CSE 501 N Fall 09 21 Introduction to
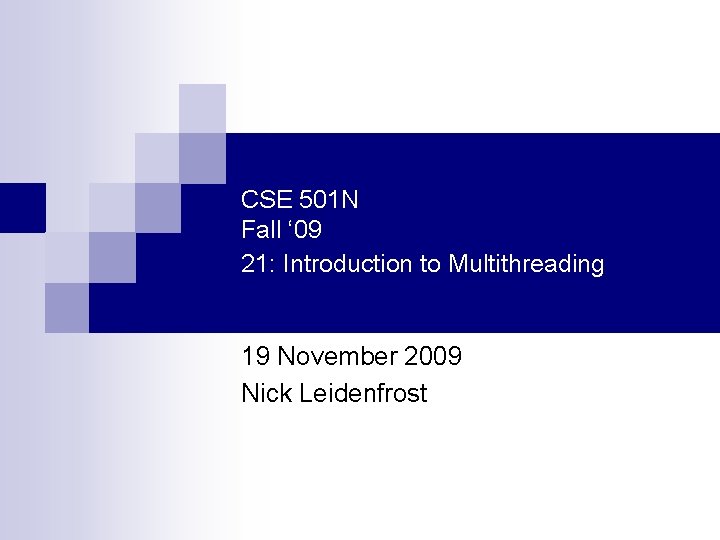
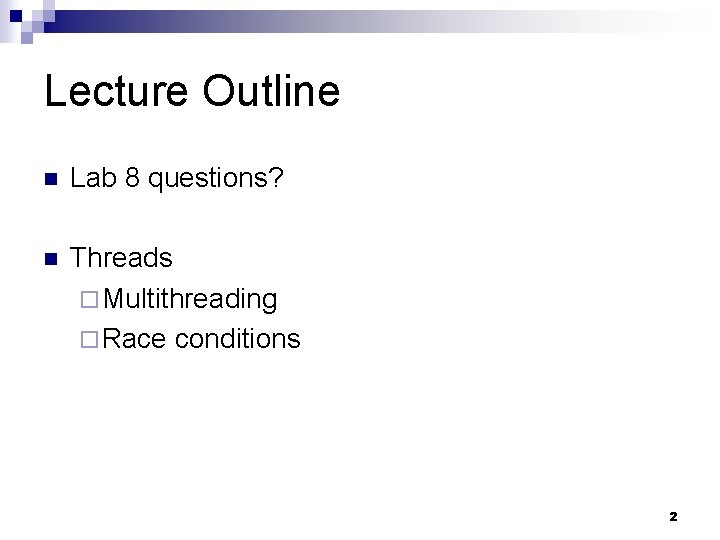
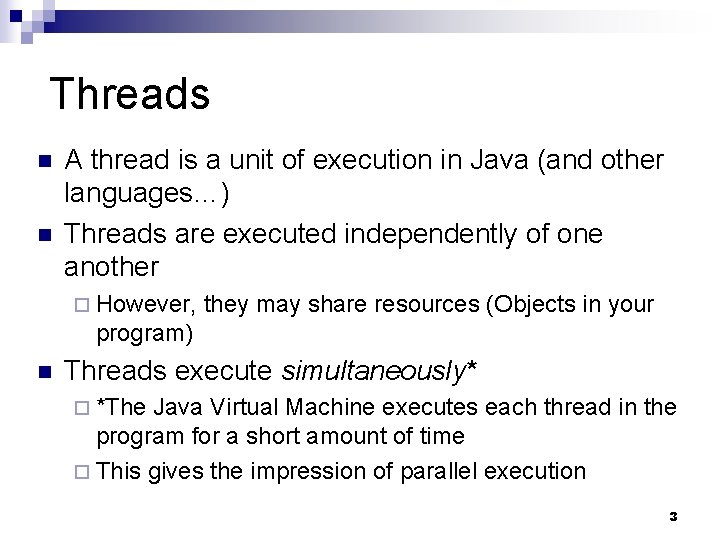
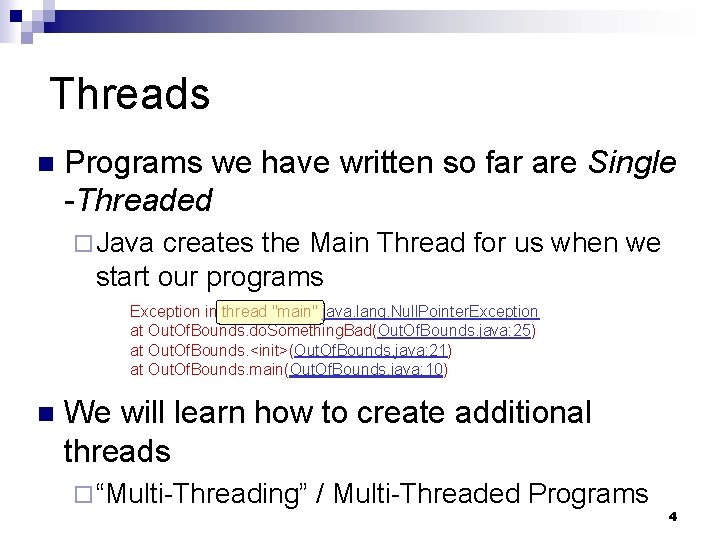
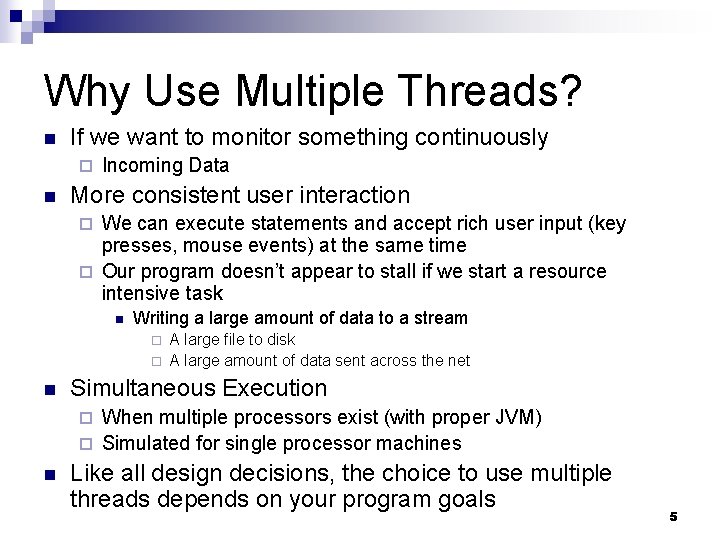
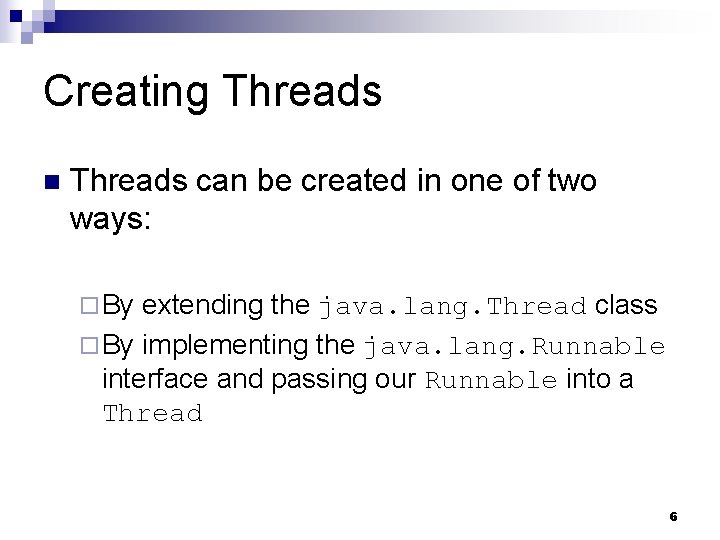
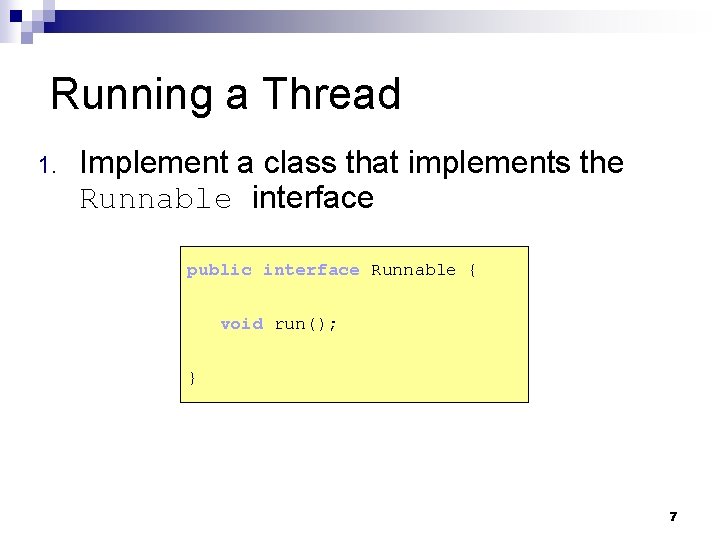
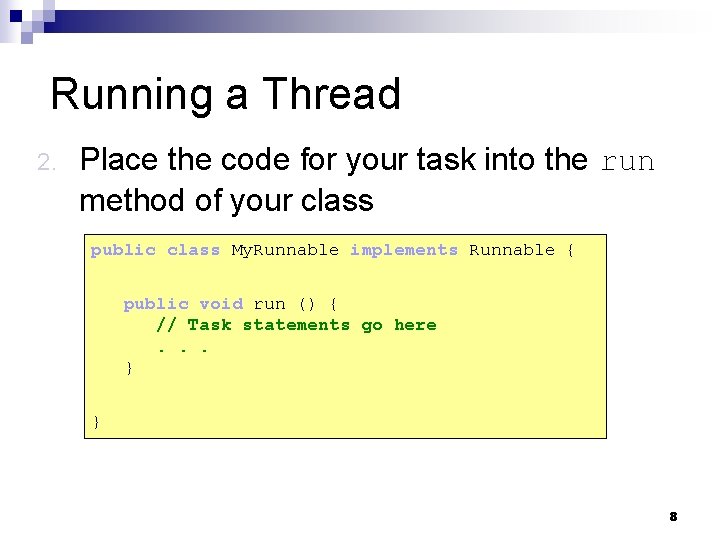
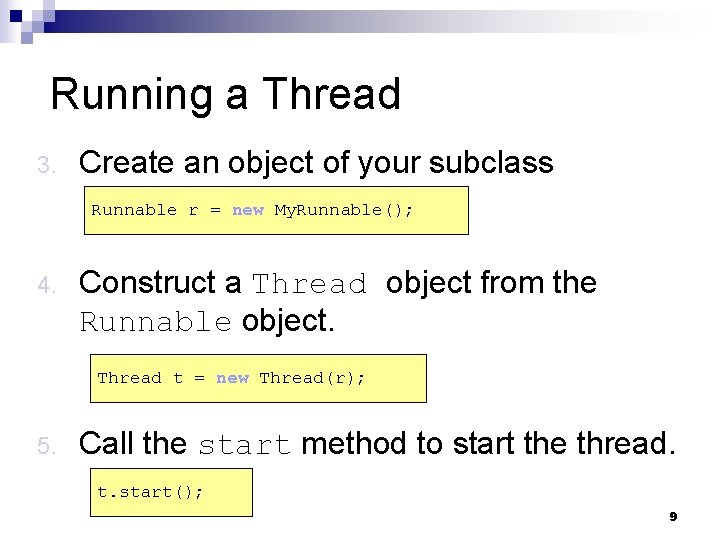
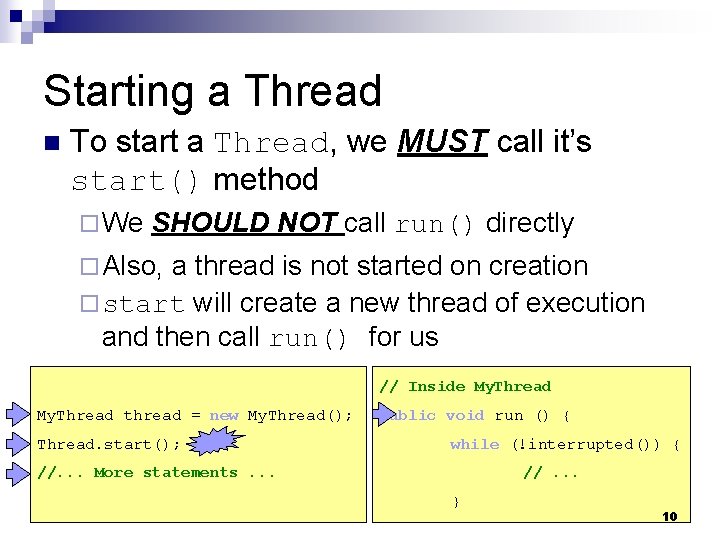
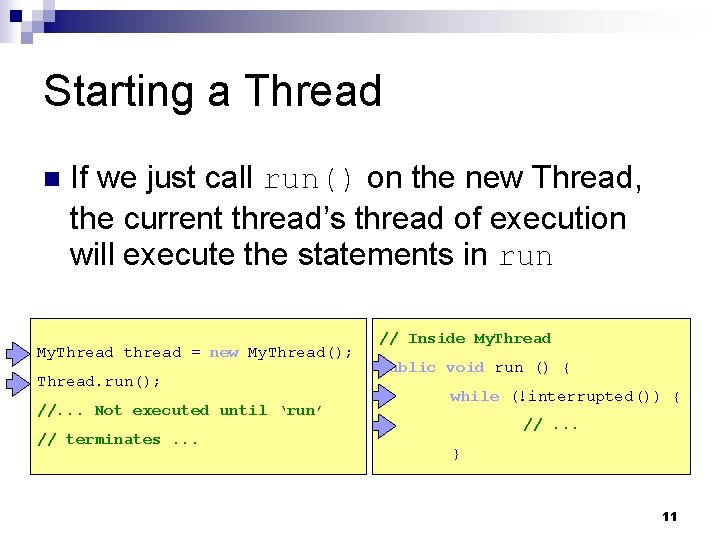
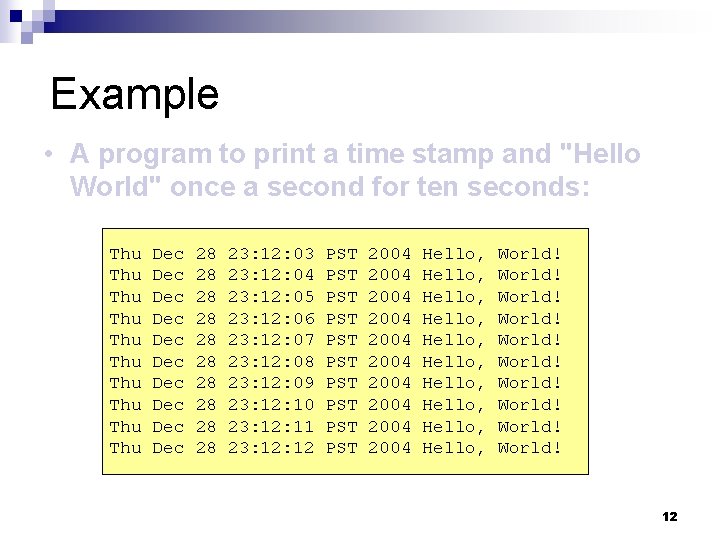
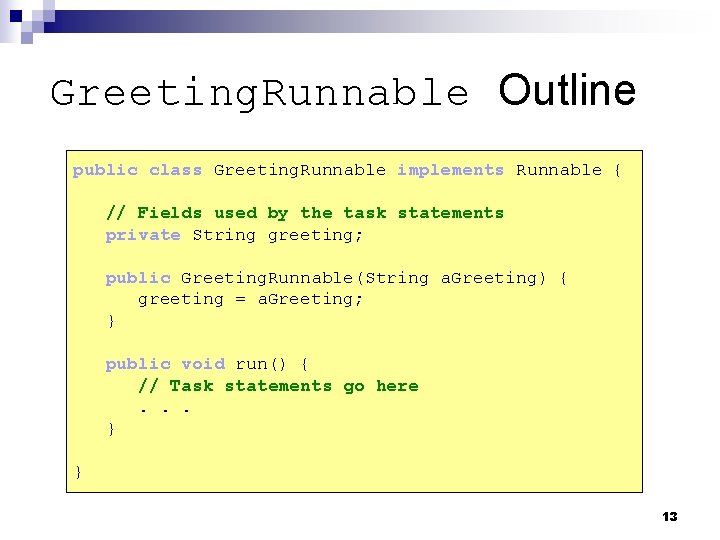
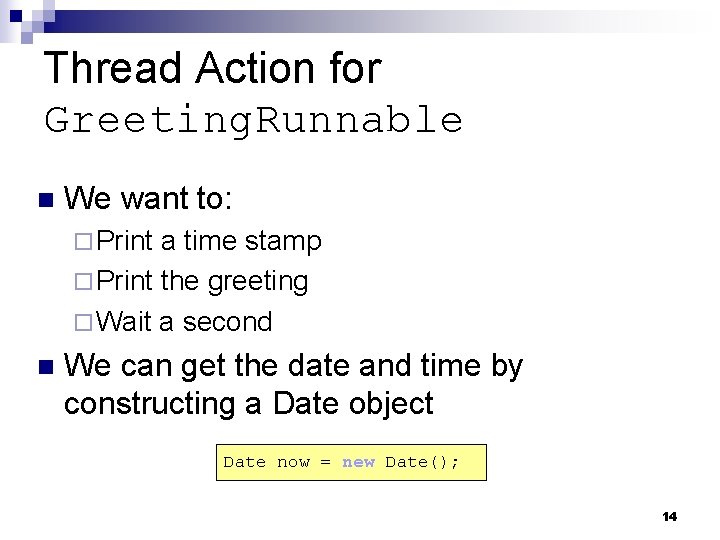
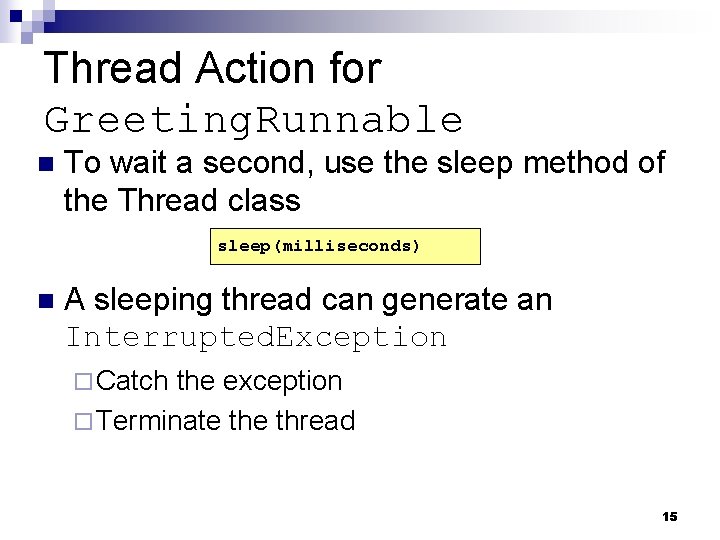
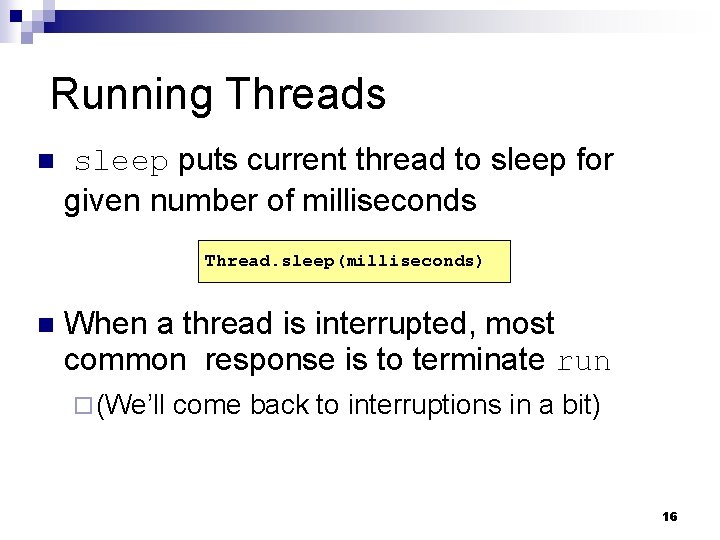
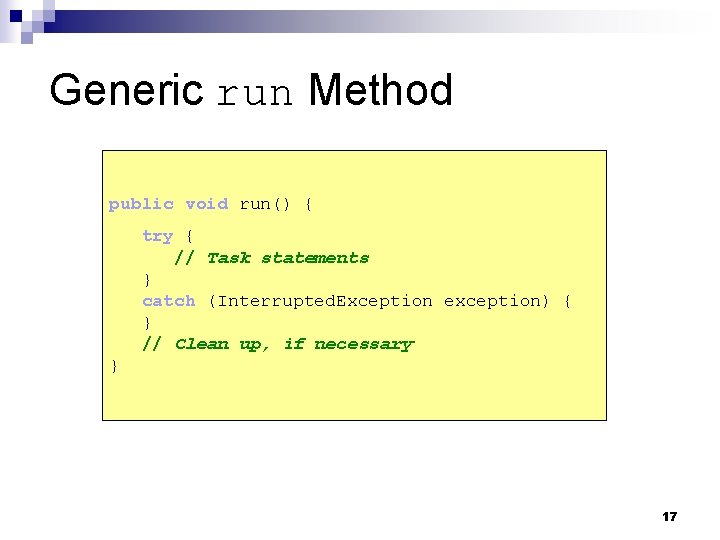
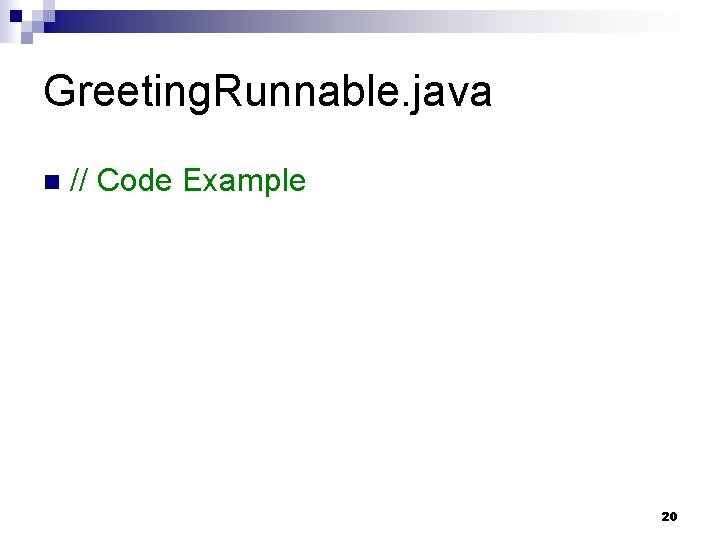
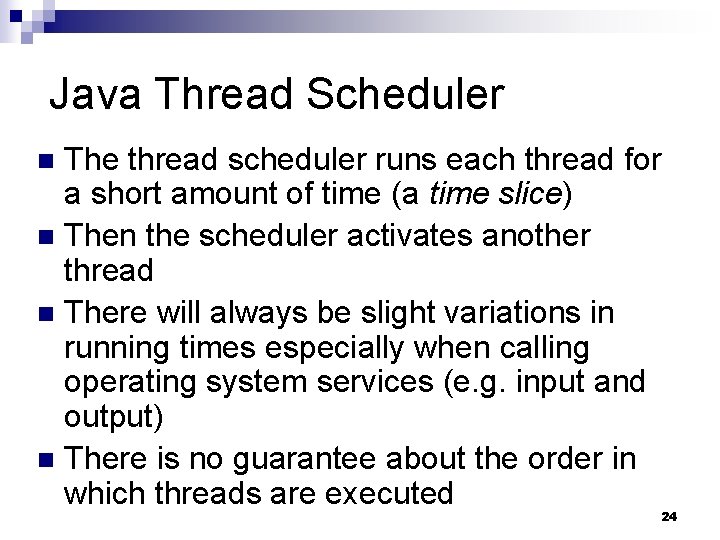
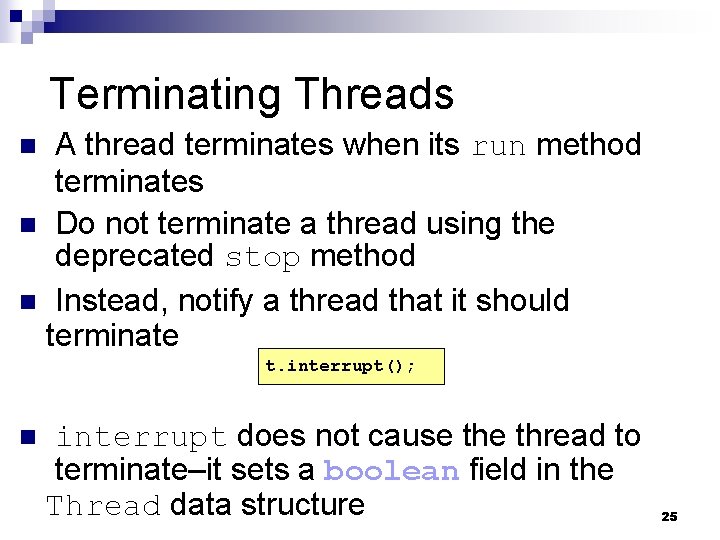
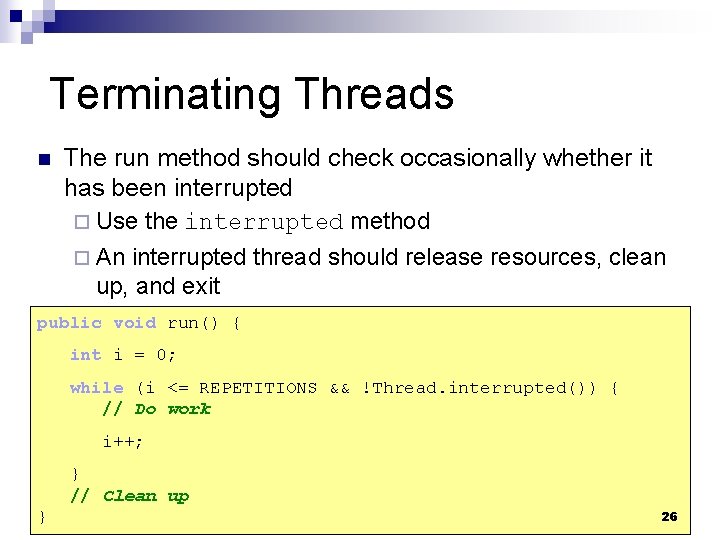
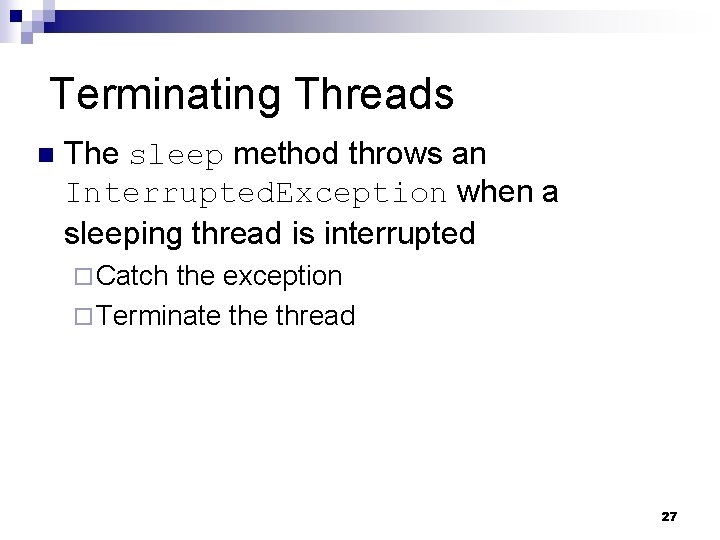
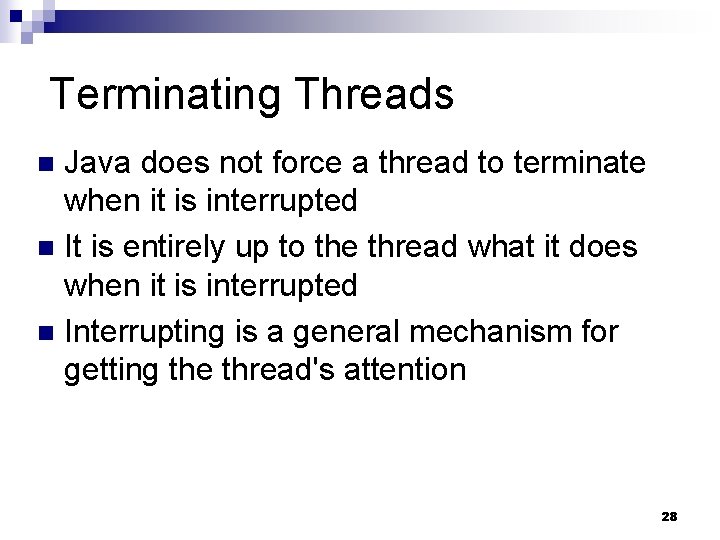
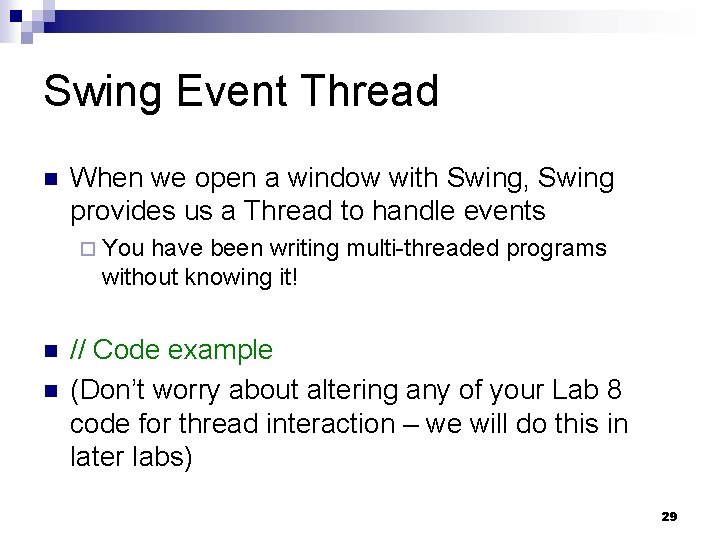
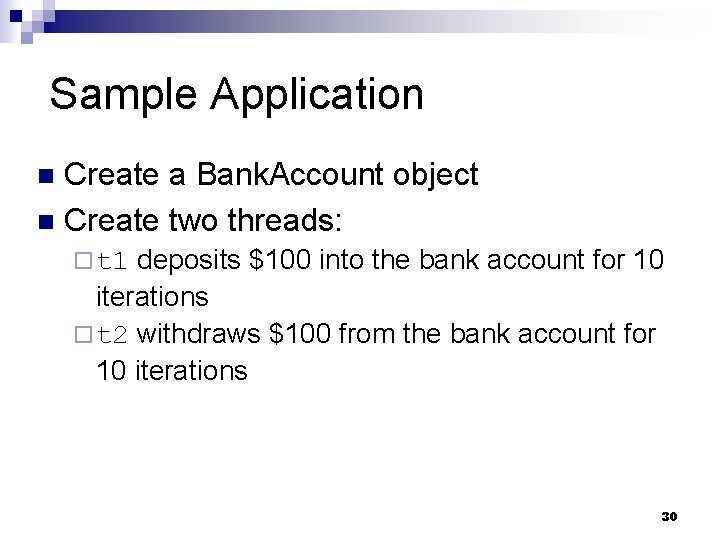
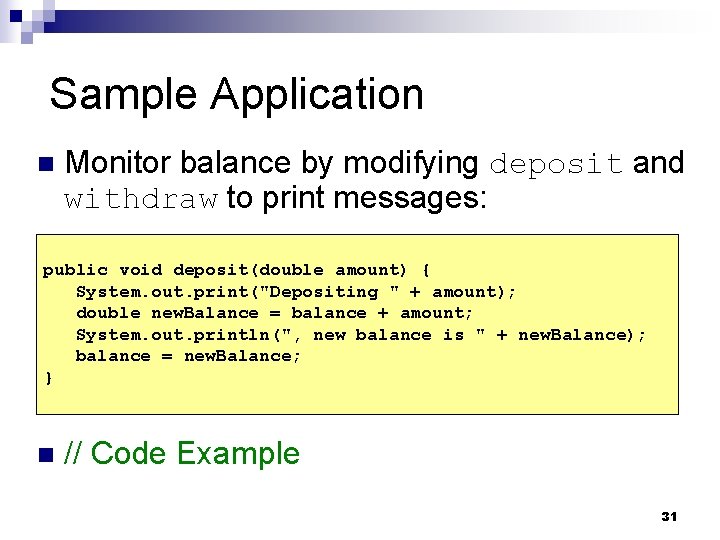
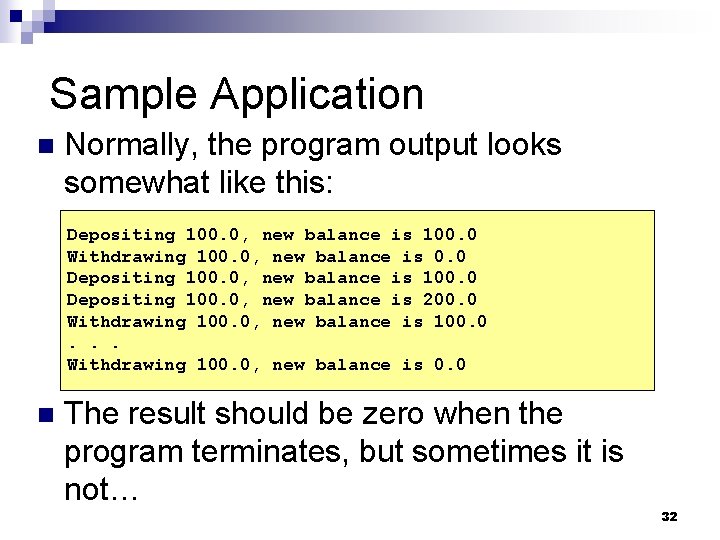
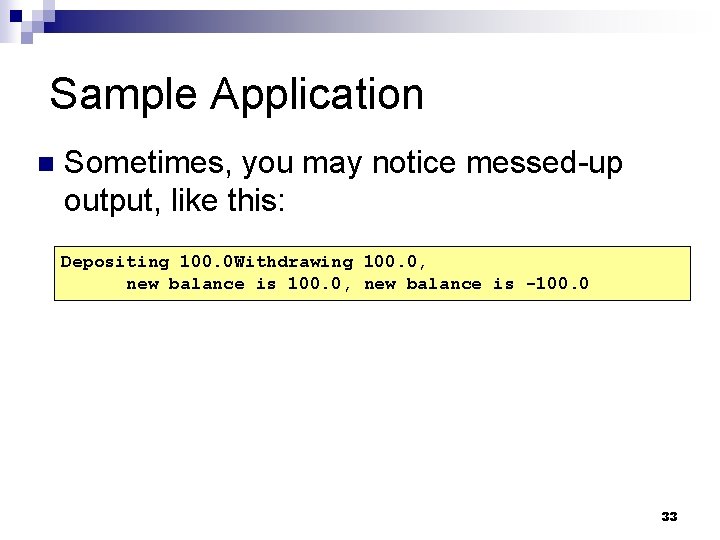
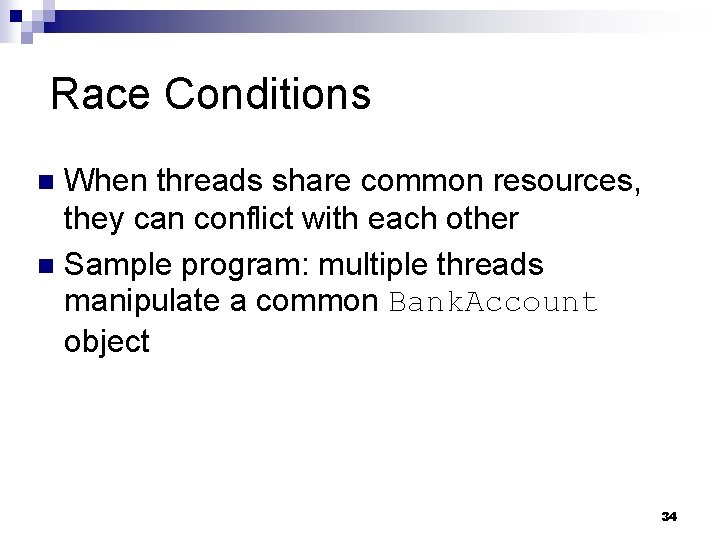
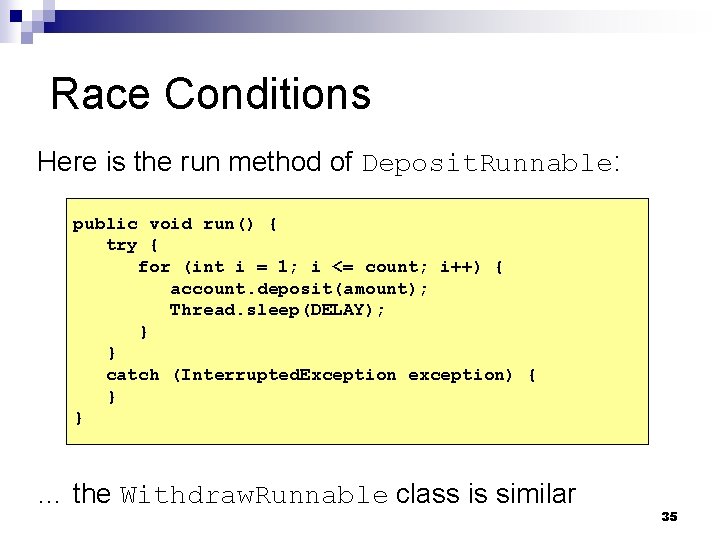
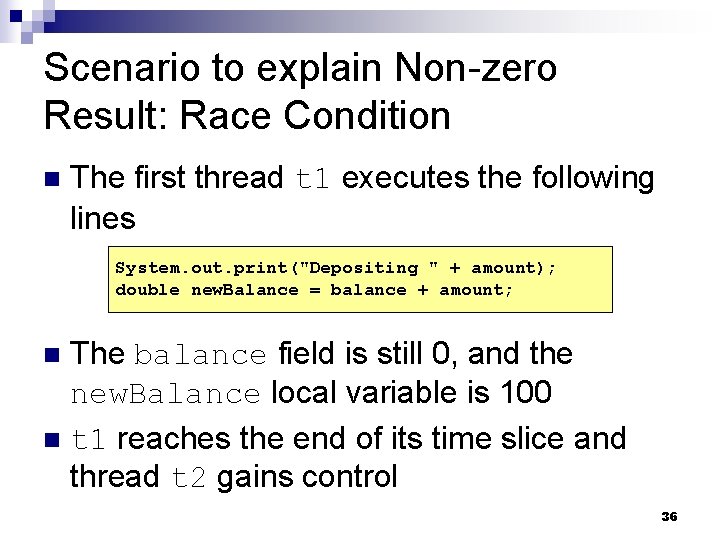
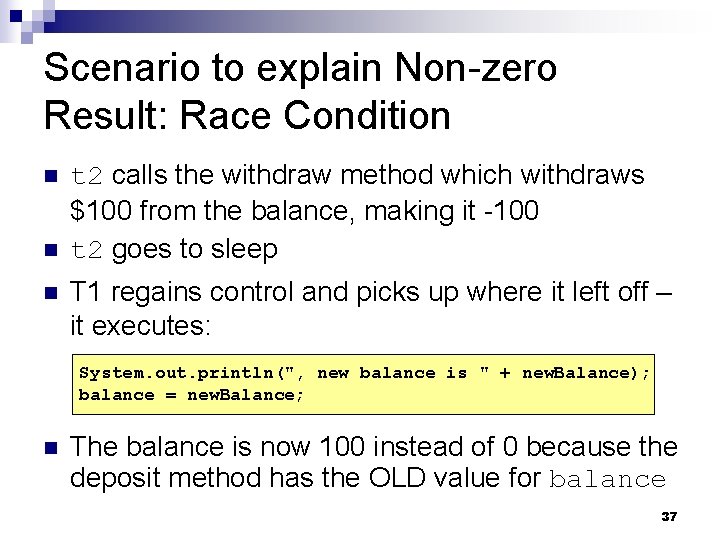
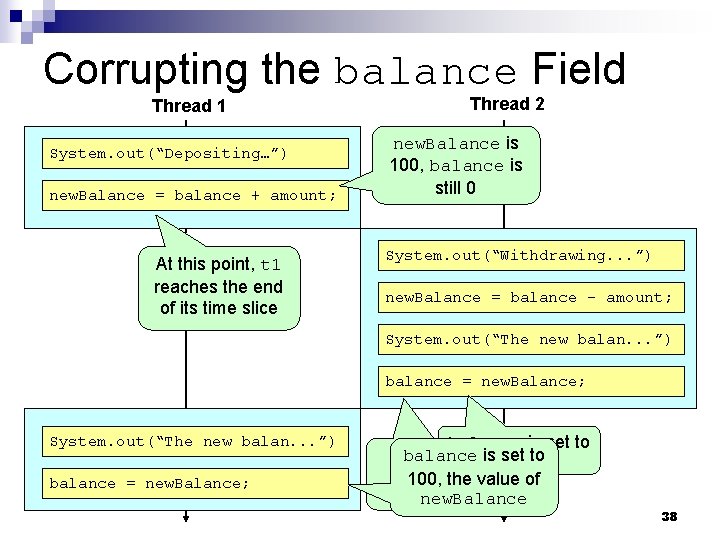
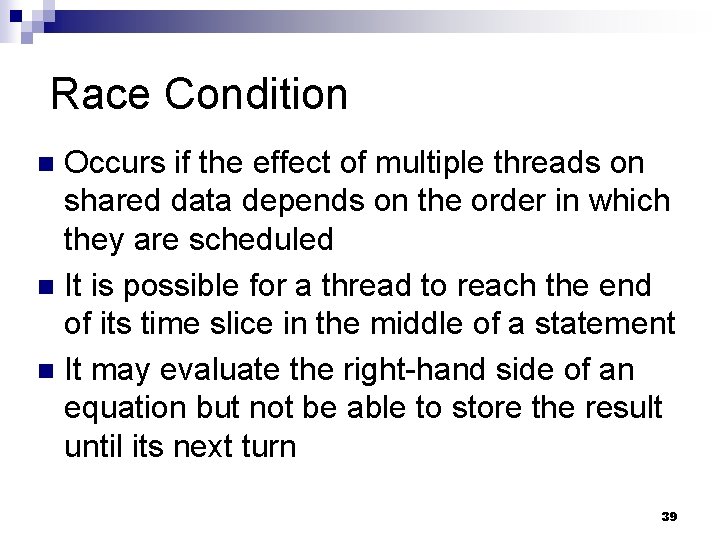
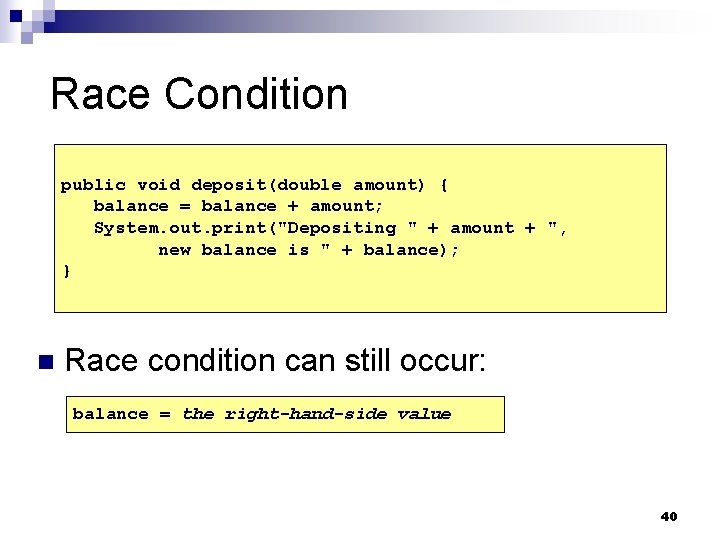
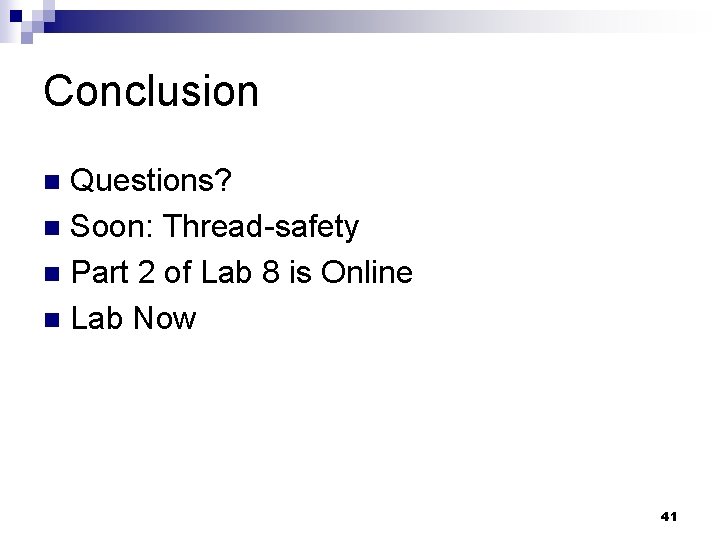
- Slides: 36
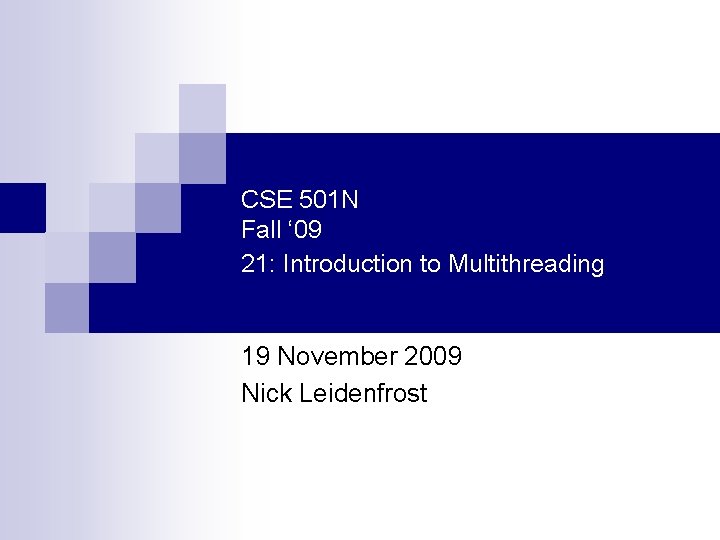
