Data Structures Get your data structures correct first
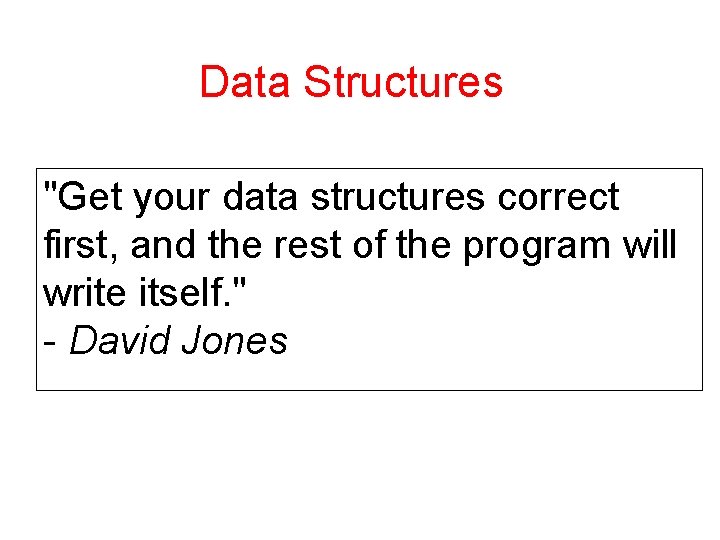
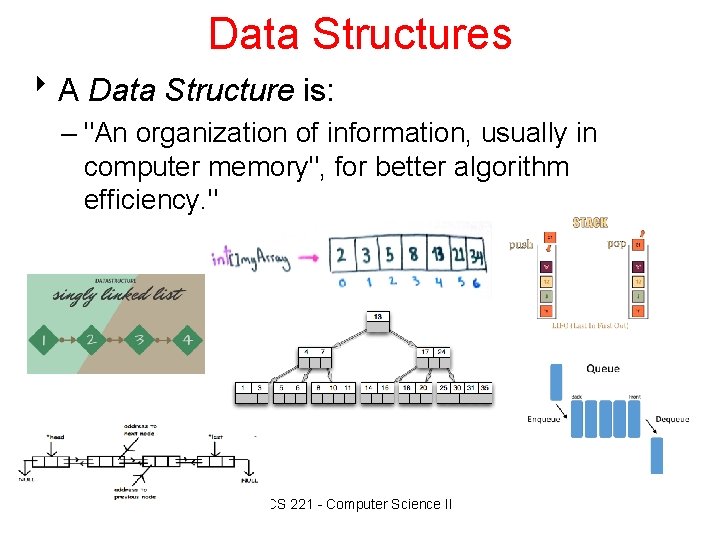
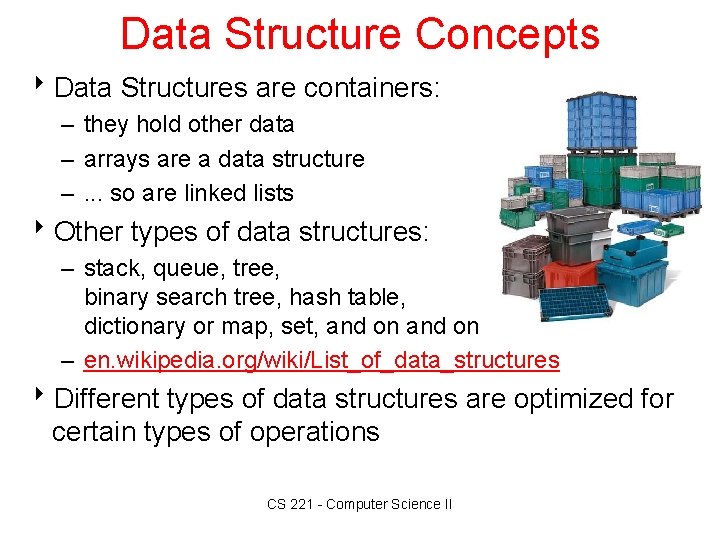
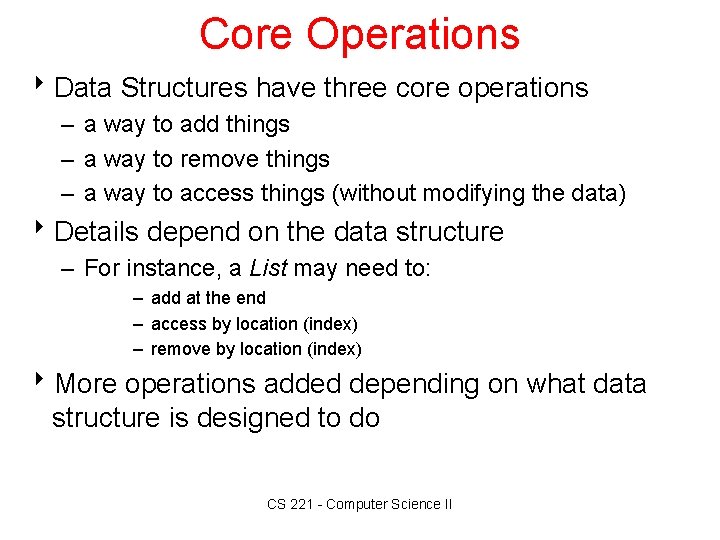
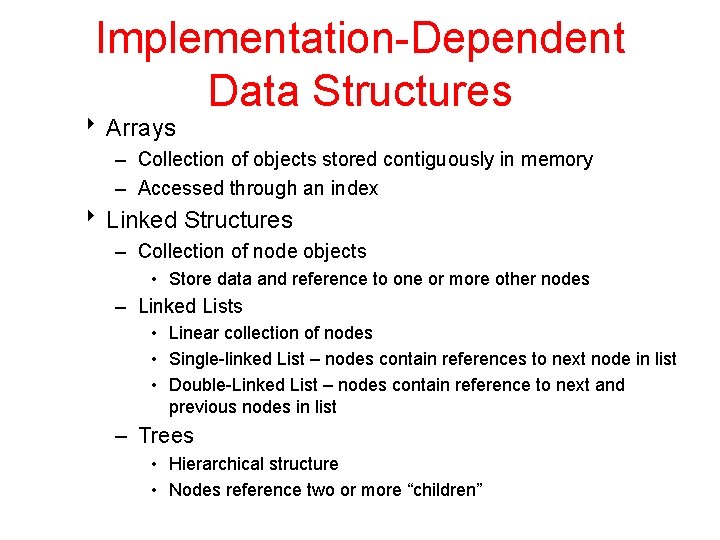
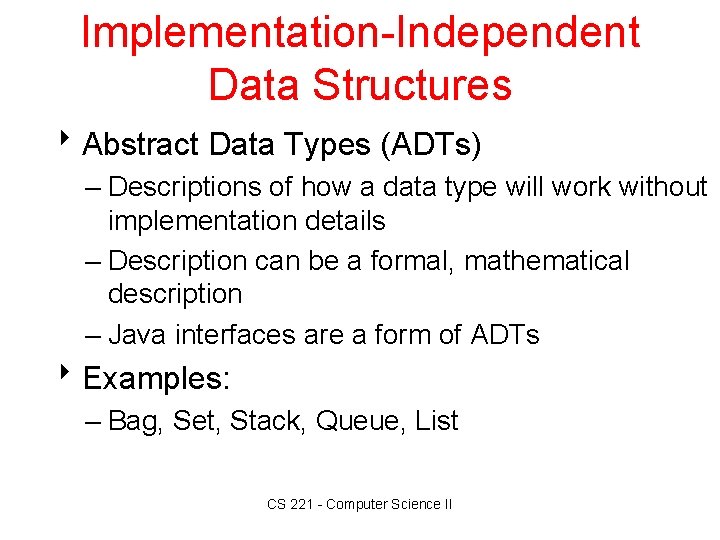
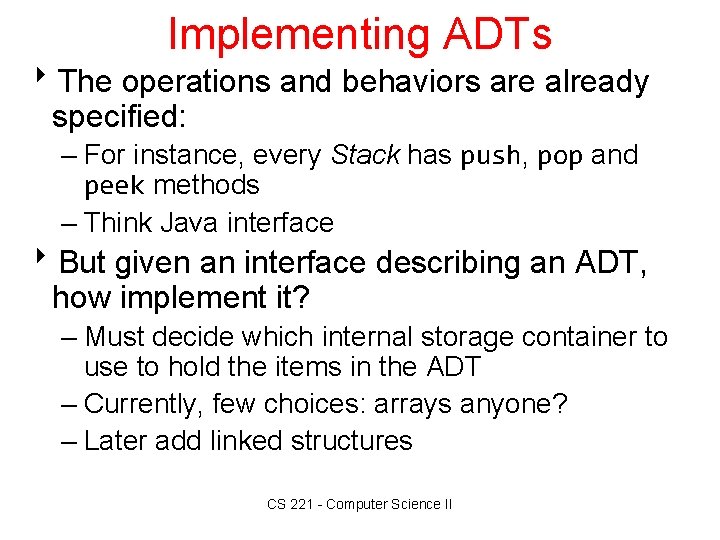
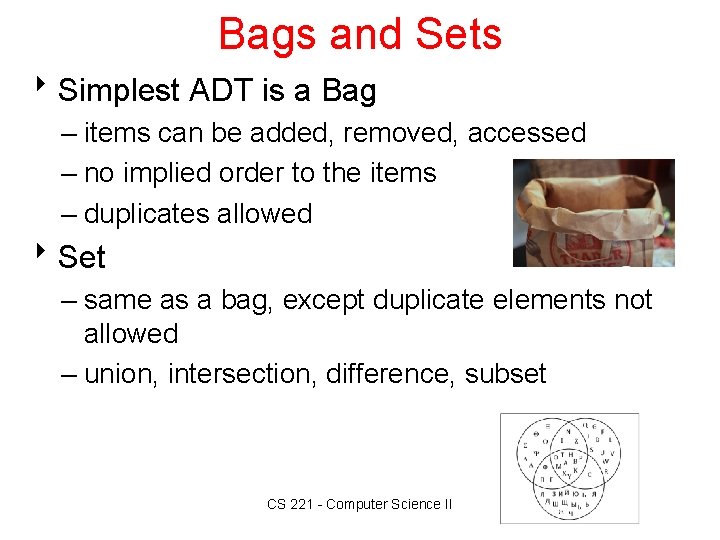
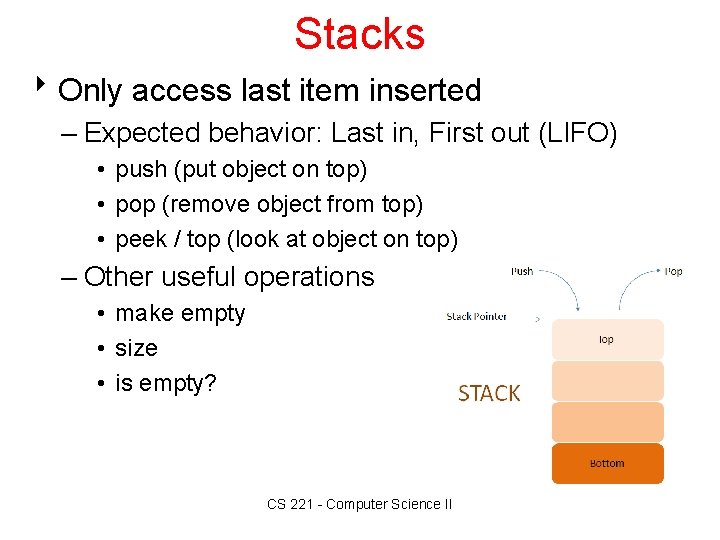
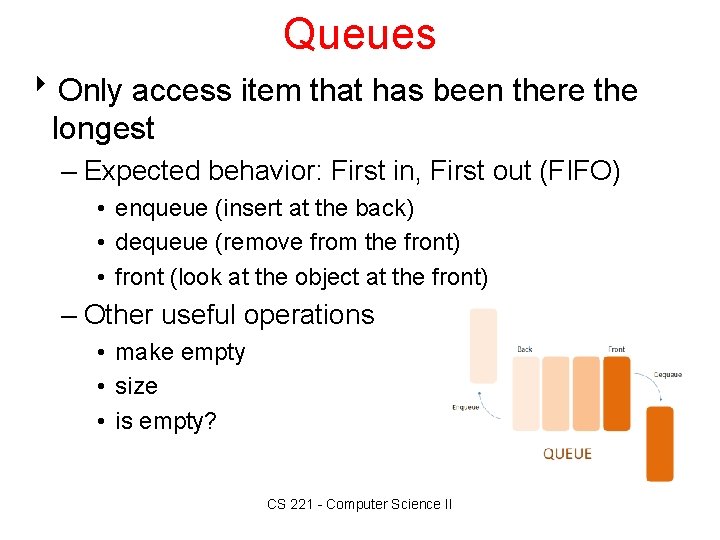
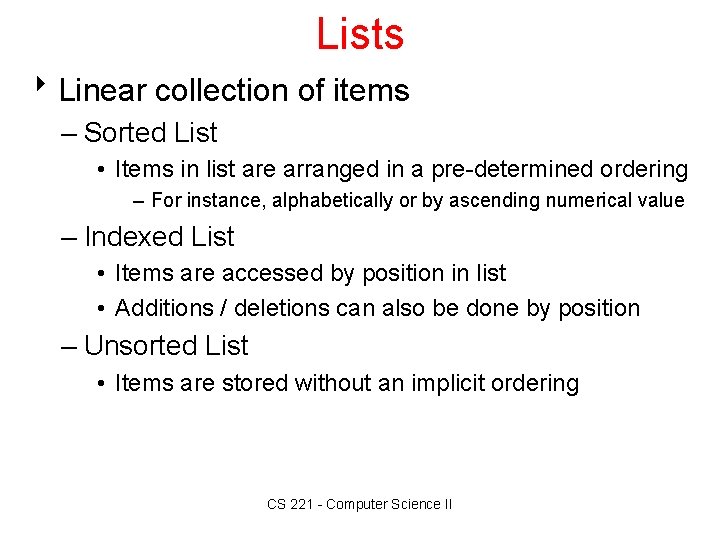
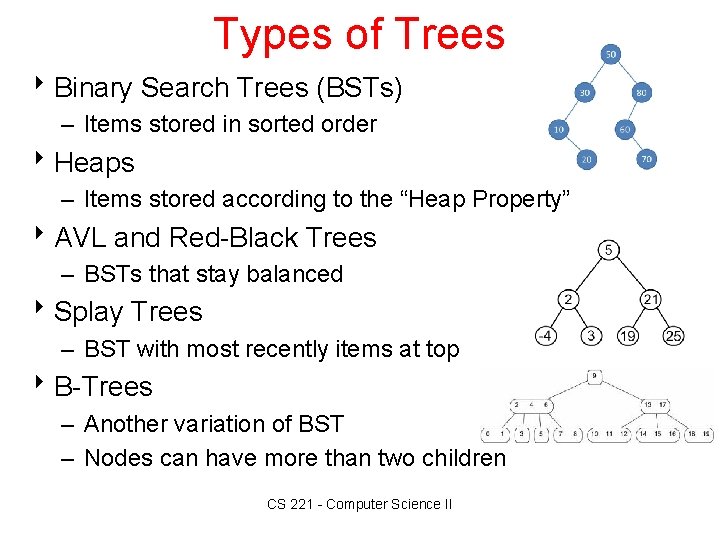
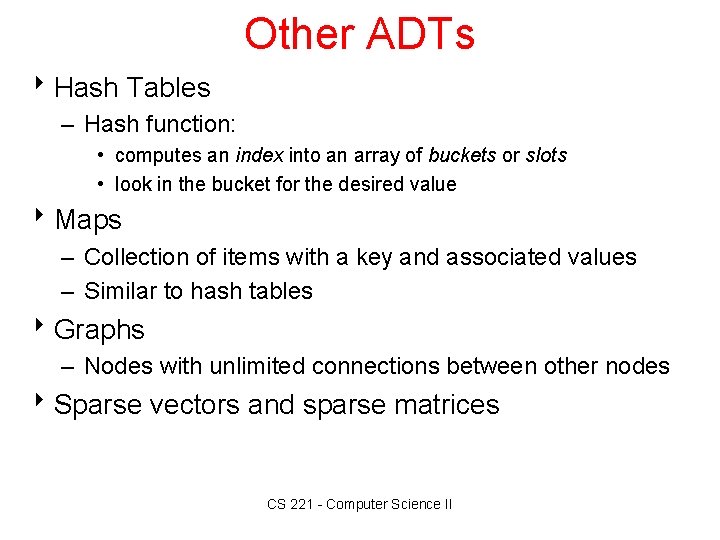
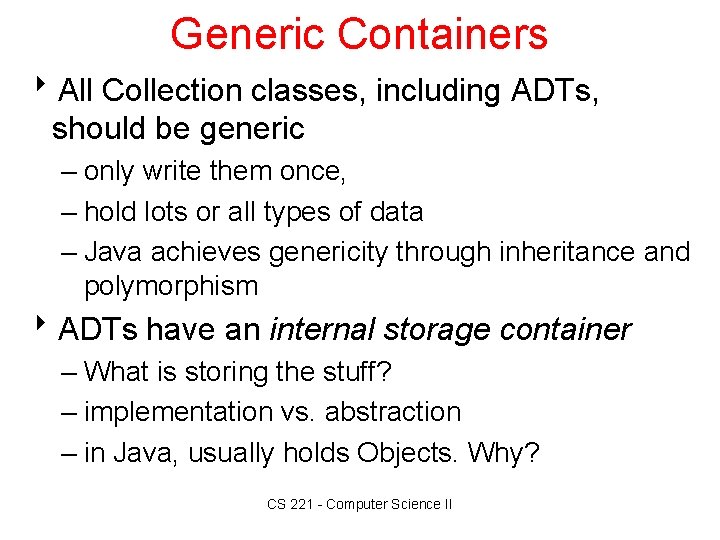
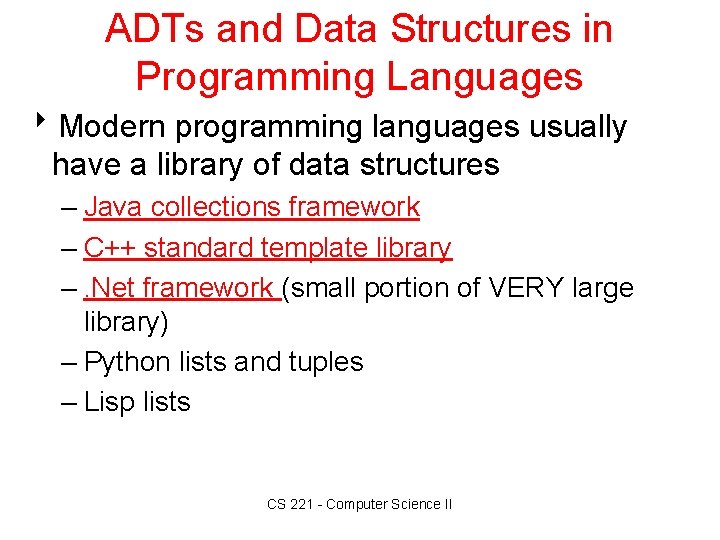
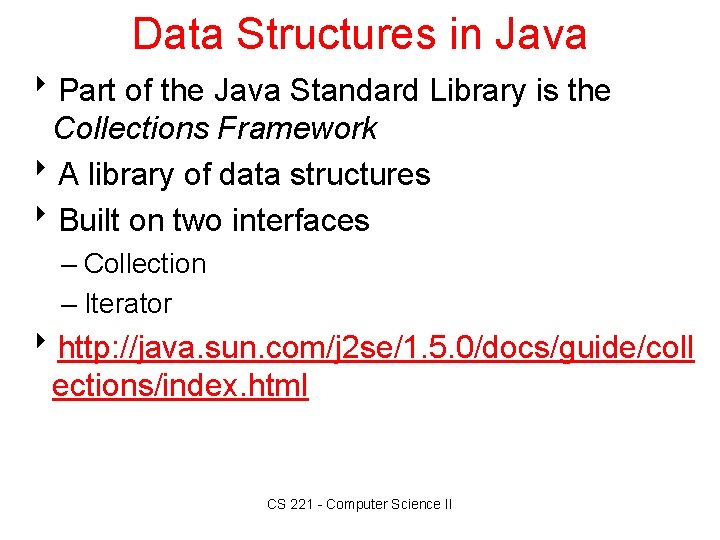
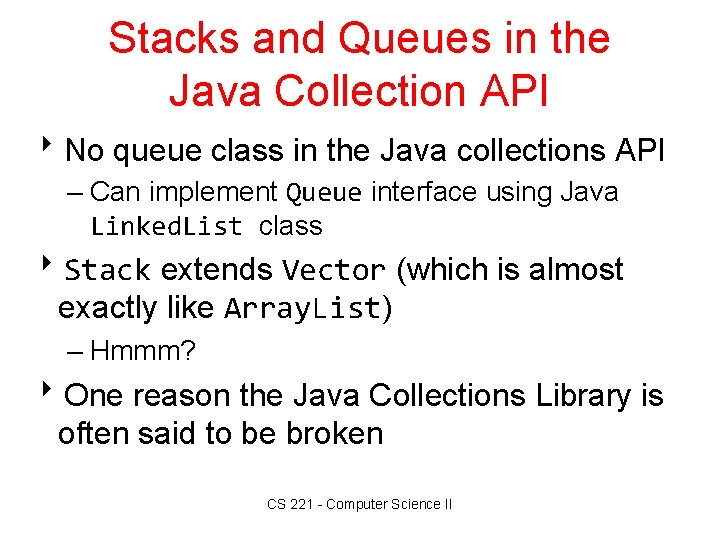
- Slides: 17
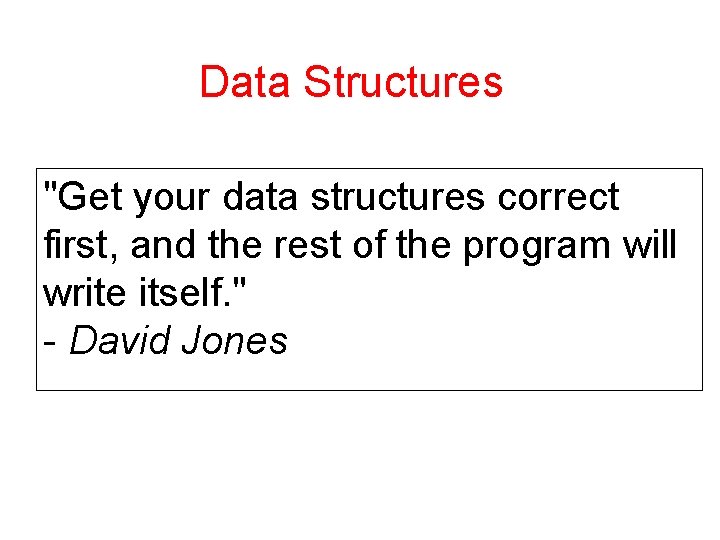
Data Structures "Get your data structures correct first, and the rest of the program will write itself. " - David Jones
