Cursors in PLSQL Includes cursor example and continuation
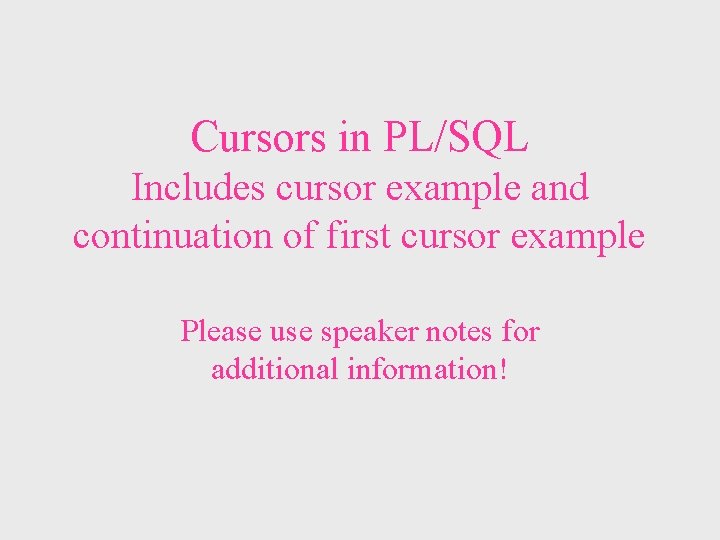
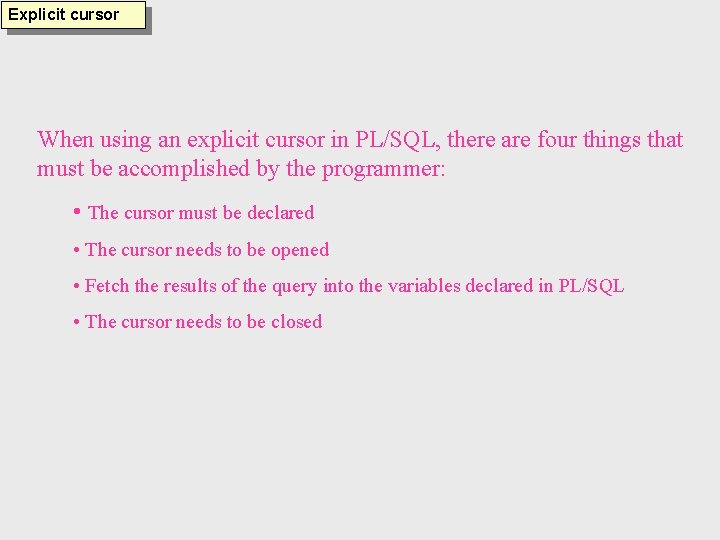
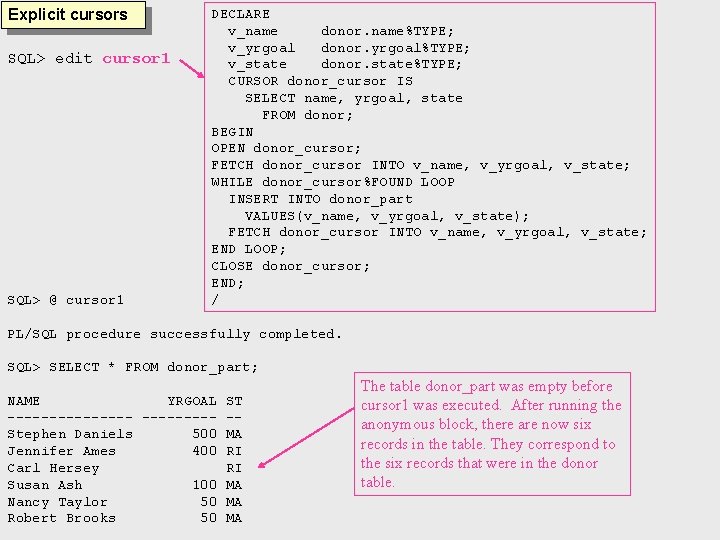
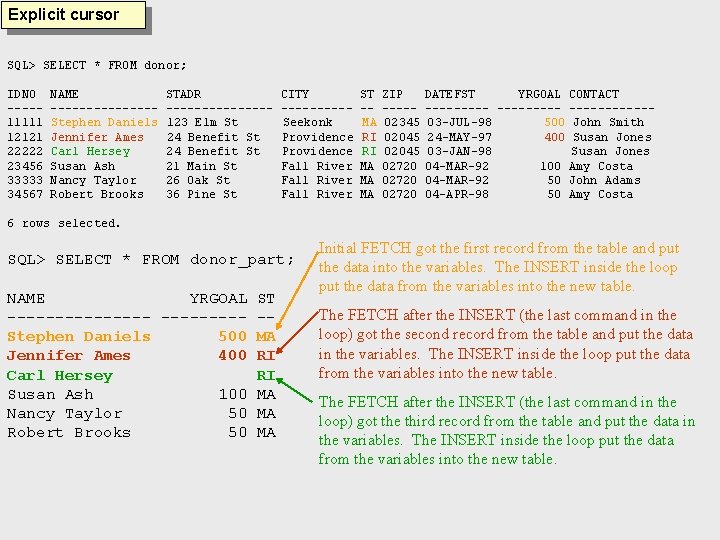
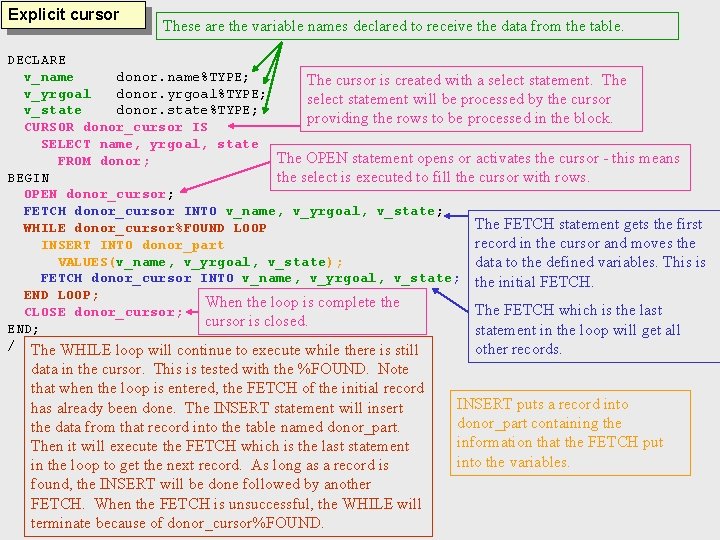
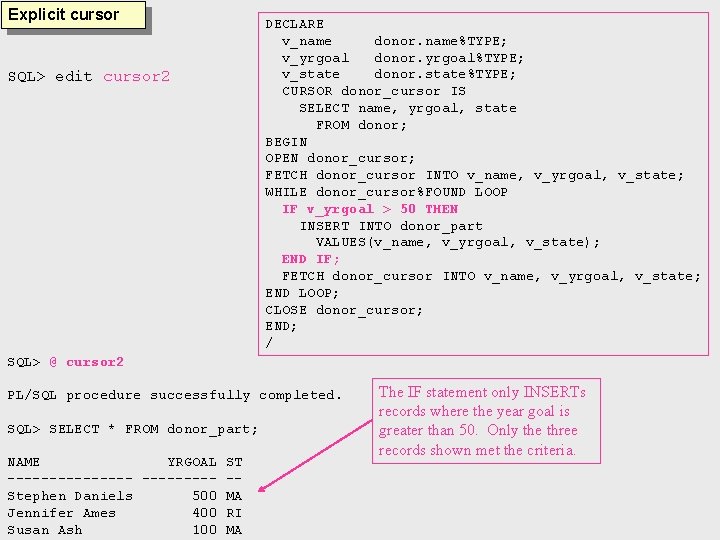
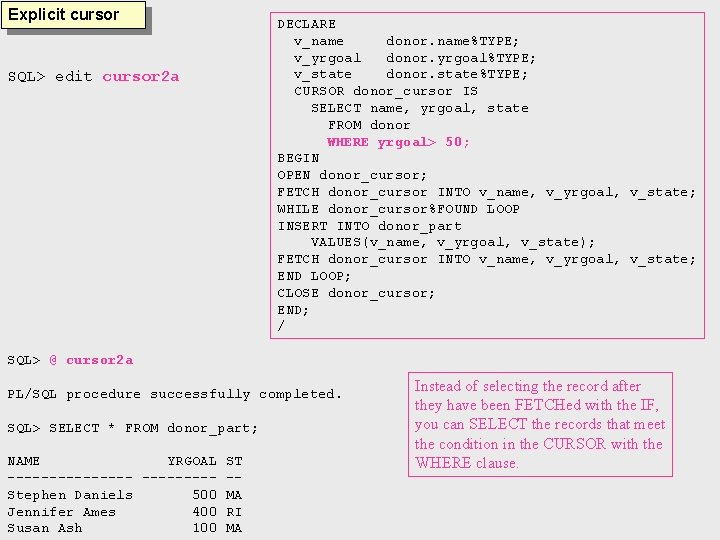
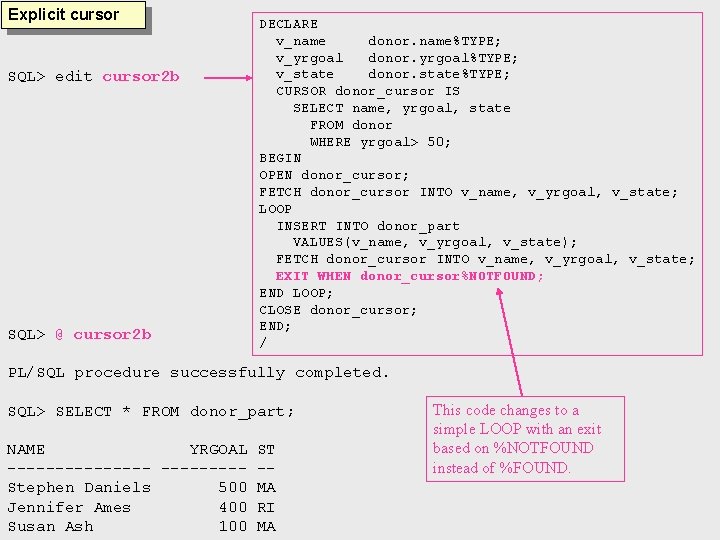
