Ctutorial Part II Prakash Linga lingacs cornell edu
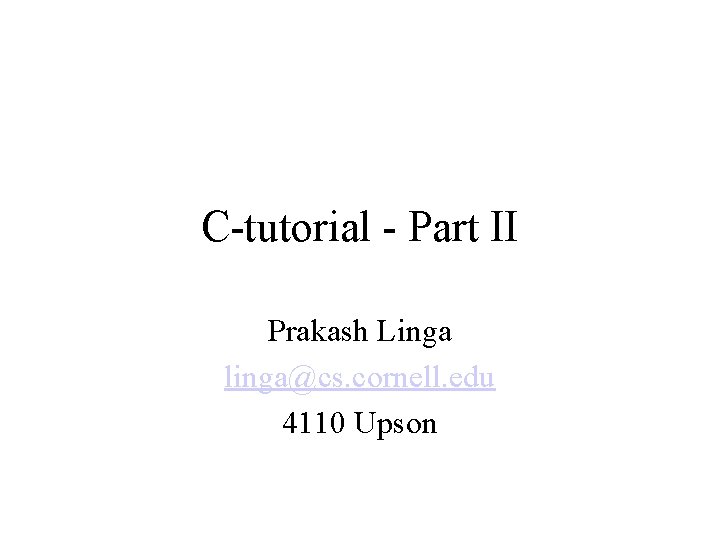
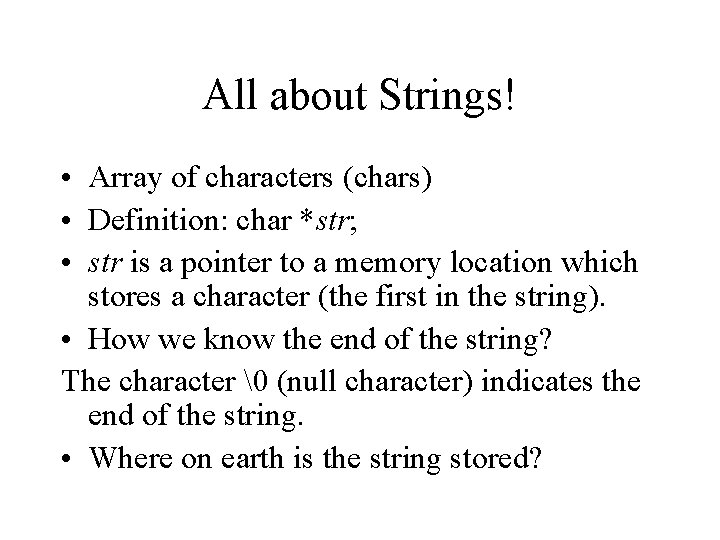
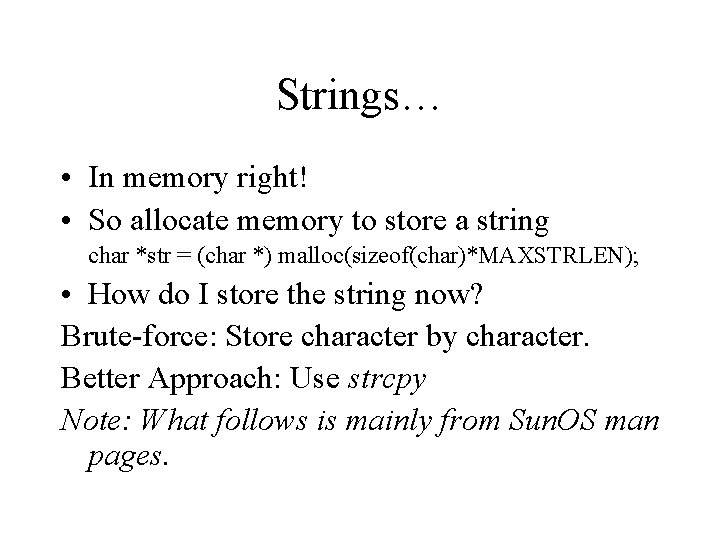
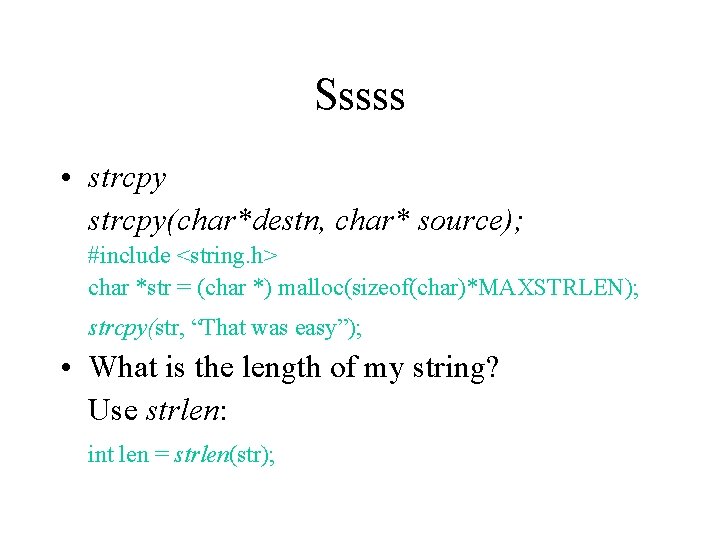
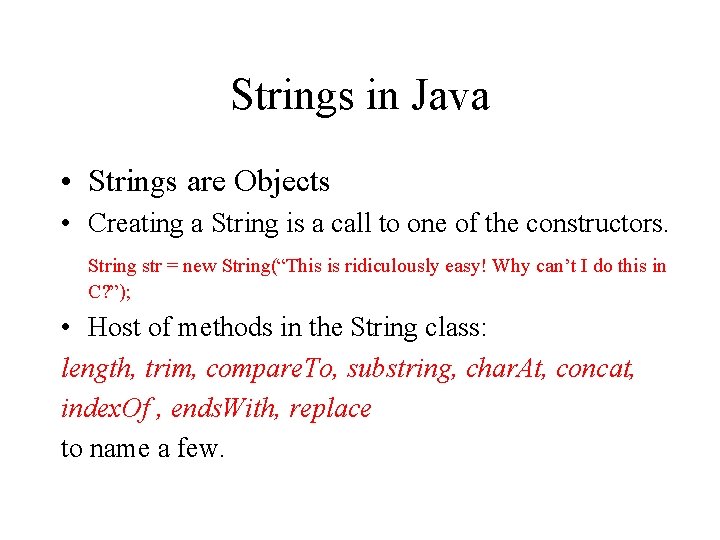
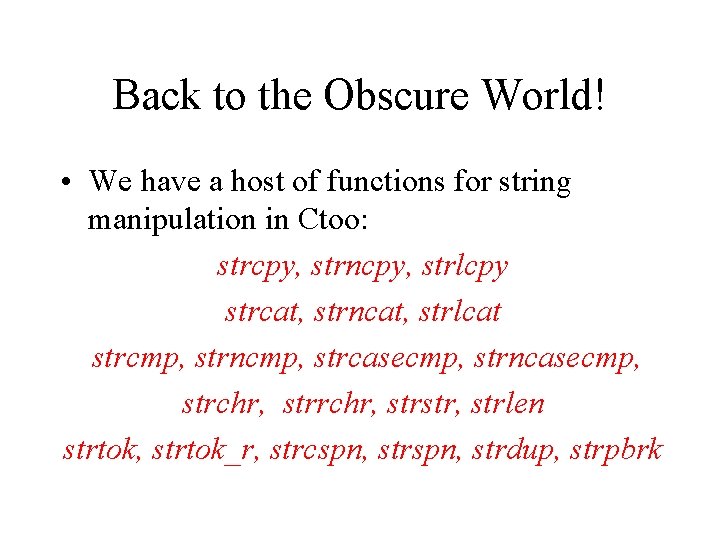
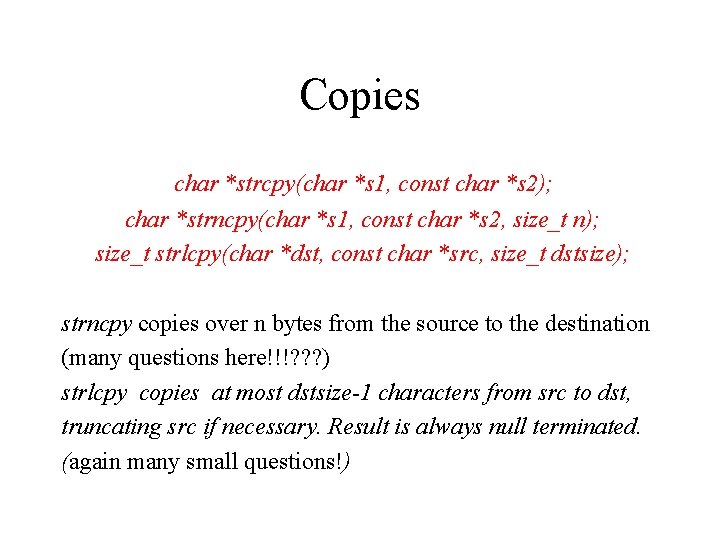
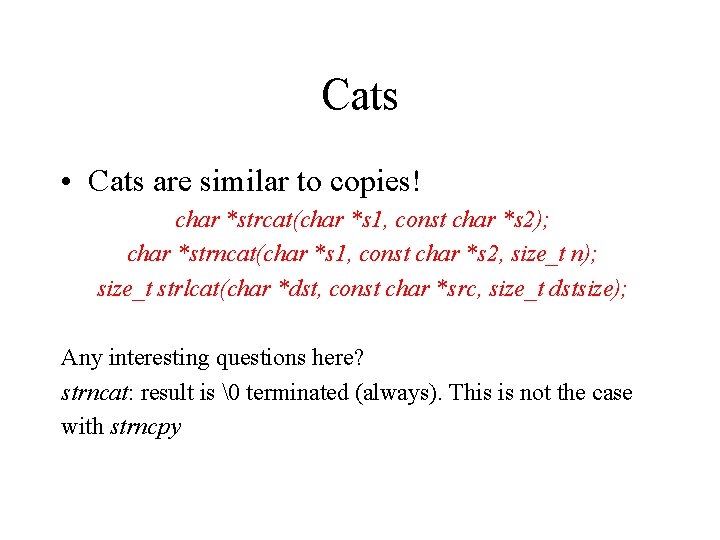