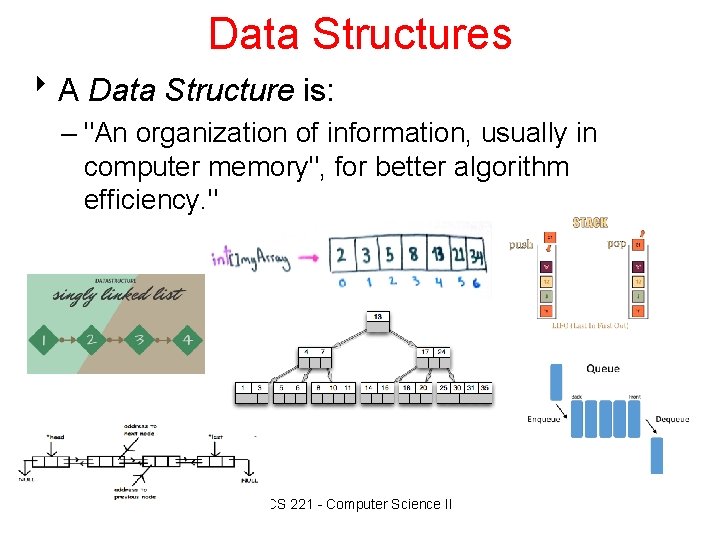
Data Structures 8 A Data Structure is: – "An organization of information, usually in computer memory", for better algorithm efficiency. " CS 221 - Computer Science II
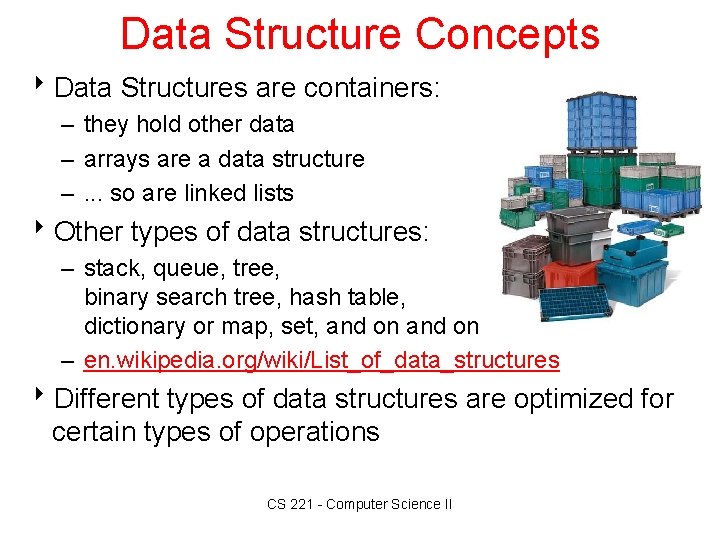
Data Structure Concepts 8 Data Structures are containers: – they hold other data – arrays are a data structure –. . . so are linked lists 8 Other types of data structures: – stack, queue, tree, binary search tree, hash table, dictionary or map, set, and on – en. wikipedia. org/wiki/List_of_data_structures 8 Different types of data structures are optimized for certain types of operations CS 221 - Computer Science II
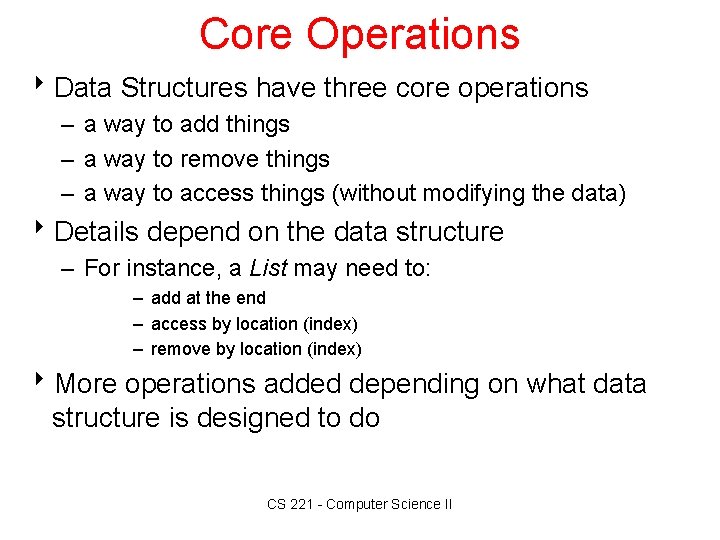
Core Operations 8 Data Structures have three core operations – a way to add things – a way to remove things – a way to access things (without modifying the data) 8 Details depend on the data structure – For instance, a List may need to: – add at