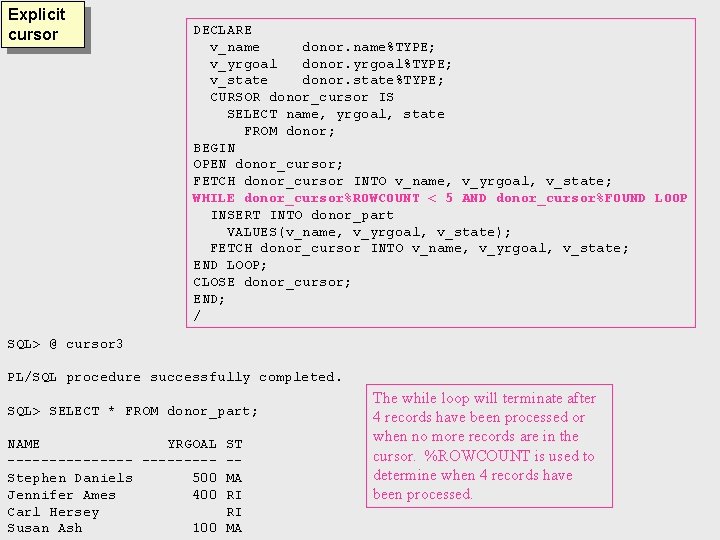
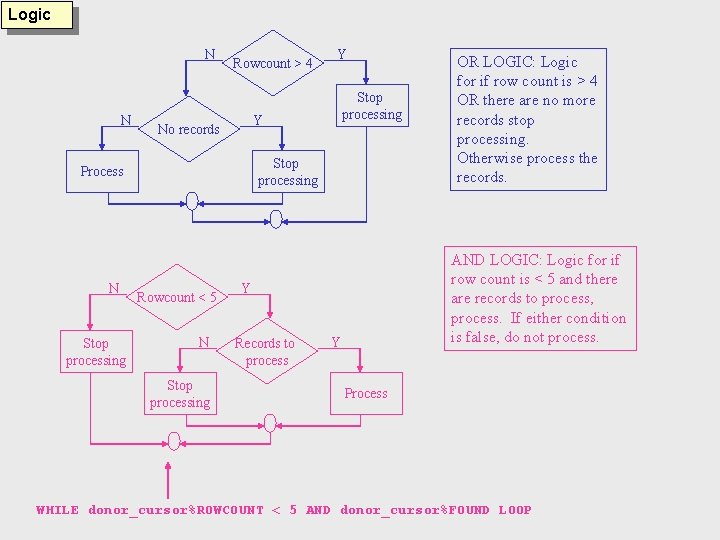
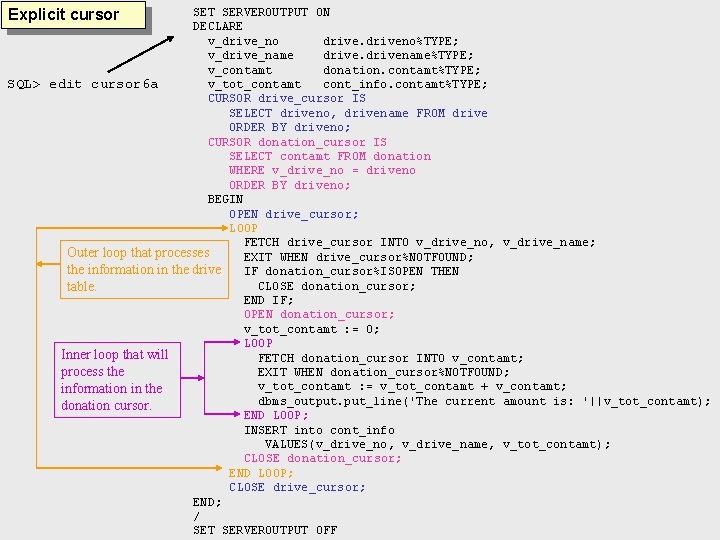
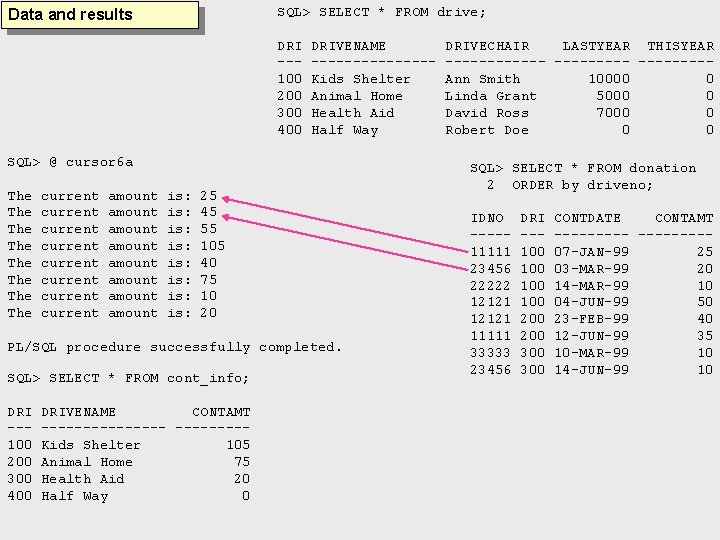
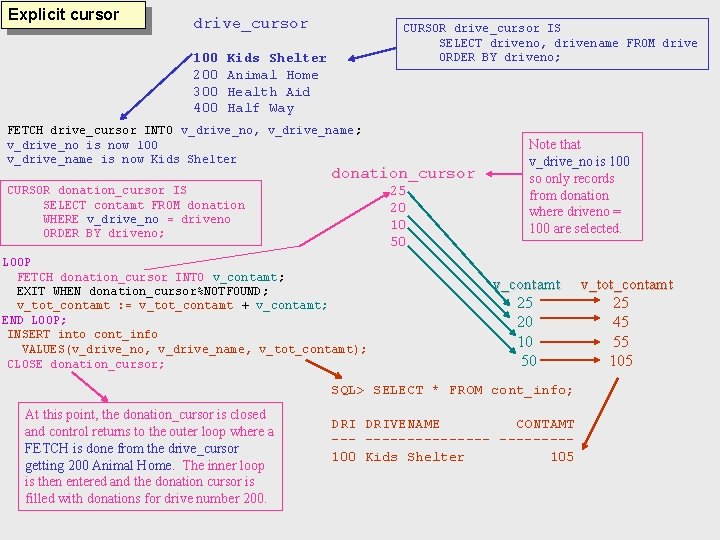
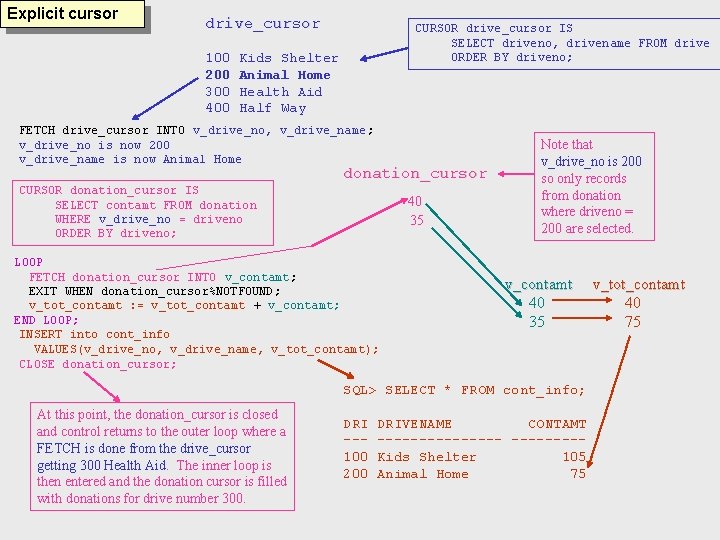
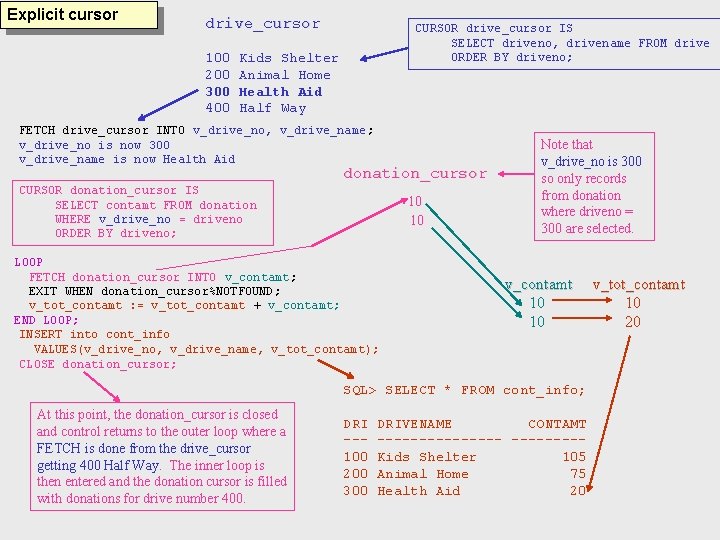
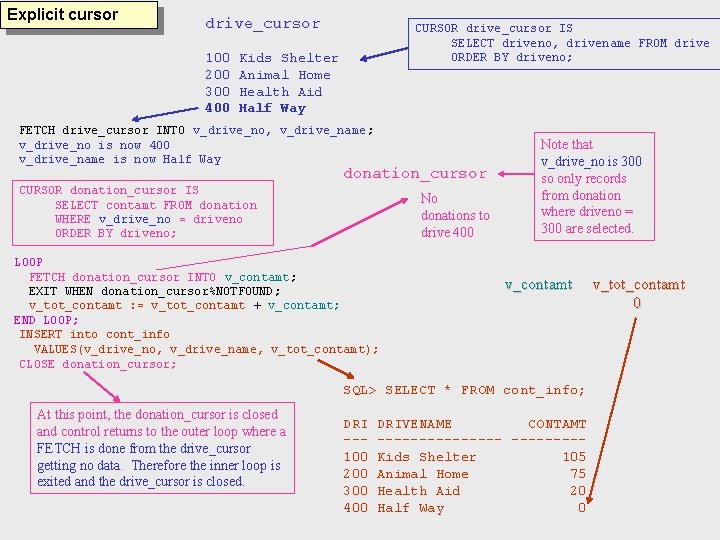
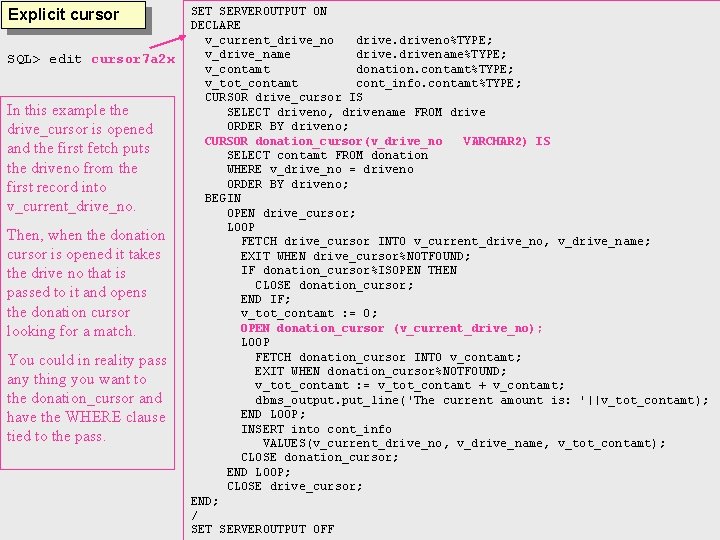