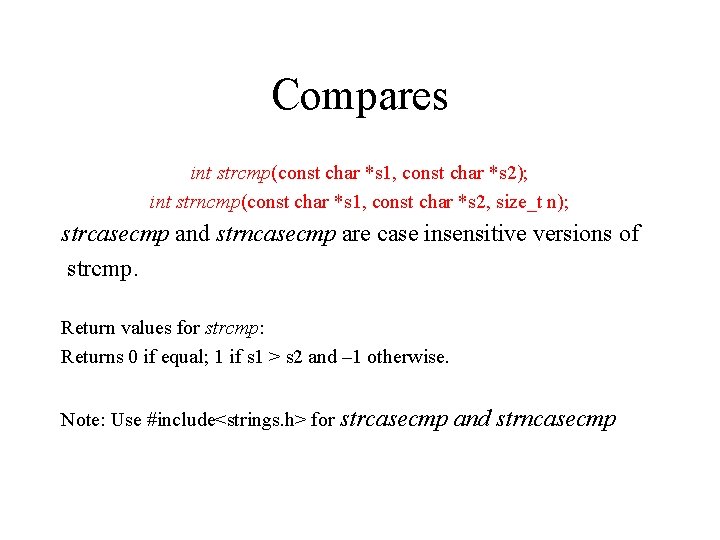
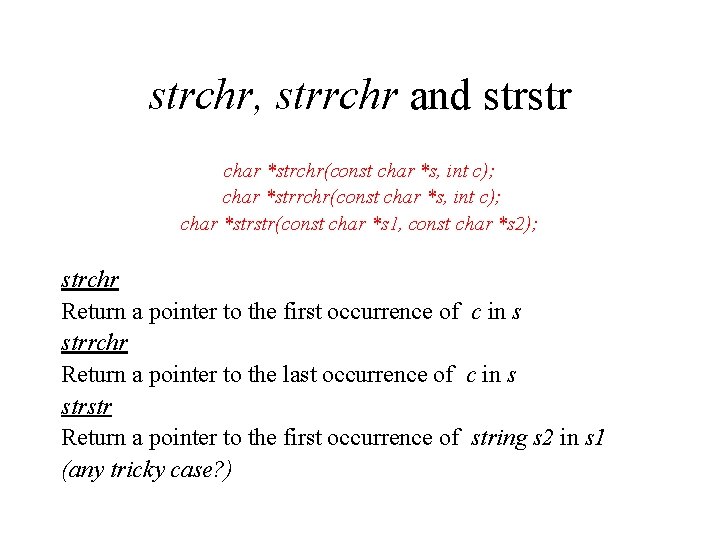
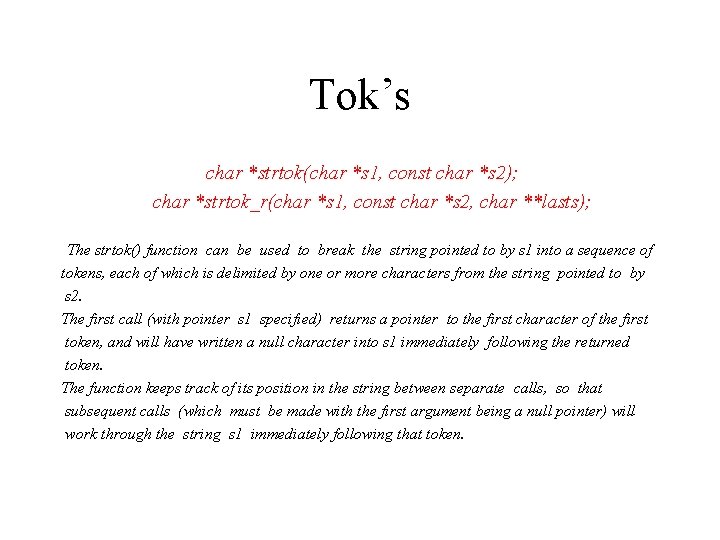
![Strtok example #include <string. h> int main () { char str[100]; char * tmp; Strtok example #include <string. h> int main () { char str[100]; char * tmp;](https://slidetodoc.com/presentation_image_h/e98a82a7713df9a11059e3c510fef3a2/image-12.jpg)
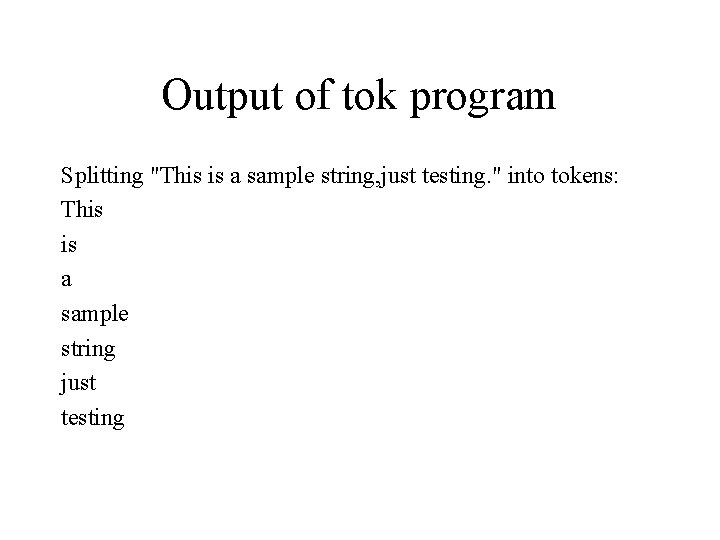