the end – access by location (index) – remove by location (index) 8 More operations added depending on what data structure is designed to do CS 221 - Computer Science II
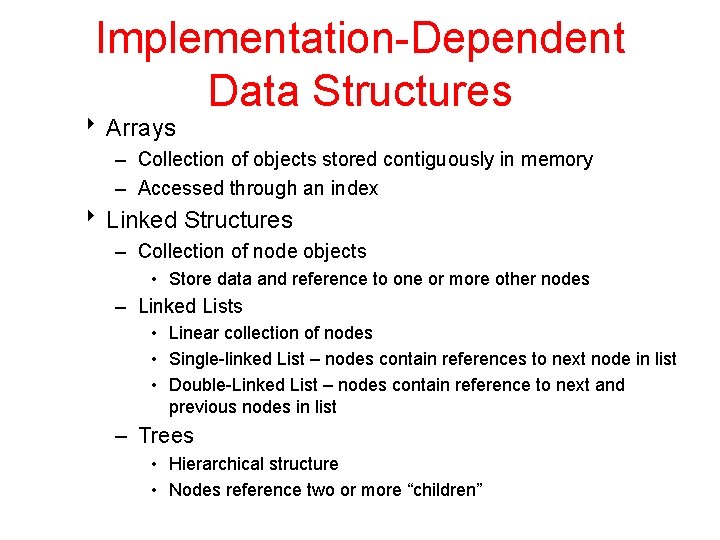
Implementation-Dependent Data Structures 8 Arrays – Collection of objects stored contiguously in memory – Accessed through an index 8 Linked Structures – Collection of node objects • Store data and reference to one or more other nodes – Linked Lists • Linear collection of nodes • Single-linked List – nodes contain references to next node in list • Double-Linked List – nodes contain reference to next and previous nodes in list – Trees • Hierarchical structure • Nodes reference two or more “children”
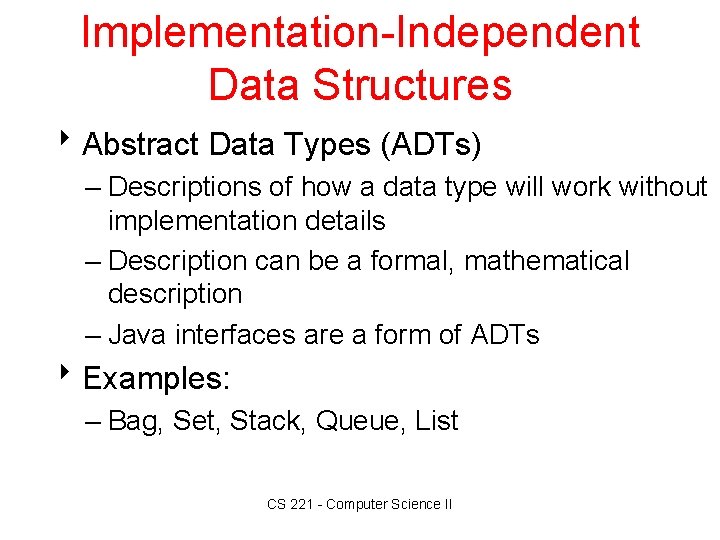
Implementation-Independent Data Structures 8 Abstract Data Types (ADTs) – Descriptions of how a data type will work without implementation details – Description can be a formal, mathematical description – Java interfaces are a form of ADTs 8 Examples: – Bag, Set, Stack, Queue, List CS 221 - Computer Science II
