CSE 501 N Fall 09 17 Exception Handling
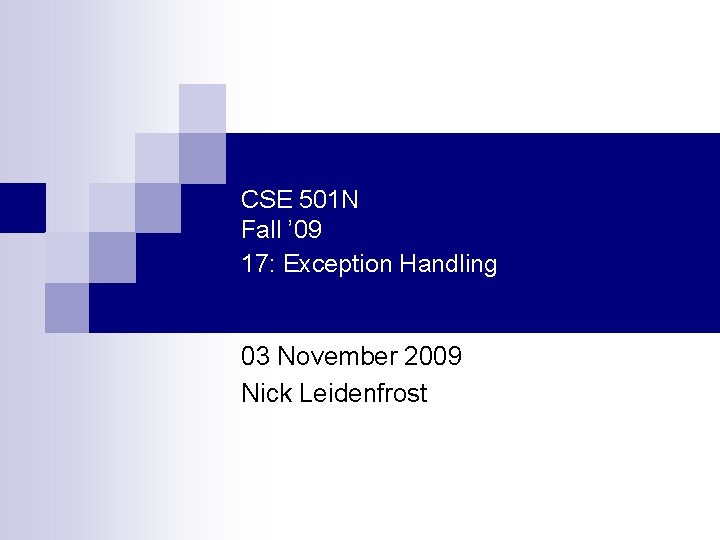
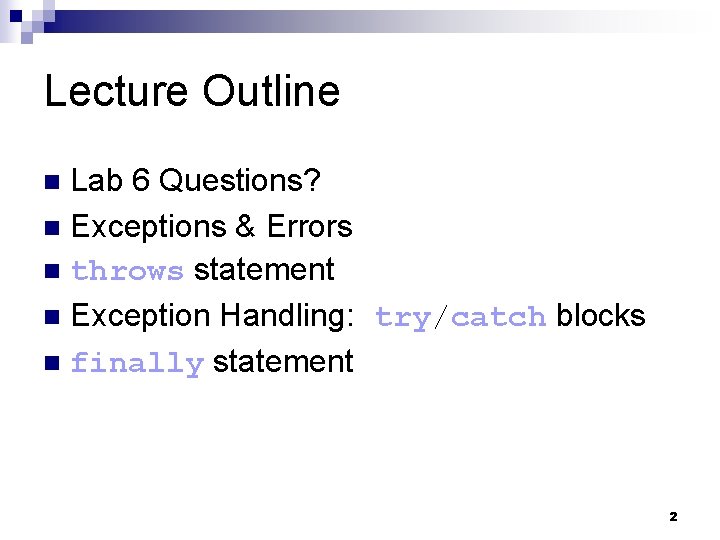
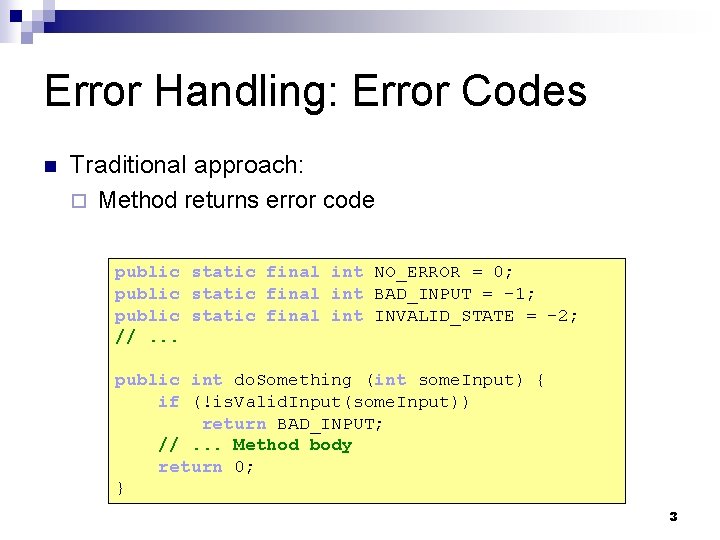
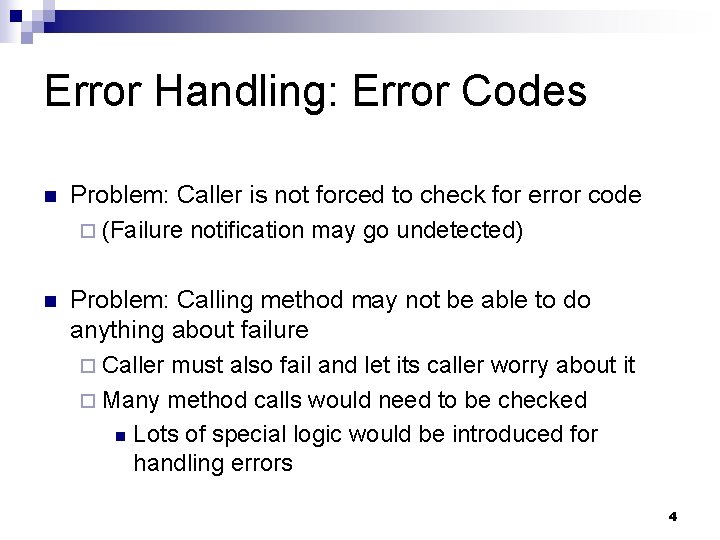
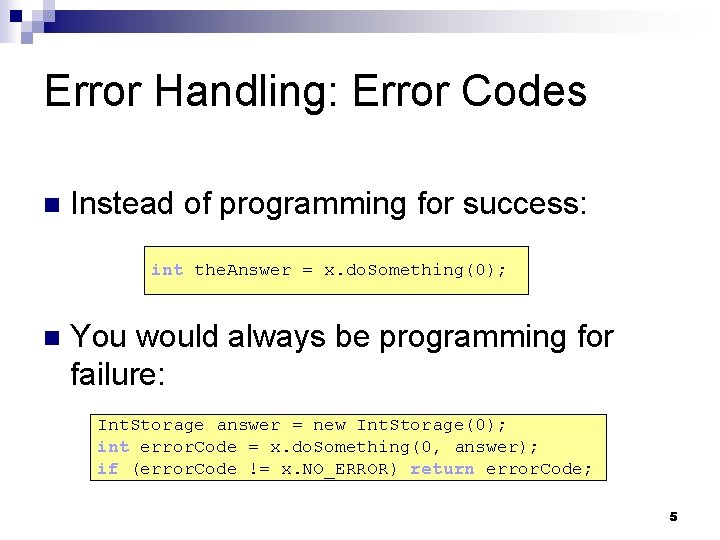
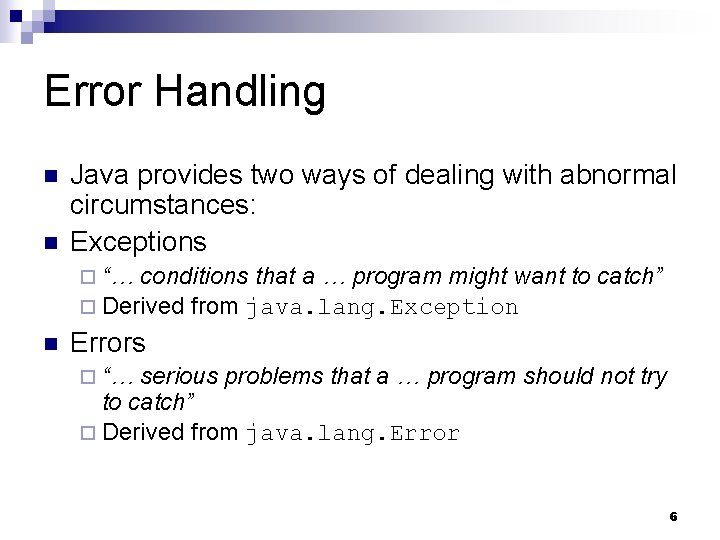
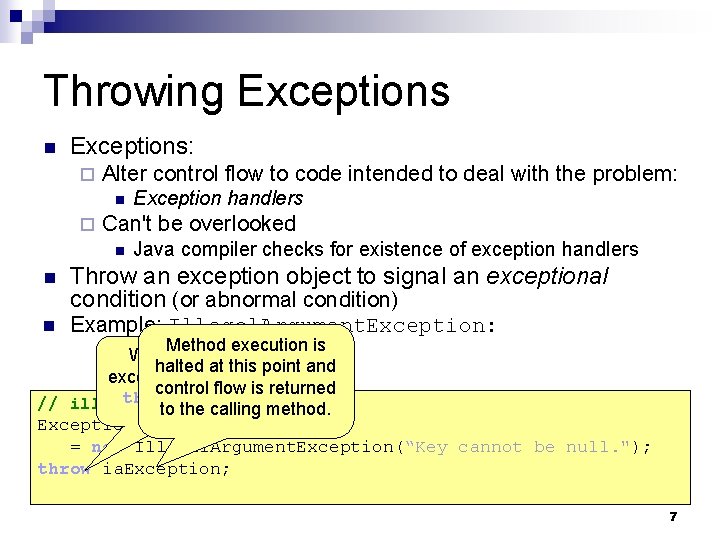
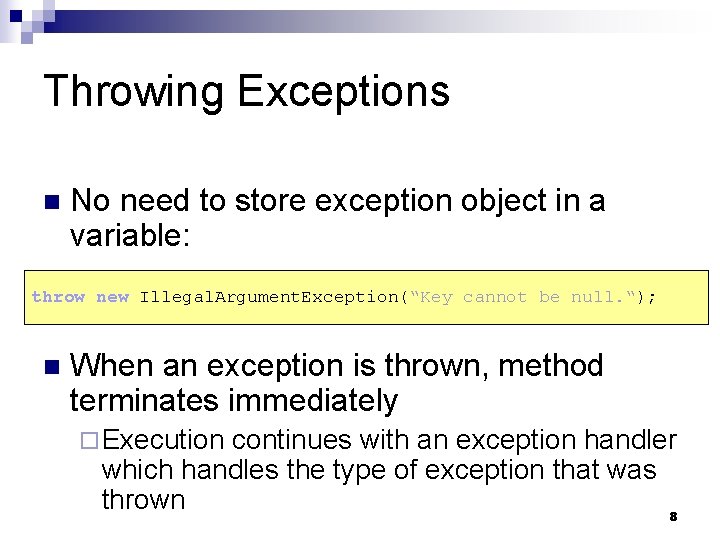
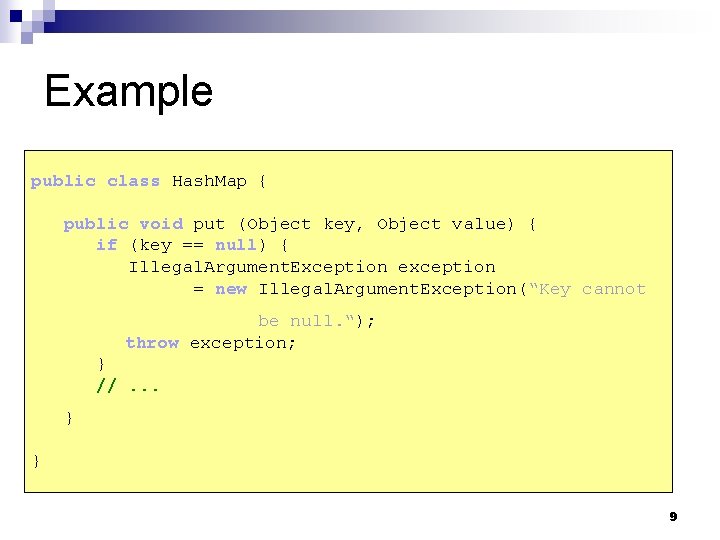
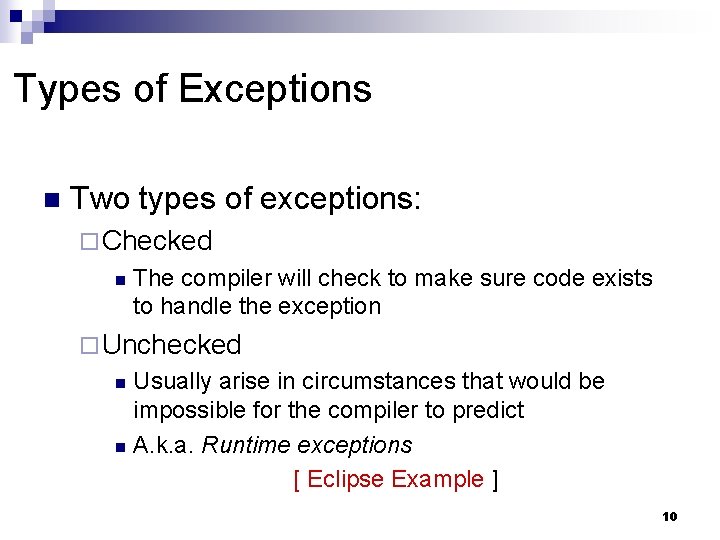
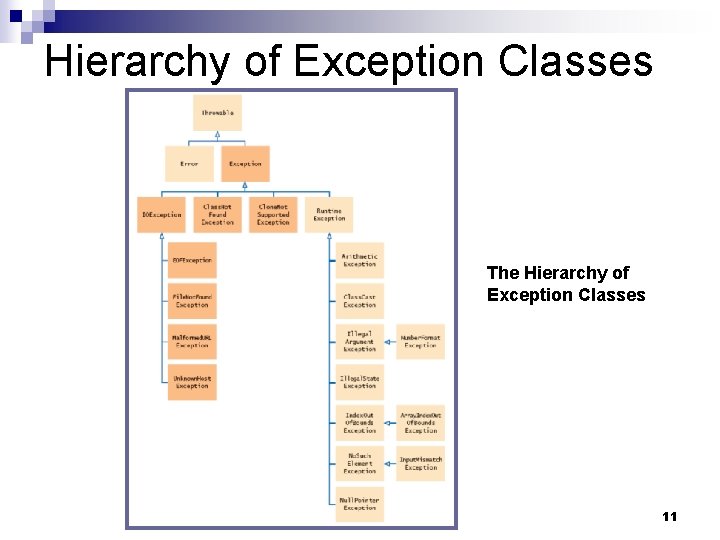
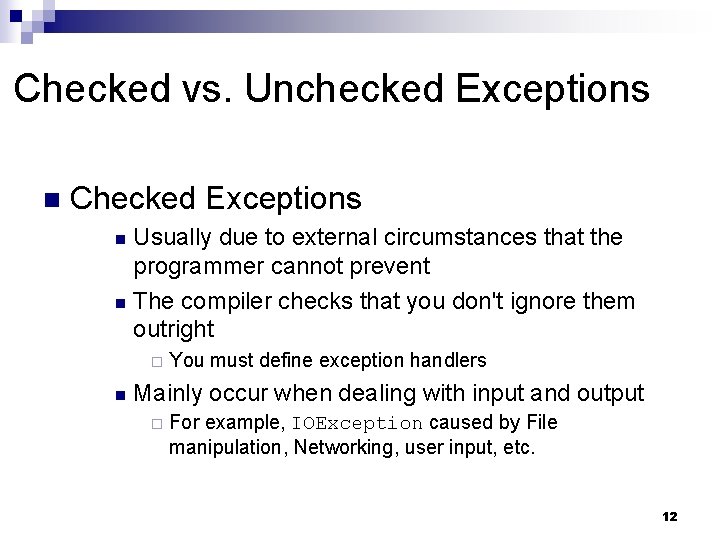
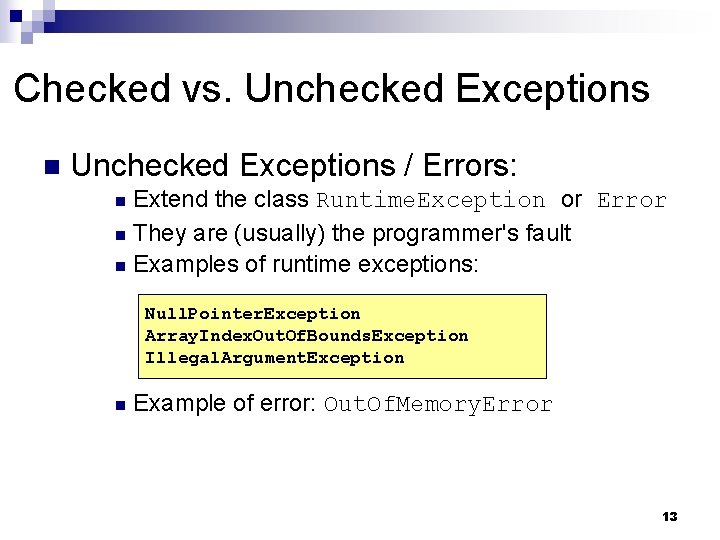
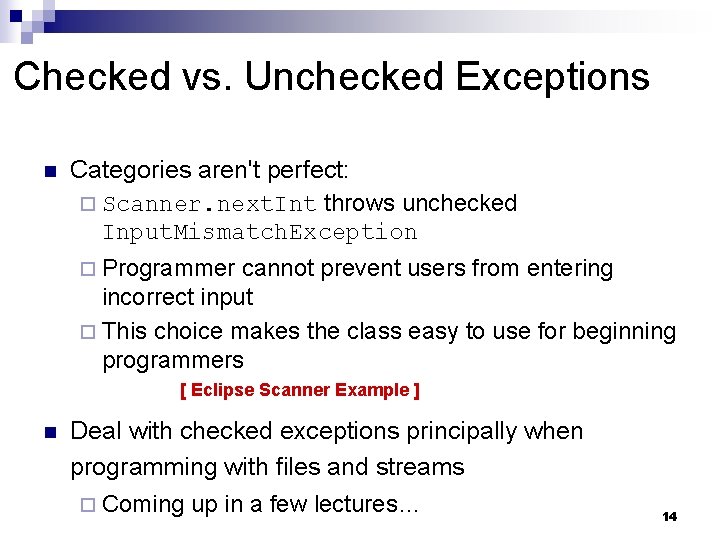
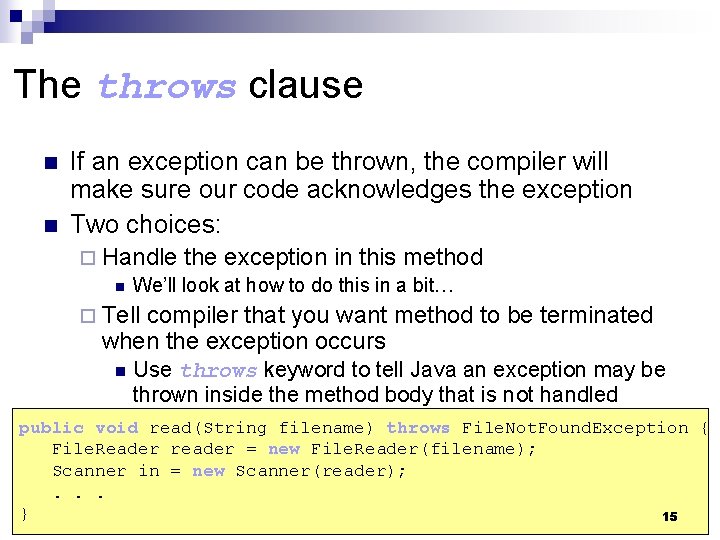
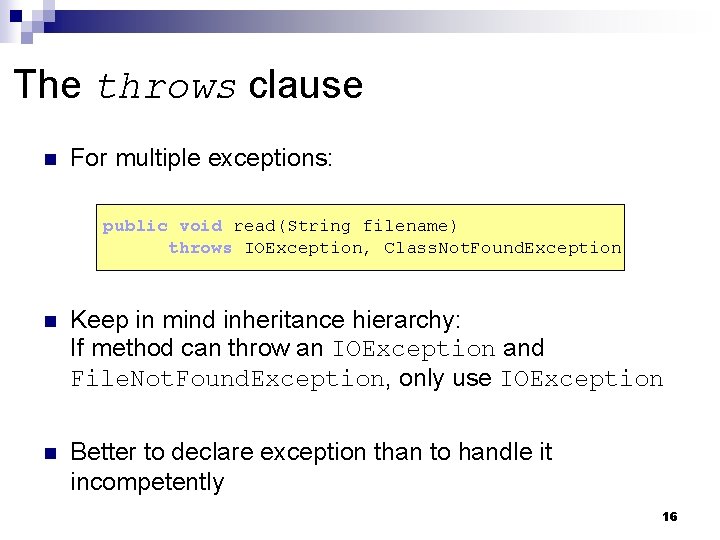
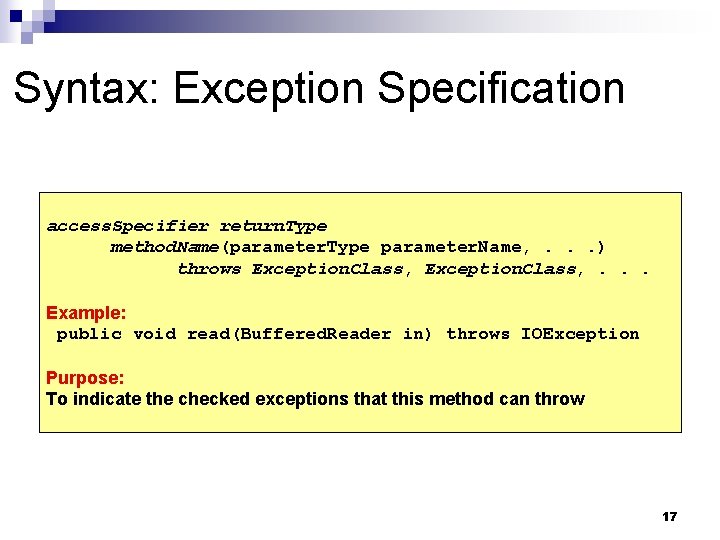
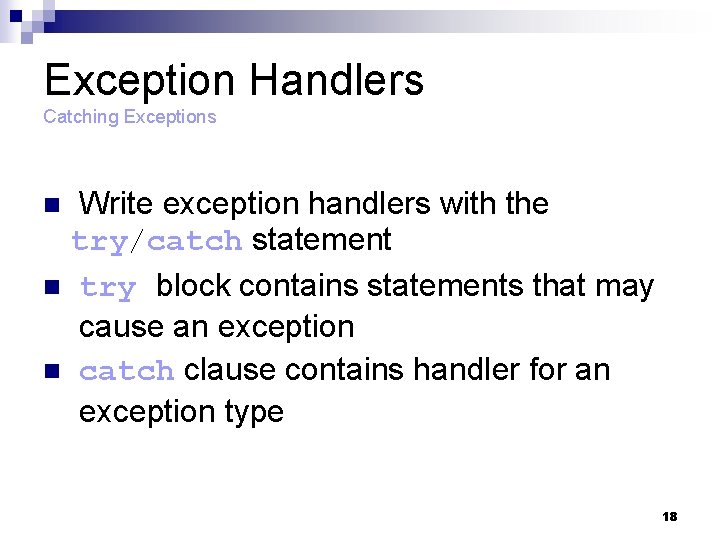
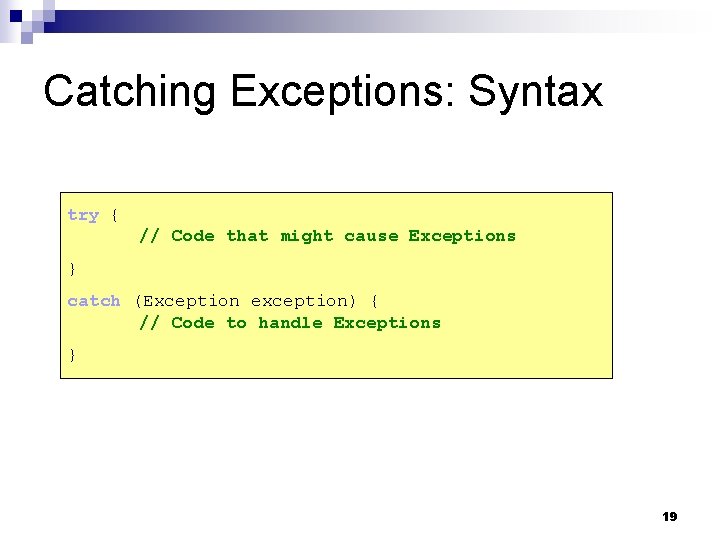
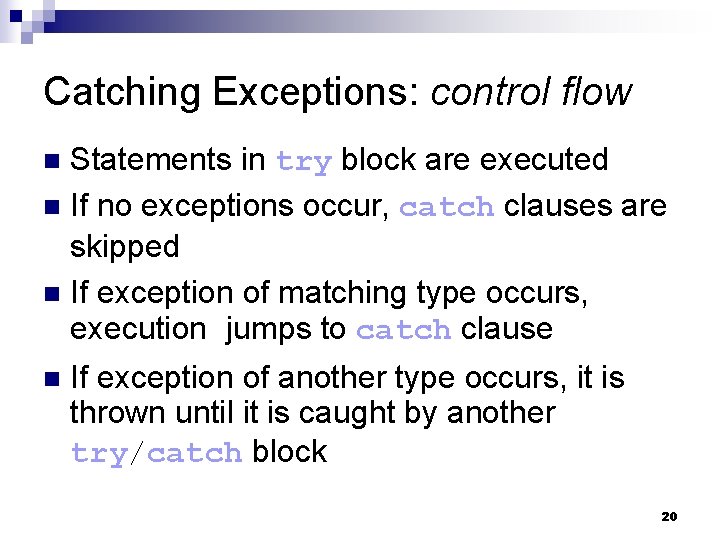
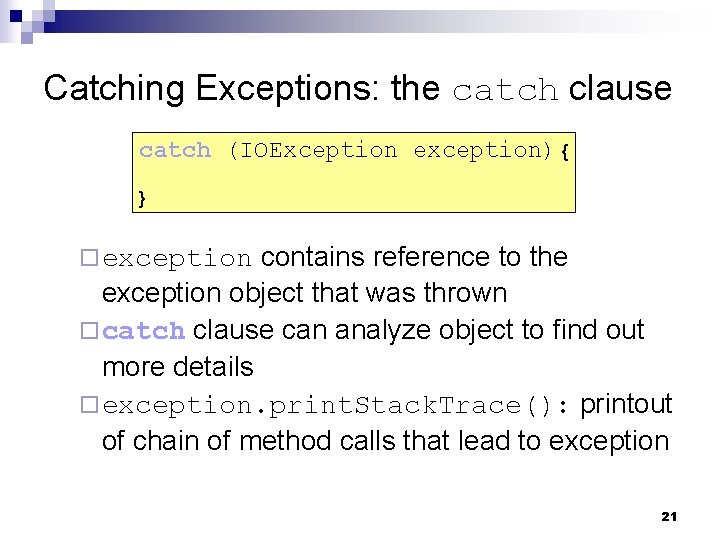
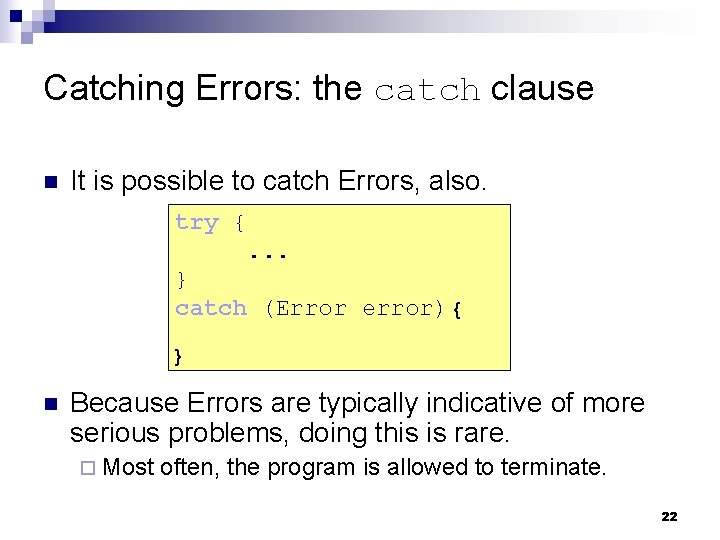
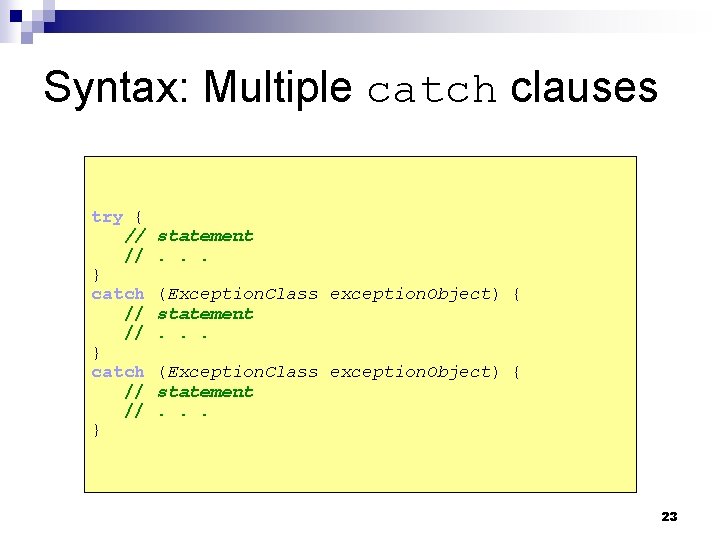
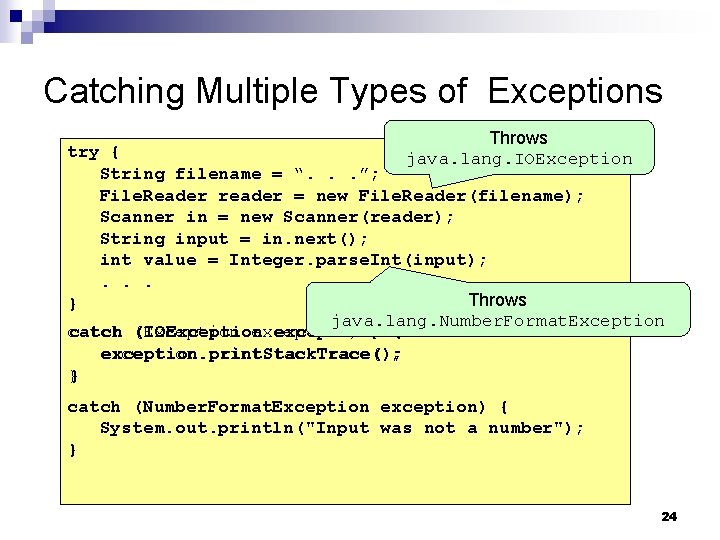
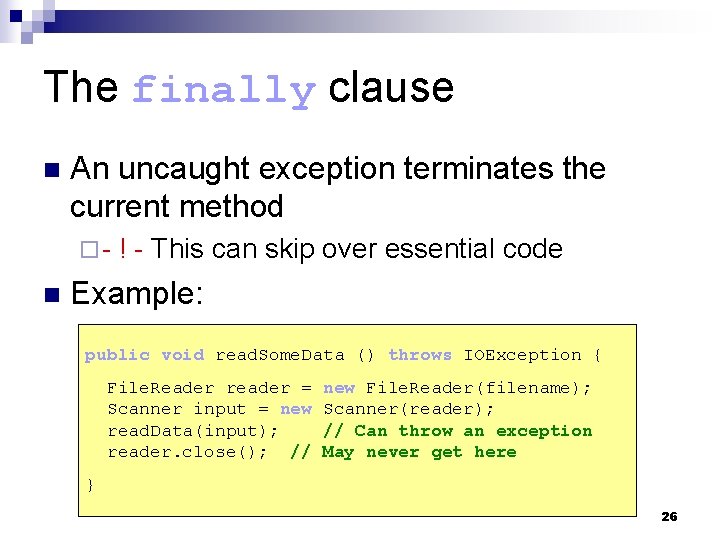
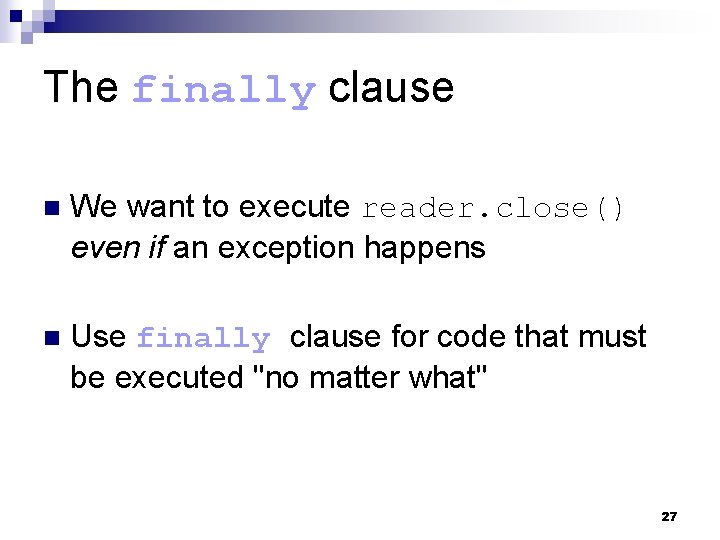
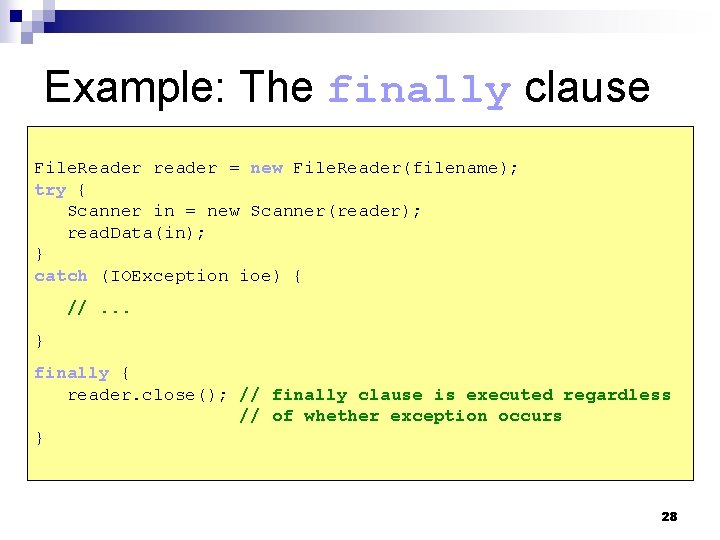
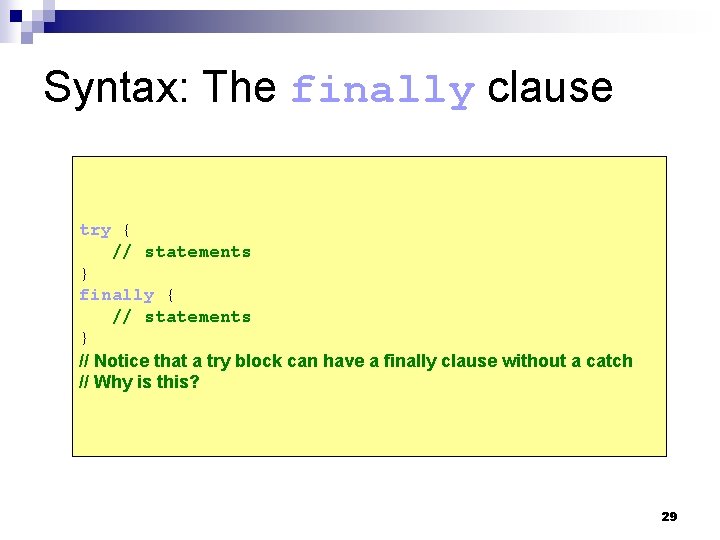
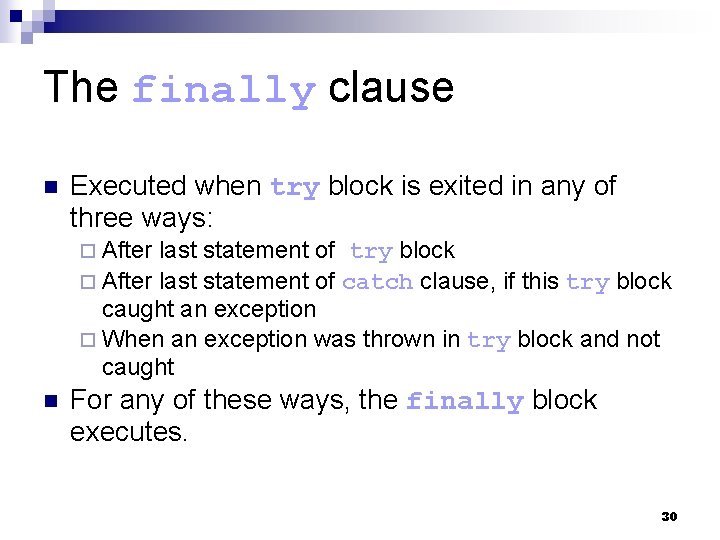
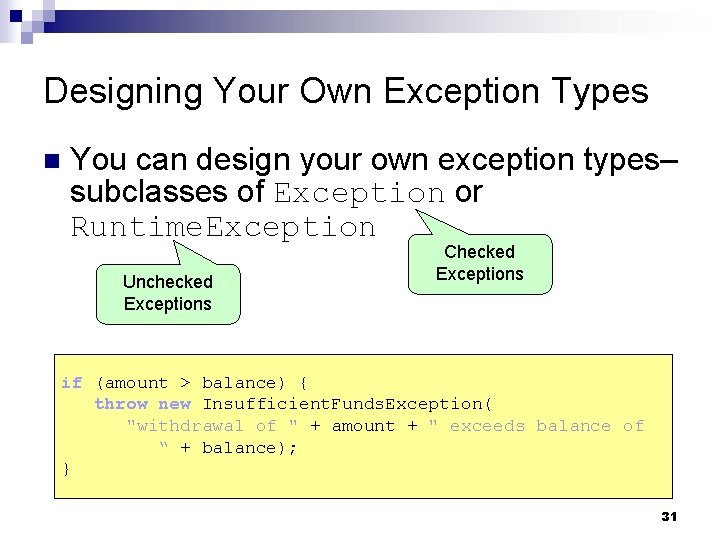
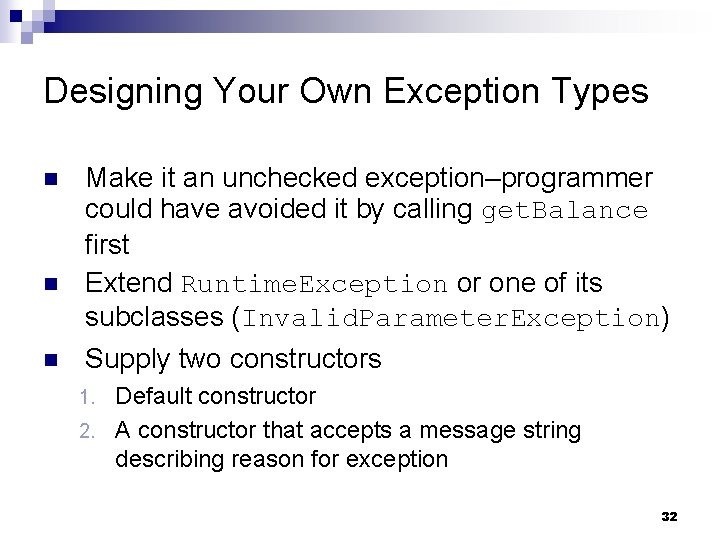
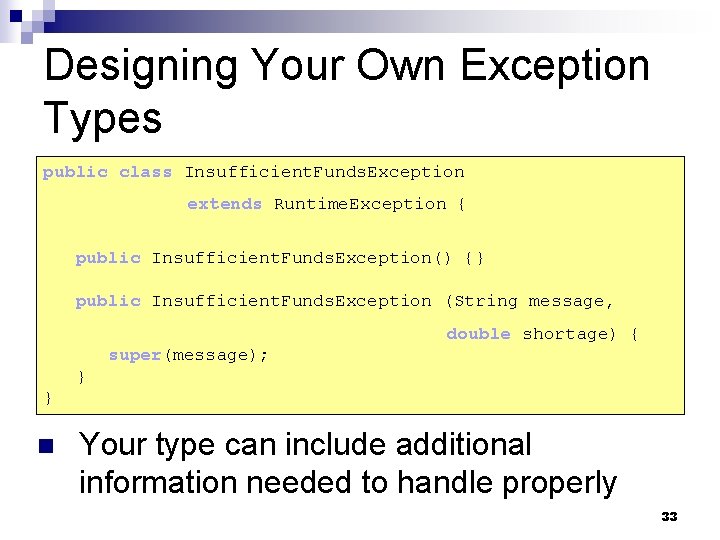
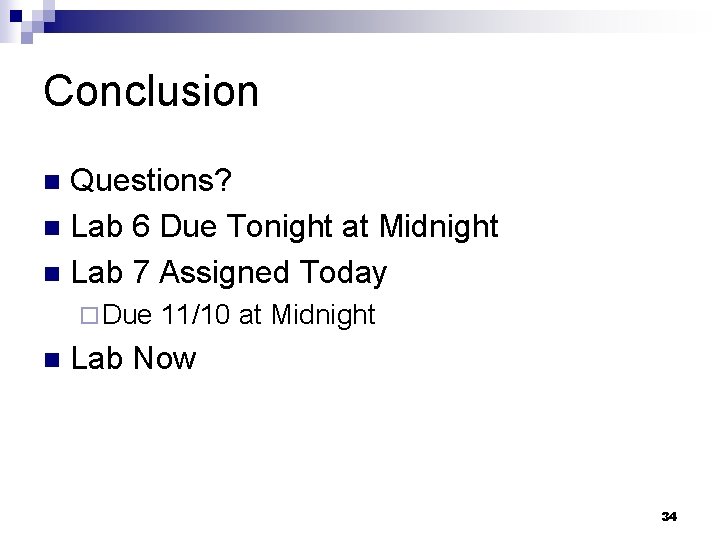
- Slides: 33
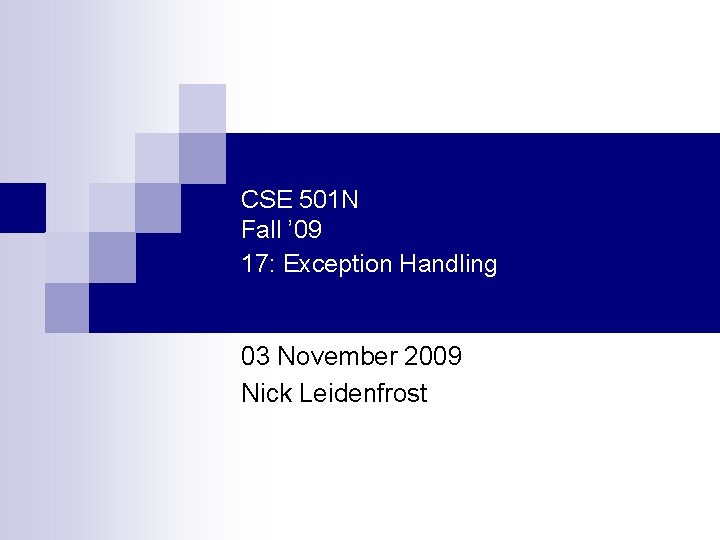
CSE 501 N Fall ’ 09 17: Exception Handling 03 November 2009 Nick Leidenfrost
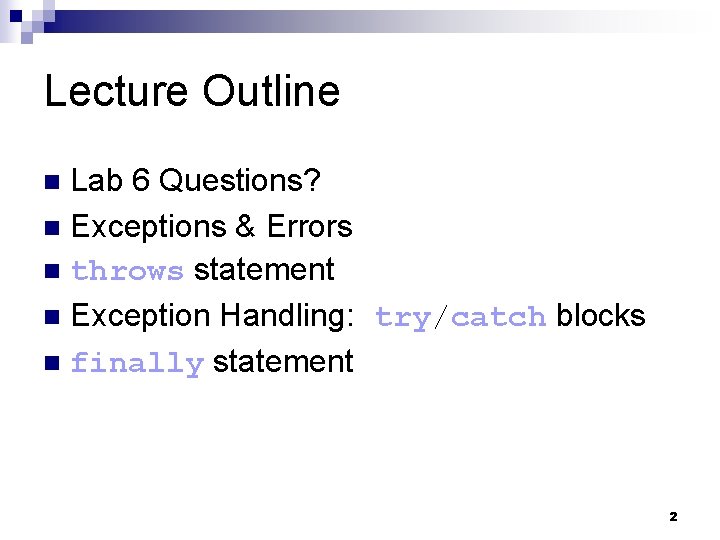
