FILES Files are accessed using the following statement
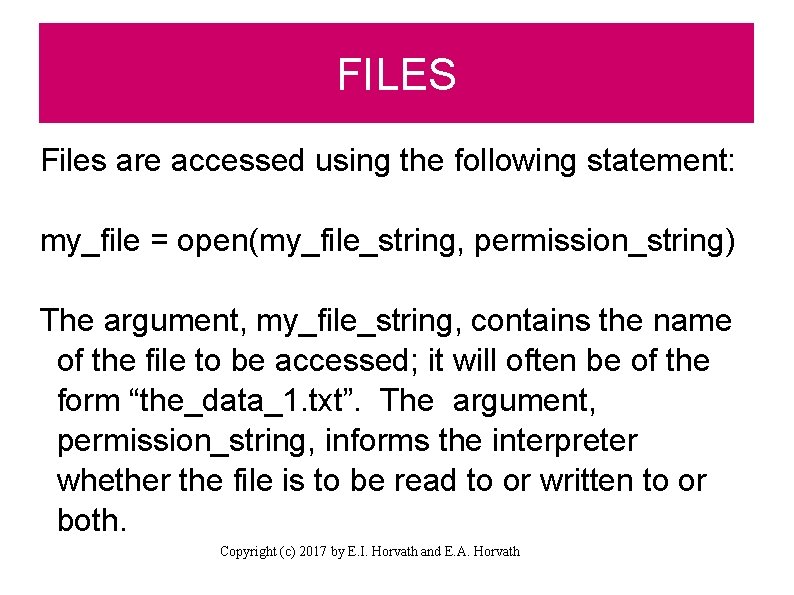
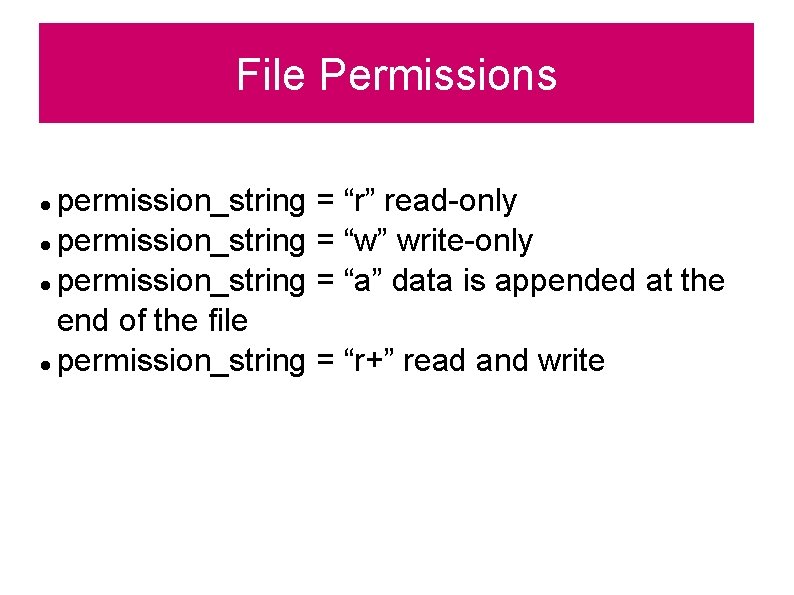
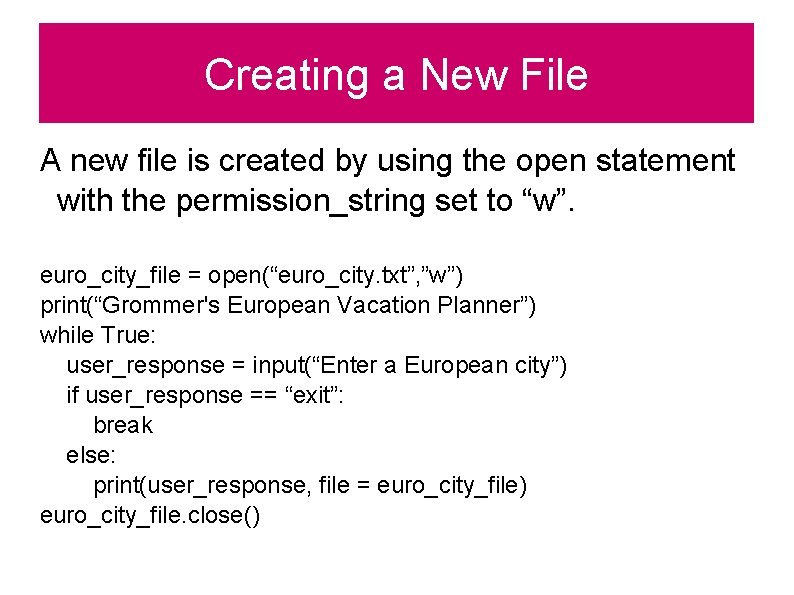
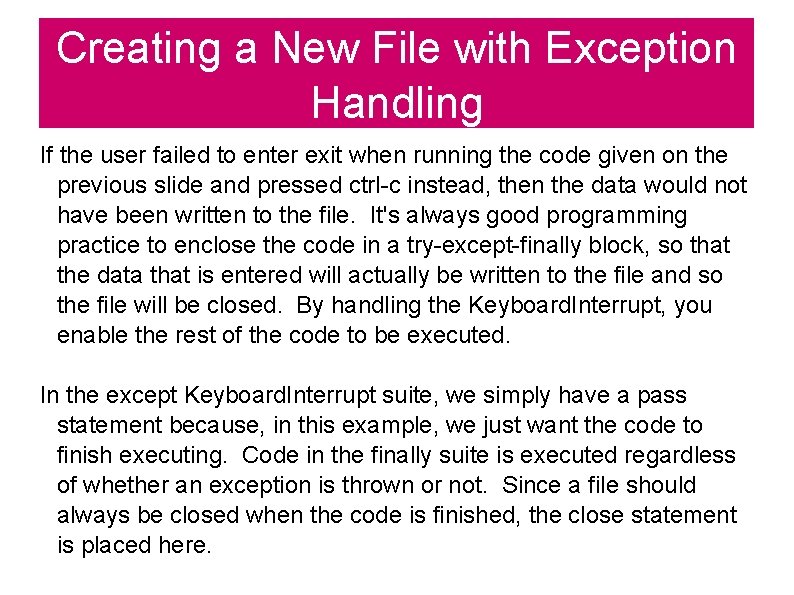
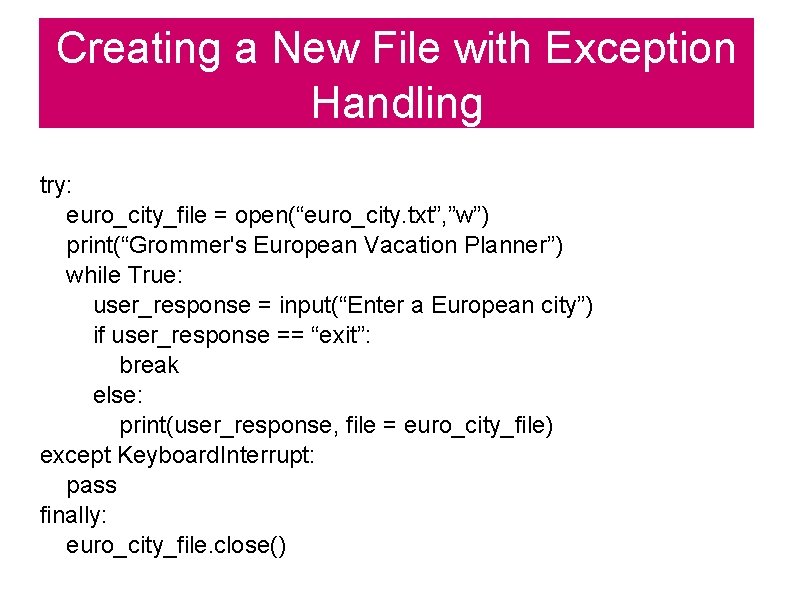
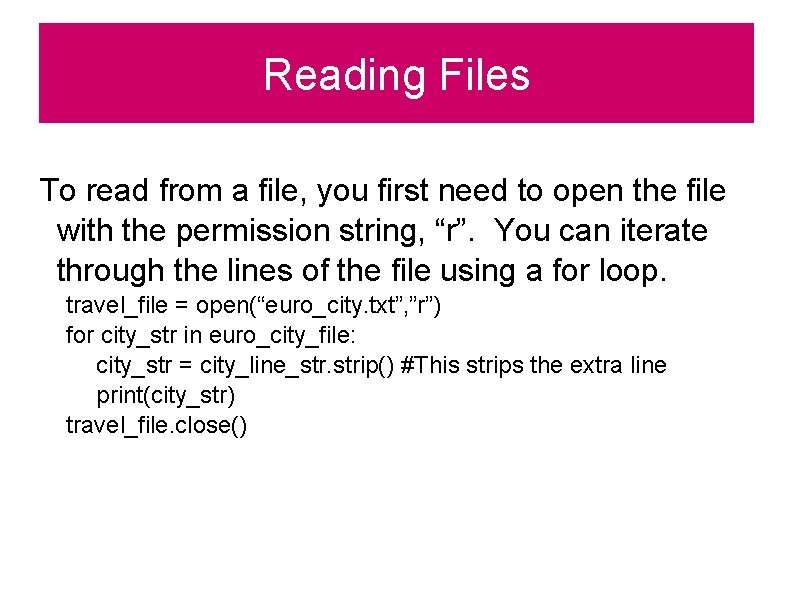
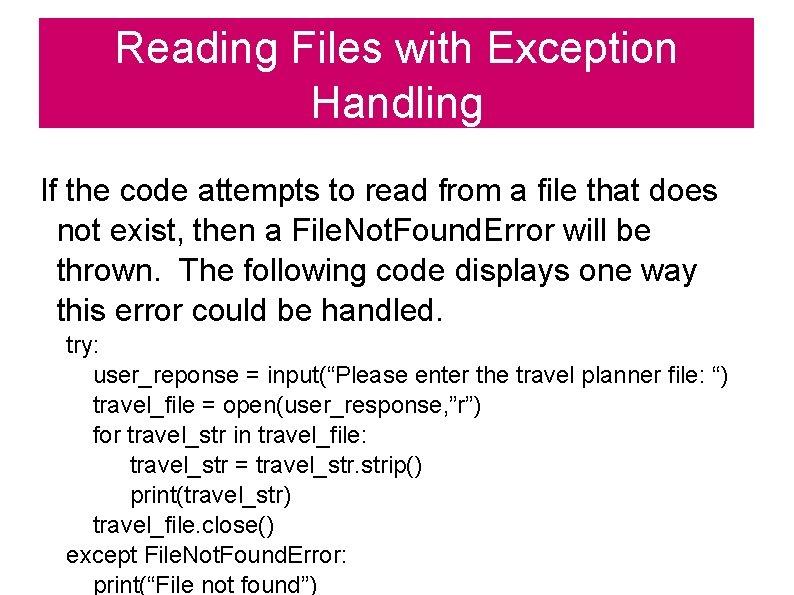
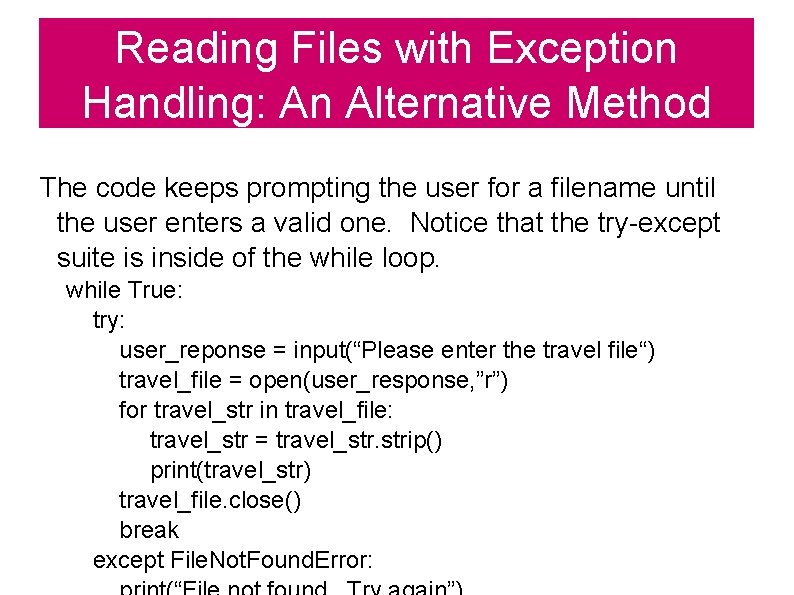
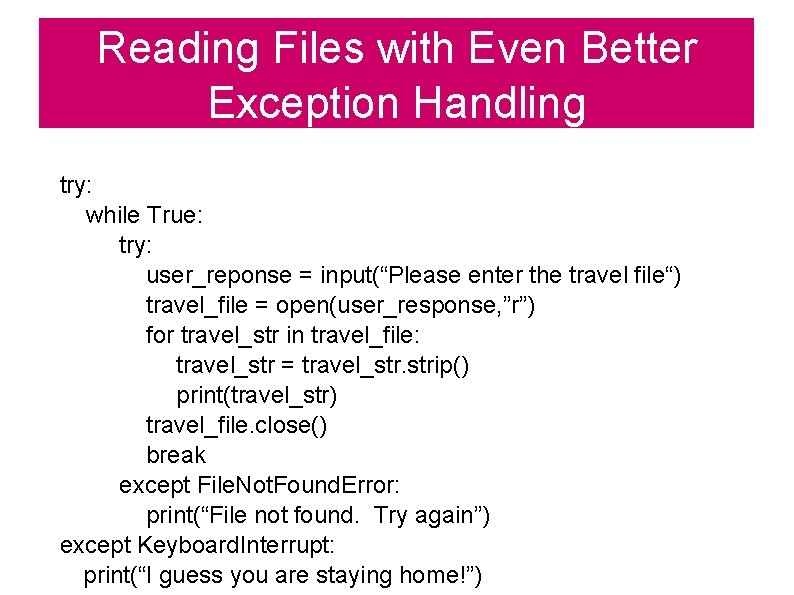
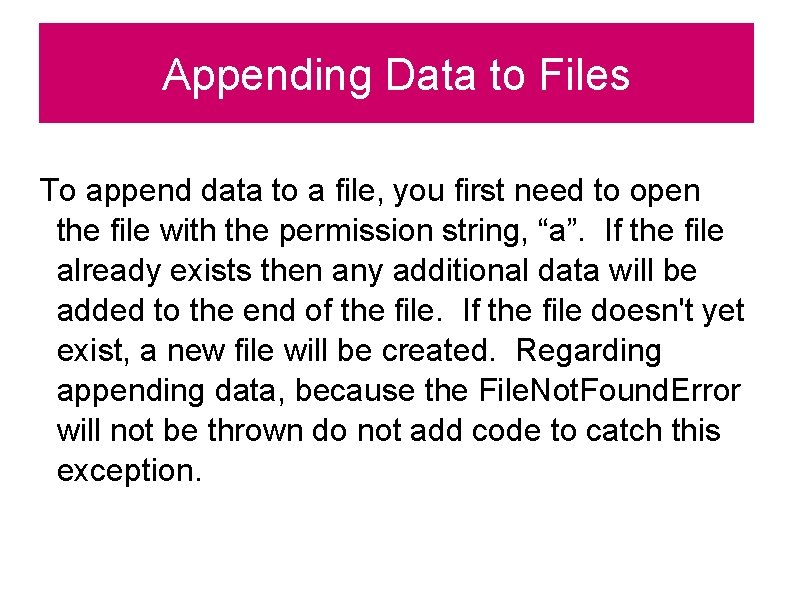
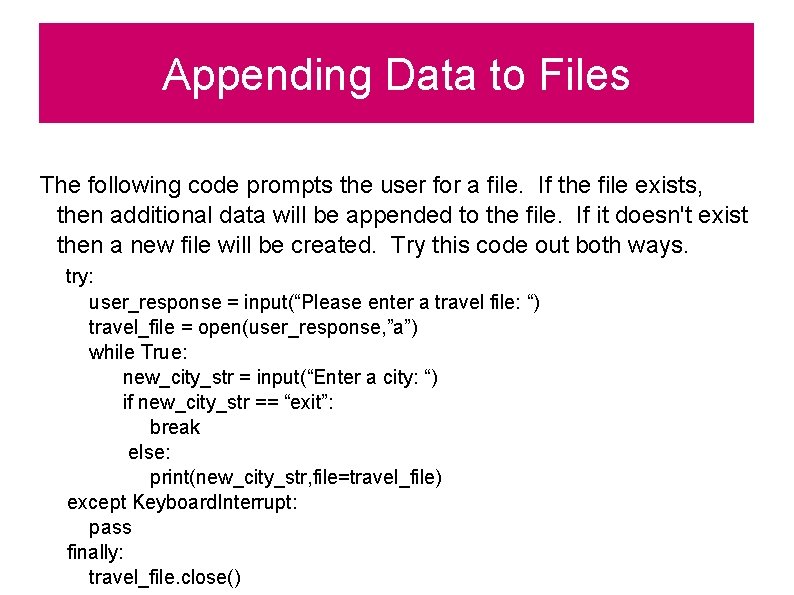
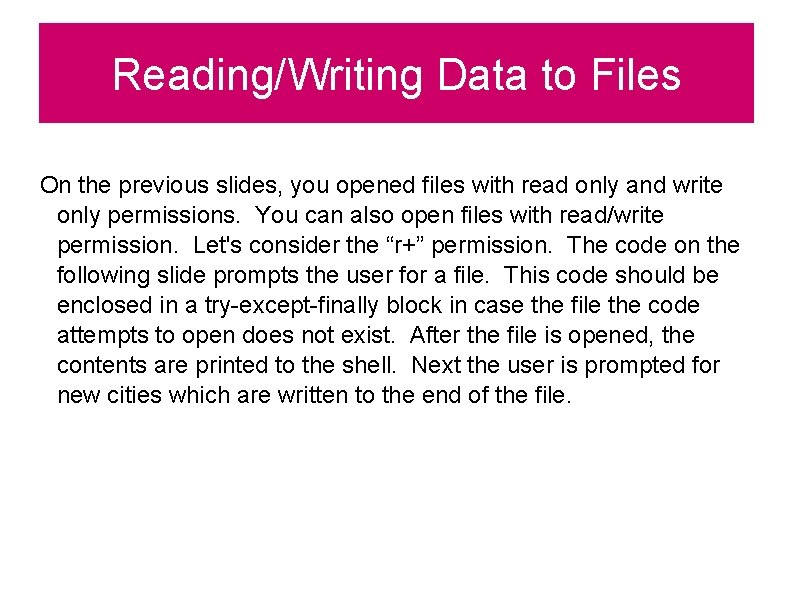
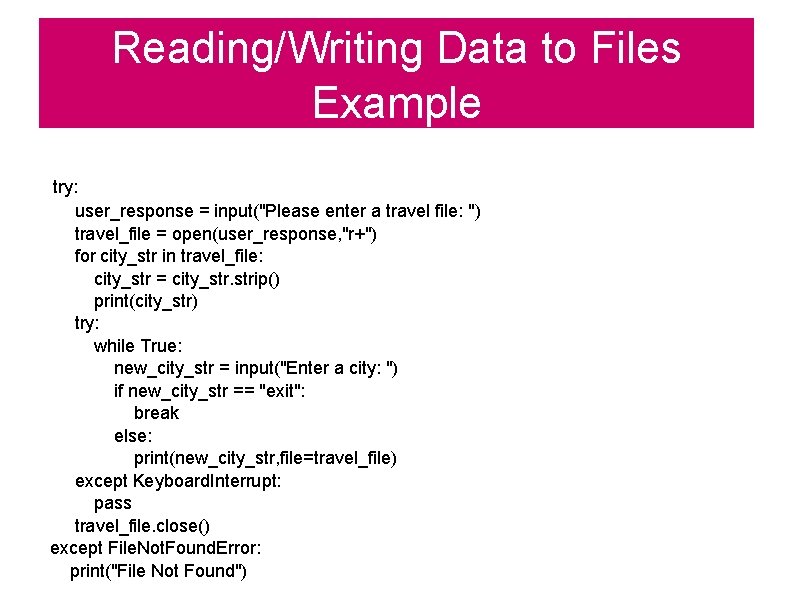
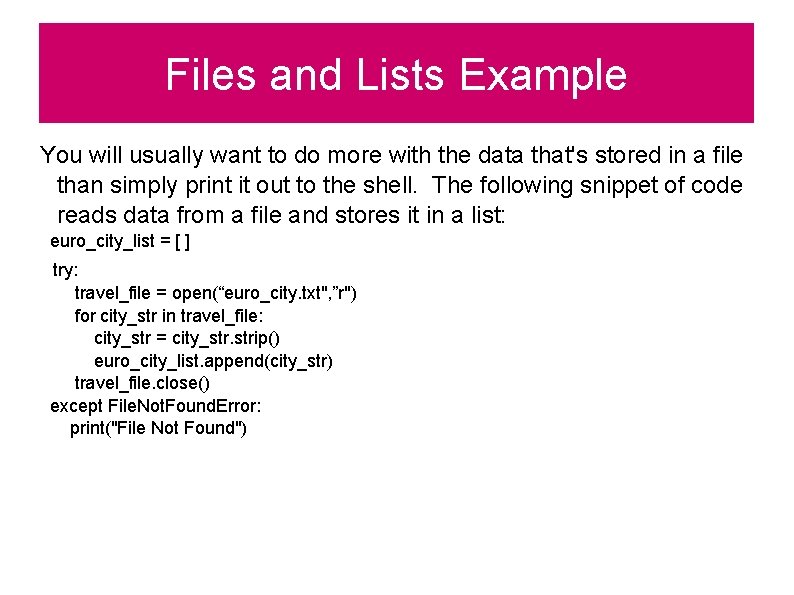
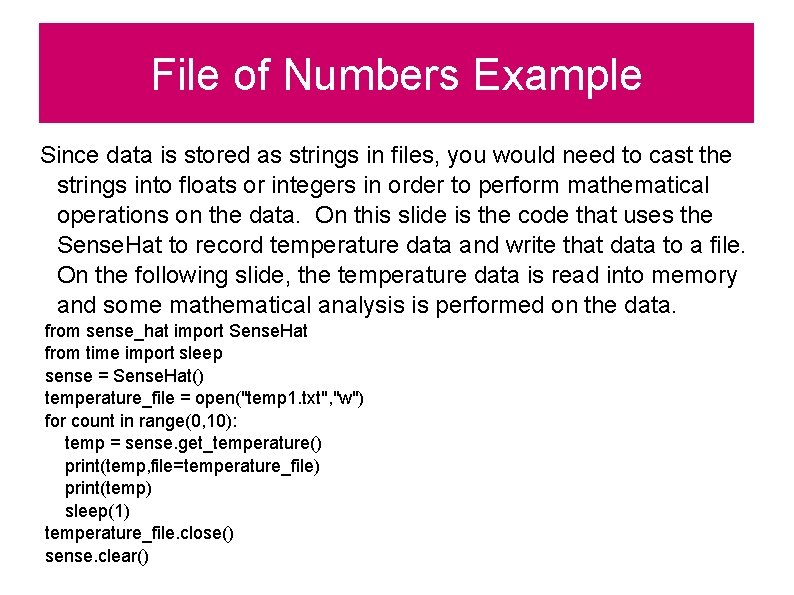
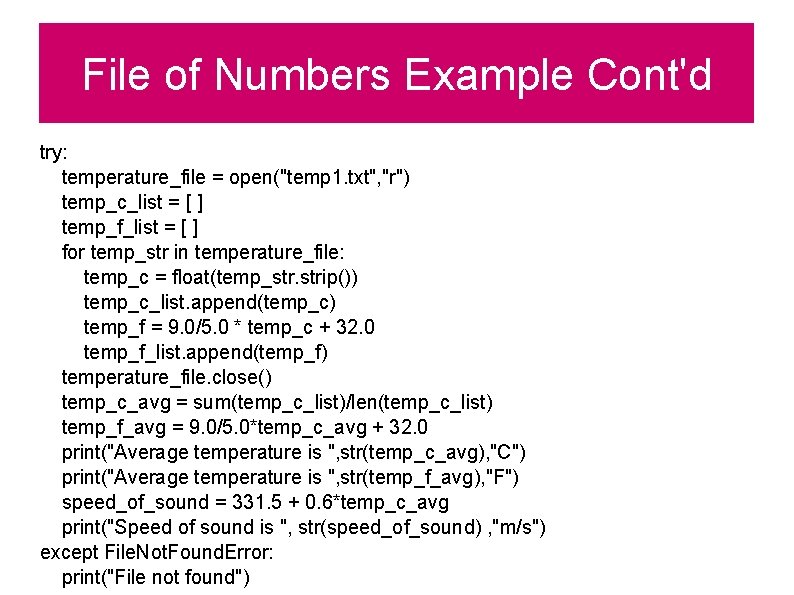
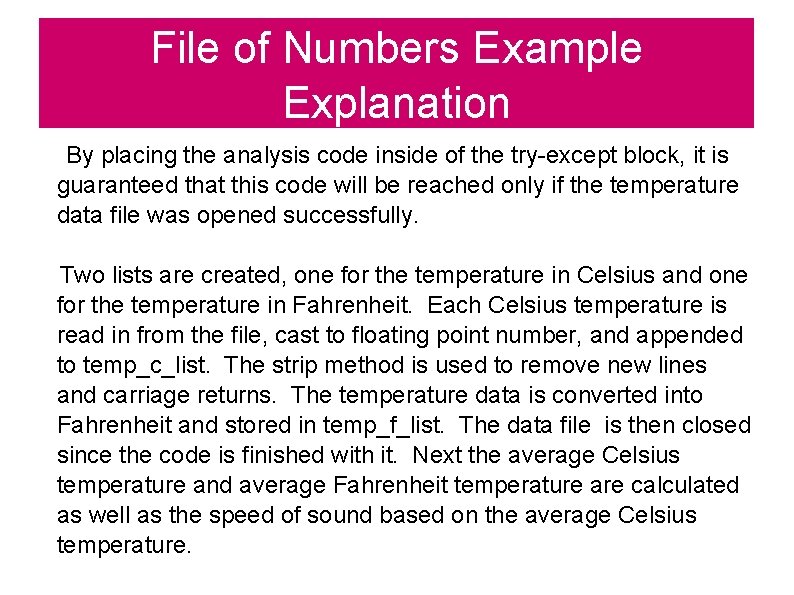
- Slides: 17
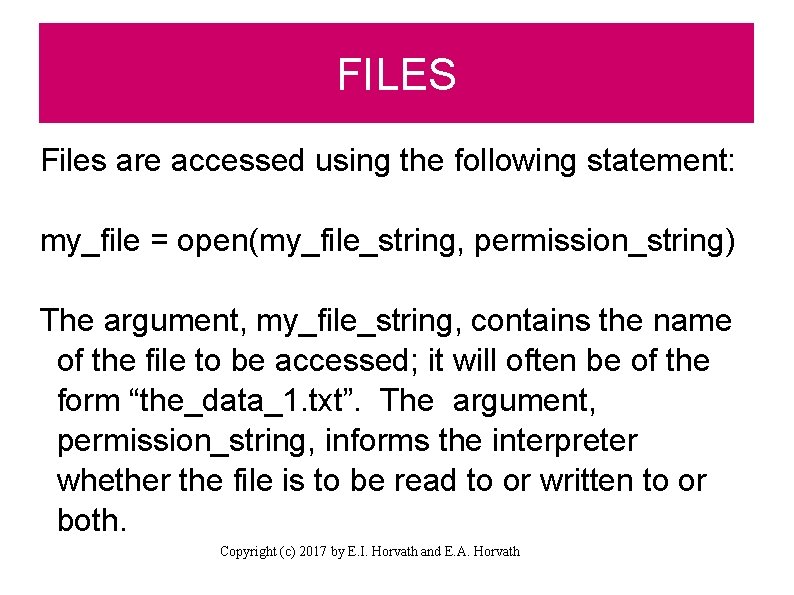