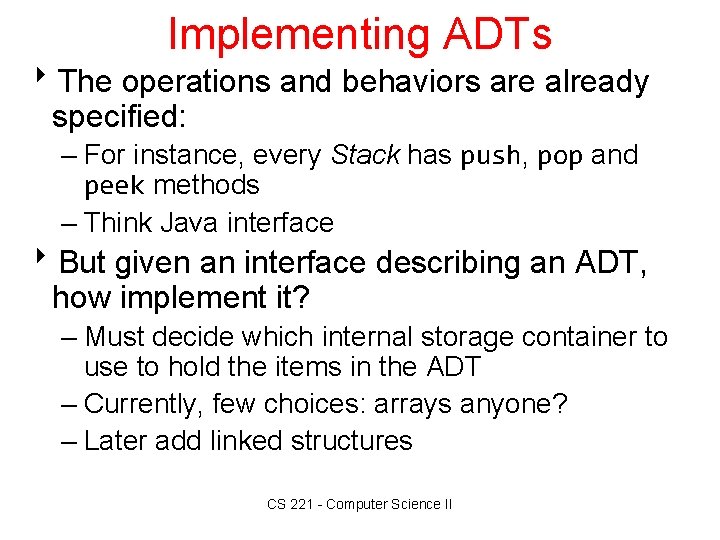
Implementing ADTs 8 The operations and behaviors are already specified: – For instance, every Stack has push, pop and peek methods – Think Java interface 8 But given an interface describing an ADT, how implement it? – Must decide which internal storage container to use to hold the items in the ADT – Currently, few choices: arrays anyone? – Later add linked structures CS 221 - Computer Science II
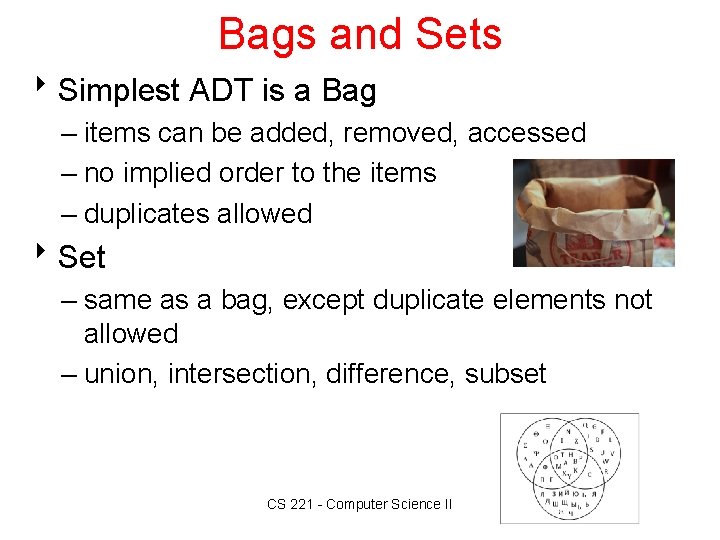
Bags and Sets 8 Simplest ADT is a Bag – items can be added, removed, accessed – no implied order to the items – duplicates allowed 8 Set – same as a bag, except duplicate elements not allowed – union, intersection, difference, subset CS 221 - Computer Science II
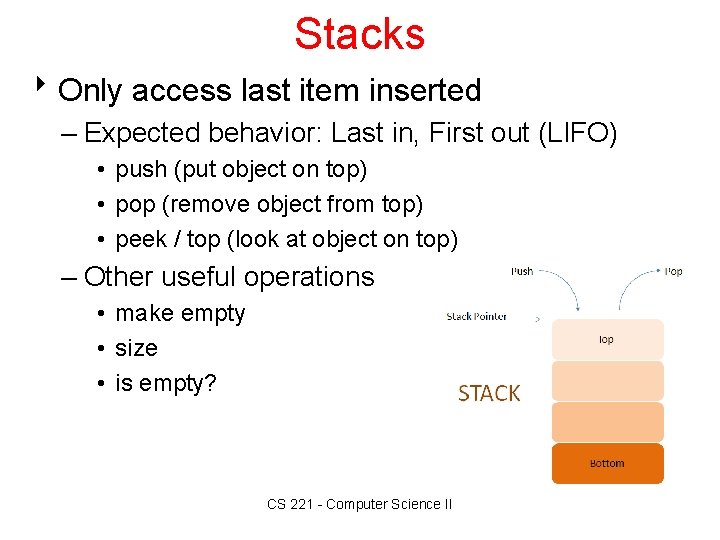
