CSE 1341 Arrays Strings 2013 Ken Howard Southern
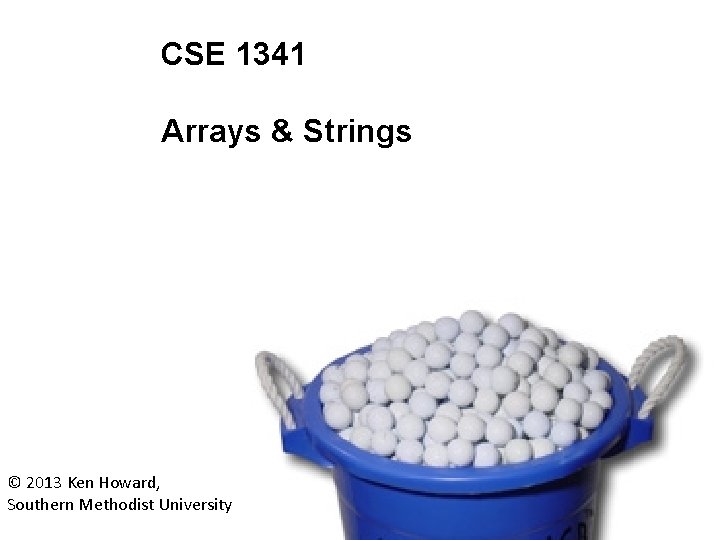
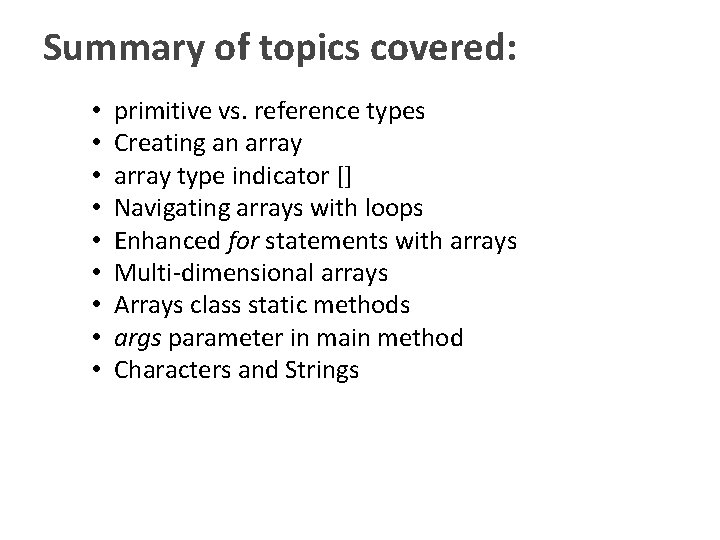
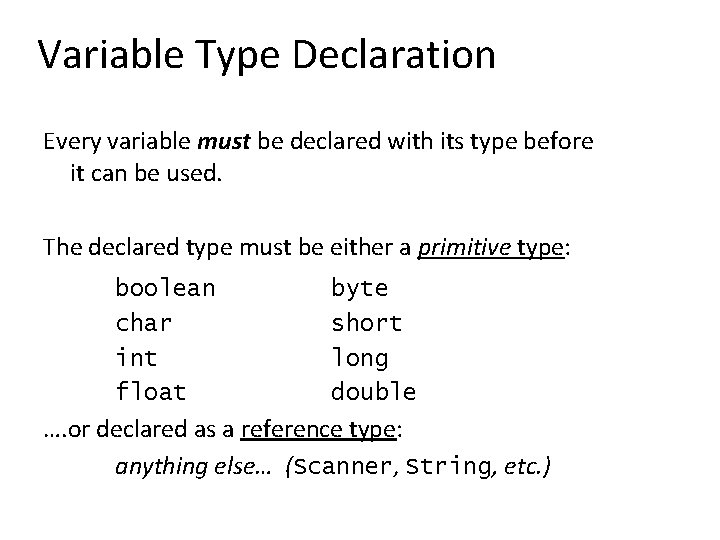
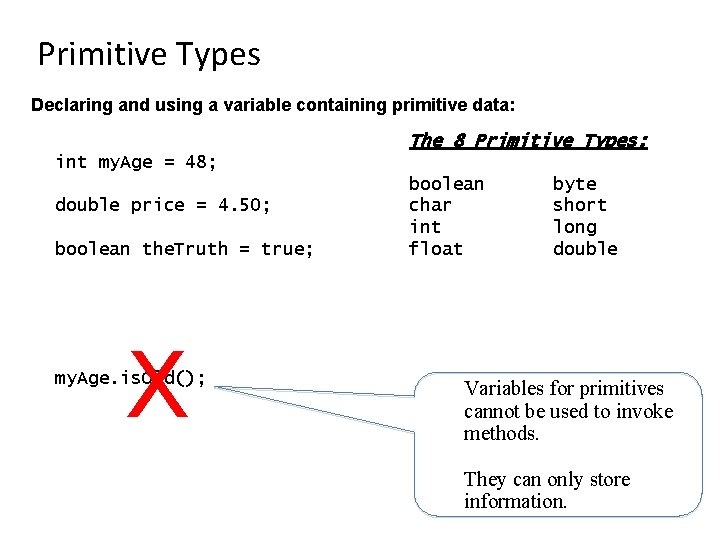
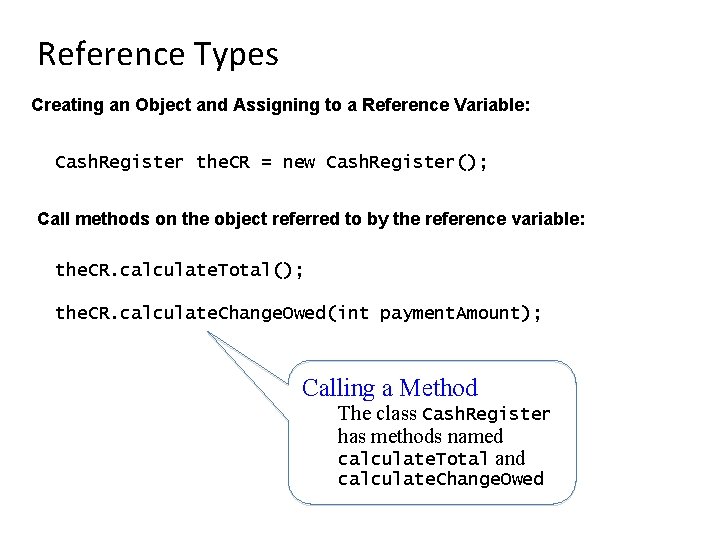
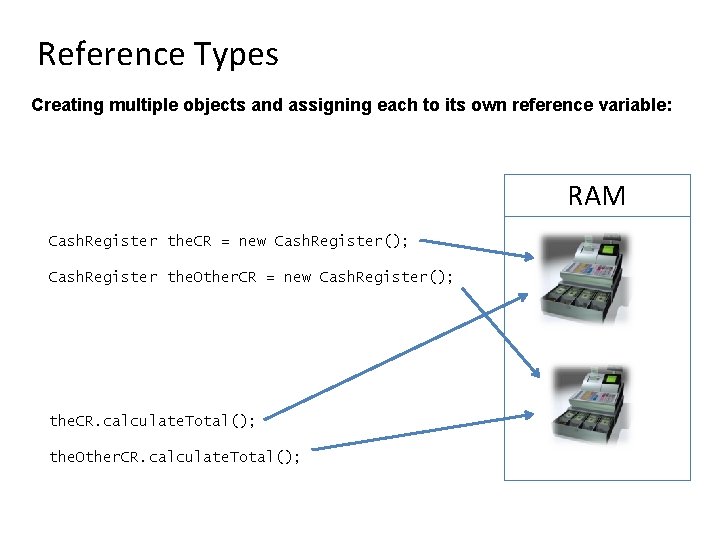
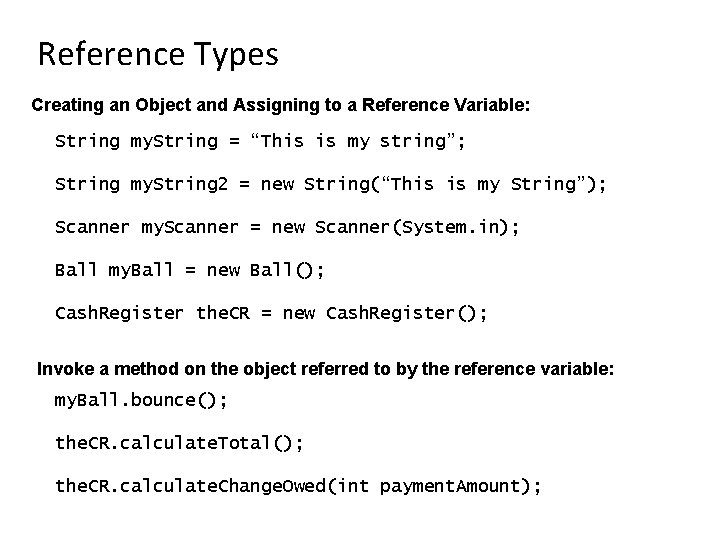
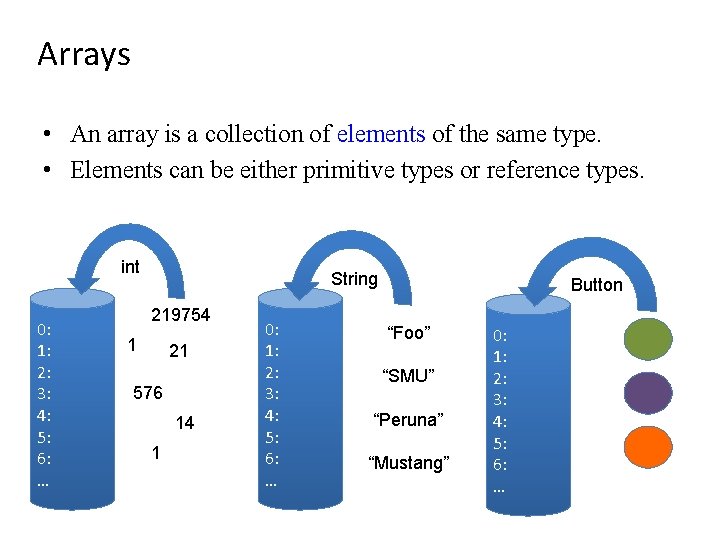
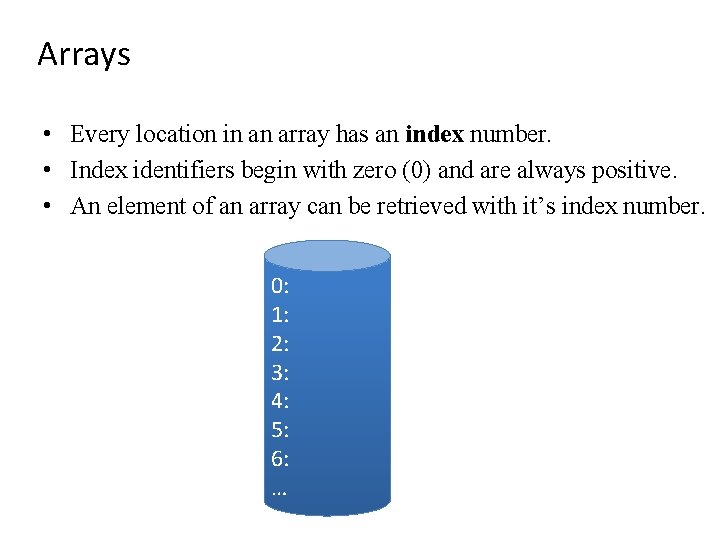
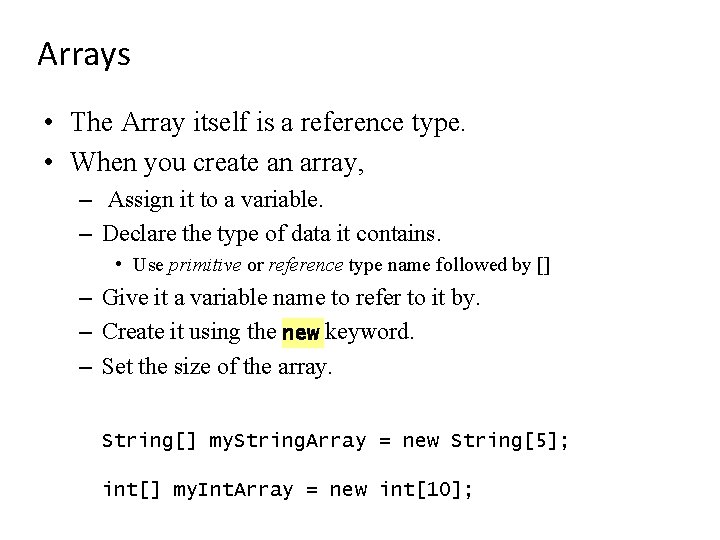
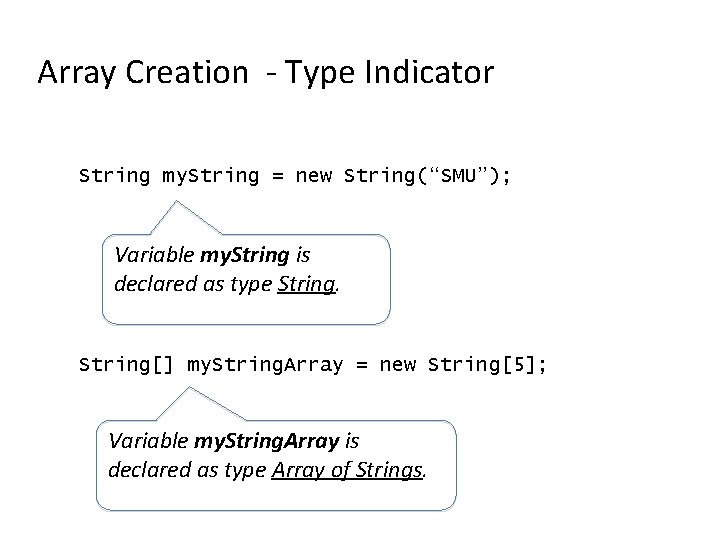
![Arrays my. Int. Array[0] 0 my. Int. Array[1] 0 my. Int. Array[2] 0 my. Arrays my. Int. Array[0] 0 my. Int. Array[1] 0 my. Int. Array[2] 0 my.](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-12.jpg)
![Arrays my. Int. Array[2] = 12; my. Int. Array[8] = 695; my. Int. Array[0] Arrays my. Int. Array[2] = 12; my. Int. Array[8] = 695; my. Int. Array[0]](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-13.jpg)
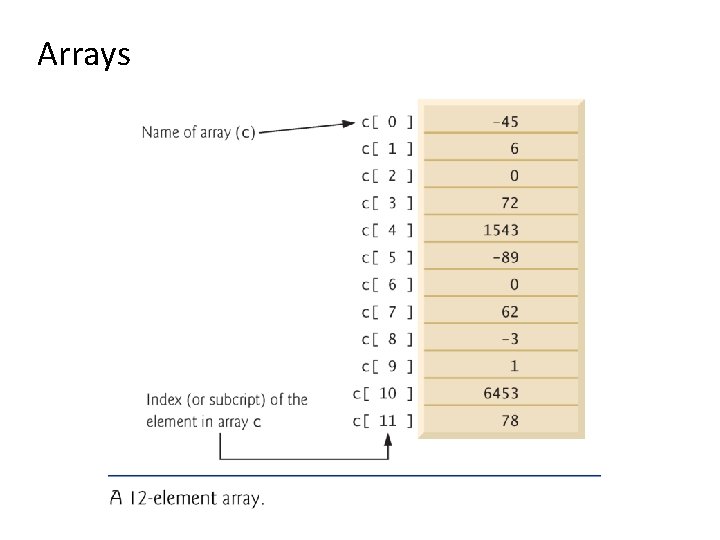
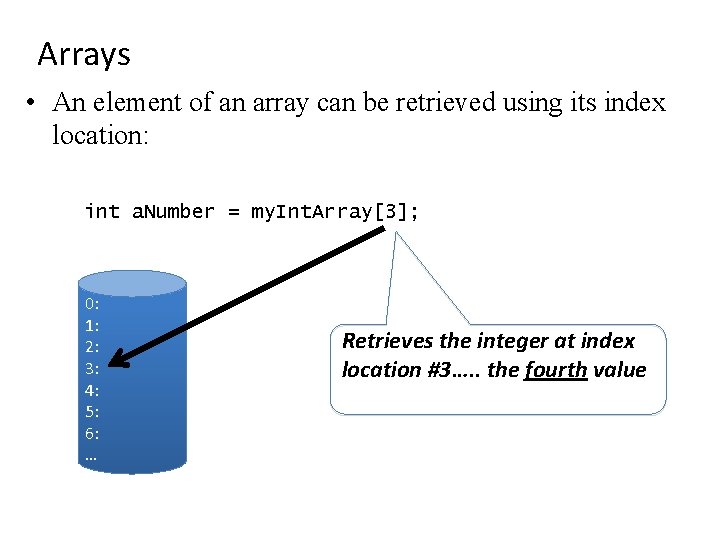
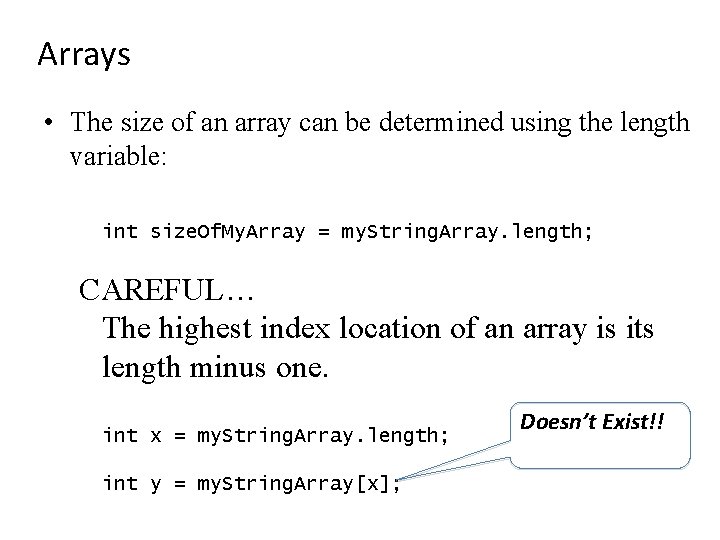
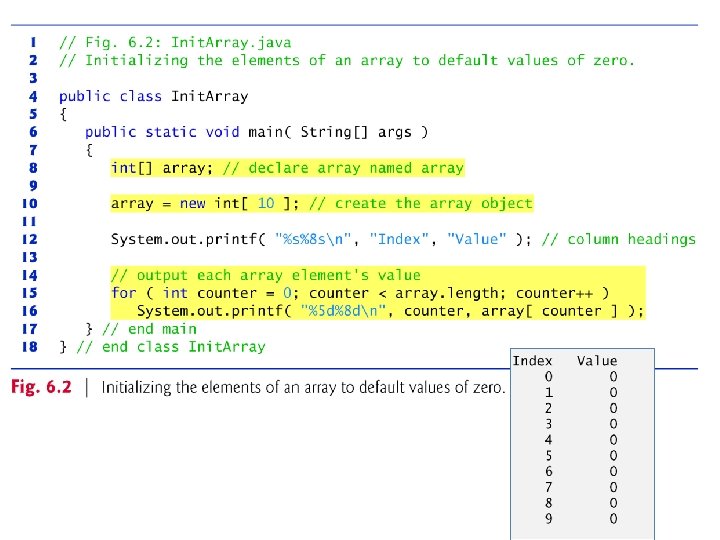
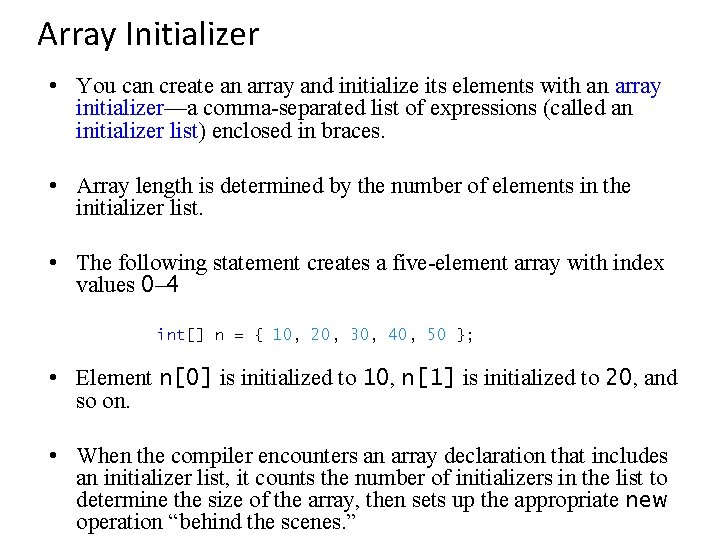
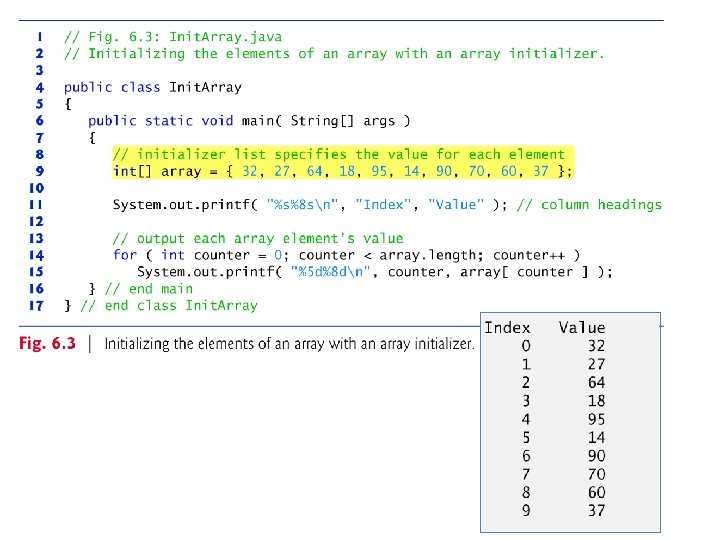
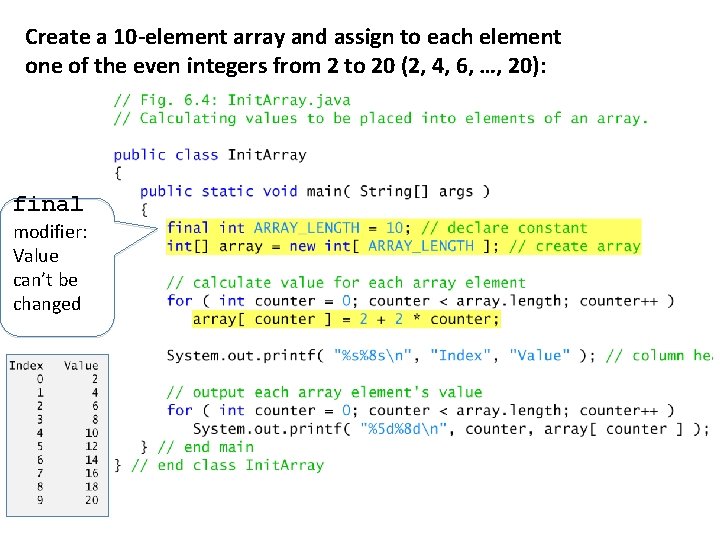
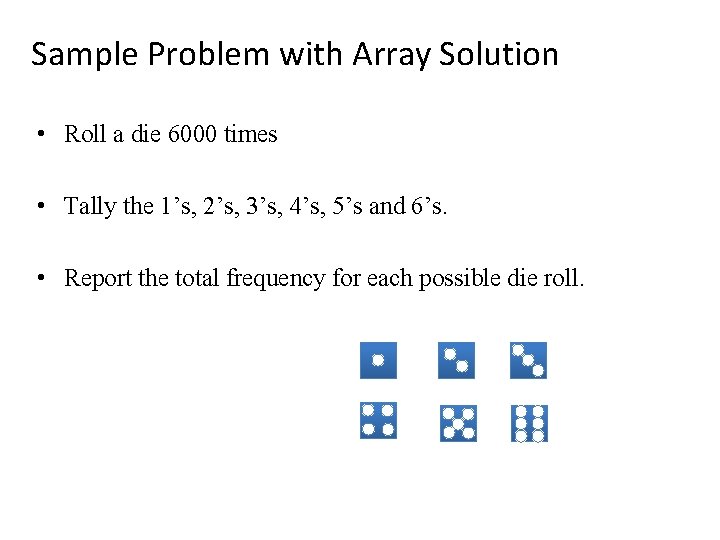