Lecture Outline Lab 6 Questions? n Exceptions & Errors n throws statement n Exception Handling: try/catch blocks n finally statement n 2
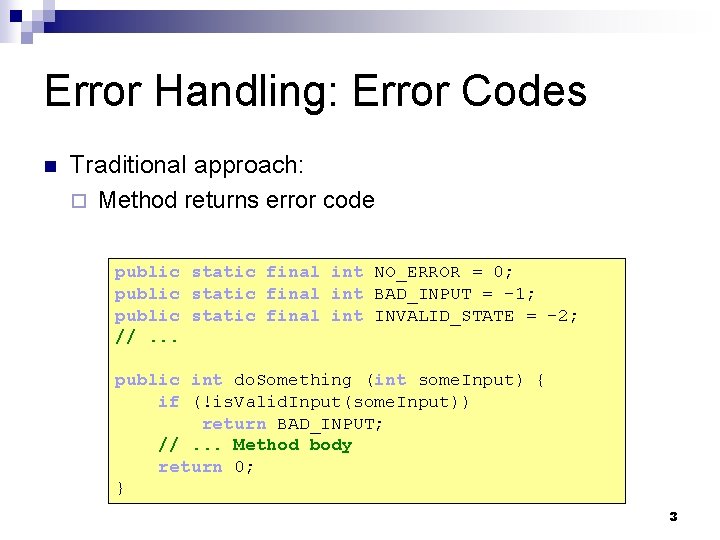
Error Handling: Error Codes n Traditional approach: ¨ Method returns error code public static final int NO_ERROR = 0; public static final int BAD_INPUT = -1; public static final int INVALID_STATE = -2; //. . . public int do. Something (int some. Input) { if (!is. Valid. Input(some. Input)) return BAD_INPUT; //. . . Method body return 0; } 3
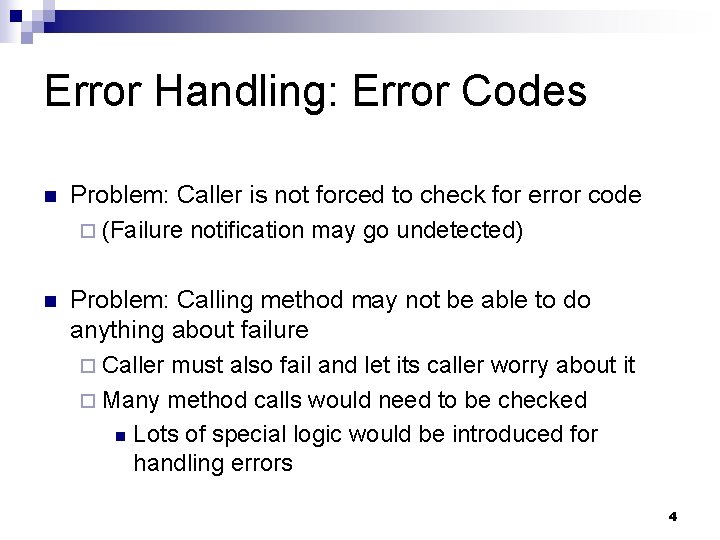
Error Handling: Error Codes n Problem: Caller is not forced to check for error code ¨ (Failure notification may go undetected) n Problem: Calling method may not be able to do anything about failure ¨ Caller must also fail and let its caller worry about it ¨ Many method calls would need to be checked n Lots of special logic would be introduced for handling errors 4
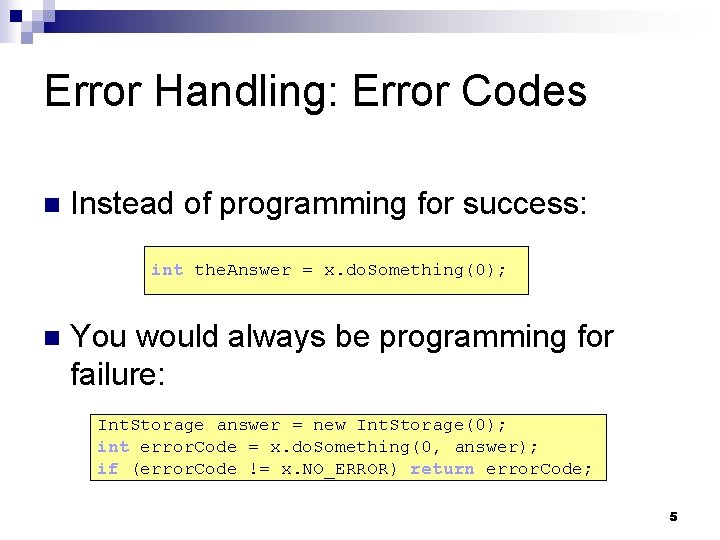
Error Handling: Error Codes n Instead of programming for success: int the. Answer = x. do. Something(0); n You would always be programming for failure: Int. Storage answer = new Int. Storage(0); int error. Code = x. do. Something(0, answer); if (error. Code != x. NO_ERROR) return error. Code; 5
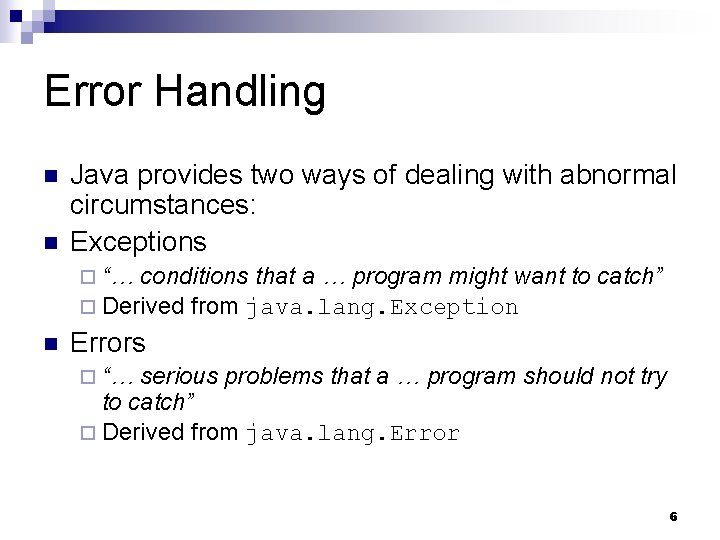
Error Handling n n Java provides two ways of dealing with abnormal circumstances: Exceptions ¨ “… conditions that a … program might want to catch” ¨ Derived from java. lang. Exception n Errors ¨ “… serious problems that a … program should not try to catch” ¨ Derived from java. lang. Error 6