Stacks 8 Only access last item inserted – Expected behavior: Last in, First out (LIFO) • push (put object on top) • pop (remove object from top) • peek / top (look at object on top) – Other useful operations • make empty • size • is empty? CS 221 - Computer Science II
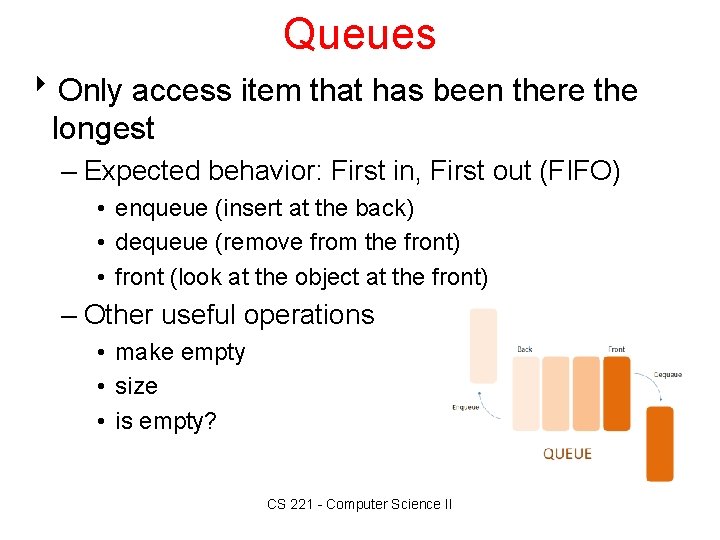
Queues 8 Only access item that has been there the longest – Expected behavior: First in, First out (FIFO) • enqueue (insert at the back) • dequeue (remove from the front) • front (look at the object at the front) – Other useful operations • make empty • size • is empty? CS 221 - Computer Science II
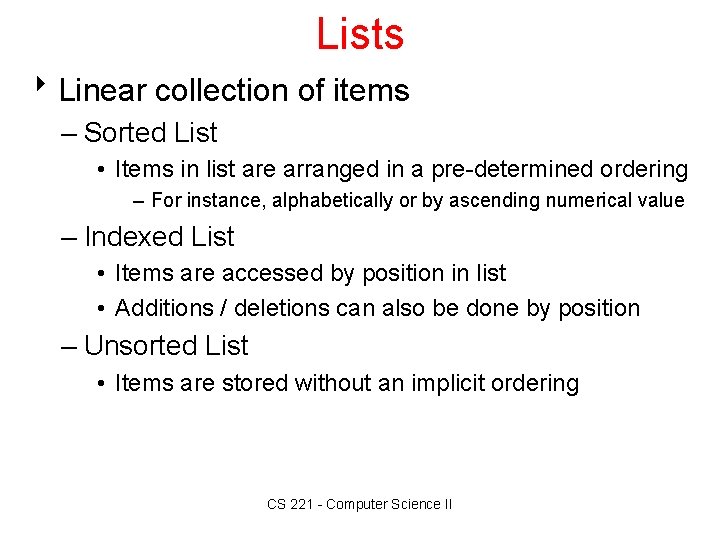
Lists 8 Linear collection of items – Sorted List • Items in list are arranged in a pre-determined ordering – For instance, alphabetically or by ascending numerical value – Indexed List • Items are accessed by position in list • Additions / deletions can also be done by position – Unsorted List • Items are stored without an implicit ordering CS 221 - Computer Science II