FILES Files are accessed using the following statement: my_file = open(my_file_string, permission_string) The argument, my_file_string, contains the name of the file to be accessed; it will often be of the form “the_data_1. txt”. The argument, permission_string, informs the interpreter whether the file is to be read to or written to or both. Copyright (c) 2017 by E. I. Horvath and E. A. Horvath
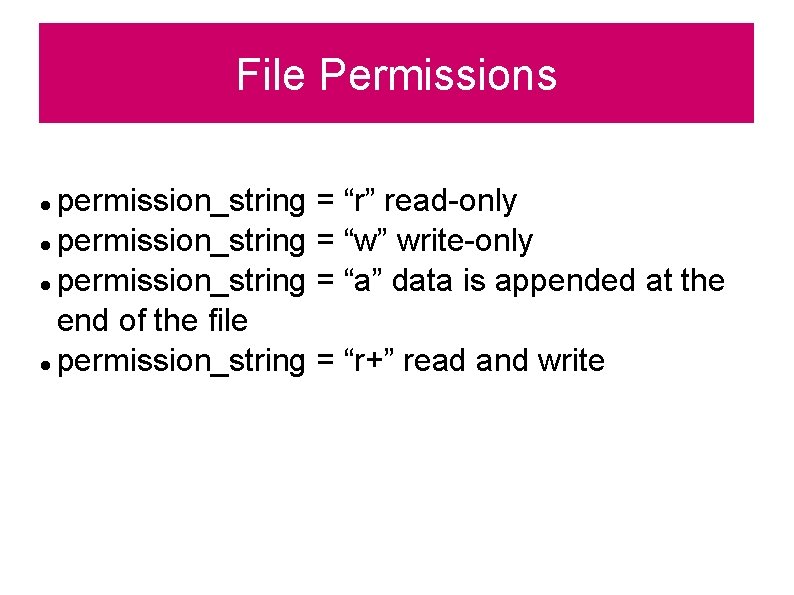
File Permissions permission_string = “r” read-only permission_string = “w” write-only permission_string = “a” data is appended at the end of the file permission_string = “r+” read and write
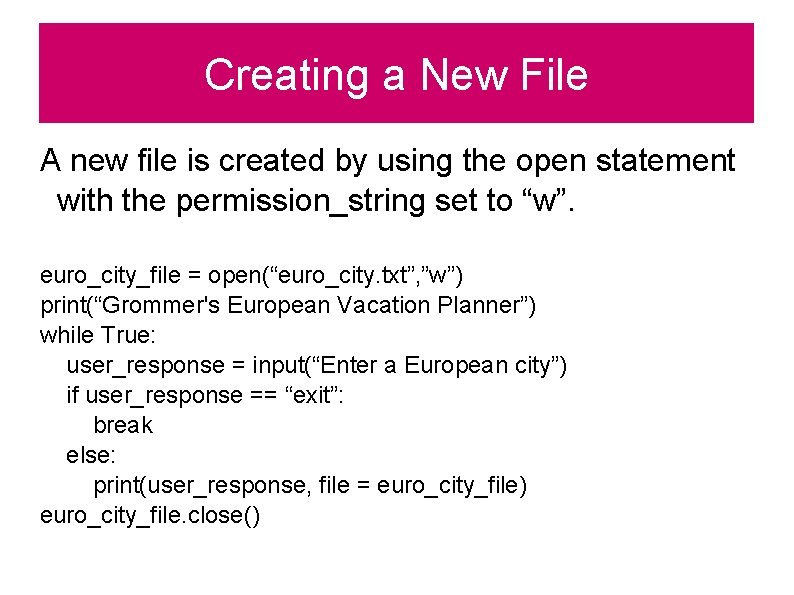
Creating a New File A new file is created by using the open statement with the permission_string set to “w”. euro_city_file = open(“euro_city. txt”, ”w”) print(“Grommer's European Vacation Planner”) while True: user_response = input(“Enter a European city”) if user_response == “exit”: break else: print(user_response, file = euro_city_file) euro_city_file. close()