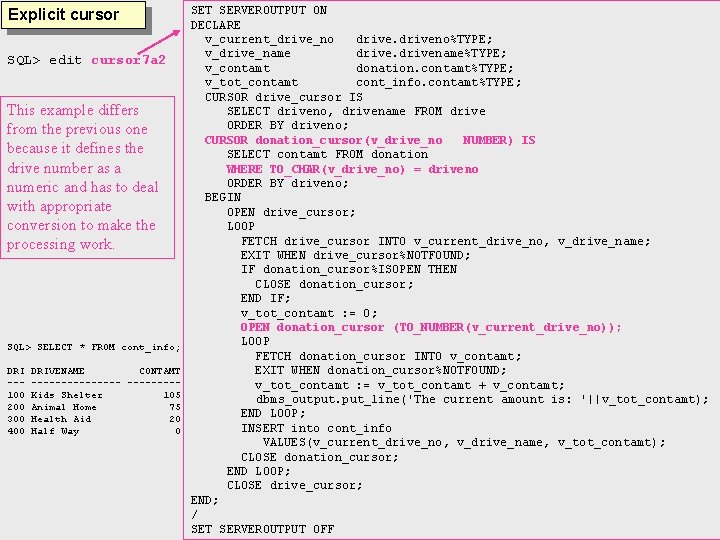
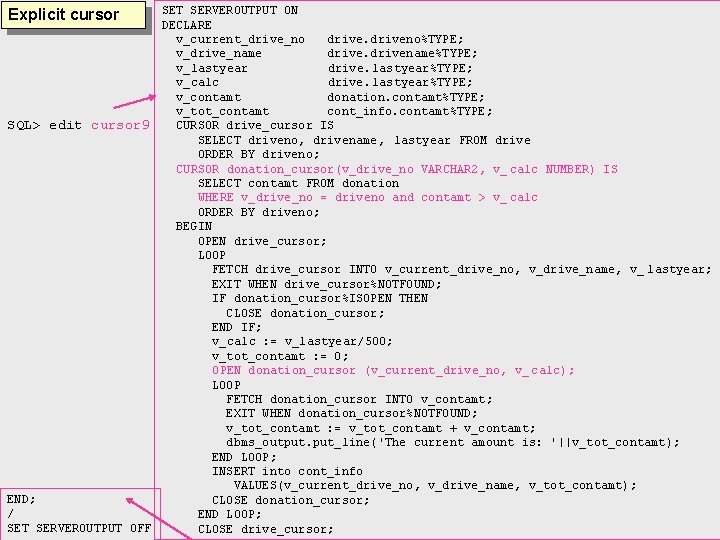
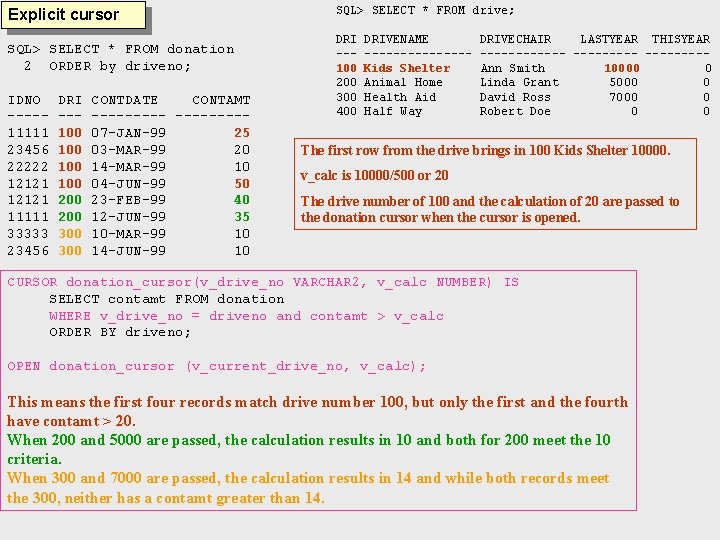
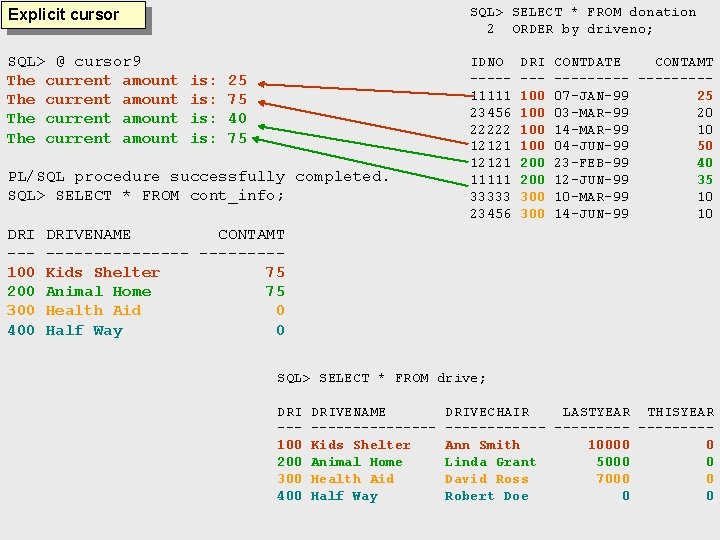
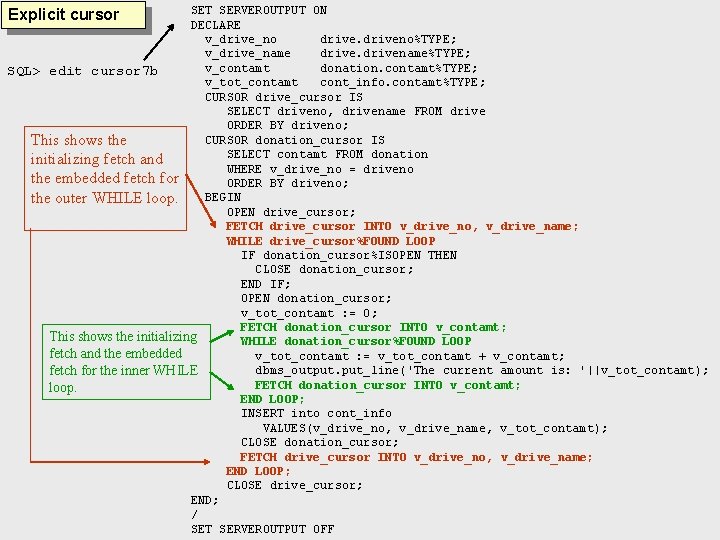
- Slides: 22
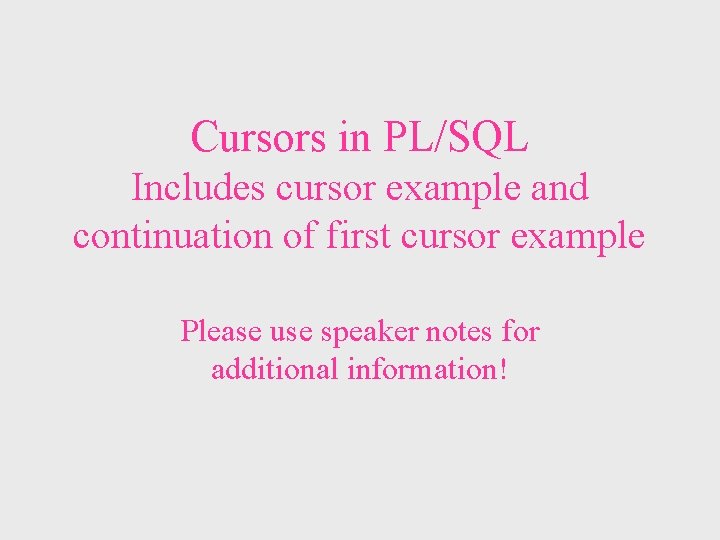
Cursors in PL/SQL Includes cursor example and continuation of first cursor example Please use speaker notes for additional information!
