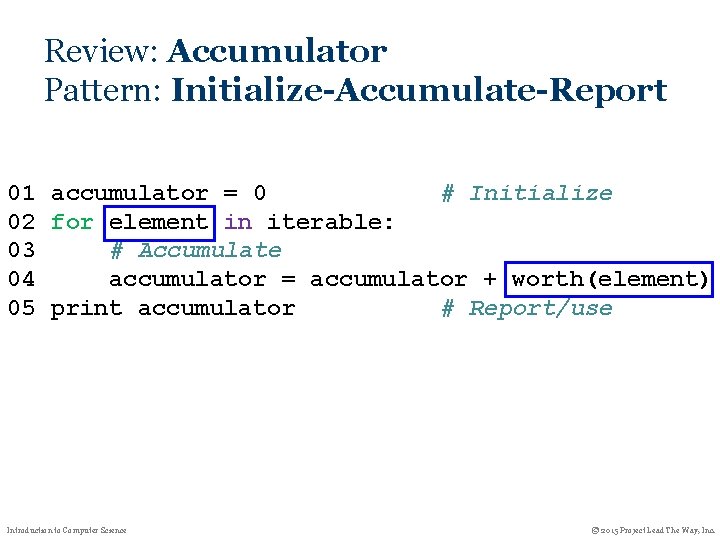
Review: Accumulator Pattern: Initialize-Accumulate-Report 01 accumulator = 0 # Initialize 02 for element in iterable: 03 # Accumulate 04 accumulator = accumulator + worth(element) 05 print accumulator # Report/use Introduction to Computer Science © 2015 Project Lead The Way, Inc.
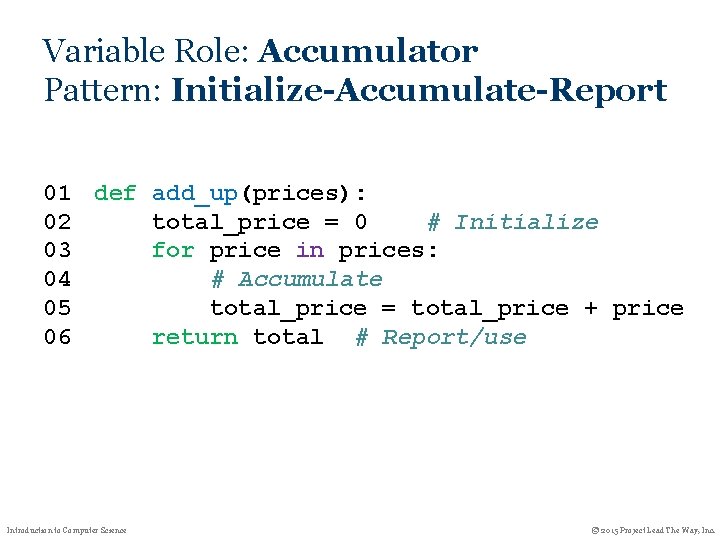
Variable Role: Accumulator Pattern: Initialize-Accumulate-Report 01 def add_up(prices): 02 total_price = 0 # Initialize 03 for price in prices: 04 # Accumulate 05 total_price = total_price + price 06 return total # Report/use Introduction to Computer Science © 2015 Project Lead The Way, Inc.
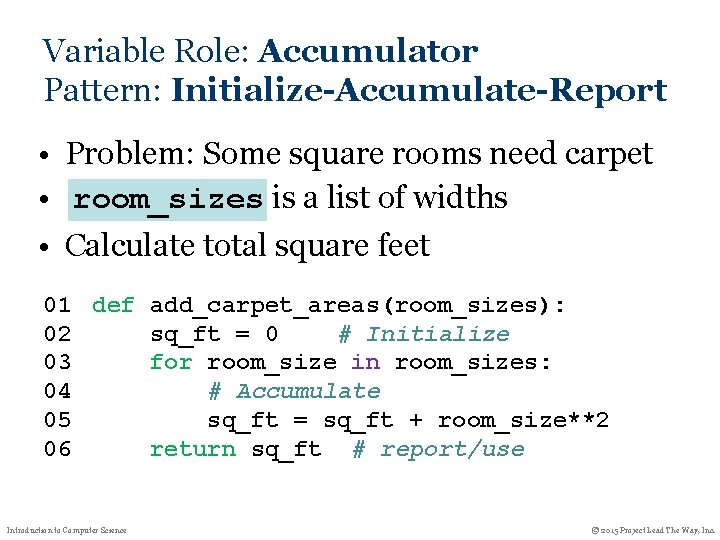
Variable Role: Accumulator Pattern: Initialize-Accumulate-Report • Problem: Some square rooms need carpet • room_sizes is a list of widths • Calculate total square feet 01 def add_carpet_areas(room_sizes): 02 sq_ft = 0 # Initialize 03 for room_size in room_sizes: 04 # Accumulate 05 sq_ft = sq_ft + room_size**2 06 return sq_ft # report/use Introduction to Computer Science © 2015 Project Lead The Way, Inc.
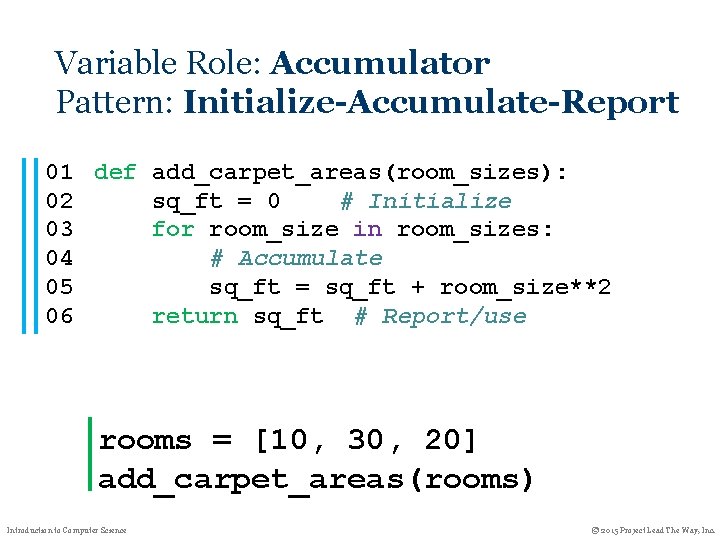
Variable Role: Accumulator Pattern: Initialize-Accumulate-Report 01 def add_carpet_areas(room_sizes): 02 sq_ft = 0 # Initialize 03 for room_size in room_sizes: 04 # Accumulate 05 sq_ft = sq_ft + room_size**2 06 return sq_ft # Report/use rooms = [10, 30, 20] add_carpet_areas(rooms) Introduction to Computer Science © 2015 Project Lead The Way, Inc.
Loops controlled by an accumulator
Accumulator function in microprocessor
Value creation value delivery value capture
Constant pointer and pointer to constant
Pointer of pointer in c
9 pointers
Metals tend to be
Equilibrium occurs when
Rate constant vs equilibrium constant
Formation constant vs equilibrium constant
Pointer constant in c
Constant pointer and pointer to constant
Formation constant vs equilibrium constant
Specific cake resistance definition
Unit of r
Variance expected value
Value of rydberg constant
Rydberg constant value