CSE 501 N Fall 09 05 Predicates and
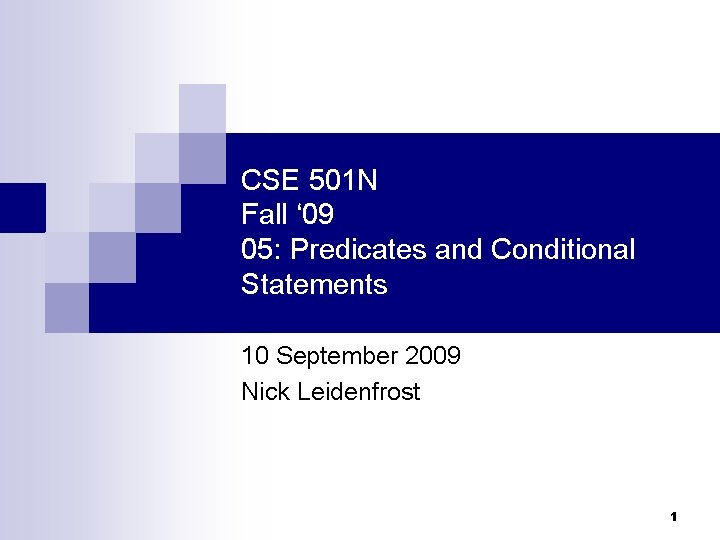
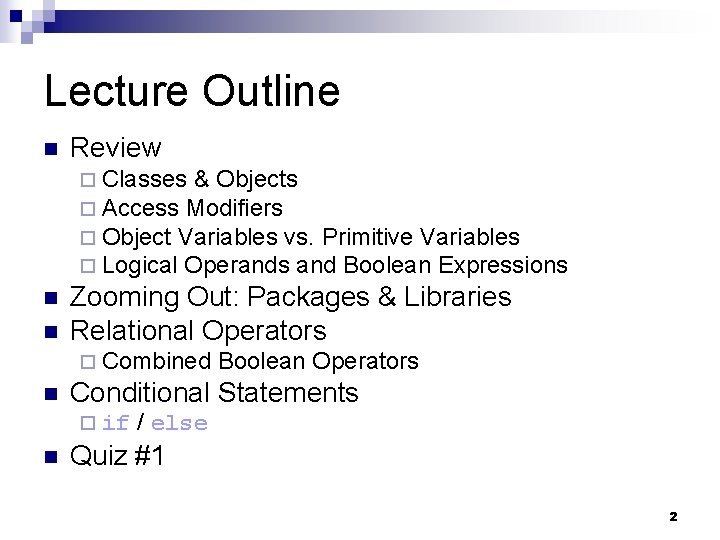
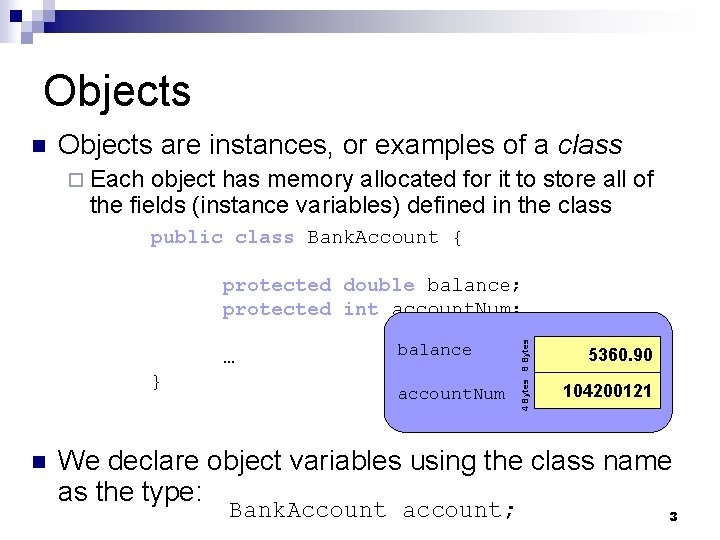
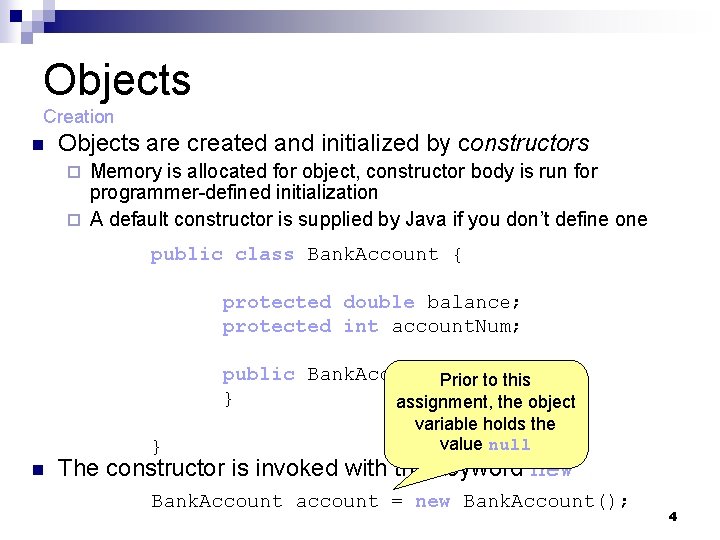
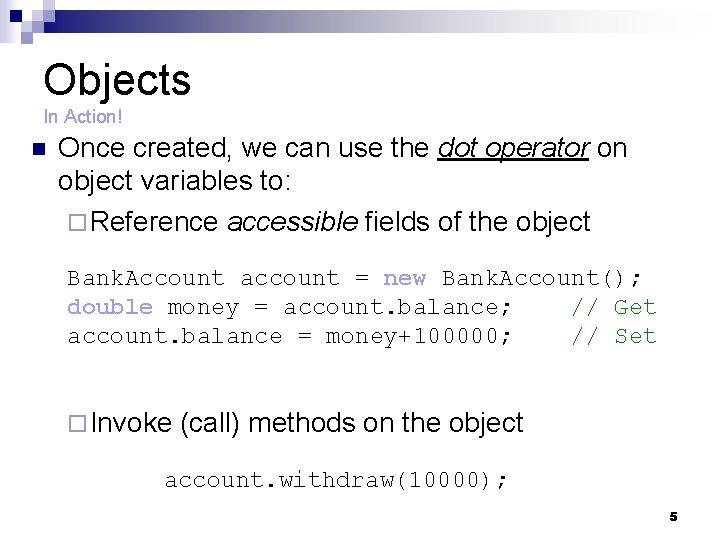
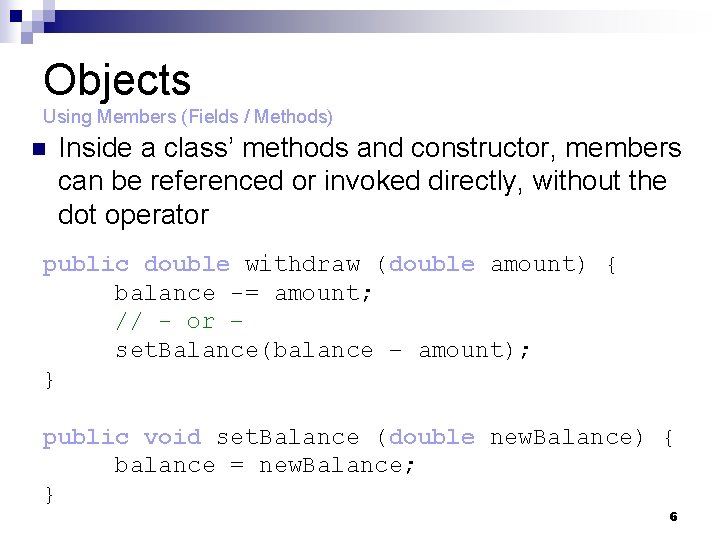
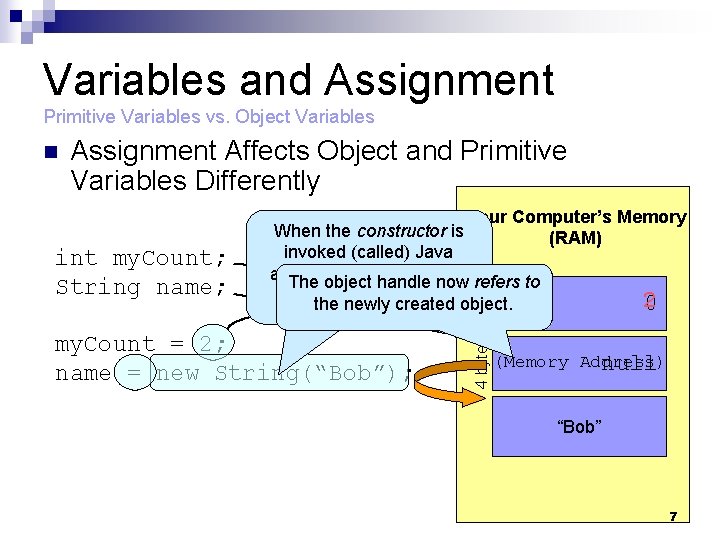
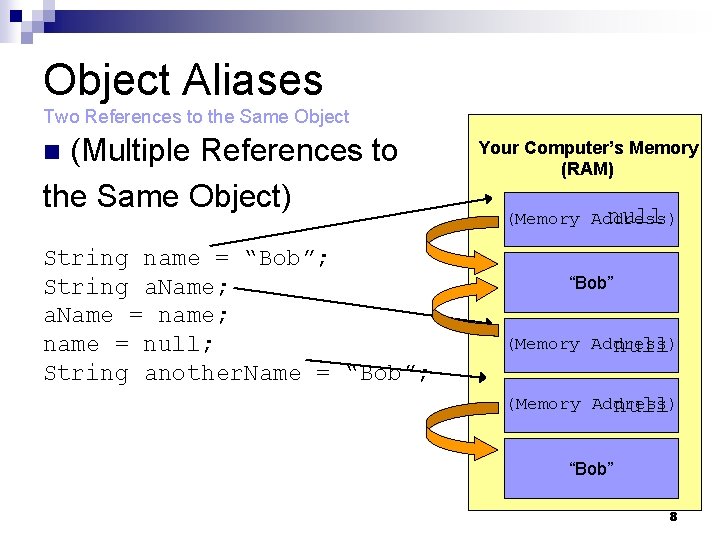
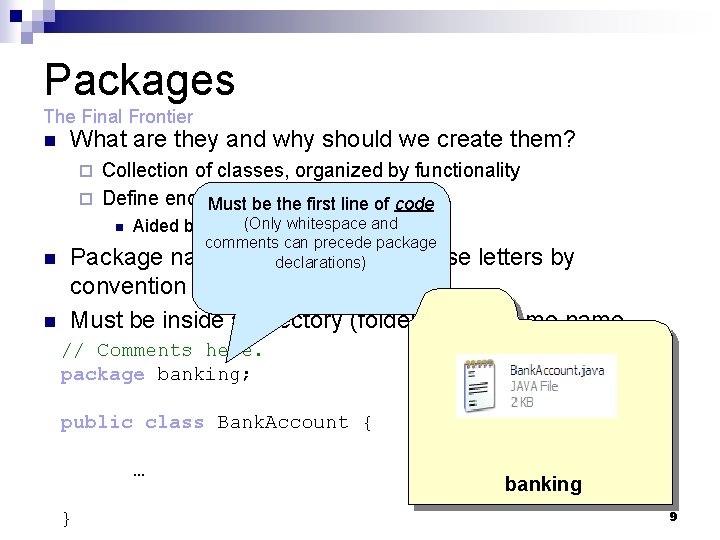
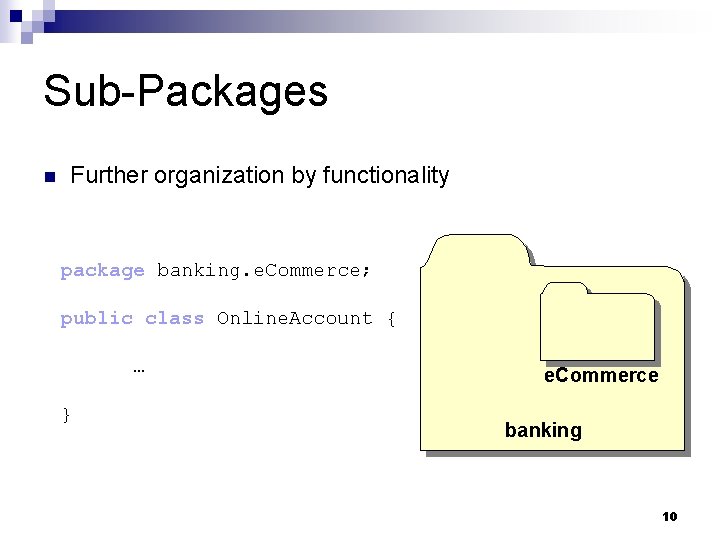
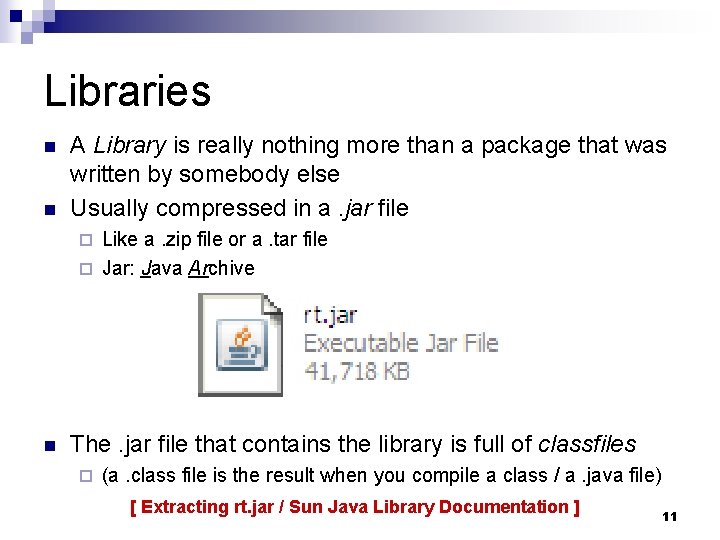
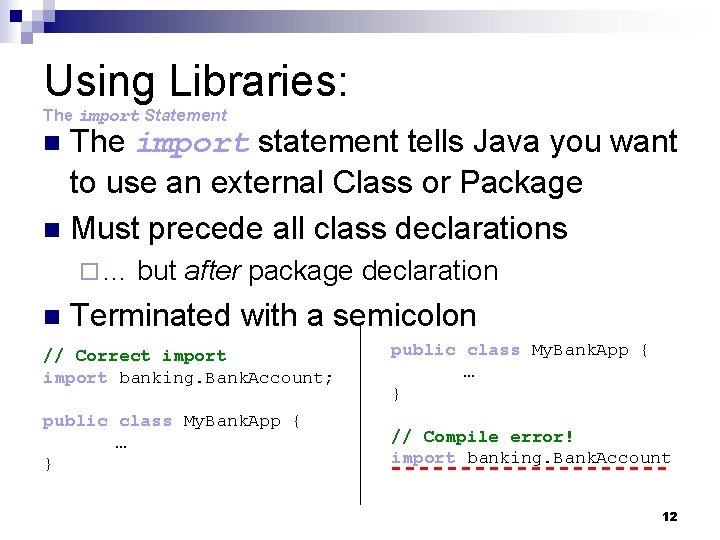
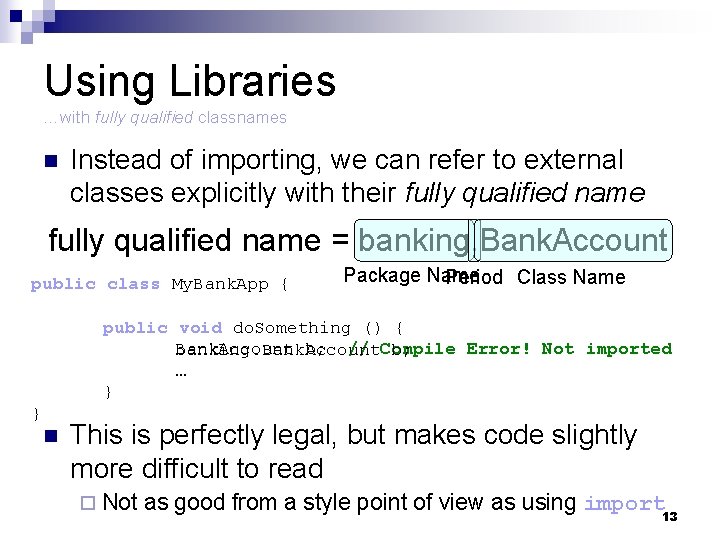
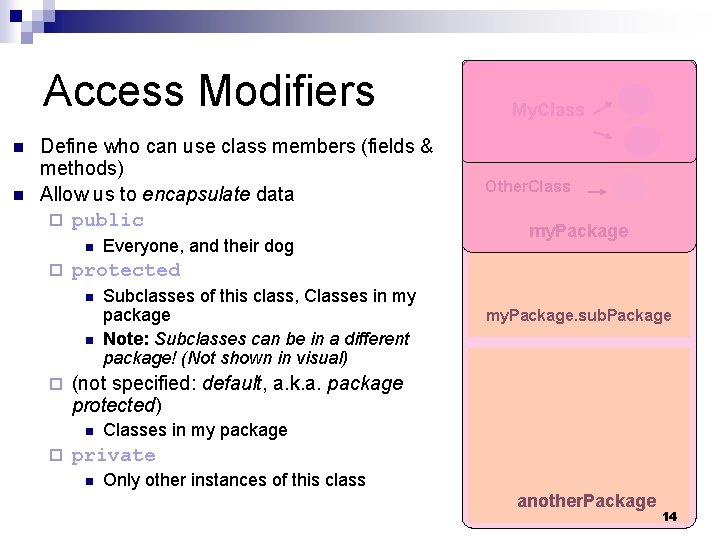
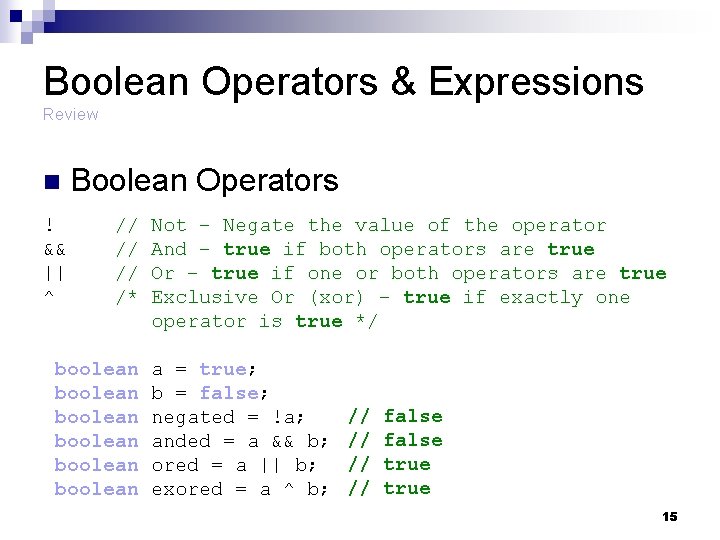
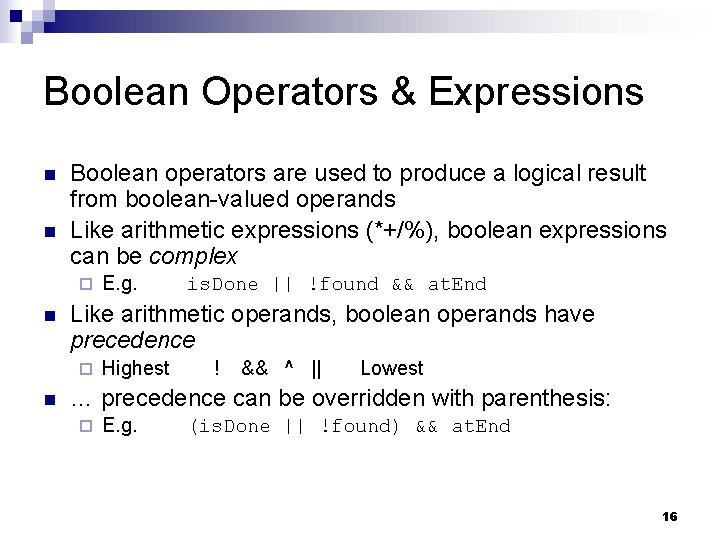
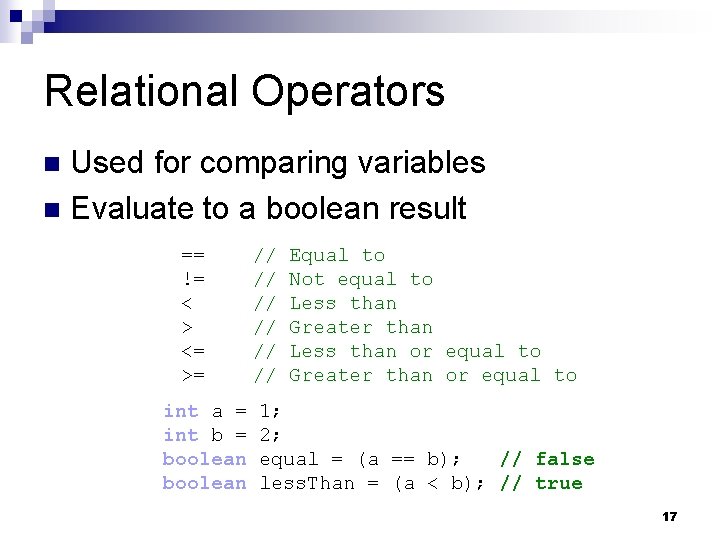
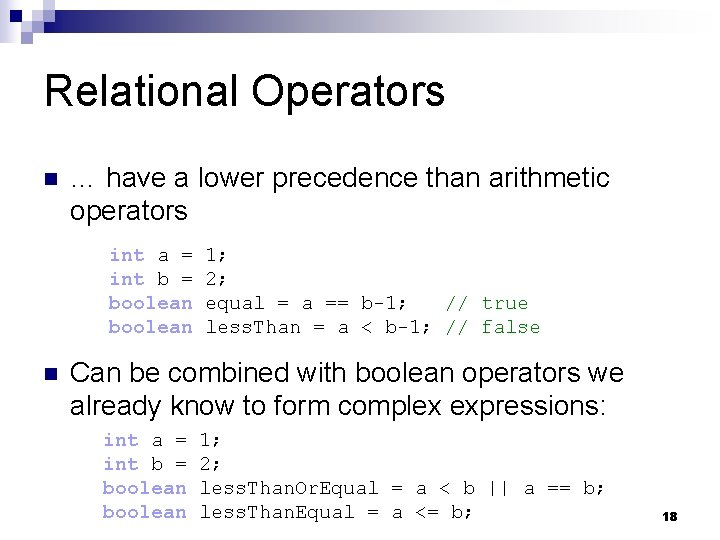
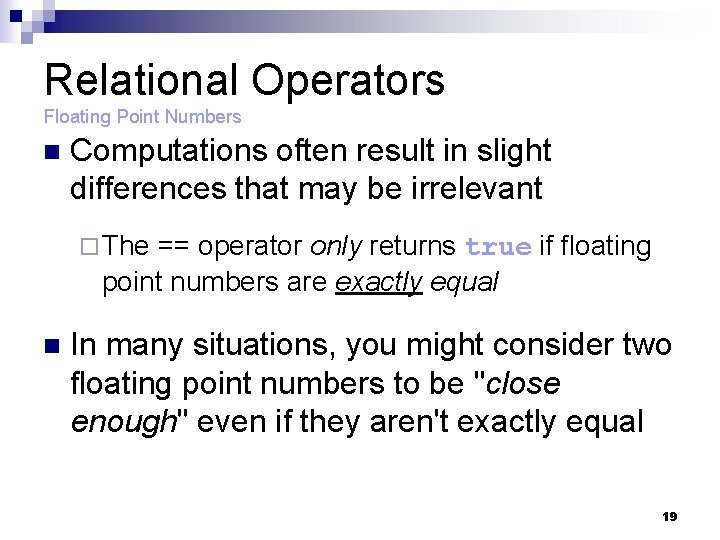
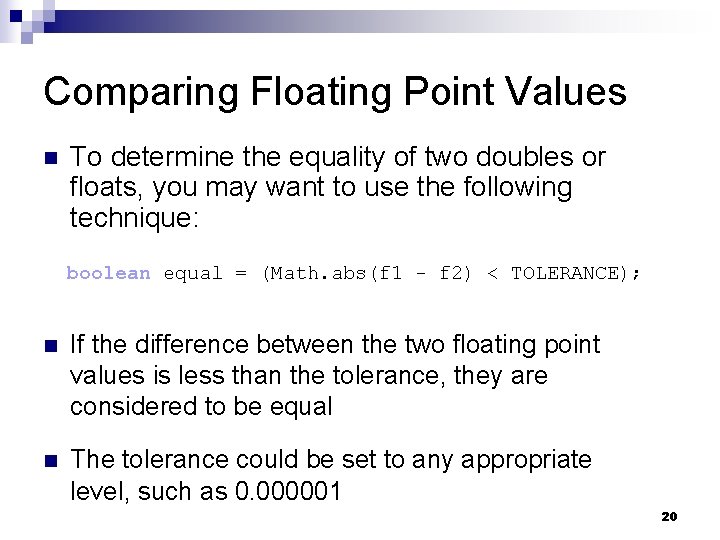
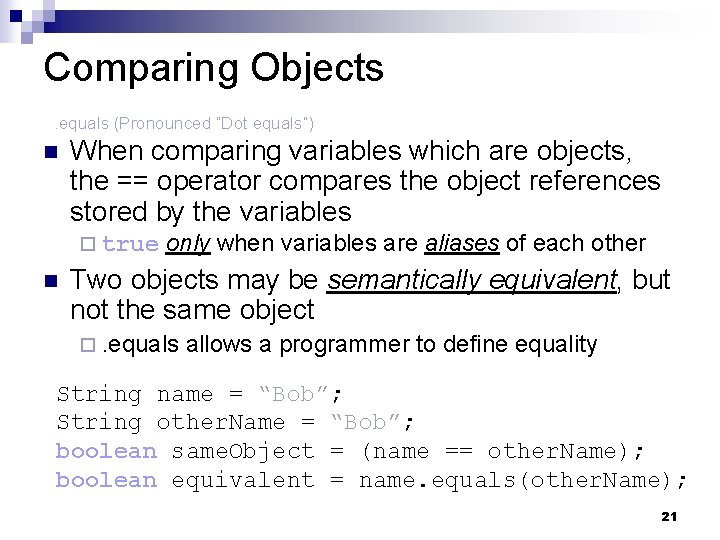
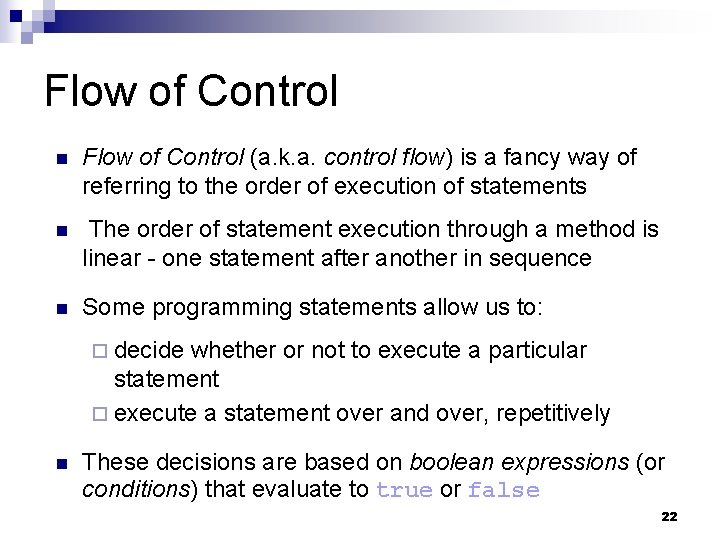
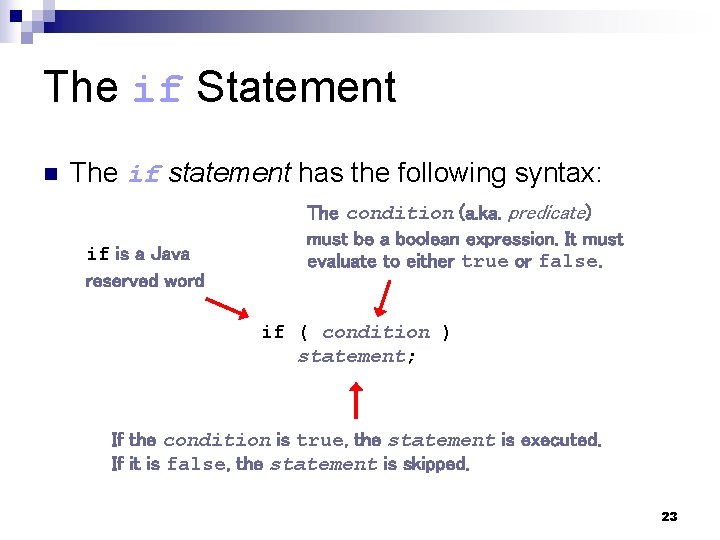
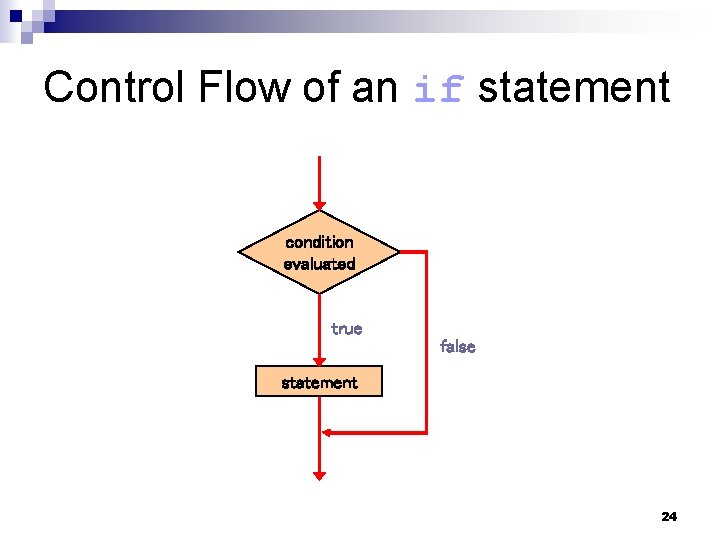
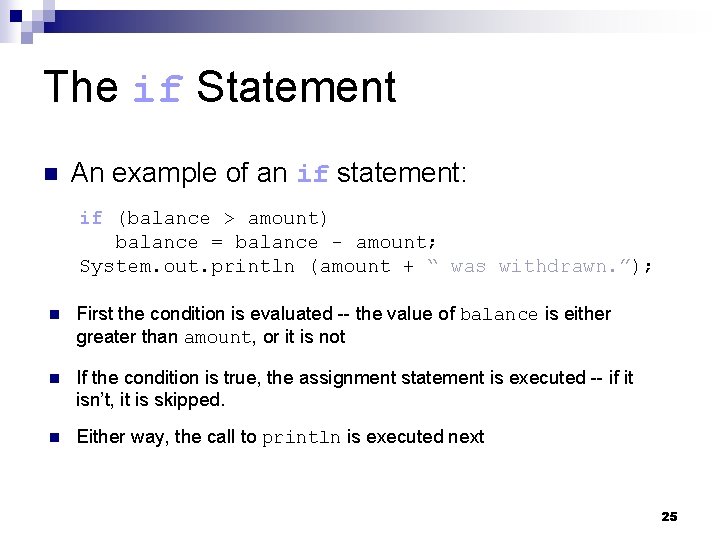
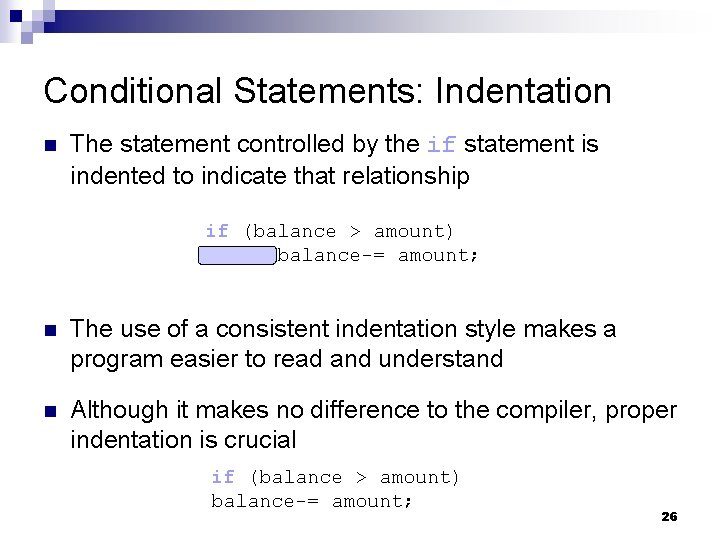
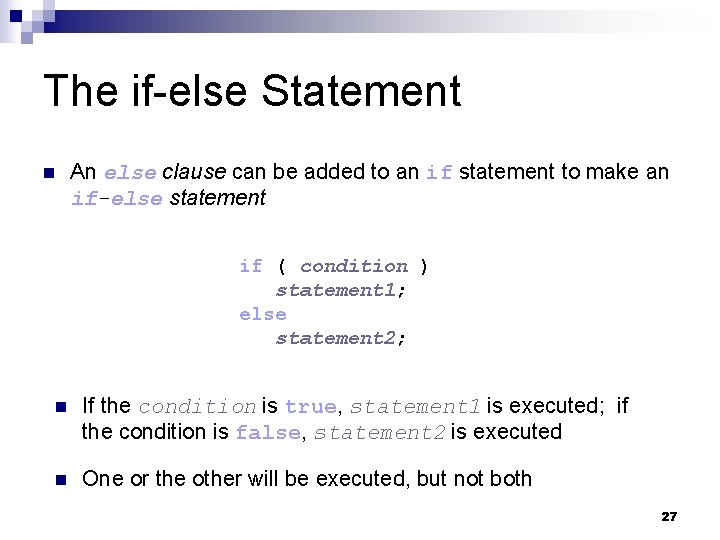
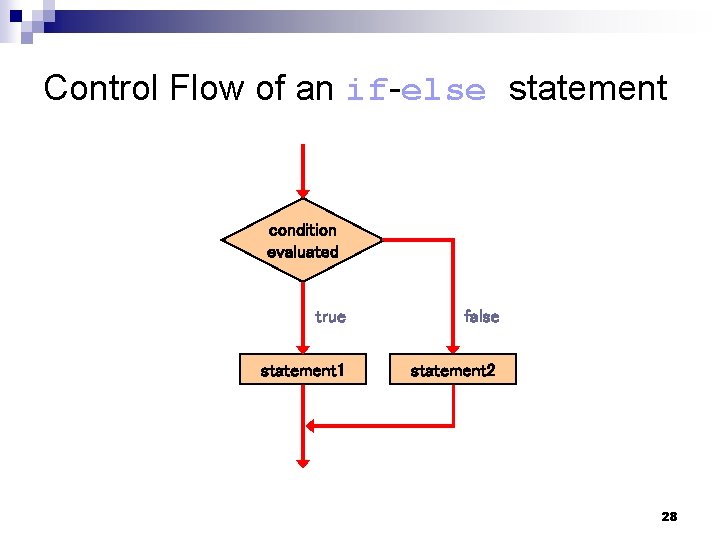
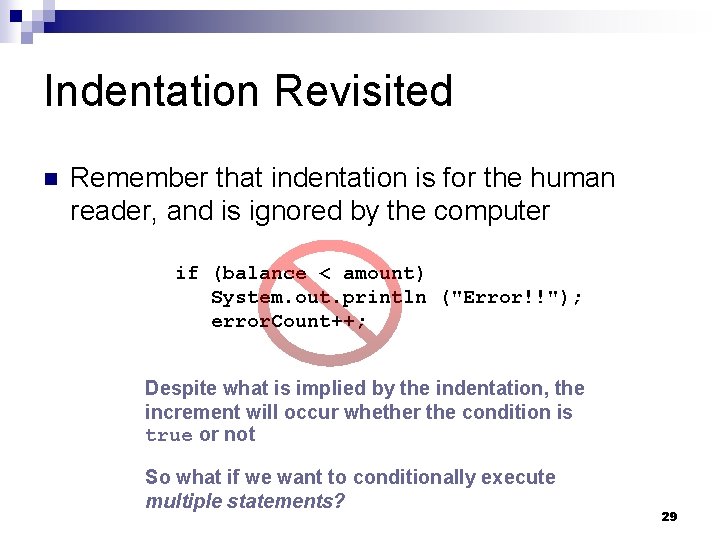
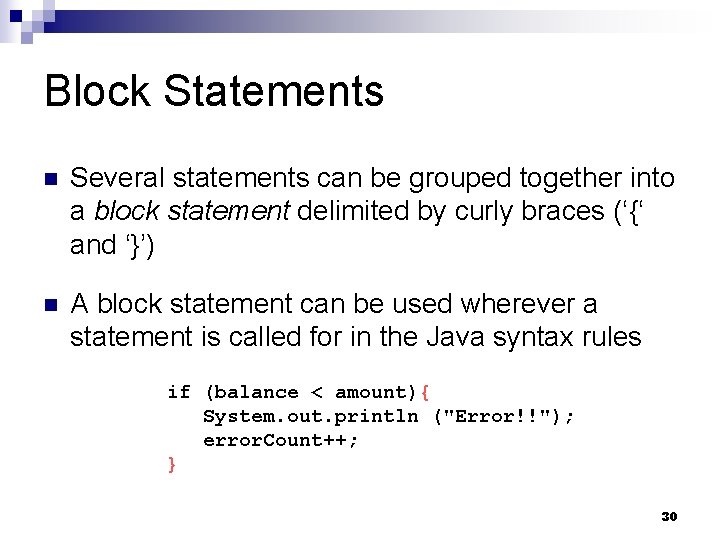
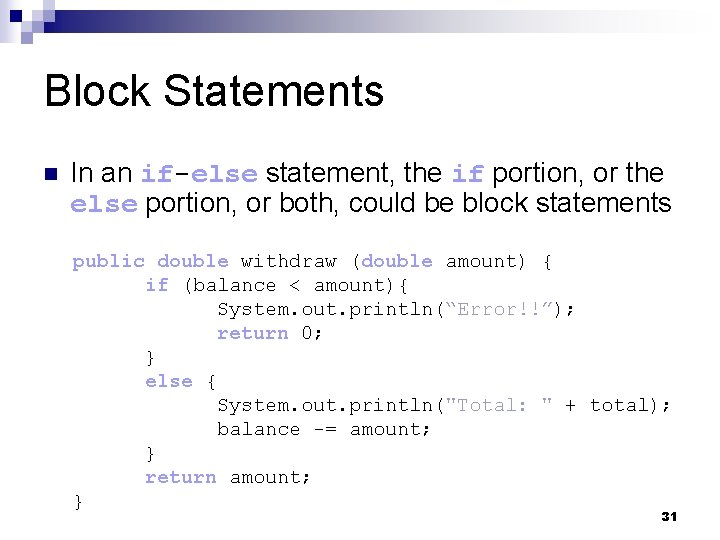
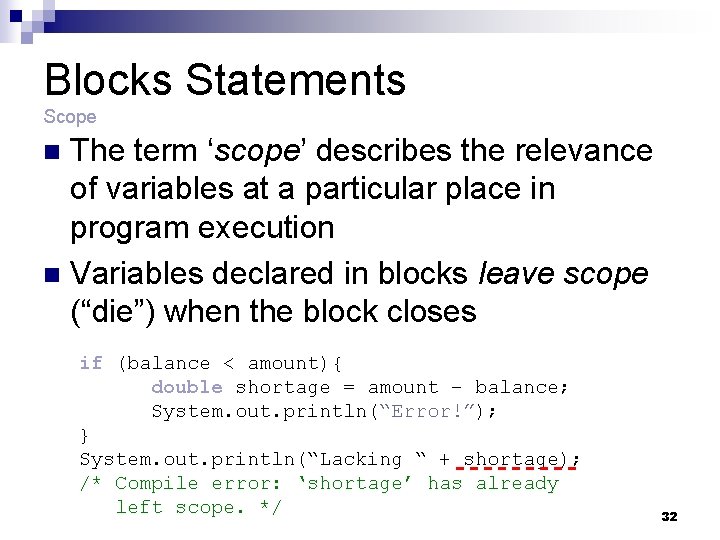
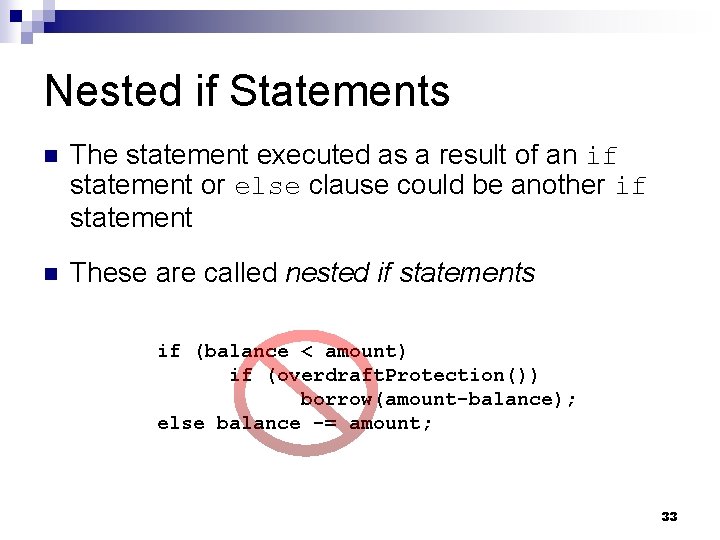
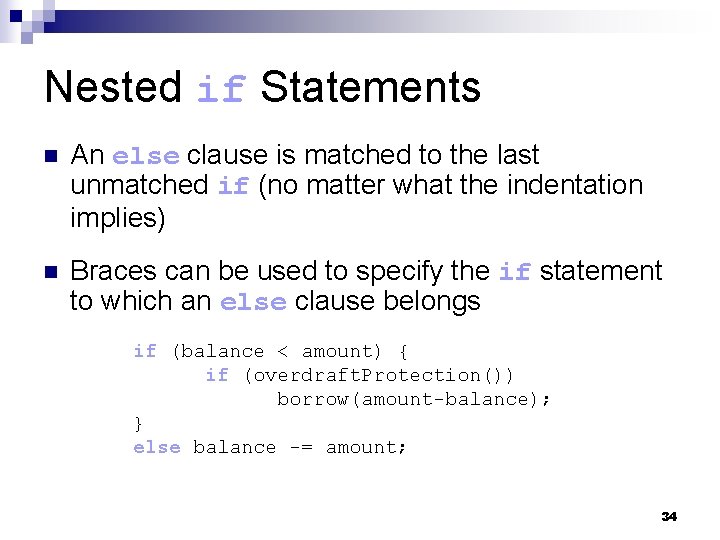
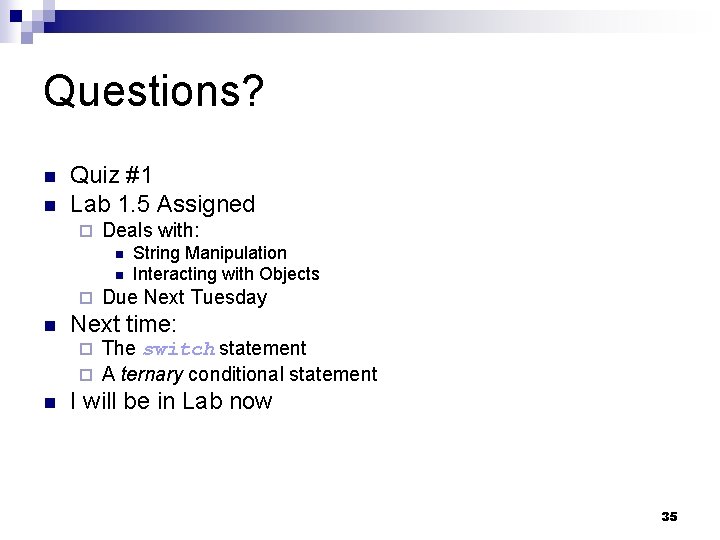
- Slides: 35
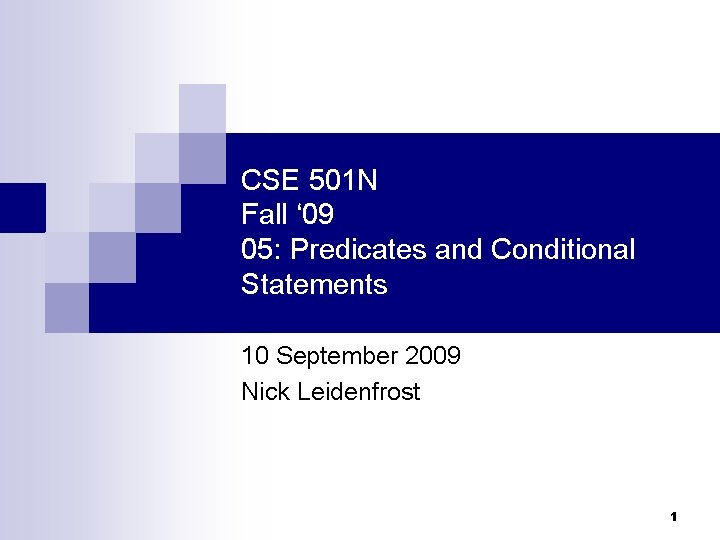
CSE 501 N Fall ‘ 09 05: Predicates and Conditional Statements 10 September 2009 Nick Leidenfrost 1
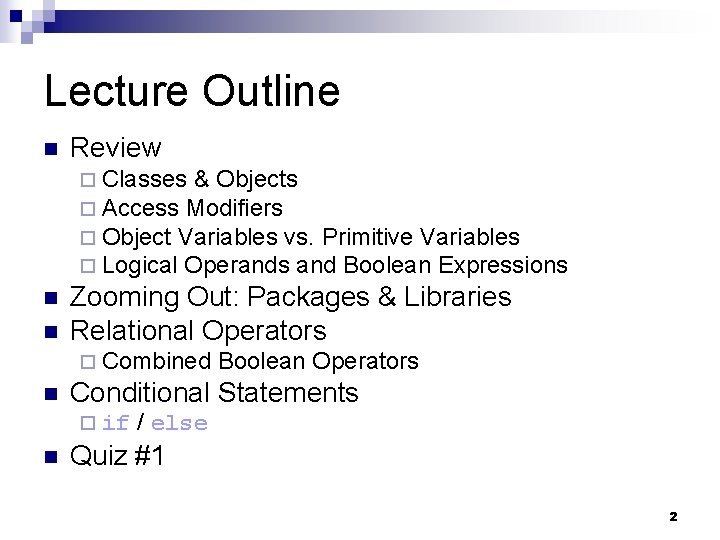
Lecture Outline n Review ¨ Classes & Objects ¨ Access Modifiers ¨ Object Variables vs. Primitive Variables ¨ Logical Operands and Boolean Expressions n n Zooming Out: Packages & Libraries Relational Operators ¨ Combined n Conditional Statements ¨ if n Boolean Operators / else Quiz #1 2