![Same example with strtok_r #include <string. h> int main () { char str[100]; char Same example with strtok_r #include <string. h> int main () { char str[100]; char](https://slidetodoc.com/presentation_image_h/e98a82a7713df9a11059e3c510fef3a2/image-14.jpg)
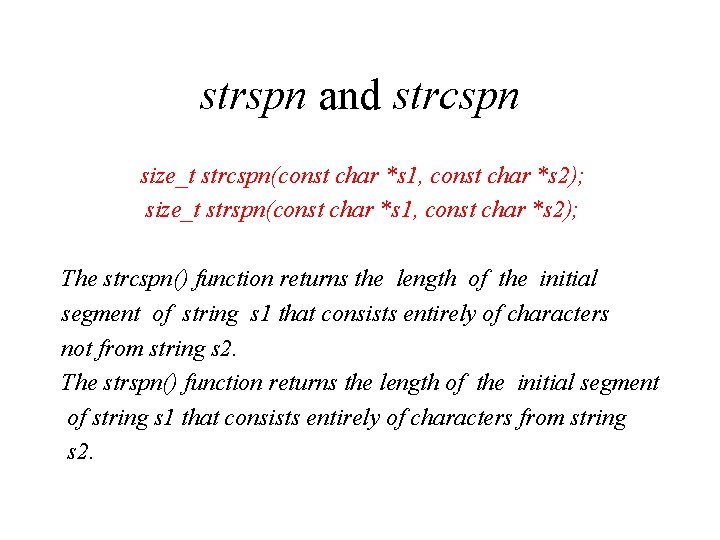
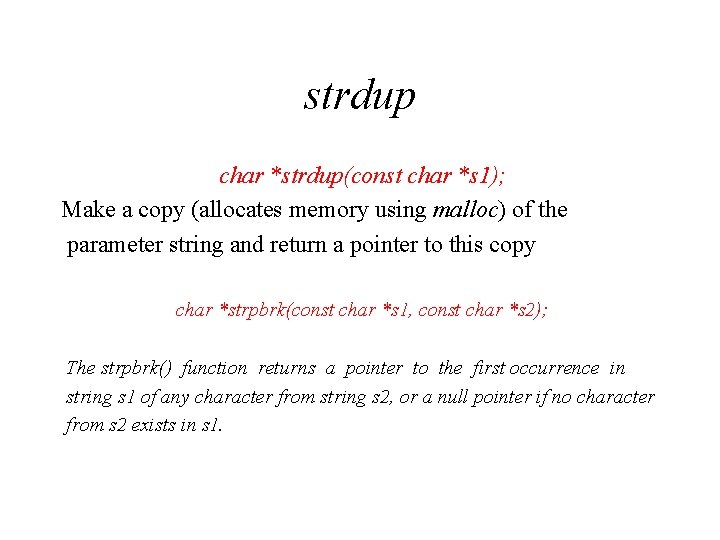
- Slides: 16
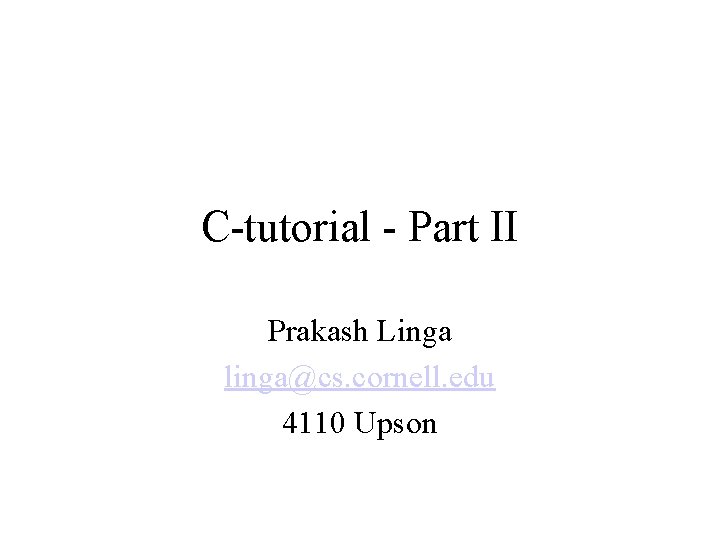