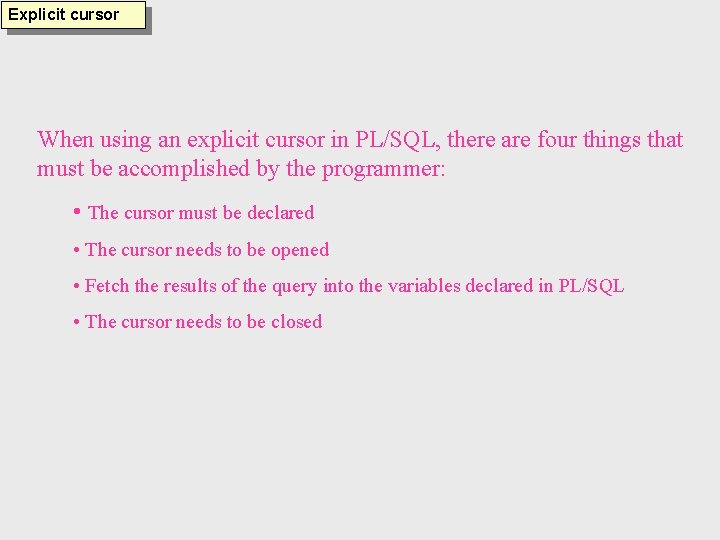
Explicit cursor When using an explicit cursor in PL/SQL, there are four things that must be accomplished by the programmer: • The cursor must be declared • The cursor needs to be opened • Fetch the results of the query into the variables declared in PL/SQL • The cursor needs to be closed
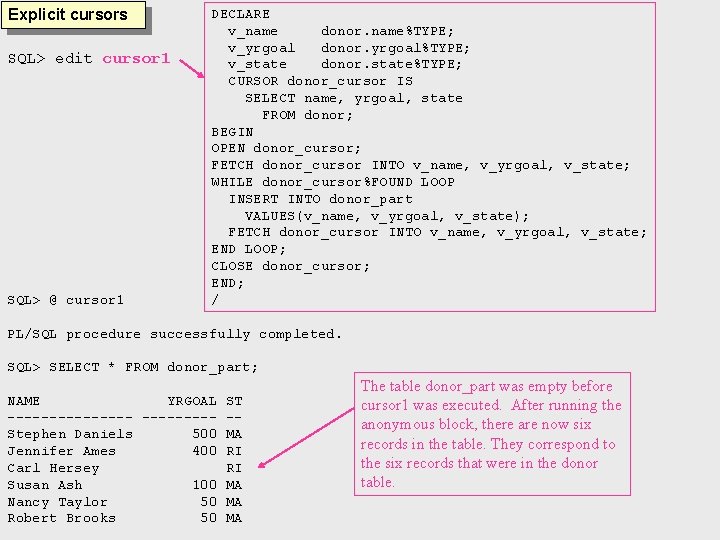
Explicit cursors SQL> edit cursor 1 SQL> @ cursor 1 DECLARE v_name donor. name%TYPE; v_yrgoal donor. yrgoal%TYPE; v_state donor. state%TYPE; CURSOR donor_cursor IS SELECT name, yrgoal, state FROM donor; BEGIN OPEN donor_cursor; FETCH donor_cursor INTO v_name, v_yrgoal, v_state; WHILE donor_cursor%FOUND LOOP INSERT INTO donor_part VALUES(v_name, v_yrgoal, v_state); FETCH donor_cursor INTO v_name, v_yrgoal, v_state; END LOOP; CLOSE donor_cursor; END; / PL/SQL procedure successfully completed. SQL> SELECT * FROM donor_part; NAME YRGOAL ST -------- -Stephen Daniels 500 MA Jennifer Ames 400 RI Carl Hersey RI Susan Ash 100 MA Nancy Taylor 50 MA Robert Brooks 50 MA The table donor_part was empty before cursor 1 was executed. After running the anonymous block, there are now six records in the table. They correspond to the six records that were in the donor table.
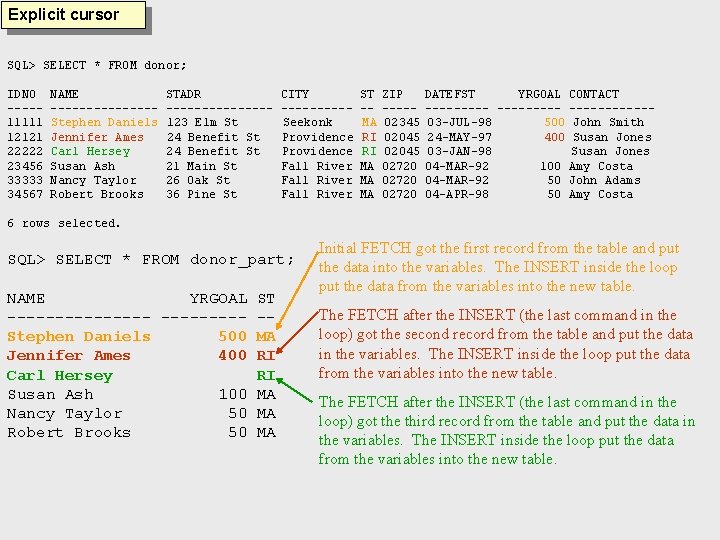
Explicit cursor SQL> SELECT * FROM donor; IDNO ----11111 12121 22222 23456 33333 34567 NAME -------Stephen Daniels Jennifer Ames Carl Hersey Susan Ash Nancy Taylor Robert Brooks STADR -------123 Elm St 24 Benefit St 21 Main St 26 Oak St 36 Pine St CITY -----Seekonk Providence Fall River ST -MA RI RI MA MA MA ZIP ----02345 02045 02720 DATEFST YRGOAL CONTACT ---------03 -JUL-98 500 John Smith 24 -MAY-97 400 Susan Jones 03 -JAN-98 Susan Jones 04 -MAR-92 100 Amy Costa 04 -MAR-92 50 John Adams 04 -APR-98 50 Amy Costa 6 rows selected. SQL> SELECT * FROM donor_part; NAME YRGOAL ST -------- -Stephen Daniels 500 MA Jennifer Ames 400 RI Carl Hersey RI Susan Ash 100 MA Nancy Taylor 50 MA Robert Brooks 50 MA Initial FETCH got the first record from the table and put the data into the variables. The INSERT inside the loop put the data from the variables into the new table. The FETCH after the INSERT (the last command in the loop) got the second record from the table and put the data in the variables. The INSERT inside the loop put the data from the variables into the new table. The FETCH after the INSERT (the last command in the loop) got the third record from the table and put the data in the variables. The INSERT inside the loop put the data from the variables into the new table.
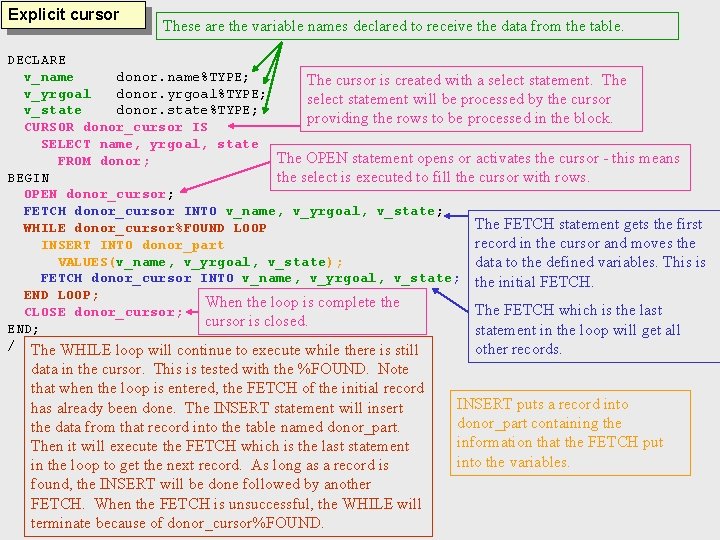
Explicit cursor These are the variable names declared to receive the data from the table. DECLARE v_name donor. name%TYPE; The cursor is created with a select statement. The v_yrgoal donor. yrgoal%TYPE; select statement will be processed by the cursor v_state donor. state%TYPE; providing the rows to be processed in the block. CURSOR donor_cursor IS SELECT name, yrgoal, state The OPEN statement opens or activates the cursor - this means FROM donor; BEGIN the select is executed to fill the cursor with rows. OPEN donor_cursor; FETCH donor_cursor INTO v_name, v_yrgoal, v_state; The FETCH statement gets the first WHILE donor_cursor%FOUND LOOP record in the cursor and moves the INSERT INTO donor_part VALUES(v_name, v_yrgoal, v_state); data to the defined variables. This is FETCH donor_cursor INTO v_name, v_yrgoal, v_state; the initial FETCH. END LOOP; When the loop is complete the The FETCH which is the last CLOSE donor_cursor; cursor is closed. END; statement in the loop will get all / The WHILE loop will continue to execute while there is still other records. data in the cursor. This is tested with the %FOUND. Note that when the loop is entered, the FETCH of the initial record has already been done. The INSERT statement will insert the data from that record into the table named donor_part. Then it will execute the FETCH which is the last statement in the loop to get the next record. As long as a record is found, the INSERT will be done followed by another FETCH. When the FETCH is unsuccessful, the WHILE will terminate because of donor_cursor%FOUND. INSERT puts a record into donor_part containing the information that the FETCH put into the variables.