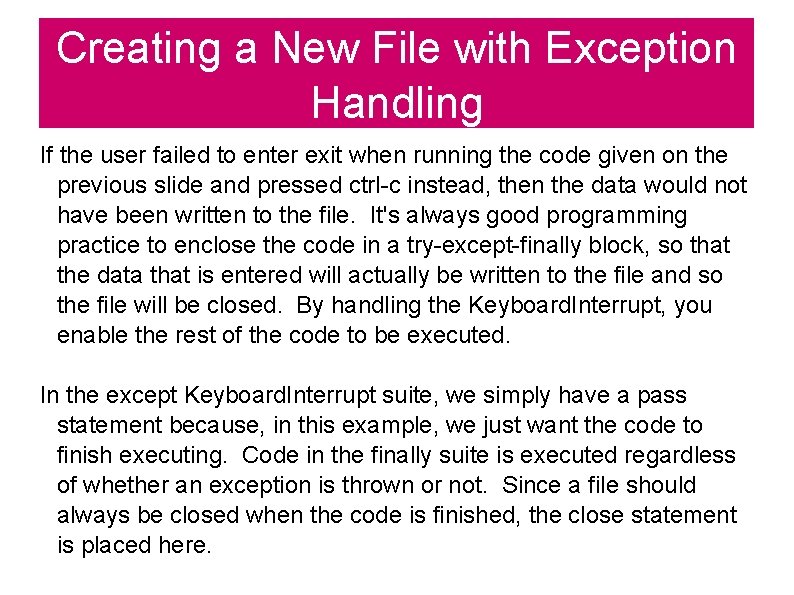
Creating a New File with Exception Handling If the user failed to enter exit when running the code given on the previous slide and pressed ctrl-c instead, then the data would not have been written to the file. It's always good programming practice to enclose the code in a try-except-finally block, so that the data that is entered will actually be written to the file and so the file will be closed. By handling the Keyboard. Interrupt, you enable the rest of the code to be executed. In the except Keyboard. Interrupt suite, we simply have a pass statement because, in this example, we just want the code to finish executing. Code in the finally suite is executed regardless of whether an exception is thrown or not. Since a file should always be closed when the code is finished, the close statement is placed here.