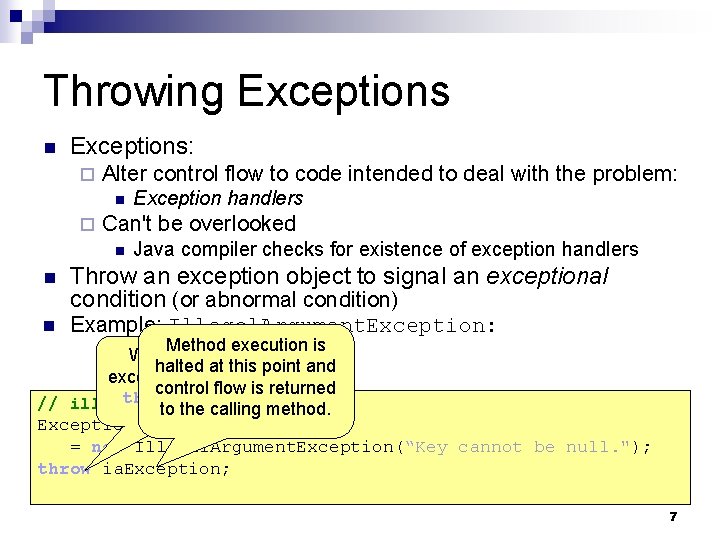
Throwing Exceptions n Exceptions: ¨ Alter control flow to code intended to deal with the problem: n ¨ Exception handlers Can't be overlooked n Java compiler checks for existence of exception handlers n Throw an exception object to signal an exceptional condition (or abnormal condition) n Example: Illegal. Argument. Exception: Method execution is We throw an halted at this point and exception with the control flow is returned throw clause. // illegal parameter to the calling value method. Exception ia. Exception = new Illegal. Argument. Exception(“Key cannot be null. "); throw ia. Exception; 7
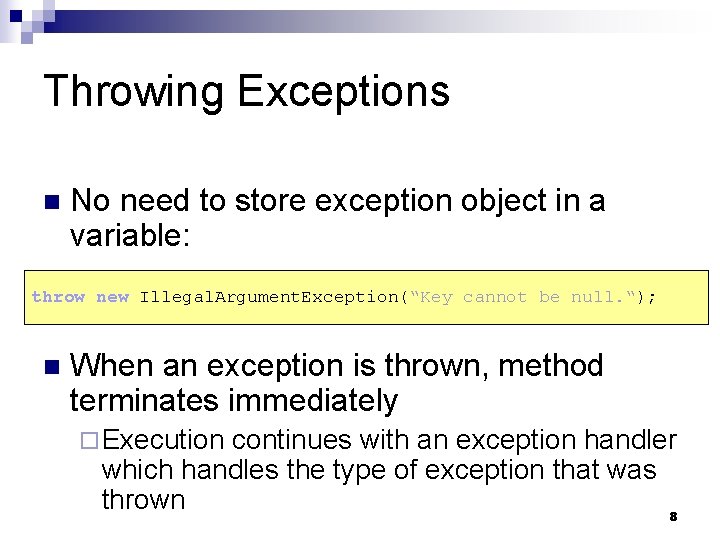
Throwing Exceptions n No need to store exception object in a variable: throw new Illegal. Argument. Exception(“Key cannot be null. “); n When an exception is thrown, method terminates immediately ¨ Execution continues with an exception handler which handles the type of exception that was thrown 8
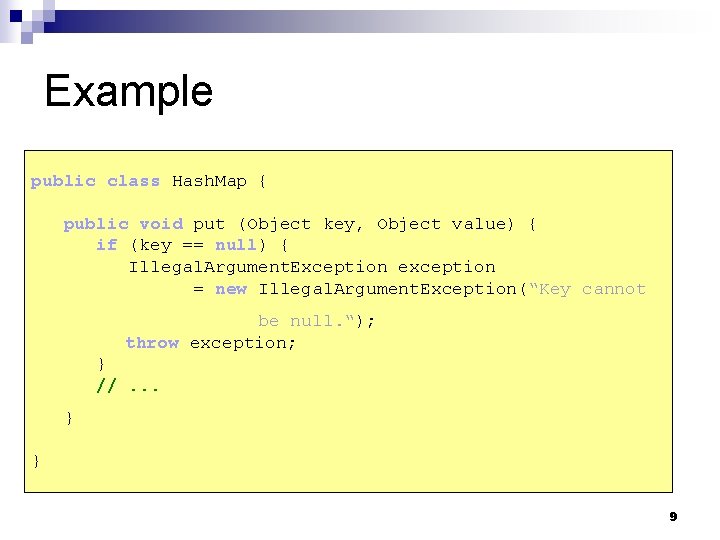
Example public class Hash. Map { public void put (Object key, Object value) { if (key == null) { Illegal. Argument. Exception exception = new Illegal. Argument. Exception(“Key cannot be null. “); throw exception; } //. . . } } 9
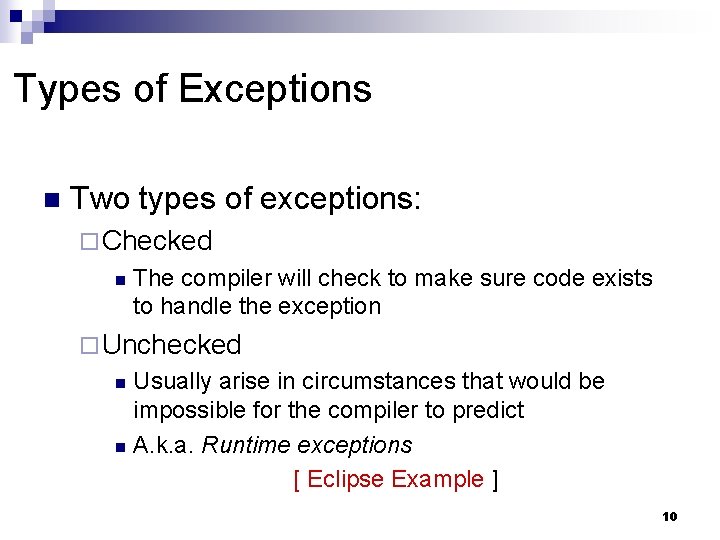
Types of Exceptions n Two types of exceptions: ¨ Checked n The compiler will check to make sure code exists to handle the exception ¨ Unchecked Usually arise in circumstances that would be impossible for the compiler to predict n A. k. a. Runtime exceptions [ Eclipse Example ] n 10
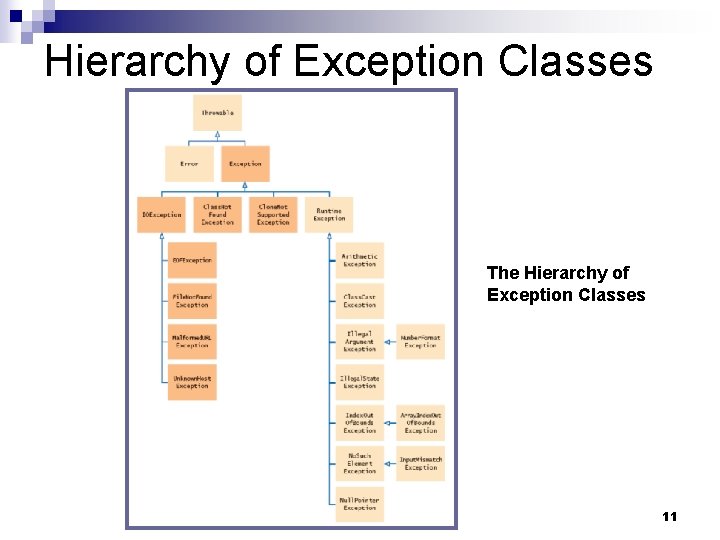