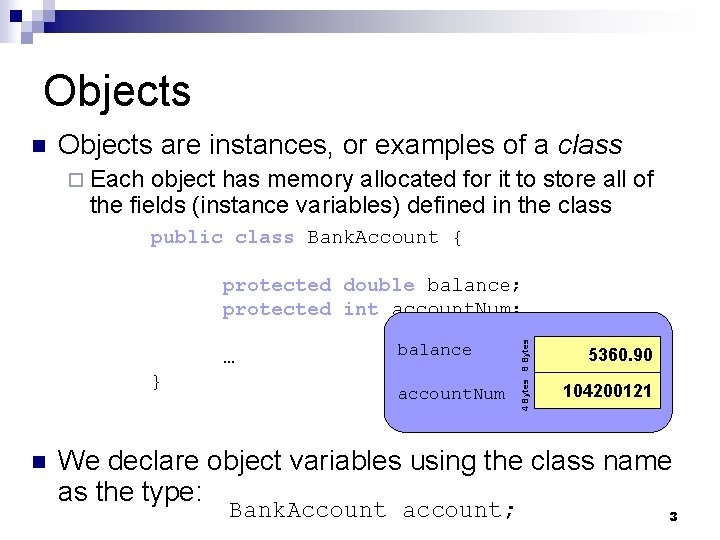
Objects n Objects are instances, or examples of a class ¨ Each object has memory allocated for it to store all of the fields (instance variables) defined in the class public class Bank. Account { … } n balance account. Num 4 Bytes 8 Bytes protected double balance; protected int account. Num; 5360. 90 104200121 We declare object variables using the class name as the type: Bank. Account account; 3
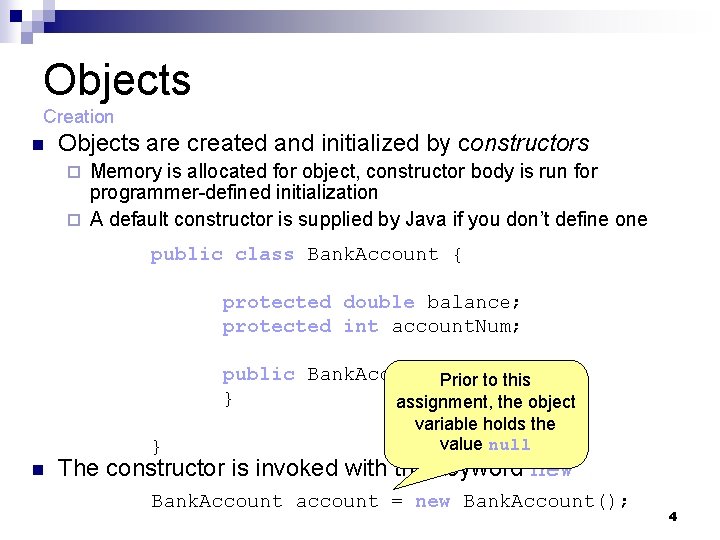
Objects Creation n Objects are created and initialized by constructors Memory is allocated for object, constructor body is run for programmer-defined initialization ¨ A default constructor is supplied by Java if you don’t define one ¨ public class Bank. Account { protected double balance; protected int account. Num; public Bank. Account Prior () to { this } assignment, the object } n variable holds the value null The constructor is invoked with the keyword new Bank. Account account = new Bank. Account(); 4
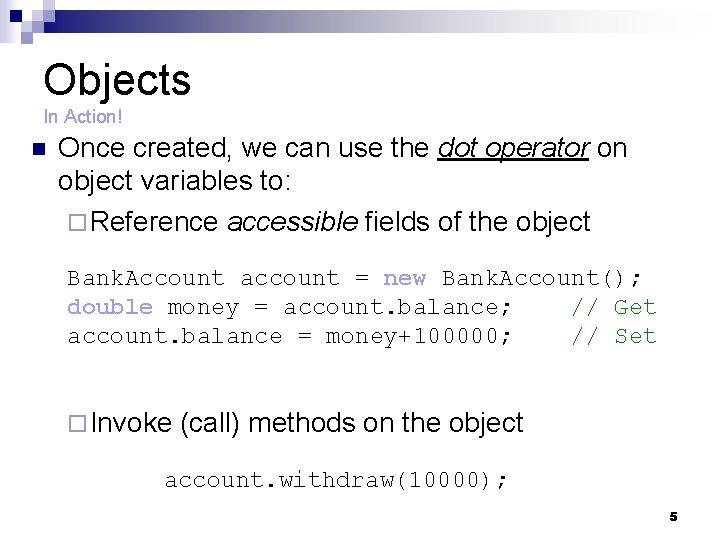
Objects In Action! n Once created, we can use the dot operator on object variables to: ¨ Reference accessible fields of the object Bank. Account account = new Bank. Account(); double money = account. balance; // Get account. balance = money+100000; // Set ¨ Invoke (call) methods on the object account. withdraw(10000); 5
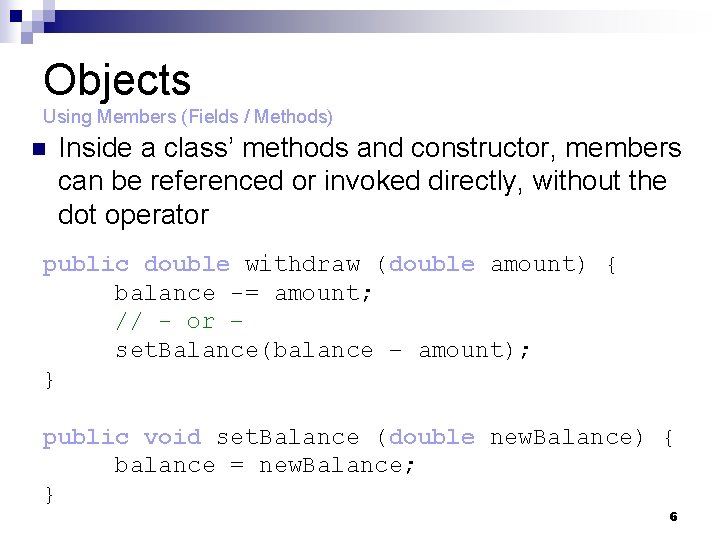
Objects Using Members (Fields / Methods) n Inside a class’ methods and constructor, members can be referenced or invoked directly, without the dot operator public double withdraw (double amount) { balance -= amount; // - or – set. Balance(balance – amount); } public void set. Balance (double new. Balance) { balance = new. Balance; } 6