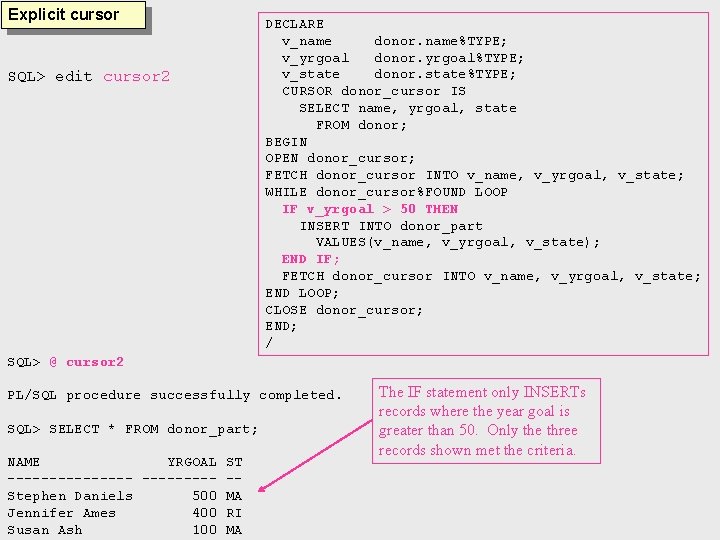
Explicit cursor SQL> edit cursor 2 DECLARE v_name donor. name%TYPE; v_yrgoal donor. yrgoal%TYPE; v_state donor. state%TYPE; CURSOR donor_cursor IS SELECT name, yrgoal, state FROM donor; BEGIN OPEN donor_cursor; FETCH donor_cursor INTO v_name, v_yrgoal, v_state; WHILE donor_cursor%FOUND LOOP IF v_yrgoal > 50 THEN INSERT INTO donor_part VALUES(v_name, v_yrgoal, v_state); END IF; FETCH donor_cursor INTO v_name, v_yrgoal, v_state; END LOOP; CLOSE donor_cursor; END; / SQL> @ cursor 2 PL/SQL procedure successfully completed. SQL> SELECT * FROM donor_part; NAME YRGOAL ST -------- -Stephen Daniels 500 MA Jennifer Ames 400 RI Susan Ash 100 MA The IF statement only INSERTs records where the year goal is greater than 50. Only the three records shown met the criteria.
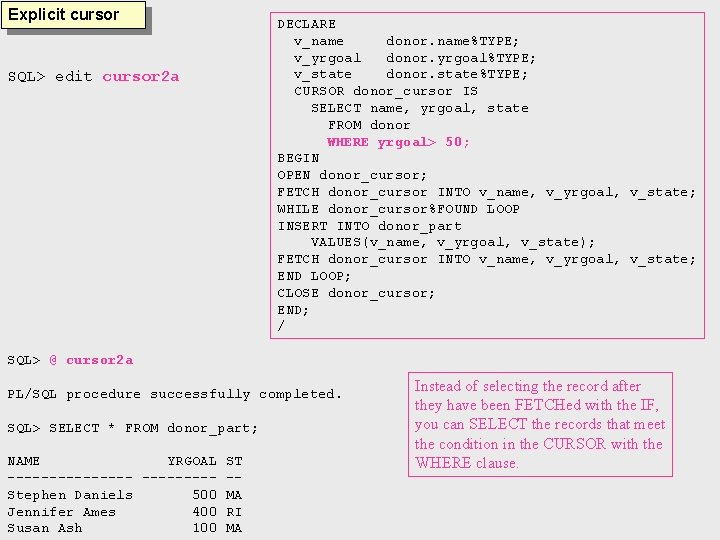
Explicit cursor SQL> edit cursor 2 a DECLARE v_name donor. name%TYPE; v_yrgoal donor. yrgoal%TYPE; v_state donor. state%TYPE; CURSOR donor_cursor IS SELECT name, yrgoal, state FROM donor WHERE yrgoal> 50; BEGIN OPEN donor_cursor; FETCH donor_cursor INTO v_name, v_yrgoal, v_state; WHILE donor_cursor%FOUND LOOP INSERT INTO donor_part VALUES(v_name, v_yrgoal, v_state); FETCH donor_cursor INTO v_name, v_yrgoal, v_state; END LOOP; CLOSE donor_cursor; END; / SQL> @ cursor 2 a PL/SQL procedure successfully completed. SQL> SELECT * FROM donor_part; NAME YRGOAL ST -------- -Stephen Daniels 500 MA Jennifer Ames 400 RI Susan Ash 100 MA Instead of selecting the record after they have been FETCHed with the IF, you can SELECT the records that meet the condition in the CURSOR with the WHERE clause.
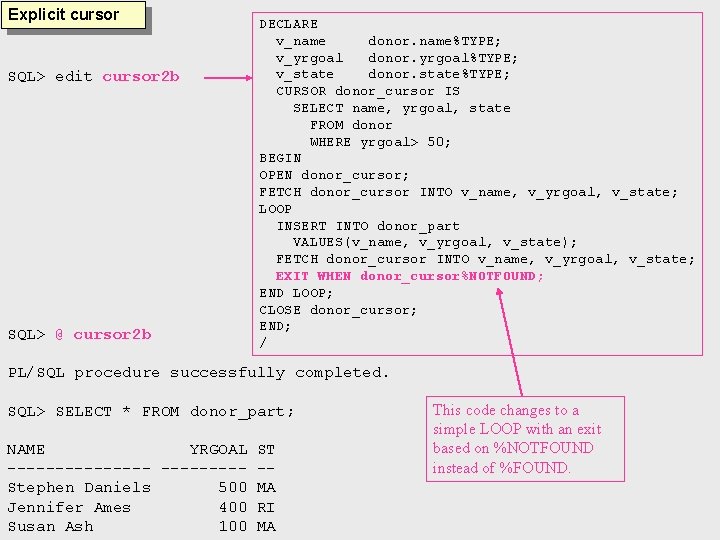
Explicit cursor SQL> edit cursor 2 b SQL> @ cursor 2 b DECLARE v_name donor. name%TYPE; v_yrgoal donor. yrgoal%TYPE; v_state donor. state%TYPE; CURSOR donor_cursor IS SELECT name, yrgoal, state FROM donor WHERE yrgoal> 50; BEGIN OPEN donor_cursor; FETCH donor_cursor INTO v_name, v_yrgoal, v_state; LOOP INSERT INTO donor_part VALUES(v_name, v_yrgoal, v_state); FETCH donor_cursor INTO v_name, v_yrgoal, v_state; EXIT WHEN donor_cursor%NOTFOUND; END LOOP; CLOSE donor_cursor; END; / PL/SQL procedure successfully completed. SQL> SELECT * FROM donor_part; NAME YRGOAL ST -------- -Stephen Daniels 500 MA Jennifer Ames 400 RI Susan Ash 100 MA This code changes to a simple LOOP with an exit based on %NOTFOUND instead of %FOUND.
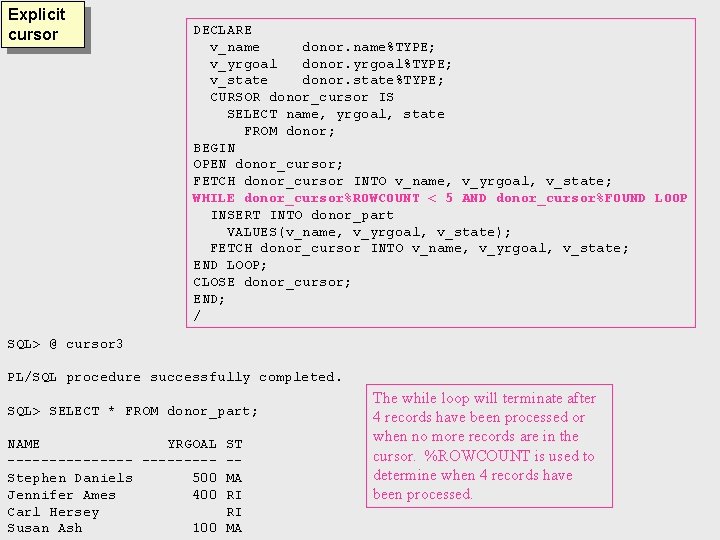
Explicit cursor DECLARE v_name donor. name%TYPE; v_yrgoal donor. yrgoal%TYPE; v_state donor. state%TYPE; CURSOR donor_cursor IS SELECT name, yrgoal, state FROM donor; BEGIN OPEN donor_cursor; FETCH donor_cursor INTO v_name, v_yrgoal, v_state; WHILE donor_cursor%ROWCOUNT < 5 AND donor_cursor%FOUND LOOP INSERT INTO donor_part VALUES(v_name, v_yrgoal, v_state); FETCH donor_cursor INTO v_name, v_yrgoal, v_state; END LOOP; CLOSE donor_cursor; END; / SQL> @ cursor 3 PL/SQL procedure successfully completed. SQL> SELECT * FROM donor_part; NAME YRGOAL ST -------- -Stephen Daniels 500 MA Jennifer Ames 400 RI Carl Hersey RI Susan Ash 100 MA The while loop will terminate after 4 records have been processed or when no more records are in the cursor. %ROWCOUNT is used to determine when 4 records have been processed.