C-tutorial - Part II Prakash Linga linga@cs. cornell. edu 4110 Upson
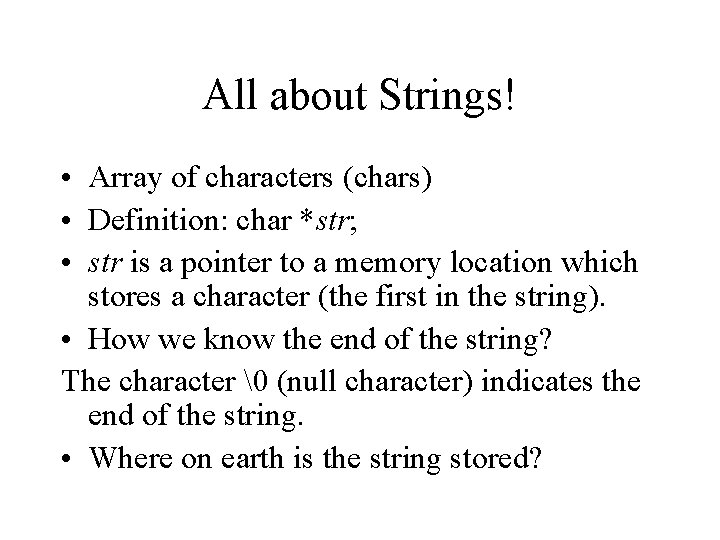
All about Strings! • Array of characters (chars) • Definition: char *str; • str is a pointer to a memory location which stores a character (the first in the string). • How we know the end of the string? The character (null character) indicates the end of the string. • Where on earth is the string stored?