CSE 501 N Fall ‘ 09 21: Introduction to Multithreading 19 November 2009 Nick Leidenfrost
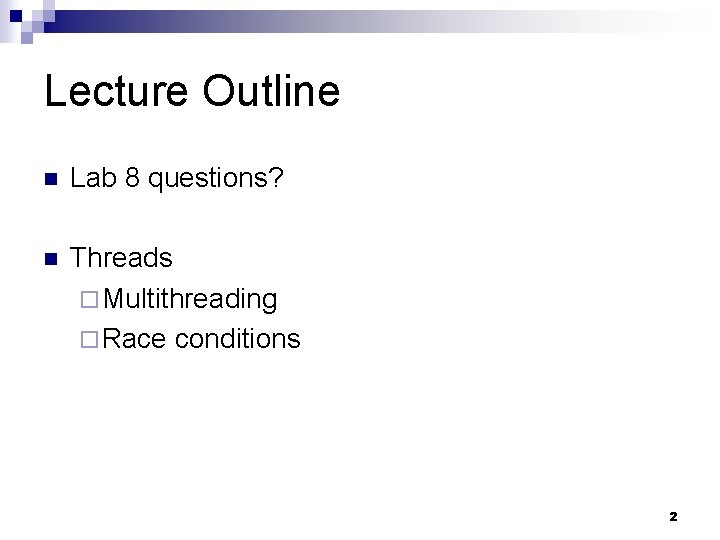
Lecture Outline n Lab 8 questions? n Threads ¨ Multithreading ¨ Race conditions 2
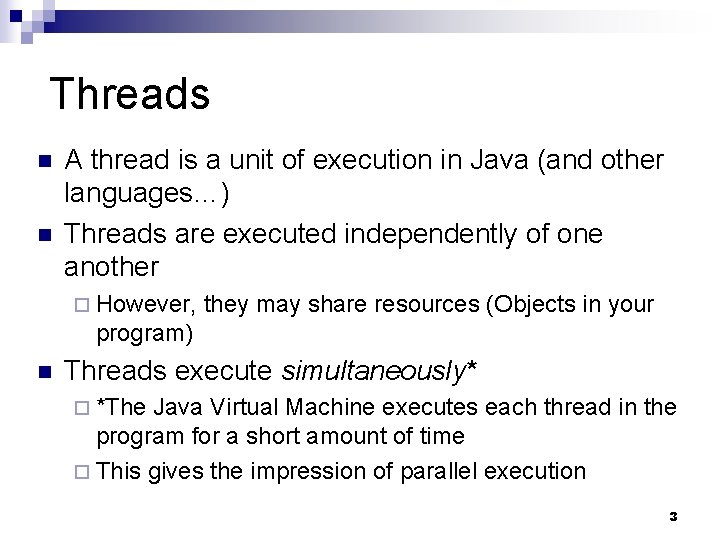
Threads n n A thread is a unit of execution in Java (and other languages…) Threads are executed independently of one another ¨ However, they may share resources (Objects in your program) n Threads execute simultaneously* ¨ *The Java Virtual Machine executes each thread in the program for a short amount of time ¨ This gives the impression of parallel execution 3
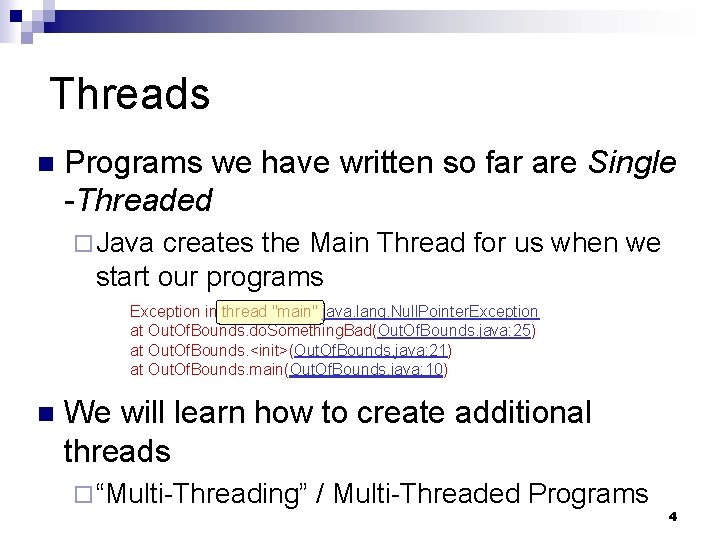
Threads n Programs we have written so far are Single -Threaded ¨ Java creates the Main Thread for us when we start our programs Exception in thread "main" java. lang. Null. Pointer. Exception at Out. Of. Bounds. do. Something. Bad(Out. Of. Bounds. java: 25) at Out. Of. Bounds. <init>(Out. Of. Bounds. java: 21) at Out. Of. Bounds. main(Out. Of. Bounds. java: 10) n We will learn how to create additional threads ¨ “Multi-Threading” / Multi-Threaded Programs 4
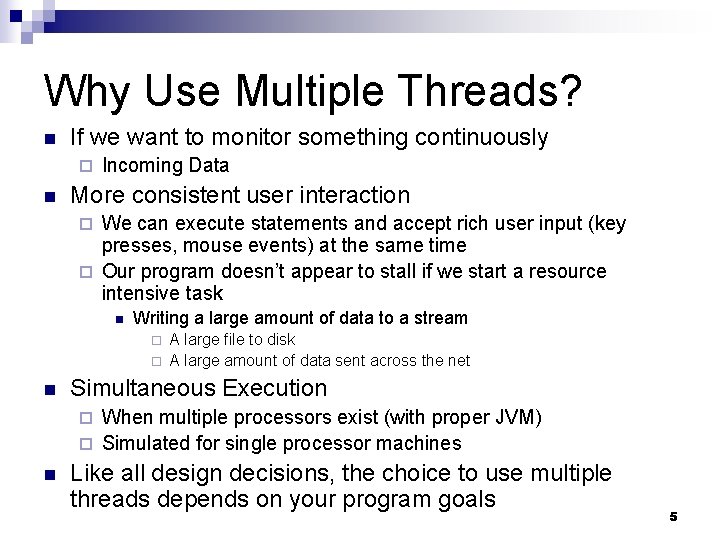
Why Use Multiple Threads? n If we want to monitor something continuously ¨ n Incoming Data More consistent user interaction We can execute statements and accept rich user input (key presses, mouse events) at the same time ¨ Our program doesn’t appear to stall if we start a resource intensive task ¨ n Writing a large amount of data to a stream ¨ ¨ n A large file to disk A large amount of data sent across the net Simultaneous Execution When multiple processors exist (with proper JVM) ¨ Simulated for single processor machines ¨ n Like all design decisions, the choice to use multiple threads depends on your program goals 5