Hierarchy of Exception Classes The Hierarchy of Exception Classes 11
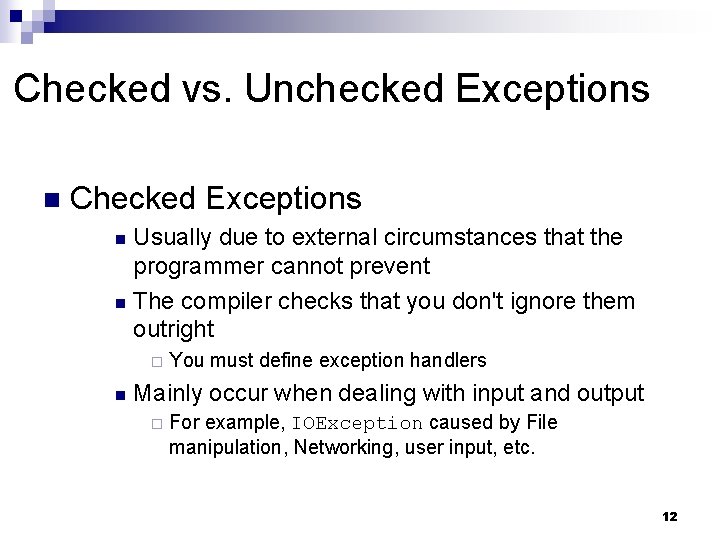
Checked vs. Unchecked Exceptions n Checked Exceptions Usually due to external circumstances that the programmer cannot prevent n The compiler checks that you don't ignore them outright n ¨ n You must define exception handlers Mainly occur when dealing with input and output ¨ For example, IOException caused by File manipulation, Networking, user input, etc. 12
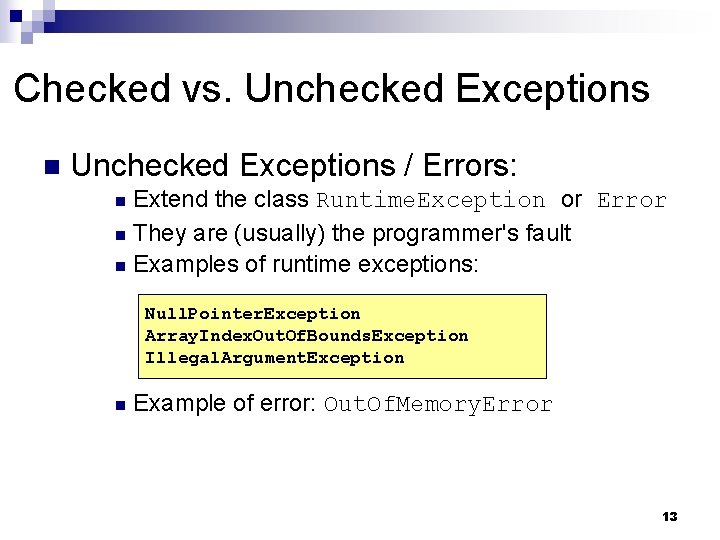
Checked vs. Unchecked Exceptions n Unchecked Exceptions / Errors: Extend the class Runtime. Exception or Error n They are (usually) the programmer's fault n Examples of runtime exceptions: n Null. Pointer. Exception Array. Index. Out. Of. Bounds. Exception Illegal. Argument. Exception n Example of error: Out. Of. Memory. Error 13
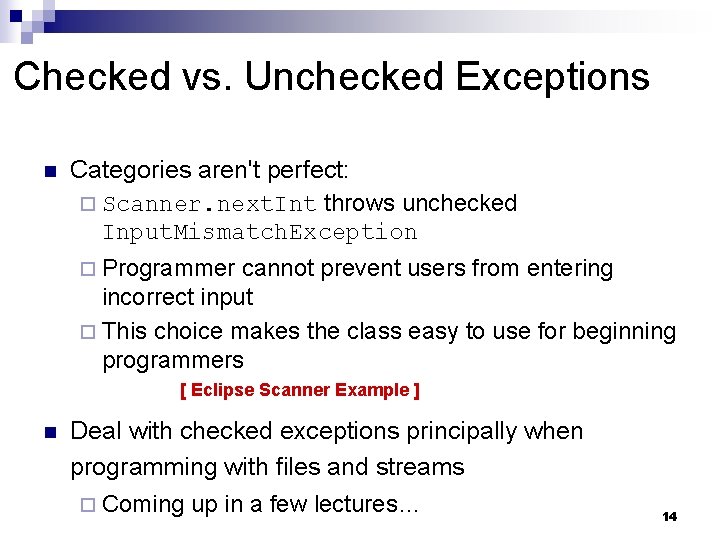
Checked vs. Unchecked Exceptions n Categories aren't perfect: ¨ Scanner. next. Int throws unchecked Input. Mismatch. Exception ¨ Programmer cannot prevent users from entering incorrect input ¨ This choice makes the class easy to use for beginning programmers [ Eclipse Scanner Example ] n Deal with checked exceptions principally when programming with files and streams ¨ Coming up in a few lectures… 14
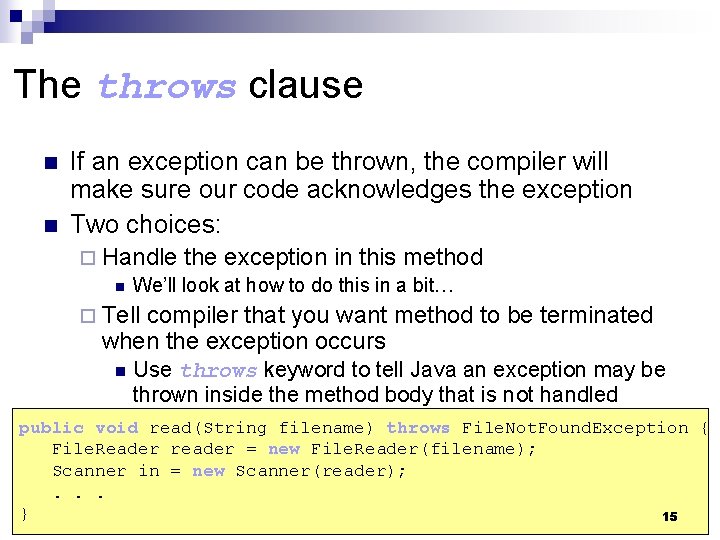