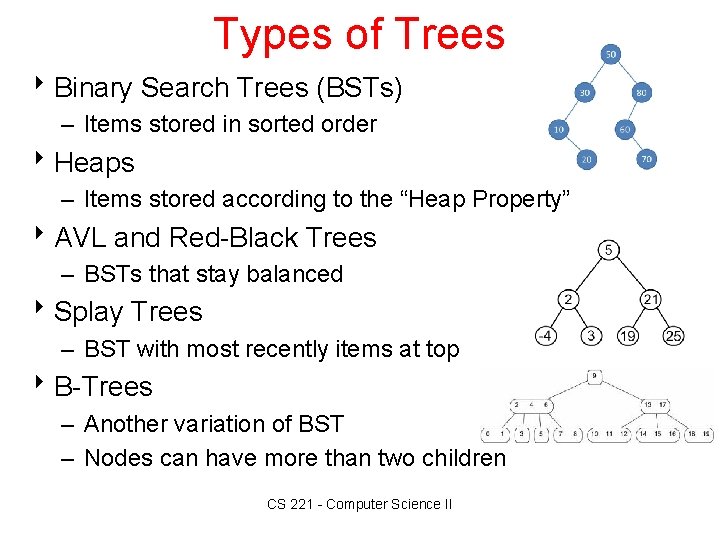
Types of Trees 8 Binary Search Trees (BSTs) – Items stored in sorted order 8 Heaps – Items stored according to the “Heap Property” 8 AVL and Red-Black Trees – BSTs that stay balanced 8 Splay Trees – BST with most recently items at top 8 B-Trees – Another variation of BST – Nodes can have more than two children CS 221 - Computer Science II
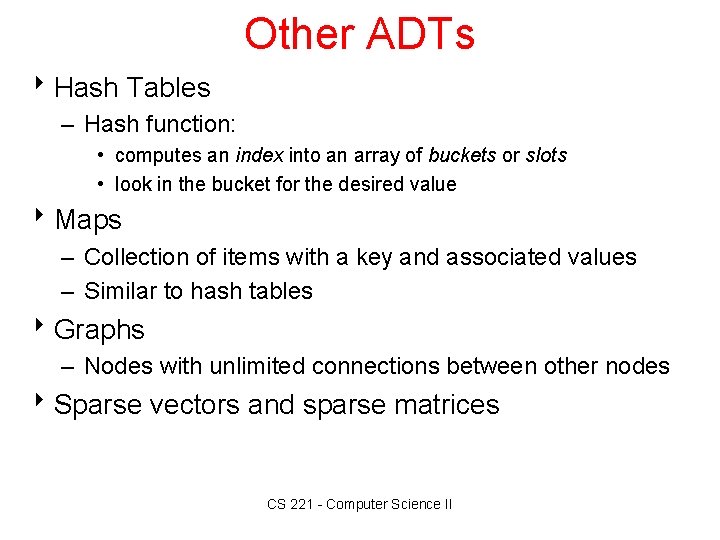
Other ADTs 8 Hash Tables – Hash function: • computes an index into an array of buckets or slots • look in the bucket for the desired value 8 Maps – Collection of items with a key and associated values – Similar to hash tables 8 Graphs – Nodes with unlimited connections between other nodes 8 Sparse vectors and sparse matrices CS 221 - Computer Science II
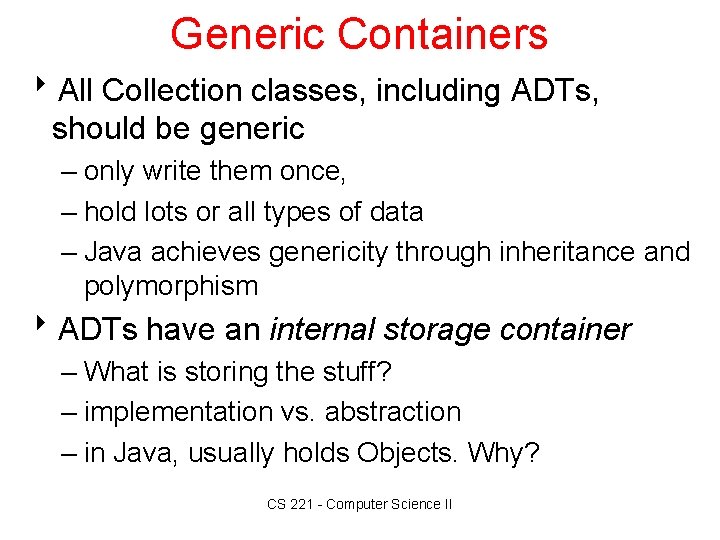