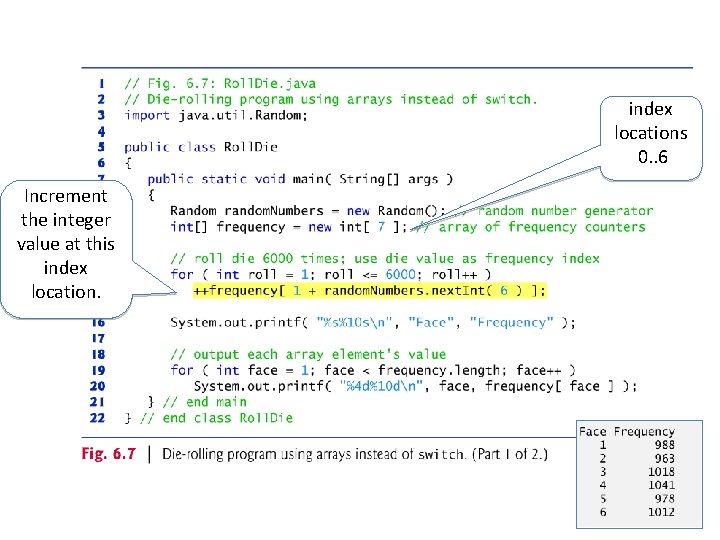
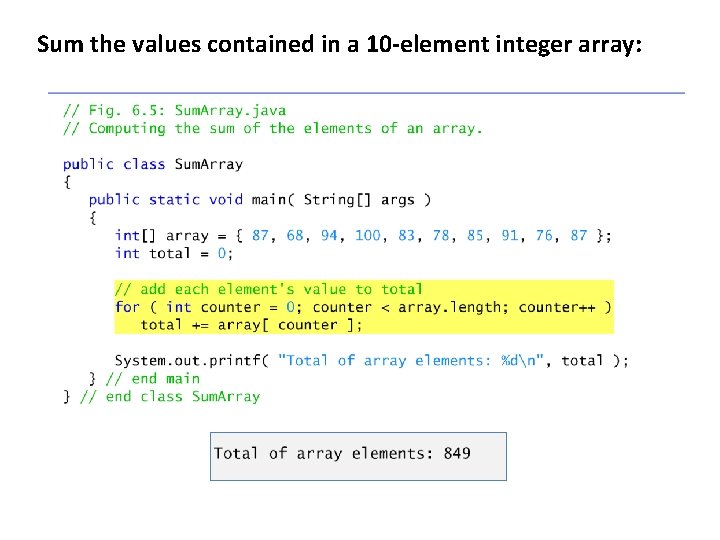
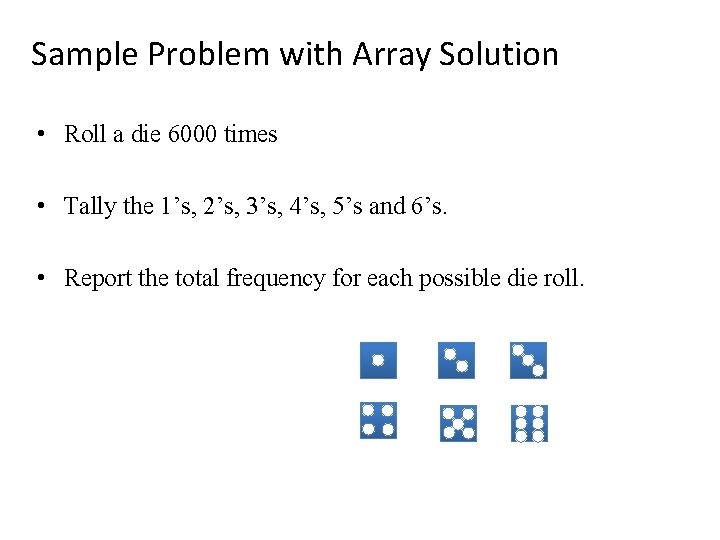
![int x = my. String. Array. length; int y = my. String. Array[x]; Will int x = my. String. Array. length; int y = my. String. Array[x]; Will](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-25.jpg)
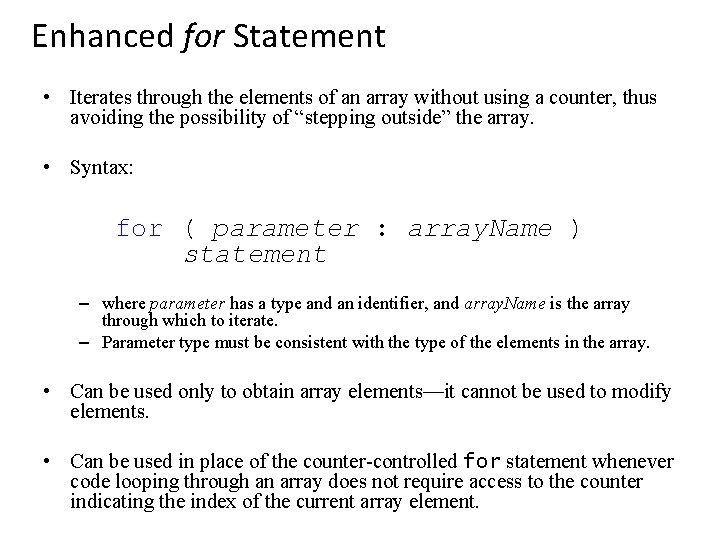
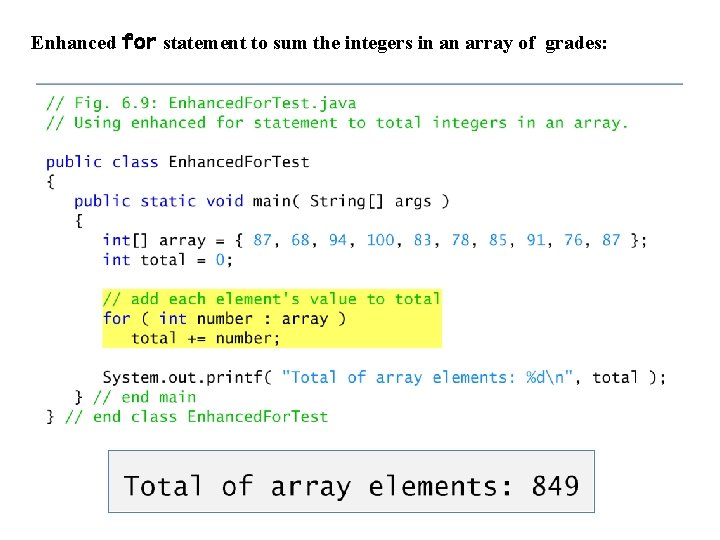
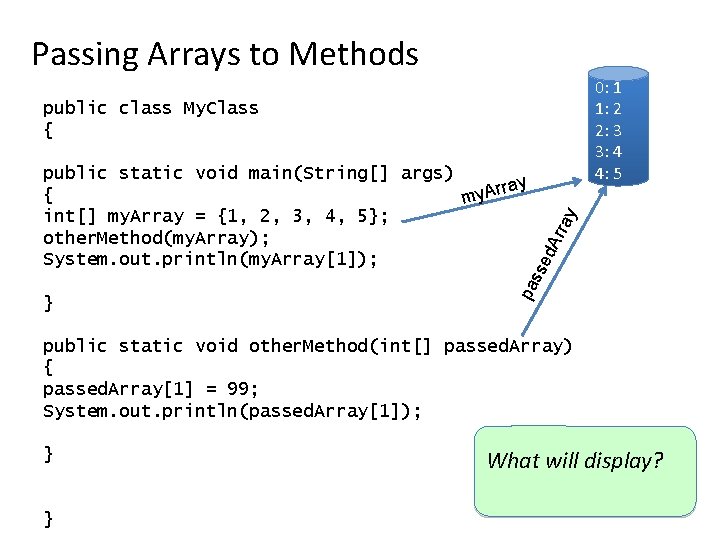
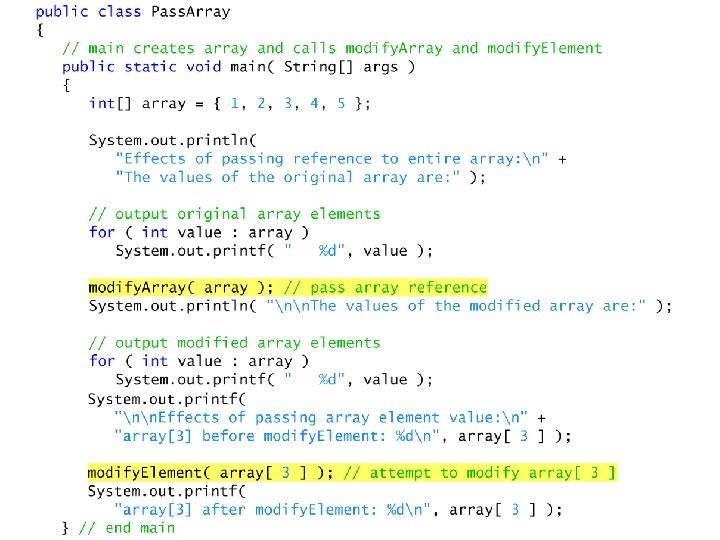
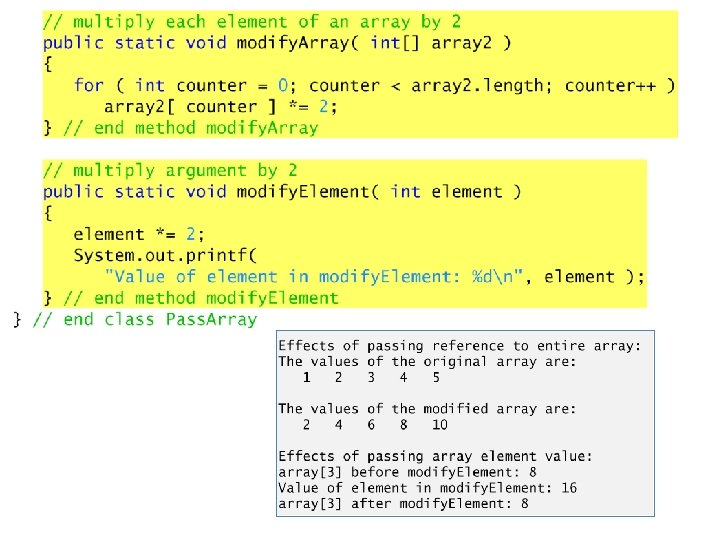
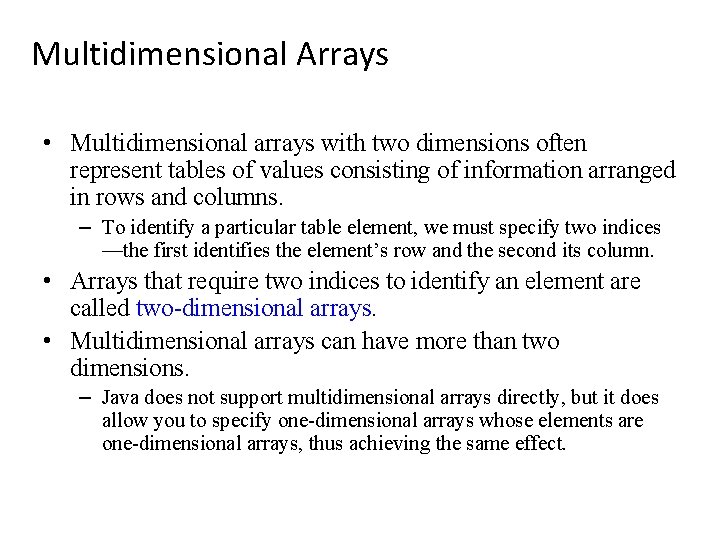
![Two Dimensional Arrays String[][] my. Array = new String[4][3]; my. Array[0][1] = “Foo” 0 Two Dimensional Arrays String[][] my. Array = new String[4][3]; my. Array[0][1] = “Foo” 0](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-32.jpg)
![Two Dimensional Arrays public class Practice { public static void main(String[] args) { String[][] Two Dimensional Arrays public class Practice { public static void main(String[] args) { String[][]](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-33.jpg)
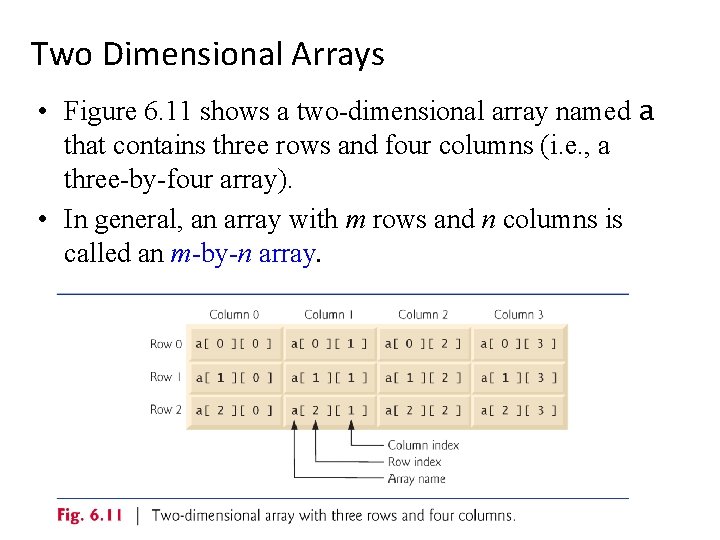
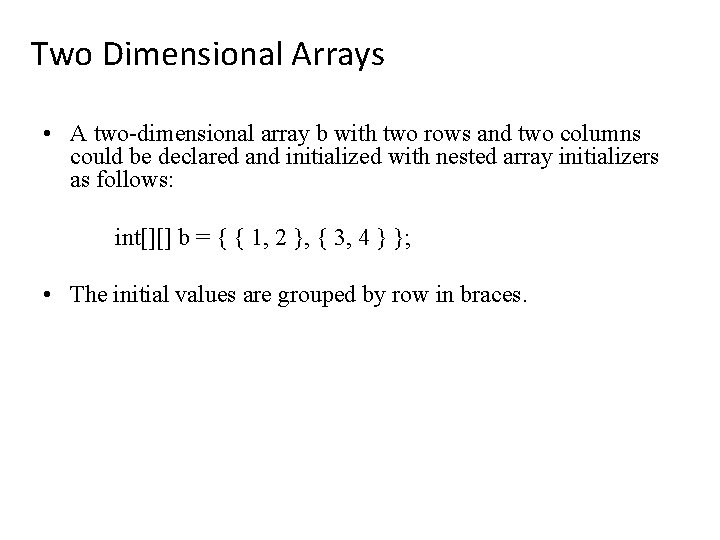