The throws clause n n If an exception can be thrown, the compiler will make sure our code acknowledges the exception Two choices: ¨ Handle the exception in this method n We’ll look at how to do this in a bit… ¨ Tell compiler that you want method to be terminated when the exception occurs n Use throws keyword to tell Java an exception may be thrown inside the method body that is not handled public void read(String filename) throws File. Not. Found. Exception { File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); . . . } 15
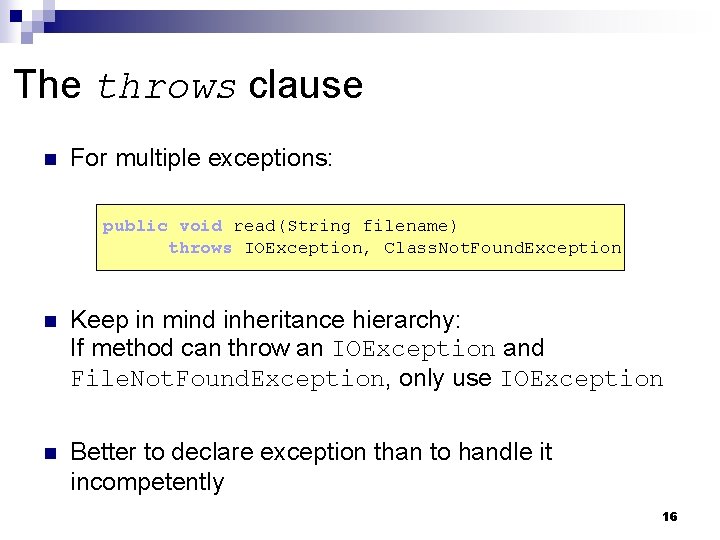
The throws clause n For multiple exceptions: public void read(String filename) throws IOException, Class. Not. Found. Exception n Keep in mind inheritance hierarchy: If method can throw an IOException and File. Not. Found. Exception, only use IOException n Better to declare exception than to handle it incompetently 16
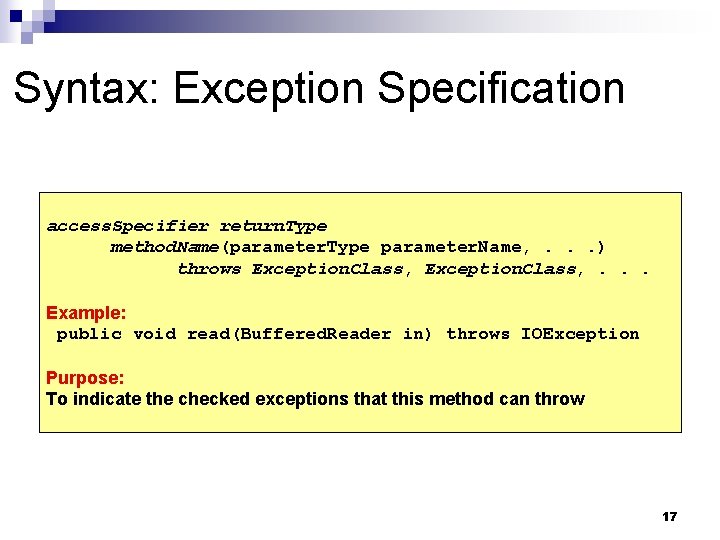
Syntax: Exception Specification access. Specifier return. Type method. Name(parameter. Type parameter. Name, . . . ) throws Exception. Class, . . . Example: public void read(Buffered. Reader in) throws IOException Purpose: To indicate the checked exceptions that this method can throw 17
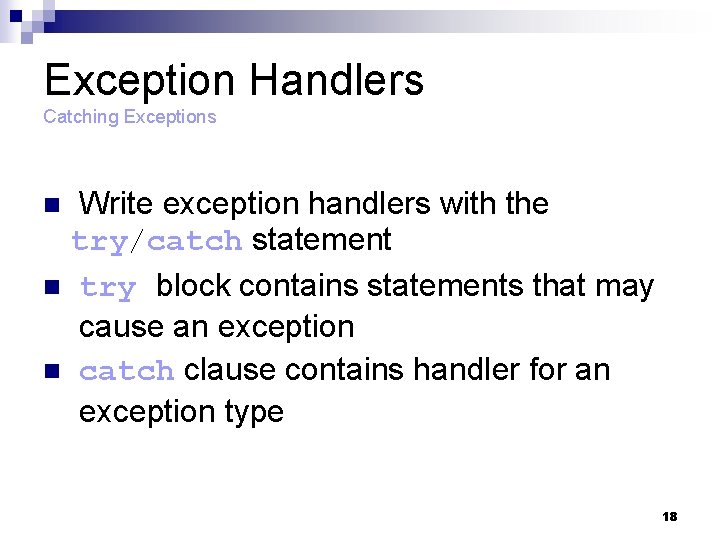
Exception Handlers Catching Exceptions Write exception handlers with the try/catch statement n try block contains statements that may cause an exception n catch clause contains handler for an exception type n 18
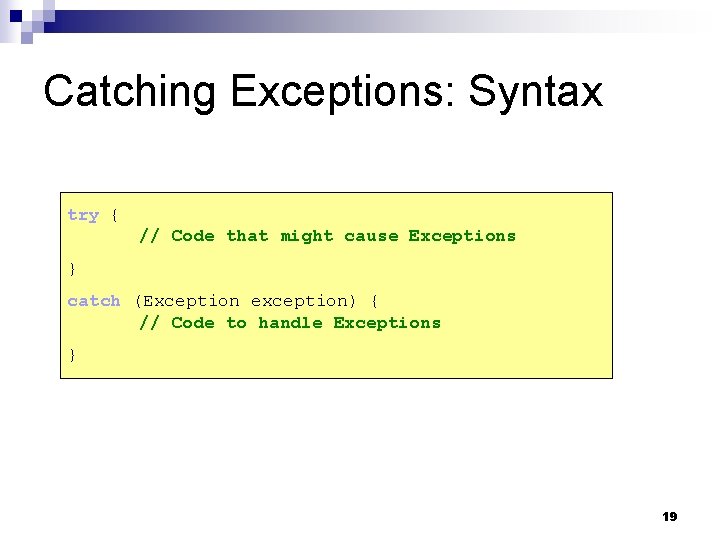
Catching Exceptions: Syntax try { // Code that might cause Exceptions } catch (Exception exception) { // Code to handle Exceptions } 19
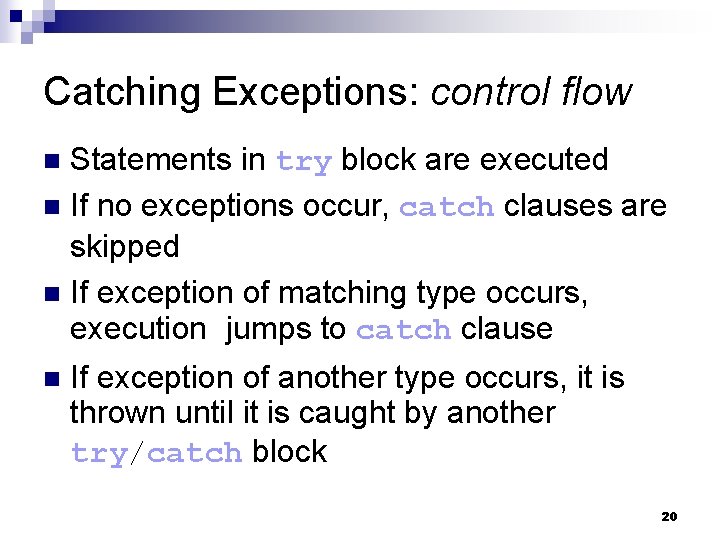