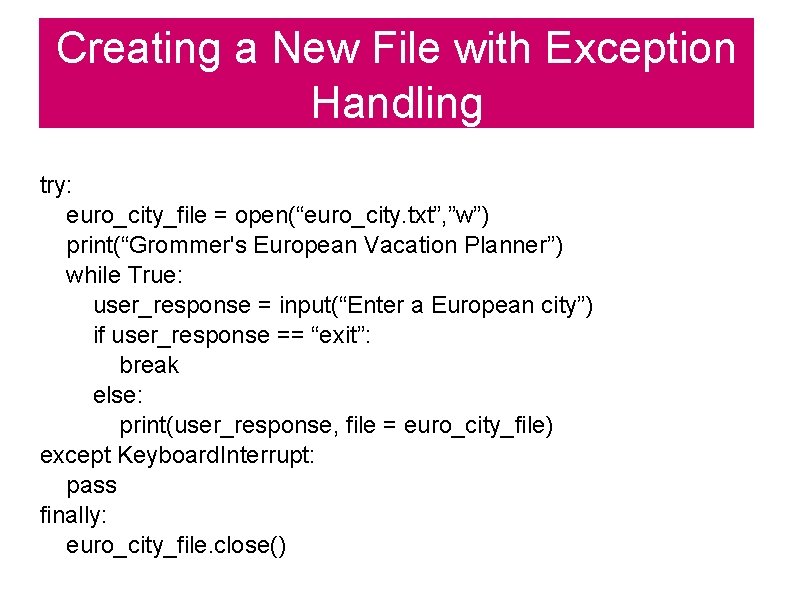
Creating a New File with Exception Handling try: euro_city_file = open(“euro_city. txt”, ”w”) print(“Grommer's European Vacation Planner”) while True: user_response = input(“Enter a European city”) if user_response == “exit”: break else: print(user_response, file = euro_city_file) except Keyboard. Interrupt: pass finally: euro_city_file. close()
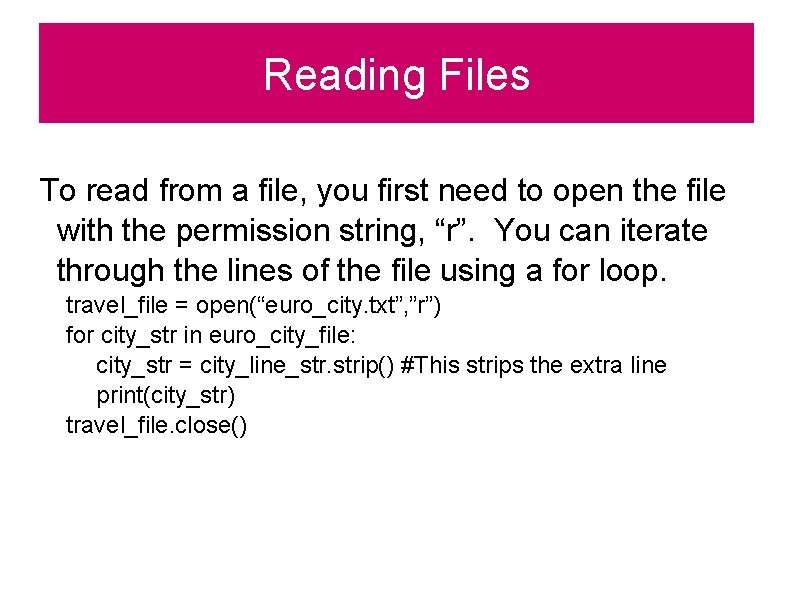
Reading Files To read from a file, you first need to open the file with the permission string, “r”. You can iterate through the lines of the file using a for loop. travel_file = open(“euro_city. txt”, ”r”) for city_str in euro_city_file: city_str = city_line_str. strip() #This strips the extra line print(city_str) travel_file. close()
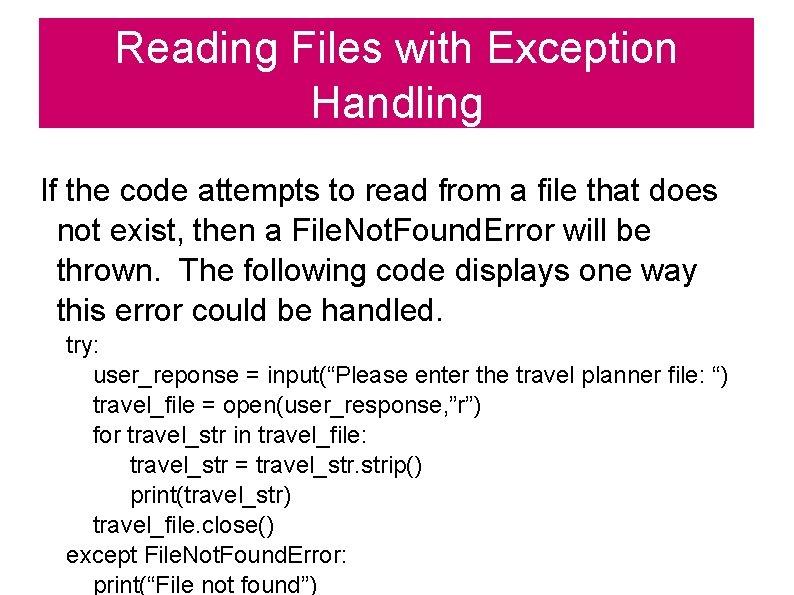