![Array with Different Row Lengths String[][] my. Array = new String[4][]; my. Array[0] = Array with Different Row Lengths String[][] my. Array = new String[4][]; my. Array[0] =](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-36.jpg)
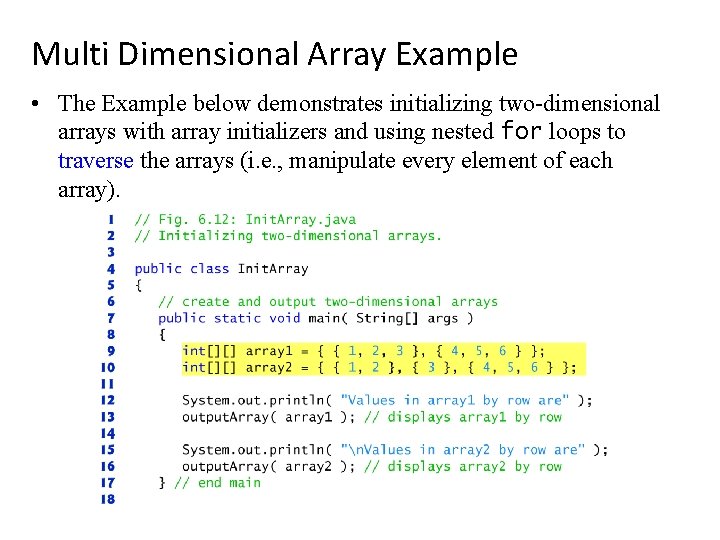
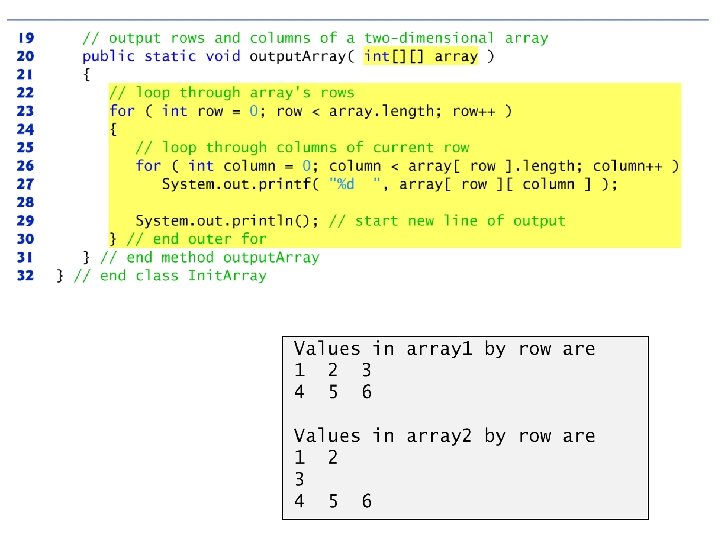
![Passing Data Into Your Java Program public class Practice { public static void main(String[] Passing Data Into Your Java Program public class Practice { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-39.jpg)
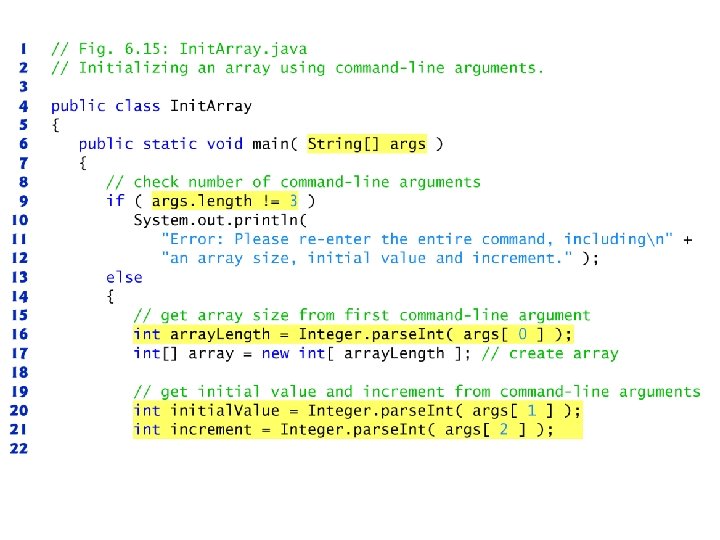
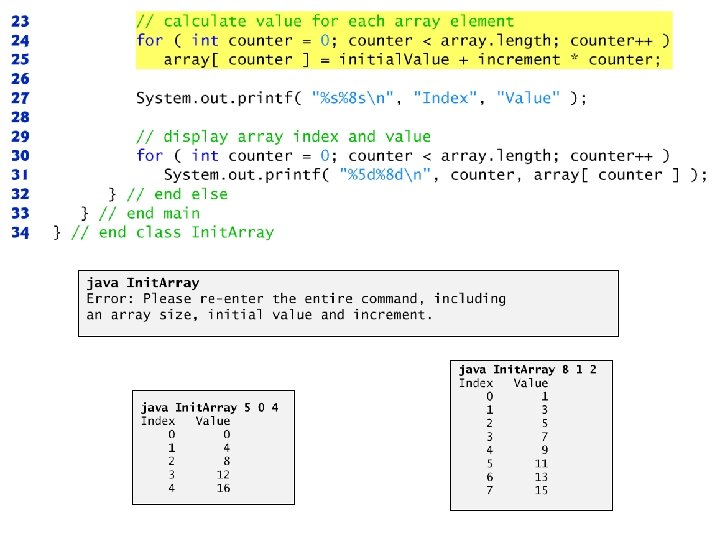
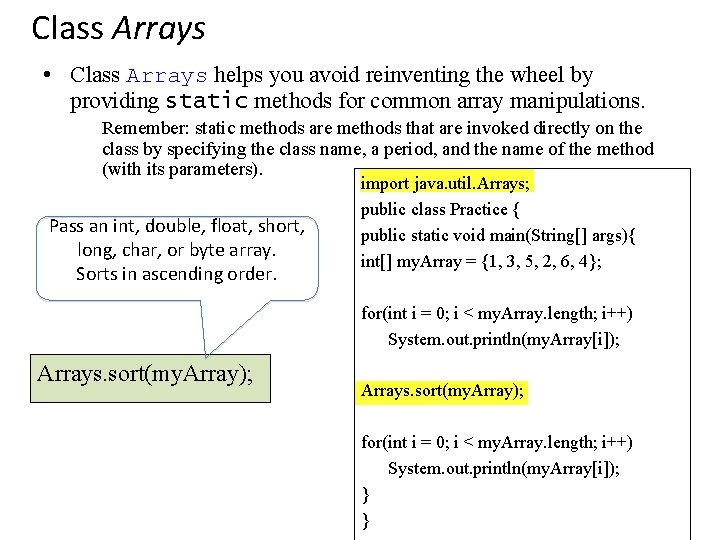
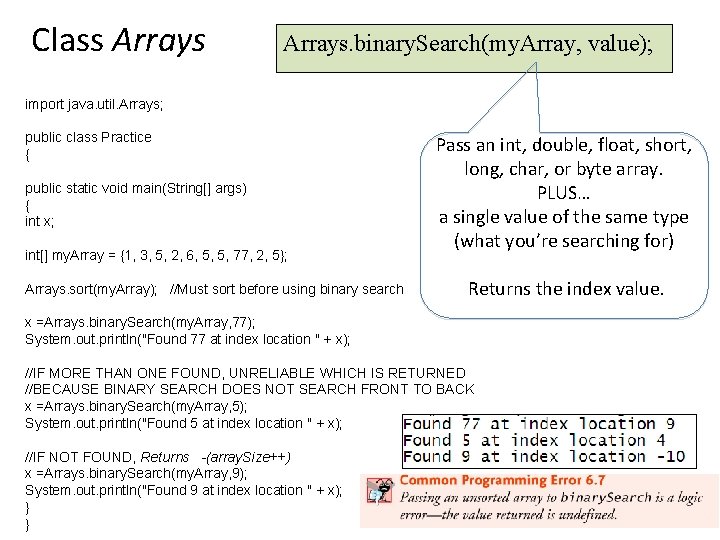
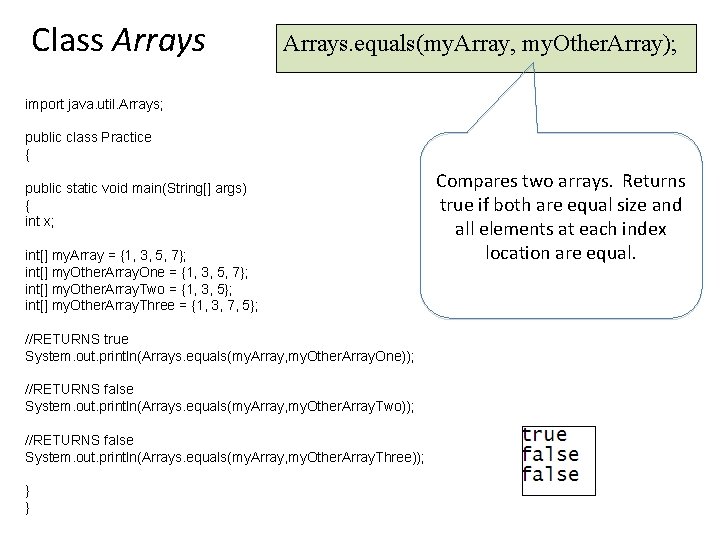
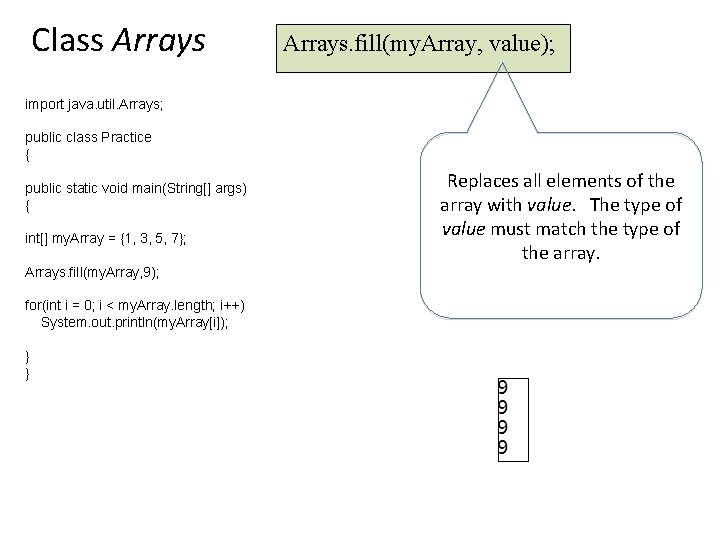
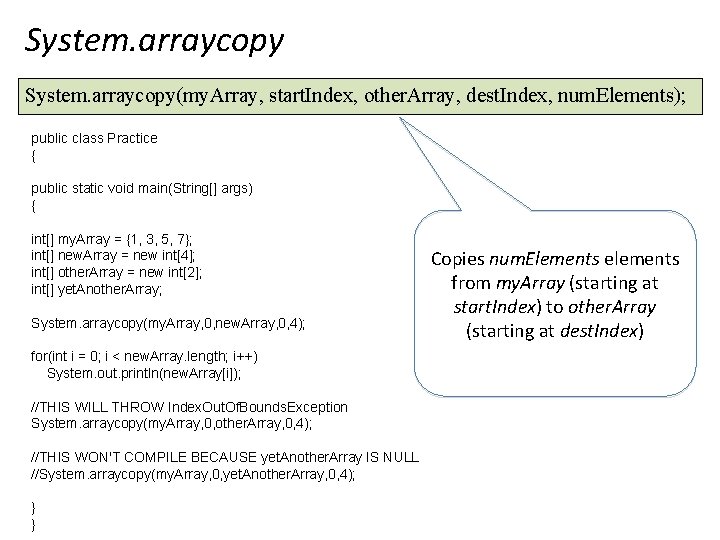
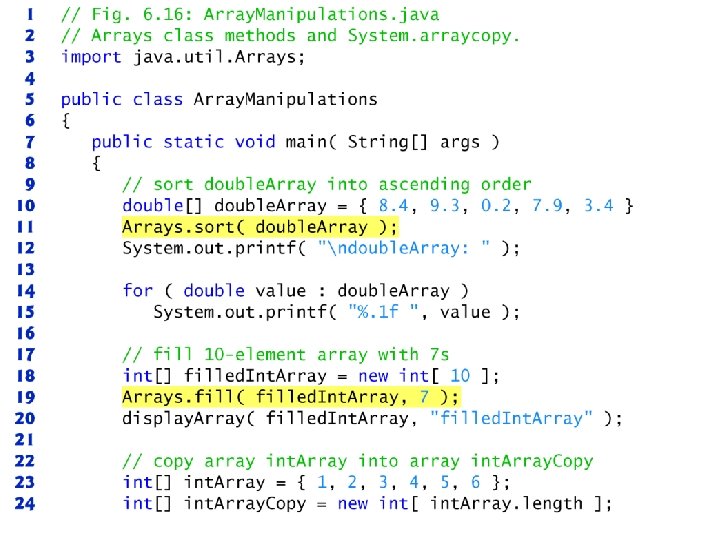
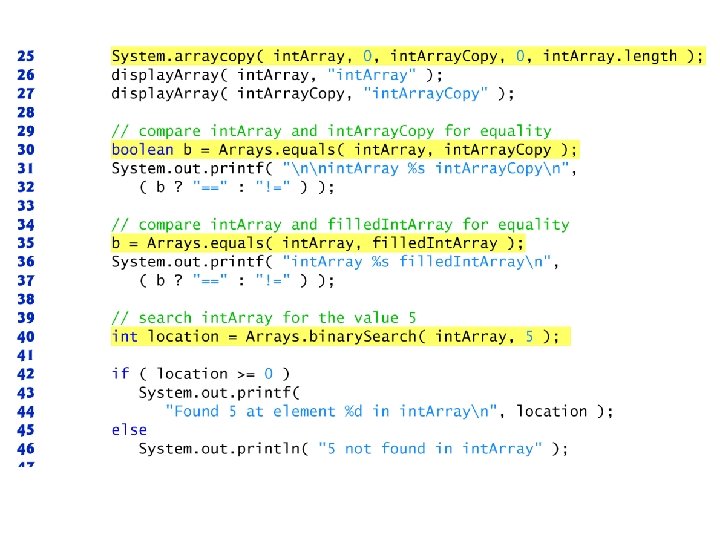
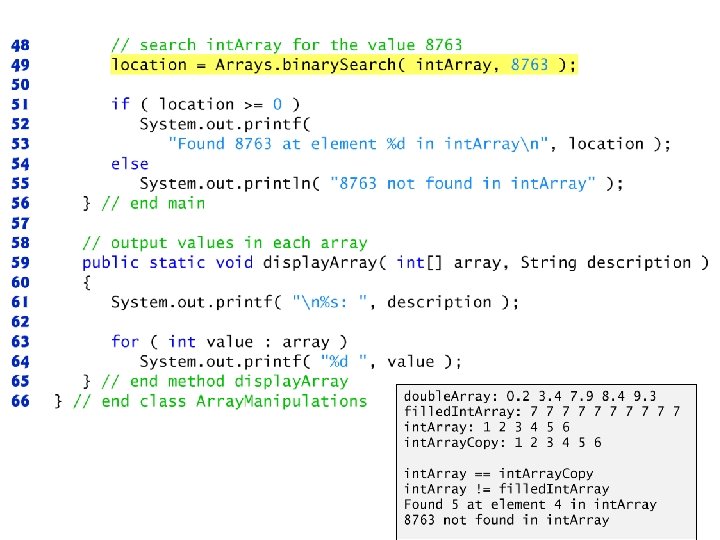
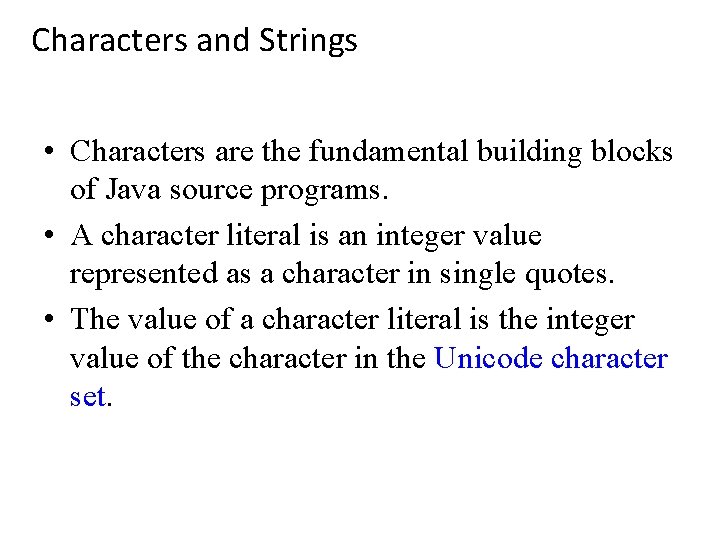
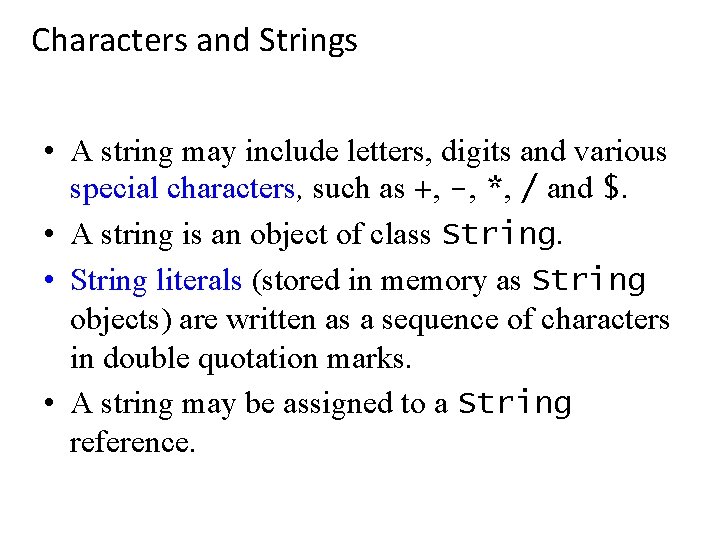
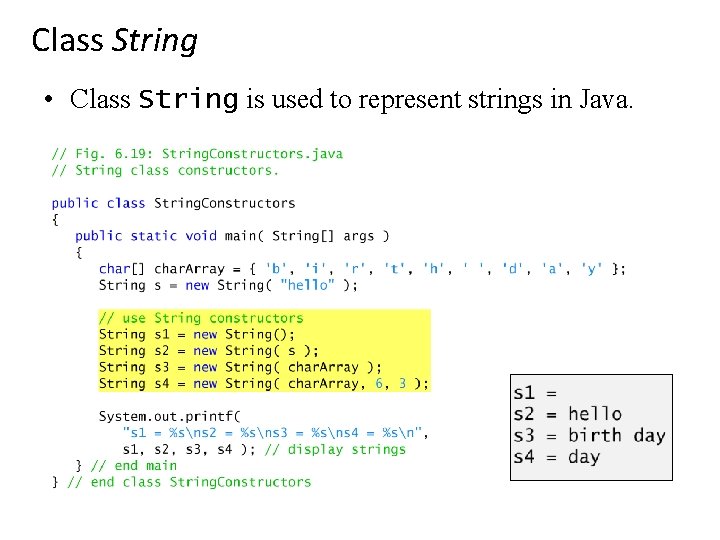
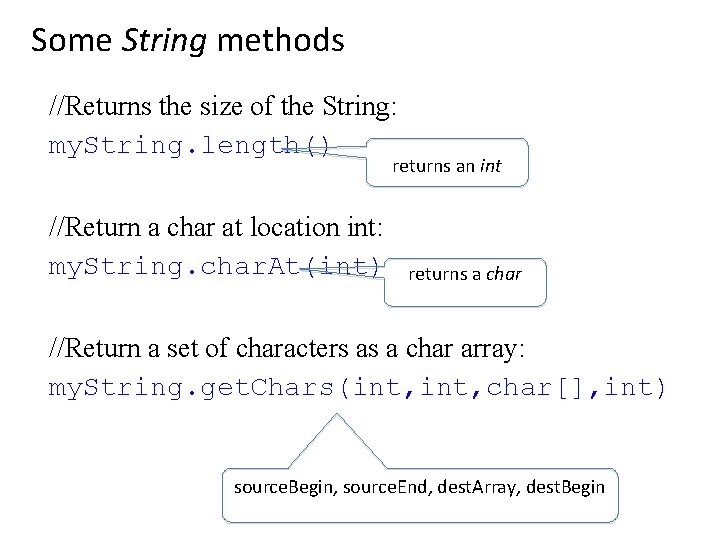
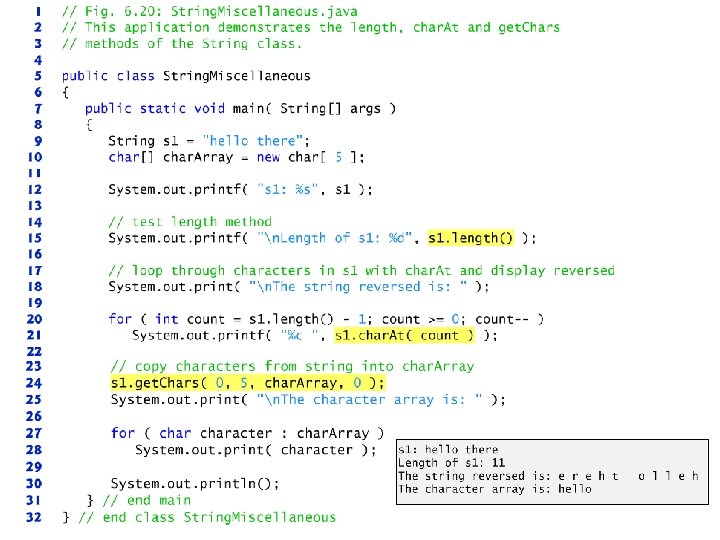
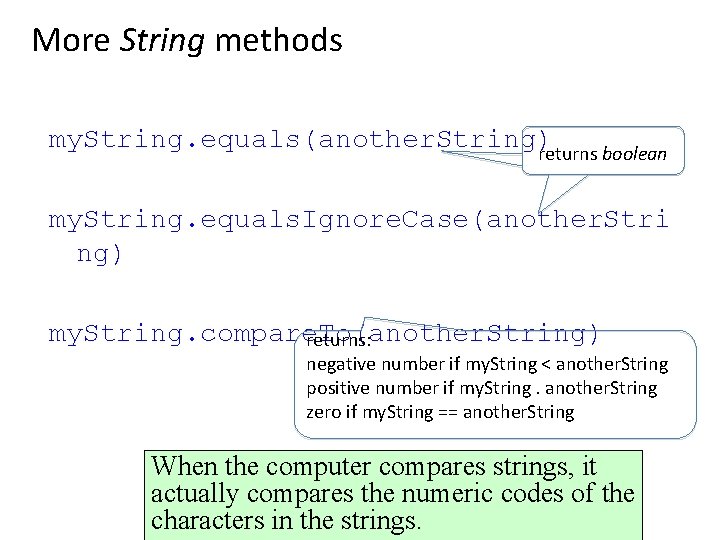
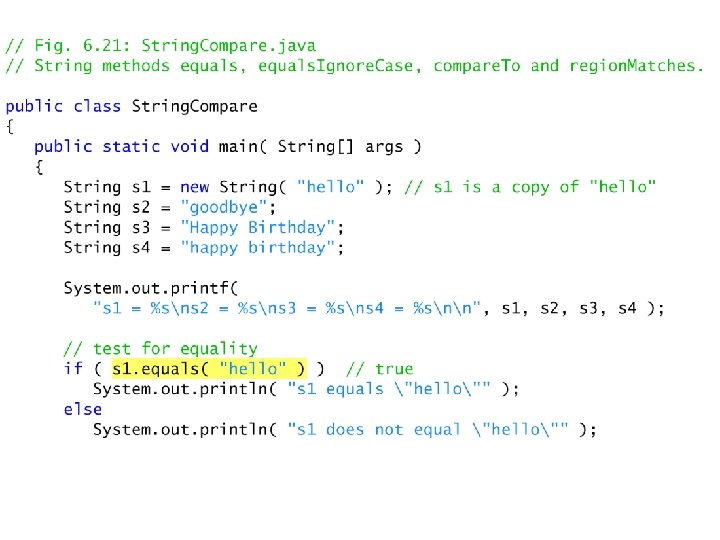
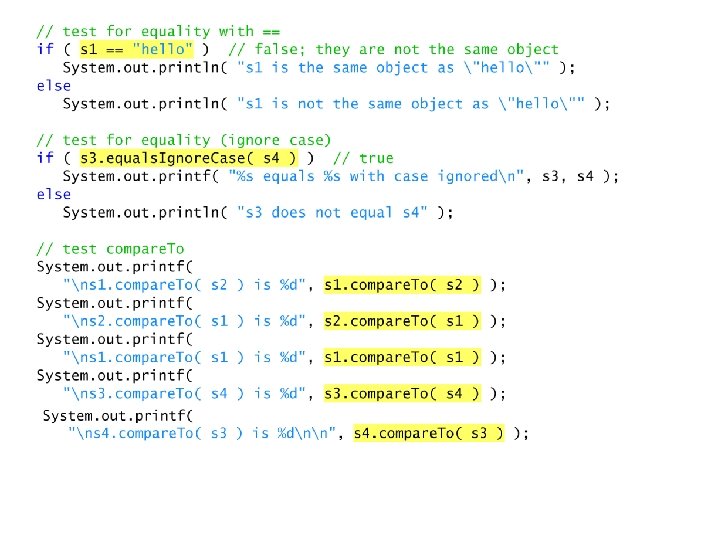
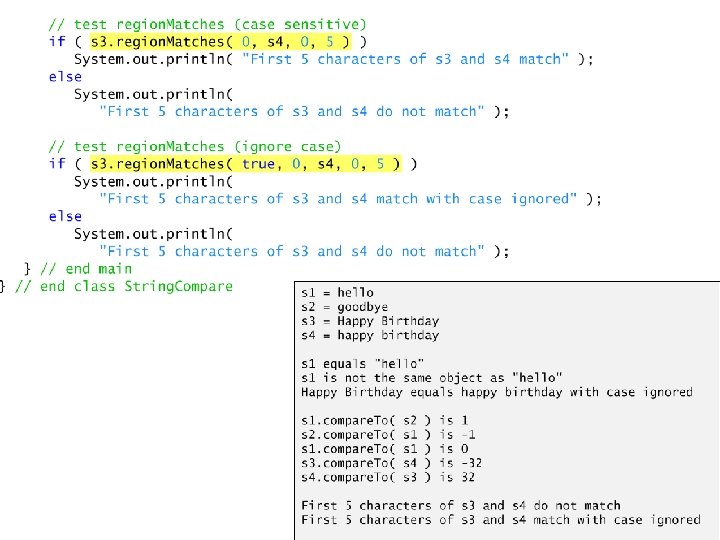
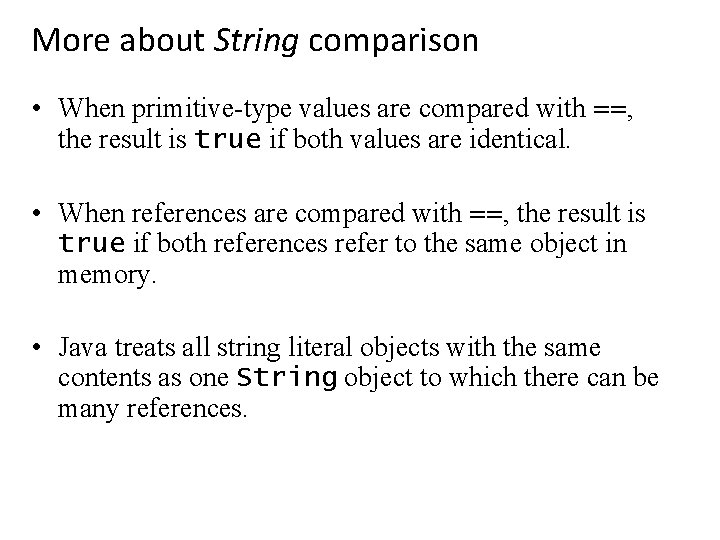