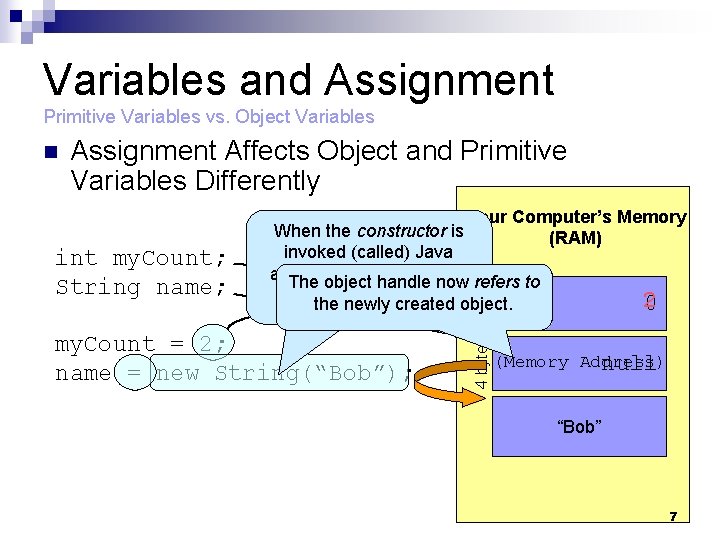
Variables and Assignment Primitive Variables vs. Object Variables Assignment Affects Object and Primitive Variables Differently int my. Count; String name; Your Computer’s Memory When the constructor is (RAM) invoked (called) Java allocates memory fornow the refers to The object handle object. 2 0 the store newly created object. my. Count = 2; name = new String(“Bob”); 4 bytes n (Memory Address) null “Bob” 7
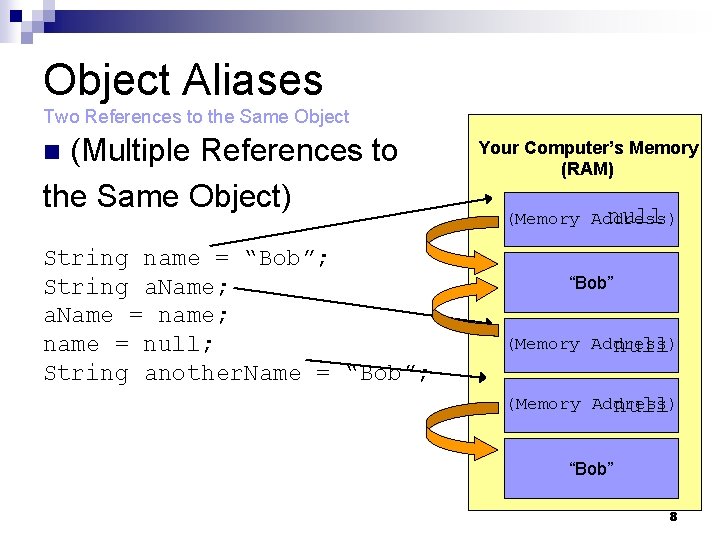
Object Aliases Two References to the Same Object (Multiple References to the Same Object) n String name = “Bob”; String a. Name; a. Name = name; name = null; String another. Name = “Bob”; Your Computer’s Memory (RAM) null (Memory Address) “Bob” (Memory Address) null “Bob” 8
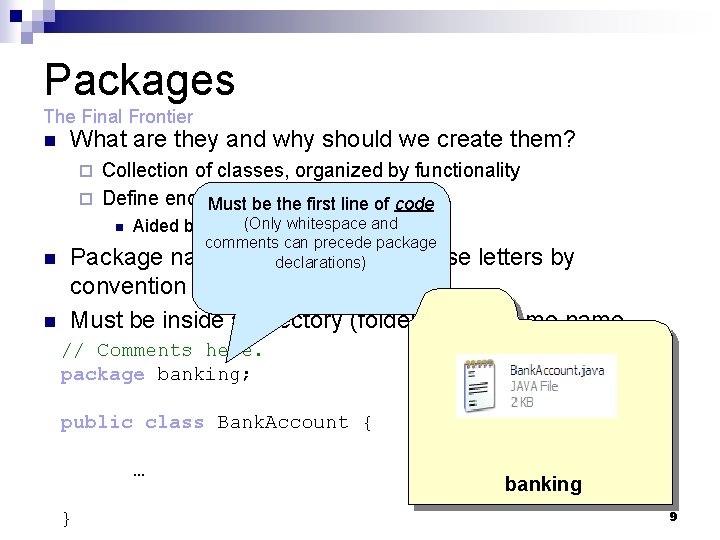
Packages The Final Frontier n What are they and why should we create them? Collection of classes, organized by functionality ¨ Define encapsulation a Class Must be theatfirst line of. Level code ¨ n n n (Only whitespace and Aided by access modifiers comments can precede package names begin with lowercase declarations) Package letters by convention Must be inside a directory (folder) of the same name // Comments here. package banking; public class Bank. Account { … } banking 9
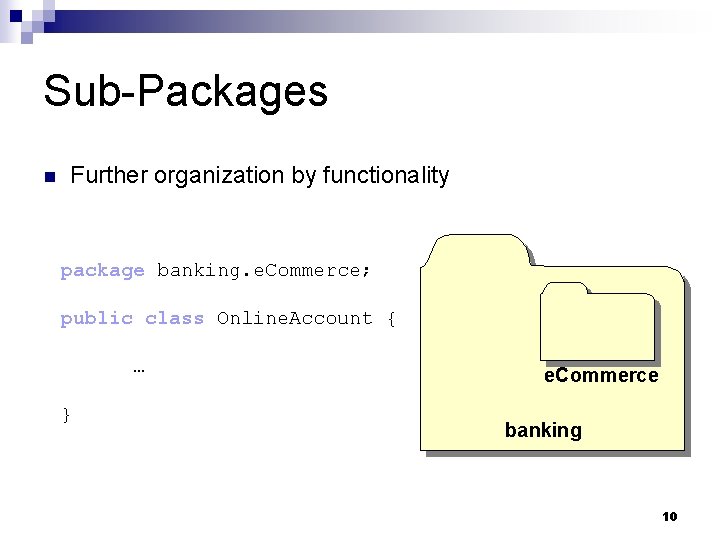
Sub-Packages n Further organization by functionality package banking. e. Commerce; public class Online. Account { … } e. Commerce banking 10
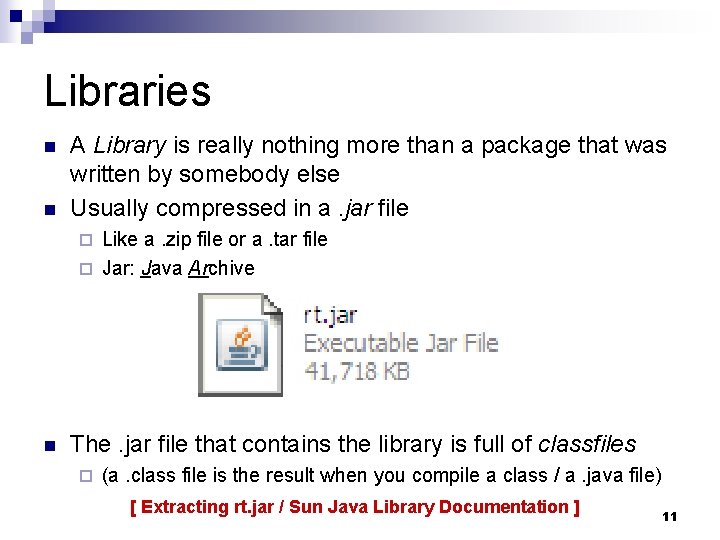
Libraries n n A Library is really nothing more than a package that was written by somebody else Usually compressed in a. jar file Like a. zip file or a. tar file ¨ Jar: Java Archive ¨ n The. jar file that contains the library is full of classfiles ¨ (a. class file is the result when you compile a class / a. java file) [ Extracting rt. jar / Sun Java Library Documentation ] 11