Catching Exceptions: control flow Statements in try block are executed n If no exceptions occur, catch clauses are skipped n If exception of matching type occurs, execution jumps to catch clause n n If exception of another type occurs, it is thrown until it is caught by another try/catch block 20
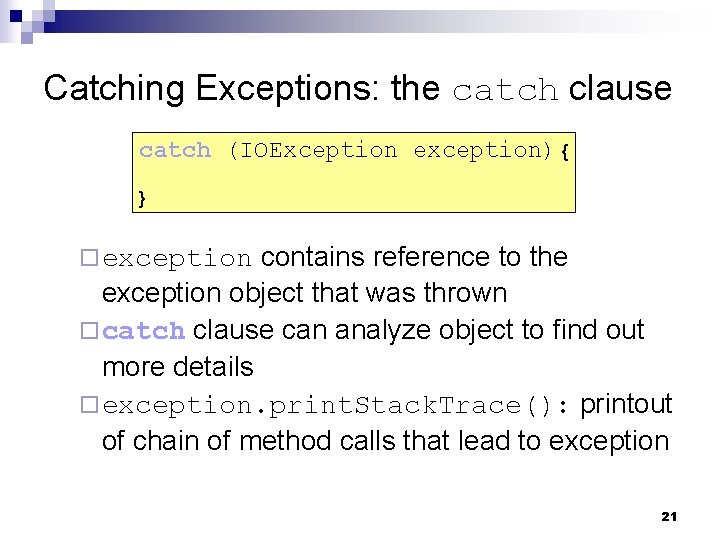
Catching Exceptions: the catch clause catch (IOException exception) { } contains reference to the exception object that was thrown ¨ catch clause can analyze object to find out more details ¨ exception. print. Stack. Trace(): printout of chain of method calls that lead to exception ¨ exception 21
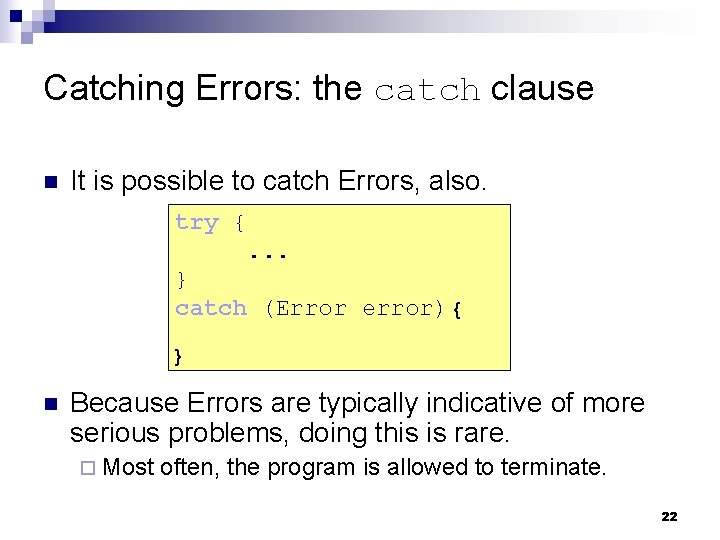
Catching Errors: the catch clause n It is possible to catch Errors, also. try {. . . } catch (Error error) { } n Because Errors are typically indicative of more serious problems, doing this is rare. ¨ Most often, the program is allowed to terminate. 22
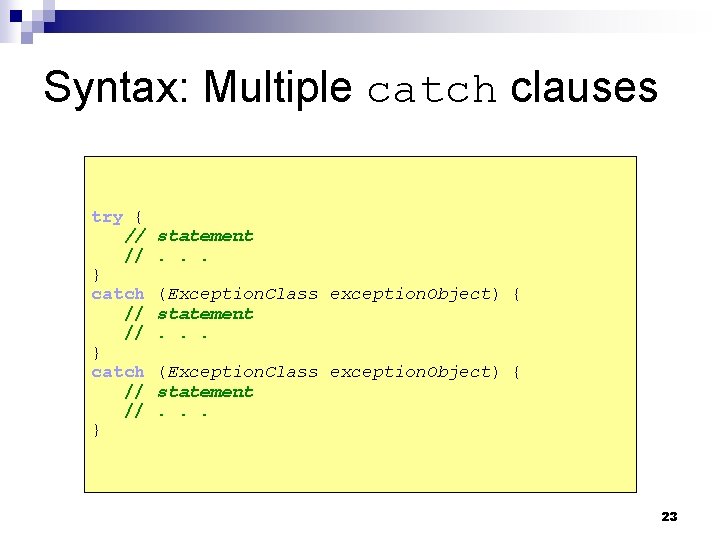
Syntax: Multiple catch clauses try { // // } catch // // } statement. . . (Exception. Class exception. Object) { statement. . . 23
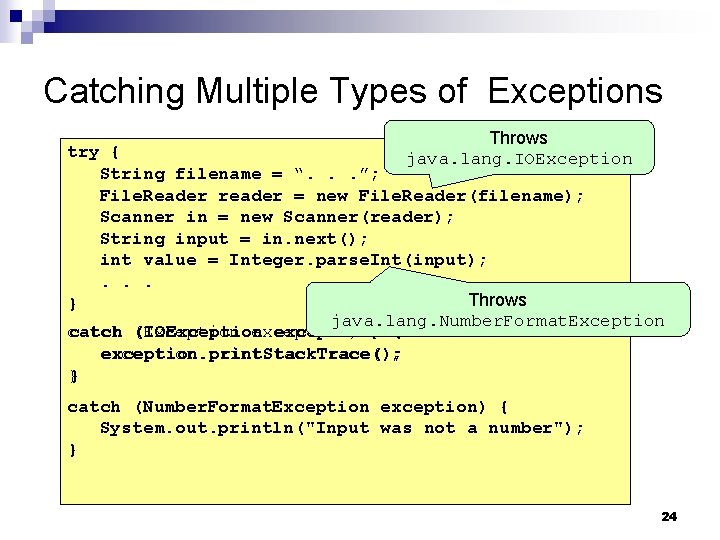
Catching Multiple Types of Exceptions Throws java. lang. IOException try { String filename = “. . . ”; File. Reader reader = new File. Reader(filename); Scanner in = new Scanner(reader); String input = in. next(); int value = Integer. parse. Int(input); . . . Throws } java. lang. Number. Format. Exception catch (Exception (IOException exception) { { exception. print. Stack. Trace(); } catch (Number. Format. Exception exception) { System. out. println("Input was not a number"); } 24
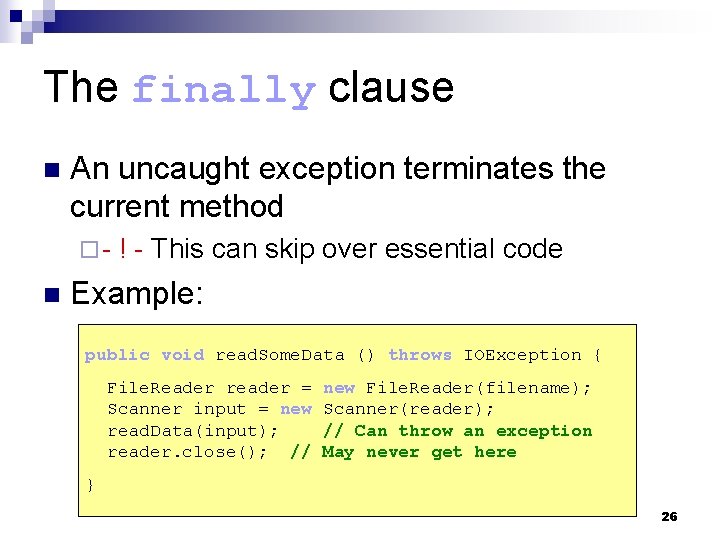