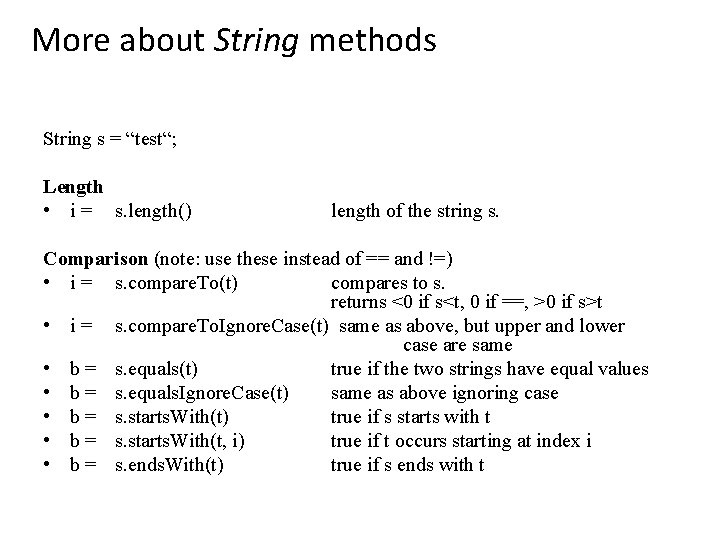
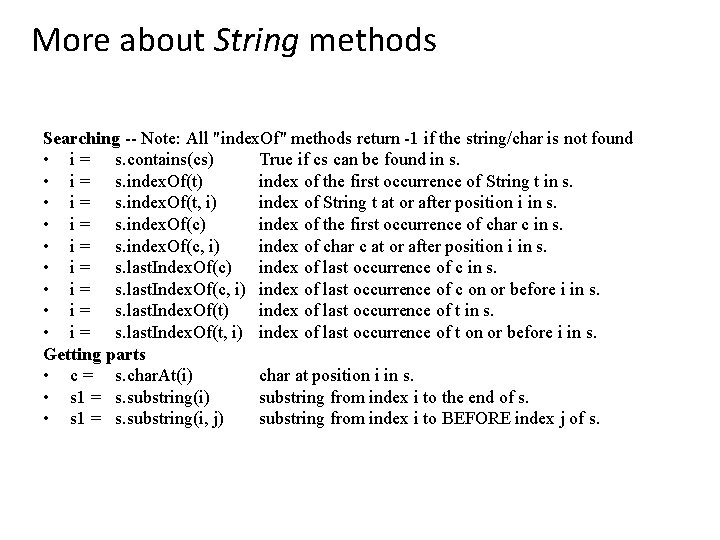
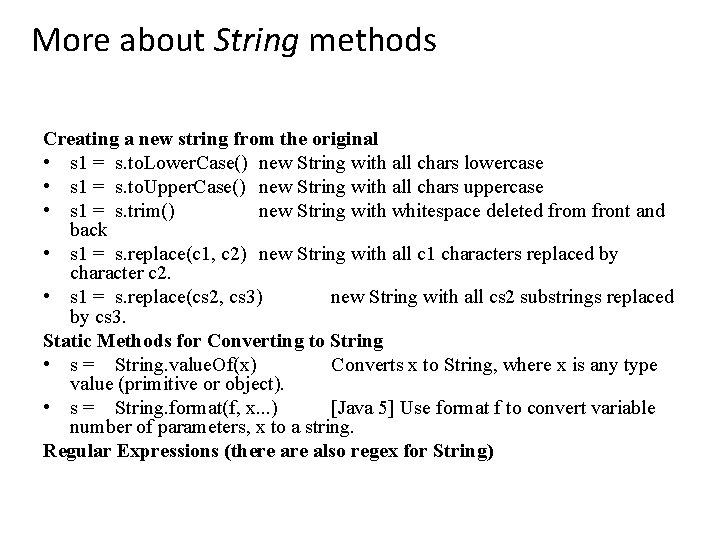
- Slides: 62
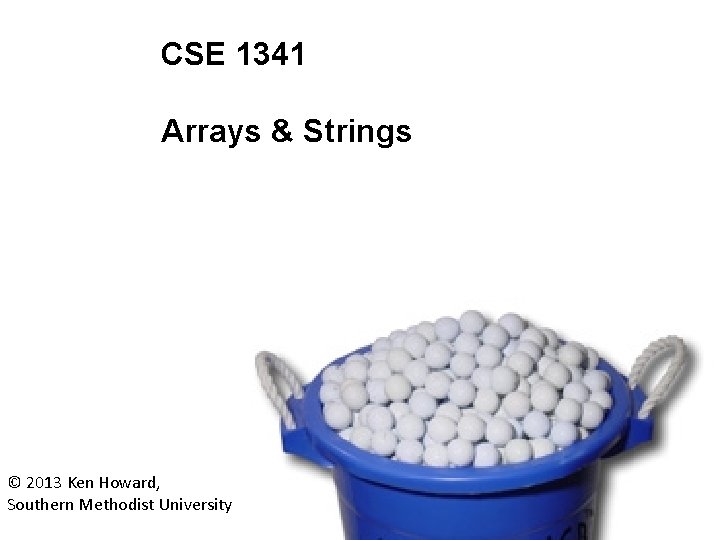
CSE 1341 Arrays & Strings © 2013 Ken Howard, Southern Methodist University
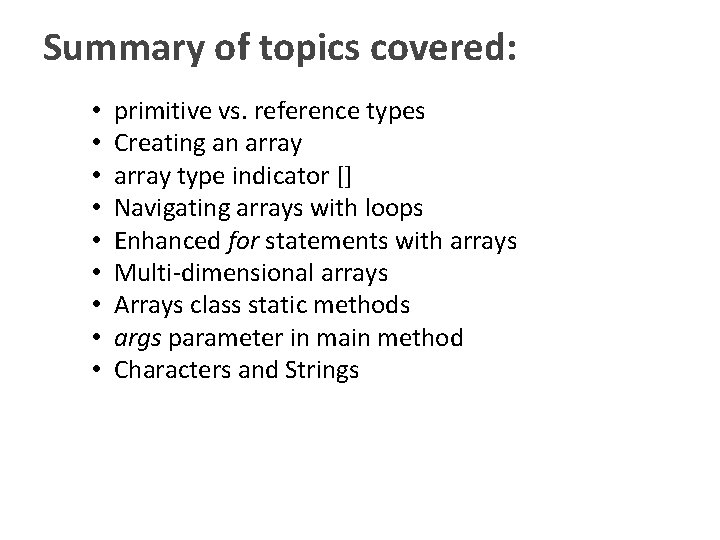
Summary of topics covered: • • • primitive vs. reference types Creating an array type indicator [] Navigating arrays with loops Enhanced for statements with arrays Multi-dimensional arrays Arrays class static methods args parameter in main method Characters and Strings
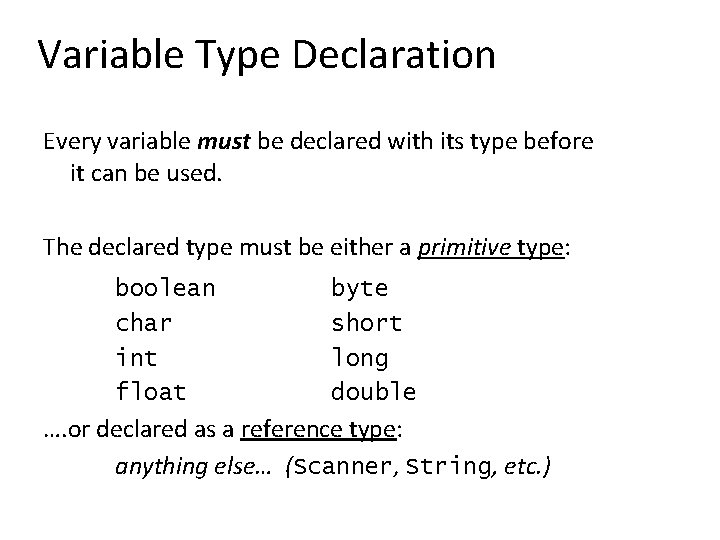
Variable Type Declaration Every variable must be declared with its type before it can be used. The declared type must be either a primitive type: boolean char int float byte short long double …. or declared as a reference type: anything else… (Scanner, String, etc. )
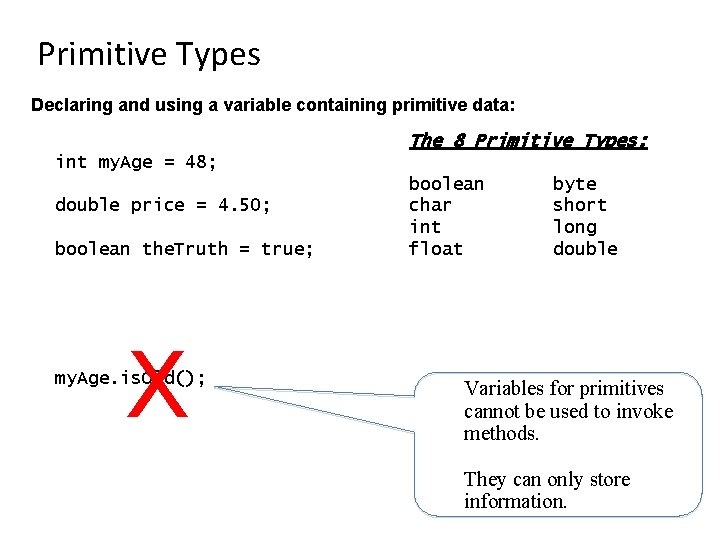
Primitive Types Declaring and using a variable containing primitive data: The 8 Primitive Types: int my. Age = 48; double price = 4. 50; boolean the. Truth = true; X my. Age. is. Old(); boolean char int float byte short long double Variables for primitives cannot be used to invoke methods. They can only store information.