Generic Containers 8 All Collection classes, including ADTs, should be generic – only write them once, – hold lots or all types of data – Java achieves genericity through inheritance and polymorphism 8 ADTs have an internal storage container – What is storing the stuff? – implementation vs. abstraction – in Java, usually holds Objects. Why? CS 221 - Computer Science II
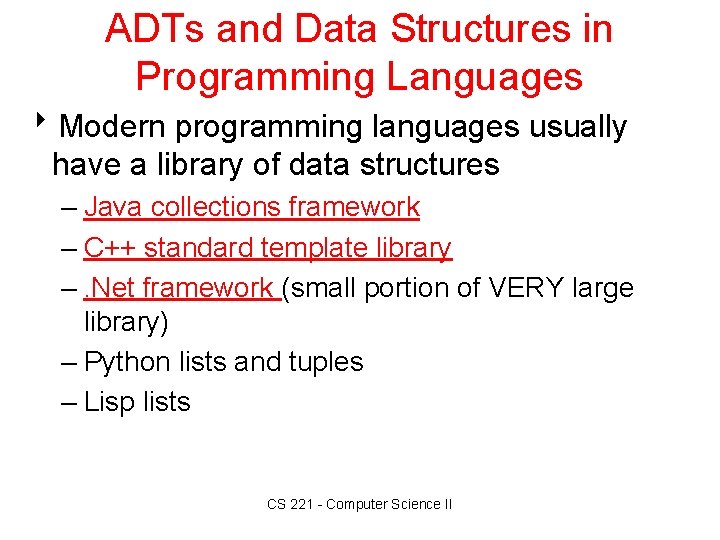
ADTs and Data Structures in Programming Languages 8 Modern programming languages usually have a library of data structures – Java collections framework – C++ standard template library –. Net framework (small portion of VERY large library) – Python lists and tuples – Lisp lists CS 221 - Computer Science II
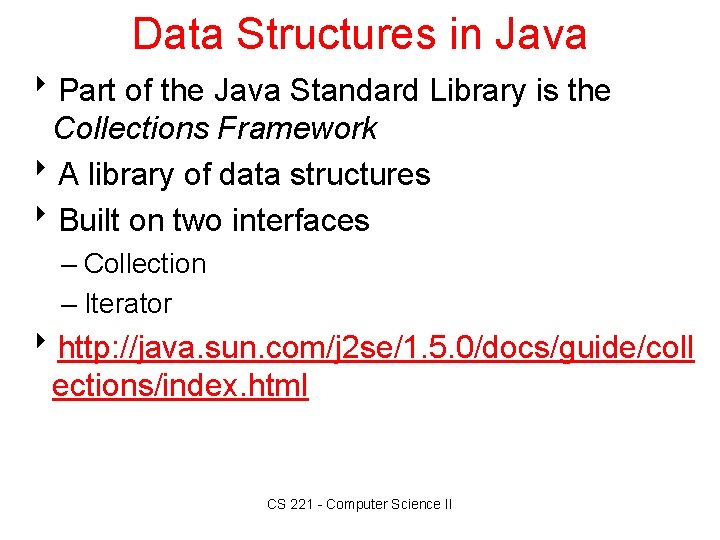
Data Structures in Java 8 Part of the Java Standard Library is the Collections Framework 8 A library of data structures 8 Built on two interfaces – Collection – Iterator 8 http: //java. sun. com/j 2 se/1. 5. 0/docs/guide/coll ections/index. html CS 221 - Computer Science II