Reading Files with Exception Handling If the code attempts to read from a file that does not exist, then a File. Not. Found. Error will be thrown. The following code displays one way this error could be handled. try: user_reponse = input(“Please enter the travel planner file: “) travel_file = open(user_response, ”r”) for travel_str in travel_file: travel_str = travel_str. strip() print(travel_str) travel_file. close() except File. Not. Found. Error: print(“File not found”)
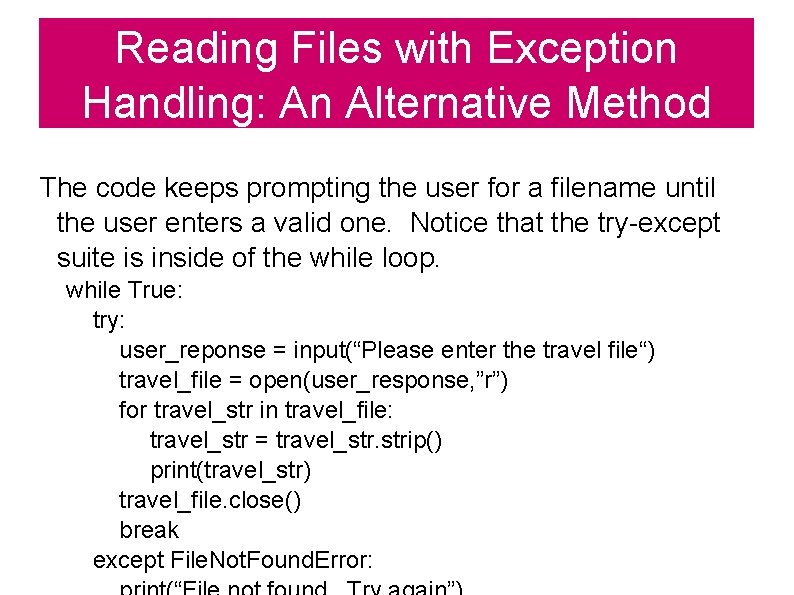
Reading Files with Exception Handling: An Alternative Method The code keeps prompting the user for a filename until the user enters a valid one. Notice that the try-except suite is inside of the while loop. while True: try: user_reponse = input(“Please enter the travel file“) travel_file = open(user_response, ”r”) for travel_str in travel_file: travel_str = travel_str. strip() print(travel_str) travel_file. close() break except File. Not. Found. Error:
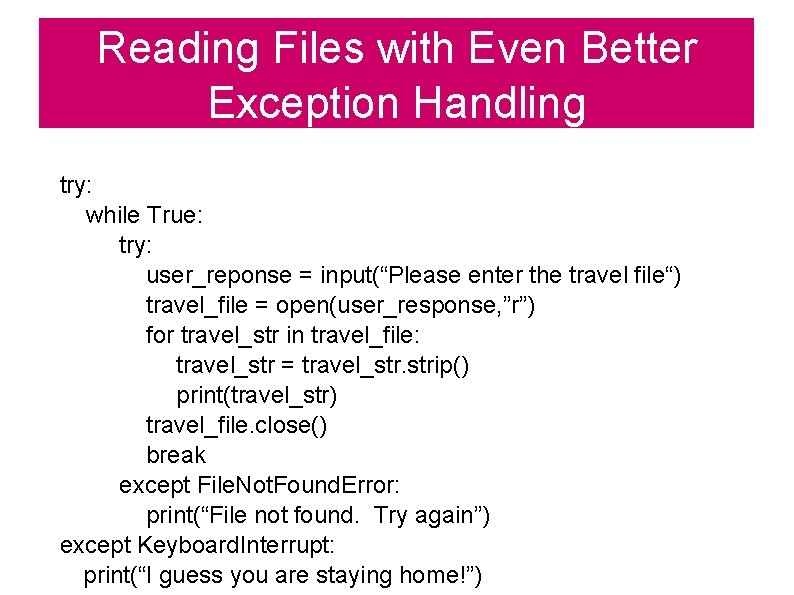
Reading Files with Even Better Exception Handling try: while True: try: user_reponse = input(“Please enter the travel file“) travel_file = open(user_response, ”r”) for travel_str in travel_file: travel_str = travel_str. strip() print(travel_str) travel_file. close() break except File. Not. Found. Error: print(“File not found. Try again”) except Keyboard. Interrupt: print(“I guess you are staying home!”)
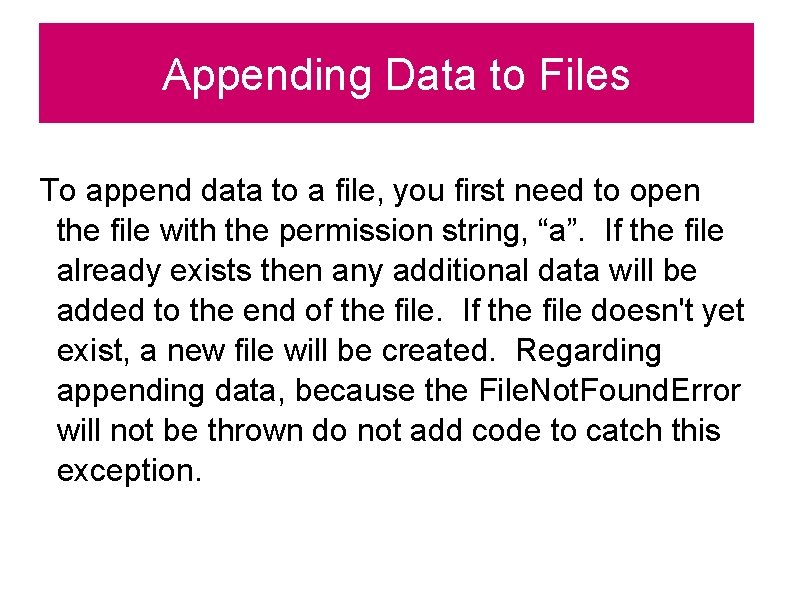
Appending Data to Files To append data to a file, you first need to open the file with the permission string, “a”. If the file already exists then any additional data will be added to the end of the file. If the file doesn't yet exist, a new file will be created. Regarding appending data, because the File. Not. Found. Error will not be thrown do not add code to catch this exception.