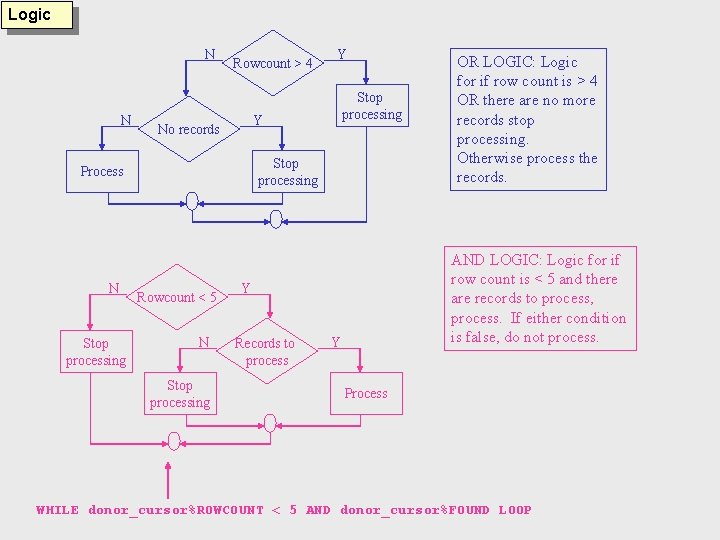
Logic N N Rowcount > 4 Stop processing Y No records Stop processing Process N Y Rowcount < 5 N Stop processing AND LOGIC: Logic for if row count is < 5 and there are records to process, process. If either condition is false, do not process. Y Records to process OR LOGIC: Logic for if row count is > 4 OR there are no more records stop processing. Otherwise process the records. Y Process WHILE donor_cursor%ROWCOUNT < 5 AND donor_cursor%FOUND LOOP
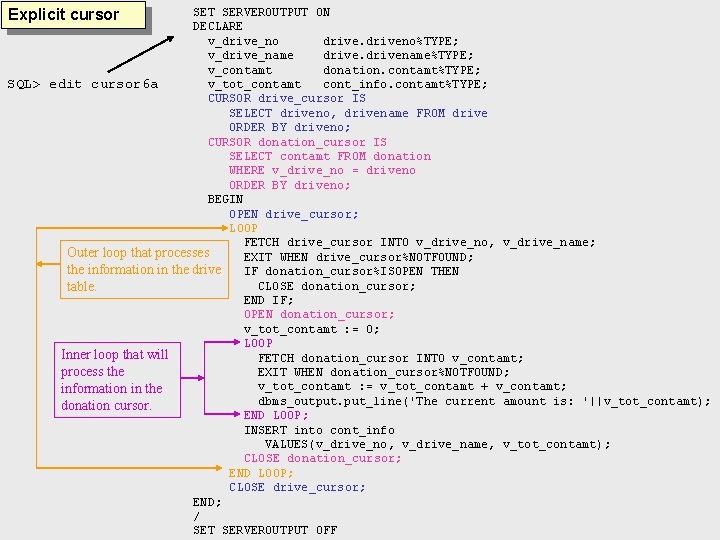
Explicit cursor SET SERVEROUTPUT ON DECLARE v_drive_no driveno%TYPE; v_drive_name drivename%TYPE; v_contamt donation. contamt%TYPE; v_tot_contamt cont_info. contamt%TYPE; SQL> edit cursor 6 a CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; CURSOR donation_cursor IS SELECT contamt FROM donation WHERE v_drive_no = driveno ORDER BY driveno; BEGIN OPEN drive_cursor; LOOP FETCH drive_cursor INTO v_drive_no, v_drive_name; Outer loop that processes EXIT WHEN drive_cursor%NOTFOUND; the information in the drive IF donation_cursor%ISOPEN THEN CLOSE donation_cursor; table. END IF; OPEN donation_cursor; v_tot_contamt : = 0; LOOP Inner loop that will FETCH donation_cursor INTO v_contamt; process the EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; information in the dbms_output. put_line('The current amount is: '||v_tot_contamt); donation cursor. END LOOP; INSERT into cont_info VALUES(v_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; END LOOP; CLOSE drive_cursor; END; / SET SERVEROUTPUT OFF
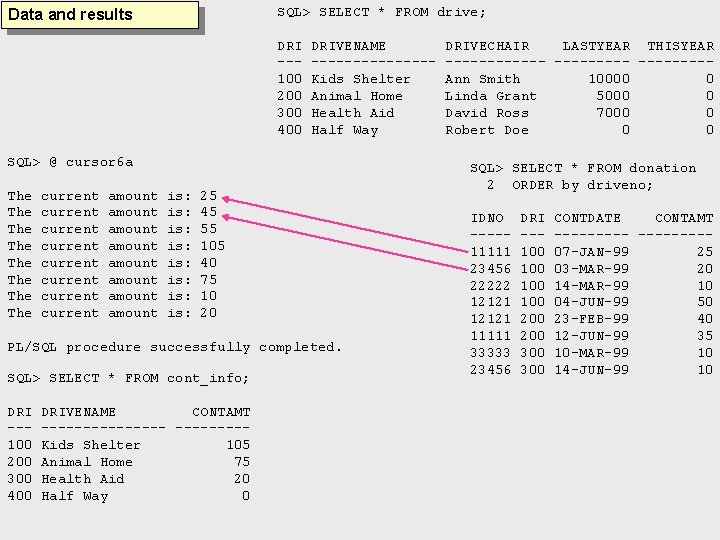
SQL> SELECT * FROM drive; Data and results DRI --100 200 300 400 DRIVENAME -------Kids Shelter Animal Home Health Aid Half Way SQL> @ cursor 6 a The The current current amount amount is: is: 25 45 55 105 40 75 10 20 PL/SQL procedure successfully completed. SQL> SELECT * FROM cont_info; DRI --100 200 300 400 DRIVENAME CONTAMT --------Kids Shelter 105 Animal Home 75 Health Aid 20 Half Way 0 DRIVECHAIR LASTYEAR THISYEAR ------ ----Ann Smith 10000 0 Linda Grant 5000 0 David Ross 7000 0 Robert Doe 0 0 SQL> SELECT * FROM donation 2 ORDER by driveno; IDNO ----11111 23456 22222 12121 11111 33333 23456 DRI --100 100 200 300 CONTDATE CONTAMT -----07 -JAN-99 25 03 -MAR-99 20 14 -MAR-99 10 04 -JUN-99 50 23 -FEB-99 40 12 -JUN-99 35 10 -MAR-99 10 14 -JUN-99 10
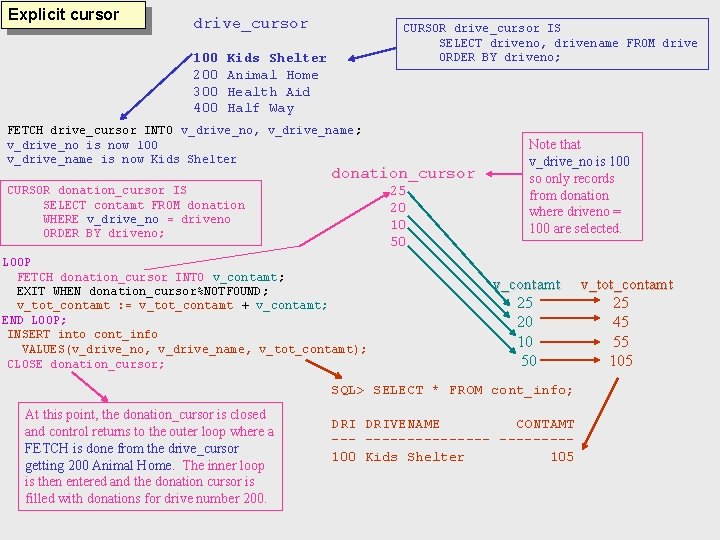
Explicit cursor drive_cursor 100 200 300 400 CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; Kids Shelter Animal Home Health Aid Half Way FETCH drive_cursor INTO v_drive_no, v_drive_name ; v_drive_no is now 100 v_drive_name is now Kids Shelter donation_cursor 25 20 10 50 CURSOR donation_cursor IS SELECT contamt FROM donation WHERE v_drive_no = driveno ORDER BY driveno; LOOP FETCH donation_cursor INTO v_contamt; EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; END LOOP; INSERT into cont_info VALUES(v_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; Note that v_drive_no is 100 so only records from donation where driveno = 100 are selected. v_contamt 25 20 10 50 SQL> SELECT * FROM cont_info; At this point, the donation_cursor is closed and control returns to the outer loop where a FETCH is done from the drive_cursor getting 200 Animal Home. The inner loop is then entered and the donation cursor is filled with donations for drive number 200. DRIVENAME CONTAMT ---------100 Kids Shelter 105 v_tot_contamt 25 45 55 105
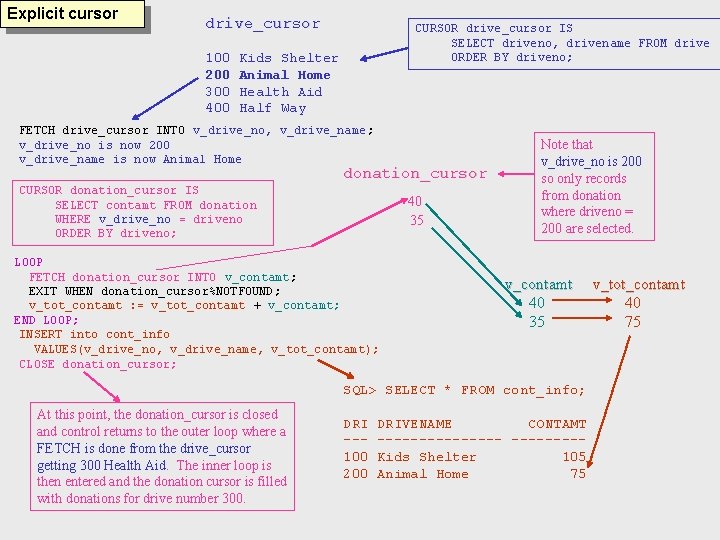
Explicit cursor drive_cursor 100 200 300 400 CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; Kids Shelter Animal Home Health Aid Half Way FETCH drive_cursor INTO v_drive_no, v_drive_name ; v_drive_no is now 200 v_drive_name is now Animal Home donation_cursor CURSOR donation_cursor IS SELECT contamt FROM donation WHERE v_drive_no = driveno ORDER BY driveno; 40 35 LOOP FETCH donation_cursor INTO v_contamt; EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; END LOOP; INSERT into cont_info VALUES(v_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; Note that v_drive_no is 200 so only records from donation where driveno = 200 are selected. v_contamt 40 35 SQL> SELECT * FROM cont_info; At this point, the donation_cursor is closed and control returns to the outer loop where a FETCH is done from the drive_cursor getting 300 Health Aid. The inner loop is then entered and the donation cursor is filled with donations for drive number 300. DRI --100 200 DRIVENAME CONTAMT --------Kids Shelter 105 Animal Home 75 v_tot_contamt 40 75
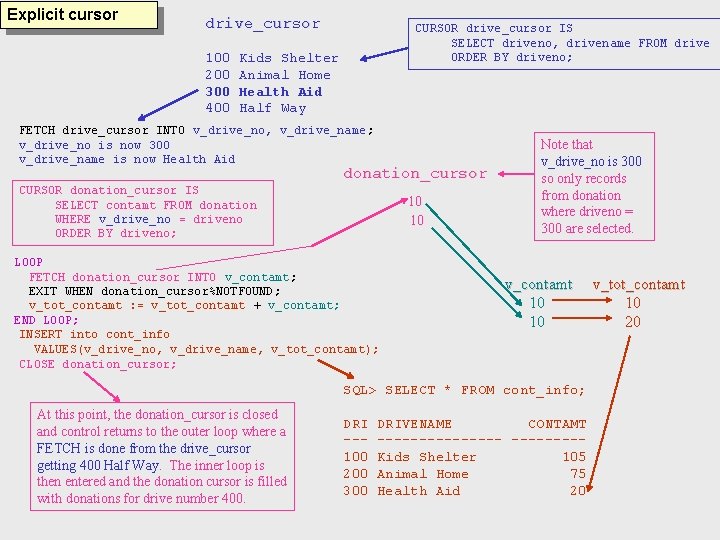