The finally clause n An uncaught exception terminates the current method ¨- n ! - This can skip over essential code Example: public void read. Some. Data () throws IOException { File. Reader reader = Scanner input = new read. Data(input); reader. close(); // new File. Reader(filename); Scanner(reader); // Can throw an exception May never get here } 26
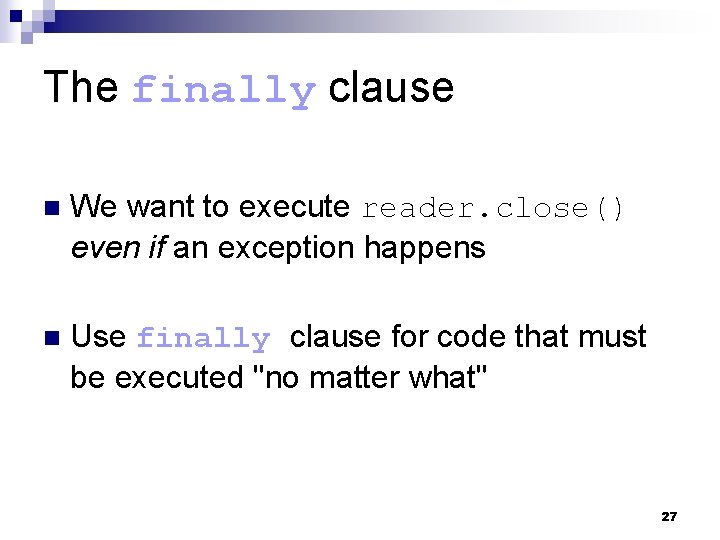
The finally clause n We want to execute reader. close() even if an exception happens n Use finally clause for code that must be executed "no matter what" 27
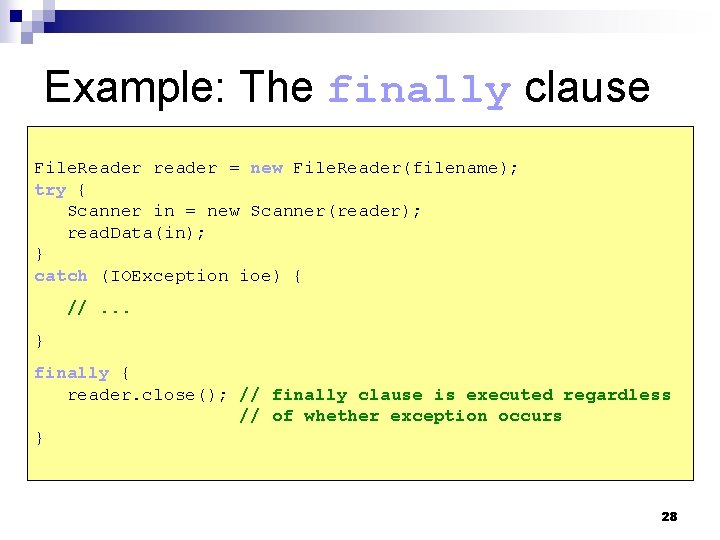
Example: The finally clause File. Reader reader = new File. Reader(filename); try { Scanner in = new Scanner(reader); read. Data(in); } catch (IOException ioe) { //. . . } finally { reader. close(); // finally clause is executed regardless // of whether exception occurs } 28
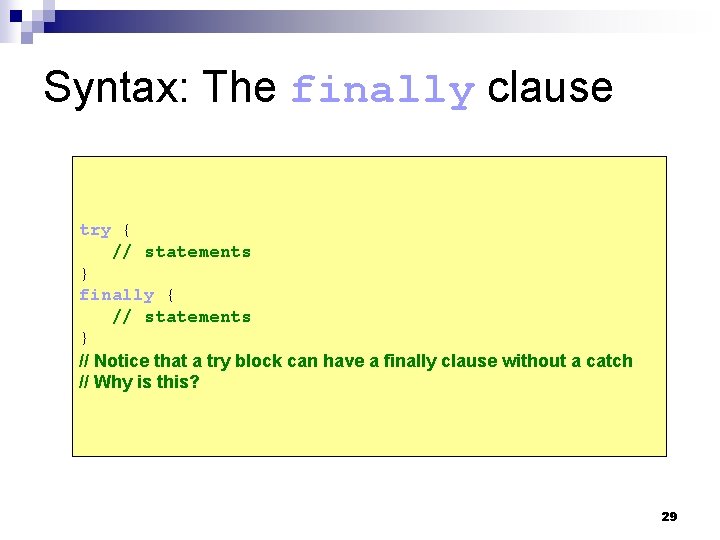
Syntax: The finally clause try { // statements } finally { // statements } // Notice that a try block can have a finally clause without a catch // Why is this? 29
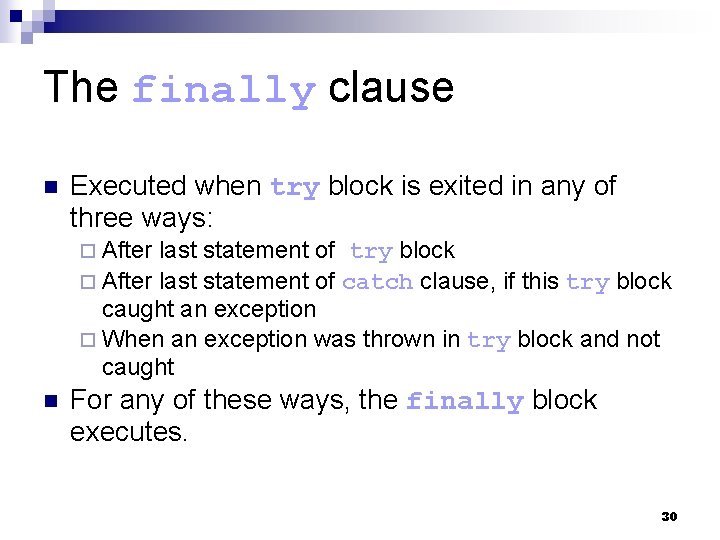
The finally clause n Executed when try block is exited in any of three ways: ¨ After last statement of try block ¨ After last statement of catch clause, if this try block caught an exception ¨ When an exception was thrown in try block and not caught n For any of these ways, the finally block executes. 30
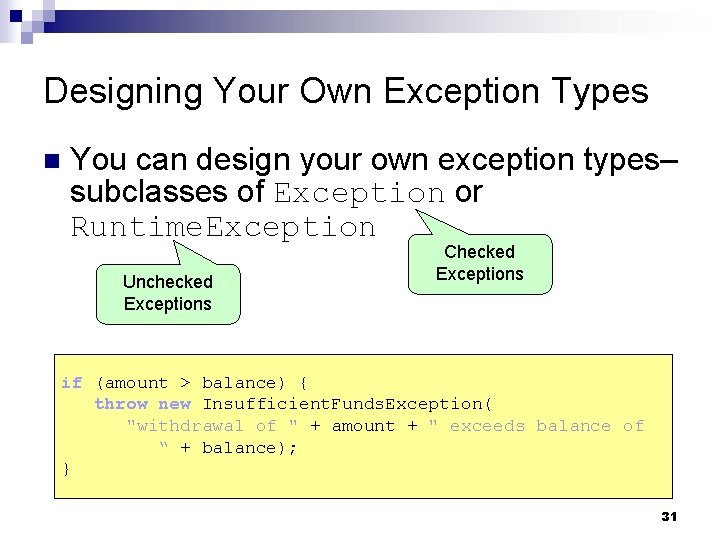
Designing Your Own Exception Types n You can design your own exception types– subclasses of Exception or Runtime. Exception Unchecked Exceptions Checked Exceptions if (amount > balance) { throw new Insufficient. Funds. Exception( "withdrawal of " + amount + " exceeds balance of “ + balance); } 31