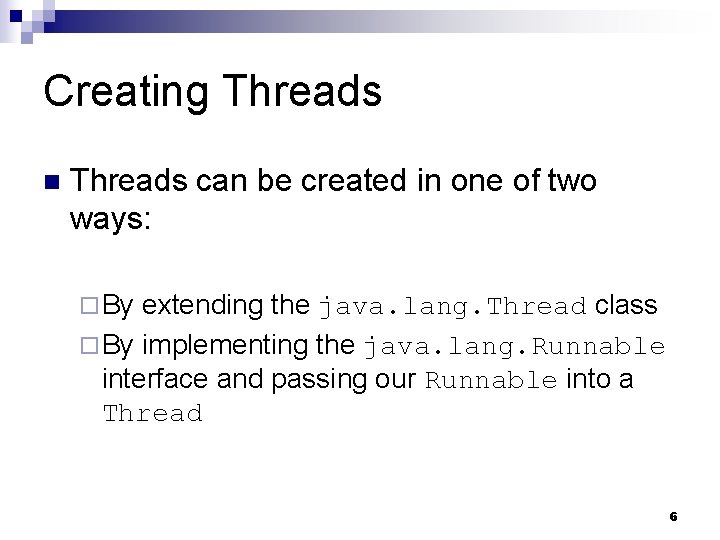
Creating Threads n Threads can be created in one of two ways: ¨ By extending the java. lang. Thread class ¨ By implementing the java. lang. Runnable interface and passing our Runnable into a Thread 6
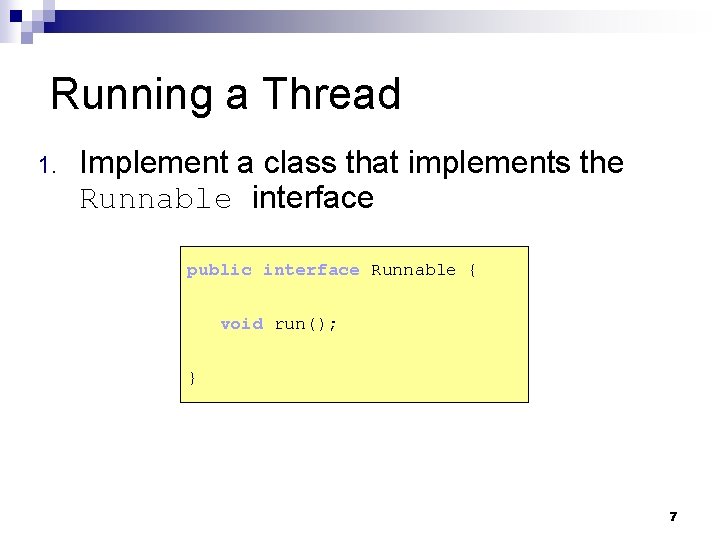
Running a Thread 1. Implement a class that implements the Runnable interface public interface Runnable { void run(); } 7
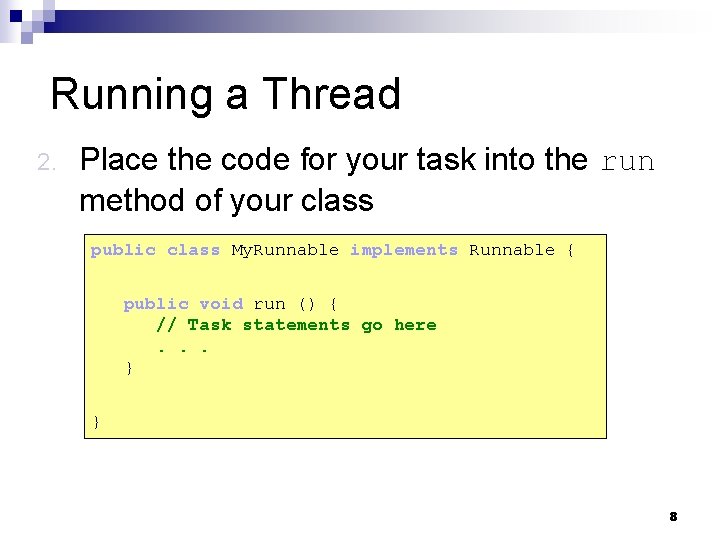
Running a Thread 2. Place the code for your task into the run method of your class public class My. Runnable implements Runnable { public void run () { // Task statements go here. . . } } 8
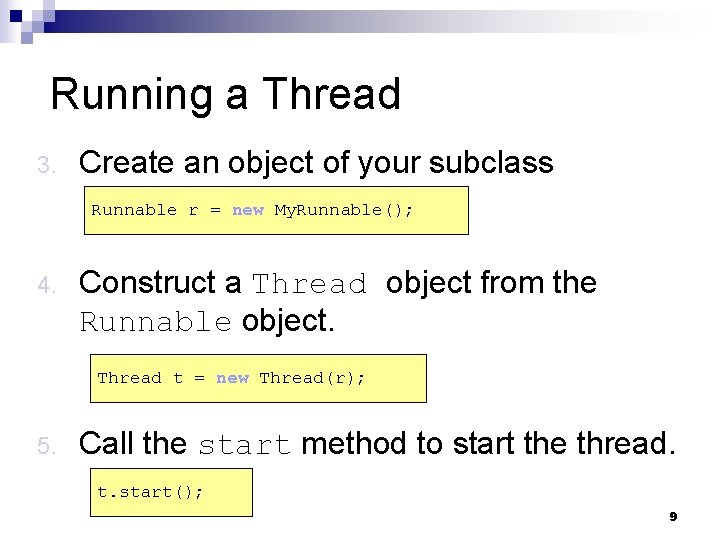
Running a Thread 3. Create an object of your subclass Runnable r = new My. Runnable(); 4. Construct a Thread object from the Runnable object. Thread t = new Thread(r); 5. Call the start method to start the thread. t. start(); 9
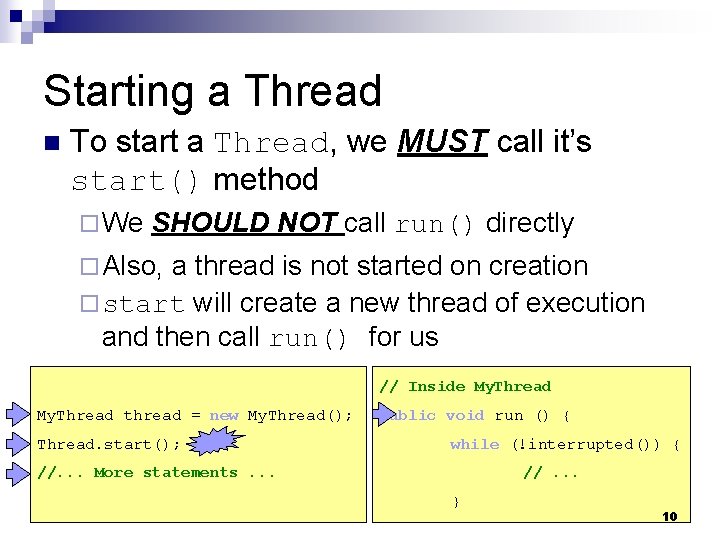
Starting a Thread n To start a Thread, we MUST call it’s start() method ¨ We SHOULD NOT call run() directly ¨ Also, a thread is not started on creation ¨ start will create a new thread of execution and then call run() for us // Inside My. Thread thread = new My. Thread(); Thread. start(); public void run () { while (!interrupted()) { //. . . More statements. . . } 10
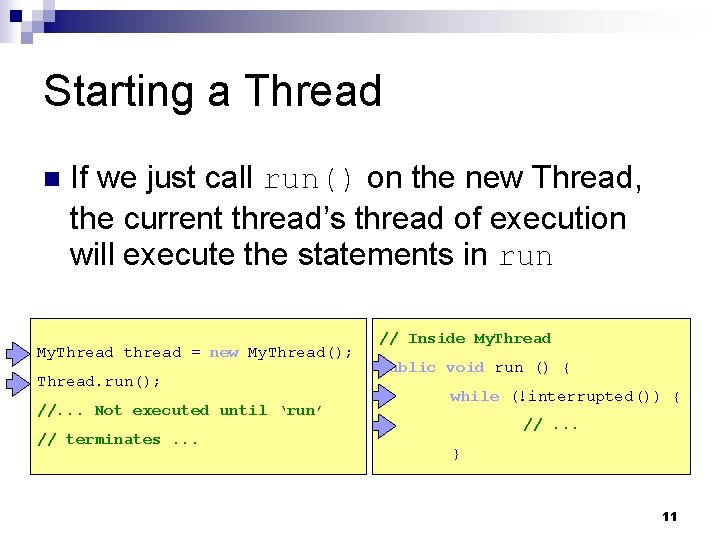