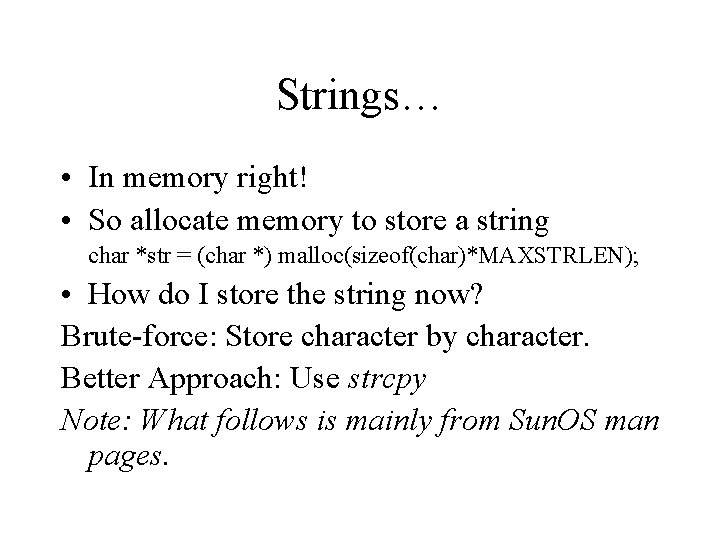
Strings… • In memory right! • So allocate memory to store a string char *str = (char *) malloc(sizeof(char)*MAXSTRLEN); • How do I store the string now? Brute-force: Store character by character. Better Approach: Use strcpy Note: What follows is mainly from Sun. OS man pages.
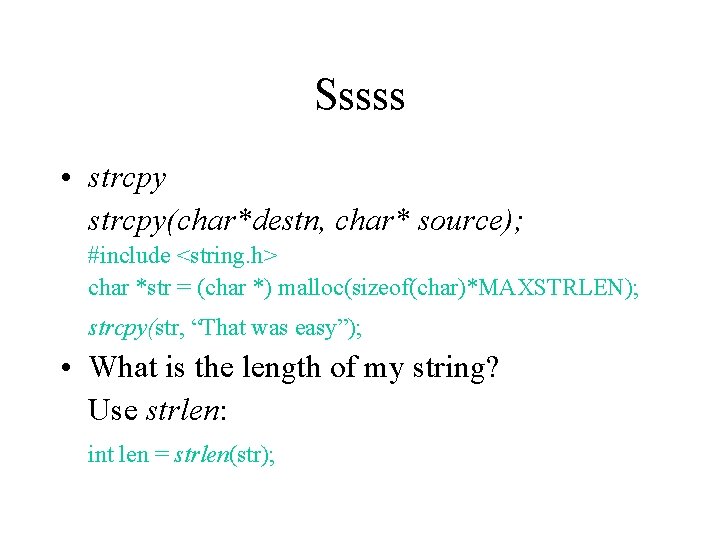
Sssss • strcpy(char*destn, char* source); #include <string. h> char *str = (char *) malloc(sizeof(char)*MAXSTRLEN); strcpy(str, “That was easy”); • What is the length of my string? Use strlen: int len = strlen(str);
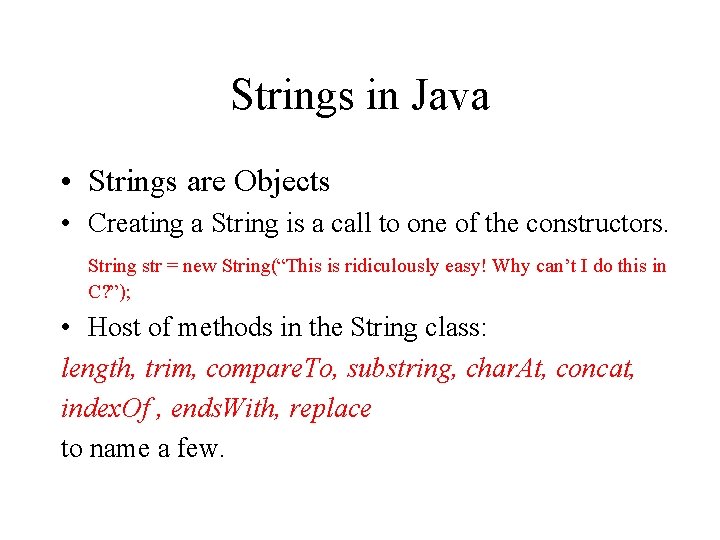
Strings in Java • Strings are Objects • Creating a String is a call to one of the constructors. String str = new String(“This is ridiculously easy! Why can’t I do this in C? ”); • Host of methods in the String class: length, trim, compare. To, substring, char. At, concat, index. Of , ends. With, replace to name a few.