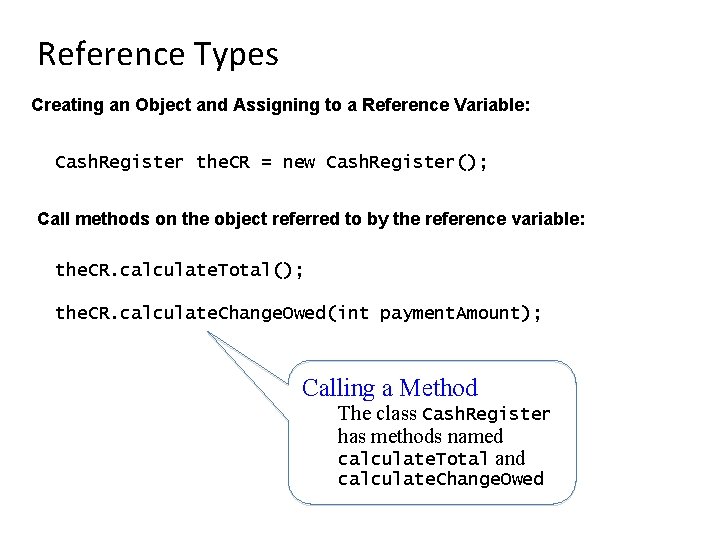
Reference Types Creating an Object and Assigning to a Reference Variable: Cash. Register the. CR = new Cash. Register(); Call methods on the object referred to by the reference variable: the. CR. calculate. Total(); the. CR. calculate. Change. Owed(int payment. Amount); Calling a Method The class Cash. Register has methods named calculate. Total and calculate. Change. Owed
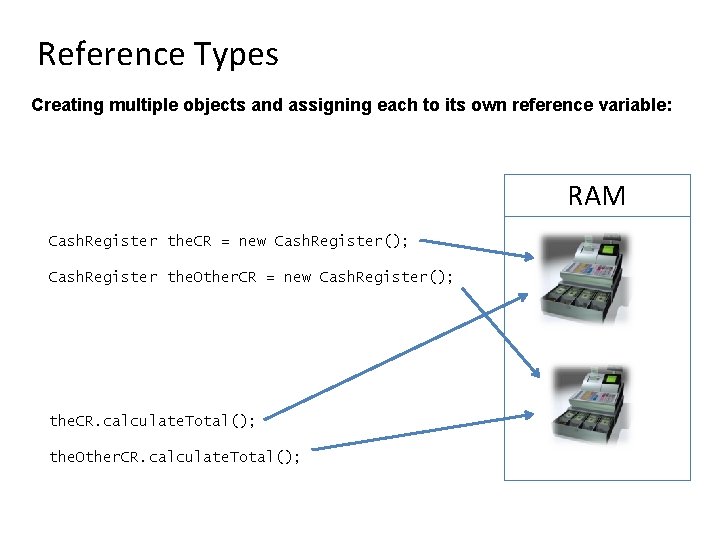
Reference Types Creating multiple objects and assigning each to its own reference variable: RAM Cash. Register the. CR = new Cash. Register(); Cash. Register the. Other. CR = new Cash. Register(); the. CR. calculate. Total(); the. Other. CR. calculate. Total();
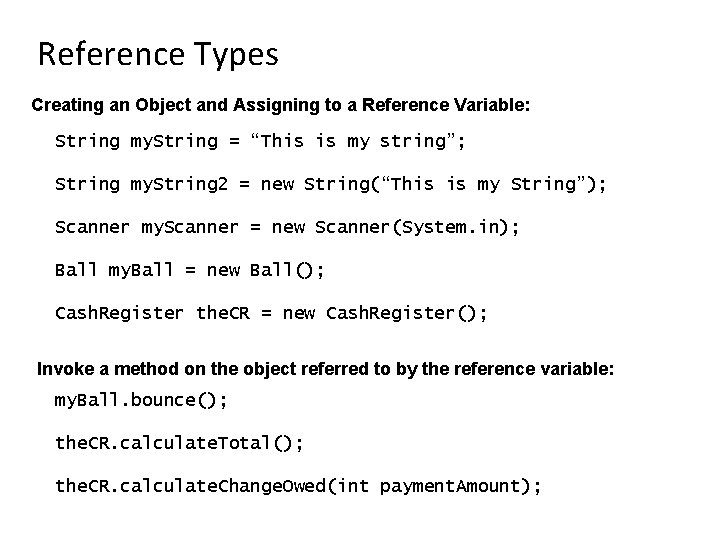
Reference Types Creating an Object and Assigning to a Reference Variable: String my. String = “This is my string”; String my. String 2 = new String(“This is my String”); Scanner my. Scanner = new Scanner(System. in); Ball my. Ball = new Ball(); Cash. Register the. CR = new Cash. Register(); Invoke a method on the object referred to by the reference variable: my. Ball. bounce(); the. CR. calculate. Total(); the. CR. calculate. Change. Owed(int payment. Amount);
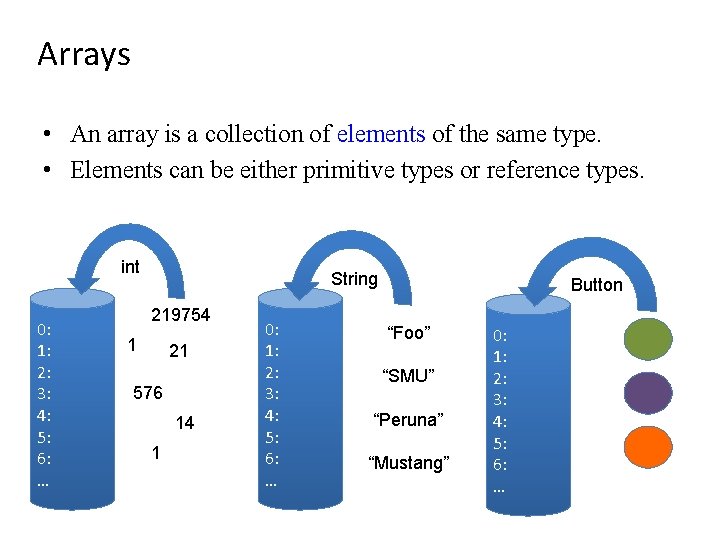
Arrays • An array is a collection of elements of the same type. • Elements can be either primitive types or reference types. int 0: 1: 2: 3: 4: 5: 6: … String 219754 1 21 576 14 1 0: 1: 2: 3: 4: 5: 6: … Button “Foo” “SMU” “Peruna” “Mustang” 0: 1: 2: 3: 4: 5: 6: …
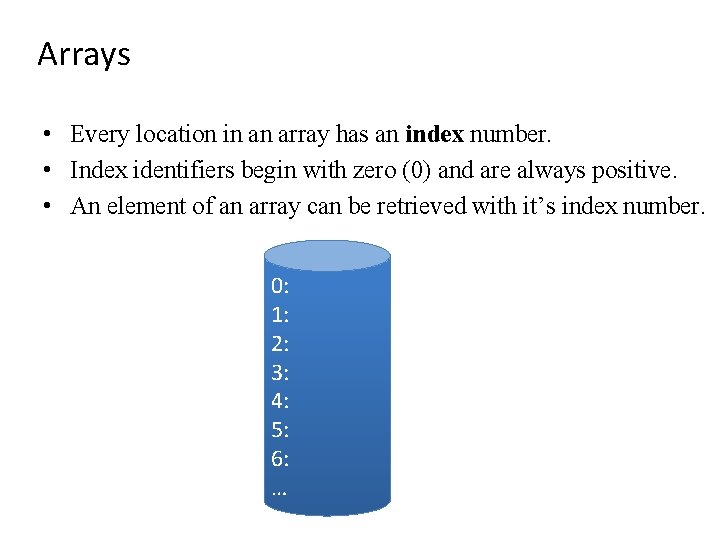
Arrays • Every location in an array has an index number. • Index identifiers begin with zero (0) and are always positive. • An element of an array can be retrieved with it’s index number. 0: 1: 2: 3: 4: 5: 6: …
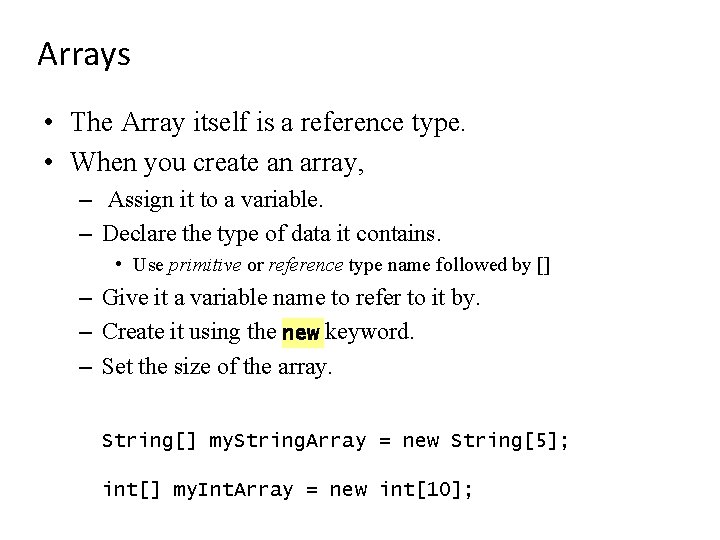
Arrays • The Array itself is a reference type. • When you create an array, – Assign it to a variable. – Declare the type of data it contains. • Use primitive or reference type name followed by [] – Give it a variable name to refer to it by. – Create it using the new keyword. – Set the size of the array. String[] my. String. Array = new String[5]; int[] my. Int. Array = new int[10];
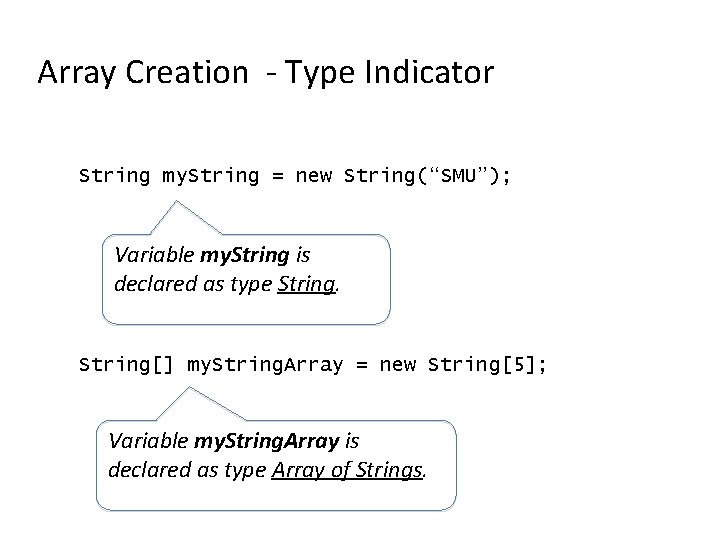
Array Creation - Type Indicator String my. String = new String(“SMU”); Variable my. String is declared as type String[] my. String. Array = new String[5]; Variable my. String. Array is declared as type Array of Strings.
![Arrays my Int Array0 0 my Int Array1 0 my Int Array2 0 my Arrays my. Int. Array[0] 0 my. Int. Array[1] 0 my. Int. Array[2] 0 my.](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-12.jpg)
Arrays my. Int. Array[0] 0 my. Int. Array[1] 0 my. Int. Array[2] 0 my. Int. Array[3] 0 my. Int. Array[4] 0 my. Int. Array[5] 0 my. Int. Array[6] 0 my. Int. Array[7] 0 my. Int. Array[8] 0 my. Int. Array[9] 0
![Arrays my Int Array2 12 my Int Array8 695 my Int Array0 Arrays my. Int. Array[2] = 12; my. Int. Array[8] = 695; my. Int. Array[0]](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-13.jpg)
Arrays my. Int. Array[2] = 12; my. Int. Array[8] = 695; my. Int. Array[0] 0 my. Int. Array[1] 0 my. Int. Array[2] 12 my. Int. Array[3] 0 my. Int. Array[4] 0 my. Int. Array[5] 0 my. Int. Array[6] 0 my. Int. Array[7] 0 my. Int. Array[8] 695 my. Int. Array[9] 0
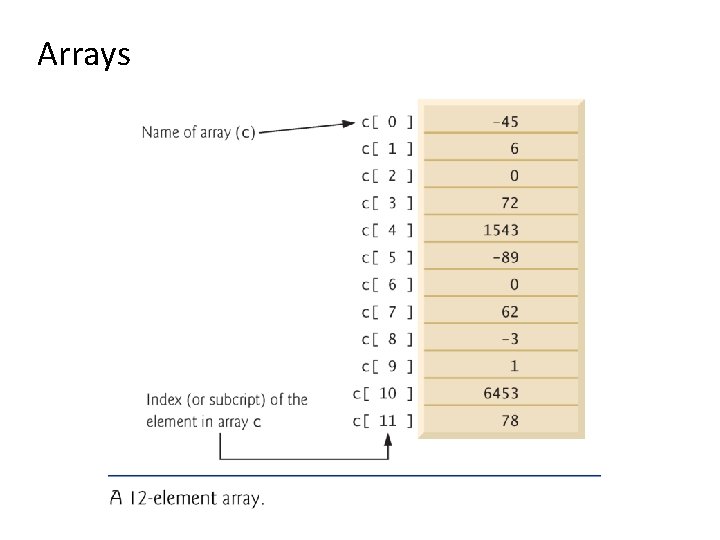
Arrays
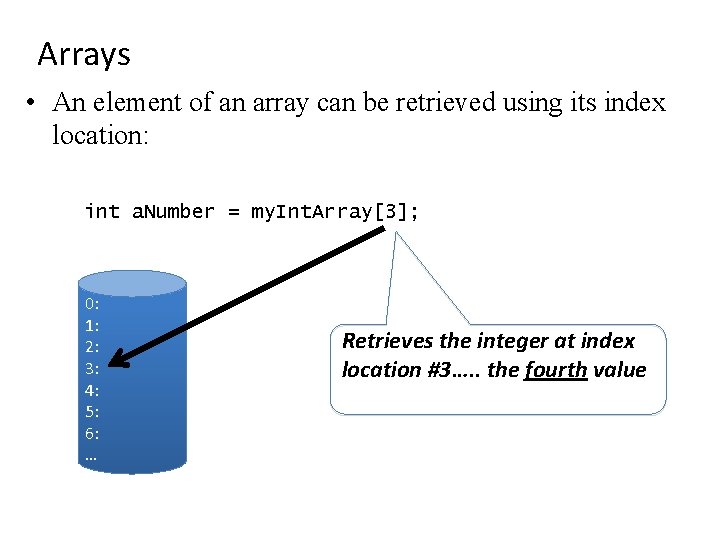
Arrays • An element of an array can be retrieved using its index location: int a. Number = my. Int. Array[3]; 0: 1: 2: 3: 4: 5: 6: … Retrieves the integer at index location #3…. . the fourth value
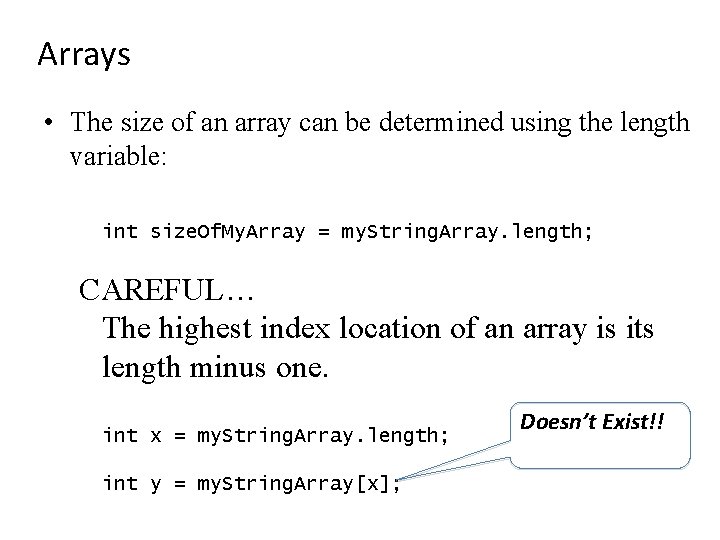
Arrays • The size of an array can be determined using the length variable: int size. Of. My. Array = my. String. Array. length; CAREFUL… The highest index location of an array is its length minus one. int x = my. String. Array. length; int y = my. String. Array[x]; Doesn’t Exist!!
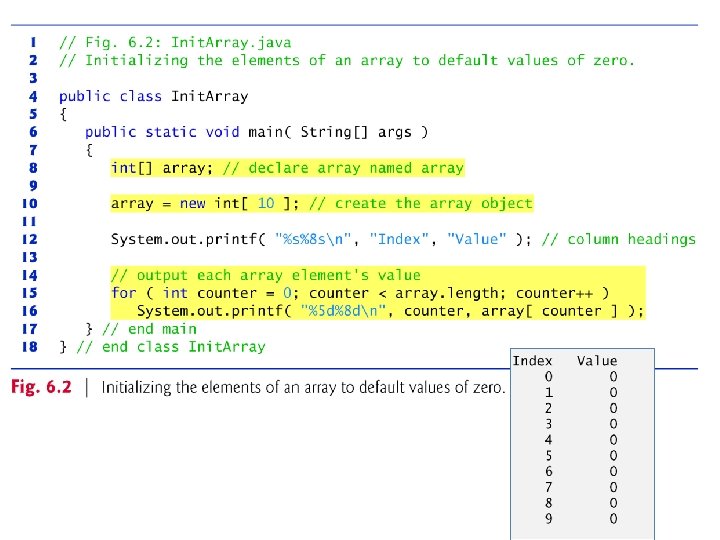
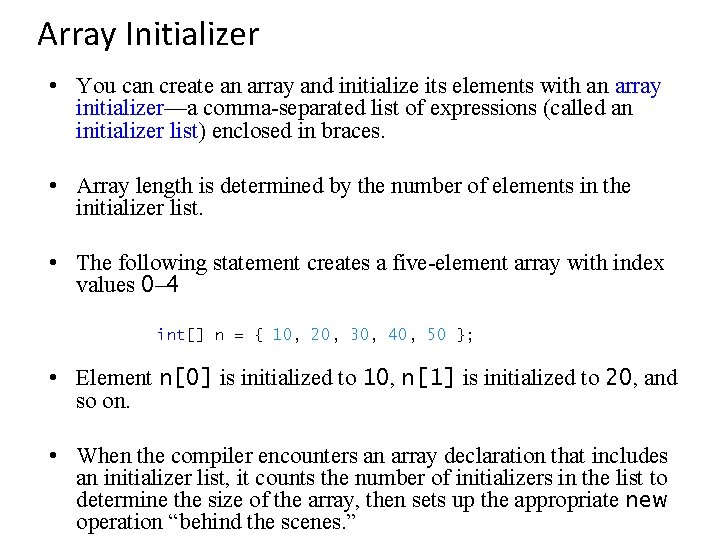
Array Initializer • You can create an array and initialize its elements with an array initializer—a comma-separated list of expressions (called an initializer list) enclosed in braces. • Array length is determined by the number of elements in the initializer list. • The following statement creates a five-element array with index values 0– 4 int[] n = { 10, 20, 30, 40, 50 }; • Element n[0] is initialized to 10, n[1] is initialized to 20, and so on. • When the compiler encounters an array declaration that includes an initializer list, it counts the number of initializers in the list to determine the size of the array, then sets up the appropriate new operation “behind the scenes. ”
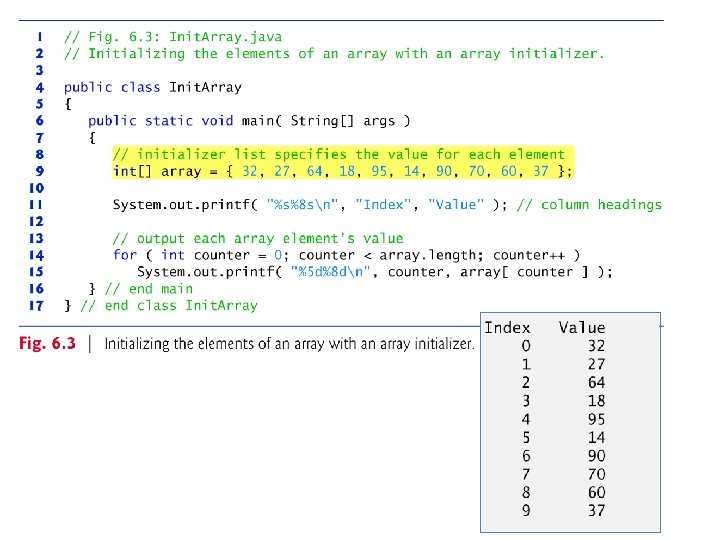
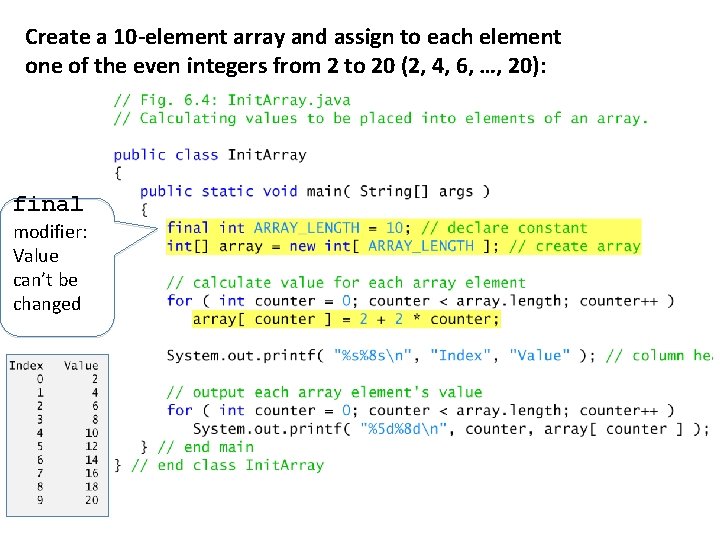
Create a 10 -element array and assign to each element one of the even integers from 2 to 20 (2, 4, 6, …, 20): final modifier: Value can’t be changed
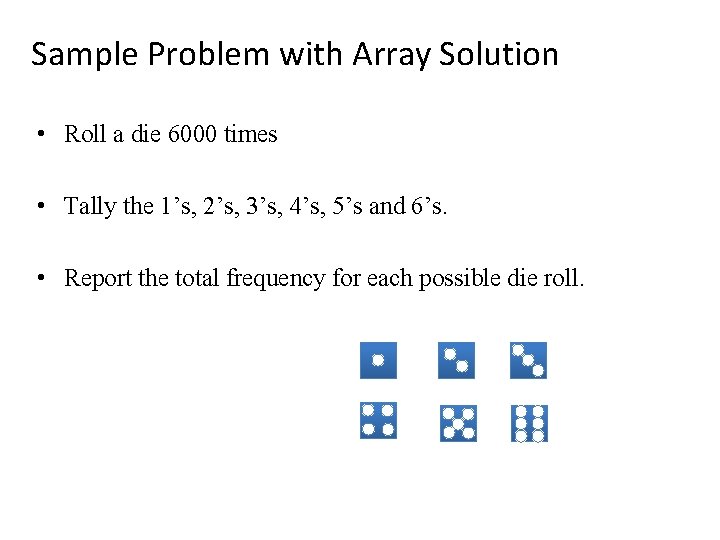
Sample Problem with Array Solution • Roll a die 6000 times • Tally the 1’s, 2’s, 3’s, 4’s, 5’s and 6’s. • Report the total frequency for each possible die roll.