Starting a Thread n If we just call run() on the new Thread, the current thread’s thread of execution will execute the statements in run My. Thread thread = new My. Thread(); Thread. run(); //. . . Not executed until ‘run’ // terminates. . . // Inside My. Thread public void run () { while (!interrupted()) { //. . . } 11
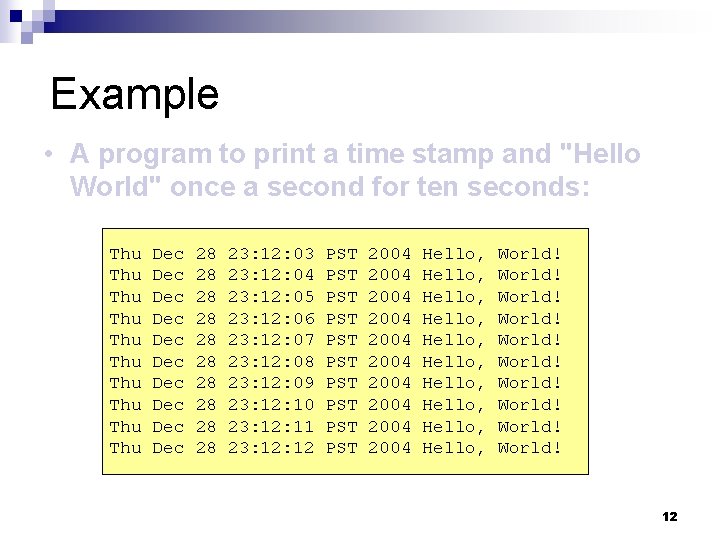
Example • A program to print a time stamp and "Hello World" once a second for ten seconds: Thu Thu Thu Dec Dec Dec 28 28 28 23: 12: 03 23: 12: 04 23: 12: 05 23: 12: 06 23: 12: 07 23: 12: 08 23: 12: 09 23: 12: 10 23: 12: 11 23: 12 PST PST PST 2004 2004 2004 Hello, Hello, Hello, World! World! World! 12
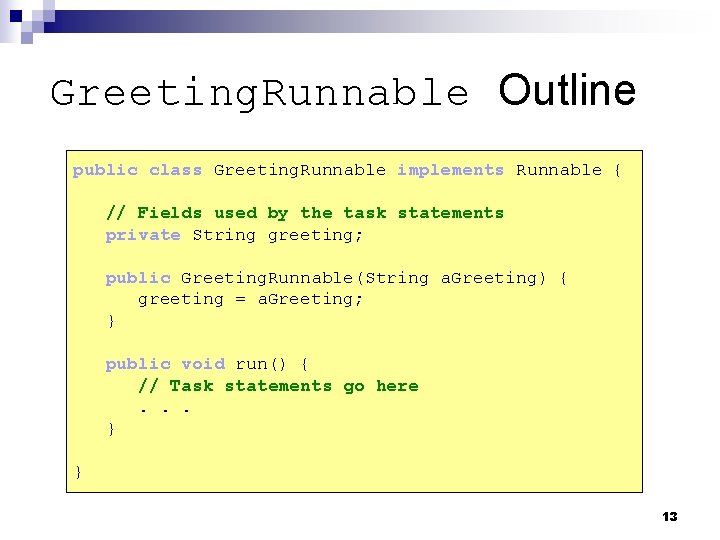
Greeting. Runnable Outline public class Greeting. Runnable implements Runnable { // Fields used by the task statements private String greeting; public Greeting. Runnable(String a. Greeting) { greeting = a. Greeting; } public void run() { // Task statements go here. . . } } 13
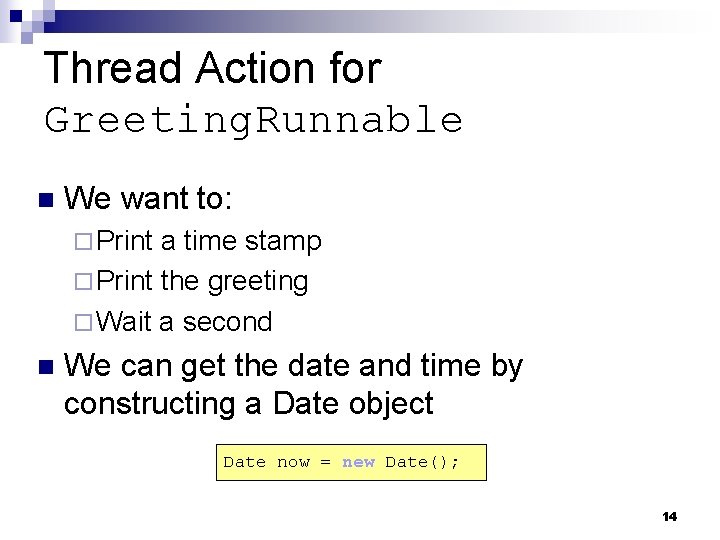
Thread Action for Greeting. Runnable n We want to: ¨ Print a time stamp ¨ Print the greeting ¨ Wait a second n We can get the date and time by constructing a Date object Date now = new Date(); 14
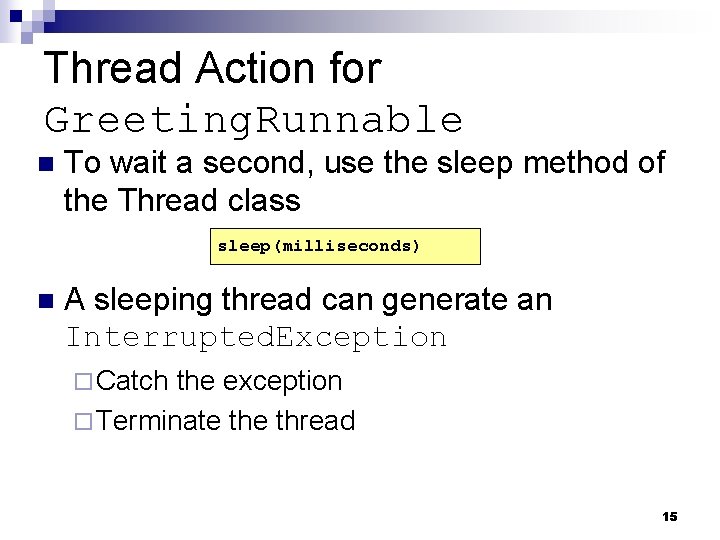
Thread Action for Greeting. Runnable n To wait a second, use the sleep method of the Thread class sleep(milliseconds) n A sleeping thread can generate an Interrupted. Exception ¨ Catch the exception ¨ Terminate thread 15
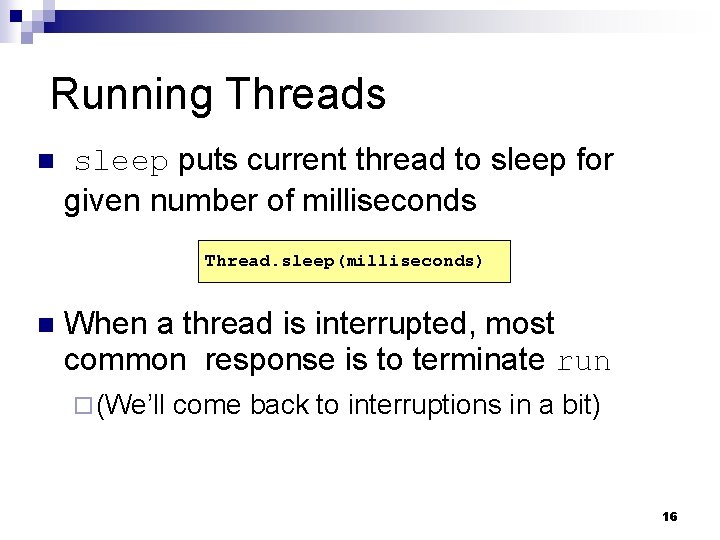
Running Threads n sleep puts current thread to sleep for given number of milliseconds Thread. sleep(milliseconds) n When a thread is interrupted, most common response is to terminate run ¨ (We’ll come back to interruptions in a bit) 16