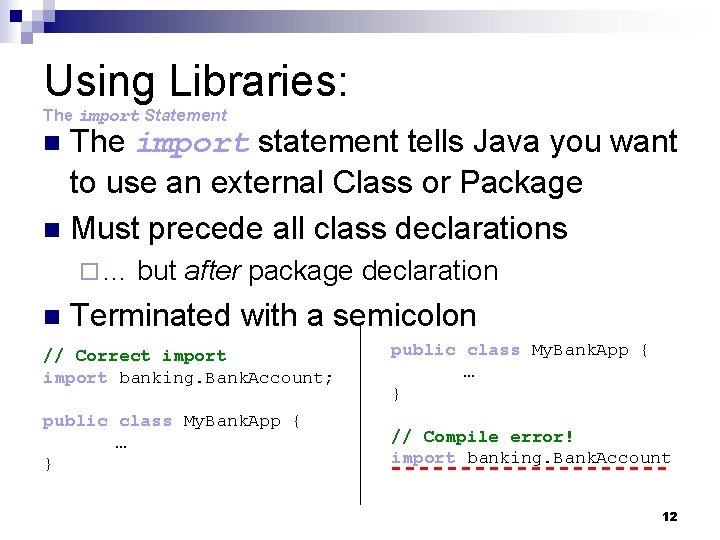
Using Libraries: The import Statement The import statement tells Java you want to use an external Class or Package n Must precede all class declarations n ¨… n but after package declaration Terminated with a semicolon // Correct import banking. Bank. Account; public class My. Bank. App { … } // Compile error! import banking. Bank. Account 12
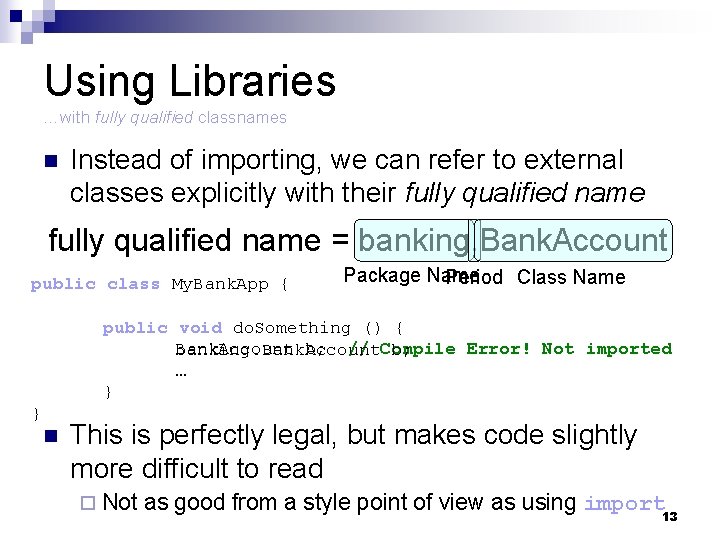
Using Libraries …with fully qualified classnames n Instead of importing, we can refer to external classes explicitly with their fully qualified name = banking. Bank. Account public class My. Bank. App { Package Name Period Class Name public void do. Something () { Bank. Account b; // Compile Error! Not imported banking. Bank. Account b; … } } n This is perfectly legal, but makes code slightly more difficult to read ¨ Not as good from a style point of view as using import 13
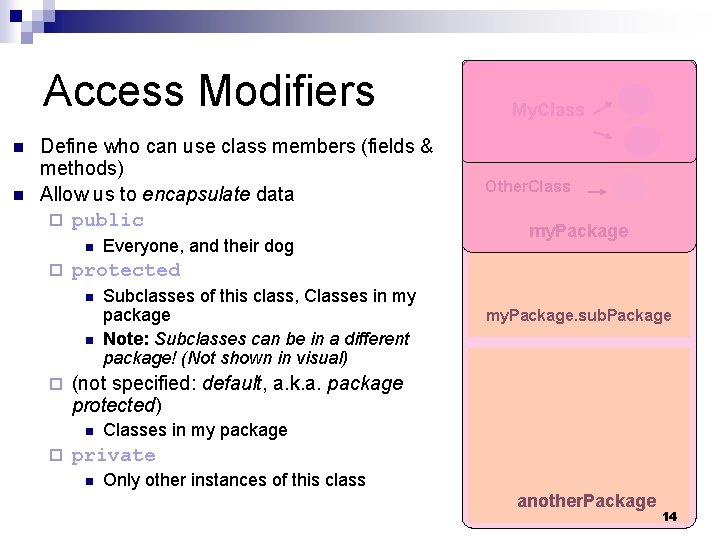
Access Modifiers n n Define who can use class members (fields & methods) Allow us to encapsulate data ¨ public n ¨ n my. Package Subclasses of this class, Classes in my package Note: Subclasses can be in a different package! (Not shown in visual) my. Package. sub. Package (not specified: default, a. k. a. package protected) n ¨ Other. Class protected n ¨ Everyone, and their dog My. Classes in my package private n Only other instances of this class another. Package 14
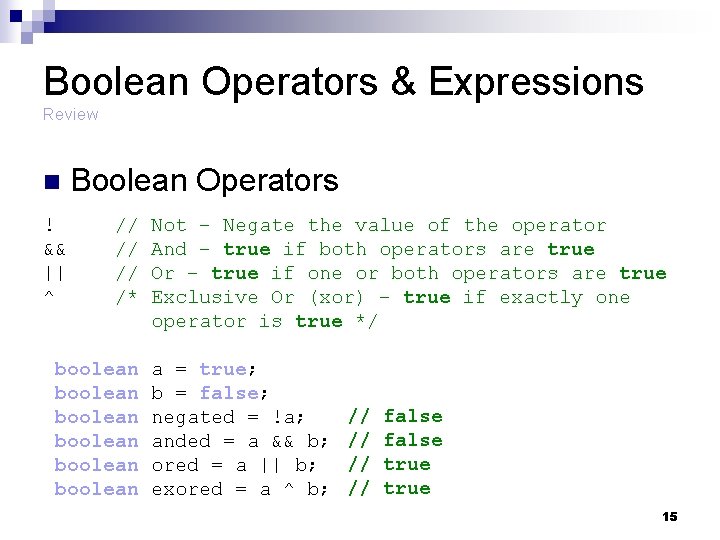
Boolean Operators & Expressions Review n ! && || ^ Boolean Operators // // // /* boolean boolean Not – Negate the value of the operator And – true if both operators are true Or – true if one or both operators are true Exclusive Or (xor) – true if exactly one operator is true */ a = true; b = false; negated = !a; anded = a && b; ored = a || b; exored = a ^ b; // // false true 15