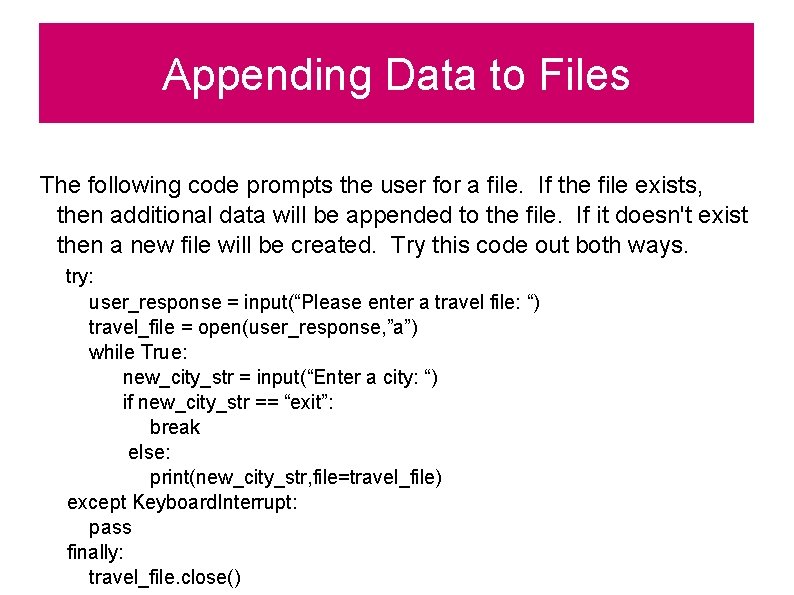
Appending Data to Files The following code prompts the user for a file. If the file exists, then additional data will be appended to the file. If it doesn't exist then a new file will be created. Try this code out both ways. try: user_response = input(“Please enter a travel file: “) travel_file = open(user_response, ”a”) while True: new_city_str = input(“Enter a city: “) if new_city_str == “exit”: break else: print(new_city_str, file=travel_file) except Keyboard. Interrupt: pass finally: travel_file. close()
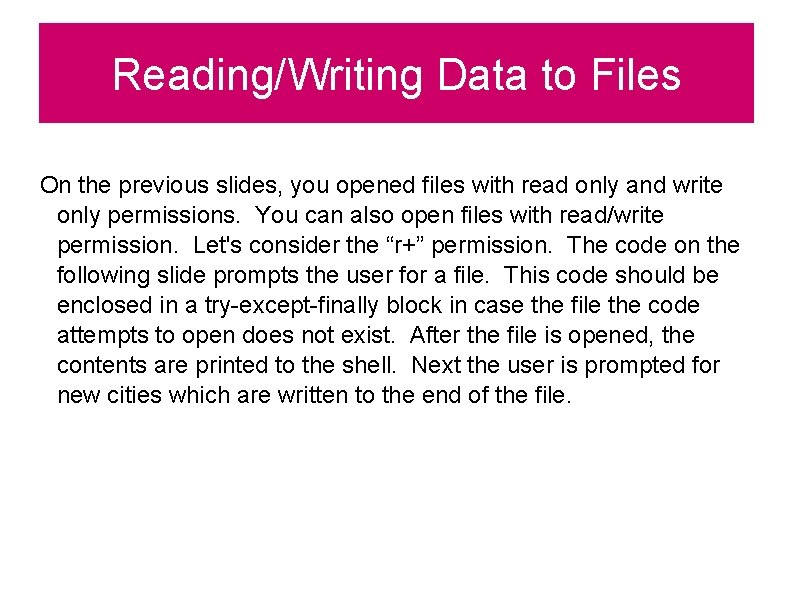
Reading/Writing Data to Files On the previous slides, you opened files with read only and write only permissions. You can also open files with read/write permission. Let's consider the “r+” permission. The code on the following slide prompts the user for a file. This code should be enclosed in a try-except-finally block in case the file the code attempts to open does not exist. After the file is opened, the contents are printed to the shell. Next the user is prompted for new cities which are written to the end of the file.
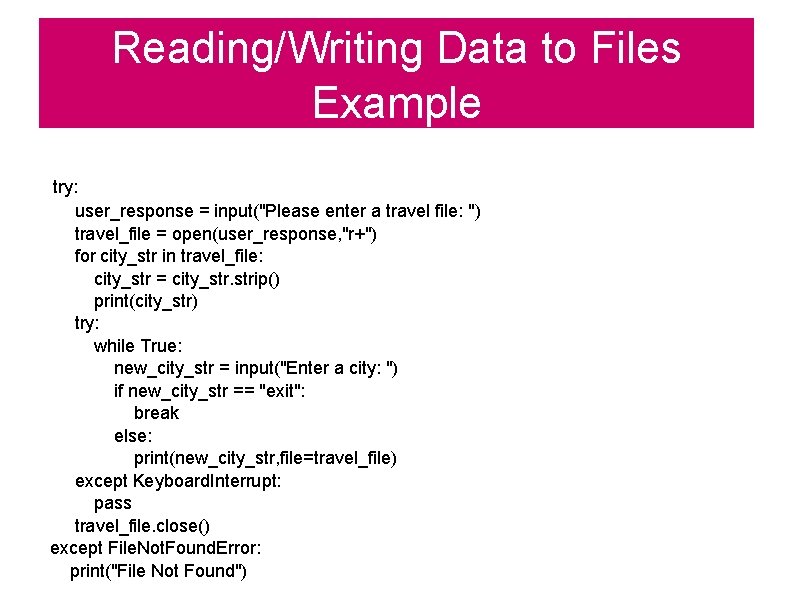
Reading/Writing Data to Files Example try: user_response = input("Please enter a travel file: ") travel_file = open(user_response, "r+") for city_str in travel_file: city_str = city_str. strip() print(city_str) try: while True: new_city_str = input("Enter a city: ") if new_city_str == "exit": break else: print(new_city_str, file=travel_file) except Keyboard. Interrupt: pass travel_file. close() except File. Not. Found. Error: print("File Not Found")
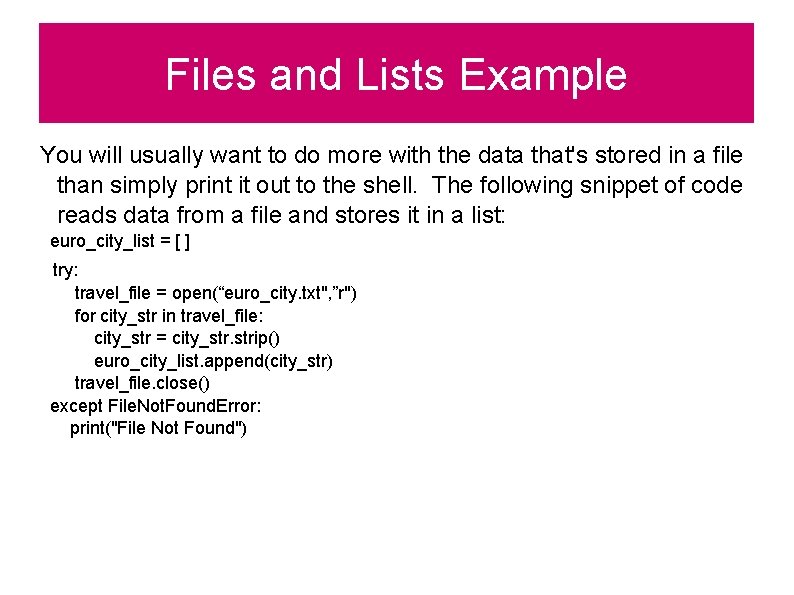
Files and Lists Example You will usually want to do more with the data that's stored in a file than simply print it out to the shell. The following snippet of code reads data from a file and stores it in a list: euro_city_list = [ ] try: travel_file = open(“euro_city. txt", ”r") for city_str in travel_file: city_str = city_str. strip() euro_city_list. append(city_str) travel_file. close() except File. Not. Found. Error: print("File Not Found")
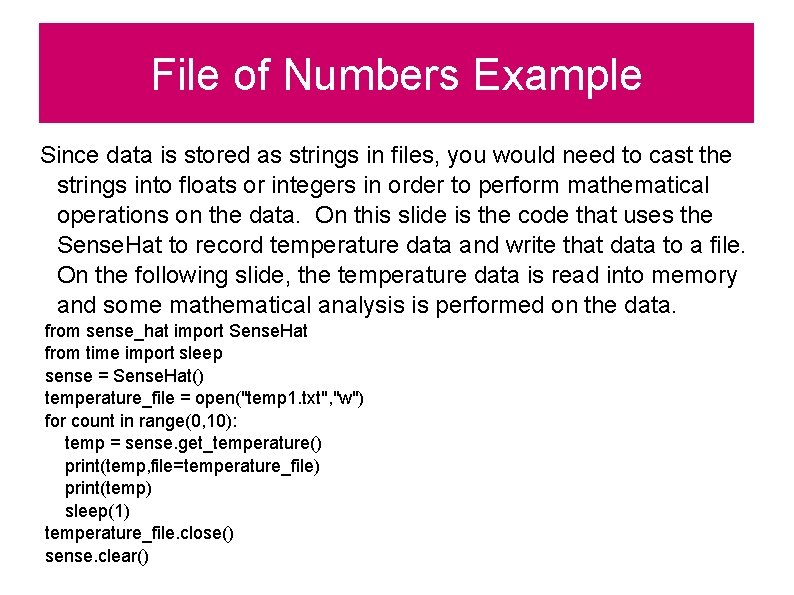