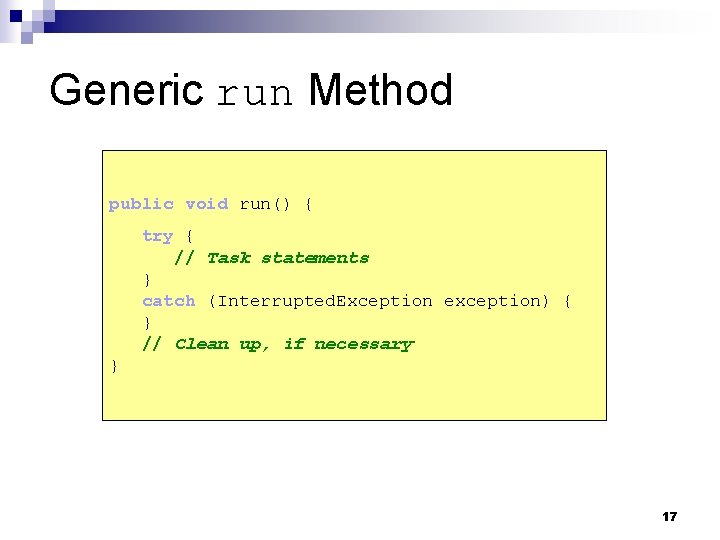
Generic run Method public void run() { try { // Task statements } catch (Interrupted. Exception exception) { } // Clean up, if necessary } 17
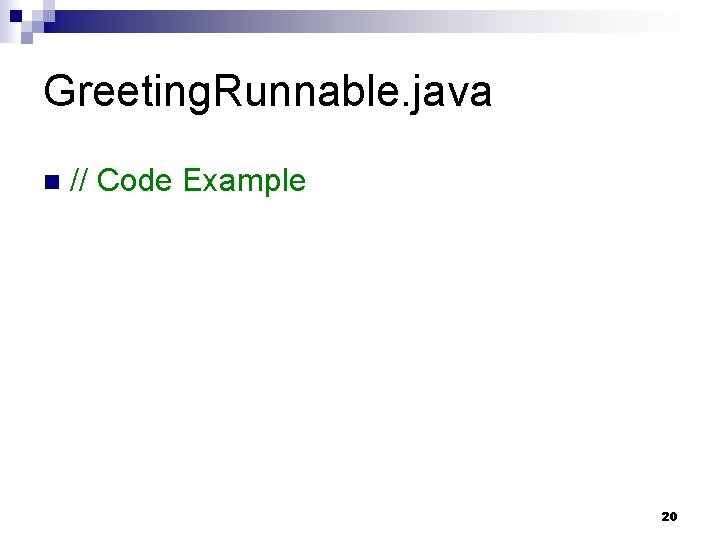
Greeting. Runnable. java n // Code Example 20
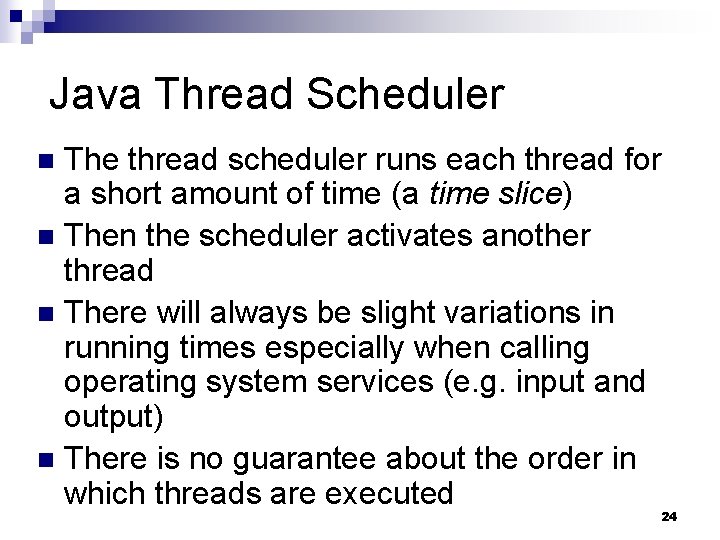
Java Thread Scheduler The thread scheduler runs each thread for a short amount of time (a time slice) n Then the scheduler activates another thread n There will always be slight variations in running times especially when calling operating system services (e. g. input and output) n There is no guarantee about the order in which threads are executed n 24
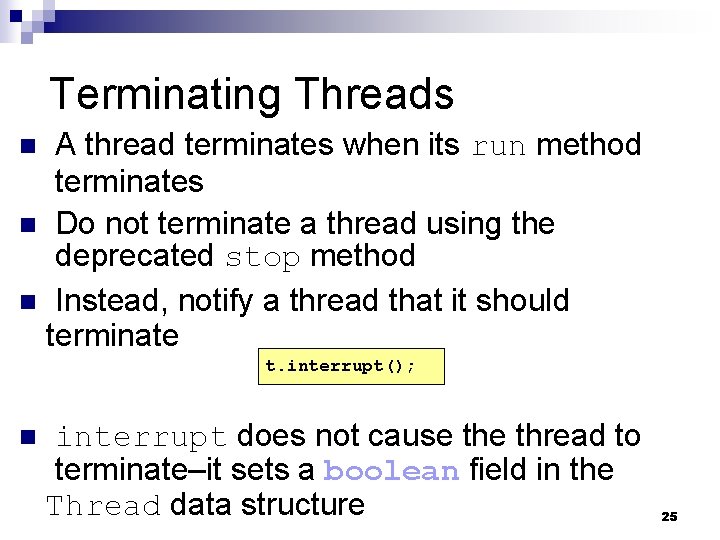
Terminating Threads A thread terminates when its run method terminates n Do not terminate a thread using the deprecated stop method n Instead, notify a thread that it should terminate n t. interrupt(); n interrupt does not cause thread to terminate–it sets a boolean field in the Thread data structure 25
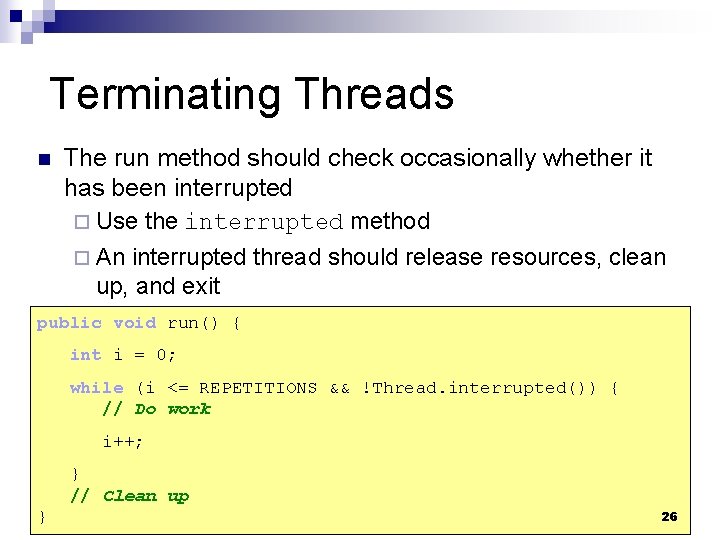
Terminating Threads n The run method should check occasionally whether it has been interrupted ¨ Use the interrupted method ¨ An interrupted thread should release resources, clean up, and exit public void run() { int i = 0; while (i <= REPETITIONS && !Thread. interrupted()) { // Do work i++; } // Clean up } 26
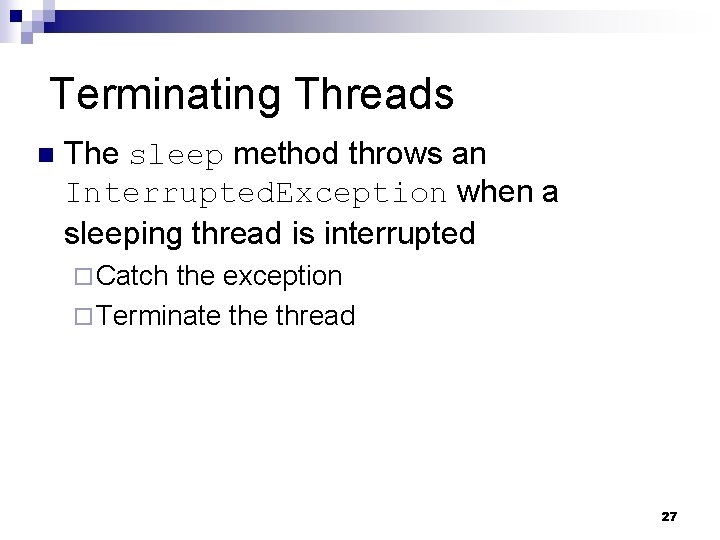
Terminating Threads n The sleep method throws an Interrupted. Exception when a sleeping thread is interrupted ¨ Catch the exception ¨ Terminate thread 27