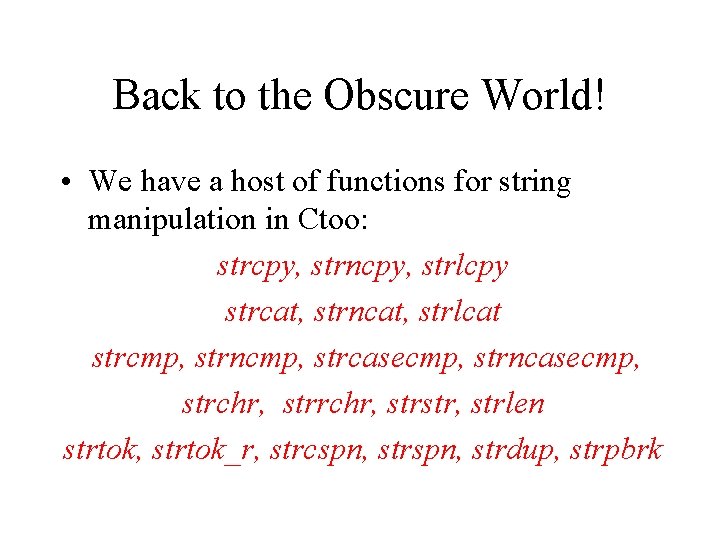
Back to the Obscure World! • We have a host of functions for string manipulation in Ctoo: strcpy, strncpy, strlcpy strcat, strncat, strlcat strcmp, strncmp, strcasecmp, strncasecmp, strchr, strstr, strlen strtok, strtok_r, strcspn, strdup, strpbrk
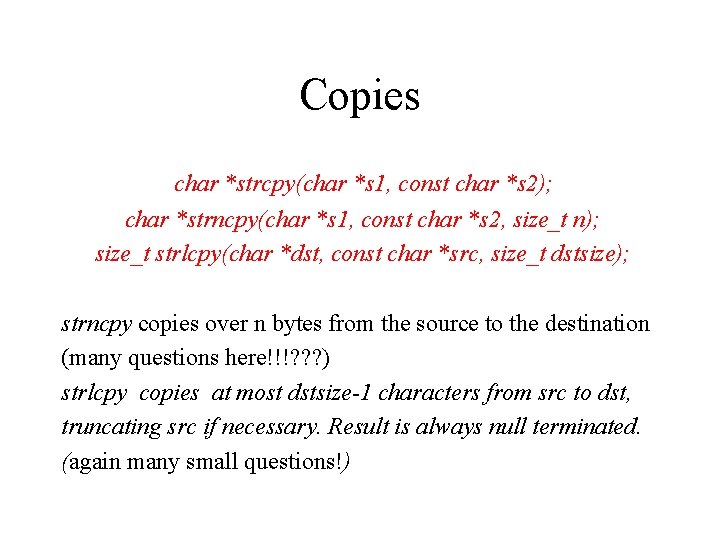
Copies char *strcpy(char *s 1, const char *s 2); char *strncpy(char *s 1, const char *s 2, size_t n); size_t strlcpy(char *dst, const char *src, size_t dstsize); strncpy copies over n bytes from the source to the destination (many questions here!!!? ? ? ) strlcpy copies at most dstsize-1 characters from src to dst, truncating src if necessary. Result is always null terminated. (again many small questions!)
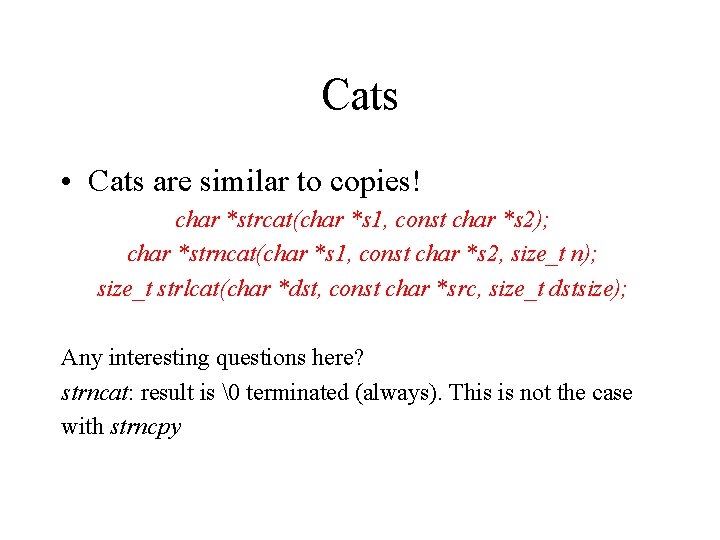
Cats • Cats are similar to copies! char *strcat(char *s 1, const char *s 2); char *strncat(char *s 1, const char *s 2, size_t n); size_t strlcat(char *dst, const char *src, size_t dstsize); Any interesting questions here? strncat: result is terminated (always). This is not the case with strncpy
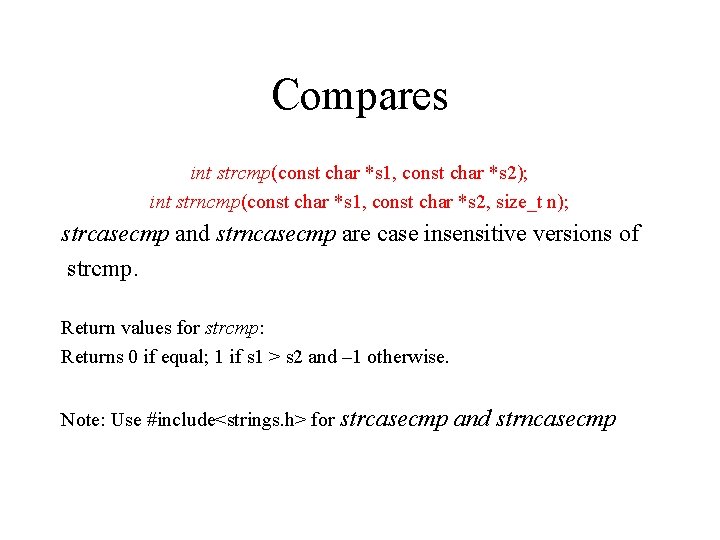
Compares int strcmp(const char *s 1, const char *s 2); int strncmp(const char *s 1, const char *s 2, size_t n); strcasecmp and strncasecmp are case insensitive versions of strcmp. Return values for strcmp: Returns 0 if equal; 1 if s 1 > s 2 and – 1 otherwise. Note: Use #include<strings. h> for strcasecmp and strncasecmp