Explicit cursor drive_cursor 100 200 300 400 CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; Kids Shelter Animal Home Health Aid Half Way FETCH drive_cursor INTO v_drive_no, v_drive_name ; v_drive_no is now 300 v_drive_name is now Health Aid donation_cursor CURSOR donation_cursor IS SELECT contamt FROM donation WHERE v_drive_no = driveno ORDER BY driveno; 10 10 LOOP FETCH donation_cursor INTO v_contamt; EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; END LOOP; INSERT into cont_info VALUES(v_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; Note that v_drive_no is 300 so only records from donation where driveno = 300 are selected. v_contamt 10 10 SQL> SELECT * FROM cont_info; At this point, the donation_cursor is closed and control returns to the outer loop where a FETCH is done from the drive_cursor getting 400 Half Way. The inner loop is then entered and the donation cursor is filled with donations for drive number 400. DRI --100 200 300 DRIVENAME CONTAMT --------Kids Shelter 105 Animal Home 75 Health Aid 20 v_tot_contamt 10 20
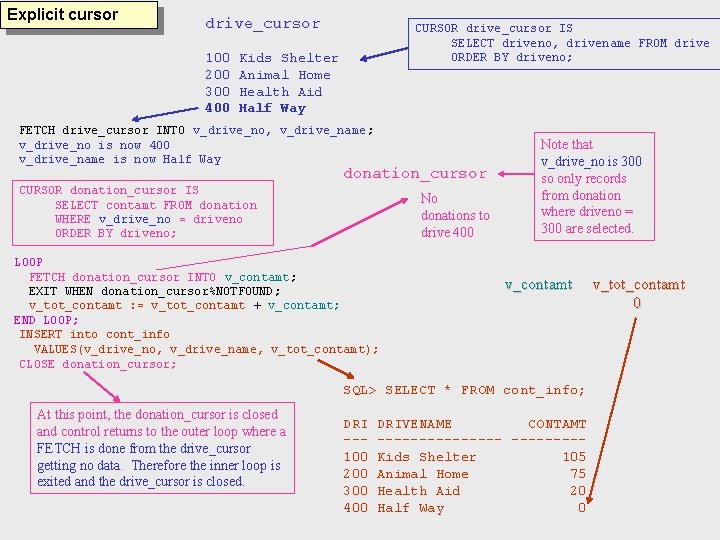
Explicit cursor drive_cursor 100 200 300 400 CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; Kids Shelter Animal Home Health Aid Half Way FETCH drive_cursor INTO v_drive_no, v_drive_name ; v_drive_no is now 400 v_drive_name is now Half Way donation_cursor CURSOR donation_cursor IS SELECT contamt FROM donation WHERE v_drive_no = driveno ORDER BY driveno; No donations to drive 400 LOOP FETCH donation_cursor INTO v_contamt; EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; END LOOP; INSERT into cont_info VALUES(v_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; Note that v_drive_no is 300 so only records from donation where driveno = 300 are selected. v_contamt SQL> SELECT * FROM cont_info; At this point, the donation_cursor is closed and control returns to the outer loop where a FETCH is done from the drive_cursor getting no data. Therefore the inner loop is exited and the drive_cursor is closed. DRI --100 200 300 400 DRIVENAME CONTAMT --------Kids Shelter 105 Animal Home 75 Health Aid 20 Half Way 0 v_tot_contamt 0
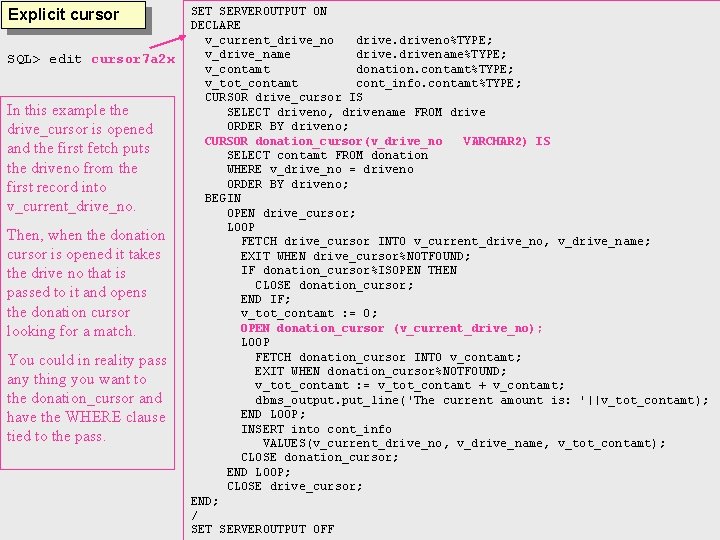
Explicit cursor SQL> edit cursor 7 a 2 x In this example the drive_cursor is opened and the first fetch puts the driveno from the first record into v_current_drive_no. Then, when the donation cursor is opened it takes the drive no that is passed to it and opens the donation cursor looking for a match. You could in reality pass any thing you want to the donation_cursor and have the WHERE clause tied to the pass. SET SERVEROUTPUT ON DECLARE v_current_drive_no driveno%TYPE; v_drive_name drivename%TYPE; v_contamt donation. contamt%TYPE; v_tot_contamt cont_info. contamt%TYPE; CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; CURSOR donation_cursor(v_drive_no VARCHAR 2) IS SELECT contamt FROM donation WHERE v_drive_no = driveno ORDER BY driveno; BEGIN OPEN drive_cursor; LOOP FETCH drive_cursor INTO v_current_drive_no, v_drive_name; EXIT WHEN drive_cursor%NOTFOUND; IF donation_cursor%ISOPEN THEN CLOSE donation_cursor; END IF; v_tot_contamt : = 0; OPEN donation_cursor (v_current_drive_no); LOOP FETCH donation_cursor INTO v_contamt; EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; dbms_output. put_line('The current amount is: '||v_tot_contamt); END LOOP; INSERT into cont_info VALUES(v_current_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; END LOOP; CLOSE drive_cursor; END; / SET SERVEROUTPUT OFF
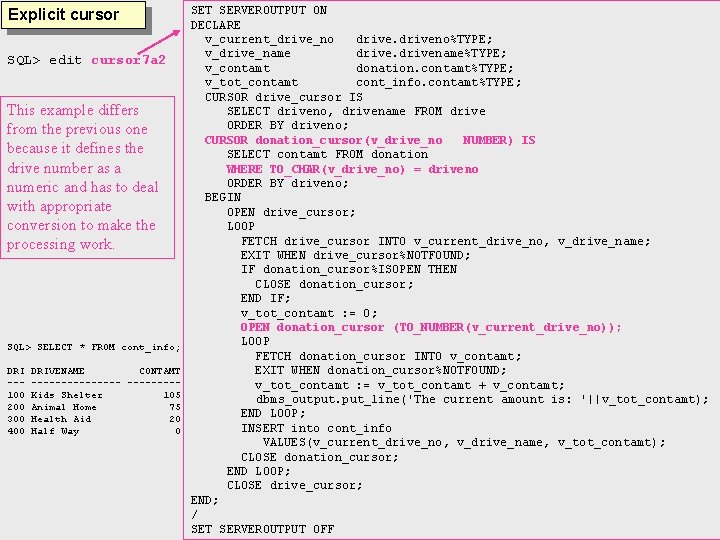
Explicit cursor SQL> edit cursor 7 a 2 This example differs from the previous one because it defines the drive number as a numeric and has to deal with appropriate conversion to make the processing work. SQL> SELECT * FROM cont_info; DRI --100 200 300 400 DRIVENAME CONTAMT --------Kids Shelter 105 Animal Home 75 Health Aid 20 Half Way 0 SET SERVEROUTPUT ON DECLARE v_current_drive_no driveno%TYPE; v_drive_name drivename%TYPE; v_contamt donation. contamt%TYPE; v_tot_contamt cont_info. contamt%TYPE; CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; CURSOR donation_cursor(v_drive_no NUMBER) IS SELECT contamt FROM donation WHERE TO_CHAR(v_drive_no) = driveno ORDER BY driveno; BEGIN OPEN drive_cursor; LOOP FETCH drive_cursor INTO v_current_drive_no, v_drive_name; EXIT WHEN drive_cursor%NOTFOUND; IF donation_cursor%ISOPEN THEN CLOSE donation_cursor; END IF; v_tot_contamt : = 0; OPEN donation_cursor (TO_NUMBER(v_current_drive_no)); LOOP FETCH donation_cursor INTO v_contamt; EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; dbms_output. put_line('The current amount is: '||v_tot_contamt); END LOOP; INSERT into cont_info VALUES(v_current_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; END LOOP; CLOSE drive_cursor; END; / SET SERVEROUTPUT OFF