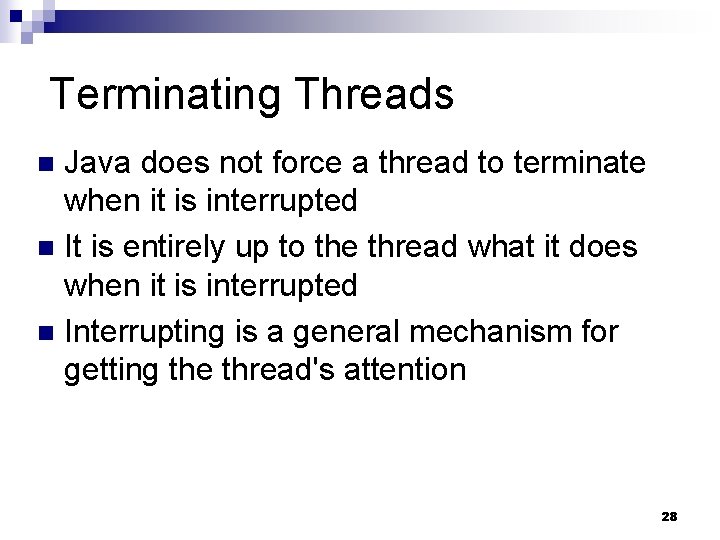
Terminating Threads Java does not force a thread to terminate when it is interrupted n It is entirely up to the thread what it does when it is interrupted n Interrupting is a general mechanism for getting the thread's attention n 28
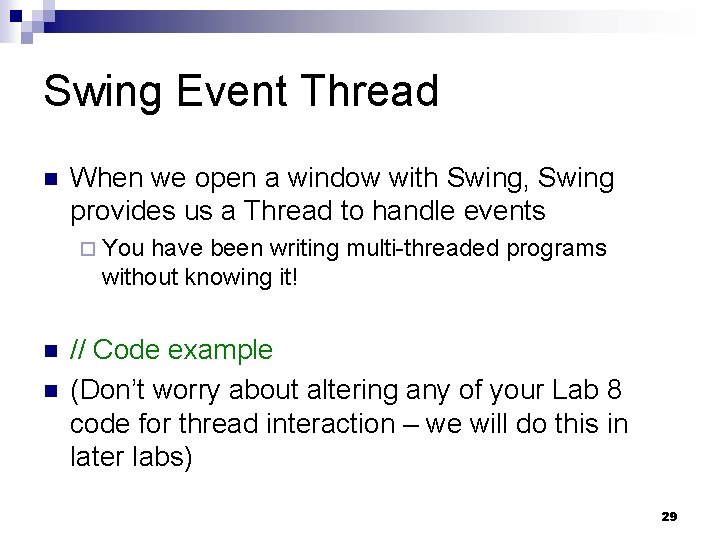
Swing Event Thread n When we open a window with Swing, Swing provides us a Thread to handle events ¨ You have been writing multi-threaded programs without knowing it! n n // Code example (Don’t worry about altering any of your Lab 8 code for thread interaction – we will do this in later labs) 29
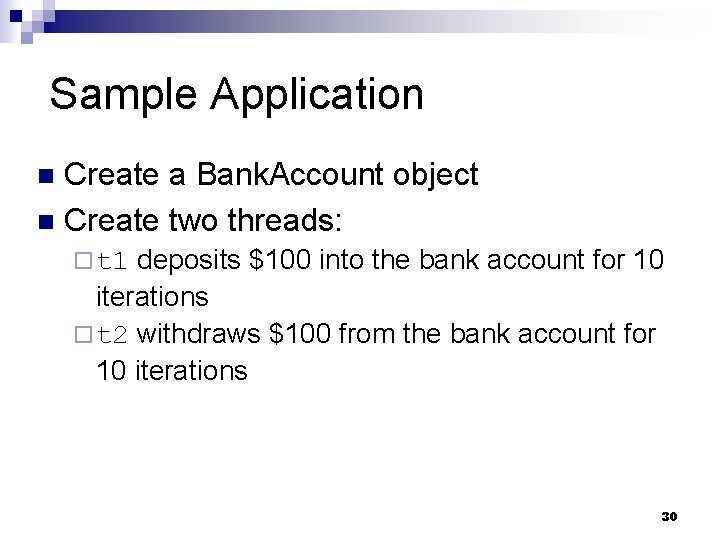
Sample Application Create a Bank. Account object n Create two threads: n deposits $100 into the bank account for 10 iterations ¨ t 2 withdraws $100 from the bank account for 10 iterations ¨ t 1 30
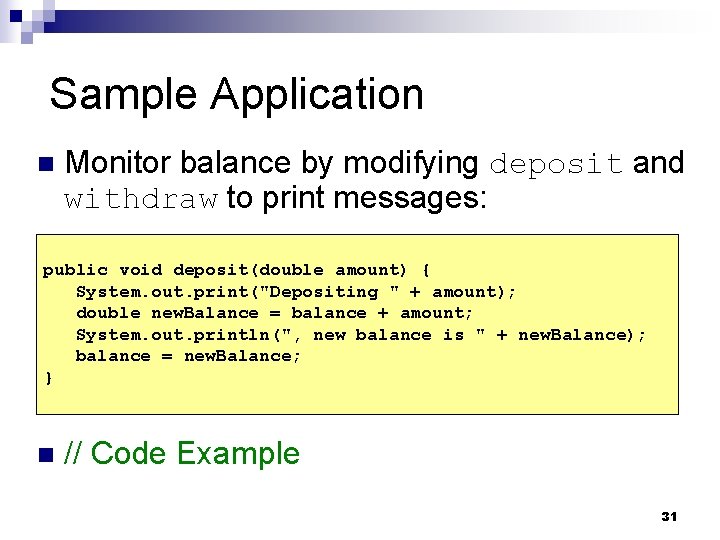
Sample Application n Monitor balance by modifying deposit and withdraw to print messages: public void deposit(double amount) { System. out. print("Depositing " + amount); double new. Balance = balance + amount; System. out. println(", new balance is " + new. Balance); balance = new. Balance; } n // Code Example 31
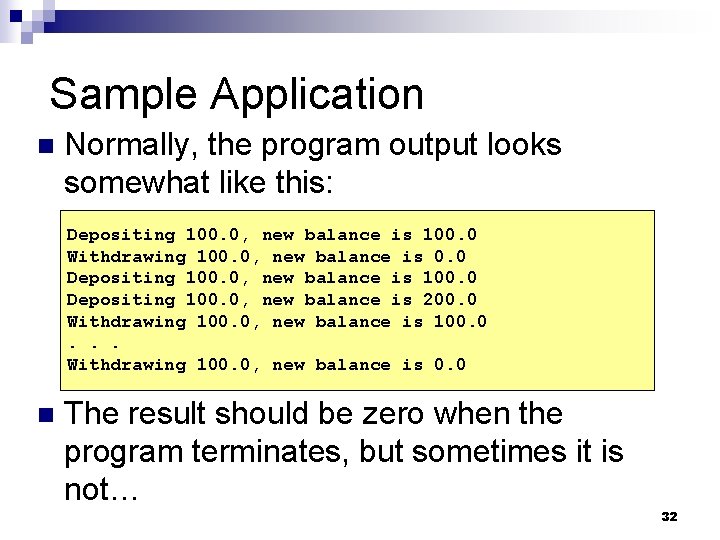
Sample Application n Normally, the program output looks somewhat like this: Depositing 100. 0, new balance is 100. 0 Withdrawing 100. 0, new balance is 0. 0 Depositing 100. 0, new balance is 100. 0 Depositing 100. 0, new balance is 200. 0 Withdrawing 100. 0, new balance is 100. 0. . . Withdrawing 100. 0, new balance is 0. 0 n The result should be zero when the program terminates, but sometimes it is not… 32