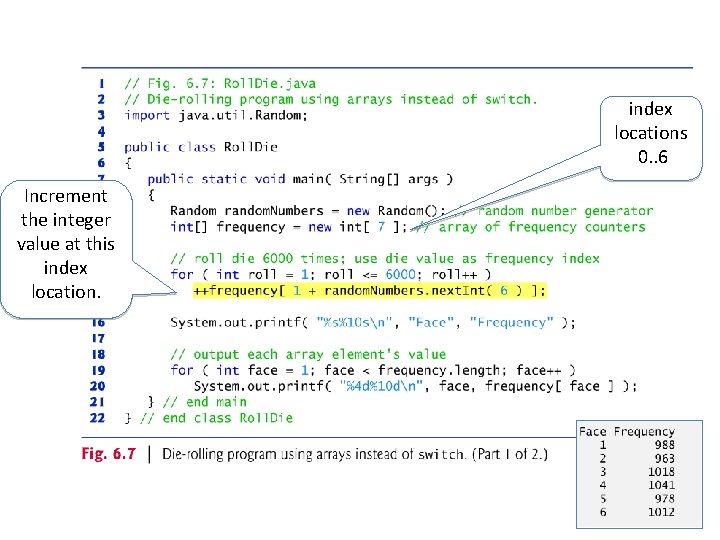
index locations 0. . 6 Increment the integer value at this index location.
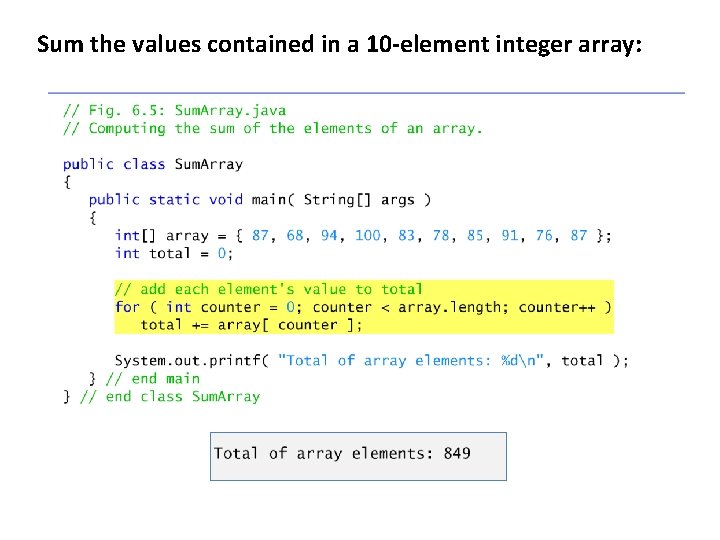
Sum the values contained in a 10 -element integer array:
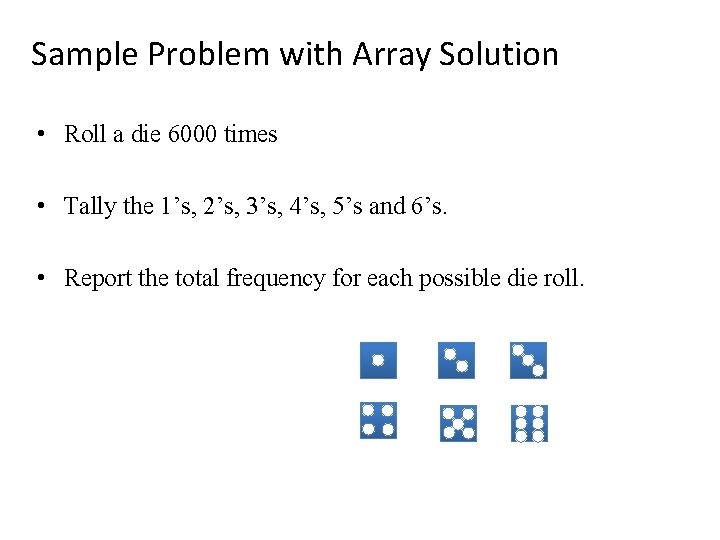
Sample Problem with Array Solution • Roll a die 6000 times • Tally the 1’s, 2’s, 3’s, 4’s, 5’s and 6’s. • Report the total frequency for each possible die roll.
![int x my String Array length int y my String Arrayx Will int x = my. String. Array. length; int y = my. String. Array[x]; Will](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-25.jpg)
int x = my. String. Array. length; int y = my. String. Array[x]; Will compile, but during runtime… Array. Index. Out. Of. Bounds. Exception
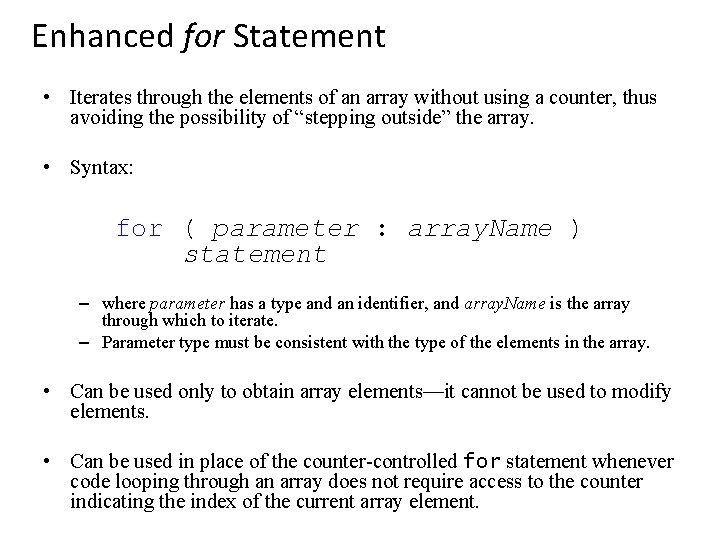
Enhanced for Statement • Iterates through the elements of an array without using a counter, thus avoiding the possibility of “stepping outside” the array. • Syntax: for ( parameter : array. Name ) statement – where parameter has a type and an identifier, and array. Name is the array through which to iterate. – Parameter type must be consistent with the type of the elements in the array. • Can be used only to obtain array elements—it cannot be used to modify elements. • Can be used in place of the counter-controlled for statement whenever code looping through an array does not require access to the counter indicating the index of the current array element.
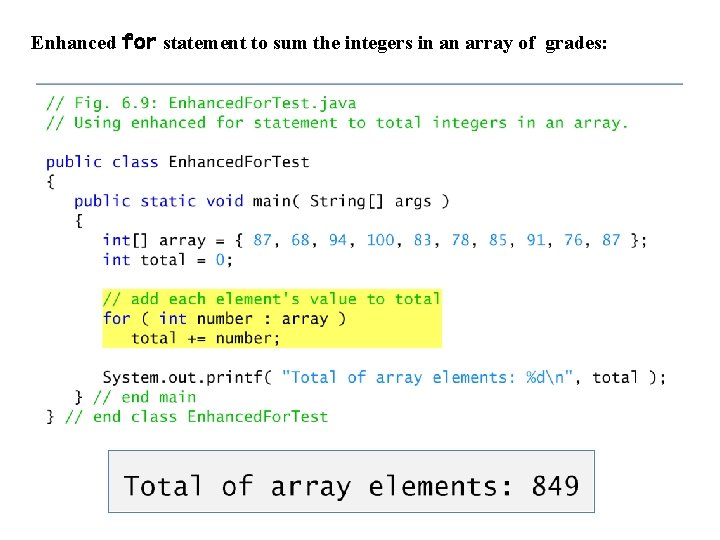
Enhanced for statement to sum the integers in an array of grades:
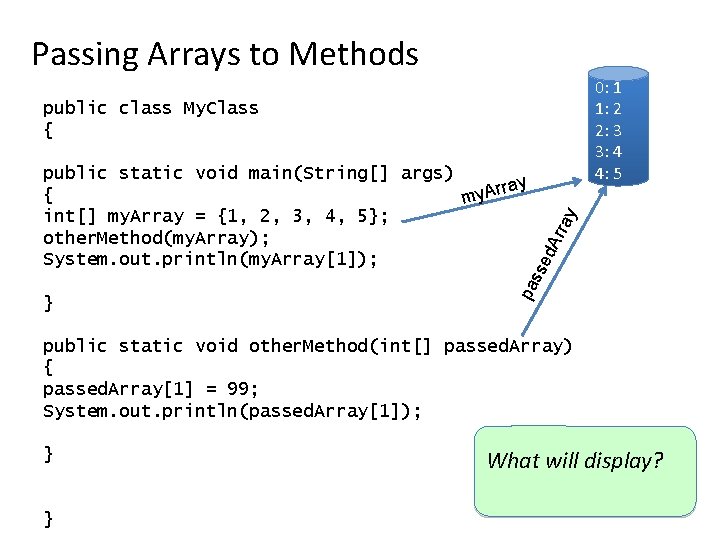
Passing Arrays to Methods 0: 1 1: 2 2: 3 3: 4 4: 5 } d. A rr pa sse public static void main(String[] args) rray A y { m int[] my. Array = {1, 2, 3, 4, 5}; other. Method(my. Array); System. out. println(my. Array[1]); ay public class My. Class { public static void other. Method(int[] passed. Array) { passed. Array[1] = 99; System. out. println(passed. Array[1]); } } What will display?
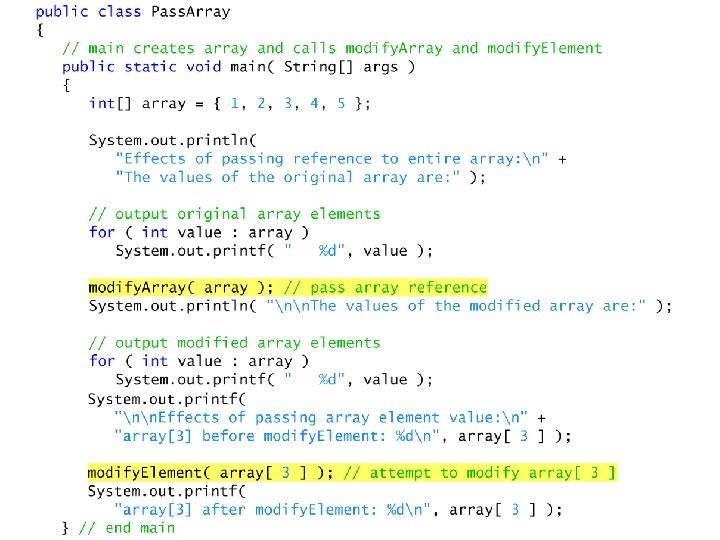
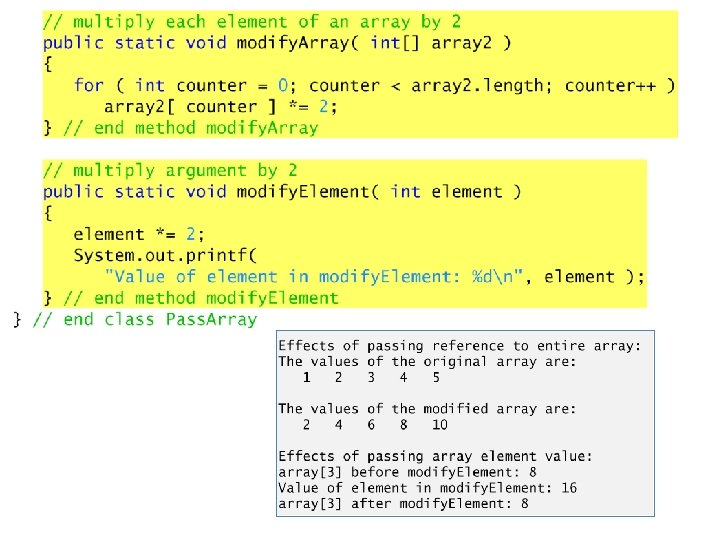
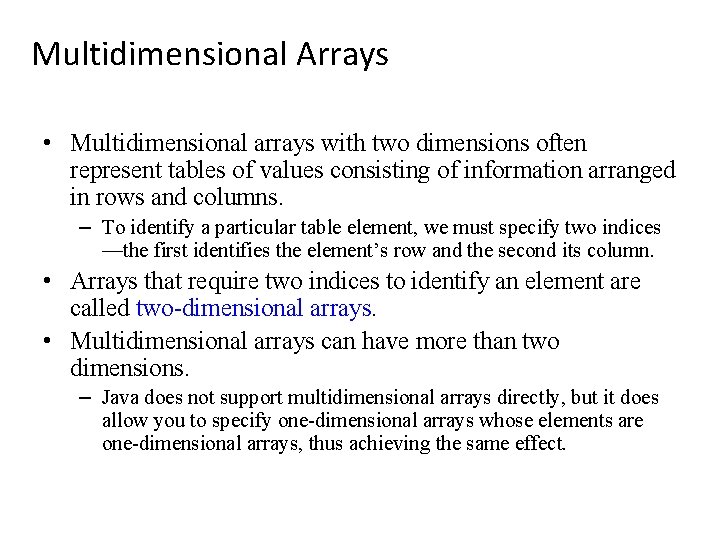
Multidimensional Arrays • Multidimensional arrays with two dimensions often represent tables of values consisting of information arranged in rows and columns. – To identify a particular table element, we must specify two indices —the first identifies the element’s row and the second its column. • Arrays that require two indices to identify an element are called two-dimensional arrays. • Multidimensional arrays can have more than two dimensions. – Java does not support multidimensional arrays directly, but it does allow you to specify one-dimensional arrays whose elements are one-dimensional arrays, thus achieving the same effect.
![Two Dimensional Arrays String my Array new String43 my Array01 Foo 0 Two Dimensional Arrays String[][] my. Array = new String[4][3]; my. Array[0][1] = “Foo” 0](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-32.jpg)
Two Dimensional Arrays String[][] my. Array = new String[4][3]; my. Array[0][1] = “Foo” 0 0 1 2 3 null my. Array[row][col] -------------my. Array[0][1] 1 Foo null 2 null
![Two Dimensional Arrays public class Practice public static void mainString args String Two Dimensional Arrays public class Practice { public static void main(String[] args) { String[][]](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-33.jpg)
Two Dimensional Arrays public class Practice { public static void main(String[] args) { String[][] my. Array = new String[4][3]; my. Array[0][1] = "Foo”; for(int i = 0; i < 4; i++) { for(int j = 0; j< 3; j++) { System. out. printf("n. Location %d , %d contains: %s", i , j, my. Array[i][j]); } }
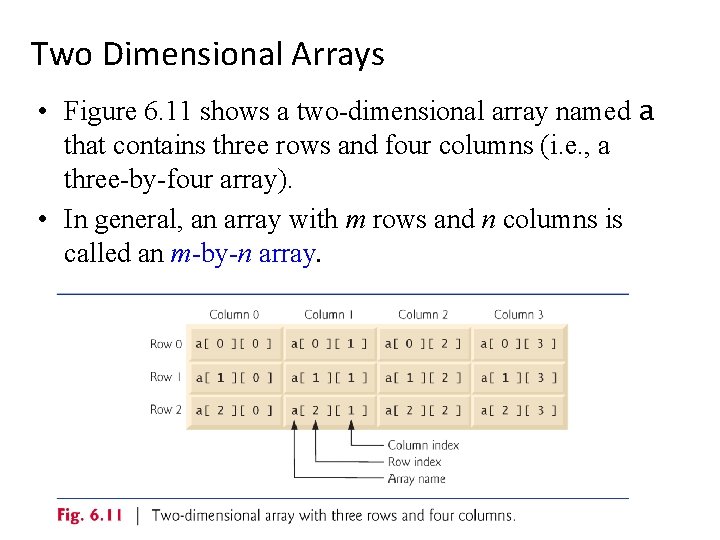
Two Dimensional Arrays • Figure 6. 11 shows a two-dimensional array named a that contains three rows and four columns (i. e. , a three-by-four array). • In general, an array with m rows and n columns is called an m-by-n array.
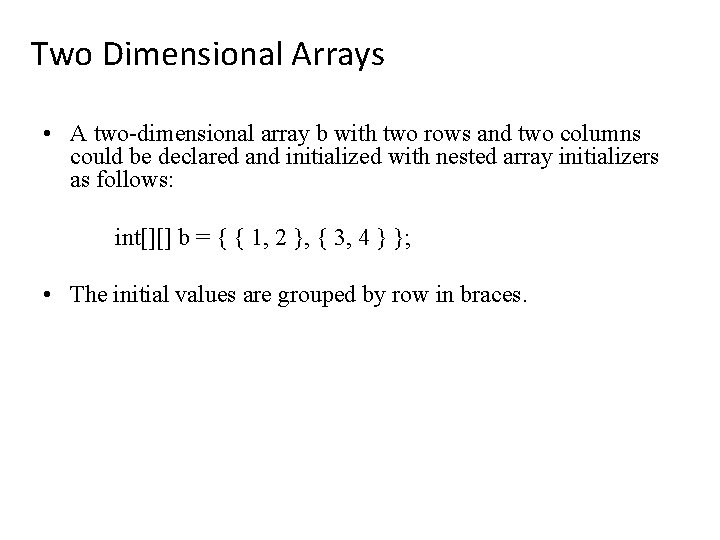
Two Dimensional Arrays • A two-dimensional array b with two rows and two columns could be declared and initialized with nested array initializers as follows: int[][] b = { { 1, 2 }, { 3, 4 } }; • The initial values are grouped by row in braces.
![Array with Different Row Lengths String my Array new String4 my Array0 Array with Different Row Lengths String[][] my. Array = new String[4][]; my. Array[0] =](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-36.jpg)
Array with Different Row Lengths String[][] my. Array = new String[4][]; my. Array[0] = new String[5]; my. Array[1] = new String[2]; my. Array[2] = new String[3]; my. Array[3][2] = “Foo”; 0 1 2 3 0 1 null null 2 null Foo 3 null 4 null
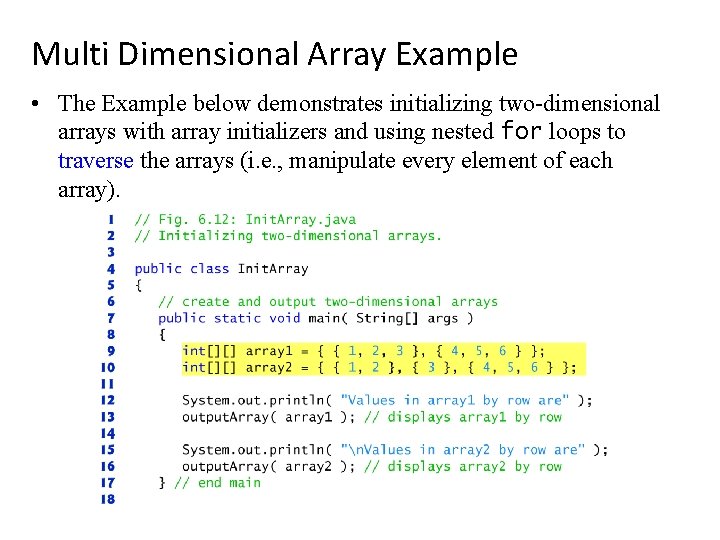
Multi Dimensional Array Example • The Example below demonstrates initializing two-dimensional arrays with array initializers and using nested for loops to traverse the arrays (i. e. , manipulate every element of each array).