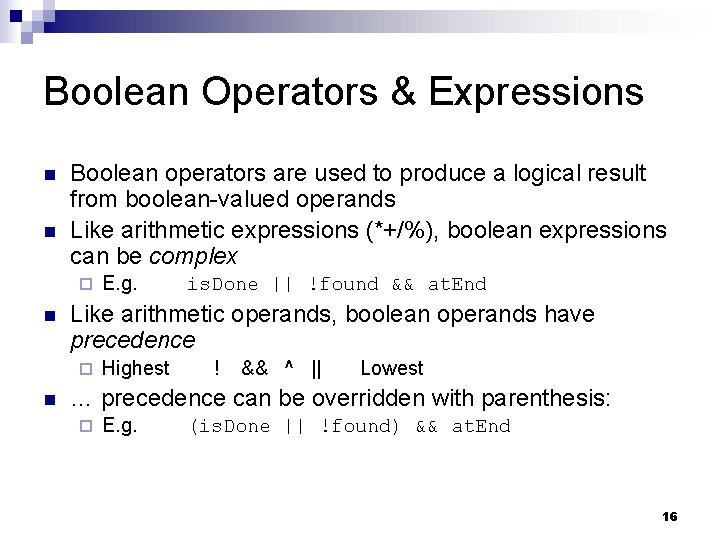
Boolean Operators & Expressions n n Boolean operators are used to produce a logical result from boolean-valued operands Like arithmetic expressions (*+/%), boolean expressions can be complex ¨ n is. Done || !found && at. End Like arithmetic operands, boolean operands have precedence ¨ n E. g. Highest ! && ^ || Lowest … precedence can be overridden with parenthesis: ¨ E. g. (is. Done || !found) && at. End 16
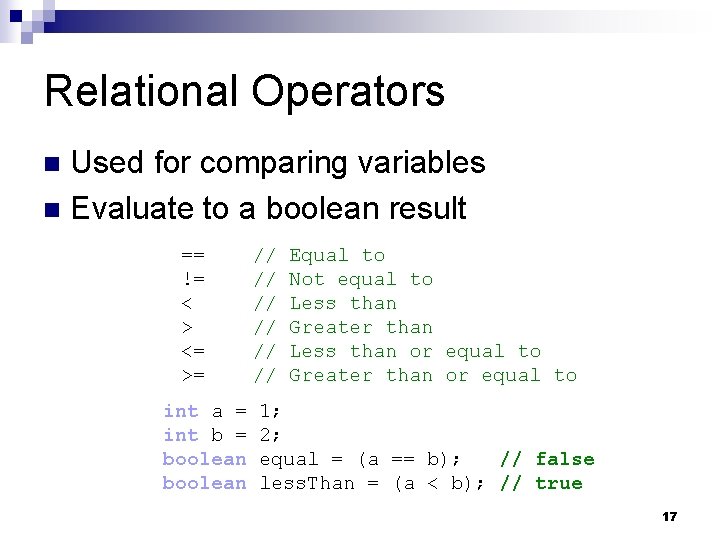
Relational Operators Used for comparing variables n Evaluate to a boolean result n == != < > <= >= int a = int b = boolean // // // Equal to Not equal to Less than Greater than Less than or equal to Greater than or equal to 1; 2; equal = (a == b); // false less. Than = (a < b); // true 17
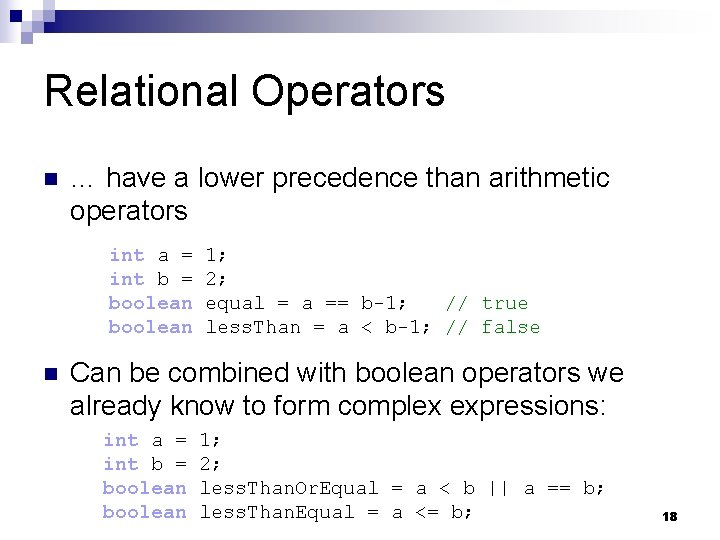
Relational Operators n … have a lower precedence than arithmetic operators int a = int b = boolean n 1; 2; equal = a == b-1; // true less. Than = a < b-1; // false Can be combined with boolean operators we already know to form complex expressions: int a = int b = boolean 1; 2; less. Than. Or. Equal = a < b || a == b; less. Than. Equal = a <= b; 18
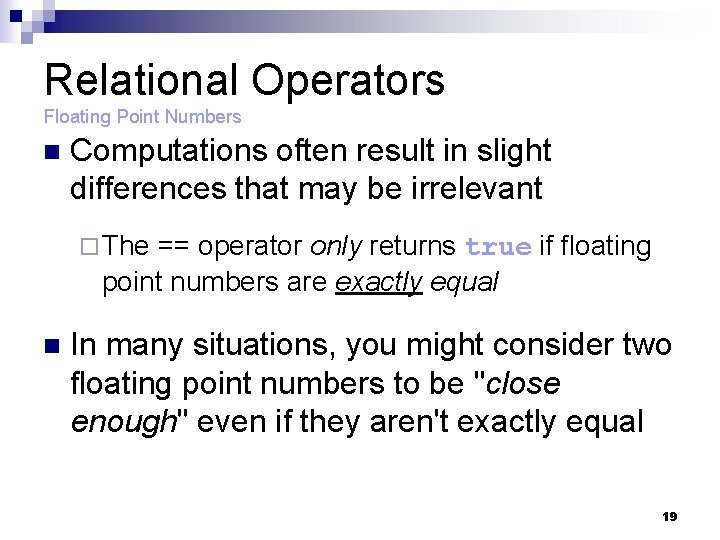
Relational Operators Floating Point Numbers n Computations often result in slight differences that may be irrelevant ¨ The == operator only returns true if floating point numbers are exactly equal n In many situations, you might consider two floating point numbers to be "close enough" even if they aren't exactly equal 19
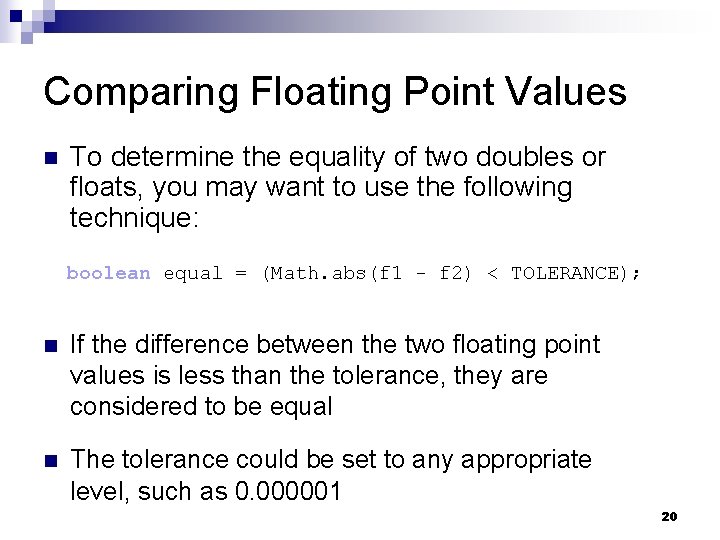