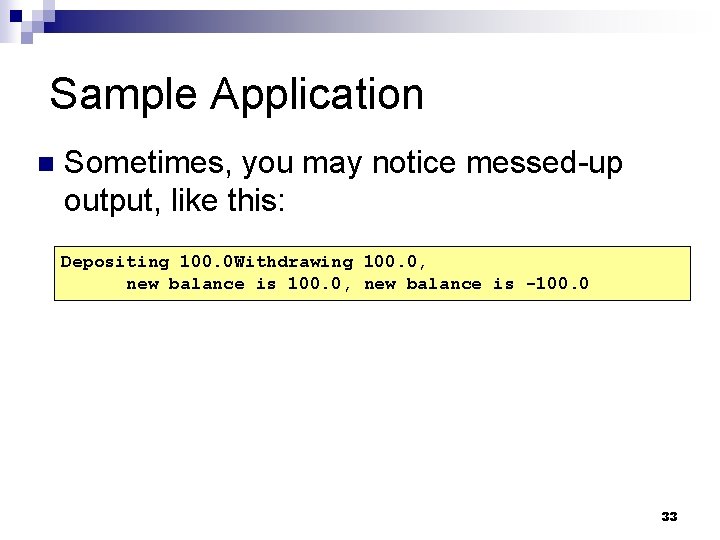
Sample Application n Sometimes, you may notice messed-up output, like this: Depositing 100. 0 Withdrawing 100. 0, new balance is -100. 0 33
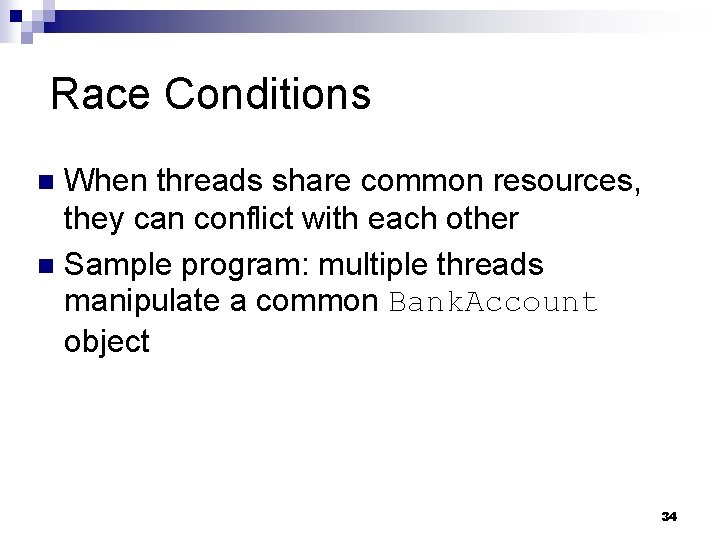
Race Conditions When threads share common resources, they can conflict with each other n Sample program: multiple threads manipulate a common Bank. Account object n 34
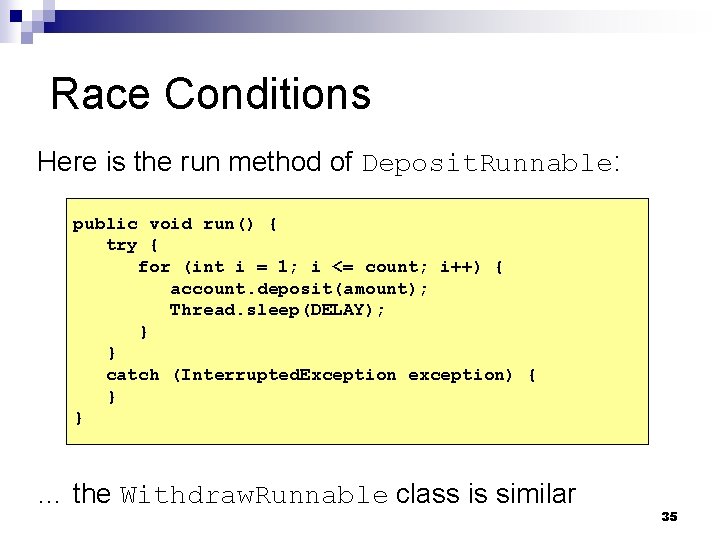
Race Conditions Here is the run method of Deposit. Runnable: public void run() { try { for (int i = 1; i <= count; i++) { account. deposit(amount); Thread. sleep(DELAY); } } catch (Interrupted. Exception exception) { } } … the Withdraw. Runnable class is similar 35
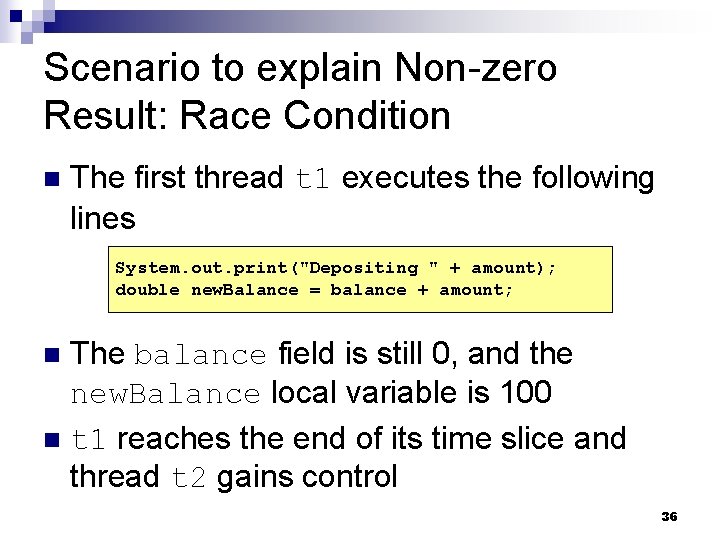
Scenario to explain Non-zero Result: Race Condition n The first thread t 1 executes the following lines System. out. print("Depositing " + amount); double new. Balance = balance + amount; The balance field is still 0, and the new. Balance local variable is 100 n t 1 reaches the end of its time slice and thread t 2 gains control n 36
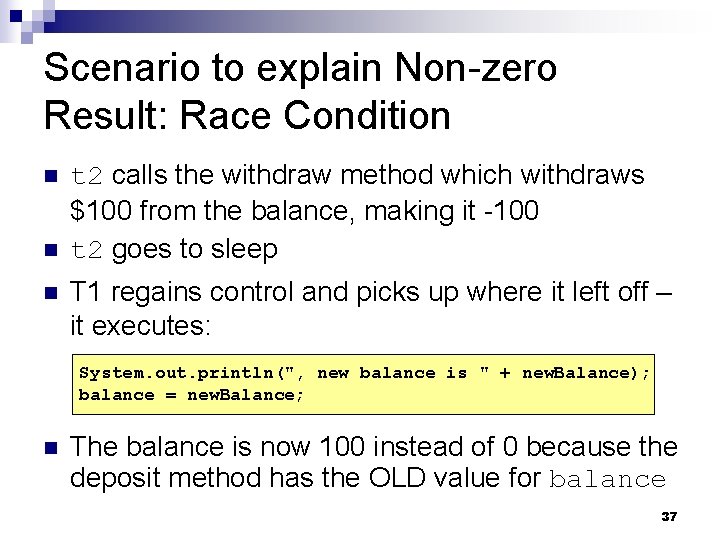
Scenario to explain Non-zero Result: Race Condition n t 2 calls the withdraw method which withdraws $100 from the balance, making it -100 t 2 goes to sleep T 1 regains control and picks up where it left off – it executes: System. out. println(", new balance is " + new. Balance); balance = new. Balance; n The balance is now 100 instead of 0 because the deposit method has the OLD value for balance 37
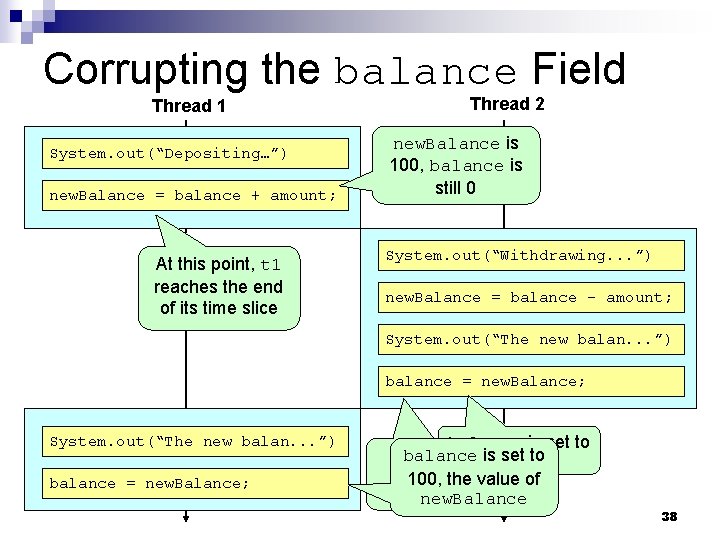