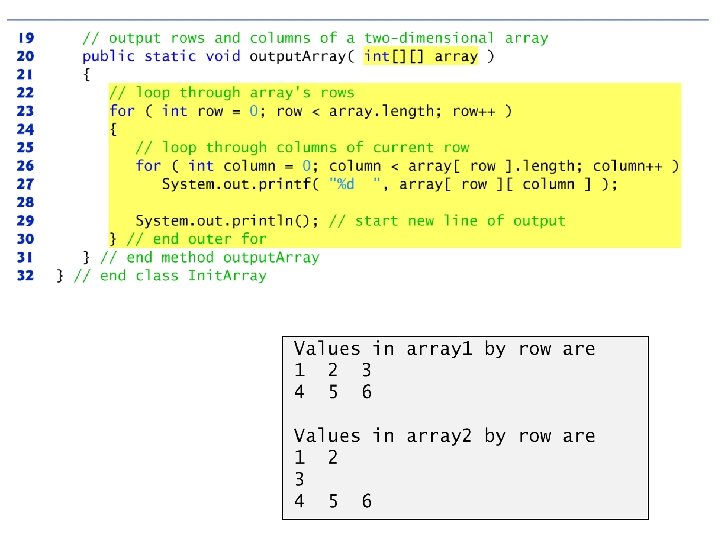
![Passing Data Into Your Java Program public class Practice public static void mainString Passing Data Into Your Java Program public class Practice { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/6d42195fe12401db816e08f3f0144a7b/image-39.jpg)
Passing Data Into Your Java Program public class Practice { public static void main(String[] args) { System. out. println(args[0]); args is an array of Strings. } } Command line arguments. C: >java My. Class Foo TRY THIS: Write a program that displays all command line arguments, where you don’t know how many values will be passed in. (Hint: args. length gives the size of the array)
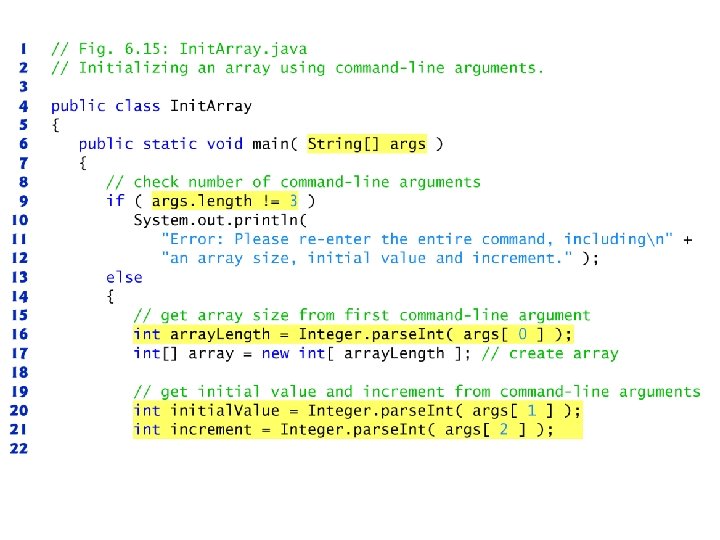
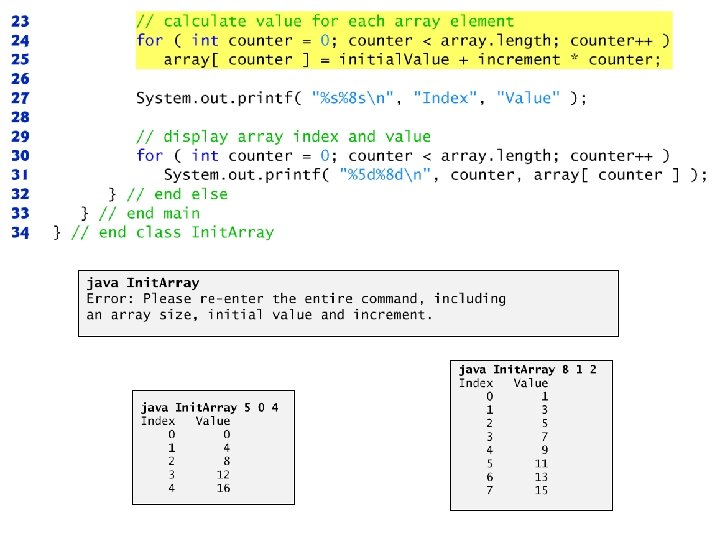
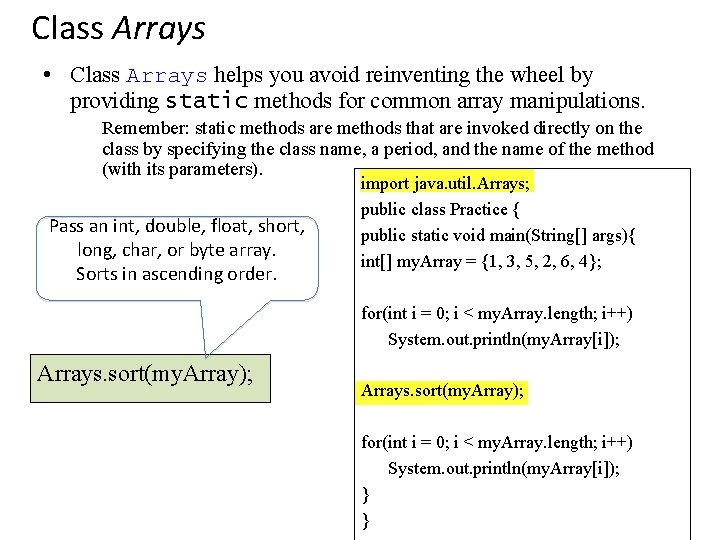
Class Arrays • Class Arrays helps you avoid reinventing the wheel by providing static methods for common array manipulations. Remember: static methods are methods that are invoked directly on the class by specifying the class name, a period, and the name of the method (with its parameters). Pass an int, double, float, short, long, char, or byte array. Sorts in ascending order. import java. util. Arrays; public class Practice { public static void main(String[] args){ int[] my. Array = {1, 3, 5, 2, 6, 4}; for(int i = 0; i < my. Array. length; i++) System. out. println(my. Array[i]); Arrays. sort(my. Array); for(int i = 0; i < my. Array. length; i++) System. out. println(my. Array[i]); } }
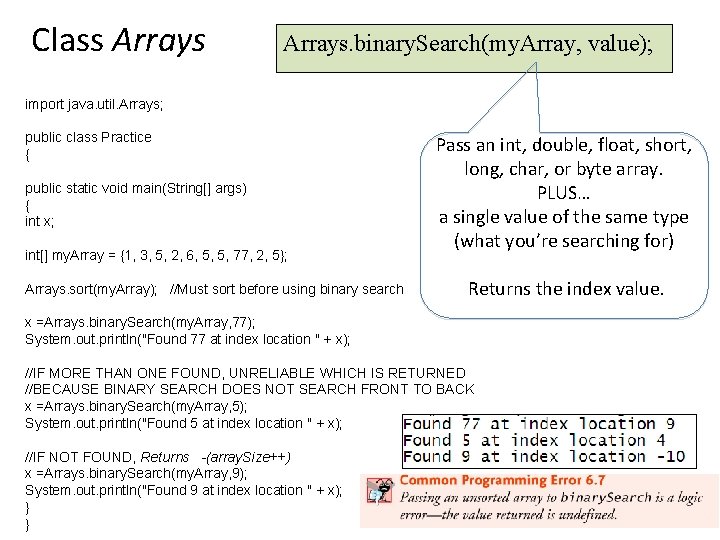
Class Arrays. binary. Search(my. Array, value); import java. util. Arrays; public class Practice { public static void main(String[] args) { int x; int[] my. Array = {1, 3, 5, 2, 6, 5, 5, 77, 2, 5}; Arrays. sort(my. Array); //Must sort before using binary search Pass an int, double, float, short, long, char, or byte array. PLUS… a single value of the same type (what you’re searching for) Returns the index value. x =Arrays. binary. Search(my. Array, 77); System. out. println("Found 77 at index location " + x); //IF MORE THAN ONE FOUND, UNRELIABLE WHICH IS RETURNED //BECAUSE BINARY SEARCH DOES NOT SEARCH FRONT TO BACK x =Arrays. binary. Search(my. Array, 5); System. out. println("Found 5 at index location " + x); //IF NOT FOUND, Returns -(array. Size++) x =Arrays. binary. Search(my. Array, 9); System. out. println("Found 9 at index location " + x); } }
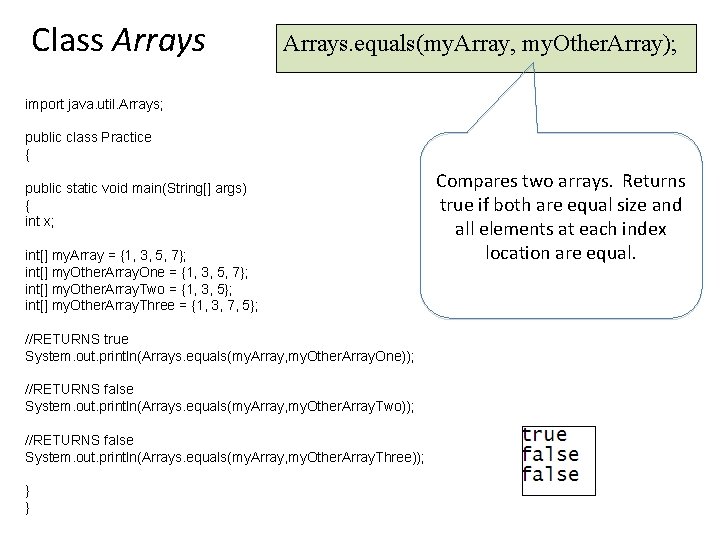
Class Arrays. equals(my. Array, my. Other. Array); import java. util. Arrays; public class Practice { public static void main(String[] args) { int x; int[] my. Array = {1, 3, 5, 7}; int[] my. Other. Array. One = {1, 3, 5, 7}; int[] my. Other. Array. Two = {1, 3, 5}; int[] my. Other. Array. Three = {1, 3, 7, 5}; //RETURNS true System. out. println(Arrays. equals(my. Array, my. Other. Array. One)); //RETURNS false System. out. println(Arrays. equals(my. Array, my. Other. Array. Two)); //RETURNS false System. out. println(Arrays. equals(my. Array, my. Other. Array. Three)); } } Compares two arrays. Returns true if both are equal size and all elements at each index location are equal.
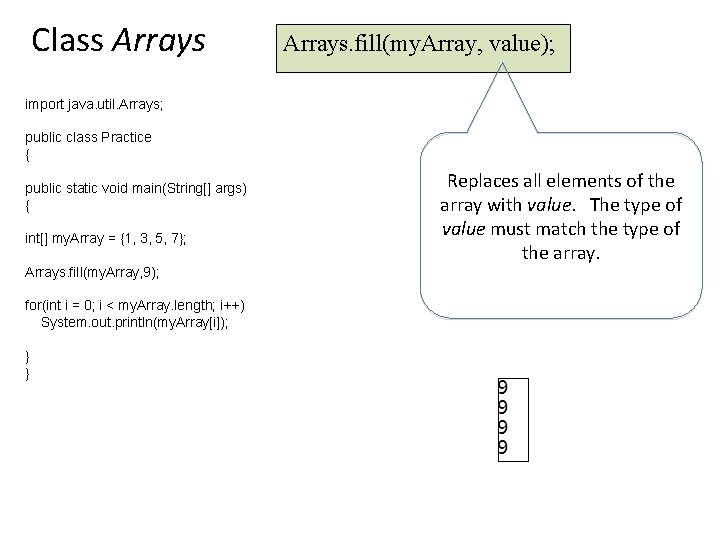
Class Arrays. fill(my. Array, value); import java. util. Arrays; public class Practice { public static void main(String[] args) { int[] my. Array = {1, 3, 5, 7}; Arrays. fill(my. Array, 9); for(int i = 0; i < my. Array. length; i++) System. out. println(my. Array[i]); } } Replaces all elements of the array with value. The type of value must match the type of the array.
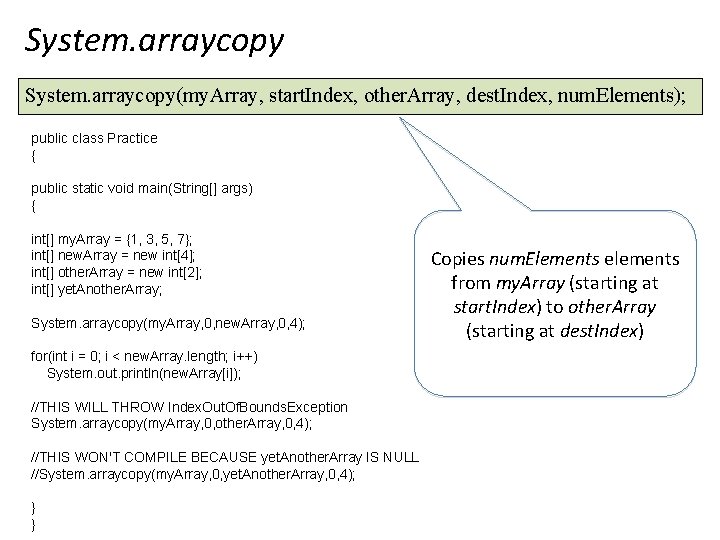
System. arraycopy(my. Array, start. Index, other. Array, dest. Index, num. Elements); public class Practice { public static void main(String[] args) { int[] my. Array = {1, 3, 5, 7}; int[] new. Array = new int[4]; int[] other. Array = new int[2]; int[] yet. Another. Array; System. arraycopy(my. Array, 0, new. Array, 0, 4); for(int i = 0; i < new. Array. length; i++) System. out. println(new. Array[i]); //THIS WILL THROW Index. Out. Of. Bounds. Exception System. arraycopy(my. Array, 0, other. Array, 0, 4); //THIS WON'T COMPILE BECAUSE yet. Another. Array IS NULL //System. arraycopy(my. Array, 0, yet. Another. Array, 0, 4); } } Copies num. Elements elements from my. Array (starting at start. Index) to other. Array (starting at dest. Index)
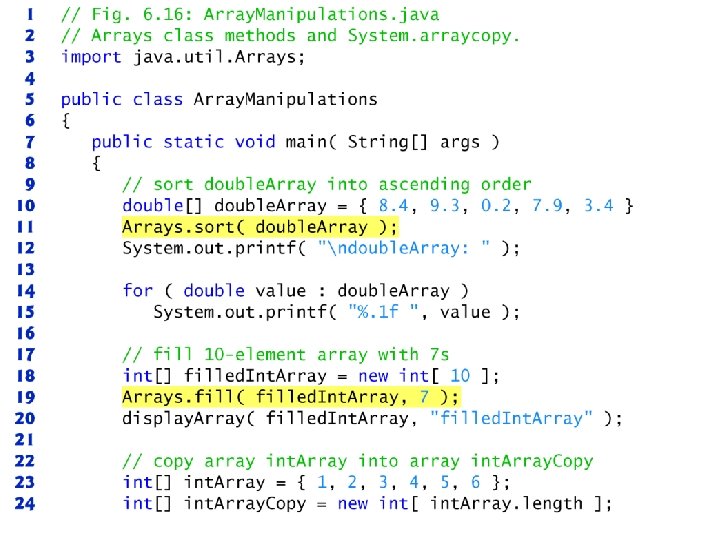
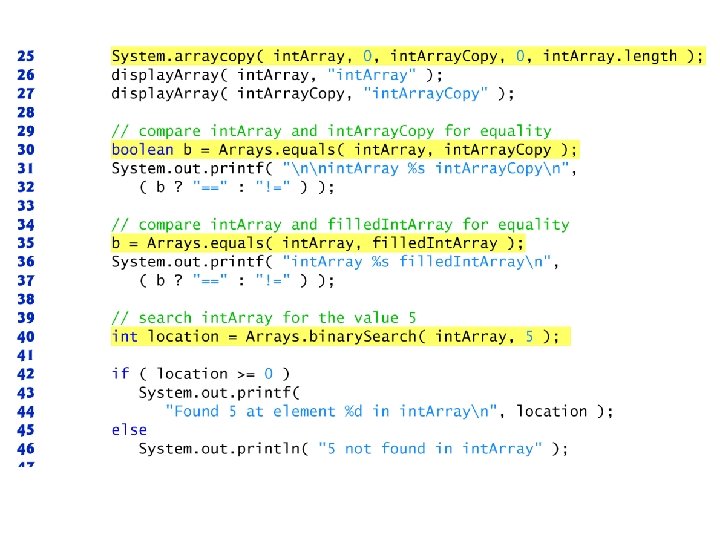
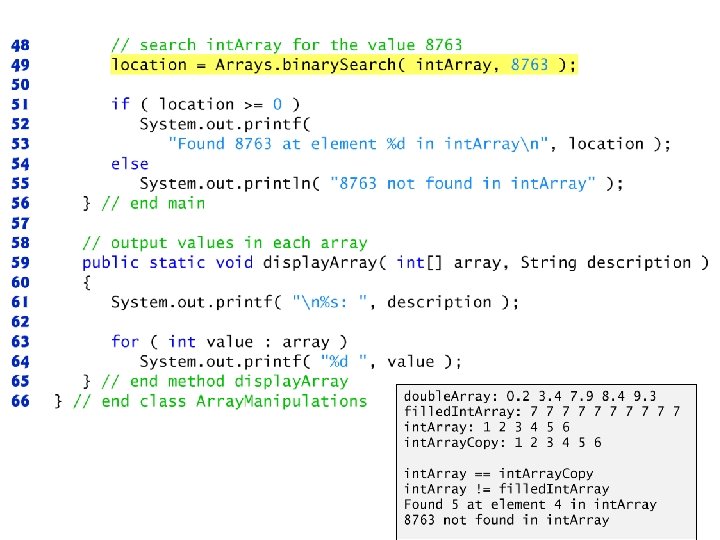
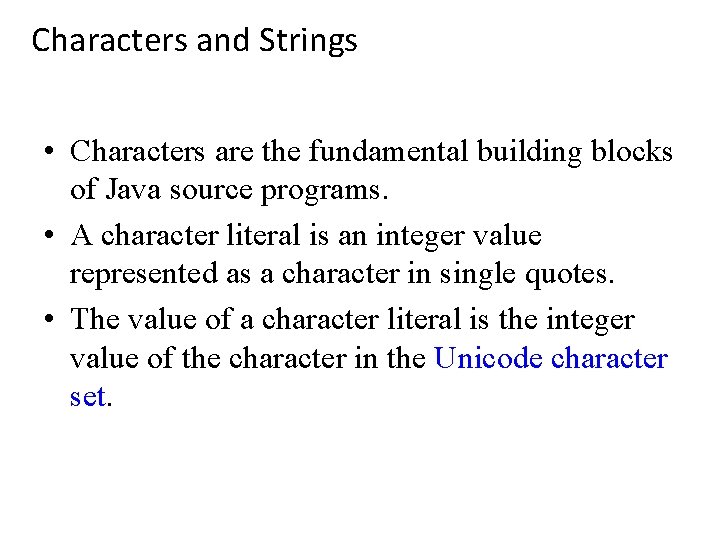
Characters and Strings • Characters are the fundamental building blocks of Java source programs. • A character literal is an integer value represented as a character in single quotes. • The value of a character literal is the integer value of the character in the Unicode character set.