Comparing Floating Point Values n To determine the equality of two doubles or floats, you may want to use the following technique: boolean equal = (Math. abs(f 1 - f 2) < TOLERANCE); n If the difference between the two floating point values is less than the tolerance, they are considered to be equal n The tolerance could be set to any appropriate level, such as 0. 000001 20
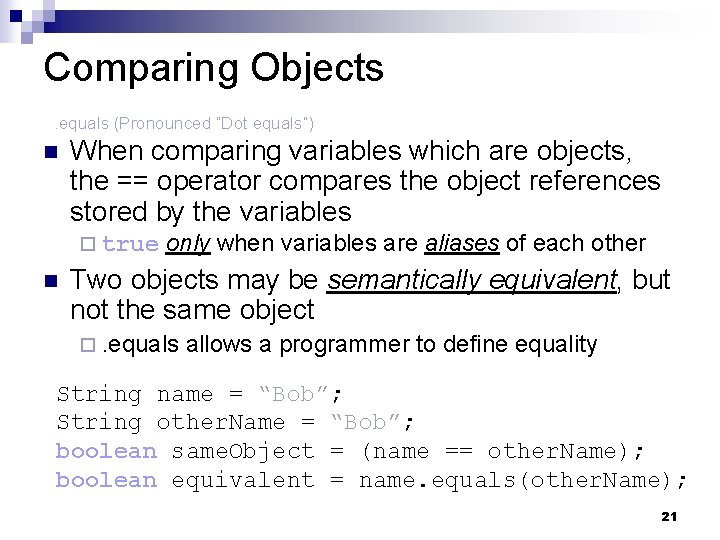
Comparing Objects. equals (Pronounced “Dot equals”) n When comparing variables which are objects, the == operator compares the object references stored by the variables ¨ true n only when variables are aliases of each other Two objects may be semantically equivalent, but not the same object ¨. equals allows a programmer to define equality String name = “Bob”; String other. Name = “Bob”; boolean same. Object = (name == other. Name); boolean equivalent = name. equals(other. Name); 21
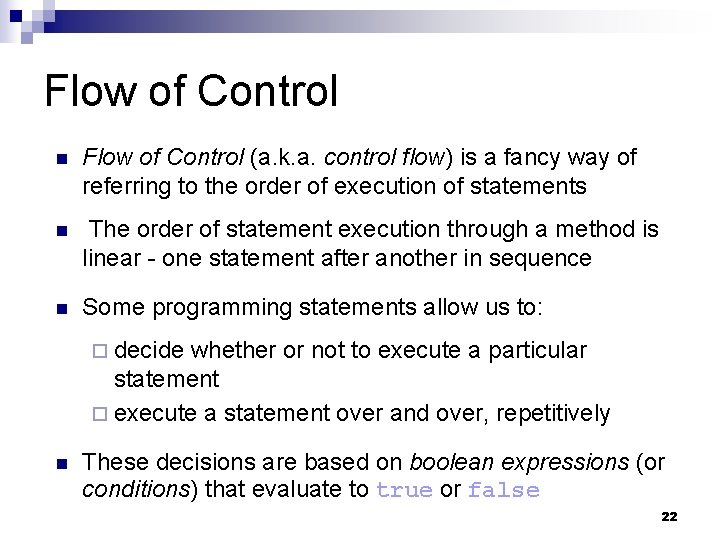
Flow of Control n Flow of Control (a. k. a. control flow) is a fancy way of referring to the order of execution of statements n The order of statement execution through a method is linear - one statement after another in sequence n Some programming statements allow us to: ¨ decide whether or not to execute a particular statement ¨ execute a statement over and over, repetitively n These decisions are based on boolean expressions (or conditions) that evaluate to true or false 22
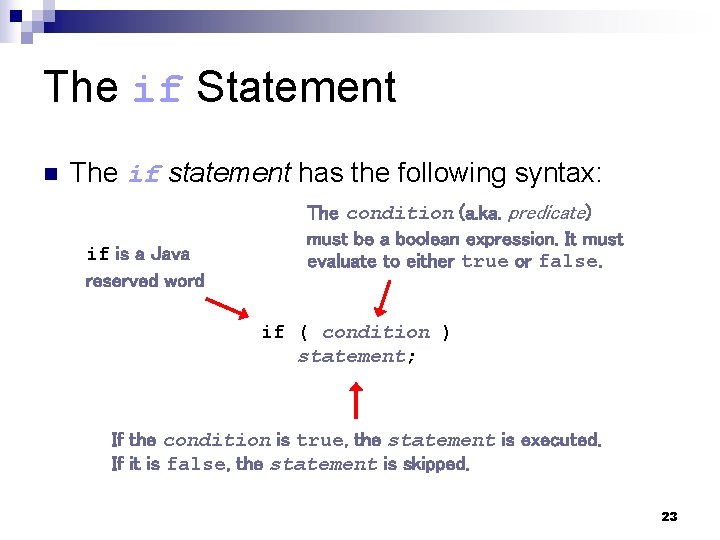
The if Statement n The if statement has the following syntax: if is a Java reserved word The condition (a. ka. predicate) must be a boolean expression. It must evaluate to either true or false. if ( condition ) statement; If the condition is true, the statement is executed. If it is false, the statement is skipped. 23
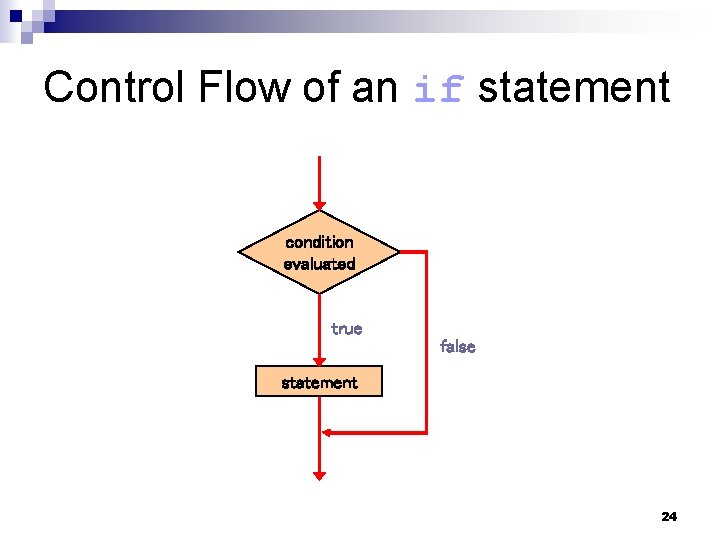
Control Flow of an if statement condition evaluated true false statement 24
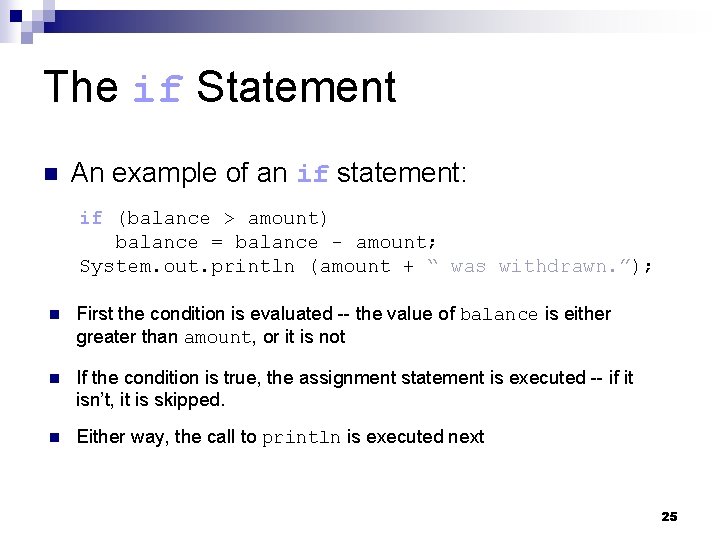