Corrupting the balance Field Thread 1 System. out(“Depositing…”) new. Balance = balance + amount; At this point, t 1 reaches the end of its time slice Thread 2 new. Balance is 100, balance is still 0 System. out(“Withdrawing. . . ”) new. Balance = balance - amount; System. out(“The new balan. . . ”) balance = new. Balance; balance is set to t 2 goes to balance is set to -100 sleep, its time 100, the value of new. Balance slice ends 38
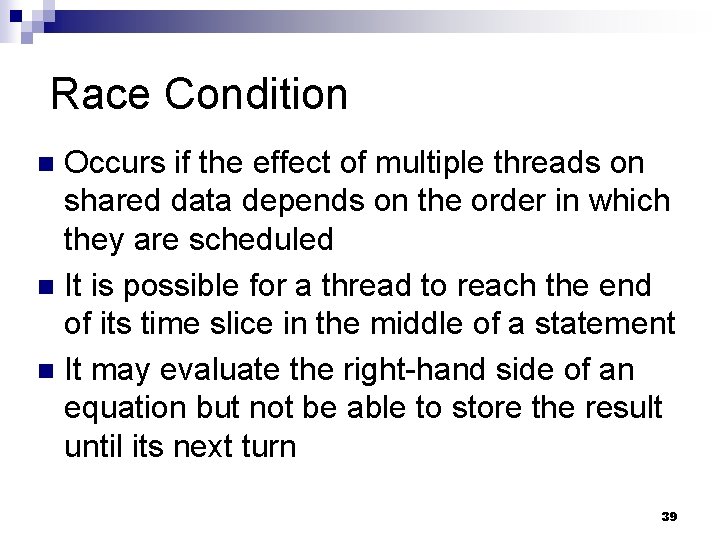
Race Condition Occurs if the effect of multiple threads on shared data depends on the order in which they are scheduled n It is possible for a thread to reach the end of its time slice in the middle of a statement n It may evaluate the right-hand side of an equation but not be able to store the result until its next turn n 39
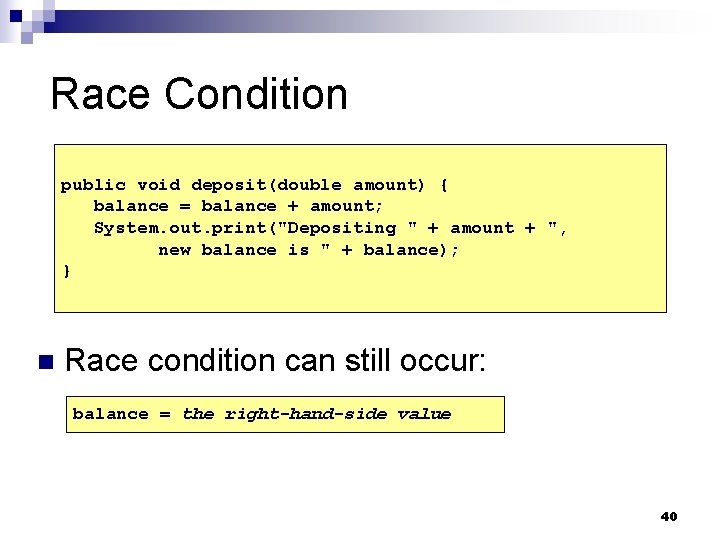
Race Condition public void deposit(double amount) { balance = balance + amount; System. out. print("Depositing " + amount + ", new balance is " + balance); } n Race condition can still occur: balance = the right-hand-side value 40
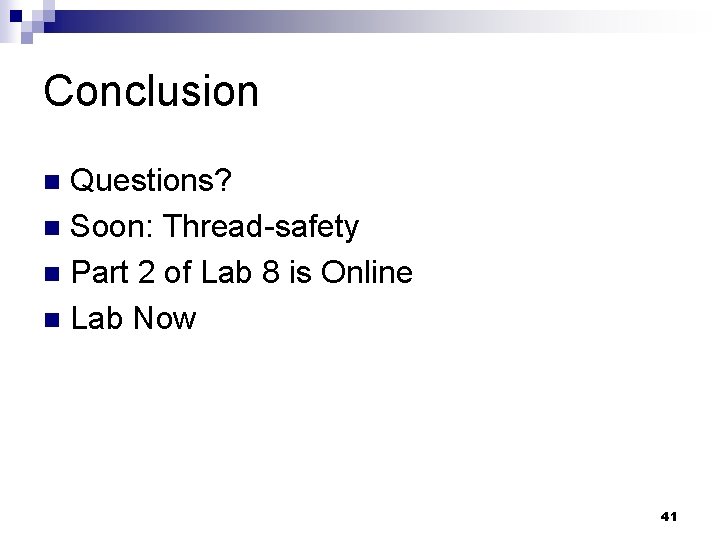
Conclusion Questions? n Soon: Thread-safety n Part 2 of Lab 8 is Online n Lab Now n 41
Barema 501 onderwijs
Rimskych 501
Bds 501
Hino número 1
Eng m 501
Ariane 5 flight 501
Dev 501
Cis 501
Cis 501
Cis 501
Cis 501
Cisco pix 501 specs
Nia 501
Tyler sis 501
Hinario 501
Cs-501
Bios 501
Bios 501
Mgt 501
Mgt 501
Cas 501
I 501
I 501
Mgt501 human resource management
Turnus 501
Simple capm
Taandme
Norma 501
Opwekking 501
W 501
Maurice cooper
Fre 501
Cis 501
Cis 501
Cis 501
Joe devietti
Conclusion paragraph format