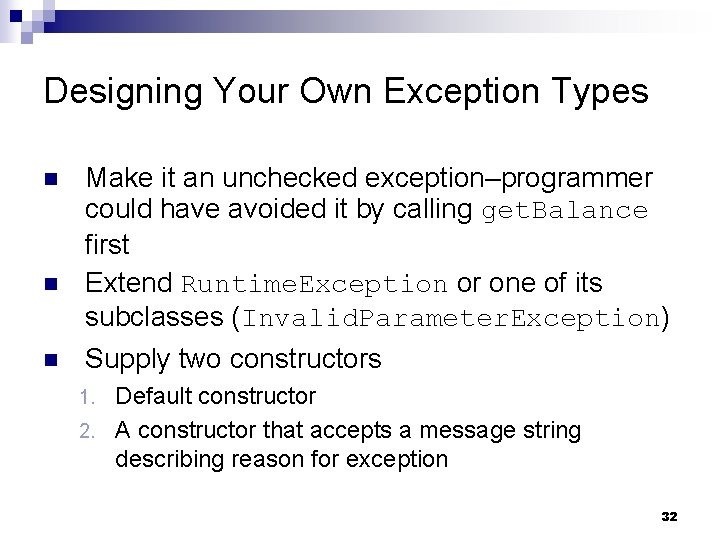
Designing Your Own Exception Types n n n Make it an unchecked exception–programmer could have avoided it by calling get. Balance first Extend Runtime. Exception or one of its subclasses (Invalid. Parameter. Exception) Supply two constructors Default constructor 2. A constructor that accepts a message string describing reason for exception 1. 32
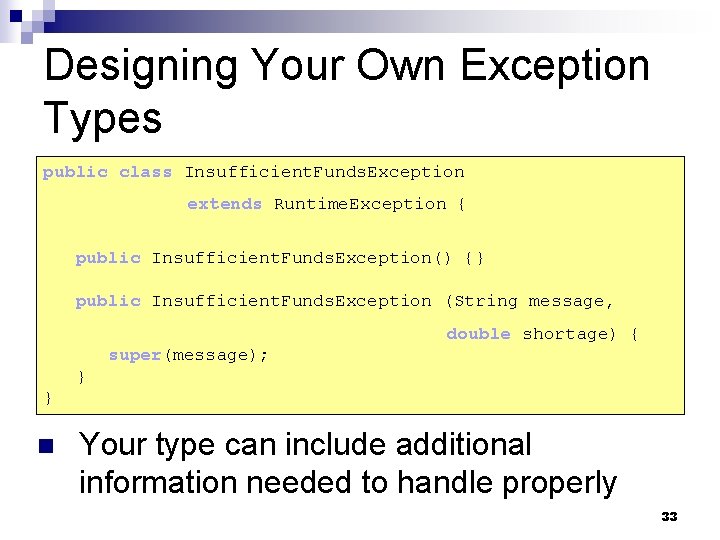
Designing Your Own Exception Types public class Insufficient. Funds. Exception extends Runtime. Exception { } } n public Insufficient. Funds. Exception() {} public Insufficient. Funds. Exception (String message, public Insufficient. Funds. Exception (String message) { double shortage) { super(message); } Your type can include additional information needed to handle properly 33
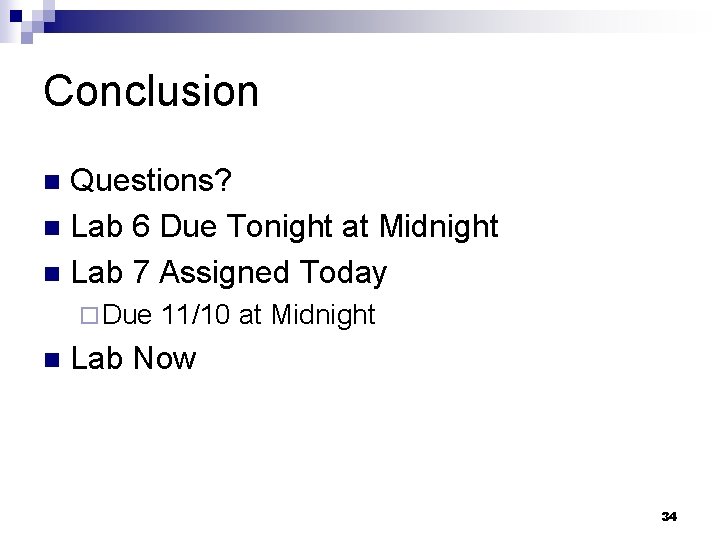
Conclusion Questions? n Lab 6 Due Tonight at Midnight n Lab 7 Assigned Today n ¨ Due n 11/10 at Midnight Lab Now 34
Php throw exception
Exception handling in vb net
Exception handling pada java
Vb.net error handling
Exception handling in java
No irq handler for vector
Apa itu exception
Exception handling in ada
Exception handling pl sql
Php exception example
Bios 501
Cas 501
Cis 501
Ubc canvs
Bds 501
Acilyse
Hinario 501
Cis 501
Opwekking 501
I 501
Mgt 501
Cisco pix 501 specs
Cis 501
Cis 501
501 hinário
Simple capm
Cs-501
Cis 501
W 501
Agodi salaris
I 501
Mgt 501
Nia inventarios
Ariane 5 flight 501