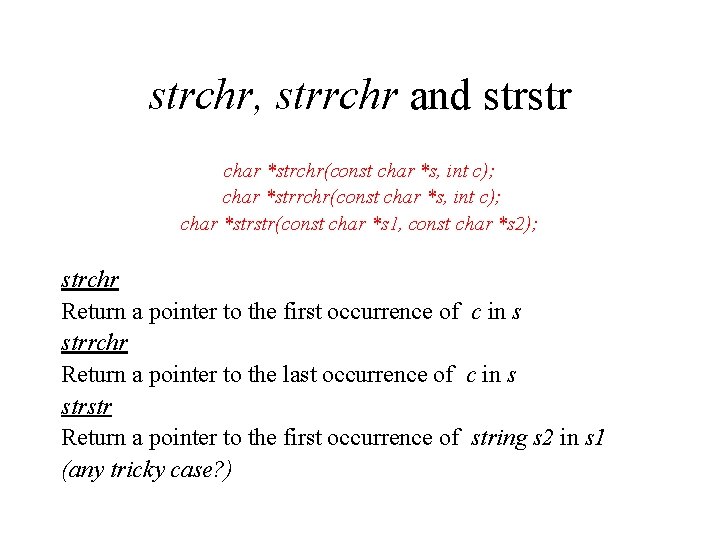
strchr, strrchr and strstr char *strchr(const char *s, int c); char *strstr(const char *s 1, const char *s 2); strchr Return a pointer to the first occurrence of c in s strrchr Return a pointer to the last occurrence of c in s strstr Return a pointer to the first occurrence of string s 2 in s 1 (any tricky case? )
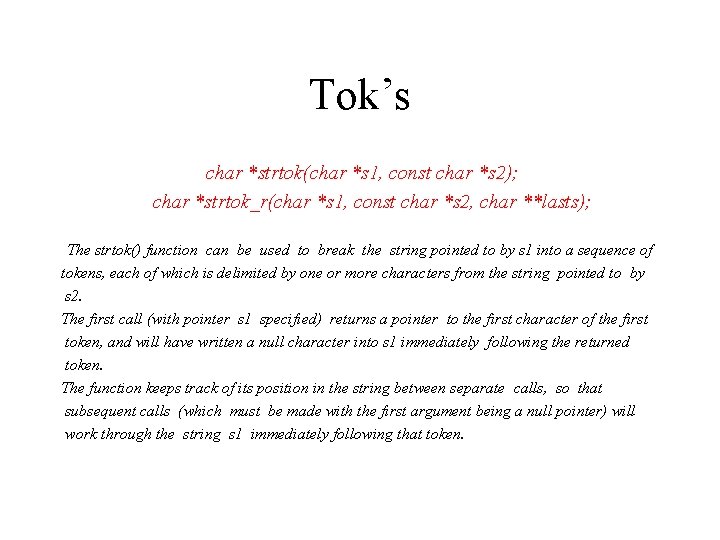
Tok’s char *strtok(char *s 1, const char *s 2); char *strtok_r(char *s 1, const char *s 2, char **lasts); The strtok() function can be used to break the string pointed to by s 1 into a sequence of tokens, each of which is delimited by one or more characters from the string pointed to by s 2. The first call (with pointer s 1 specified) returns a pointer to the first character of the first token, and will have written a null character into s 1 immediately following the returned token. The function keeps track of its position in the string between separate calls, so that subsequent calls (which must be made with the first argument being a null pointer) will work through the string s 1 immediately following that token.