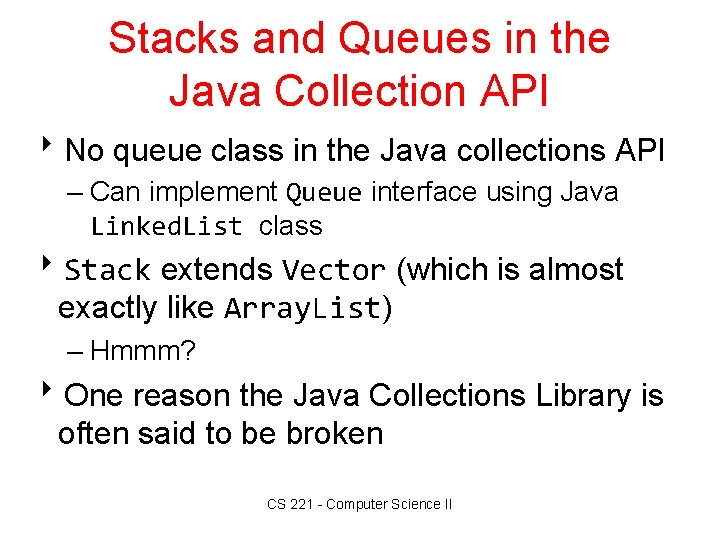
Stacks and Queues in the Java Collection API 8 No queue class in the Java collections API – Can implement Queue interface using Java Linked. List class 8 Stack extends Vector (which is almost exactly like Array. List) – Hmmm? 8 One reason the Java Collections Library is often said to be broken CS 221 - Computer Science II
Get on / get off transport
One direction songs one thing
Get up get moving quiz
Get up get moving quiz
Get up get moving quiz
Example of selection in pseudocode
Get focused get results
Get up get moving quiz
Circle the correct alternatives we won't get
Give us your hungry your tired your poor
Homologous structure
If you get home the first
How long does a dog stay on heat
You never get second chance make first impression
Karakia for protection
5 sentences about my best friend
Get your password shivaji university
How to win your parents trust back