File of Numbers Example Since data is stored as strings in files, you would need to cast the strings into floats or integers in order to perform mathematical operations on the data. On this slide is the code that uses the Sense. Hat to record temperature data and write that data to a file. On the following slide, the temperature data is read into memory and some mathematical analysis is performed on the data. from sense_hat import Sense. Hat from time import sleep sense = Sense. Hat() temperature_file = open("temp 1. txt", "w") for count in range(0, 10): temp = sense. get_temperature() print(temp, file=temperature_file) print(temp) sleep(1) temperature_file. close() sense. clear()
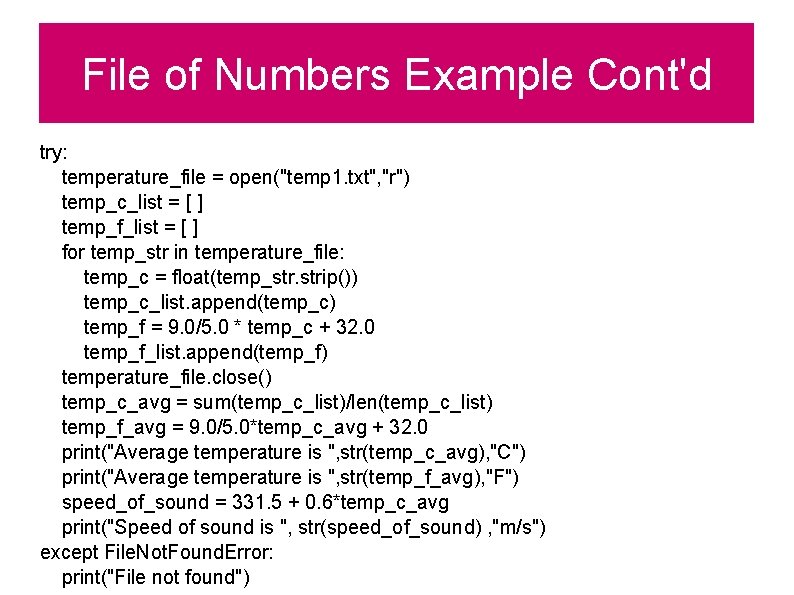
File of Numbers Example Cont'd try: temperature_file = open("temp 1. txt", "r") temp_c_list = [ ] temp_f_list = [ ] for temp_str in temperature_file: temp_c = float(temp_str. strip()) temp_c_list. append(temp_c) temp_f = 9. 0/5. 0 * temp_c + 32. 0 temp_f_list. append(temp_f) temperature_file. close() temp_c_avg = sum(temp_c_list)/len(temp_c_list) temp_f_avg = 9. 0/5. 0*temp_c_avg + 32. 0 print("Average temperature is ", str(temp_c_avg), "C") print("Average temperature is ", str(temp_f_avg), "F") speed_of_sound = 331. 5 + 0. 6*temp_c_avg print("Speed of sound is ", str(speed_of_sound) , "m/s") except File. Not. Found. Error: print("File not found")
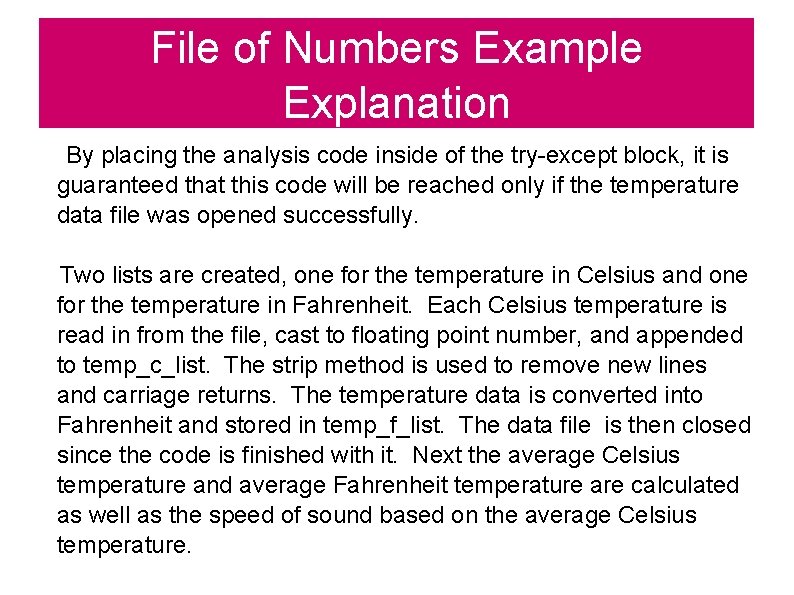
File of Numbers Example Explanation By placing the analysis code inside of the try-except block, it is guaranteed that this code will be reached only if the temperature data file was opened successfully. Two lists are created, one for the temperature in Celsius and one for the temperature in Fahrenheit. Each Celsius temperature is read in from the file, cast to floating point number, and appended to temp_c_list. The strip method is used to remove new lines and carriage returns. The temperature data is converted into Fahrenheit and stored in temp_f_list. The data file is then closed since the code is finished with it. Next the average Celsius temperature and average Fahrenheit temperature are calculated as well as the speed of sound based on the average Celsius temperature.
Insidan region jh
Array element is accessed using
File mode python
Cjis policy and security awareness test
Cjis meaning
An access specifier indicates how a class may be accessed.
How is the medisoft report designer accessed
Operating system definition
Pyright self is not accessed
Hát kết hợp bộ gõ cơ thể
Ng-html
Bổ thể
Tỉ lệ cơ thể trẻ em
Chó sói
Tư thế worm breton
Chúa sống lại
Các môn thể thao bắt đầu bằng tiếng chạy
Thế nào là hệ số cao nhất