![Strtok example include string h int main char str100 char tmp Strtok example #include <string. h> int main () { char str[100]; char * tmp;](https://slidetodoc.com/presentation_image_h/e98a82a7713df9a11059e3c510fef3a2/image-12.jpg)
Strtok example #include <string. h> int main () { char str[100]; char * tmp; strcpy(str, "This is a sample string, just testing. "); printf ("Splitting "%s" into tokens: n", str); tmp = strtok (str, " , . "); while (tmp != NULL) { printf ("%sn", tmp); tmp = strtok (NULL, " , . "); } return 0; }
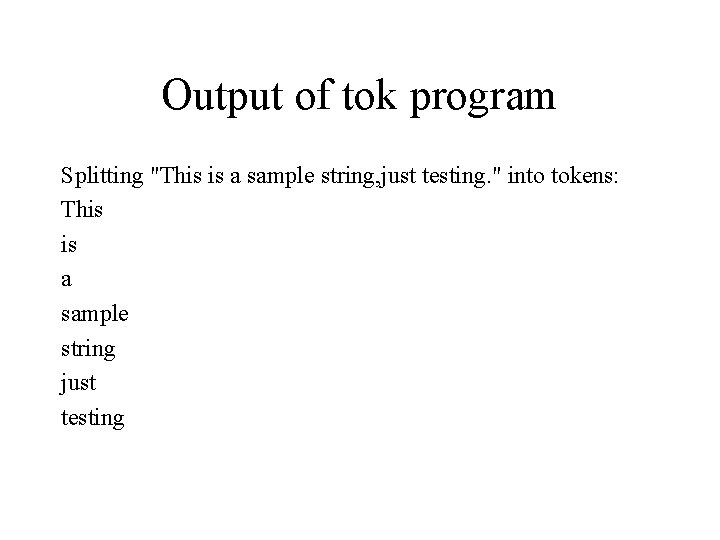
Output of tok program Splitting "This is a sample string, just testing. " into tokens: This is a sample string just testing
![Same example with strtokr include string h int main char str100 char Same example with strtok_r #include <string. h> int main () { char str[100]; char](https://slidetodoc.com/presentation_image_h/e98a82a7713df9a11059e3c510fef3a2/image-14.jpg)
Same example with strtok_r #include <string. h> int main () { char str[100]; char * tmp, *last; strcpy(str, "This is a sample string, just testing. "); printf ("Splitting "%s" into tokens: n", str); tmp = strtok_r (str, " , &last); while (tmp != NULL) { printf ("%sn", tmp); tmp = strtok_r (NULL, " , &last); } return 0; }
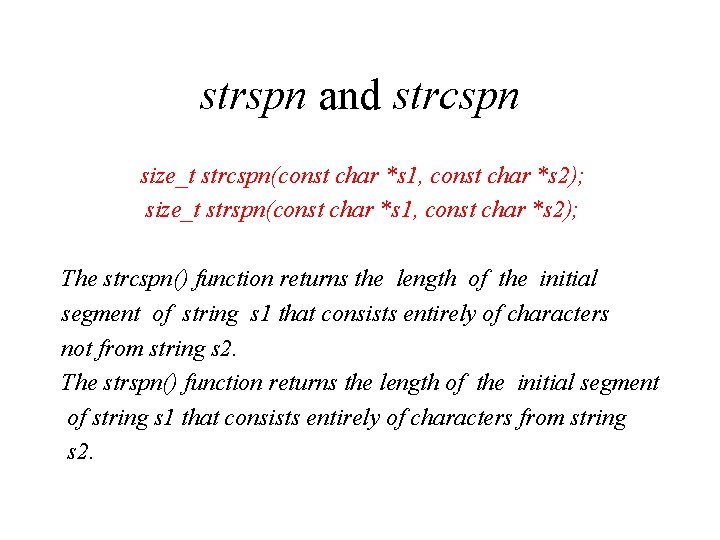
strspn and strcspn size_t strcspn(const char *s 1, const char *s 2); size_t strspn(const char *s 1, const char *s 2); The strcspn() function returns the length of the initial segment of string s 1 that consists entirely of characters not from string s 2. The strspn() function returns the length of the initial segment of string s 1 that consists entirely of characters from string s 2.
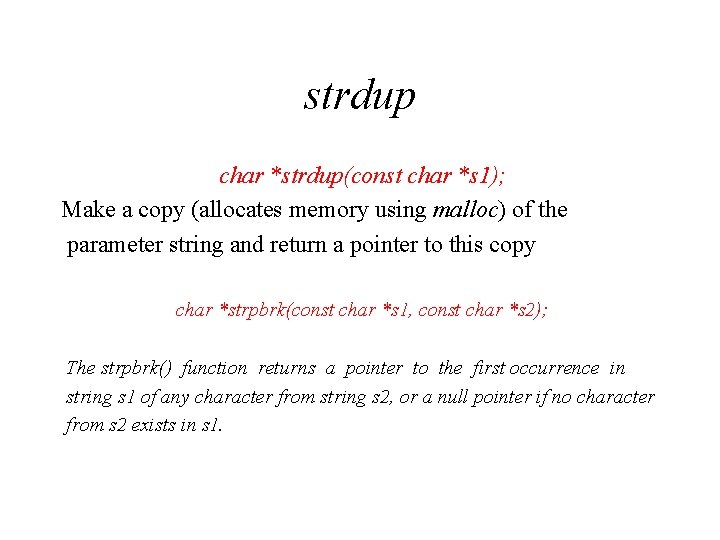
strdup char *strdup(const char *s 1); Make a copy (allocates memory using malloc) of the parameter string and return a pointer to this copy char *strpbrk(const char *s 1, const char *s 2); The strpbrk() function returns a pointer to the first occurrence in string s 1 of any character from string s 2, or a null pointer if no character from s 2 exists in s 1.
Cornell cahrs
Varun mudra
Chetan prakash vs met institute
Nirupama prakash kumar
Arati prakash
Anuradha prakash
Prakash panangaden
Prakash balan nsf
Prakash naidoo
Blackboard cornell edu
Edu.sharif.edu
Brainpop ratios
Parts of a bar graph
Technical description examples
Part whole model subtraction
The phase of the moon you see depends on ______.
Unit ratio definition