The if Statement n An example of an if statement: if (balance > amount) balance = balance - amount; System. out. println (amount + “ was withdrawn. ”); n First the condition is evaluated -- the value of balance is either greater than amount, or it is not n If the condition is true, the assignment statement is executed -- if it isn’t, it is skipped. n Either way, the call to println is executed next 25
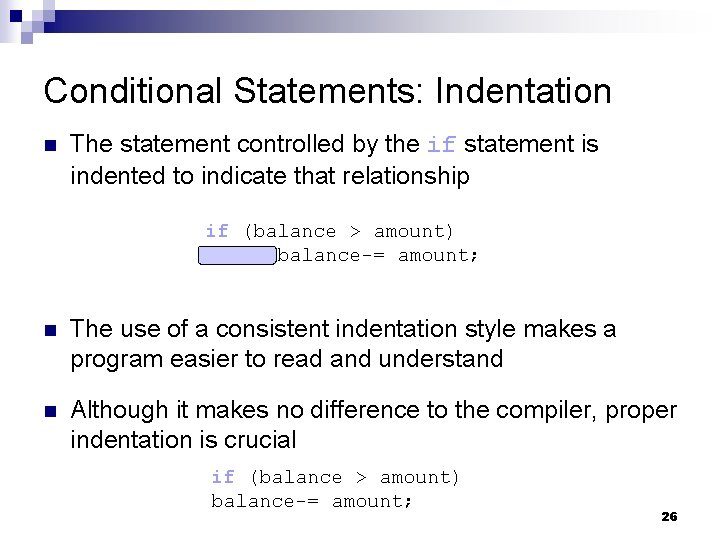
Conditional Statements: Indentation n The statement controlled by the if statement is indented to indicate that relationship if (balance > amount) balance-= amount; n The use of a consistent indentation style makes a program easier to read and understand n Although it makes no difference to the compiler, proper indentation is crucial if (balance > amount) balance-= amount; 26
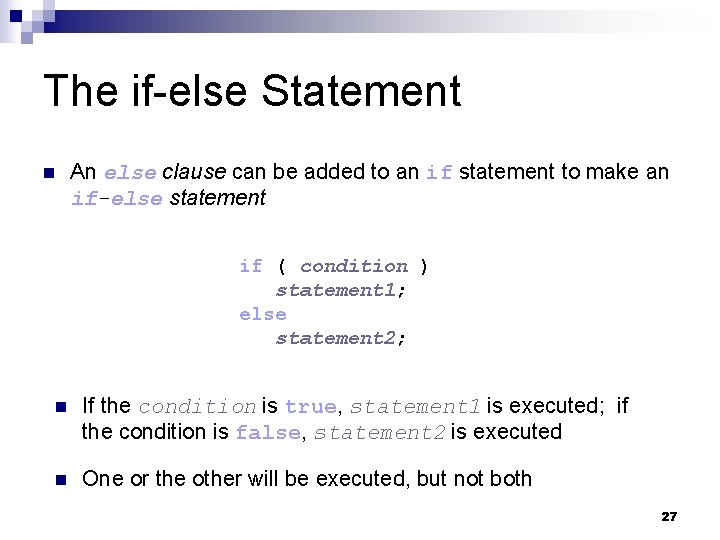
The if-else Statement n An else clause can be added to an if statement to make an if-else statement if ( condition ) statement 1; else statement 2; n If the condition is true, statement 1 is executed; if the condition is false, statement 2 is executed n One or the other will be executed, but not both 27
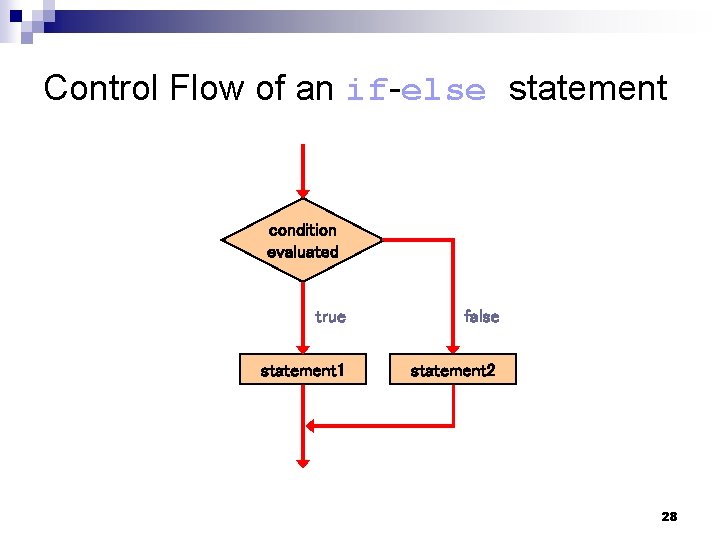
Control Flow of an if-else statement condition evaluated true false statement 1 statement 2 28
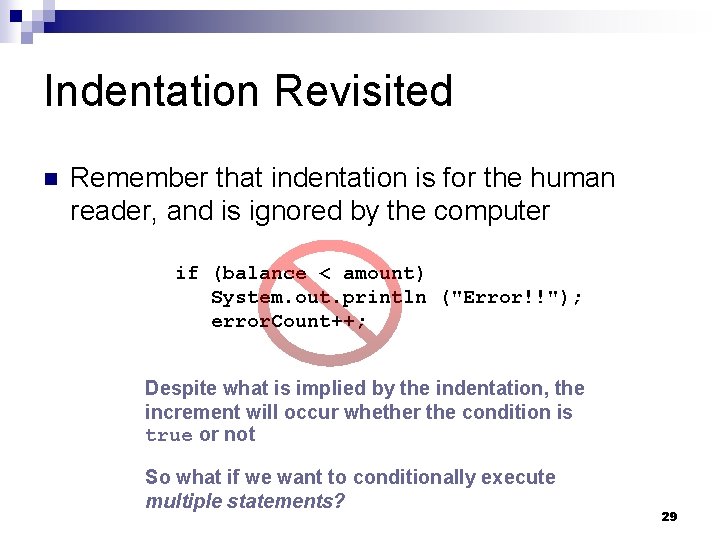
Indentation Revisited n Remember that indentation is for the human reader, and is ignored by the computer if (balance < amount) System. out. println ("Error!!"); error. Count++; Despite what is implied by the indentation, the increment will occur whether the condition is true or not So what if we want to conditionally execute multiple statements? 29
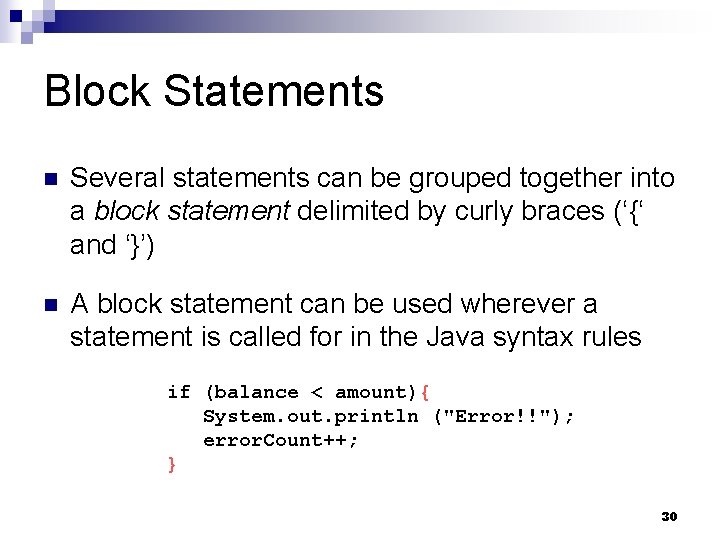
Block Statements n Several statements can be grouped together into a block statement delimited by curly braces (‘{‘ and ‘}’) n A block statement can be used wherever a statement is called for in the Java syntax rules if (balance < amount){ System. out. println ("Error!!"); error. Count++; } 30