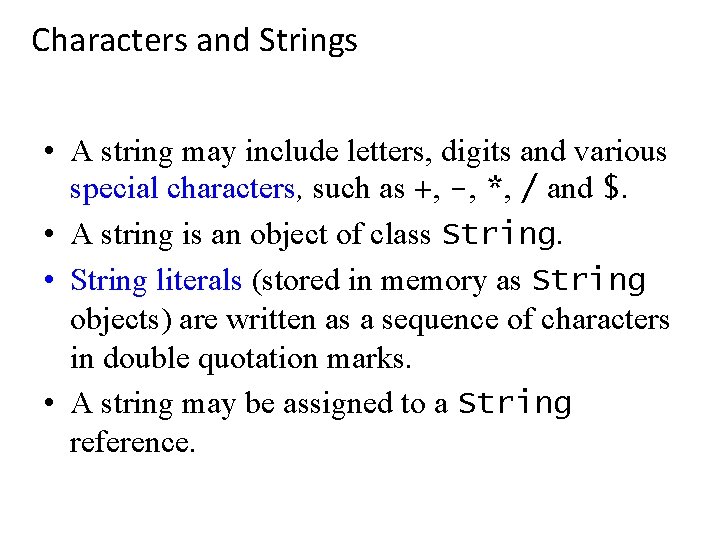
Characters and Strings • A string may include letters, digits and various special characters, such as +, -, *, / and $. • A string is an object of class String. • String literals (stored in memory as String objects) are written as a sequence of characters in double quotation marks. • A string may be assigned to a String reference.
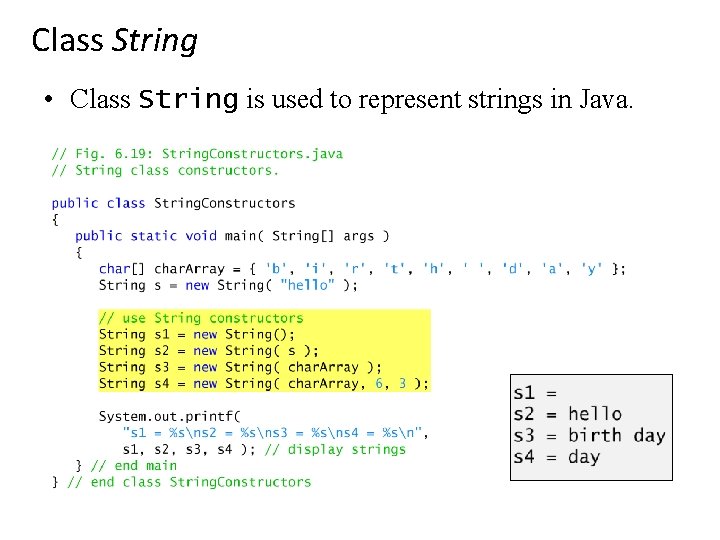
Class String • Class String is used to represent strings in Java.
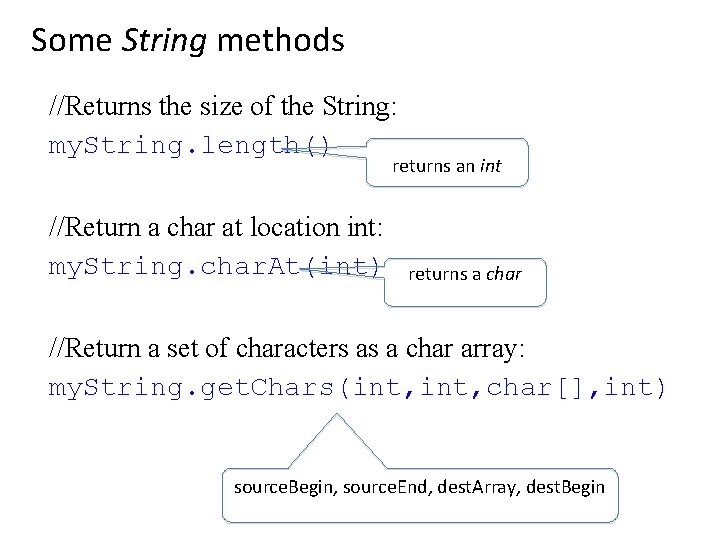
Some String methods //Returns the size of the String: my. String. length() returns an int //Return a char at location int: my. String. char. At(int) returns a char //Return a set of characters as a char array: my. String. get. Chars(int, char[], int) source. Begin, source. End, dest. Array, dest. Begin
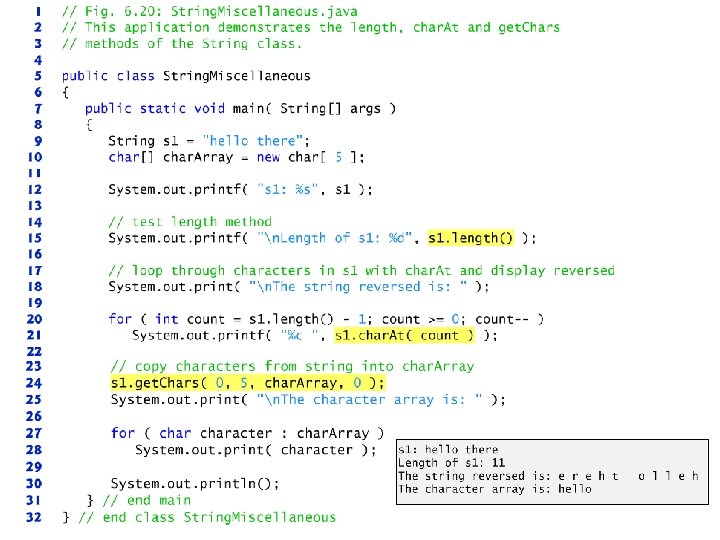
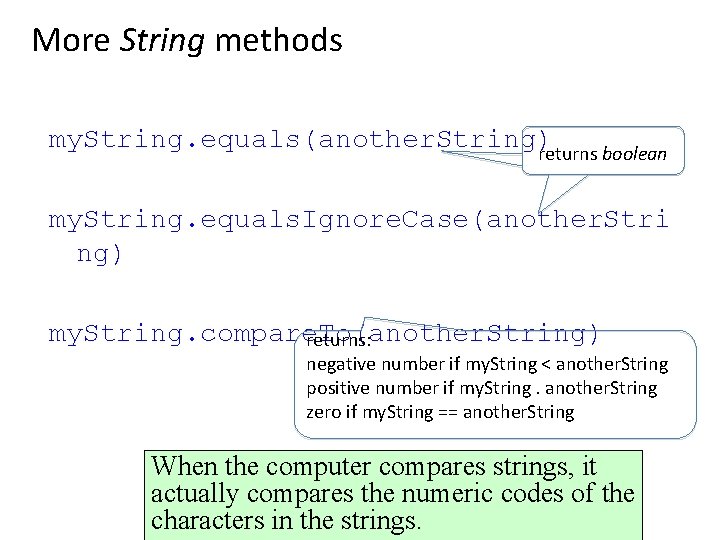
More String methods my. String. equals(another. String) returns boolean my. String. equals. Ignore. Case(another. Stri ng) my. String. compare. To(another. String) returns: negative number if my. String < another. String positive number if my. String. another. String zero if my. String == another. String When the computer compares strings, it actually compares the numeric codes of the characters in the strings.
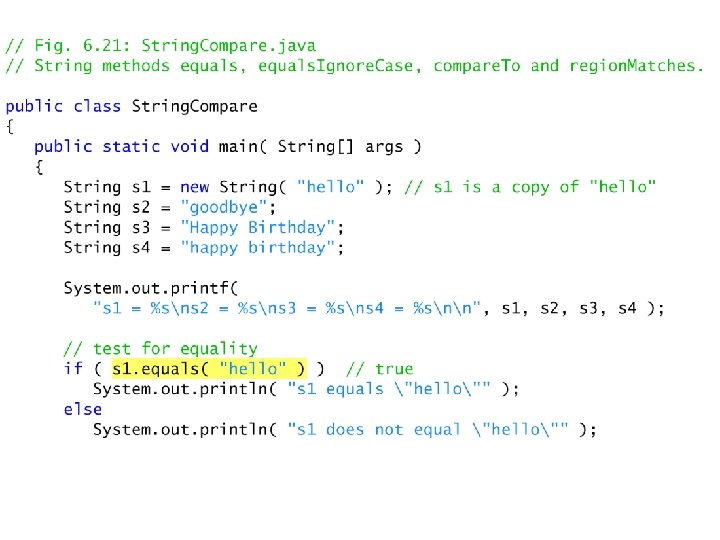
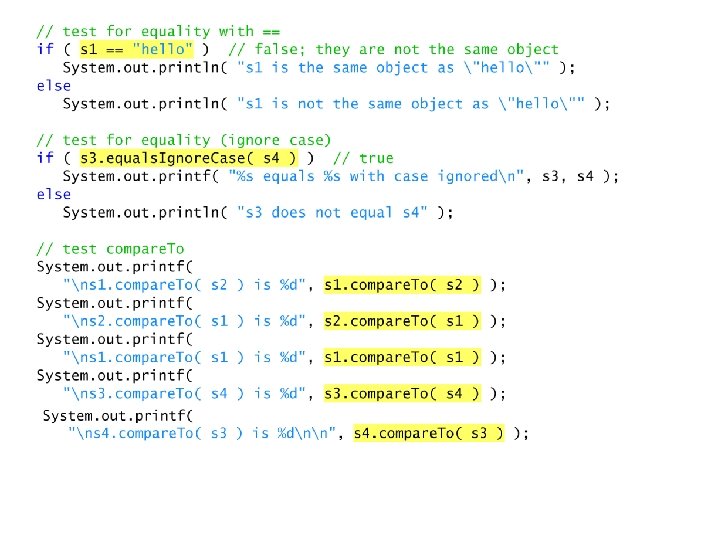
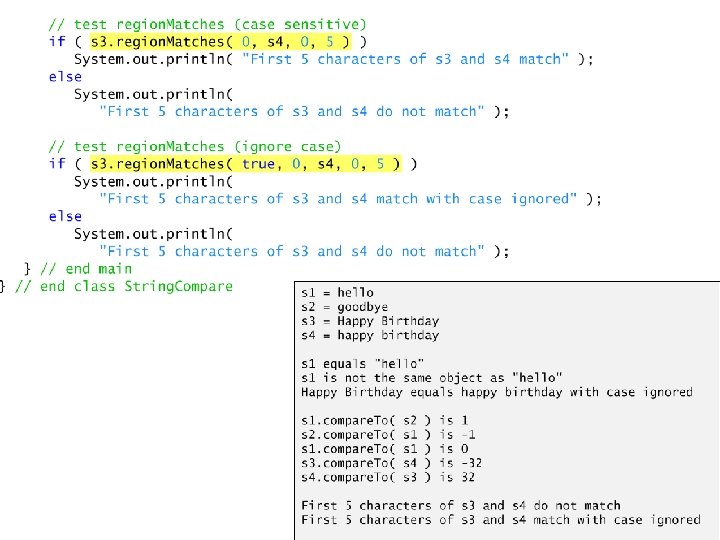
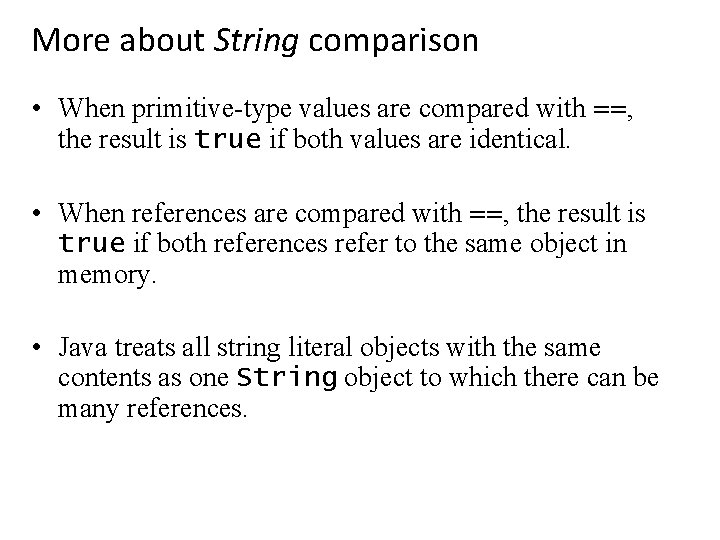
More about String comparison • When primitive-type values are compared with ==, the result is true if both values are identical. • When references are compared with ==, the result is true if both references refer to the same object in memory. • Java treats all string literal objects with the same contents as one String object to which there can be many references.
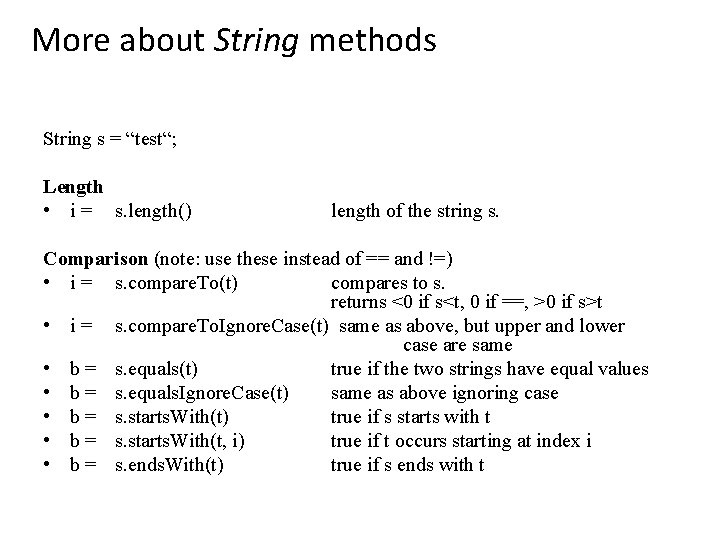
More about String methods String s = “test“; Length • i = s. length() length of the string s. Comparison (note: use these instead of == and !=) • i = s. compare. To(t) compares to s. returns <0 if s<t, 0 if ==, >0 if s>t • i = s. compare. To. Ignore. Case(t) same as above, but upper and lower case are same • b = s. equals(t) true if the two strings have equal values • b = s. equals. Ignore. Case(t) same as above ignoring case • b = s. starts. With(t) true if s starts with t • b = s. starts. With(t, i) true if t occurs starting at index i • b = s. ends. With(t) true if s ends with t
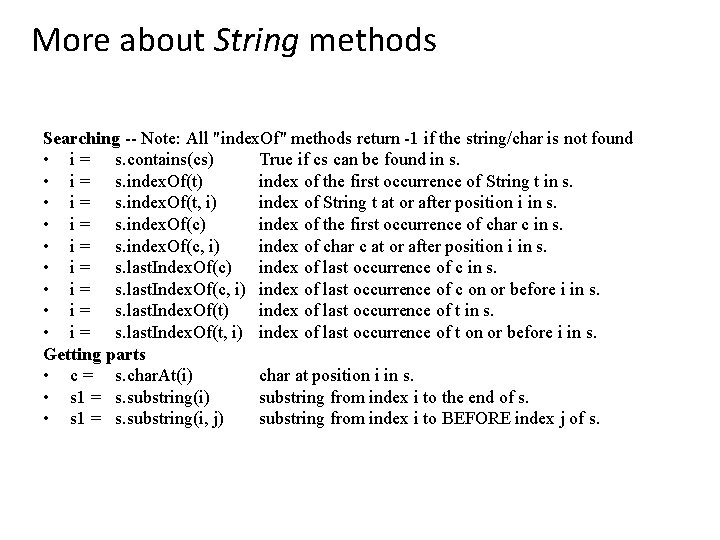
More about String methods Searching -- Note: All "index. Of" methods return -1 if the string/char is not found • i = s. contains(cs) True if cs can be found in s. • i = s. index. Of(t) index of the first occurrence of String t in s. • i = s. index. Of(t, i) index of String t at or after position i in s. • i = s. index. Of(c) index of the first occurrence of char c in s. • i = s. index. Of(c, i) index of char c at or after position i in s. • i = s. last. Index. Of(c) index of last occurrence of c in s. • i = s. last. Index. Of(c, i) index of last occurrence of c on or before i in s. • i = s. last. Index. Of(t) index of last occurrence of t in s. • i = s. last. Index. Of(t, i) index of last occurrence of t on or before i in s. Getting parts • c = s. char. At(i) char at position i in s. • s 1 = s. substring(i) substring from index i to the end of s. • s 1 = s. substring(i, j) substring from index i to BEFORE index j of s.
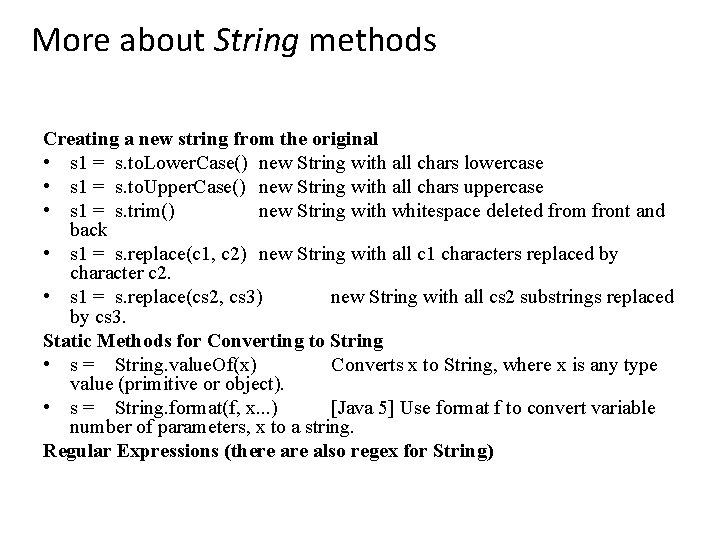
More about String methods Creating a new string from the original • s 1 = s. to. Lower. Case() new String with all chars lowercase • s 1 = s. to. Upper. Case() new String with all chars uppercase • s 1 = s. trim() new String with whitespace deleted from front and back • s 1 = s. replace(c 1, c 2) new String with all c 1 characters replaced by character c 2. • s 1 = s. replace(cs 2, cs 3) new String with all cs 2 substrings replaced by cs 3. Static Methods for Converting to String • s = String. value. Of(x) Converts x to String, where x is any type value (primitive or object). • s = String. format(f, x. . . ) [Java 5] Use format f to convert variable number of parameters, x to a string. Regular Expressions (there also regex for String)
Computer science arrays
Python list of arrays
Dynamic arrays and amortized analysis
How many arrays in 24
Are vectors dynamic arrays
Small basic arrays
Polynomial representation using arrays
Adt of array
潘仁義
Arrays visual basic
Searching and sorting arrays in c++
Arreglo java
Arrays
Parallel arrays in c
Arrays in pascal examples
Facts about arrays
Strings in assembly language
Partially filled array java
C++ parallel arrays
Python parallel arrays
Advantages of dynamic memory allocation
Arrays bidimensionales java
Day 3: arrays
Array of arrays c++
Dynamic array mips
Global arrays in c
Redundancy array of independent disk
Why do we need arrays?
Array advantages and disadvantages
Microled arrays
Redundant array of inexpensive disks
Array mips
Creating arrays matlab
Java array operations
Two equal mass rocks tied to strings
Language c string
Pointers and strings
What are strings in c
A type of cipher that uses multiple alphabetic strings.
Three masses are connected by strings
Flexible pattern matching in strings
Ottawa suzuki strings
Ida strings
Vortex strings
Sludoy instrument description
Array of structs c
Concatenation discrete math
Stos assembly
Rate of energy transfer by sinusoidal waves on strings
Combinations and permutations
Declare a two dimensional array of strings named chessboard
Muscle strings
Michael league gear
Import java.util.string
Jmp to excel
Upx unpacker
Compare strings in python
Ida pro strings
String and other things
Mrs sprockett's strange machine
Things not strings
Springs and strings
Howard gardner hendricks