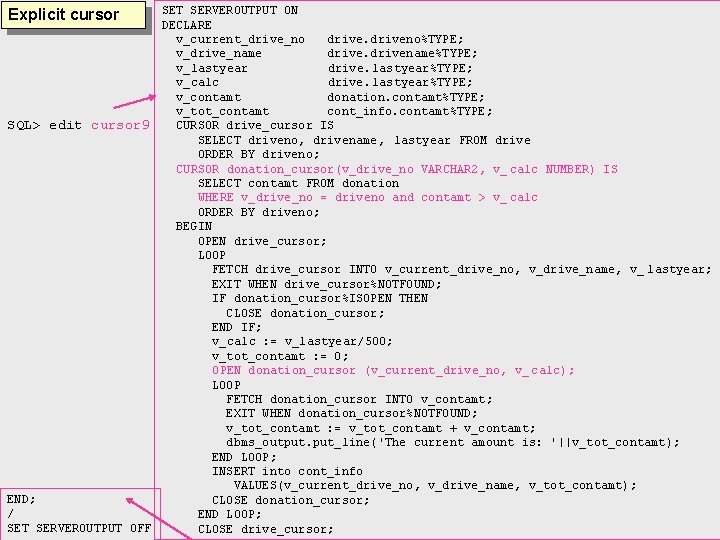
SET SERVEROUTPUT ON DECLARE v_current_drive_no driveno%TYPE; v_drive_name drivename%TYPE; v_lastyear drive. lastyear%TYPE; v_calc drive. lastyear%TYPE; v_contamt donation. contamt%TYPE; v_tot_contamt cont_info. contamt%TYPE; SQL> edit cursor 9 CURSOR drive_cursor IS SELECT driveno, drivename, lastyear FROM drive ORDER BY driveno; CURSOR donation_cursor(v_drive_no VARCHAR 2, v_ calc NUMBER) IS SELECT contamt FROM donation WHERE v_drive_no = driveno and contamt > v_ calc ORDER BY driveno; BEGIN OPEN drive_cursor; LOOP FETCH drive_cursor INTO v_current_drive_no, v_drive_name, v_ lastyear; EXIT WHEN drive_cursor%NOTFOUND; IF donation_cursor%ISOPEN THEN CLOSE donation_cursor; END IF; v_calc : = v_lastyear/500; v_tot_contamt : = 0; OPEN donation_cursor (v_current_drive_no, v_ calc); LOOP FETCH donation_cursor INTO v_contamt; EXIT WHEN donation_cursor%NOTFOUND; v_tot_contamt : = v_tot_contamt + v_contamt; dbms_output. put_line('The current amount is: '||v_tot_contamt); END LOOP; INSERT into cont_info VALUES(v_current_drive_no, v_drive_name, v_tot_contamt); END; CLOSE donation_cursor; / END LOOP; SET SERVEROUTPUT OFF CLOSE drive_cursor; Explicit cursor
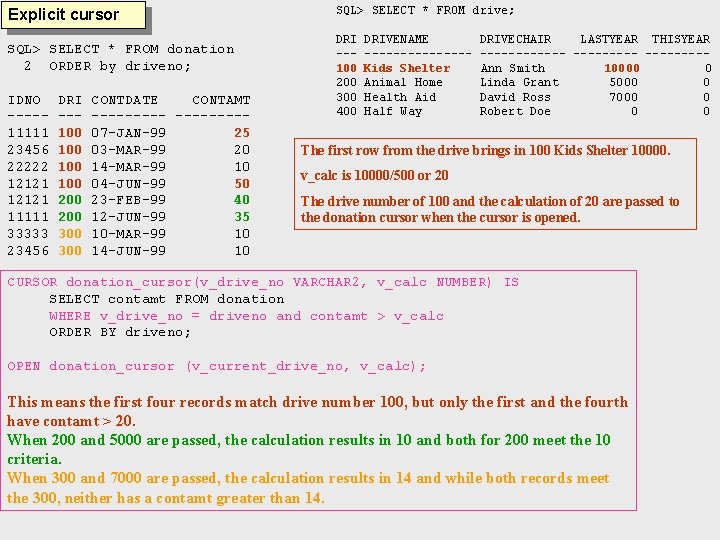
Explicit cursor SQL> SELECT * FROM donation 2 ORDER by driveno; IDNO ----11111 23456 22222 12121 11111 33333 23456 DRI --100 100 200 300 CONTDATE CONTAMT -----07 -JAN-99 25 03 -MAR-99 20 14 -MAR-99 10 04 -JUN-99 50 23 -FEB-99 40 12 -JUN-99 35 10 -MAR-99 10 14 -JUN-99 10 SQL> SELECT * FROM drive; DRI --100 200 300 400 DRIVENAME -------Kids Shelter Animal Home Health Aid Half Way DRIVECHAIR LASTYEAR THISYEAR ------ ----Ann Smith 10000 0 Linda Grant 5000 0 David Ross 7000 0 Robert Doe 0 0 The first row from the drive brings in 100 Kids Shelter 10000. v_calc is 10000/500 or 20 The drive number of 100 and the calculation of 20 are passed to the donation cursor when the cursor is opened. CURSOR donation_cursor(v_drive_no VARCHAR 2, v_calc NUMBER) IS SELECT contamt FROM donation WHERE v_drive_no = driveno and contamt > v_calc ORDER BY driveno; OPEN donation_cursor (v_current_drive_no, v_calc); This means the first four records match drive number 100, but only the first and the fourth have contamt > 20. When 200 and 5000 are passed, the calculation results in 10 and both for 200 meet the 10 criteria. When 300 and 7000 are passed, the calculation results in 14 and while both records meet the 300, neither has a contamt greater than 14.
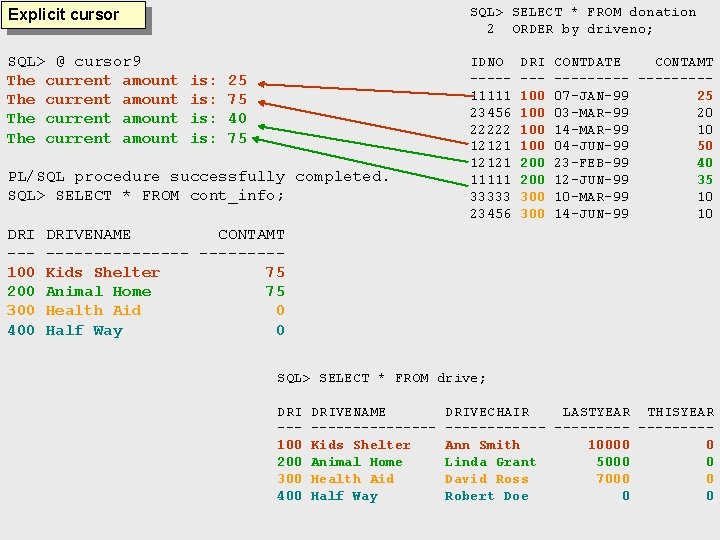
Explicit cursor SQL> SELECT * FROM donation 2 ORDER by driveno; SQL> @ cursor 9 The current amount IDNO ----11111 23456 22222 12121 11111 33333 23456 is: is: 25 75 40 75 PL/SQL procedure successfully completed. SQL> SELECT * FROM cont_info; DRI --100 200 300 400 DRI --100 100 200 300 CONTDATE CONTAMT -----07 -JAN-99 25 03 -MAR-99 20 14 -MAR-99 10 04 -JUN-99 50 23 -FEB-99 40 12 -JUN-99 35 10 -MAR-99 10 14 -JUN-99 10 DRIVENAME CONTAMT --------Kids Shelter 75 Animal Home 75 Health Aid 0 Half Way 0 SQL> SELECT * FROM drive; DRI --100 200 300 400 DRIVENAME -------Kids Shelter Animal Home Health Aid Half Way DRIVECHAIR LASTYEAR THISYEAR ------ ----Ann Smith 10000 0 Linda Grant 5000 0 David Ross 7000 0 Robert Doe 0 0
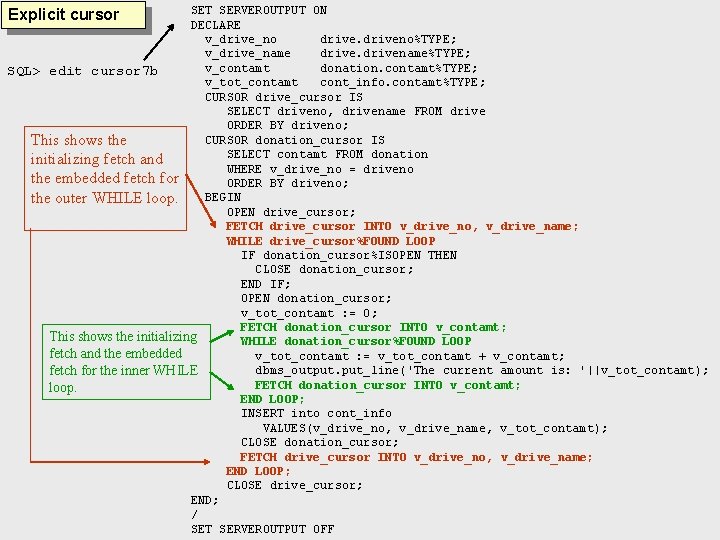
SET SERVEROUTPUT ON DECLARE v_drive_no driveno%TYPE; v_drive_name drivename%TYPE; v_contamt donation. contamt%TYPE; SQL> edit cursor 7 b v_tot_contamt cont_info. contamt%TYPE; CURSOR drive_cursor IS SELECT driveno, drivename FROM drive ORDER BY driveno; CURSOR donation_cursor IS This shows the SELECT contamt FROM donation initializing fetch and WHERE v_drive_no = driveno the embedded fetch for ORDER BY driveno; BEGIN the outer WHILE loop. OPEN drive_cursor; FETCH drive_cursor INTO v_drive_no, v_drive_name; WHILE drive_cursor%FOUND LOOP IF donation_cursor%ISOPEN THEN CLOSE donation_cursor; END IF; OPEN donation_cursor; v_tot_contamt : = 0; FETCH donation_cursor INTO v_contamt; This shows the initializing WHILE donation_cursor%FOUND LOOP fetch and the embedded v_tot_contamt : = v_tot_contamt + v_contamt; dbms_output. put_line('The current amount is: '||v_tot_contamt); fetch for the inner WHILE FETCH donation_cursor INTO v_contamt; loop. END LOOP; INSERT into cont_info VALUES(v_drive_no, v_drive_name, v_tot_contamt); CLOSE donation_cursor; FETCH drive_cursor INTO v_drive_no, v_drive_name; END LOOP; CLOSE drive_cursor; END; / SET SERVEROUTPUT OFF Explicit cursor