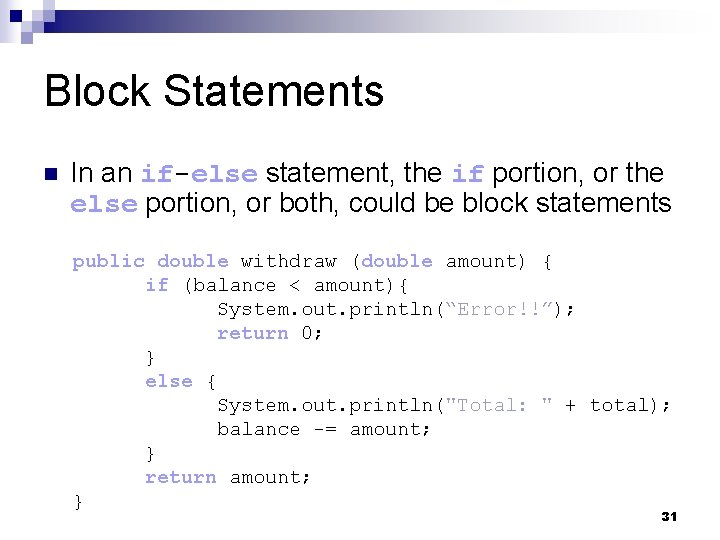
Block Statements n In an if-else statement, the if portion, or the else portion, or both, could be block statements public double withdraw (double amount) { if (balance < amount){ System. out. println(“Error!!”); return 0; } else { System. out. println("Total: " + total); balance -= amount; } return amount; } 31
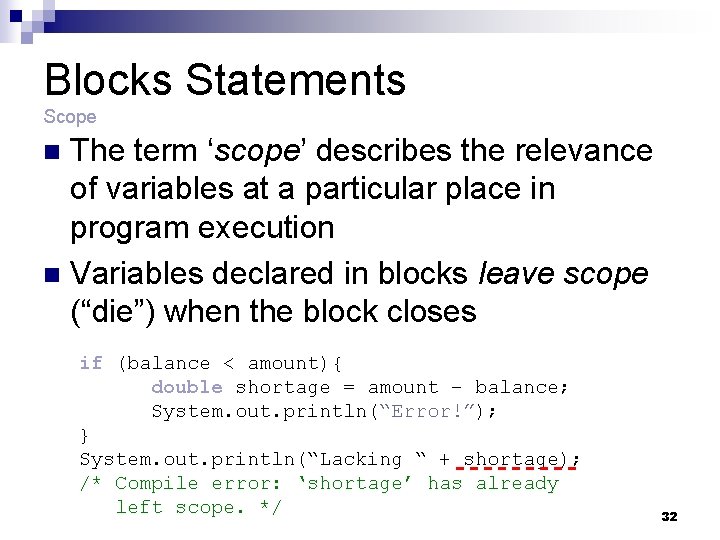
Blocks Statements Scope The term ‘scope’ describes the relevance of variables at a particular place in program execution n Variables declared in blocks leave scope (“die”) when the block closes n if (balance < amount){ double shortage = amount – balance; System. out. println(“Error!”); } System. out. println(“Lacking “ + shortage); /* Compile error: ‘shortage’ has already left scope. */ 32
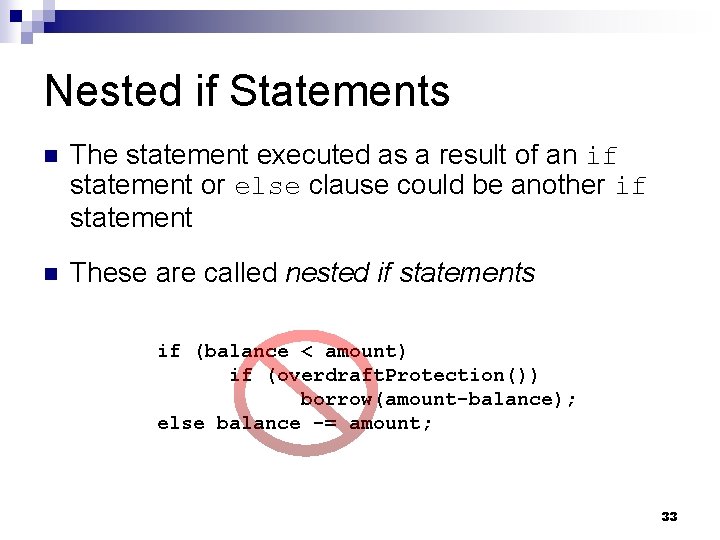
Nested if Statements n The statement executed as a result of an if statement or else clause could be another if statement n These are called nested if statements if (balance < amount) if (overdraft. Protection()) borrow(amount-balance); else balance -= amount; 33
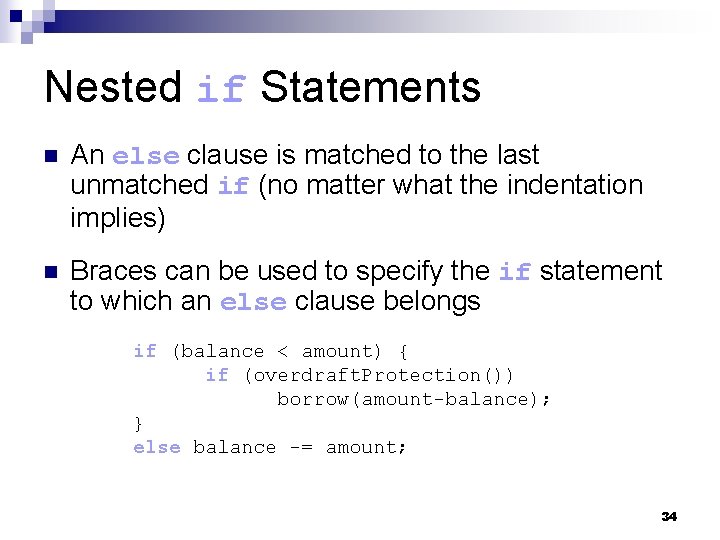
Nested if Statements n An else clause is matched to the last unmatched if (no matter what the indentation implies) n Braces can be used to specify the if statement to which an else clause belongs if (balance < amount) { if (overdraft. Protection()) borrow(amount-balance); } else balance -= amount; 34
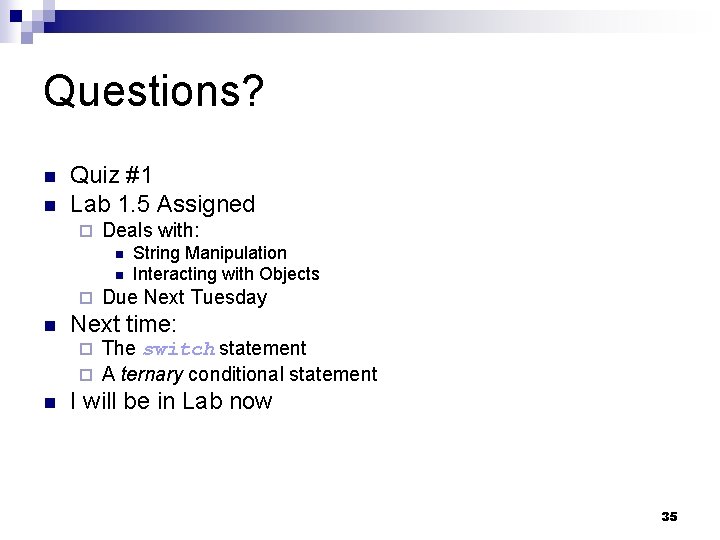
Questions? n n Quiz #1 Lab 1. 5 Assigned ¨ Deals with: n n ¨ n String Manipulation Interacting with Objects Due Next Tuesday Next time: The switch statement ¨ A ternary conditional statement ¨ n I will be in Lab now 35